LIS 651 lecture 3 taming PHP Thomas Krichel
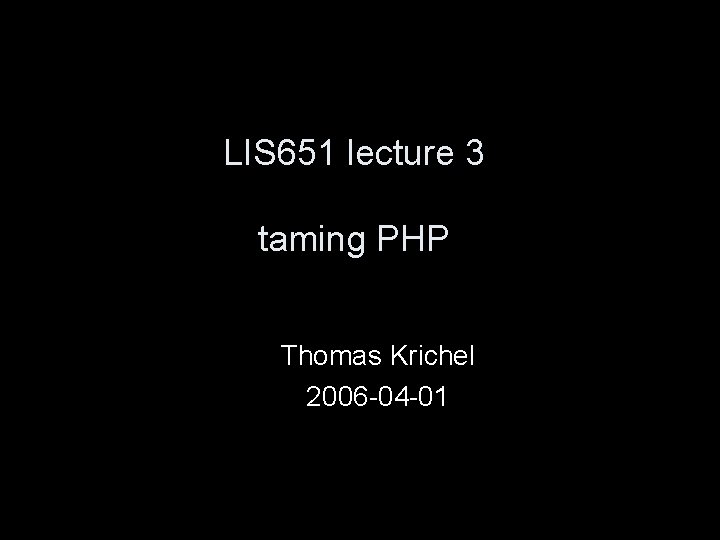
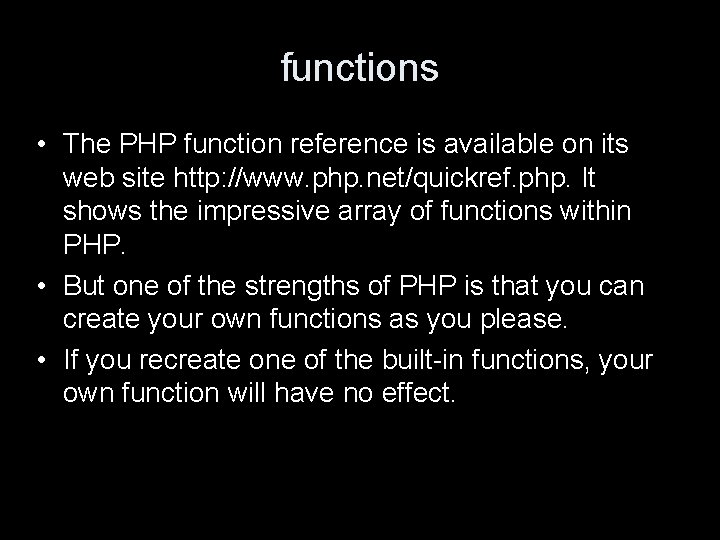
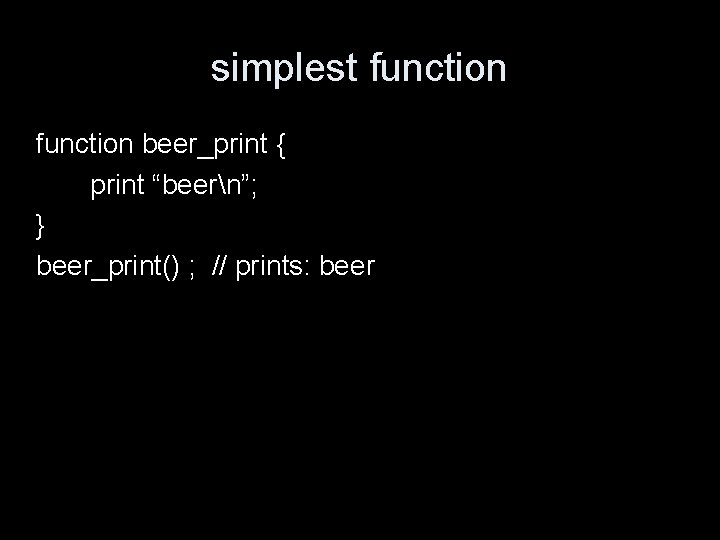
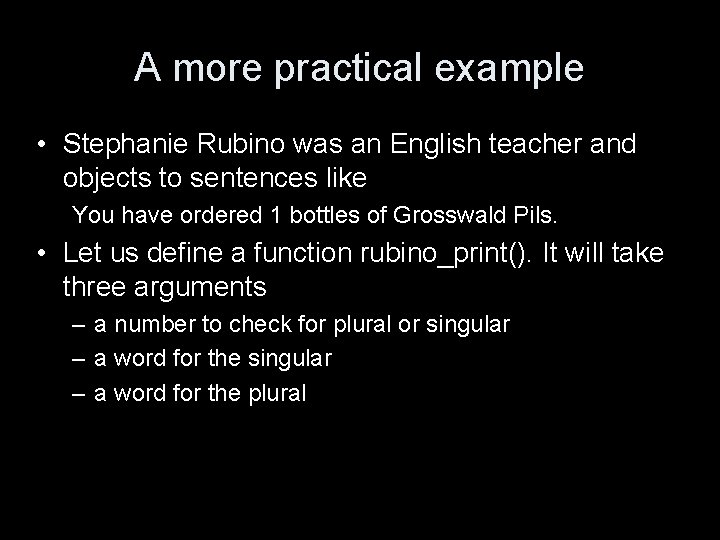
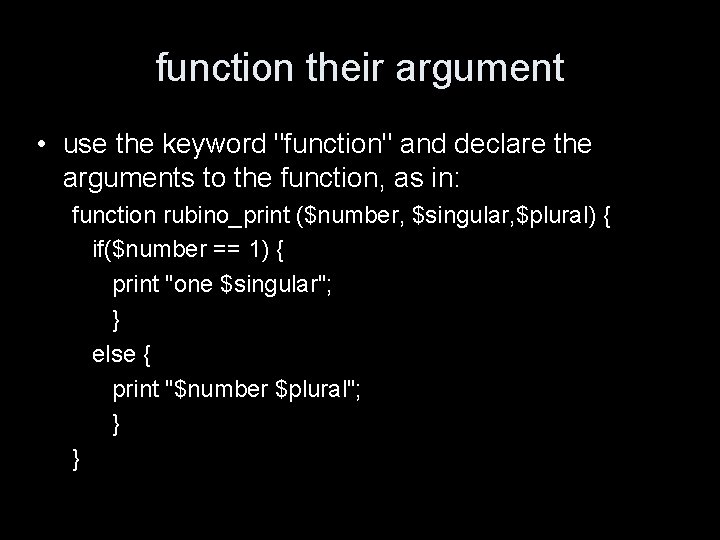
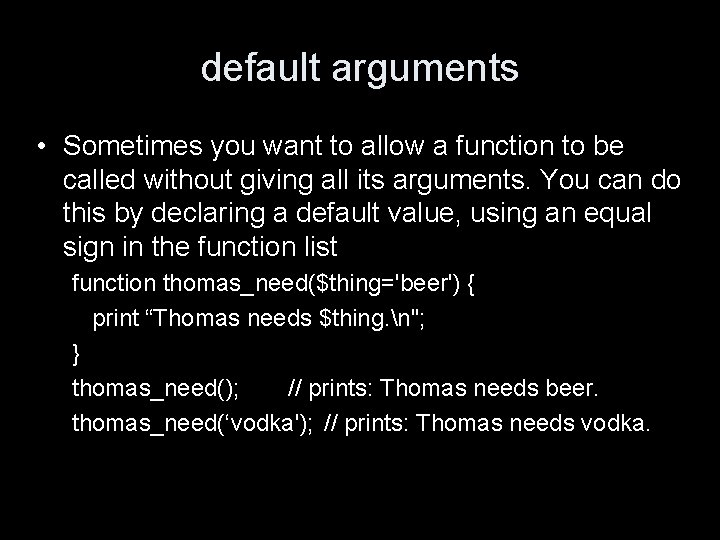
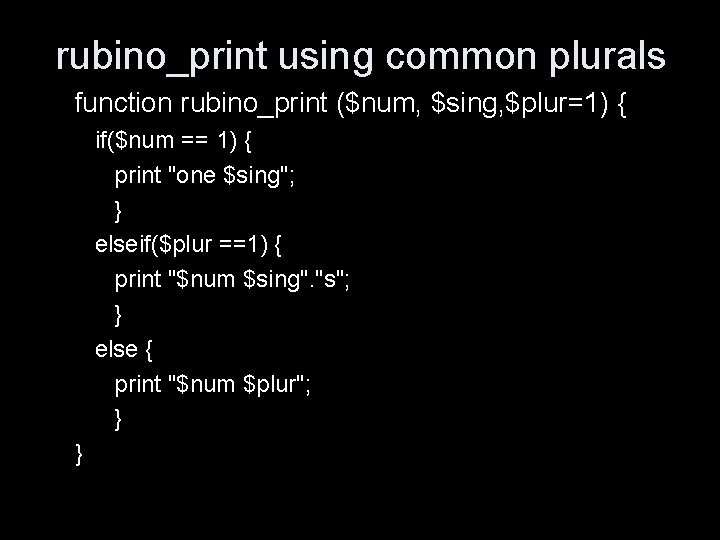
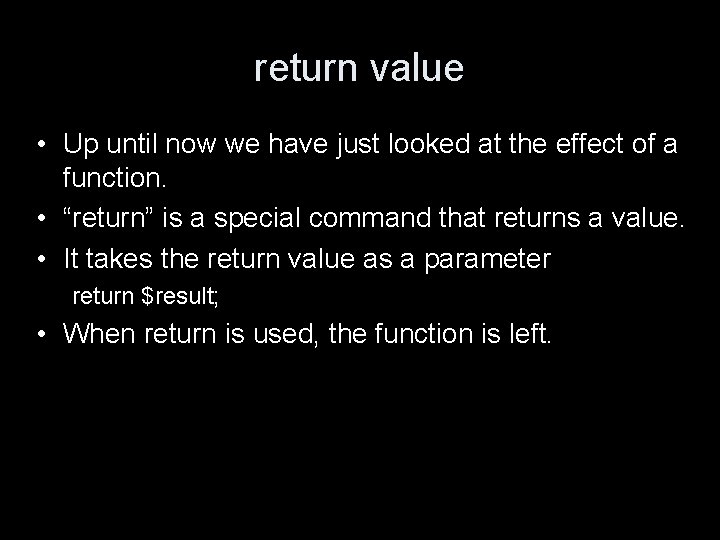
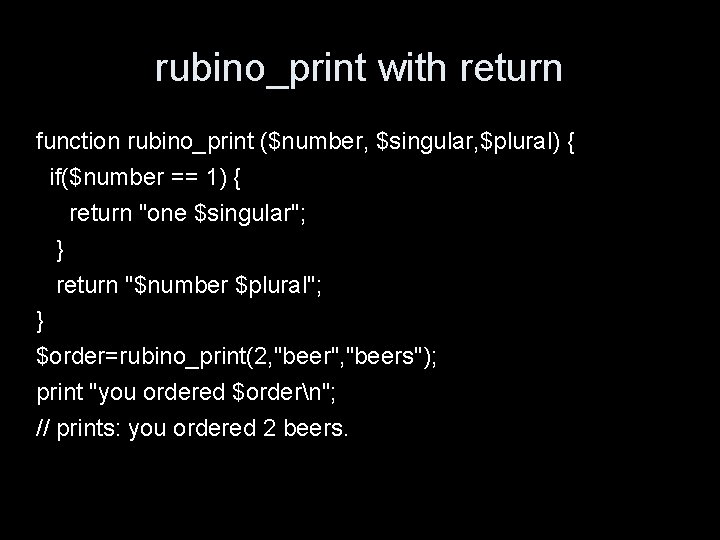
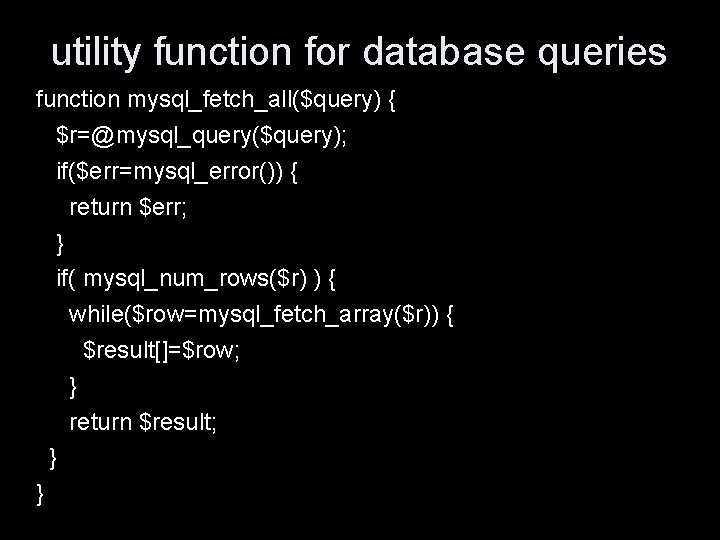
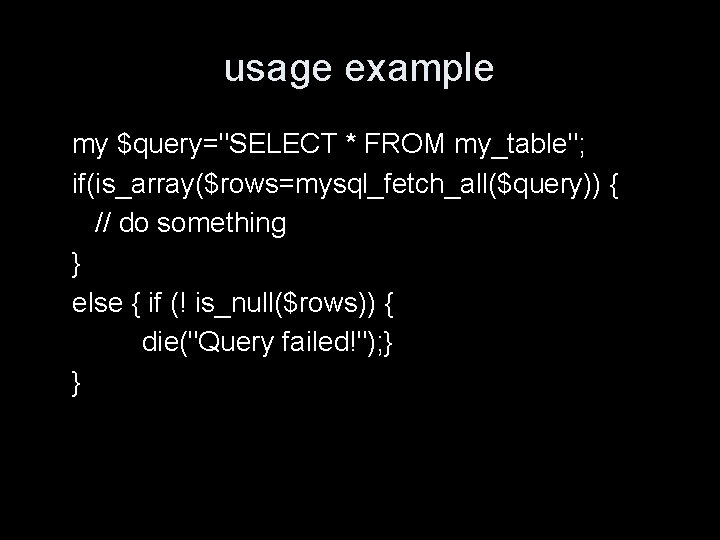
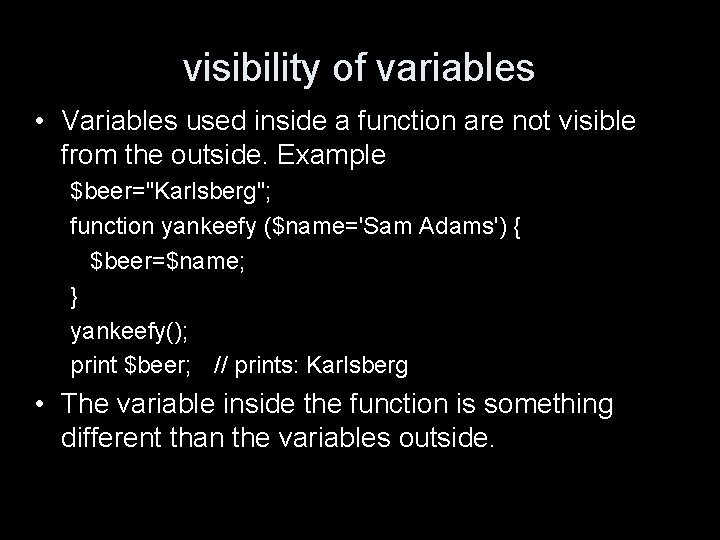
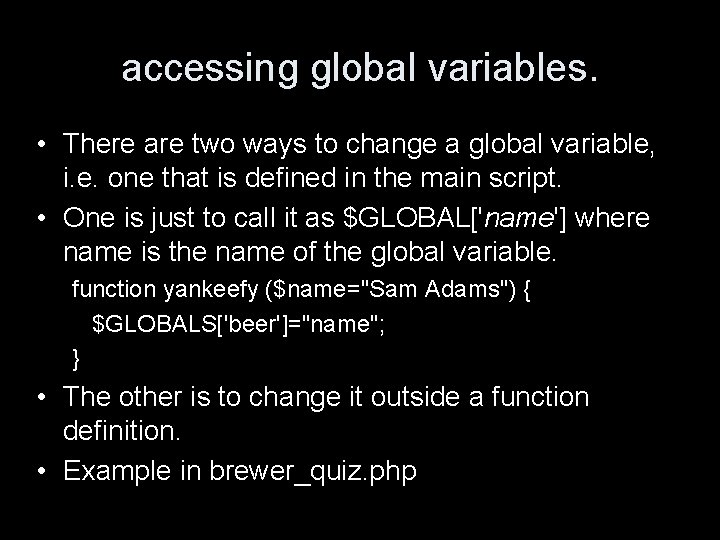
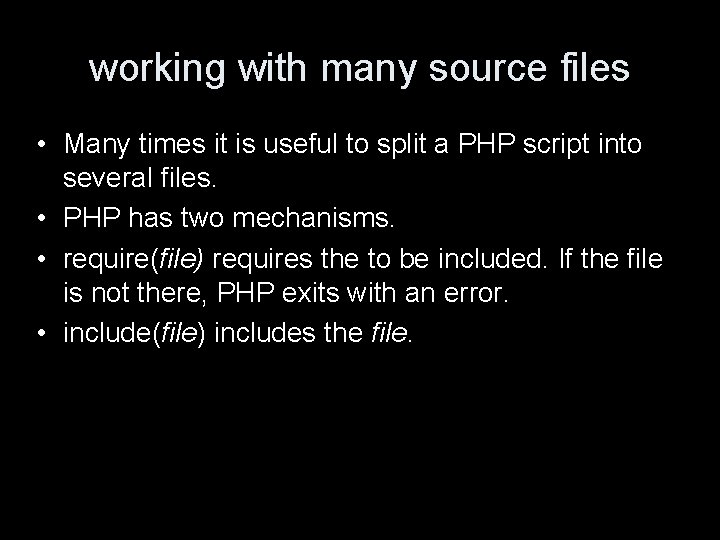
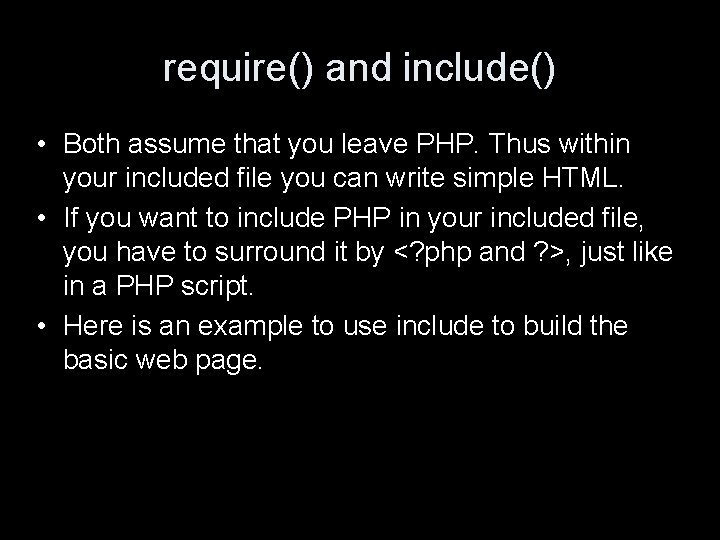
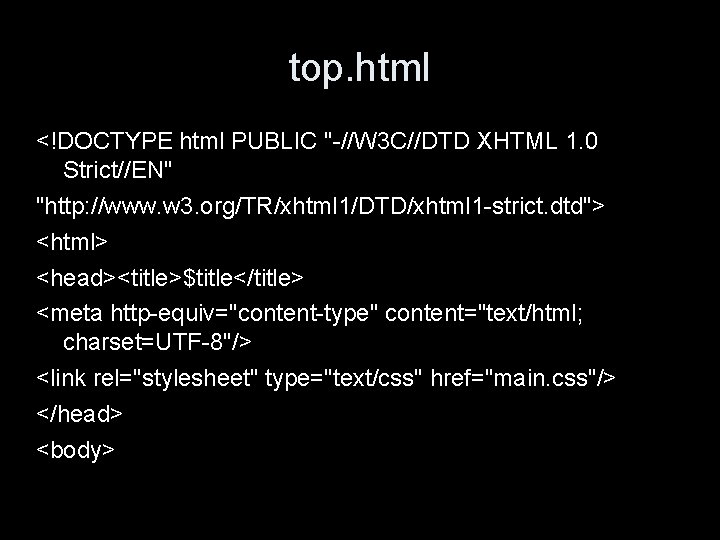
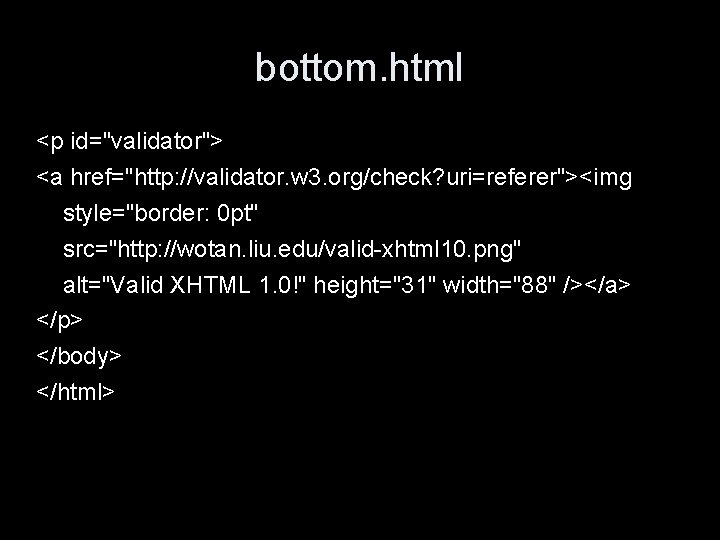
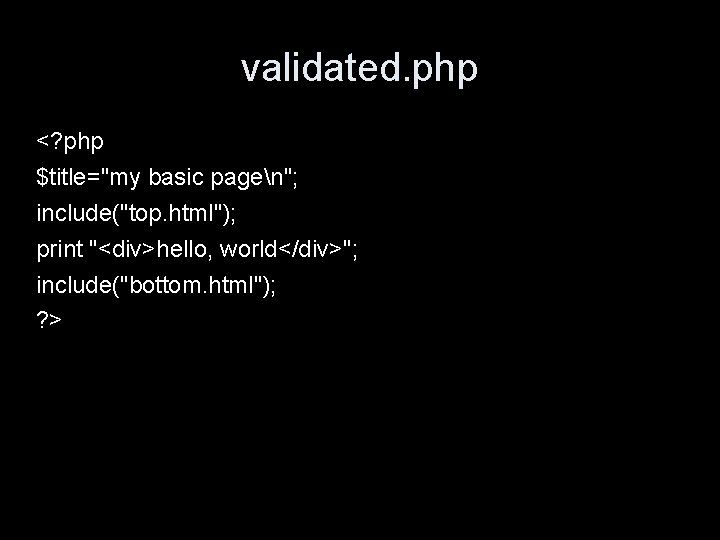
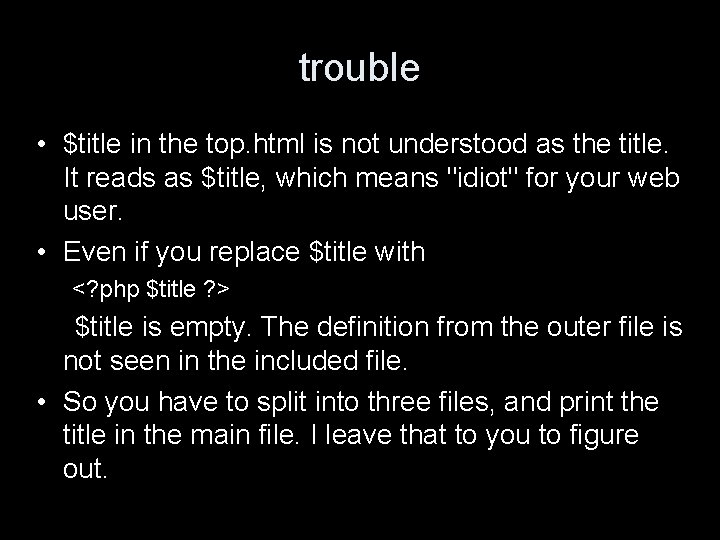
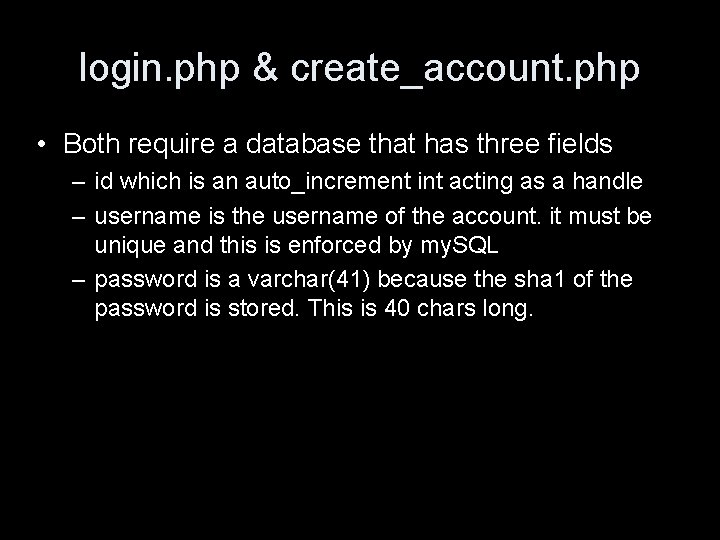
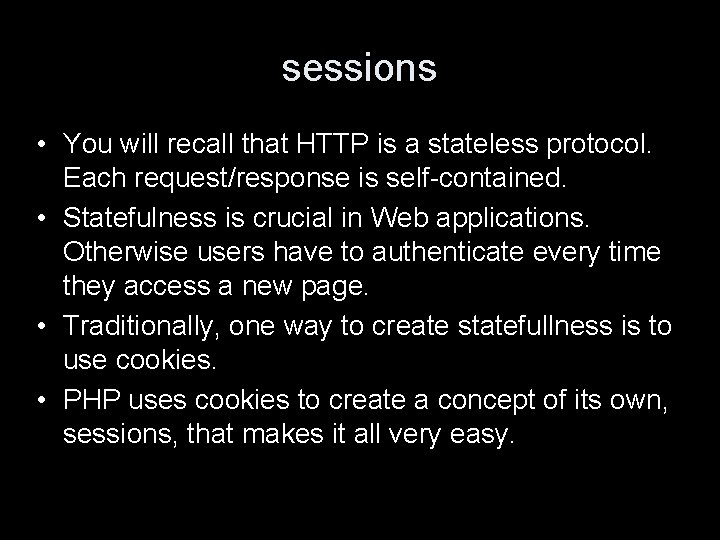
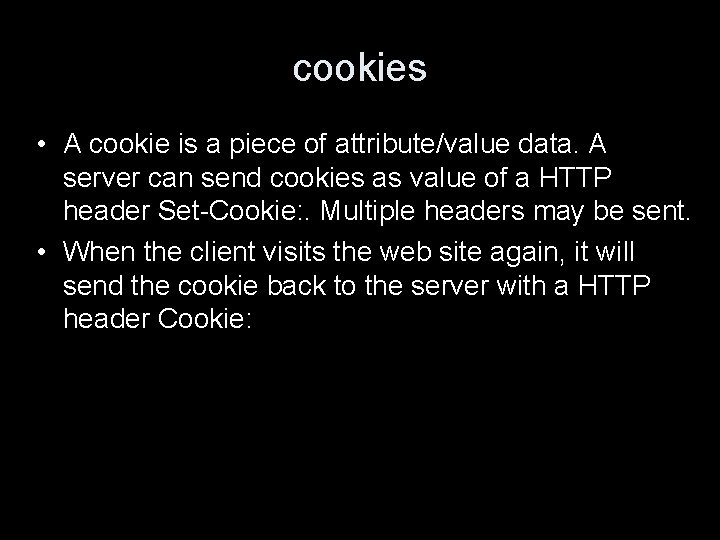
![Set-Cookie • Set-Cookie: name=value; [expires= date; ] [path=path; ] [domain= domain] [secure] • where Set-Cookie • Set-Cookie: name=value; [expires= date; ] [path=path; ] [domain= domain] [secure] • where](https://slidetodoc.com/presentation_image/529d1b5a089412286eb3c73da5a6c0a5/image-23.jpg)
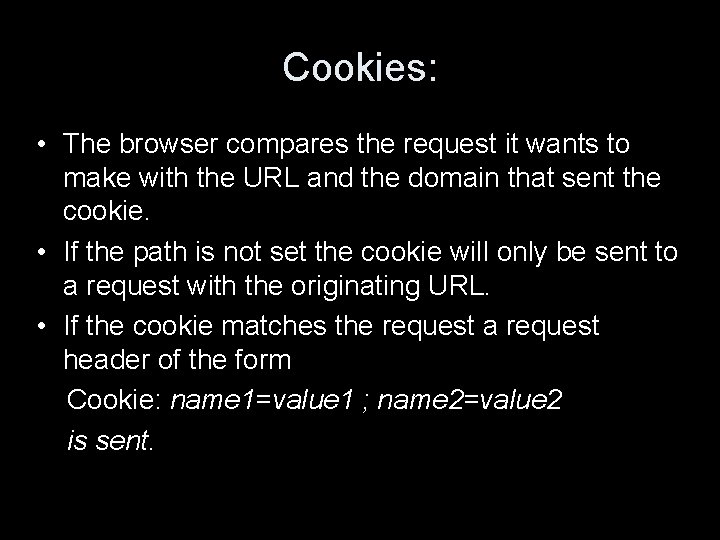
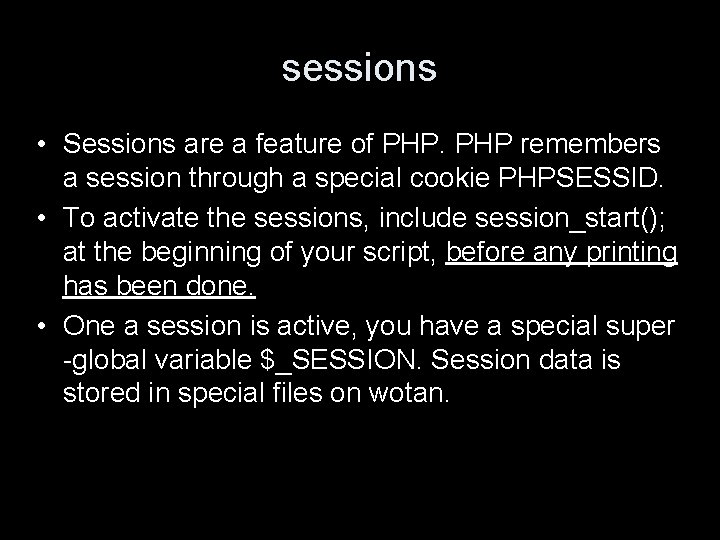
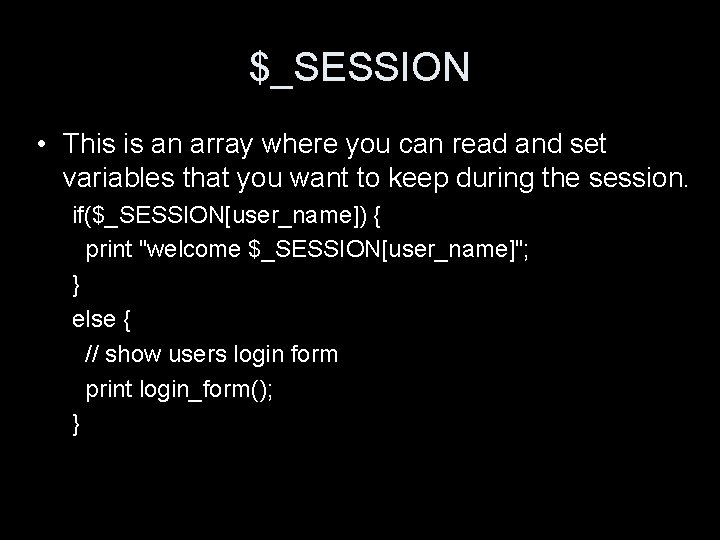
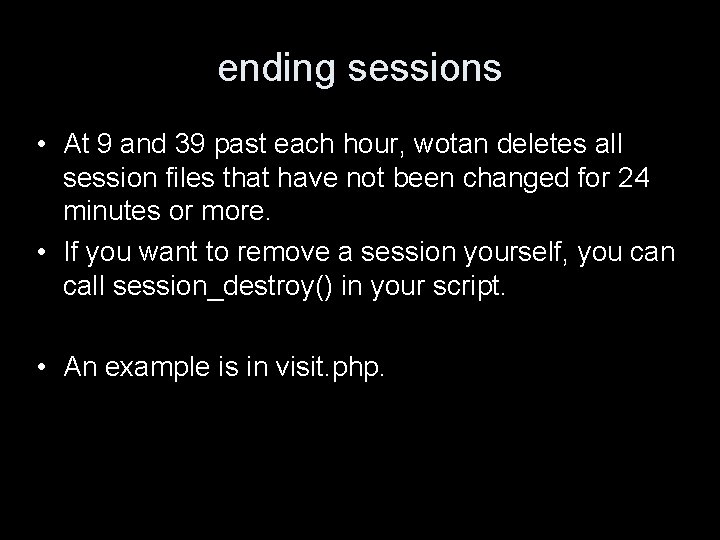
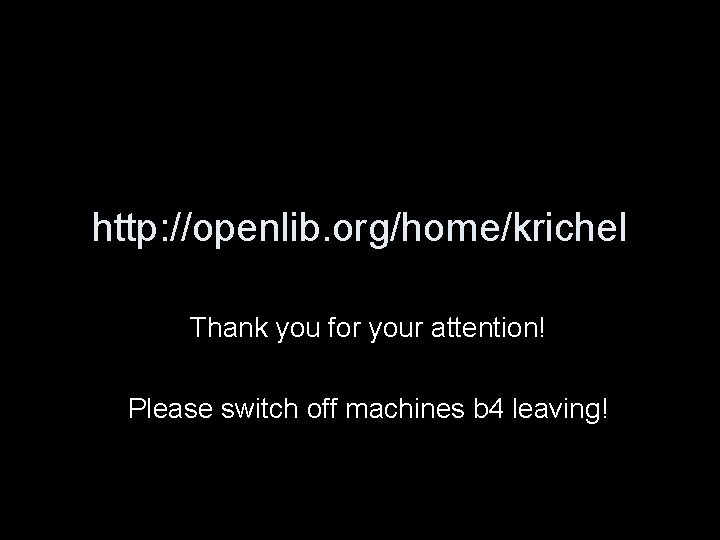
- Slides: 28
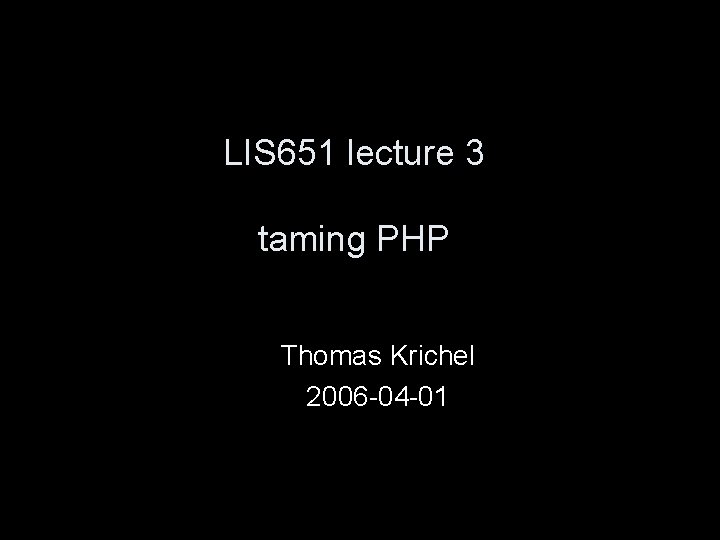
LIS 651 lecture 3 taming PHP Thomas Krichel 2006 -04 -01
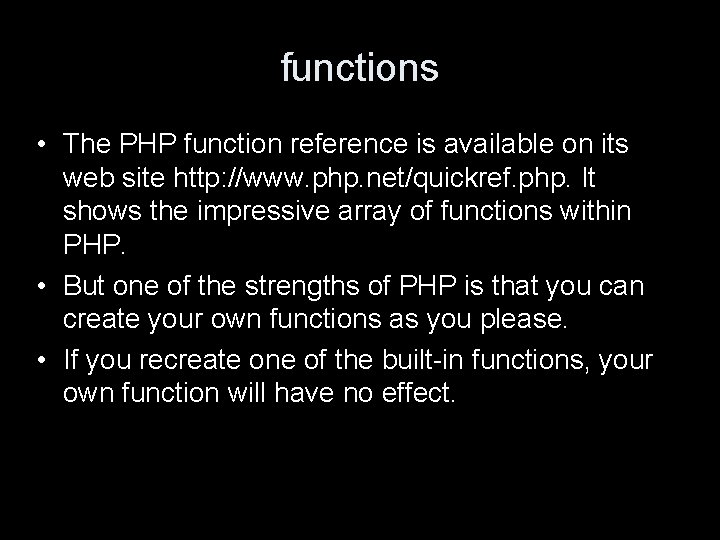
functions • The PHP function reference is available on its web site http: //www. php. net/quickref. php. It shows the impressive array of functions within PHP. • But one of the strengths of PHP is that you can create your own functions as you please. • If you recreate one of the built-in functions, your own function will have no effect.
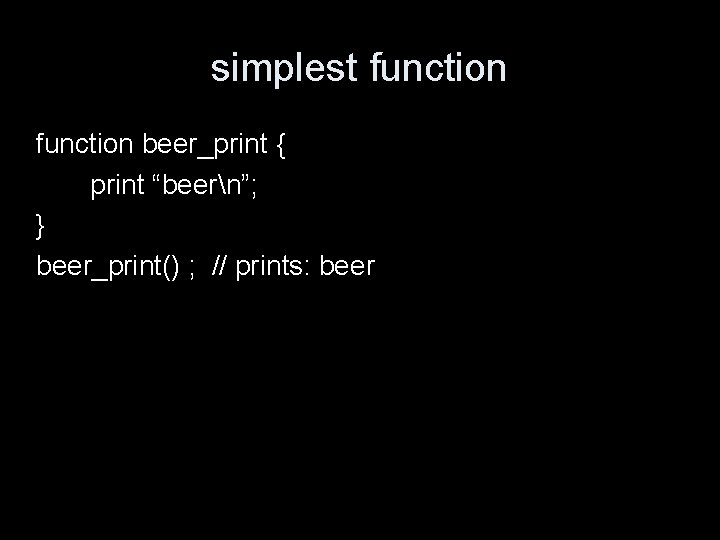
simplest function beer_print { print “beern”; } beer_print() ; // prints: beer
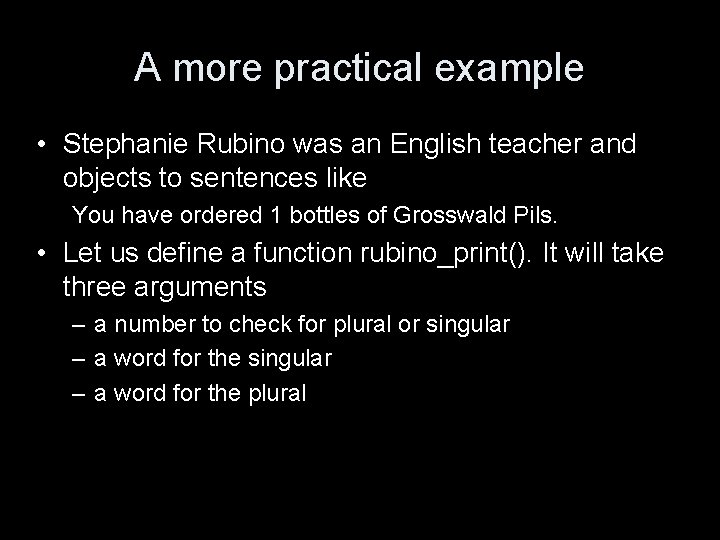
A more practical example • Stephanie Rubino was an English teacher and objects to sentences like You have ordered 1 bottles of Grosswald Pils. • Let us define a function rubino_print(). It will take three arguments – a number to check for plural or singular – a word for the plural
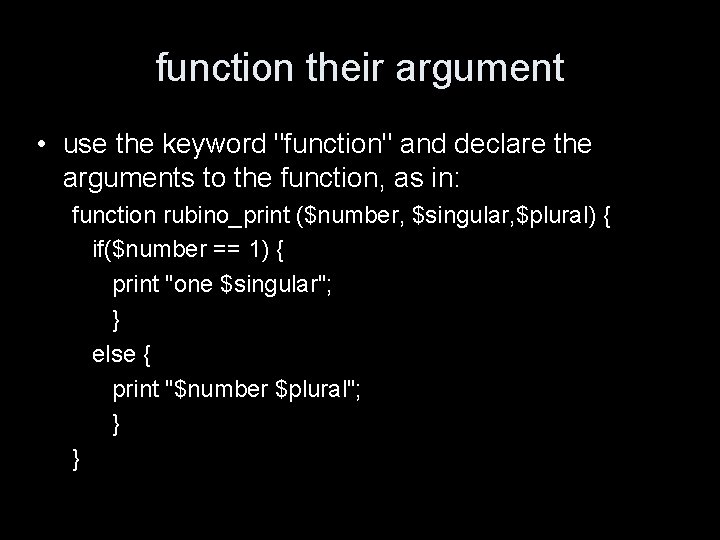
function their argument • use the keyword "function" and declare the arguments to the function, as in: function rubino_print ($number, $singular, $plural) { if($number == 1) { print "one $singular"; } else { print "$number $plural"; } }
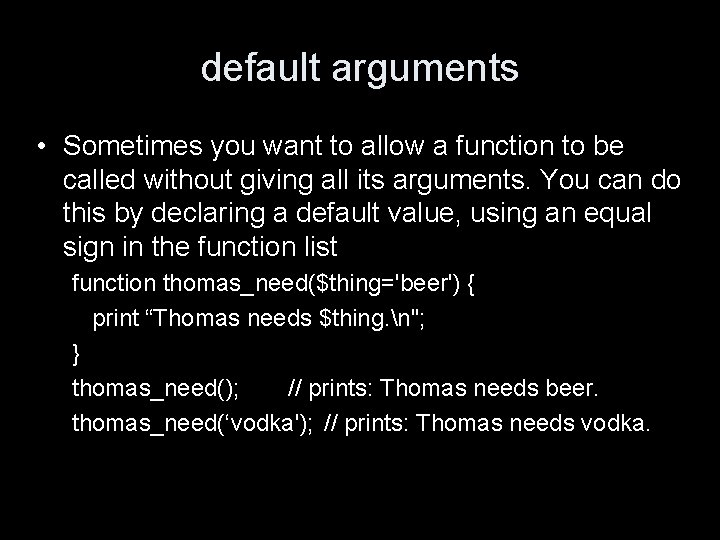
default arguments • Sometimes you want to allow a function to be called without giving all its arguments. You can do this by declaring a default value, using an equal sign in the function list function thomas_need($thing='beer') { print “Thomas needs $thing. n"; } thomas_need(); // prints: Thomas needs beer. thomas_need(‘vodka'); // prints: Thomas needs vodka.
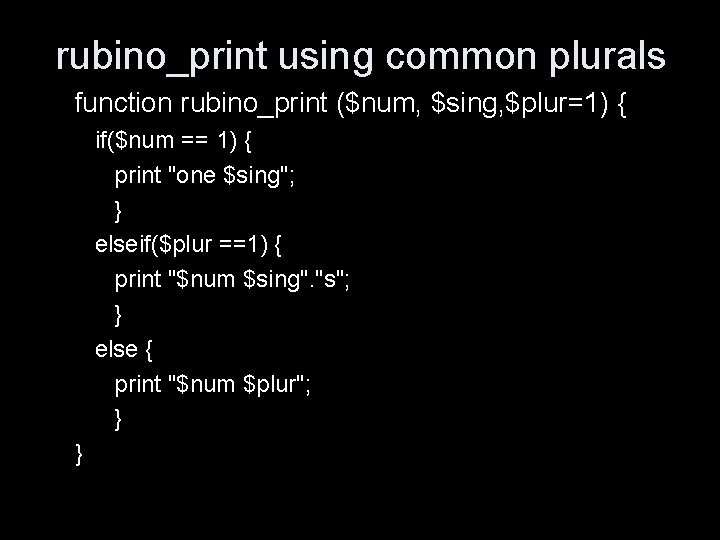
rubino_print using common plurals function rubino_print ($num, $sing, $plur=1) { if($num == 1) { print "one $sing"; } elseif($plur ==1) { print "$num $sing". "s"; } else { print "$num $plur"; } }
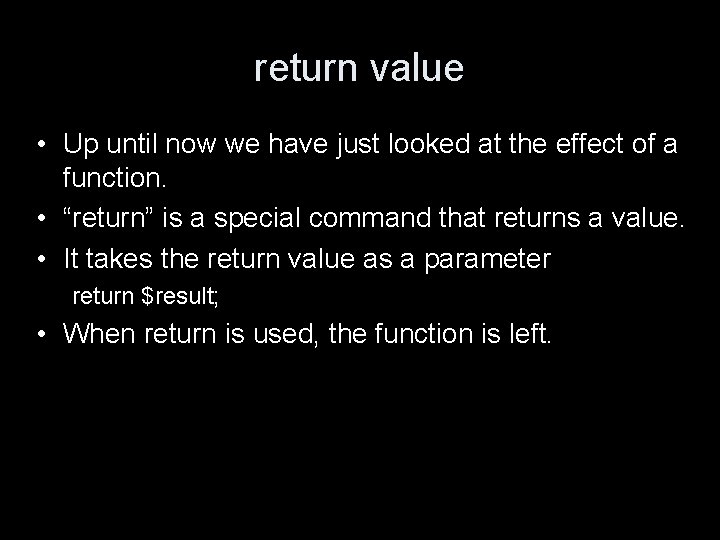
return value • Up until now we have just looked at the effect of a function. • “return” is a special command that returns a value. • It takes the return value as a parameter return $result; • When return is used, the function is left.
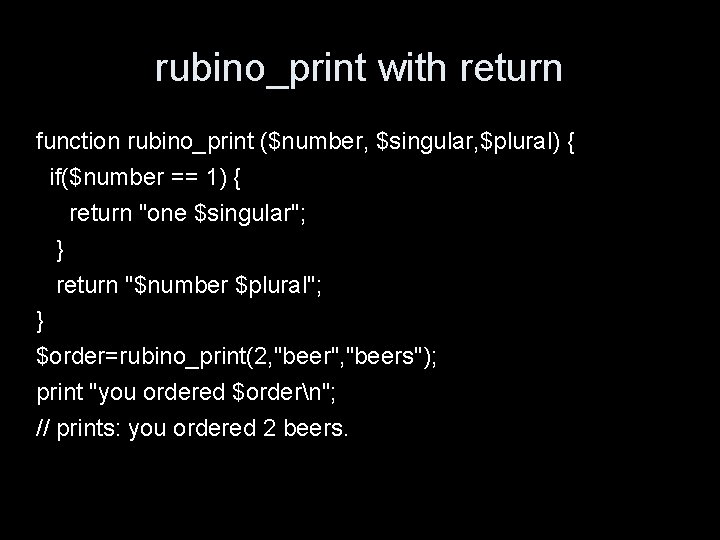
rubino_print with return function rubino_print ($number, $singular, $plural) { if($number == 1) { return "one $singular"; } return "$number $plural"; } $order=rubino_print(2, "beer", "beers"); print "you ordered $ordern"; // prints: you ordered 2 beers.
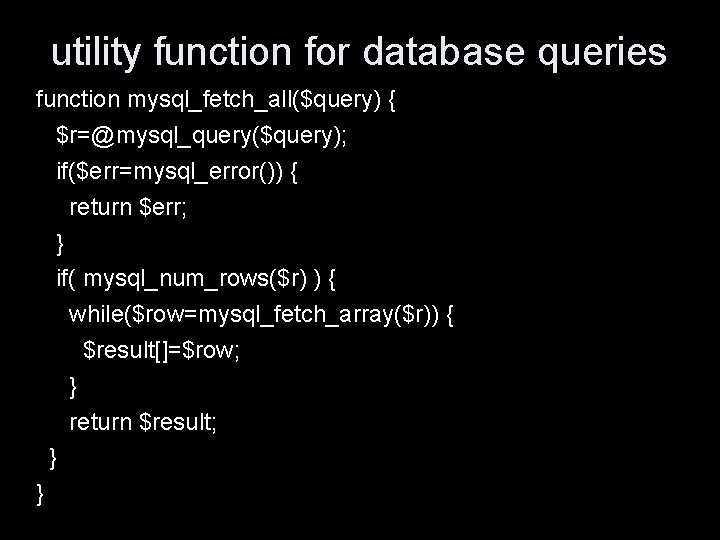
utility function for database queries function mysql_fetch_all($query) { $r=@mysql_query($query); if($err=mysql_error()) { return $err; } if( mysql_num_rows($r) ) { while($row=mysql_fetch_array($r)) { $result[]=$row; } return $result; } }
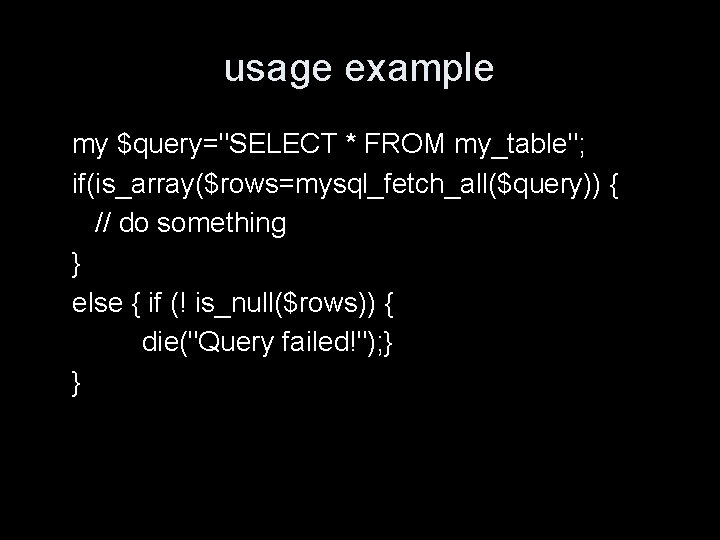
usage example my $query="SELECT * FROM my_table"; if(is_array($rows=mysql_fetch_all($query)) { // do something } else { if (! is_null($rows)) { die("Query failed!"); } }
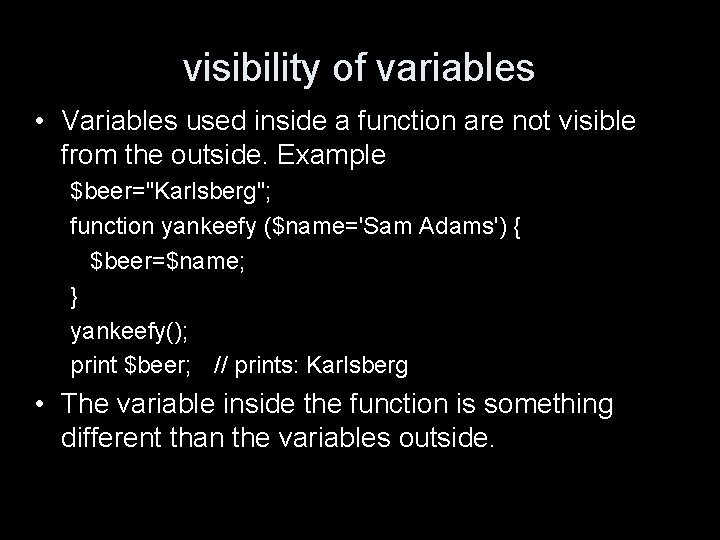
visibility of variables • Variables used inside a function are not visible from the outside. Example $beer="Karlsberg"; function yankeefy ($name='Sam Adams') { $beer=$name; } yankeefy(); print $beer; // prints: Karlsberg • The variable inside the function is something different than the variables outside.
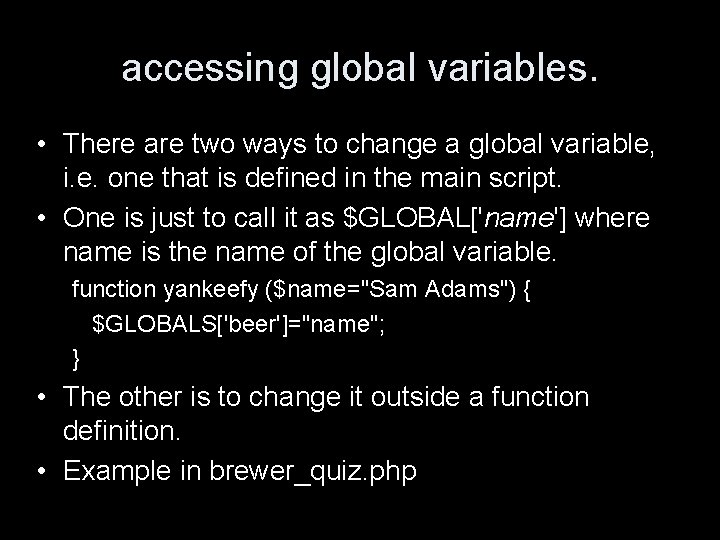
accessing global variables. • There are two ways to change a global variable, i. e. one that is defined in the main script. • One is just to call it as $GLOBAL['name'] where name is the name of the global variable. function yankeefy ($name="Sam Adams") { $GLOBALS['beer']="name"; } • The other is to change it outside a function definition. • Example in brewer_quiz. php
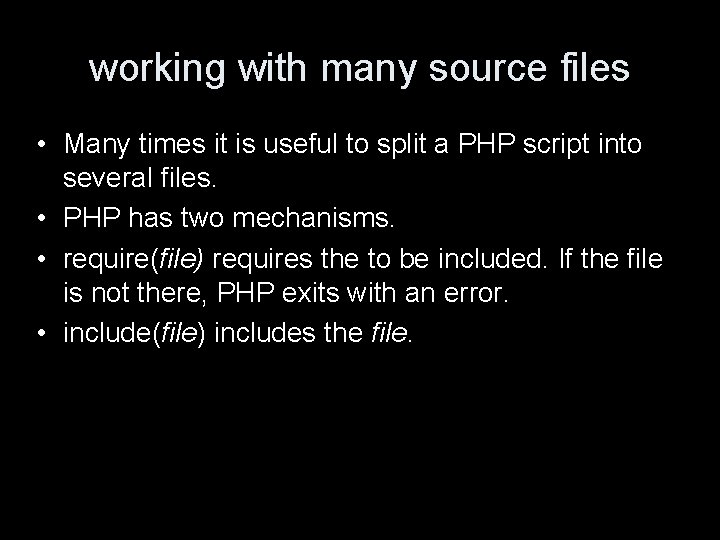
working with many source files • Many times it is useful to split a PHP script into several files. • PHP has two mechanisms. • require(file) requires the to be included. If the file is not there, PHP exits with an error. • include(file) includes the file.
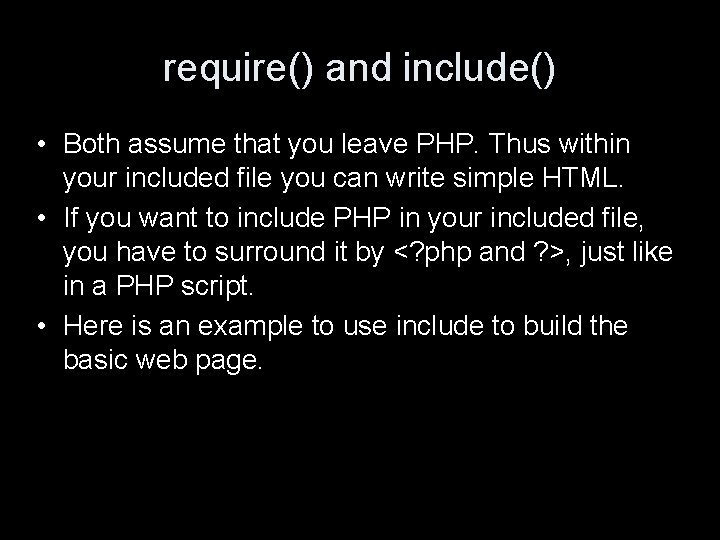
require() and include() • Both assume that you leave PHP. Thus within your included file you can write simple HTML. • If you want to include PHP in your included file, you have to surround it by <? php and ? >, just like in a PHP script. • Here is an example to use include to build the basic web page.
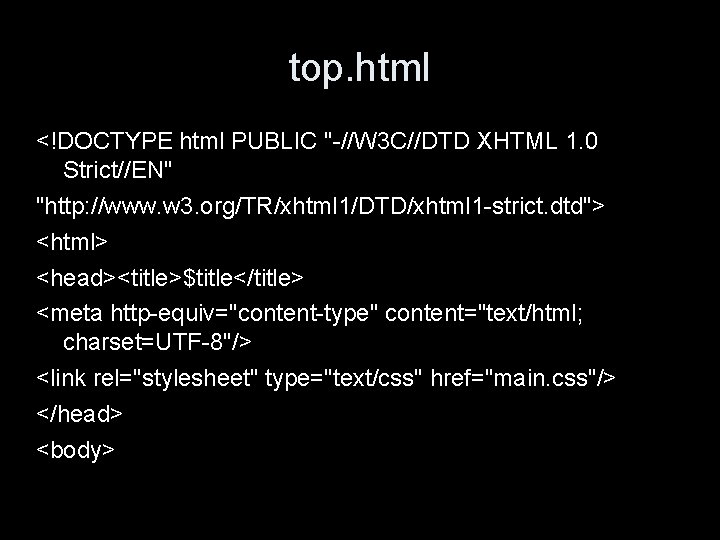
top. html <!DOCTYPE html PUBLIC "-//W 3 C//DTD XHTML 1. 0 Strict//EN" "http: //www. w 3. org/TR/xhtml 1/DTD/xhtml 1 -strict. dtd"> <html> <head><title>$title</title> <meta http-equiv="content-type" content="text/html; charset=UTF-8"/> <link rel="stylesheet" type="text/css" href="main. css"/> </head> <body>
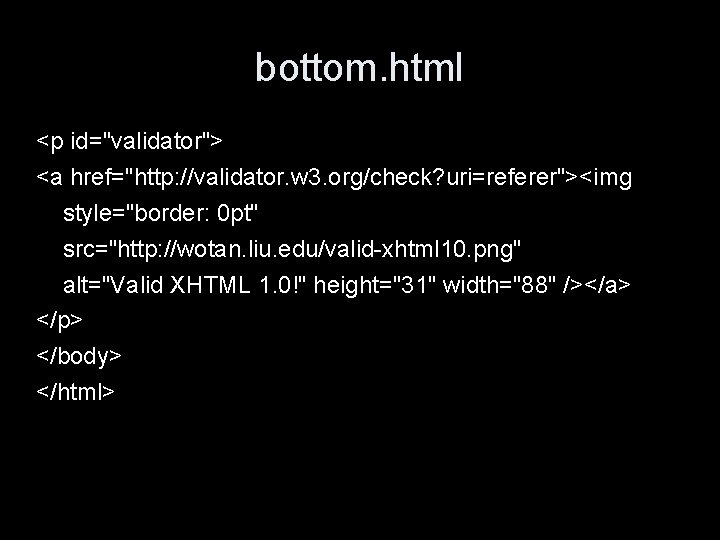
bottom. html <p id="validator"> <a href="http: //validator. w 3. org/check? uri=referer"><img style="border: 0 pt" src="http: //wotan. liu. edu/valid-xhtml 10. png" alt="Valid XHTML 1. 0!" height="31" width="88" /></a> </p> </body> </html>
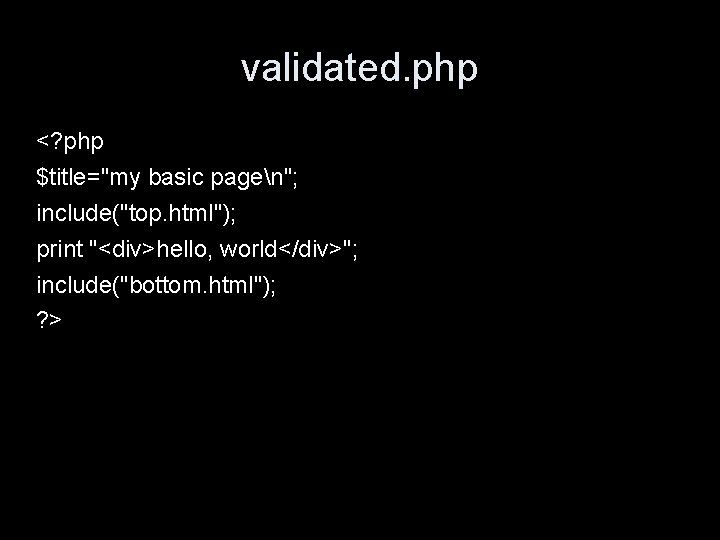
validated. php <? php $title="my basic pagen"; include("top. html"); print "<div>hello, world</div>"; include("bottom. html"); ? >
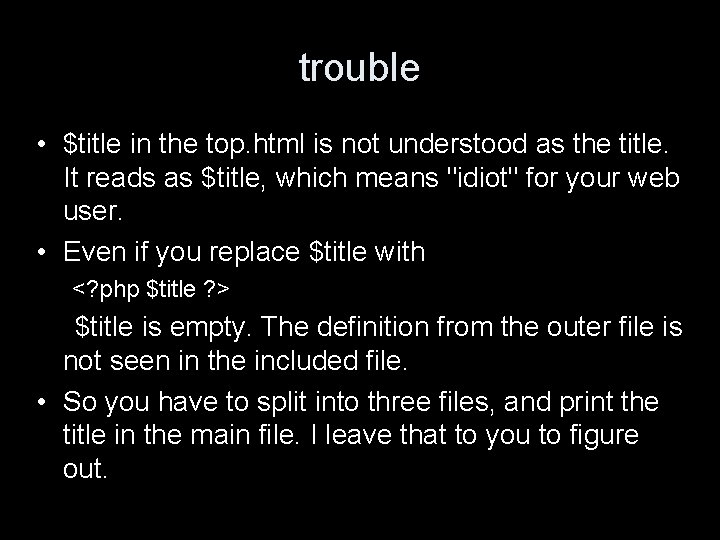
trouble • $title in the top. html is not understood as the title. It reads as $title, which means "idiot" for your web user. • Even if you replace $title with <? php $title ? > $title is empty. The definition from the outer file is not seen in the included file. • So you have to split into three files, and print the title in the main file. I leave that to you to figure out.
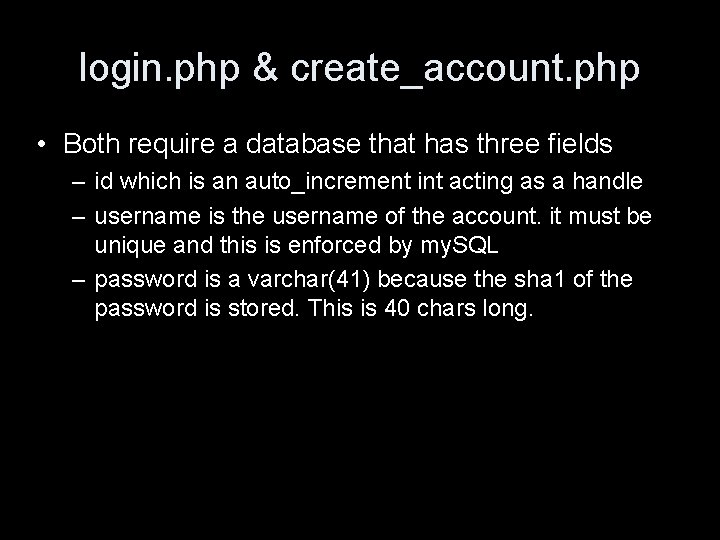
login. php & create_account. php • Both require a database that has three fields – id which is an auto_increment int acting as a handle – username is the username of the account. it must be unique and this is enforced by my. SQL – password is a varchar(41) because the sha 1 of the password is stored. This is 40 chars long.
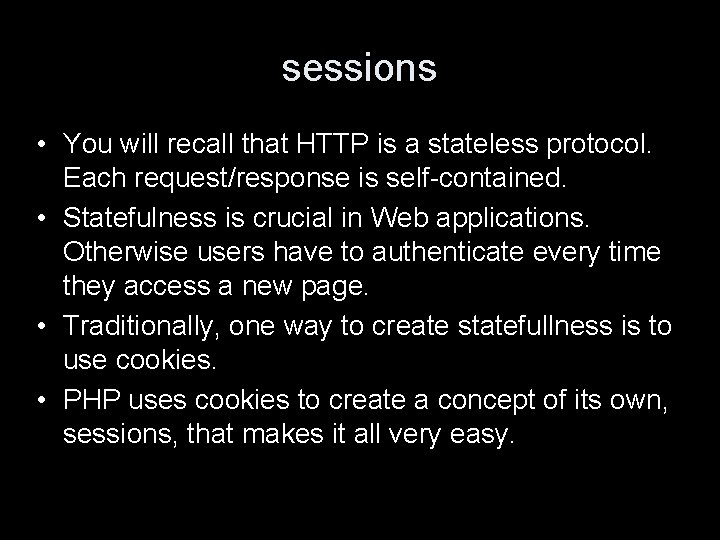
sessions • You will recall that HTTP is a stateless protocol. Each request/response is self-contained. • Statefulness is crucial in Web applications. Otherwise users have to authenticate every time they access a new page. • Traditionally, one way to create statefullness is to use cookies. • PHP uses cookies to create a concept of its own, sessions, that makes it all very easy.
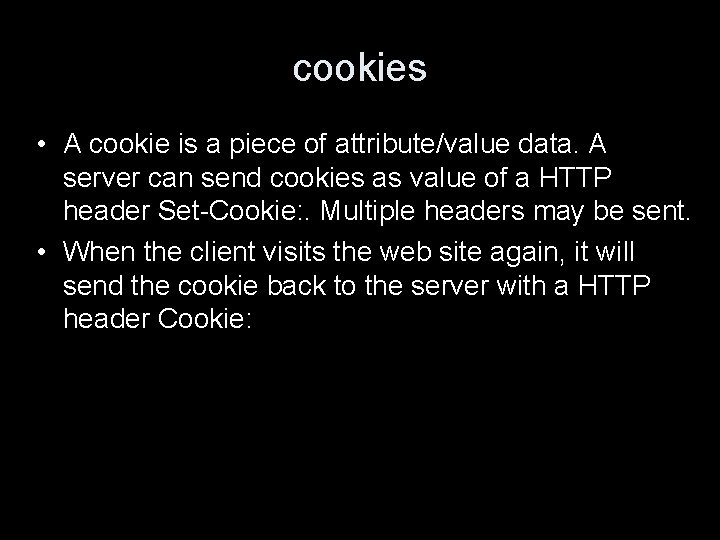
cookies • A cookie is a piece of attribute/value data. A server can send cookies as value of a HTTP header Set-Cookie: . Multiple headers may be sent. • When the client visits the web site again, it will send the cookie back to the server with a HTTP header Cookie:
![SetCookie SetCookie namevalue expires date pathpath domain domain secure where Set-Cookie • Set-Cookie: name=value; [expires= date; ] [path=path; ] [domain= domain] [secure] • where](https://slidetodoc.com/presentation_image/529d1b5a089412286eb3c73da5a6c0a5/image-23.jpg)
Set-Cookie • Set-Cookie: name=value; [expires= date; ] [path=path; ] [domain= domain] [secure] • where – – name= is the variable name set in the cookie value= is the variable's value date= is a date when the cookie expires path= restricts the cookie to be sent only when requests to a path starting with path are made – domain= restricts the sending of the cookie to a certain domain – secure restricts transmission to https
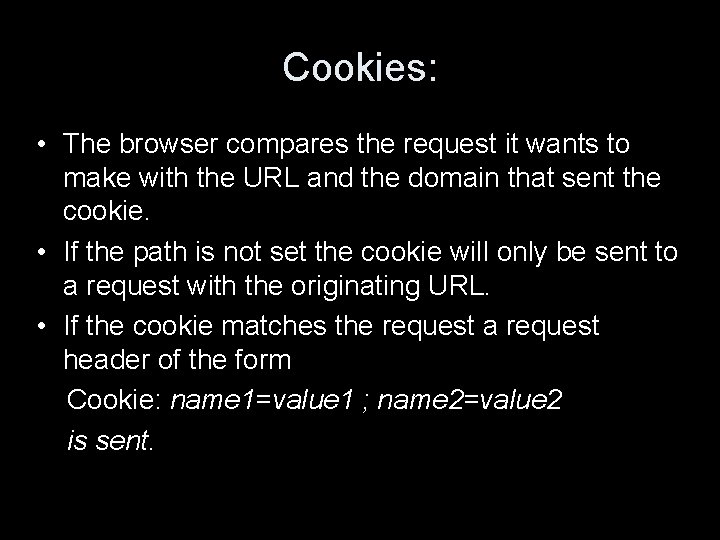
Cookies: • The browser compares the request it wants to make with the URL and the domain that sent the cookie. • If the path is not set the cookie will only be sent to a request with the originating URL. • If the cookie matches the request a request header of the form Cookie: name 1=value 1 ; name 2=value 2 is sent.
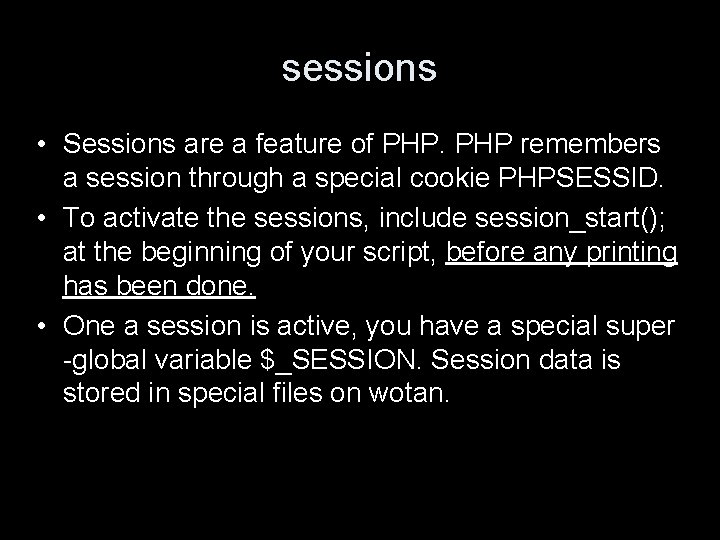
sessions • Sessions are a feature of PHP remembers a session through a special cookie PHPSESSID. • To activate the sessions, include session_start(); at the beginning of your script, before any printing has been done. • One a session is active, you have a special super -global variable $_SESSION. Session data is stored in special files on wotan.
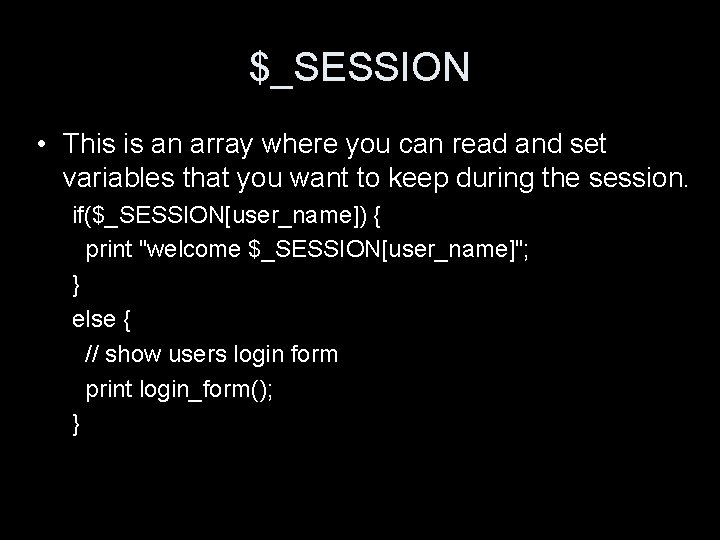
$_SESSION • This is an array where you can read and set variables that you want to keep during the session. if($_SESSION[user_name]) { print "welcome $_SESSION[user_name]"; } else { // show users login form print login_form(); }
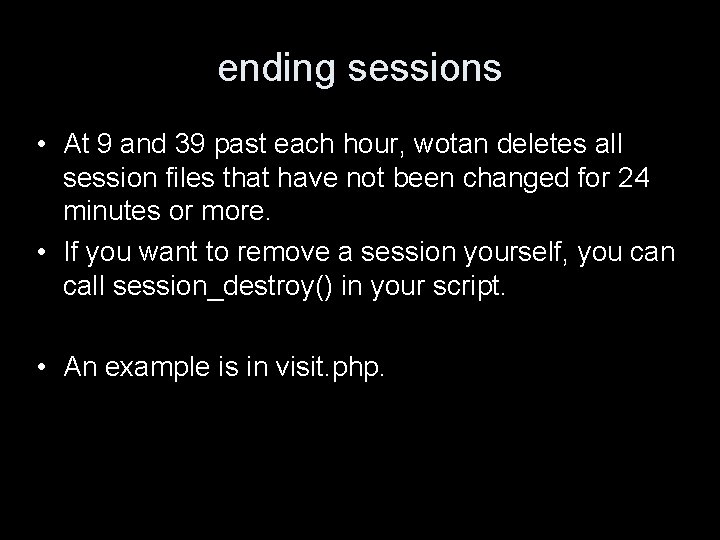
ending sessions • At 9 and 39 past each hour, wotan deletes all session files that have not been changed for 24 minutes or more. • If you want to remove a session yourself, you can call session_destroy() in your script. • An example is in visit. php.
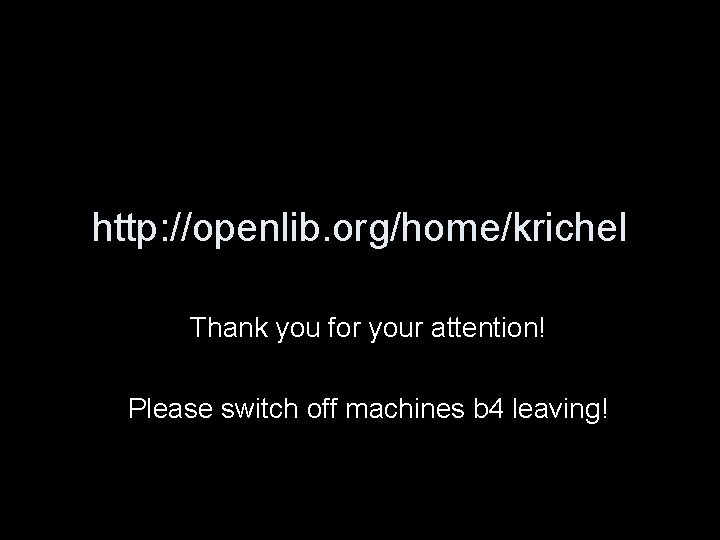
http: //openlib. org/home/krichel Thank you for your attention! Please switch off machines b 4 leaving!