LIS 651 lecture 3 functions and arrays Thomas
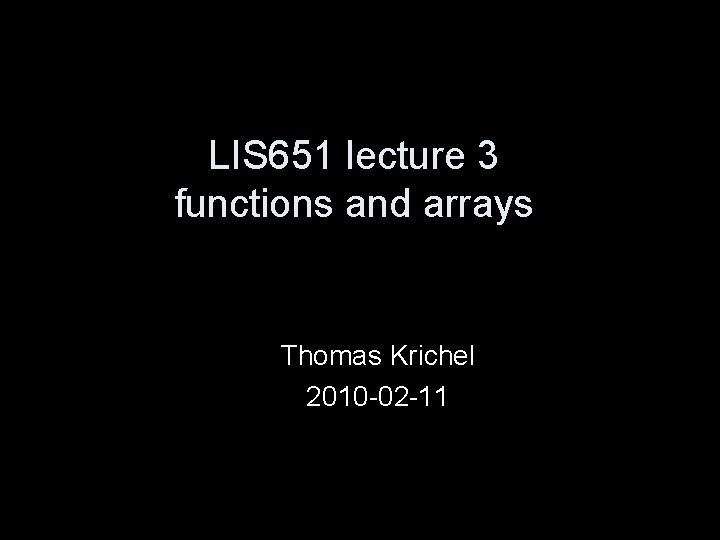
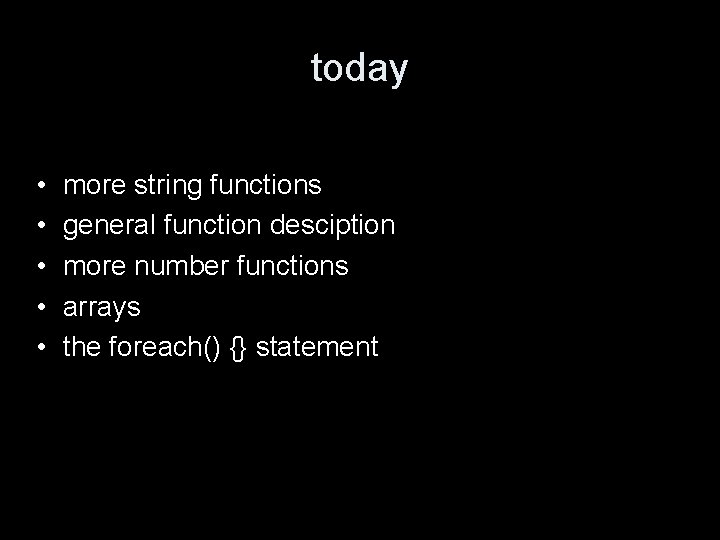
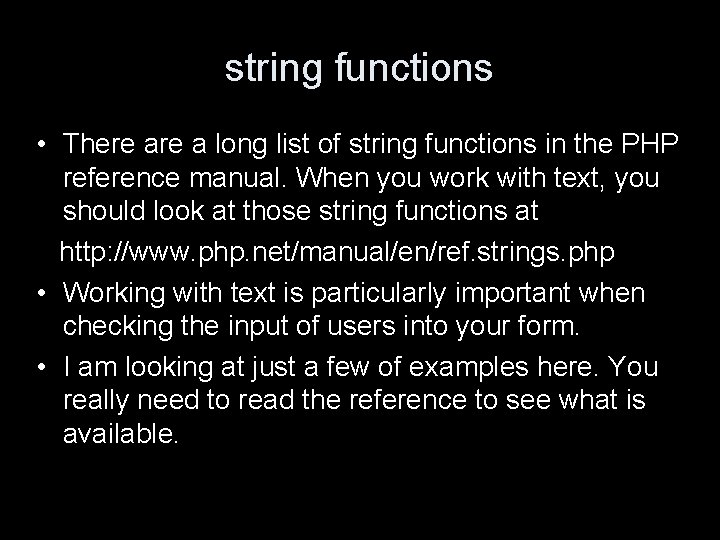
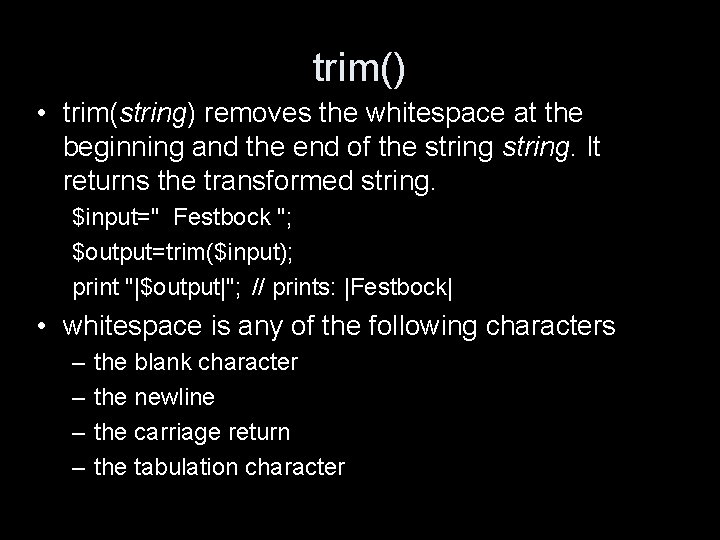
![strlen() • strlen(string) returns the length of the string. $zip=trim($_POST['zipcode']); $zip_length=strlen($zip); print $zip_length; // strlen() • strlen(string) returns the length of the string. $zip=trim($_POST['zipcode']); $zip_length=strlen($zip); print $zip_length; //](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-5.jpg)
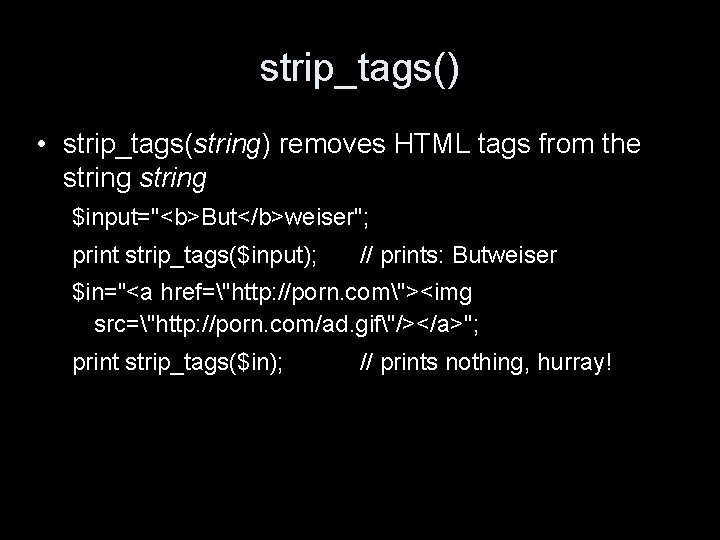
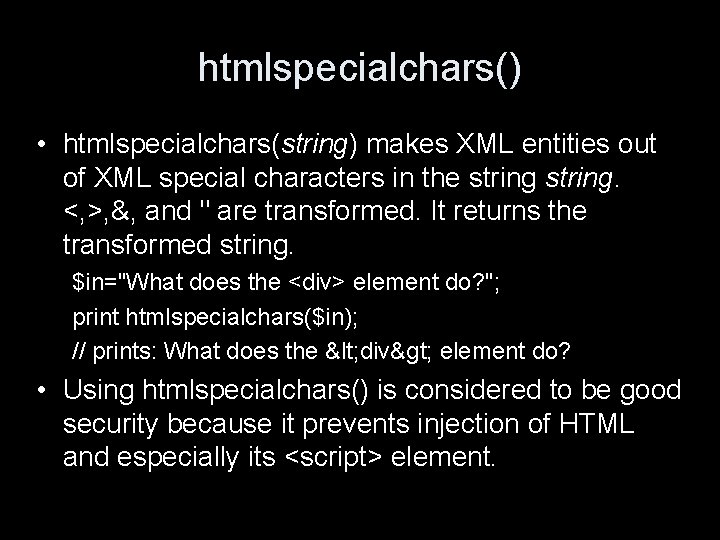
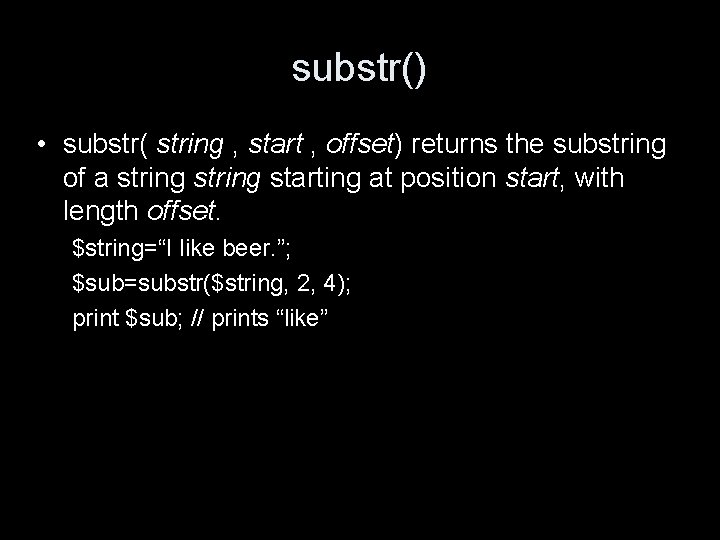
![documentation: description • • string trim ( string $str [, string $charlist ] ) documentation: description • • string trim ( string $str [, string $charlist ] )](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-9.jpg)
![argument list • string $str [, string $charlist ] • This argument list suggests argument list • string $str [, string $charlist ] • This argument list suggests](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-10.jpg)
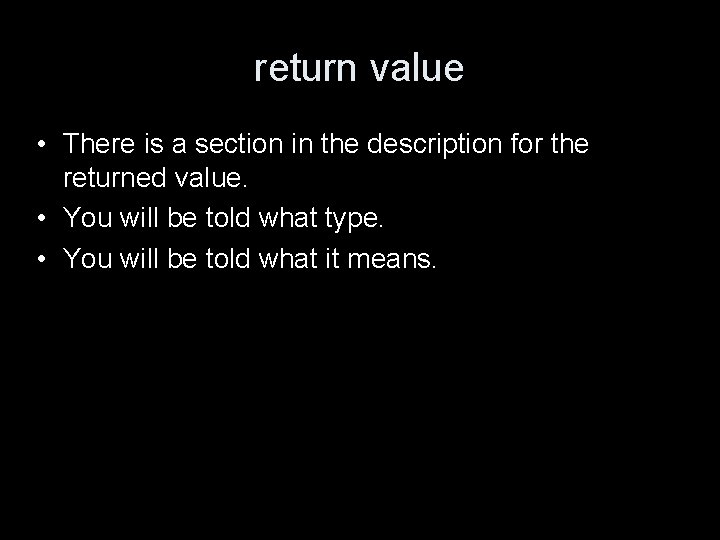
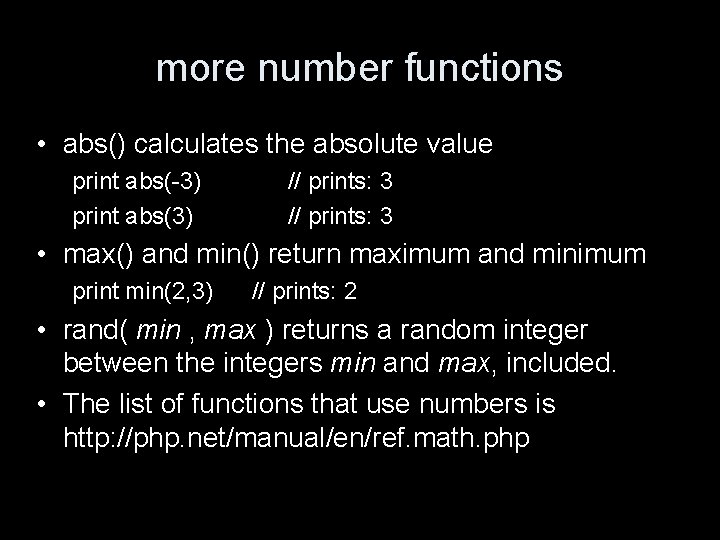
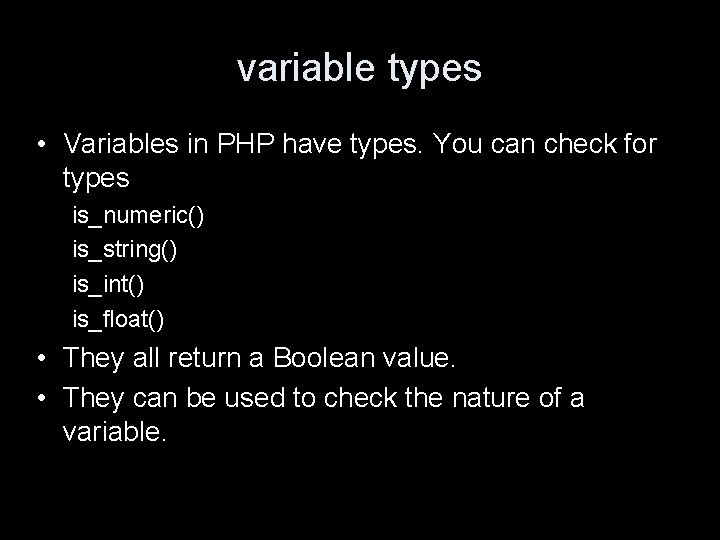
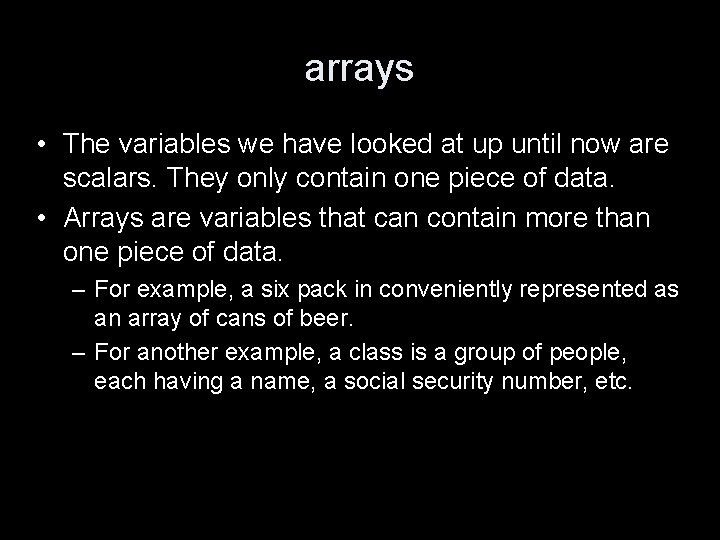
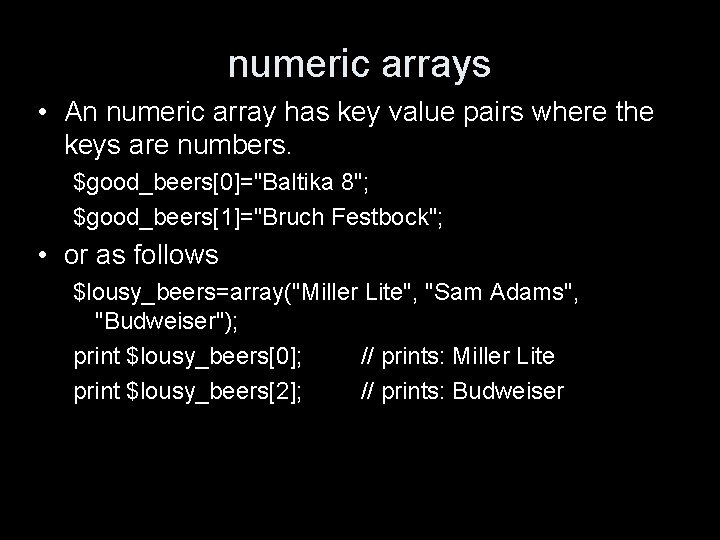
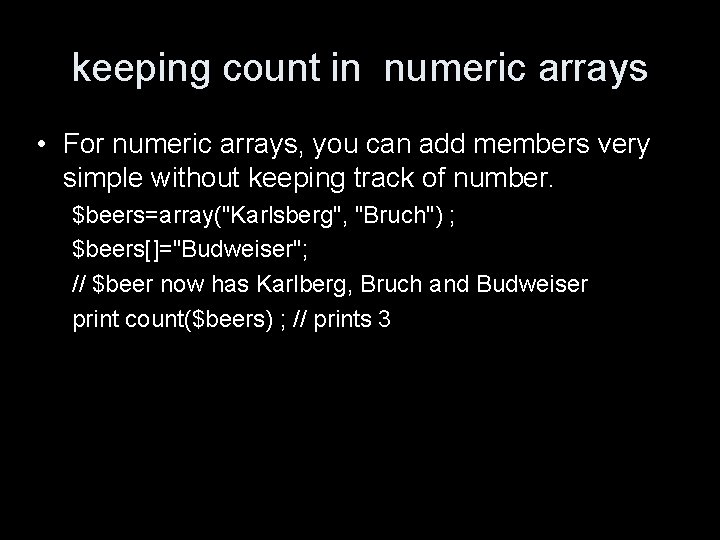
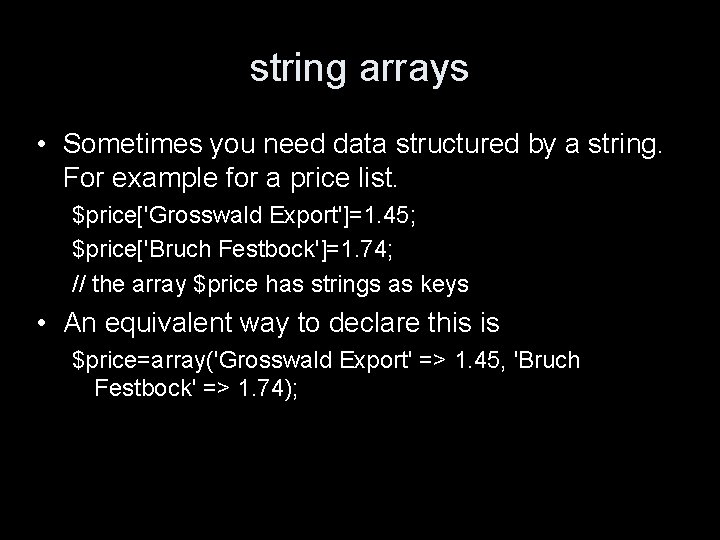
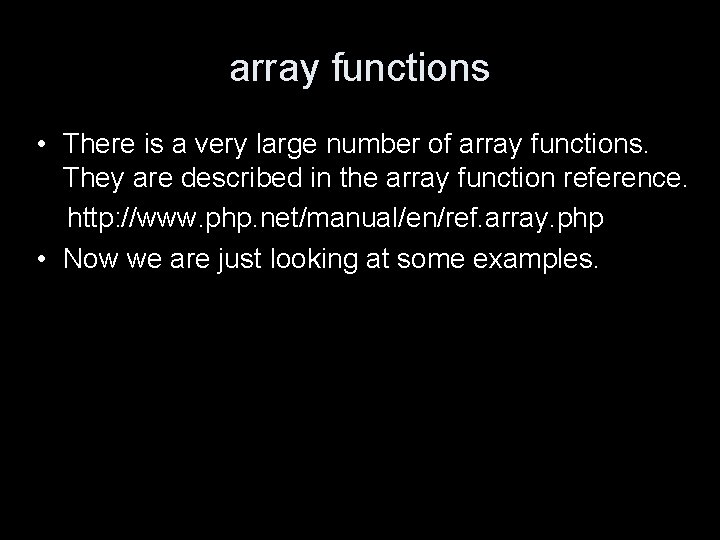
![count() • count() returns the size of an array $price['Grosswald Export']=1. 45; $price['Bruch Festbock']=1. count() • count() returns the size of an array $price['Grosswald Export']=1. 45; $price['Bruch Festbock']=1.](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-19.jpg)
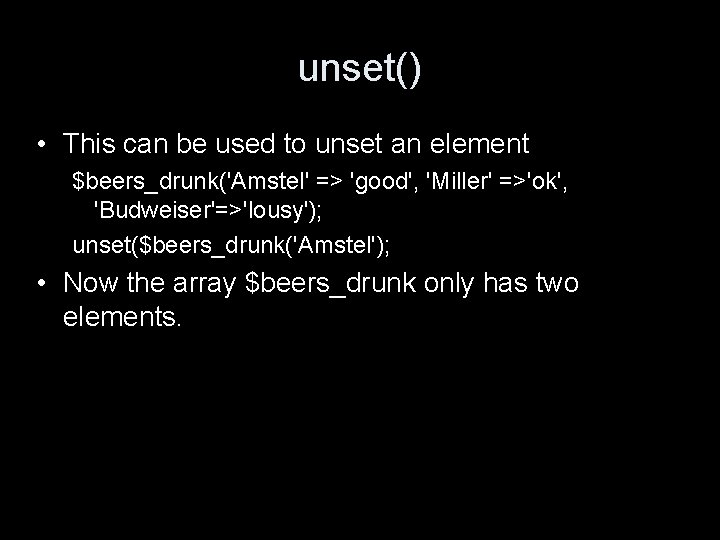
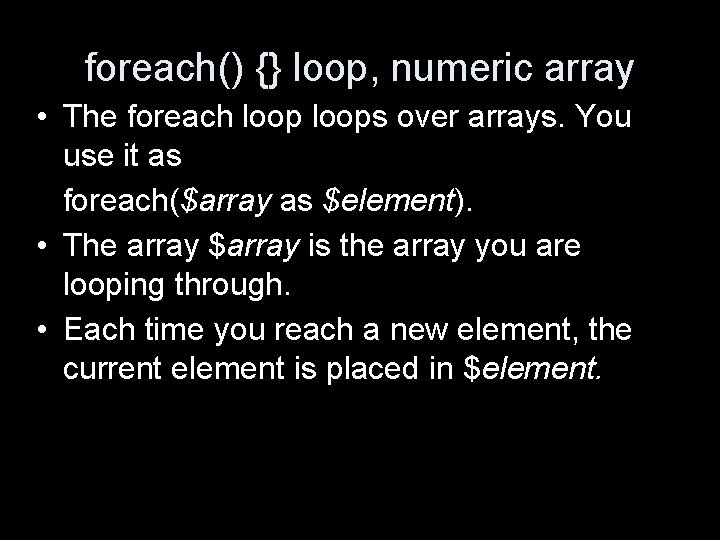
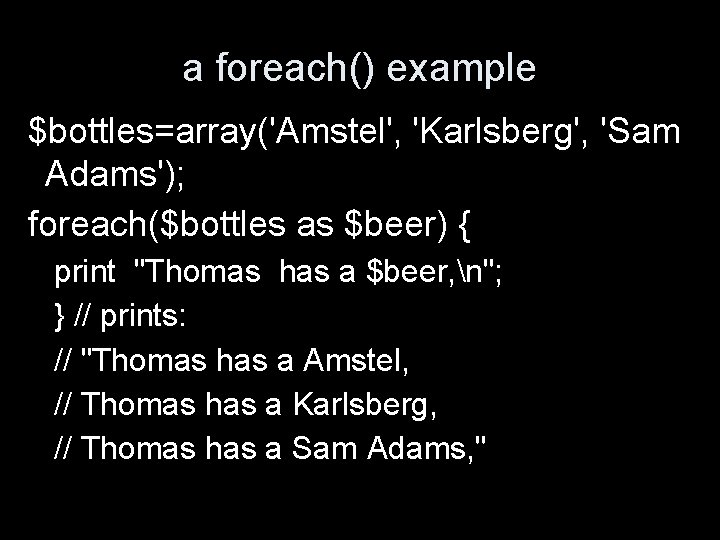
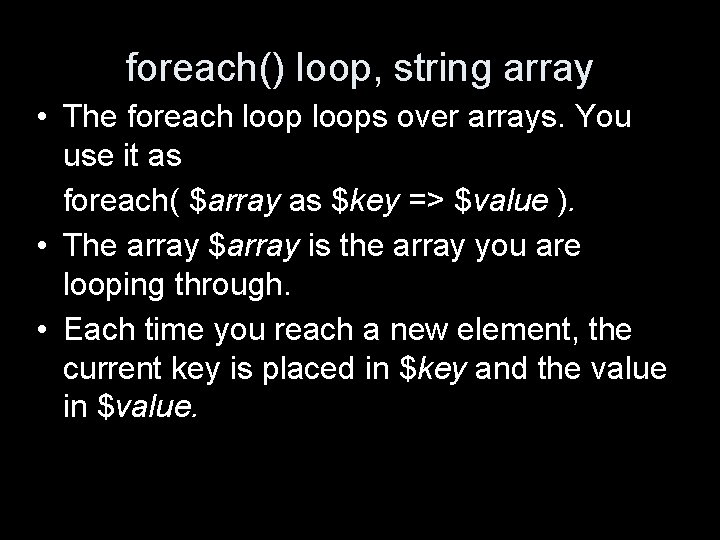
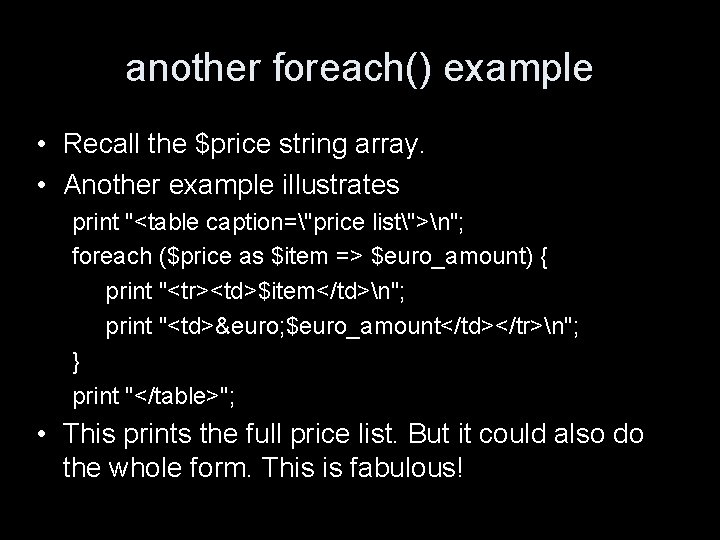
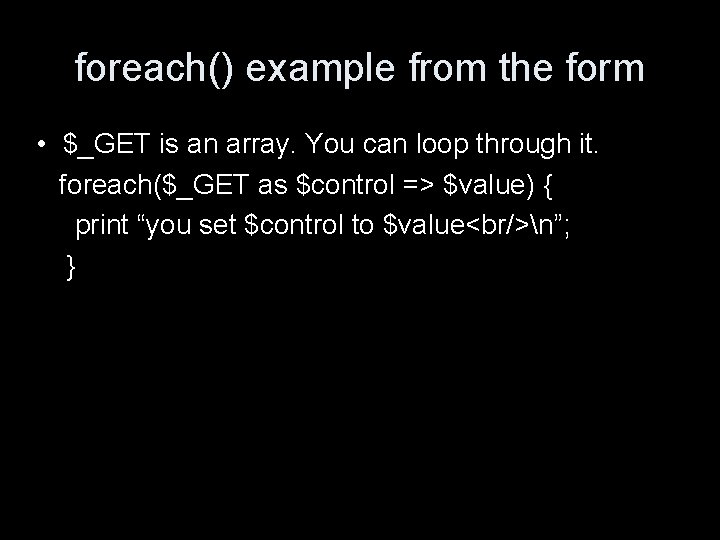
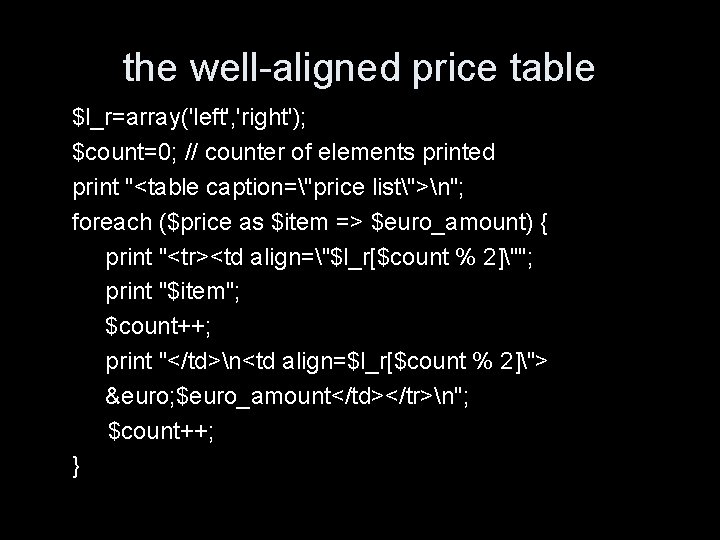
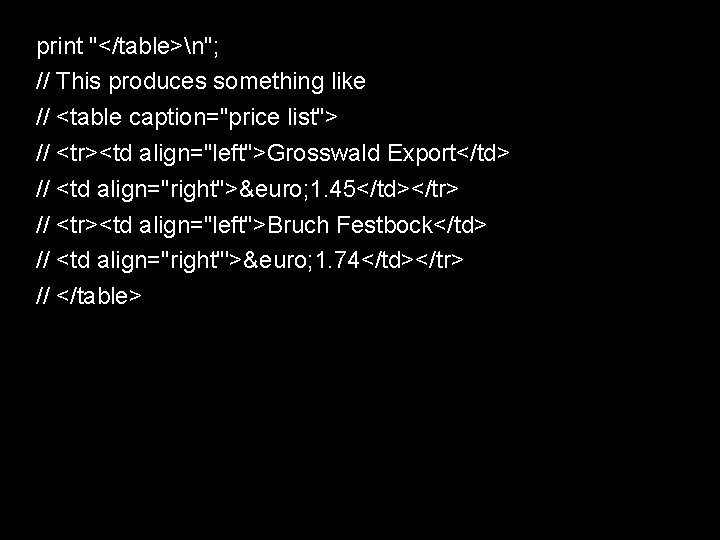
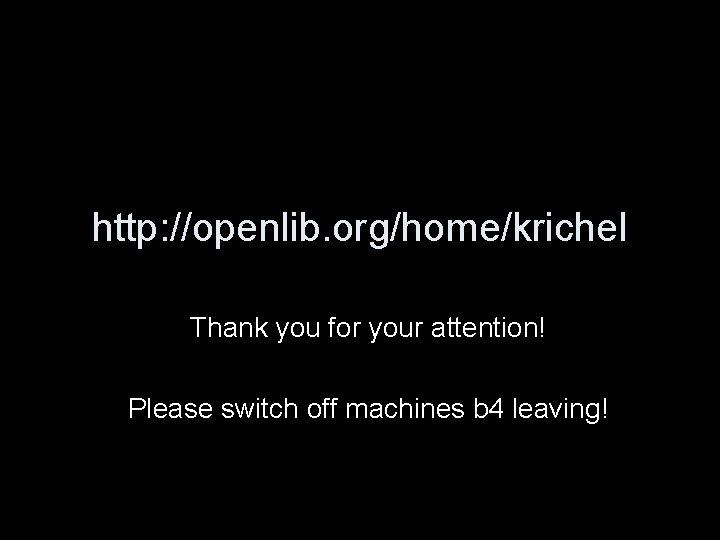
- Slides: 28
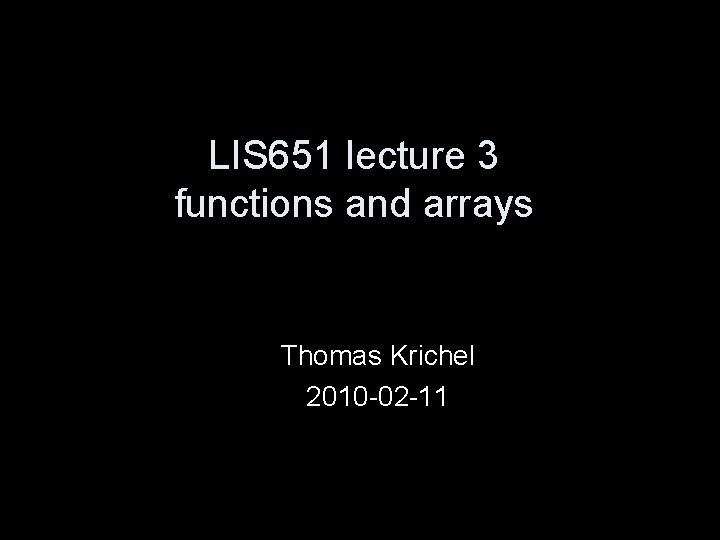
LIS 651 lecture 3 functions and arrays Thomas Krichel 2010 -02 -11
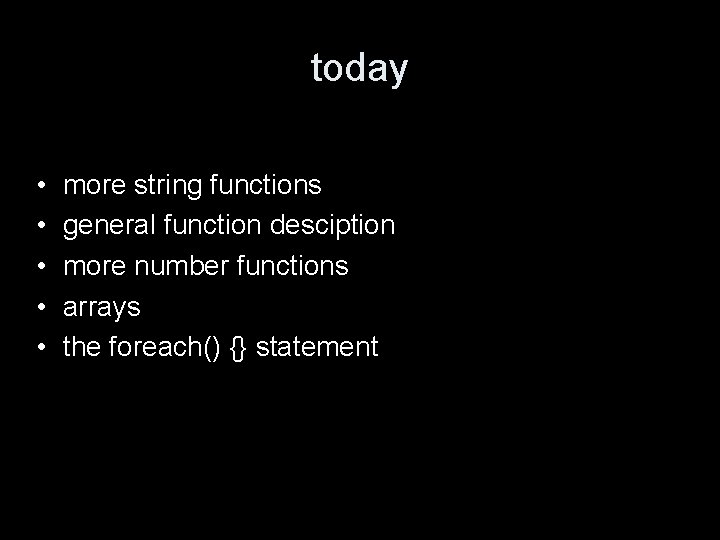
today • • • more string functions general function desciption more number functions arrays the foreach() {} statement
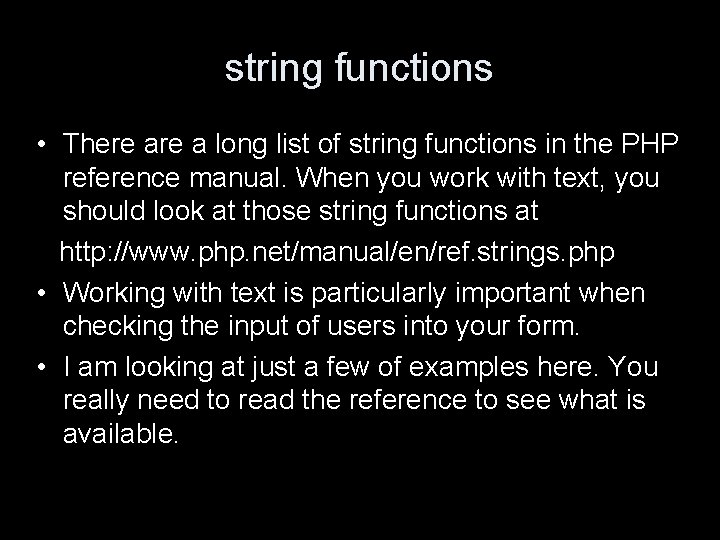
string functions • There a long list of string functions in the PHP reference manual. When you work with text, you should look at those string functions at http: //www. php. net/manual/en/ref. strings. php • Working with text is particularly important when checking the input of users into your form. • I am looking at just a few of examples here. You really need to read the reference to see what is available.
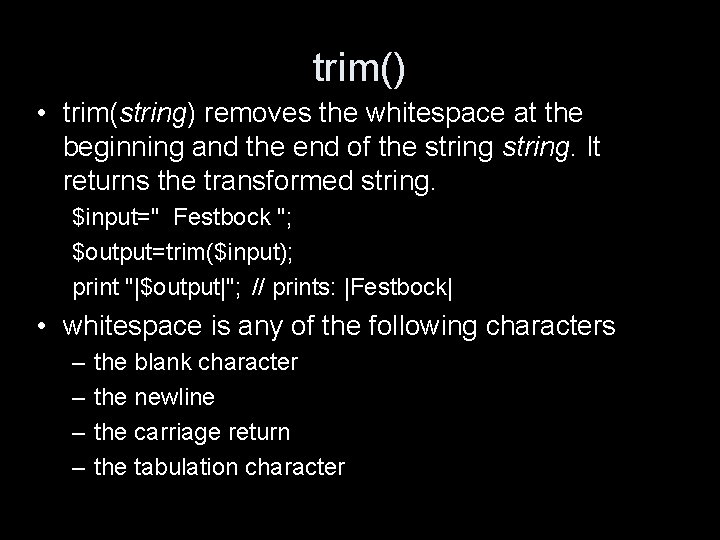
trim() • trim(string) removes the whitespace at the beginning and the end of the string. It returns the transformed string. $input=" Festbock "; $output=trim($input); print "|$output|"; // prints: |Festbock| • whitespace is any of the following characters – – the blank character the newline the carriage return the tabulation character
![strlen strlenstring returns the length of the string ziptrimPOSTzipcode ziplengthstrlenzip print ziplength strlen() • strlen(string) returns the length of the string. $zip=trim($_POST['zipcode']); $zip_length=strlen($zip); print $zip_length; //](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-5.jpg)
strlen() • strlen(string) returns the length of the string. $zip=trim($_POST['zipcode']); $zip_length=strlen($zip); print $zip_length; // hopefully, prints 5
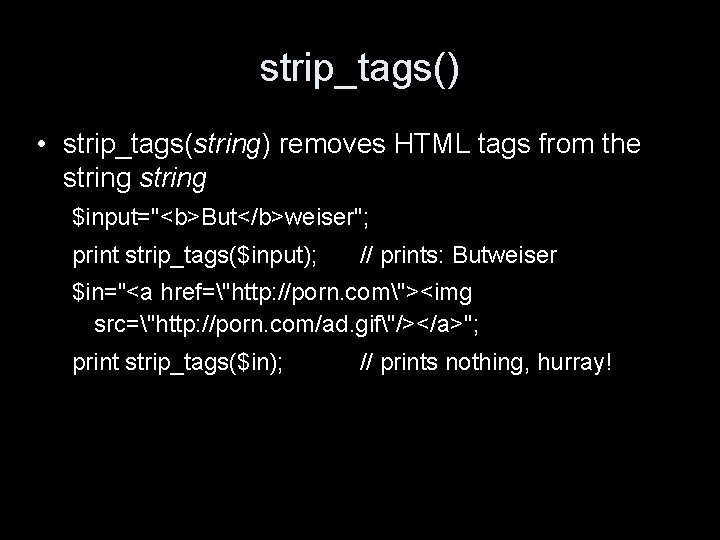
strip_tags() • strip_tags(string) removes HTML tags from the string $input="<b>But</b>weiser"; print strip_tags($input); // prints: Butweiser $in="<a href="http: //porn. com"><img src="http: //porn. com/ad. gif"/></a>"; print strip_tags($in); // prints nothing, hurray!
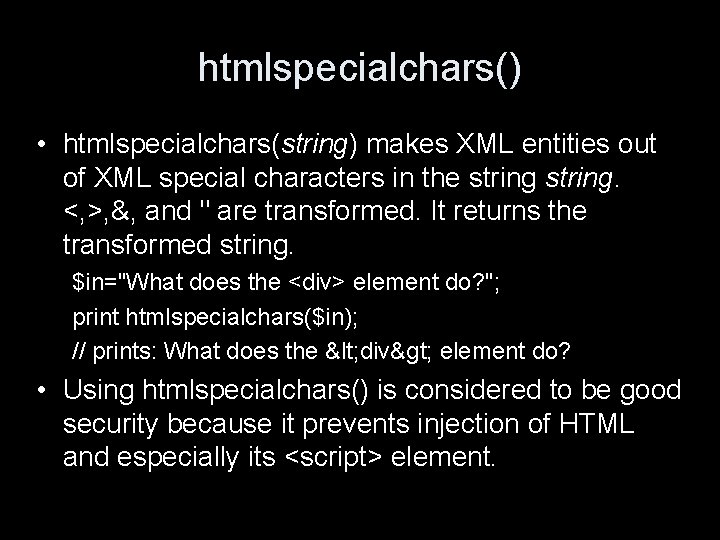
htmlspecialchars() • htmlspecialchars(string) makes XML entities out of XML special characters in the string. <, >, &, and " are transformed. It returns the transformed string. $in="What does the <div> element do? "; print htmlspecialchars($in); // prints: What does the < div> element do? • Using htmlspecialchars() is considered to be good security because it prevents injection of HTML and especially its <script> element.
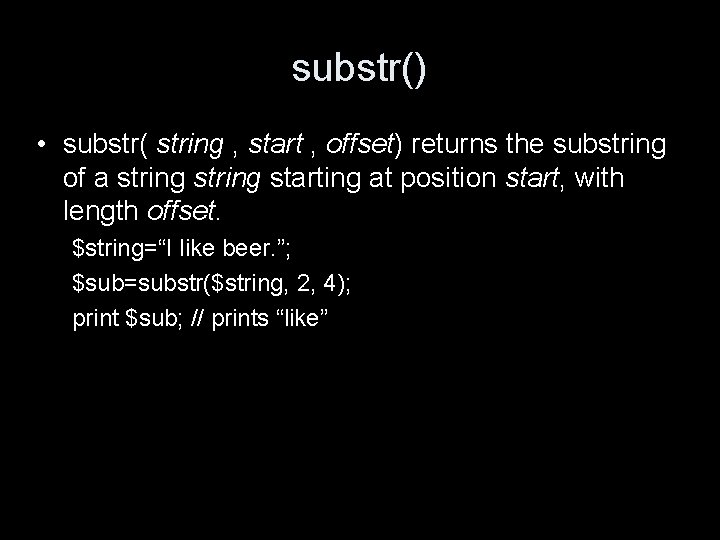
substr() • substr( string , start , offset) returns the substring of a string starting at position start, with length offset. $string=“I like beer. ”; $sub=substr($string, 2, 4); print $sub; // prints “like”
![documentation description string trim string str string charlist documentation: description • • string trim ( string $str [, string $charlist ] )](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-9.jpg)
documentation: description • • string trim ( string $str [, string $charlist ] ) here "string" at the beginning tells us that the function returns a variable of type string. The name of the function is written in bold. Then comes the parenthesis. It encloses the arguments. Anything that is optional is enclosed in square brackets. Don't put the square bracket in the function call.
![argument list string str string charlist This argument list suggests argument list • string $str [, string $charlist ] • This argument list suggests](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-10.jpg)
argument list • string $str [, string $charlist ] • This argument list suggests – that you have to give a first argument that is a string (or will be converted to it) – that there is an optional argument that also is a string – arguments are separated by comma. • The arguments are described in the parameters section.
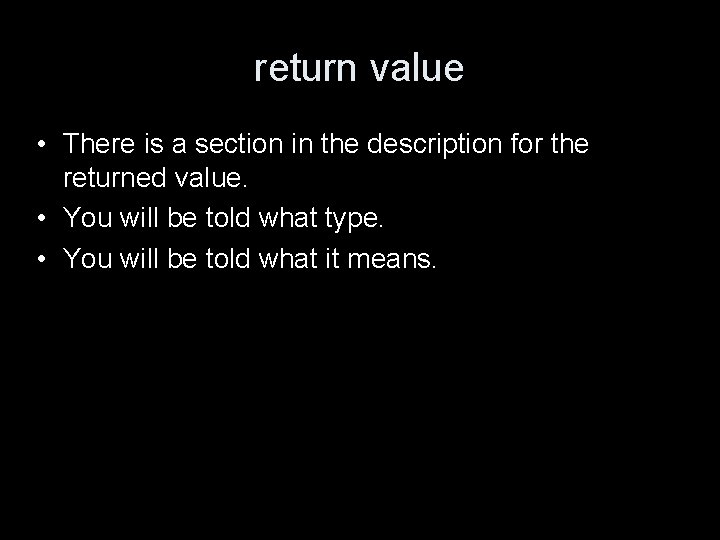
return value • There is a section in the description for the returned value. • You will be told what type. • You will be told what it means.
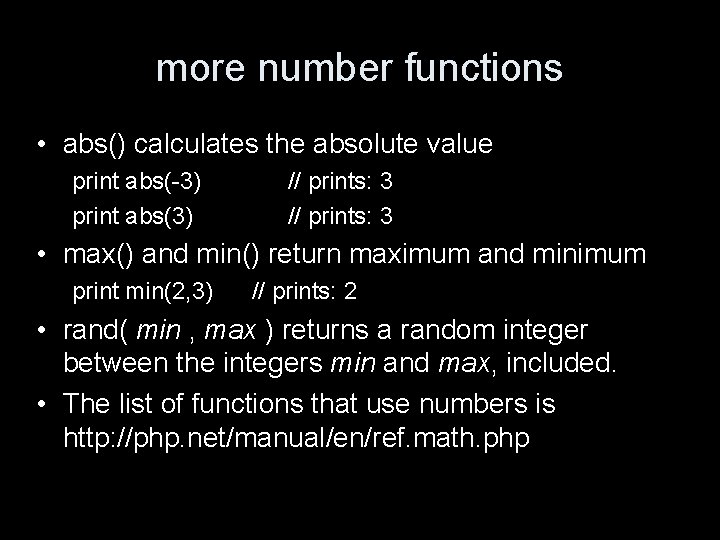
more number functions • abs() calculates the absolute value print abs(-3) print abs(3) // prints: 3 • max() and min() return maximum and minimum print min(2, 3) // prints: 2 • rand( min , max ) returns a random integer between the integers min and max, included. • The list of functions that use numbers is http: //php. net/manual/en/ref. math. php
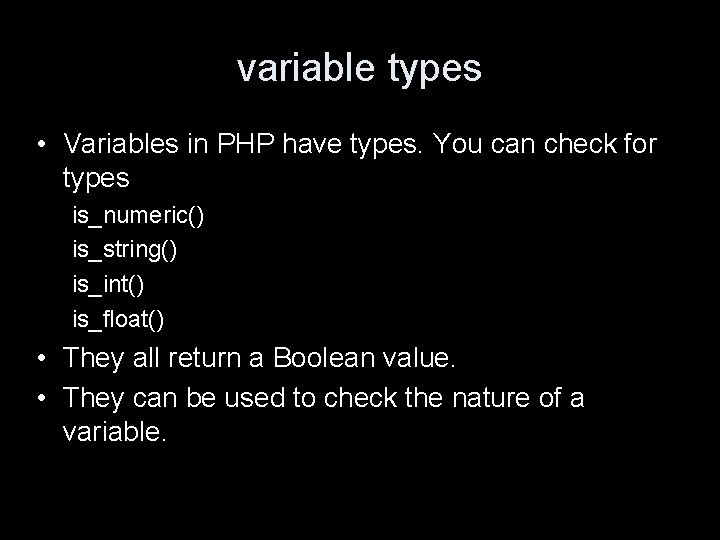
variable types • Variables in PHP have types. You can check for types is_numeric() is_string() is_int() is_float() • They all return a Boolean value. • They can be used to check the nature of a variable.
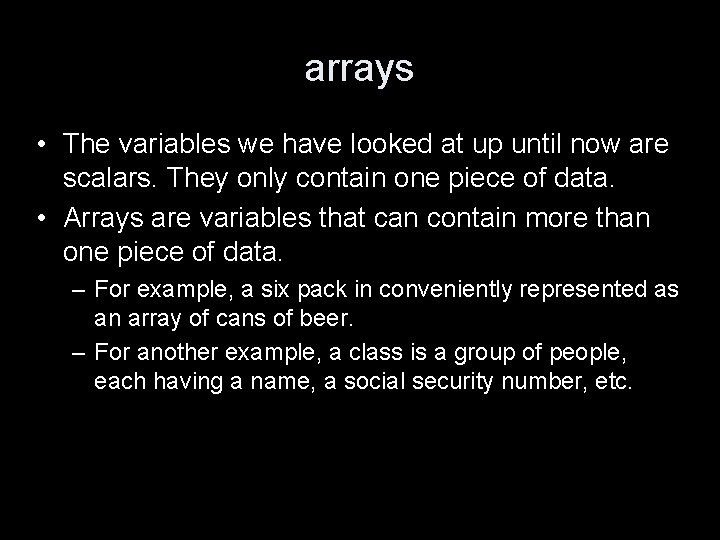
arrays • The variables we have looked at up until now are scalars. They only contain one piece of data. • Arrays are variables that can contain more than one piece of data. – For example, a six pack in conveniently represented as an array of cans of beer. – For another example, a class is a group of people, each having a name, a social security number, etc.
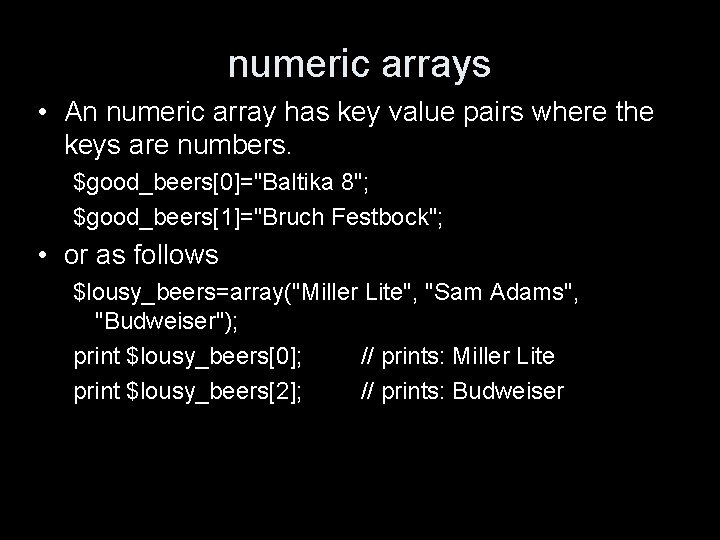
numeric arrays • An numeric array has key value pairs where the keys are numbers. $good_beers[0]="Baltika 8"; $good_beers[1]="Bruch Festbock"; • or as follows $lousy_beers=array("Miller Lite", "Sam Adams", "Budweiser"); print $lousy_beers[0]; // prints: Miller Lite print $lousy_beers[2]; // prints: Budweiser
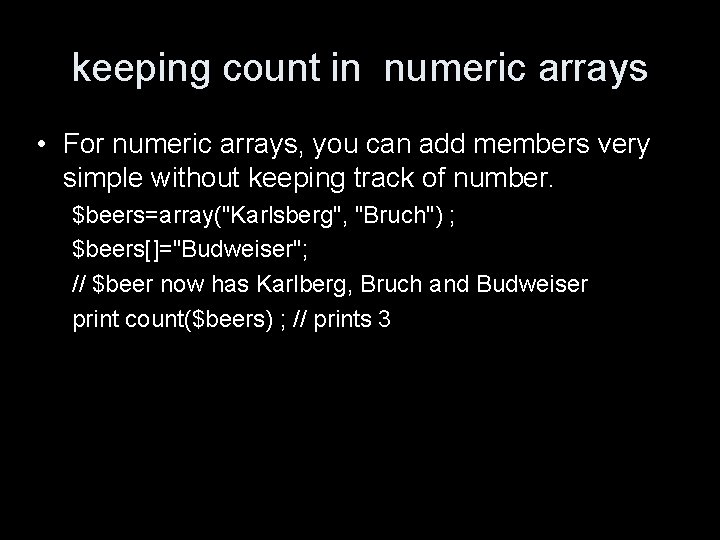
keeping count in numeric arrays • For numeric arrays, you can add members very simple without keeping track of number. $beers=array("Karlsberg", "Bruch") ; $beers[]="Budweiser"; // $beer now has Karlberg, Bruch and Budweiser print count($beers) ; // prints 3
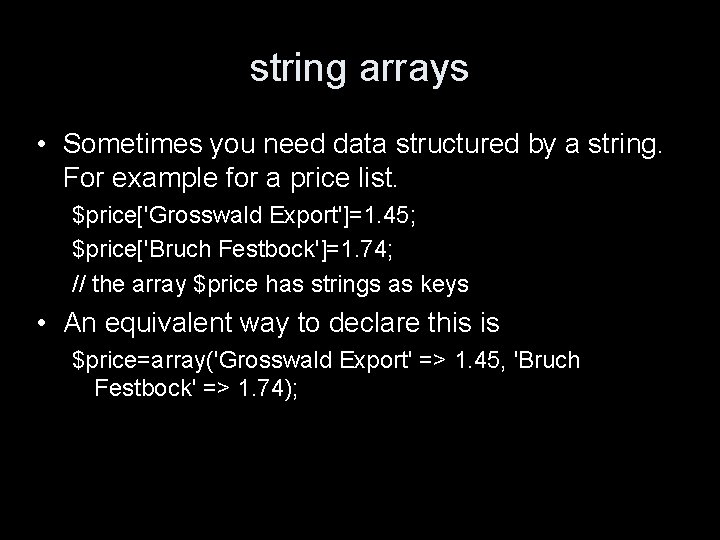
string arrays • Sometimes you need data structured by a string. For example for a price list. $price['Grosswald Export']=1. 45; $price['Bruch Festbock']=1. 74; // the array $price has strings as keys • An equivalent way to declare this is $price=array('Grosswald Export' => 1. 45, 'Bruch Festbock' => 1. 74);
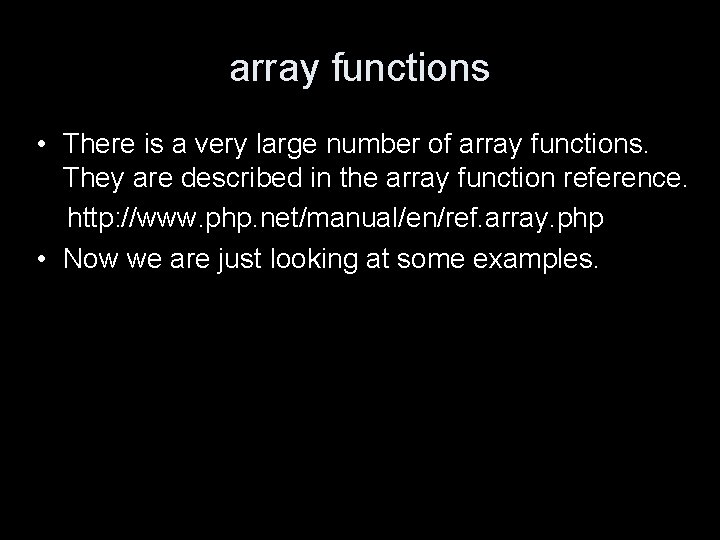
array functions • There is a very large number of array functions. They are described in the array function reference. http: //www. php. net/manual/en/ref. array. php • Now we are just looking at some examples.
![count count returns the size of an array priceGrosswald Export1 45 priceBruch Festbock1 count() • count() returns the size of an array $price['Grosswald Export']=1. 45; $price['Bruch Festbock']=1.](https://slidetodoc.com/presentation_image_h2/a7357530cb5a9a5f0560b5a0cd630f4d/image-19.jpg)
count() • count() returns the size of an array $price['Grosswald Export']=1. 45; $price['Bruch Festbock']=1. 74; $product_number=count($price); print "We have $product_number products for you today. "; // prints: We have 2 products for you today.
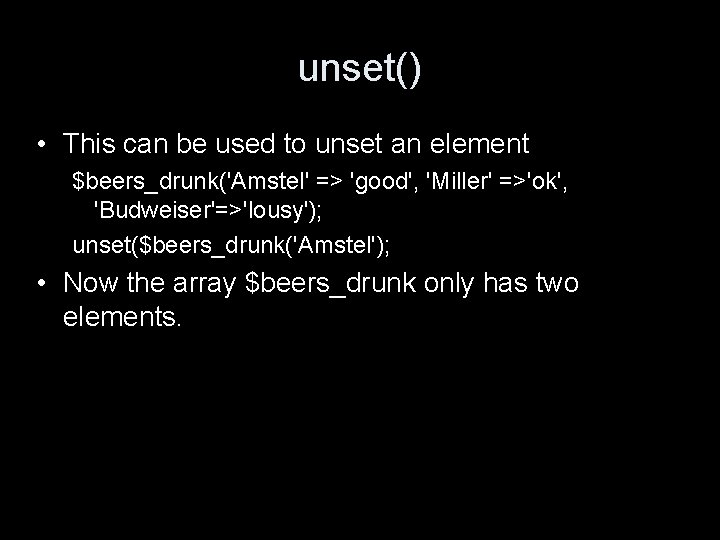
unset() • This can be used to unset an element $beers_drunk('Amstel' => 'good', 'Miller' =>'ok', 'Budweiser'=>'lousy'); unset($beers_drunk('Amstel'); • Now the array $beers_drunk only has two elements.
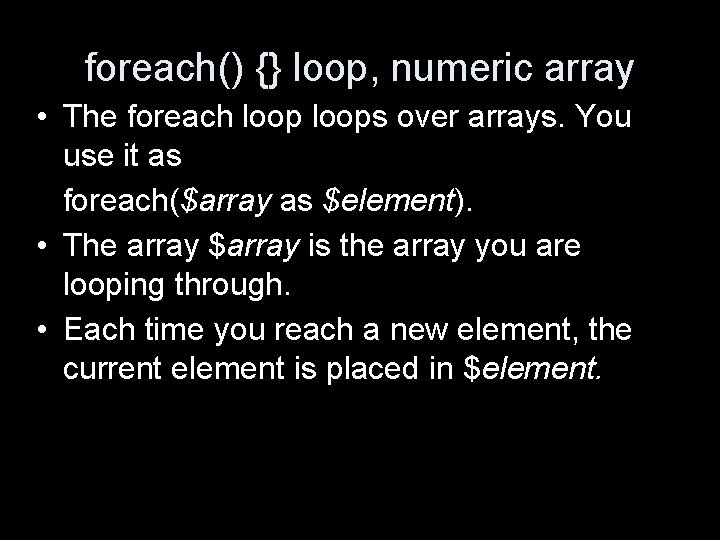
foreach() {} loop, numeric array • The foreach loops over arrays. You use it as foreach($array as $element). • The array $array is the array you are looping through. • Each time you reach a new element, the current element is placed in $element.
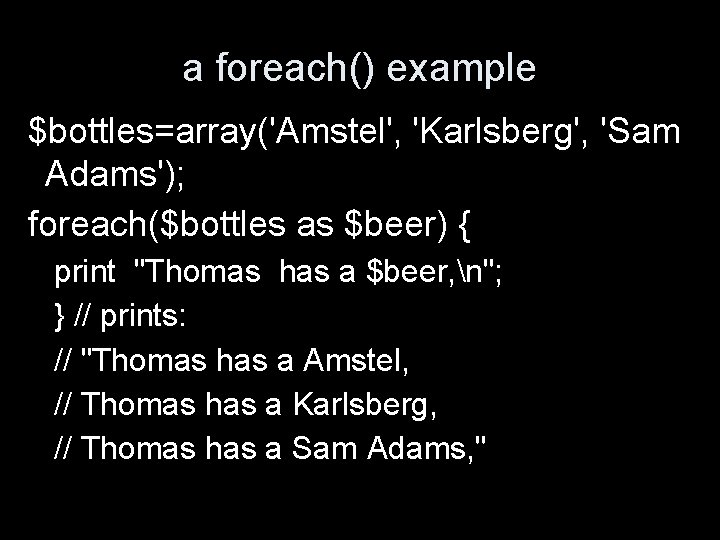
a foreach() example $bottles=array('Amstel', 'Karlsberg', 'Sam Adams'); foreach($bottles as $beer) { print "Thomas has a $beer, n"; } // prints: // "Thomas has a Amstel, // Thomas has a Karlsberg, // Thomas has a Sam Adams, "
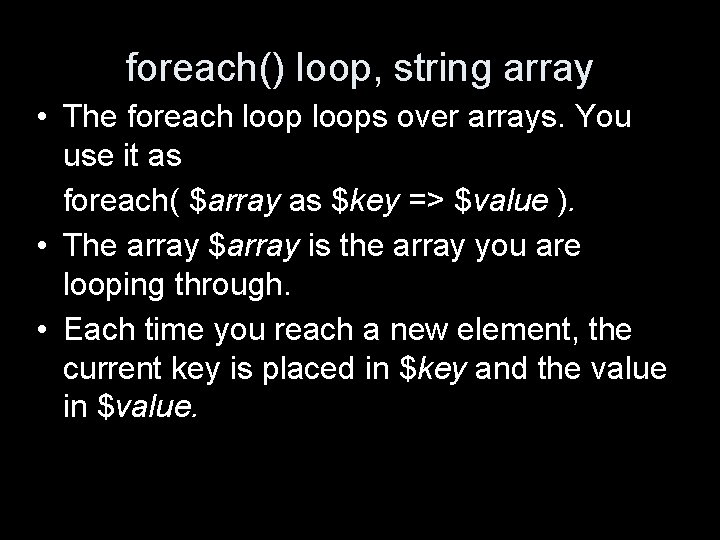
foreach() loop, string array • The foreach loops over arrays. You use it as foreach( $array as $key => $value ). • The array $array is the array you are looping through. • Each time you reach a new element, the current key is placed in $key and the value in $value.
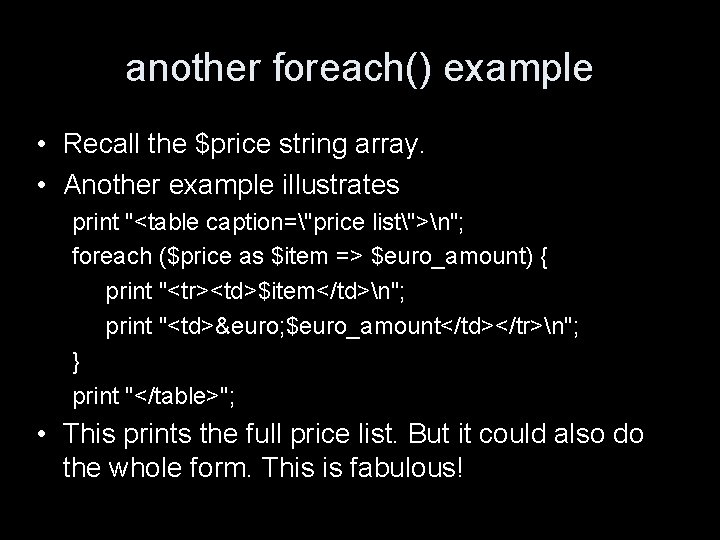
another foreach() example • Recall the $price string array. • Another example illustrates print "<table caption="price list">n"; foreach ($price as $item => $euro_amount) { print "<tr><td>$item</td>n"; print "<td>€ $euro_amount</td></tr>n"; } print "</table>"; • This prints the full price list. But it could also do the whole form. This is fabulous!
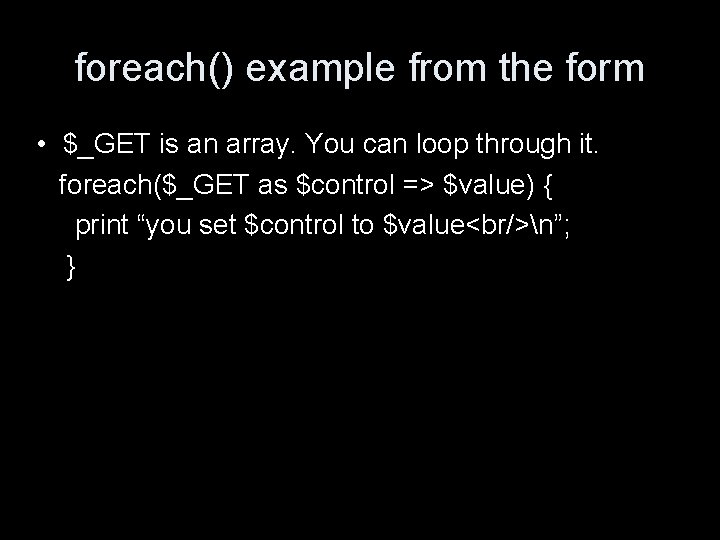
foreach() example from the form • $_GET is an array. You can loop through it. foreach($_GET as $control => $value) { print “you set $control to $value<br/>n”; }
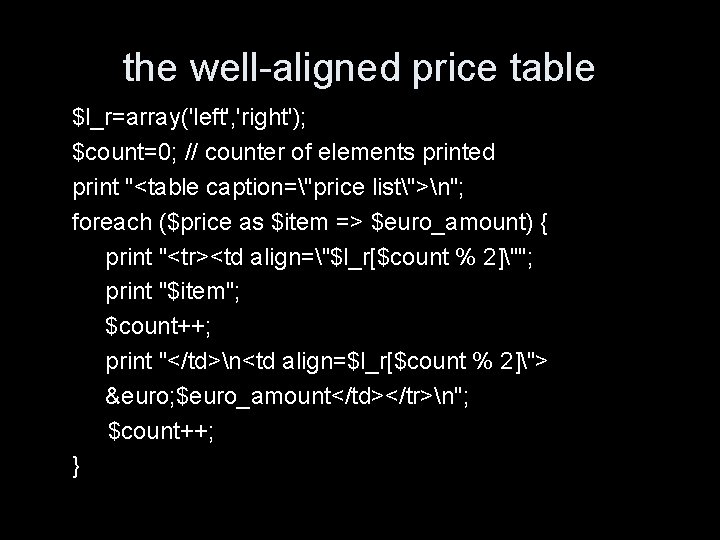
the well-aligned price table $l_r=array('left', 'right'); $count=0; // counter of elements printed print "<table caption="price list">n"; foreach ($price as $item => $euro_amount) { print "<tr><td align="$l_r[$count % 2]""; print "$item"; $count++; print "</td>n<td align=$l_r[$count % 2]"> € $euro_amount</td></tr>n"; $count++; }
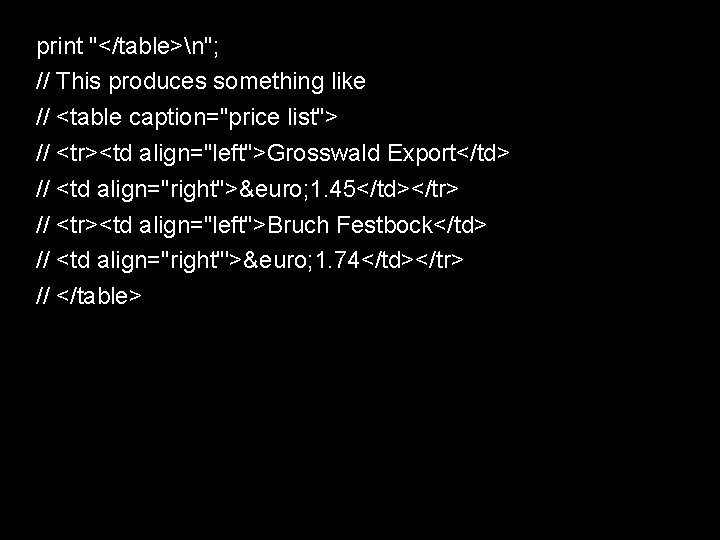
print "</table>n"; // This produces something like // <table caption="price list"> // <tr><td align="left">Grosswald Export</td> // <td align="right">€ 1. 45</td></tr> // <tr><td align="left">Bruch Festbock</td> // <td align="right"'>€ 1. 74</td></tr> // </table>
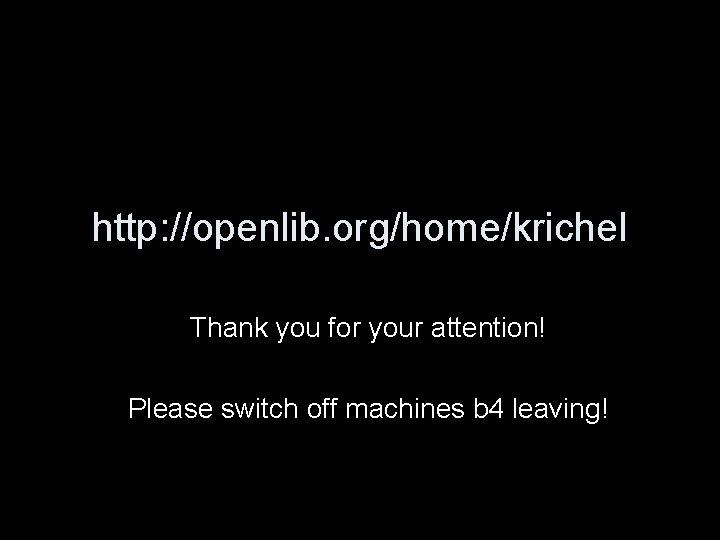
http: //openlib. org/home/krichel Thank you for your attention! Please switch off machines b 4 leaving!
500-651 exam dumps
Ece 651
Ece 651
Lei 12 651
01:640:244 lecture notes - lecture 15: plat, idah, farad
Forensic pathologist vs forensic anthropologist
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
What are the disadvantages of arrays
Parallel arrays python
Array of arrays c++
Parallel arrays
Partially filled arrays
C++ parallel arrays
Why do we need arrays?
Suma de arreglos unidimensionales en c
Arreglos bidimensionales en java
Arrays mips
Polynomial representation using arrays
Arrays in arm assembly
Global arrays in c
Computer science arrays
Arrays visual basic
Python parallel arrays
I wonder is it possible
Pascal multidimensional array
Mips dynamic array
Creating arrays matlab
Adt of array