Life Cycle of an Applet along with response
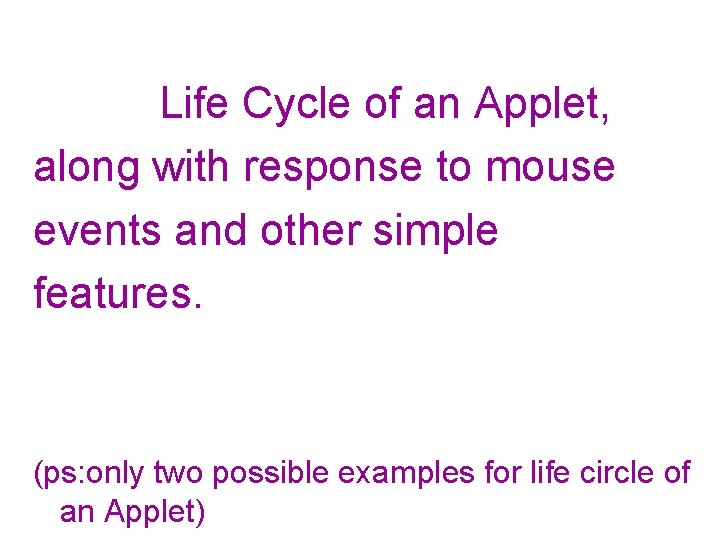
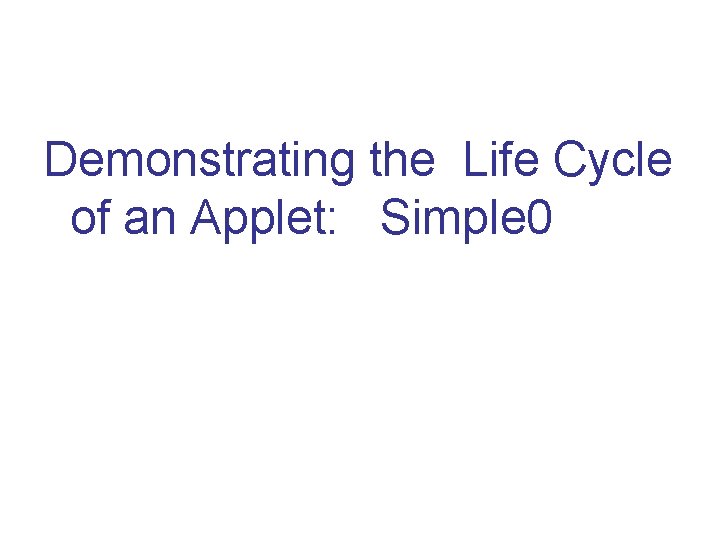
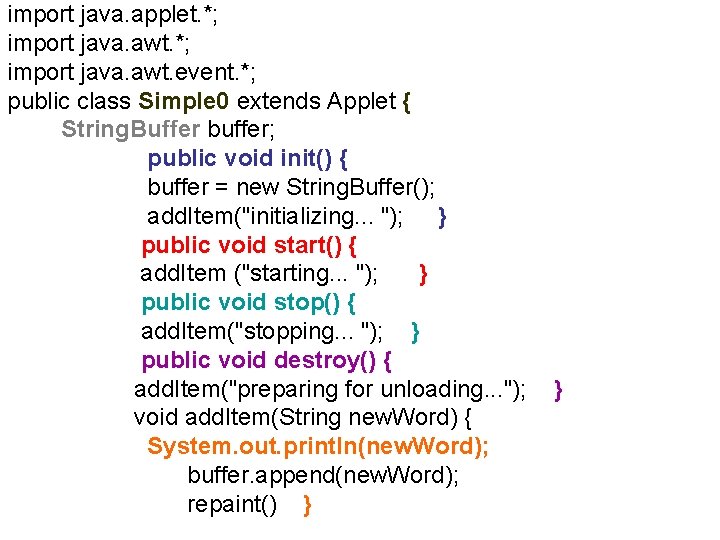
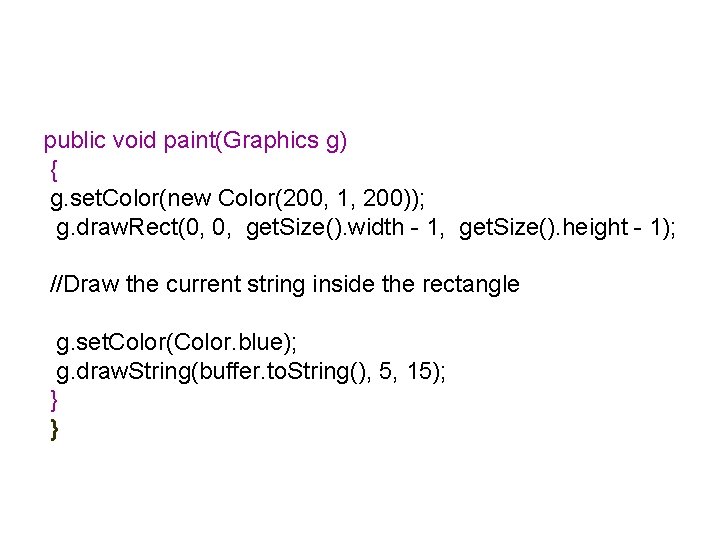
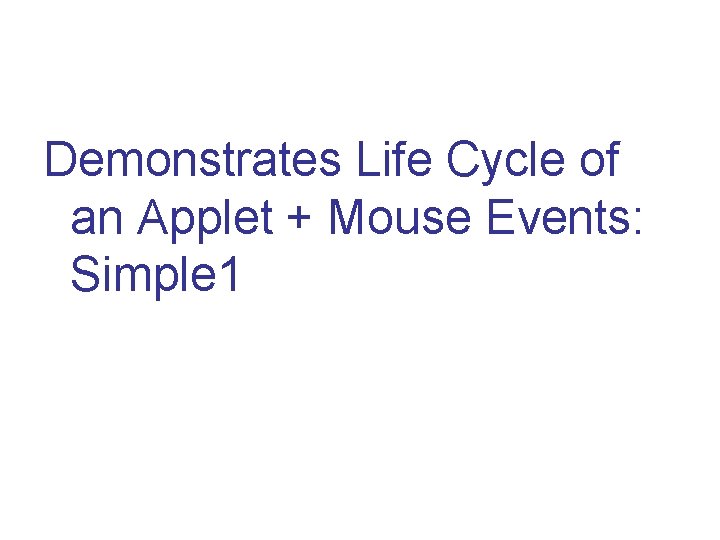
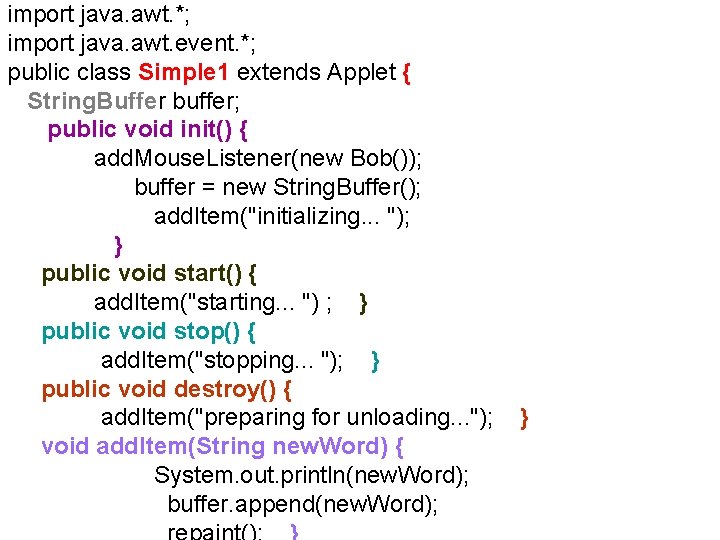
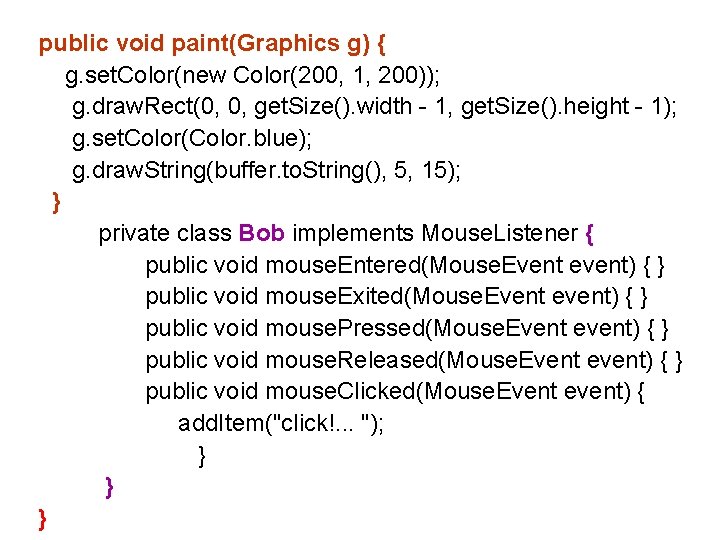
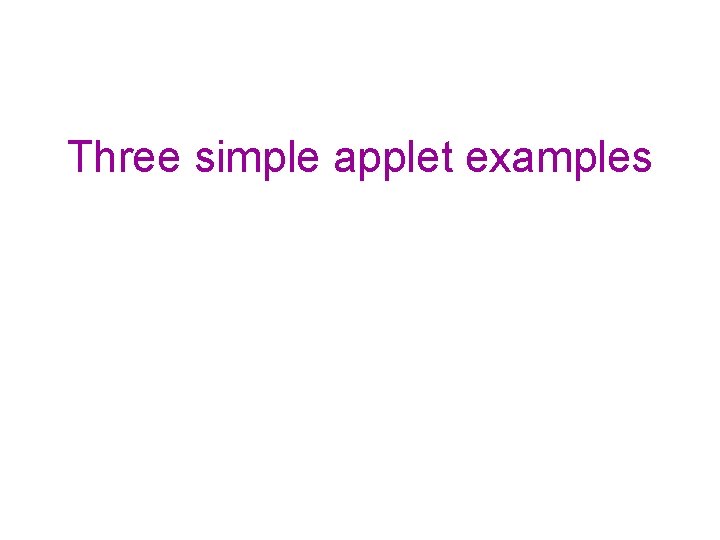
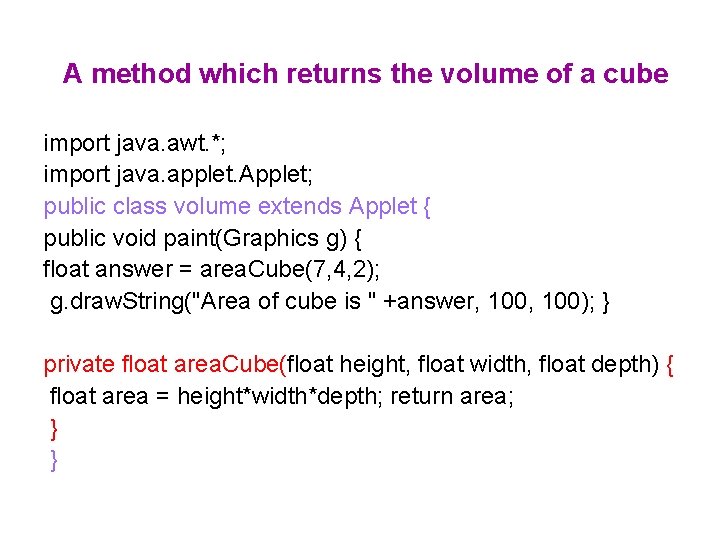
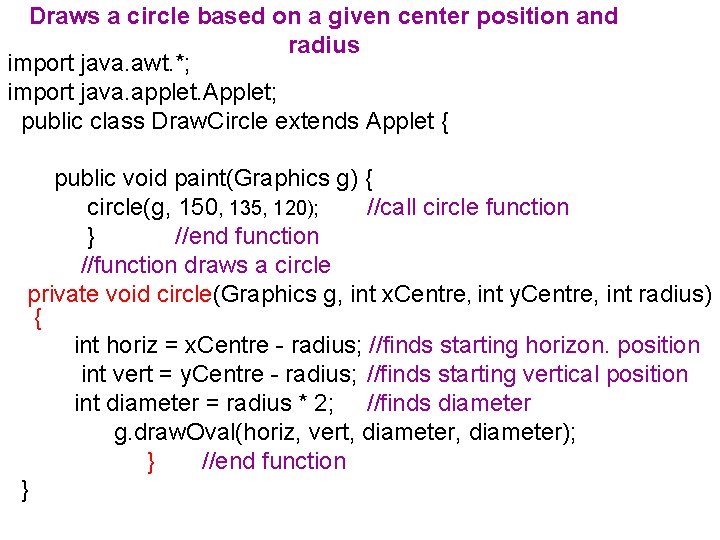
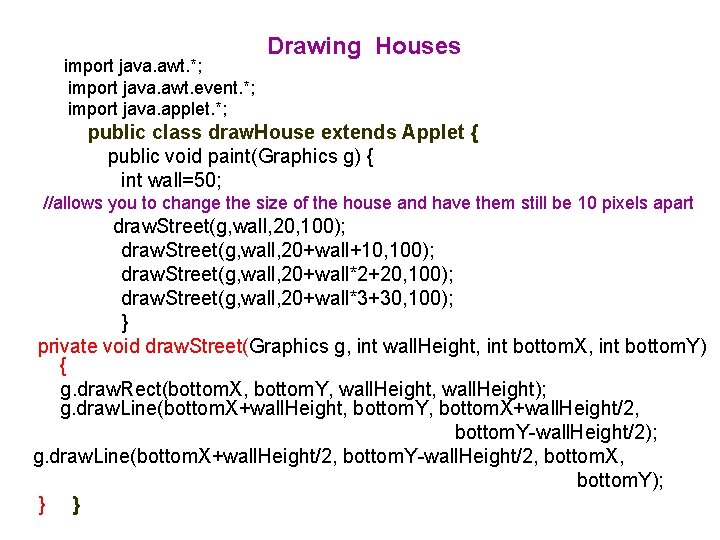
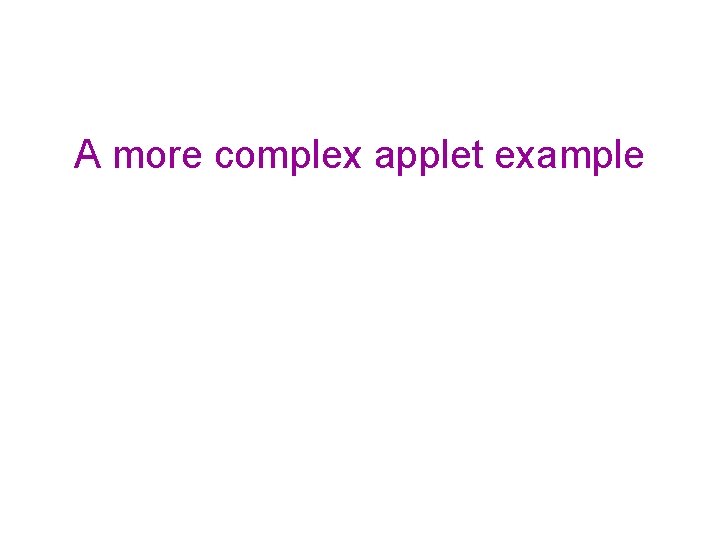
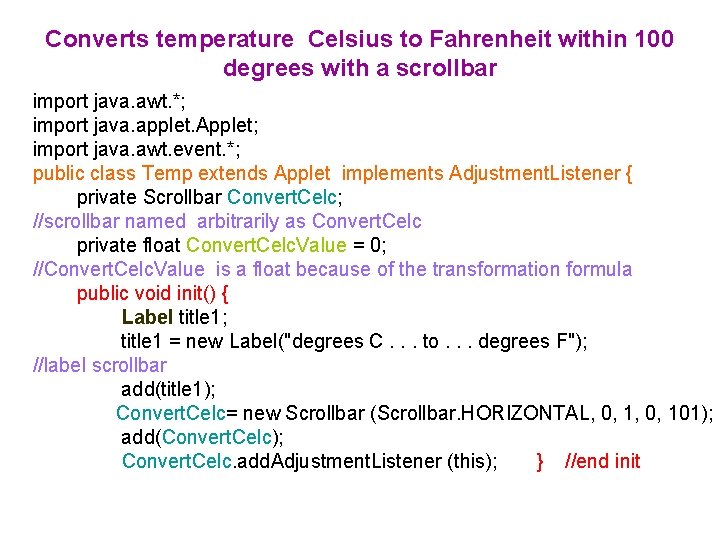
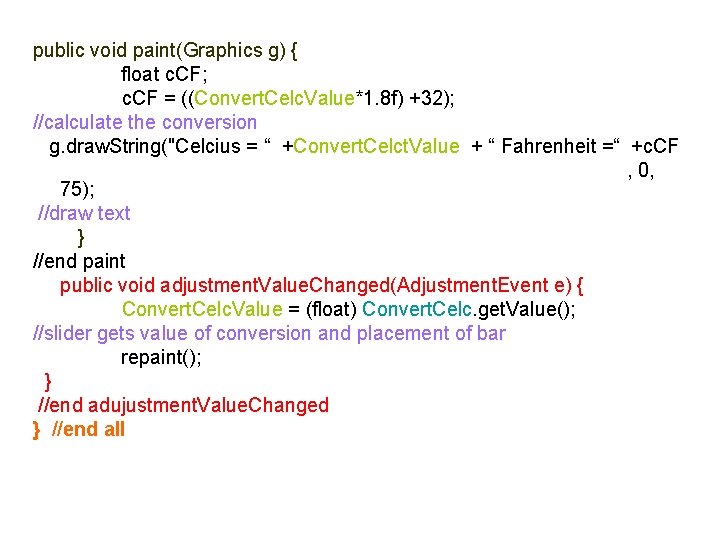
- Slides: 14
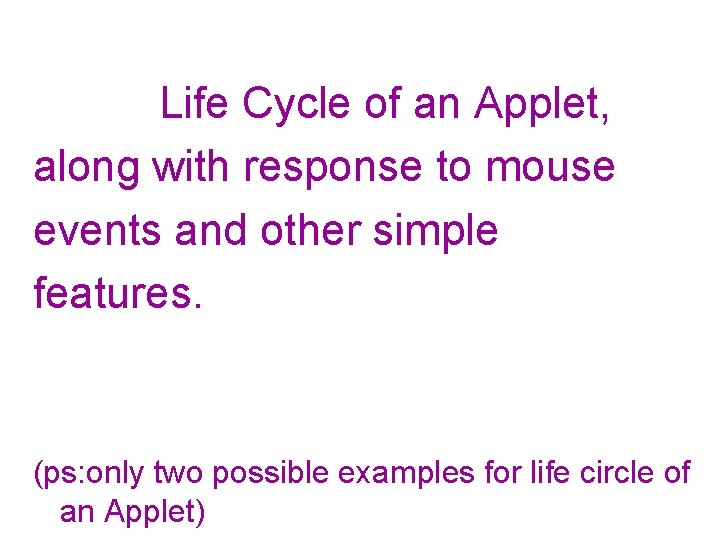
Life Cycle of an Applet, along with response to mouse events and other simple features. (ps: only two possible examples for life circle of an Applet)
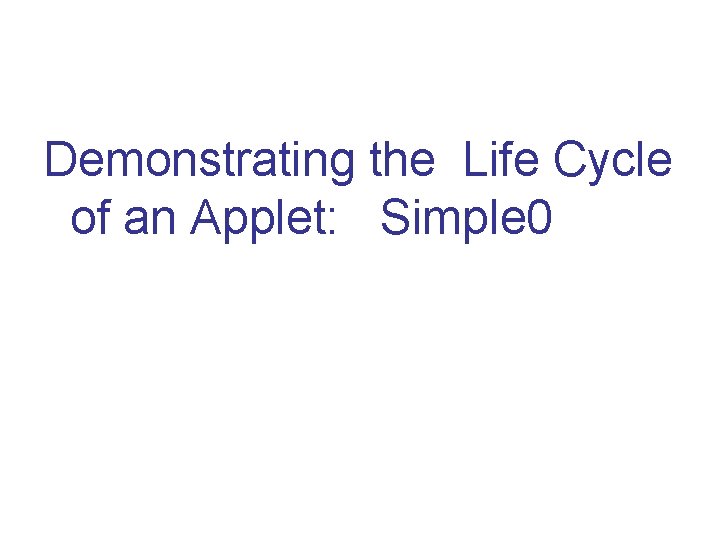
Demonstrating the Life Cycle of an Applet: Simple 0
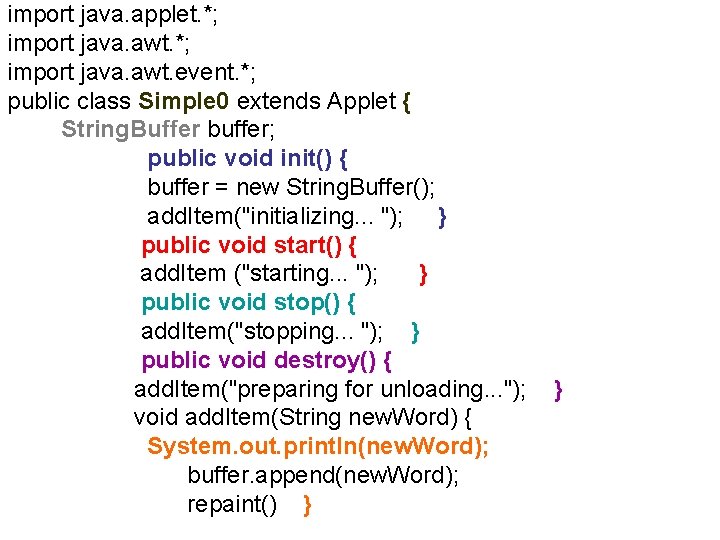
import java. applet. *; import java. awt. event. *; public class Simple 0 extends Applet { String. Buffer buffer; public void init() { buffer = new String. Buffer(); add. Item("initializing. . . "); } public void start() { add. Item ("starting. . . "); } public void stop() { add. Item("stopping. . . "); } public void destroy() { add. Item("preparing for unloading. . . "); void add. Item(String new. Word) { System. out. println(new. Word); buffer. append(new. Word); repaint() } }
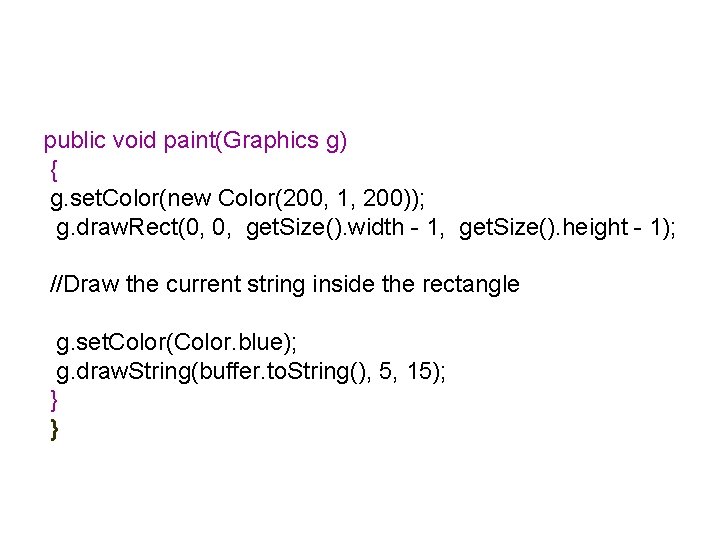
public void paint(Graphics g) { g. set. Color(new Color(200, 1, 200)); g. draw. Rect(0, 0, get. Size(). width - 1, get. Size(). height - 1); //Draw the current string inside the rectangle g. set. Color(Color. blue); g. draw. String(buffer. to. String(), 5, 15); } }
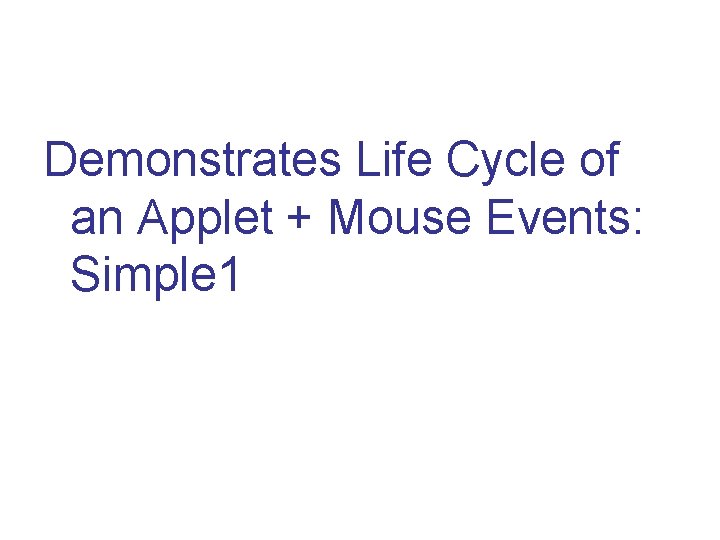
Demonstrates Life Cycle of an Applet + Mouse Events: Simple 1
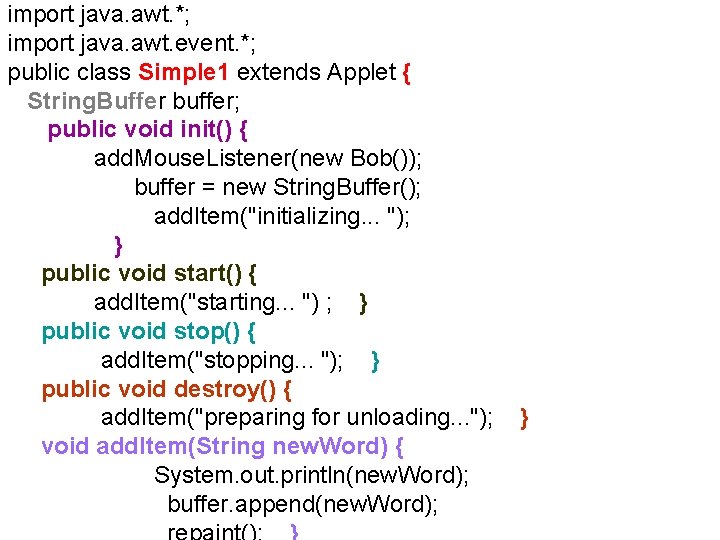
import java. awt. *; import java. awt. event. *; public class Simple 1 extends Applet { String. Buffer buffer; public void init() { add. Mouse. Listener(new Bob()); buffer = new String. Buffer(); add. Item("initializing. . . "); } public void start() { add. Item("starting. . . ") ; } public void stop() { add. Item("stopping. . . "); } public void destroy() { add. Item("preparing for unloading. . . "); void add. Item(String new. Word) { System. out. println(new. Word); buffer. append(new. Word); }
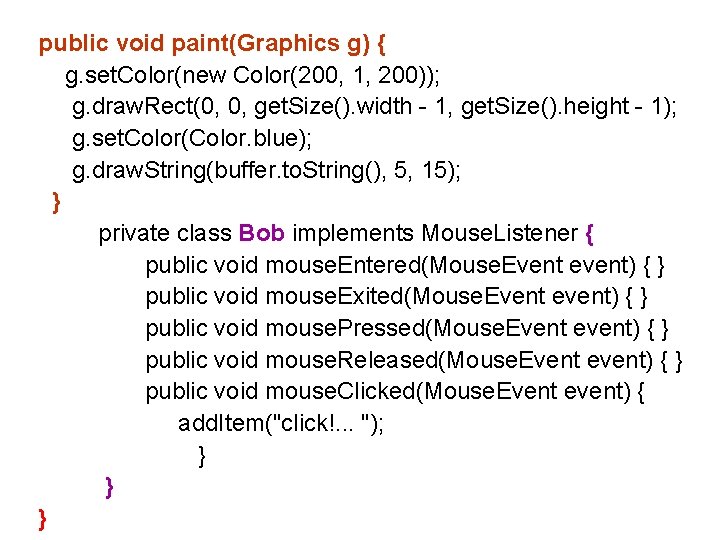
public void paint(Graphics g) { g. set. Color(new Color(200, 1, 200)); g. draw. Rect(0, 0, get. Size(). width - 1, get. Size(). height - 1); g. set. Color(Color. blue); g. draw. String(buffer. to. String(), 5, 15); } private class Bob implements Mouse. Listener { public void mouse. Entered(Mouse. Event event) { } public void mouse. Exited(Mouse. Event event) { } public void mouse. Pressed(Mouse. Event event) { } public void mouse. Released(Mouse. Event event) { } public void mouse. Clicked(Mouse. Event event) { add. Item("click!. . . "); } } }
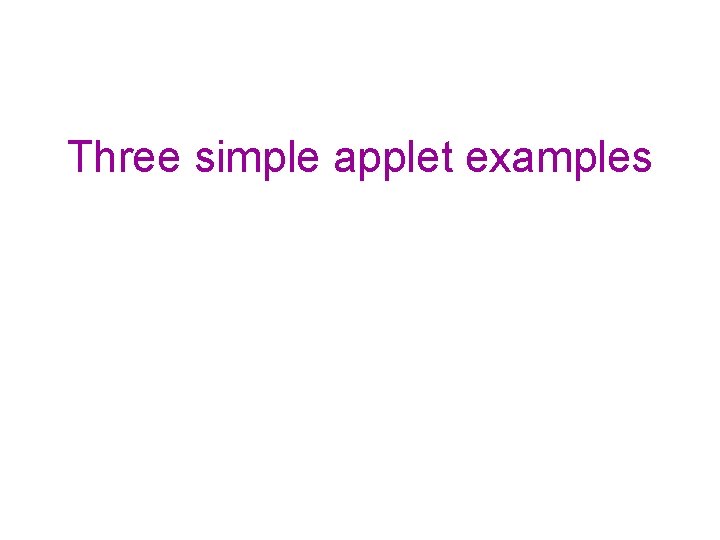
Three simple applet examples
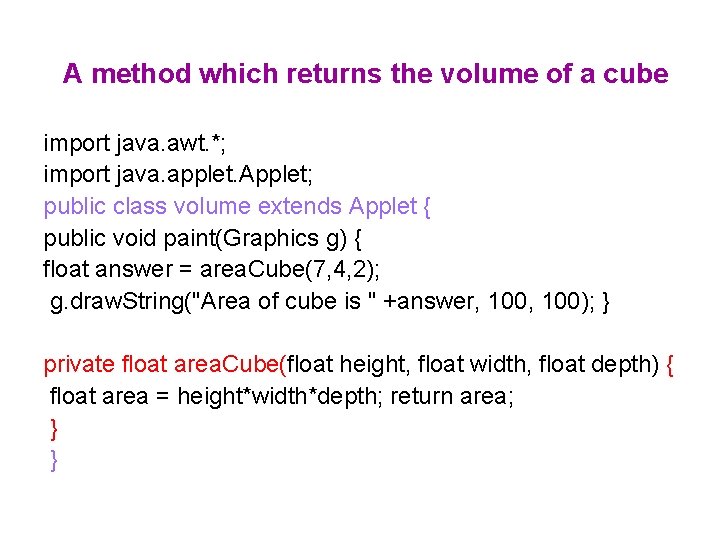
A method which returns the volume of a cube import java. awt. *; import java. applet. Applet; public class volume extends Applet { public void paint(Graphics g) { float answer = area. Cube(7, 4, 2); g. draw. String("Area of cube is " +answer, 100); } private float area. Cube(float height, float width, float depth) { float area = height*width*depth; return area; } }
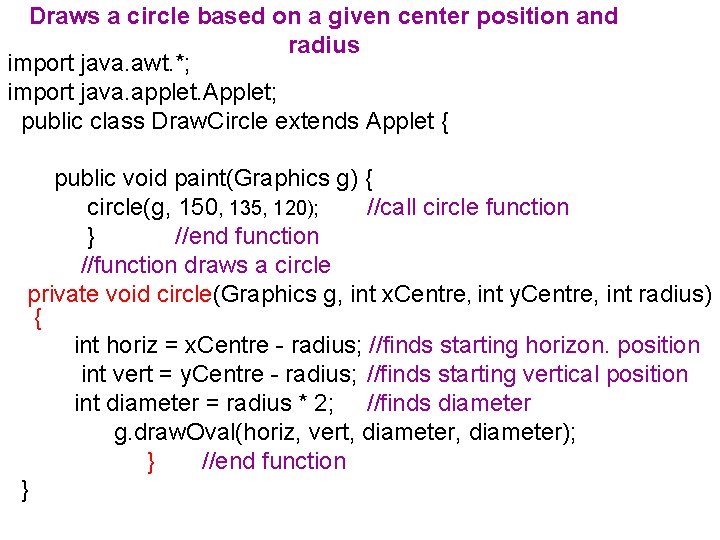
Draws a circle based on a given center position and radius import java. awt. *; import java. applet. Applet; public class Draw. Circle extends Applet { public void paint(Graphics g) { circle(g, 150, 135, 120); //call circle function } //end function //function draws a circle private void circle(Graphics g, int x. Centre, int y. Centre, int radius) { int horiz = x. Centre - radius; //finds starting horizon. position int vert = y. Centre - radius; //finds starting vertical position int diameter = radius * 2; //finds diameter g. draw. Oval(horiz, vert, diameter); } //end function }
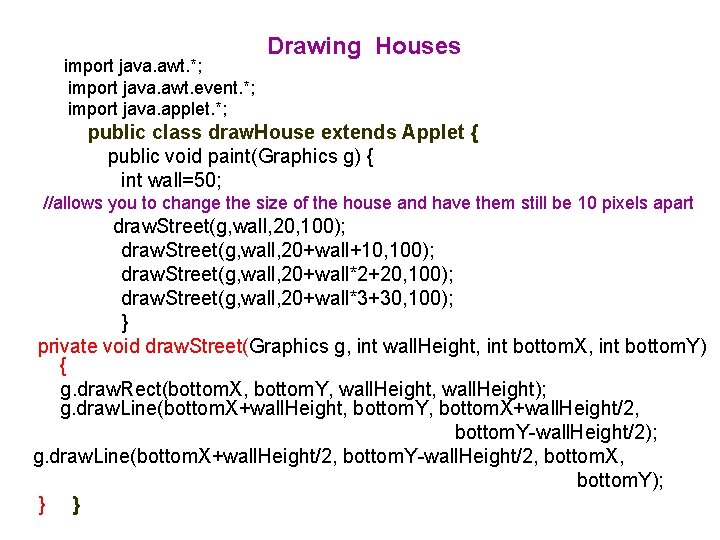
import java. awt. *; import java. awt. event. *; import java. applet. *; Drawing Houses public class draw. House extends Applet { public void paint(Graphics g) { int wall=50; //allows you to change the size of the house and have them still be 10 pixels apart draw. Street(g, wall, 20, 100); draw. Street(g, wall, 20+wall+10, 100); draw. Street(g, wall, 20+wall*2+20, 100); draw. Street(g, wall, 20+wall*3+30, 100); } private void draw. Street(Graphics g, int wall. Height, int bottom. X, int bottom. Y) { g. draw. Rect(bottom. X, bottom. Y, wall. Height); g. draw. Line(bottom. X+wall. Height, bottom. Y, bottom. X+wall. Height/2, bottom. Y-wall. Height/2); g. draw. Line(bottom. X+wall. Height/2, bottom. Y-wall. Height/2, bottom. X, bottom. Y); } }
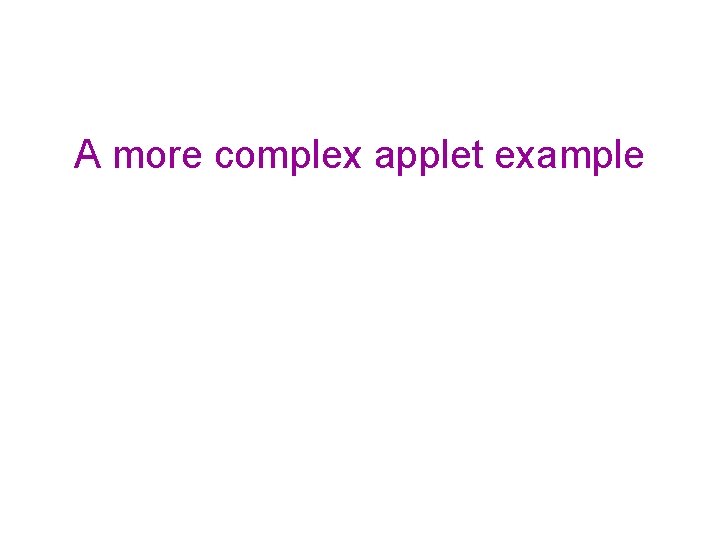
A more complex applet example
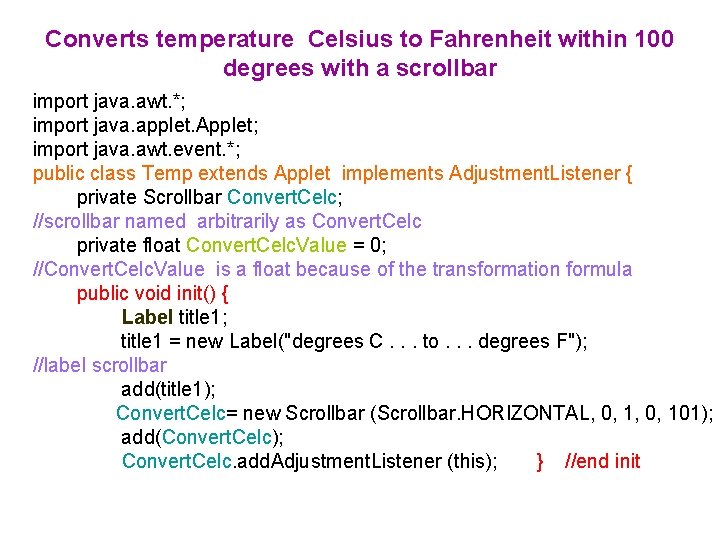
Converts temperature Celsius to Fahrenheit within 100 degrees with a scrollbar import java. awt. *; import java. applet. Applet; import java. awt. event. *; public class Temp extends Applet implements Adjustment. Listener { private Scrollbar Convert. Celc; //scrollbar named arbitrarily as Convert. Celc private float Convert. Celc. Value = 0; //Convert. Celc. Value is a float because of the transformation formula public void init() { Label title 1; title 1 = new Label("degrees C. . . to. . . degrees F"); //label scrollbar add(title 1); Convert. Celc= new Scrollbar (Scrollbar. HORIZONTAL, 0, 101); add(Convert. Celc); Convert. Celc. add. Adjustment. Listener (this); } //end init
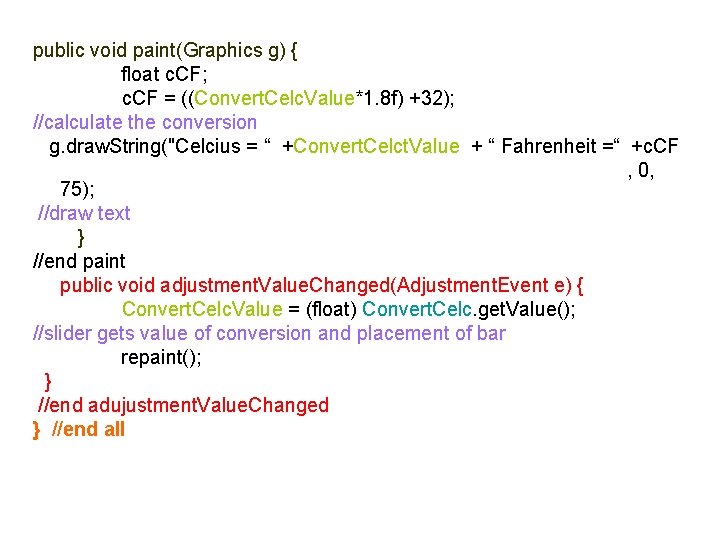
public void paint(Graphics g) { float c. CF; c. CF = ((Convert. Celc. Value*1. 8 f) +32); //calculate the conversion g. draw. String("Celcius = “ +Convert. Celct. Value + “ Fahrenheit =“ +c. CF , 0, 75); //draw text } //end paint public void adjustment. Value. Changed(Adjustment. Event e) { Convert. Celc. Value = (float) Convert. Celc. get. Value(); //slider gets value of conversion and placement of bar repaint(); } //end adujustment. Value. Changed } //end all
Swing vs awt
Applet class
Tanslate
G.draw
Import java.applet.applet
Bagaimana cara menjalankan applet melalui applet viewer?
Difference between local and remote applet
Java applet
Explain the applet life cycle
Steps involved in developing and running a local applet
Natural response and forced response
Natural response and forced response example
Primary immune response and secondary immune response
Difference between applet and awt
Zdistribution