Lecture 2 Android Concepts Topics Framework Components Intent
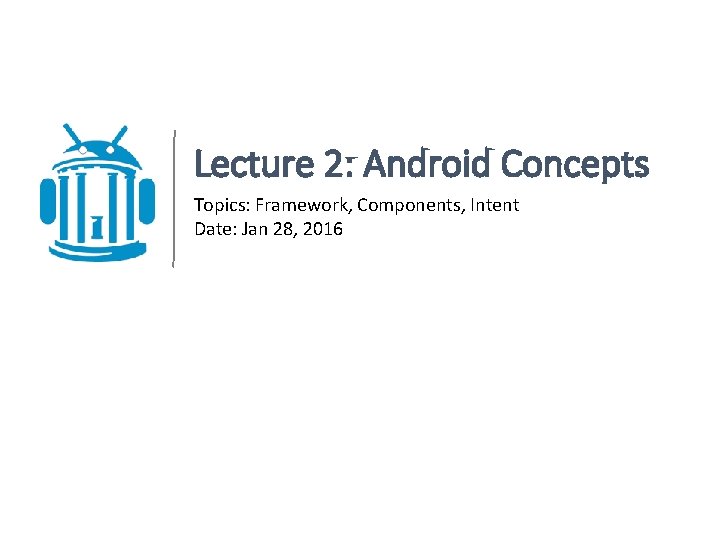
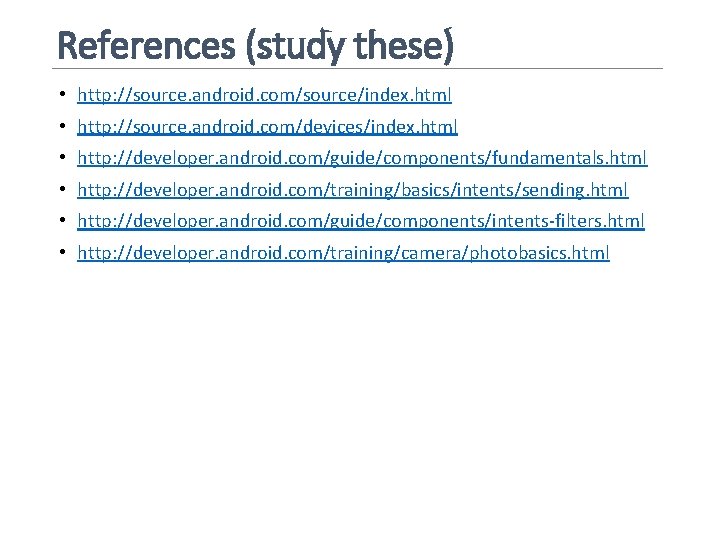
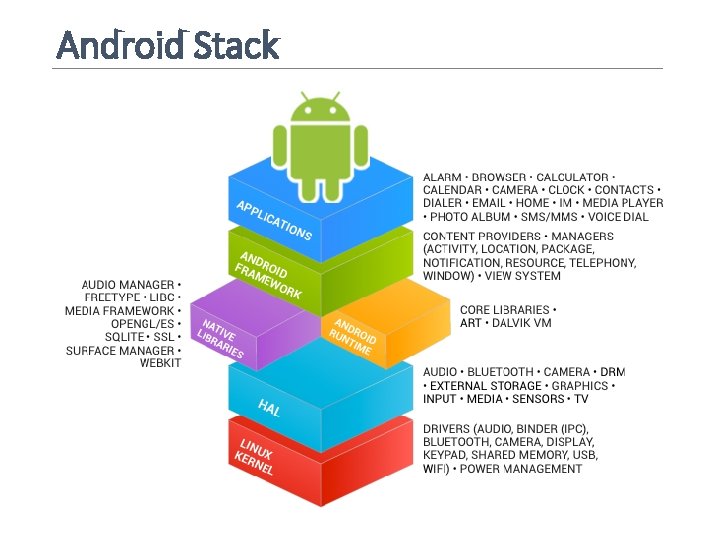
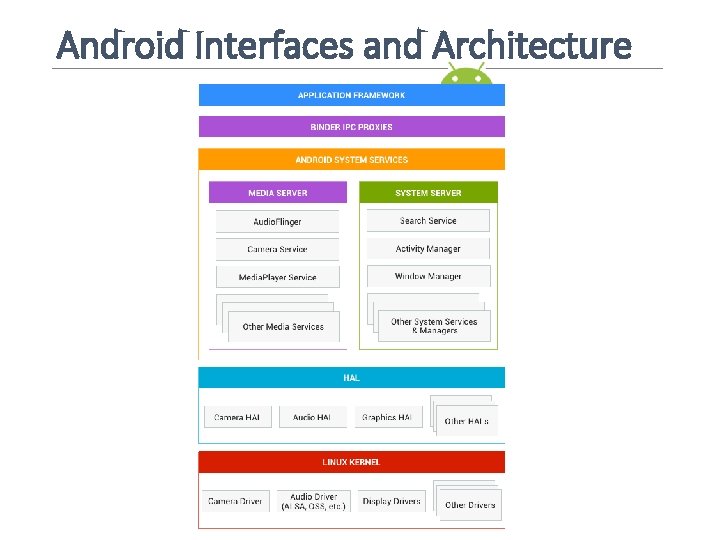
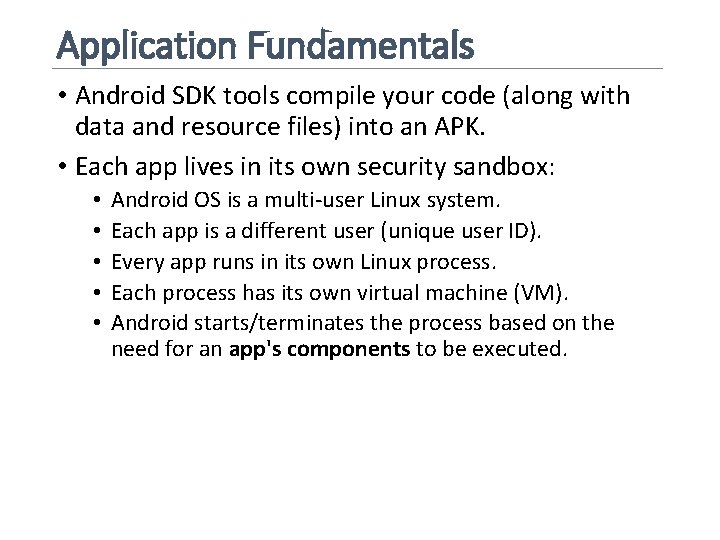
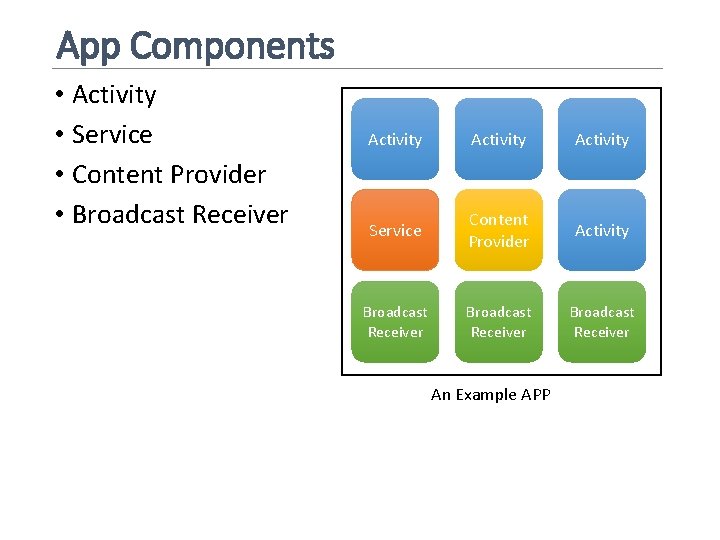
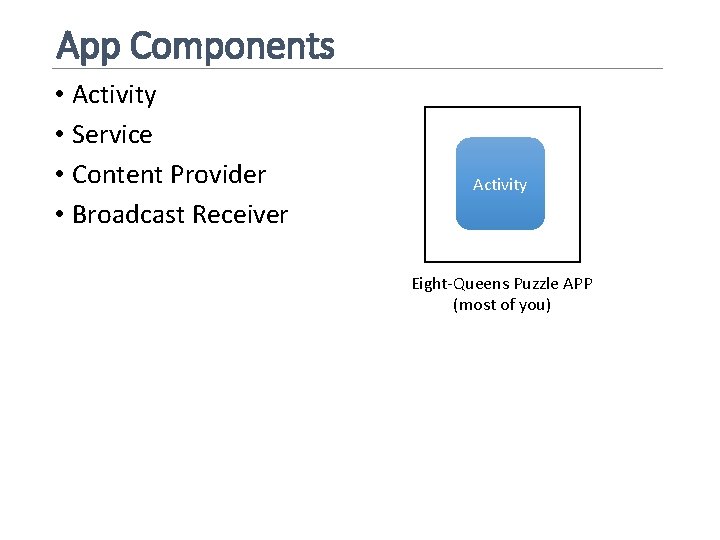
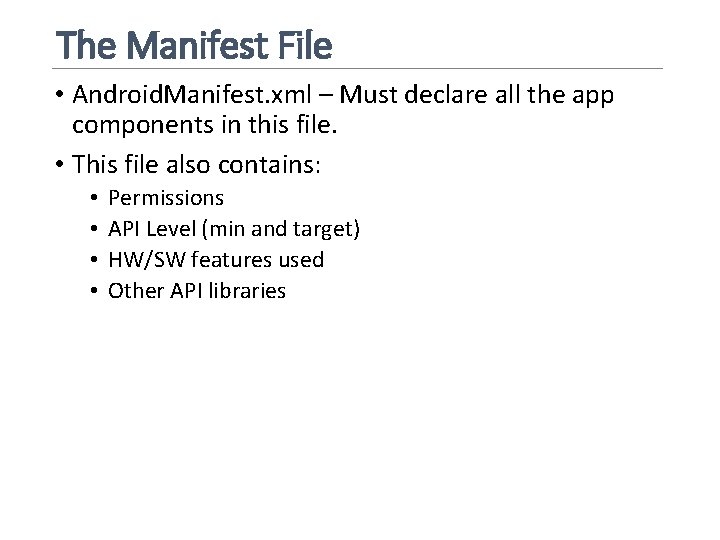
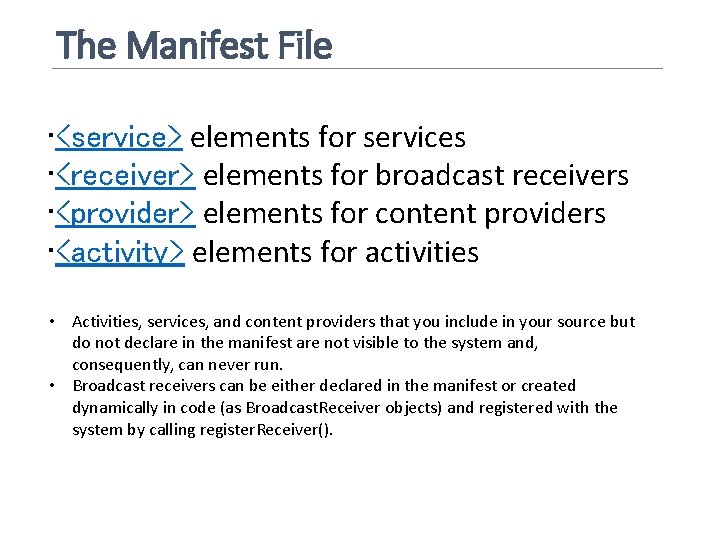
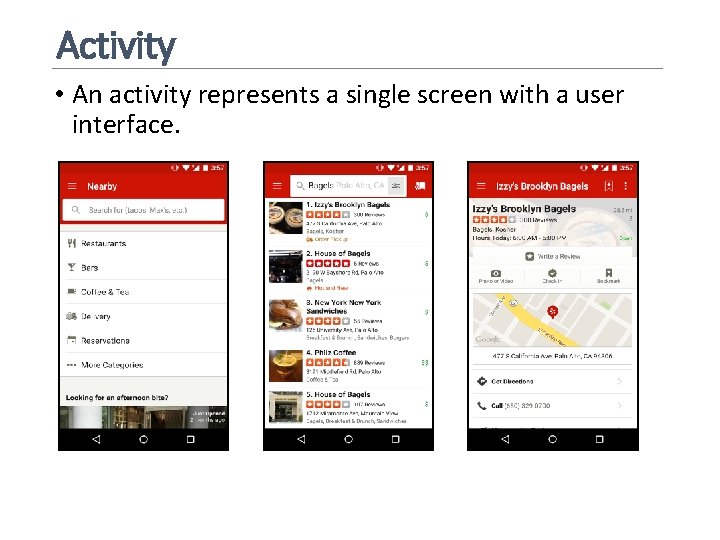
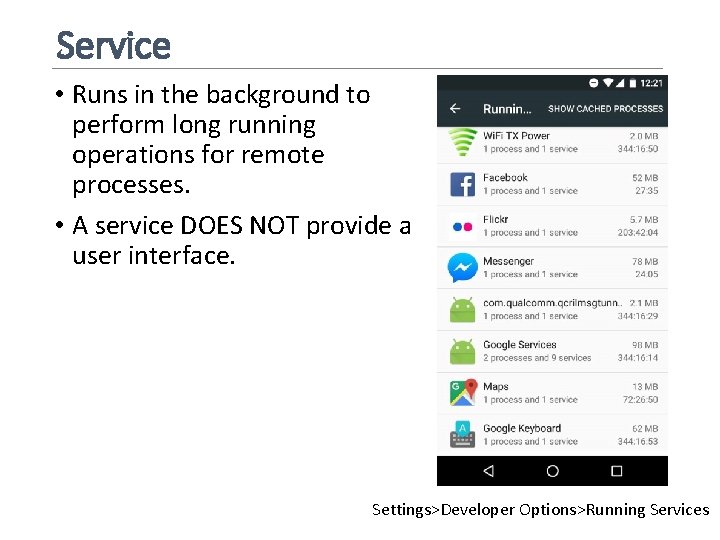
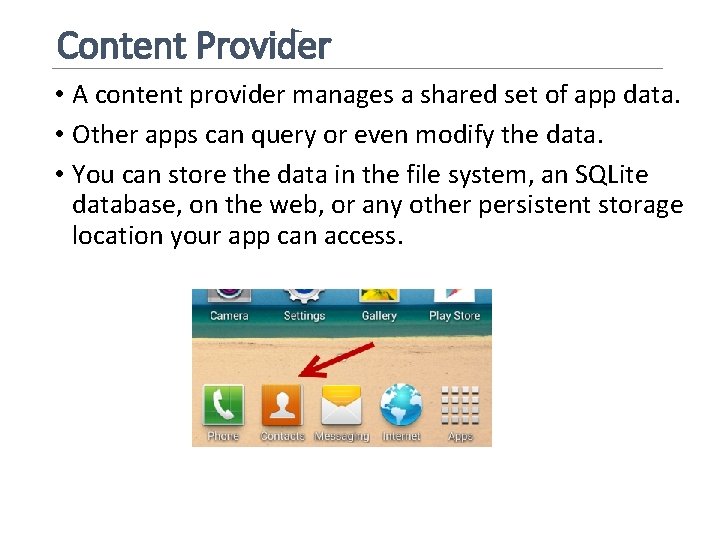
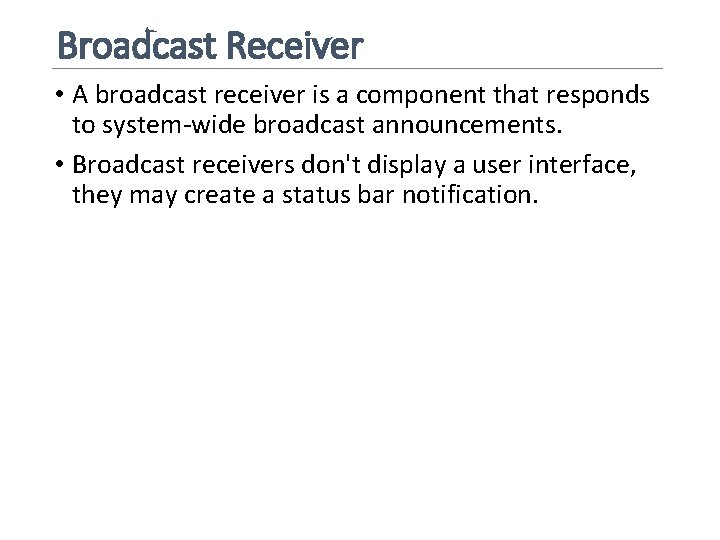
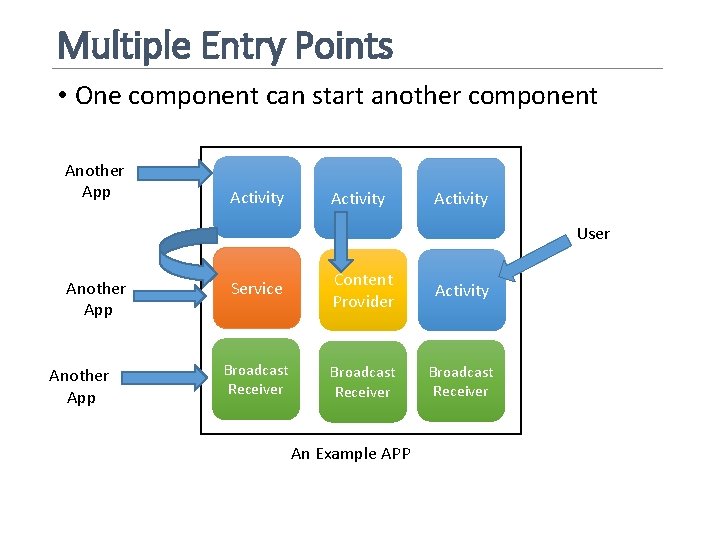
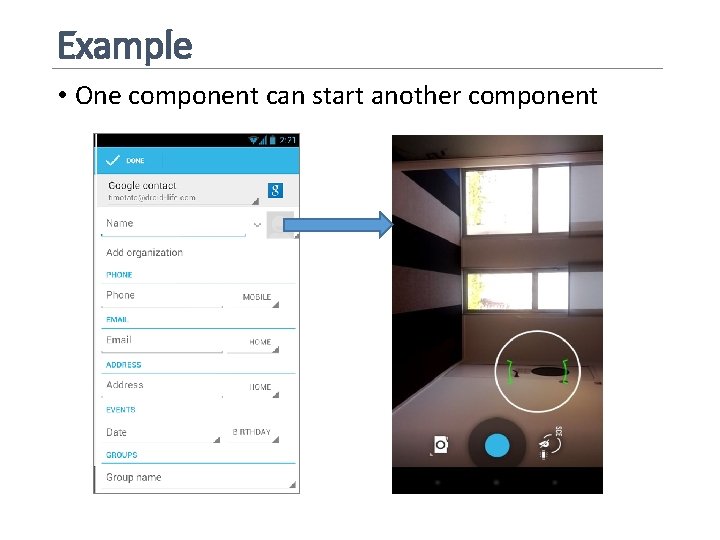
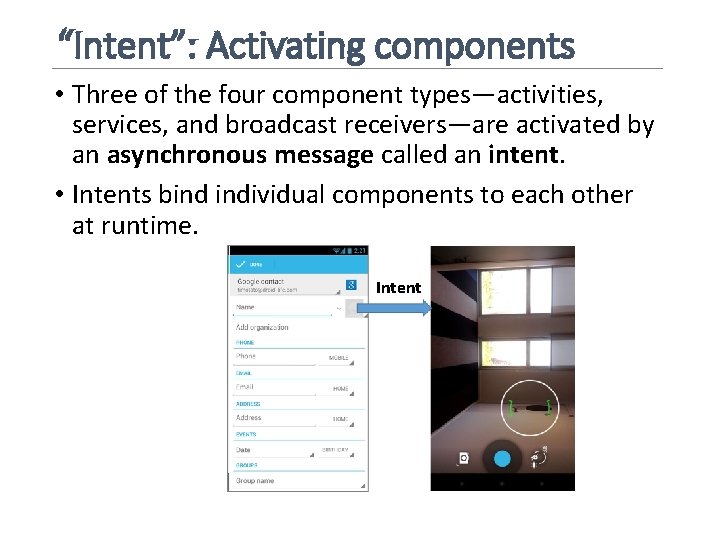
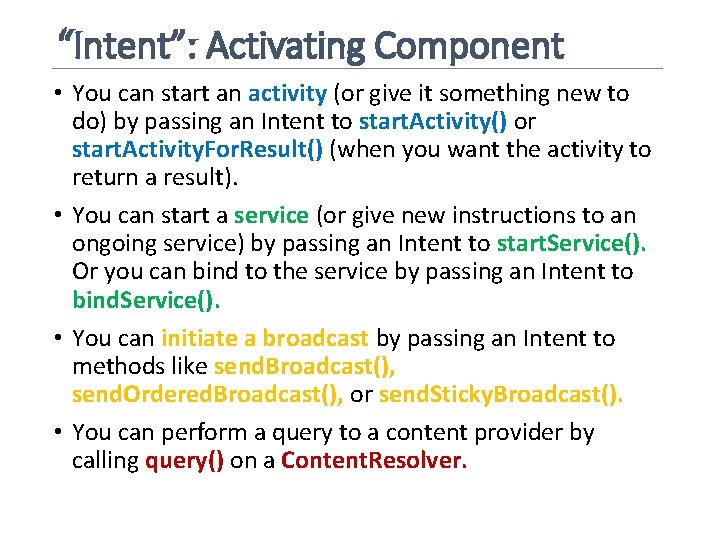
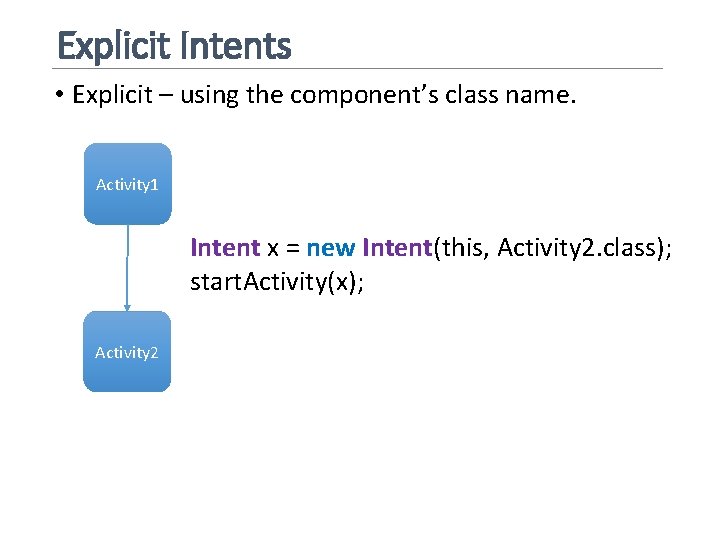
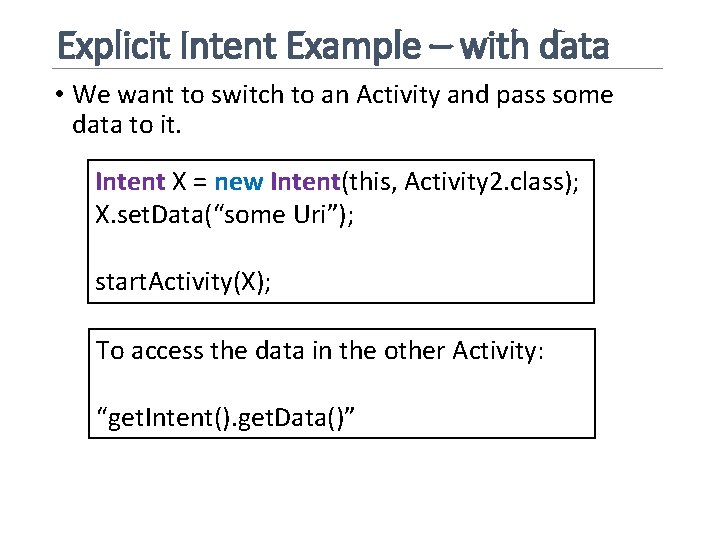
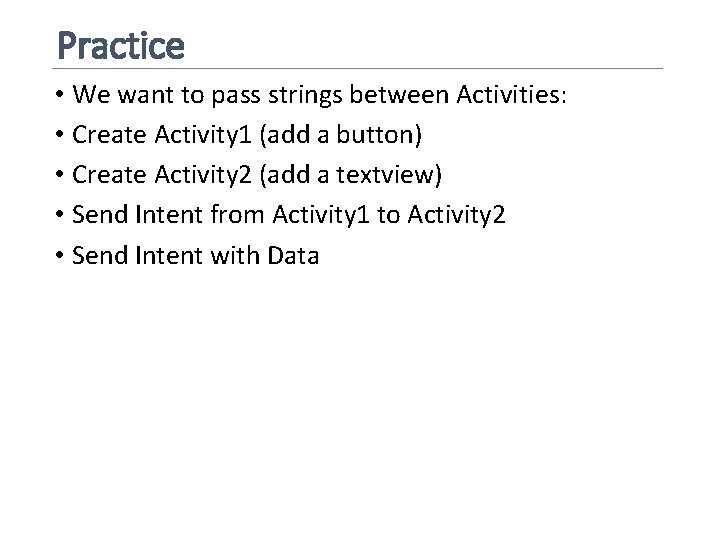
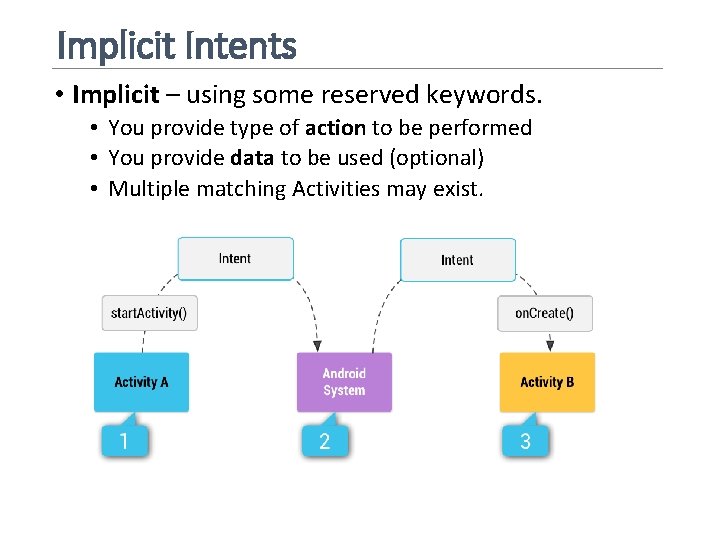
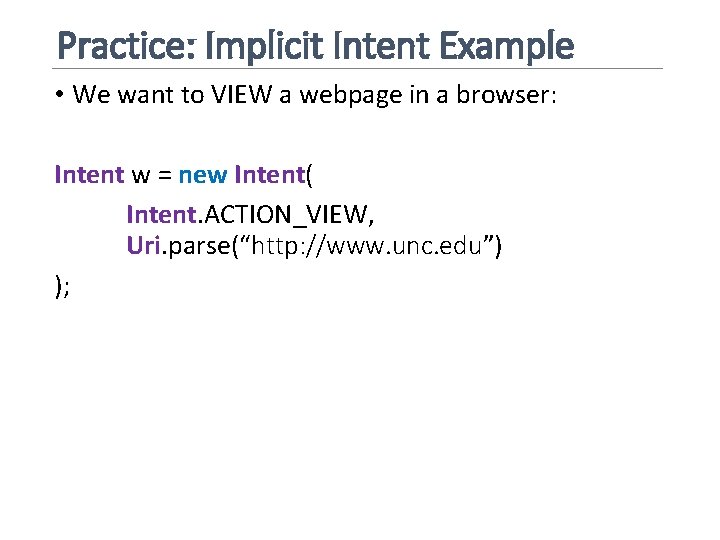
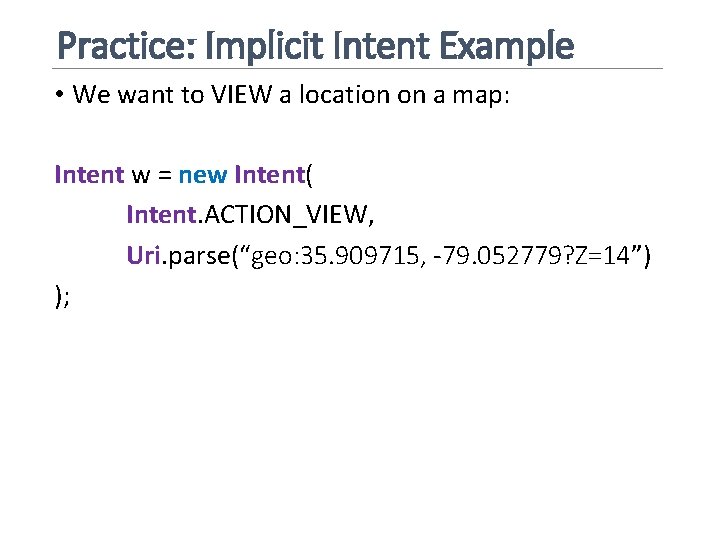
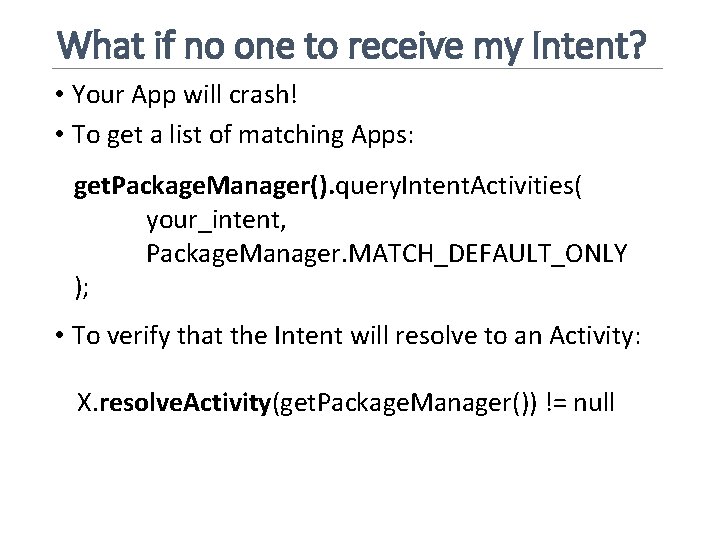
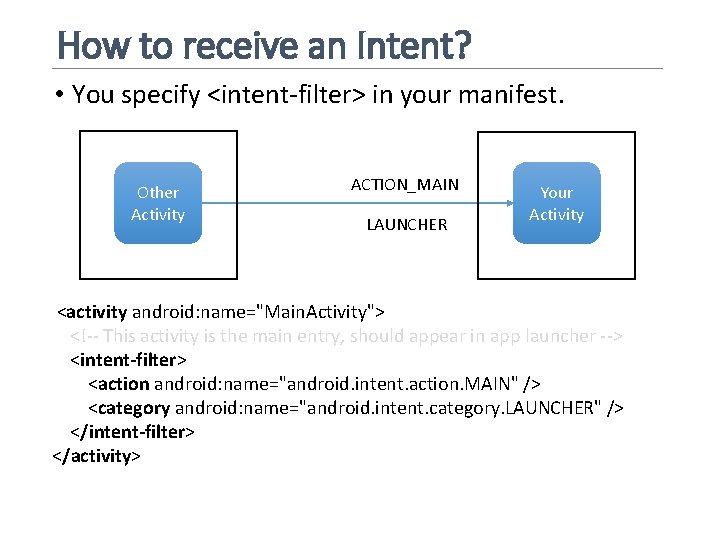
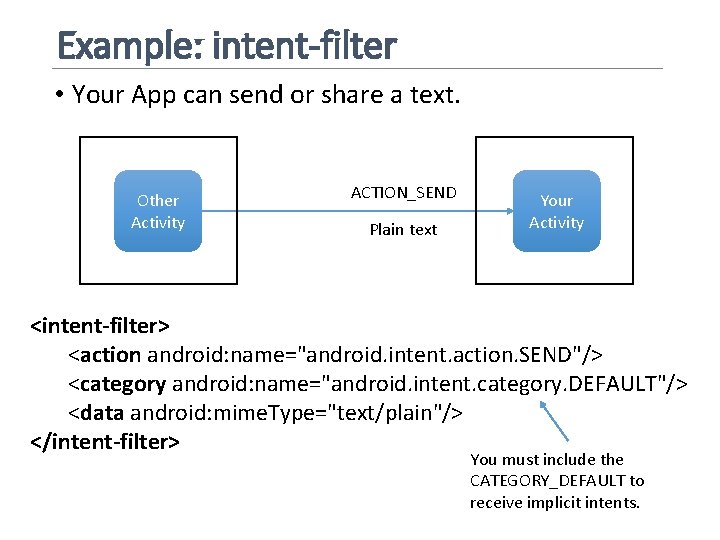
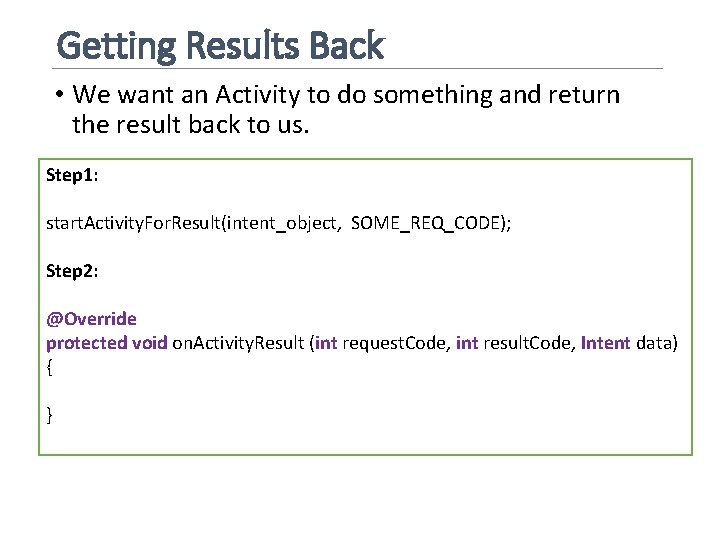
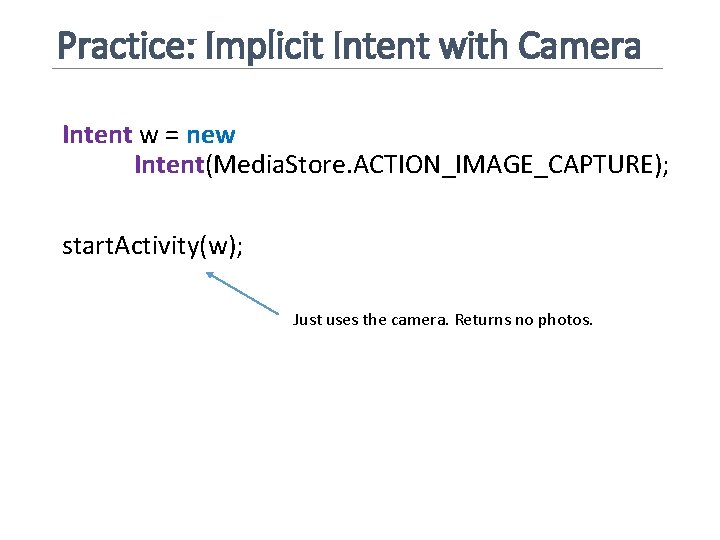
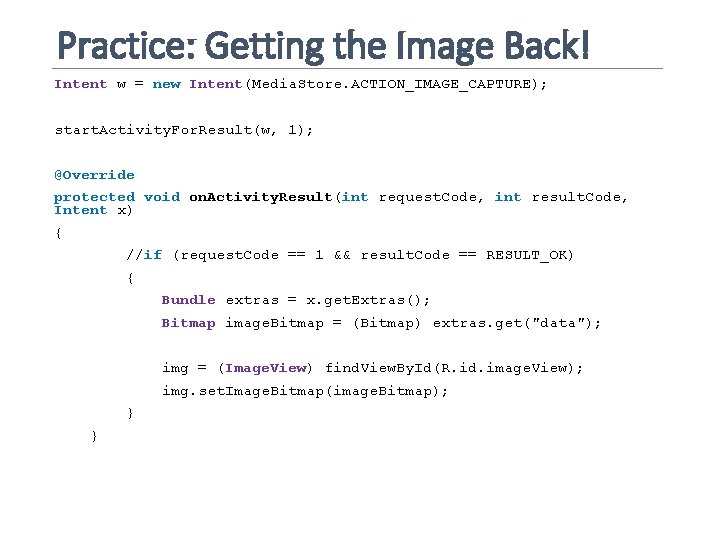
- Slides: 29
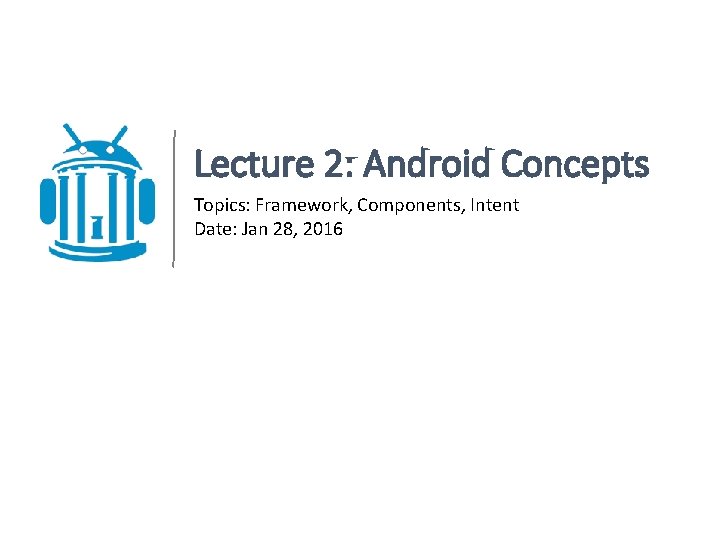
Lecture 2: Android Concepts Topics: Framework, Components, Intent Date: Jan 28, 2016
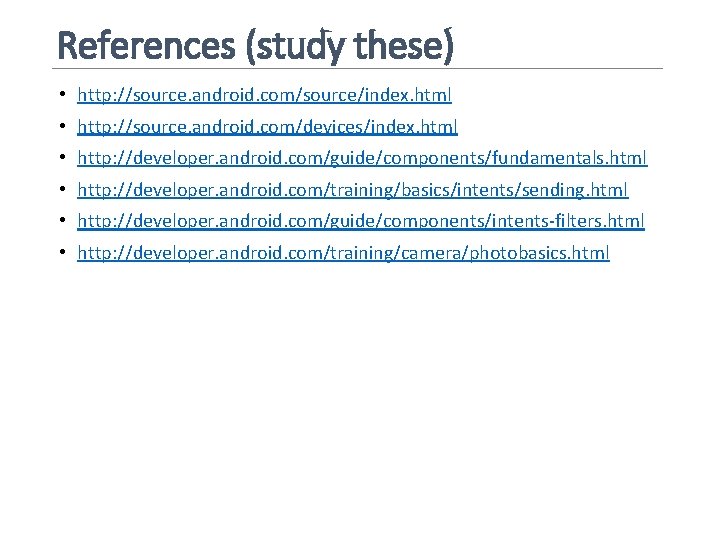
References (study these) • http: //source. android. com/source/index. html • http: //source. android. com/devices/index. html • http: //developer. android. com/guide/components/fundamentals. html • http: //developer. android. com/training/basics/intents/sending. html • http: //developer. android. com/guide/components/intents-filters. html • http: //developer. android. com/training/camera/photobasics. html
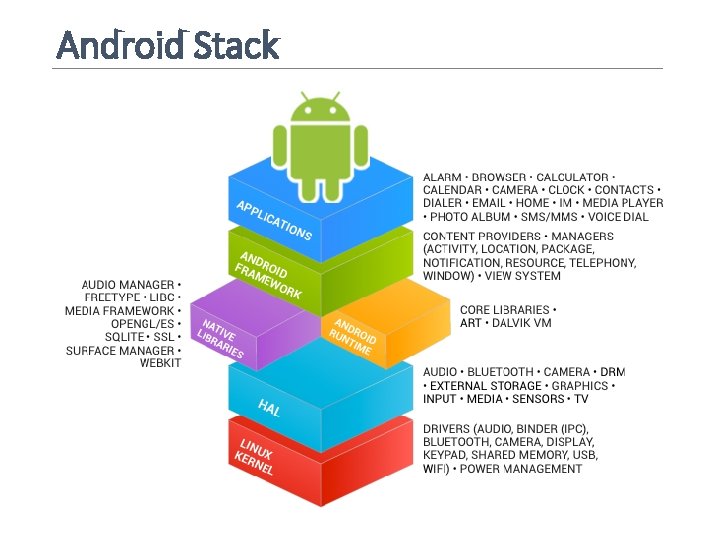
Android Stack
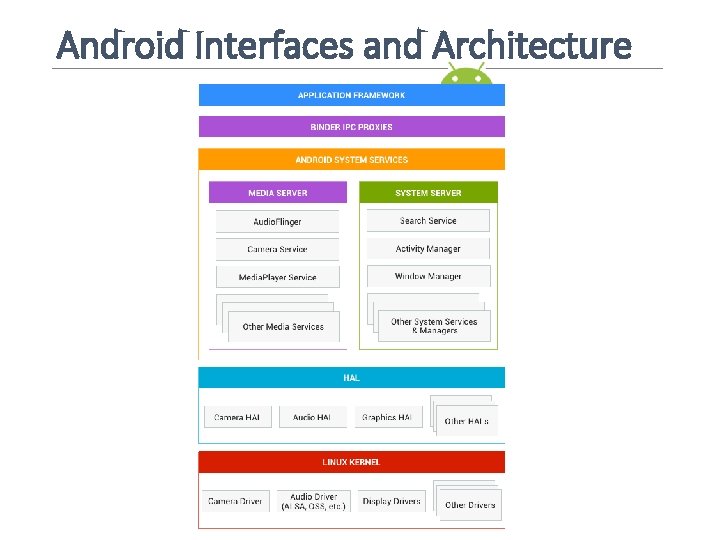
Android Interfaces and Architecture
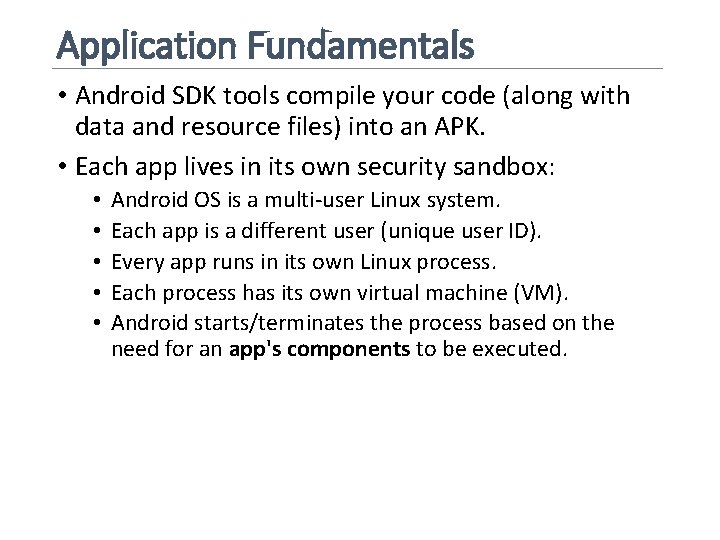
Application Fundamentals • Android SDK tools compile your code (along with data and resource files) into an APK. • Each app lives in its own security sandbox: • • • Android OS is a multi-user Linux system. Each app is a different user (unique user ID). Every app runs in its own Linux process. Each process has its own virtual machine (VM). Android starts/terminates the process based on the need for an app's components to be executed.
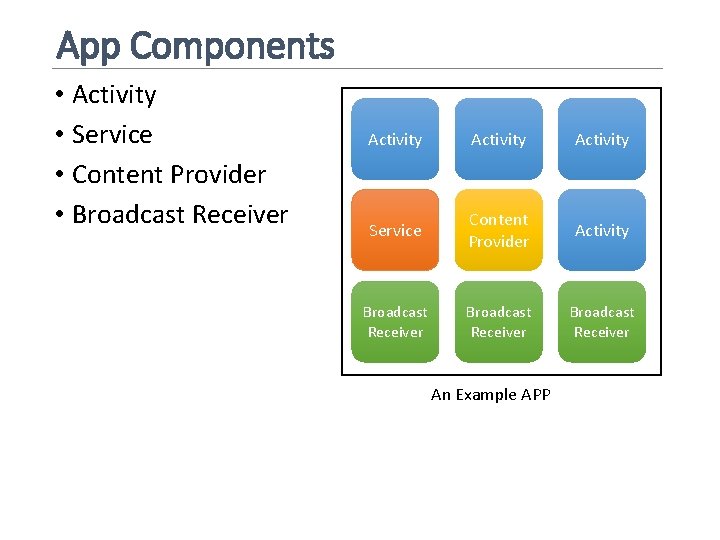
App Components • Activity • Service • Content Provider • Broadcast Receiver Activity Service Content Provider Activity Broadcast Receiver An Example APP
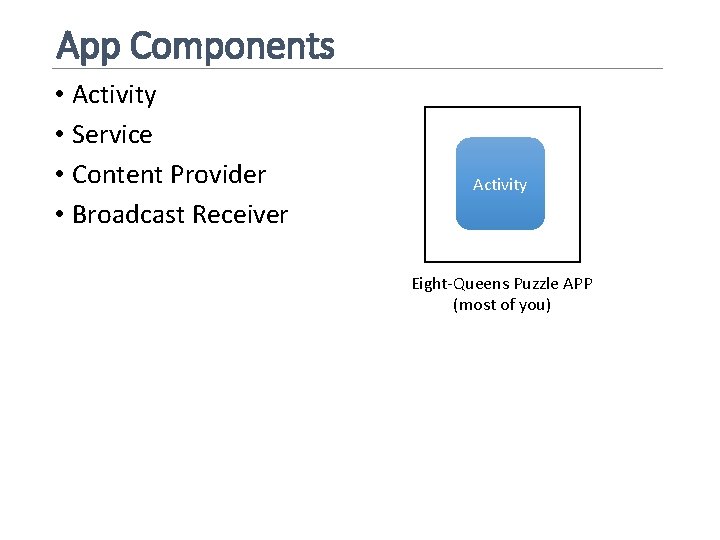
App Components • Activity • Service • Content Provider • Broadcast Receiver Activity Eight-Queens Puzzle APP (most of you)
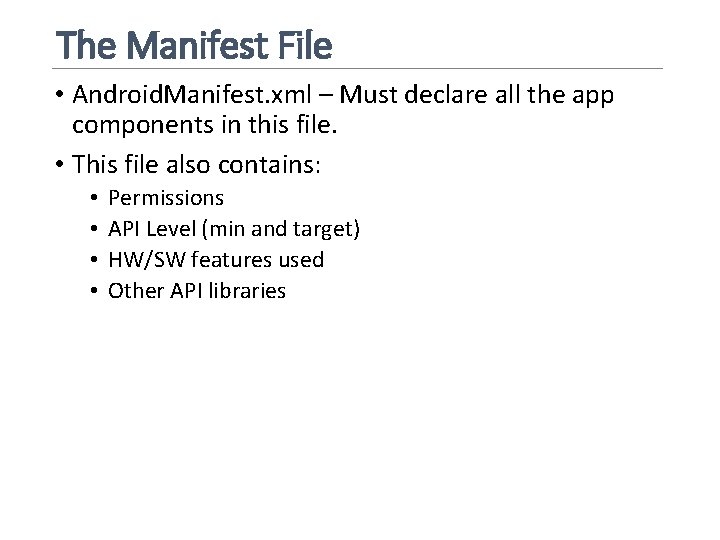
The Manifest File • Android. Manifest. xml – Must declare all the app components in this file. • This file also contains: • • Permissions API Level (min and target) HW/SW features used Other API libraries
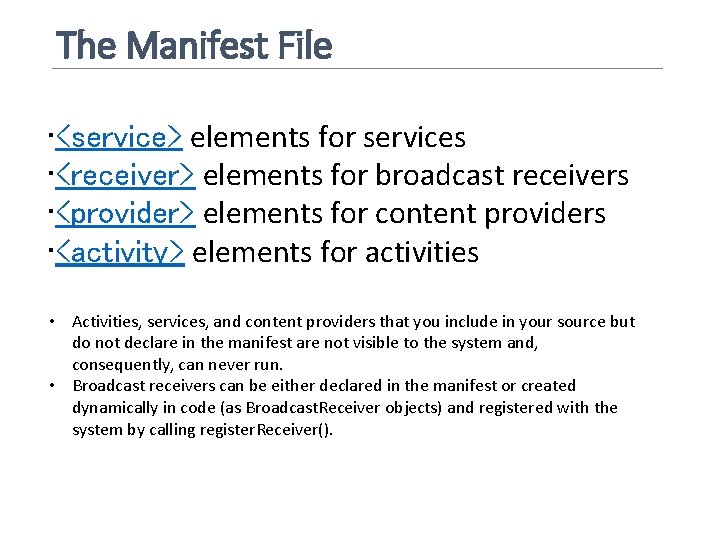
The Manifest File • <service> elements for services • <receiver> elements for broadcast receivers • <provider> elements for content providers • <activity> elements for activities • Activities, services, and content providers that you include in your source but do not declare in the manifest are not visible to the system and, consequently, can never run. • Broadcast receivers can be either declared in the manifest or created dynamically in code (as Broadcast. Receiver objects) and registered with the system by calling register. Receiver().
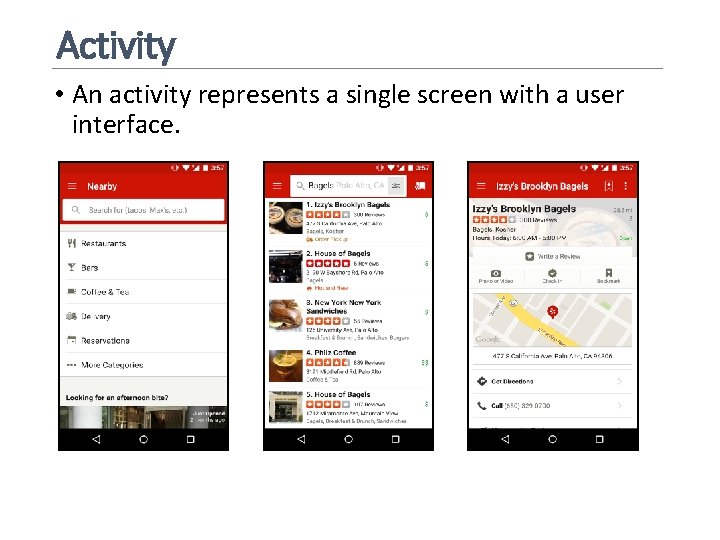
Activity • An activity represents a single screen with a user interface.
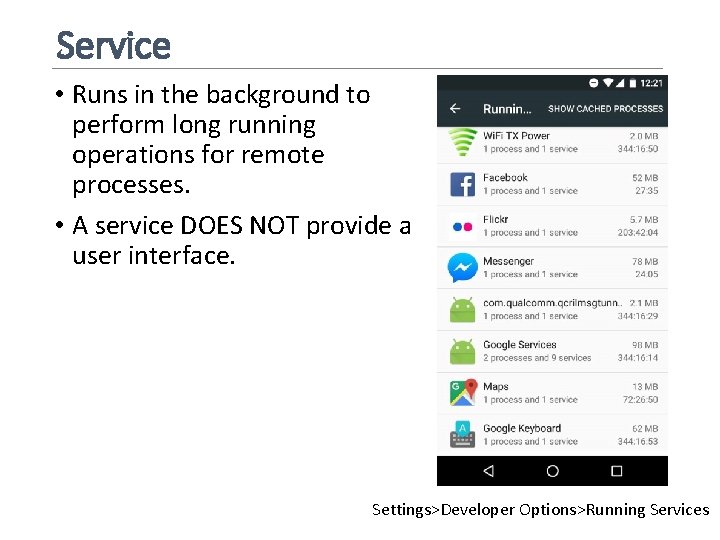
Service • Runs in the background to perform long running operations for remote processes. • A service DOES NOT provide a user interface. Settings>Developer Options>Running Services
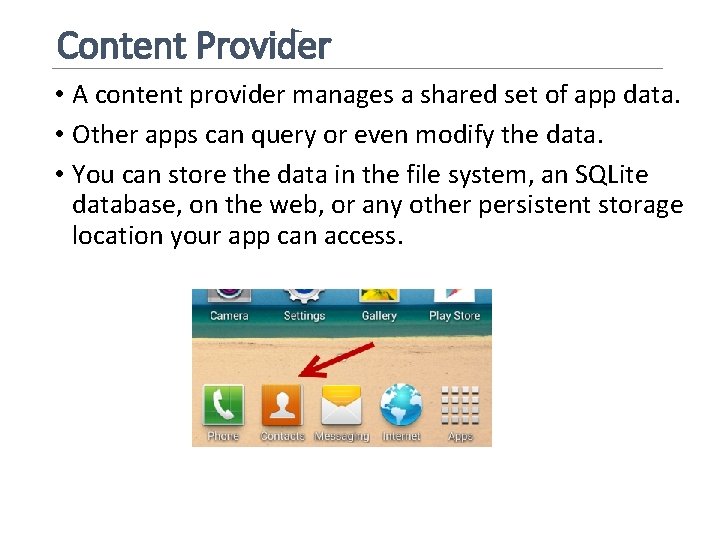
Content Provider • A content provider manages a shared set of app data. • Other apps can query or even modify the data. • You can store the data in the file system, an SQLite database, on the web, or any other persistent storage location your app can access.
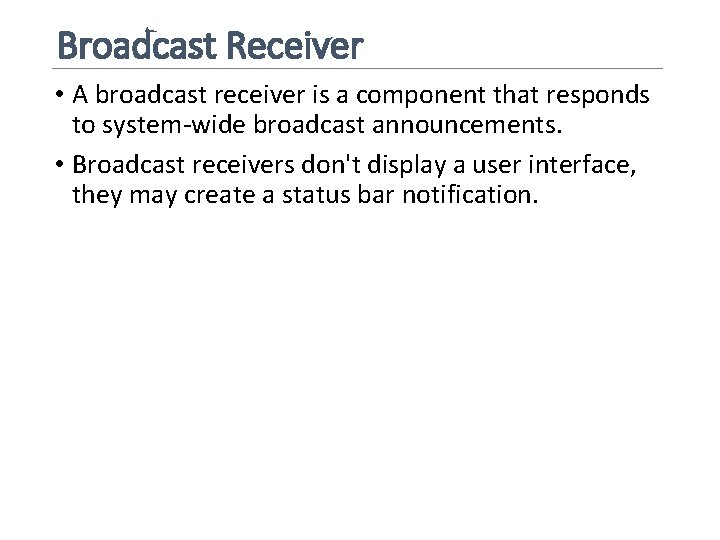
Broadcast Receiver • A broadcast receiver is a component that responds to system-wide broadcast announcements. • Broadcast receivers don't display a user interface, they may create a status bar notification.
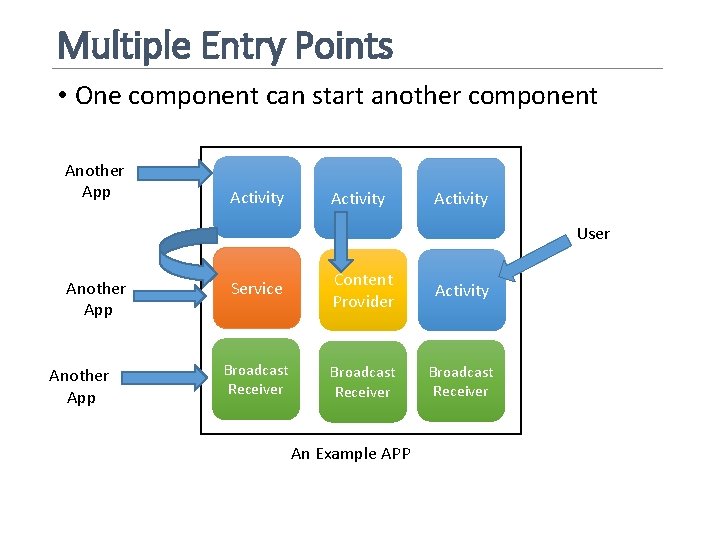
Multiple Entry Points • One component can start another component Another App Activity User Another App Service Content Provider Activity Broadcast Receiver An Example APP
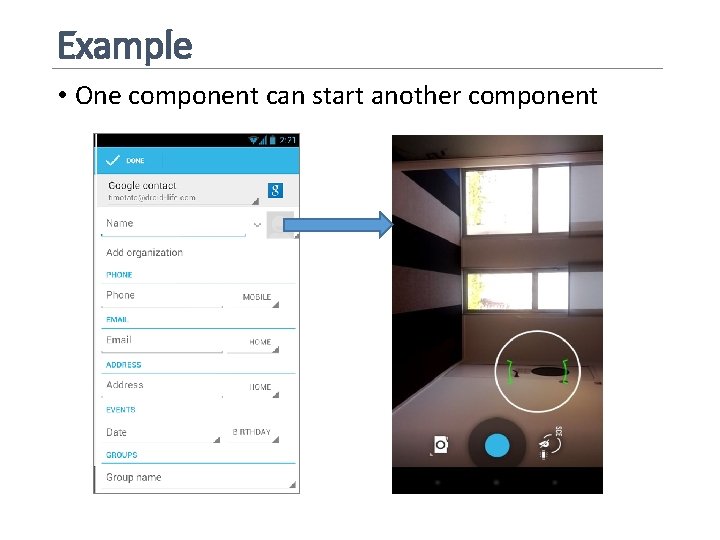
Example • One component can start another component
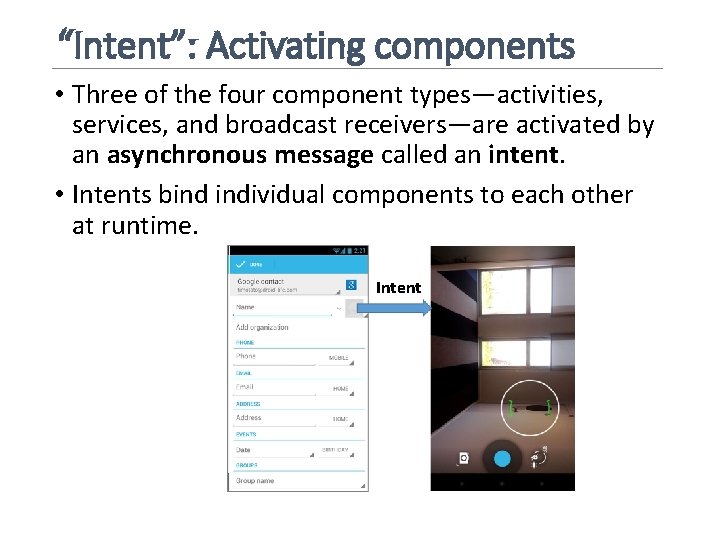
“Intent”: Activating components • Three of the four component types—activities, services, and broadcast receivers—are activated by an asynchronous message called an intent. • Intents bind individual components to each other at runtime. Intent
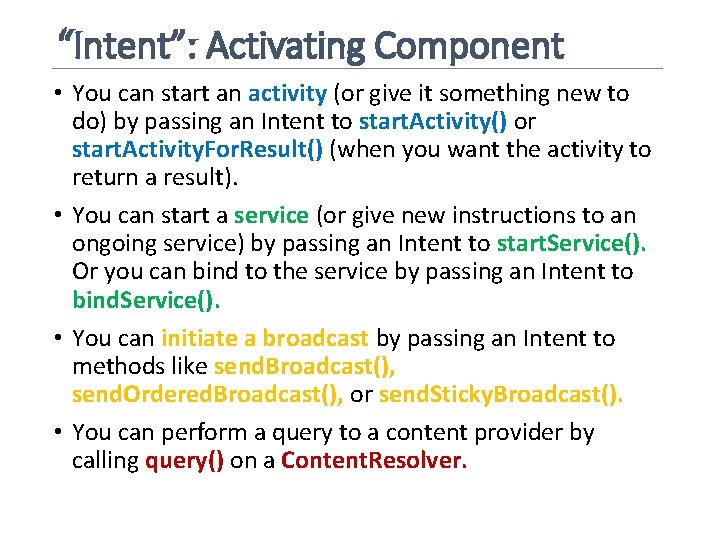
“Intent”: Activating Component • You can start an activity (or give it something new to do) by passing an Intent to start. Activity() or start. Activity. For. Result() (when you want the activity to return a result). • You can start a service (or give new instructions to an ongoing service) by passing an Intent to start. Service(). Or you can bind to the service by passing an Intent to bind. Service(). • You can initiate a broadcast by passing an Intent to methods like send. Broadcast(), send. Ordered. Broadcast(), or send. Sticky. Broadcast(). • You can perform a query to a content provider by calling query() on a Content. Resolver.
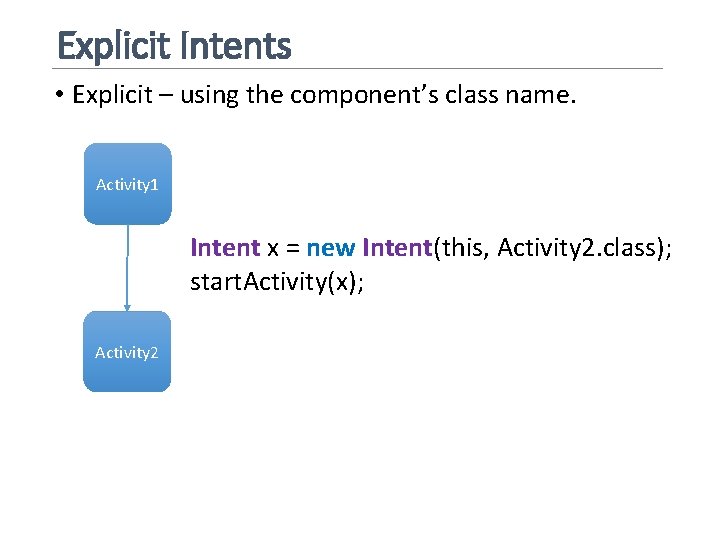
Explicit Intents • Explicit – using the component’s class name. Activity 1 Intent x = new Intent(this, Activity 2. class); start. Activity(x); Activity 2
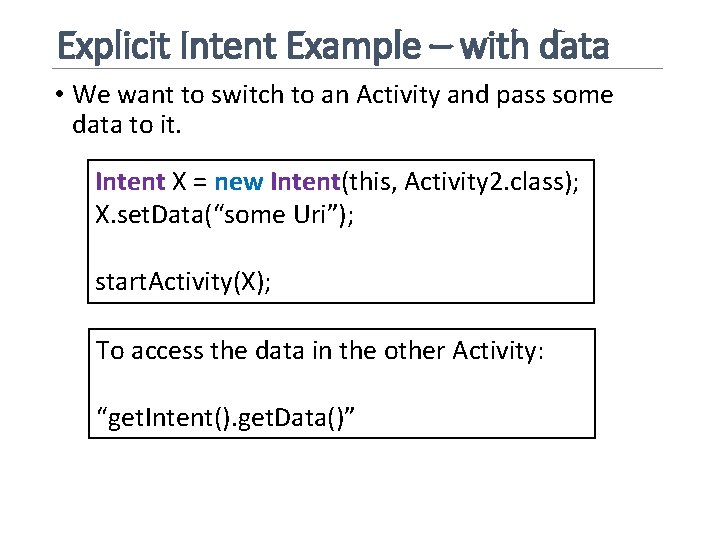
Explicit Intent Example – with data • We want to switch to an Activity and pass some data to it. Intent X = new Intent(this, Activity 2. class); X. set. Data(“some Uri”); start. Activity(X); To access the data in the other Activity: “get. Intent(). get. Data()”
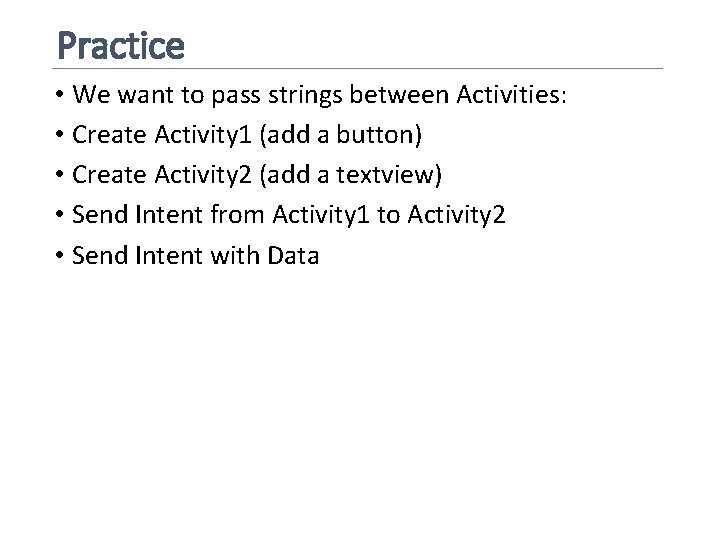
Practice • We want to pass strings between Activities: • Create Activity 1 (add a button) • Create Activity 2 (add a textview) • Send Intent from Activity 1 to Activity 2 • Send Intent with Data
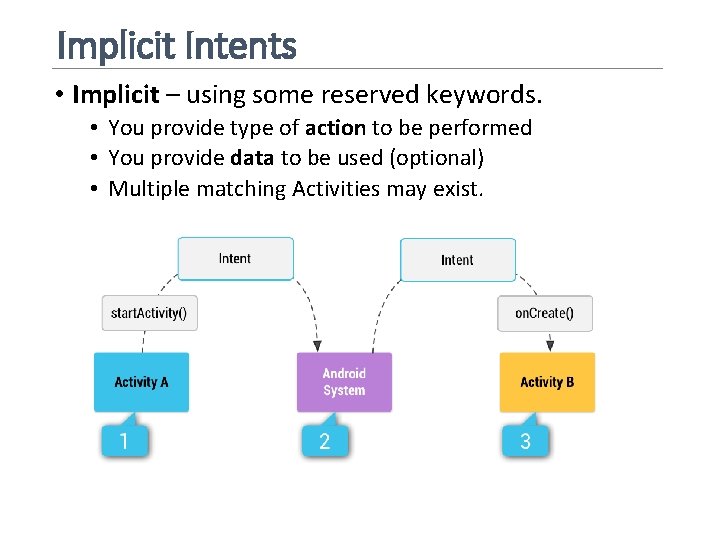
Implicit Intents • Implicit – using some reserved keywords. • You provide type of action to be performed • You provide data to be used (optional) • Multiple matching Activities may exist.
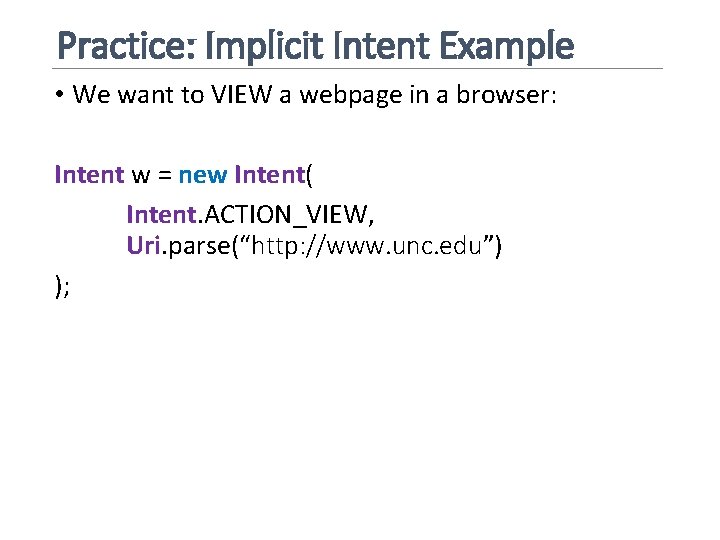
Practice: Implicit Intent Example • We want to VIEW a webpage in a browser: Intent w = new Intent( Intent. ACTION_VIEW, Uri. parse(“http: //www. unc. edu”) );
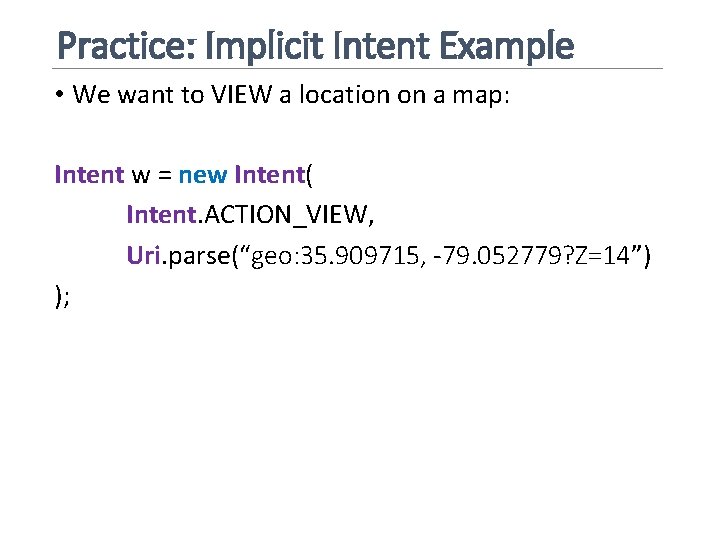
Practice: Implicit Intent Example • We want to VIEW a location on a map: Intent w = new Intent( Intent. ACTION_VIEW, Uri. parse(“geo: 35. 909715, -79. 052779? Z=14”) );
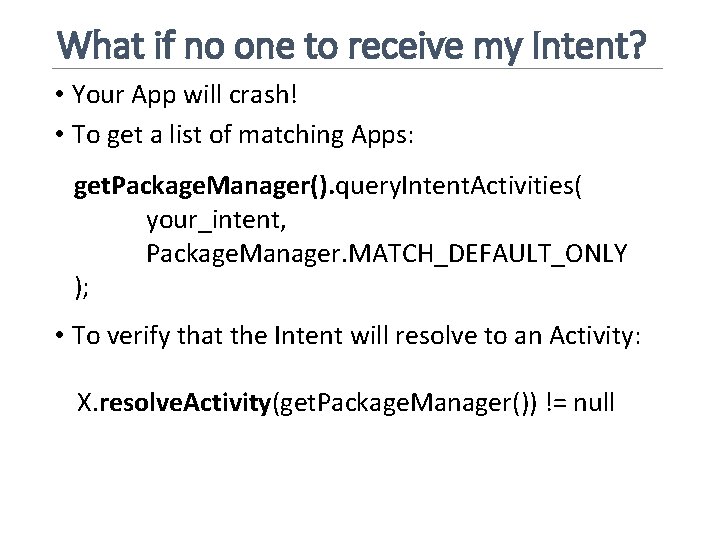
What if no one to receive my Intent? • Your App will crash! • To get a list of matching Apps: get. Package. Manager(). query. Intent. Activities( your_intent, Package. Manager. MATCH_DEFAULT_ONLY ); • To verify that the Intent will resolve to an Activity: X. resolve. Activity(get. Package. Manager()) != null
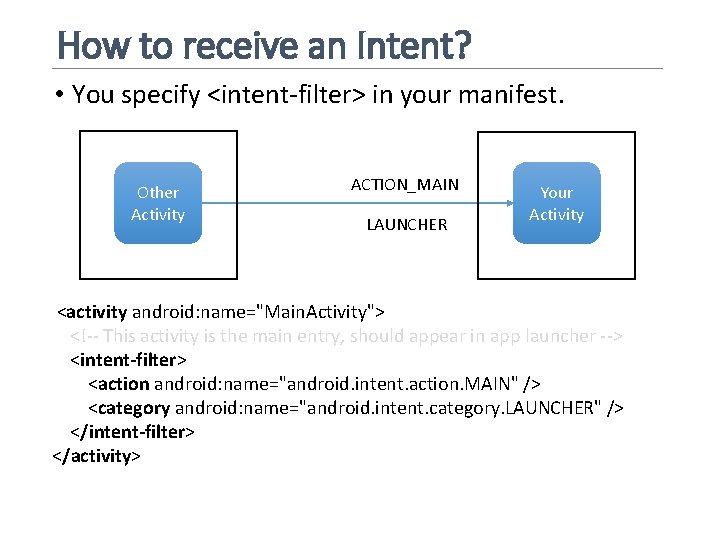
How to receive an Intent? • You specify <intent-filter> in your manifest. Other Activity ACTION_MAIN LAUNCHER Your Activity <activity android: name="Main. Activity"> <!-- This activity is the main entry, should appear in app launcher --> <intent-filter> <action android: name="android. intent. action. MAIN" /> <category android: name="android. intent. category. LAUNCHER" /> </intent-filter> </activity>
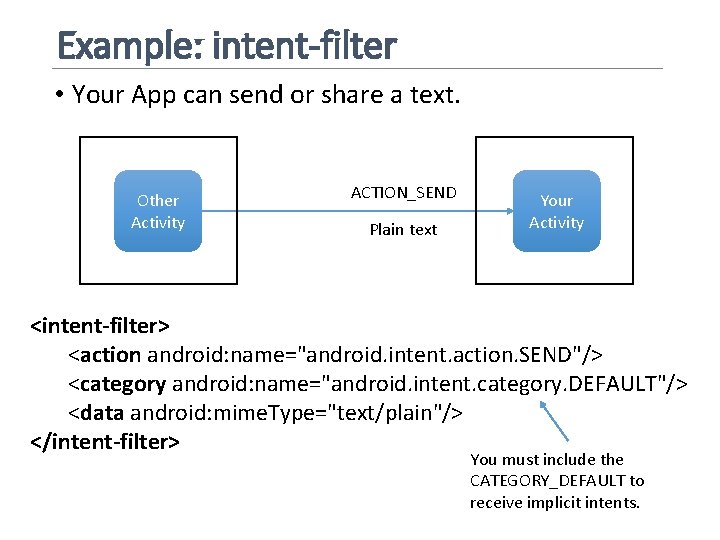
Example: intent-filter • Your App can send or share a text. Other Activity ACTION_SEND Plain text Your Activity <intent-filter> <action android: name="android. intent. action. SEND"/> <category android: name="android. intent. category. DEFAULT"/> <data android: mime. Type="text/plain"/> </intent-filter> You must include the CATEGORY_DEFAULT to receive implicit intents.
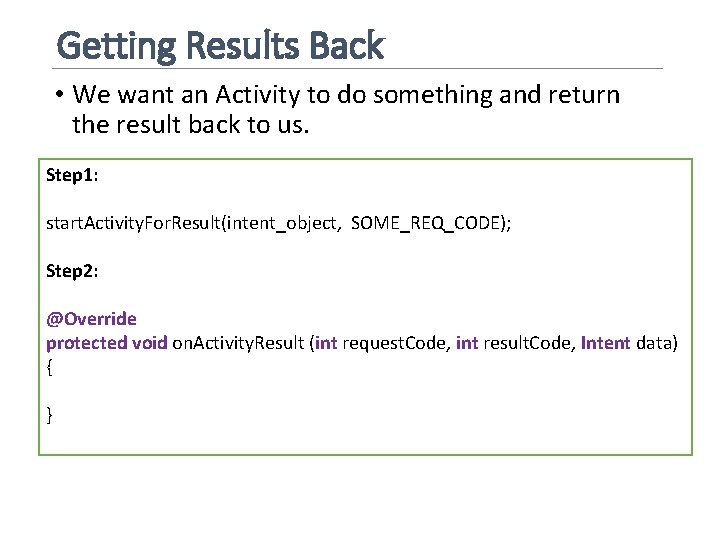
Getting Results Back • We want an Activity to do something and return the result back to us. Step 1: start. Activity. For. Result(intent_object, SOME_REQ_CODE); Step 2: @Override protected void on. Activity. Result (int request. Code, int result. Code, Intent data) { }
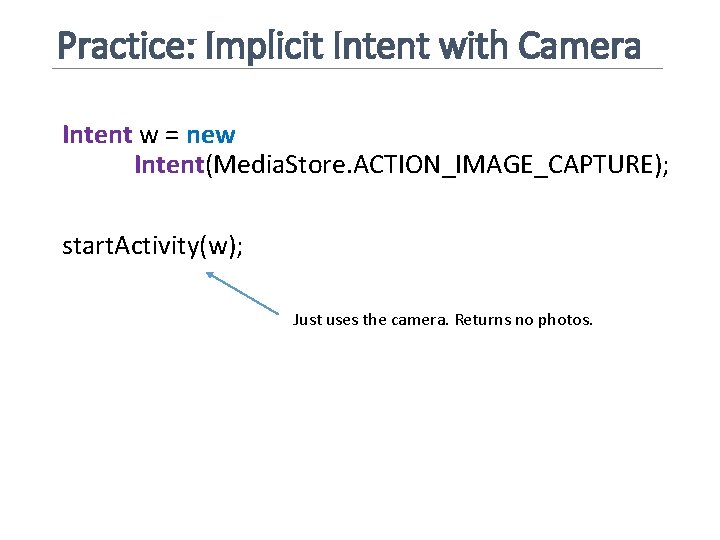
Practice: Implicit Intent with Camera Intent w = new Intent(Media. Store. ACTION_IMAGE_CAPTURE); start. Activity(w); Just uses the camera. Returns no photos.
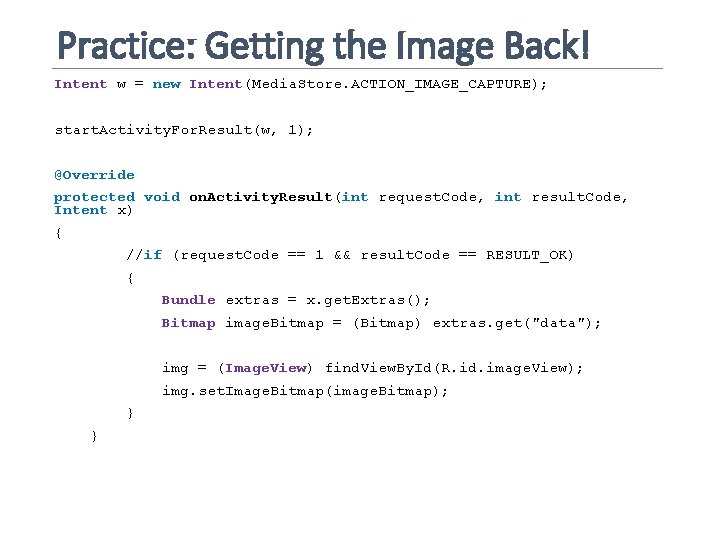
Practice: Getting the Image Back! Intent w = new Intent(Media. Store. ACTION_IMAGE_CAPTURE); start. Activity. For. Result(w, 1); @Override protected void on. Activity. Result(int request. Code, int result. Code, Intent x) { //if (request. Code == 1 && result. Code == RESULT_OK) { Bundle extras = x. get. Extras(); Bitmap image. Bitmap = (Bitmap) extras. get("data"); img = (Image. View) find. View. By. Id(R. id. image. View); img. set. Image. Bitmap(image. Bitmap); } }
Implicit intent example
Android.intent.action.phone_state
Android.intent.action.action_power_connected
Android basic components
Android advanced topics
Android lecture
01:640:244 lecture notes - lecture 15: plat, idah, farad
Mediastore.action_image_capture android 11
Android basic components
Android telephony architecture
Resource manager in android framework
Android game framework
Emory ras intent to submit
Always assume positive intent
Strategic fit vs strategic intent
Define strategic management
Ndia intent guide
Army pir examples
Intent resolution
Declaring intent lol
Essential intent
Creative intent meaning
Charred document example
Commander's intent example
Composers expressed nationalism in their music by
Fpmseta
User intent modeling
Icc rendering intent to wcs gamut mapping
National letter of intent sample
Example of intentional fallacy