Lecture 12 Iterators ADS 2 Lecture 12 1
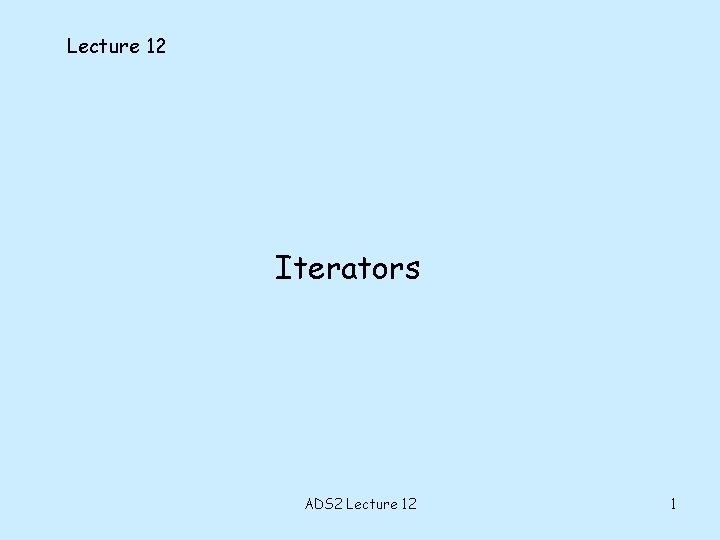
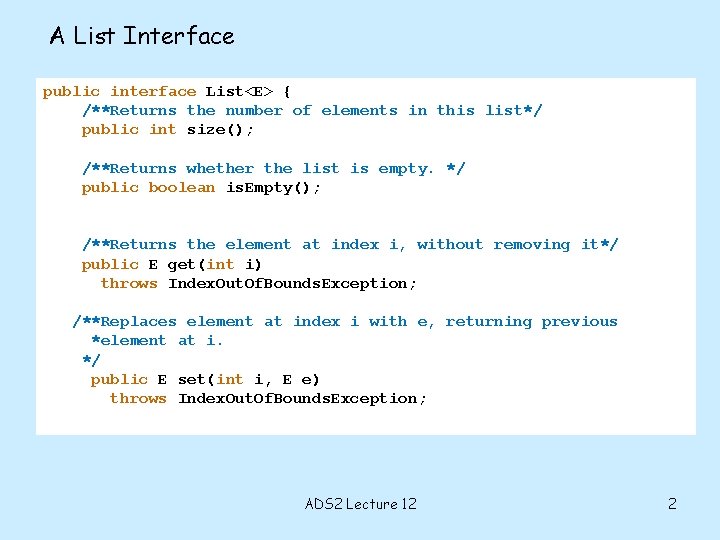
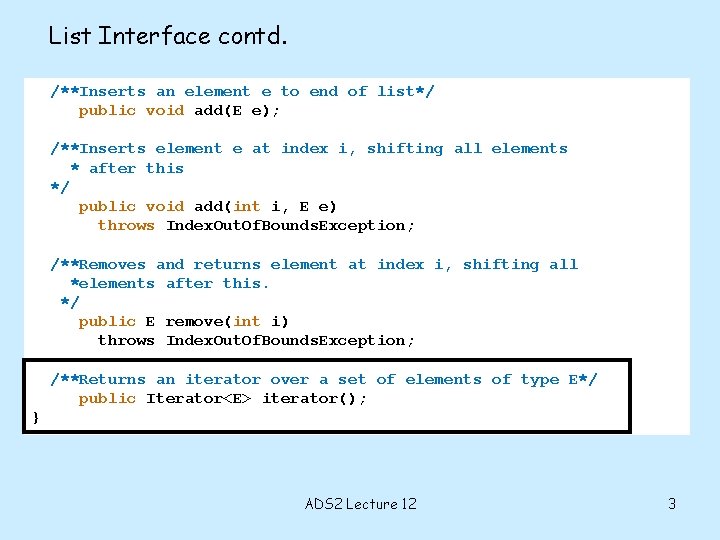
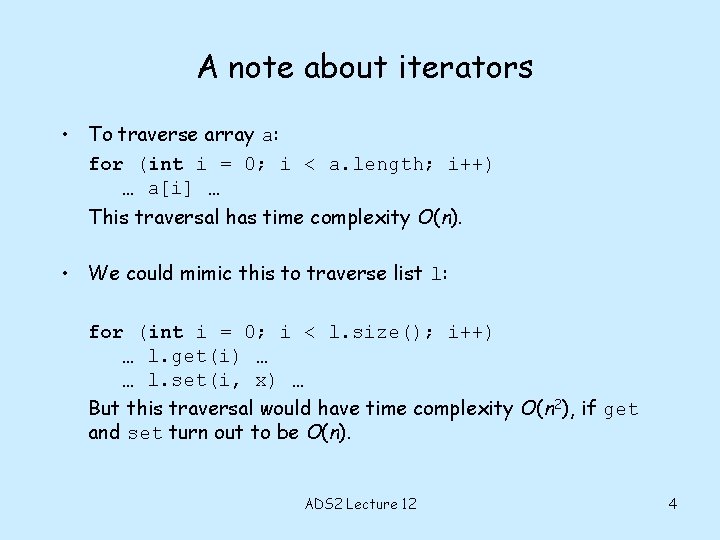
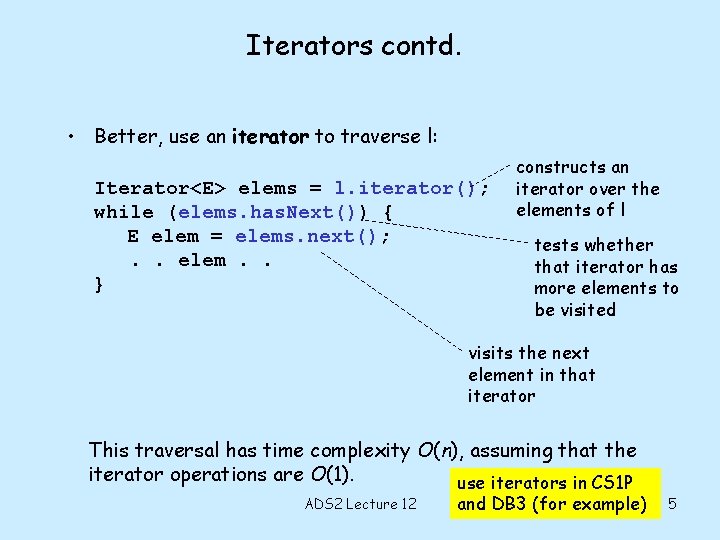
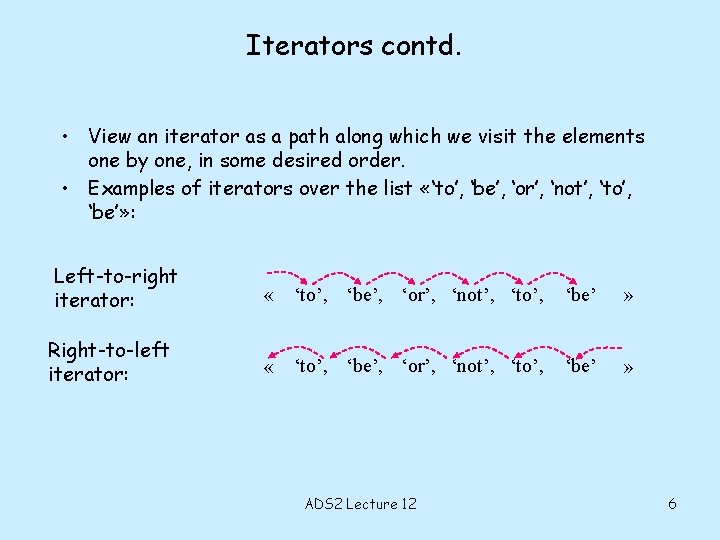
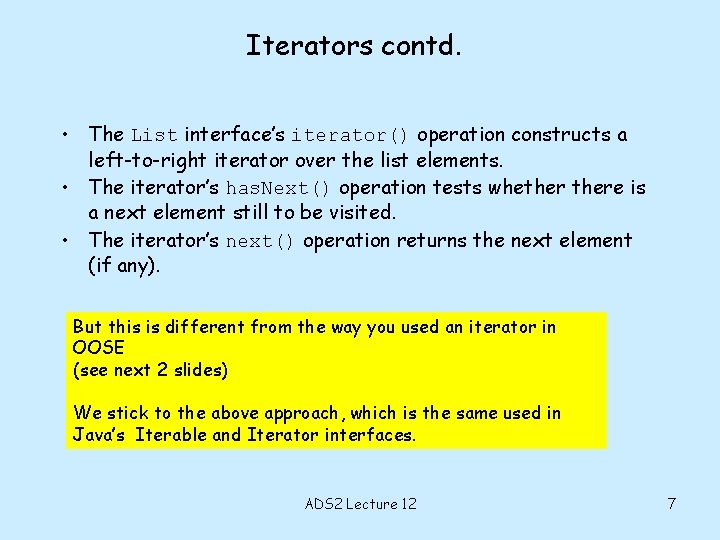
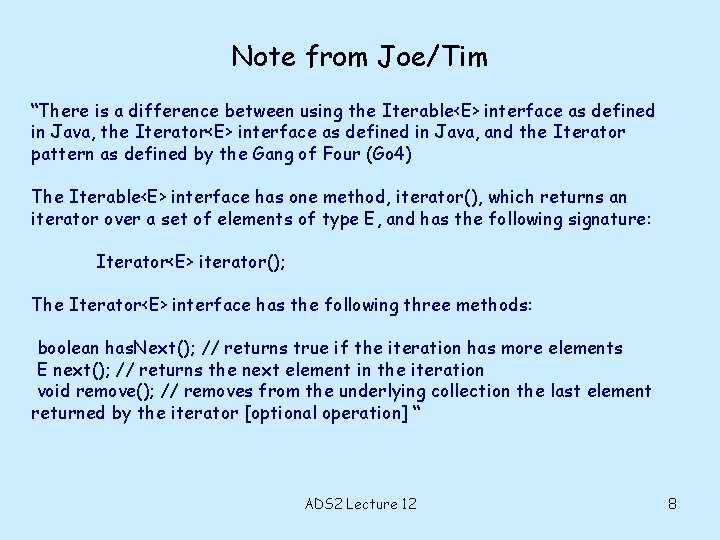
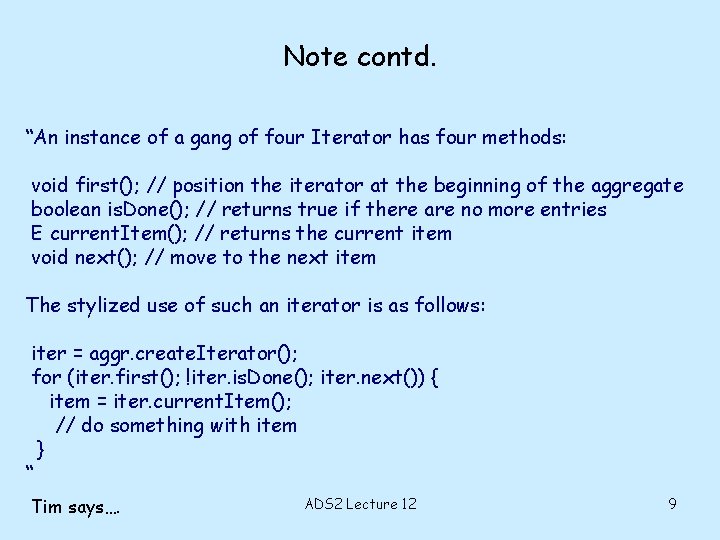
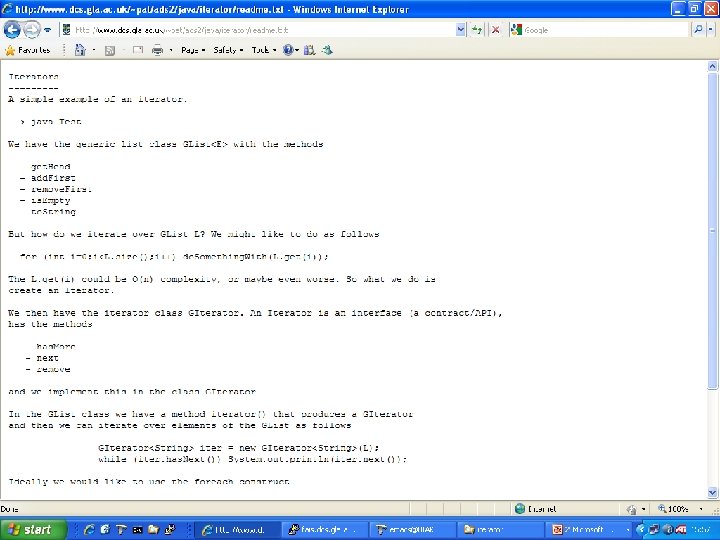
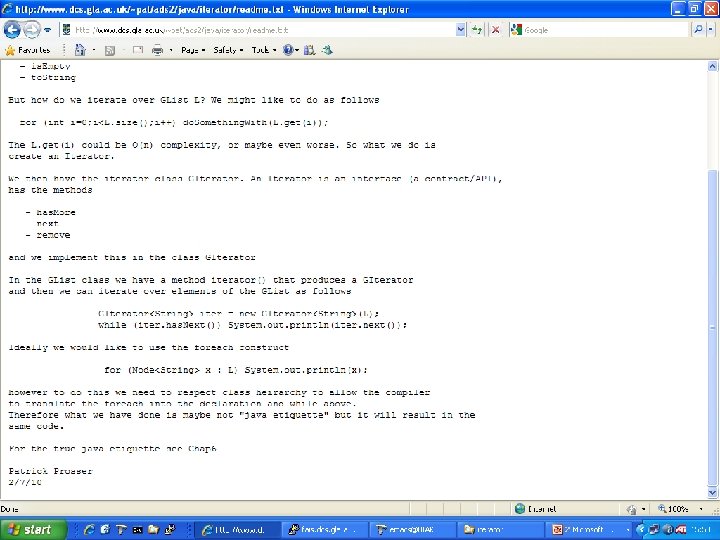
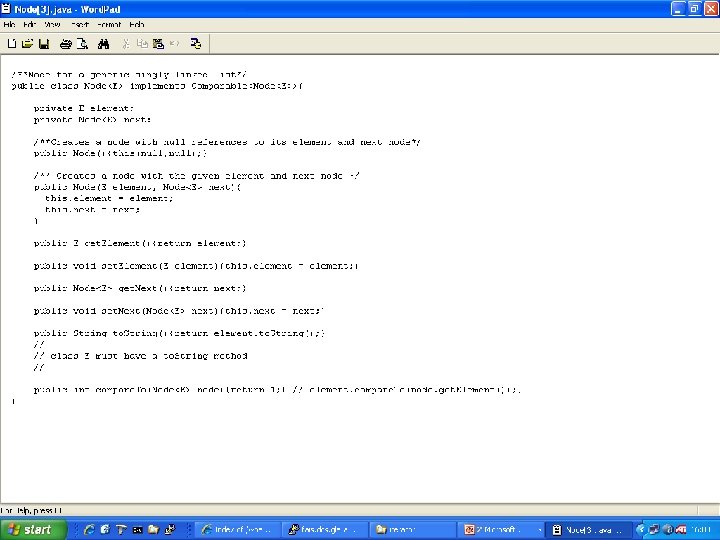
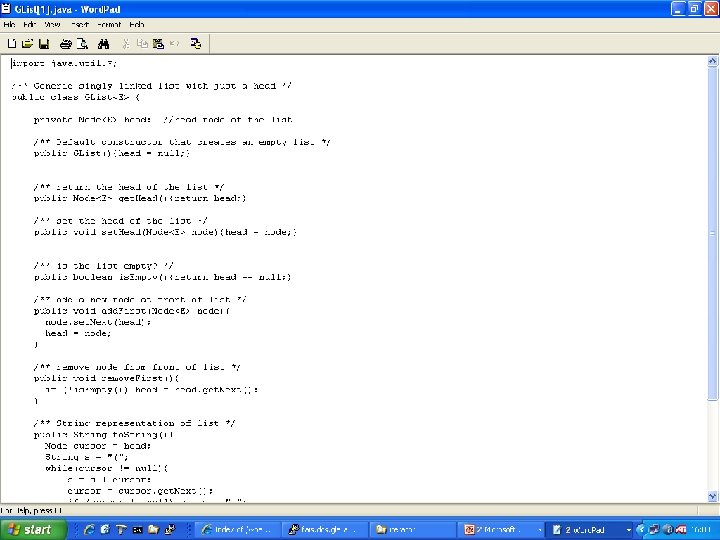
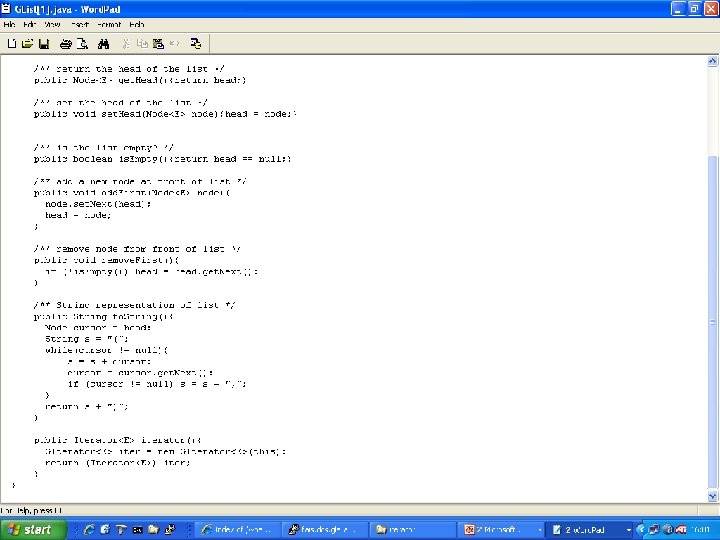
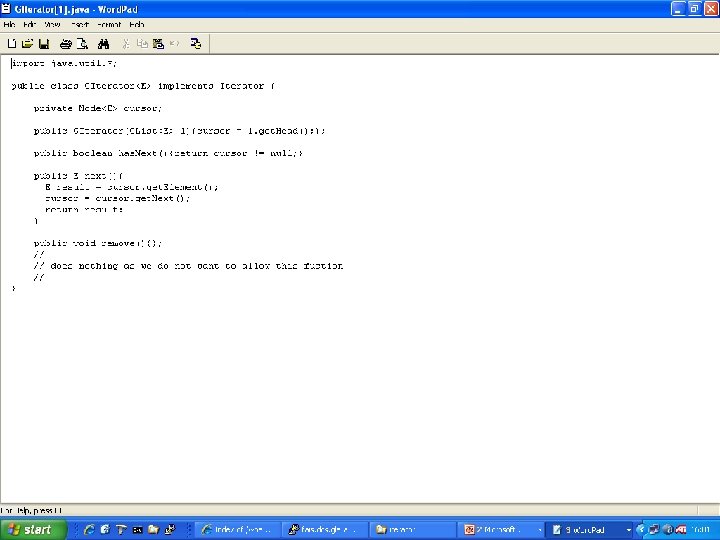
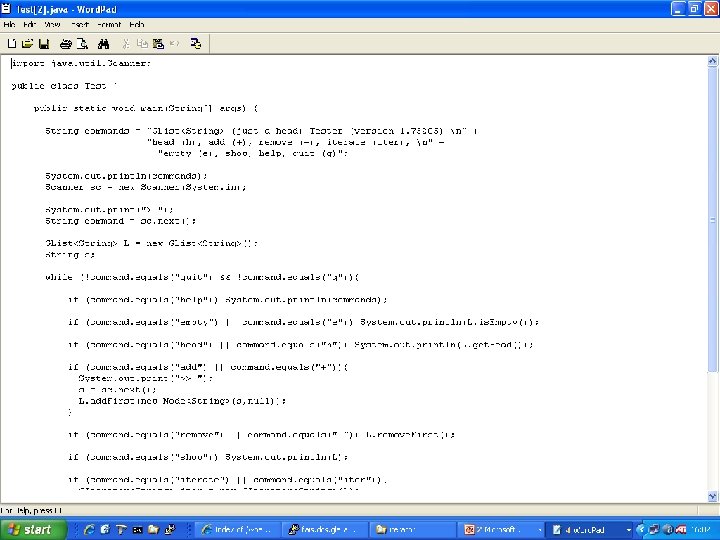
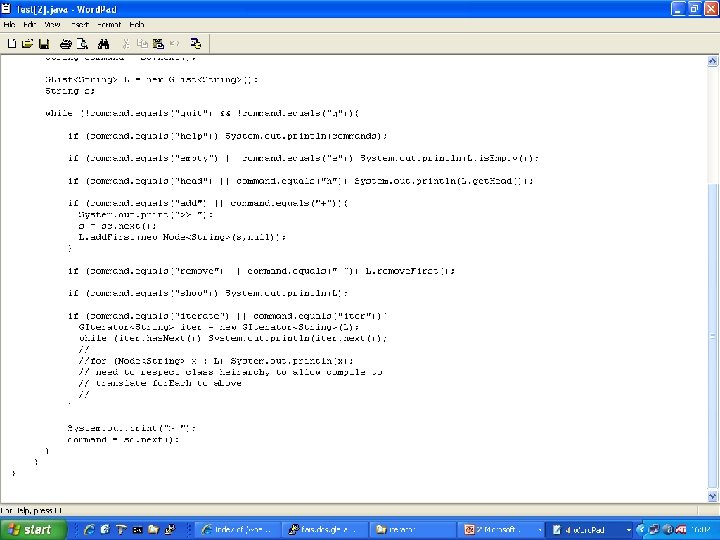
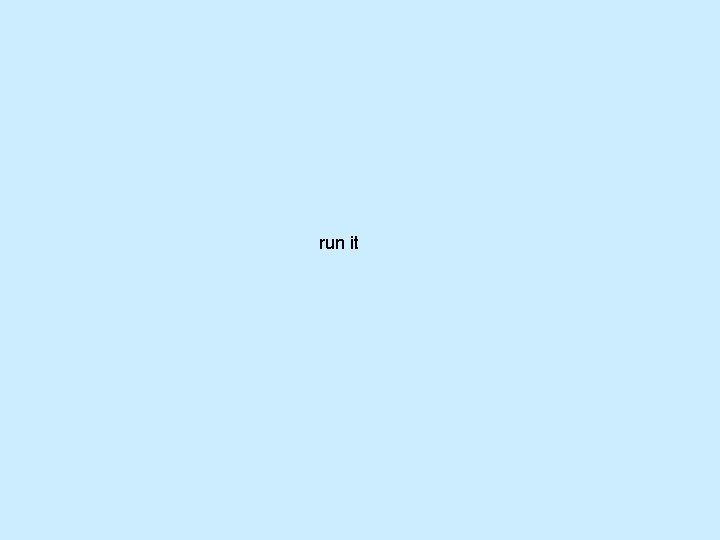
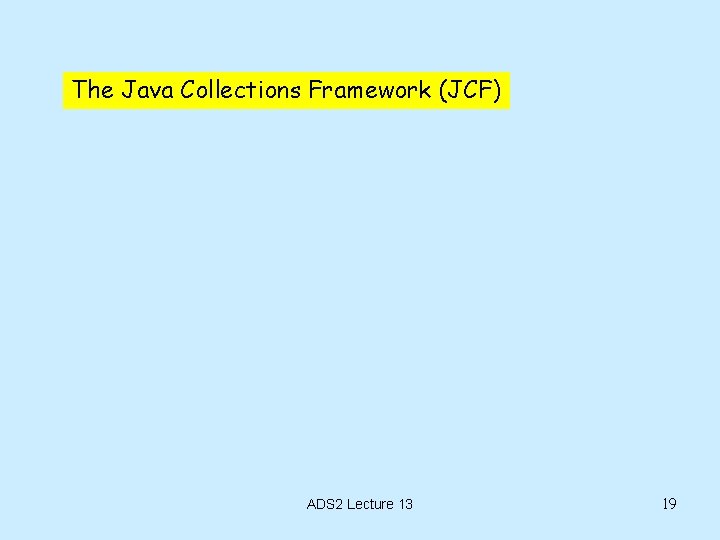
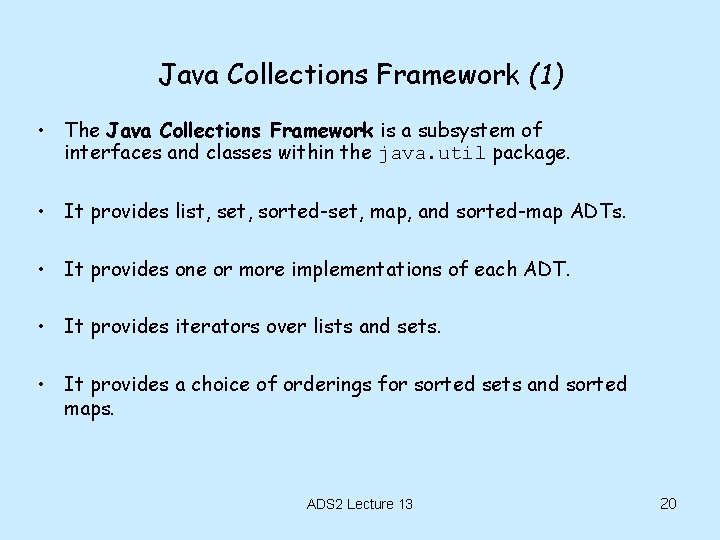
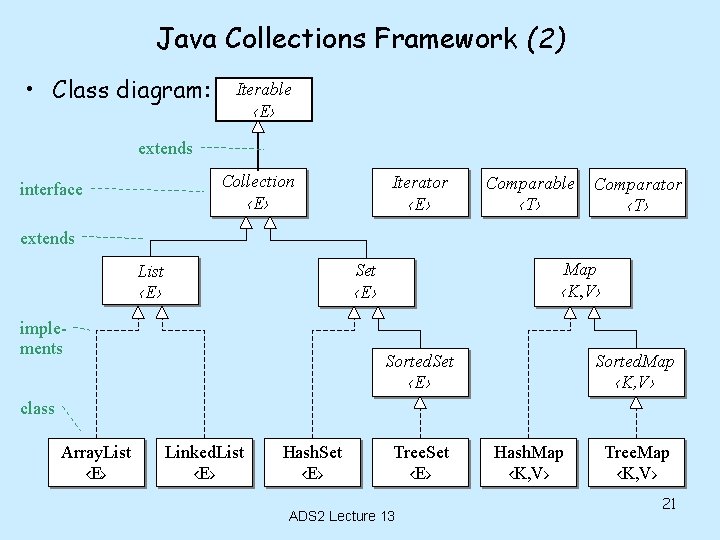
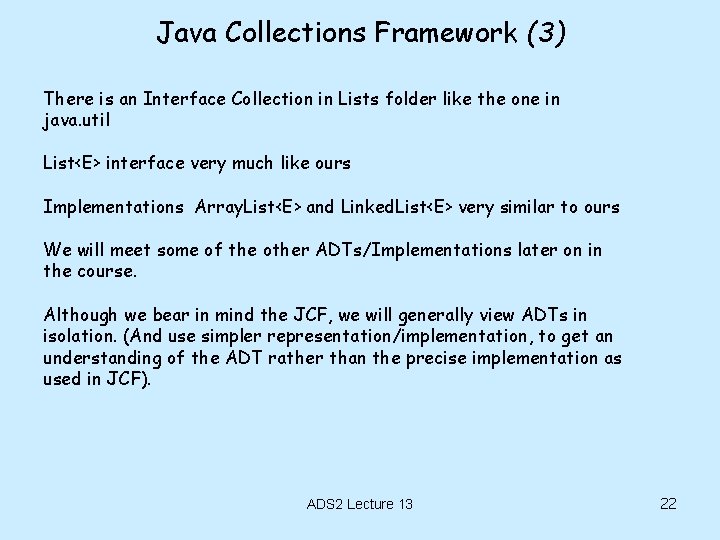
- Slides: 22
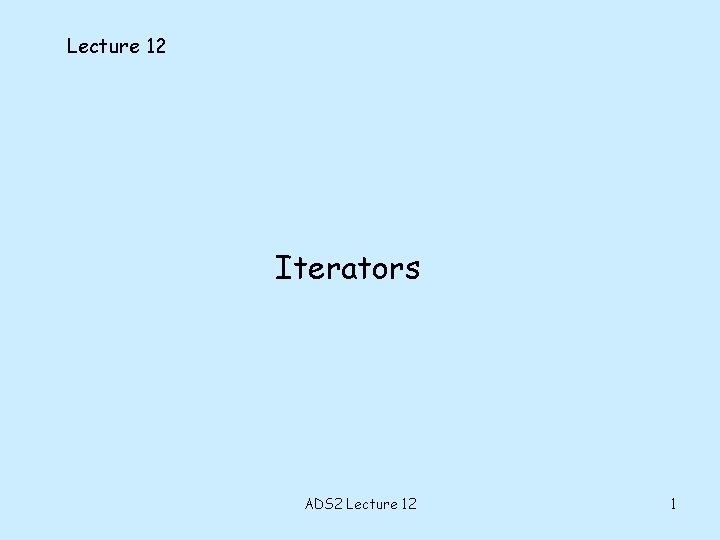
Lecture 12 Iterators ADS 2 Lecture 12 1
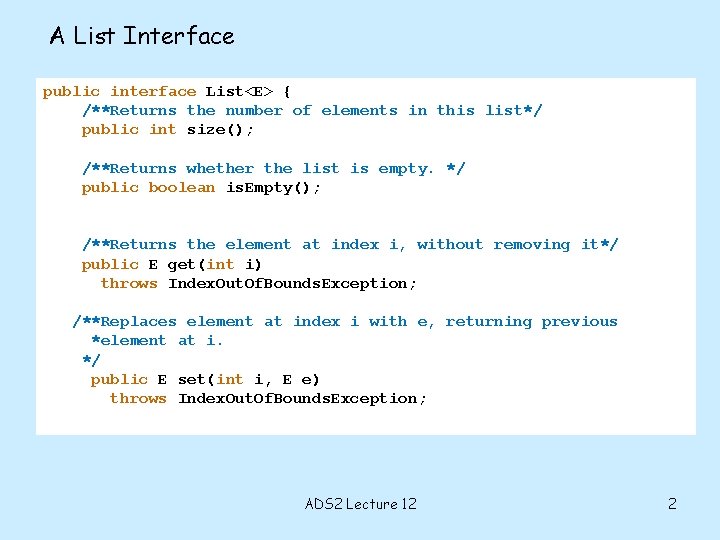
A List Interface public interface List<E> { /**Returns the number of elements in this list*/ public int size(); /**Returns whether the list is empty. */ public boolean is. Empty(); /**Returns the element at index i, without removing it*/ public E get(int i) throws Index. Out. Of. Bounds. Exception; /**Replaces element at index i with e, returning previous *element at i. */ public E set(int i, E e) throws Index. Out. Of. Bounds. Exception; ADS 2 Lecture 12 2
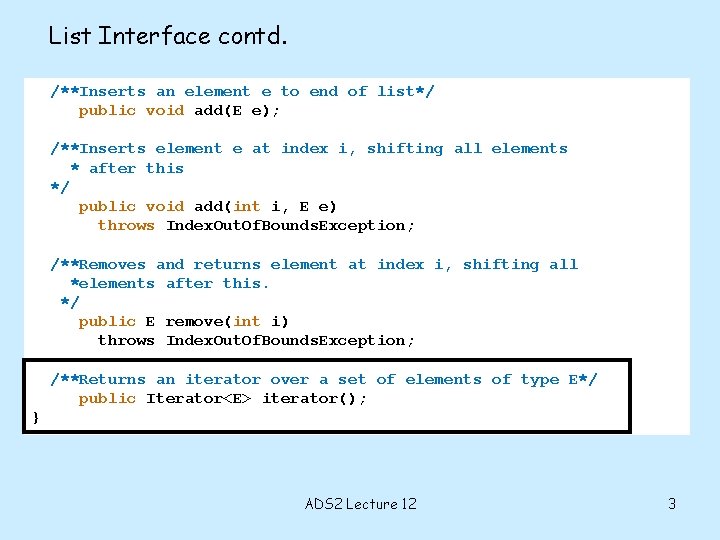
List Interface contd. /**Inserts an element e to end of list*/ public void add(E e); /**Inserts element e at index i, shifting all elements * after this */ public void add(int i, E e) throws Index. Out. Of. Bounds. Exception; /**Removes and returns element at index i, shifting all *elements after this. */ public E remove(int i) throws Index. Out. Of. Bounds. Exception; /**Returns an iterator over a set of elements of type E*/ public Iterator<E> iterator(); } ADS 2 Lecture 12 3
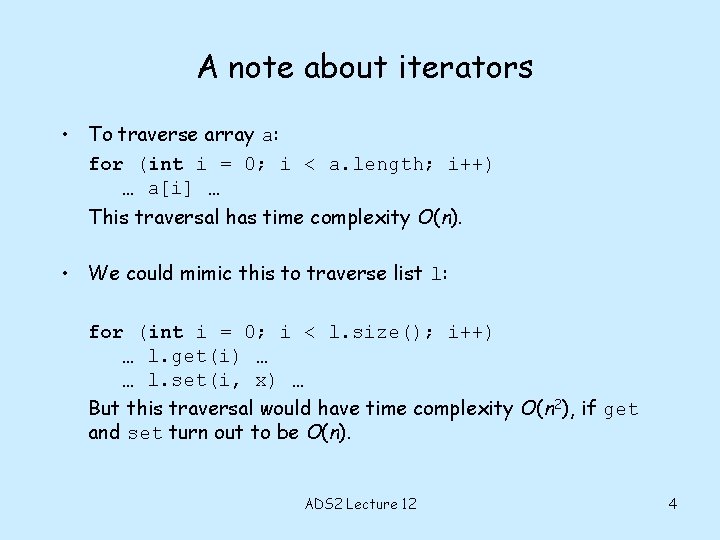
A note about iterators • To traverse array a: for (int i = 0; i < a. length; i++) … a[i] … This traversal has time complexity O(n). • We could mimic this to traverse list l: for (int i = 0; i < l. size(); i++) … l. get(i) … … l. set(i, x) … But this traversal would have time complexity O(n 2), if get and set turn out to be O(n). ADS 2 Lecture 12 4
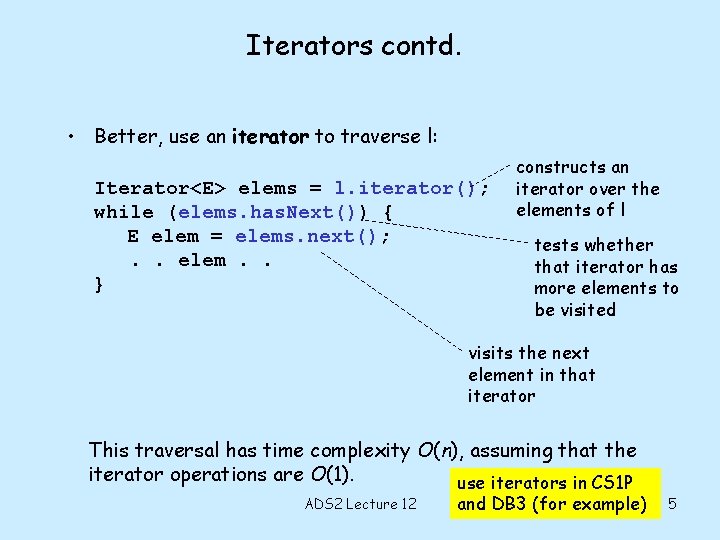
Iterators contd. • Better, use an iterator to traverse l: Iterator<E> elems = l. iterator(); while (elems. has. Next()) { E elem = elems. next(); . . elem. . } constructs an iterator over the elements of l tests whether that iterator has more elements to be visited visits the next element in that iterator This traversal has time complexity O(n), assuming that the iterator operations are O(1). use iterators in CS 1 P ADS 2 Lecture 12 and DB 3 (for example) 5
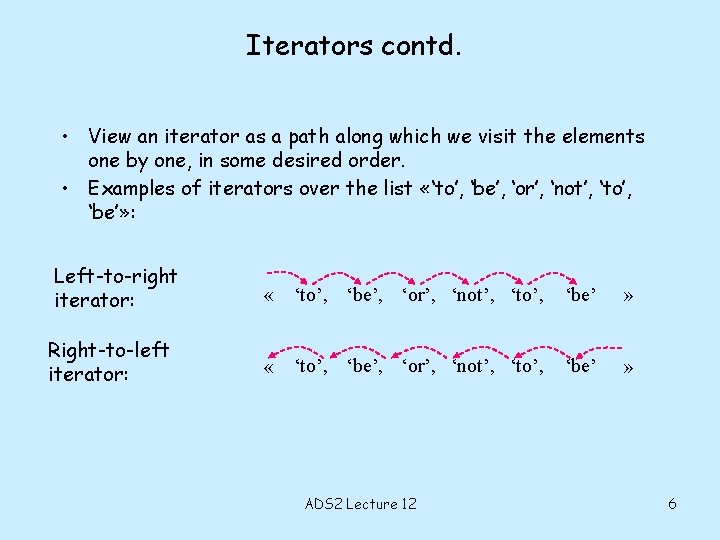
Iterators contd. • View an iterator as a path along which we visit the elements one by one, in some desired order. • Examples of iterators over the list «‘to’, ‘be’, ‘or’, ‘not’, ‘to’, ‘be’» : Left-to-right iterator: « ‘to’, ‘be’, ‘or’, ‘not’, ‘to’, ‘be’ » Right-to-left iterator: « ‘to’, ‘be’, ‘or’, ‘not’, ‘to’, ‘be’ » ADS 2 Lecture 12 6
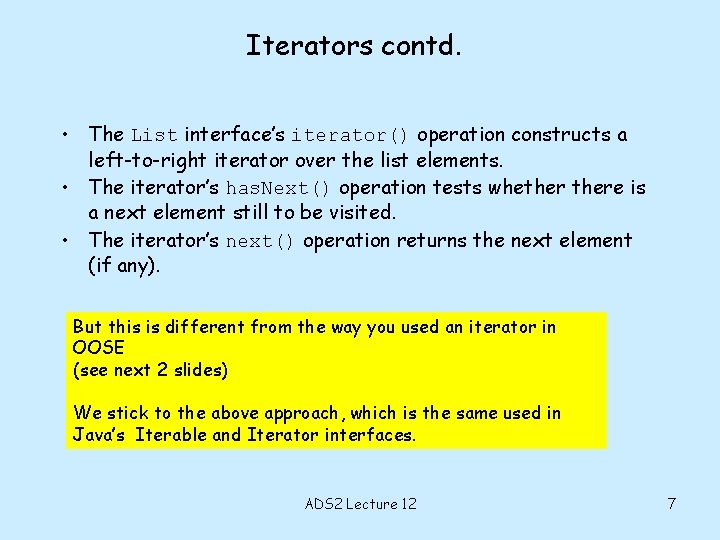
Iterators contd. • The List interface’s iterator() operation constructs a left-to-right iterator over the list elements. • The iterator’s has. Next() operation tests whethere is a next element still to be visited. • The iterator’s next() operation returns the next element (if any). But this is different from the way you used an iterator in OOSE (see next 2 slides) We stick to the above approach, which is the same used in Java’s Iterable and Iterator interfaces. ADS 2 Lecture 12 7
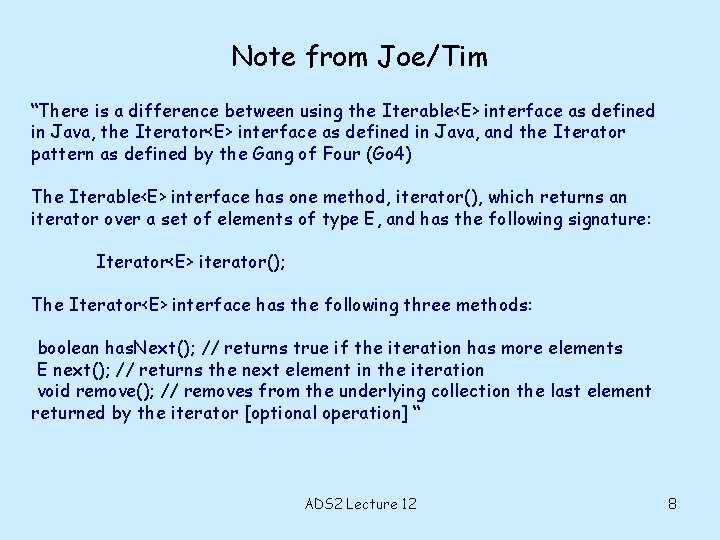
Note from Joe/Tim “There is a difference between using the Iterable<E> interface as defined in Java, the Iterator<E> interface as defined in Java, and the Iterator pattern as defined by the Gang of Four (Go 4) The Iterable<E> interface has one method, iterator(), which returns an iterator over a set of elements of type E, and has the following signature: Iterator<E> iterator(); The Iterator<E> interface has the following three methods: boolean has. Next(); // returns true if the iteration has more elements E next(); // returns the next element in the iteration void remove(); // removes from the underlying collection the last element returned by the iterator [optional operation] “ ADS 2 Lecture 12 8
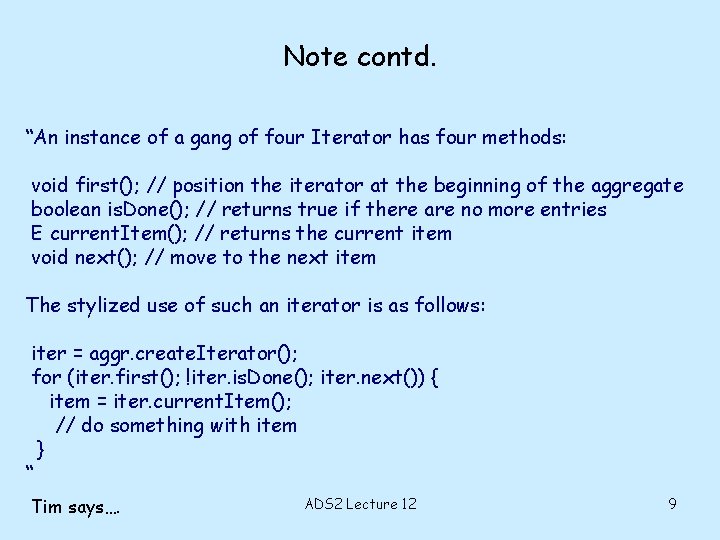
Note contd. “An instance of a gang of four Iterator has four methods: void first(); // position the iterator at the beginning of the aggregate boolean is. Done(); // returns true if there are no more entries E current. Item(); // returns the current item void next(); // move to the next item The stylized use of such an iterator is as follows: iter = aggr. create. Iterator(); for (iter. first(); !iter. is. Done(); iter. next()) { item = iter. current. Item(); // do something with item } “ Tim says…. ADS 2 Lecture 12 9
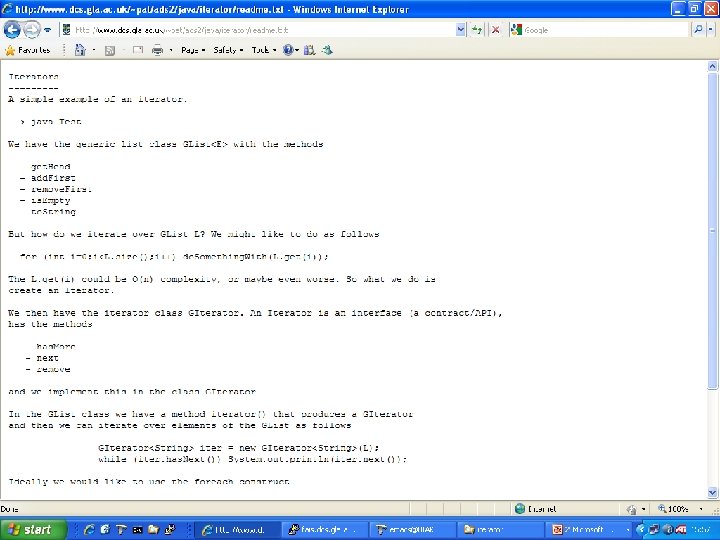
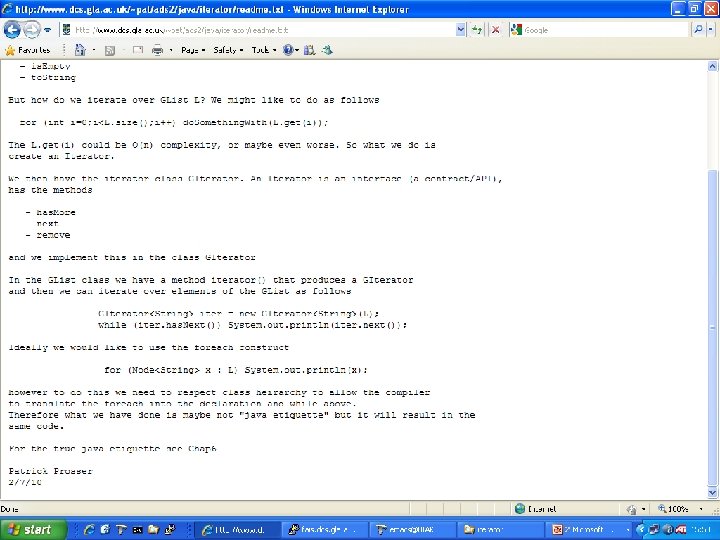
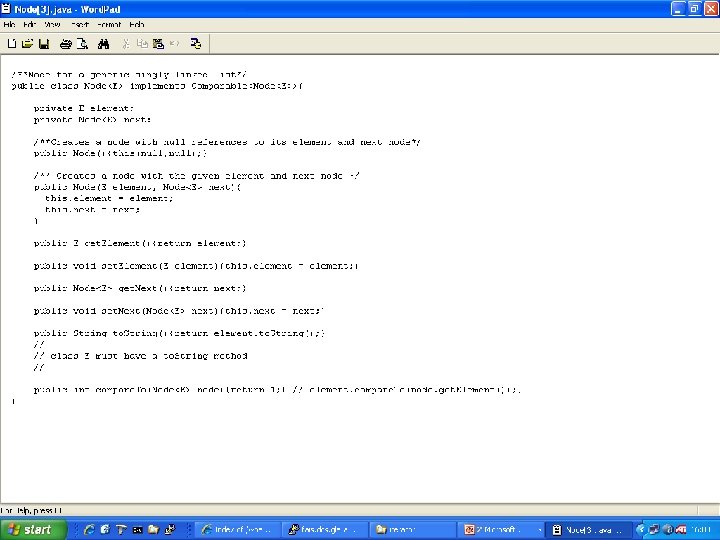
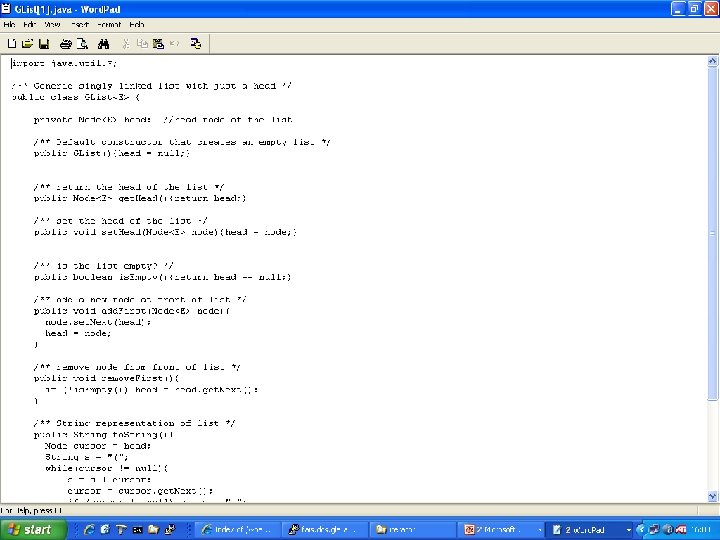
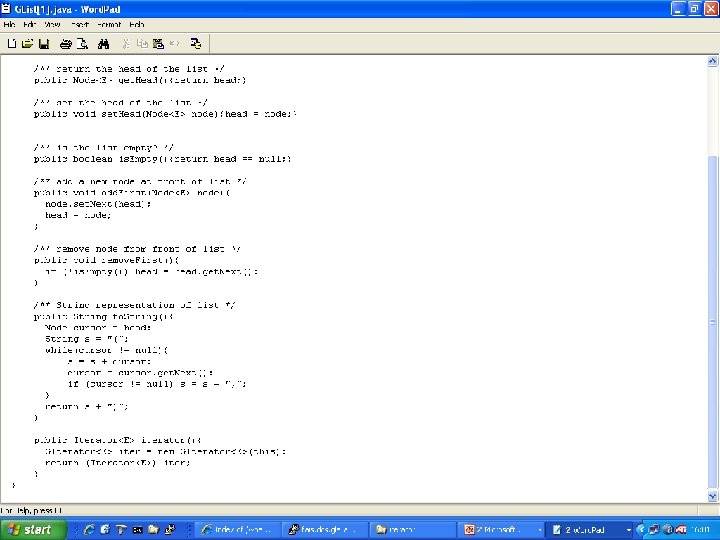
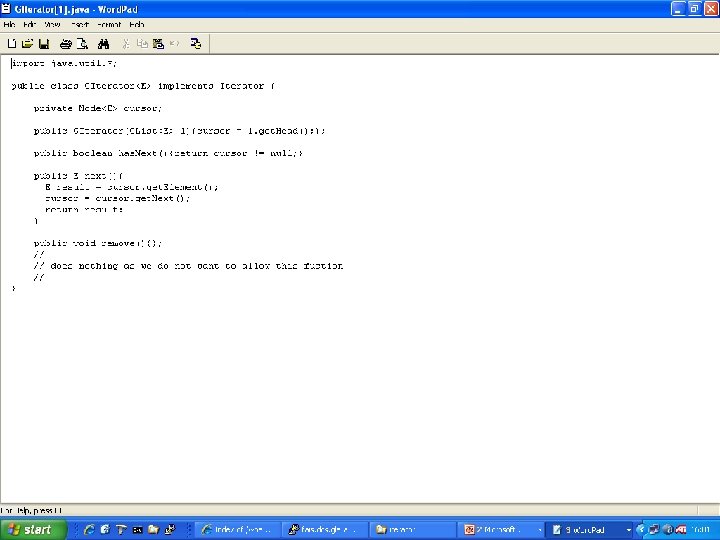
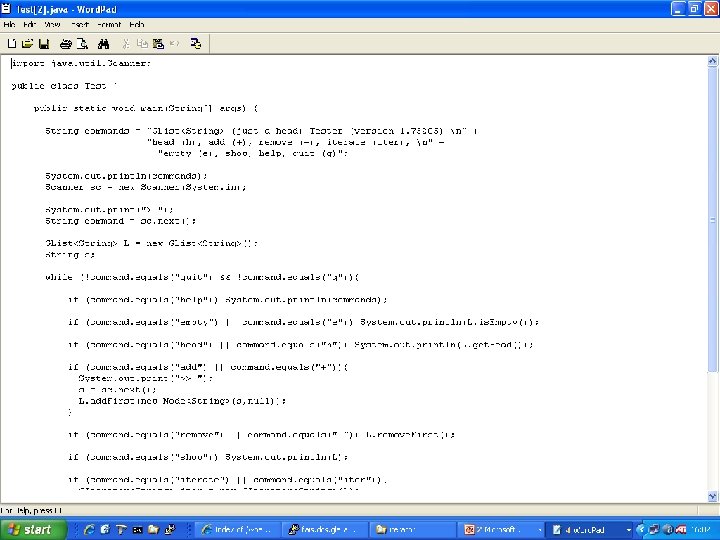
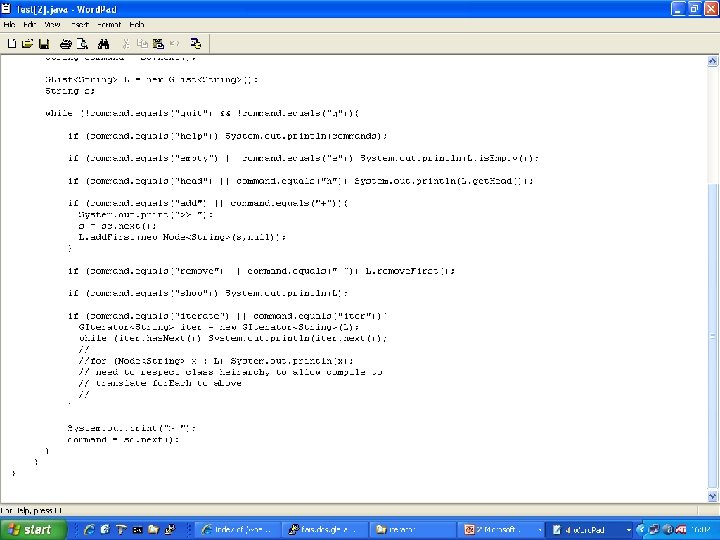
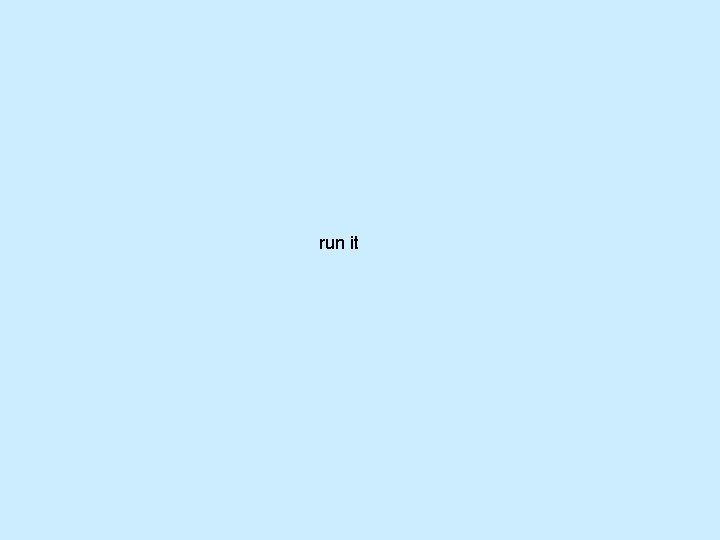
run it
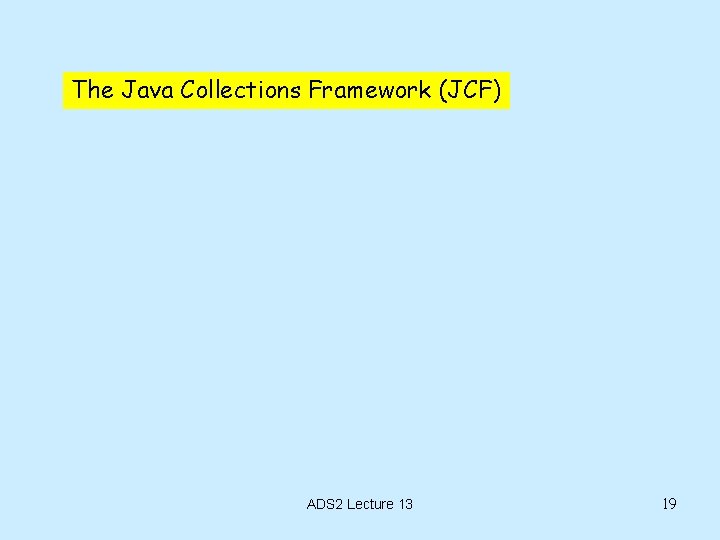
The Java Collections Framework (JCF) ADS 2 Lecture 13 19
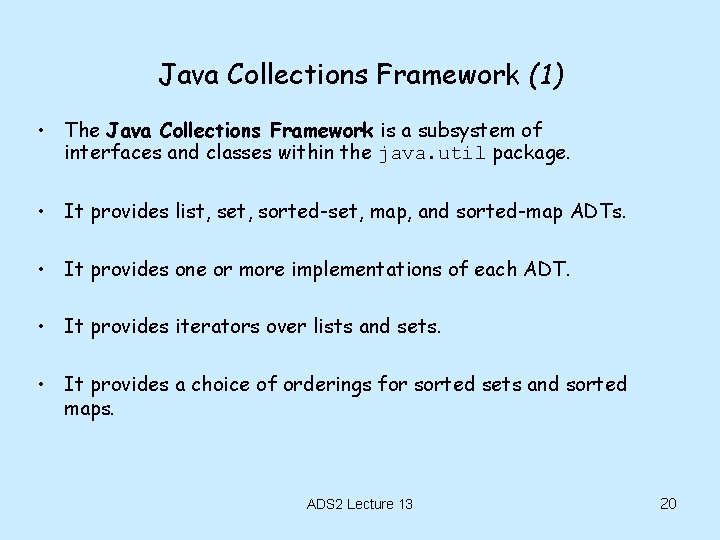
Java Collections Framework (1) • The Java Collections Framework is a subsystem of interfaces and classes within the java. util package. • It provides list, set, sorted-set, map, and sorted-map ADTs. • It provides one or more implementations of each ADT. • It provides iterators over lists and sets. • It provides a choice of orderings for sorted sets and sorted maps. ADS 2 Lecture 13 20
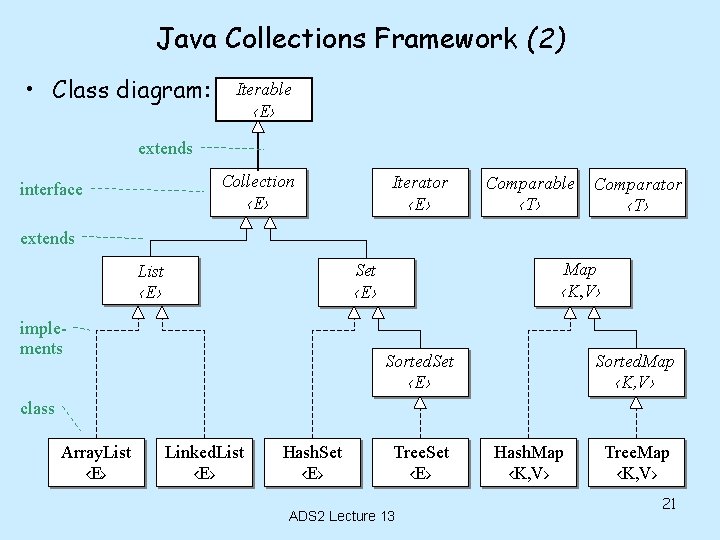
Java Collections Framework (2) • Class diagram: Iterable ‹E› extends Collection ‹E› interface Iterator ‹E› Comparable ‹T› Comparator ‹T› extends Map ‹K, V› Set ‹E› List ‹E› implements Sorted. Set ‹E› Sorted. Map ‹K, V› class Array. List ‹E› Linked. List ‹E› Hash. Set ‹E› Tree. Set ‹E› ADS 2 Lecture 13 Hash. Map ‹K, V› Tree. Map ‹K, V› 21
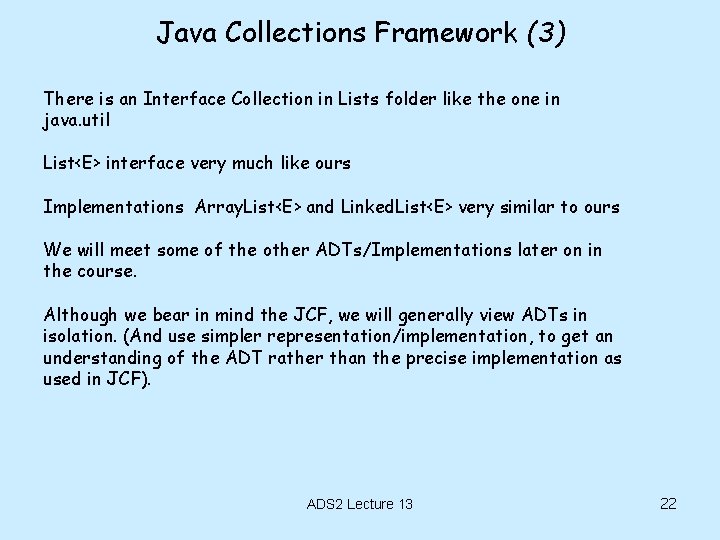
Java Collections Framework (3) There is an Interface Collection in Lists folder like the one in java. util List<E> interface very much like ours Implementations Array. List<E> and Linked. List<E> very similar to ours We will meet some of the other ADTs/Implementations later on in the course. Although we bear in mind the JCF, we will generally view ADTs in isolation. (And use simpler representation/implementation, to get an understanding of the ADT rather than the precise implementation as used in JCF). ADS 2 Lecture 13 22
Python iterators and generators
Maps and sets support bidirectional iterators.
Task 1833 in adm
Indoor and outdoor advertising media
01:640:244 lecture notes - lecture 15: plat, idah, farad
Slogan ruffles
Ad power amplifier
Game ads
Testimonial technique propaganda
Unfinished claim ads examples
Conative ads
Allusions in advertising
Card stacking propaganda
Dns server for raspberry pi
Usaid ads 579
Name calling persuasive technique
Adbusters
Eid mubarak mcdonald's ad
Power flarm
Ads menetlusrakendus
Ads girl
Loaded language ads
Public service advertisment