Lecture 11 q Enumeration Type q Userdefined Structure
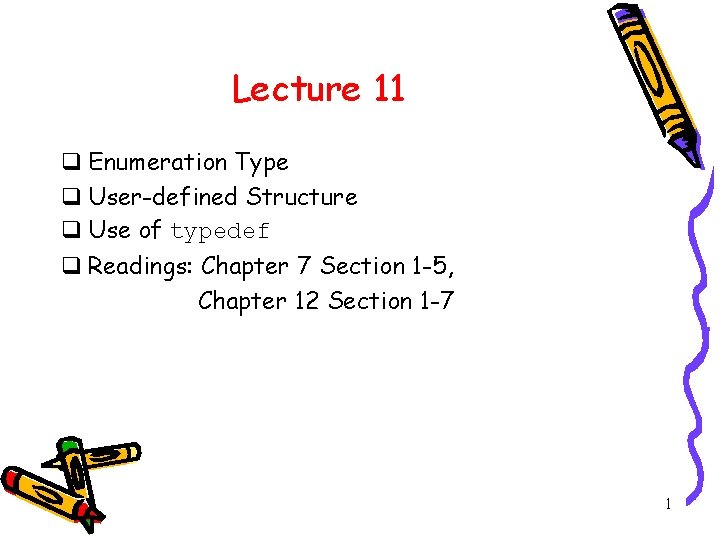
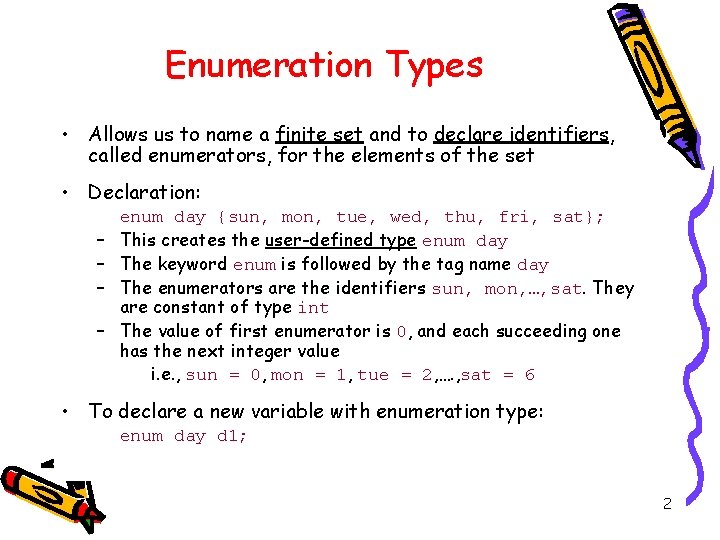
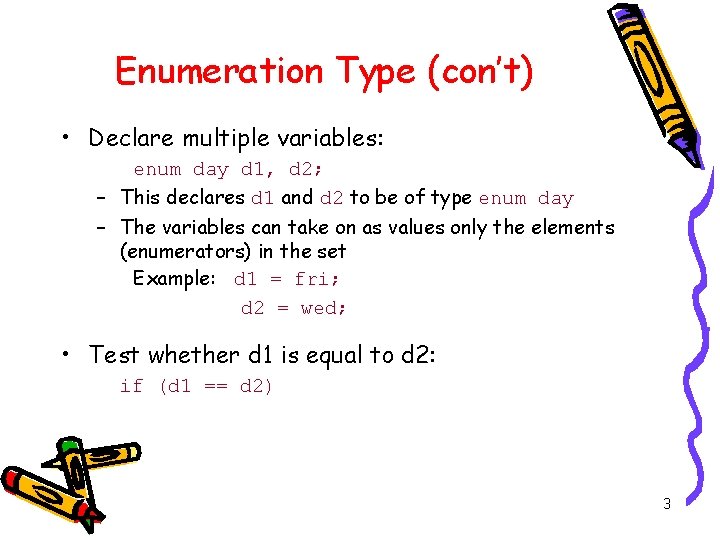
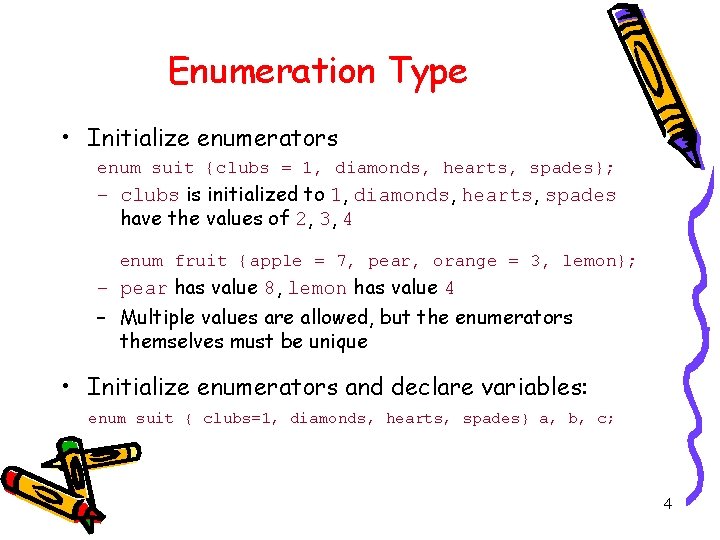
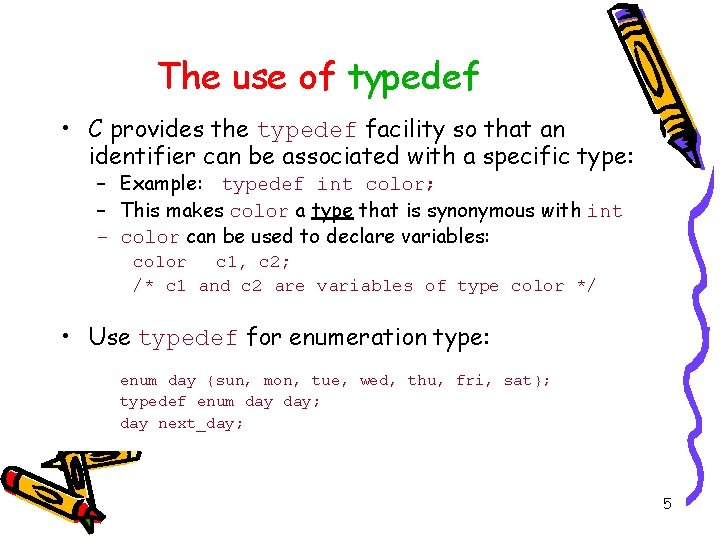
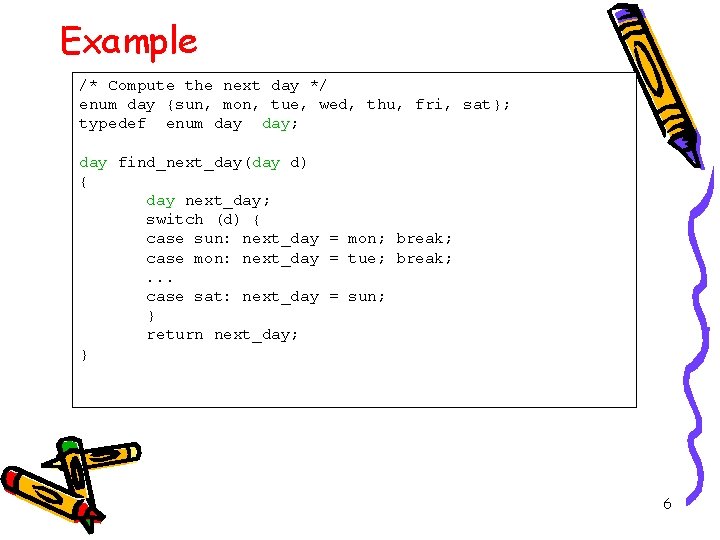
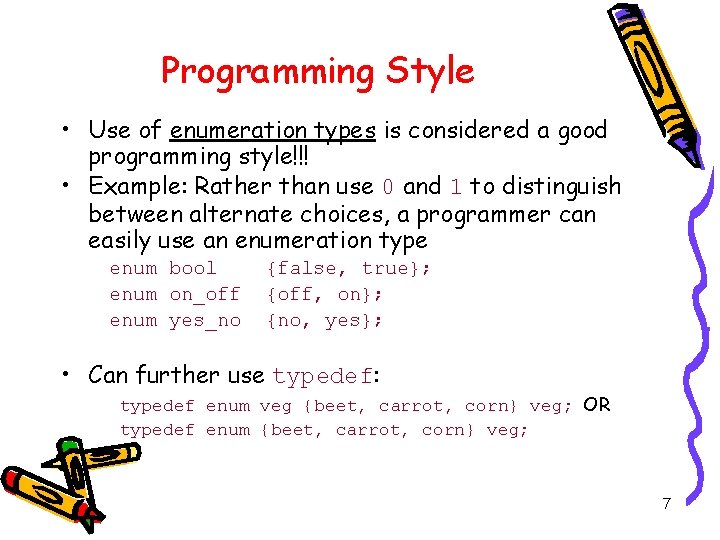
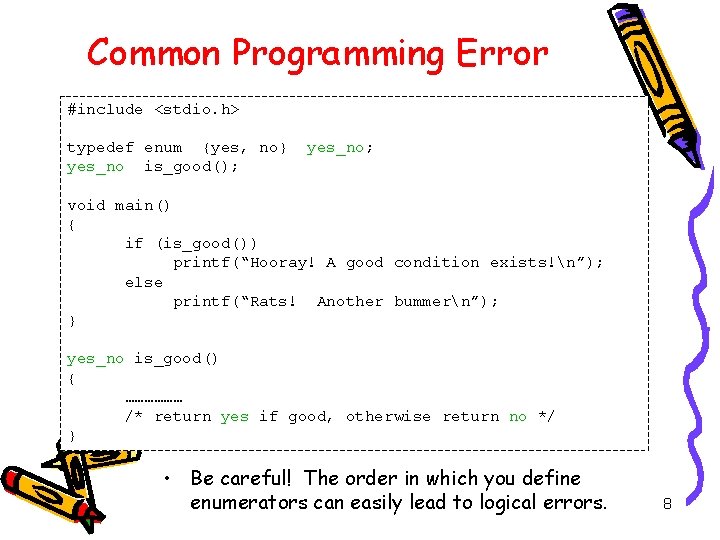
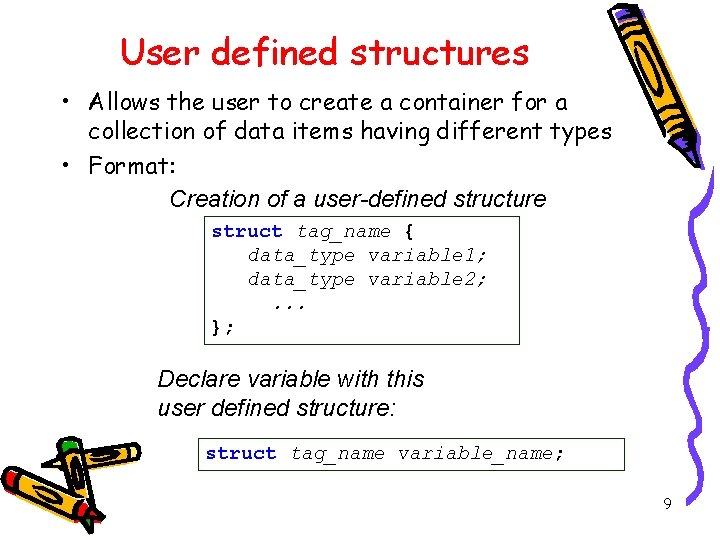
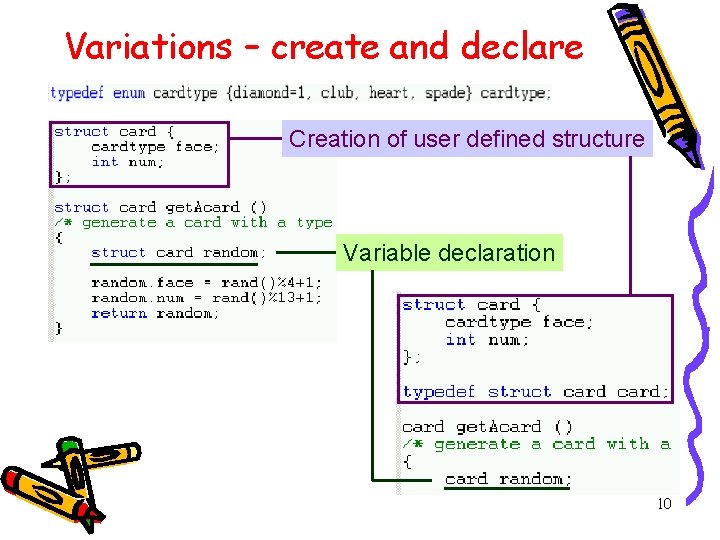
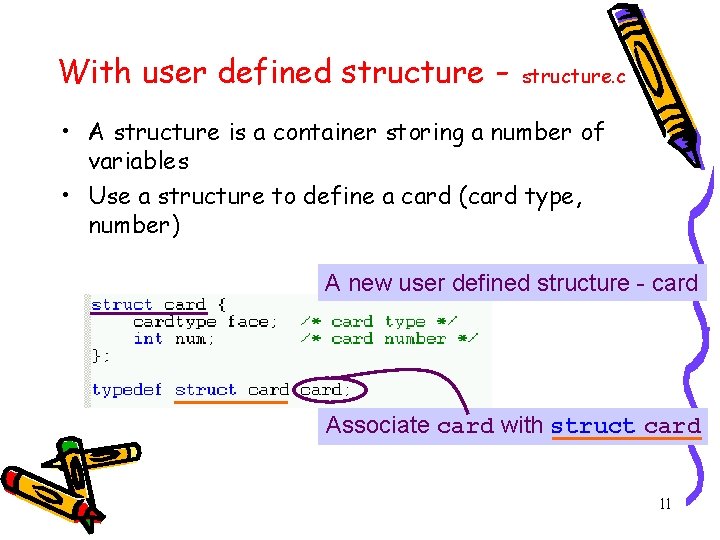
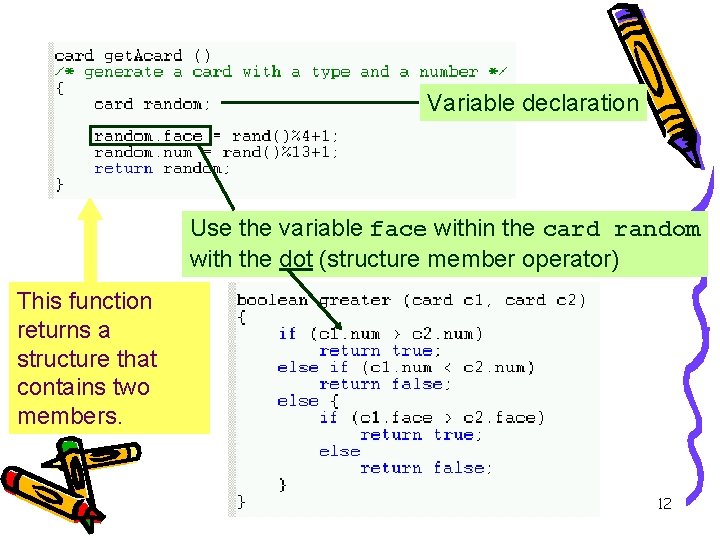
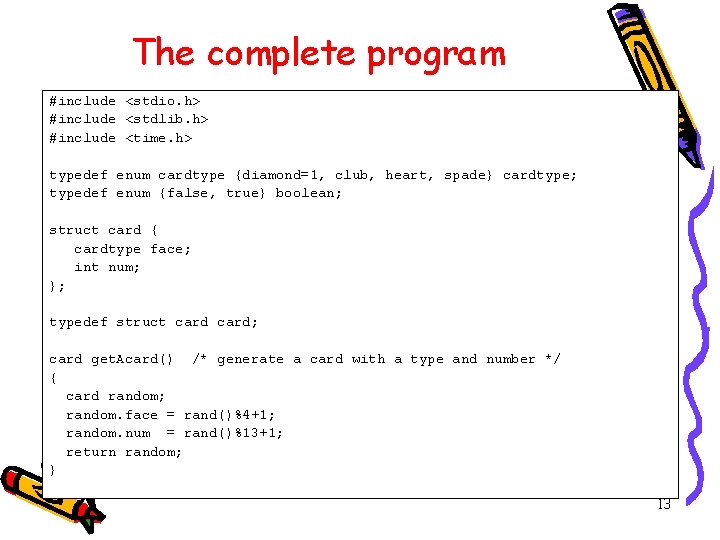
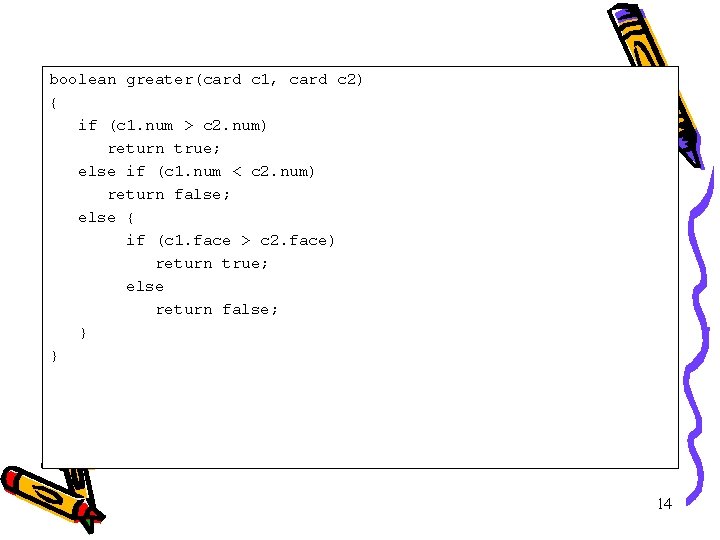
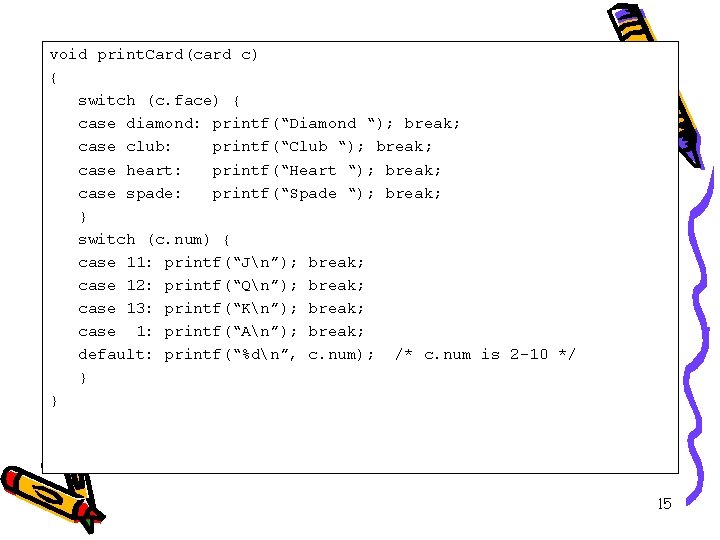
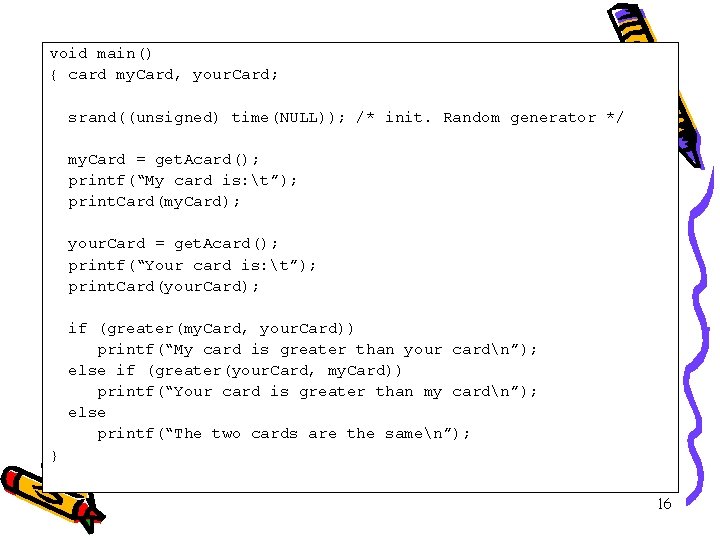
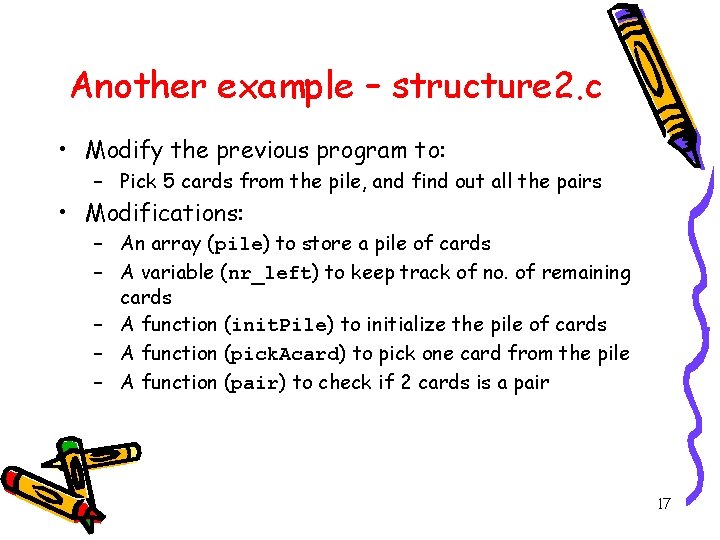
![card pile[52]; int nr_left; void init. Pile() { int i; for (i=0; i<52; i++) card pile[52]; int nr_left; void init. Pile() { int i; for (i=0; i<52; i++)](https://slidetodoc.com/presentation_image_h2/f0355f54f2da5f67b39cadcc3b918b64/image-18.jpg)
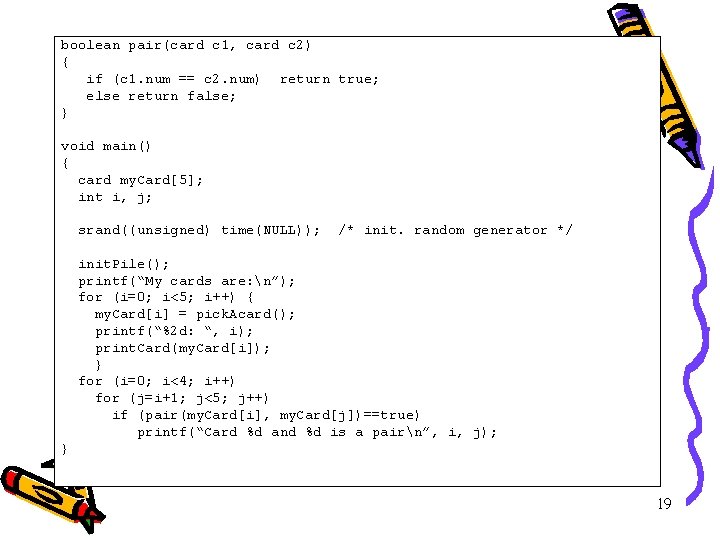
- Slides: 19
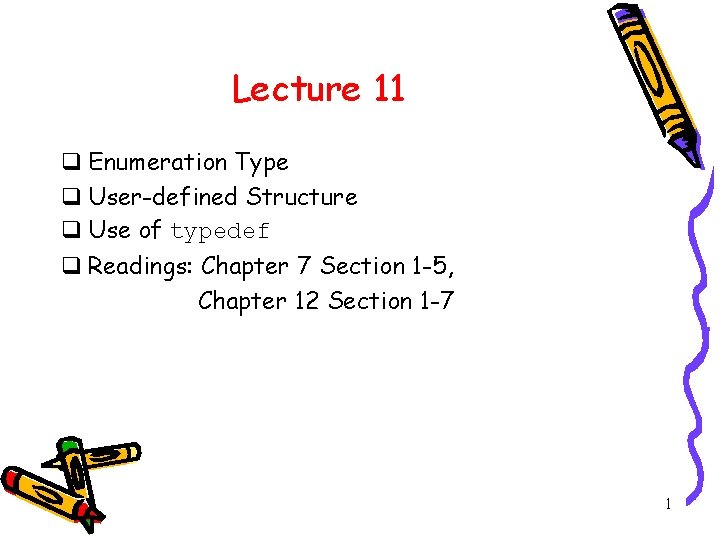
Lecture 11 q Enumeration Type q User-defined Structure q Use of typedef q Readings: Chapter 7 Section 1 -5, Chapter 12 Section 1 -7 1
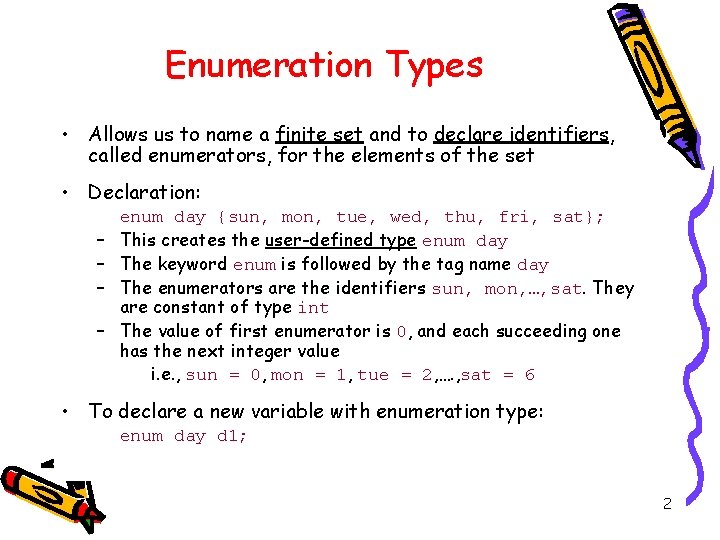
Enumeration Types • Allows us to name a finite set and to declare identifiers, called enumerators, for the elements of the set • Declaration: – – enum day {sun, mon, tue, wed, thu, fri, sat}; This creates the user-defined type enum day The keyword enum is followed by the tag name day The enumerators are the identifiers sun, mon, …, sat. They are constant of type int The value of first enumerator is 0, and each succeeding one has the next integer value i. e. , sun = 0, mon = 1, tue = 2, …. , sat = 6 • To declare a new variable with enumeration type: enum day d 1; 2
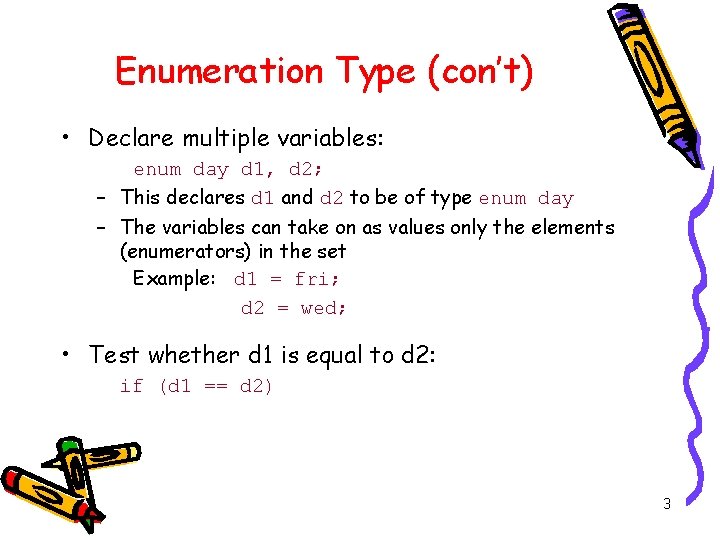
Enumeration Type (con’t) • Declare multiple variables: enum day d 1, d 2; – This declares d 1 and d 2 to be of type enum day – The variables can take on as values only the elements (enumerators) in the set Example: d 1 = fri; d 2 = wed; • Test whether d 1 is equal to d 2: if (d 1 == d 2) 3
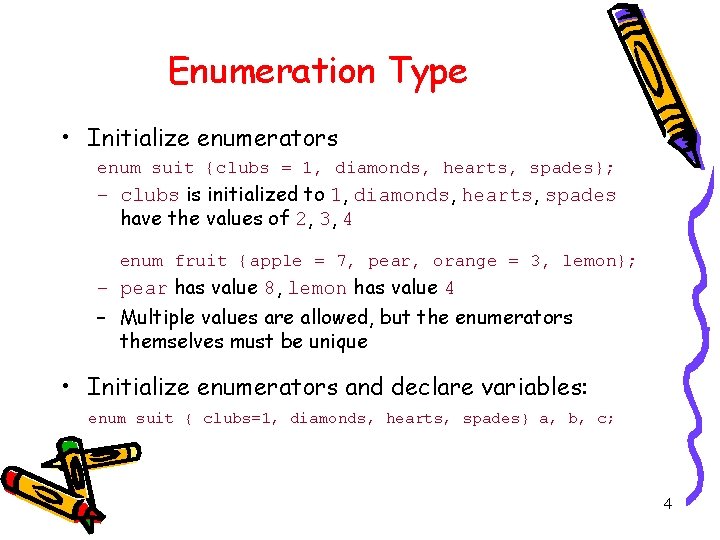
Enumeration Type • Initialize enumerators enum suit {clubs = 1, diamonds, hearts, spades}; – clubs is initialized to 1, diamonds, hearts, spades have the values of 2, 3, 4 enum fruit {apple = 7, pear, orange = 3, lemon}; – pear has value 8, lemon has value 4 – Multiple values are allowed, but the enumerators themselves must be unique • Initialize enumerators and declare variables: enum suit { clubs=1, diamonds, hearts, spades} a, b, c; 4
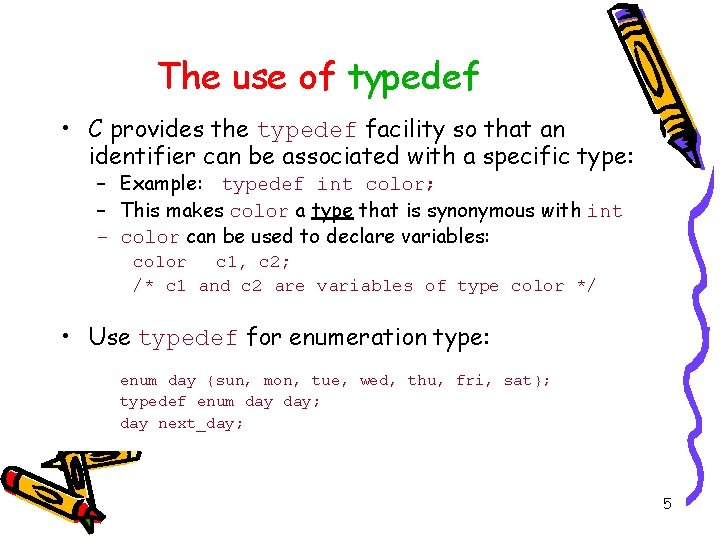
The use of typedef • C provides the typedef facility so that an identifier can be associated with a specific type: – Example: typedef int color; – This makes color a type that is synonymous with int – color can be used to declare variables: color c 1, c 2; /* c 1 and c 2 are variables of type color */ • Use typedef for enumeration type: enum day {sun, mon, tue, wed, thu, fri, sat}; typedef enum day; day next_day; 5
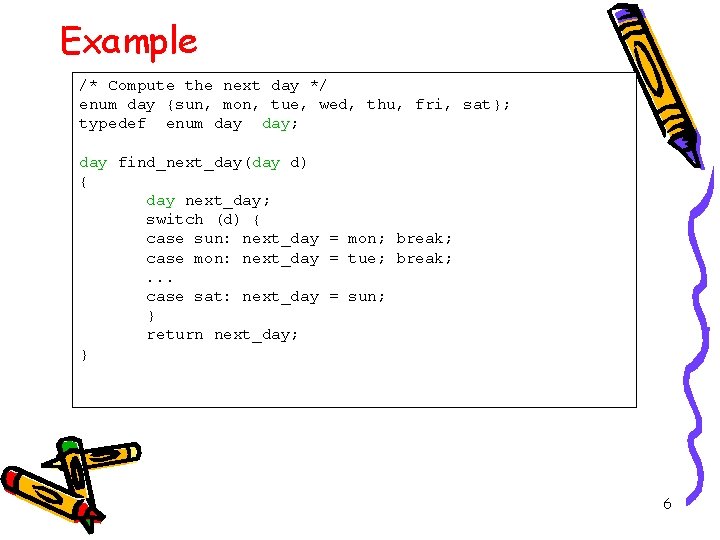
Example /* Compute the next day */ enum day {sun, mon, tue, wed, thu, fri, sat}; typedef enum day; day find_next_day(day d) { day next_day; switch (d) { case sun: next_day = mon; break; case mon: next_day = tue; break; . . . case sat: next_day = sun; } return next_day; } 6
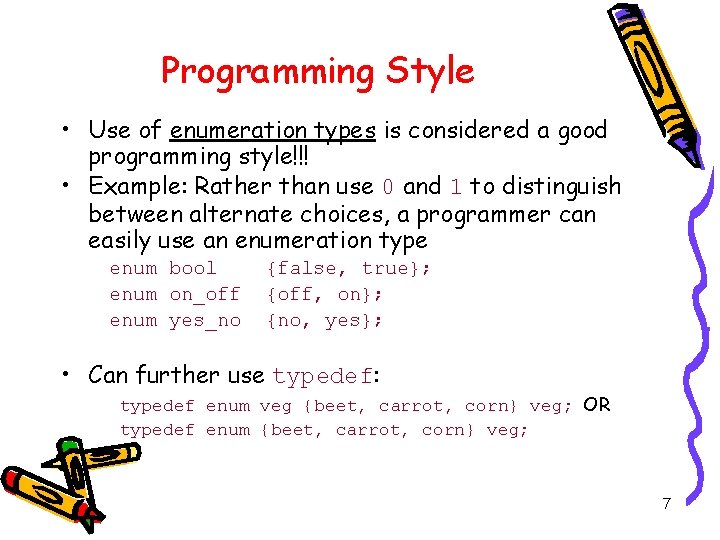
Programming Style • Use of enumeration types is considered a good programming style!!! • Example: Rather than use 0 and 1 to distinguish between alternate choices, a programmer can easily use an enumeration type enum bool enum on_off enum yes_no {false, true}; {off, on}; {no, yes}; • Can further use typedef: typedef enum veg {beet, carrot, corn} veg; OR typedef enum {beet, carrot, corn} veg; 7
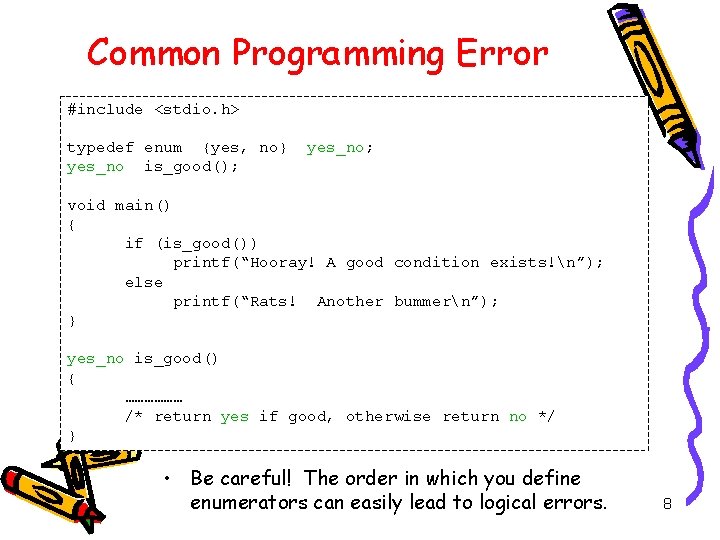
Common Programming Error #include <stdio. h> typedef enum {yes, no} yes_no is_good(); yes_no; void main() { if (is_good()) printf(“Hooray! A good condition exists!n”); else printf(“Rats! Another bummern”); } yes_no is_good() { ……………… /* return yes if good, otherwise return no */ } • Be careful! The order in which you define enumerators can easily lead to logical errors. 8
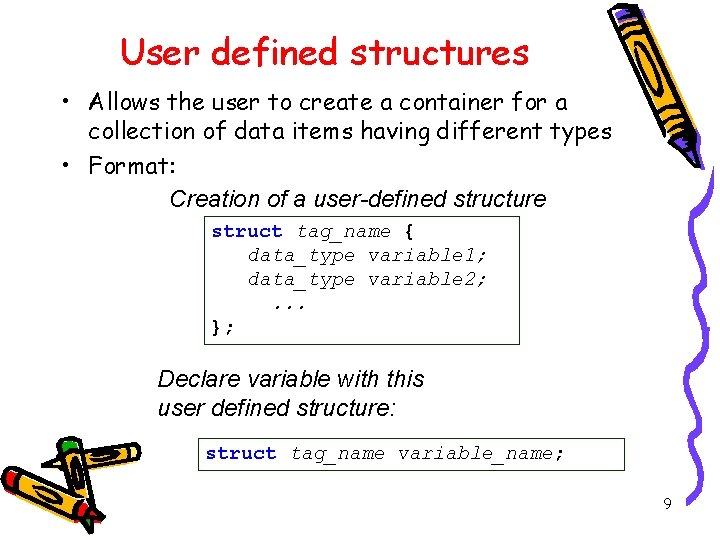
User defined structures • Allows the user to create a container for a collection of data items having different types • Format: Creation of a user-defined structure struct tag_name { data_type variable 1; data_type variable 2; . . . }; Declare variable with this user defined structure: struct tag_name variable_name; 9
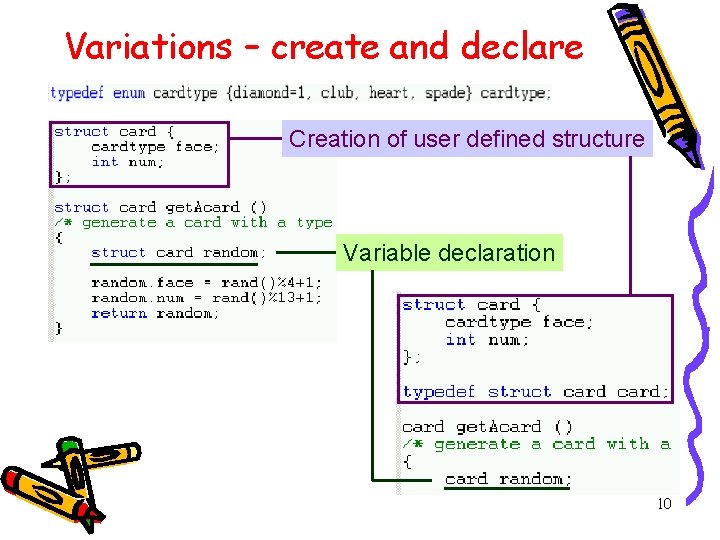
Variations – create and declare Creation of user defined structure Variable declaration 10
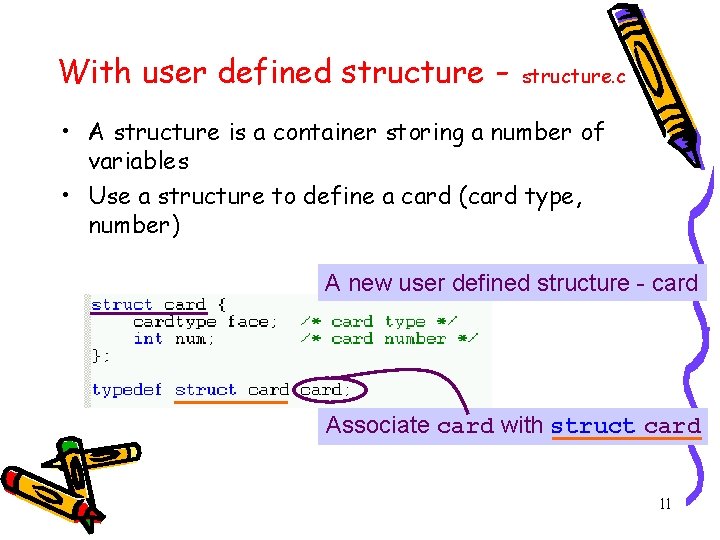
With user defined structure - structure. c • A structure is a container storing a number of variables • Use a structure to define a card (card type, number) A new user defined structure - card Associate card with struct card 11
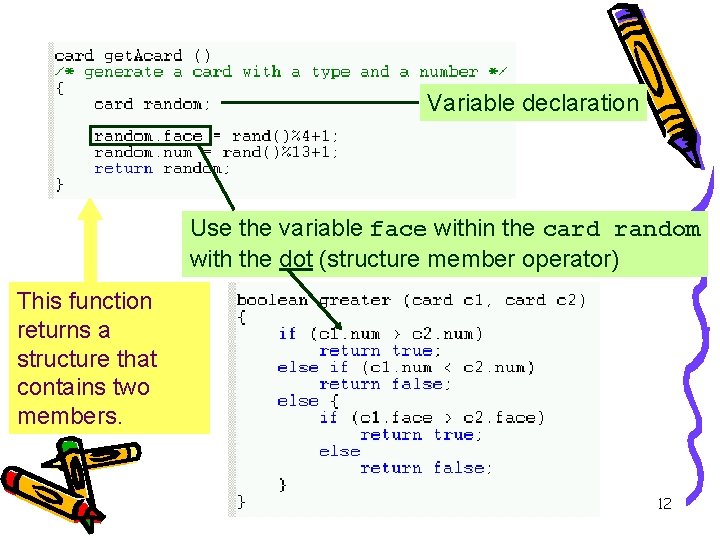
Variable declaration Use the variable face within the card random with the dot (structure member operator) This function returns a structure that contains two members. 12
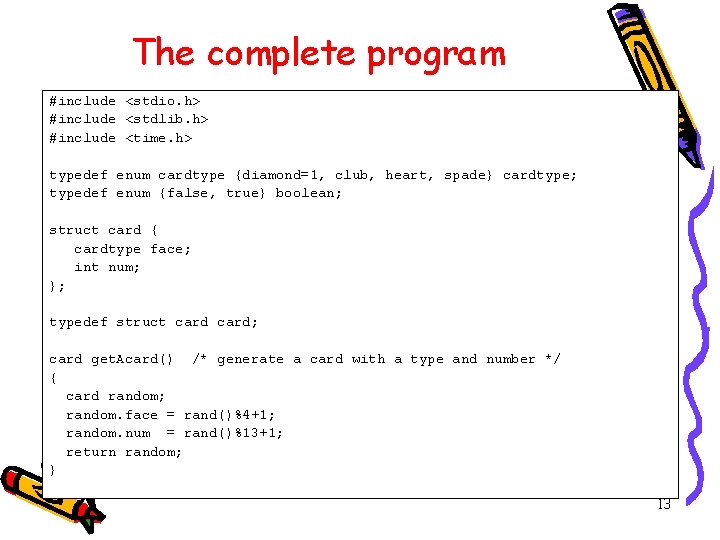
The complete program #include <stdio. h> #include <stdlib. h> #include <time. h> typedef enum cardtype {diamond=1, club, heart, spade} cardtype; typedef enum {false, true} boolean; struct card { cardtype face; int num; }; typedef struct card; card get. Acard() /* generate a card with a type and number */ { card random; random. face = rand()%4+1; random. num = rand()%13+1; return random; } 13
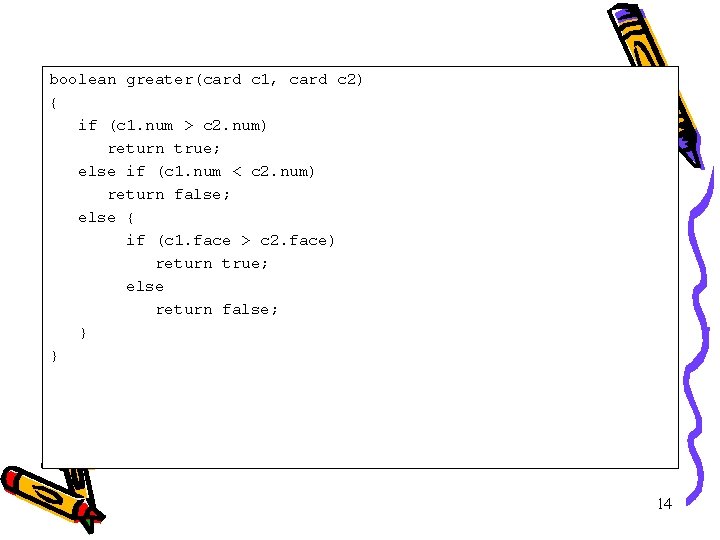
boolean greater(card c 1, card c 2) { if (c 1. num > c 2. num) return true; else if (c 1. num < c 2. num) return false; else { if (c 1. face > c 2. face) return true; else return false; } } 14
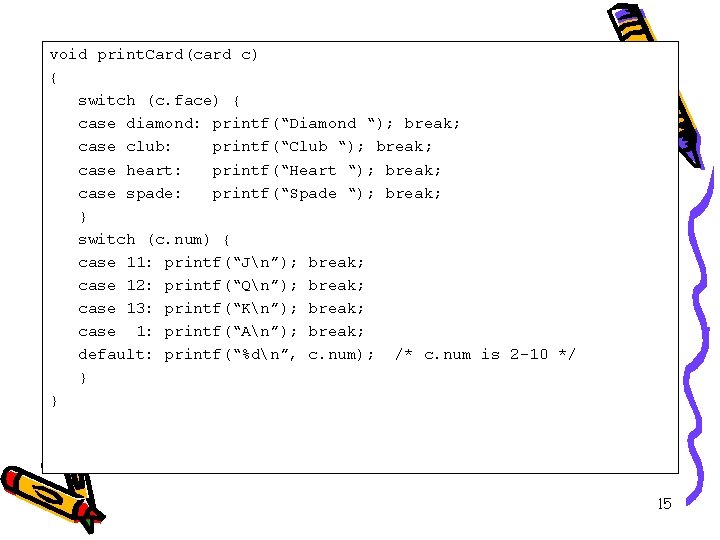
void print. Card(card c) { switch (c. face) { case diamond: printf(“Diamond “); break; case club: printf(“Club “); break; case heart: printf(“Heart “); break; case spade: printf(“Spade “); break; } switch (c. num) { case 11: printf(“Jn”); break; case 12: printf(“Qn”); break; case 13: printf(“Kn”); break; case 1: printf(“An”); break; default: printf(“%dn”, c. num); /* c. num is 2 -10 */ } } 15
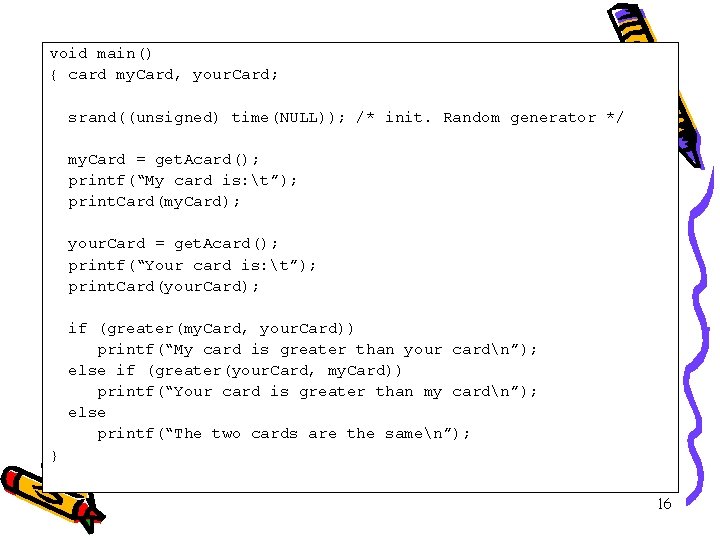
void main() { card my. Card, your. Card; srand((unsigned) time(NULL)); /* init. Random generator */ my. Card = get. Acard(); printf(“My card is: t”); print. Card(my. Card); your. Card = get. Acard(); printf(“Your card is: t”); print. Card(your. Card); if (greater(my. Card, your. Card)) printf(“My card is greater than your cardn”); else if (greater(your. Card, my. Card)) printf(“Your card is greater than my cardn”); else printf(“The two cards are the samen”); } 16
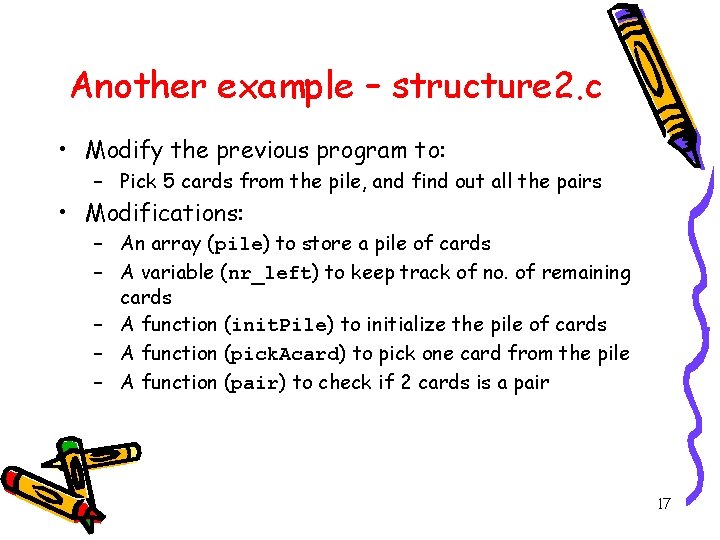
Another example – structure 2. c • Modify the previous program to: – Pick 5 cards from the pile, and find out all the pairs • Modifications: – An array (pile) to store a pile of cards – A variable (nr_left) to keep track of no. of remaining cards – A function (init. Pile) to initialize the pile of cards – A function (pick. Acard) to pick one card from the pile – A function (pair) to check if 2 cards is a pair 17
![card pile52 int nrleft void init Pile int i for i0 i52 i card pile[52]; int nr_left; void init. Pile() { int i; for (i=0; i<52; i++)](https://slidetodoc.com/presentation_image_h2/f0355f54f2da5f67b39cadcc3b918b64/image-18.jpg)
card pile[52]; int nr_left; void init. Pile() { int i; for (i=0; i<52; i++) { pile[i]. face = (i/13)+1; pile[i]. num = (i%13)+1; } nr_left=52; } card pick. Acard() { card random; int i; i = rand()%nr_left; random = pile[i]; pile[i] = pile[nr_left-1]; nr_left--; return random; } 18
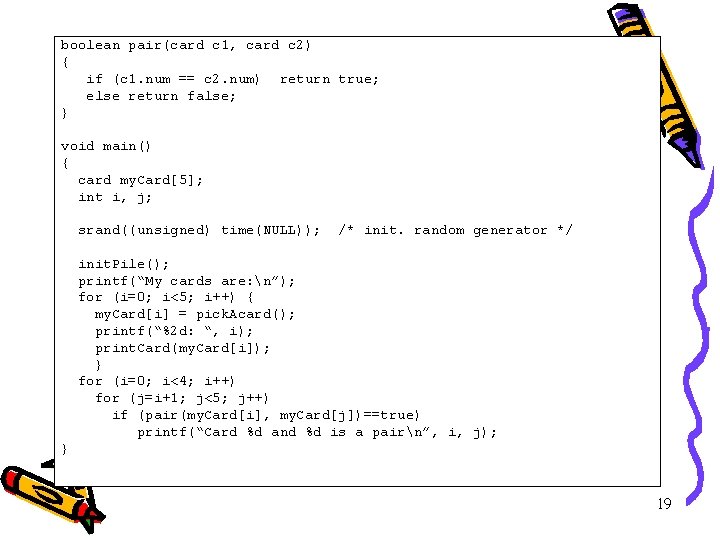
boolean pair(card c 1, card c 2) { if (c 1. num == c 2. num) return true; else return false; } void main() { card my. Card[5]; int i, j; srand((unsigned) time(NULL)); /* init. random generator */ init. Pile(); printf(“My cards are: n”); for (i=0; i<5; i++) { my. Card[i] = pick. Acard(); printf(“%2 d: “, i); print. Card(my. Card[i]); } for (i=0; i<4; i++) for (j=i+1; j<5; j++) if (pair(my. Card[i], my. Card[j])==true) printf(“Card %d and %d is a pairn”, i, j); } 19
Example of enumeration
What is enumeration text type
Influences on language design
Enumeration text type
Example of enumeration text type
Logos definition
Feelings of hatred towards somebody
Organizational text features
Enumeration/listing signal words
Chronological signal words
01:640:244 lecture notes - lecture 15: plat, idah, farad
Name type compatibility and structure type compatibility
Cve common vulnerability enumeration
Common configuration enumeration
Post enumeration survey
Spatial occupancy enumeration in cad
Spatial occupancy enumeration
Enumeration transition words
Inference by enumeration in artificial intelligence
Inference by enumeration in artificial intelligence