Lecture 09 Applets Introduction to Applets l l
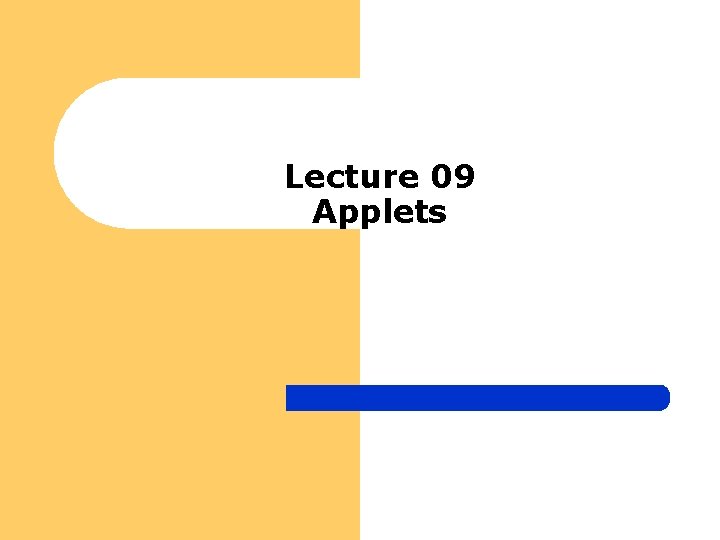
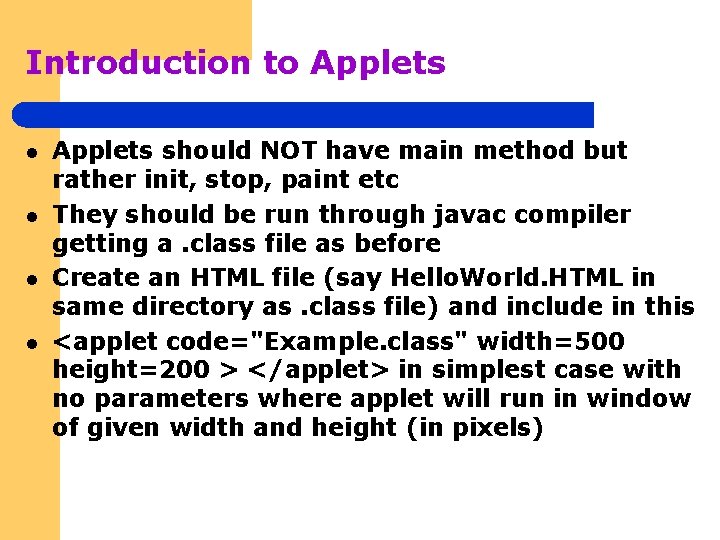
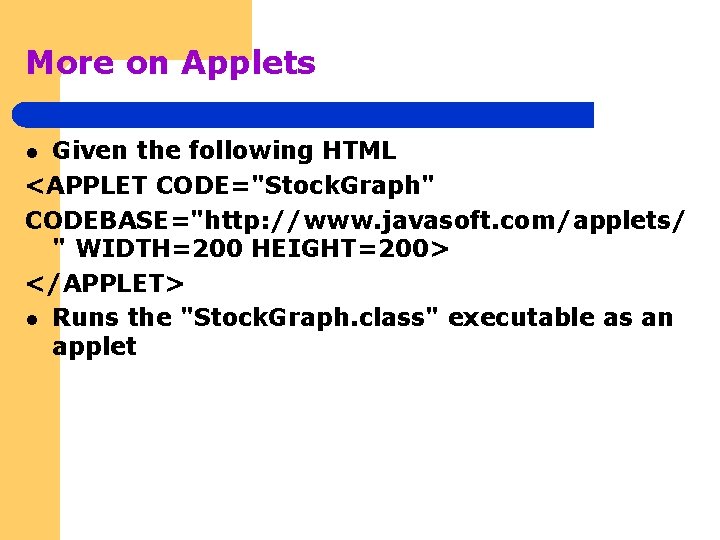
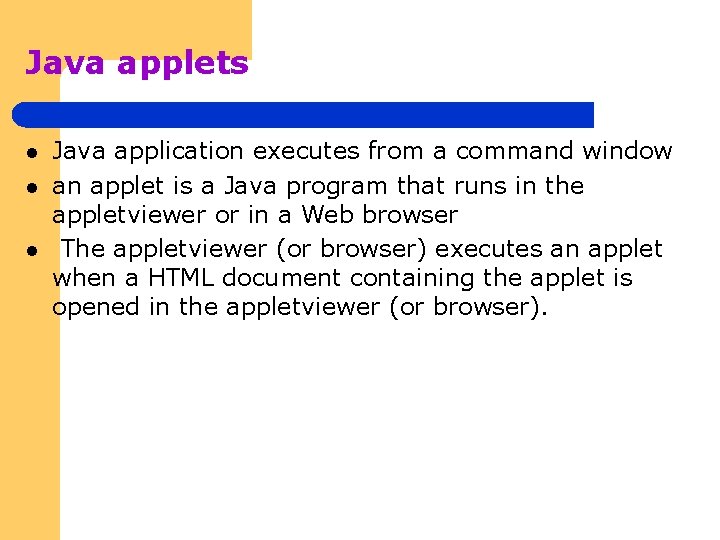
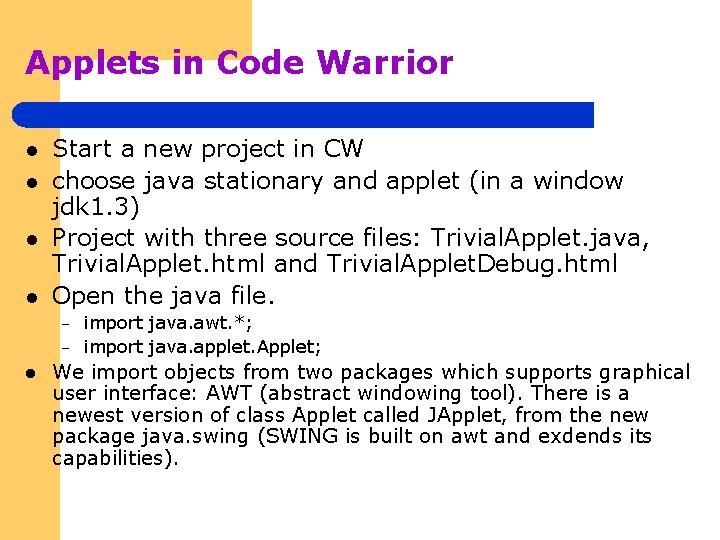
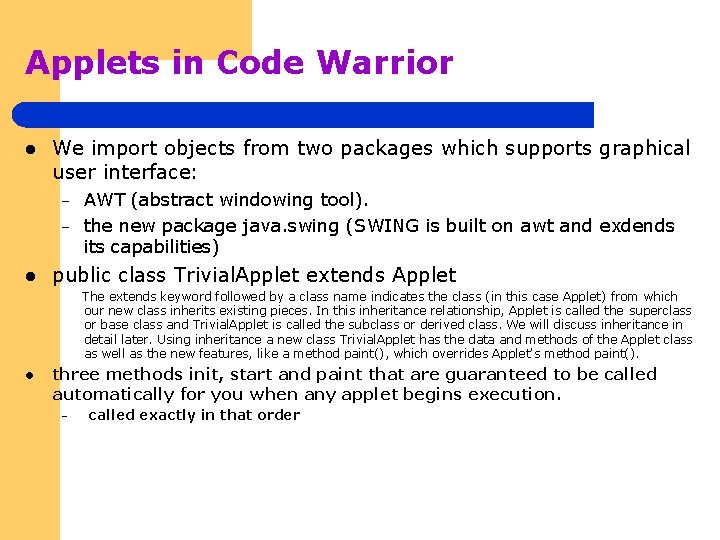
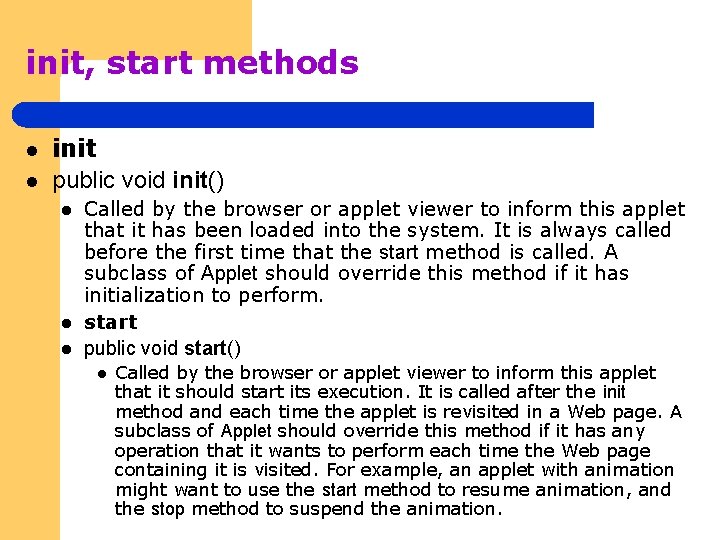
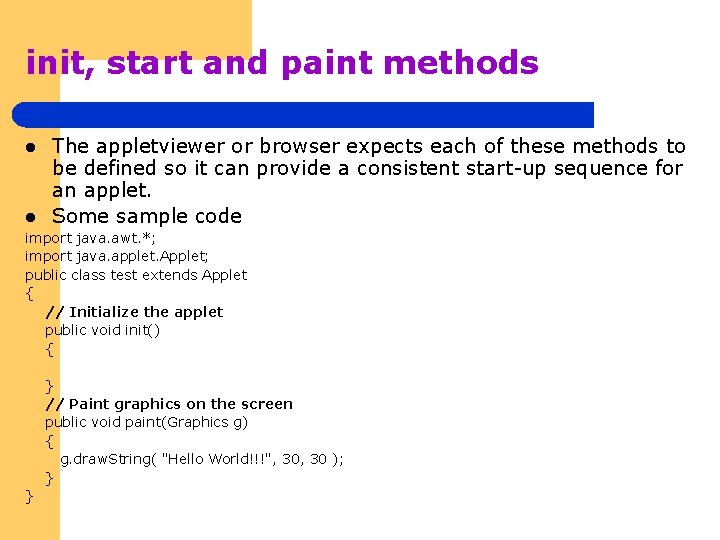
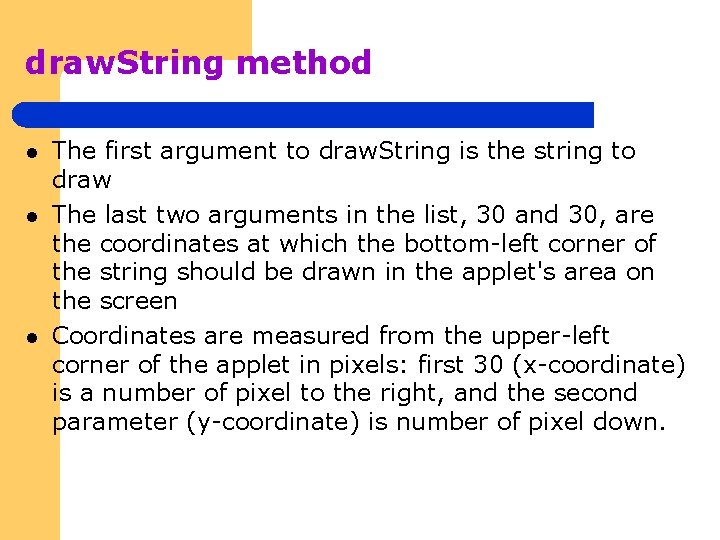
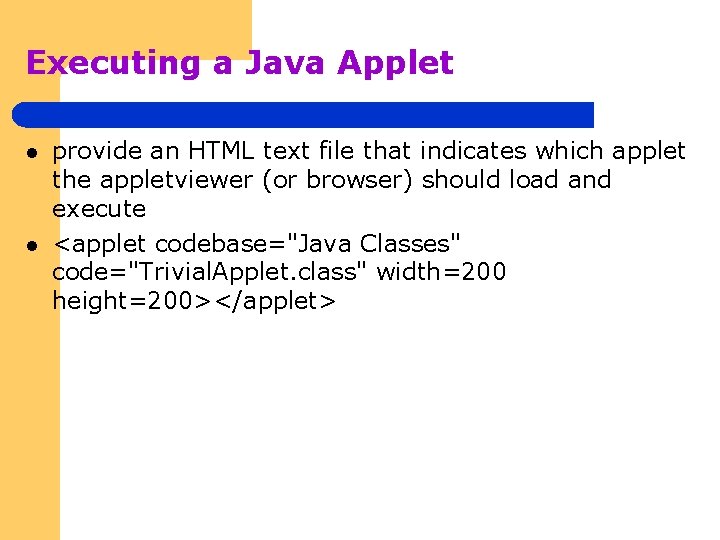
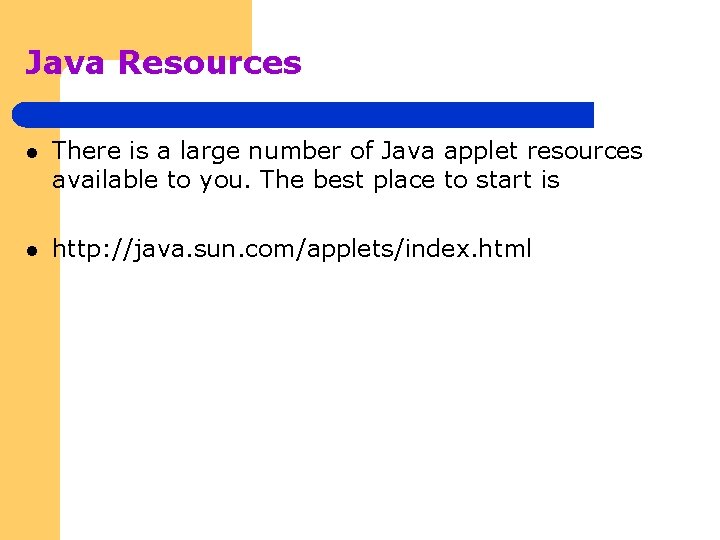
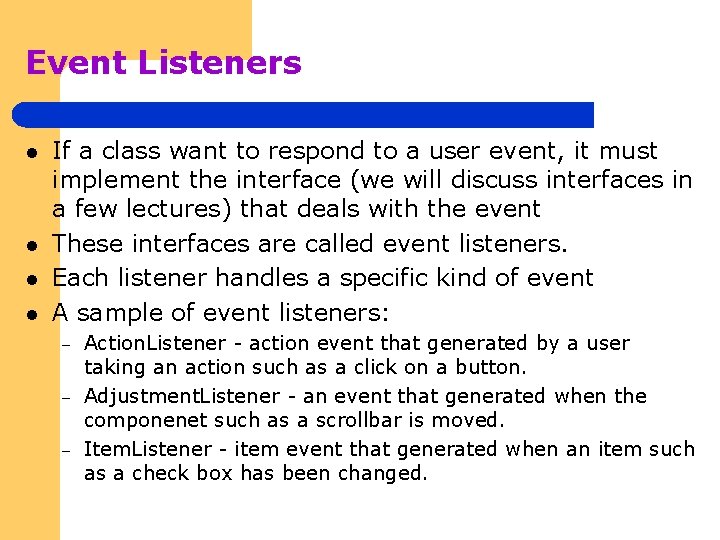
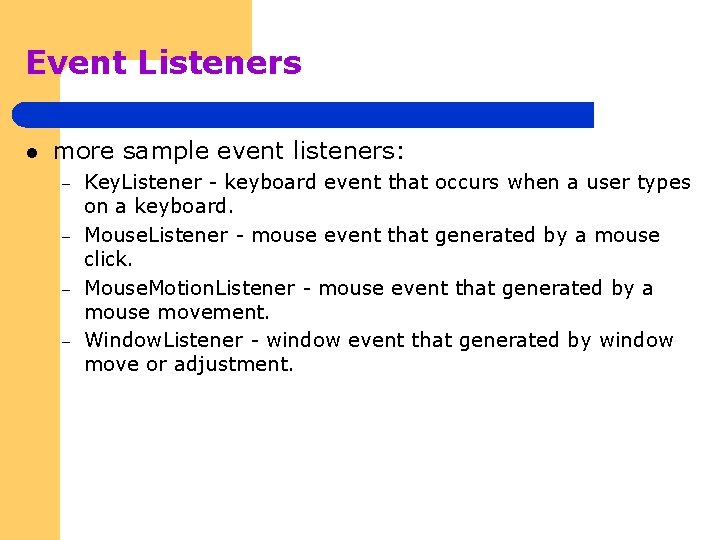
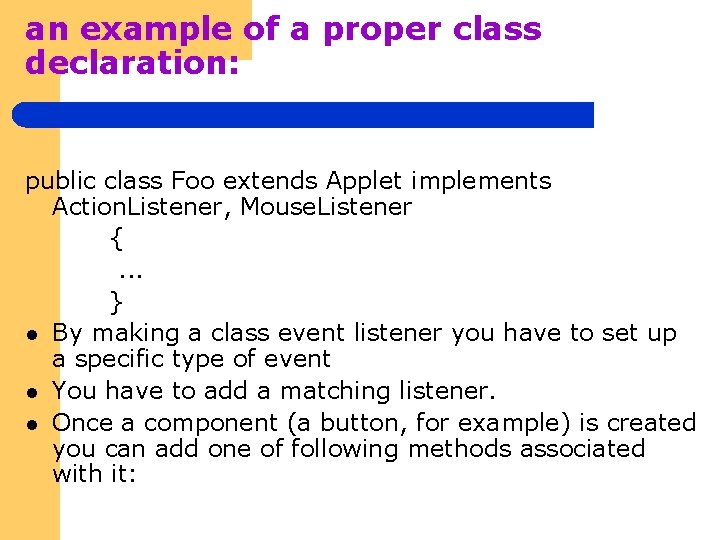
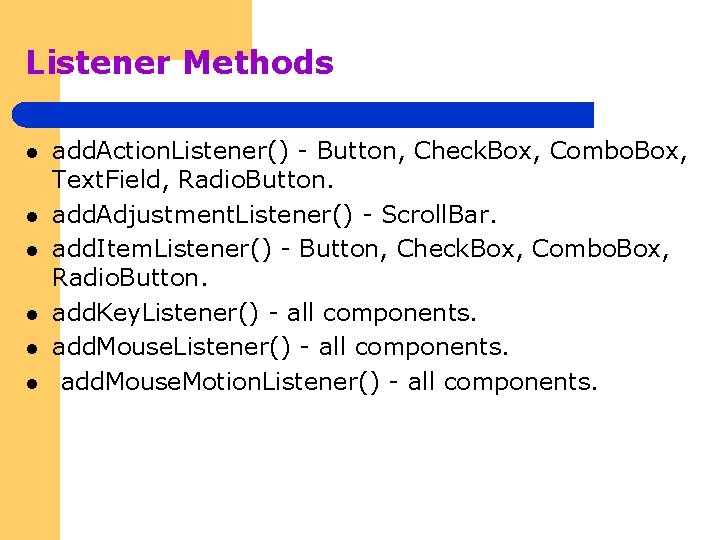
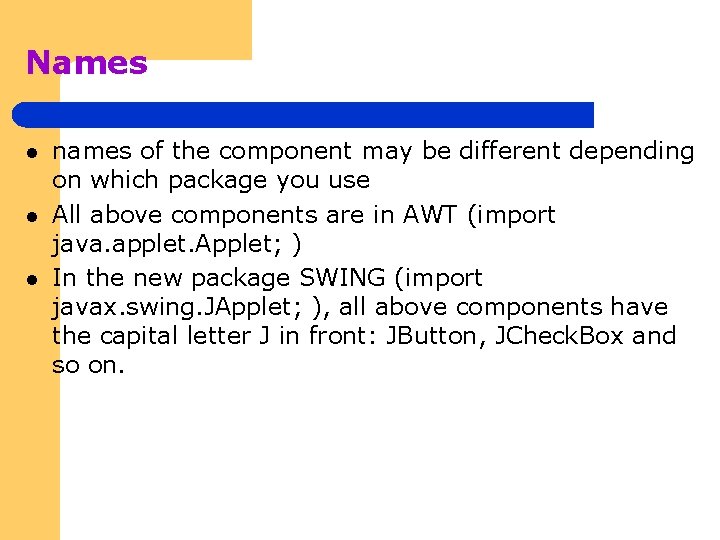
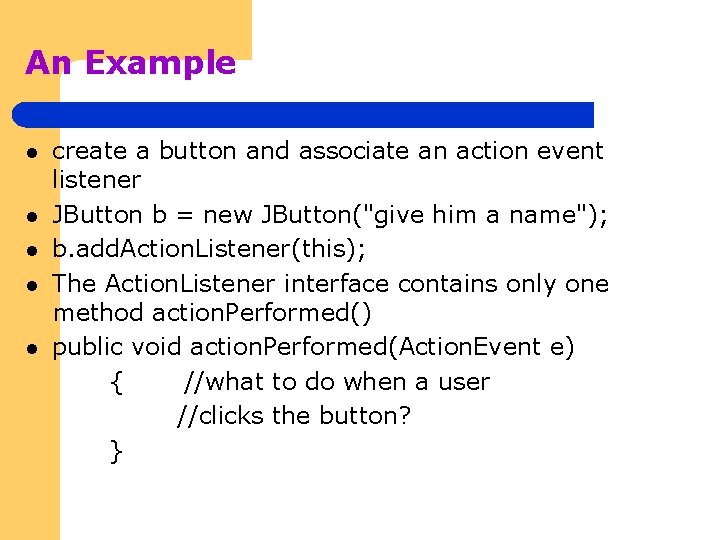
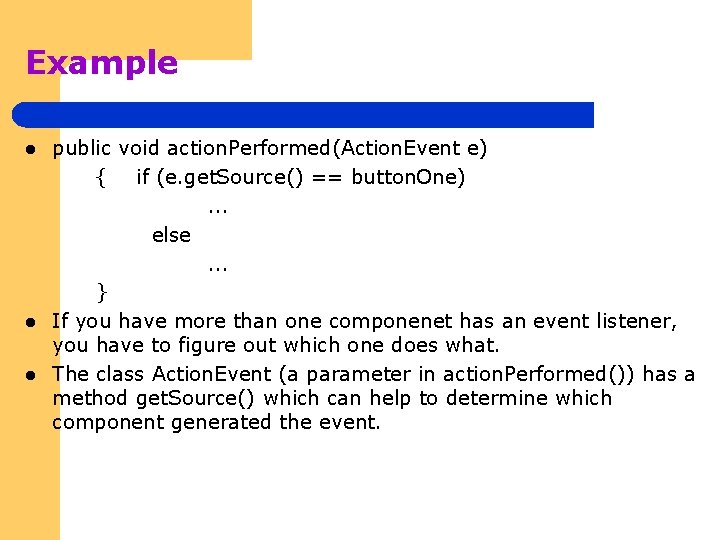
- Slides: 18
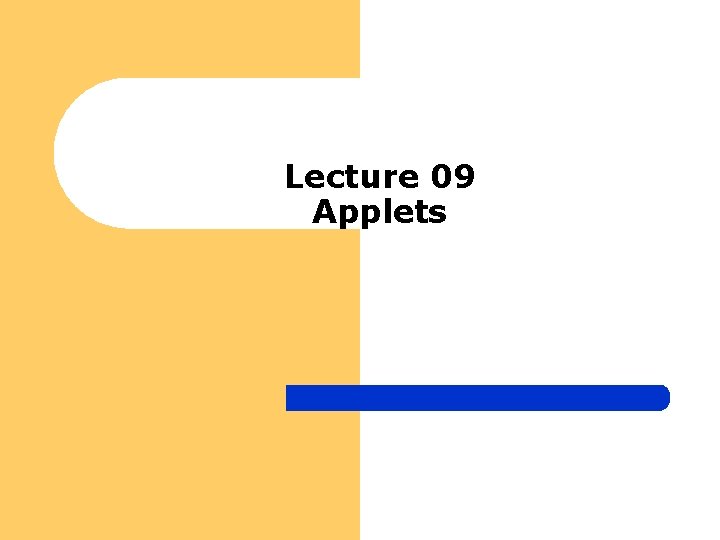
Lecture 09 Applets
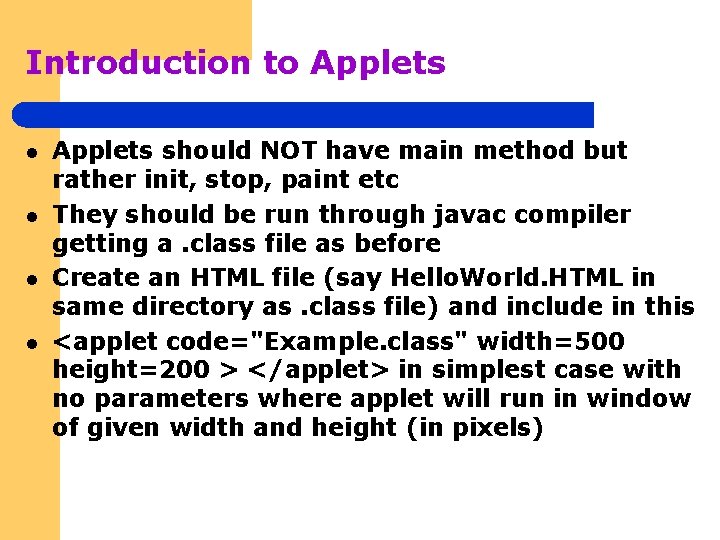
Introduction to Applets l l Applets should NOT have main method but rather init, stop, paint etc They should be run through javac compiler getting a. class file as before Create an HTML file (say Hello. World. HTML in same directory as. class file) and include in this <applet code="Example. class" width=500 height=200 > </applet> in simplest case with no parameters where applet will run in window of given width and height (in pixels)
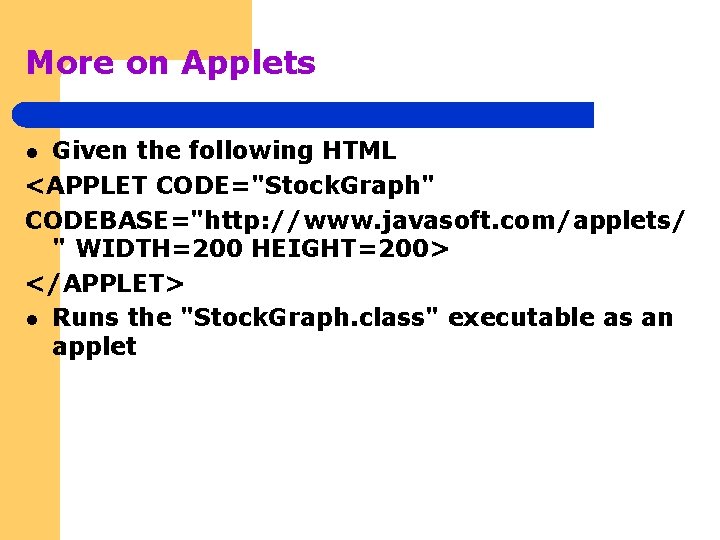
More on Applets Given the following HTML <APPLET CODE="Stock. Graph" CODEBASE="http: //www. javasoft. com/applets/ " WIDTH=200 HEIGHT=200> </APPLET> l Runs the "Stock. Graph. class" executable as an applet l
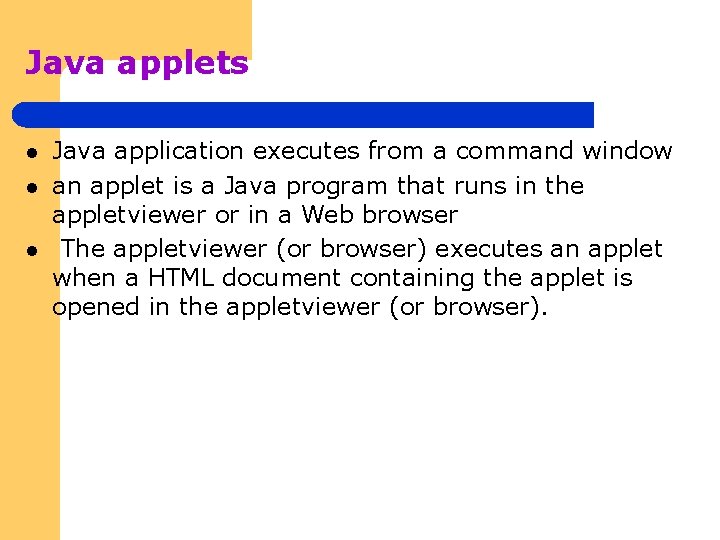
Java applets l l l Java application executes from a command window an applet is a Java program that runs in the appletviewer or in a Web browser The appletviewer (or browser) executes an applet when a HTML document containing the applet is opened in the appletviewer (or browser).
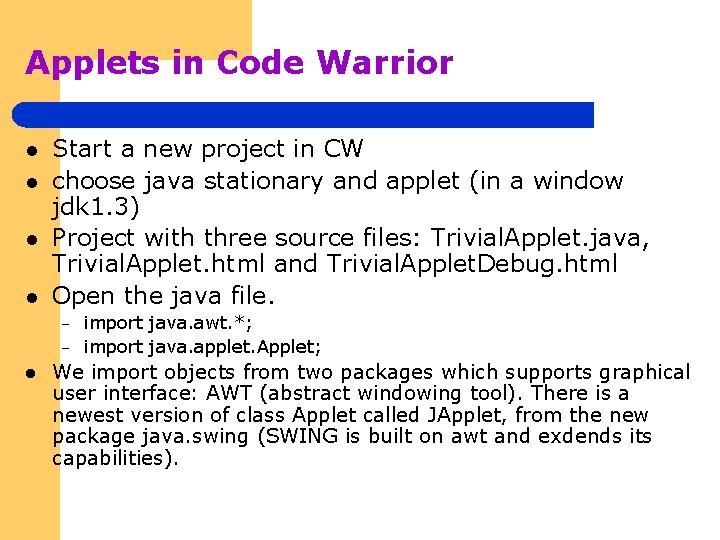
Applets in Code Warrior l l Start a new project in CW choose java stationary and applet (in a window jdk 1. 3) Project with three source files: Trivial. Applet. java, Trivial. Applet. html and Trivial. Applet. Debug. html Open the java file. – – l import java. awt. *; import java. applet. Applet; We import objects from two packages which supports graphical user interface: AWT (abstract windowing tool). There is a newest version of class Applet called JApplet, from the new package java. swing (SWING is built on awt and exdends its capabilities).
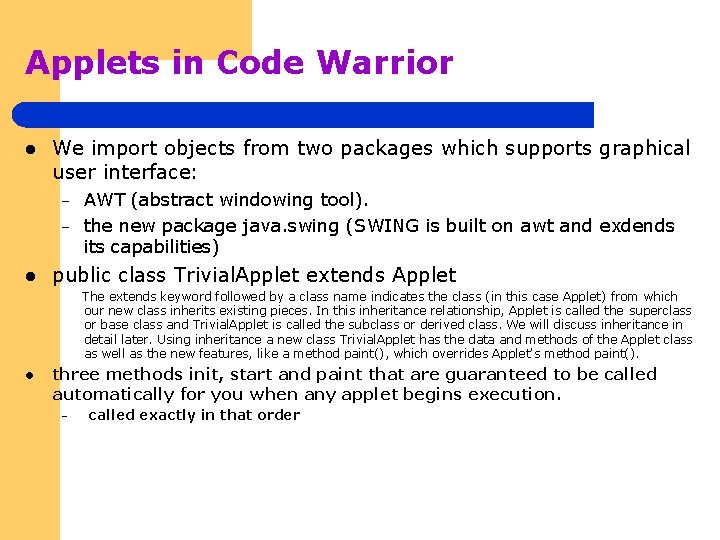
Applets in Code Warrior l We import objects from two packages which supports graphical user interface: – – l AWT (abstract windowing tool). the new package java. swing (SWING is built on awt and exdends its capabilities) public class Trivial. Applet extends Applet The extends keyword followed by a class name indicates the class (in this case Applet) from which our new class inherits existing pieces. In this inheritance relationship, Applet is called the superclass or base class and Trivial. Applet is called the subclass or derived class. We will discuss inheritance in detail later. Using inheritance a new class Trivial. Applet has the data and methods of the Applet class as well as the new features, like a method paint(), which overrides Applet's method paint(). l three methods init, start and paint that are guaranteed to be called automatically for you when any applet begins execution. – called exactly in that order
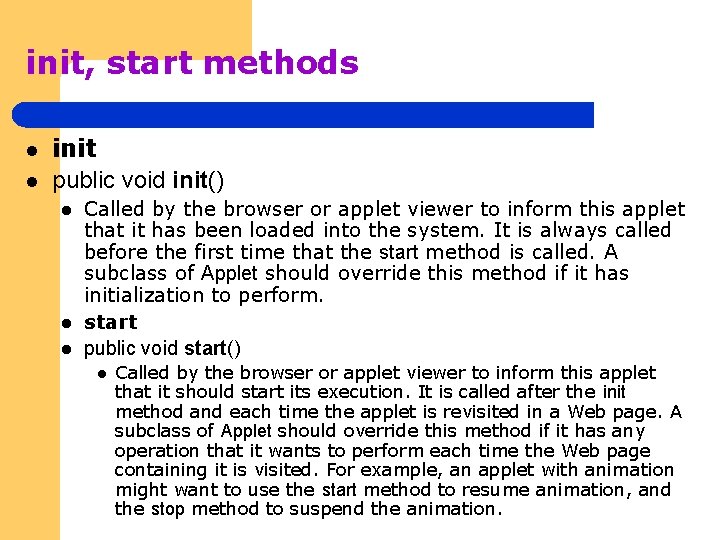
init, start methods l l init public void init() l l l Called by the browser or applet viewer to inform this applet that it has been loaded into the system. It is always called before the first time that the start method is called. A subclass of Applet should override this method if it has initialization to perform. start public void start() l Called by the browser or applet viewer to inform this applet that it should start its execution. It is called after the init method and each time the applet is revisited in a Web page. A subclass of Applet should override this method if it has any operation that it wants to perform each time the Web page containing it is visited. For example, an applet with animation might want to use the start method to resume animation, and the stop method to suspend the animation.
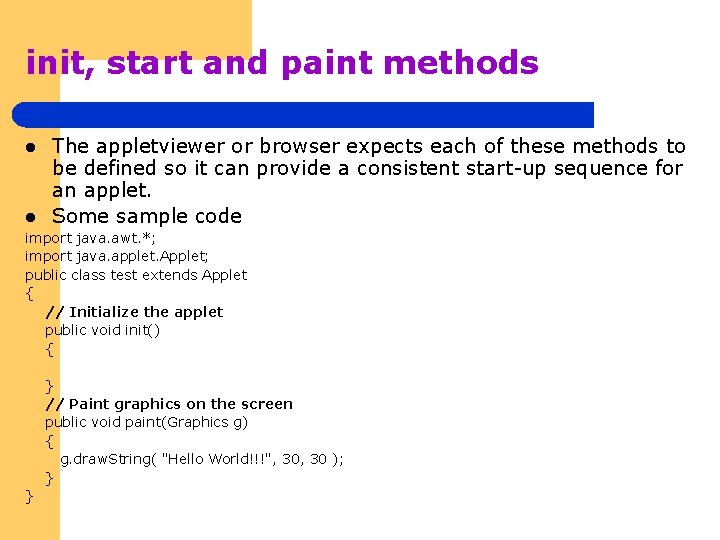
init, start and paint methods l l The appletviewer or browser expects each of these methods to be defined so it can provide a consistent start-up sequence for an applet. Some sample code import java. awt. *; import java. applet. Applet; public class test extends Applet { // Initialize the applet public void init() { } // Paint graphics on the screen public void paint(Graphics g) { g. draw. String( "Hello World!!!", 30 ); } }
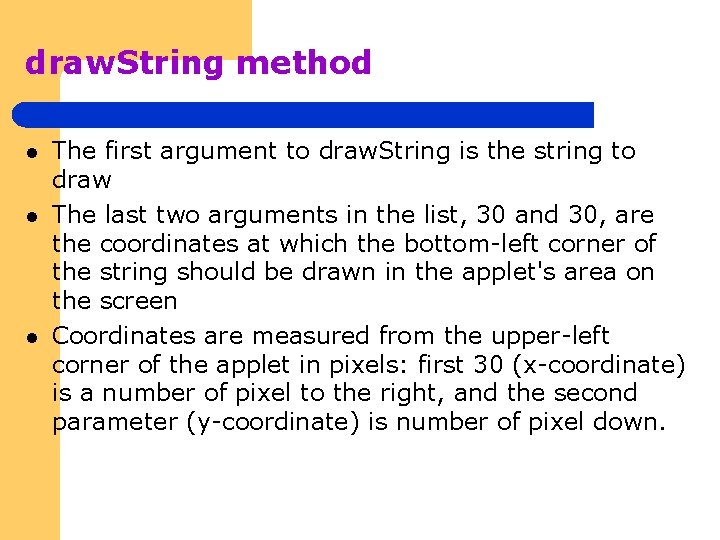
draw. String method l l l The first argument to draw. String is the string to draw The last two arguments in the list, 30 and 30, are the coordinates at which the bottom-left corner of the string should be drawn in the applet's area on the screen Coordinates are measured from the upper-left corner of the applet in pixels: first 30 (x-coordinate) is a number of pixel to the right, and the second parameter (y-coordinate) is number of pixel down.
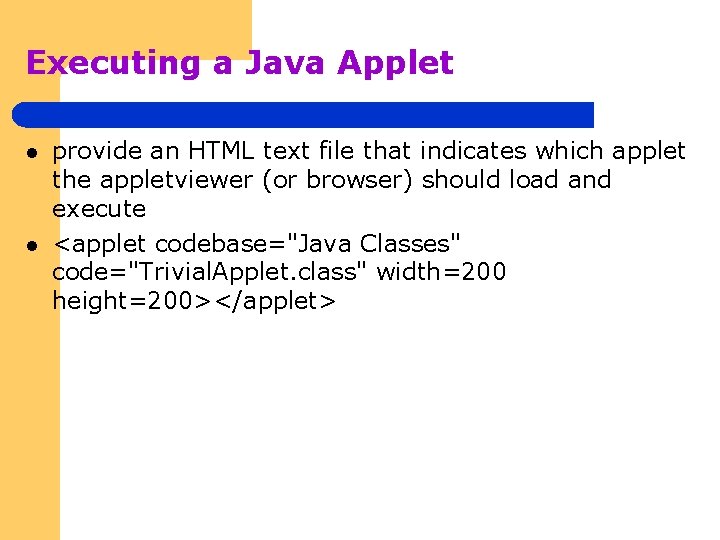
Executing a Java Applet l l provide an HTML text file that indicates which applet the appletviewer (or browser) should load and execute <applet codebase="Java Classes" code="Trivial. Applet. class" width=200 height=200></applet>
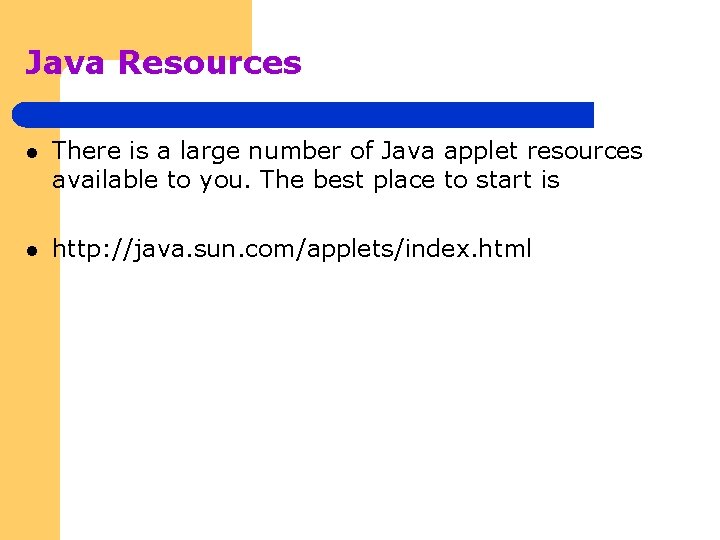
Java Resources l There is a large number of Java applet resources available to you. The best place to start is l http: //java. sun. com/applets/index. html
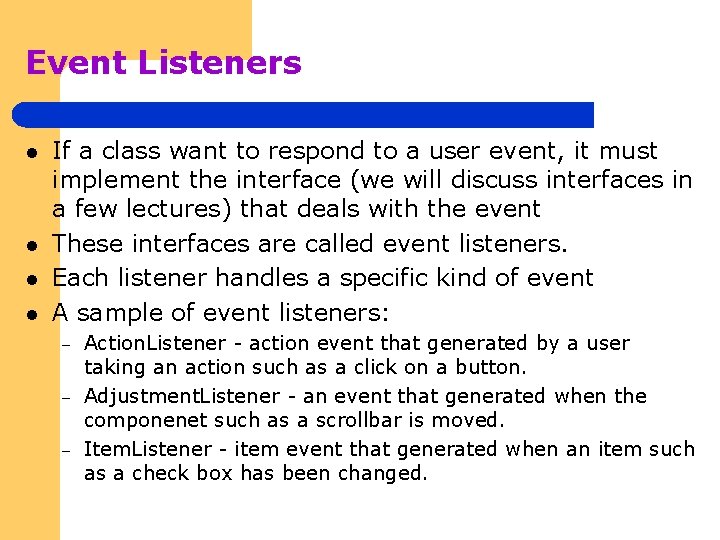
Event Listeners l l If a class want to respond to a user event, it must implement the interface (we will discuss interfaces in a few lectures) that deals with the event These interfaces are called event listeners. Each listener handles a specific kind of event A sample of event listeners: – – – Action. Listener - action event that generated by a user taking an action such as a click on a button. Adjustment. Listener - an event that generated when the componenet such as a scrollbar is moved. Item. Listener - item event that generated when an item such as a check box has been changed.
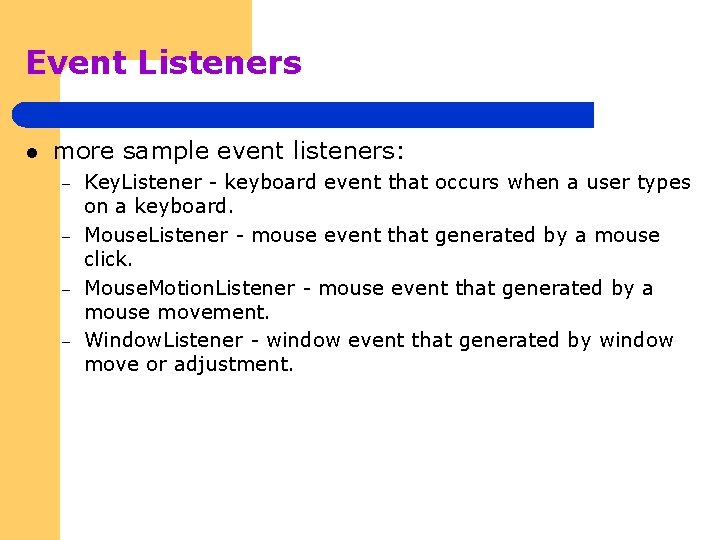
Event Listeners l more sample event listeners: – – Key. Listener - keyboard event that occurs when a user types on a keyboard. Mouse. Listener - mouse event that generated by a mouse click. Mouse. Motion. Listener - mouse event that generated by a mouse movement. Window. Listener - window event that generated by window move or adjustment.
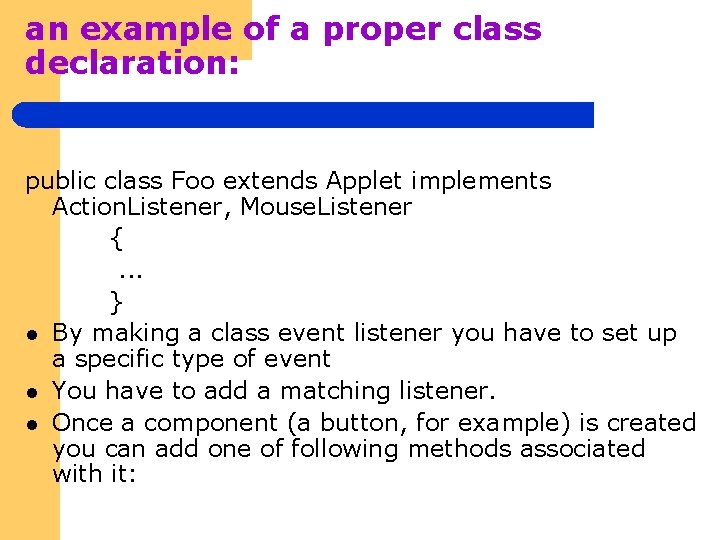
an example of a proper class declaration: public class Foo extends Applet implements Action. Listener, Mouse. Listener {. . . } l By making a class event listener you have to set up a specific type of event l You have to add a matching listener. l Once a component (a button, for example) is created you can add one of following methods associated with it:
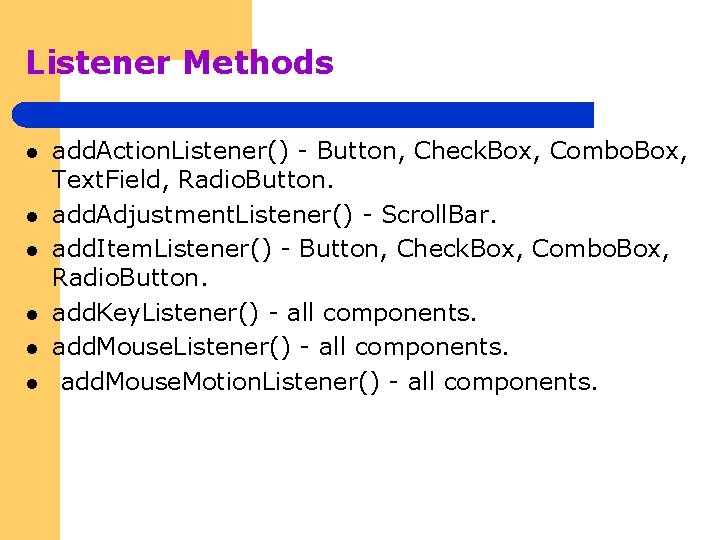
Listener Methods l l l add. Action. Listener() - Button, Check. Box, Combo. Box, Text. Field, Radio. Button. add. Adjustment. Listener() - Scroll. Bar. add. Item. Listener() - Button, Check. Box, Combo. Box, Radio. Button. add. Key. Listener() - all components. add. Mouse. Motion. Listener() - all components.
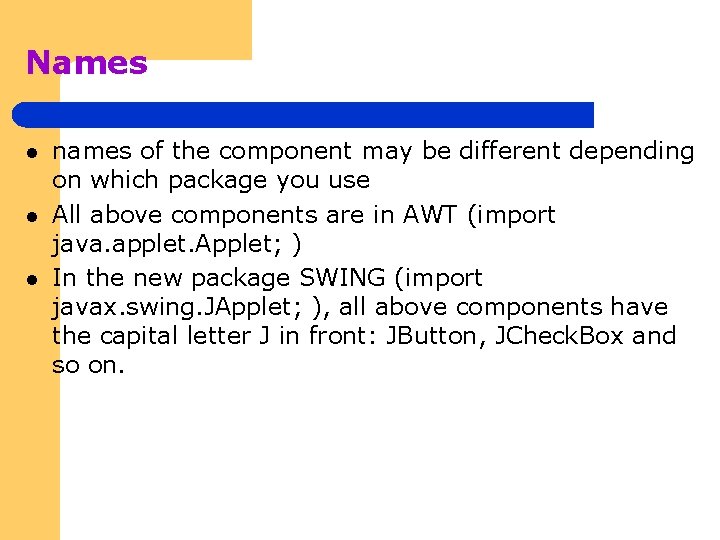
Names l l l names of the component may be different depending on which package you use All above components are in AWT (import java. applet. Applet; ) In the new package SWING (import javax. swing. JApplet; ), all above components have the capital letter J in front: JButton, JCheck. Box and so on.
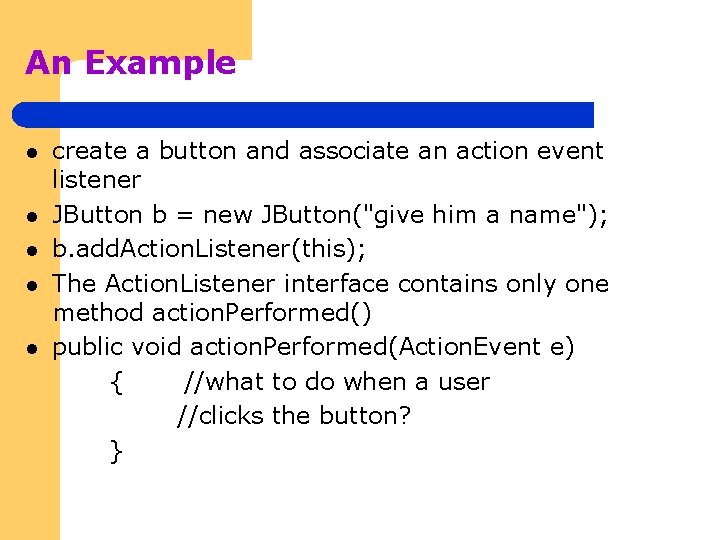
An Example l l l create a button and associate an action event listener JButton b = new JButton("give him a name"); b. add. Action. Listener(this); The Action. Listener interface contains only one method action. Performed() public void action. Performed(Action. Event e) { //what to do when a user //clicks the button? }
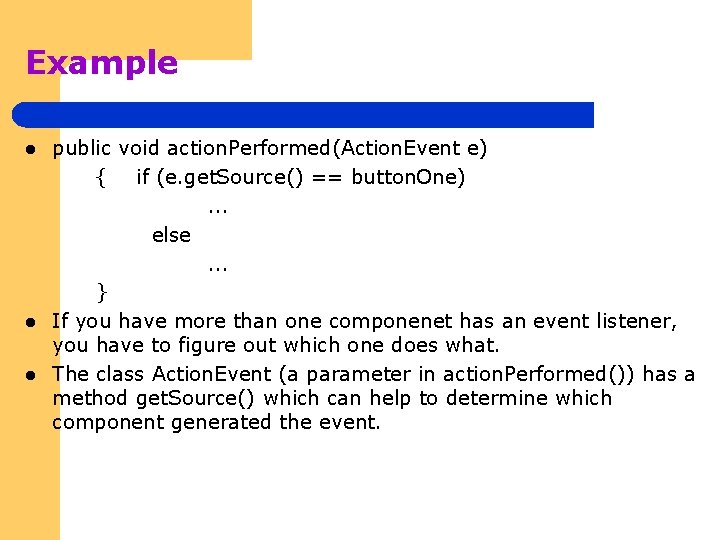
Example l l l public void action. Performed(Action. Event e) { if (e. get. Source() == button. One). . . else. . . } If you have more than one componenet has an event listener, you have to figure out which one does what. The class Action. Event (a parameter in action. Performed()) has a method get. Source() which can help to determine which component generated the event.
Wisweb applets
Bouwgeschillen oplossen
Reese's pieces simulation
Erik poll
01:640:244 lecture notes - lecture 15: plat, idah, farad
Introduction to biochemistry lecture notes
Introduction to psychology lecture
Introduction to algorithms lecture notes
Body paragraph
Project procurement management lecture notes
Lecture about sport
Healthy lifestyle wrap up lecture
Whats nihilism
Meaning of this
Randy pausch the last lecture summary
Tensorflow lecture
Theology proper lecture notes
Strategic management lecture
Geology lecture series