JAVA SWINGS WHAT IS SWINGS Swing is a
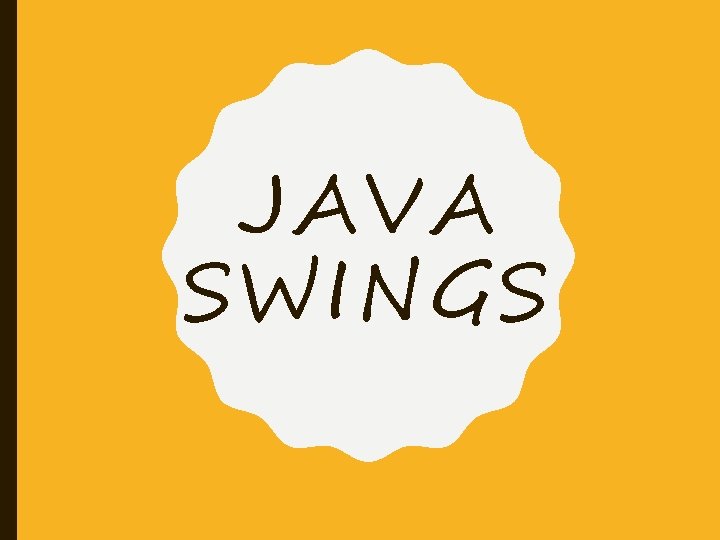
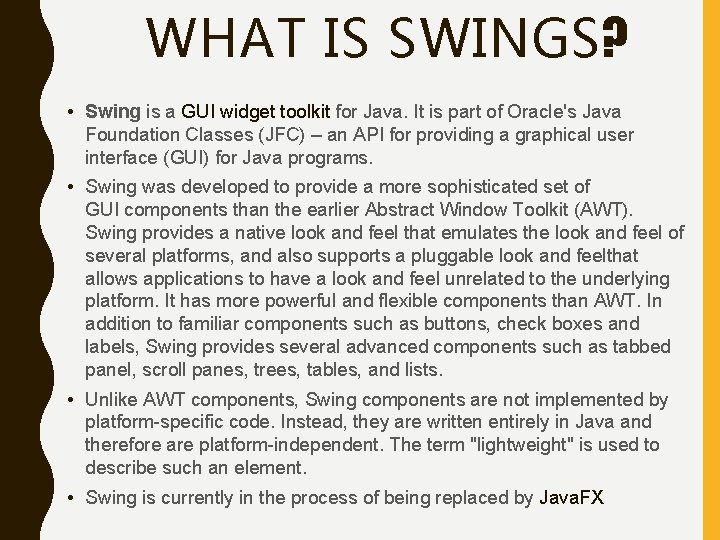
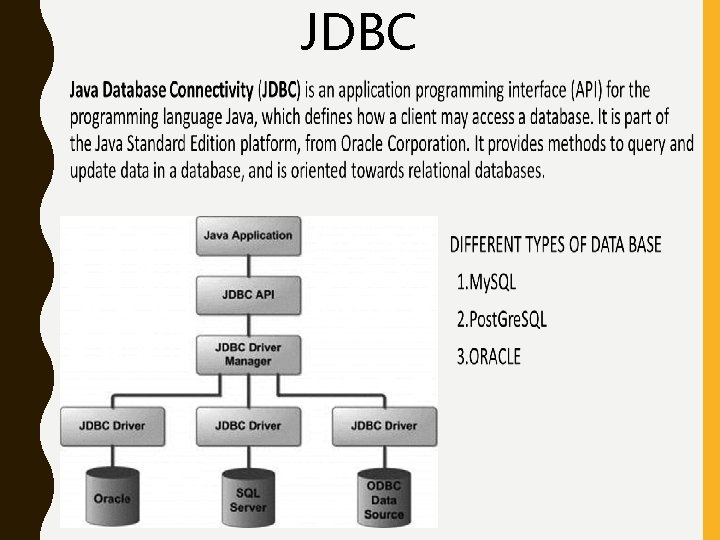
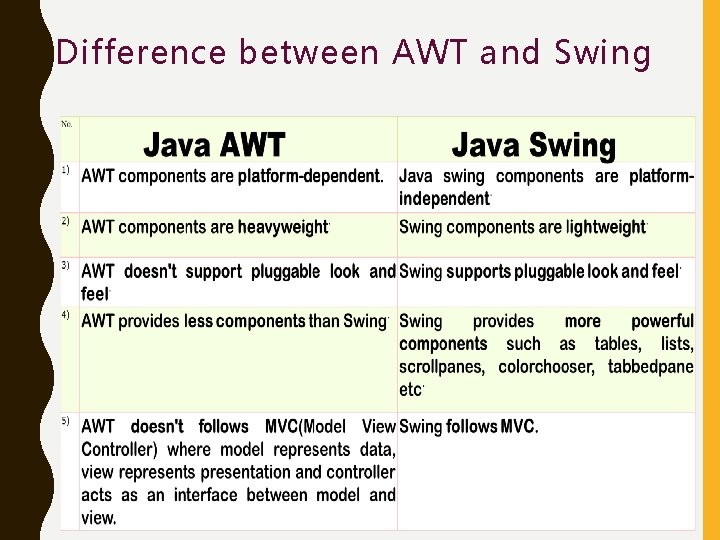
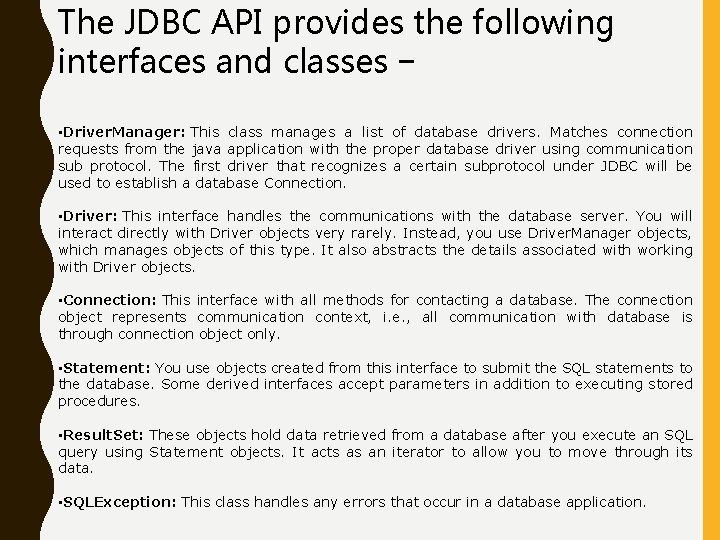
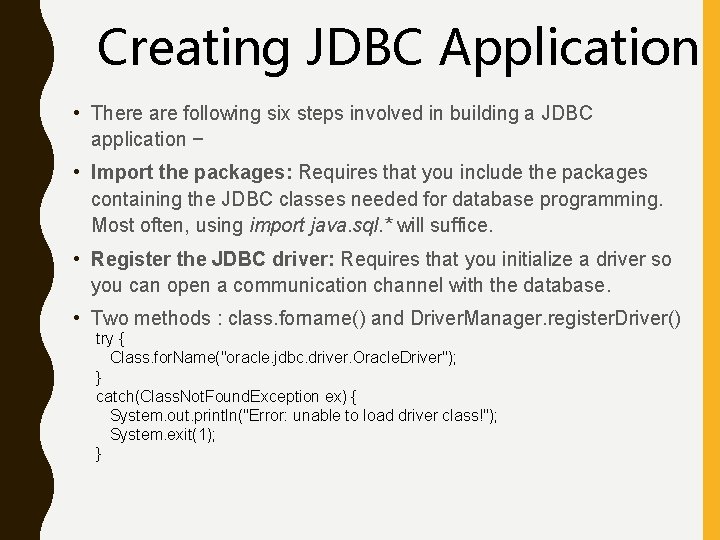
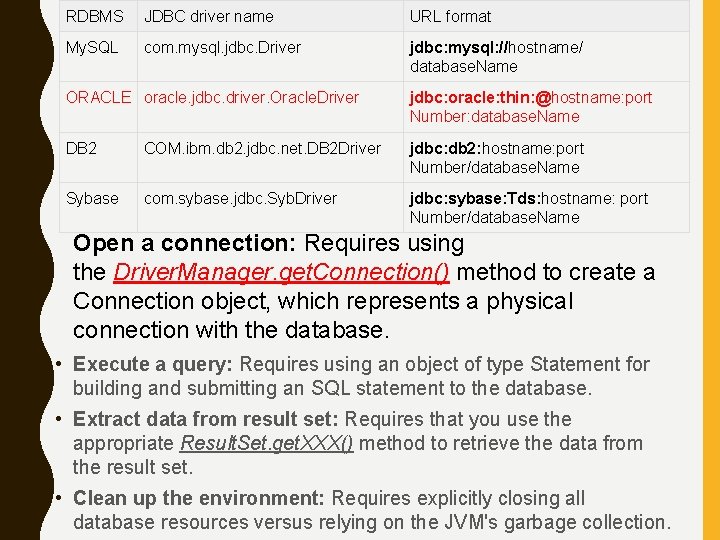
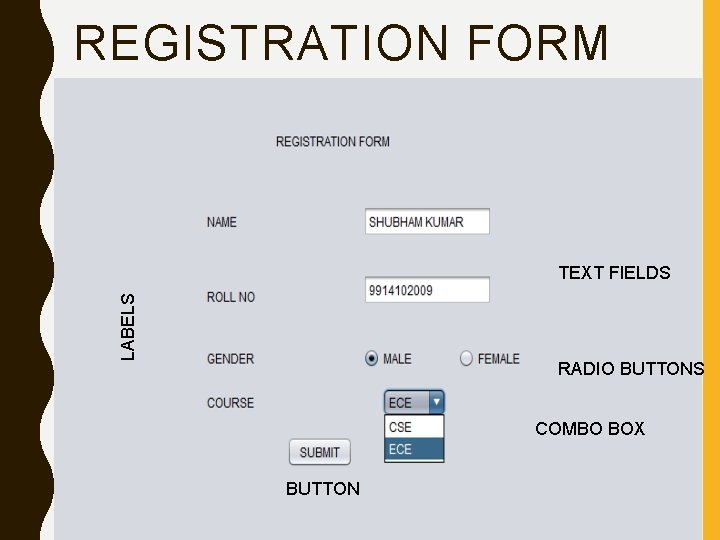
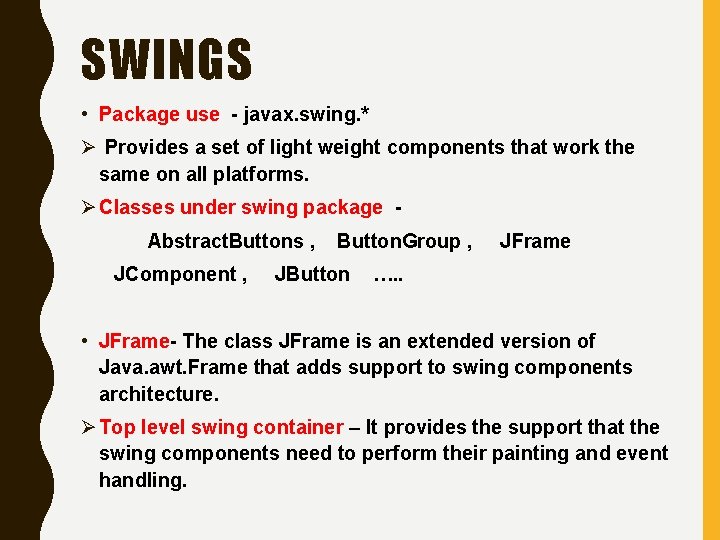
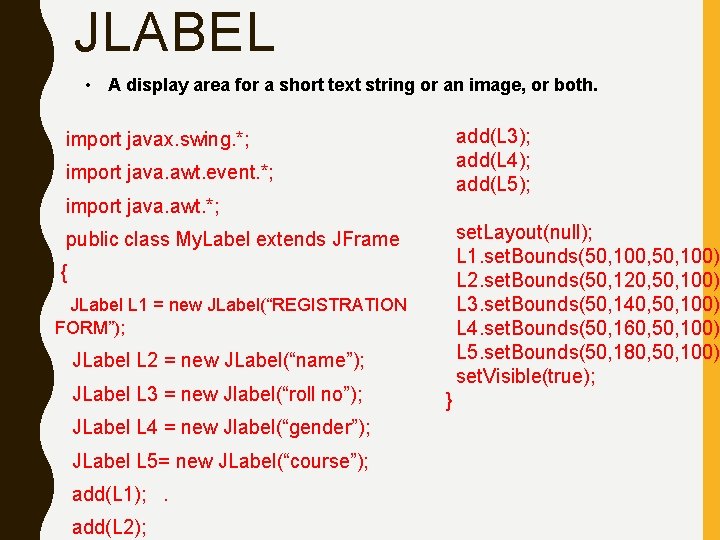
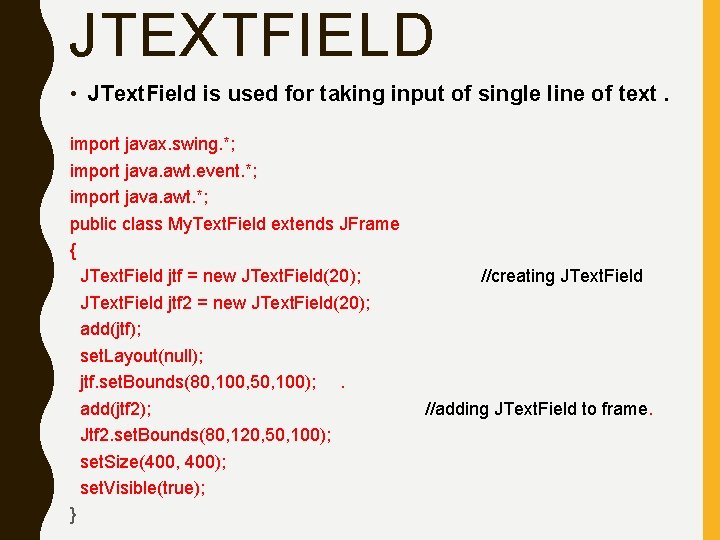
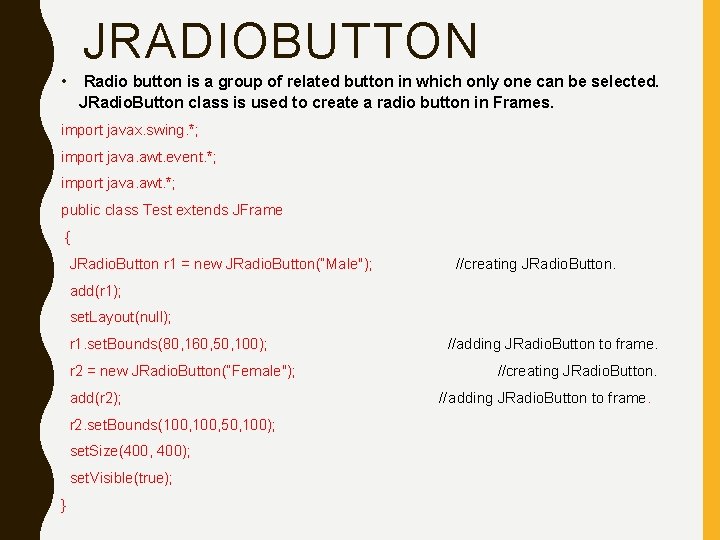
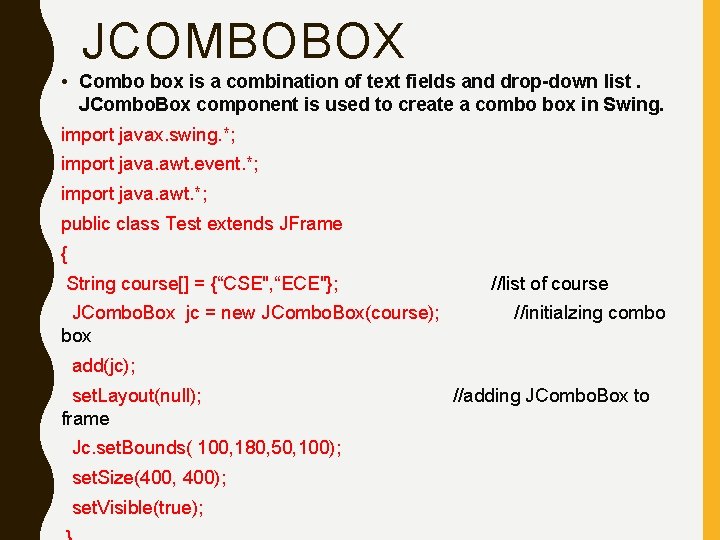
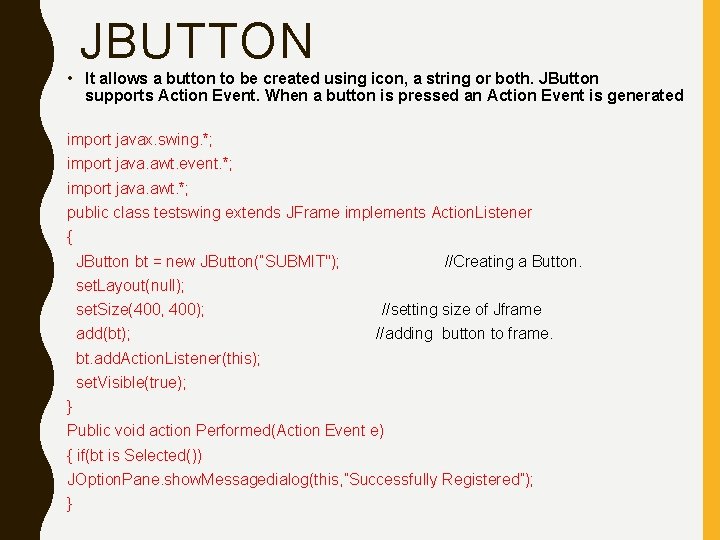
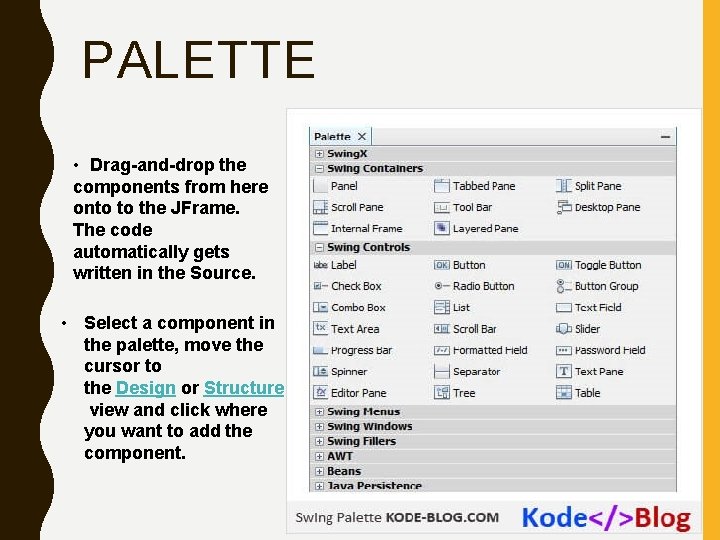
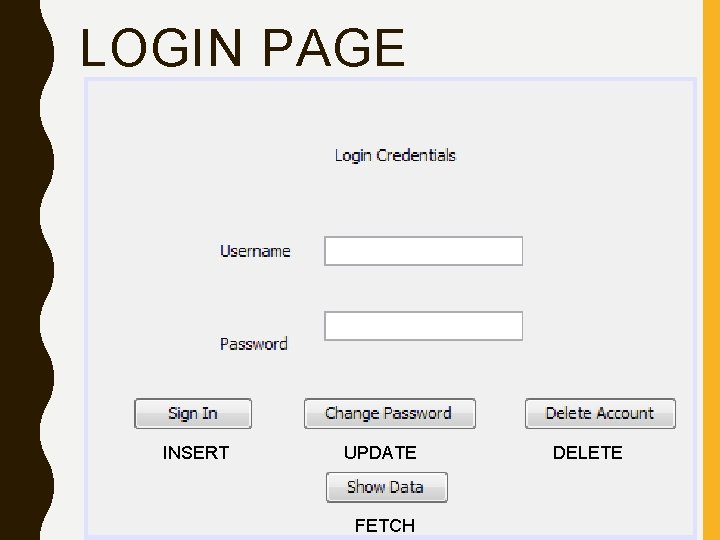
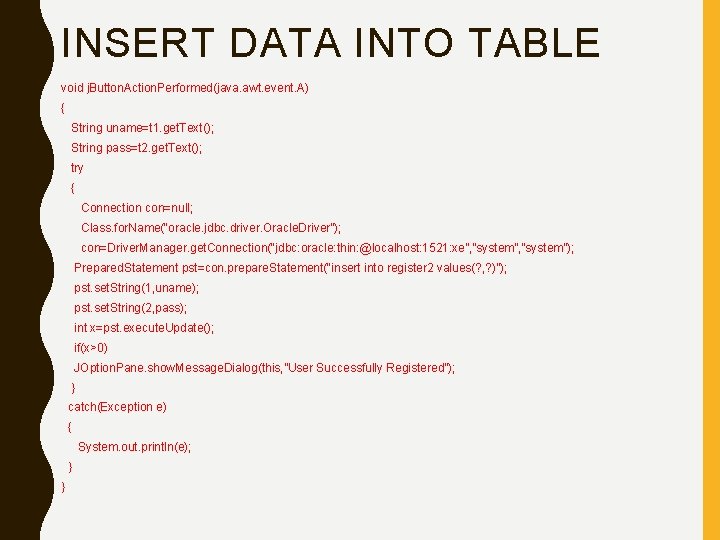
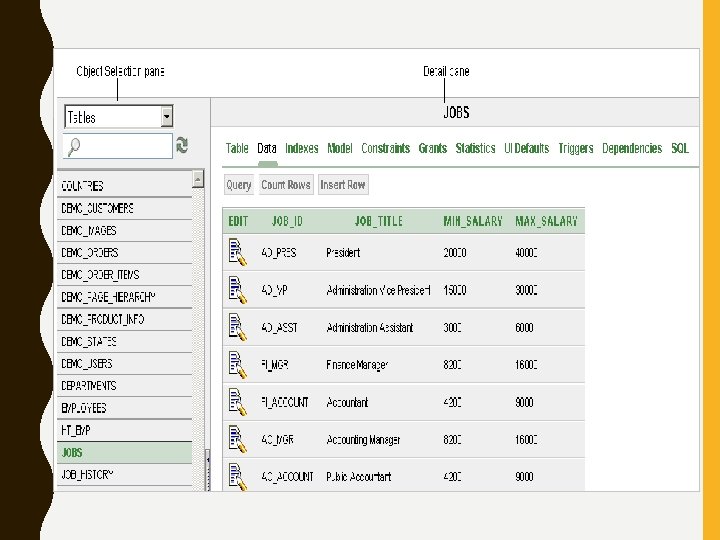
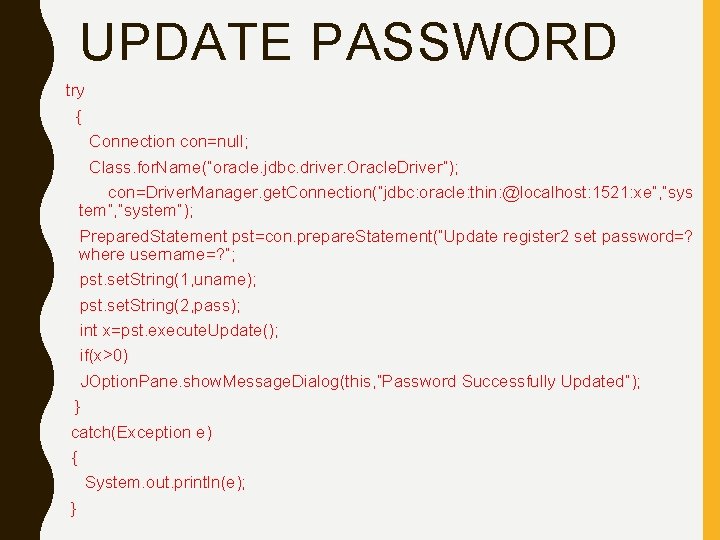
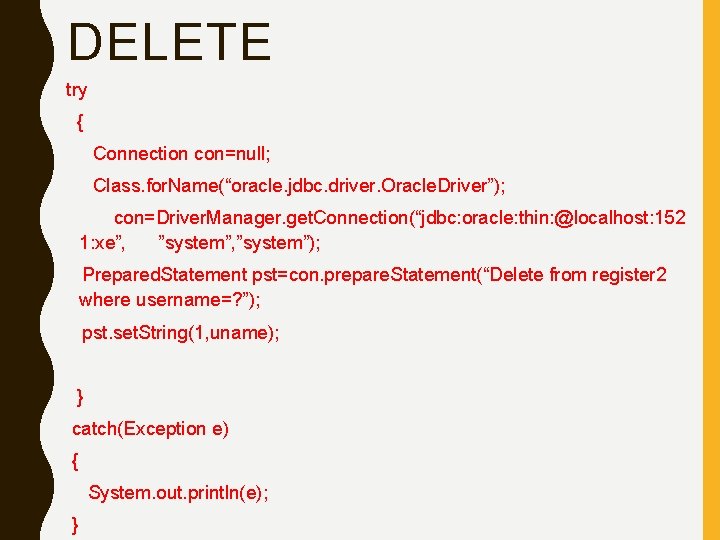
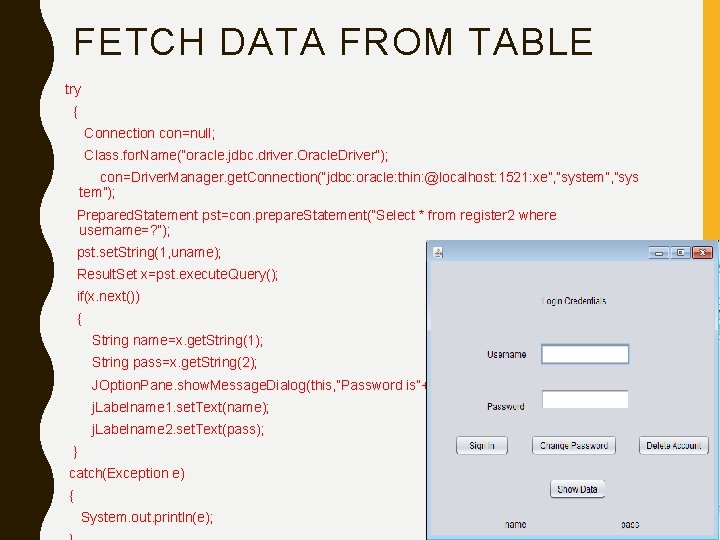
- Slides: 21
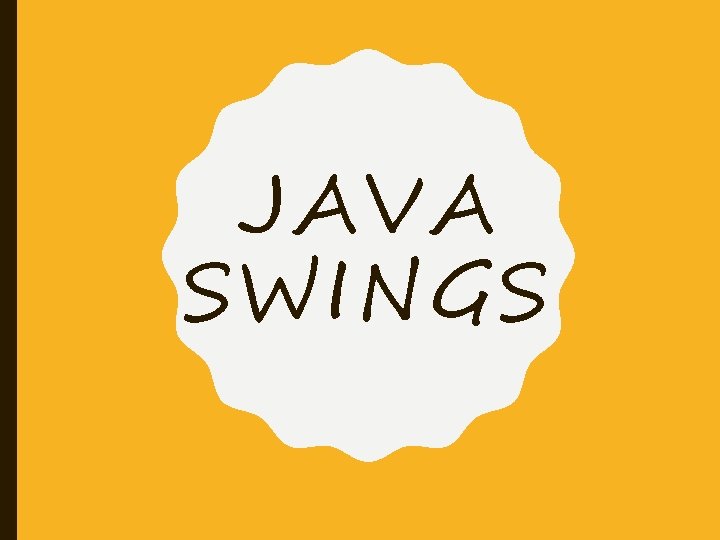
JAVA SWINGS
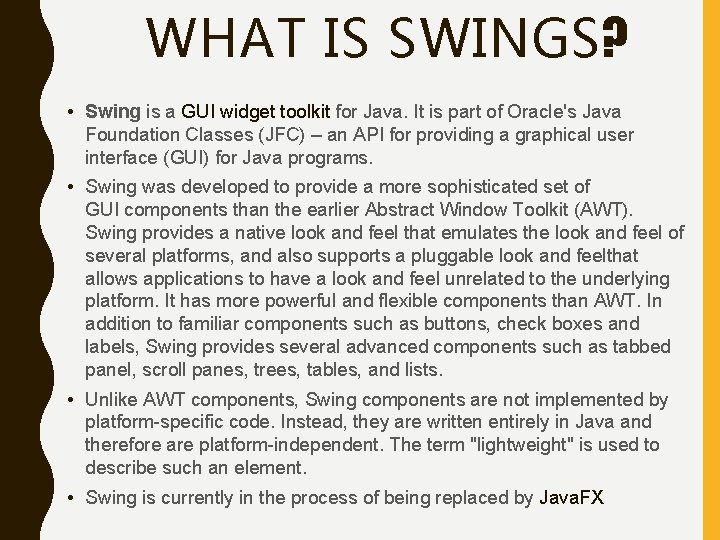
WHAT IS SWINGS? • Swing is a GUI widget toolkit for Java. It is part of Oracle's Java Foundation Classes (JFC) – an API for providing a graphical user interface (GUI) for Java programs. • Swing was developed to provide a more sophisticated set of GUI components than the earlier Abstract Window Toolkit (AWT). Swing provides a native look and feel that emulates the look and feel of several platforms, and also supports a pluggable look and feelthat allows applications to have a look and feel unrelated to the underlying platform. It has more powerful and flexible components than AWT. In addition to familiar components such as buttons, check boxes and labels, Swing provides several advanced components such as tabbed panel, scroll panes, trees, tables, and lists. • Unlike AWT components, Swing components are not implemented by platform-specific code. Instead, they are written entirely in Java and therefore are platform-independent. The term "lightweight" is used to describe such an element. • Swing is currently in the process of being replaced by Java. FX
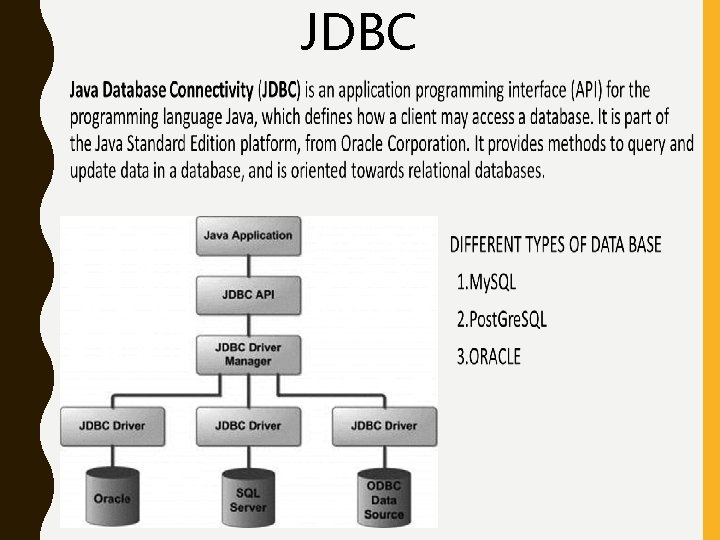
JDBC
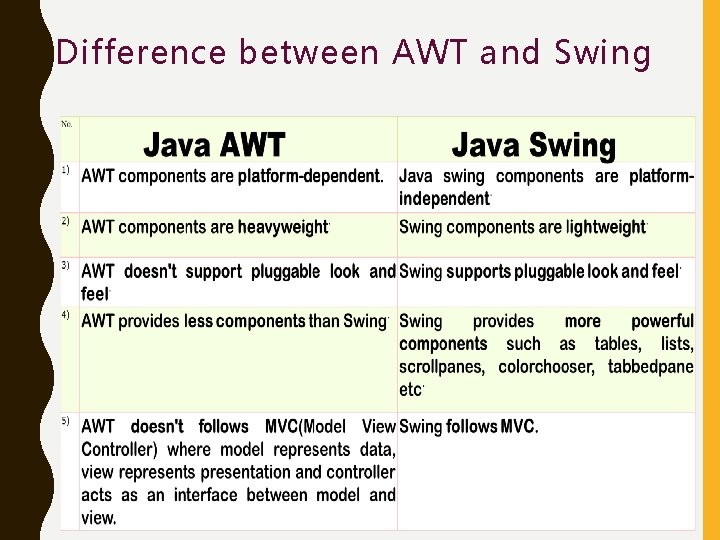
Difference between AWT and Swing
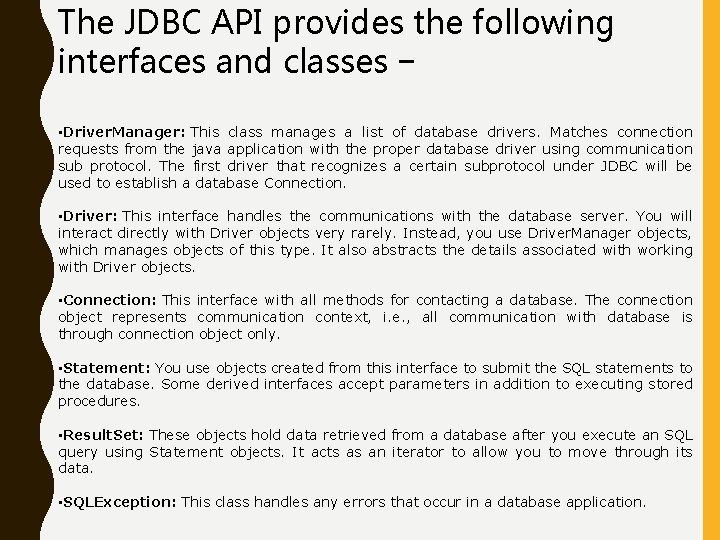
The JDBC API provides the following interfaces and classes − • Driver. Manager: This class manages a list of database drivers. Matches connection requests from the java application with the proper database driver using communication sub protocol. The first driver that recognizes a certain subprotocol under JDBC will be used to establish a database Connection. • Driver: This interface handles the communications with the database server. You will interact directly with Driver objects very rarely. Instead, you use Driver. Manager objects, which manages objects of this type. It also abstracts the details associated with working with Driver objects. • Connection: This interface with all methods for contacting a database. The connection object represents communication context, i. e. , all communication with database is through connection object only. • Statement: You use objects created from this interface to submit the SQL statements to the database. Some derived interfaces accept parameters in addition to executing stored procedures. • Result. Set: These objects hold data retrieved from a database after you execute an SQL query using Statement objects. It acts as an iterator to allow you to move through its data. • SQLException: This class handles any errors that occur in a database application.
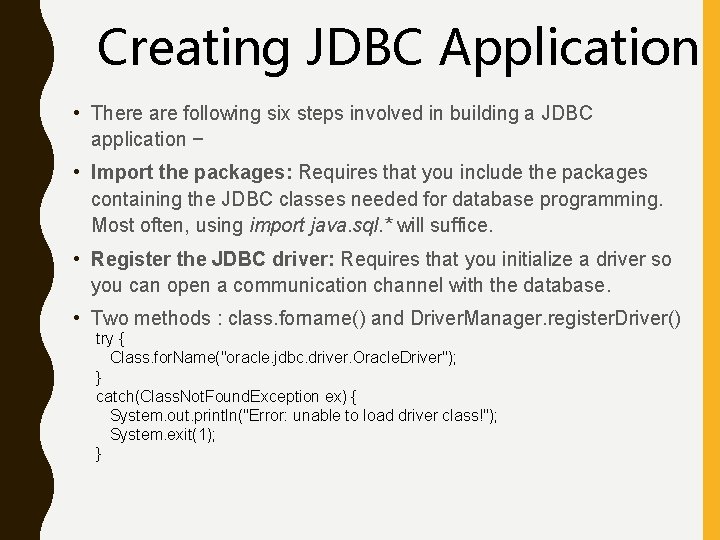
Creating JDBC Application • There are following six steps involved in building a JDBC application − • Import the packages: Requires that you include the packages containing the JDBC classes needed for database programming. Most often, using import java. sql. * will suffice. • Register the JDBC driver: Requires that you initialize a driver so you can open a communication channel with the database. • Two methods : class. forname() and Driver. Manager. register. Driver() try { Class. for. Name("oracle. jdbc. driver. Oracle. Driver"); } catch(Class. Not. Found. Exception ex) { System. out. println("Error: unable to load driver class!"); System. exit(1); }
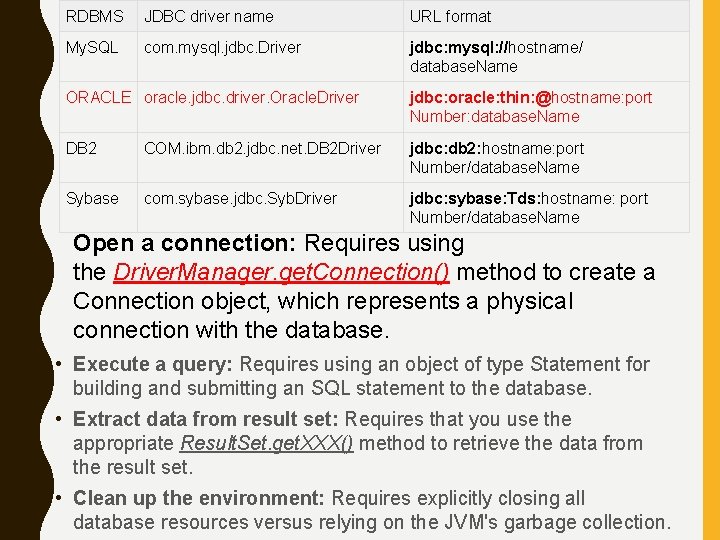
RDBMS JDBC driver name URL format My. SQL com. mysql. jdbc. Driver jdbc: mysql: //hostname/ database. Name ORACLE oracle. jdbc. driver. Oracle. Driver jdbc: oracle: thin: @hostname: port Number: database. Name DB 2 COM. ibm. db 2. jdbc. net. DB 2 Driver jdbc: db 2: hostname: port Number/database. Name Sybase com. sybase. jdbc. Syb. Driver jdbc: sybase: Tds: hostname: port Number/database. Name Open a connection: Requires using the Driver. Manager. get. Connection() method to create a Connection object, which represents a physical connection with the database. • Execute a query: Requires using an object of type Statement for building and submitting an SQL statement to the database. • Extract data from result set: Requires that you use the appropriate Result. Set. get. XXX() method to retrieve the data from the result set. • Clean up the environment: Requires explicitly closing all database resources versus relying on the JVM's garbage collection.
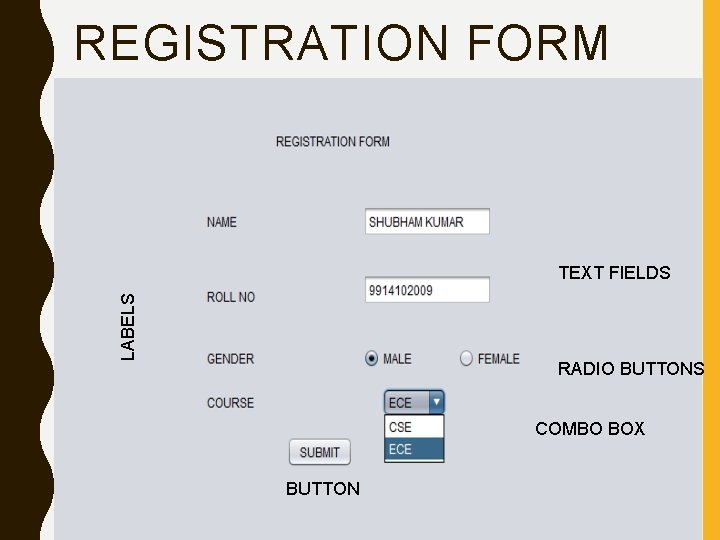
REGISTRATION FORM LABELS TEXT FIELDS RADIO BUTTONS COMBO BOX BUTTON
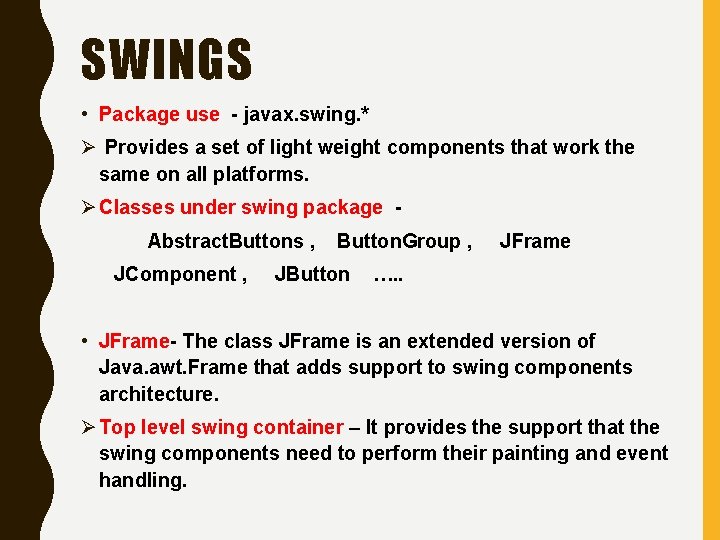
SWINGS • Package use - javax. swing. * Ø Provides a set of light weight components that work the same on all platforms. Ø Classes under swing package Abstract. Buttons , JComponent , Button. Group , JButton JFrame …. . • JFrame- The class JFrame is an extended version of Java. awt. Frame that adds support to swing components architecture. Ø Top level swing container – It provides the support that the swing components need to perform their painting and event handling.
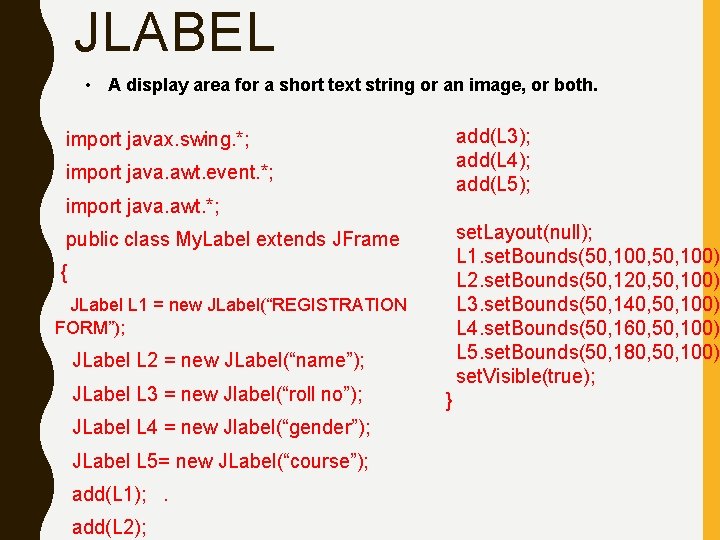
JLABEL • A display area for a short text string or an image, or both. add(L 3); add(L 4); add(L 5); import javax. swing. *; import java. awt. event. *; import java. awt. *; set. Layout(null); L 1. set. Bounds(50, 100, 50, 100); L 2. set. Bounds(50, 120, 50, 100); L 3. set. Bounds(50, 140, 50, 100); L 4. set. Bounds(50, 160, 50, 100); L 5. set. Bounds(50, 180, 50, 100); set. Visible(true); public class My. Label extends JFrame { JLabel L 1 = new JLabel(“REGISTRATION FORM”); JLabel L 2 = new JLabel(“name”); JLabel L 3 = new Jlabel(“roll no”); JLabel L 4 = new Jlabel(“gender”); JLabel L 5= new JLabel(“course”); add(L 1); . add(L 2); }
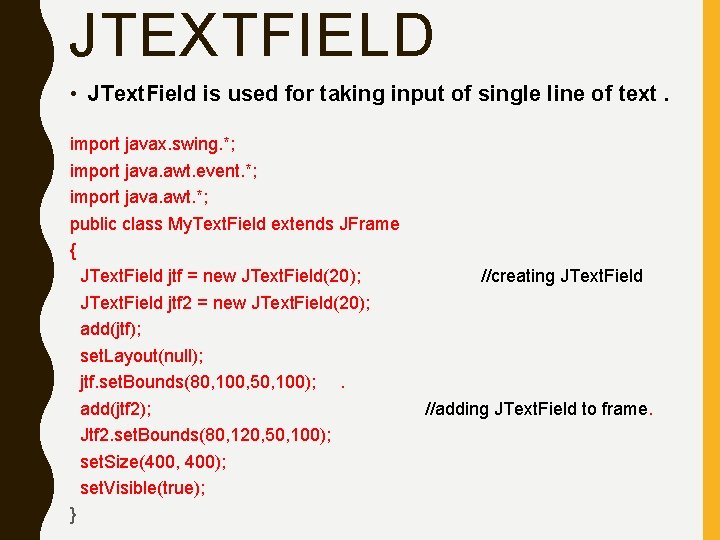
JTEXTFIELD • JText. Field is used for taking input of single line of text. import javax. swing. *; import java. awt. event. *; import java. awt. *; public class My. Text. Field extends JFrame { JText. Field jtf = new JText. Field(20); JText. Field jtf 2 = new JText. Field(20); add(jtf); set. Layout(null); jtf. set. Bounds(80, 100, 50, 100); . add(jtf 2); Jtf 2. set. Bounds(80, 120, 50, 100); set. Size(400, 400); set. Visible(true); } //creating JText. Field //adding JText. Field to frame.
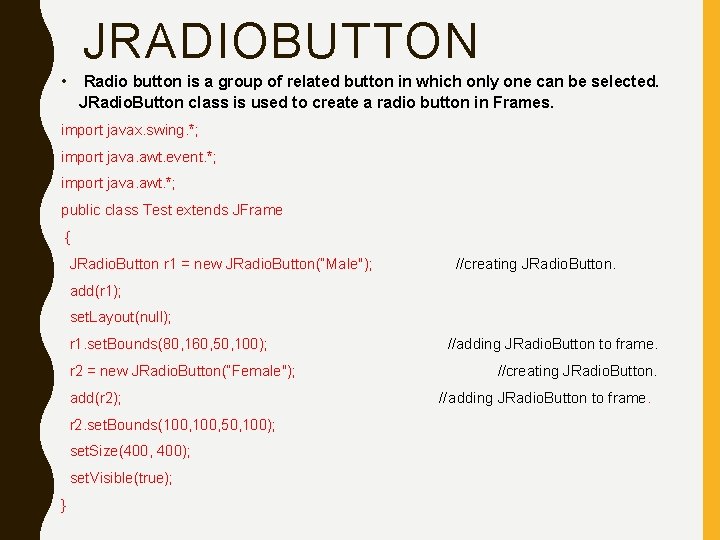
JRADIOBUTTON • Radio button is a group of related button in which only one can be selected. JRadio. Button class is used to create a radio button in Frames. import javax. swing. *; import java. awt. event. *; import java. awt. *; public class Test extends JFrame { JRadio. Button r 1 = new JRadio. Button(“Male"); //creating JRadio. Button. add(r 1); set. Layout(null); r 1. set. Bounds(80, 160, 50, 100); r 2 = new JRadio. Button(“Female"); add(r 2); r 2. set. Bounds(100, 50, 100); set. Size(400, 400); set. Visible(true); } //adding JRadio. Button to frame. //creating JRadio. Button. //adding JRadio. Button to frame.
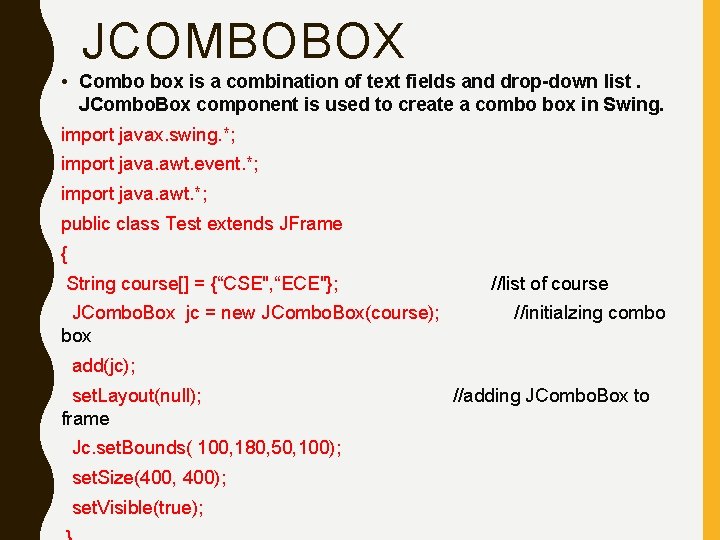
JCOMBOBOX • Combo box is a combination of text fields and drop-down list. JCombo. Box component is used to create a combo box in Swing. import javax. swing. *; import java. awt. event. *; import java. awt. *; public class Test extends JFrame { String course[] = {“CSE", “ECE"}; JCombo. Box jc = new JCombo. Box(course); box //list of course //initialzing combo add(jc); set. Layout(null); frame Jc. set. Bounds( 100, 180, 50, 100); set. Size(400, 400); set. Visible(true); //adding JCombo. Box to
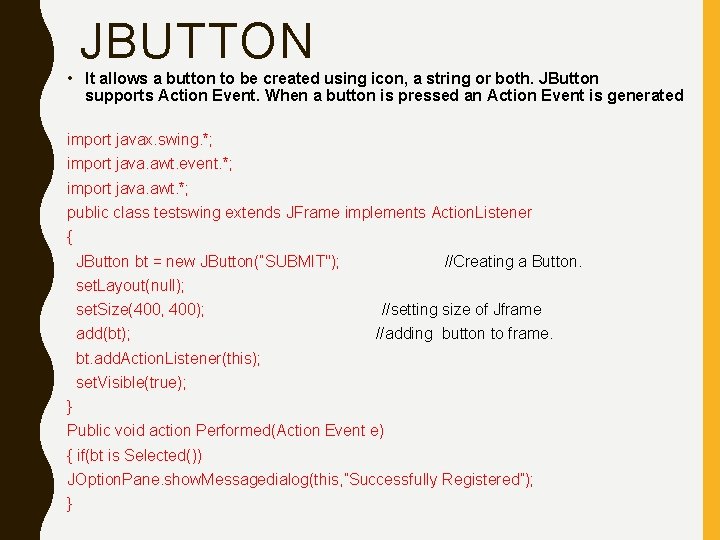
JBUTTON • It allows a button to be created using icon, a string or both. JButton supports Action Event. When a button is pressed an Action Event is generated import javax. swing. *; import java. awt. event. *; import java. awt. *; public class testswing extends JFrame implements Action. Listener { JButton bt = new JButton(“SUBMIT"); //Creating a Button. set. Layout(null); set. Size(400, 400); add(bt); //setting size of Jframe //adding button to frame. bt. add. Action. Listener(this); set. Visible(true); } Public void action Performed(Action Event e) { if(bt is Selected()) JOption. Pane. show. Messagedialog(this, ”Successfully Registered”); }
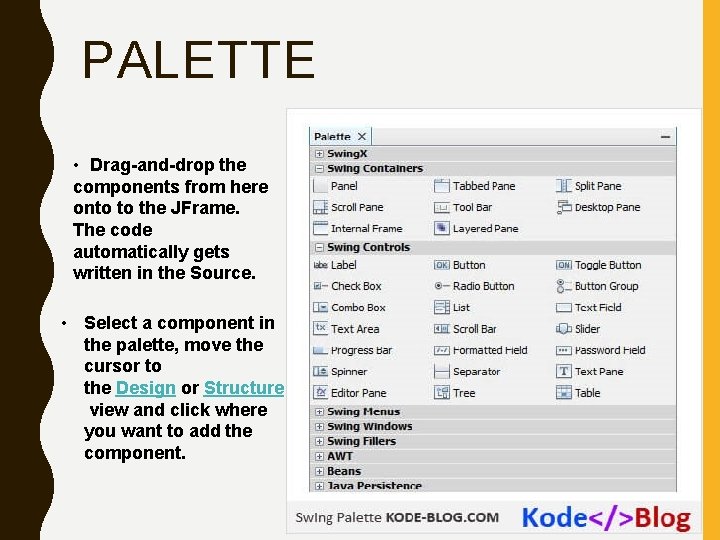
PALETTE • Drag-and-drop the components from here onto to the JFrame. The code automatically gets written in the Source. • Select a component in the palette, move the cursor to the Design or Structure view and click where you want to add the component.
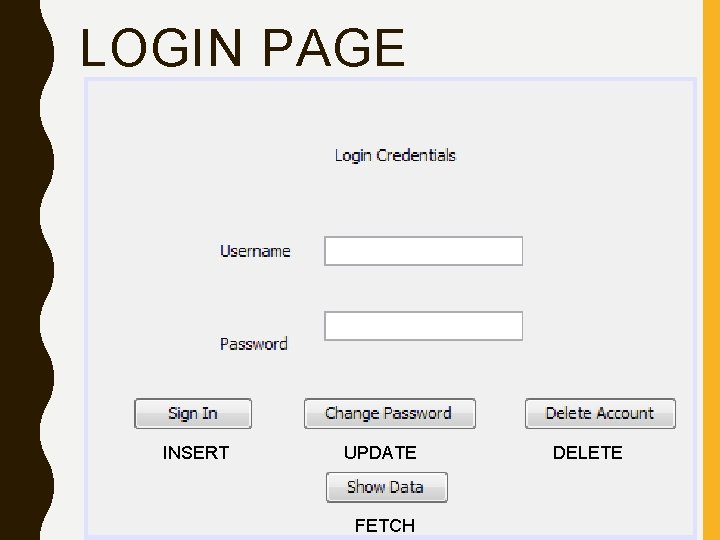
LOGIN PAGE INSERT UPDATE FETCH DELETE
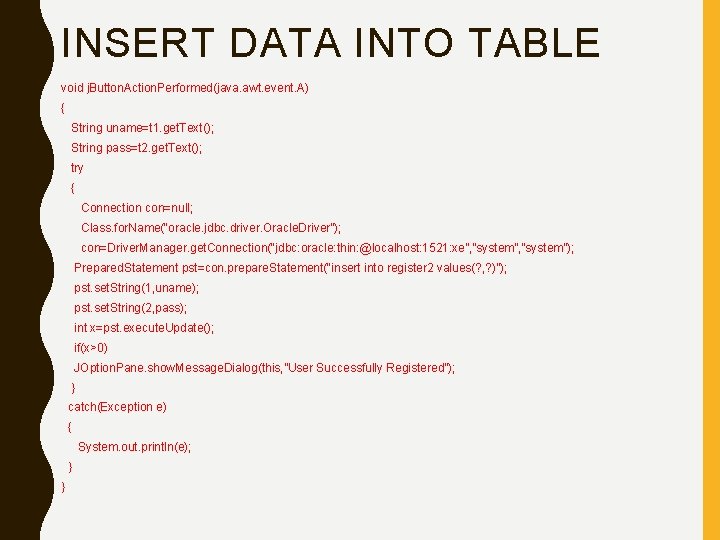
INSERT DATA INTO TABLE void j. Button. Action. Performed(java. awt. event. A) { String uname=t 1. get. Text(); String pass=t 2. get. Text(); try { Connection con=null; Class. for. Name(“oracle. jdbc. driver. Oracle. Driver”); con=Driver. Manager. get. Connection(“jdbc: oracle: thin: @localhost: 1521: xe”, ”system”); Prepared. Statement pst=con. prepare. Statement(“insert into register 2 values(? , ? )”); pst. set. String(1, uname); pst. set. String(2, pass); int x=pst. execute. Update(); if(x>0) JOption. Pane. show. Message. Dialog(this, ”User Successfully Registered”); } catch(Exception e) { System. out. println(e); } }
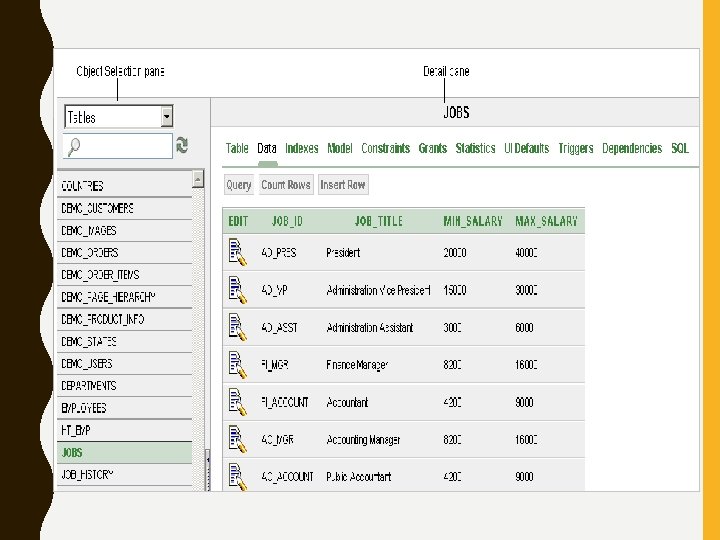
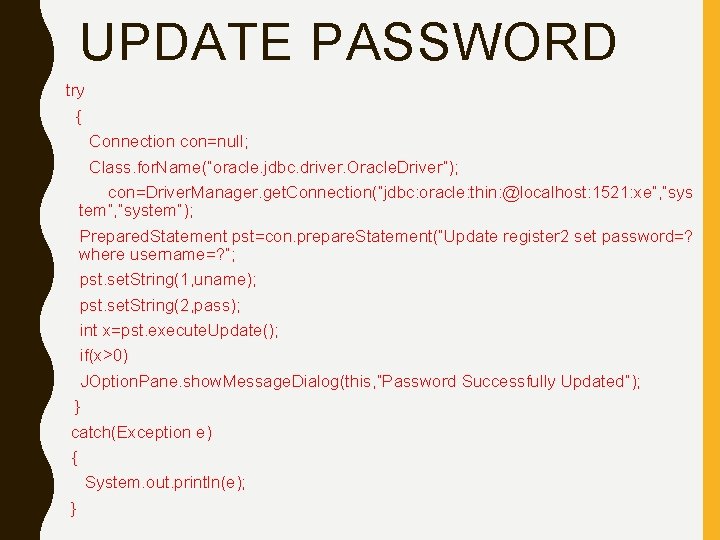
UPDATE PASSWORD try { Connection con=null; Class. for. Name(“oracle. jdbc. driver. Oracle. Driver”); con=Driver. Manager. get. Connection(“jdbc: oracle: thin: @localhost: 1521: xe”, ”sys tem”, ”system”); Prepared. Statement pst=con. prepare. Statement(“Update register 2 set password=? where username=? ”; pst. set. String(1, uname); pst. set. String(2, pass); int x=pst. execute. Update(); if(x>0) JOption. Pane. show. Message. Dialog(this, ”Password Successfully Updated”); } catch(Exception e) { System. out. println(e); }
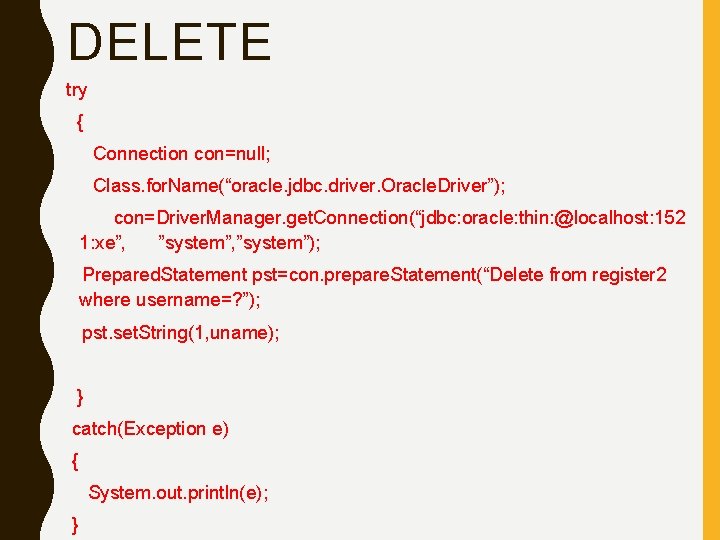
DELETE try { Connection con=null; Class. for. Name(“oracle. jdbc. driver. Oracle. Driver”); con=Driver. Manager. get. Connection(“jdbc: oracle: thin: @localhost: 152 1: xe”, ”system”); Prepared. Statement pst=con. prepare. Statement(“Delete from register 2 where username=? ”); pst. set. String(1, uname); } catch(Exception e) { System. out. println(e); }
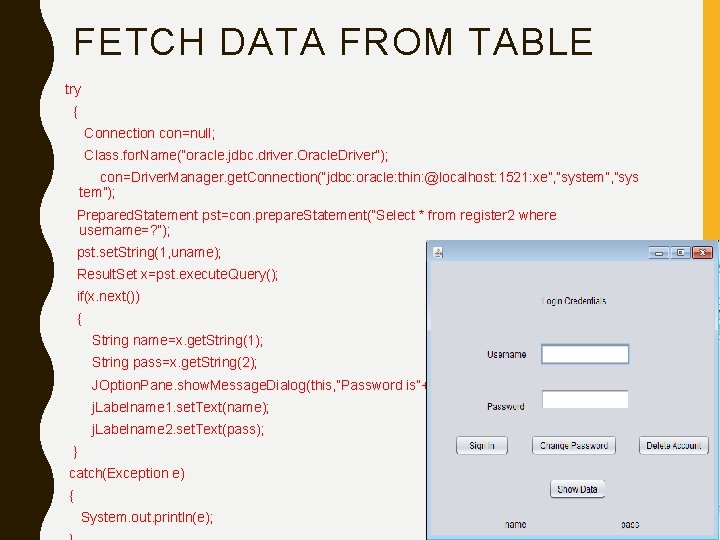
FETCH DATA FROM TABLE try { Connection con=null; Class. for. Name(“oracle. jdbc. driver. Oracle. Driver”); con=Driver. Manager. get. Connection(“jdbc: oracle: thin: @localhost: 1521: xe”, ”system”, ”sys tem”); Prepared. Statement pst=con. prepare. Statement(“Select * from register 2 where username=? ”); pst. set. String(1, uname); Result. Set x=pst. execute. Query(); if(x. next()) { String name=x. get. String(1); String pass=x. get. String(2); JOption. Pane. show. Message. Dialog(this, ”Password is”+pass); j. Labelname 1. set. Text(name); j. Labelname 2. set. Text(pass); } catch(Exception e) { System. out. println(e);
A person swings on a swing when the person sits still
Perbedaan antara java swing dan awt adalah
Tutorial java swing
Java swings
Java swing
Abstract windowing toolkit
Java swing tutorial
Null layout in java
Java swing
Java swing responsive
Java awt swing
Java gui libraries
Java graphics set color
Java swing browser
Java swing actionlistener multiple buttons
Java swing frame
A the top of the gui containment hierarchy
Java swing form example
Swing layout
Swing mvc
Java swing mvc example
Yoshi