Java Coding 6 Collections David Davenport Computer Eng
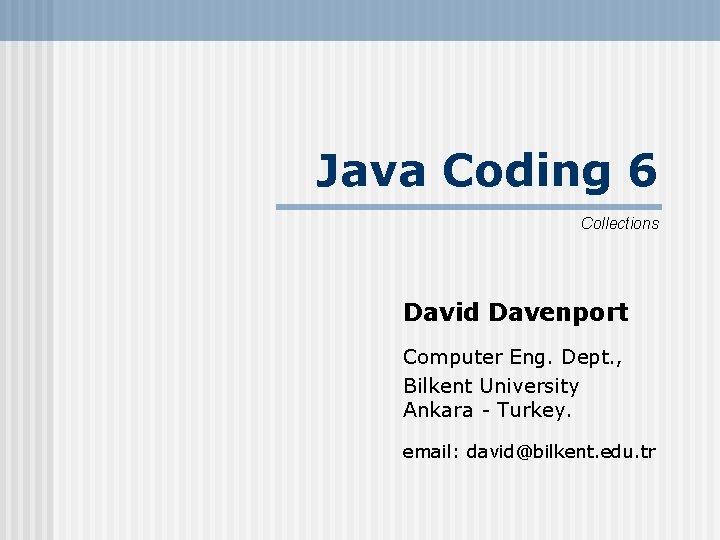
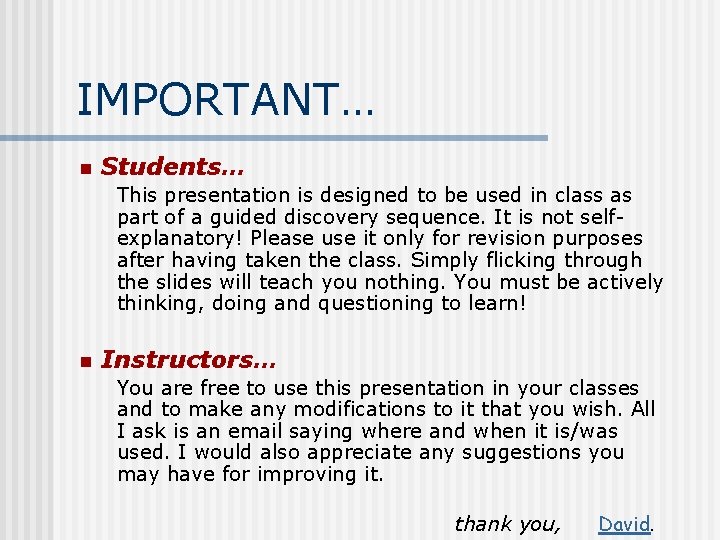
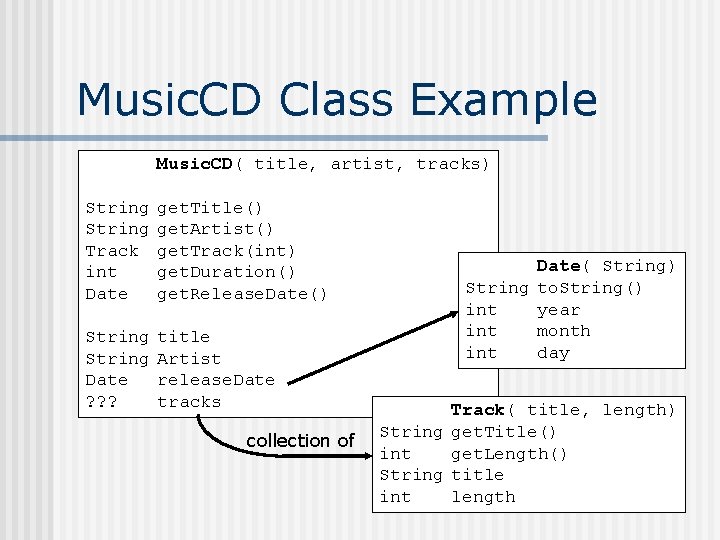
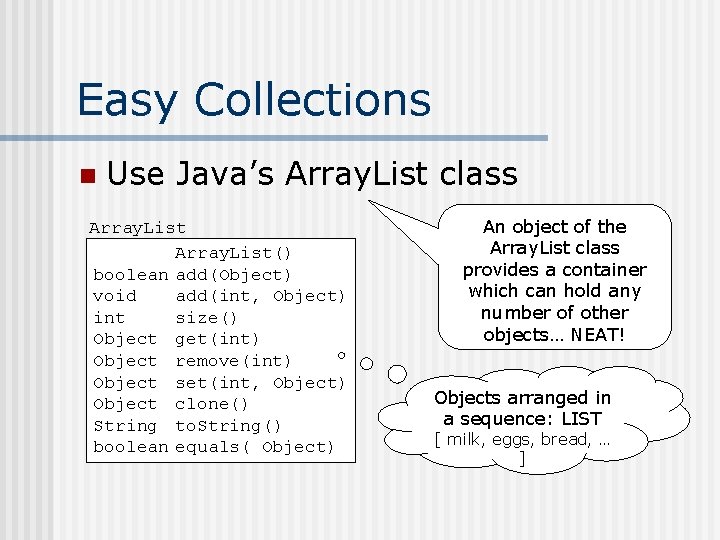
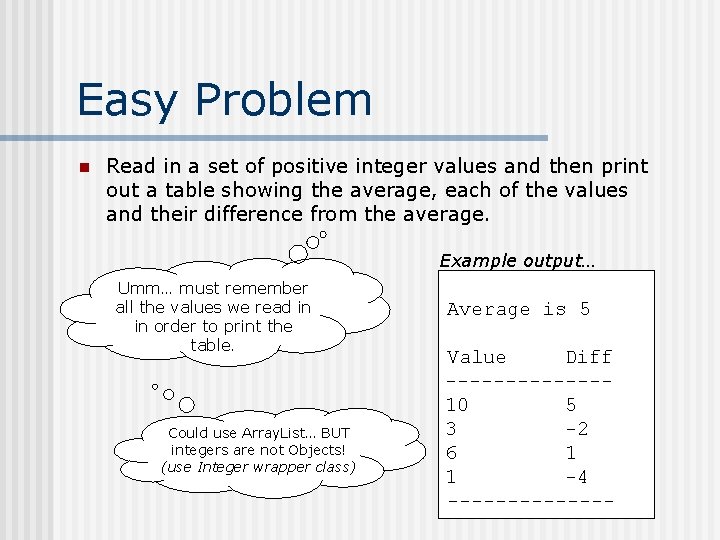
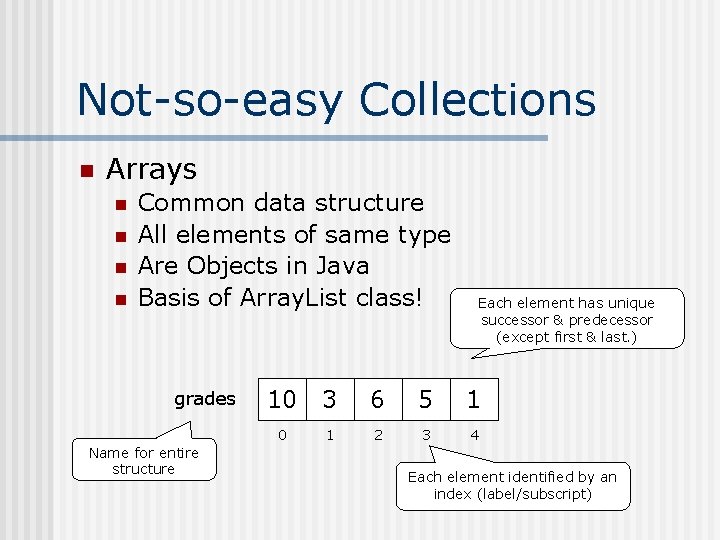
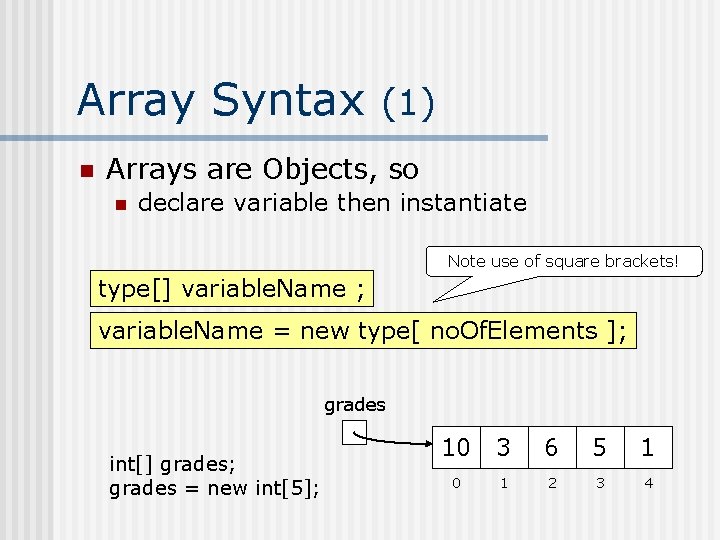
![Array Syntax (2) n Referring to an individual element variable. Name[index] n examples grades[0] Array Syntax (2) n Referring to an individual element variable. Name[index] n examples grades[0]](https://slidetodoc.com/presentation_image_h2/83121bdc80b89e2740867c4764fe2ae8/image-8.jpg)
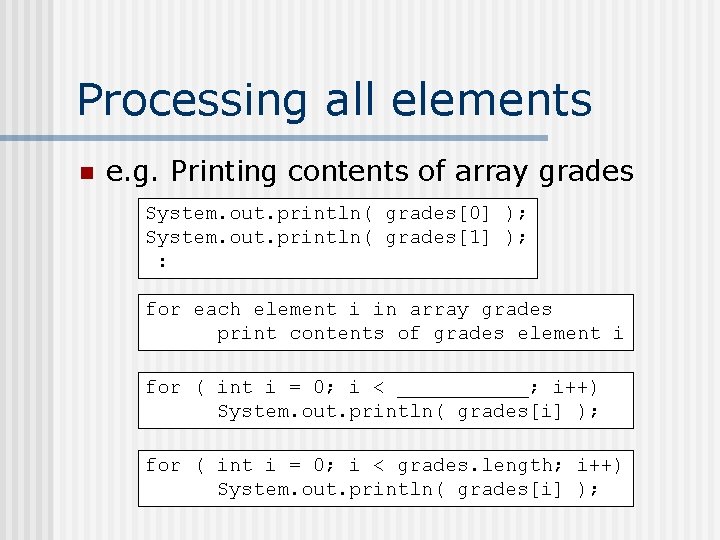
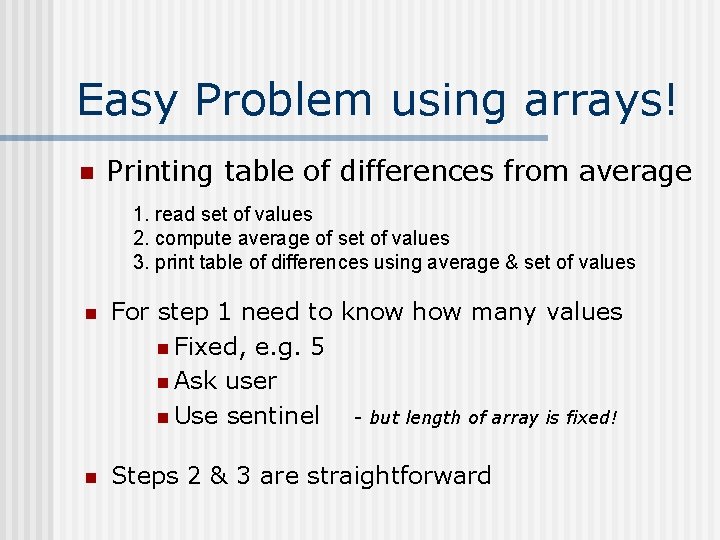
![Arrays of objects n Array contains only references to objects Track[] tracks; tracks = Arrays of objects n Array contains only references to objects Track[] tracks; tracks =](https://slidetodoc.com/presentation_image_h2/83121bdc80b89e2740867c4764fe2ae8/image-11.jpg)
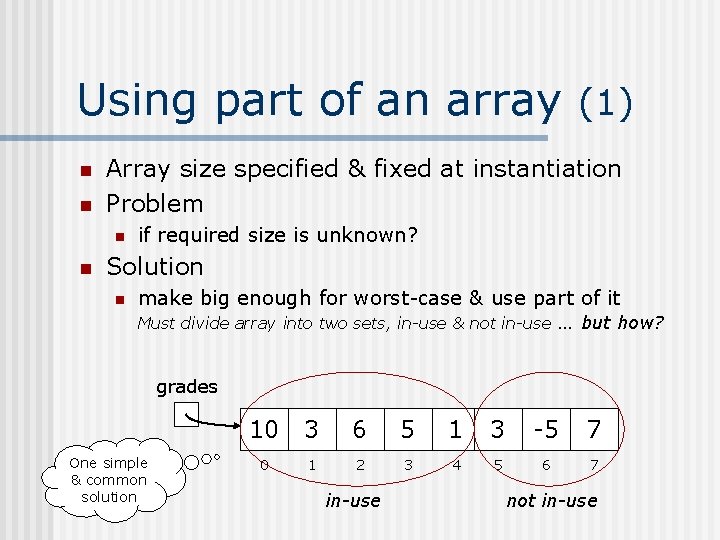
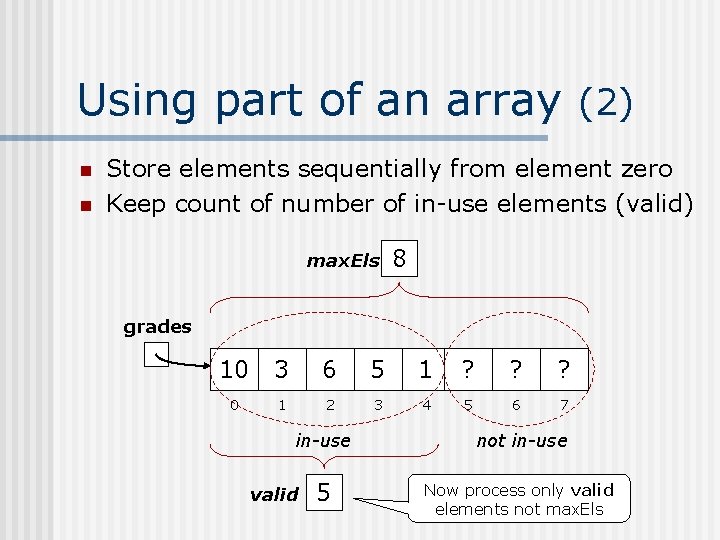
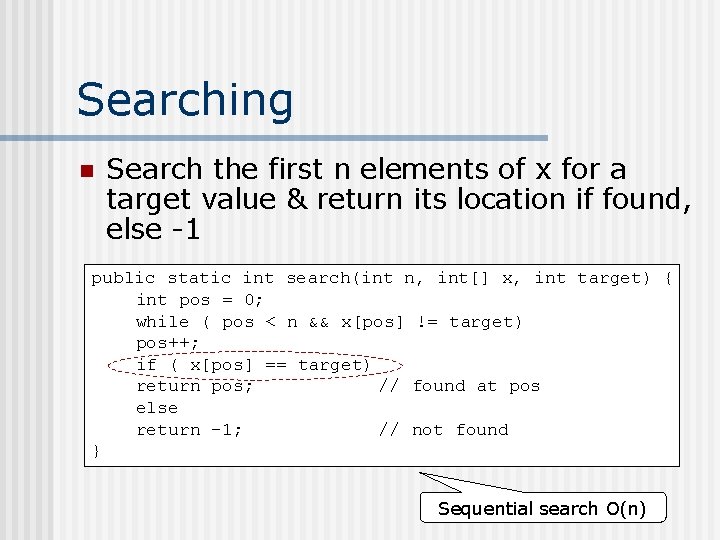
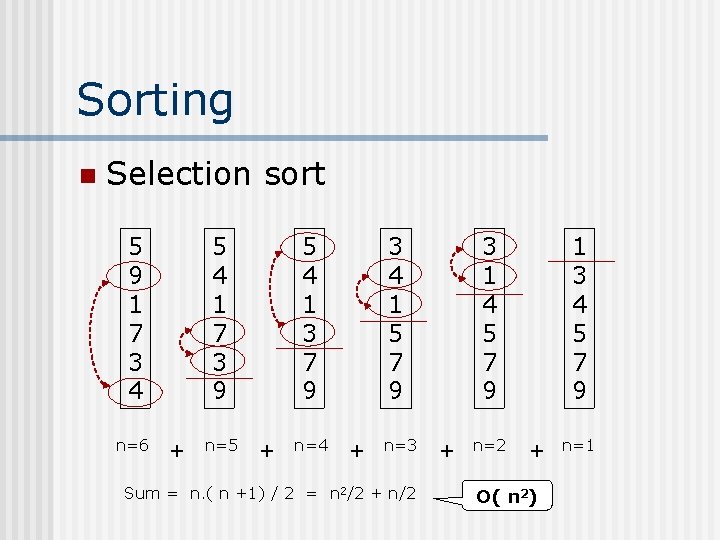
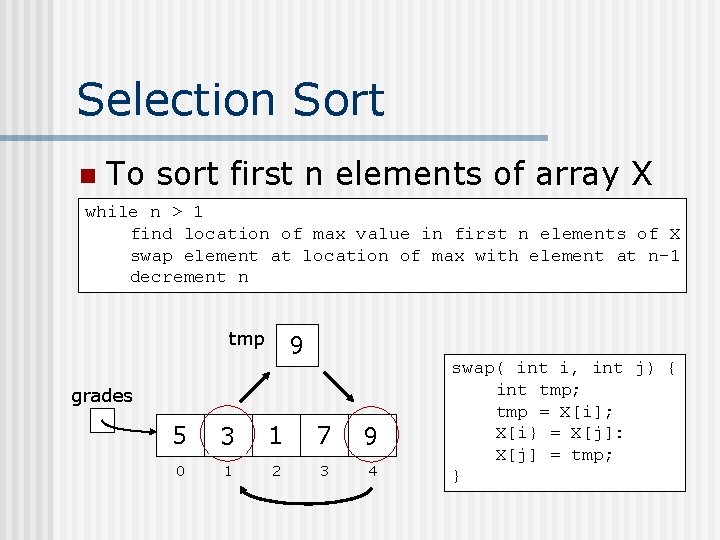
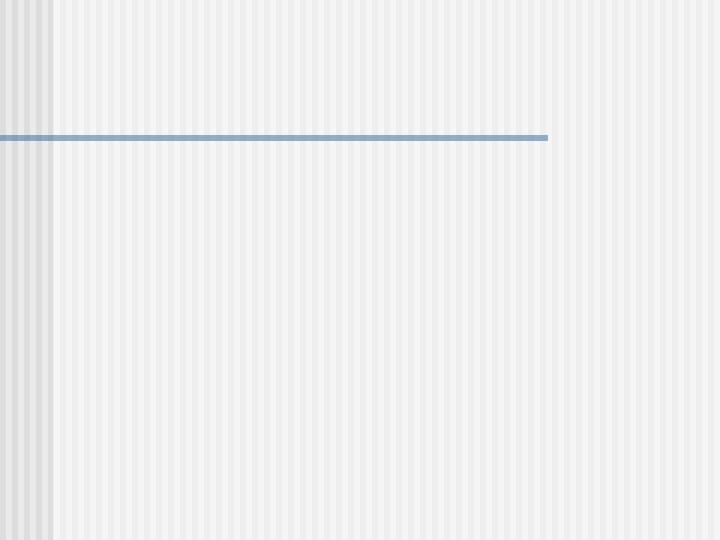
- Slides: 17
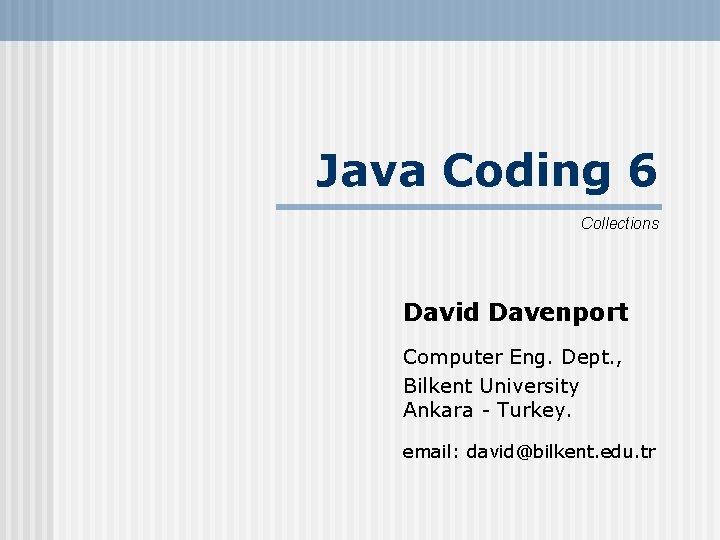
Java Coding 6 Collections David Davenport Computer Eng. Dept. , Bilkent University Ankara - Turkey. email: david@bilkent. edu. tr
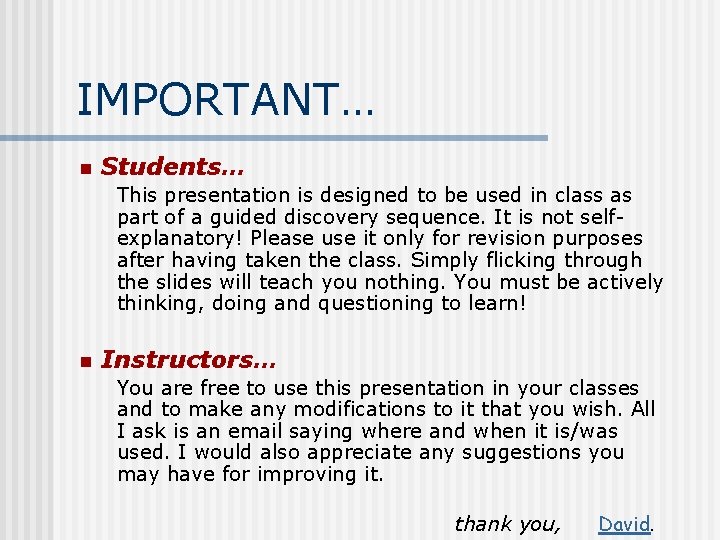
IMPORTANT… n Students… This presentation is designed to be used in class as part of a guided discovery sequence. It is not selfexplanatory! Please use it only for revision purposes after having taken the class. Simply flicking through the slides will teach you nothing. You must be actively thinking, doing and questioning to learn! n Instructors… You are free to use this presentation in your classes and to make any modifications to it that you wish. All I ask is an email saying where and when it is/was used. I would also appreciate any suggestions you may have for improving it. thank you, David.
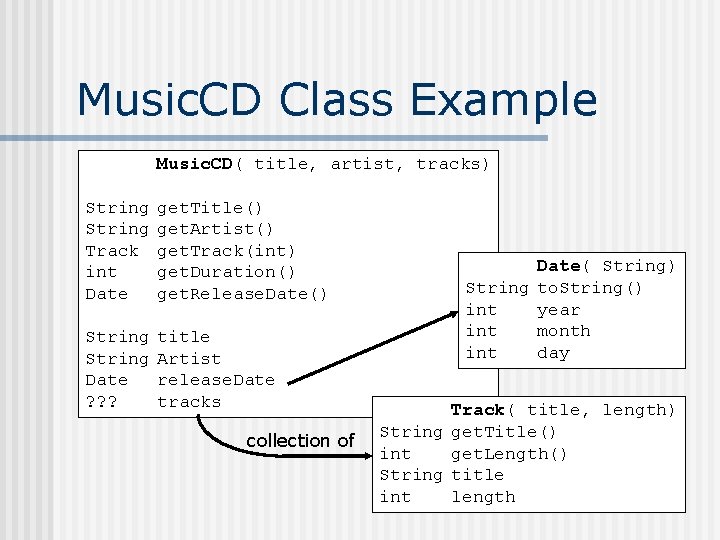
Music. CD Class Example Music. CD( title, artist, tracks) String Track int Date get. Title() get. Artist() get. Track(int) get. Duration() get. Release. Date() String Date ? ? ? title Artist release. Date tracks collection of Date( String) String to. String() int year int month int day Track( title, length) String get. Title() int get. Length() String title int length
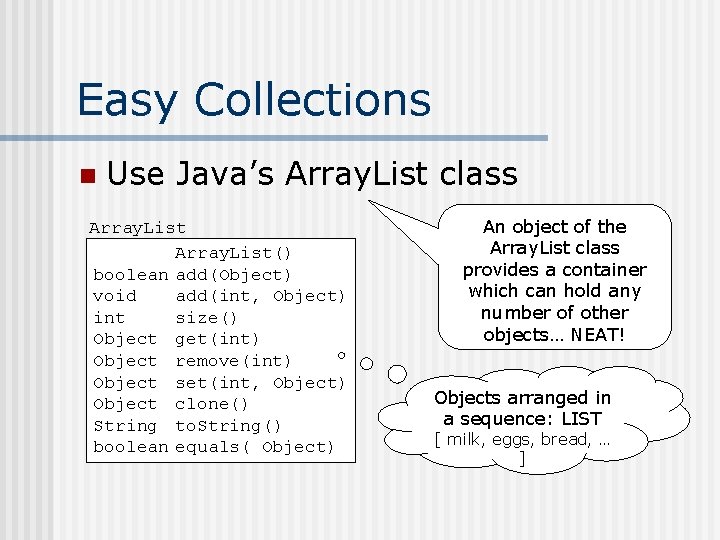
Easy Collections n Use Java’s Array. List class Array. List() boolean add(Object) void add(int, Object) int size() Object get(int) Object remove(int) Object set(int, Object) Object clone() String to. String() boolean equals( Object) An object of the Array. List class provides a container which can hold any number of other objects… NEAT! Objects arranged in a sequence: LIST [ milk, eggs, bread, … ]
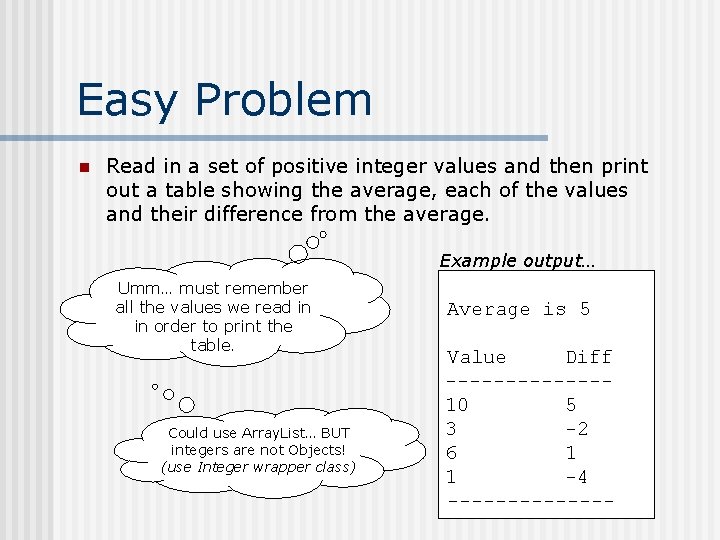
Easy Problem n Read in a set of positive integer values and then print out a table showing the average, each of the values and their difference from the average. Example output… Umm… must remember all the values we read in in order to print the table. Could use Array. List… BUT integers are not Objects! (use Integer wrapper class) Average is 5 Value Diff -------10 5 3 -2 6 1 1 -4 -------
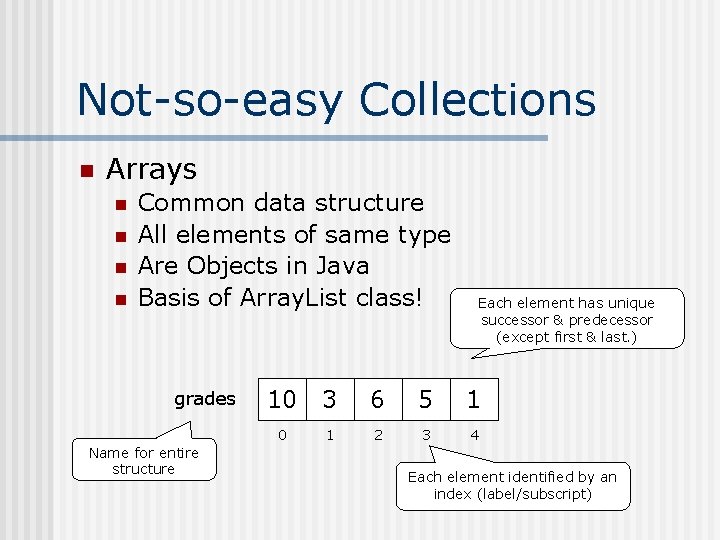
Not-so-easy Collections n Arrays n n Common data structure All elements of same type Are Objects in Java Basis of Array. List class! grades Name for entire structure Each element has unique successor & predecessor (except first & last. ) 10 3 6 5 1 0 1 2 3 4 Each element identified by an index (label/subscript)
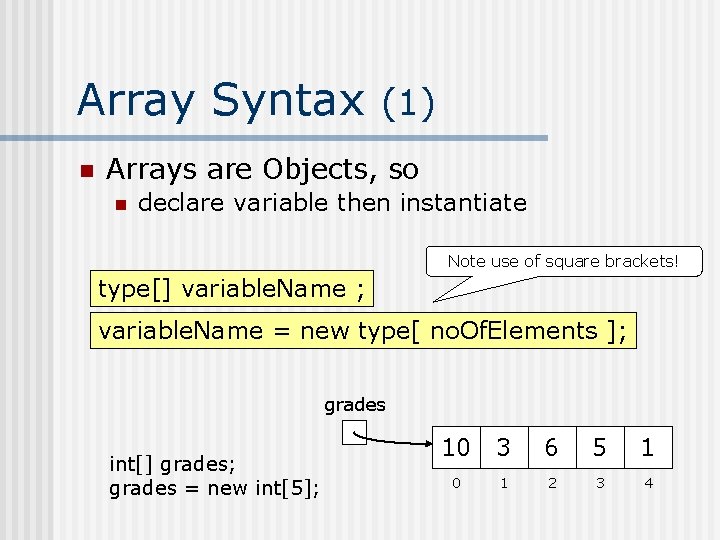
Array Syntax (1) n Arrays are Objects, so n declare variable then instantiate Note use of square brackets! type[] variable. Name ; variable. Name = new type[ no. Of. Elements ]; grades int[] grades; grades = new int[5]; 10 3 6 5 1 0 1 2 3 4
![Array Syntax 2 n Referring to an individual element variable Nameindex n examples grades0 Array Syntax (2) n Referring to an individual element variable. Name[index] n examples grades[0]](https://slidetodoc.com/presentation_image_h2/83121bdc80b89e2740867c4764fe2ae8/image-8.jpg)
Array Syntax (2) n Referring to an individual element variable. Name[index] n examples grades[0] grades[1] names[99] grades[ i+1] names[ FIRST] grades[0] = 10; grades[1] = grades[0] + 2; System. out. println( grades[0]); names[99] = Keyboard. read. String();
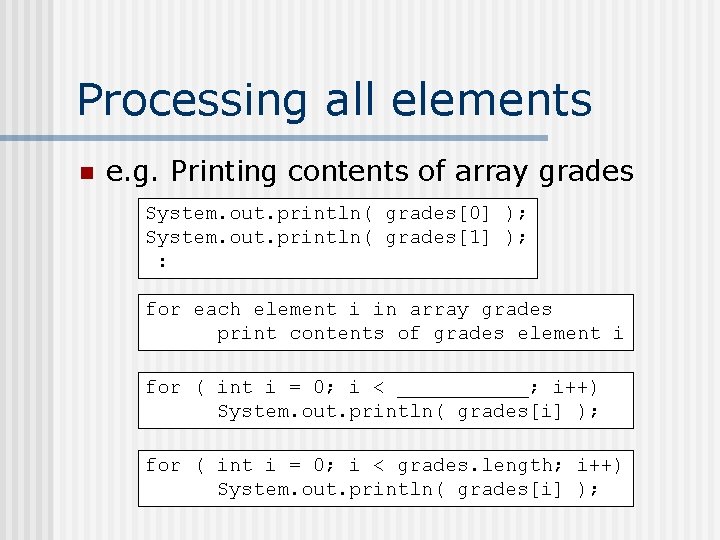
Processing all elements n e. g. Printing contents of array grades System. out. println( grades[0] ); System. out. println( grades[1] ); : for each element i in array grades print contents of grades element i for ( int i = 0; i < ______; i++) System. out. println( grades[i] ); for ( int i = 0; i < grades. length; i++) System. out. println( grades[i] );
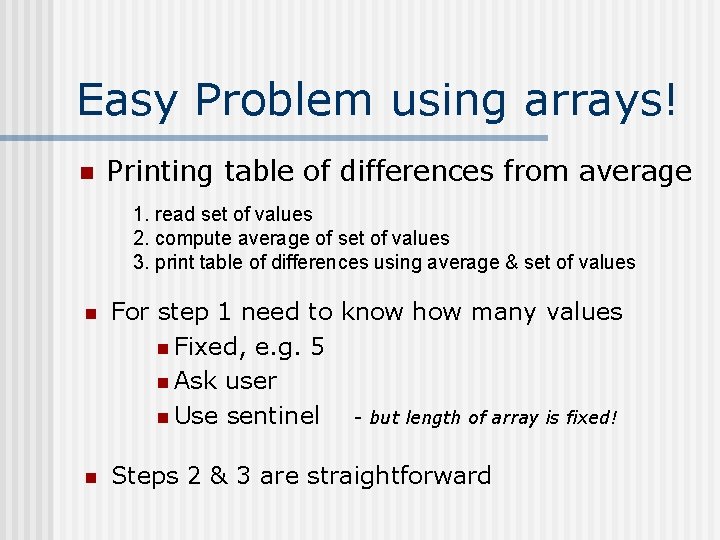
Easy Problem using arrays! n Printing table of differences from average 1. read set of values 2. compute average of set of values 3. print table of differences using average & set of values n For step 1 need to know how many values n Fixed, e. g. 5 n Ask user n Use sentinel - but length of array is fixed! n Steps 2 & 3 are straightforward
![Arrays of objects n Array contains only references to objects Track tracks tracks Arrays of objects n Array contains only references to objects Track[] tracks; tracks =](https://slidetodoc.com/presentation_image_h2/83121bdc80b89e2740867c4764fe2ae8/image-11.jpg)
Arrays of objects n Array contains only references to objects Track[] tracks; tracks = new Track[5]; n Still need to create actual objects tracks[0] = new Track( “David”, 100); tracks[1] = new Track( “Gunes”, 200); tracks 0 David 100 1 2 Gunes 200 3 4 tracks[0]. get. Title() tracks[4]. get. Title()
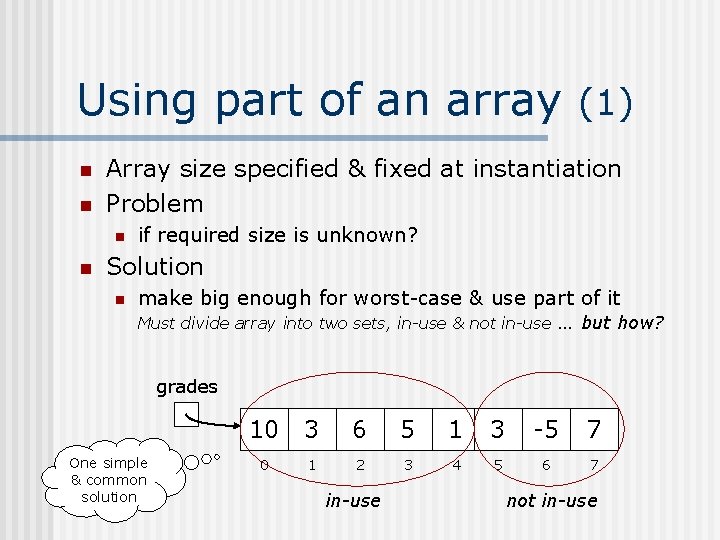
Using part of an array (1) n n Array size specified & fixed at instantiation Problem n n if required size is unknown? Solution n make big enough for worst-case & use part of it Must divide array into two sets, in-use & not in-use … but how? grades One simple & common solution 10 3 6 5 1 3 -5 7 0 1 2 3 4 5 6 7 in-use not in-use
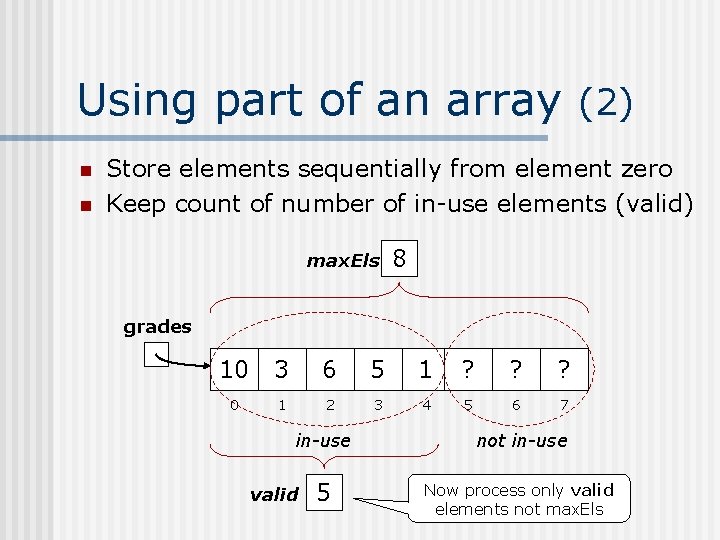
Using part of an array (2) n n Store elements sequentially from element zero Keep count of number of in-use elements (valid) max. Els 8 grades 10 3 6 5 1 ? ? ? 0 1 2 3 4 5 6 7 in-use not in-use 5 Now process only valid elements not max. Els valid
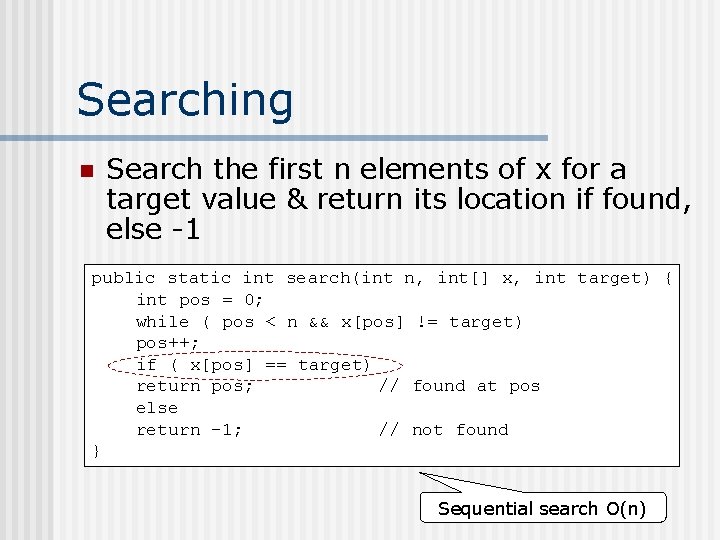
Searching n Search the first n elements of x for a target value & return its location if found, else -1 public static int search(int n, int[] x, int target) { int pos = 0; while ( pos < n && x[pos] != target) pos++; if ( x[pos] == target) return pos; // found at pos else return – 1; // not found } Sequential search O(n)
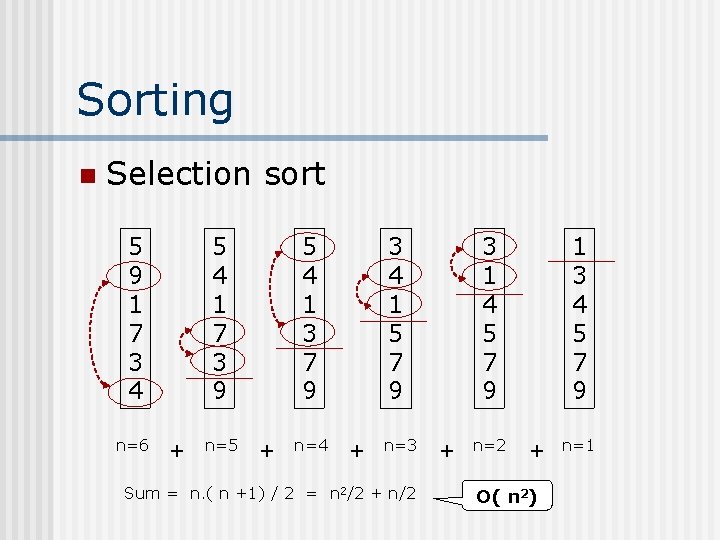
Sorting n Selection sort 5 4 1 7 3 9 5 9 1 7 3 4 n=6 + n=5 5 4 1 3 7 9 + n=4 3 4 1 5 7 9 + n=3 Sum = n. ( n +1) / 2 = n 2/2 + n/2 3 1 4 5 7 9 + n=2 1 3 4 5 7 9 + O( n 2) n=1
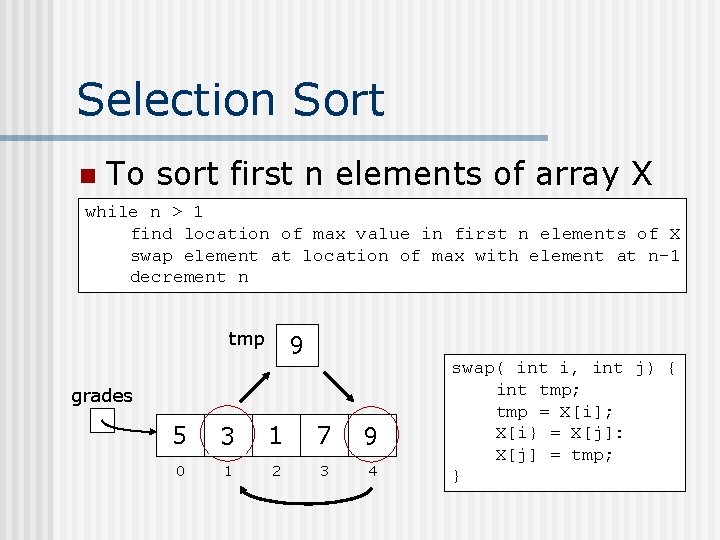
Selection Sort n To sort first n elements of array X while n > 1 find location of max value in first n elements of X swap element at location of max with element at n-1 decrement n tmp 9 grades 5 9 3 1 7 3 9 0 1 2 3 4 swap( int i, int j) { int tmp; tmp = X[i]; X[i} = X[j]: X[j] = tmp; }
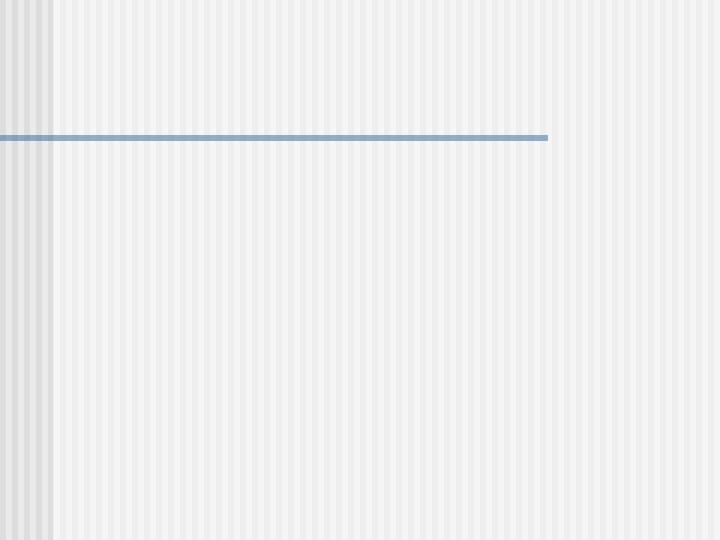
Contoh open coding, axial coding selective coding
Contoh axial coding
Eng katta va eng kichik qiymatlari
Bilkent cs
David davenport bilkent
David davenport bilkent
Minimax java
Java collections overview
Java collections framework diagram
Java collection hierarchy
Import java.util.* class test collection 15
Google guava collections
History of qualitative research
Coding dna and non coding dna
Diagramme de davenport
Diagramme de davenport
Homer davenport saltville va
Deans pest control