Java Coding 4 Method madness David Davenport Computer
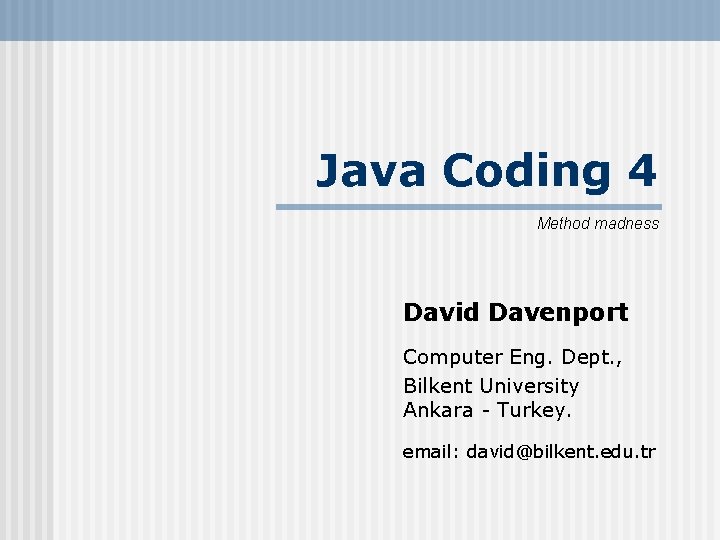
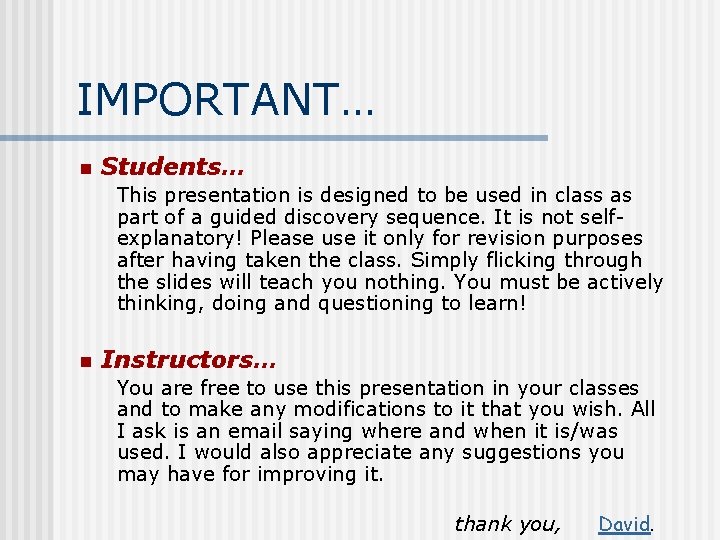
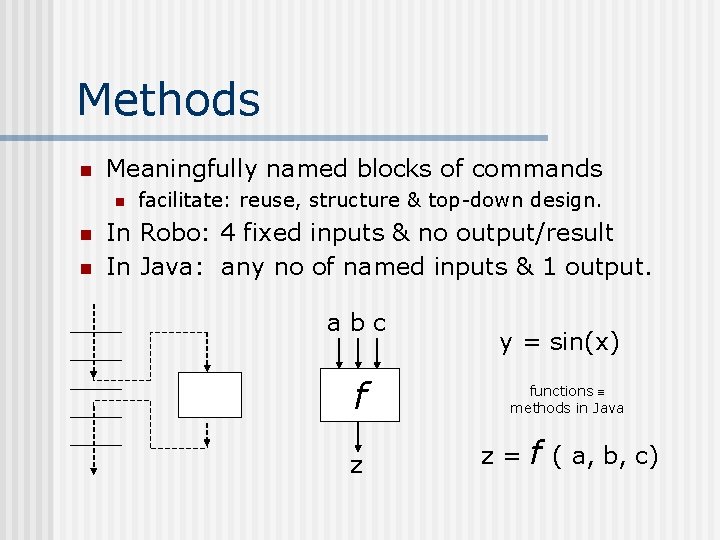
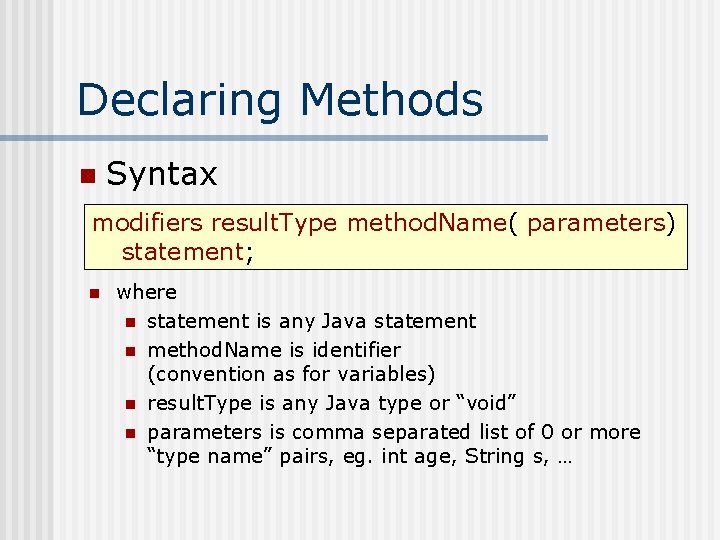
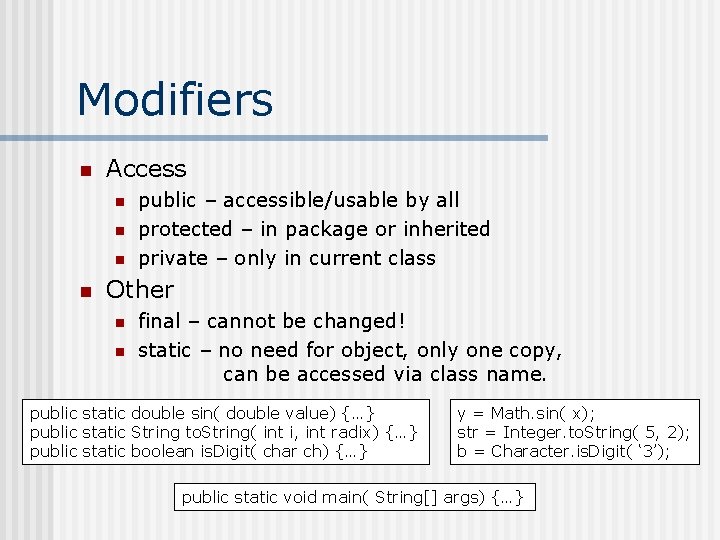
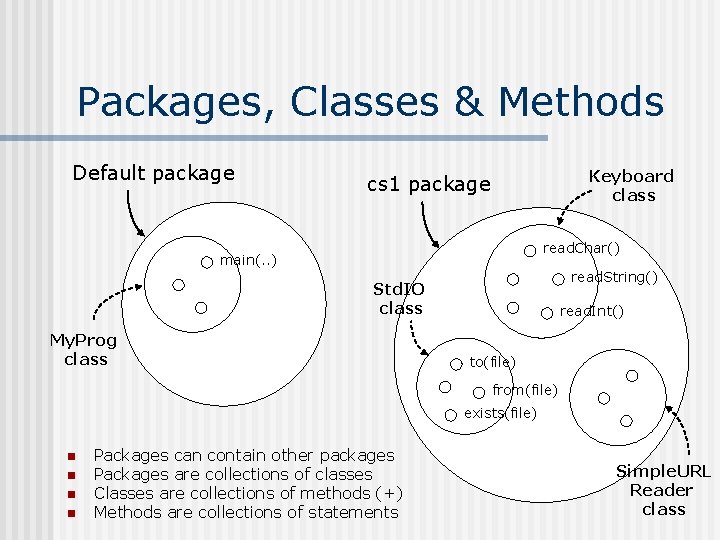
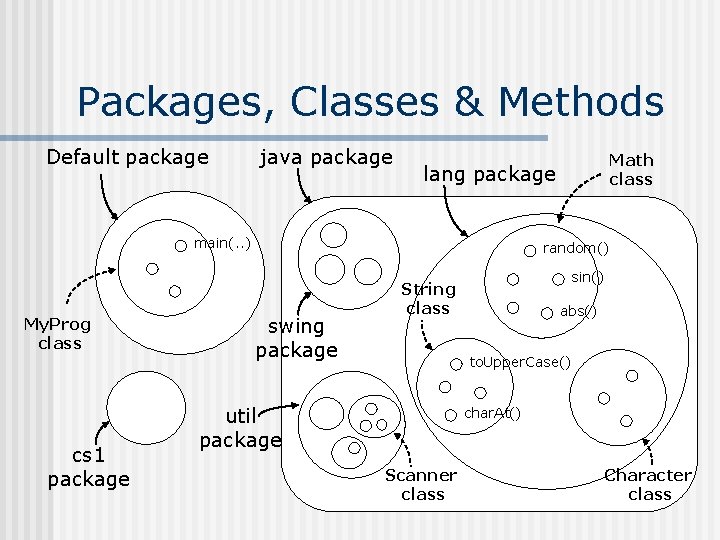
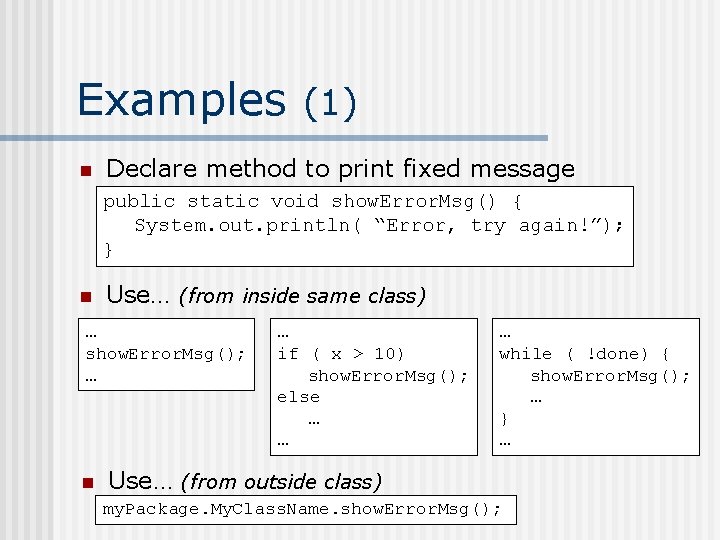
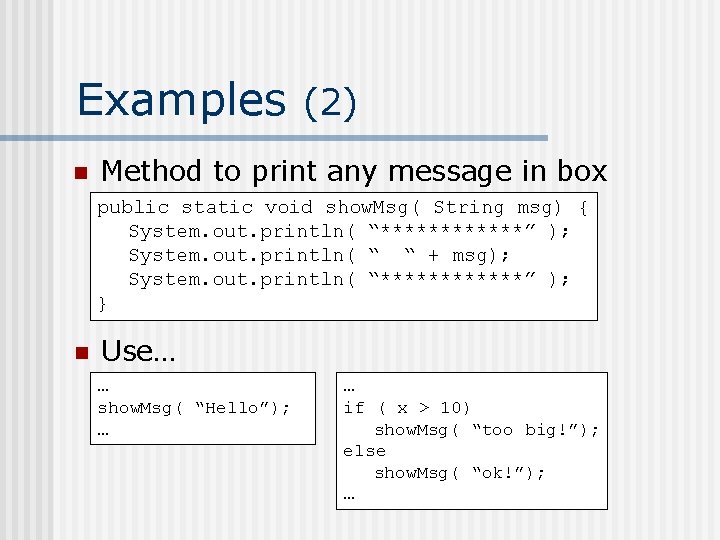
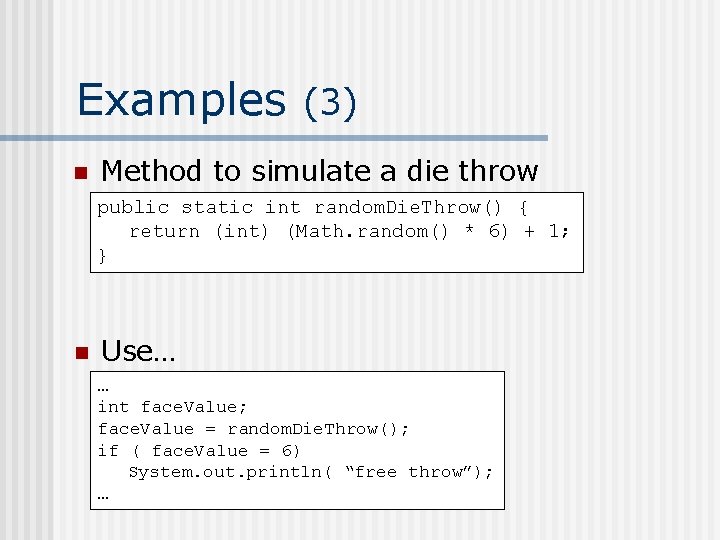
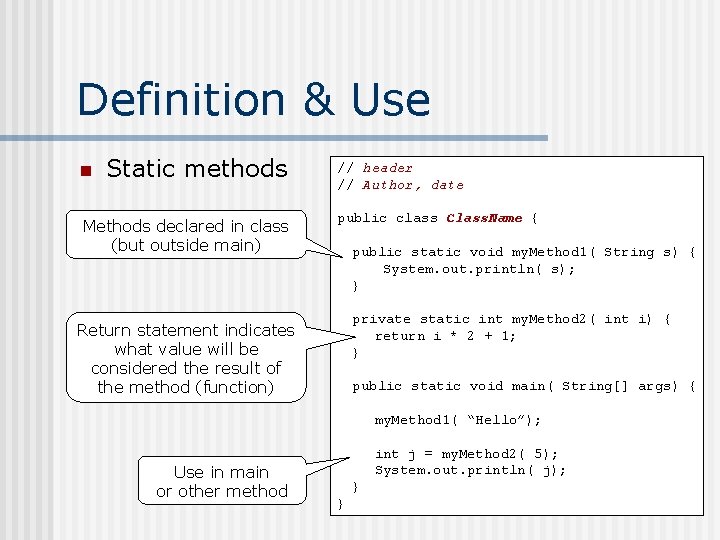
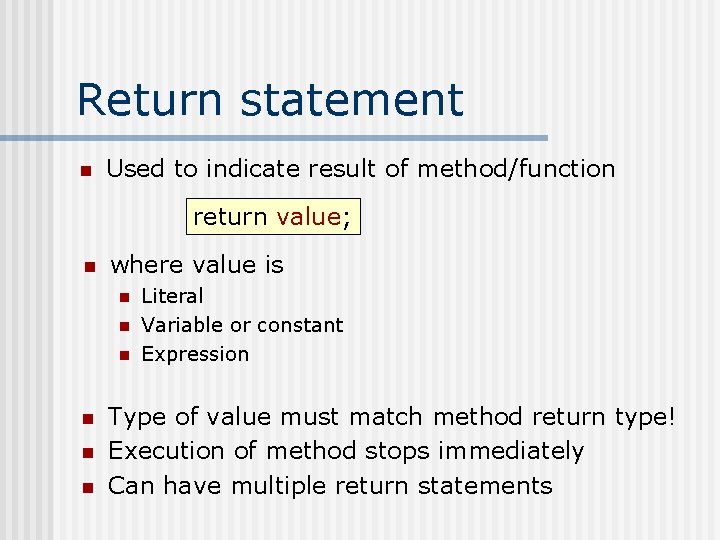
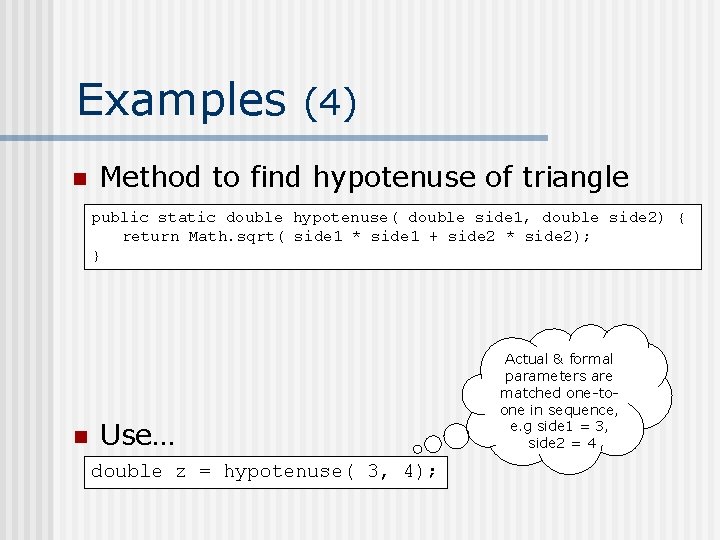
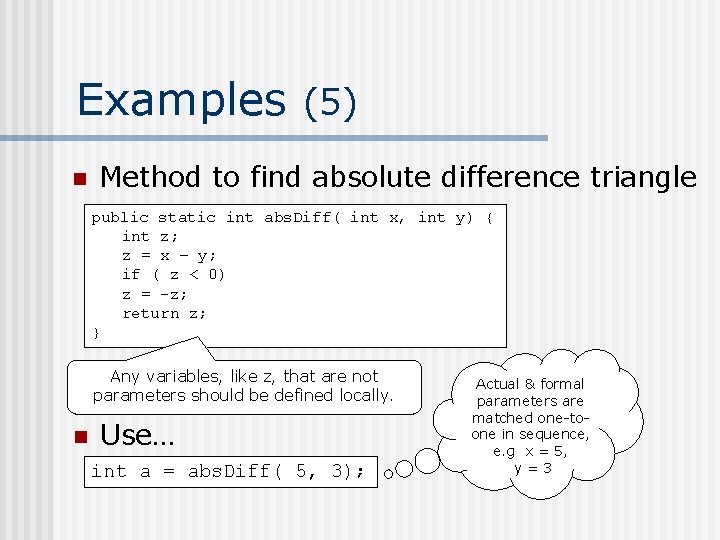
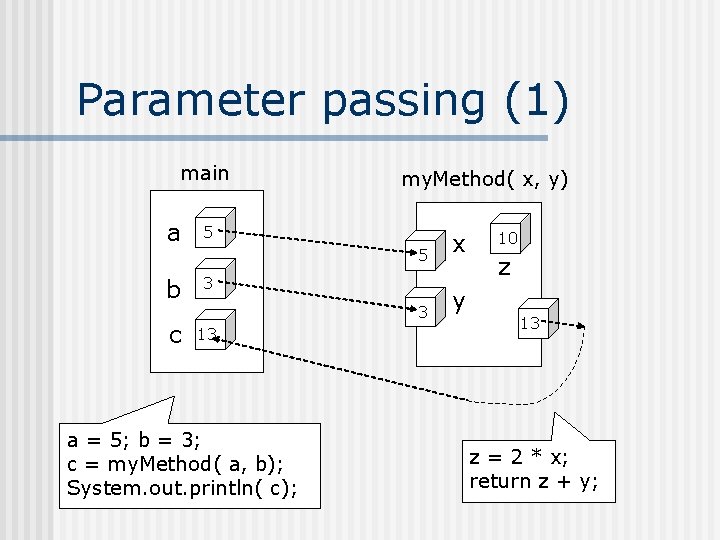
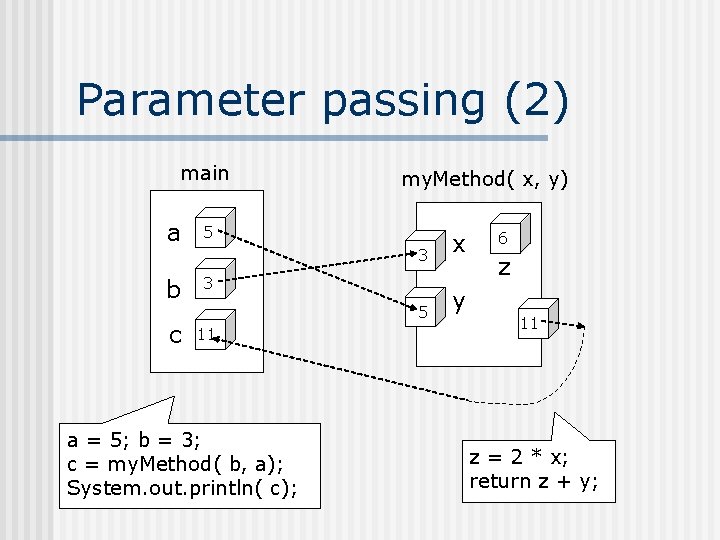
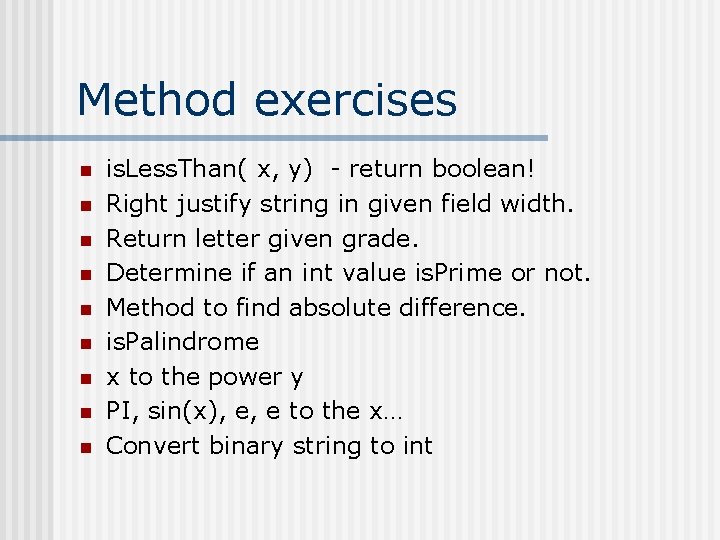
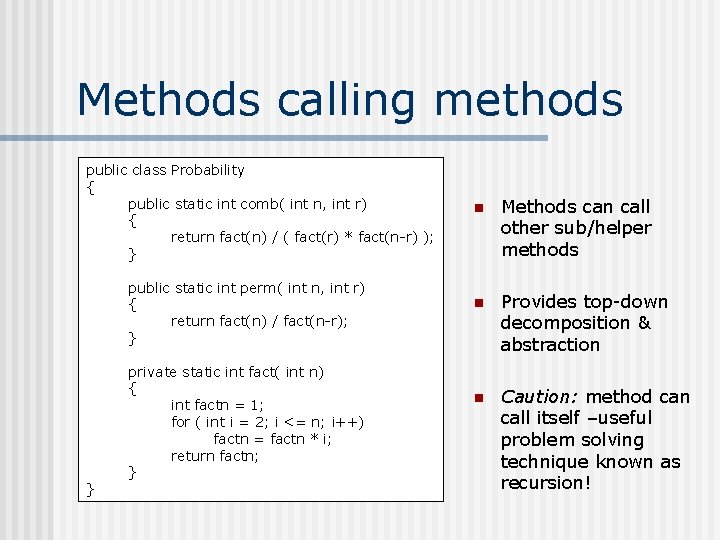
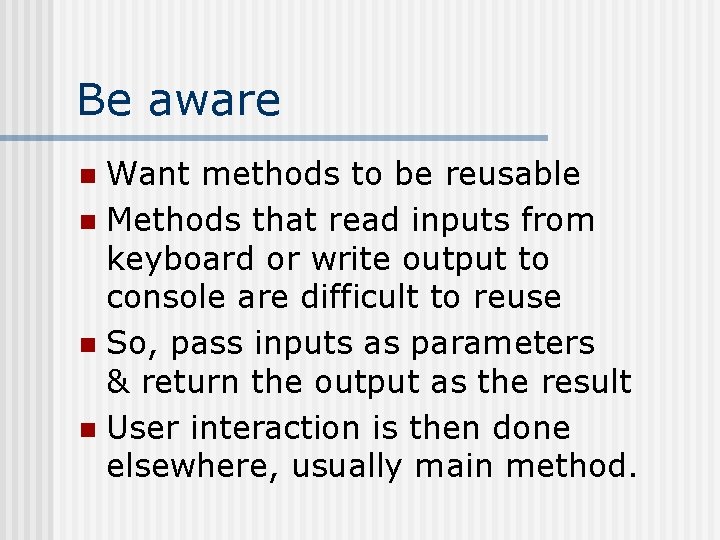
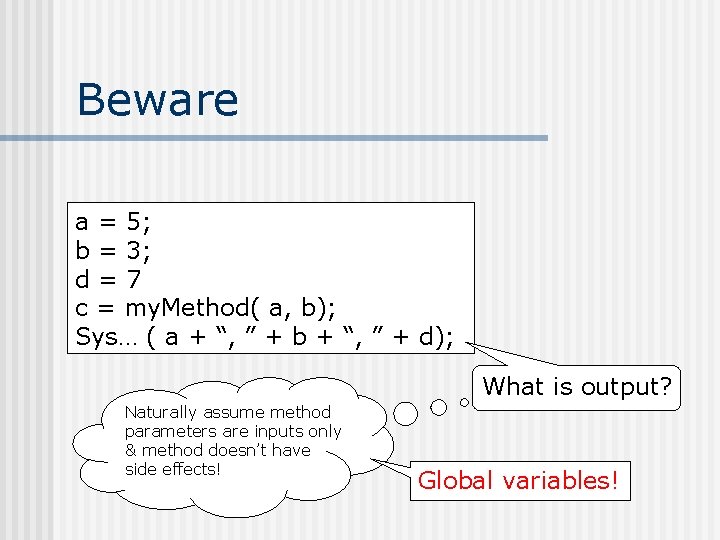
- Slides: 20
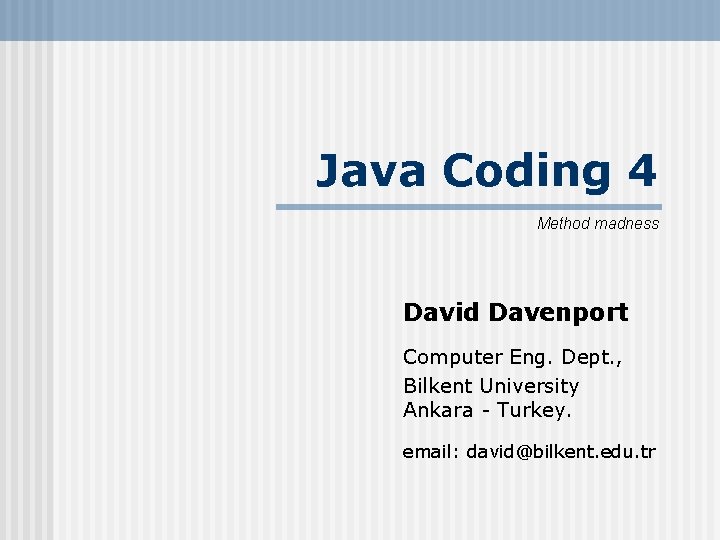
Java Coding 4 Method madness David Davenport Computer Eng. Dept. , Bilkent University Ankara - Turkey. email: david@bilkent. edu. tr
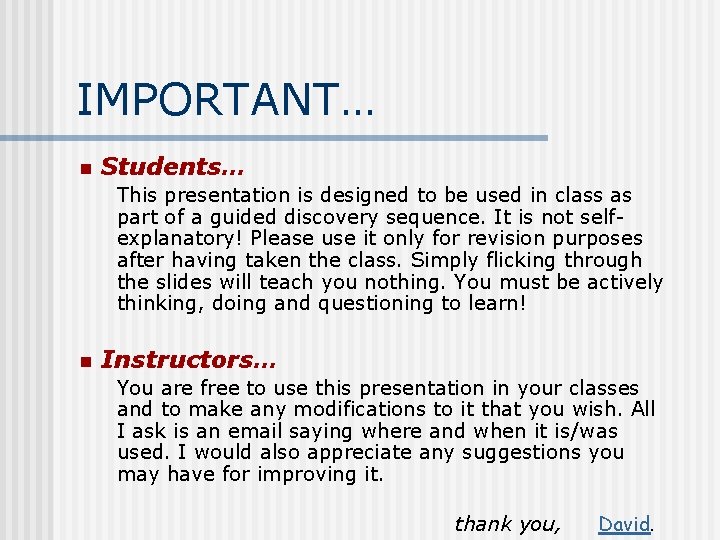
IMPORTANT… n Students… This presentation is designed to be used in class as part of a guided discovery sequence. It is not selfexplanatory! Please use it only for revision purposes after having taken the class. Simply flicking through the slides will teach you nothing. You must be actively thinking, doing and questioning to learn! n Instructors… You are free to use this presentation in your classes and to make any modifications to it that you wish. All I ask is an email saying where and when it is/was used. I would also appreciate any suggestions you may have for improving it. thank you, David.
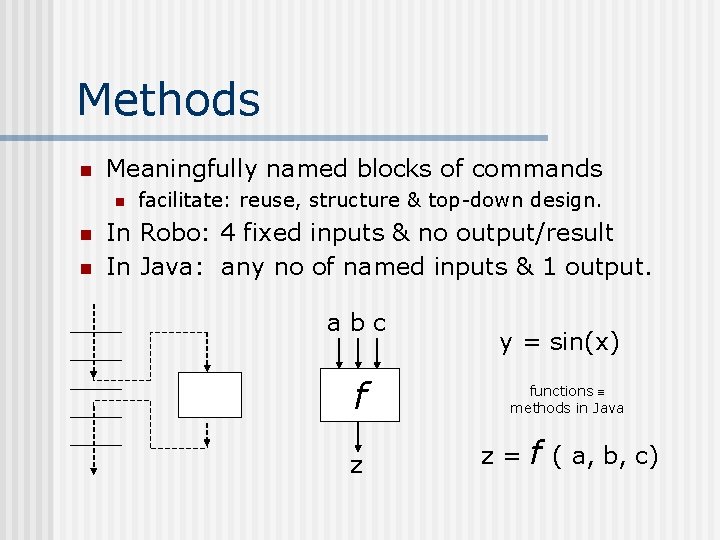
Methods n Meaningfully named blocks of commands n n n facilitate: reuse, structure & top-down design. In Robo: 4 fixed inputs & no output/result In Java: any no of named inputs & 1 output. abc f z y = sin(x) functions methods in Java z = f ( a, b, c)
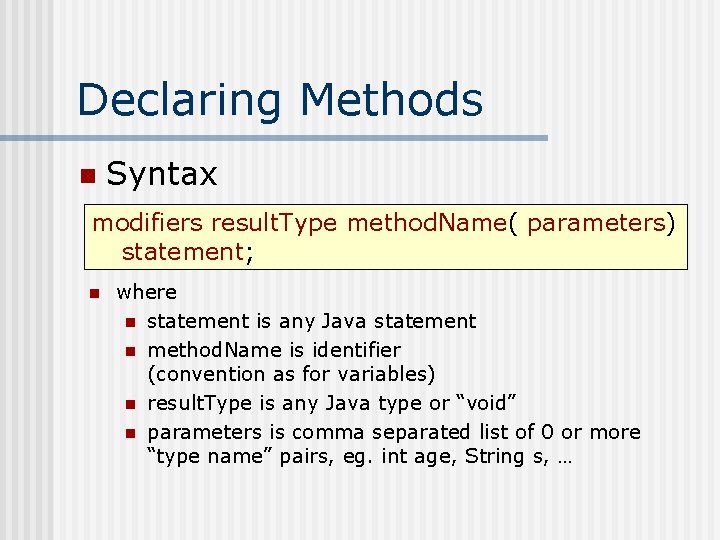
Declaring Methods n Syntax modifiers result. Type method. Name( parameters) statement; n where n statement is any Java statement n method. Name is identifier (convention as for variables) n result. Type is any Java type or “void” n parameters is comma separated list of 0 or more “type name” pairs, eg. int age, String s, …
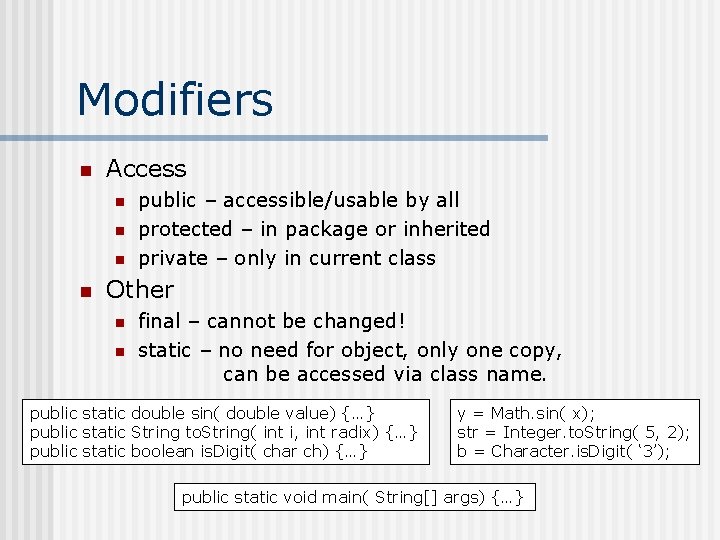
Modifiers n Access n n public – accessible/usable by all protected – in package or inherited private – only in current class Other n n final – cannot be changed! static – no need for object, only one copy, can be accessed via class name. public static double sin( double value) {…} public static String to. String( int i, int radix) {…} public static boolean is. Digit( char ch) {…} y = Math. sin( x); str = Integer. to. String( 5, 2); b = Character. is. Digit( ‘ 3’); public static void main( String[] args) {…}
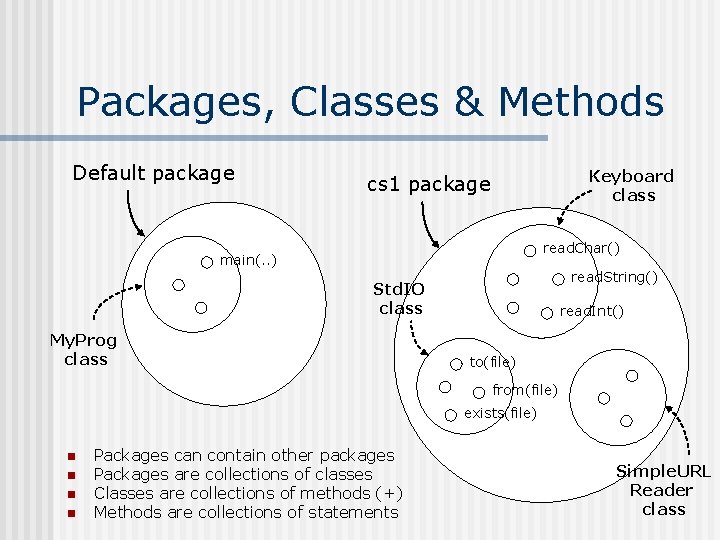
Packages, Classes & Methods Default package Keyboard class cs 1 package read. Char() main(. . ) read. String() Std. IO class My. Prog class read. Int() to(file) from(file) exists(file) n n Packages can contain other packages Packages are collections of classes Classes are collections of methods (+) Methods are collections of statements Simple. URL Reader class
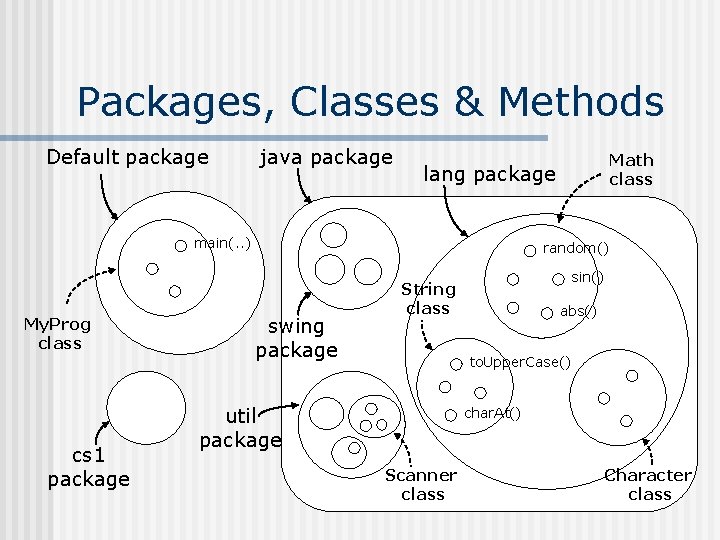
Packages, Classes & Methods Default package java package lang package main(. . ) My. Prog class cs 1 package Math class random() swing package sin() String class abs() to. Upper. Case() util package char. At() Scanner class Character class
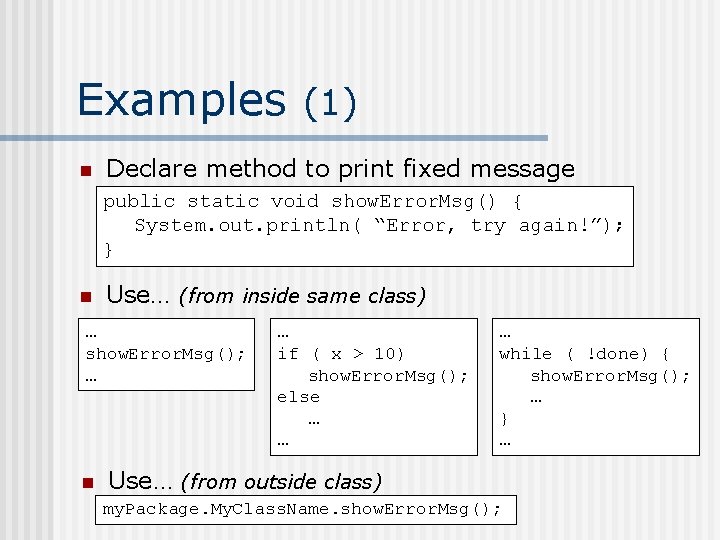
Examples (1) n Declare method to print fixed message public static void show. Error. Msg() { System. out. println( “Error, try again!”); } n Use… (from inside same class) … show. Error. Msg(); … n … if ( x > 10) show. Error. Msg(); else … … … while ( !done) { show. Error. Msg(); … } … Use… (from outside class) my. Package. My. Class. Name. show. Error. Msg();
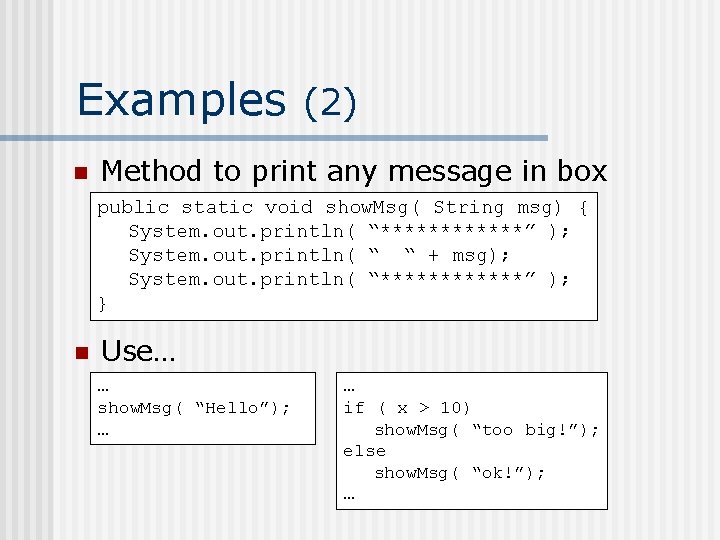
Examples (2) n Method to print any message in box public static void show. Msg( String msg) { System. out. println( “******” ); System. out. println( “ “ + msg); System. out. println( “******” ); } n Use… … show. Msg( “Hello”); … … if ( x > 10) show. Msg( “too big!”); else show. Msg( “ok!”); …
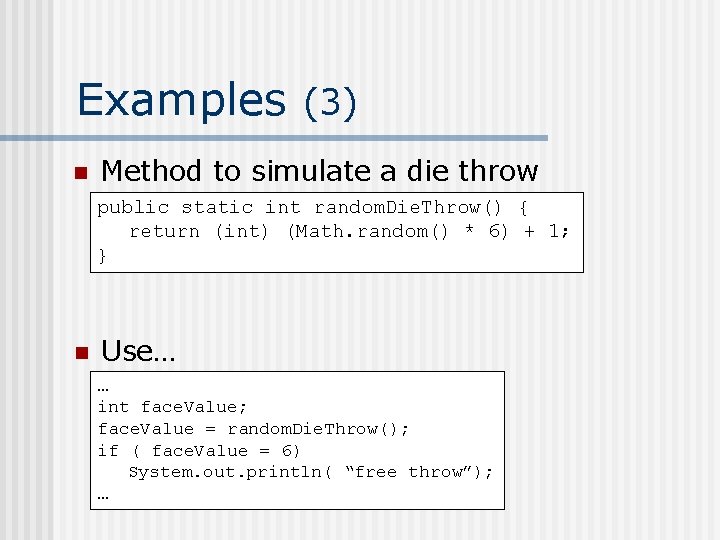
Examples (3) n Method to simulate a die throw public static int random. Die. Throw() { return (int) (Math. random() * 6) + 1; } n Use… … int face. Value; face. Value = random. Die. Throw(); if ( face. Value = 6) System. out. println( “free throw”); …
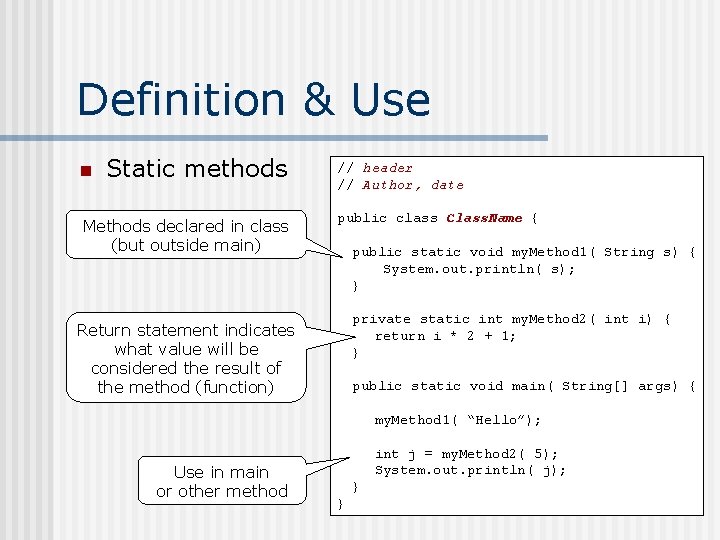
Definition & Use n Static methods Methods declared in class (but outside main) // header // Author, date public class Class. Name { public static void my. Method 1( String s) { System. out. println( s); } private static int my. Method 2( int i) { return i * 2 + 1; } Return statement indicates what value will be considered the result of the method (function) public static void main( String[] args) { my. Method 1( “Hello”); Use in main or other method int j = my. Method 2( 5); System. out. println( j); } }
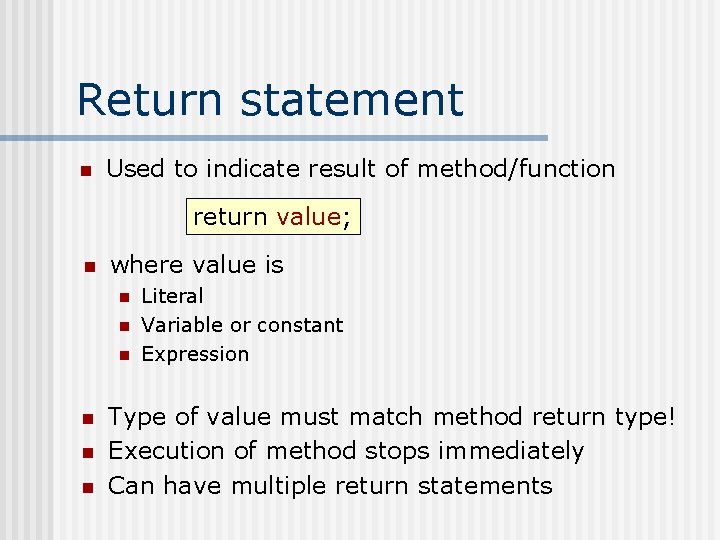
Return statement n Used to indicate result of method/function return value; n where value is n n n Literal Variable or constant Expression Type of value must match method return type! Execution of method stops immediately Can have multiple return statements
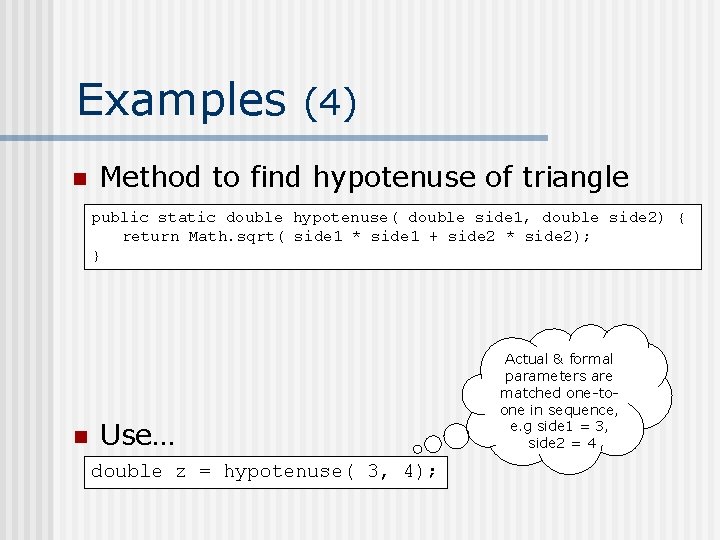
Examples (4) n Method to find hypotenuse of triangle public static double hypotenuse( double side 1, double side 2) { return Math. sqrt( side 1 * side 1 + side 2 * side 2); } n Use… double z = hypotenuse( 3, 4); Actual & formal parameters are matched one-toone in sequence, e. g side 1 = 3, side 2 = 4
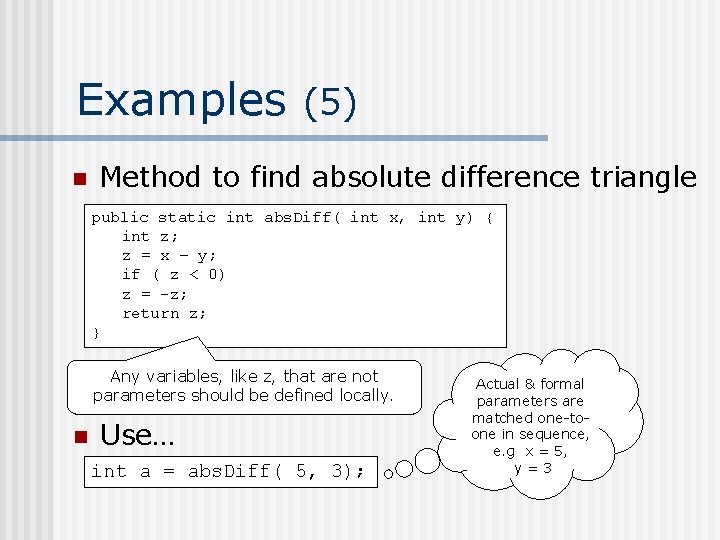
Examples (5) n Method to find absolute difference triangle public static int abs. Diff( int x, int y) { int z; z = x – y; if ( z < 0) z = -z; return z; } Any variables, like z, that are not parameters should be defined locally. n Use… int a = abs. Diff( 5, 3); Actual & formal parameters are matched one-toone in sequence, e. g x = 5, y = 3
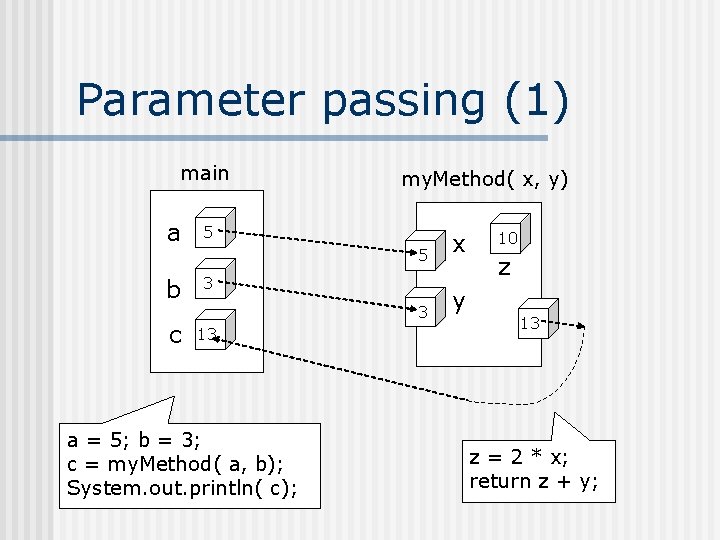
Parameter passing (1) main a my. Method( x, y) 5 b 3 c 13 a = 5; b = 3; c = my. Method( a, b); System. out. println( c); 5 x 3 y 10 z 13 z = 2 * x; return z + y;
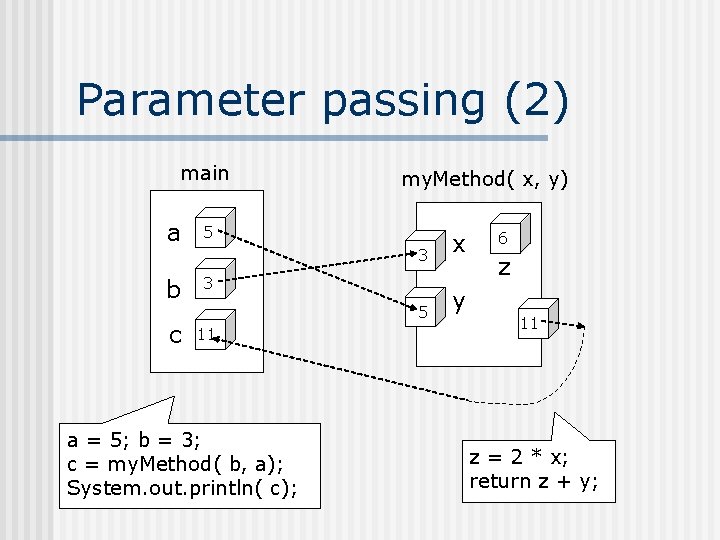
Parameter passing (2) main a my. Method( x, y) 5 b 3 c 11 a = 5; b = 3; c = my. Method( b, a); System. out. println( c); 3 x 5 y 6 z 11 z = 2 * x; return z + y;
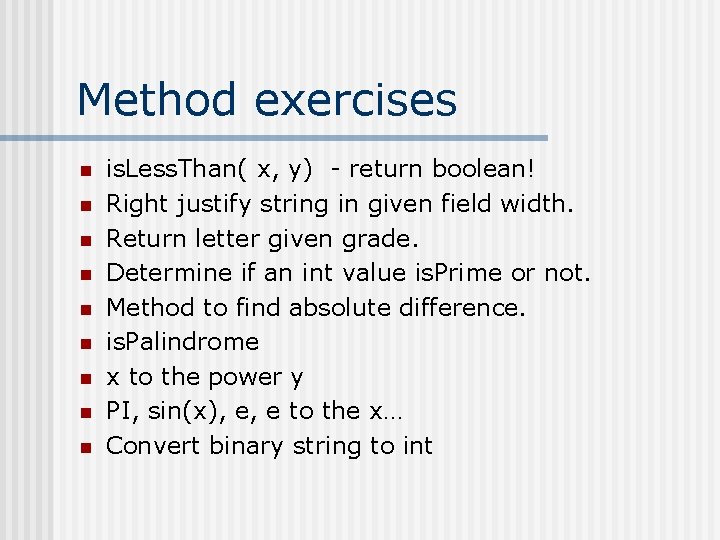
Method exercises n n n n n is. Less. Than( x, y) - return boolean! Right justify string in given field width. Return letter given grade. Determine if an int value is. Prime or not. Method to find absolute difference. is. Palindrome x to the power y PI, sin(x), e, e to the x… Convert binary string to int
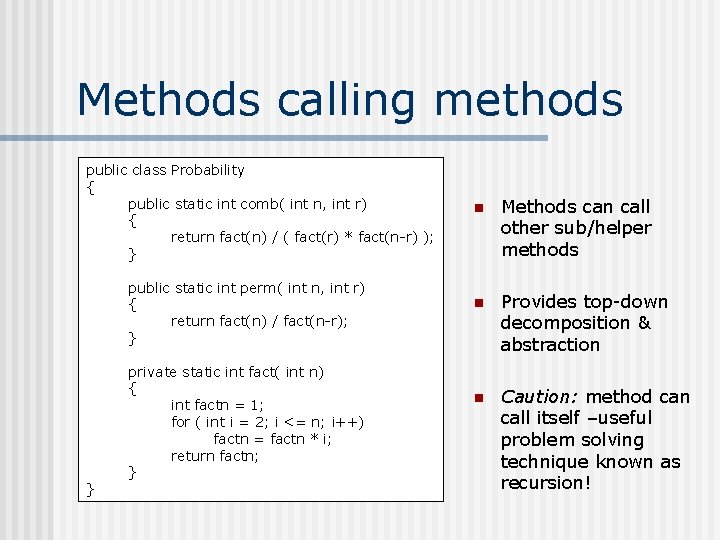
Methods calling methods public class Probability { public static int comb( int n, int r) { return fact(n) / ( fact(r) * fact(n-r) ); } } public static int perm( int n, int r) { return fact(n) / fact(n-r); } private static int fact( int n) { int factn = 1; for ( int i = 2; i <= n; i++) factn = factn * i; return factn; } n Methods can call other sub/helper methods n Provides top-down decomposition & abstraction n Caution: method can call itself –useful problem solving technique known as recursion!
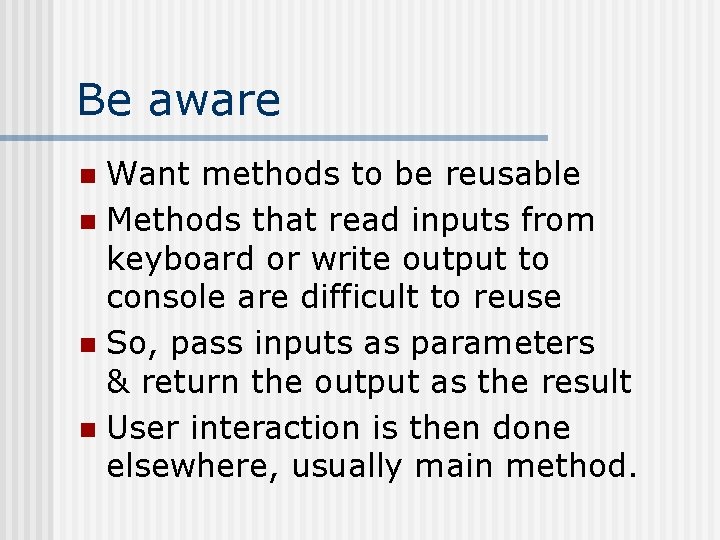
Be aware Want methods to be reusable n Methods that read inputs from keyboard or write output to console are difficult to reuse n So, pass inputs as parameters & return the output as the result n User interaction is then done elsewhere, usually main method. n
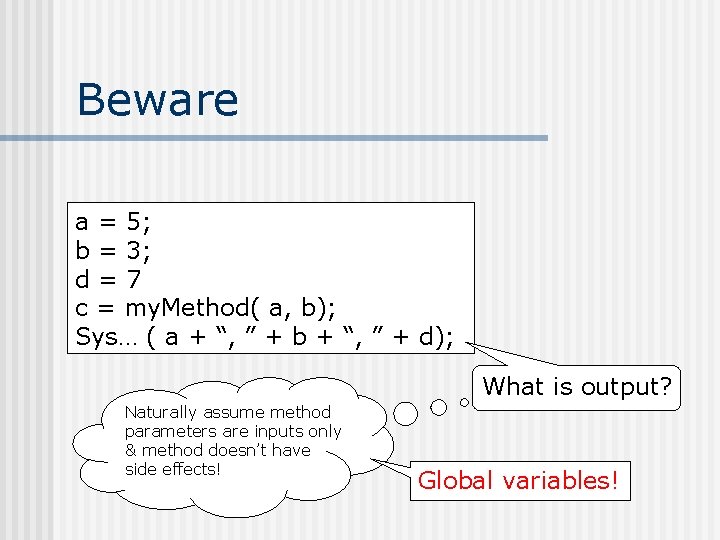
Beware a = 5; b = 3; d = 7 c = my. Method( a, b); Sys… ( a + “, ” + b + “, ” + d); What is output? Naturally assume method parameters are inputs only & method doesn’t have side effects! Global variables!
Apa itu open coding
Contoh selective coding
David davenport bilkent
David davenport bilkent
David davenport bilkent
History of qualitative research
Coding dna and non coding dna
Goethe hamlet
Davenport diagramme
Diagramme de davenport
Homer davenport saltville va
Purina davenport iowa
Dr craig davenport
Dr michelle davenport
Escp
Leon davenport
Mark a. davenport
Davenport v cotton hope plantation
Java secure coding
Playground markup
Java code review checklist pdf