Interface Ashima Wadhwa Interface An Interface is a
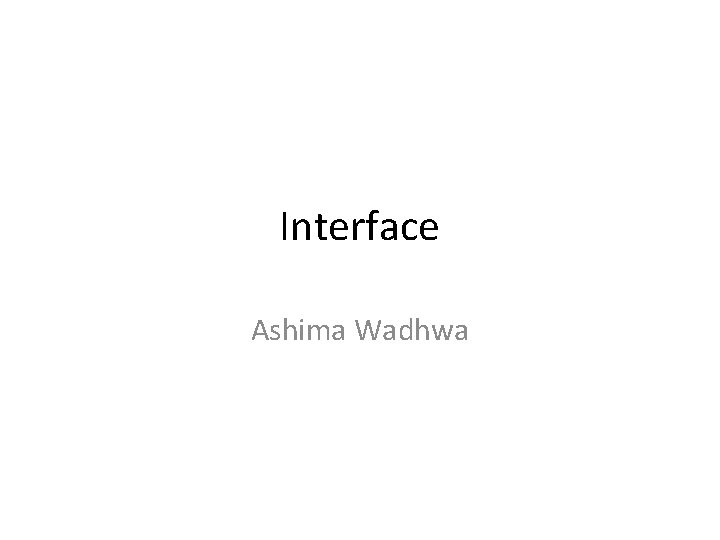
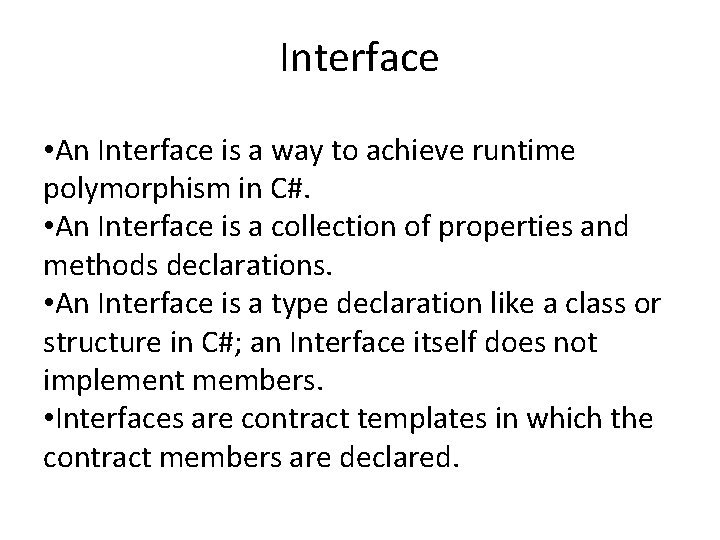
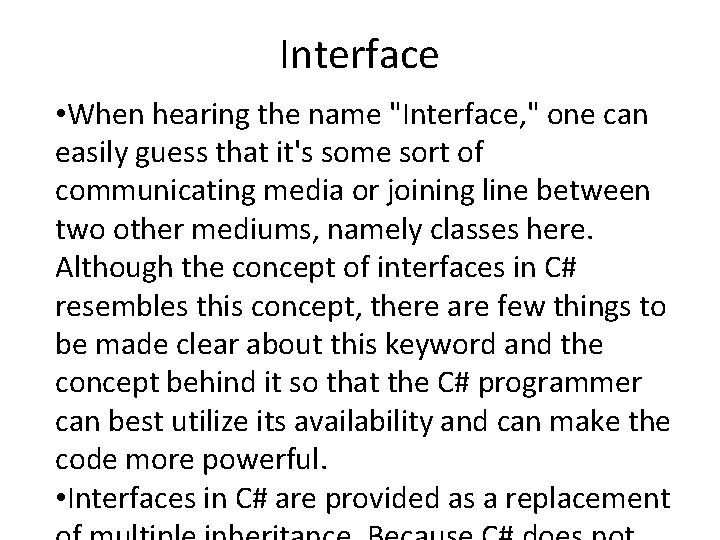
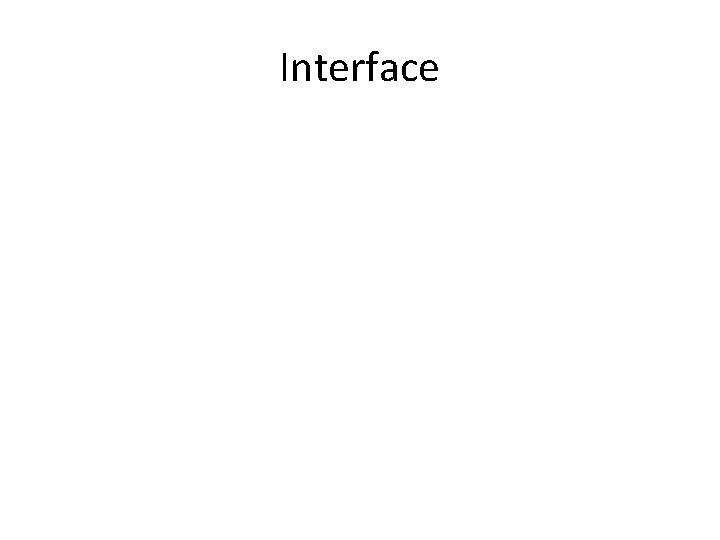
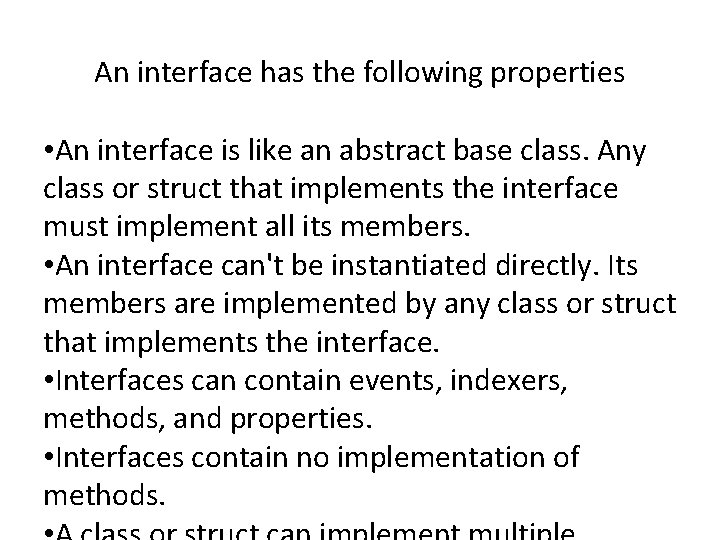
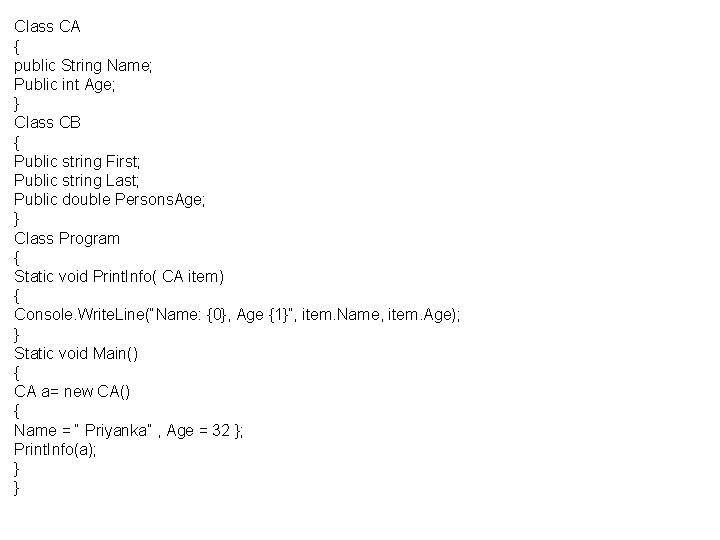
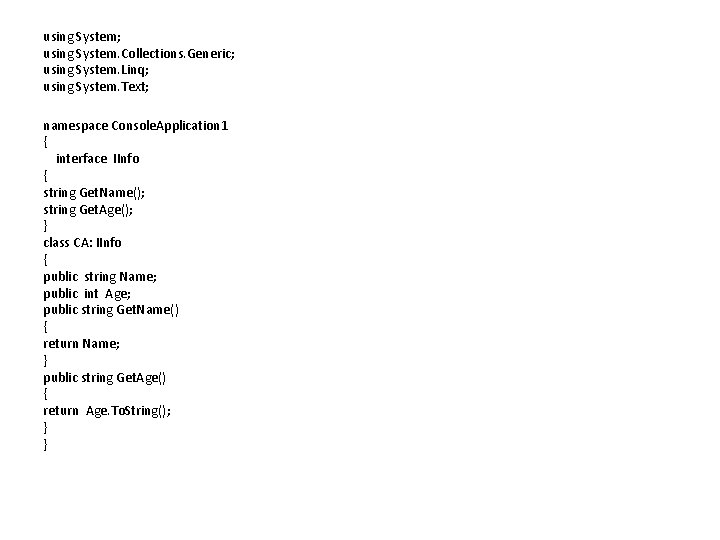
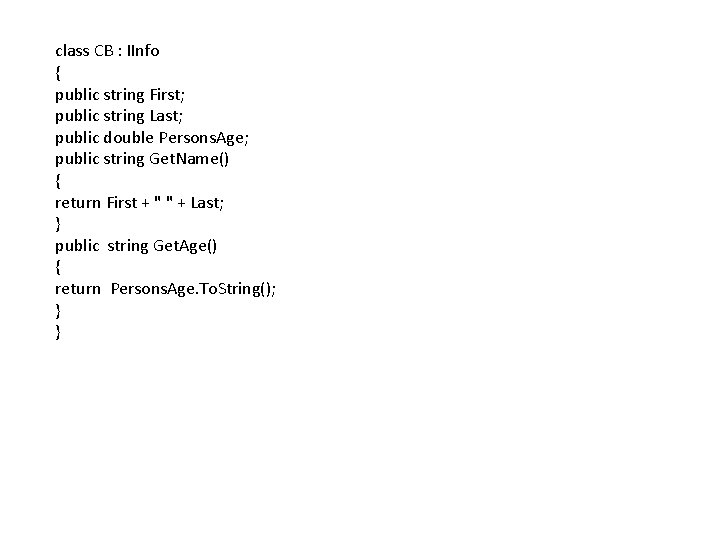
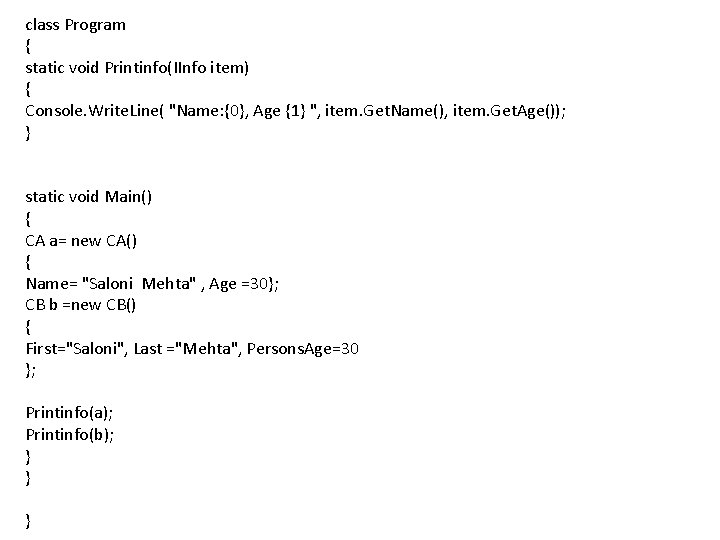
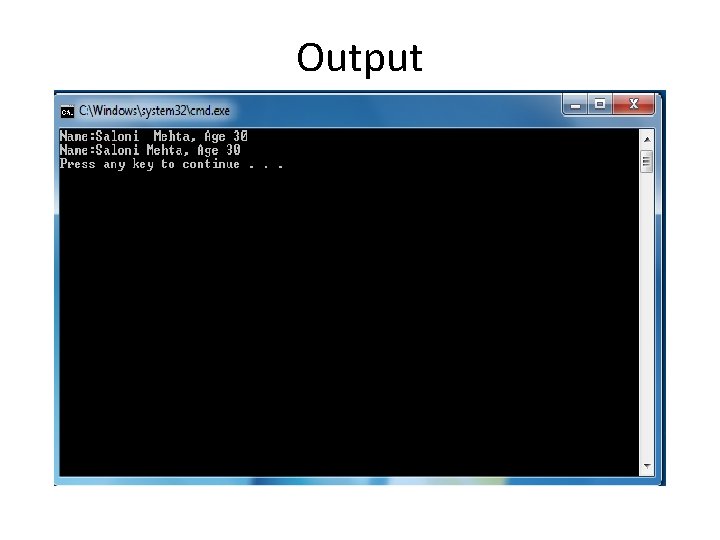
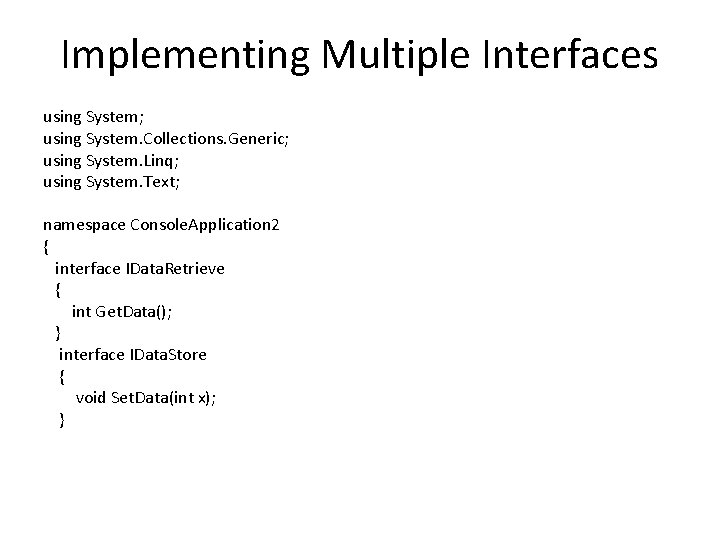
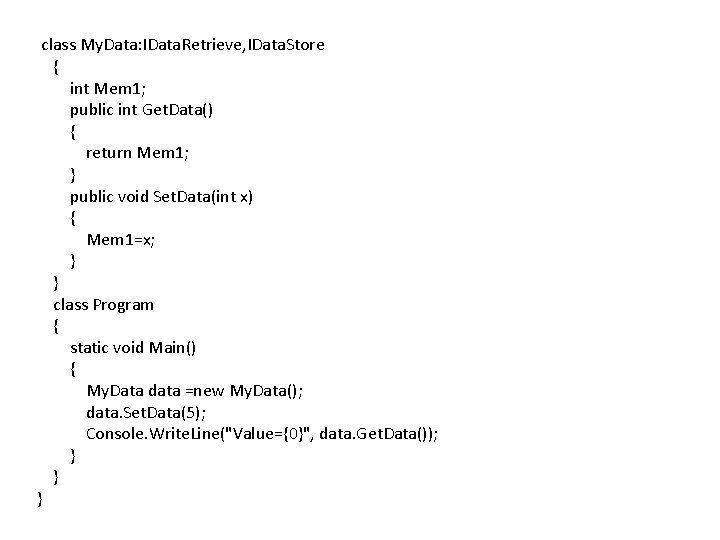
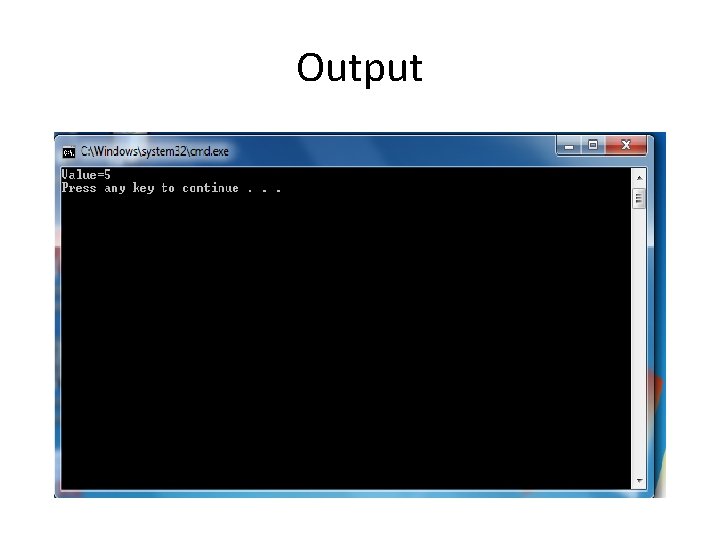
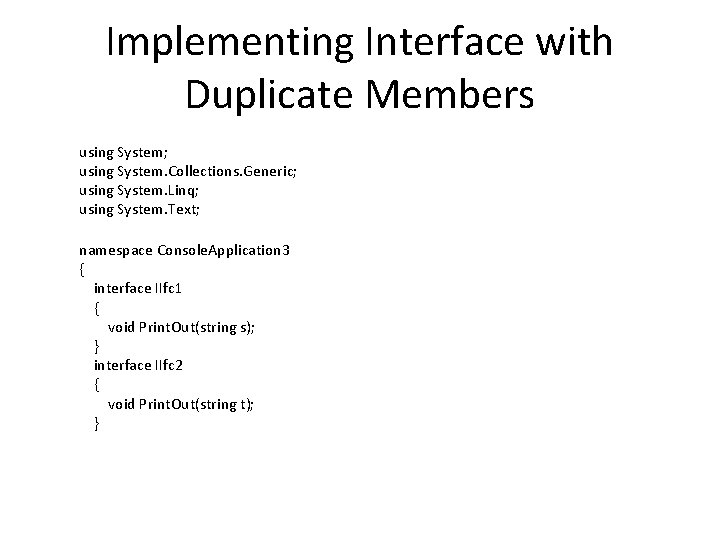
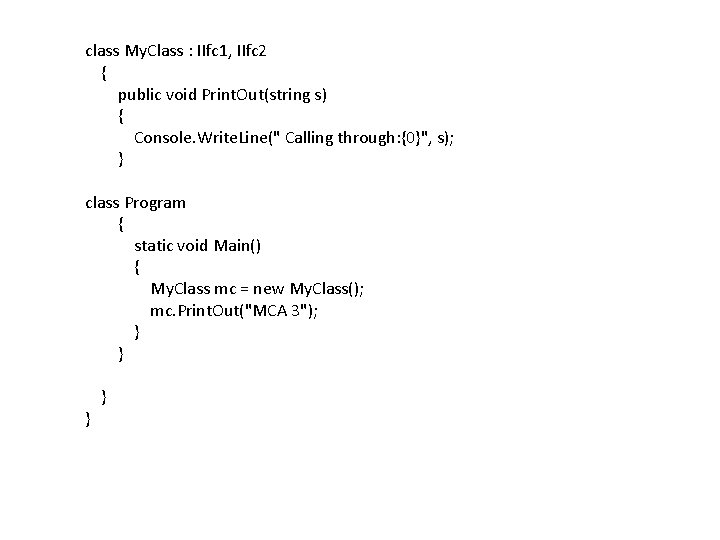
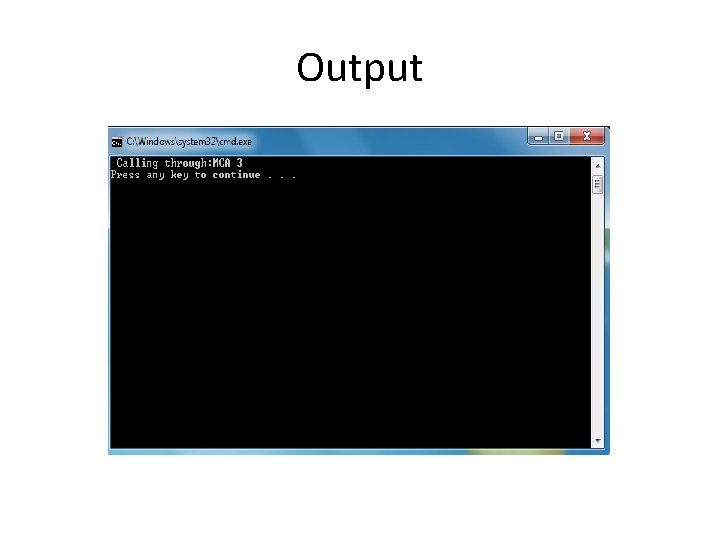
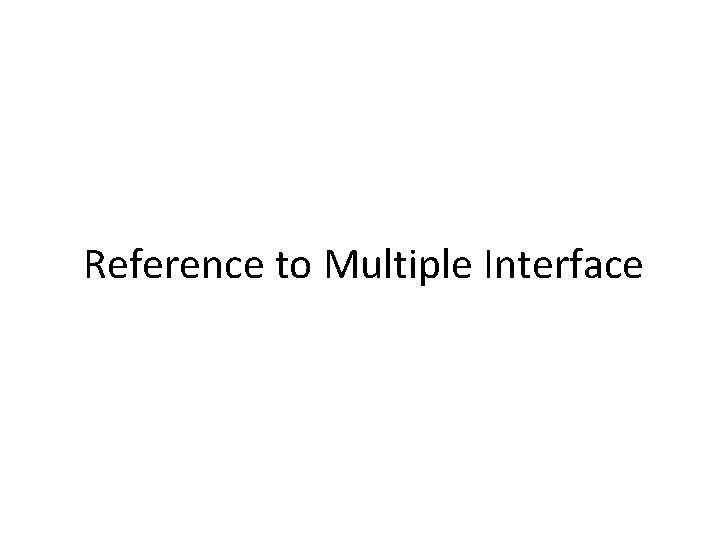
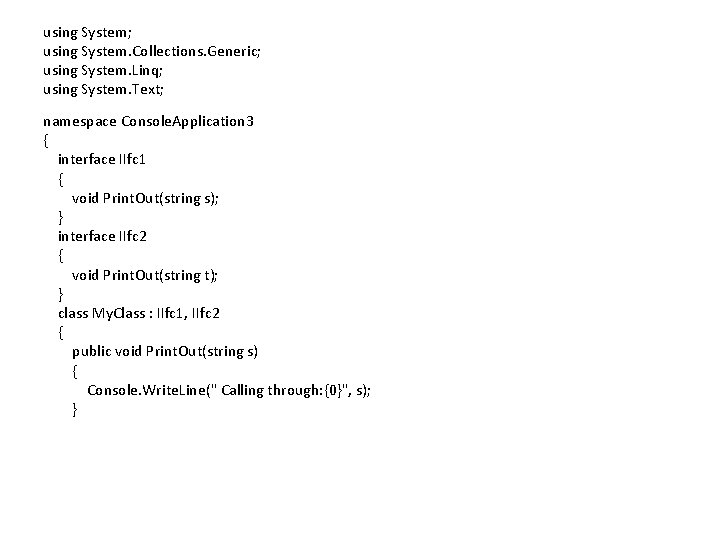
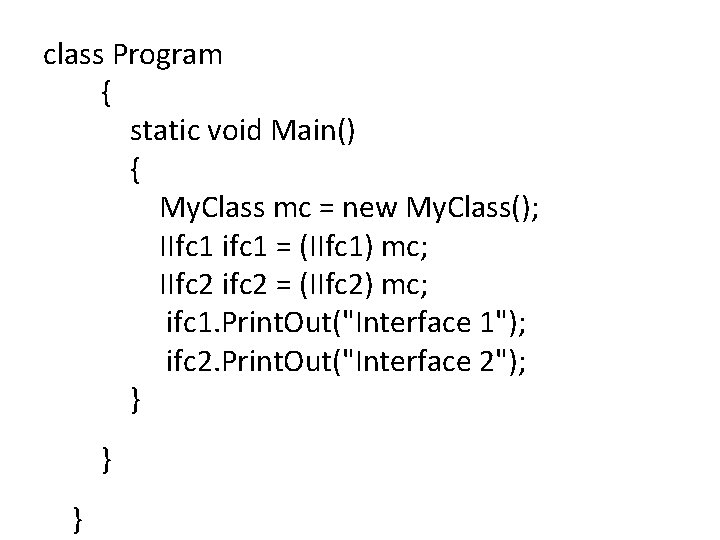
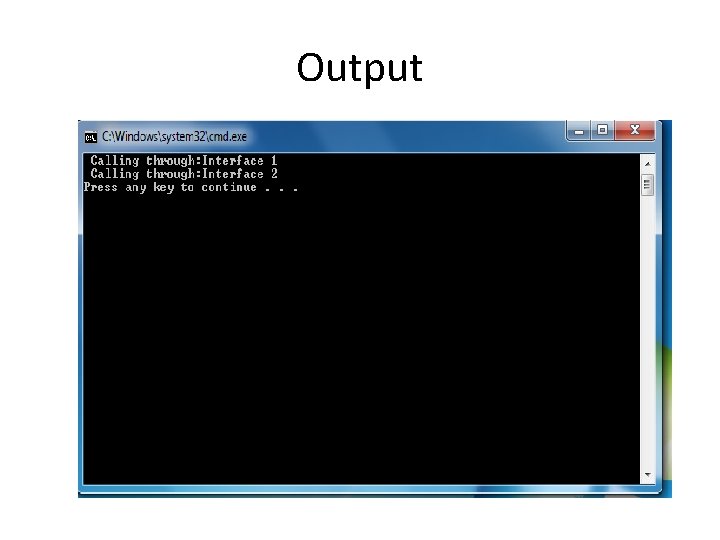
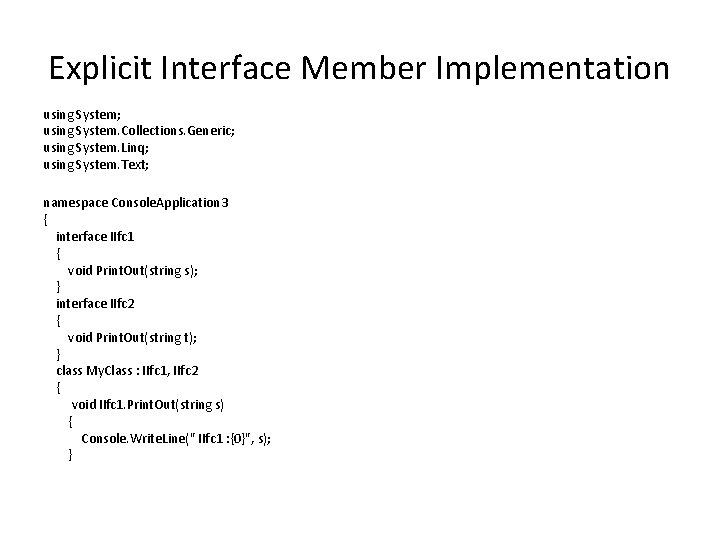
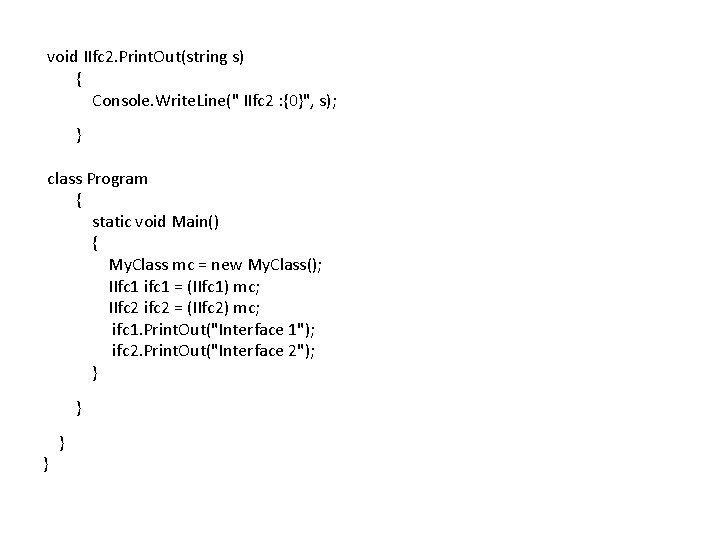
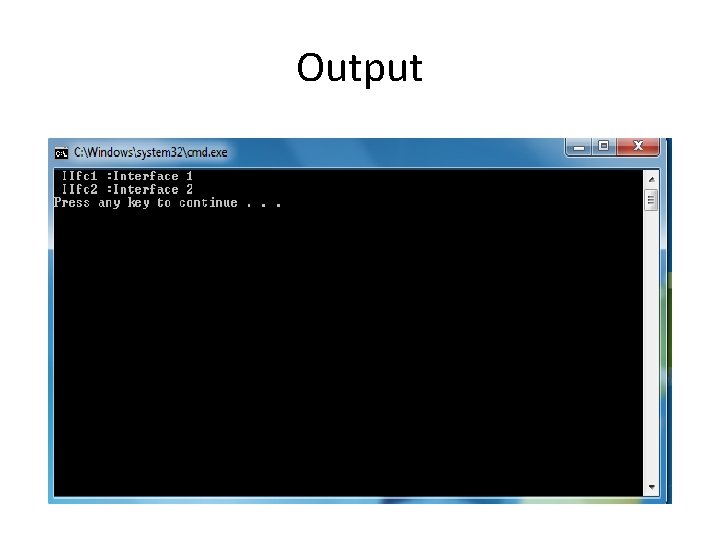
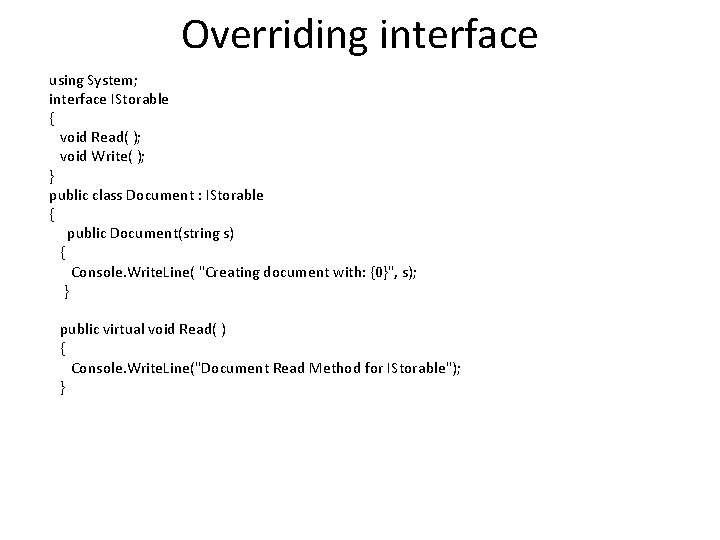
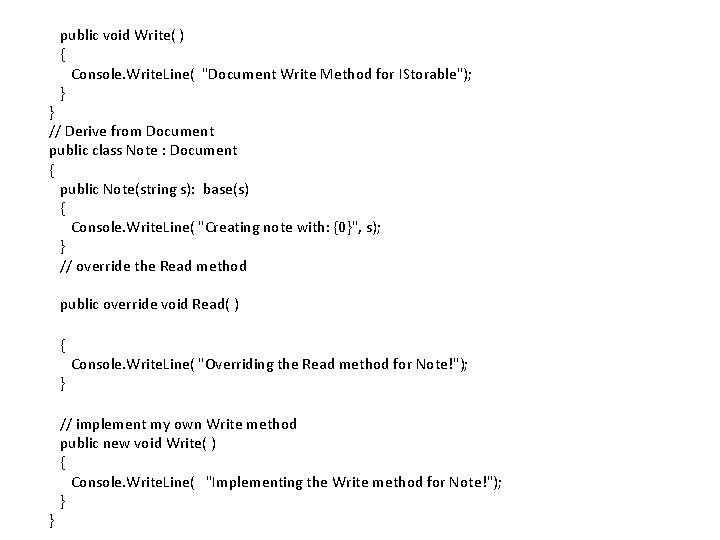
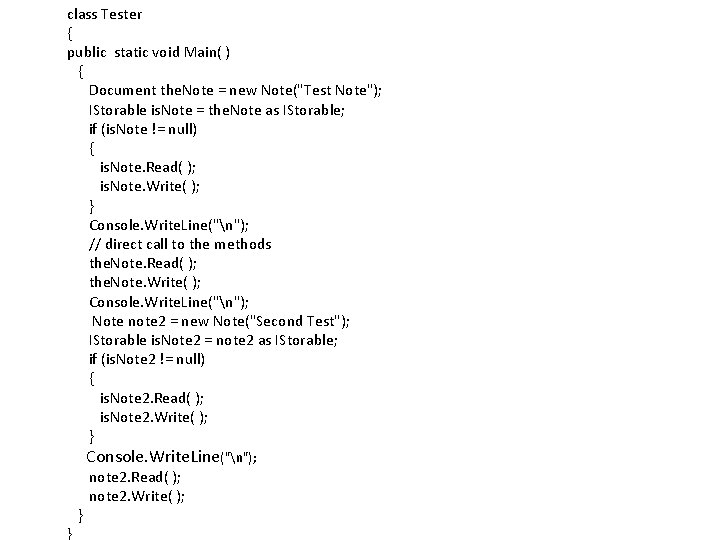
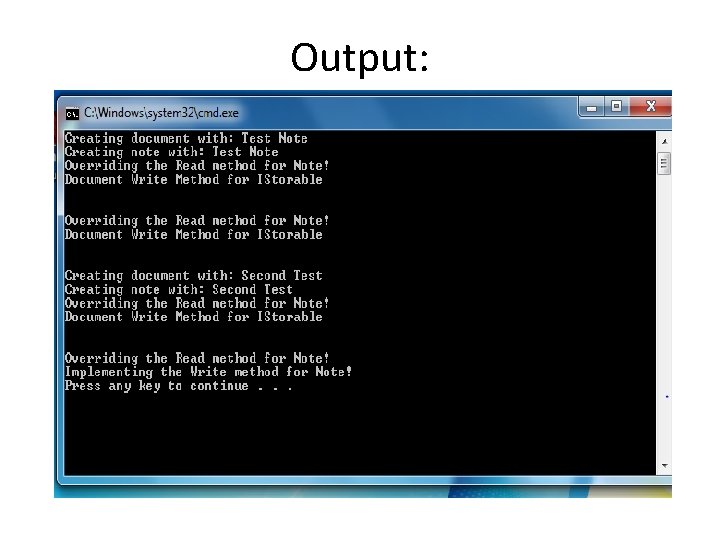
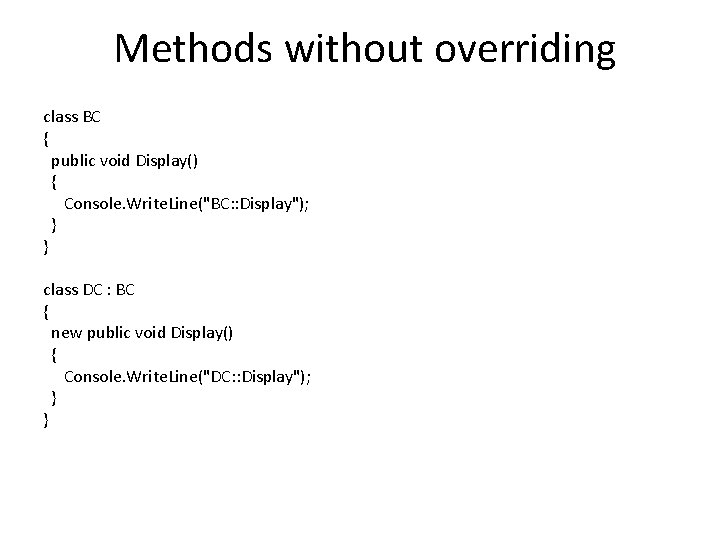
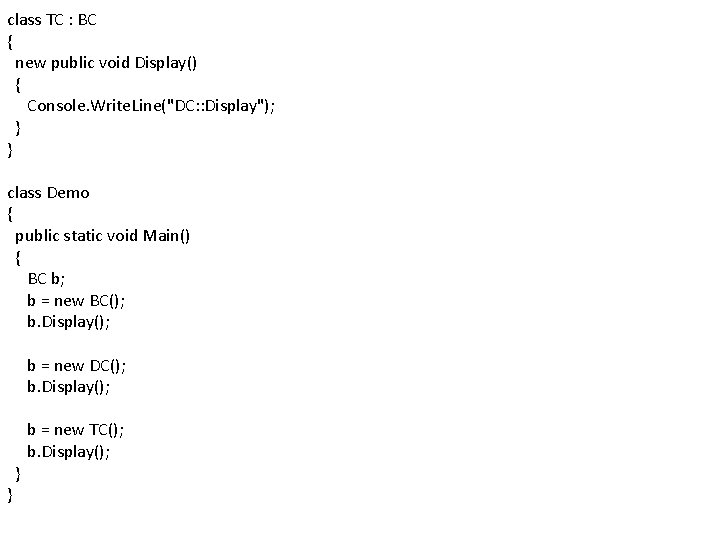
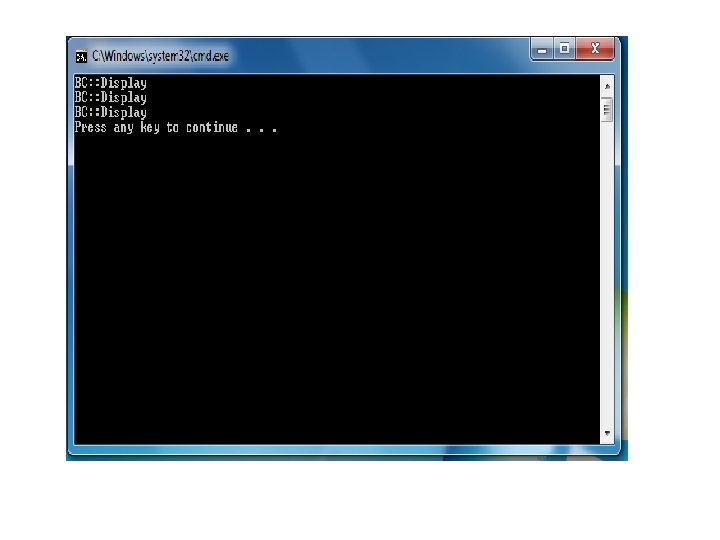
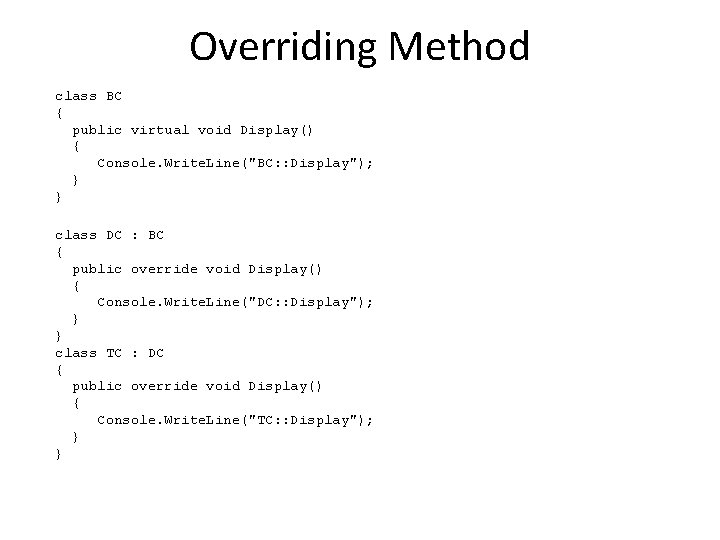
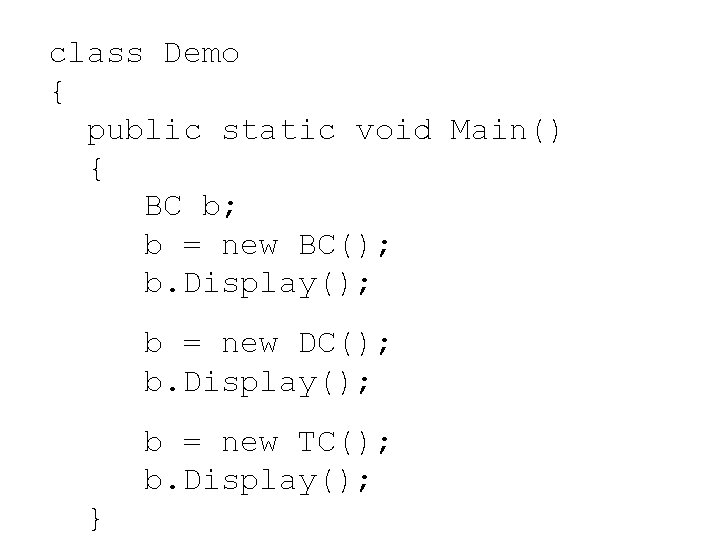
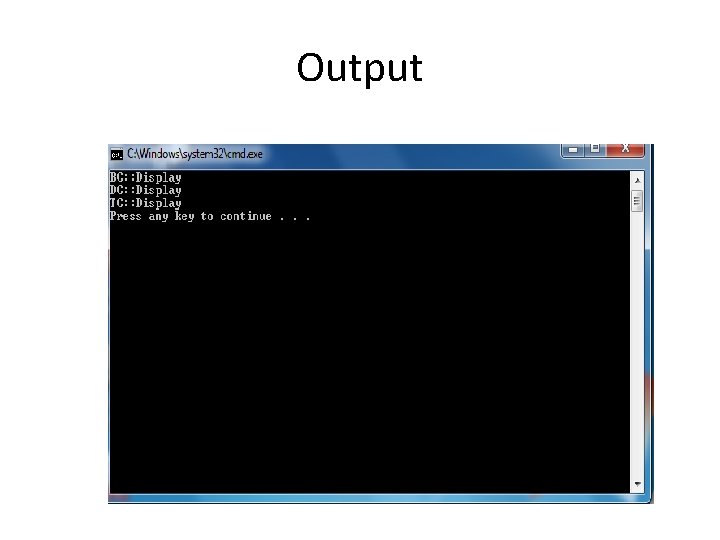
- Slides: 33
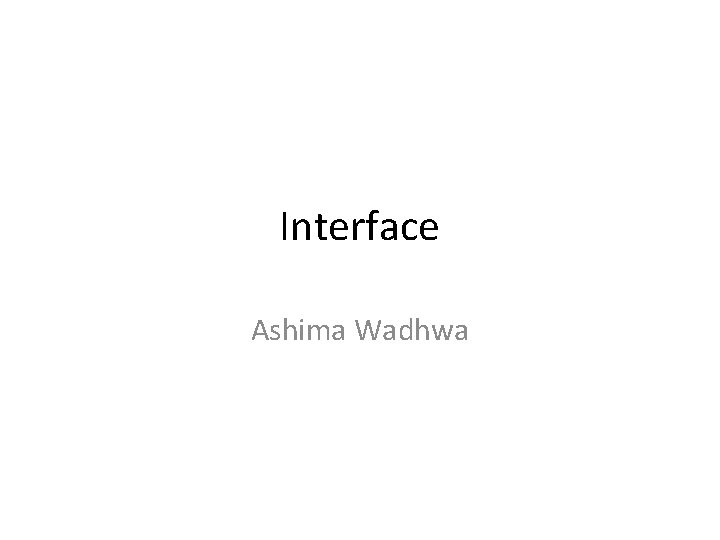
Interface Ashima Wadhwa
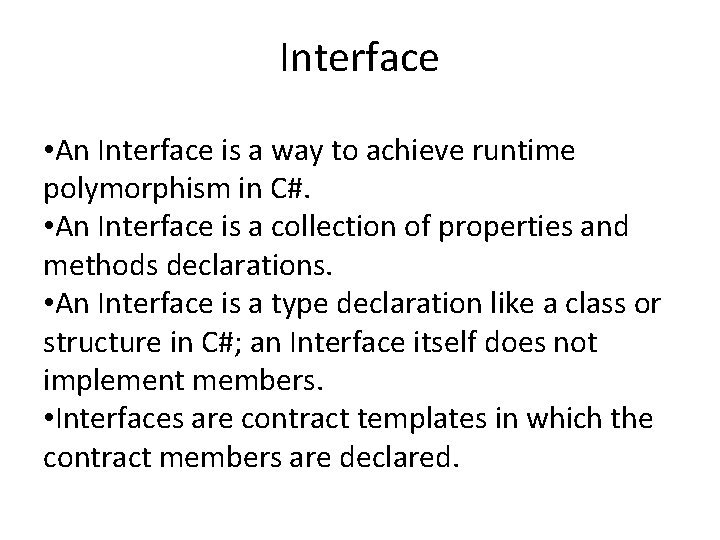
Interface • An Interface is a way to achieve runtime polymorphism in C#. • An Interface is a collection of properties and methods declarations. • An Interface is a type declaration like a class or structure in C#; an Interface itself does not implement members. • Interfaces are contract templates in which the contract members are declared.
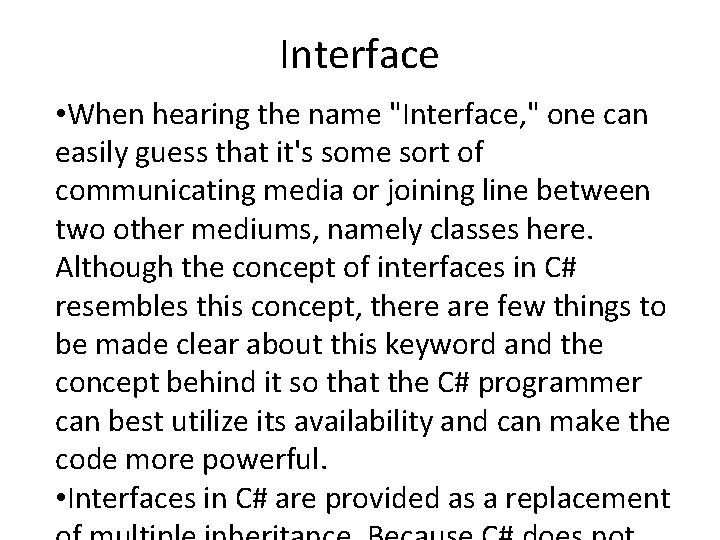
Interface • When hearing the name "Interface, " one can easily guess that it's some sort of communicating media or joining line between two other mediums, namely classes here. Although the concept of interfaces in C# resembles this concept, there are few things to be made clear about this keyword and the concept behind it so that the C# programmer can best utilize its availability and can make the code more powerful. • Interfaces in C# are provided as a replacement
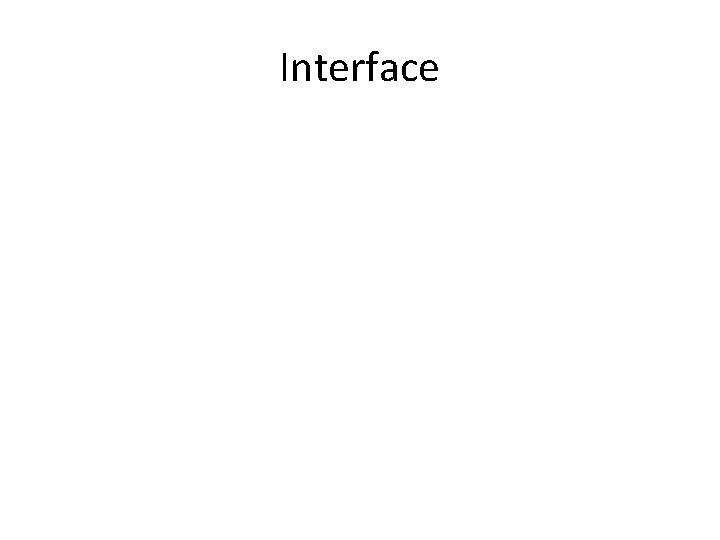
Interface
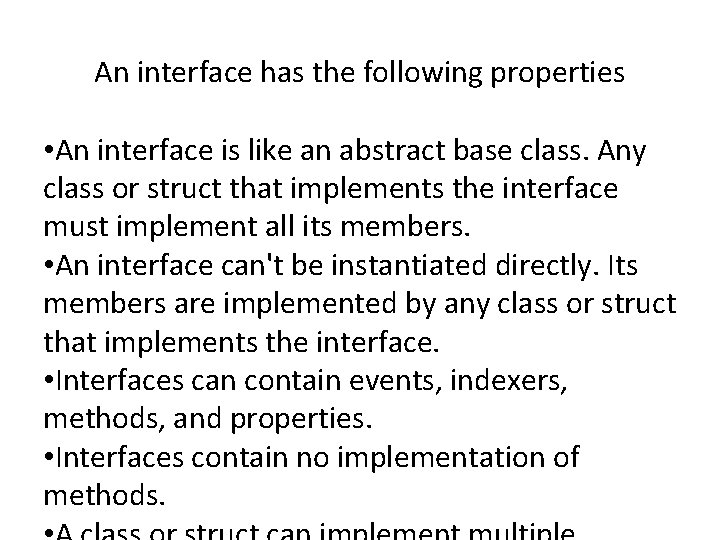
An interface has the following properties • An interface is like an abstract base class. Any class or struct that implements the interface must implement all its members. • An interface can't be instantiated directly. Its members are implemented by any class or struct that implements the interface. • Interfaces can contain events, indexers, methods, and properties. • Interfaces contain no implementation of methods.
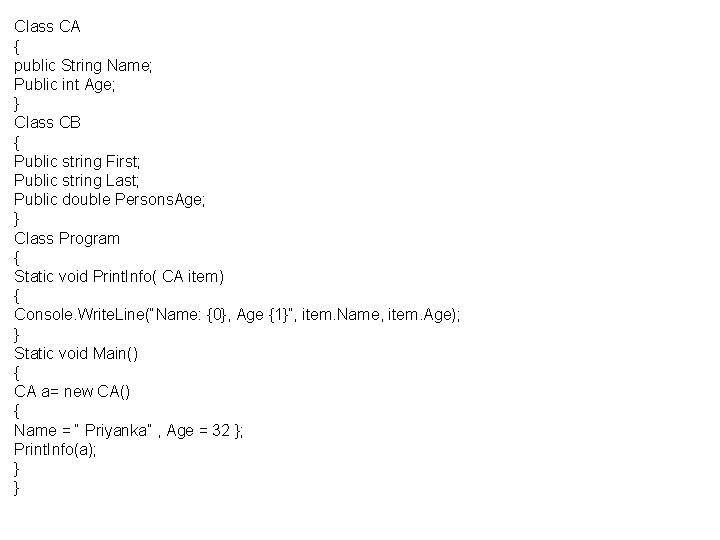
Class CA { public String Name; Public int Age; } Class CB { Public string First; Public string Last; Public double Persons. Age; } Class Program { Static void Print. Info( CA item) { Console. Write. Line(“Name: {0}, Age {1}”, item. Name, item. Age); } Static void Main() { CA a= new CA() { Name = “ Priyanka” , Age = 32 }; Print. Info(a); } }
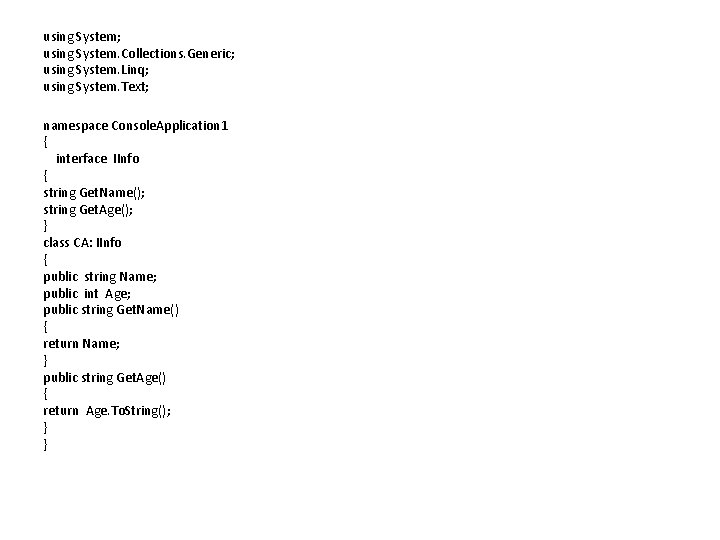
using System; using System. Collections. Generic; using System. Linq; using System. Text; namespace Console. Application 1 { interface IInfo { string Get. Name(); string Get. Age(); } class CA: IInfo { public string Name; public int Age; public string Get. Name() { return Name; } public string Get. Age() { return Age. To. String(); } }
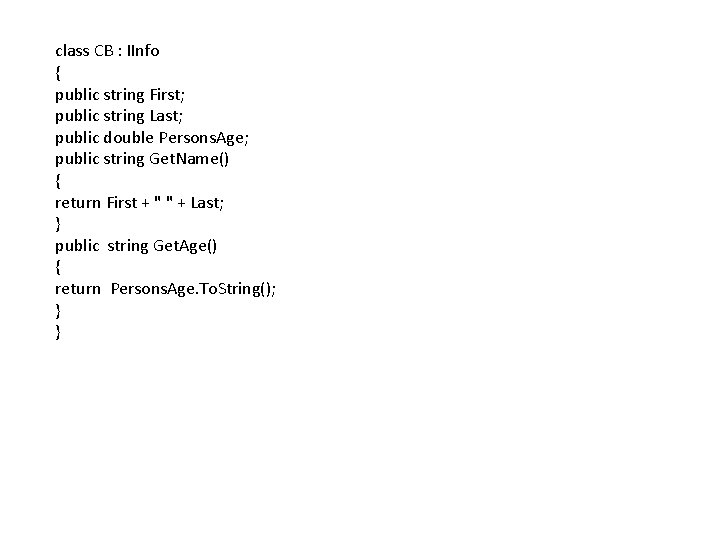
class CB : IInfo { public string First; public string Last; public double Persons. Age; public string Get. Name() { return First + " " + Last; } public string Get. Age() { return Persons. Age. To. String(); } }
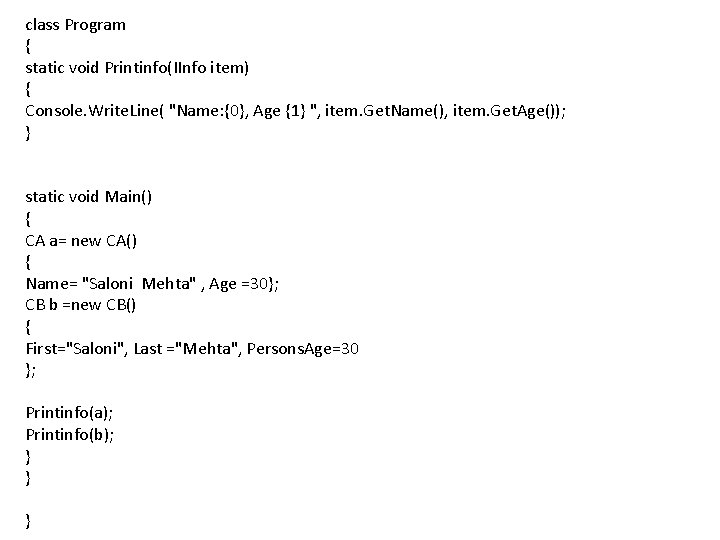
class Program { static void Printinfo(IInfo item) { Console. Write. Line( "Name: {0}, Age {1} ", item. Get. Name(), item. Get. Age()); } static void Main() { CA a= new CA() { Name= "Saloni Mehta" , Age =30}; CB b =new CB() { First="Saloni", Last ="Mehta", Persons. Age=30 }; Printinfo(a); Printinfo(b); } } }
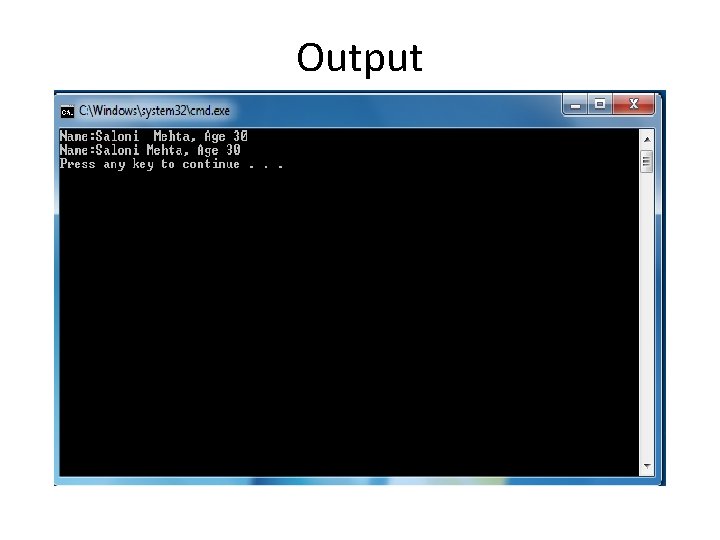
Output
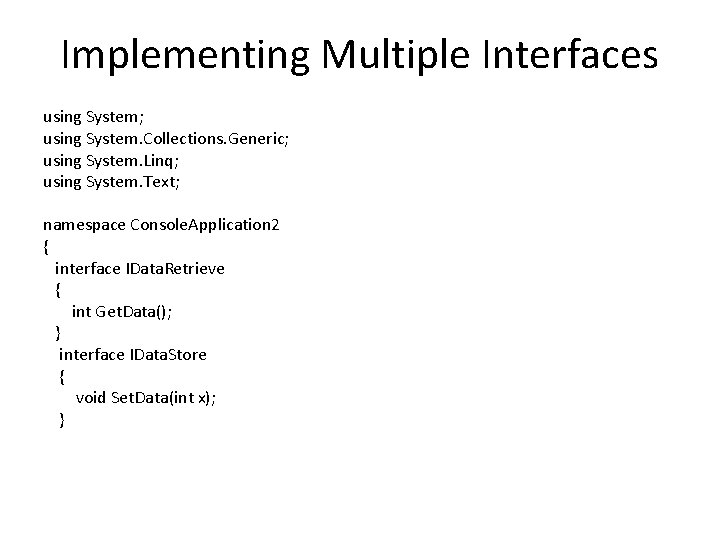
Implementing Multiple Interfaces using System; using System. Collections. Generic; using System. Linq; using System. Text; namespace Console. Application 2 { interface IData. Retrieve { int Get. Data(); } interface IData. Store { void Set. Data(int x); }
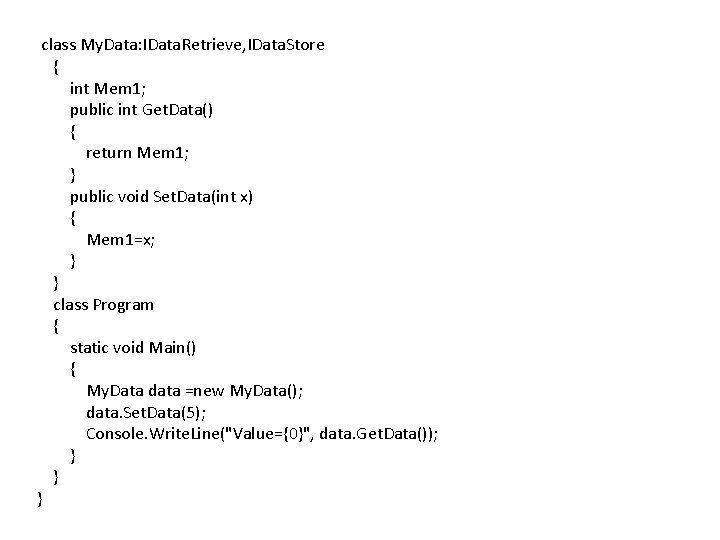
class My. Data: IData. Retrieve, IData. Store { int Mem 1; public int Get. Data() { return Mem 1; } public void Set. Data(int x) { Mem 1=x; } } class Program { static void Main() { My. Data data =new My. Data(); data. Set. Data(5); Console. Write. Line("Value={0}", data. Get. Data()); } } }
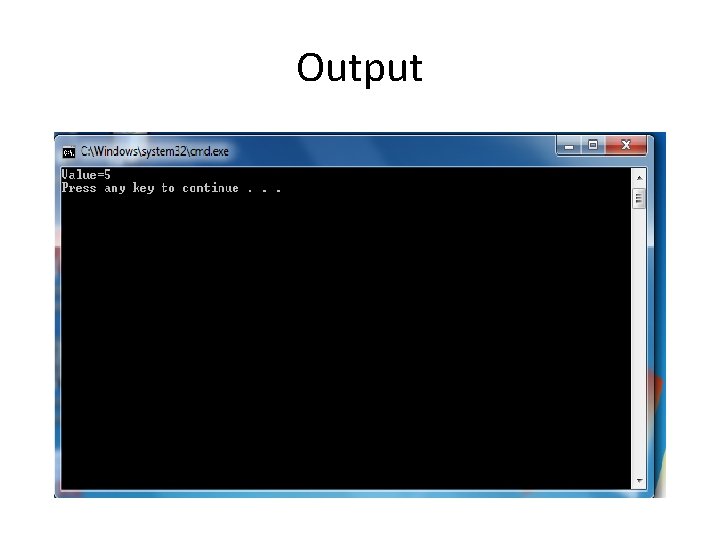
Output
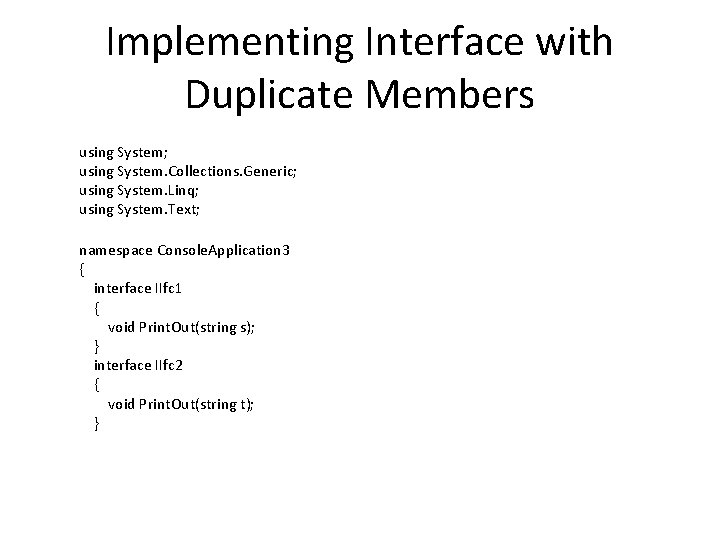
Implementing Interface with Duplicate Members using System; using System. Collections. Generic; using System. Linq; using System. Text; namespace Console. Application 3 { interface IIfc 1 { void Print. Out(string s); } interface IIfc 2 { void Print. Out(string t); }
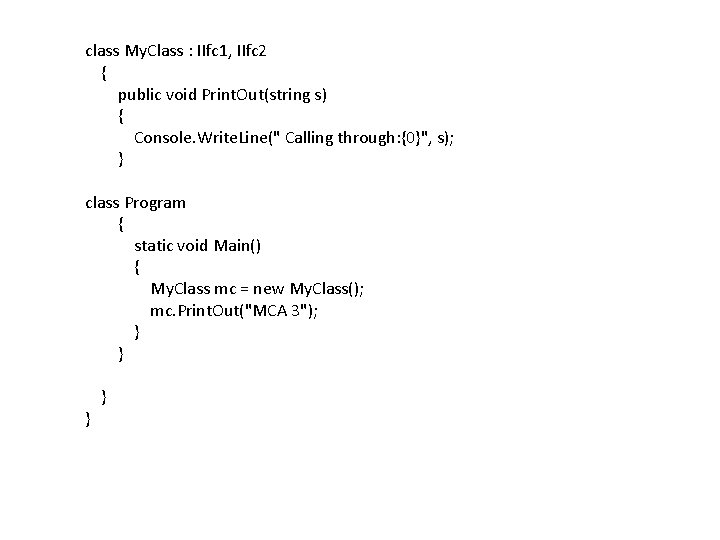
class My. Class : IIfc 1, IIfc 2 { public void Print. Out(string s) { Console. Write. Line(" Calling through: {0}", s); } class Program { static void Main() { My. Class mc = new My. Class(); mc. Print. Out("MCA 3"); } }
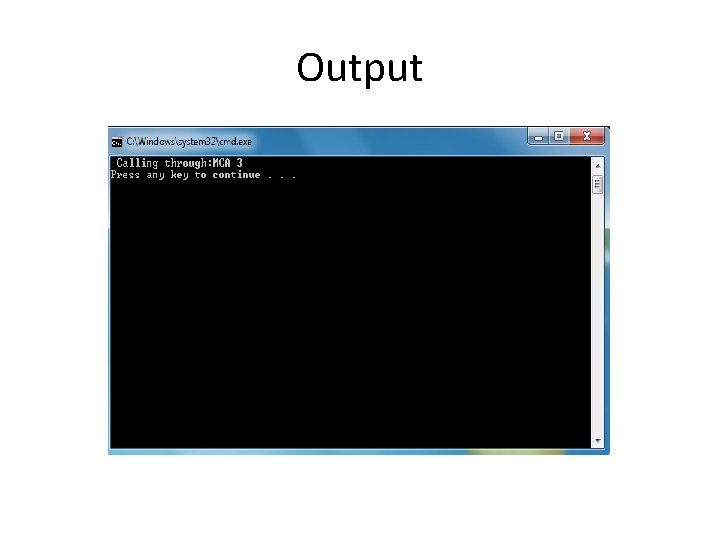
Output
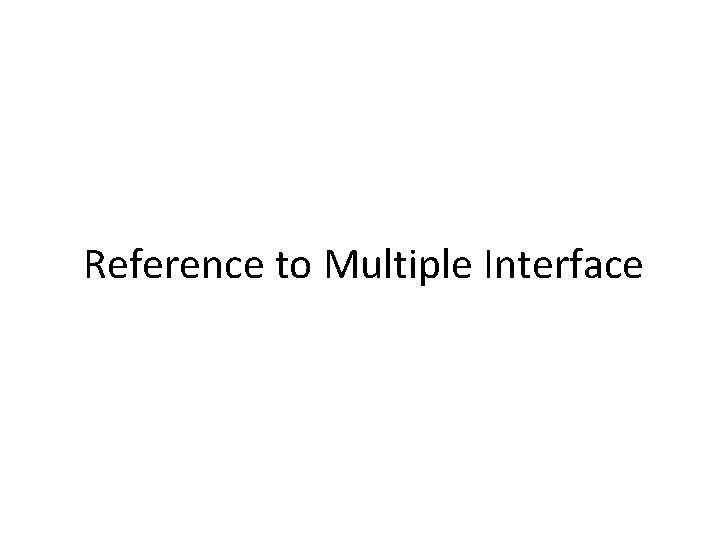
Reference to Multiple Interface
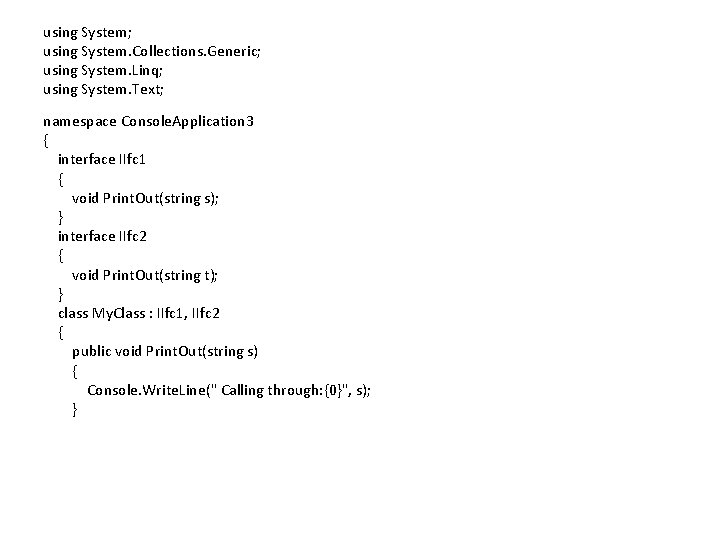
using System; using System. Collections. Generic; using System. Linq; using System. Text; namespace Console. Application 3 { interface IIfc 1 { void Print. Out(string s); } interface IIfc 2 { void Print. Out(string t); } class My. Class : IIfc 1, IIfc 2 { public void Print. Out(string s) { Console. Write. Line(" Calling through: {0}", s); }
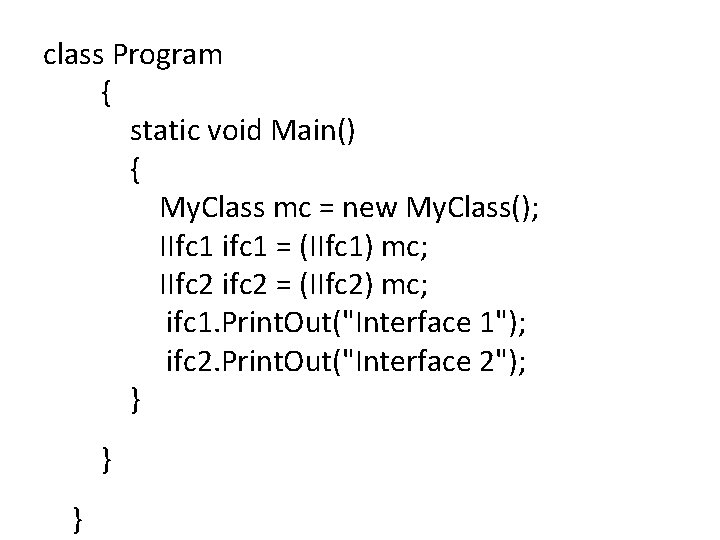
class Program { static void Main() { My. Class mc = new My. Class(); IIfc 1 ifc 1 = (IIfc 1) mc; IIfc 2 ifc 2 = (IIfc 2) mc; ifc 1. Print. Out("Interface 1"); ifc 2. Print. Out("Interface 2"); } } }
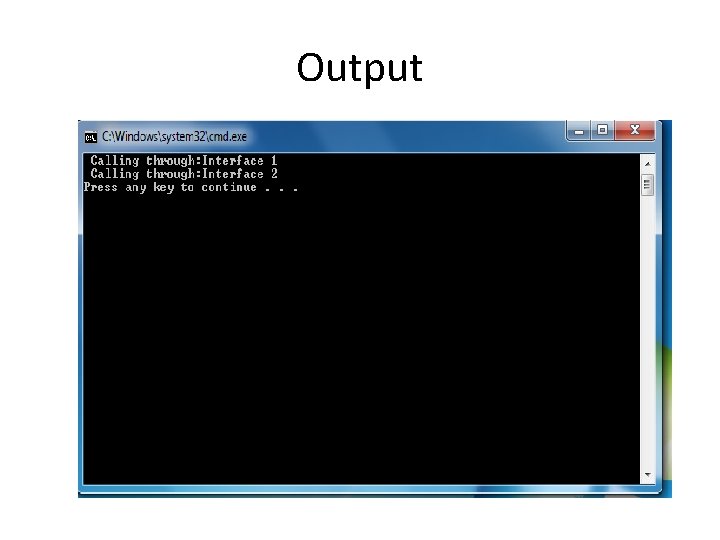
Output
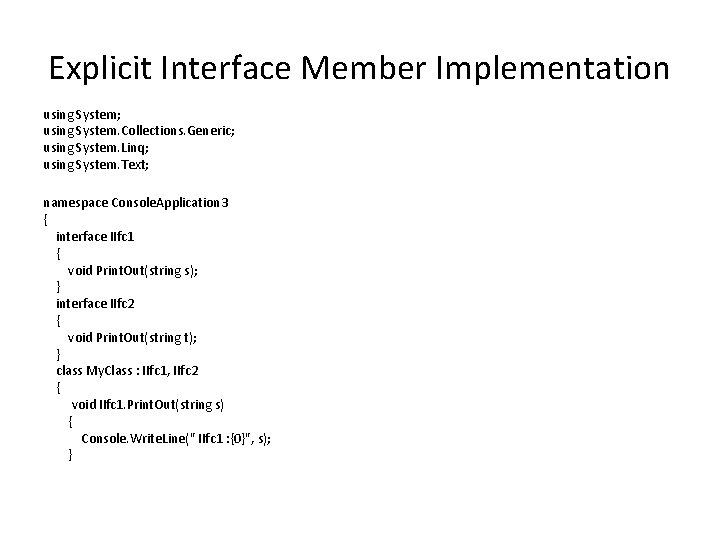
Explicit Interface Member Implementation using System; using System. Collections. Generic; using System. Linq; using System. Text; namespace Console. Application 3 { interface IIfc 1 { void Print. Out(string s); } interface IIfc 2 { void Print. Out(string t); } class My. Class : IIfc 1, IIfc 2 { void IIfc 1. Print. Out(string s) { Console. Write. Line(" IIfc 1 : {0}", s); }
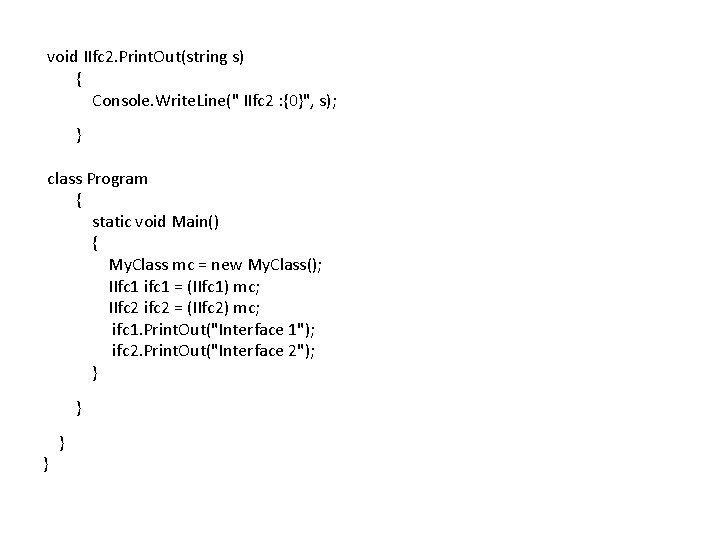
void IIfc 2. Print. Out(string s) { Console. Write. Line(" IIfc 2 : {0}", s); } class Program { static void Main() { My. Class mc = new My. Class(); IIfc 1 ifc 1 = (IIfc 1) mc; IIfc 2 ifc 2 = (IIfc 2) mc; ifc 1. Print. Out("Interface 1"); ifc 2. Print. Out("Interface 2"); } }
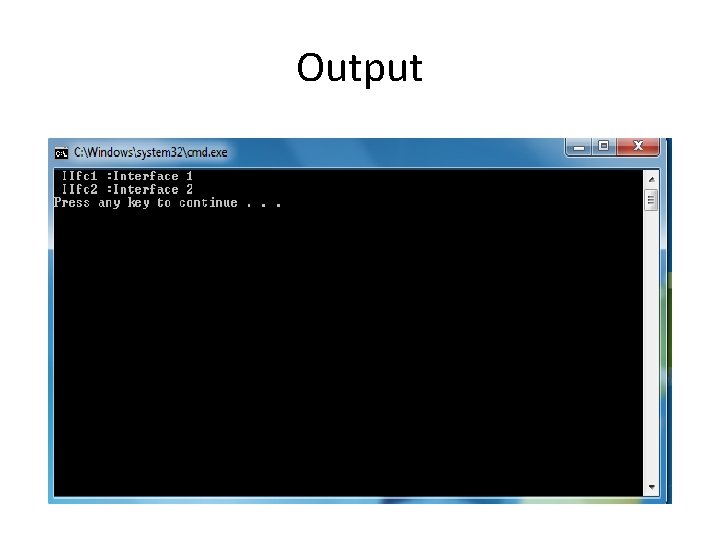
Output
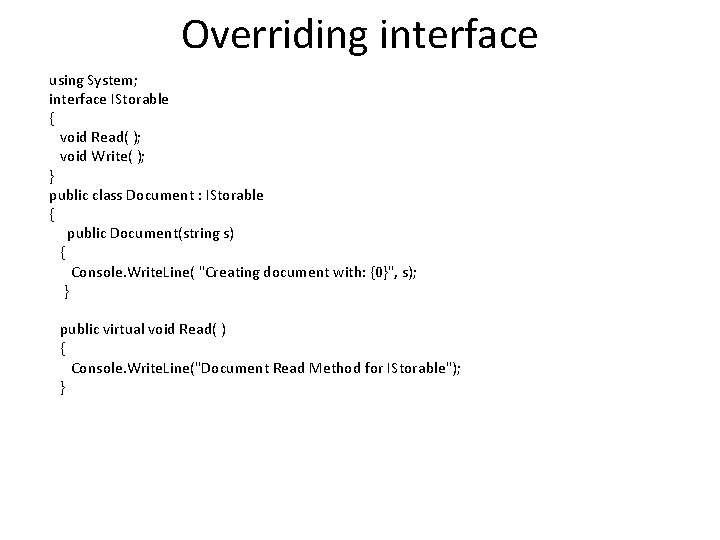
Overriding interface using System; interface IStorable { void Read( ); void Write( ); } public class Document : IStorable { public Document(string s) { Console. Write. Line( "Creating document with: {0}", s); } public virtual void Read( ) { Console. Write. Line("Document Read Method for IStorable"); }
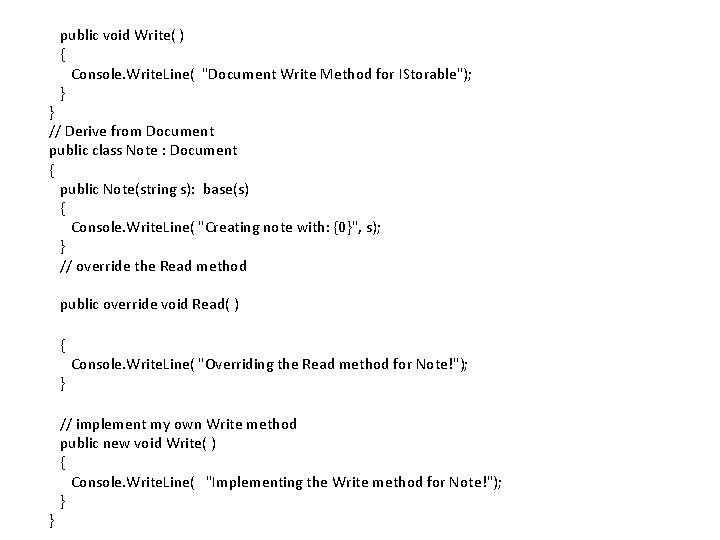
public void Write( ) { Console. Write. Line( "Document Write Method for IStorable"); } } // Derive from Document public class Note : Document { public Note(string s): base(s) { Console. Write. Line( "Creating note with: {0}", s); } // override the Read method public override void Read( ) { } } Console. Write. Line( "Overriding the Read method for Note!"); // implement my own Write method public new void Write( ) { Console. Write. Line( "Implementing the Write method for Note!"); }
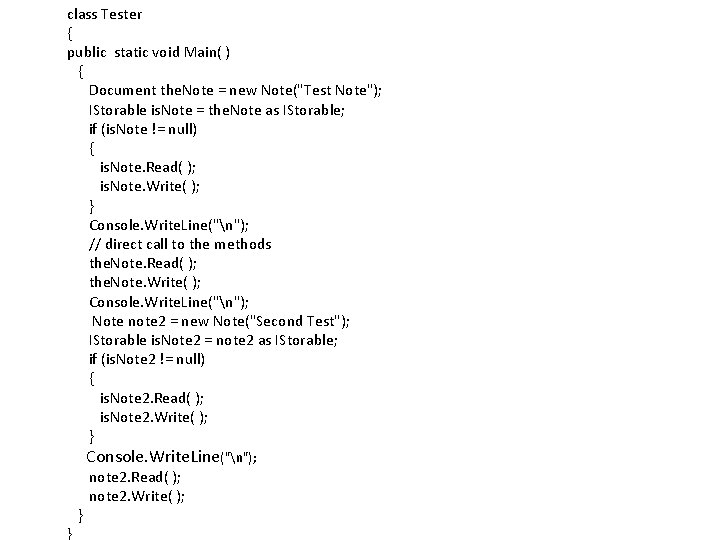
class Tester { public static void Main( ) { Document the. Note = new Note("Test Note"); IStorable is. Note = the. Note as IStorable; if (is. Note != null) { is. Note. Read( ); is. Note. Write( ); } Console. Write. Line("n"); // direct call to the methods the. Note. Read( ); the. Note. Write( ); Console. Write. Line("n"); Note note 2 = new Note("Second Test"); IStorable is. Note 2 = note 2 as IStorable; if (is. Note 2 != null) { is. Note 2. Read( ); is. Note 2. Write( ); } Console. Write. Line("n"); } } note 2. Read( ); note 2. Write( );
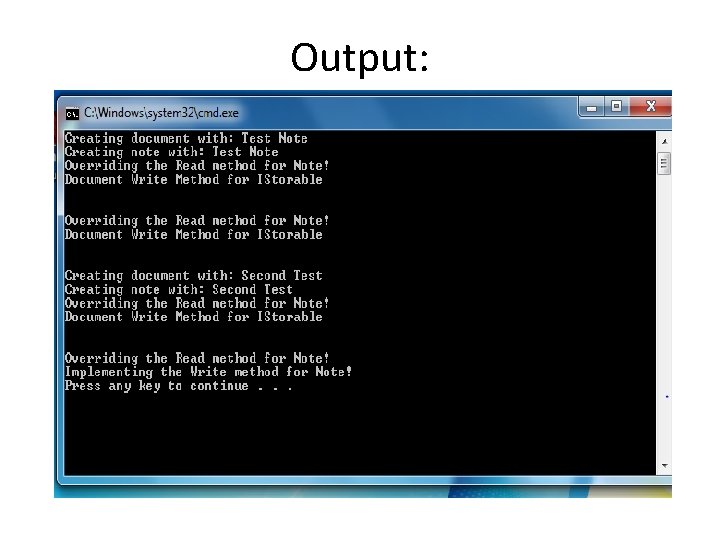
Output:
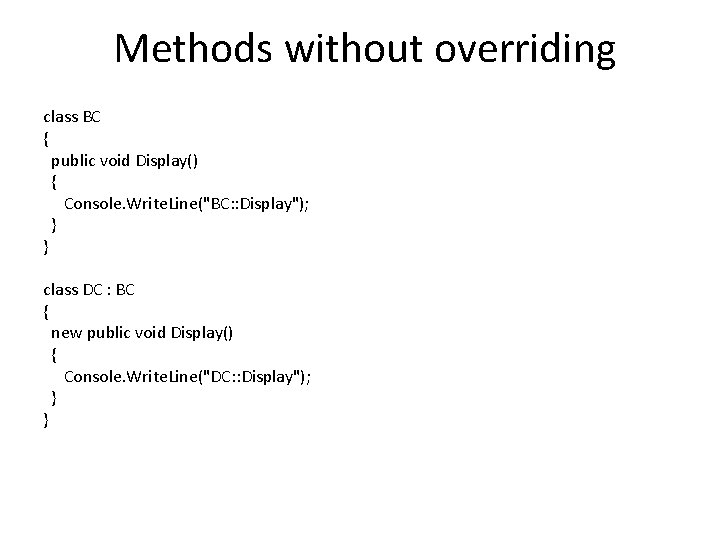
Methods without overriding class BC { public void Display() { Console. Write. Line("BC: : Display"); } } class DC : BC { new public void Display() { Console. Write. Line("DC: : Display"); } }
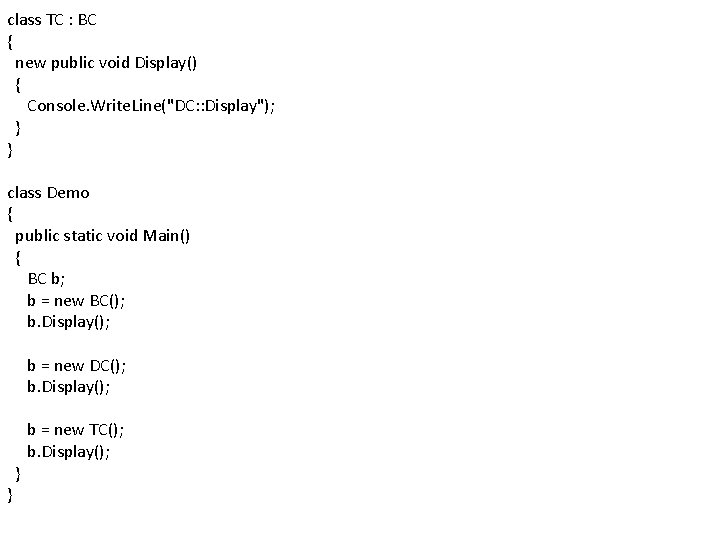
class TC : BC { new public void Display() { Console. Write. Line("DC: : Display"); } } class Demo { public static void Main() { BC b; b = new BC(); b. Display(); b = new DC(); b. Display(); } } b = new TC(); b. Display();
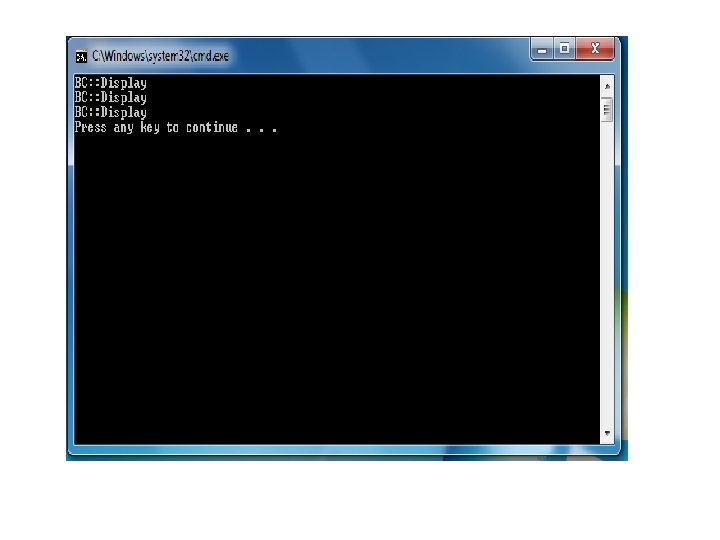
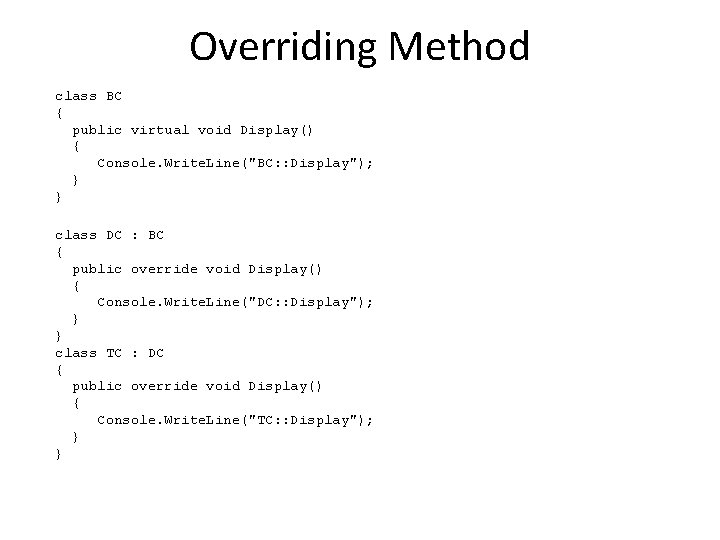
Overriding Method class BC { public virtual void Display() { Console. Write. Line("BC: : Display"); } } class DC : BC { public override void Display() { Console. Write. Line("DC: : Display"); } } class TC : DC { public override void Display() { Console. Write. Line("TC: : Display"); } }
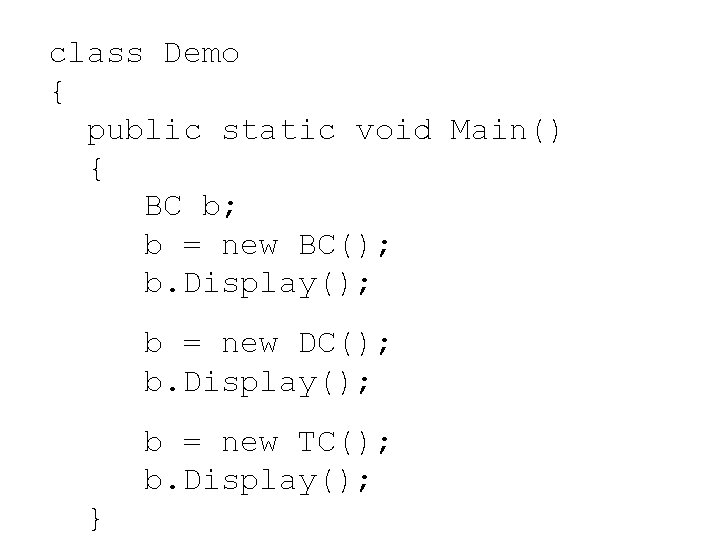
class Demo { public static void Main() { BC b; b = new BC(); b. Display(); b = new DC(); b. Display(); b = new TC(); b. Display(); }
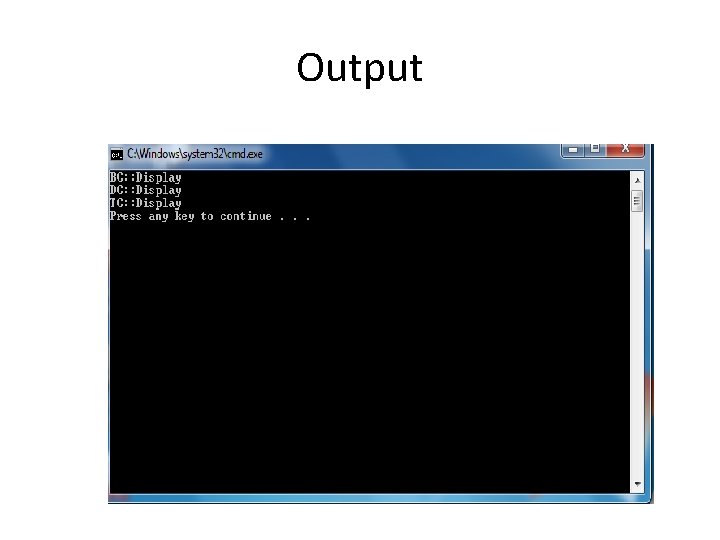
Output