int max 3int x int y int z
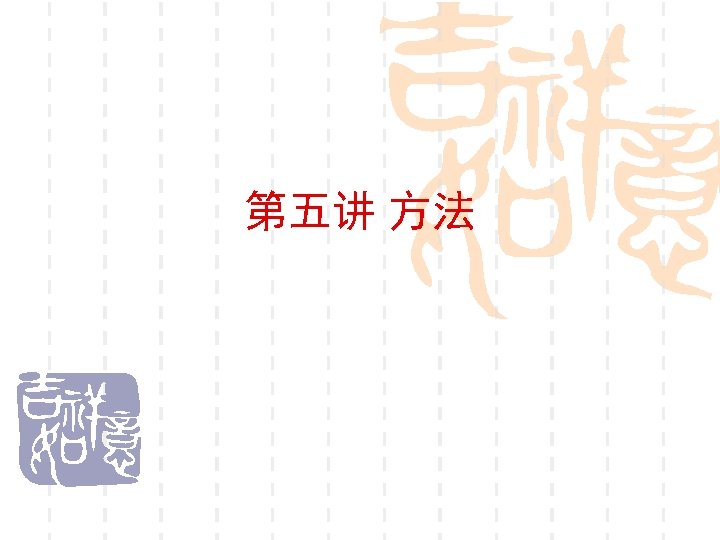
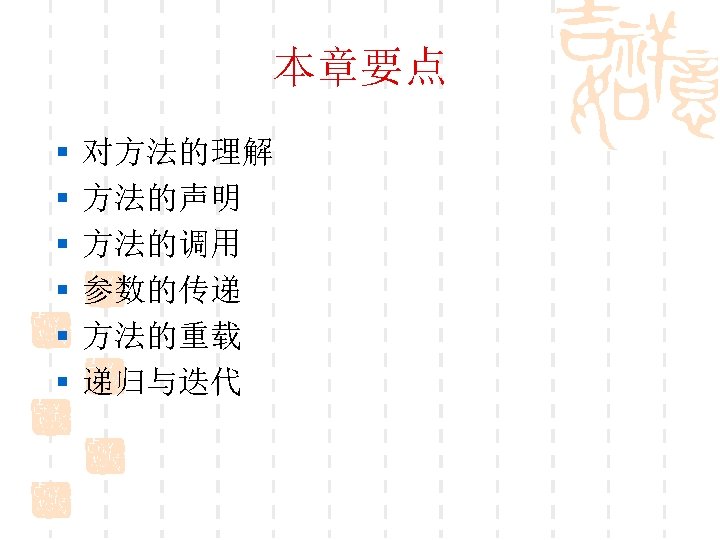
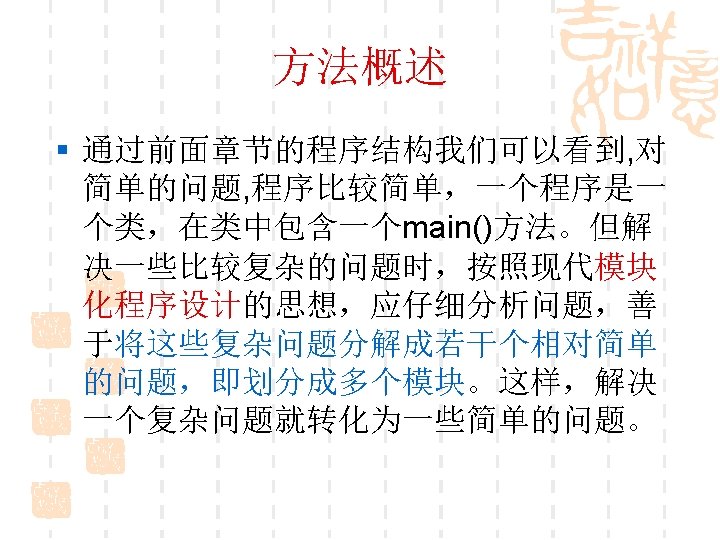
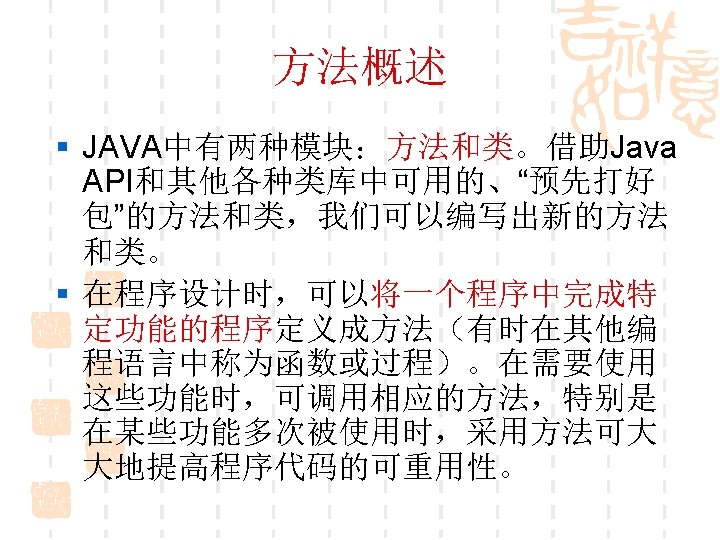
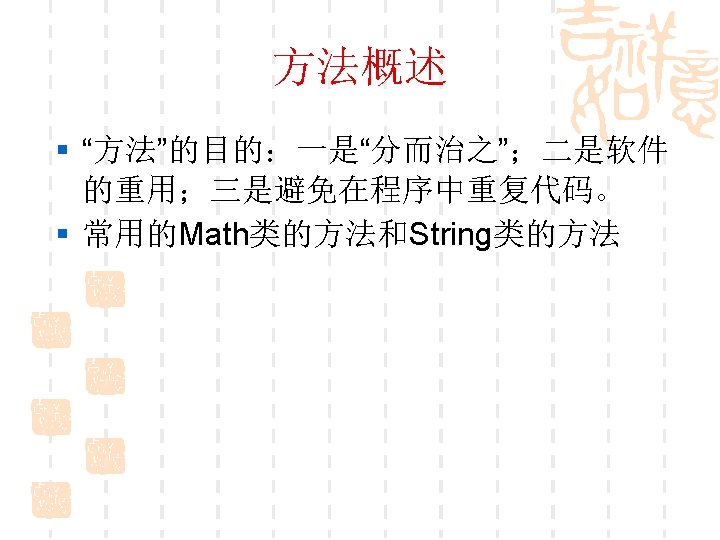
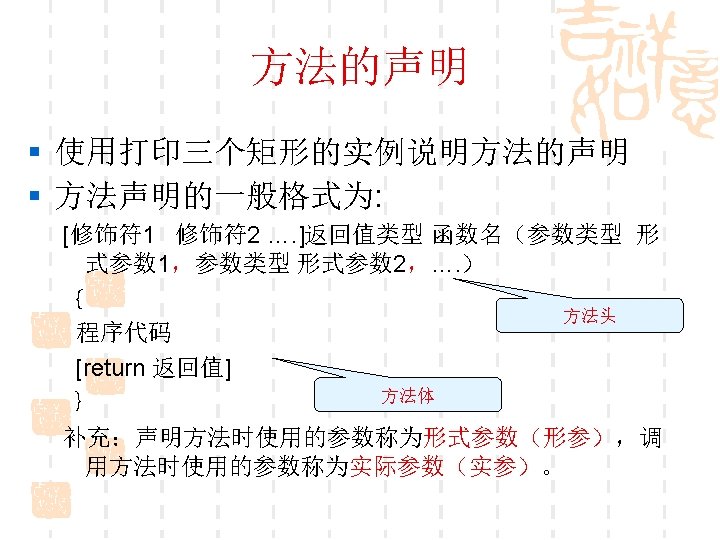
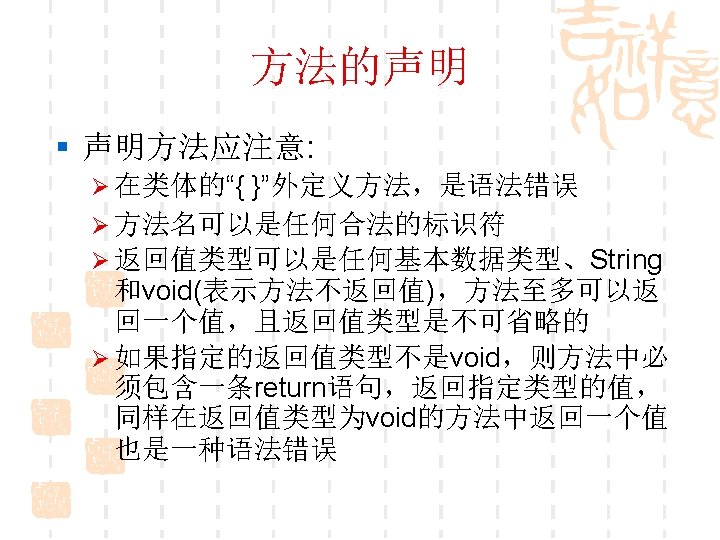
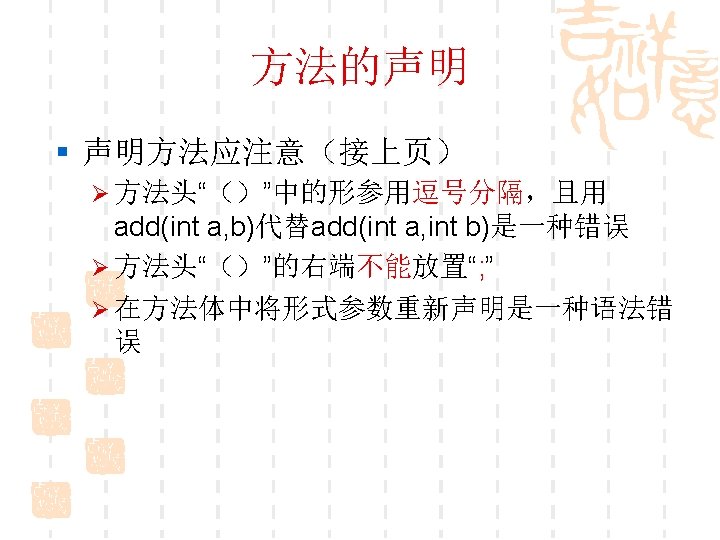
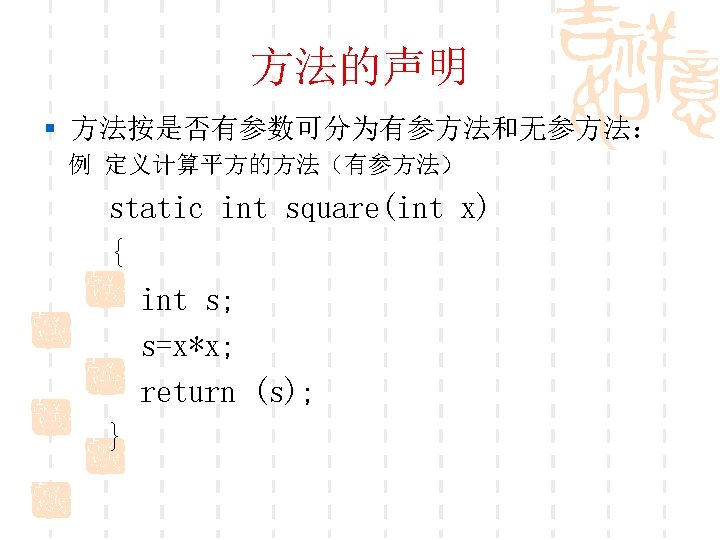
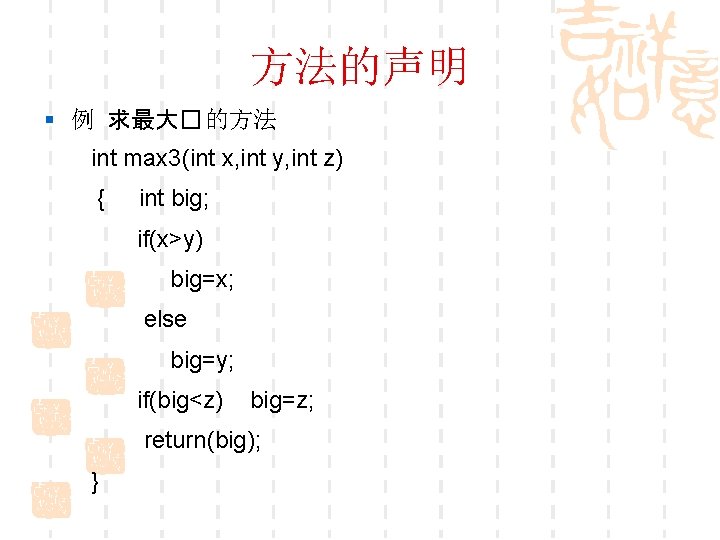
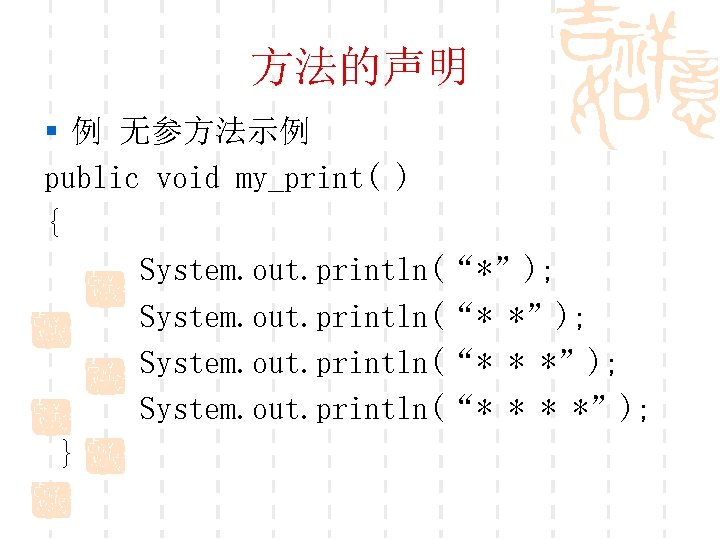
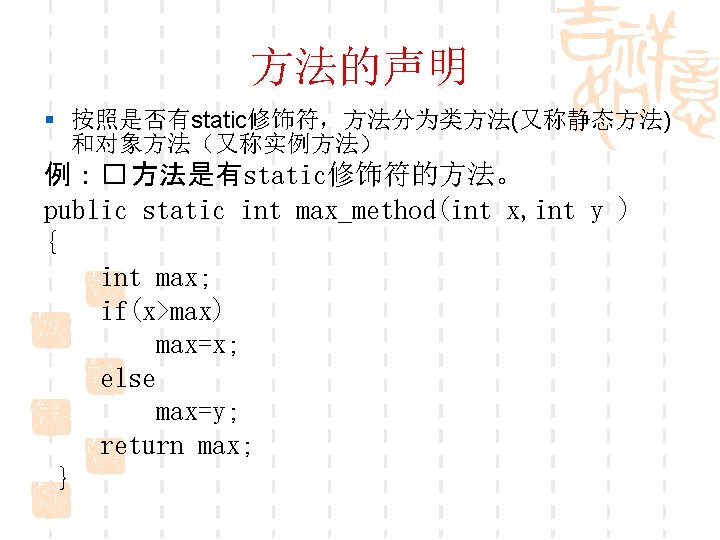
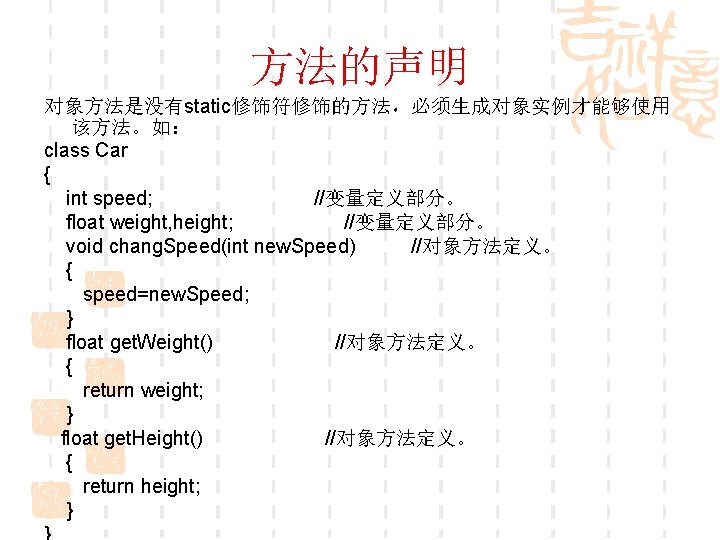
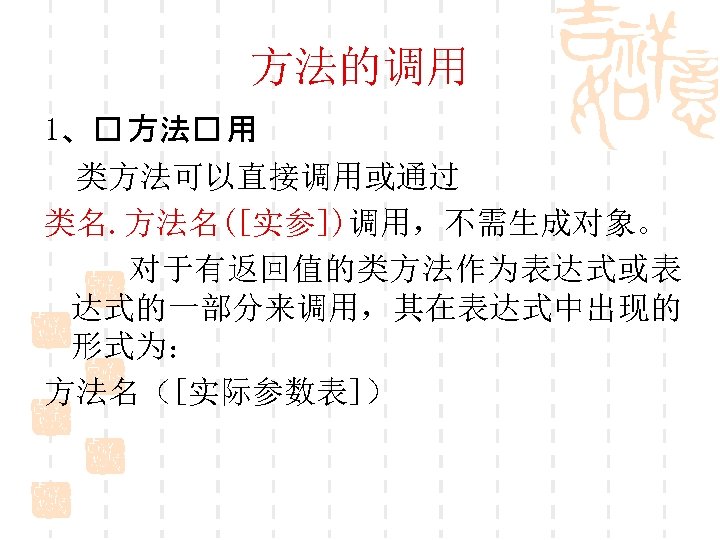
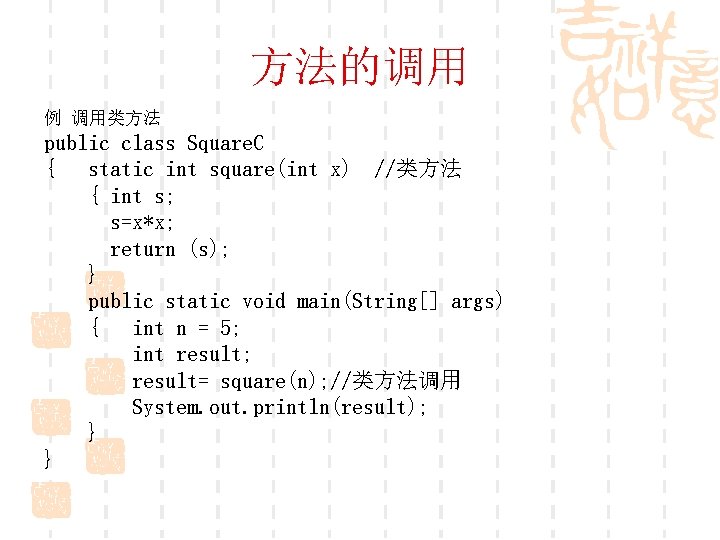
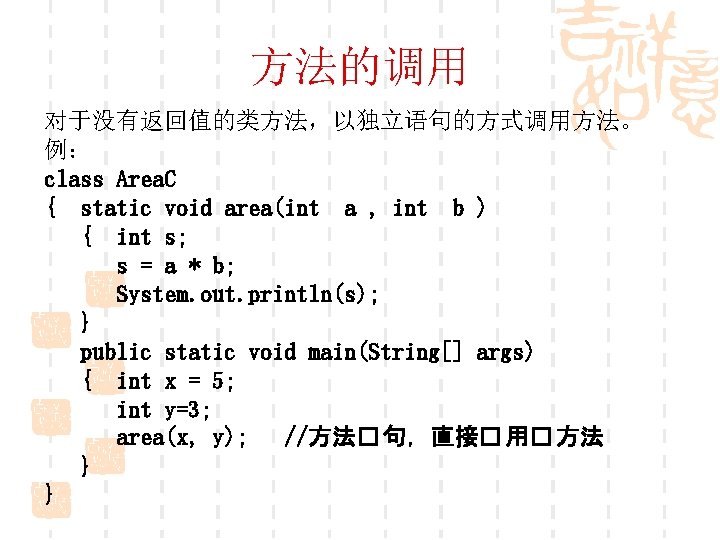
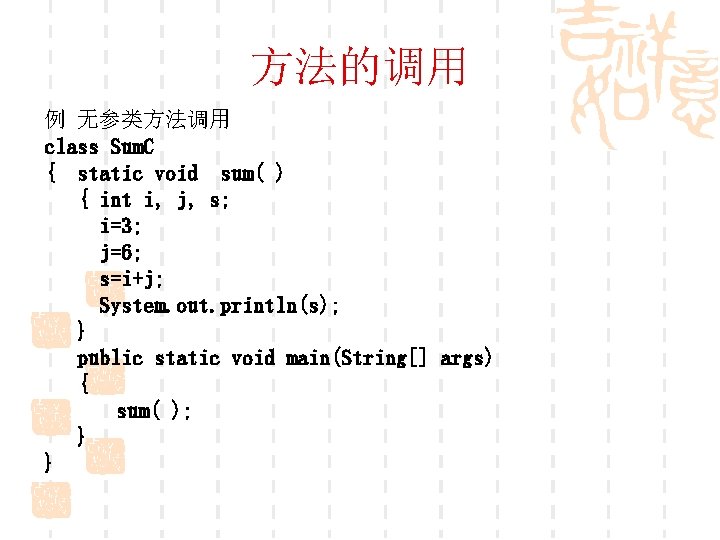
![方法的调用 2、对象方法调用 � 于� 象方法,必� 先生成� 象� 例,然后用如下形式� 用方法: � 象名. 方法名([� 参]) class 方法的调用 2、对象方法调用 � 于� 象方法,必� 先生成� 象� 例,然后用如下形式� 用方法: � 象名. 方法名([� 参]) class](https://slidetodoc.com/presentation_image_h2/7ed5381bf68b536fb301255cf30d4652/image-18.jpg)
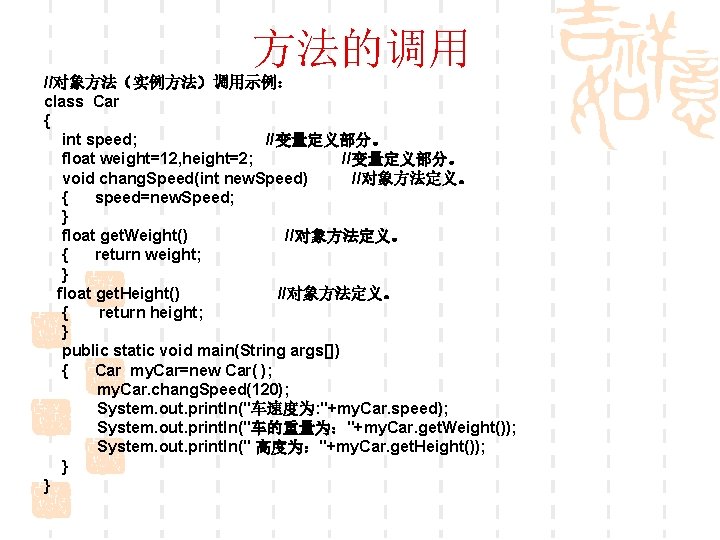
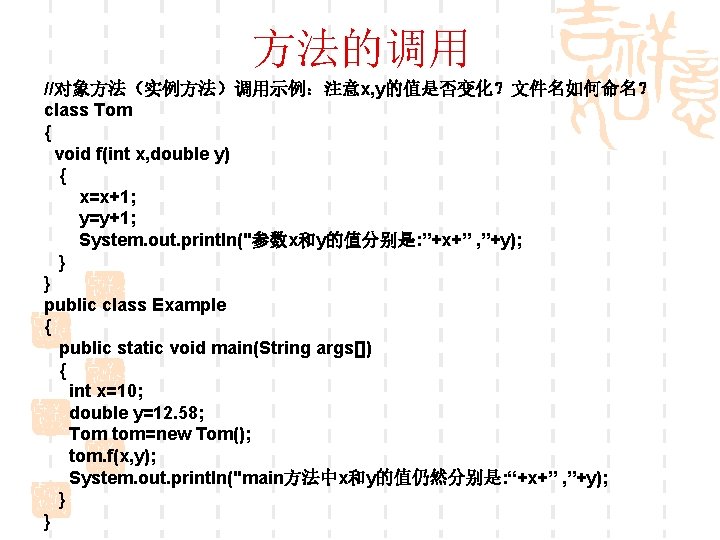
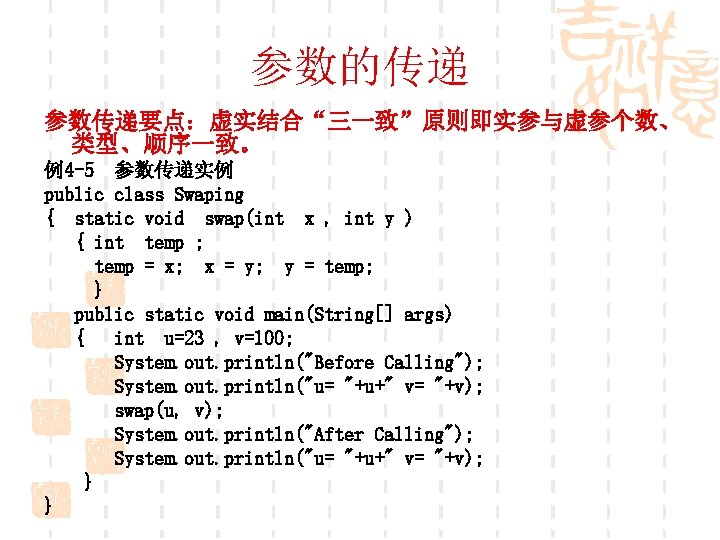
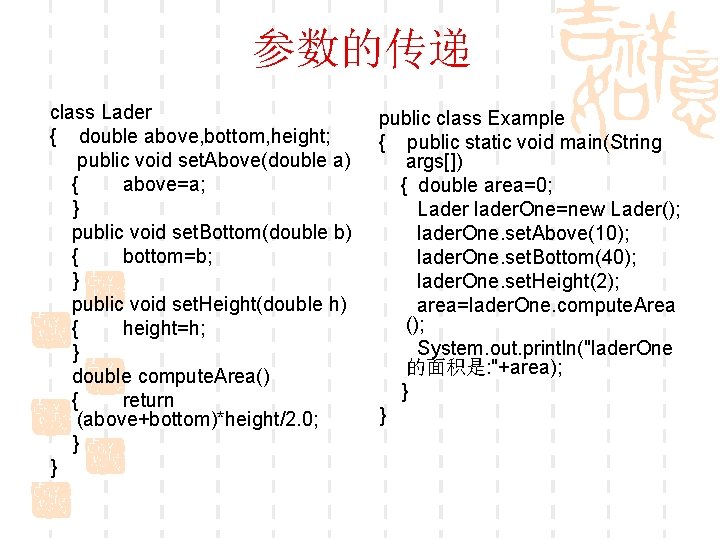
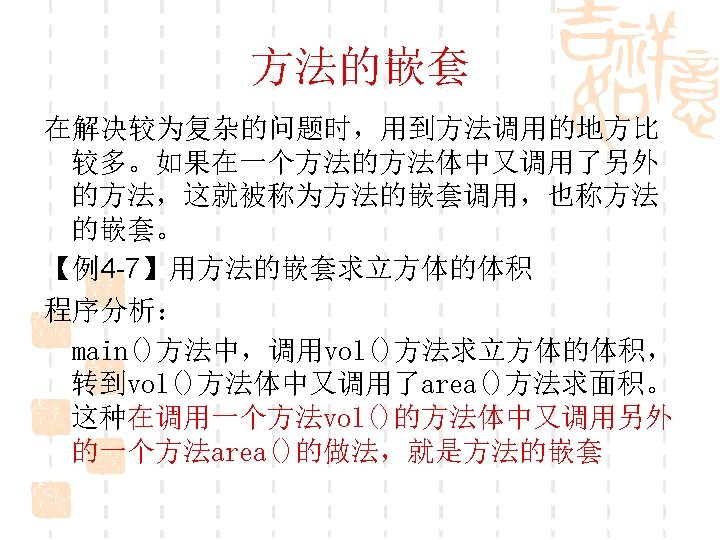
![方法的嵌套 public class Volume { public static void main(String args[ ]) { int i=5, 方法的嵌套 public class Volume { public static void main(String args[ ]) { int i=5,](https://slidetodoc.com/presentation_image_h2/7ed5381bf68b536fb301255cf30d4652/image-24.jpg)
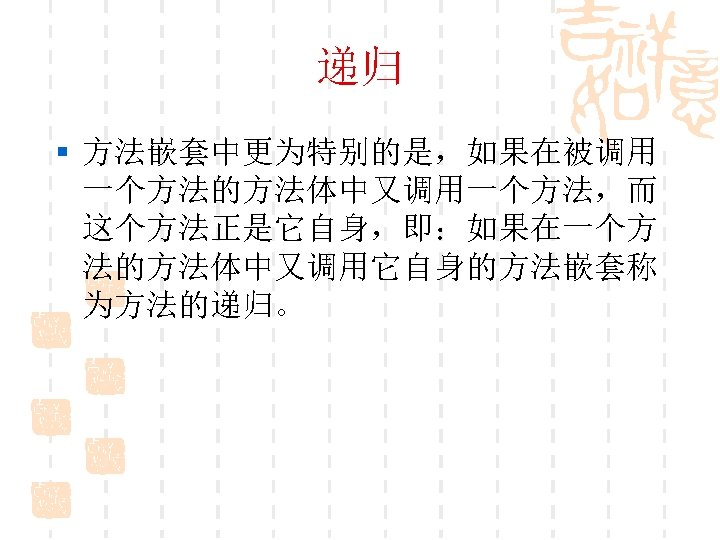
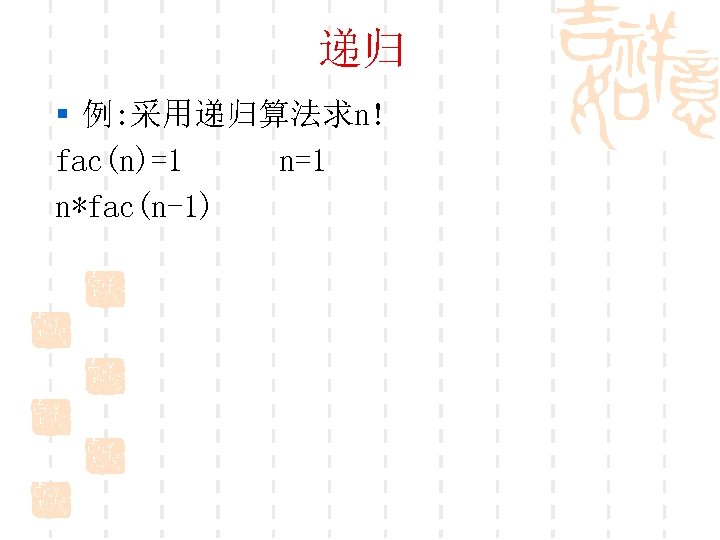
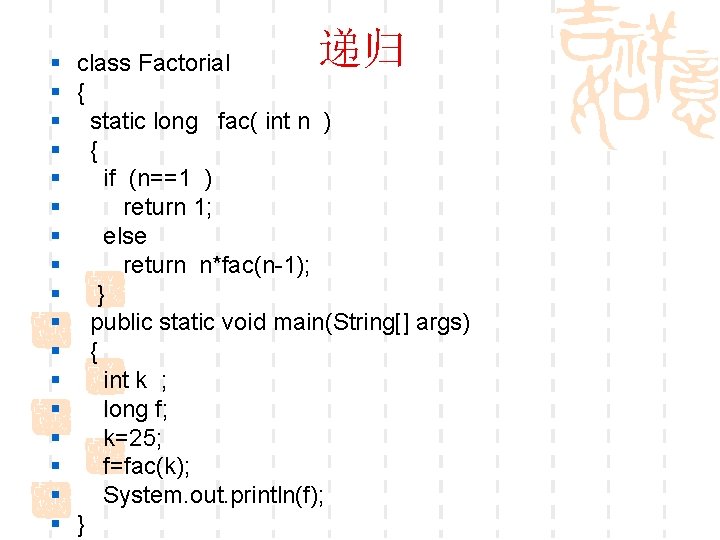
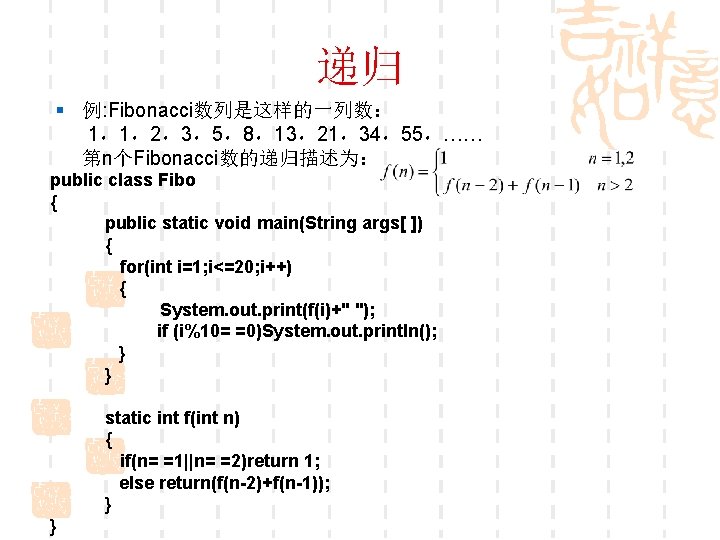
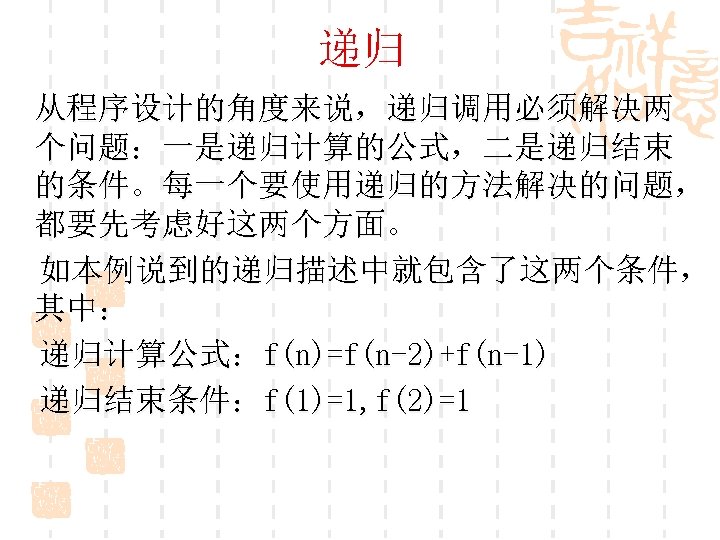
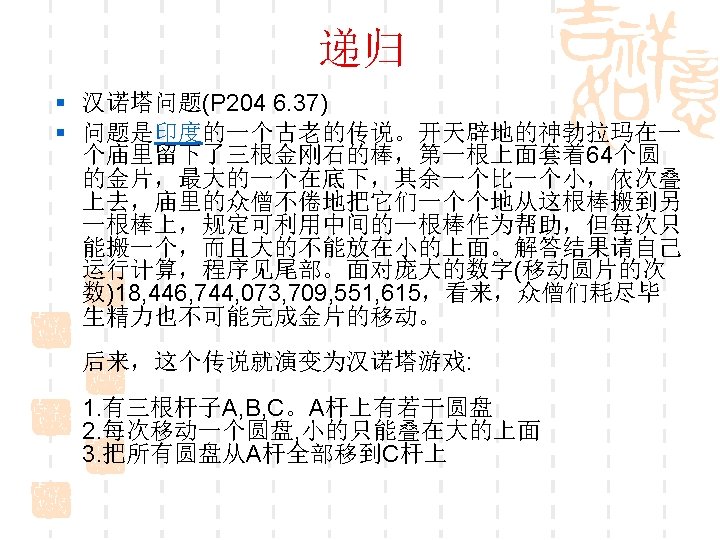
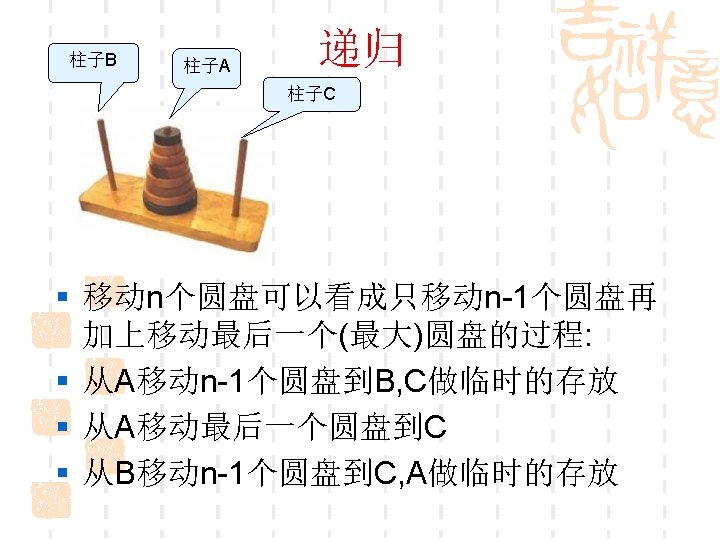
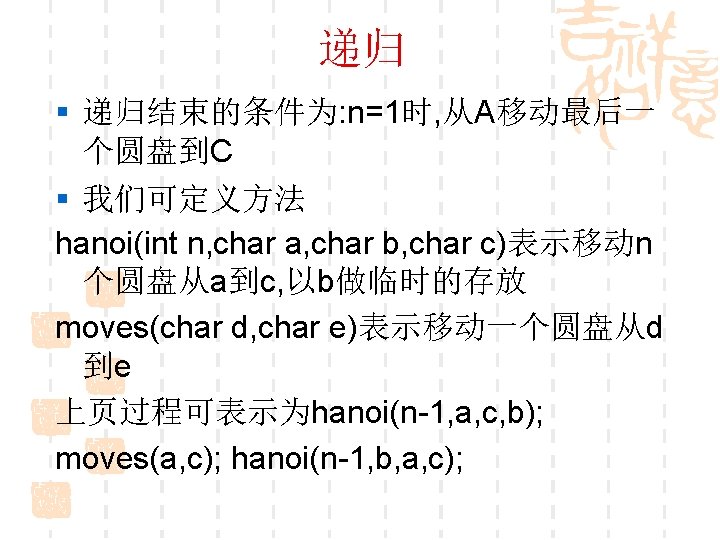
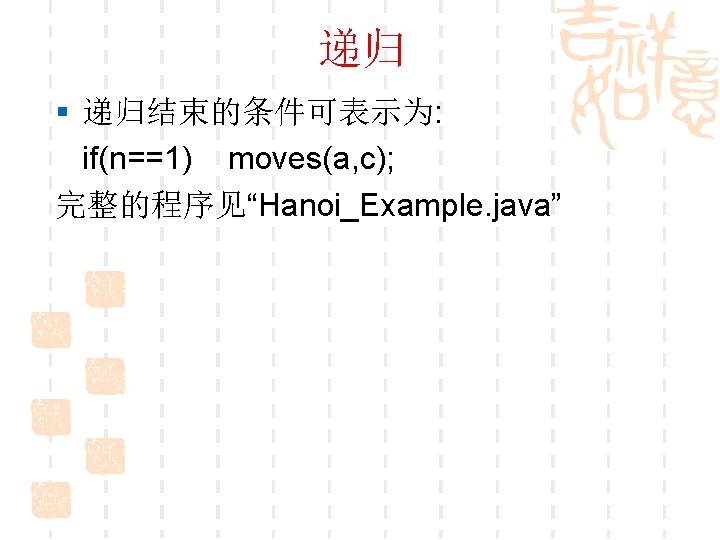
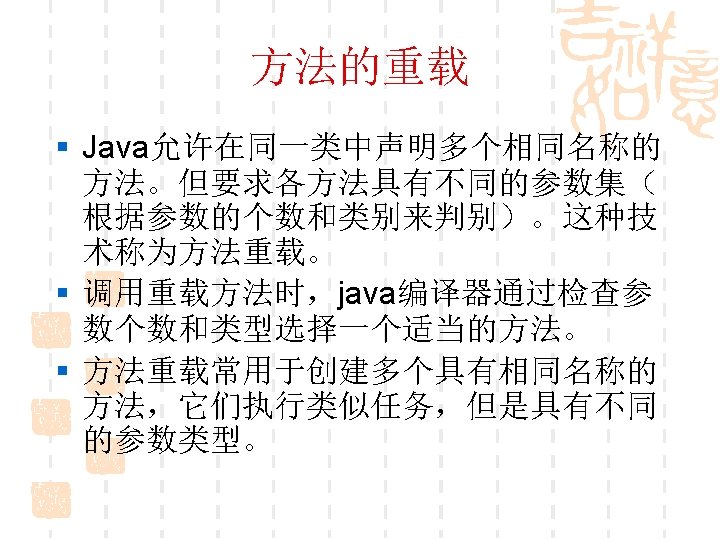
- Slides: 34
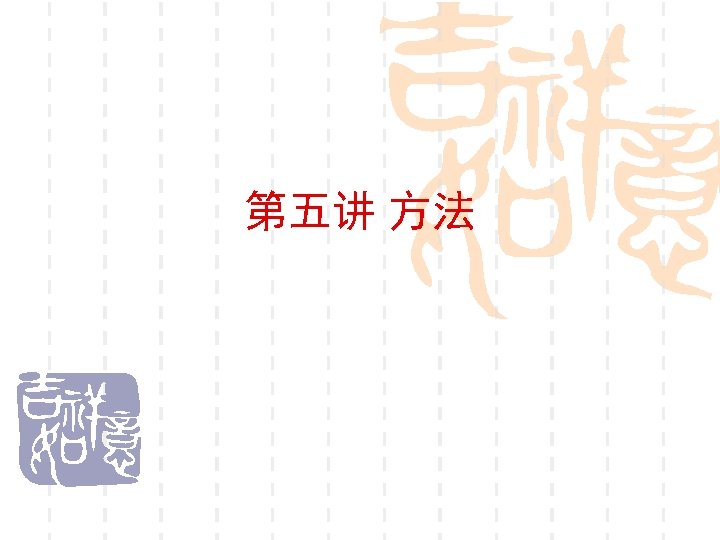
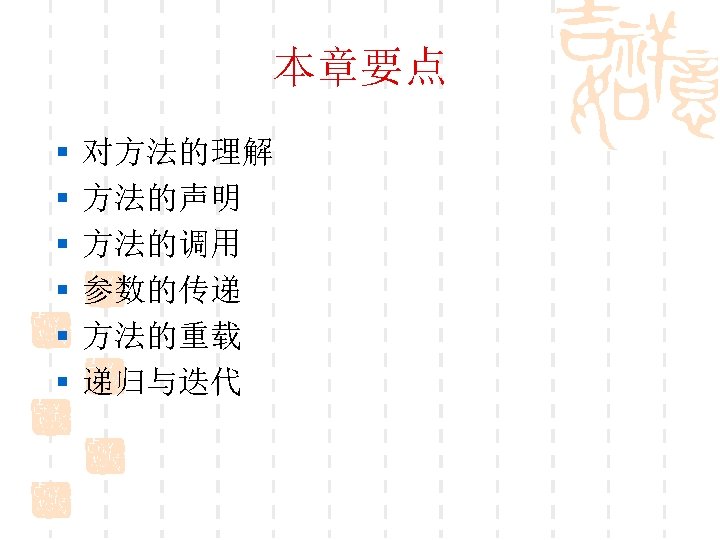
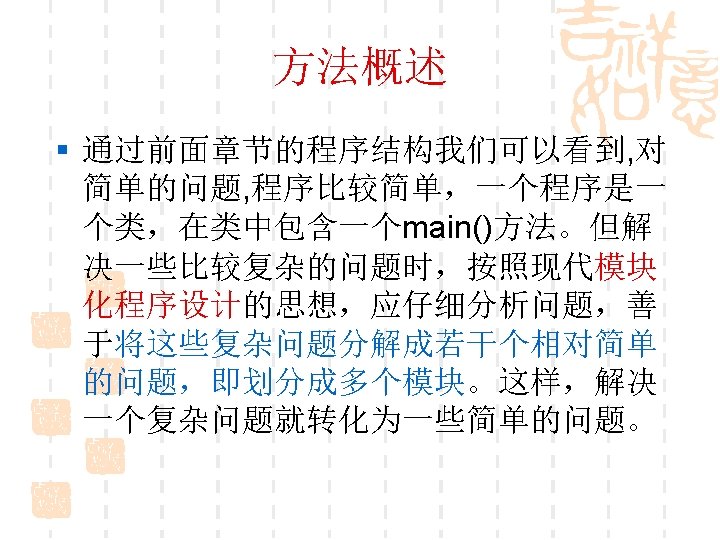
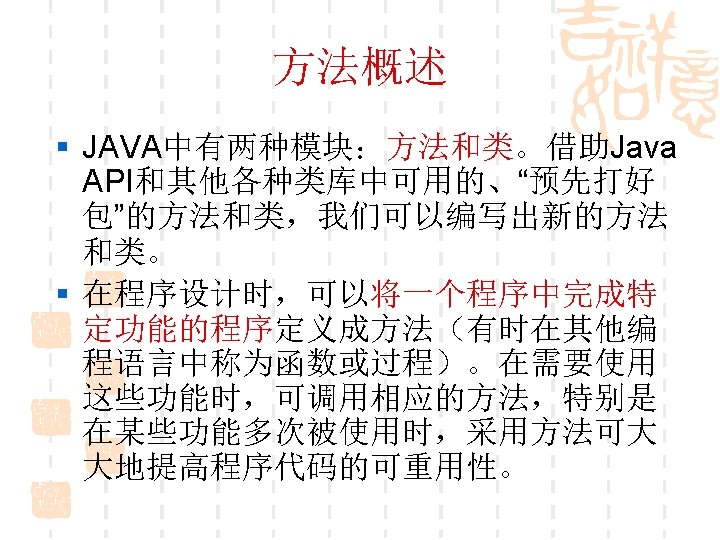
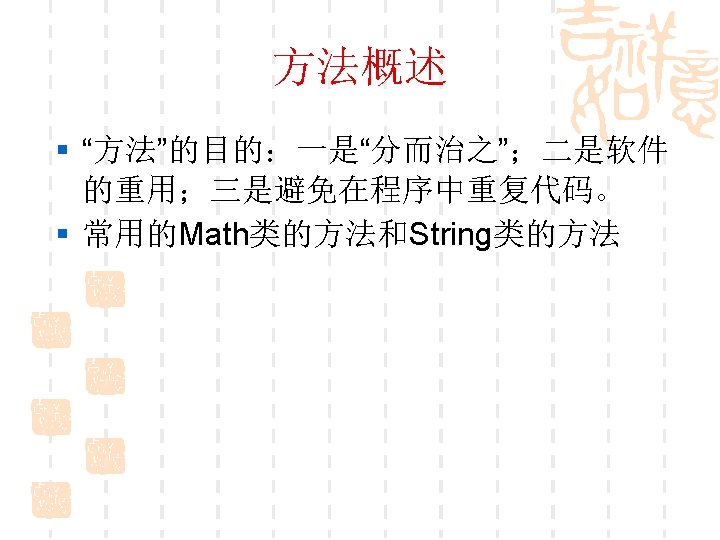
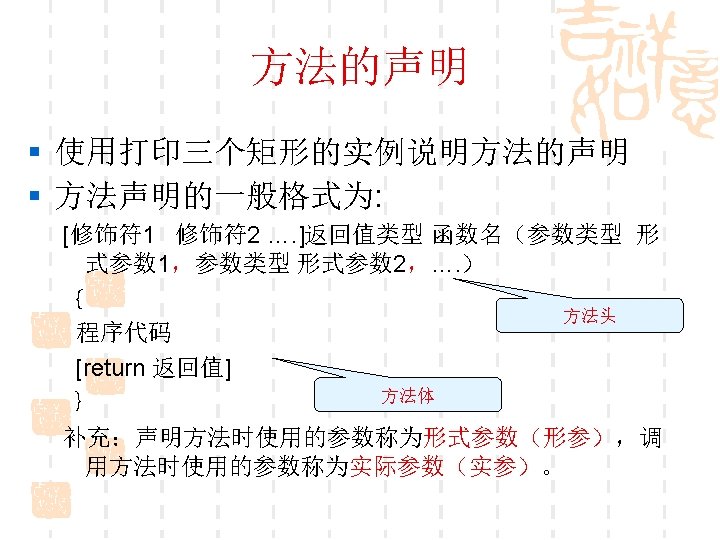
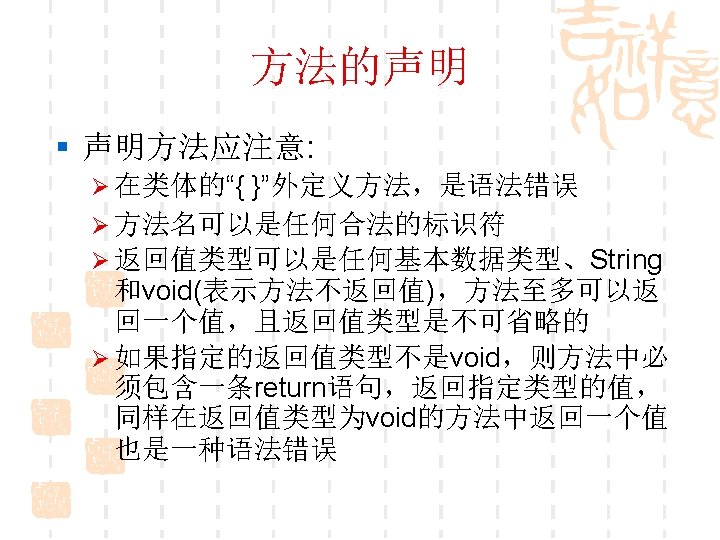
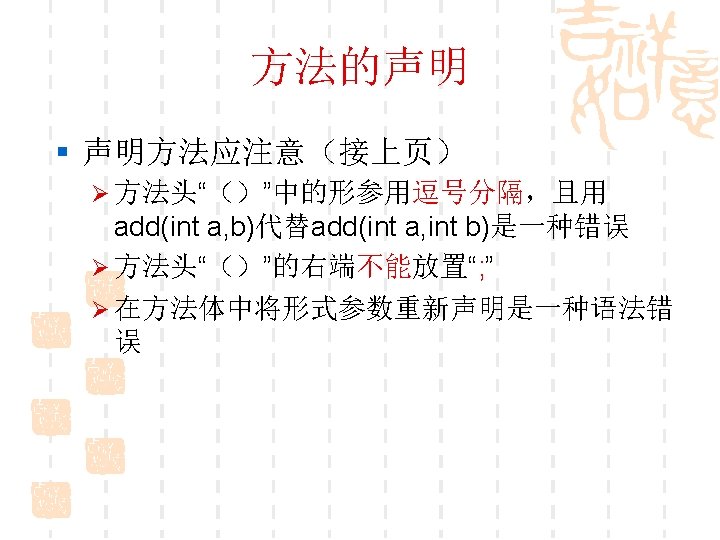
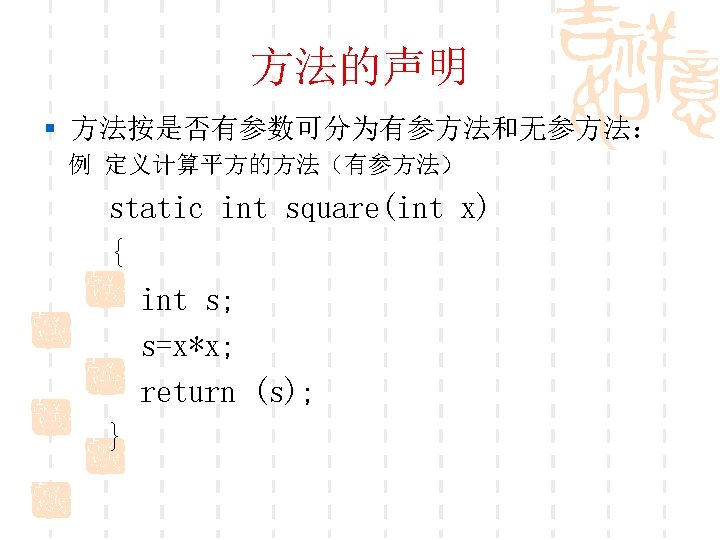
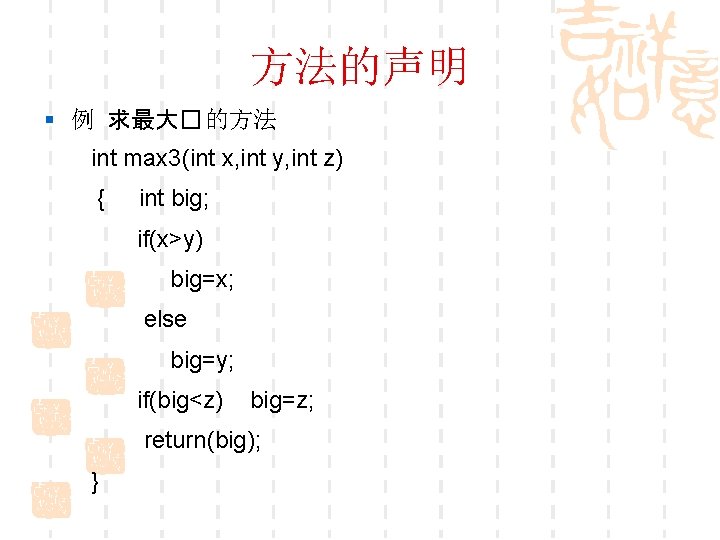
方法的声明 § 例 求最大� 的方法 int max 3(int x, int y, int z) { int big; if(x>y) big=x; else big=y; if(big<z) big=z; return(big); }
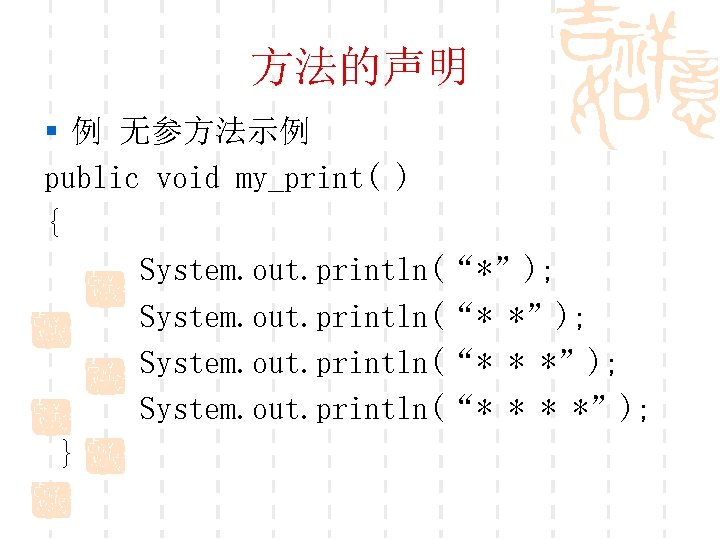
方法的声明 § 例 无参方法示例 public void my_print( ) { System. out. println(“*”); System. out. println(“* * * *”); }
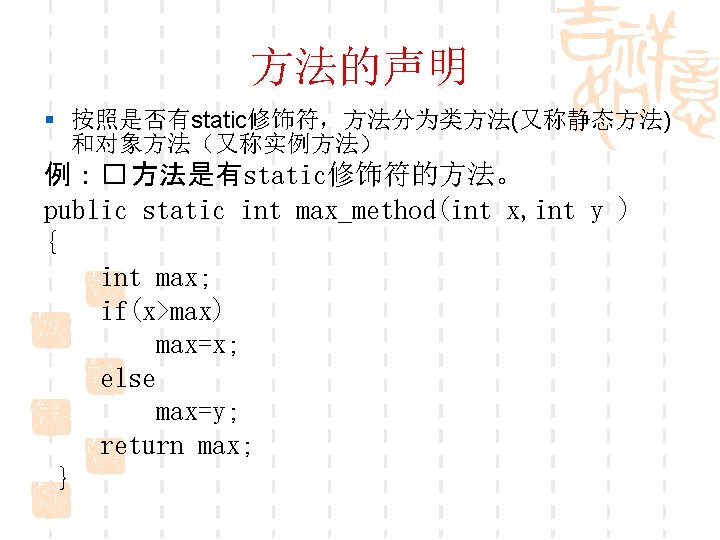
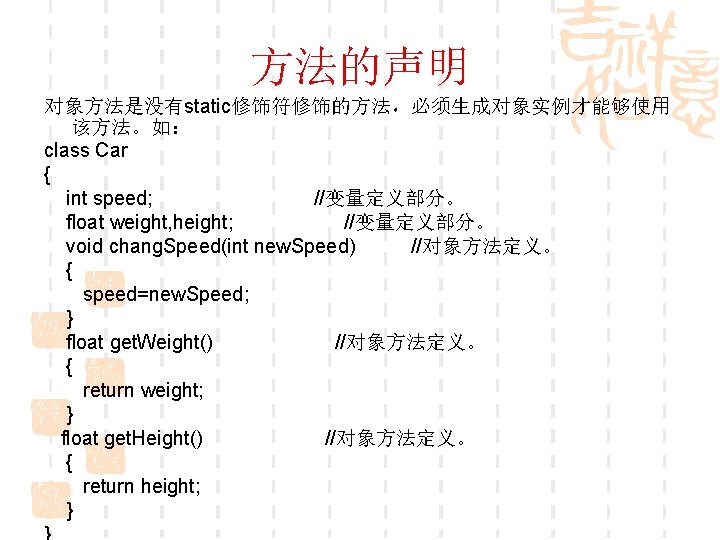
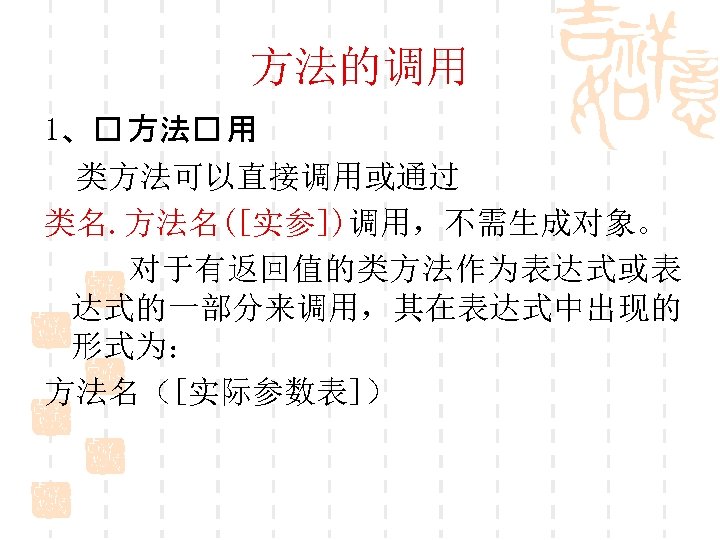
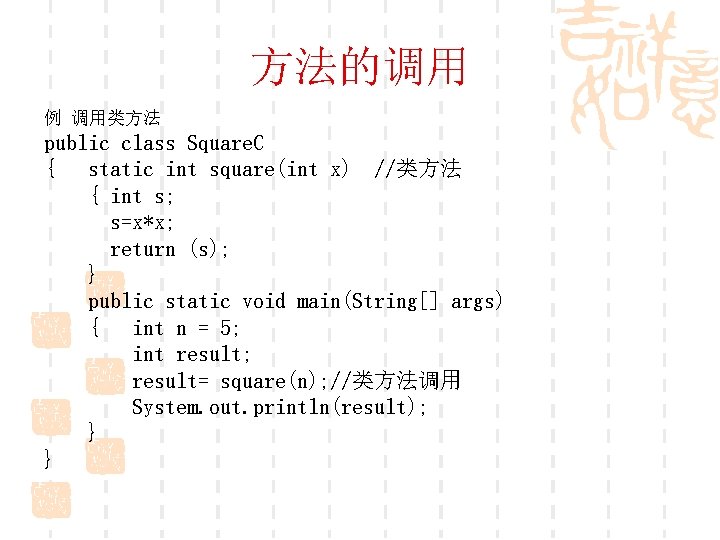
方法的调用 例 调用类方法 public class Square. C { static int square(int x) //类方法 { int s; s=x*x; return (s); } public static void main(String[] args) { int n = 5; int result; result= square(n); //类方法调用 System. out. println(result); } }
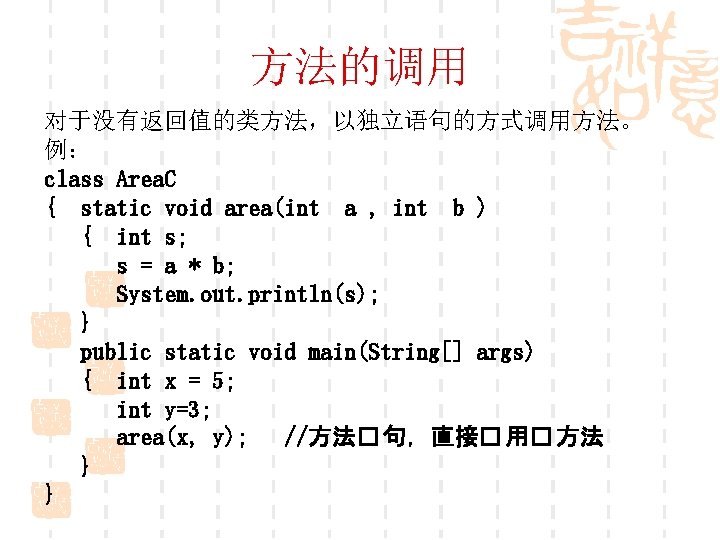
方法的调用 对于没有返回值的类方法,以独立语句的方式调用方法。 例: class Area. C { static void area(int a , int b ) { int s; s = a * b; System. out. println(s); } public static void main(String[] args) { int x = 5; int y=3; area(x, y); //方法� 句,直接� 用� 方法 } }
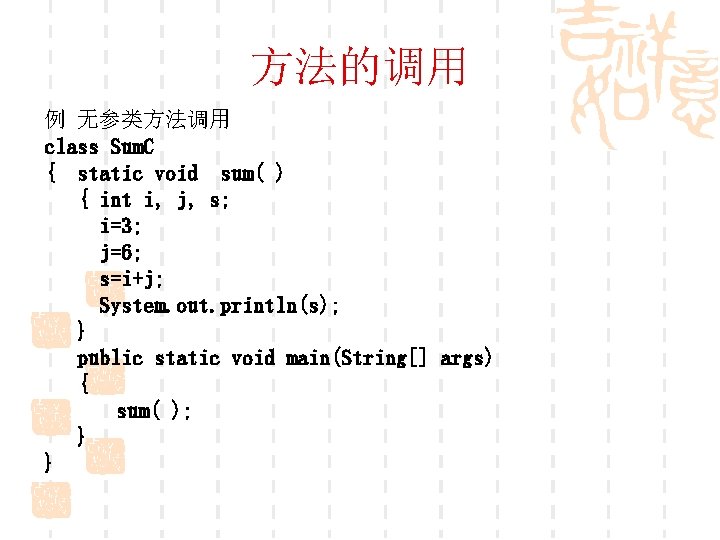
方法的调用 例 无参类方法调用 class Sum. C { static void sum( ) { int i, j, s; i=3; j=6; s=i+j; System. out. println(s); } public static void main(String[] args) { sum( ); } }
![方法的调用 2对象方法调用 于 象方法必 先生成 象 例然后用如下形式 用方法 象名 方法名 参 class 方法的调用 2、对象方法调用 � 于� 象方法,必� 先生成� 象� 例,然后用如下形式� 用方法: � 象名. 方法名([� 参]) class](https://slidetodoc.com/presentation_image_h2/7ed5381bf68b536fb301255cf30d4652/image-18.jpg)
方法的调用 2、对象方法调用 � 于� 象方法,必� 先生成� 象� 例,然后用如下形式� 用方法: � 象名. 方法名([� 参]) class My. Object { static int x=10; static int y=20; void method(int k, int m) { int sum; sum=k+m; System. out. println("sum="+sum); } public static void main(String args[]) { My. Object obj=new My. Object(); //生成对象 obj. method(x, y); //调用对象方法 } }
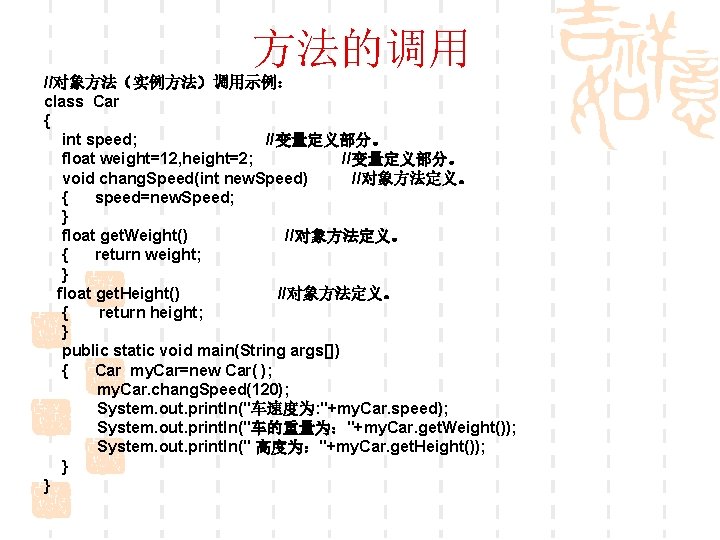
方法的调用 //对象方法(实例方法)调用示例: class Car { int speed; //变量定义部分。 float weight=12, height=2; //变量定义部分。 void chang. Speed(int new. Speed) //对象方法定义。 { speed=new. Speed; } float get. Weight() //对象方法定义。 { return weight; } float get. Height() //对象方法定义。 { return height; } public static void main(String args[]) { Car my. Car=new Car( ); my. Car. chang. Speed(120); System. out. println("车速度为: "+my. Car. speed); System. out. println("车的重量为:"+my. Car. get. Weight()); System. out. println(" 高度为:"+my. Car. get. Height()); } }
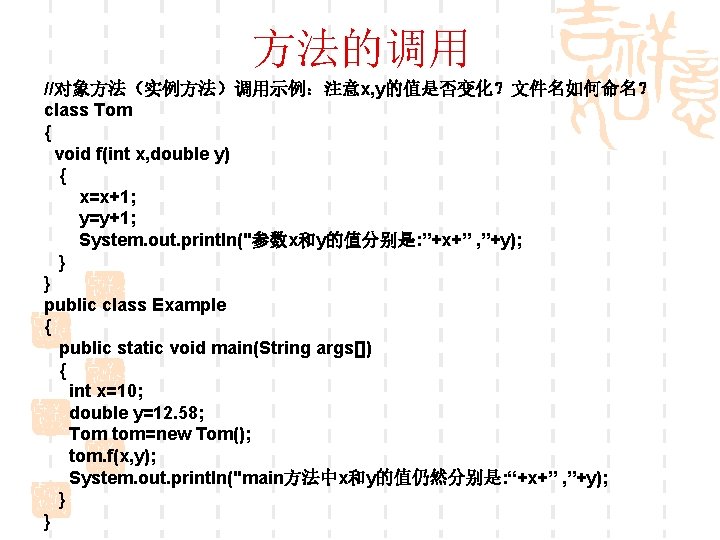
方法的调用 //对象方法(实例方法)调用示例:注意x, y的值是否变化?文件名如何命名? class Tom { void f(int x, double y) { x=x+1; y=y+1; System. out. println("参数x和y的值分别是: ”+x+” , ”+y); } } public class Example { public static void main(String args[]) { int x=10; double y=12. 58; Tom tom=new Tom(); tom. f(x, y); System. out. println("main方法中x和y的值仍然分别是: “+x+” , ”+y); } }
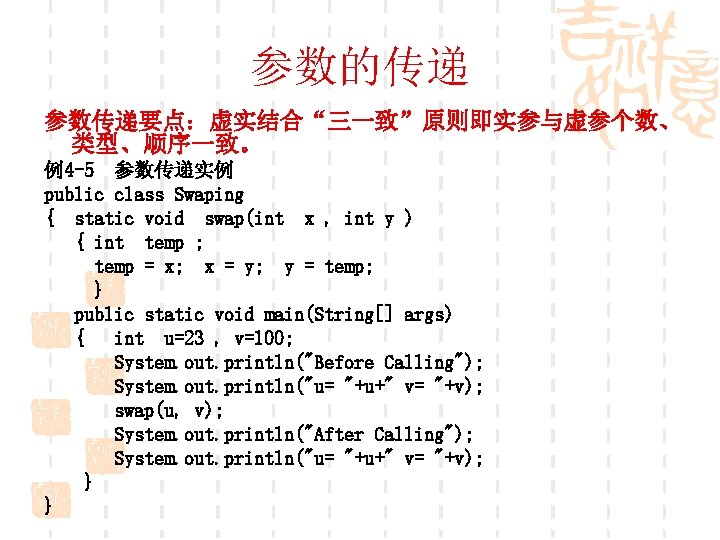
参数的传递 参数传递要点:虚实结合“三一致”原则即实参与虚参个数、 类型、顺序一致。 例4 -5 参数传递实例 public class Swaping { static void swap(int x , int y ) { int temp ; temp = x; x = y; y = temp; } public static void main(String[] args) { int u=23 , v=100; System. out. println("Before Calling"); System. out. println("u= "+u+" v= "+v); swap(u, v); System. out. println("After Calling"); System. out. println("u= "+u+" v= "+v); } }
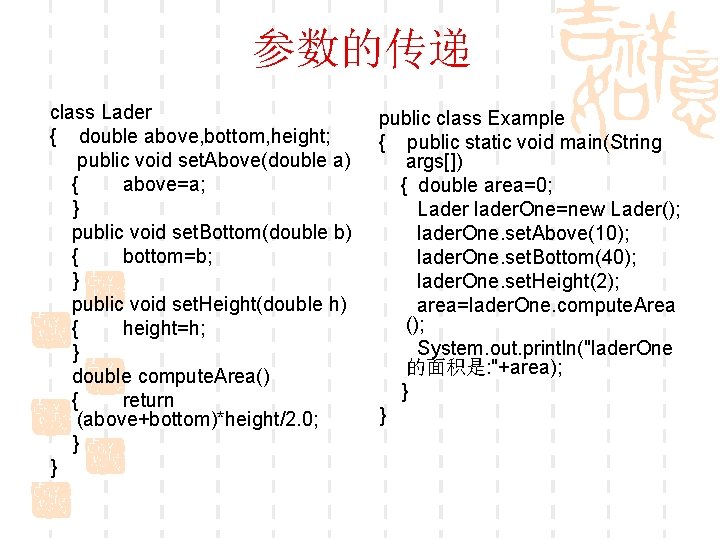
参数的传递 class Lader { double above, bottom, height; public void set. Above(double a) { above=a; } public void set. Bottom(double b) { bottom=b; } public void set. Height(double h) { height=h; } double compute. Area() { return (above+bottom)*height/2. 0; } } public class Example { public static void main(String args[]) { double area=0; Lader lader. One=new Lader(); lader. One. set. Above(10); lader. One. set. Bottom(40); lader. One. set. Height(2); area=lader. One. compute. Area (); System. out. println("lader. One 的面积是: "+area); } }
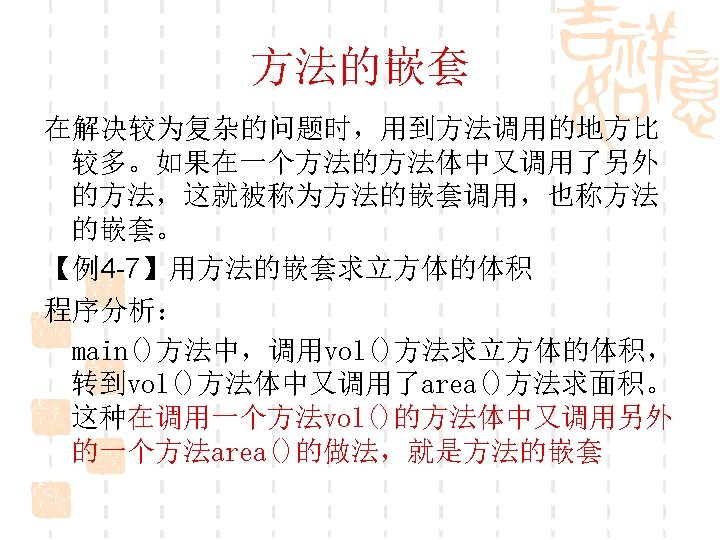
![方法的嵌套 public class Volume public static void mainString args int i5 方法的嵌套 public class Volume { public static void main(String args[ ]) { int i=5,](https://slidetodoc.com/presentation_image_h2/7ed5381bf68b536fb301255cf30d4652/image-24.jpg)
方法的嵌套 public class Volume { public static void main(String args[ ]) { int i=5, j=6, k=7, v; v=vol(i, j, k); System. out. println("立方体的体积为:"+v); } static int vol(int a, int b, int c) { return(a*area(b, c)); } static int area(int x, int y) { return(x*y); } } //求体积的vol()方法 //求面积的area()方法
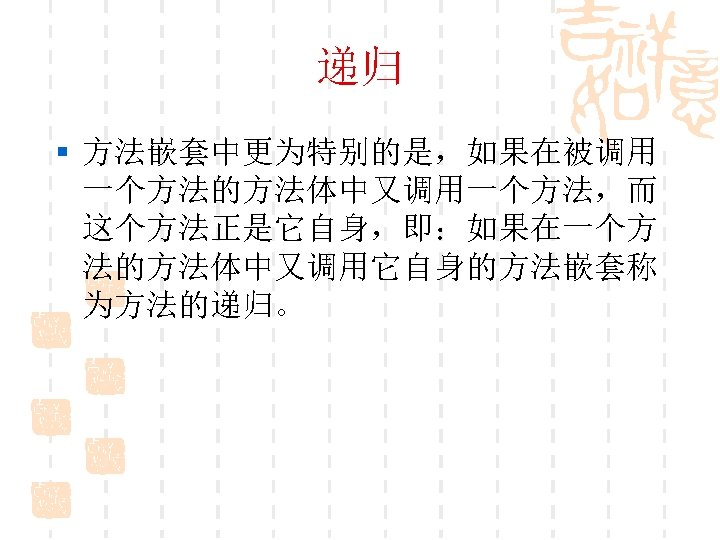
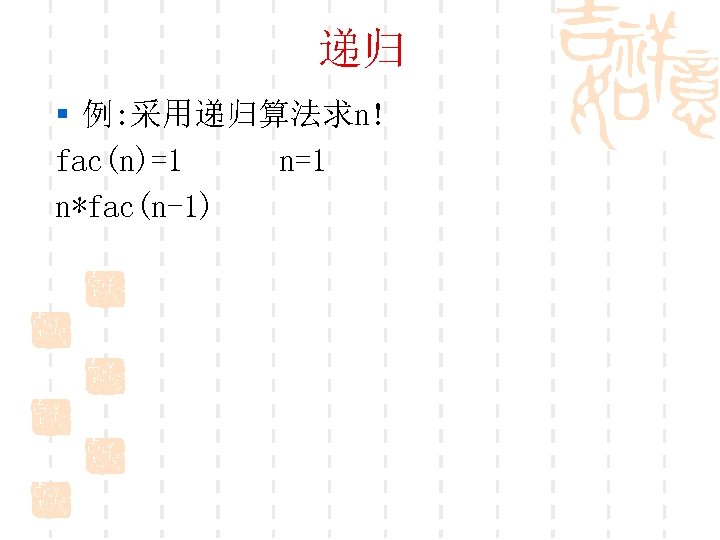
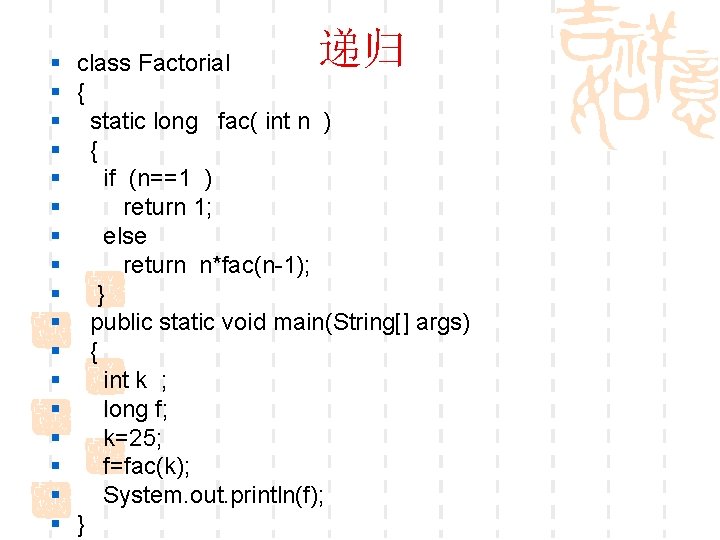
递归 § class Factorial § { § static long fac( int n ) § { § if (n==1 ) § return 1; § else § return n*fac(n-1); § } § public static void main(String[] args) § { § int k ; § long f; § k=25; § f=fac(k); § System. out. println(f); § }
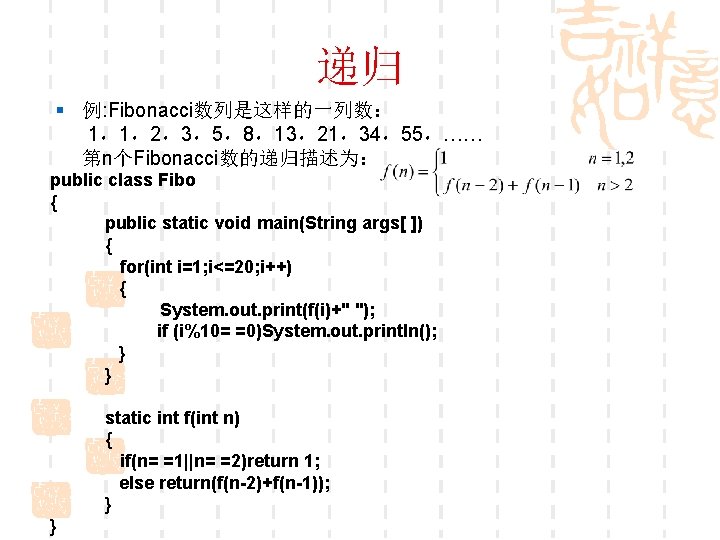
递归 § 例: Fibonacci数列是这样的一列数: 1,1,2,3,5,8,13,21,34,55,…… 第n个Fibonacci数的递归描述为: public class Fibo { public static void main(String args[ ]) { for(int i=1; i<=20; i++) { System. out. print(f(i)+" "); if (i%10= =0)System. out. println(); } } static int f(int n) { if(n= =1||n= =2)return 1; else return(f(n-2)+f(n-1)); } }
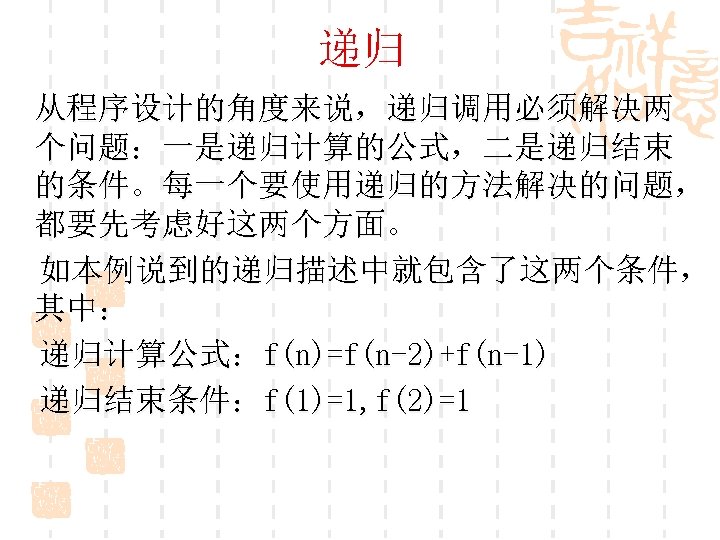
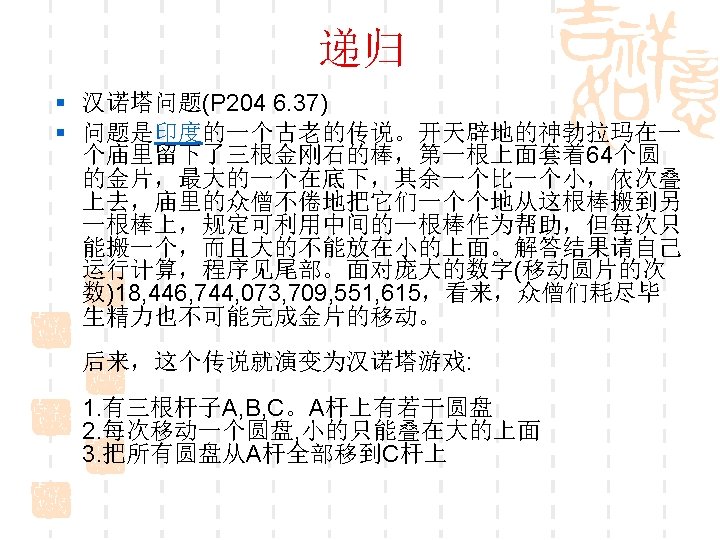
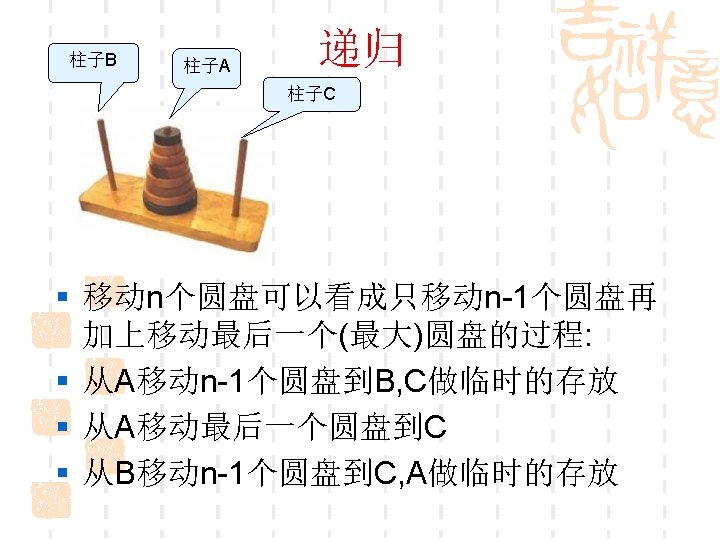
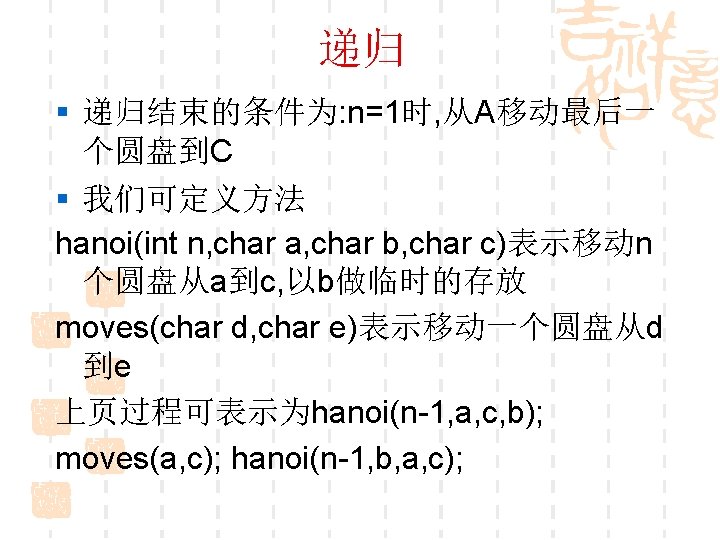
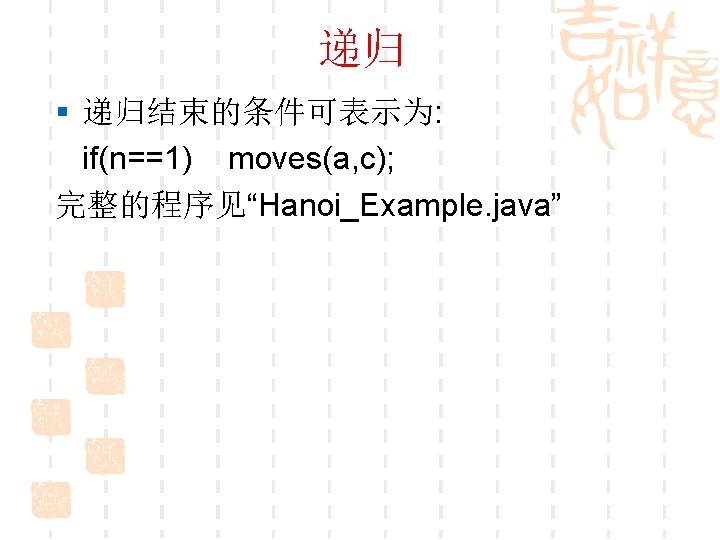
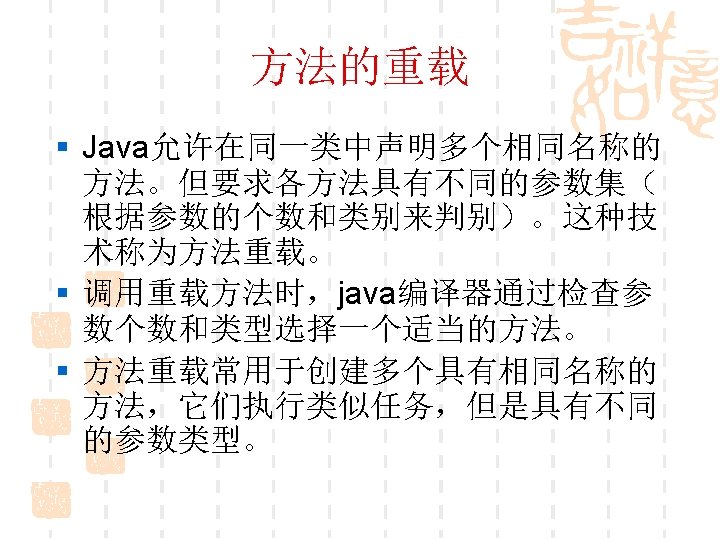
Int max(int x int y)
Sum0
Int.max
Public void drawsquare(int x, int y, int len)
Divideint
Interface calculator public int add class test
Absolute max vs local max
Max int c++
Maxint
Int factorial(int n)
Void swap
Interface myinterface int foo(int x)
Void
Const int arduino adalah
Int main(int argc, char** argv)
Mainint
Int main() int num=4
Disadvantages of bureaucracy by max weber
Frotáž umělci
Pr max budowski
Flow g cut
Max weber bureaucracy characteristics
Alireza akhavan github
Altera
Alienação marx
Max hickey
Hermeneutic phenomenology
Pr max budowski
Max's restaurant value proposition
Max z = 3x1 + 5x2 metodo simplex
Max wertheimer gestalt psychology
Max weber zhaw
Political democracy cartoon
Omega plus max