Game Extras Pepper Arrays Vs Array List for
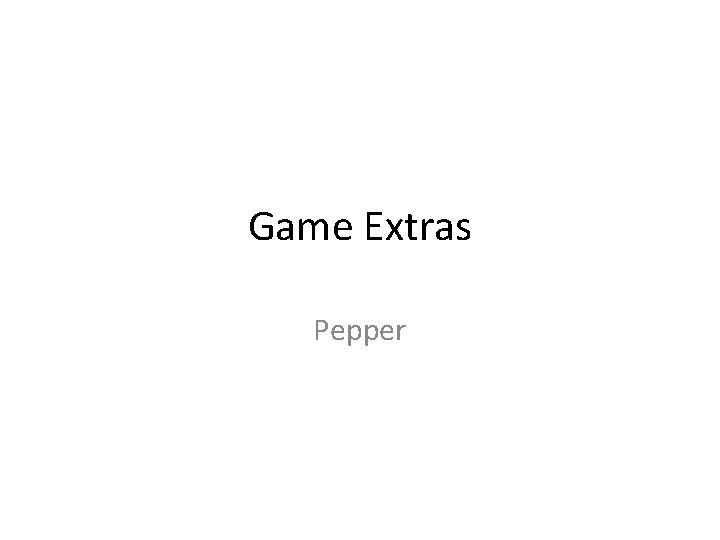
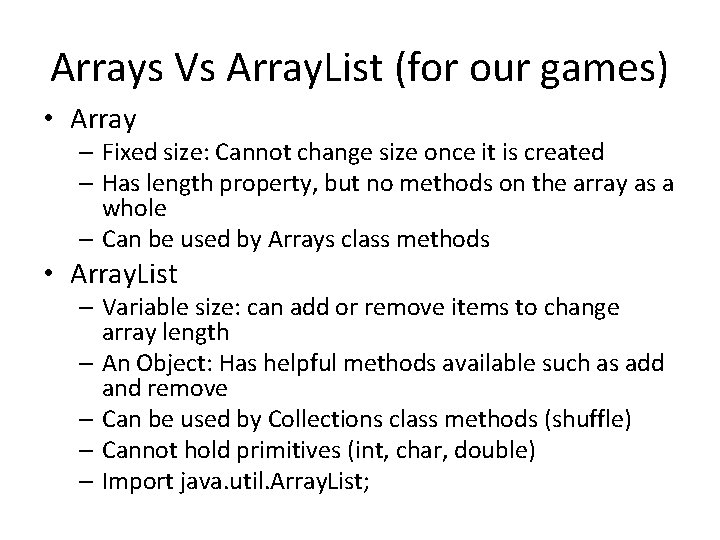
![Review Array syntax • Card[] c : creates variable to hold an array of Review Array syntax • Card[] c : creates variable to hold an array of](https://slidetodoc.com/presentation_image_h2/ab7771f04a5f5a570753f68de3e341b6/image-3.jpg)
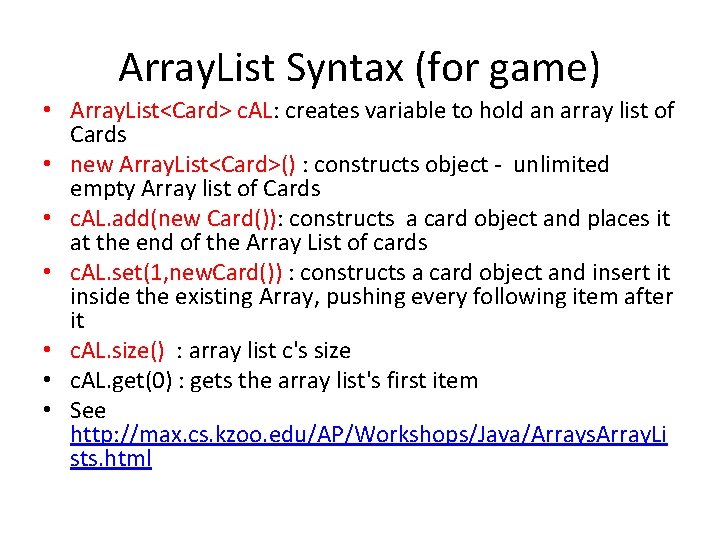
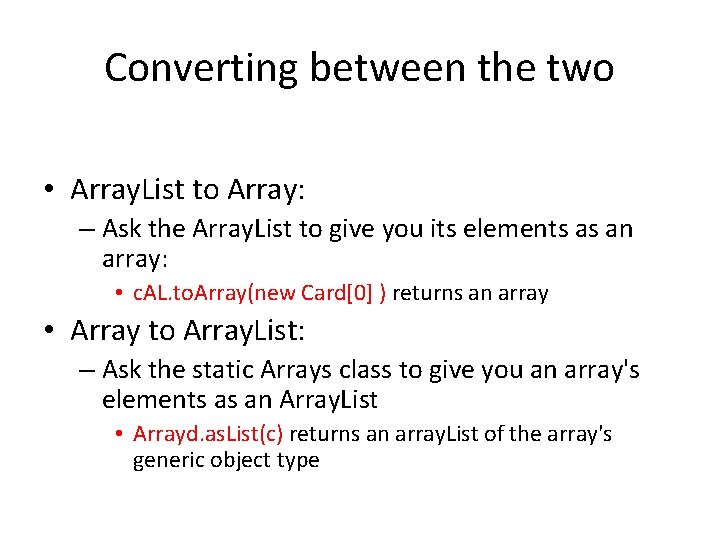
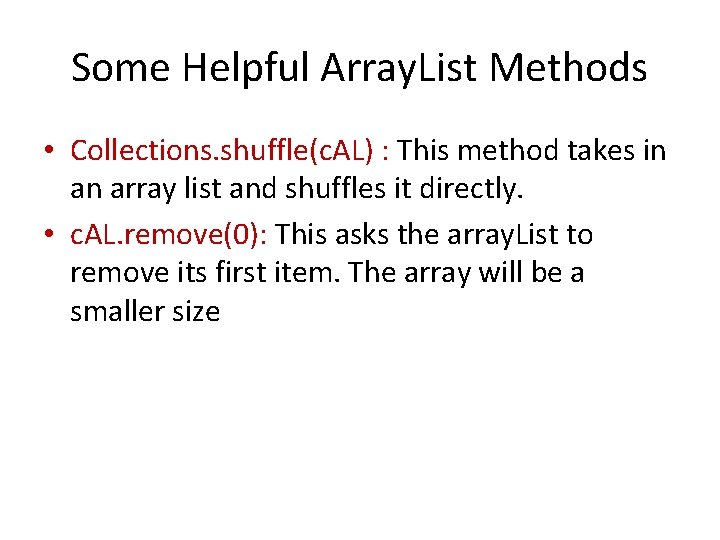
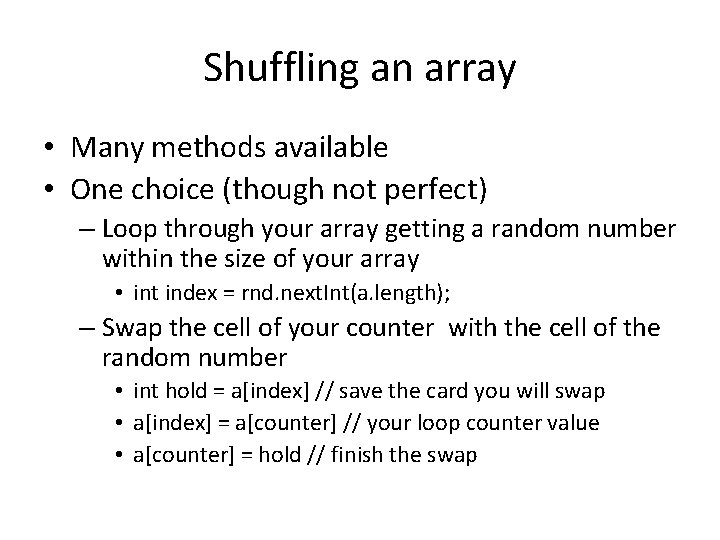
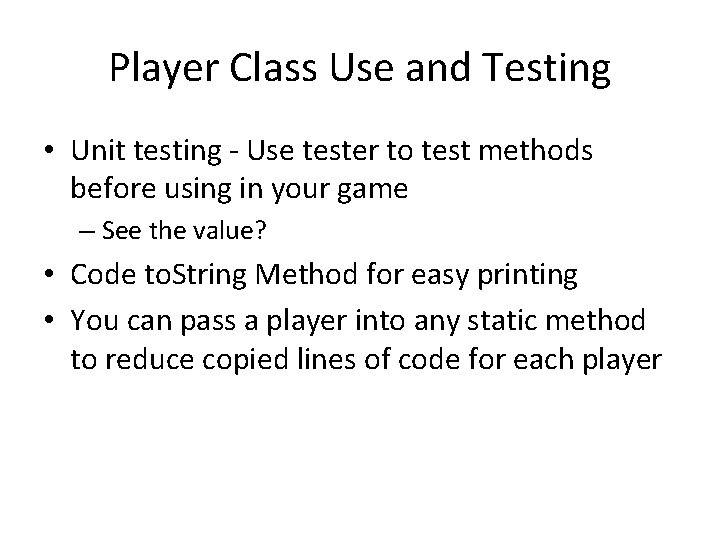
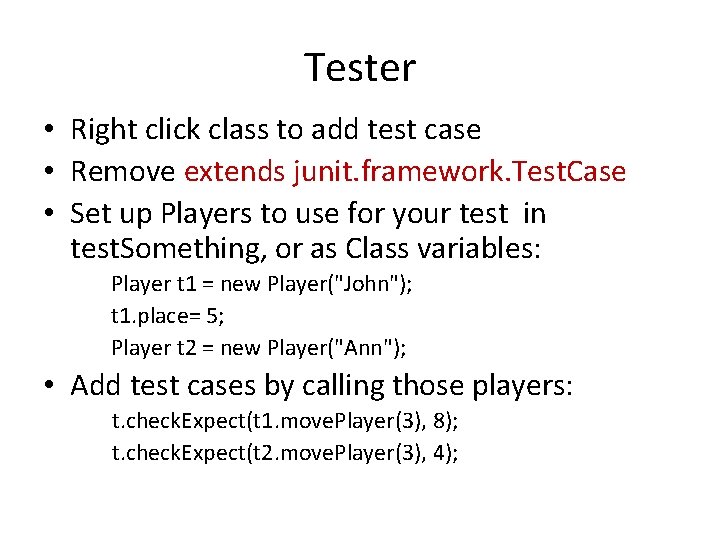
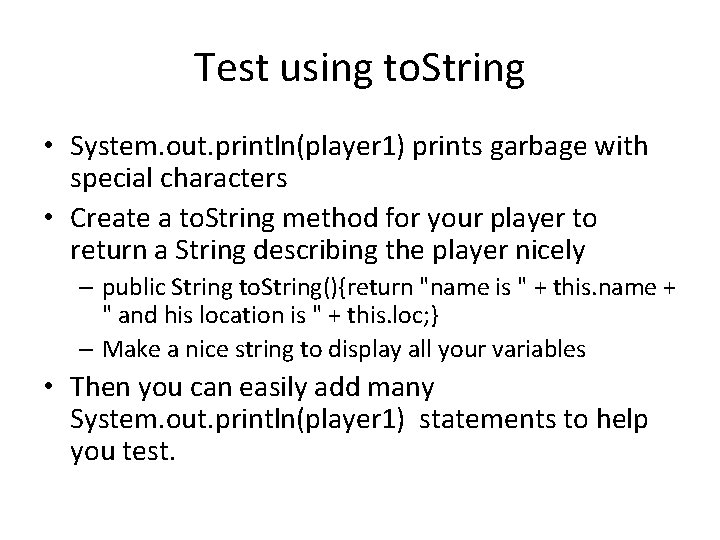
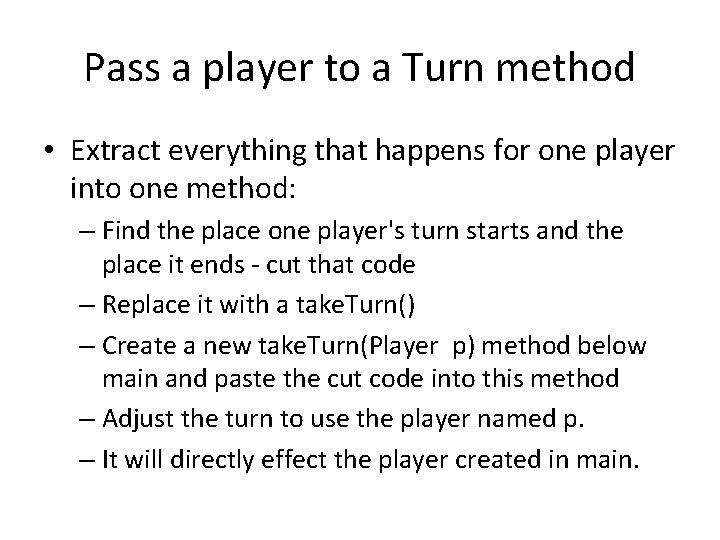
- Slides: 11
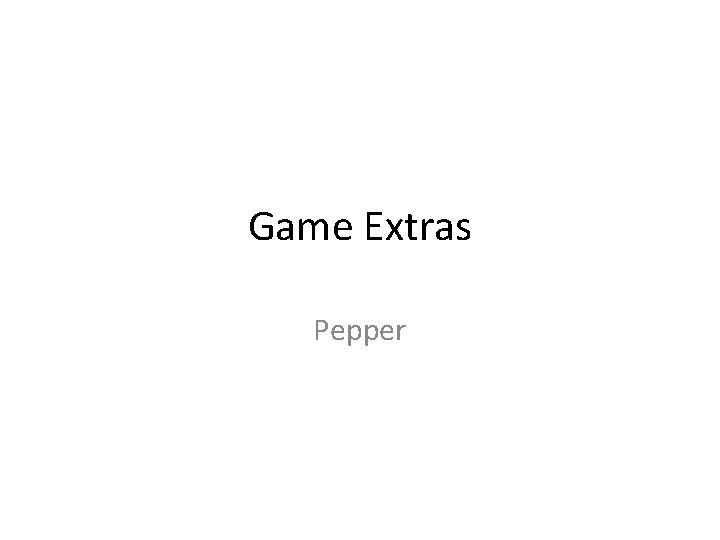
Game Extras Pepper
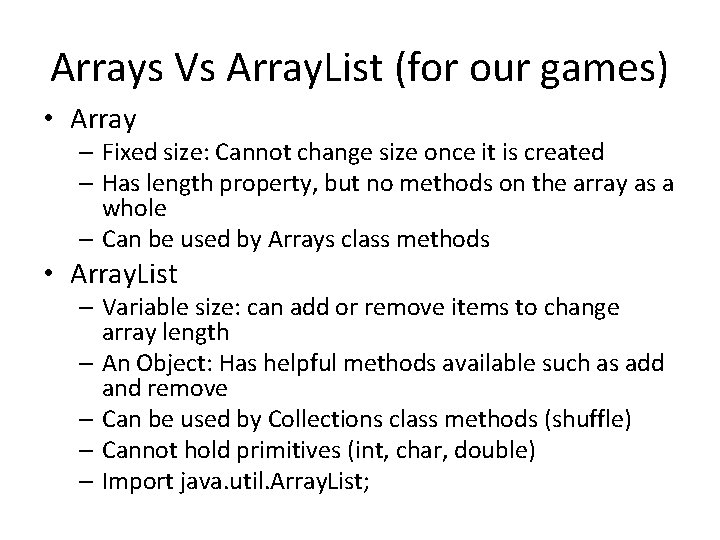
Arrays Vs Array. List (for our games) • Array – Fixed size: Cannot change size once it is created – Has length property, but no methods on the array as a whole – Can be used by Arrays class methods • Array. List – Variable size: can add or remove items to change array length – An Object: Has helpful methods available such as add and remove – Can be used by Collections class methods (shuffle) – Cannot hold primitives (int, char, double) – Import java. util. Array. List;
![Review Array syntax Card c creates variable to hold an array of Review Array syntax • Card[] c : creates variable to hold an array of](https://slidetodoc.com/presentation_image_h2/ab7771f04a5f5a570753f68de3e341b6/image-3.jpg)
Review Array syntax • Card[] c : creates variable to hold an array of Cards • new Card[5] : constructs object - 5 empty array spots for Cards • c[0] = new Card() : constructs a card object and places it into c[0] • c. length : array c's size
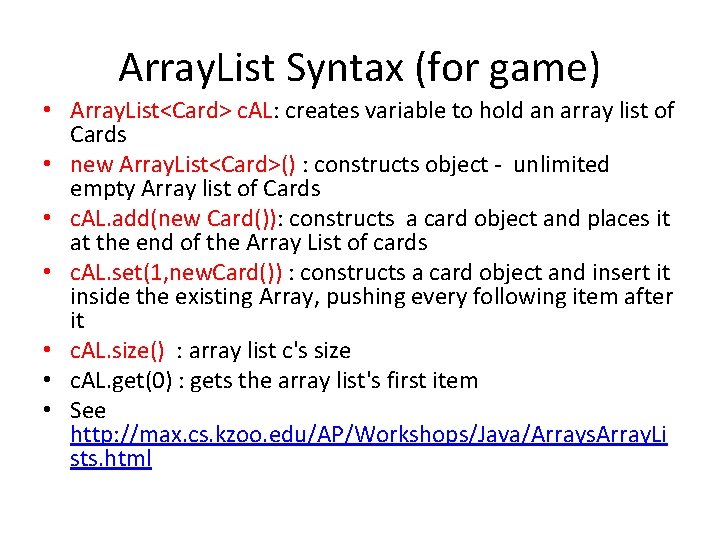
Array. List Syntax (for game) • Array. List<Card> c. AL: creates variable to hold an array list of Cards • new Array. List<Card>() : constructs object - unlimited empty Array list of Cards • c. AL. add(new Card()): constructs a card object and places it at the end of the Array List of cards • c. AL. set(1, new. Card()) : constructs a card object and insert it inside the existing Array, pushing every following item after it • c. AL. size() : array list c's size • c. AL. get(0) : gets the array list's first item • See http: //max. cs. kzoo. edu/AP/Workshops/Java/Arrays. Array. Li sts. html
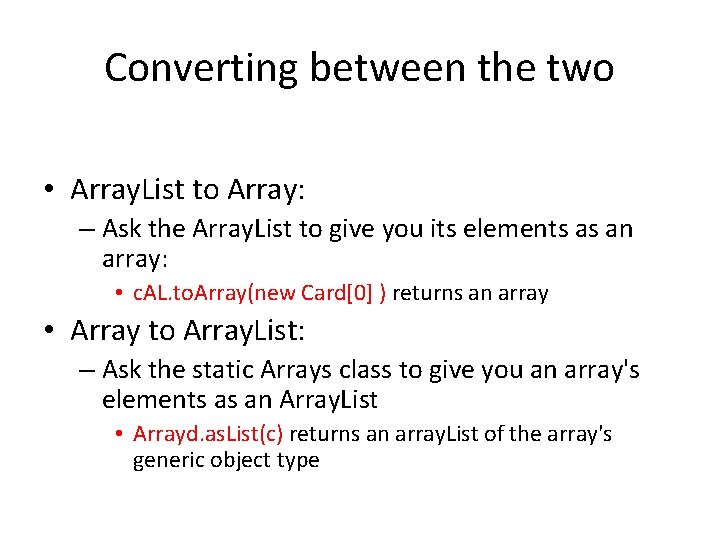
Converting between the two • Array. List to Array: – Ask the Array. List to give you its elements as an array: • c. AL. to. Array(new Card[0] ) returns an array • Array to Array. List: – Ask the static Arrays class to give you an array's elements as an Array. List • Arrayd. as. List(c) returns an array. List of the array's generic object type
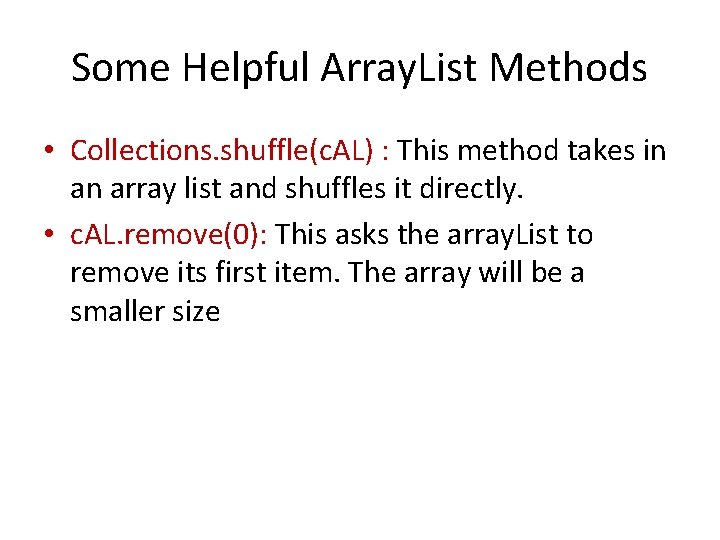
Some Helpful Array. List Methods • Collections. shuffle(c. AL) : This method takes in an array list and shuffles it directly. • c. AL. remove(0): This asks the array. List to remove its first item. The array will be a smaller size
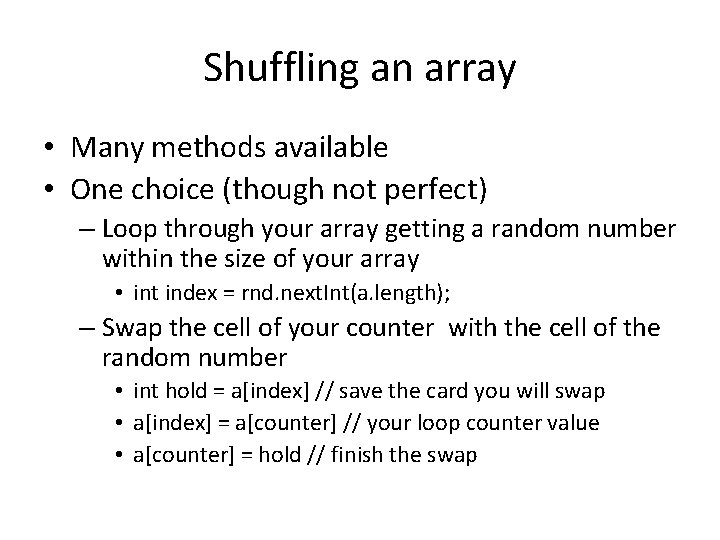
Shuffling an array • Many methods available • One choice (though not perfect) – Loop through your array getting a random number within the size of your array • int index = rnd. next. Int(a. length); – Swap the cell of your counter with the cell of the random number • int hold = a[index] // save the card you will swap • a[index] = a[counter] // your loop counter value • a[counter] = hold // finish the swap
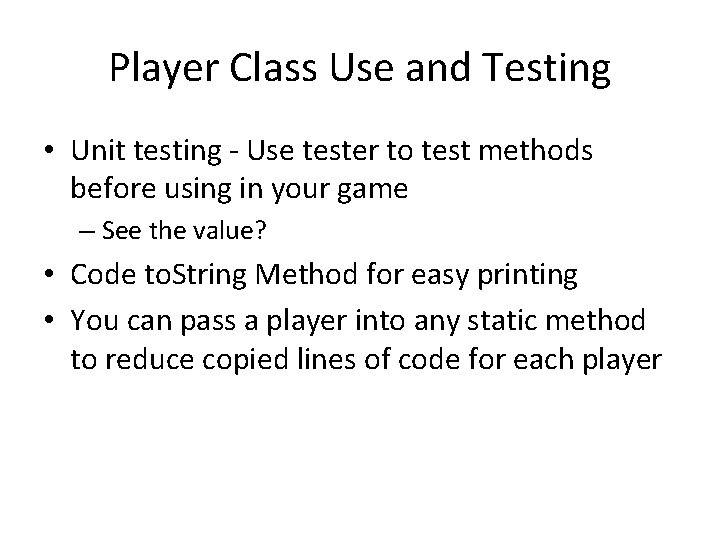
Player Class Use and Testing • Unit testing - Use tester to test methods before using in your game – See the value? • Code to. String Method for easy printing • You can pass a player into any static method to reduce copied lines of code for each player
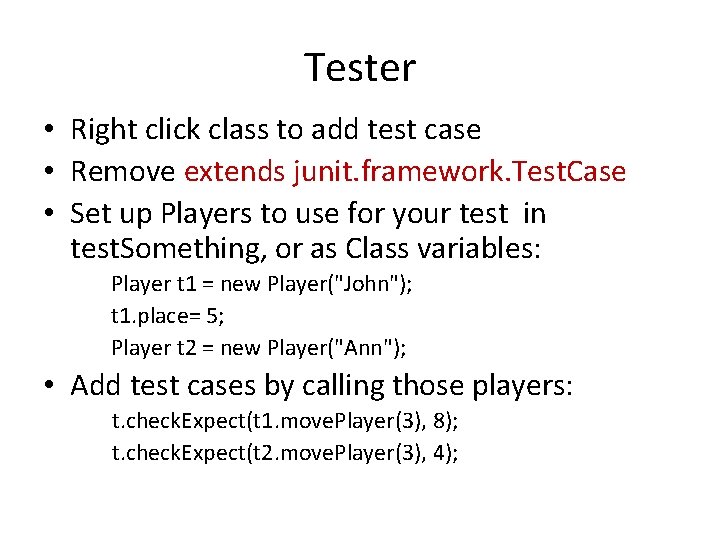
Tester • Right click class to add test case • Remove extends junit. framework. Test. Case • Set up Players to use for your test in test. Something, or as Class variables: Player t 1 = new Player("John"); t 1. place= 5; Player t 2 = new Player("Ann"); • Add test cases by calling those players: t. check. Expect(t 1. move. Player(3), 8); t. check. Expect(t 2. move. Player(3), 4);
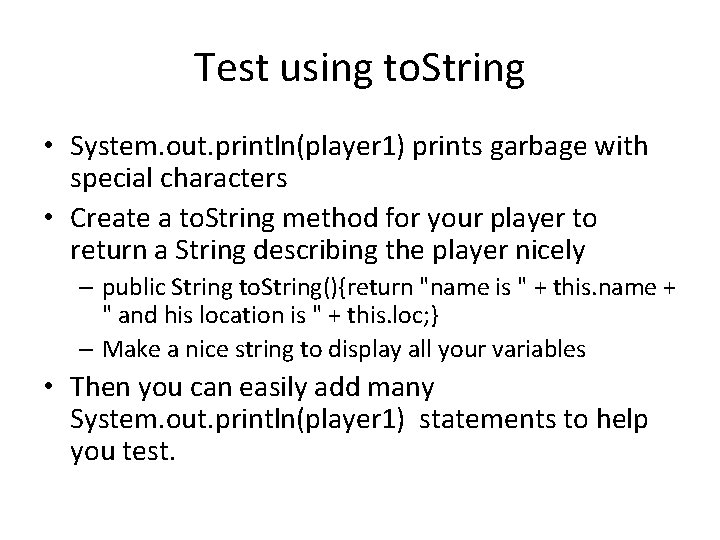
Test using to. String • System. out. println(player 1) prints garbage with special characters • Create a to. String method for your player to return a String describing the player nicely – public String to. String(){return "name is " + this. name + " and his location is " + this. loc; } – Make a nice string to display all your variables • Then you can easily add many System. out. println(player 1) statements to help you test.
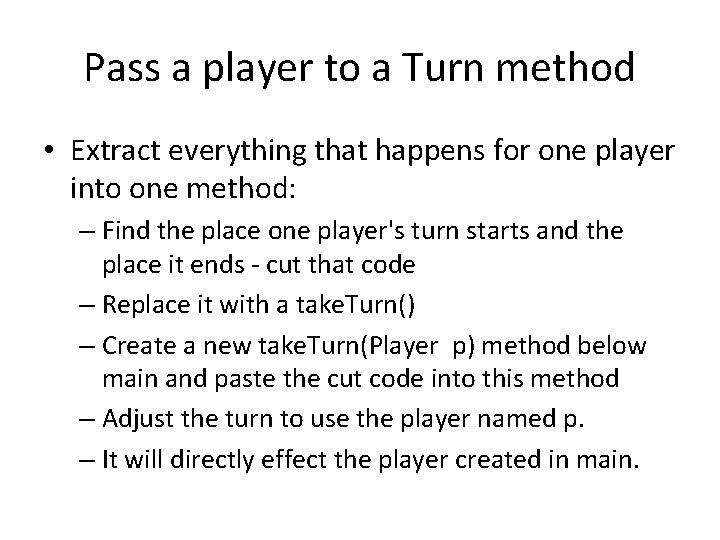
Pass a player to a Turn method • Extract everything that happens for one player into one method: – Find the place one player's turn starts and the place it ends - cut that code – Replace it with a take. Turn() – Create a new take. Turn(Player p) method below main and paste the cut code into this method – Adjust the turn to use the player named p. – It will directly effect the player created in main.