From C to C Why C is much
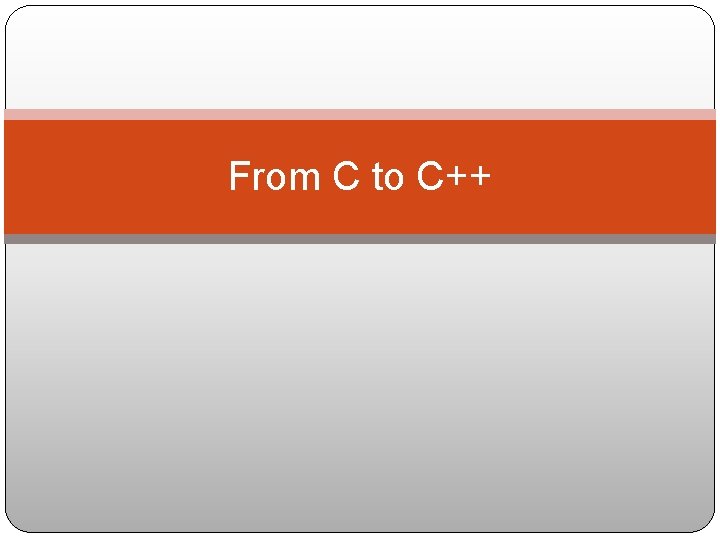
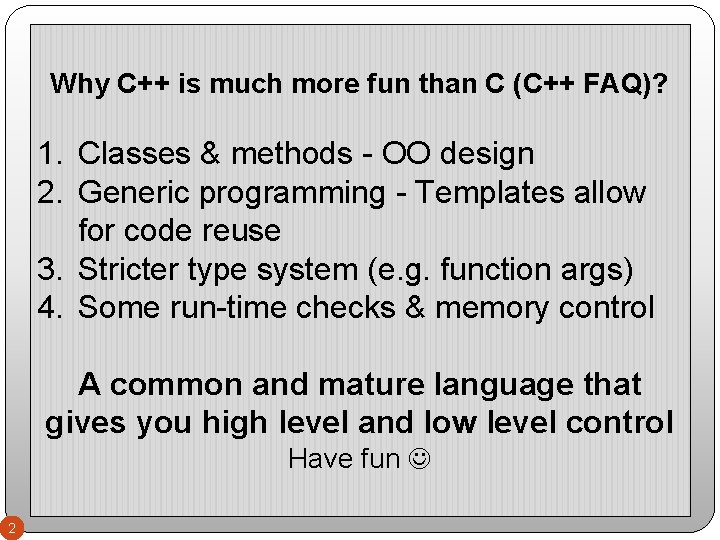
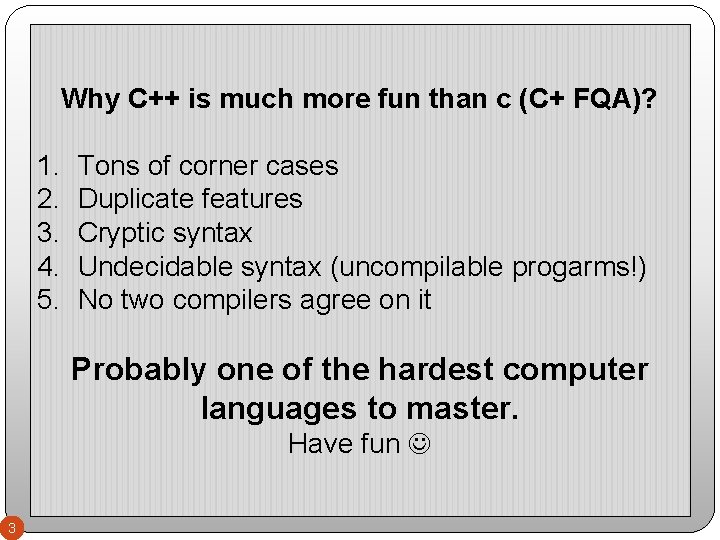
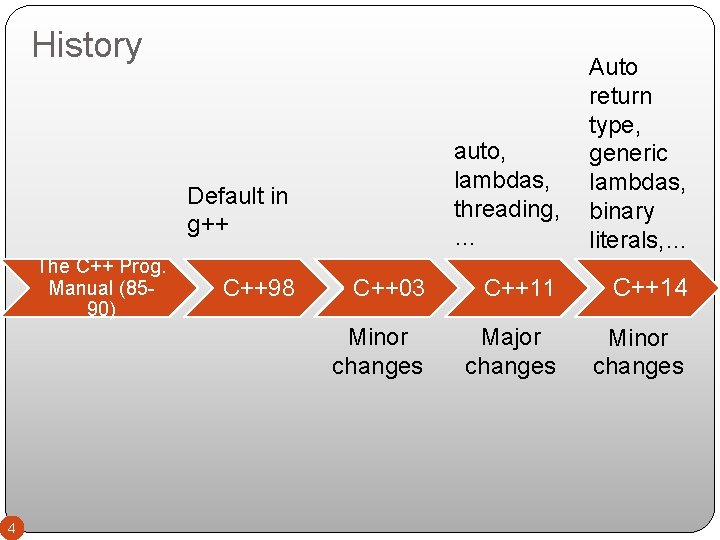
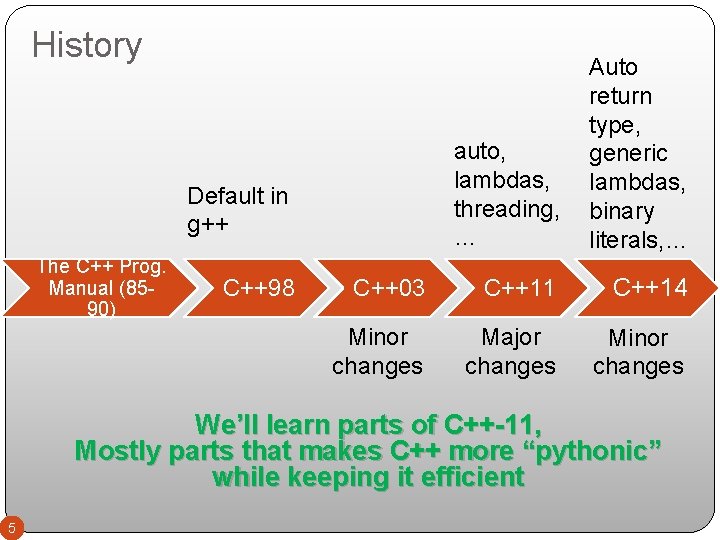
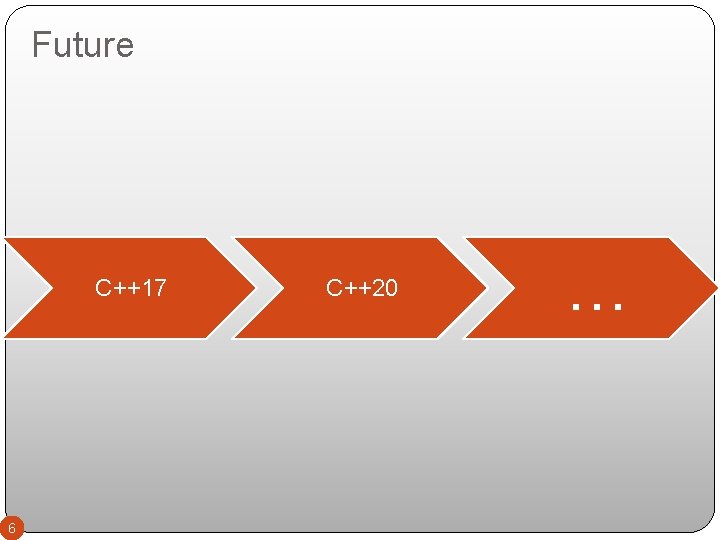
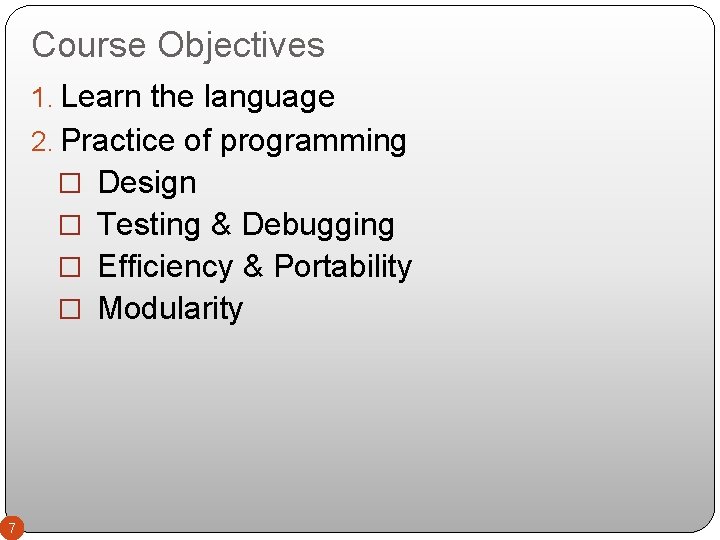
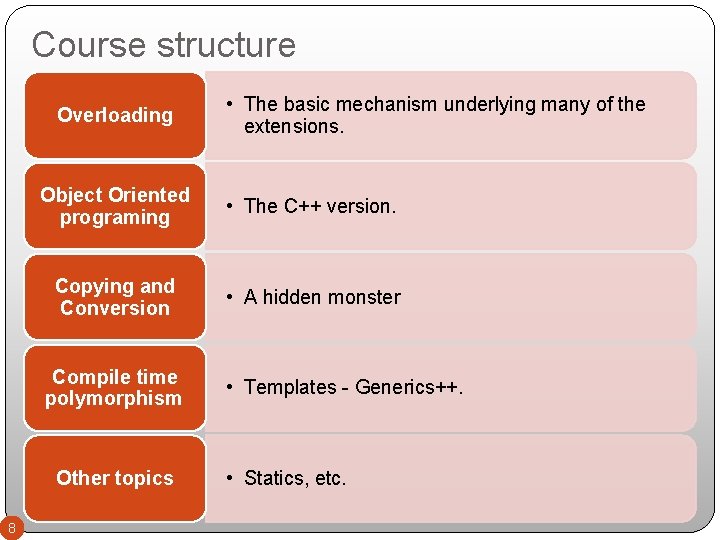
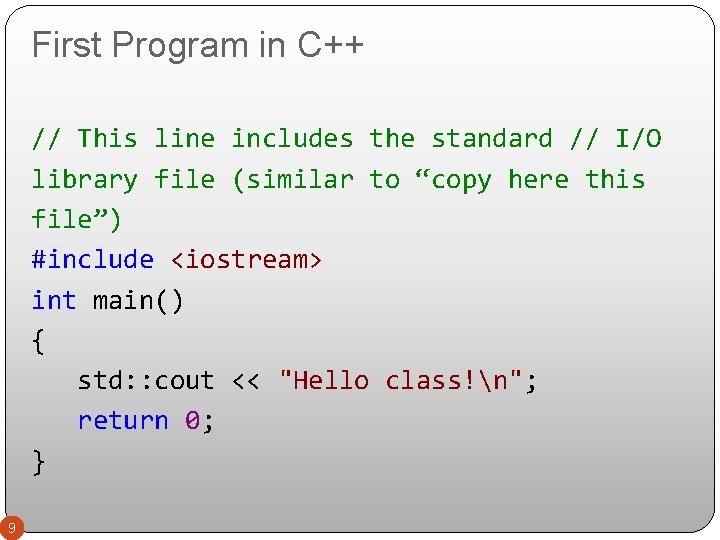
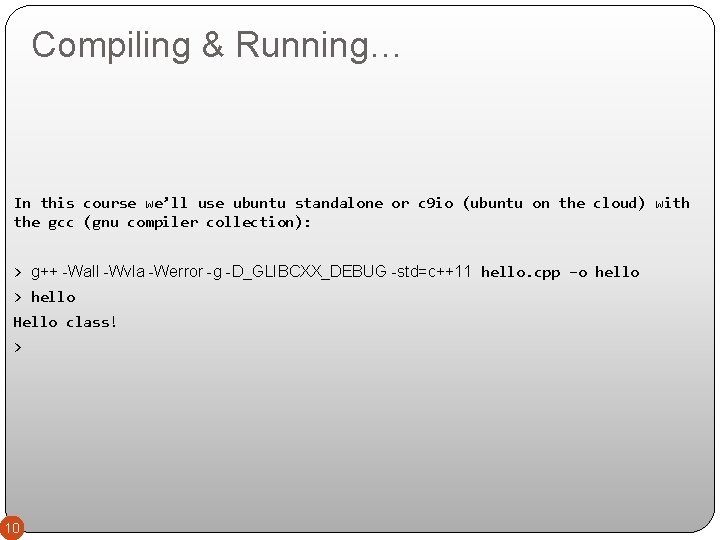
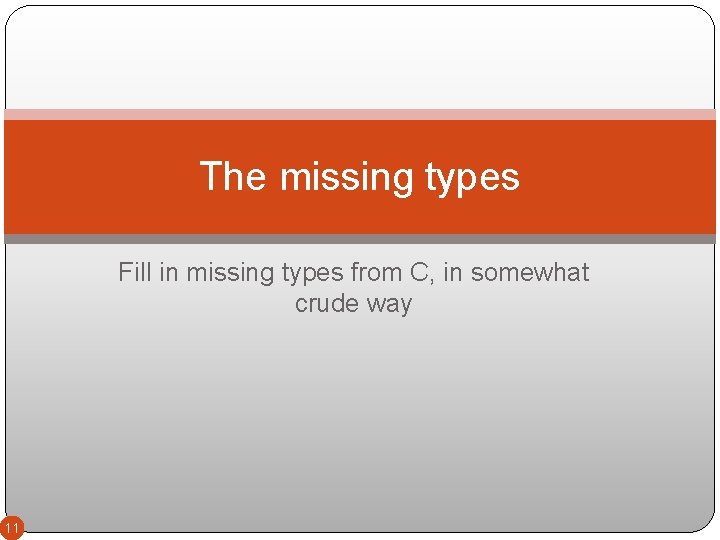
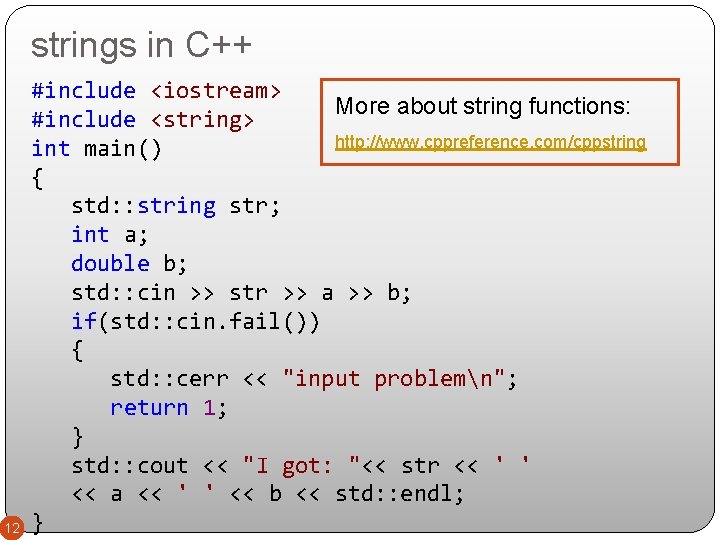
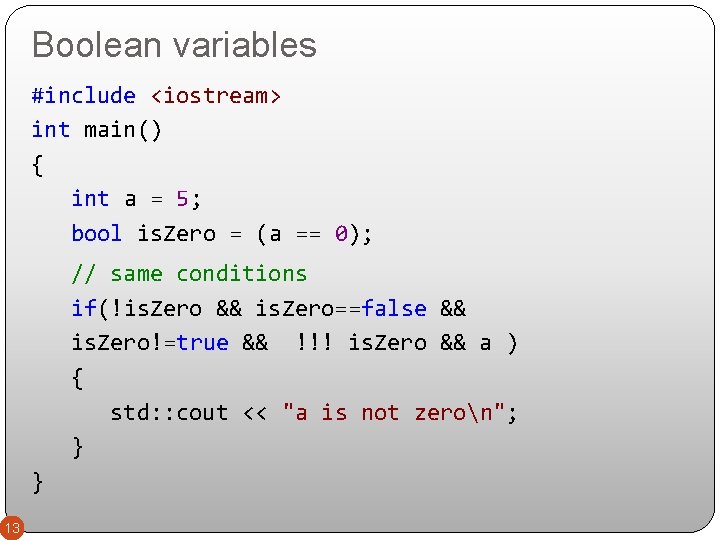
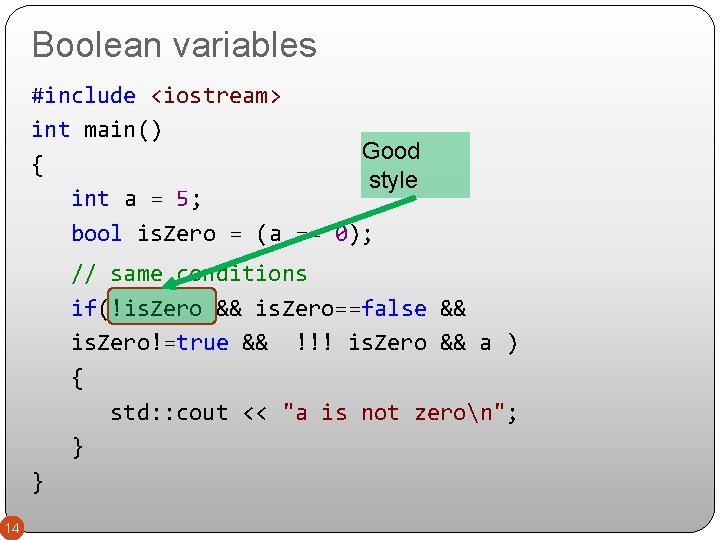
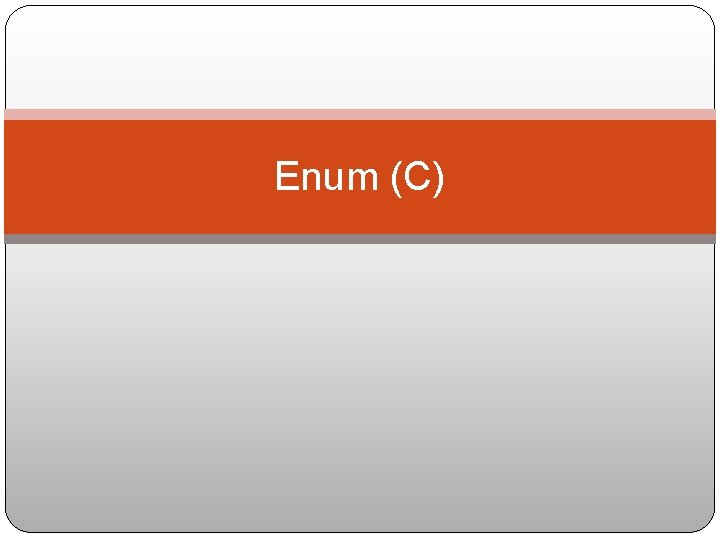
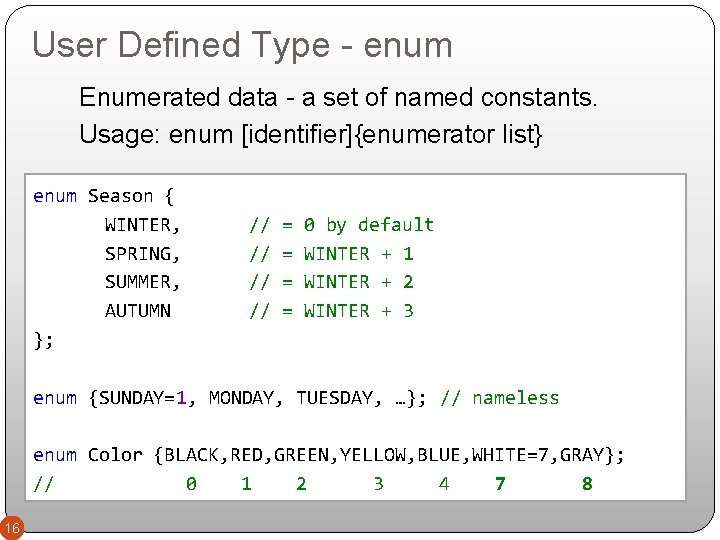
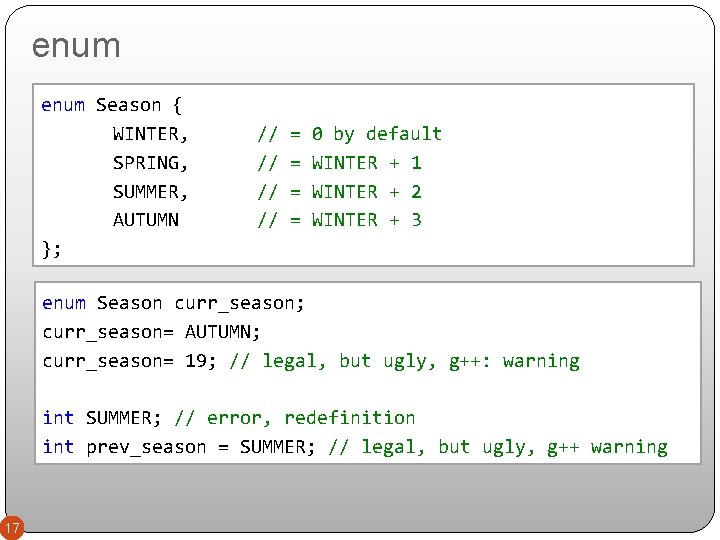
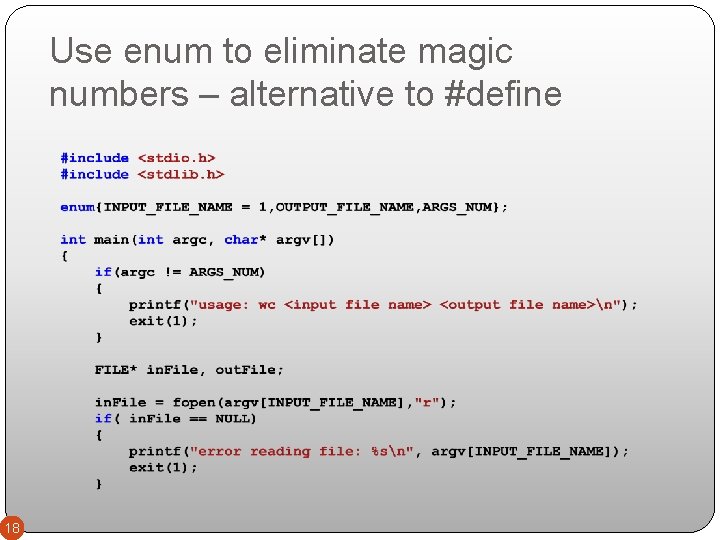
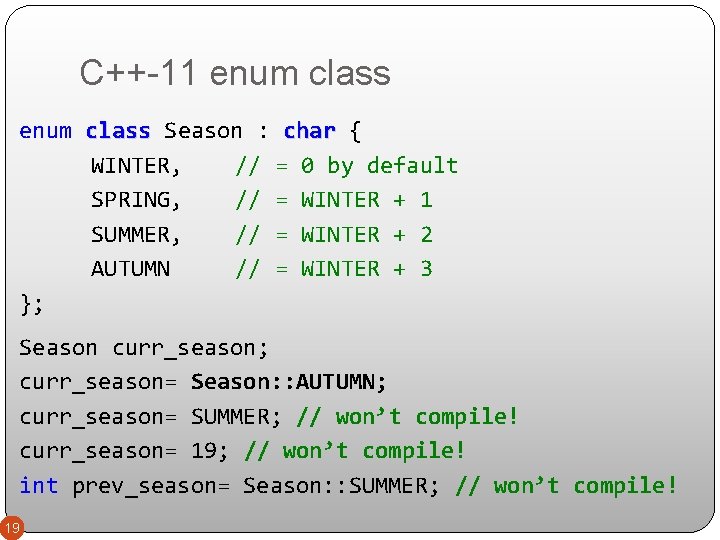
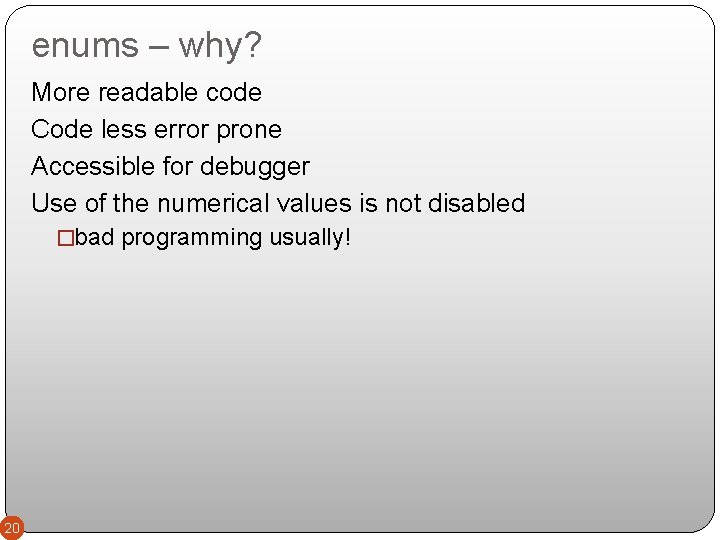
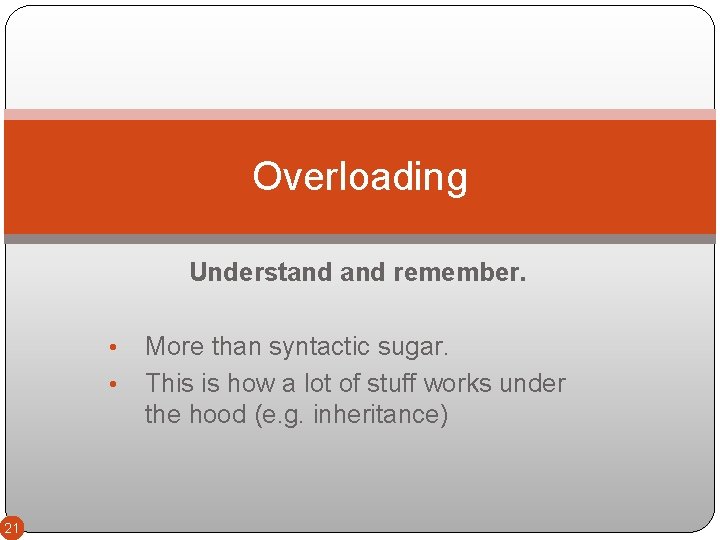
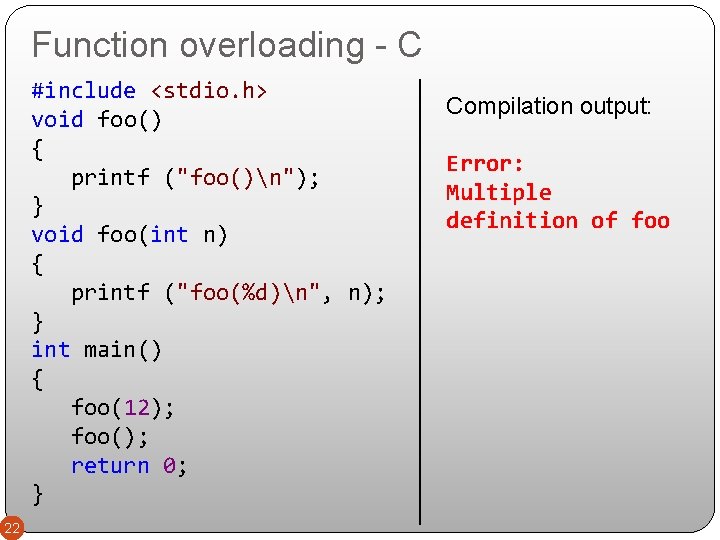
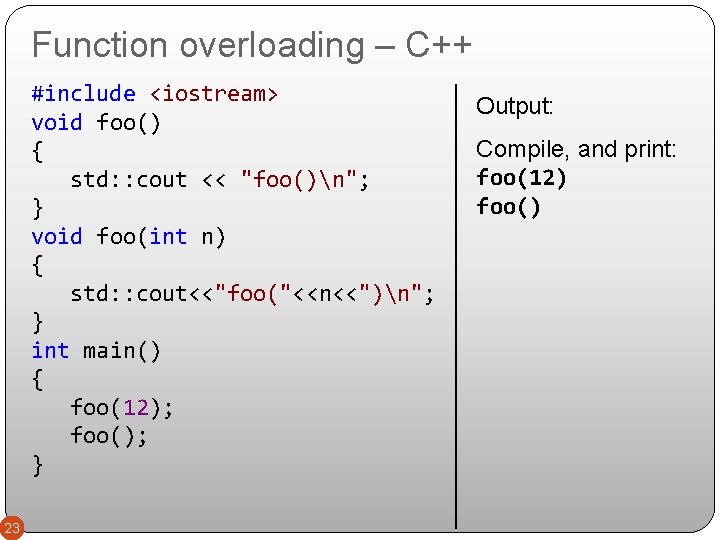
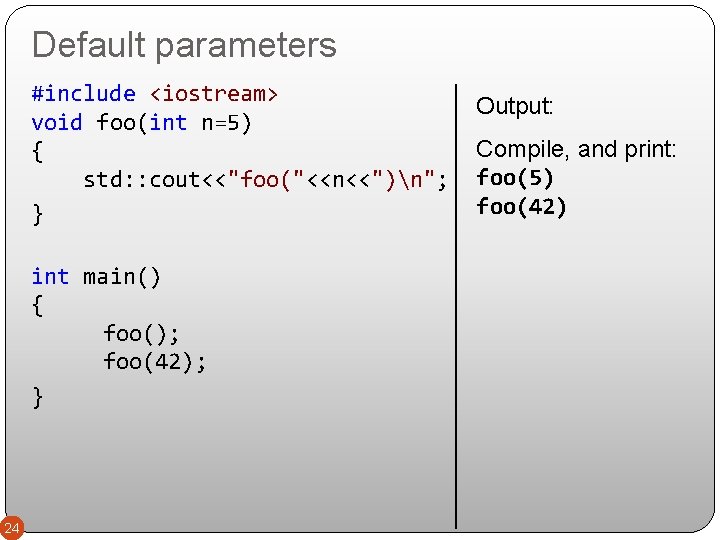
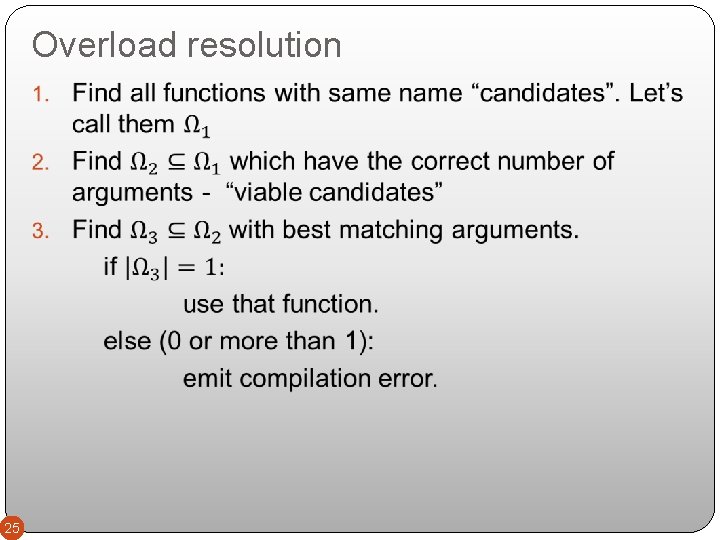
- Slides: 25
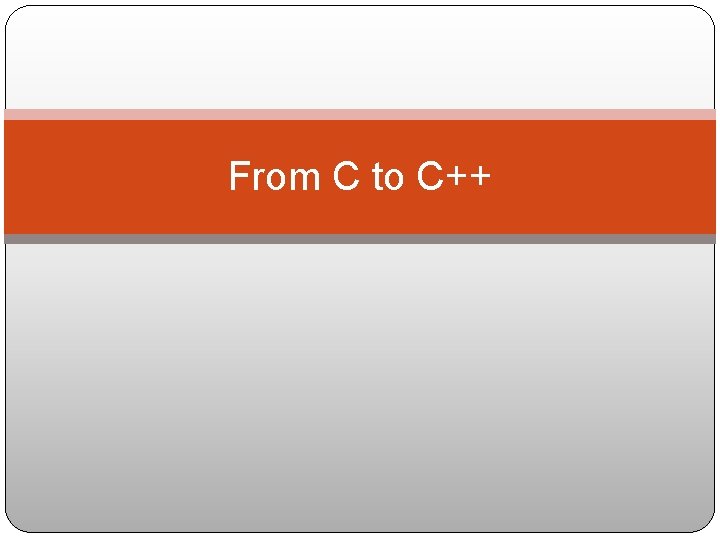
From C to C++
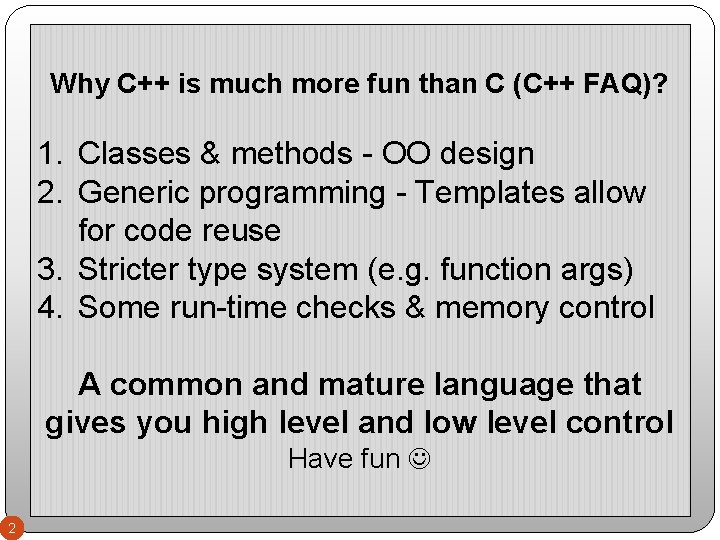
Why C++ is much more fun than C (C++ FAQ)? 1. Classes & methods - OO design 2. Generic programming - Templates allow for code reuse 3. Stricter type system (e. g. function args) 4. Some run-time checks & memory control A common and mature language that gives you high level and low level control Have fun 2
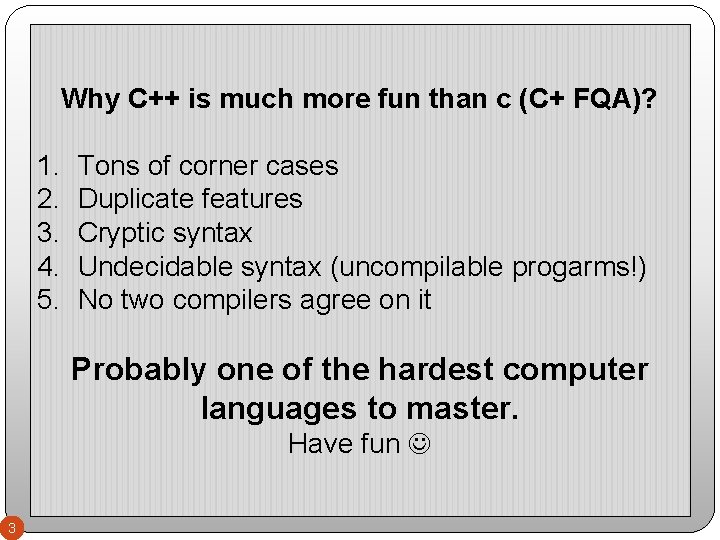
Why C++ is much more fun than c (C+ FQA)? 1. 2. 3. 4. 5. Tons of corner cases Duplicate features Cryptic syntax Undecidable syntax (uncompilable progarms!) No two compilers agree on it Probably one of the hardest computer languages to master. Have fun 3
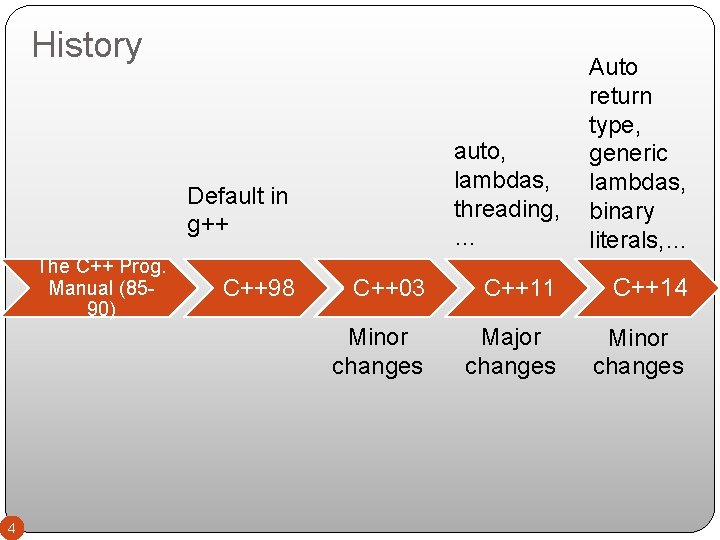
History auto, lambdas, threading, … Auto return type, generic lambdas, binary literals, … C++03 C++11 C++14 Minor changes Major changes Default in g++ The C++ Prog. Manual (8590) 4 C++98 Minor changes
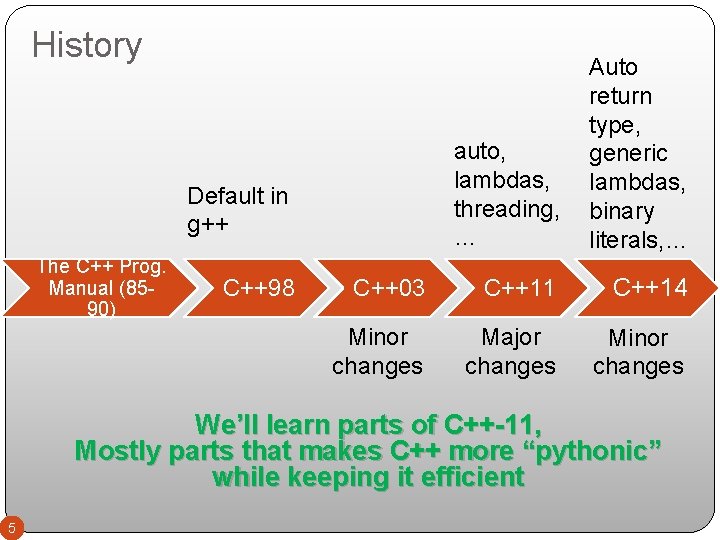
History auto, lambdas, threading, … Auto return type, generic lambdas, binary literals, … C++03 C++11 C++14 Minor changes Major changes Default in g++ The C++ Prog. Manual (8590) C++98 Minor changes We’ll learn parts of C++-11, Mostly parts that makes C++ more “pythonic” while keeping it efficient 5
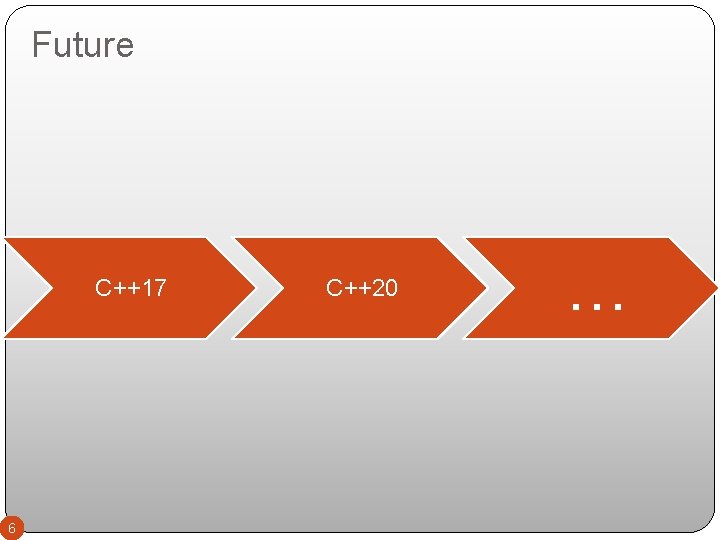
Future C++17 6 C++20 …
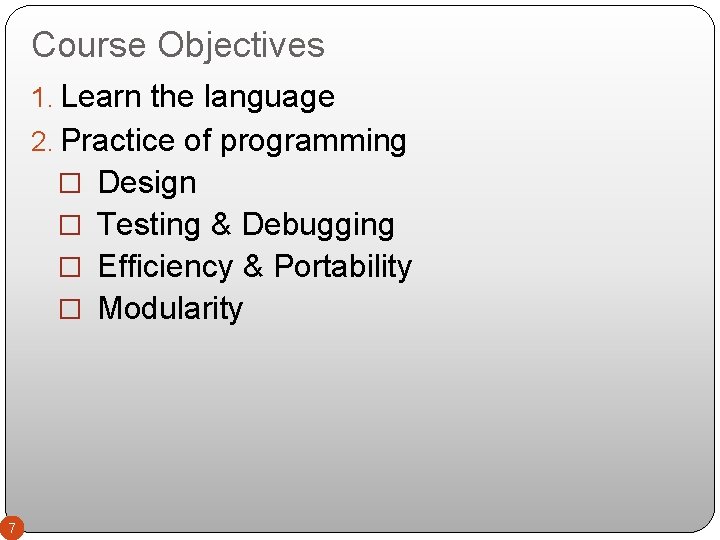
Course Objectives 1. Learn the language 2. Practice of programming � Design � Testing & Debugging � Efficiency & Portability � Modularity 7
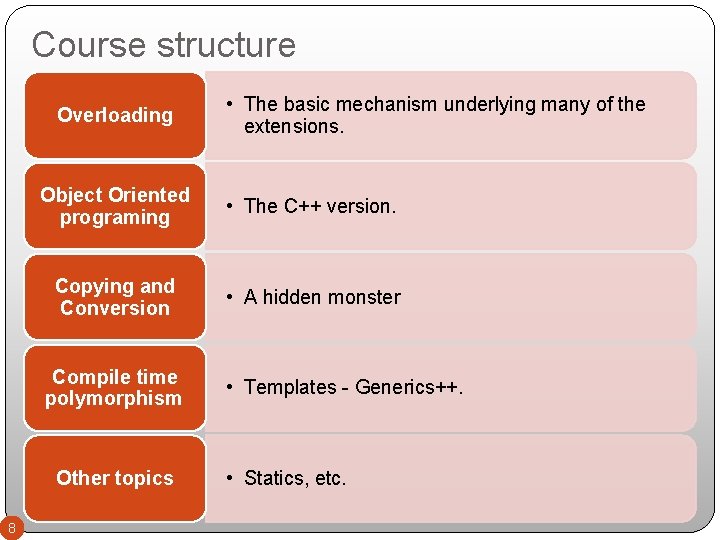
Course structure Overloading Object Oriented programing • The C++ version. Copying and Conversion • A hidden monster Compile time polymorphism Other topics 8 • The basic mechanism underlying many of the extensions. • Templates - Generics++. • Statics, etc.
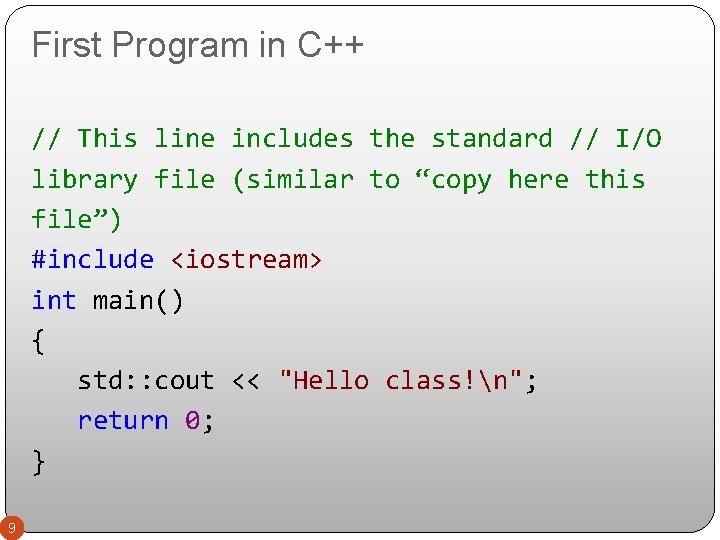
First Program in C++ // This line includes the standard // I/O library file (similar to “copy here this file”) #include <iostream> int main() { std: : cout << "Hello class!n"; return 0; } 9
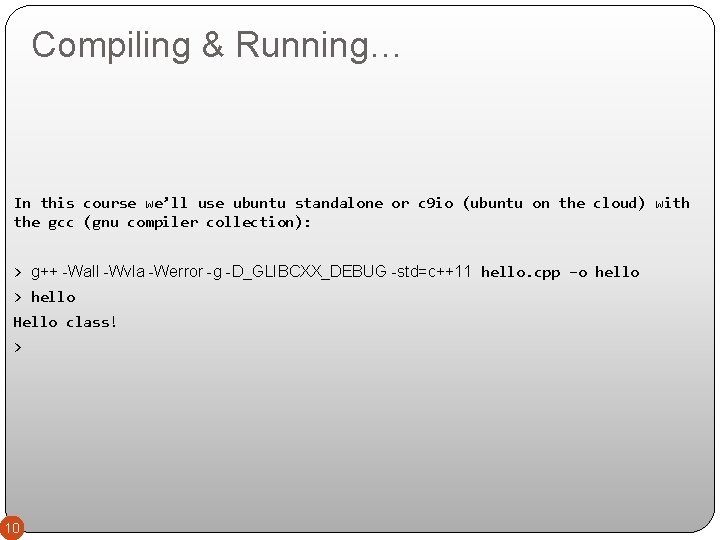
Compiling & Running… In this course we’ll use ubuntu standalone or c 9 io (ubuntu on the cloud) with the gcc (gnu compiler collection): > g++ -Wall -Wvla -Werror -g -D_GLIBCXX_DEBUG -std=c++11 hello. cpp –o hello > hello Hello class! > 10
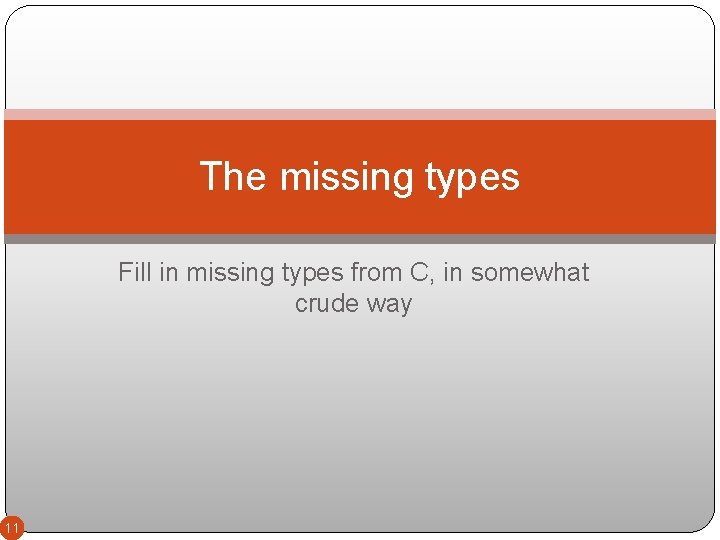
The missing types Fill in missing types from C, in somewhat crude way 11
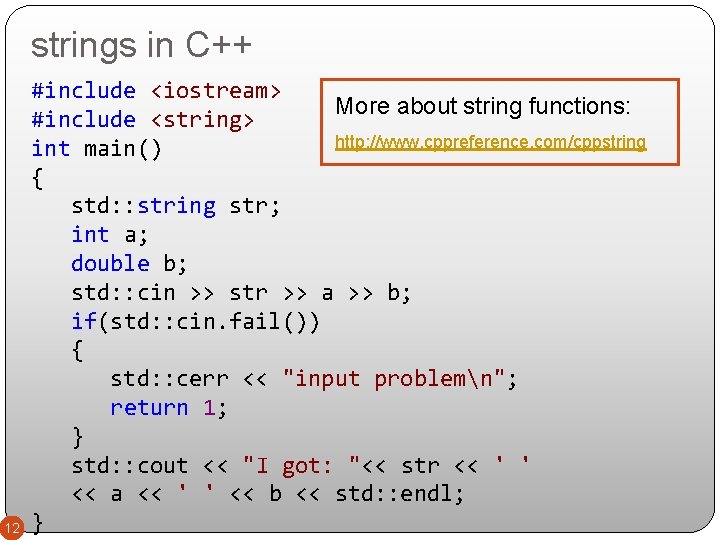
strings in C++ 12 #include <iostream> More about string functions: #include <string> http: //www. cppreference. com/cppstring int main() { std: : string str; int a; double b; std: : cin >> str >> a >> b; if(std: : cin. fail()) { std: : cerr << "input problemn"; return 1; } std: : cout << "I got: "<< str << ' ' << a << ' ' << b << std: : endl; }
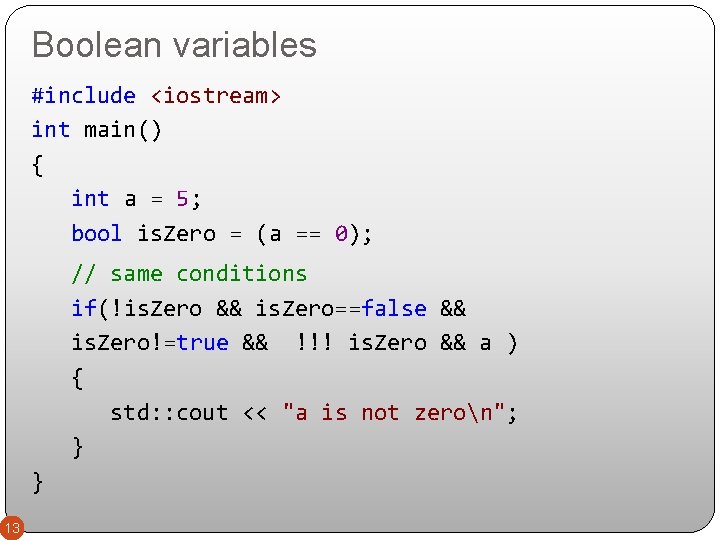
Boolean variables #include <iostream> int main() { int a = 5; bool is. Zero = (a == 0); // same conditions if(!is. Zero && is. Zero==false && is. Zero!=true && !!! is. Zero && a ) { std: : cout << "a is not zeron"; } } 13
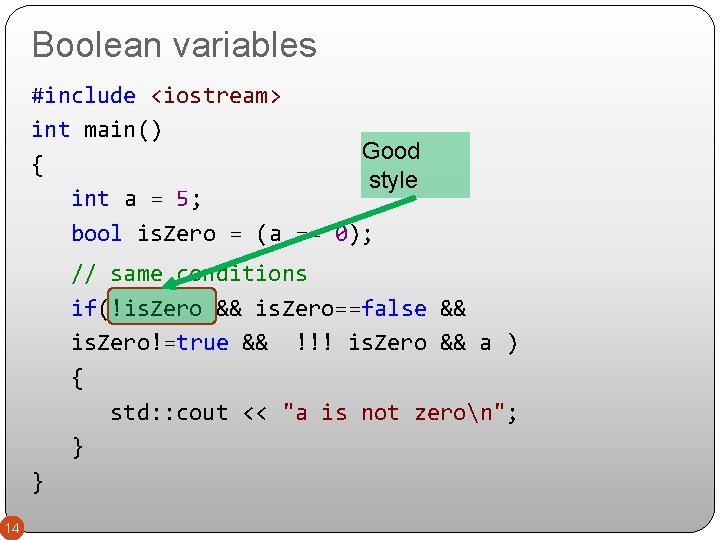
Boolean variables #include <iostream> int main() Good { style int a = 5; bool is. Zero = (a == 0); // same conditions if(!is. Zero && is. Zero==false && is. Zero!=true && !!! is. Zero && a ) { std: : cout << "a is not zeron"; } } 14
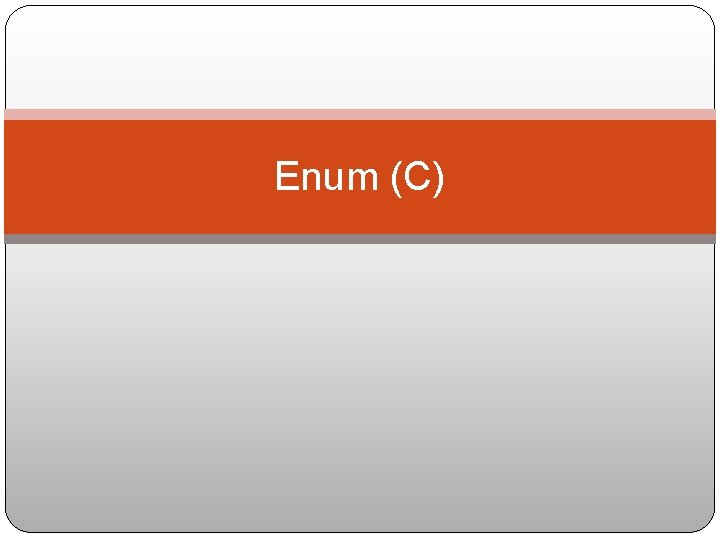
Enum (C)
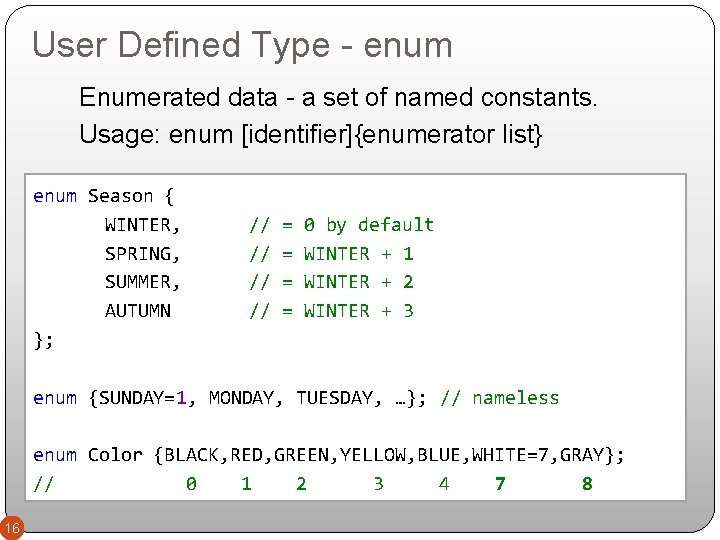
User Defined Type - enum Enumerated data - a set of named constants. Usage: enum [identifier]{enumerator list} enum Season { WINTER, SPRING, SUMMER, AUTUMN }; // = 0 by default // = WINTER + 1 // = WINTER + 2 // = WINTER + 3 enum {SUNDAY=1, MONDAY, TUESDAY, …}; // nameless enum Color {BLACK, RED, GREEN, YELLOW, BLUE, WHITE=7, GRAY}; // 0 1 2 3 4 7 8 16
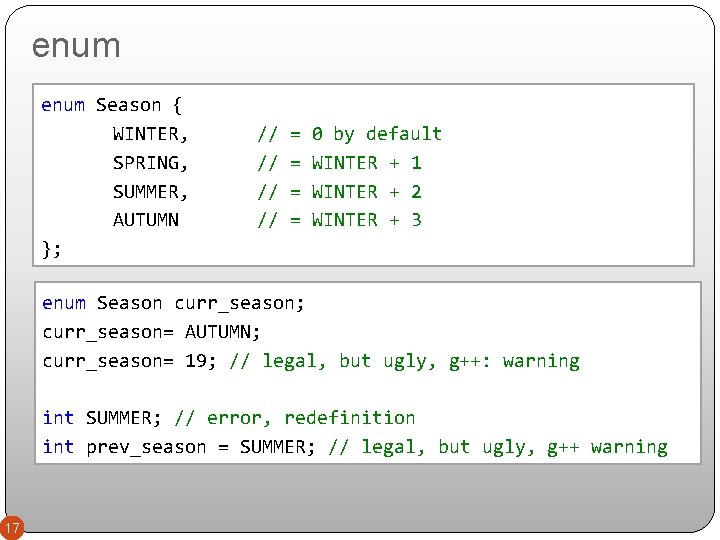
enum Season { WINTER, SPRING, SUMMER, AUTUMN }; // = 0 by default // = WINTER + 1 // = WINTER + 2 // = WINTER + 3 enum Season curr_season; curr_season= AUTUMN; curr_season= 19; // legal, but ugly, g++: warning int SUMMER; // error, redefinition int prev_season = SUMMER; // legal, but ugly, g++ warning 17
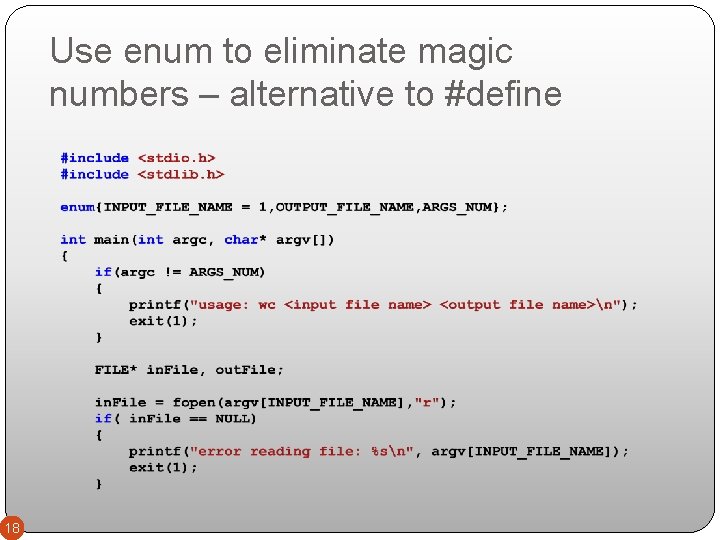
Use enum to eliminate magic numbers – alternative to #define 18
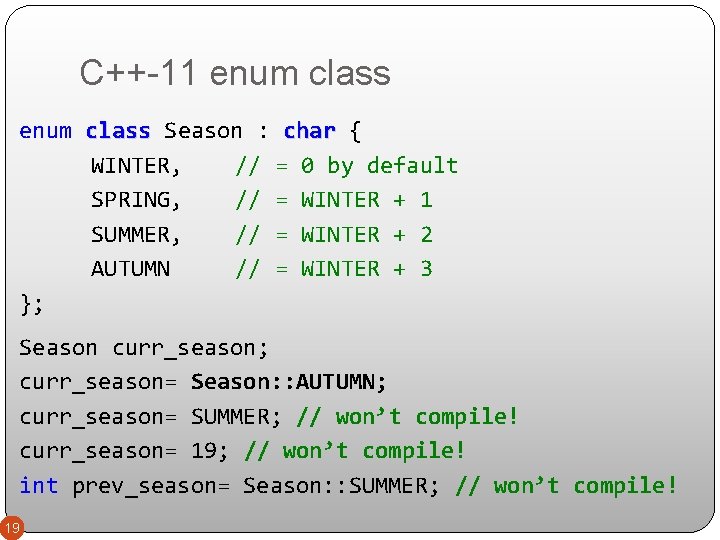
C++-11 enum class Season : char { WINTER, // = 0 by default SPRING, // = WINTER + 1 SUMMER, // = WINTER + 2 AUTUMN // = WINTER + 3 }; Season curr_season; curr_season= Season: : AUTUMN; curr_season= SUMMER; // won’t compile! curr_season= 19; // won’t compile! int prev_season= Season: : SUMMER; // won’t compile! 19
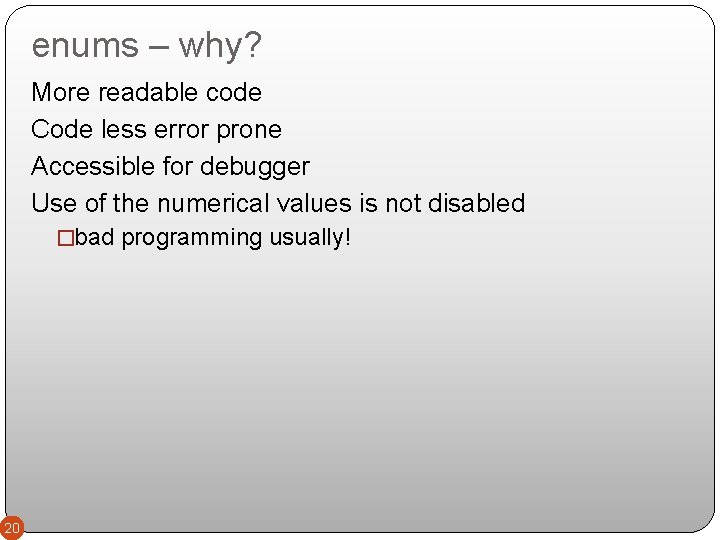
enums – why? More readable code Code less error prone Accessible for debugger Use of the numerical values is not disabled �bad programming usually! 20
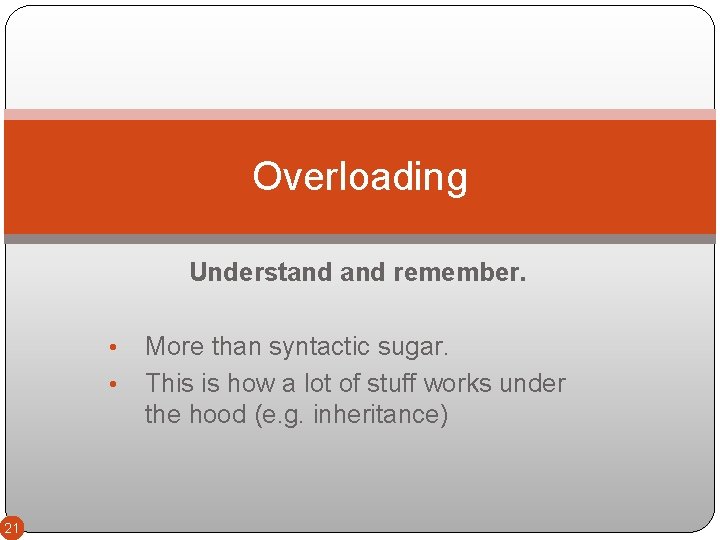
Overloading Understand remember. • • 21 More than syntactic sugar. This is how a lot of stuff works under the hood (e. g. inheritance)
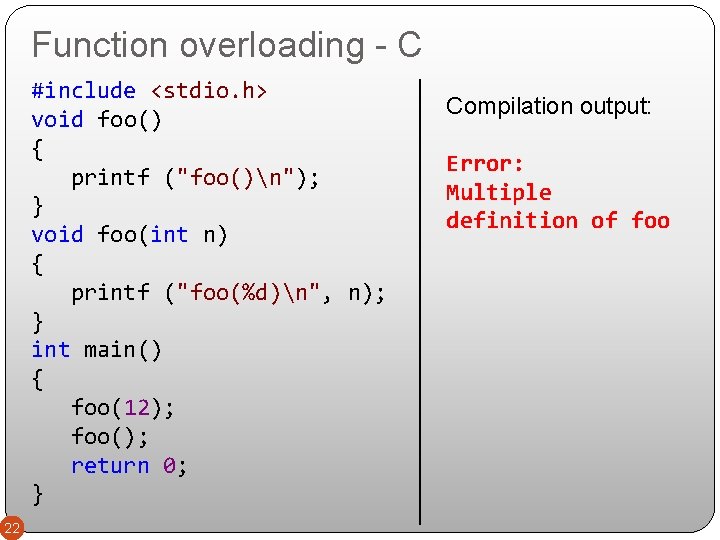
Function overloading - C #include <stdio. h> void foo() { printf ("foo()n"); } void foo(int n) { printf ("foo(%d)n", n); } int main() { foo(12); foo(); return 0; } 22 Compilation output: Error: Multiple definition of foo
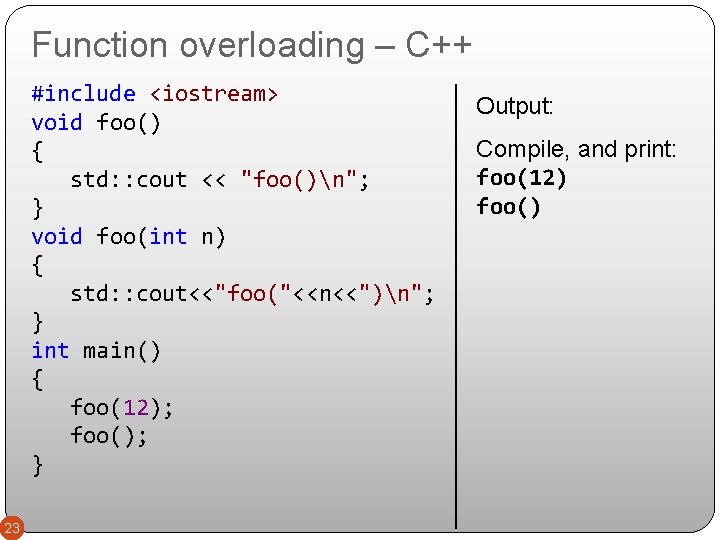
Function overloading – C++ #include <iostream> void foo() { std: : cout << "foo()n"; } void foo(int n) { std: : cout<<"foo("<<n<<")n"; } int main() { foo(12); foo(); } 23 Output: Compile, and print: foo(12) foo()
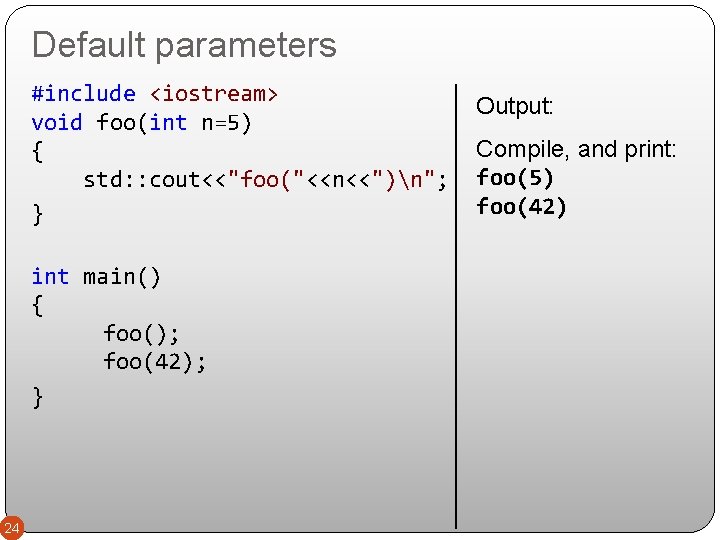
Default parameters #include <iostream> void foo(int n=5) { std: : cout<<"foo("<<n<<")n"; } int main() { foo(); foo(42); } 24 Output: Compile, and print: foo(5) foo(42)
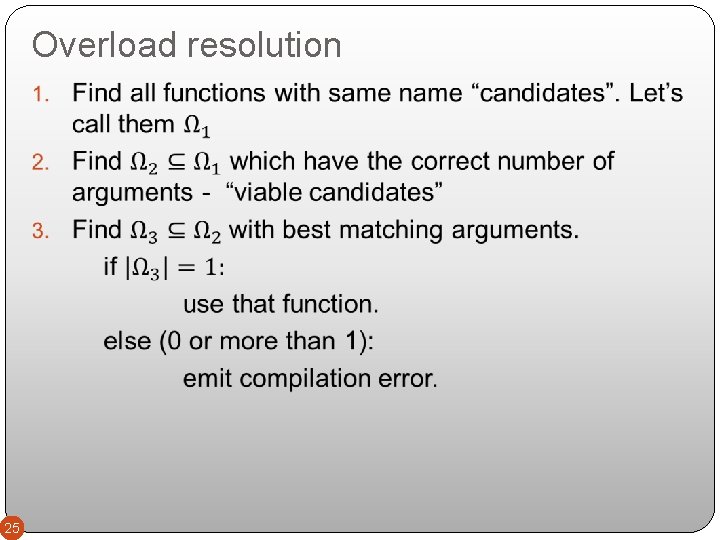
Overload resolution 25
Hey bye bye
How much
True or false katniss dismisses haymitch advice
How much is too much plagiarism
Don't ask why why why
What does janie say to jody on his deathbed?
Aims and objectives of plastic pollution
Why-why analysis
Why do you cry, willie
Does the table represent a function why or why not
What does the image represent
Why or why not
Analisa akar masalah
3 types of alcohol
How much does 1 million look like
Who is he
Does africa have water
How much can they safely carry answer key
Much madness is divinest sense analysis
Much ado about nothing allusions
The world is too much with us imagery
What is the tone in the world is too much with us
The world is too much with us figurative language
A an some any exercises
How much water is there on earth
Thank you for your attention