Divide and Conquer Merge Sort Divide and Conquer
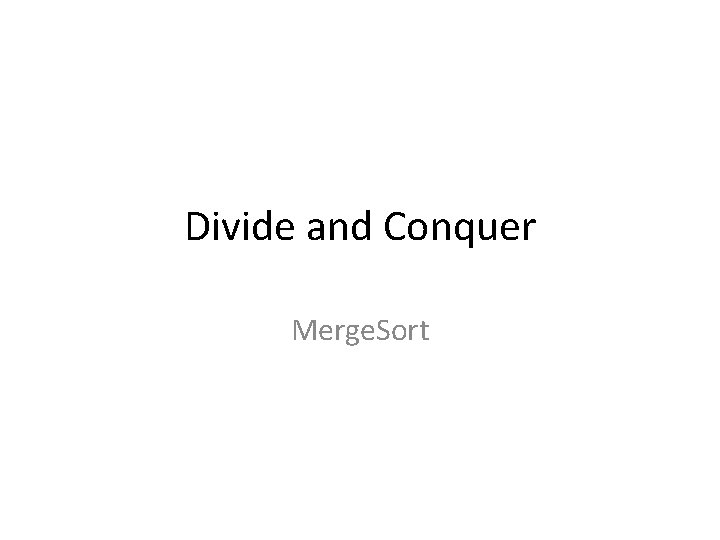
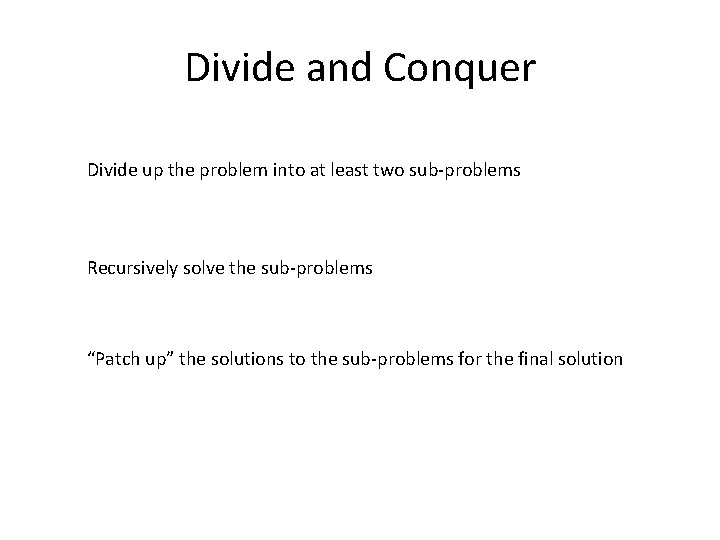
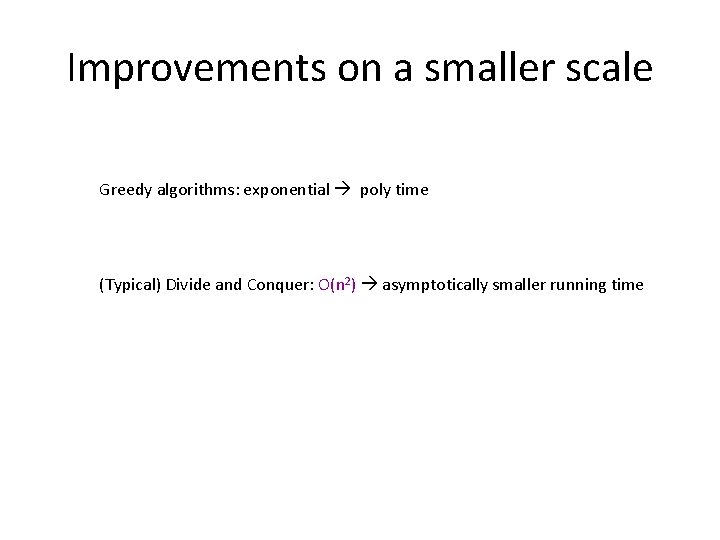
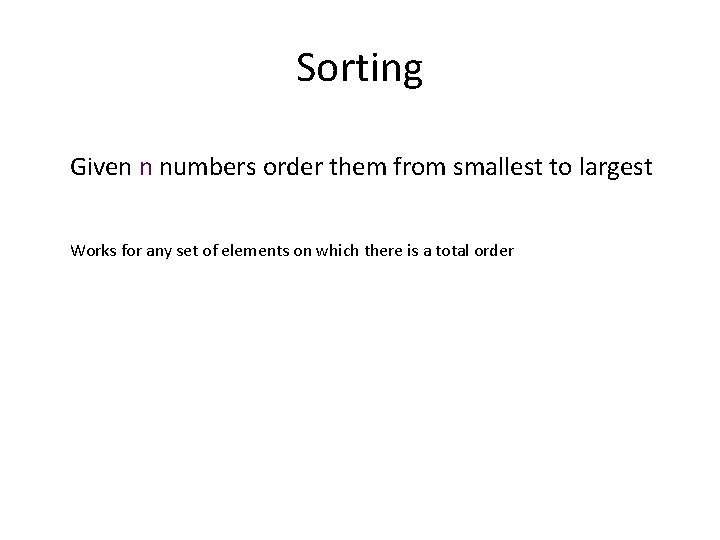
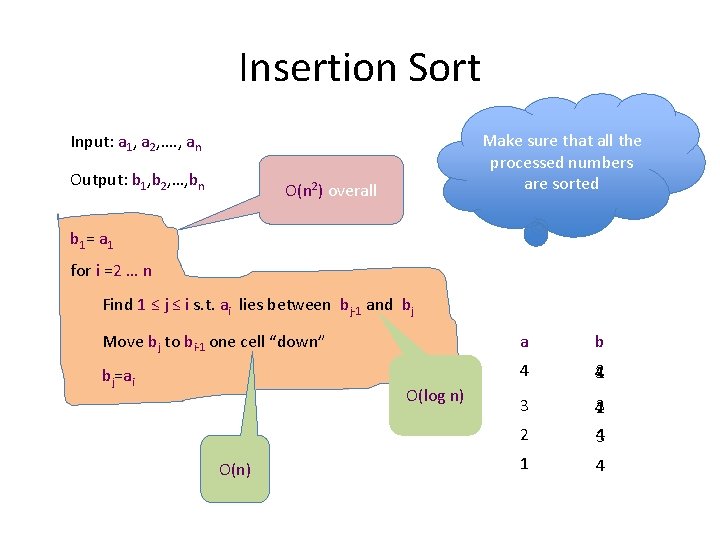
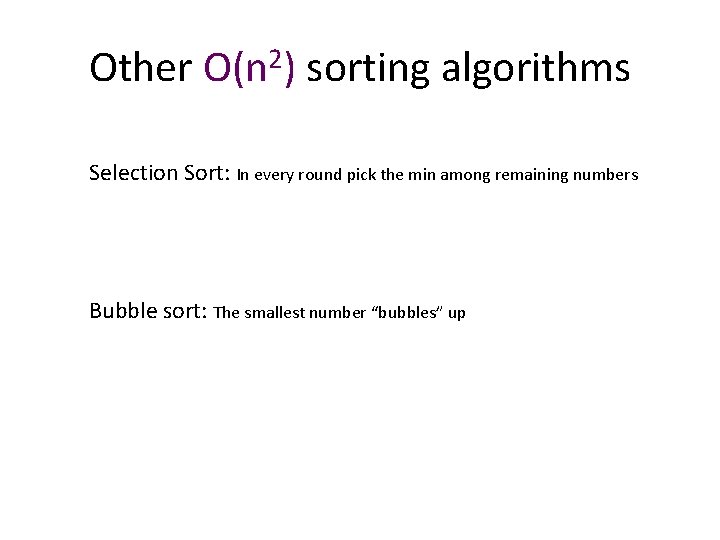
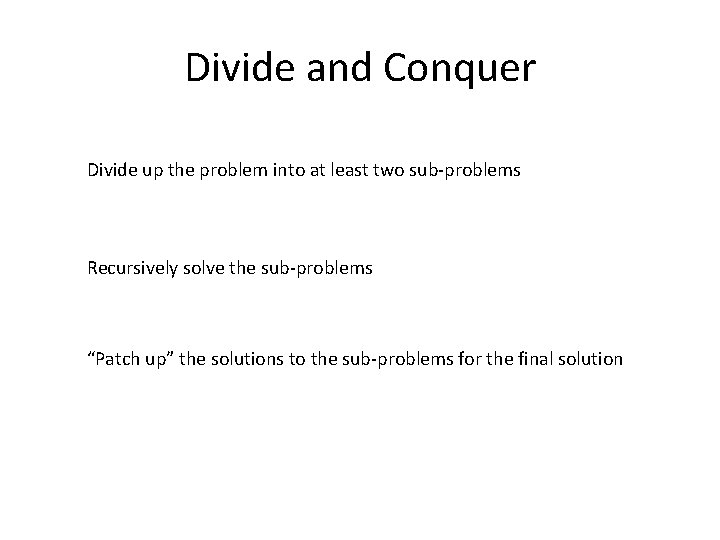
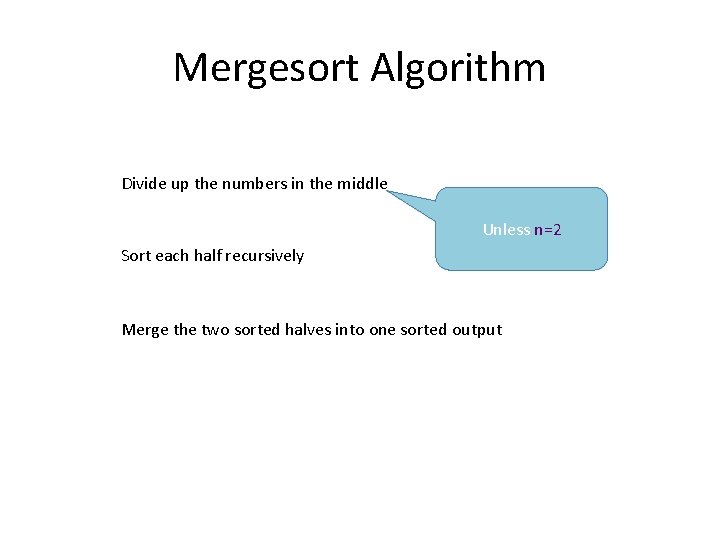
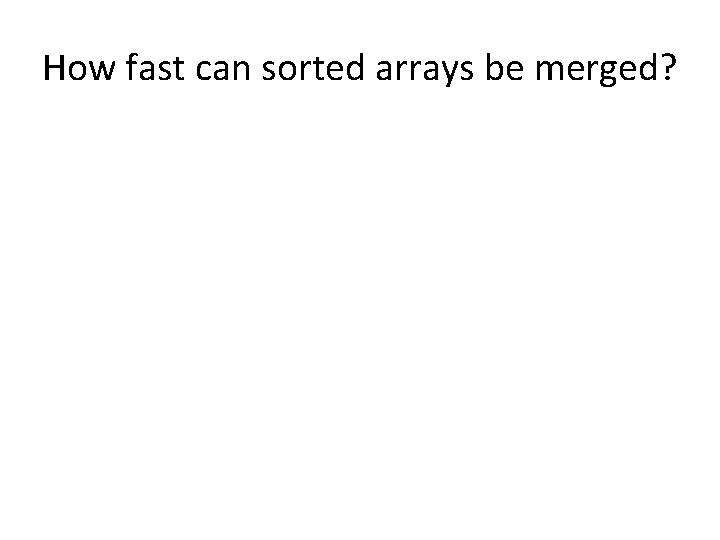
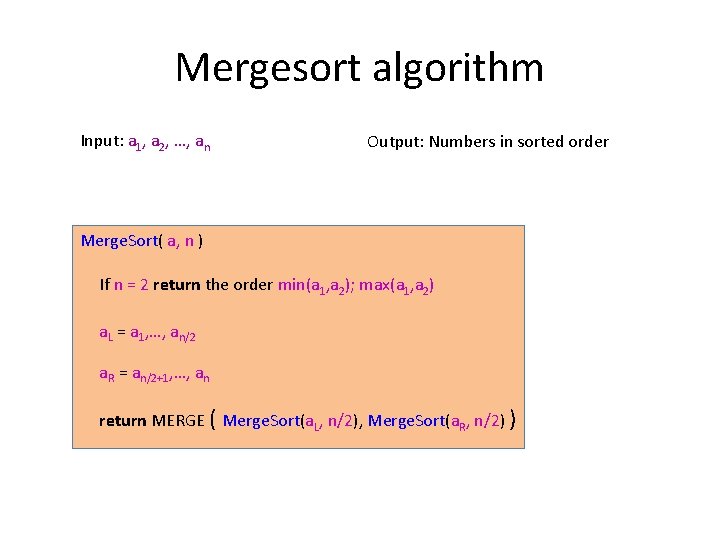
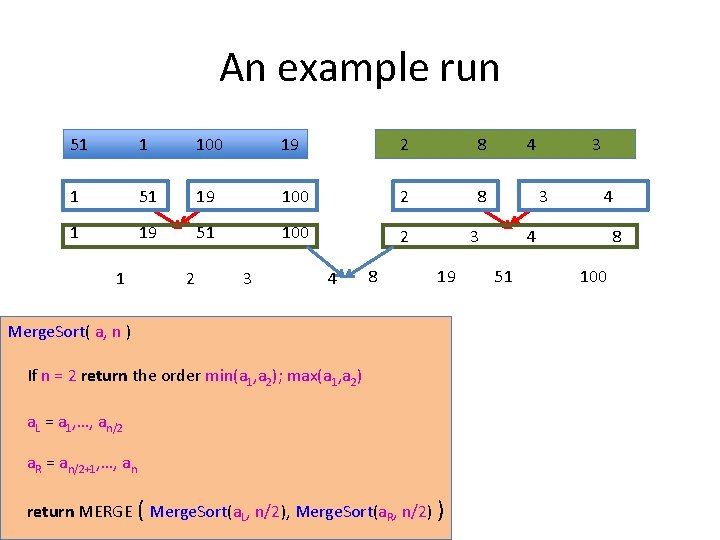
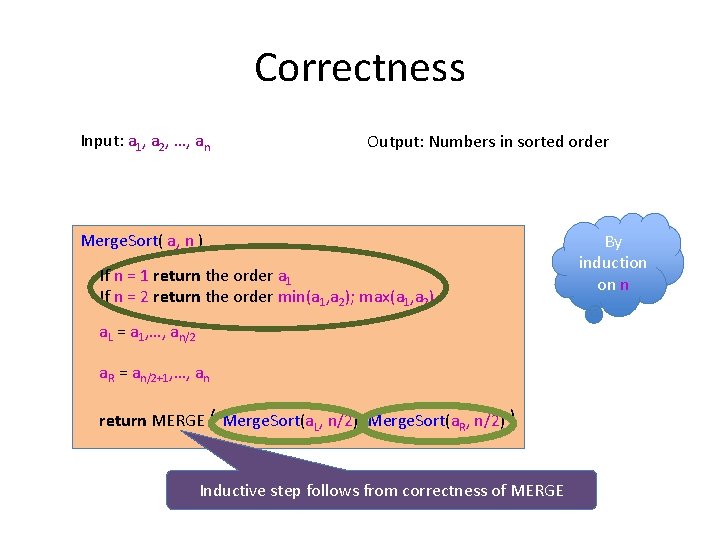
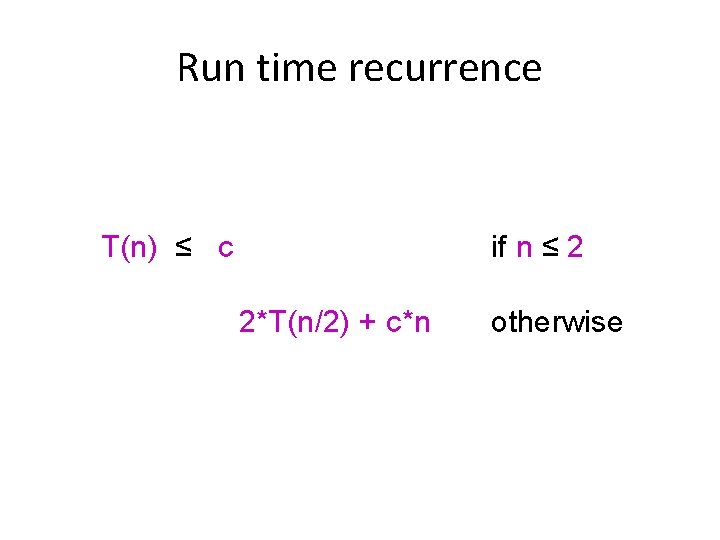
- Slides: 13
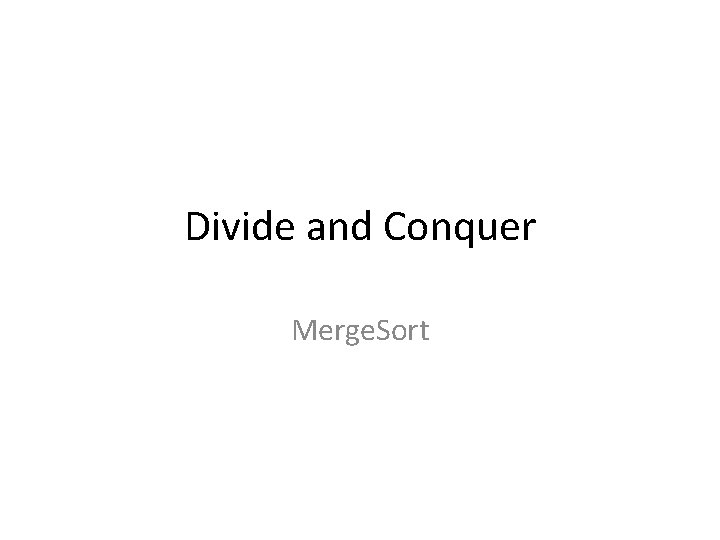
Divide and Conquer Merge. Sort
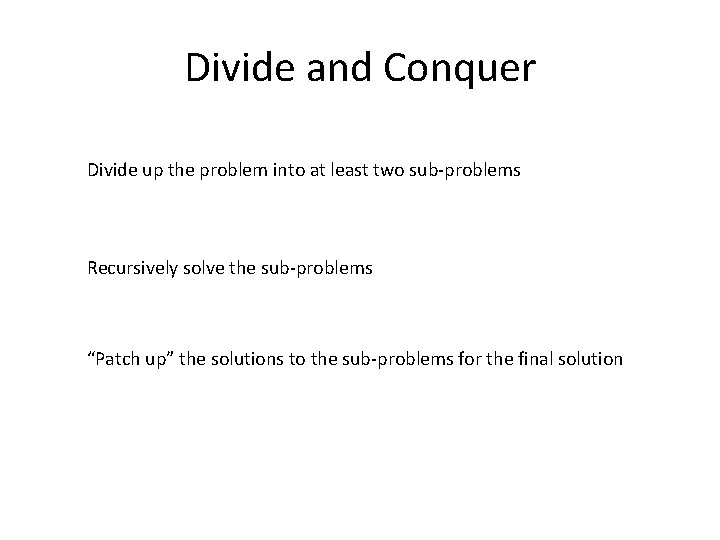
Divide and Conquer Divide up the problem into at least two sub-problems Recursively solve the sub-problems “Patch up” the solutions to the sub-problems for the final solution
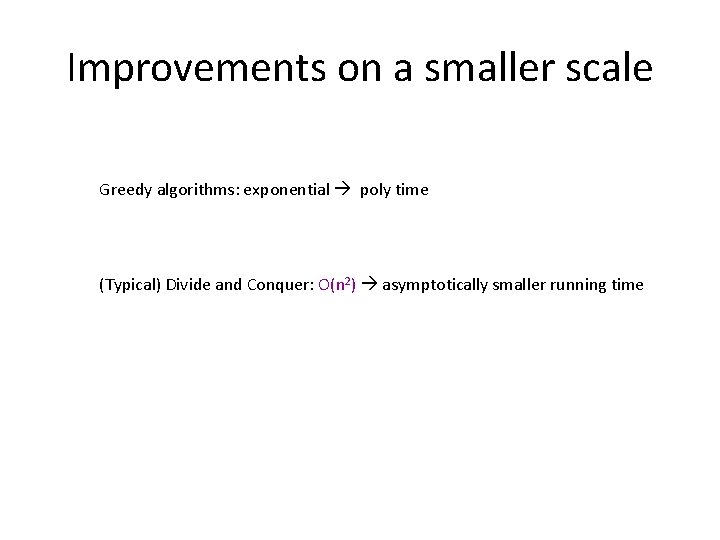
Improvements on a smaller scale Greedy algorithms: exponential poly time (Typical) Divide and Conquer: O(n 2) asymptotically smaller running time
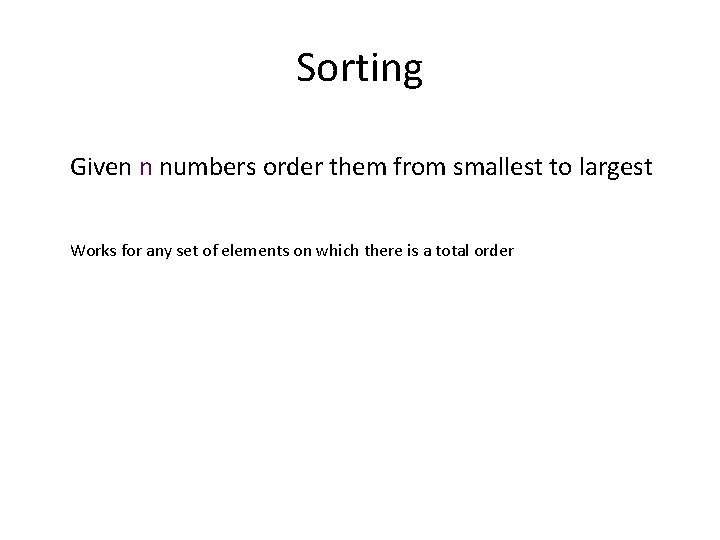
Sorting Given n numbers order them from smallest to largest Works for any set of elements on which there is a total order
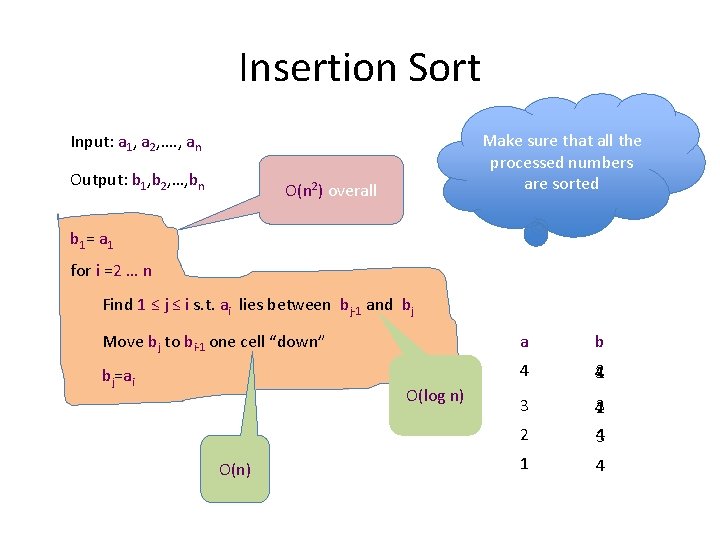
Insertion Sort Make sure that all the processed numbers are sorted Input: a 1, a 2, …. , an Output: b 1, b 2, …, bn O(n 2) overall b 1= a 1 for i =2 … n Find 1 ≤ j ≤ i s. t. ai lies between bj-1 and bj Move bj to bi-1 one cell “down” a b bj=ai 4 2 431 3 2 2 43 4 3 1 4 O(log n) O(n)
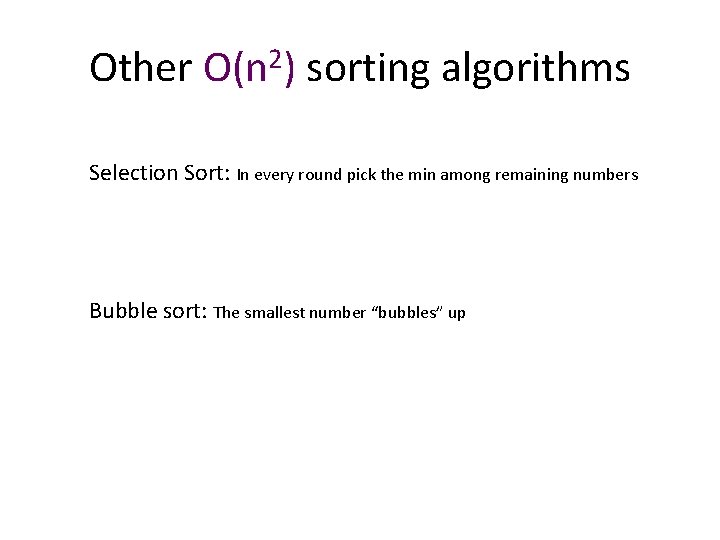
Other O(n 2) sorting algorithms Selection Sort: In every round pick the min among remaining numbers Bubble sort: The smallest number “bubbles” up
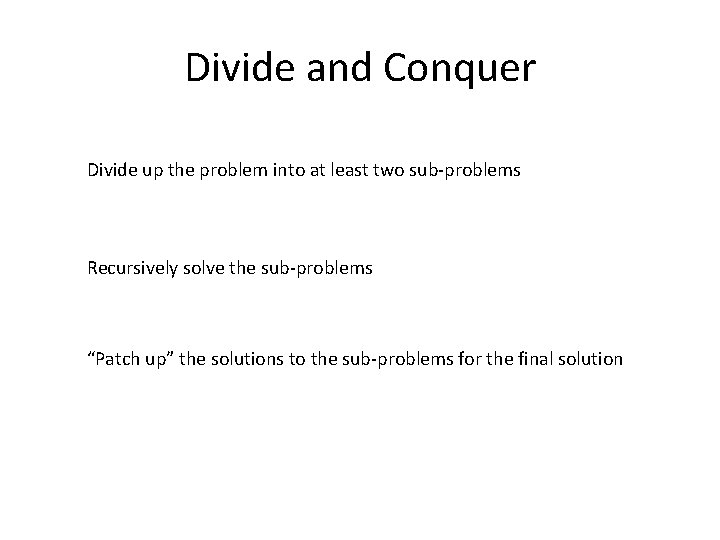
Divide and Conquer Divide up the problem into at least two sub-problems Recursively solve the sub-problems “Patch up” the solutions to the sub-problems for the final solution
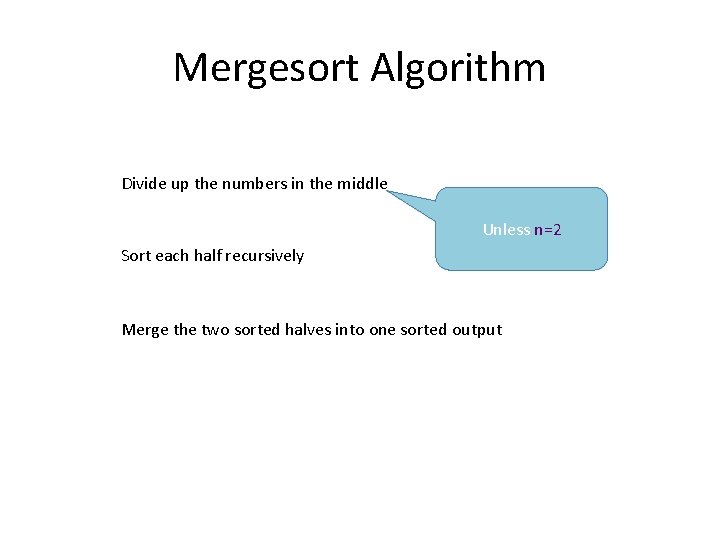
Mergesort Algorithm Divide up the numbers in the middle Unless n=2 Sort each half recursively Merge the two sorted halves into one sorted output
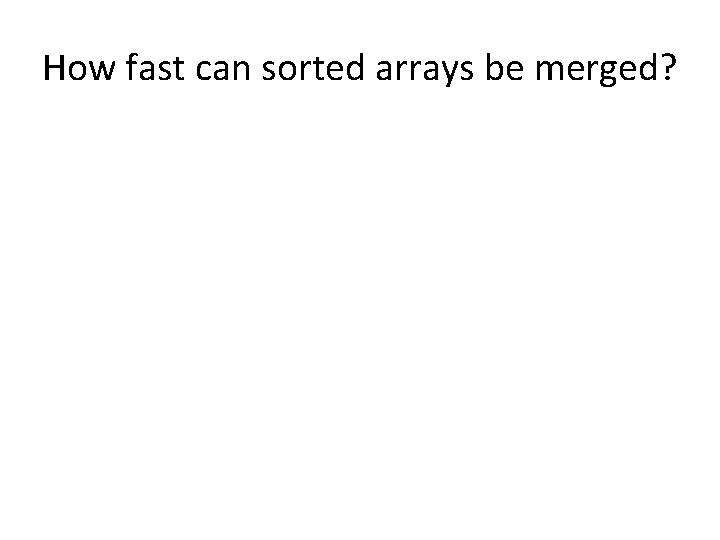
How fast can sorted arrays be merged?
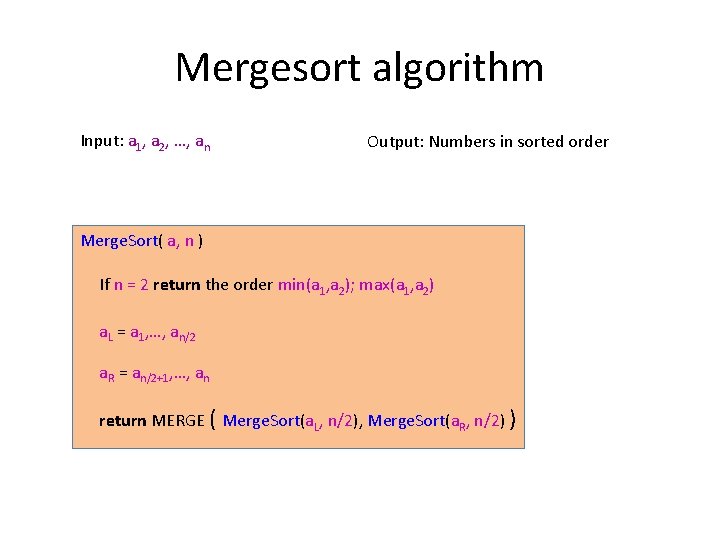
Mergesort algorithm Input: a 1, a 2, …, an Output: Numbers in sorted order Merge. Sort( a, n ) If n = 2 return the order min(a 1, a 2); max(a 1, a 2) a. L = a 1, …, an/2 a. R = an/2+1, …, an return MERGE ( Merge. Sort(a. L, n/2), Merge. Sort(a. R, n/2) )
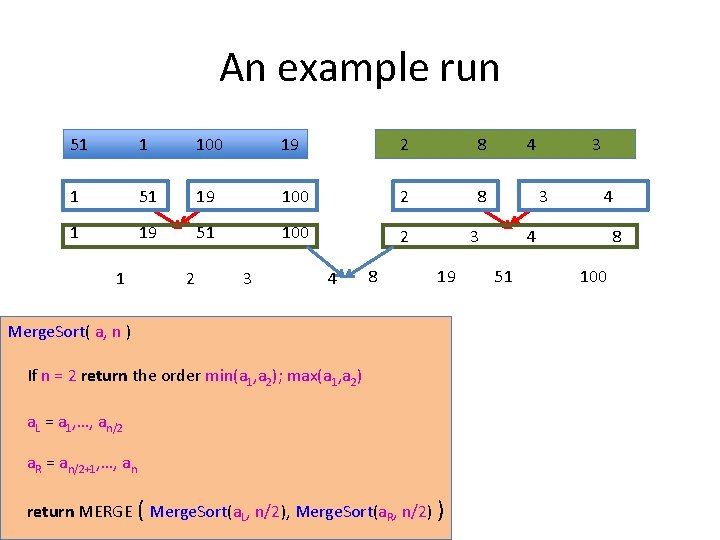
An example run 51 1 100 19 2 8 1 51 19 100 2 8 1 19 51 100 2 1 2 3 4 8 4 3 3 19 Merge. Sort( a, n ) If n = 2 return the order min(a 1, a 2); max(a 1, a 2) a. L = a 1, …, an/2 a. R = an/2+1, …, an return MERGE ( Merge. Sort(a. L, n/2), Merge. Sort(a. R, n/2) ) 3 4 4 51 8 100
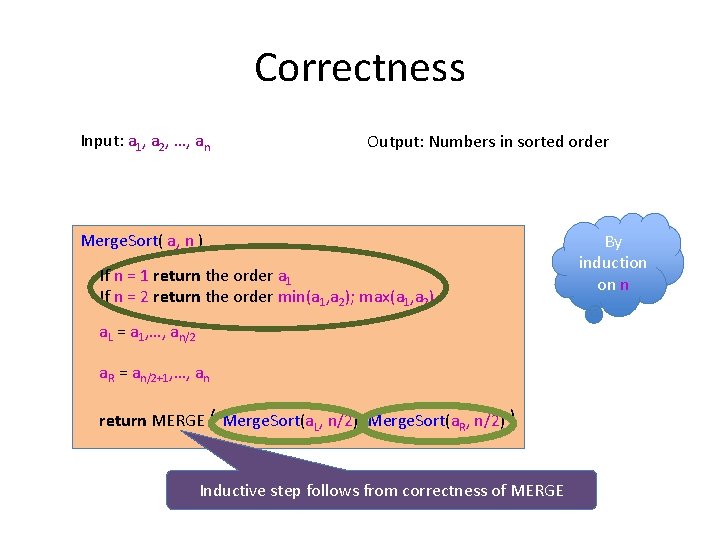
Correctness Input: a 1, a 2, …, an Output: Numbers in sorted order Merge. Sort( a, n ) If n = 1 return the order a 1 If n = 2 return the order min(a 1, a 2); max(a 1, a 2) a. L = a 1, …, an/2 a. R = an/2+1, …, an return MERGE ( Merge. Sort(a. L, n/2), Merge. Sort(a. R, n/2) ) Inductive step follows from correctness of MERGE By induction on n
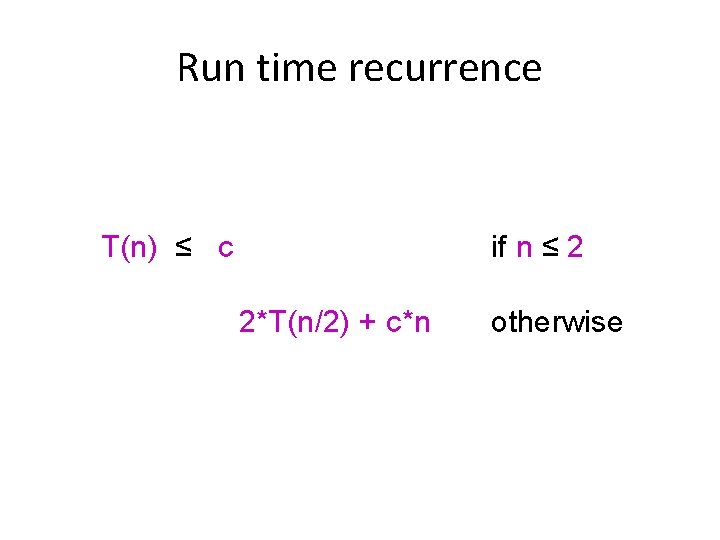
Run time recurrence T(n) ≤ c if n ≤ 2 2*T(n/2) + c*n otherwise
Quick sort merge sort
Quick sort merge sort
Is shell sort divide and conquer
Sort and merge in cobol
Recurrence relation of bubble sort
Recurrence relation
Why is merge sort n log n
Merge sort complexity
Bottom up merge sort
Binary merge sort
Merge sort
Why is merge sort n log n
Merge sort mips implementation
Merge sort medium