Sorting Merge Sort Merge Sort divide and conquer
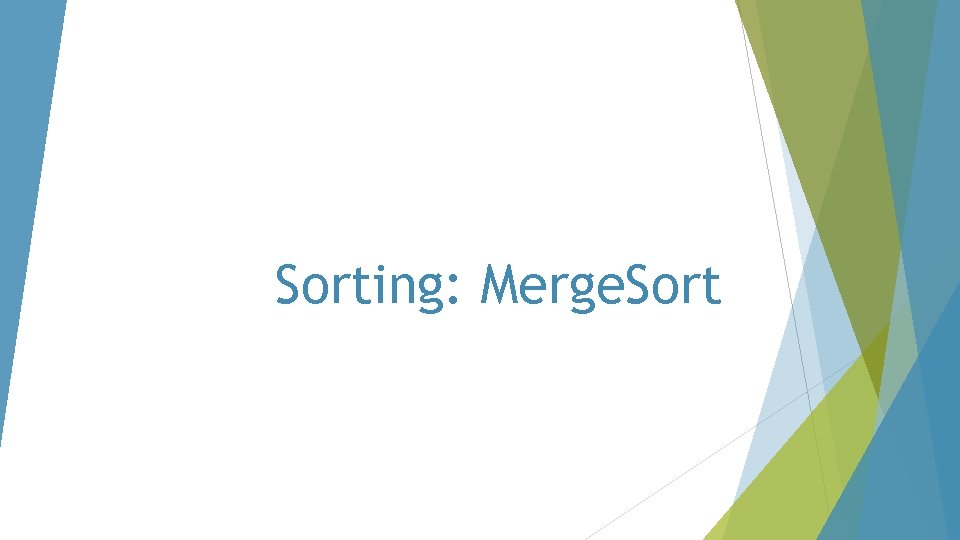
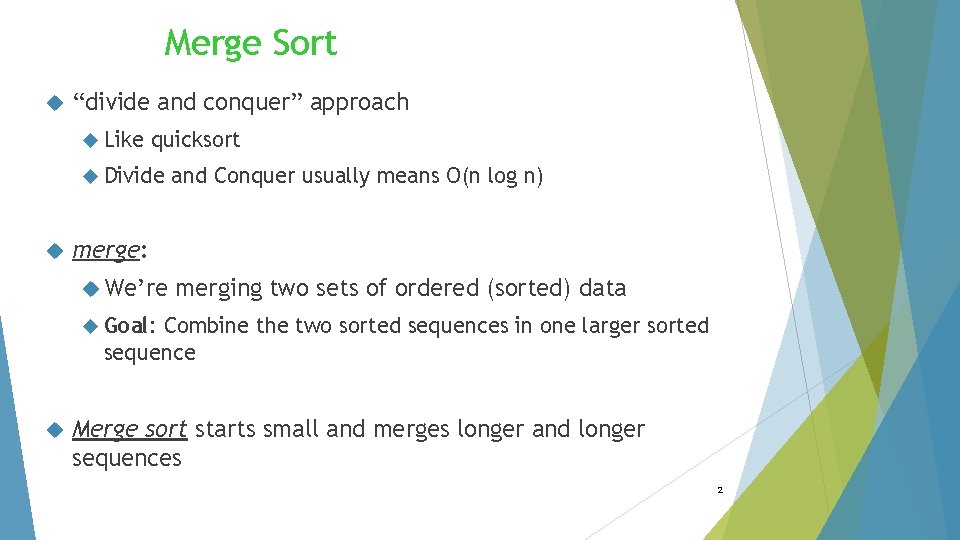
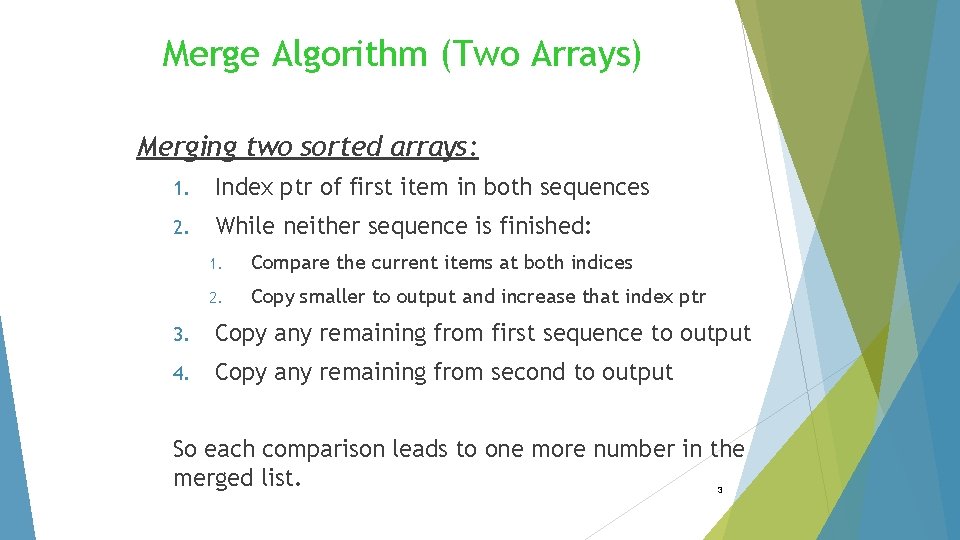
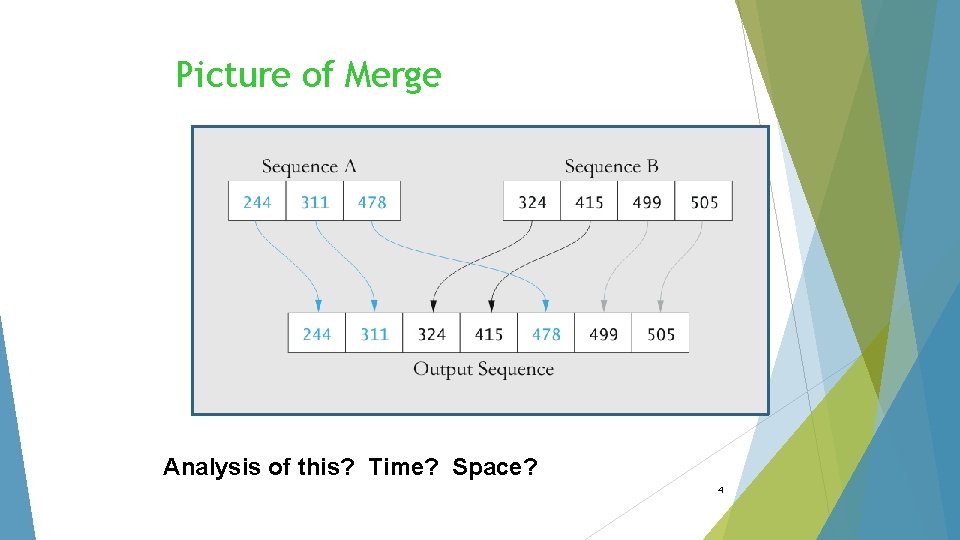
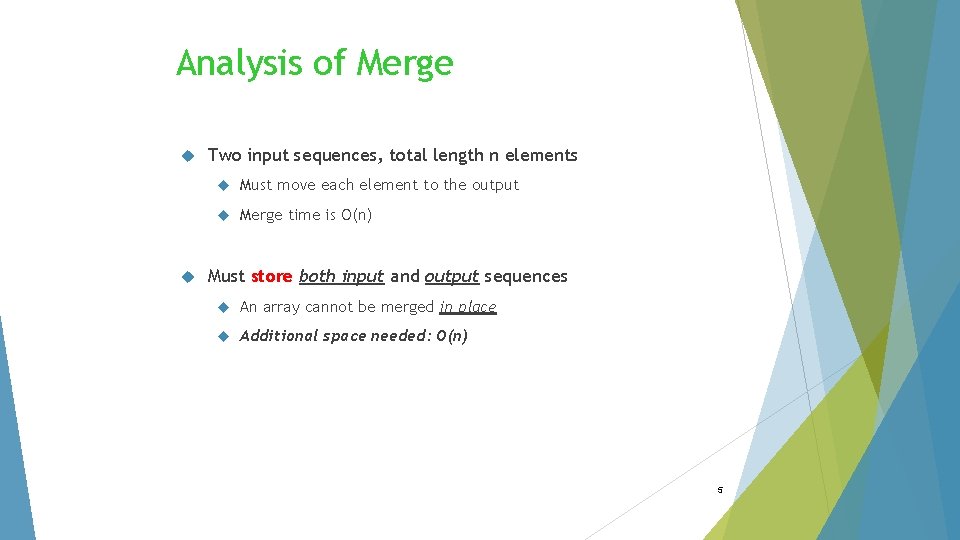
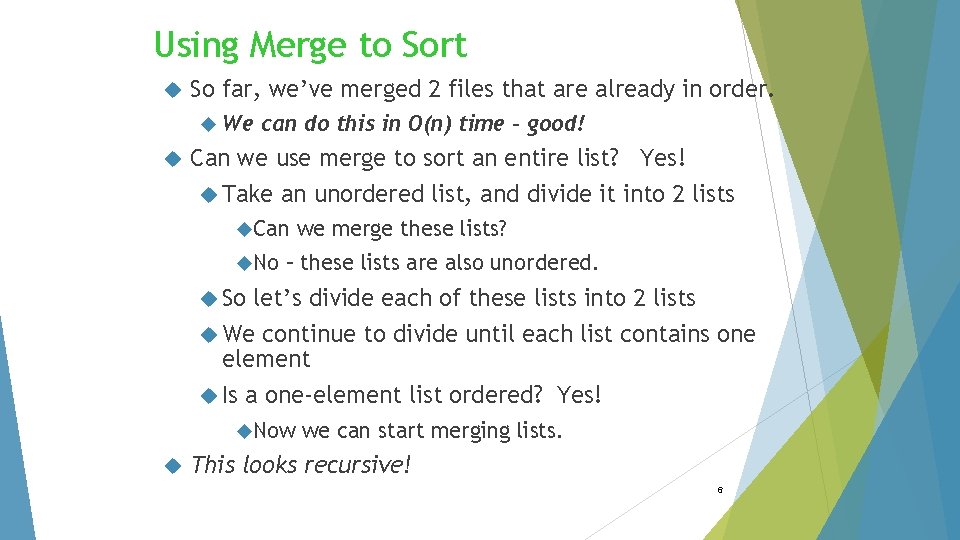
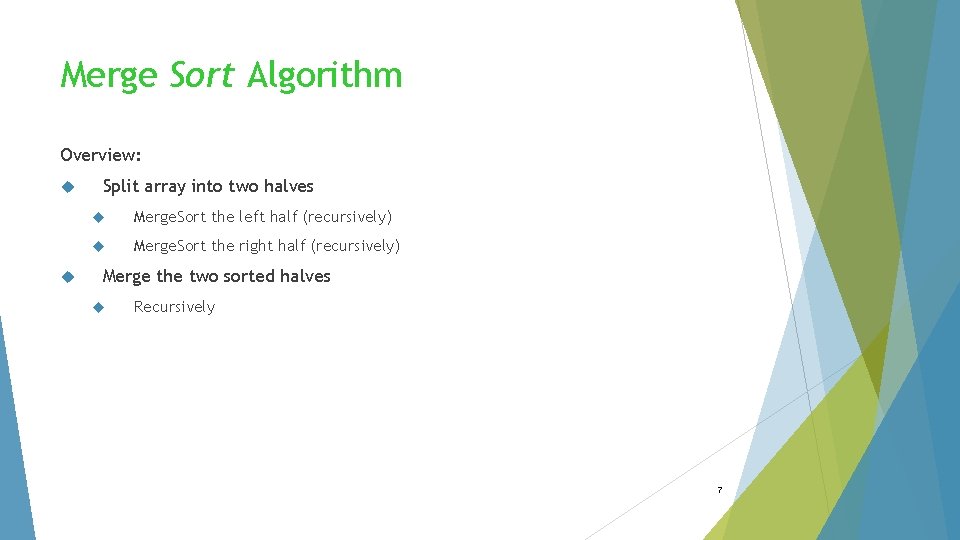
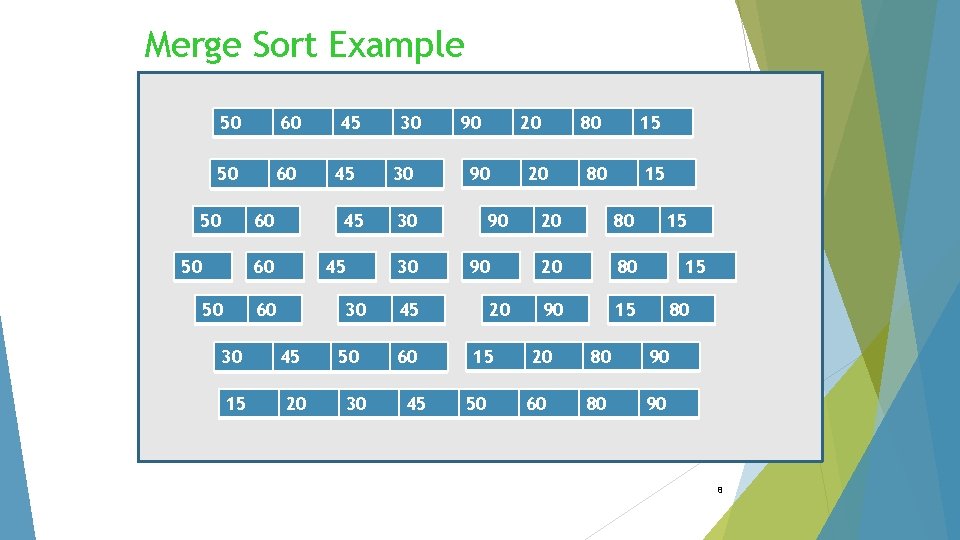
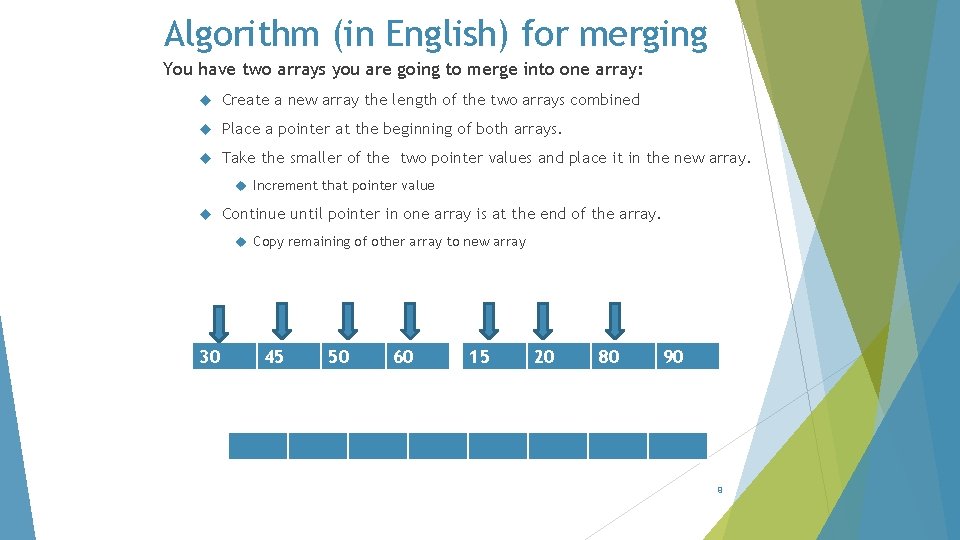
![void merge(int arr[], int l, int m, int r) { int i, j, k; void merge(int arr[], int l, int m, int r) { int i, j, k;](https://slidetodoc.com/presentation_image_h2/35d1cc76e79bfb757dff5db9334a2048/image-10.jpg)
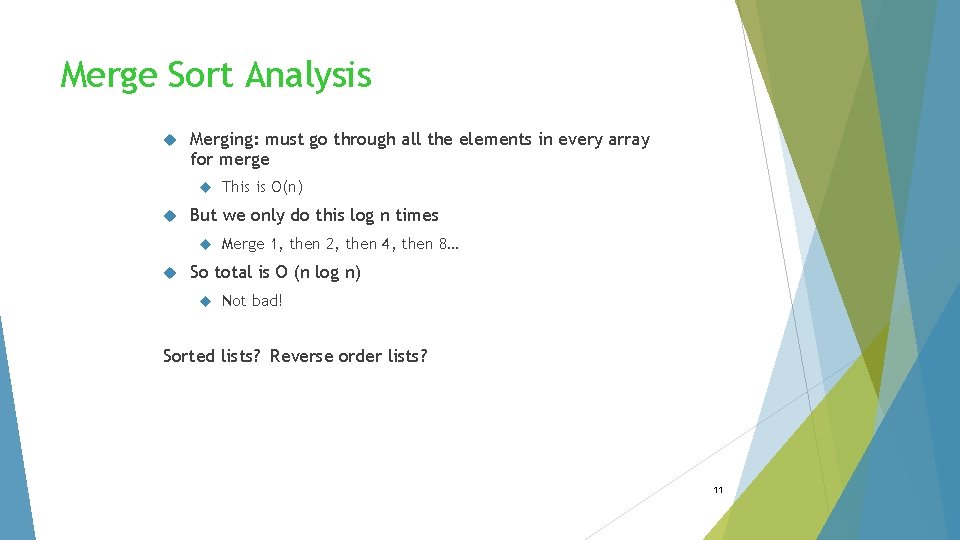
- Slides: 11
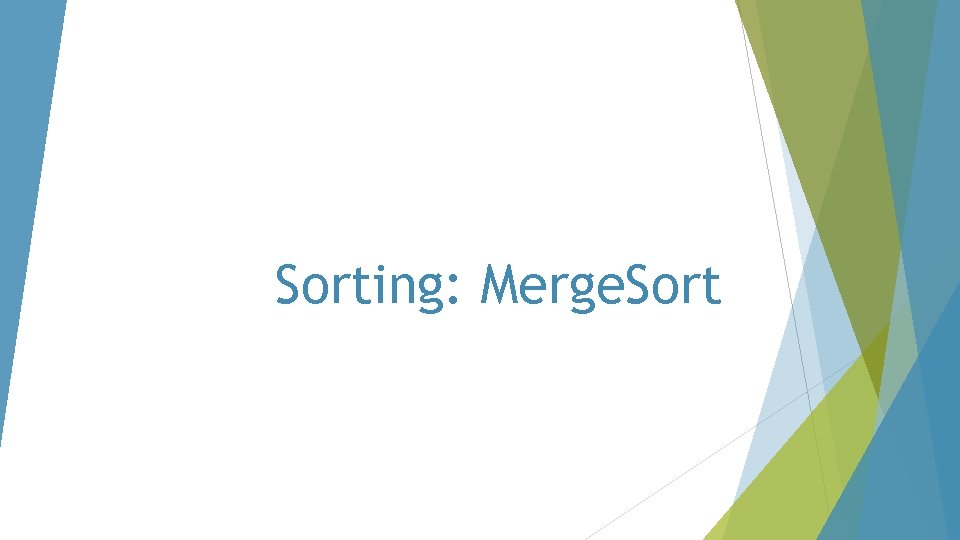
Sorting: Merge. Sort
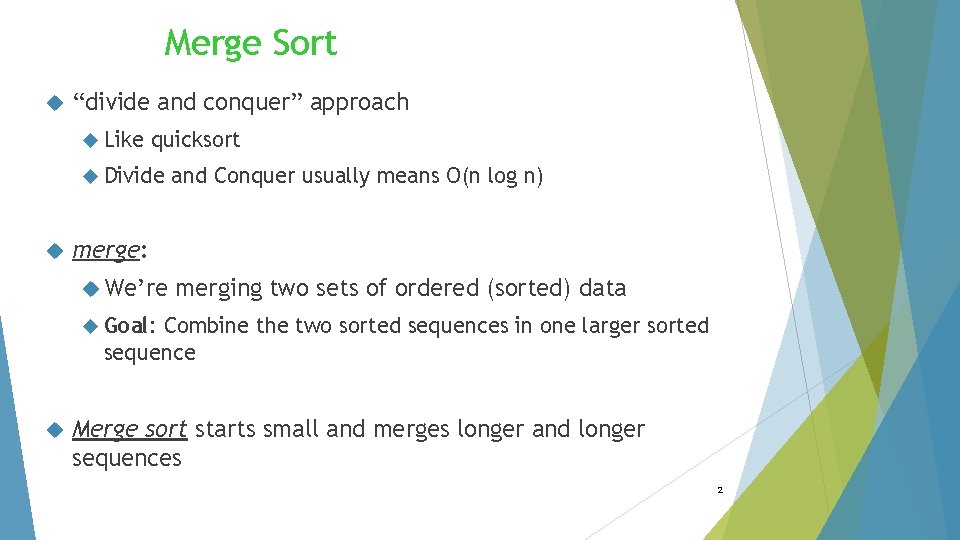
Merge Sort “divide and conquer” approach Like quicksort Divide and Conquer usually means O(n log n) merge: We’re merging two sets of ordered (sorted) data Goal: Combine the two sorted sequences in one larger sorted sequence Merge sort starts small and merges longer and longer sequences 2
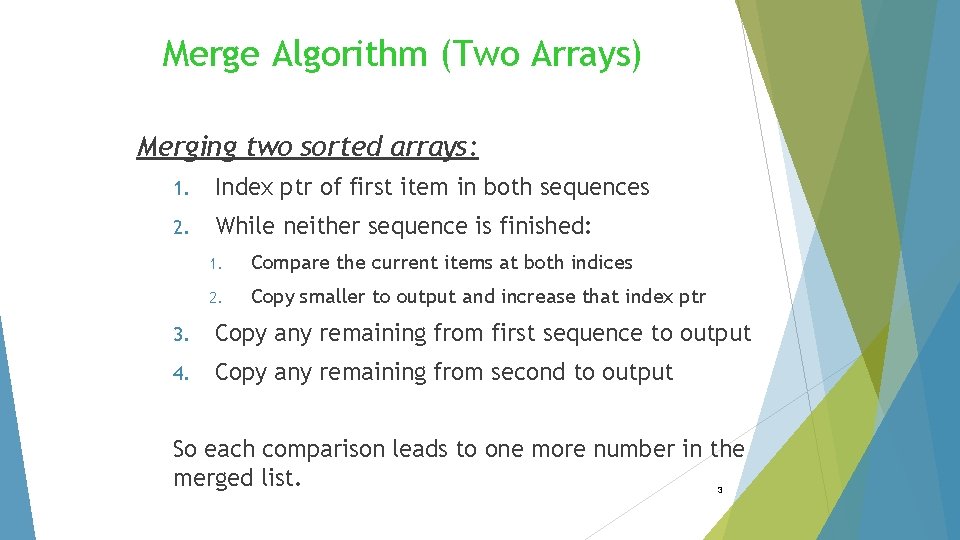
Merge Algorithm (Two Arrays) Merging two sorted arrays: 1. Index ptr of first item in both sequences 2. While neither sequence is finished: 1. Compare the current items at both indices 2. Copy smaller to output and increase that index ptr 3. Copy any remaining from first sequence to output 4. Copy any remaining from second to output So each comparison leads to one more number in the merged list. 3
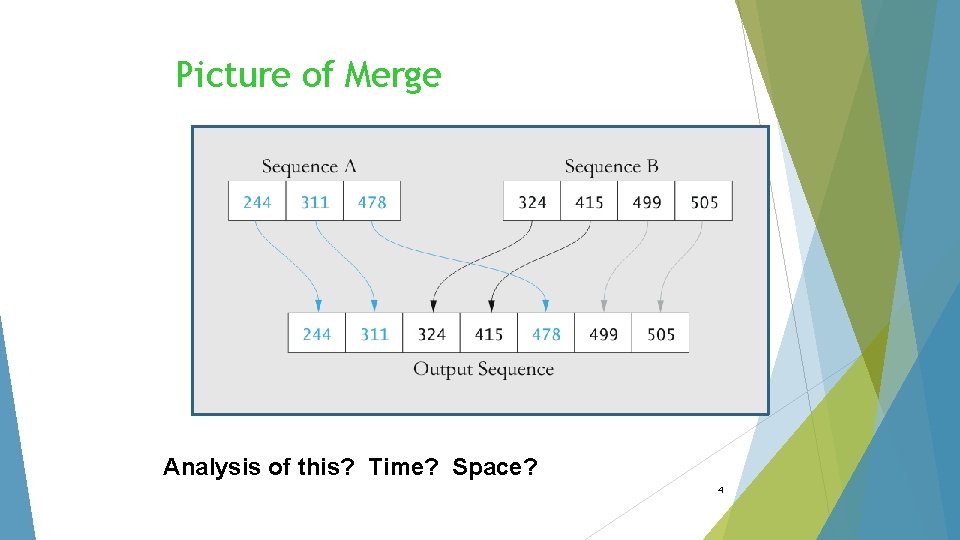
Picture of Merge Analysis of this? Time? Space? 4
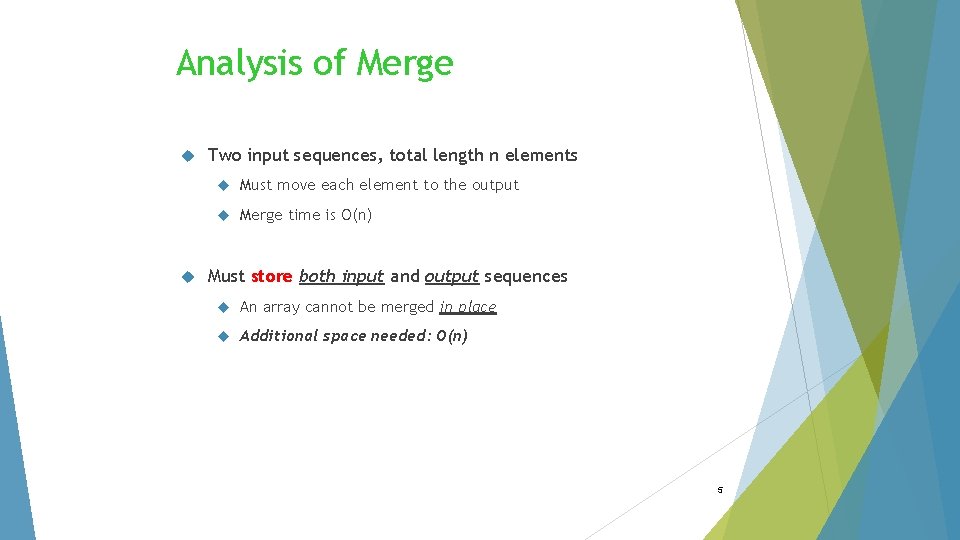
Analysis of Merge Two input sequences, total length n elements Must move each element to the output Merge time is O(n) Must store both input and output sequences An array cannot be merged in place Additional space needed: O(n) 5
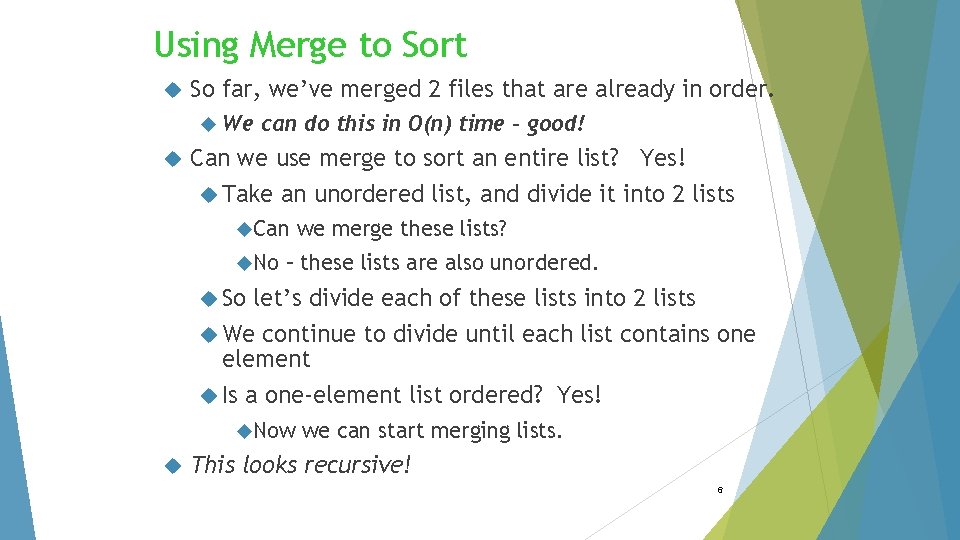
Using Merge to Sort So far, we’ve merged 2 files that are already in order. We can do this in O(n) time – good! Can we use merge to sort an entire list? Yes! Take an unordered list, and divide it into 2 lists Can No we merge these lists? – these lists are also unordered. So let’s divide each of these lists into 2 lists We continue to divide until each list contains one element Is a one-element list ordered? Yes! Now we can start merging lists. This looks recursive! 6
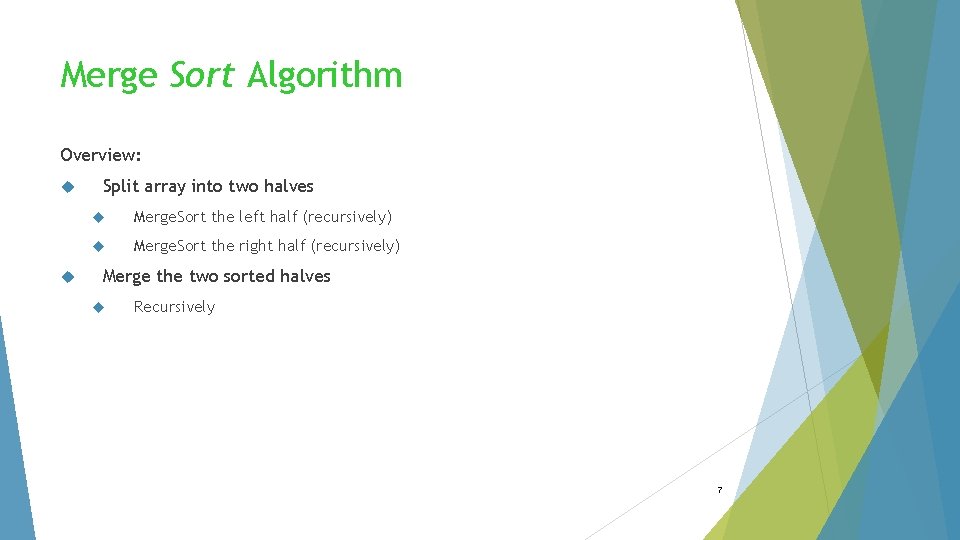
Merge Sort Algorithm Overview: Split array into two halves Merge. Sort the left half (recursively) Merge. Sort the right half (recursively) Merge the two sorted halves Recursively 7
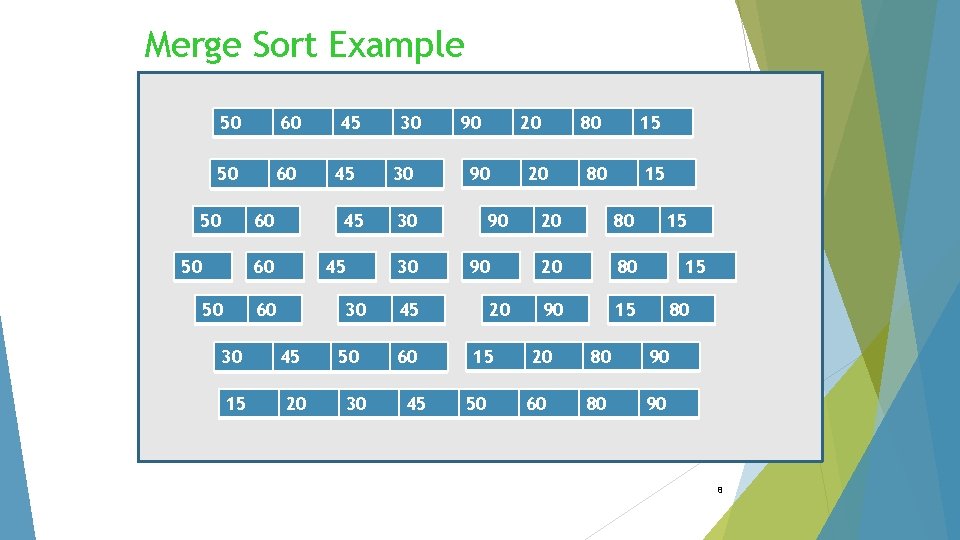
Merge Sort Example 50 60 50 45 60 30 15 45 45 60 50 45 30 45 20 50 30 30 30 90 90 45 60 45 20 20 15 50 80 20 15 80 15 20 80 90 15 20 60 15 15 80 80 90 8
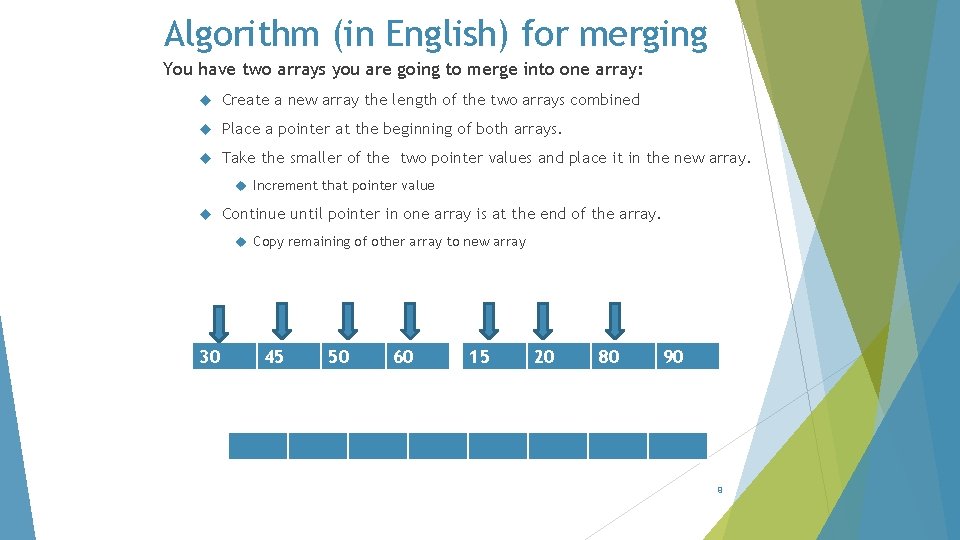
Algorithm (in English) for merging You have two arrays you are going to merge into one array: Create a new array the length of the two arrays combined Place a pointer at the beginning of both arrays. Take the smaller of the two pointer values and place it in the new array. Increment that pointer value Continue until pointer in one array is at the end of the array. Copy remaining of other array to new array 30 45 15 50 20 60 30 15 45 50 20 80 60 80 90 90 9
![void mergeint arr int l int m int r int i j k void merge(int arr[], int l, int m, int r) { int i, j, k;](https://slidetodoc.com/presentation_image_h2/35d1cc76e79bfb757dff5db9334a2048/image-10.jpg)
void merge(int arr[], int l, int m, int r) { int i, j, k; int n 1 = m - l + 1; int n 2 = r - m; Merge Sort Code int L[n 1], R[n 2]; /* create temp arrays */ for(i = 0; i < n 1; i++) /* Copy data to temp arrays L[] and R[] */ L[i] = arr[l + i]; for(j = 0; j < n 2; j++) R[j] = arr[m + 1+ j]; i = 0; /* Merge the temp arrays back into arr[l. . r]*/ j = 0; k = l; while (i < n 1 && j < n 2) { if (L[i] <= R[j]) { arr[k] = L[i]; i++; } else { arr[k] = R[j]; j++; } k++; } while (i < n 1) {/* Copy the remaining elements of L[], if there any */ arr[k] = L[i]; i++; k++; } } while (j < n 2) {/* Copy the remaining elements of R[], if there any */ arr[k] = R[j]; j++; k++; } 10
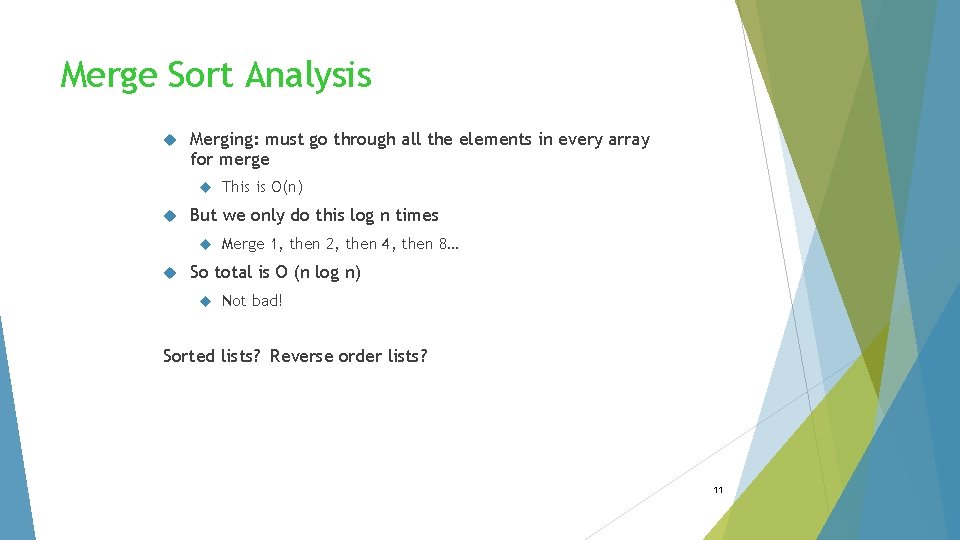
Merge Sort Analysis Merging: must go through all the elements in every array for merge But we only do this log n times This is O(n) Merge 1, then 2, then 4, then 8… So total is O (n log n) Not bad! Sorted lists? Reverse order lists? 11
Insertion sort divide and conquer
Quick sort merge sort
Quick sort merge sort
Difference between external and internal sorting
Divide and conquer advantages and disadvantages
Divide and conquer vs greedy
Voronoi diagram
Delaunay triangulation divide and conquer algorithm
Counting inversions divide and conquer
Metoda divide et impera c++ probleme rezolvate
Dynamic programming bottom up
Dynamic programming vs divide and conquer