CSE 452 Programming Languages Expressions and Control Flow
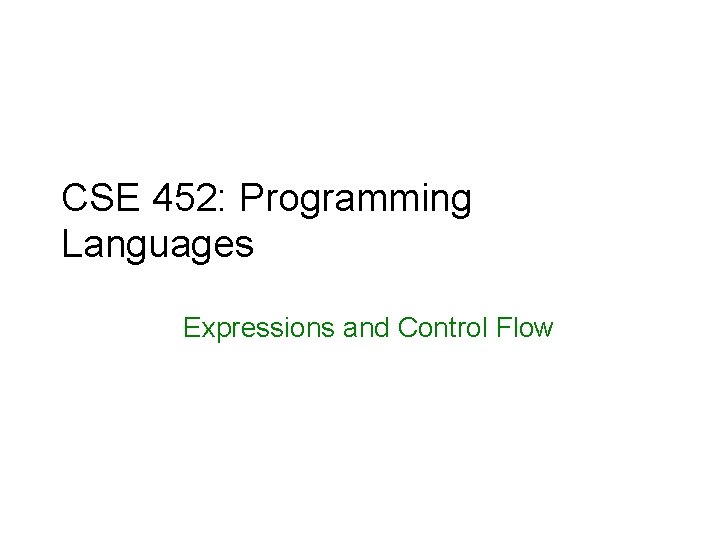
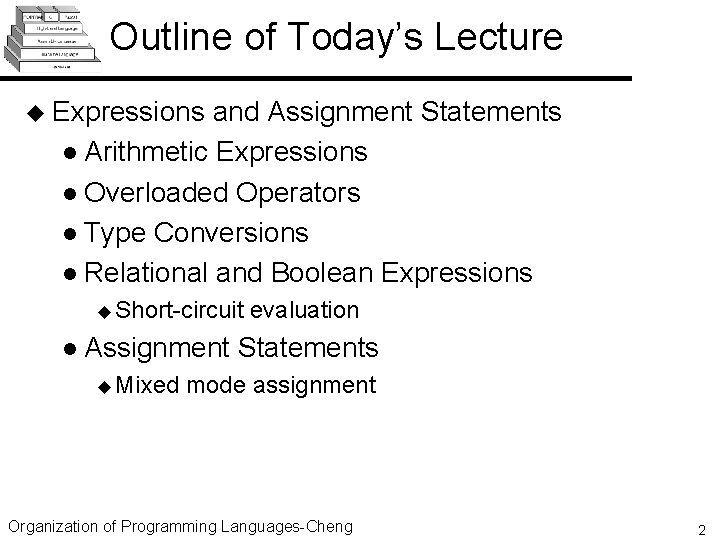
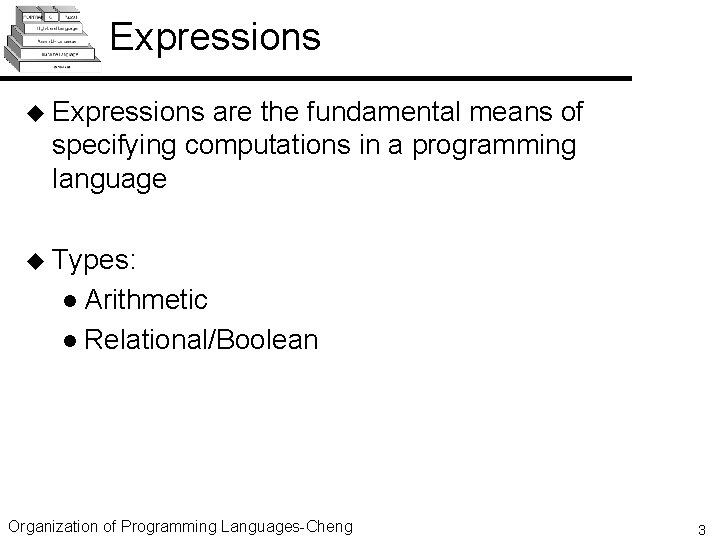
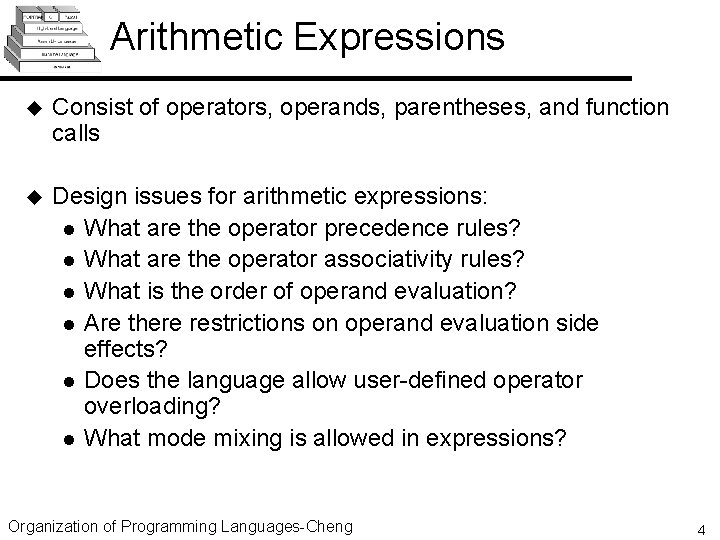
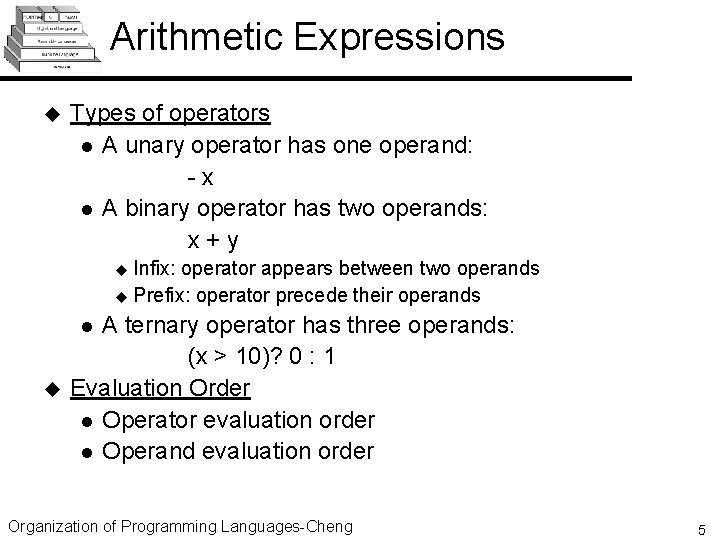
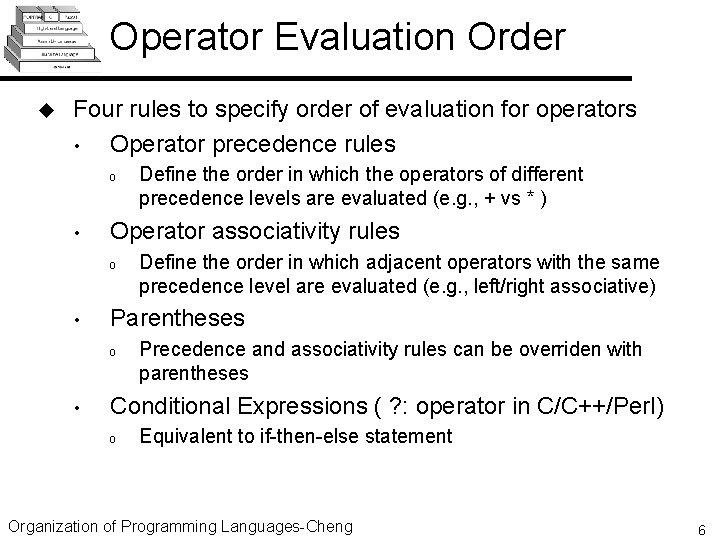
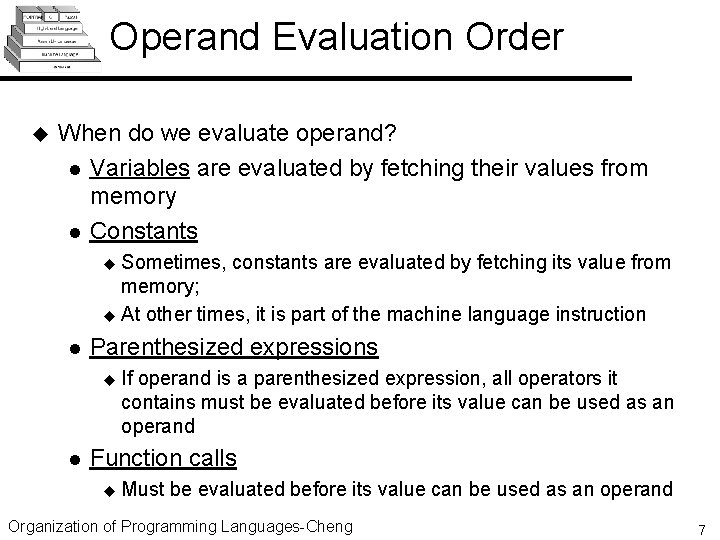
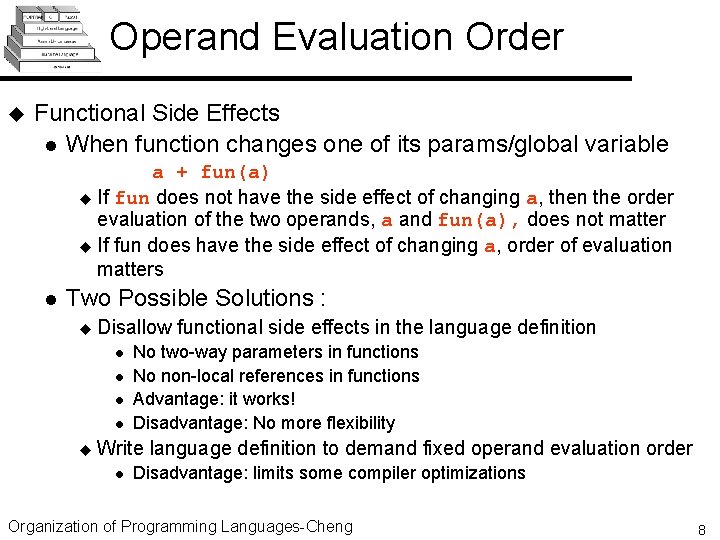
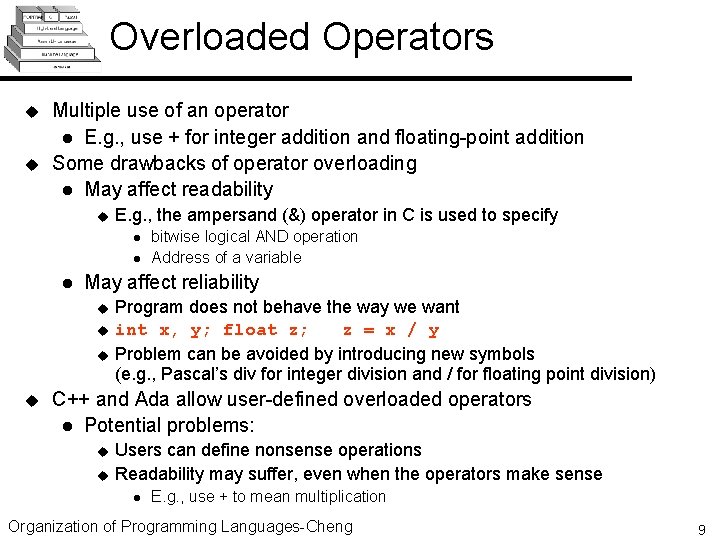
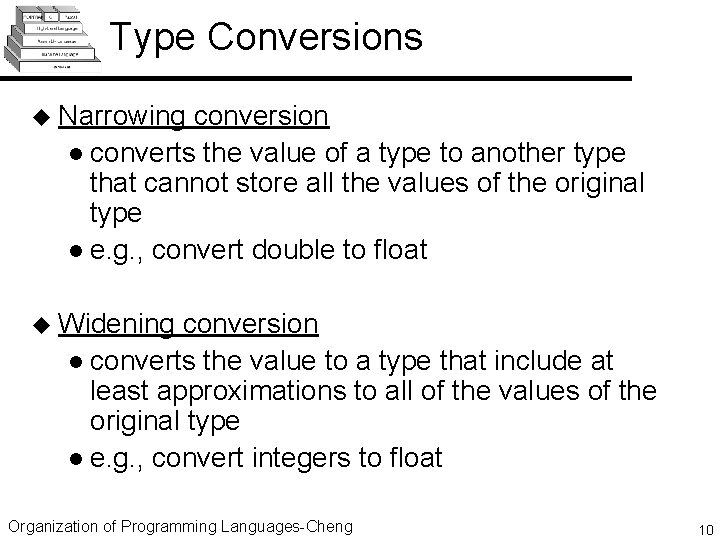
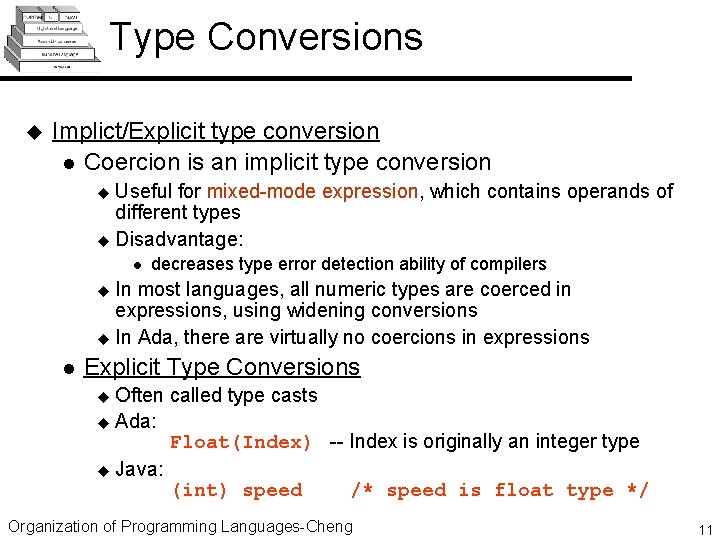
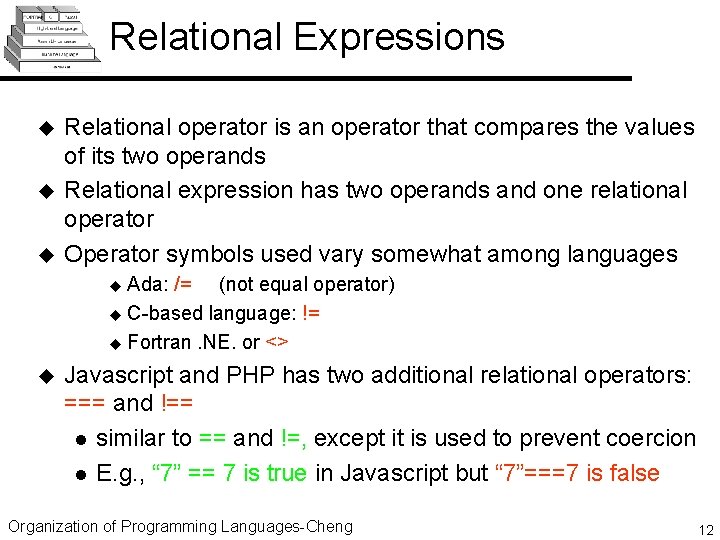
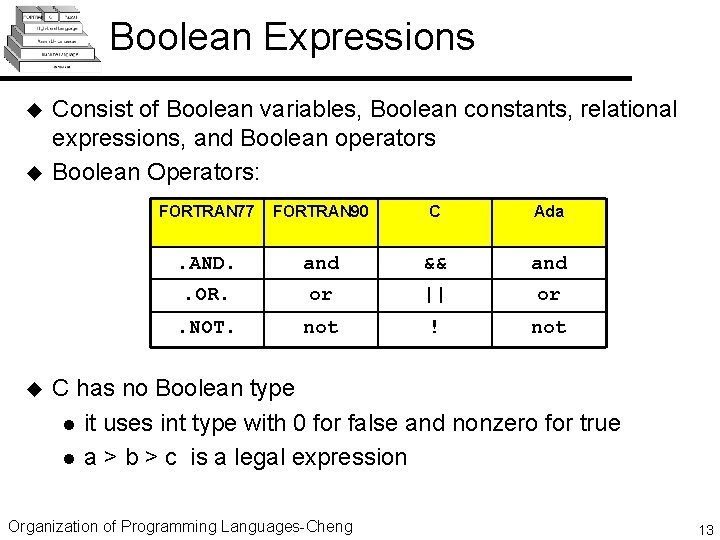
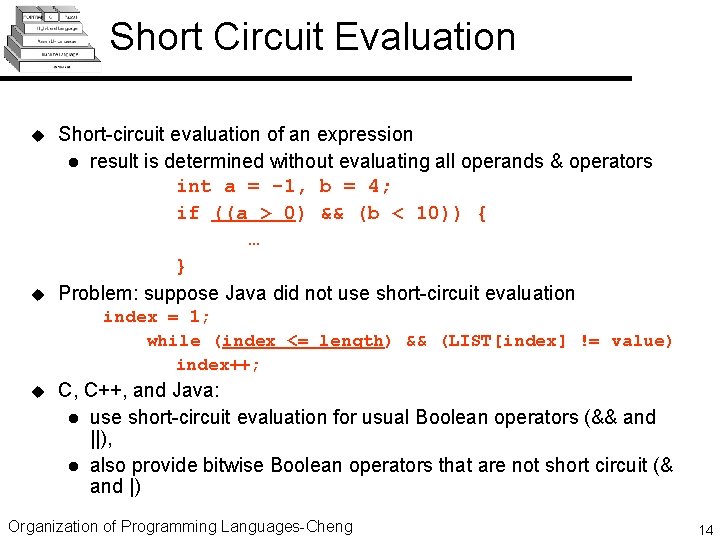
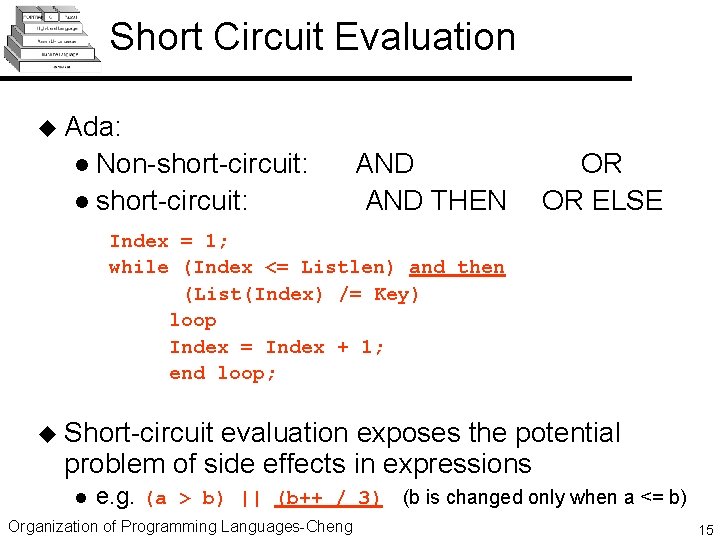
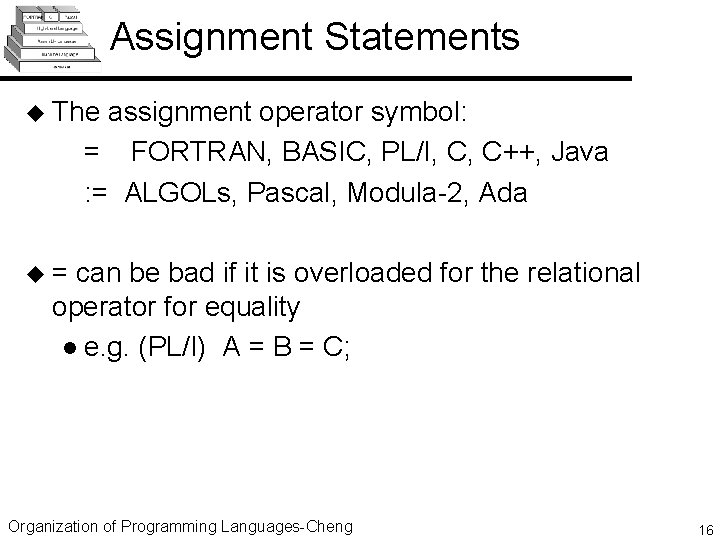
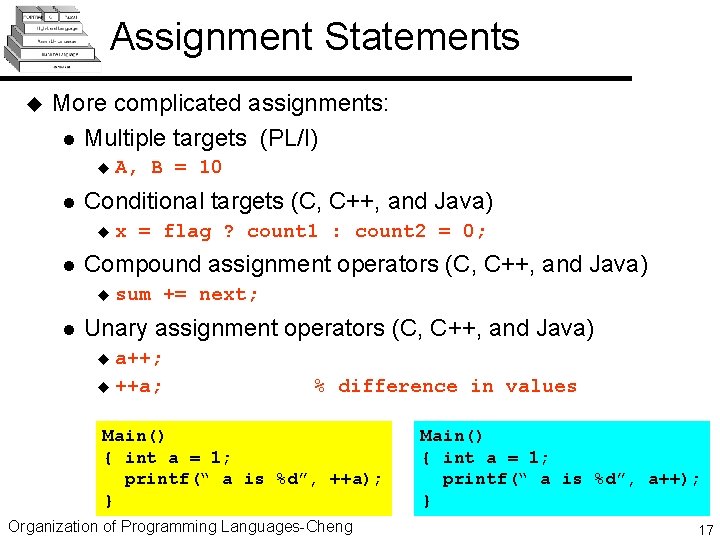
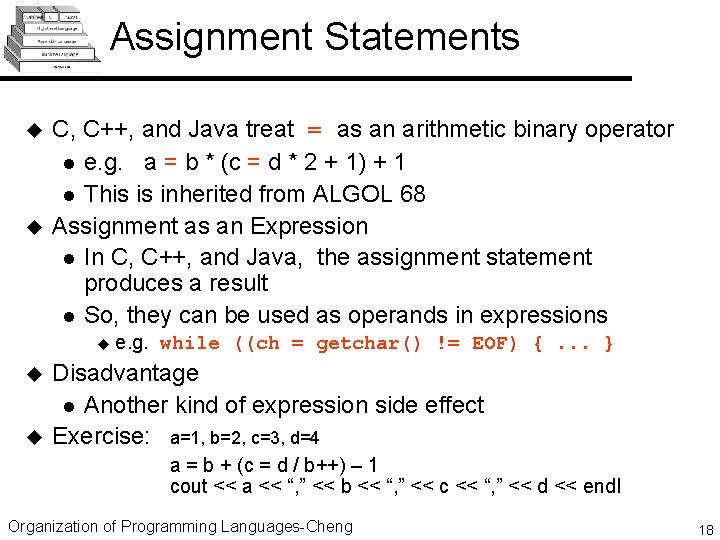
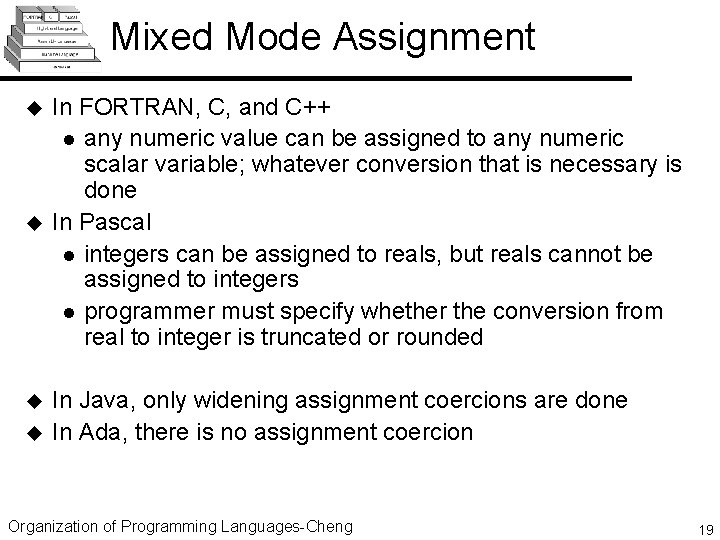
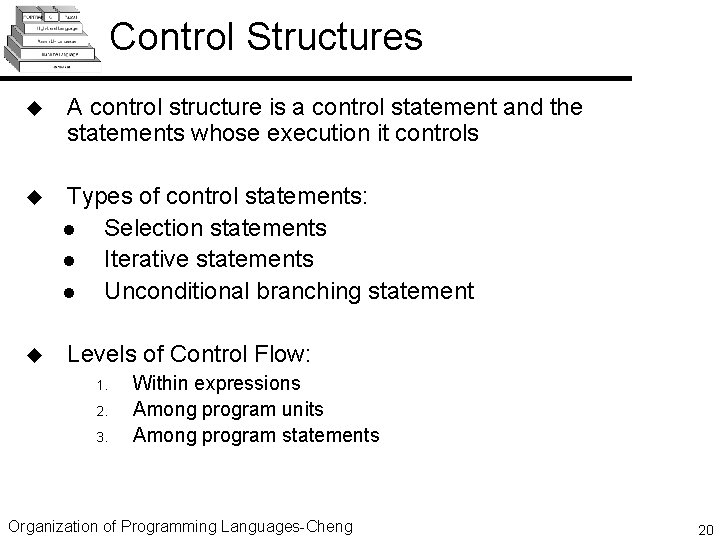
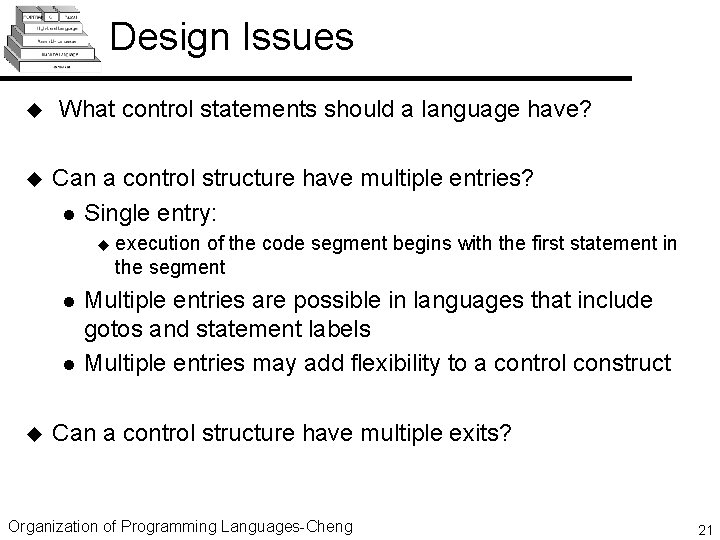
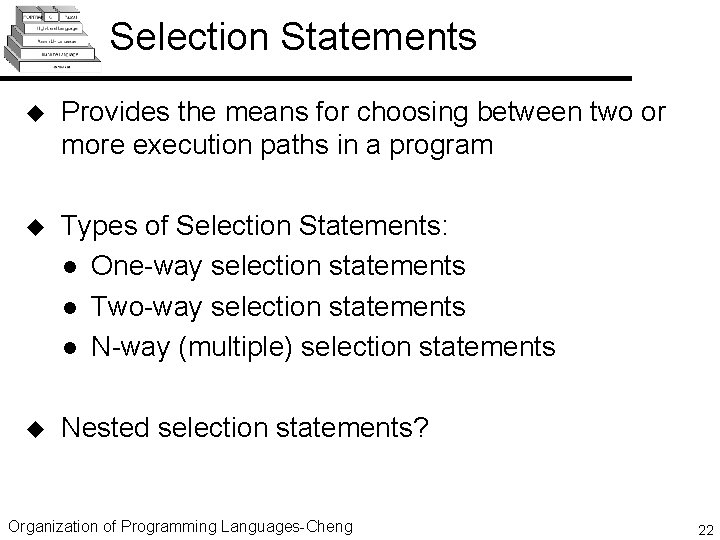
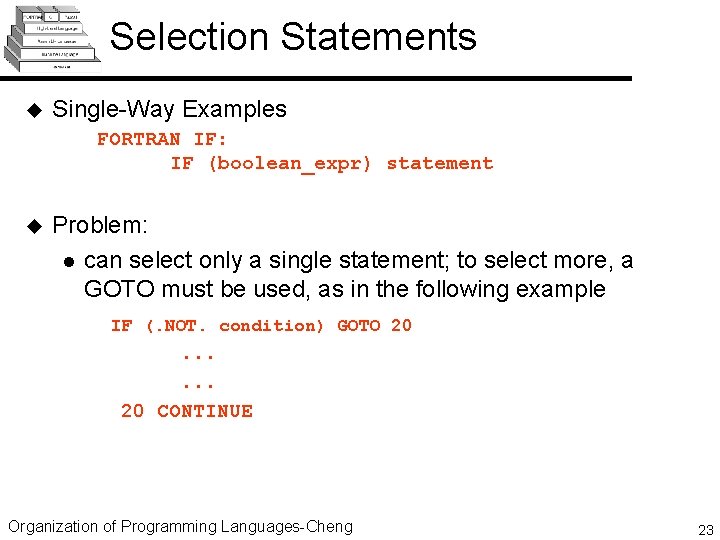
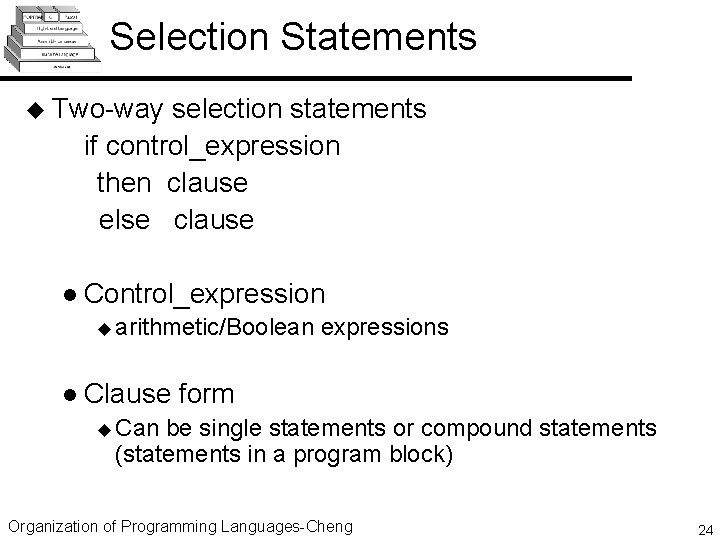
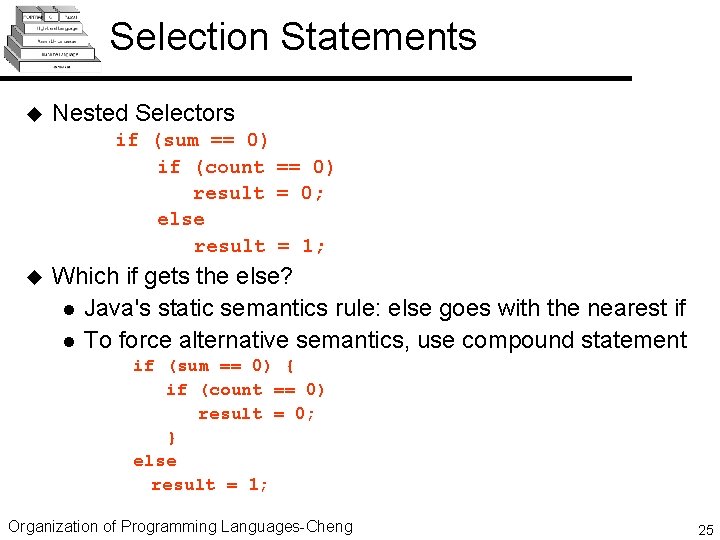
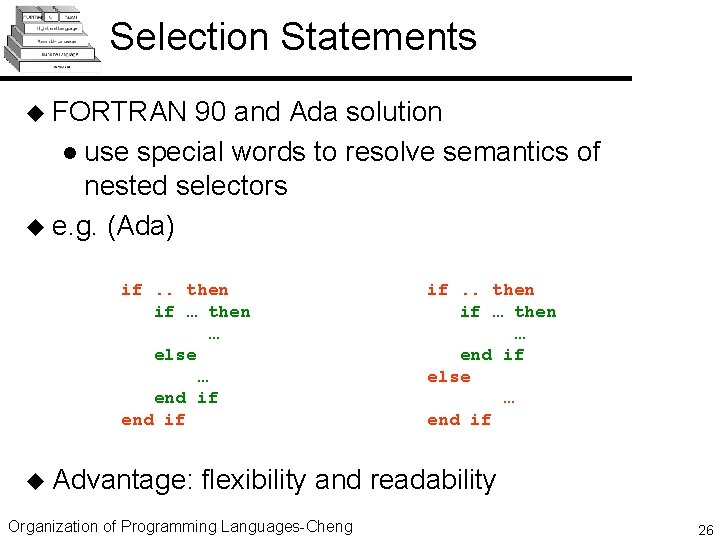
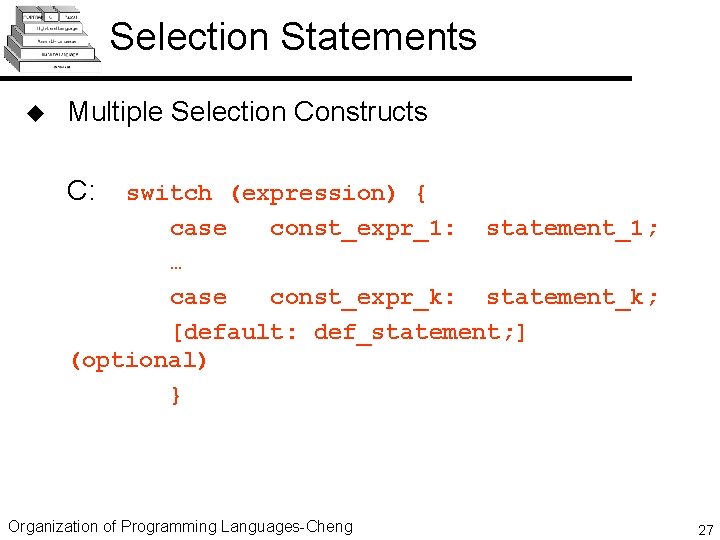
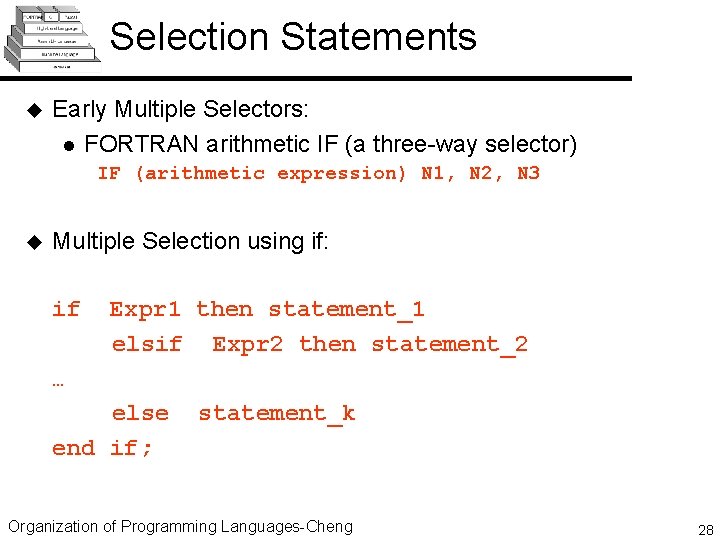
- Slides: 28
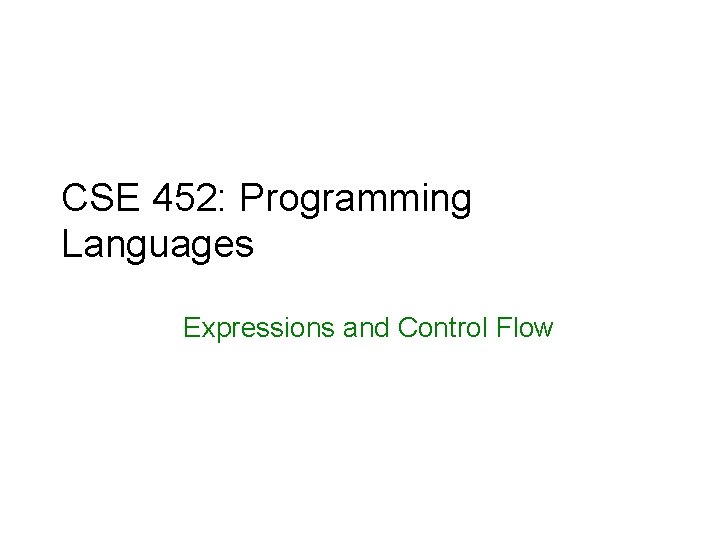
CSE 452: Programming Languages Expressions and Control Flow
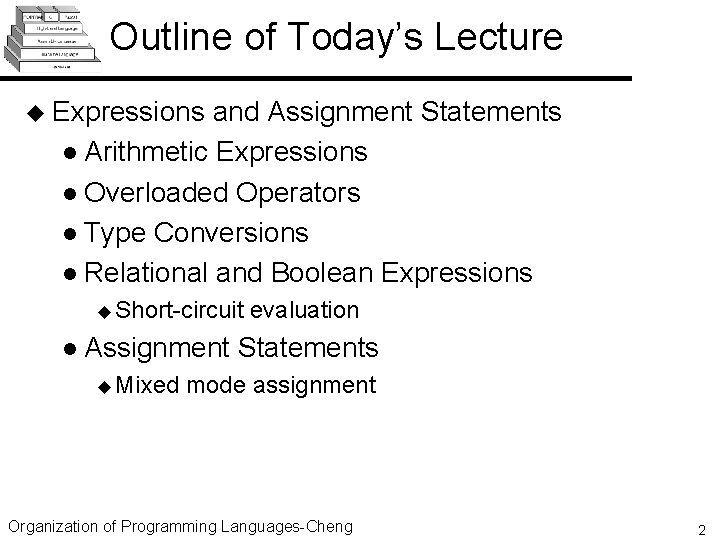
Outline of Today’s Lecture u Expressions and Assignment Statements l Arithmetic Expressions l Overloaded Operators l Type Conversions l Relational and Boolean Expressions u Short-circuit l Assignment u Mixed evaluation Statements mode assignment Organization of Programming Languages-Cheng 2
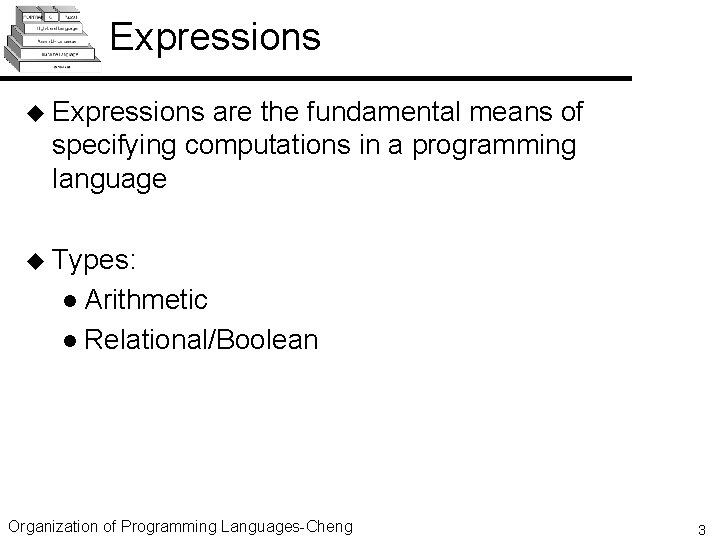
Expressions u Expressions are the fundamental means of specifying computations in a programming language u Types: l Arithmetic l Relational/Boolean Organization of Programming Languages-Cheng 3
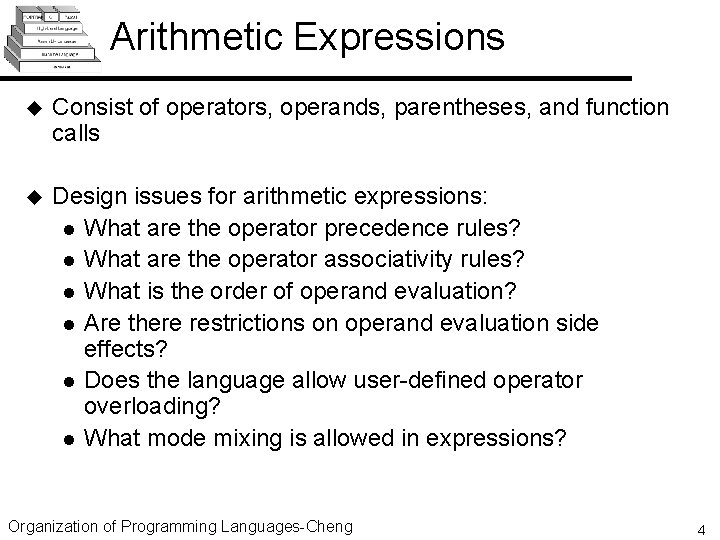
Arithmetic Expressions u Consist of operators, operands, parentheses, and function calls u Design issues for arithmetic expressions: l What are the operator precedence rules? l What are the operator associativity rules? l What is the order of operand evaluation? l Are there restrictions on operand evaluation side effects? l Does the language allow user-defined operator overloading? l What mode mixing is allowed in expressions? Organization of Programming Languages-Cheng 4
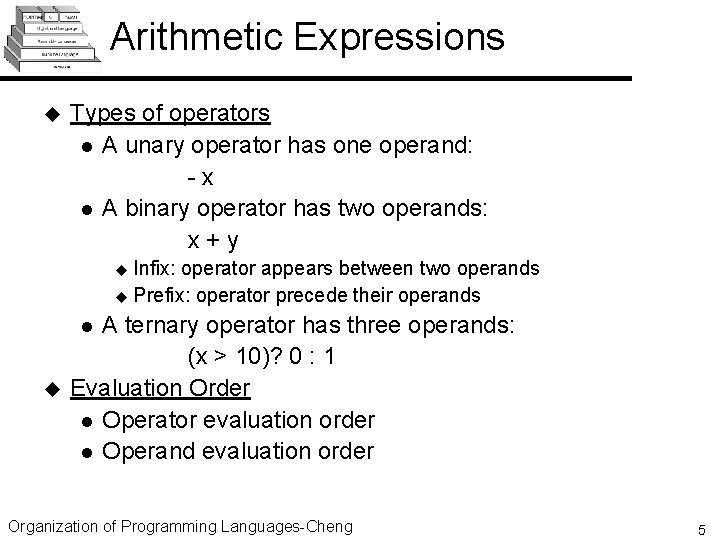
Arithmetic Expressions u Types of operators l A unary operator has one operand: -x l A binary operator has two operands: x+y Infix: operator appears between two operands u Prefix: operator precede their operands u A ternary operator has three operands: (x > 10)? 0 : 1 Evaluation Order l Operator evaluation order l Operand evaluation order l u Organization of Programming Languages-Cheng 5
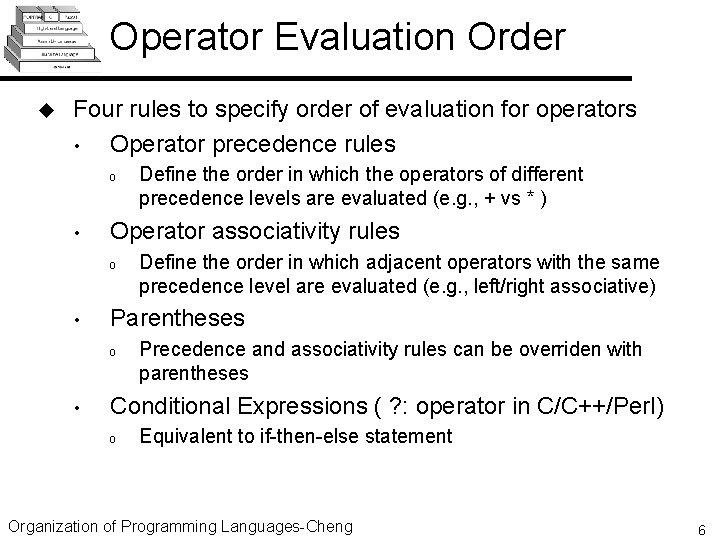
Operator Evaluation Order u Four rules to specify order of evaluation for operators • Operator precedence rules o • Operator associativity rules o • Define the order in which adjacent operators with the same precedence level are evaluated (e. g. , left/right associative) Parentheses o • Define the order in which the operators of different precedence levels are evaluated (e. g. , + vs * ) Precedence and associativity rules can be overriden with parentheses Conditional Expressions ( ? : operator in C/C++/Perl) o Equivalent to if-then-else statement Organization of Programming Languages-Cheng 6
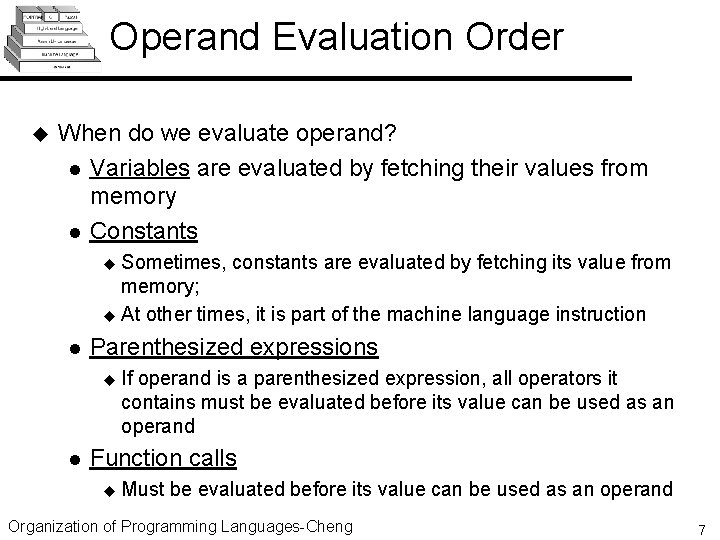
Operand Evaluation Order u When do we evaluate operand? l Variables are evaluated by fetching their values from memory l Constants Sometimes, constants are evaluated by fetching its value from memory; u At other times, it is part of the machine language instruction u l Parenthesized expressions u l If operand is a parenthesized expression, all operators it contains must be evaluated before its value can be used as an operand Function calls u Must be evaluated before its value can be used as an operand Organization of Programming Languages-Cheng 7
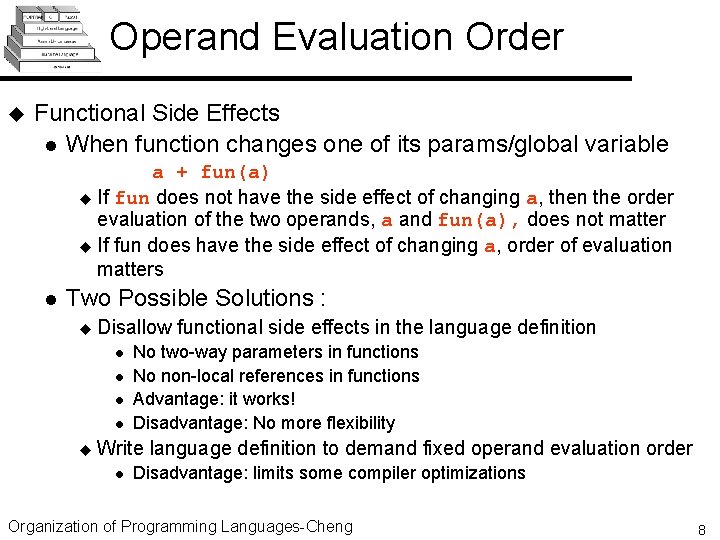
Operand Evaluation Order u Functional Side Effects l When function changes one of its params/global variable a + fun(a) u If fun does not have the side effect of changing a, then the order evaluation of the two operands, a and fun(a), does not matter u If fun does have the side effect of changing a, order of evaluation matters l Two Possible Solutions : u Disallow functional side effects in the language definition l l u No two-way parameters in functions No non-local references in functions Advantage: it works! Disadvantage: No more flexibility Write language definition to demand fixed operand evaluation order l Disadvantage: limits some compiler optimizations Organization of Programming Languages-Cheng 8
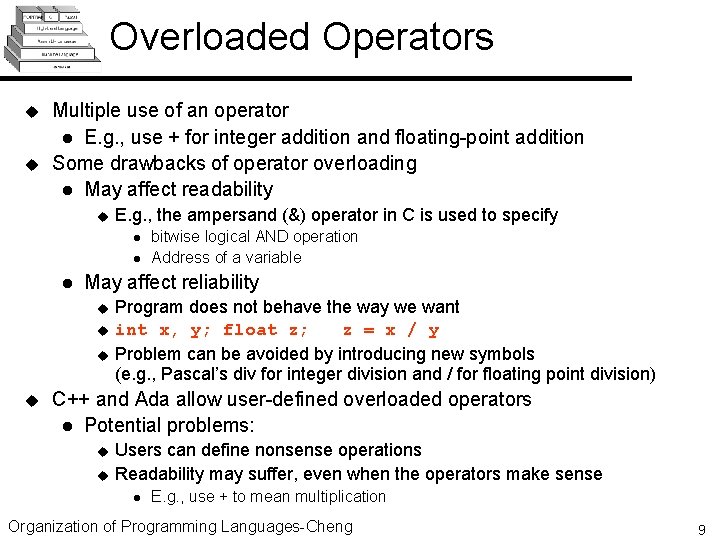
Overloaded Operators u u Multiple use of an operator l E. g. , use + for integer addition and floating-point addition Some drawbacks of operator overloading l May affect readability u E. g. , the ampersand (&) operator in C is used to specify l l l May affect reliability u u bitwise logical AND operation Address of a variable Program does not behave the way we want int x, y; float z; z = x / y Problem can be avoided by introducing new symbols (e. g. , Pascal’s div for integer division and / for floating point division) C++ and Ada allow user-defined overloaded operators l Potential problems: u u Users can define nonsense operations Readability may suffer, even when the operators make sense l E. g. , use + to mean multiplication Organization of Programming Languages-Cheng 9
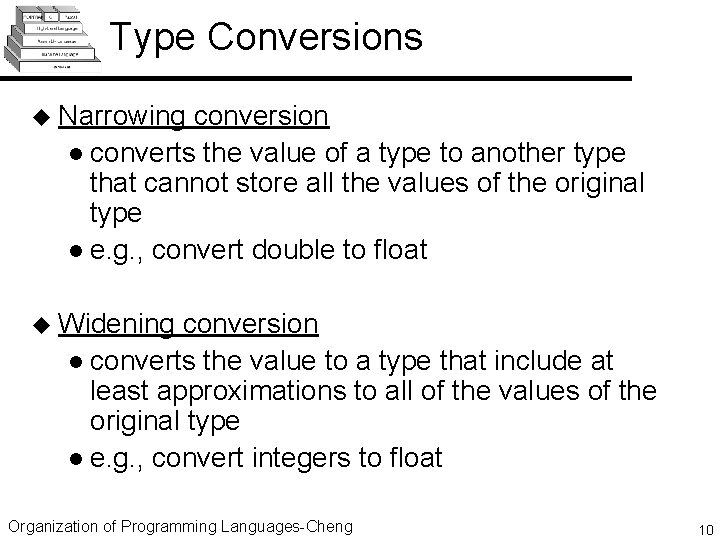
Type Conversions u Narrowing conversion l converts the value of a type to another type that cannot store all the values of the original type l e. g. , convert double to float u Widening conversion l converts the value to a type that include at least approximations to all of the values of the original type l e. g. , convert integers to float Organization of Programming Languages-Cheng 10
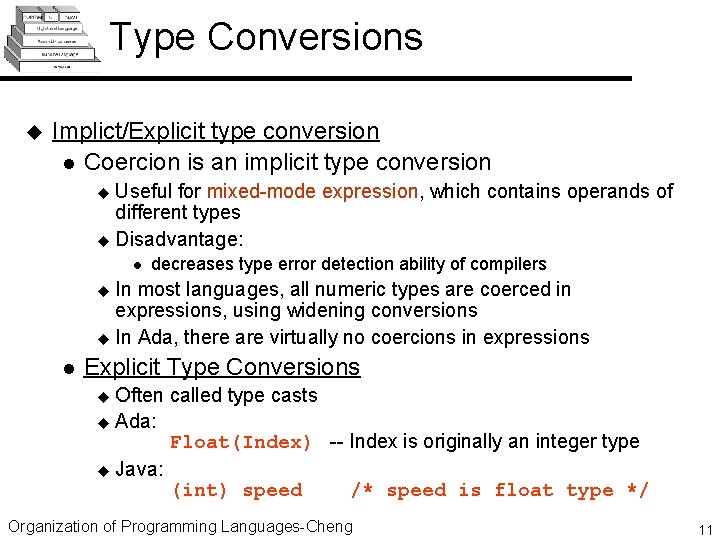
Type Conversions u Implict/Explicit type conversion l Coercion is an implicit type conversion Useful for mixed-mode expression, which contains operands of different types u Disadvantage: u l decreases type error detection ability of compilers In most languages, all numeric types are coerced in expressions, using widening conversions u In Ada, there are virtually no coercions in expressions u l Explicit Type Conversions Often called type casts u Ada: Float(Index) -- Index is originally an integer type u Java: (int) speed /* speed is float type */ u Organization of Programming Languages-Cheng 11
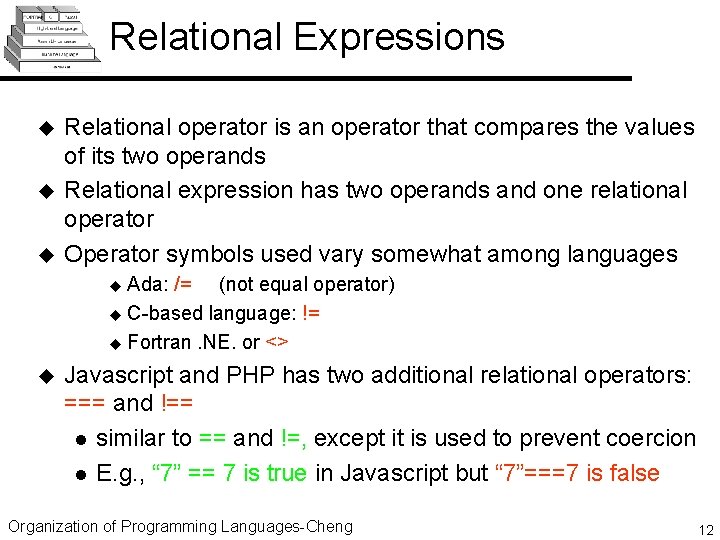
Relational Expressions u u u Relational operator is an operator that compares the values of its two operands Relational expression has two operands and one relational operator Operator symbols used vary somewhat among languages Ada: /= (not equal operator) u C-based language: != u Fortran. NE. or <> u u Javascript and PHP has two additional relational operators: === and !== l similar to == and !=, except it is used to prevent coercion l E. g. , “ 7” == 7 is true in Javascript but “ 7”===7 is false Organization of Programming Languages-Cheng 12
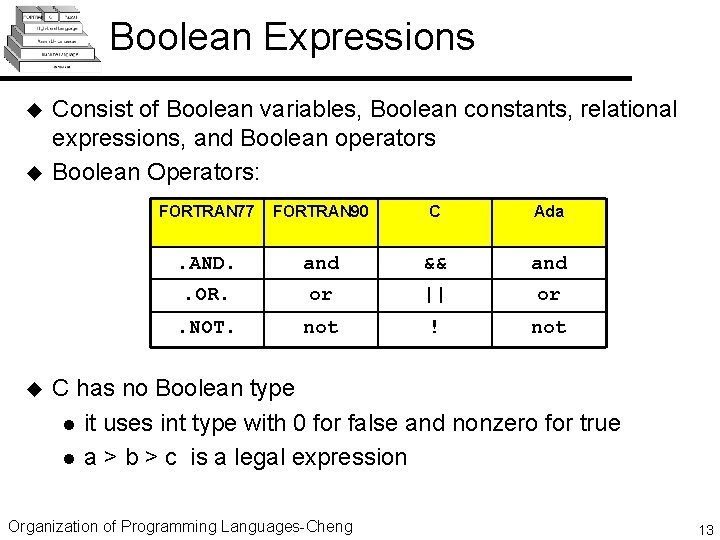
Boolean Expressions u u u Consist of Boolean variables, Boolean constants, relational expressions, and Boolean operators Boolean Operators: FORTRAN 77 FORTRAN 90 C Ada . AND. and && and . OR. or || or . NOT. not ! not C has no Boolean type l it uses int type with 0 for false and nonzero for true l a > b > c is a legal expression Organization of Programming Languages-Cheng 13
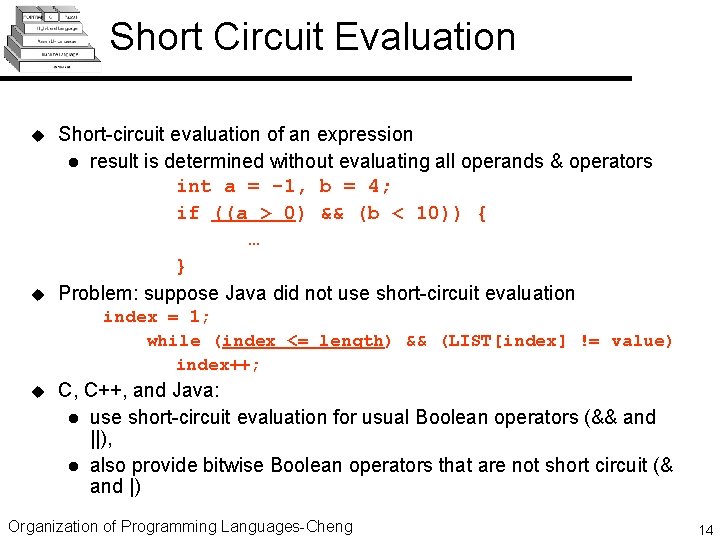
Short Circuit Evaluation u u Short-circuit evaluation of an expression l result is determined without evaluating all operands & operators int a = -1, b = 4; if ((a > 0) && (b < 10)) { … } Problem: suppose Java did not use short-circuit evaluation index = 1; while (index <= length) && (LIST[index] != value) index++; u C, C++, and Java: l use short-circuit evaluation for usual Boolean operators (&& and ||), l also provide bitwise Boolean operators that are not short circuit (& and |) Organization of Programming Languages-Cheng 14
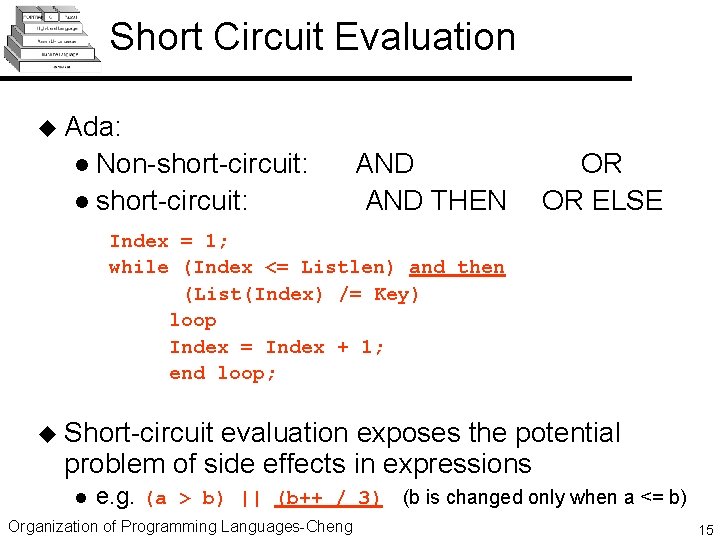
Short Circuit Evaluation u Ada: l Non-short-circuit: l short-circuit: AND THEN OR OR ELSE Index = 1; while (Index <= Listlen) and then (List(Index) /= Key) loop Index = Index + 1; end loop; u Short-circuit evaluation exposes the potential problem of side effects in expressions l e. g. (a > b) || (b++ / 3) (b is changed only when a <= b) Organization of Programming Languages-Cheng 15
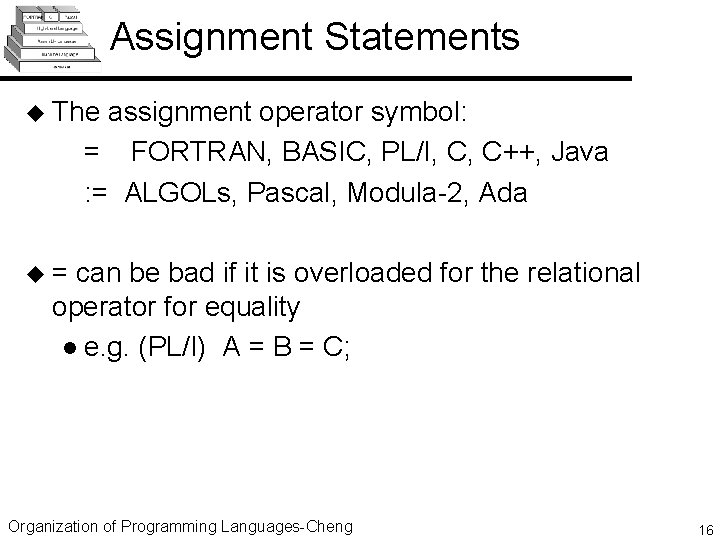
Assignment Statements u The assignment operator symbol: = FORTRAN, BASIC, PL/I, C, C++, Java : = ALGOLs, Pascal, Modula-2, Ada u= can be bad if it is overloaded for the relational operator for equality l e. g. (PL/I) A = B = C; Organization of Programming Languages-Cheng 16
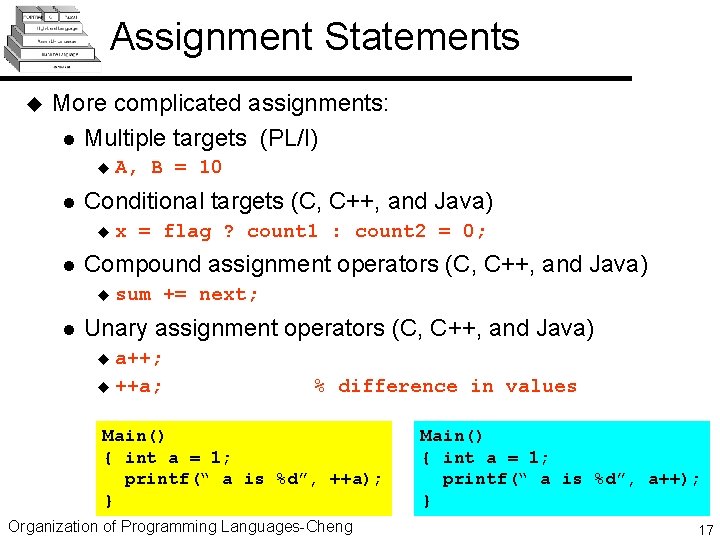
Assignment Statements u More complicated assignments: l Multiple targets (PL/I) u l Conditional targets (C, C++, and Java) u l x = flag ? count 1 : count 2 = 0; Compound assignment operators (C, C++, and Java) u l A, B = 10 sum += next; Unary assignment operators (C, C++, and Java) a++; u ++a; u % difference in values Main() { int a = 1; printf(“ a is %d”, ++a); } Organization of Programming Languages-Cheng Main() { int a = 1; printf(“ a is %d”, a++); } 17
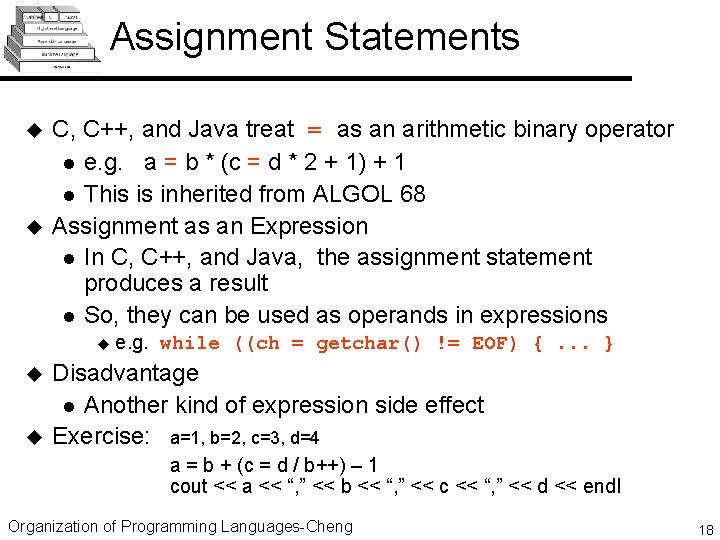
Assignment Statements u u C, C++, and Java treat = as an arithmetic binary operator l e. g. a = b * (c = d * 2 + 1) + 1 l This is inherited from ALGOL 68 Assignment as an Expression l In C, C++, and Java, the assignment statement produces a result l So, they can be used as operands in expressions u u u e. g. while ((ch = getchar() != EOF) {. . . } Disadvantage l Another kind of expression side effect Exercise: a=1, b=2, c=3, d=4 a = b + (c = d / b++) – 1 cout << a << “, ” << b << “, ” << c << “, ” << d << endl Organization of Programming Languages-Cheng 18
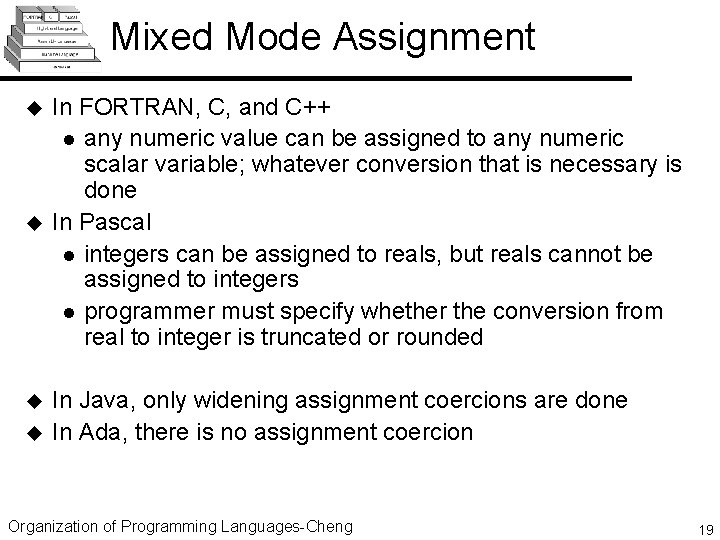
Mixed Mode Assignment u u In FORTRAN, C, and C++ l any numeric value can be assigned to any numeric scalar variable; whatever conversion that is necessary is done In Pascal l integers can be assigned to reals, but reals cannot be assigned to integers l programmer must specify whether the conversion from real to integer is truncated or rounded In Java, only widening assignment coercions are done In Ada, there is no assignment coercion Organization of Programming Languages-Cheng 19
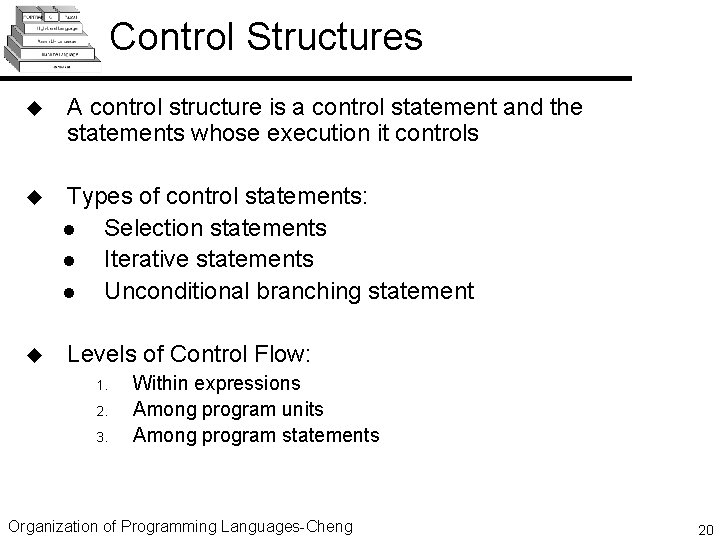
Control Structures u A control structure is a control statement and the statements whose execution it controls u Types of control statements: l Selection statements l Iterative statements l Unconditional branching statement u Levels of Control Flow: 1. 2. 3. Within expressions Among program units Among program statements Organization of Programming Languages-Cheng 20
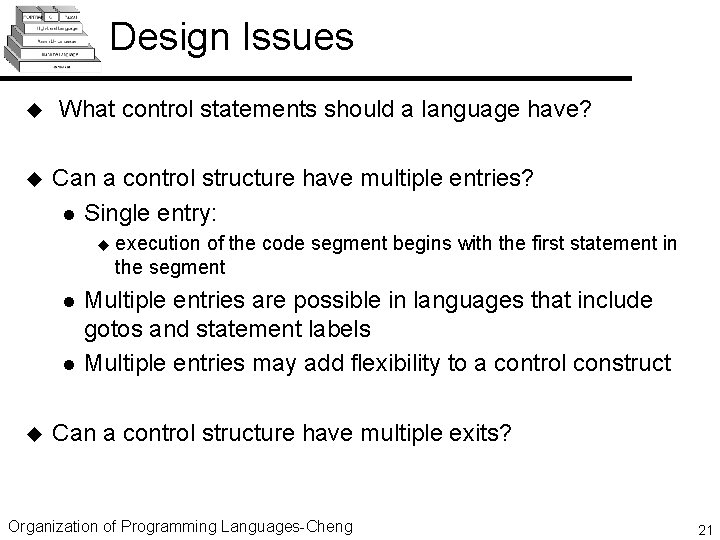
Design Issues u u What control statements should a language have? Can a control structure have multiple entries? l Single entry: u l l u execution of the code segment begins with the first statement in the segment Multiple entries are possible in languages that include gotos and statement labels Multiple entries may add flexibility to a control construct Can a control structure have multiple exits? Organization of Programming Languages-Cheng 21
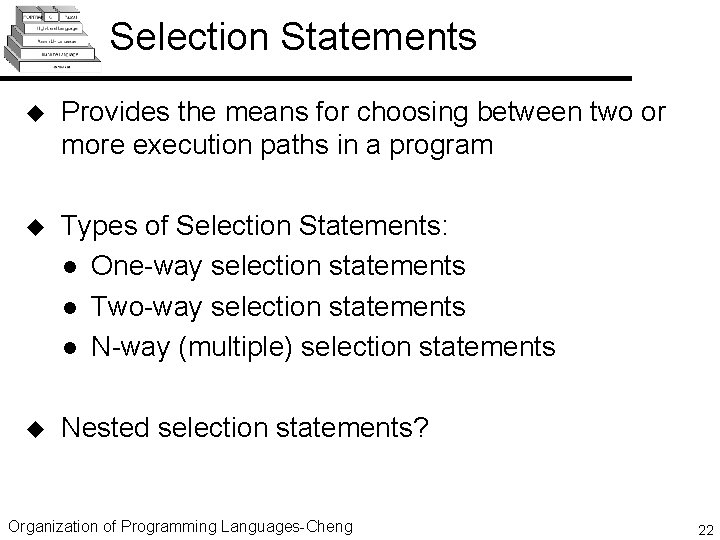
Selection Statements u Provides the means for choosing between two or more execution paths in a program u Types of Selection Statements: l One-way selection statements l Two-way selection statements l N-way (multiple) selection statements u Nested selection statements? Organization of Programming Languages-Cheng 22
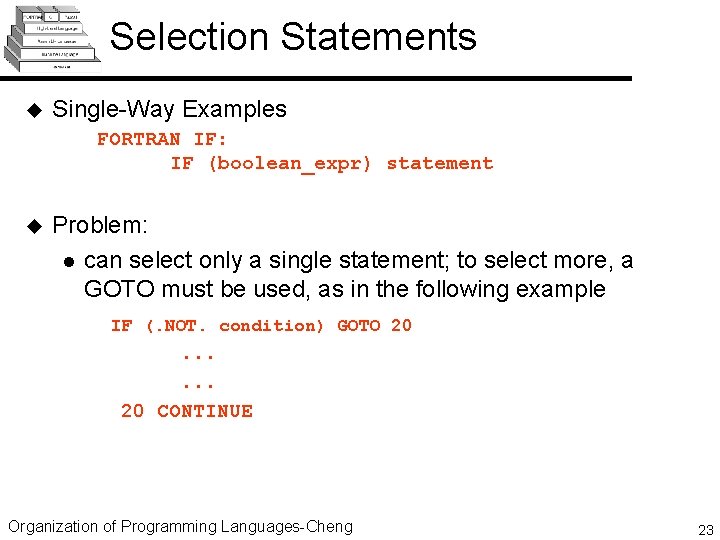
Selection Statements u Single-Way Examples FORTRAN IF: IF (boolean_expr) statement u Problem: l can select only a single statement; to select more, a GOTO must be used, as in the following example IF (. NOT. condition) GOTO 20 . . . 20 CONTINUE Organization of Programming Languages-Cheng 23
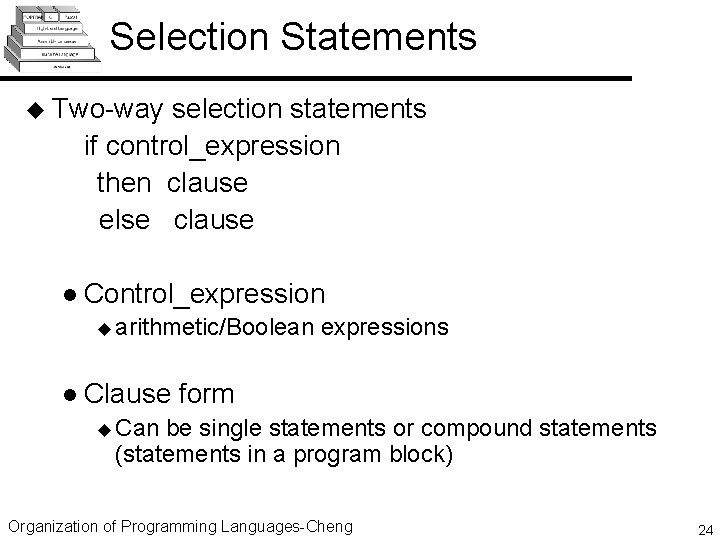
Selection Statements u Two-way selection statements if control_expression then clause else clause l Control_expression u arithmetic/Boolean l Clause expressions form u Can be single statements or compound statements (statements in a program block) Organization of Programming Languages-Cheng 24
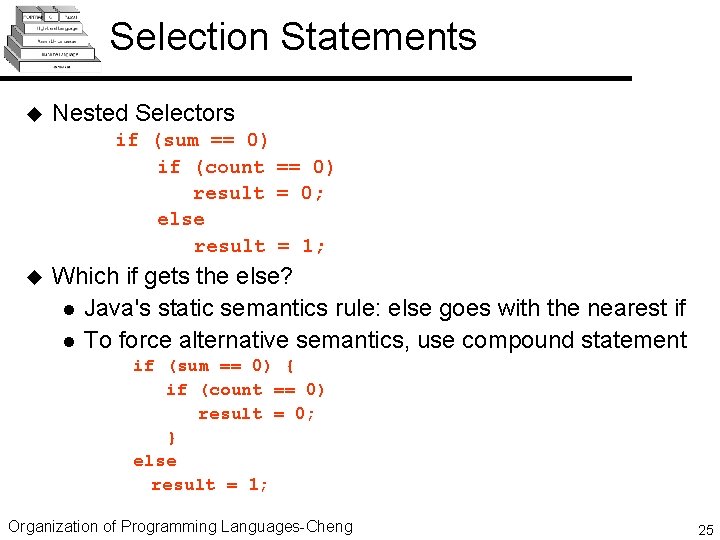
Selection Statements u Nested Selectors if (sum == 0) if (count == 0) result = 0; else result = 1; u Which if gets the else? l Java's static semantics rule: else goes with the nearest if l To force alternative semantics, use compound statement if (sum == 0) { if (count == 0) result = 0; } else result = 1; Organization of Programming Languages-Cheng 25
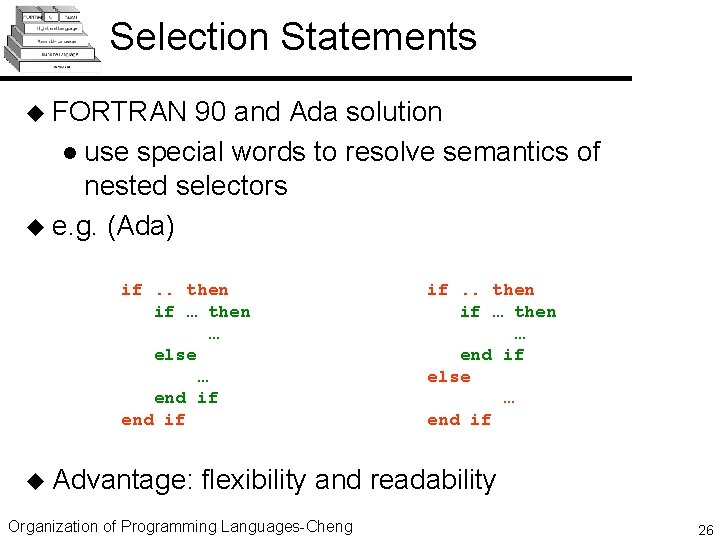
Selection Statements u FORTRAN 90 and Ada solution l use special words to resolve semantics of nested selectors u e. g. (Ada) if. . then if … then … else … end if u Advantage: if. . then if … then … end if else … end if flexibility and readability Organization of Programming Languages-Cheng 26
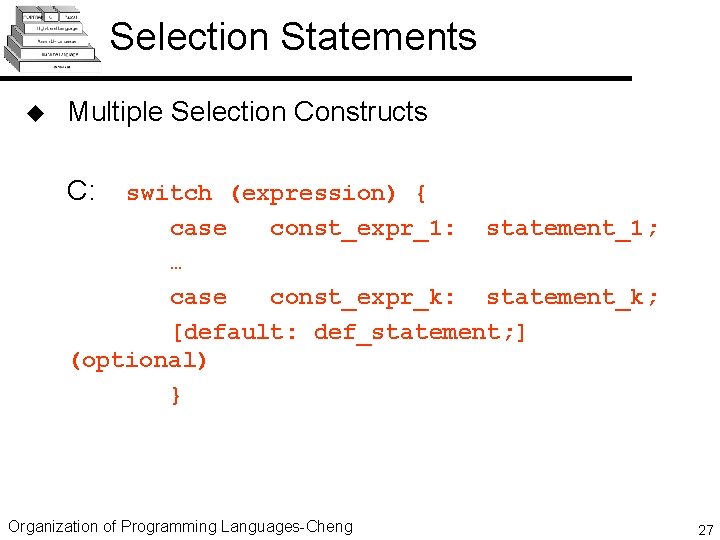
Selection Statements u Multiple Selection Constructs C: switch (expression) { case const_expr_1: statement_1; … case const_expr_k: statement_k; [default: def_statement; ] (optional) } Organization of Programming Languages-Cheng 27
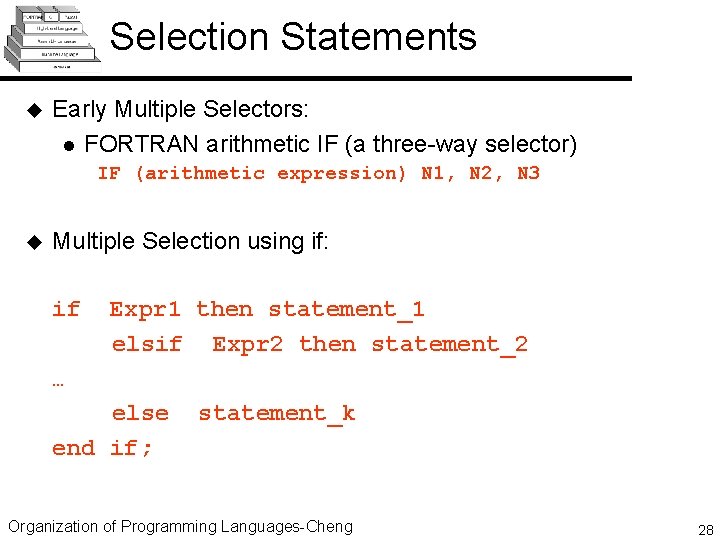
Selection Statements u Early Multiple Selectors: l FORTRAN arithmetic IF (a three-way selector) IF (arithmetic expression) N 1, N 2, N 3 u Multiple Selection using if: if Expr 1 then statement_1 elsif Expr 2 then statement_2 … else end if; statement_k Organization of Programming Languages-Cheng 28
Cse 340 principles of programming languages
Cse 340 principles of programming languages
Cse 452
Cse452
Cse 452
Cse 452
Cse 452
Cse 452
Cse 452
Cse 452
Control flow and data flow computers
Real-time systems and programming languages
Cs 421
Advantages of high level language
Real time programming language
Cs 421 programming languages and compilers
Flow and error control
Data flow vs control flow
Transaction flow graph
Multithreading program in java
Cxc it
Introduction to programming languages
Plc coding language
Procedural programming languages
Imperative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Types of programming languages