CSE 3302 Programming Languages Control I Expressions and
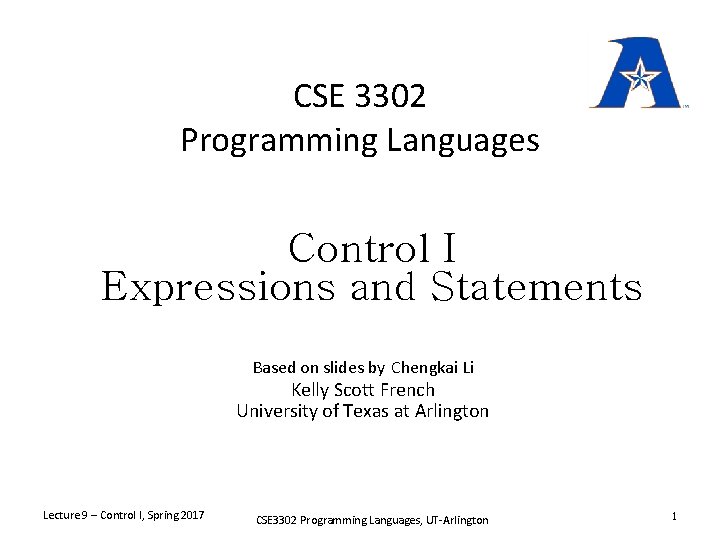
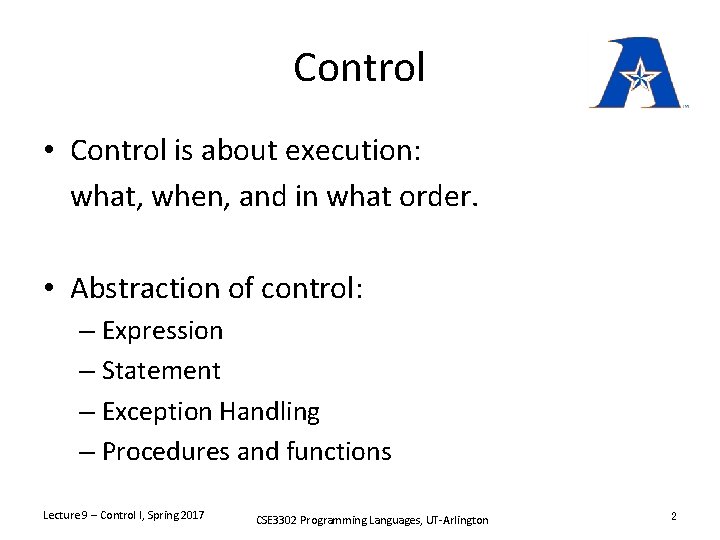
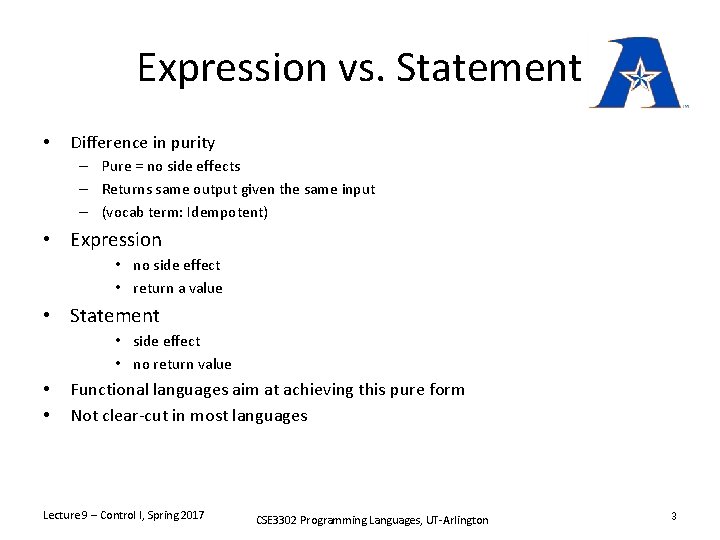
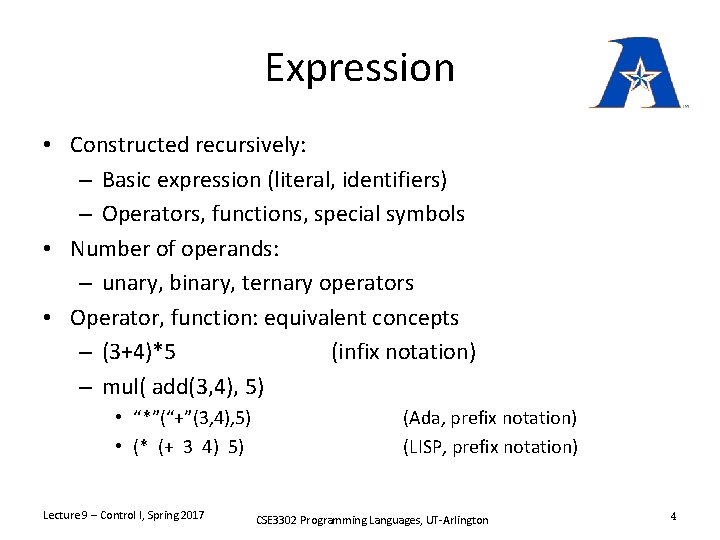
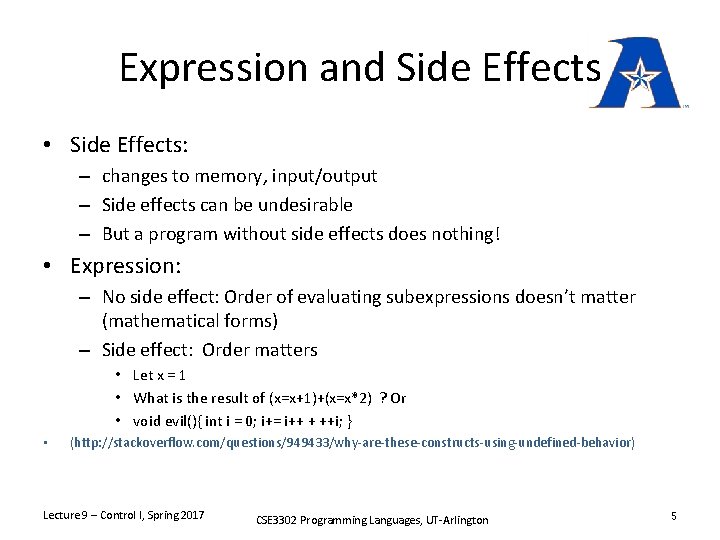
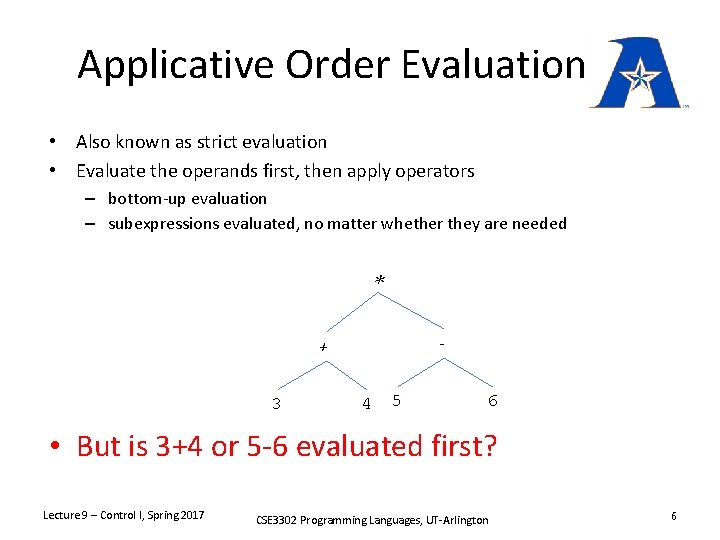
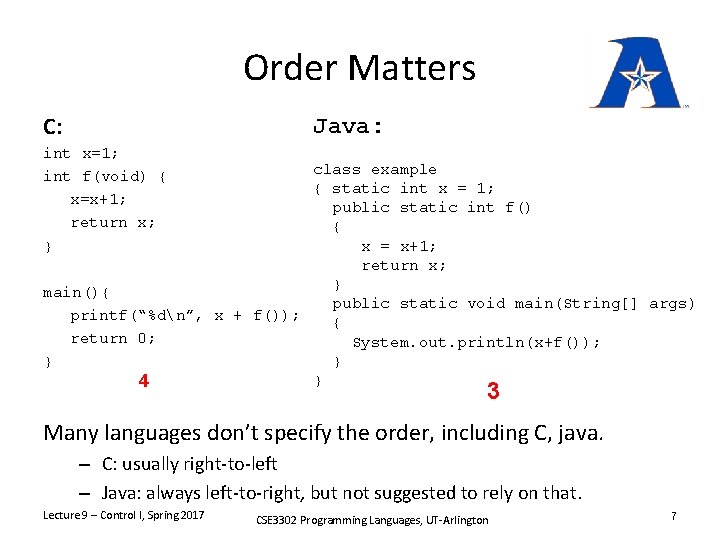
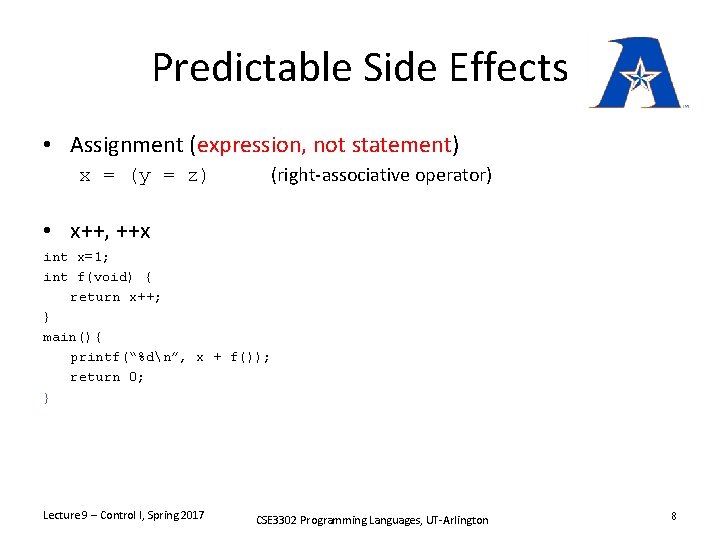
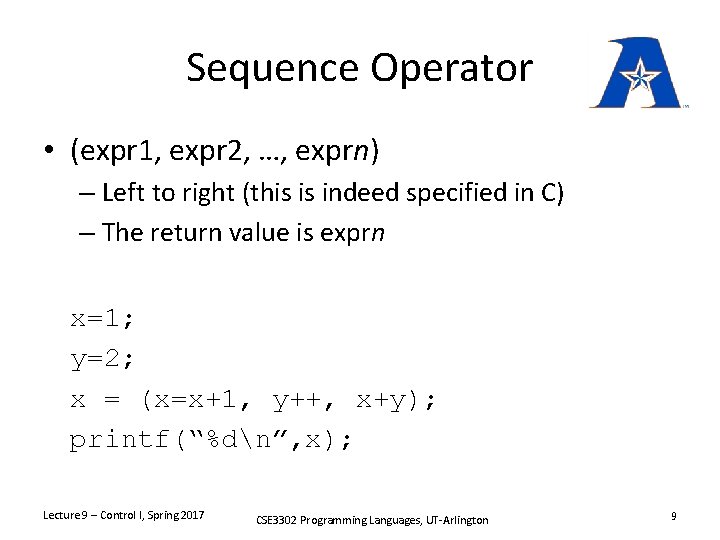
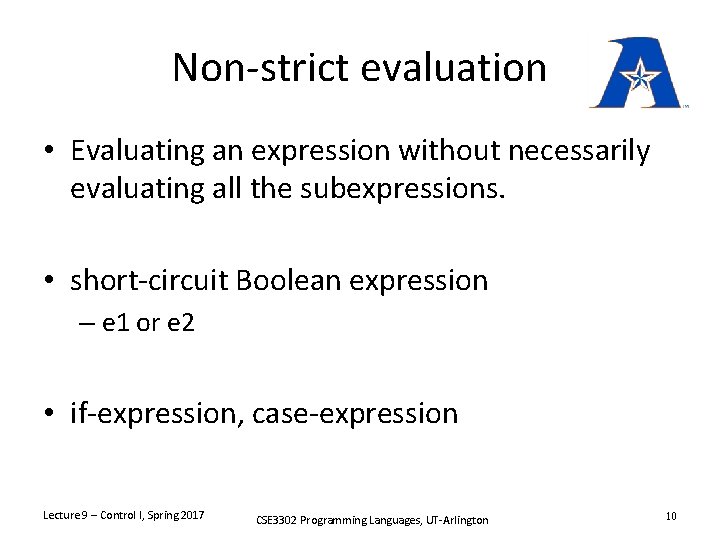
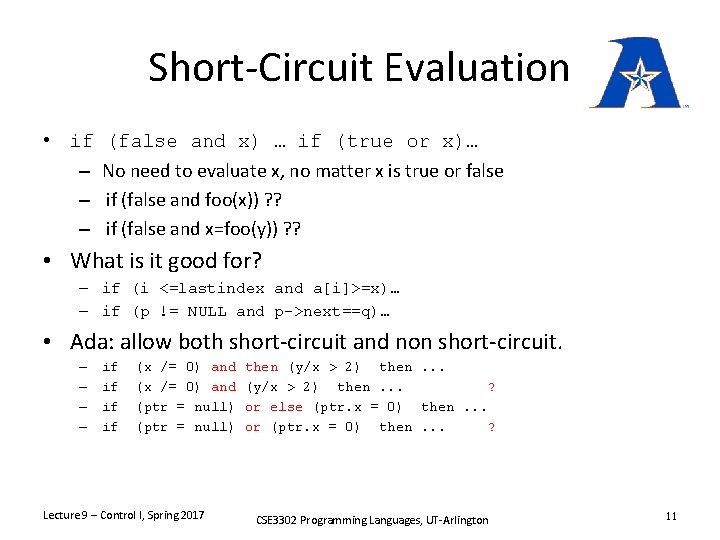
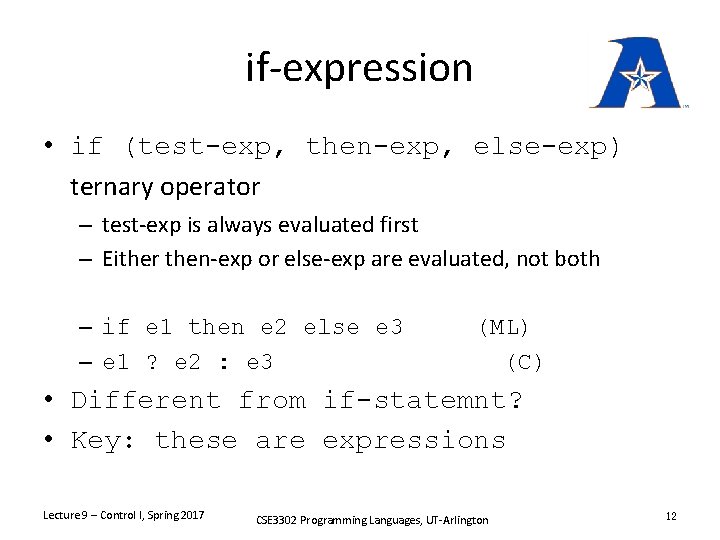
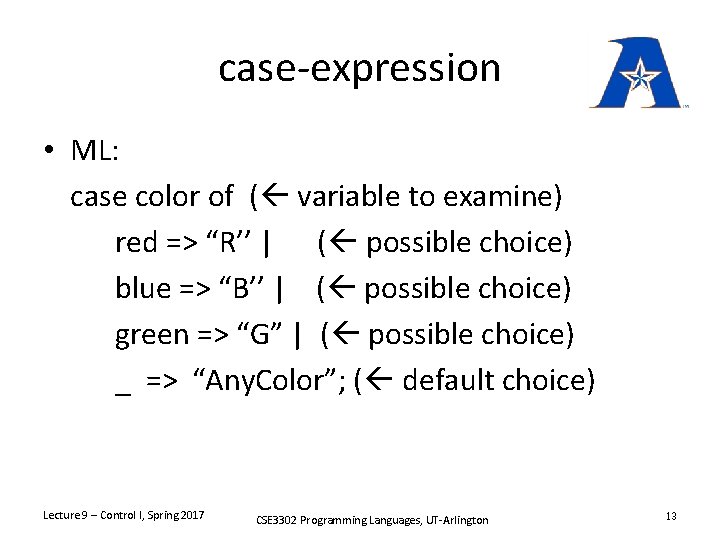
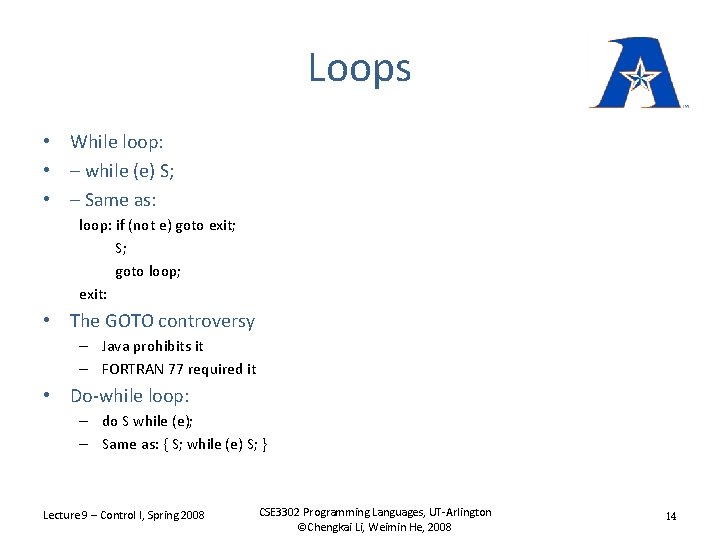
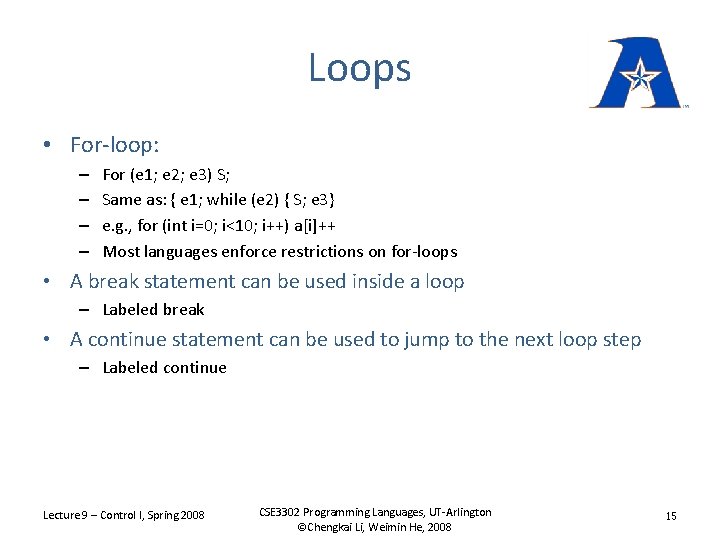
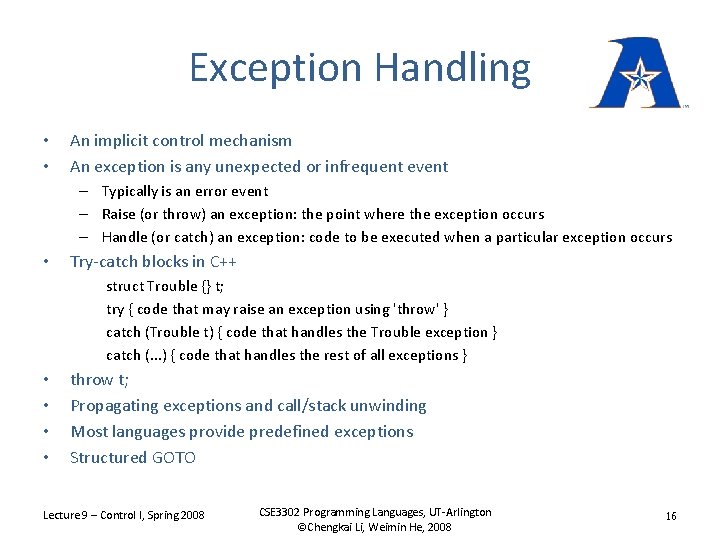
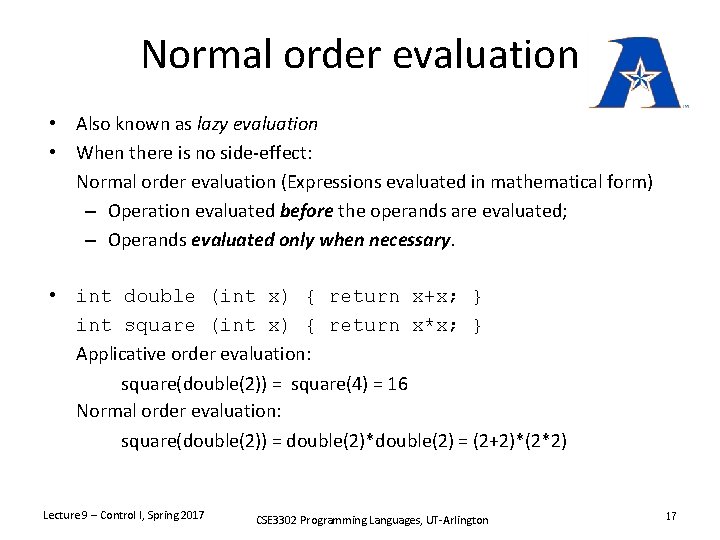
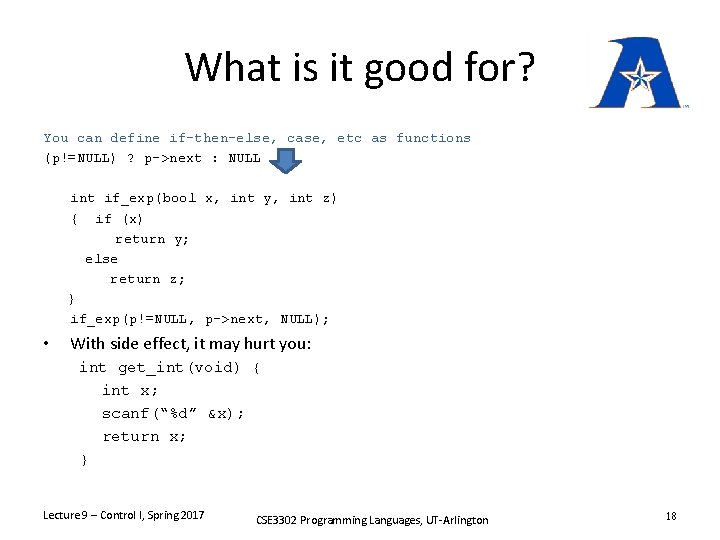
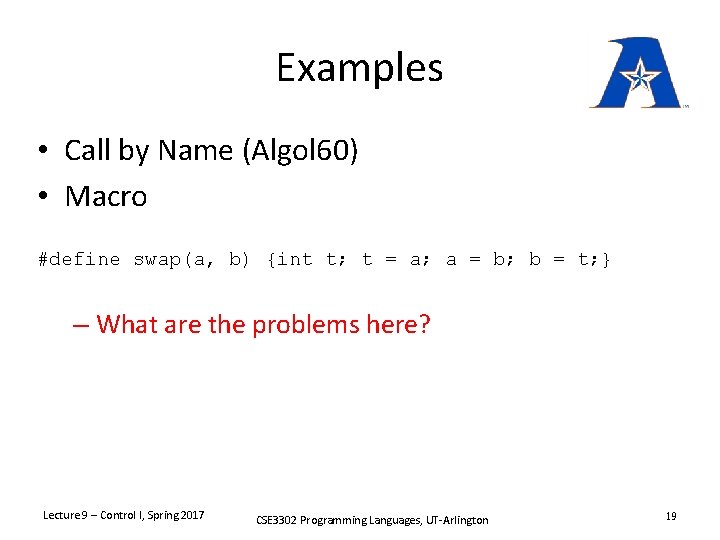
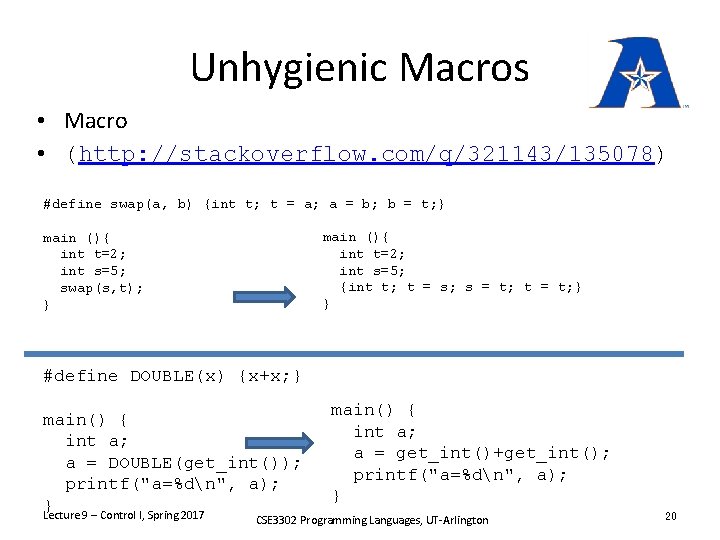
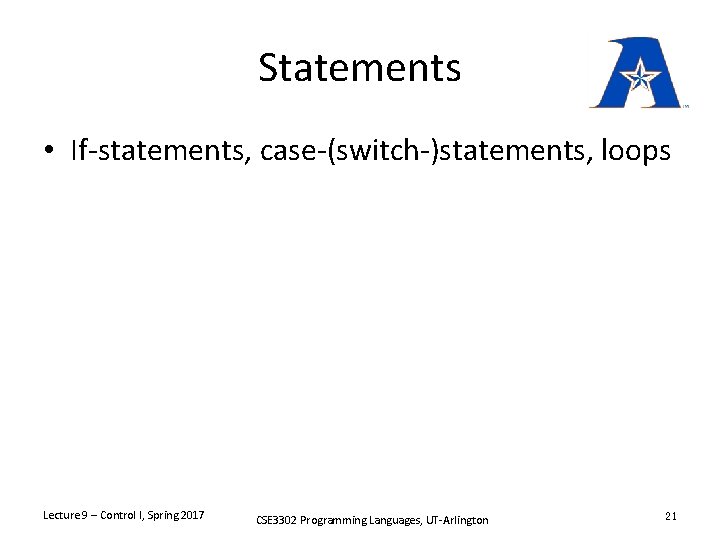
- Slides: 21
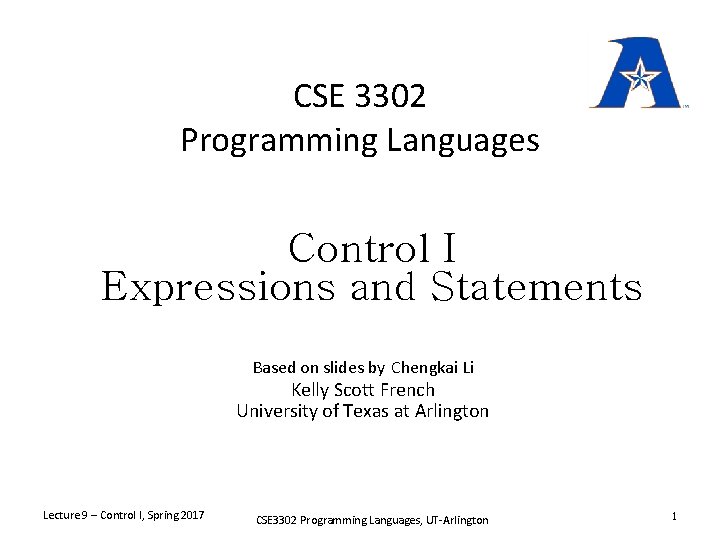
CSE 3302 Programming Languages Control I Expressions and Statements Based on slides by Chengkai Li Kelly Scott French University of Texas at Arlington Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 1
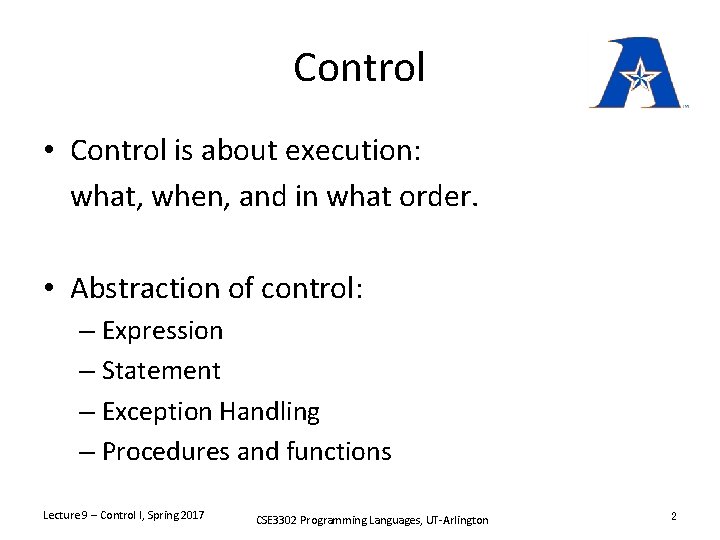
Control • Control is about execution: what, when, and in what order. • Abstraction of control: – Expression – Statement – Exception Handling – Procedures and functions Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 2
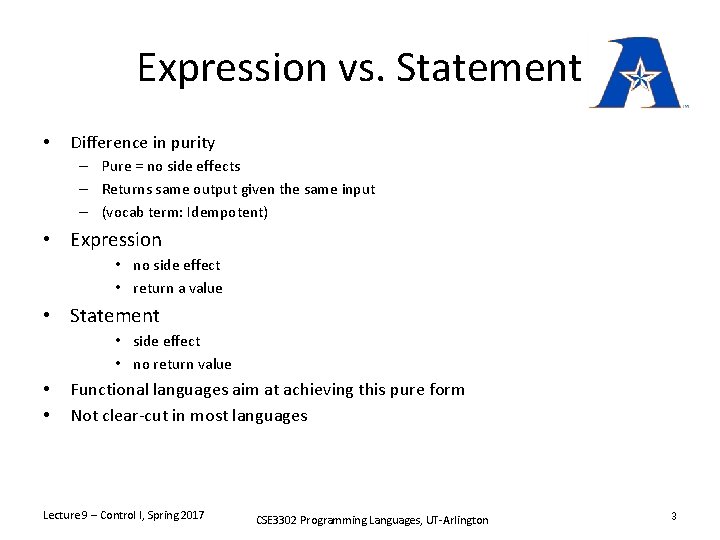
Expression vs. Statement • Difference in purity – Pure = no side effects – Returns same output given the same input – (vocab term: Idempotent) • Expression • no side effect • return a value • Statement • side effect • no return value • • Functional languages aim at achieving this pure form Not clear-cut in most languages Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 3
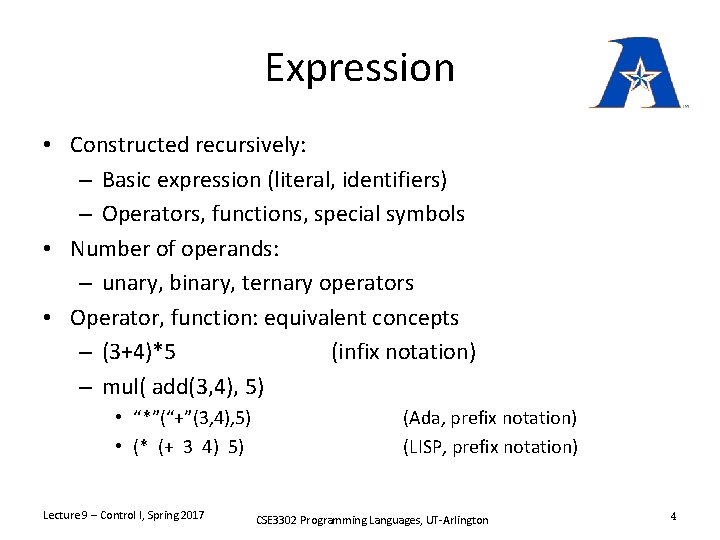
Expression • Constructed recursively: – Basic expression (literal, identifiers) – Operators, functions, special symbols • Number of operands: – unary, binary, ternary operators • Operator, function: equivalent concepts – (3+4)*5 (infix notation) – mul( add(3, 4), 5) • “*”(“+”(3, 4), 5) • (* (+ 3 4) 5) Lecture 9 – Control I, Spring 2017 (Ada, prefix notation) (LISP, prefix notation) CSE 3302 Programming Languages, UT-Arlington 4
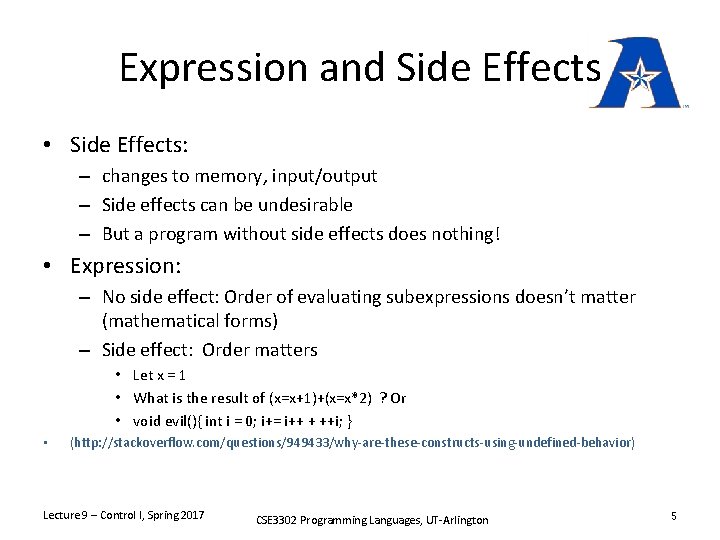
Expression and Side Effects • Side Effects: – changes to memory, input/output – Side effects can be undesirable – But a program without side effects does nothing! • Expression: – No side effect: Order of evaluating subexpressions doesn’t matter (mathematical forms) – Side effect: Order matters • Let x = 1 • What is the result of (x=x+1)+(x=x*2) ? Or • void evil(){ int i = 0; i+= i++ + ++i; } • (http: //stackoverflow. com/questions/949433/why-are-these-constructs-using-undefined-behavior) Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 5
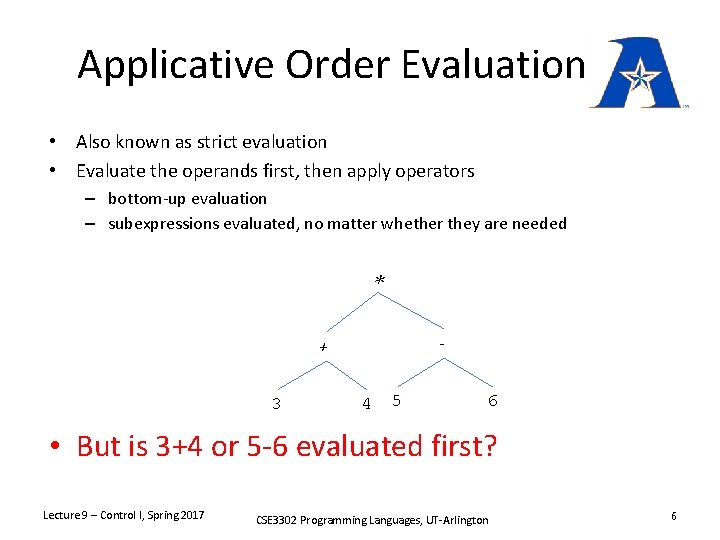
Applicative Order Evaluation • Also known as strict evaluation • Evaluate the operands first, then apply operators – bottom-up evaluation – subexpressions evaluated, no matter whether they are needed * - + 3 4 5 6 • But is 3+4 or 5 -6 evaluated first? Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 6
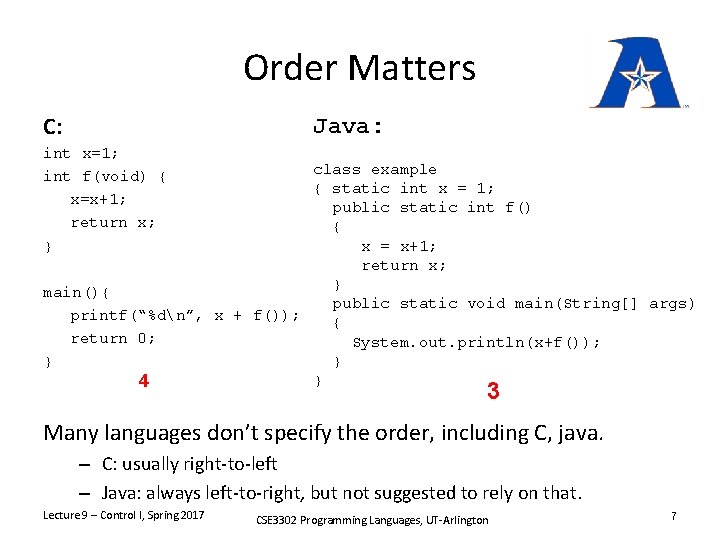
Order Matters C: Java: int x=1; int f(void) { x=x+1; return x; } class example { static int x = 1; public static int f() { x = x+1; return x; } main(){ public static void main(String[] args) printf(“%dn”, x + f()); { return 0; System. out. println(x+f()); } } } 4 3 Many languages don’t specify the order, including C, java. – C: usually right-to-left – Java: always left-to-right, but not suggested to rely on that. Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 7
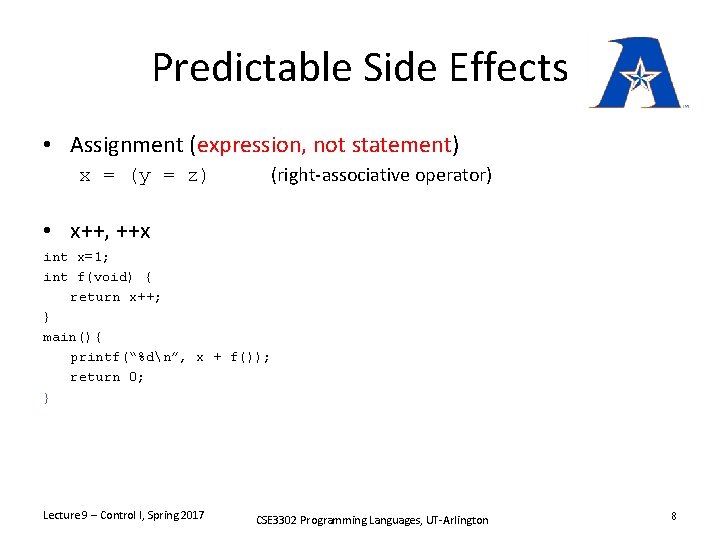
Predictable Side Effects • Assignment (expression, not statement) x = (y = z) (right-associative operator) • x++, ++x int x=1; int f(void) { return x++; } main(){ printf(“%dn”, x + f()); return 0; } Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 8
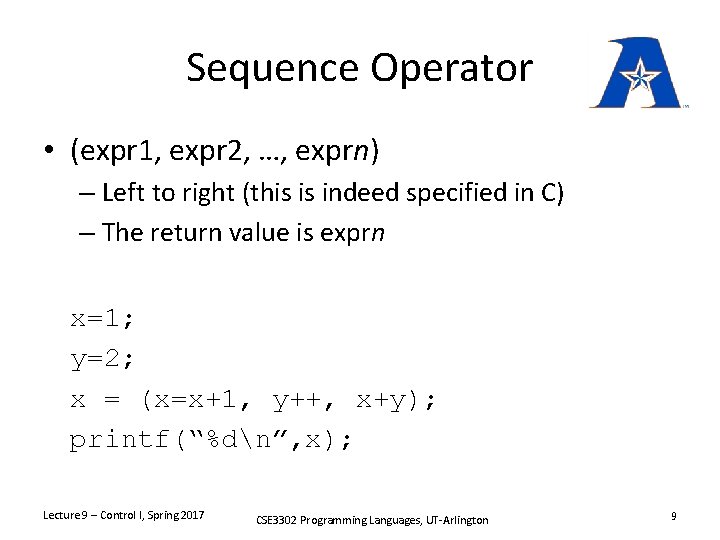
Sequence Operator • (expr 1, expr 2, …, exprn) – Left to right (this is indeed specified in C) – The return value is exprn x=1; y=2; x = (x=x+1, y++, x+y); printf(“%dn”, x); Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 9
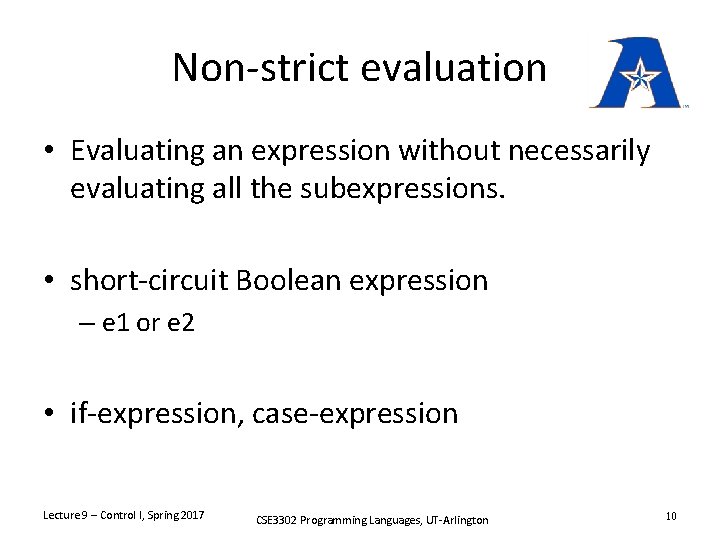
Non-strict evaluation • Evaluating an expression without necessarily evaluating all the subexpressions. • short-circuit Boolean expression – e 1 or e 2 • if-expression, case-expression Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 10
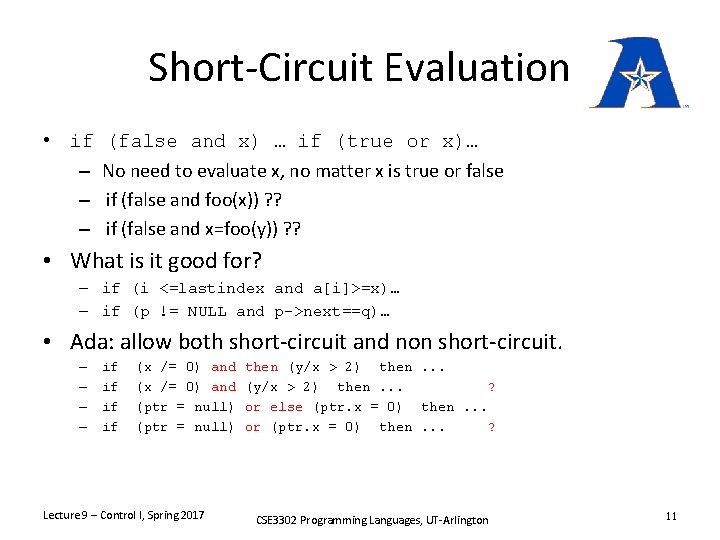
Short-Circuit Evaluation • if – – – (false and x) … if (true or x)… No need to evaluate x, no matter x is true or false if (false and foo(x)) ? ? if (false and x=foo(y)) ? ? • What is it good for? – if (i <=lastindex and a[i]>=x)… – if (p != NULL and p->next==q)… • Ada: allow both short-circuit and non short-circuit. – – if if (x /= 0) and (ptr = null) Lecture 9 – Control I, Spring 2017 then (y/x > 2) then. . . ? or else (ptr. x = 0) then. . . or (ptr. x = 0) then. . . ? CSE 3302 Programming Languages, UT-Arlington 11
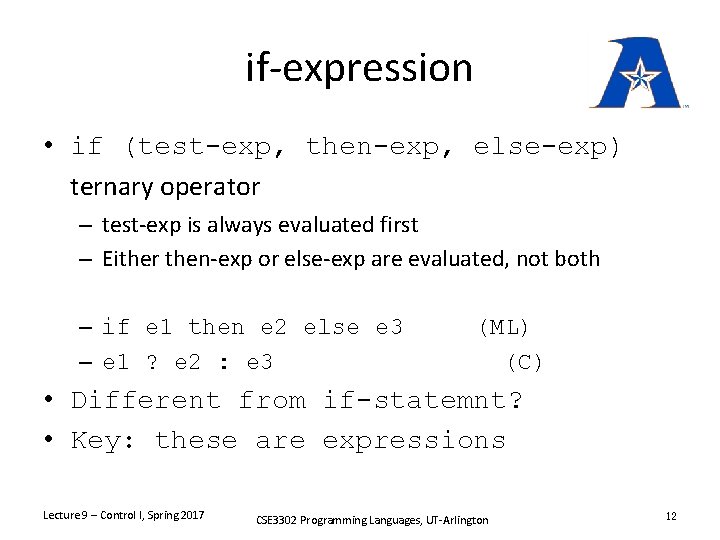
if-expression • if (test-exp, then-exp, else-exp) ternary operator – test-exp is always evaluated first – Either then-exp or else-exp are evaluated, not both – if e 1 then e 2 else e 3 – e 1 ? e 2 : e 3 (ML) (C) • Different from if-statemnt? • Key: these are expressions Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 12
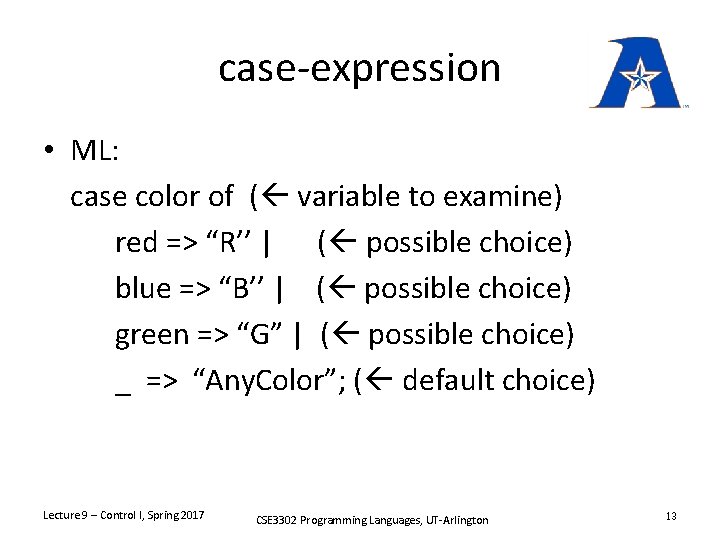
case-expression • ML: case color of ( variable to examine) red => “R’’ | ( possible choice) blue => “B’’ | ( possible choice) green => “G” | ( possible choice) _ => “Any. Color”; ( default choice) Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 13
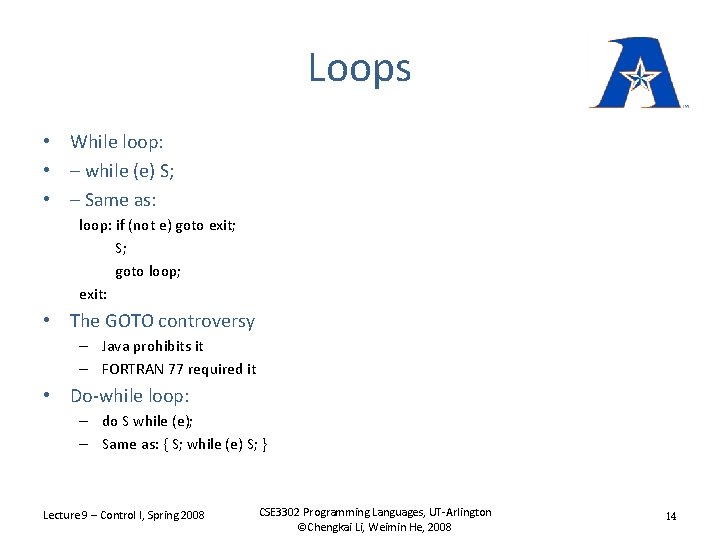
Loops • While loop: • – while (e) S; • – Same as: loop: if (not e) goto exit; S; goto loop; exit: • The GOTO controversy – Java prohibits it – FORTRAN 77 required it • Do-while loop: – do S while (e); – Same as: { S; while (e) S; } Lecture 9 – Control I, Spring 2008 CSE 3302 Programming Languages, UT-Arlington ©Chengkai Li, Weimin He, 2008 14
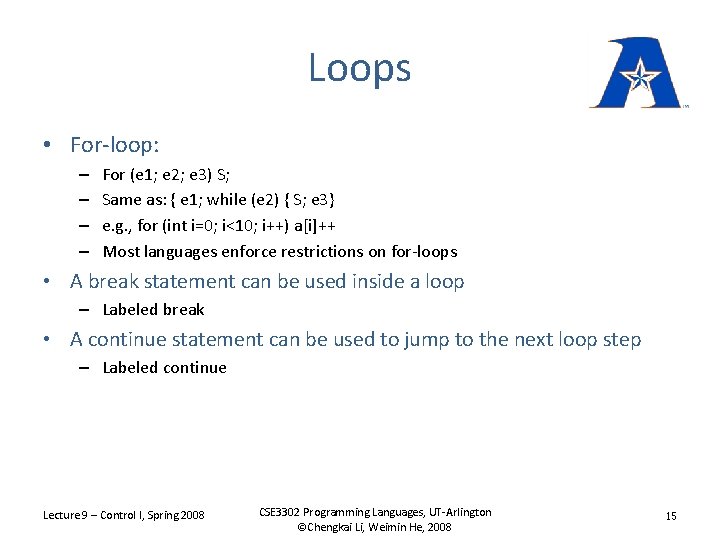
Loops • For-loop: – – For (e 1; e 2; e 3) S; Same as: { e 1; while (e 2) { S; e 3} e. g. , for (int i=0; i<10; i++) a[i]++ Most languages enforce restrictions on for-loops • A break statement can be used inside a loop – Labeled break • A continue statement can be used to jump to the next loop step – Labeled continue Lecture 9 – Control I, Spring 2008 CSE 3302 Programming Languages, UT-Arlington ©Chengkai Li, Weimin He, 2008 15
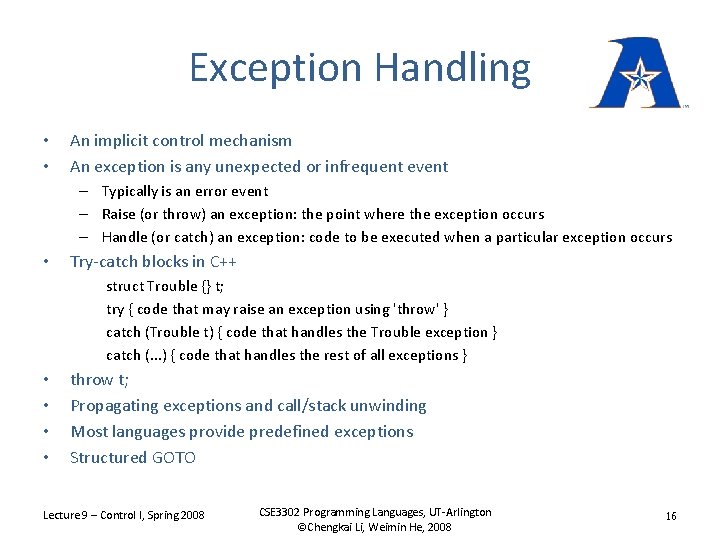
Exception Handling • • An implicit control mechanism An exception is any unexpected or infrequent event – Typically is an error event – Raise (or throw) an exception: the point where the exception occurs – Handle (or catch) an exception: code to be executed when a particular exception occurs • Try-catch blocks in C++ struct Trouble {} t; try { code that may raise an exception using 'throw' } catch (Trouble t) { code that handles the Trouble exception } catch (. . . ) { code that handles the rest of all exceptions } • • throw t; Propagating exceptions and call/stack unwinding Most languages provide predefined exceptions Structured GOTO Lecture 9 – Control I, Spring 2008 CSE 3302 Programming Languages, UT-Arlington ©Chengkai Li, Weimin He, 2008 16
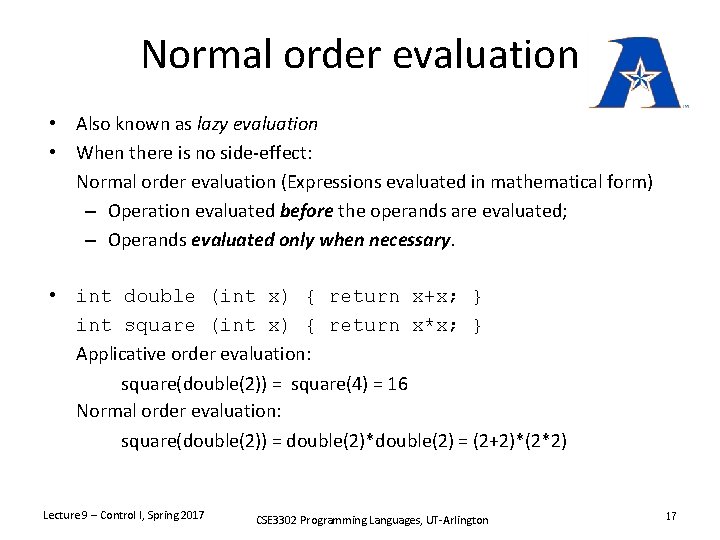
Normal order evaluation • Also known as lazy evaluation • When there is no side-effect: Normal order evaluation (Expressions evaluated in mathematical form) – Operation evaluated before the operands are evaluated; – Operands evaluated only when necessary. • int double (int x) { return x+x; } int square (int x) { return x*x; } Applicative order evaluation: square(double(2)) = square(4) = 16 Normal order evaluation: square(double(2)) = double(2)*double(2) = (2+2)*(2*2) Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 17
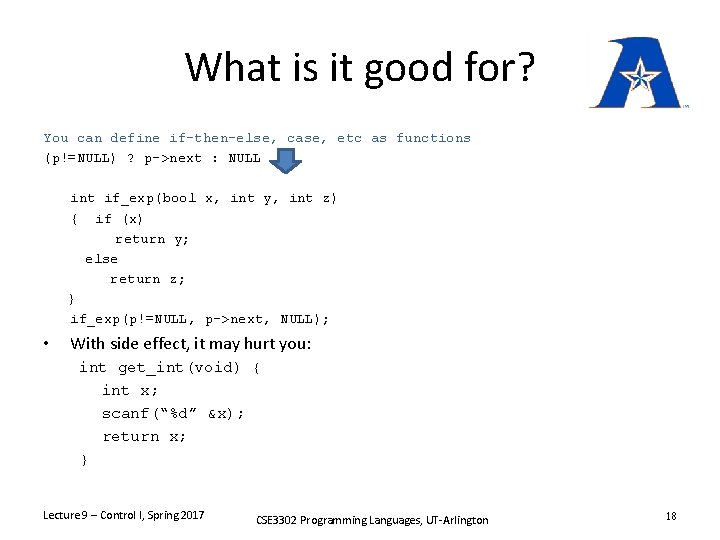
What is it good for? You can define if-then-else, case, etc as functions (p!=NULL) ? p->next : NULL int if_exp(bool x, int y, int z) { if (x) return y; else return z; } if_exp(p!=NULL, p->next, NULL); • With side effect, it may hurt you: int get_int(void) { int x; scanf(“%d” &x); return x; } Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 18
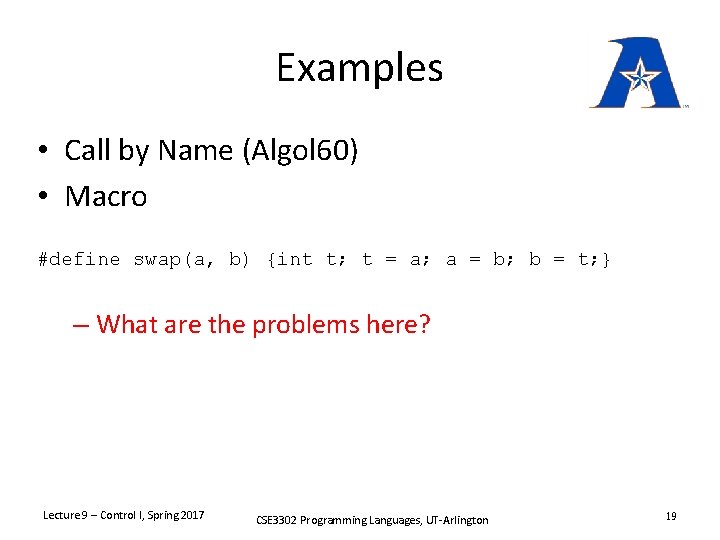
Examples • Call by Name (Algol 60) • Macro #define swap(a, b) {int t; t = a; a = b; b = t; } – What are the problems here? Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 19
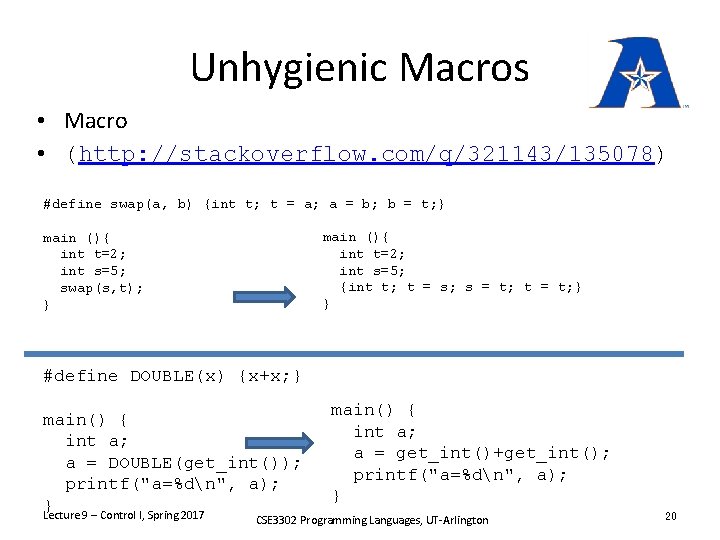
Unhygienic Macros • Macro • (http: //stackoverflow. com/q/321143/135078) #define swap(a, b) {int t; t = a; a = b; b = t; } main (){ int t=2; int s=5; {int t; t = s; s = t; t = t; } } main (){ int t=2; int s=5; swap(s, t); } #define DOUBLE(x) {x+x; } main() { int a; a = DOUBLE(get_int()); printf("a=%dn", a); } Lecture 9 – Control I, Spring 2017 main() { int a; a = get_int()+get_int(); printf("a=%dn", a); } CSE 3302 Programming Languages, UT-Arlington 20
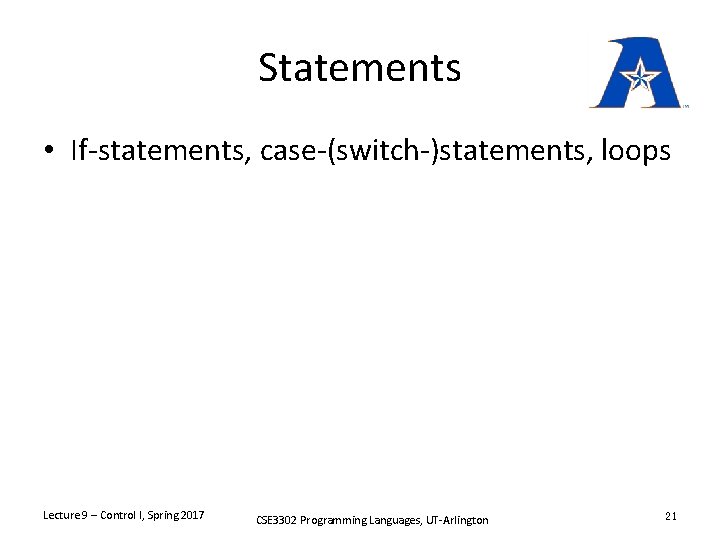
Statements • If-statements, case-(switch-)statements, loops Lecture 9 – Control I, Spring 2017 CSE 3302 Programming Languages, UT-Arlington 21