CSE 326 Linear ADTs Lists Stacks and Queues
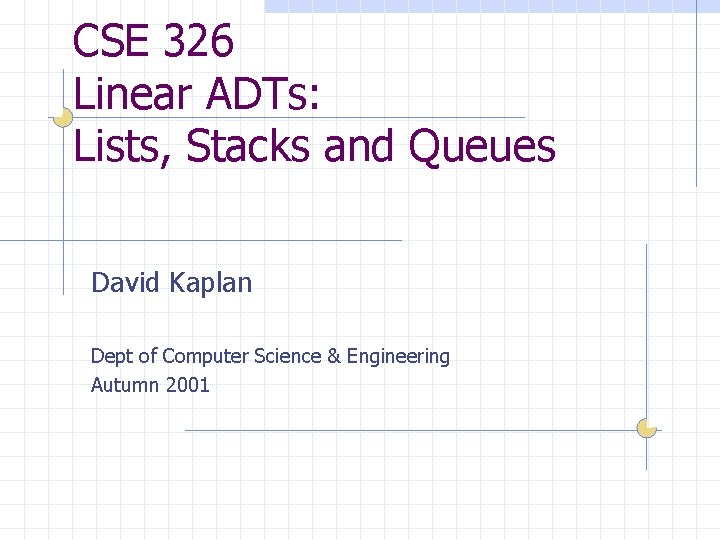
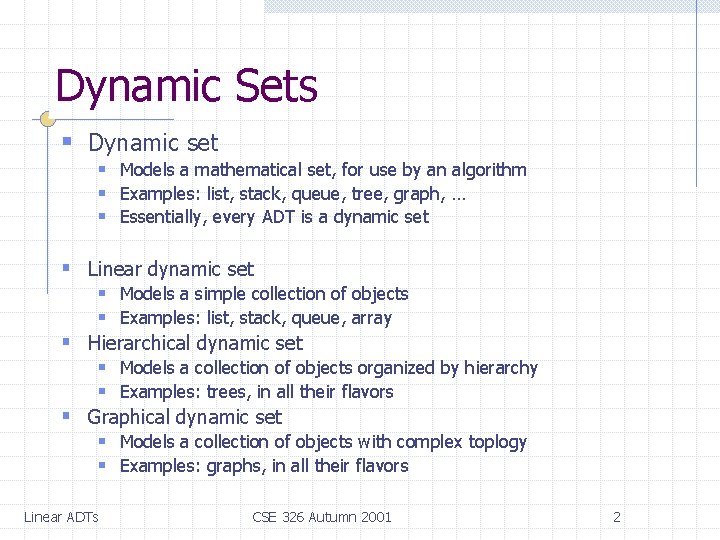
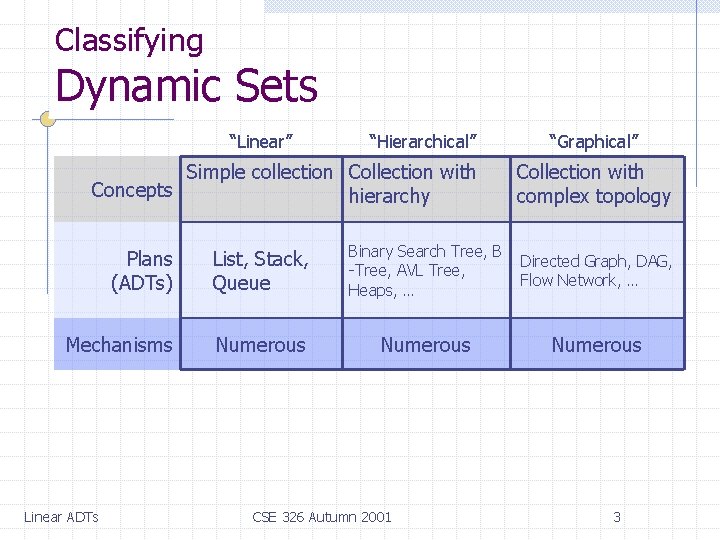
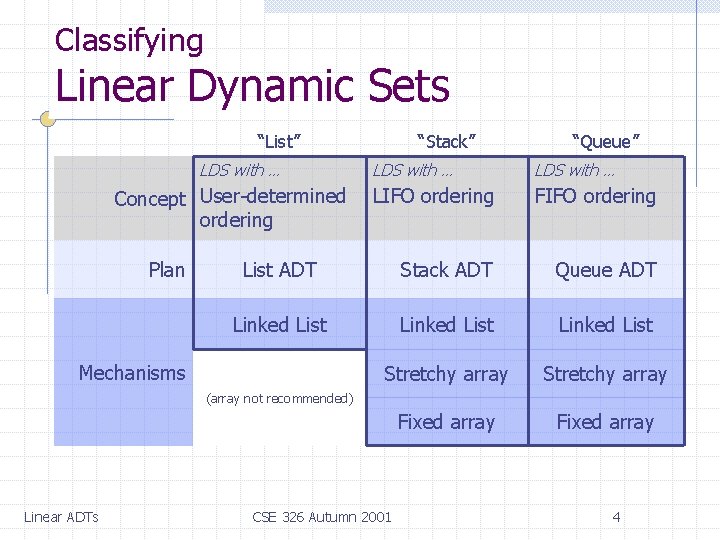
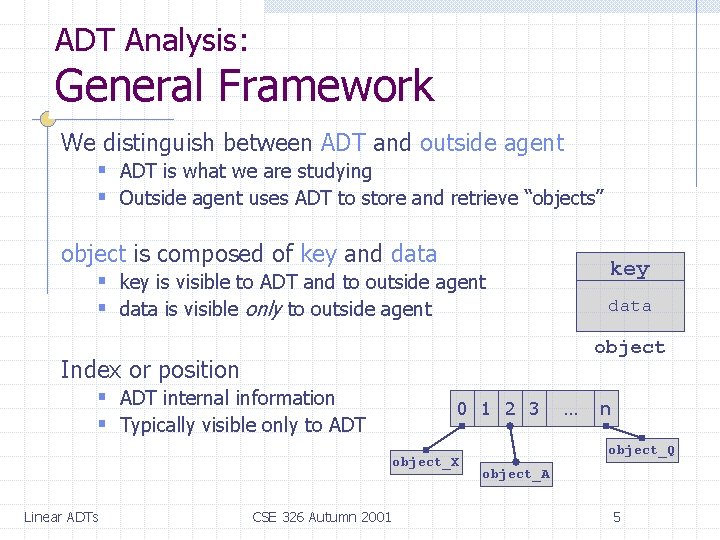
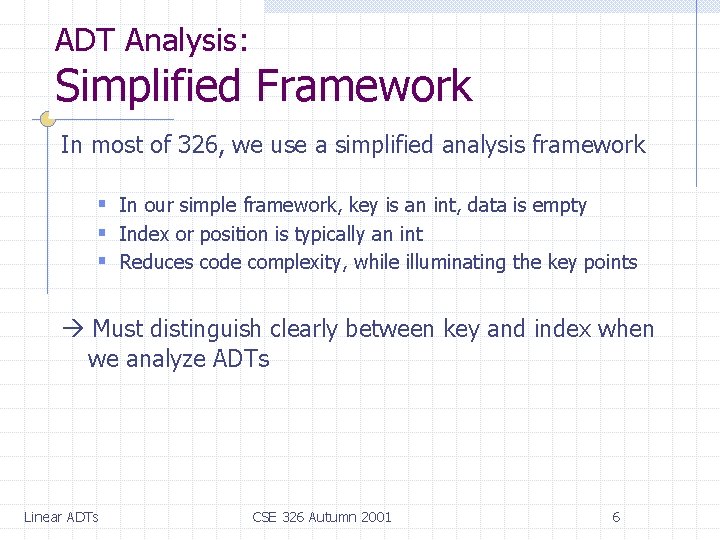
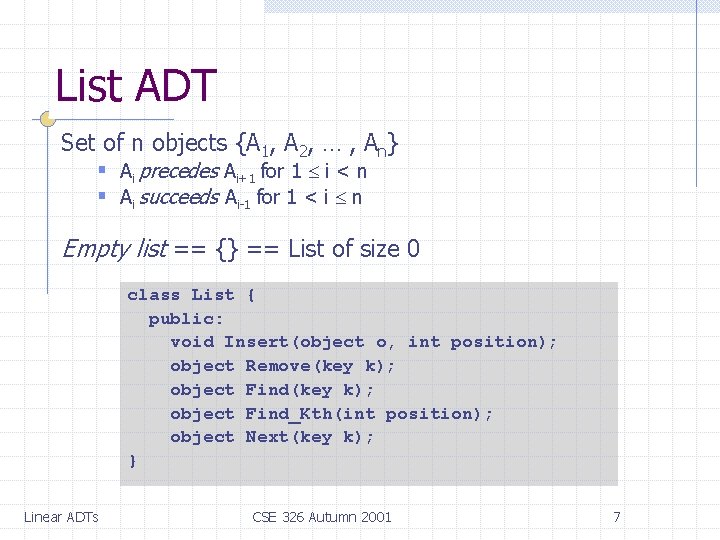
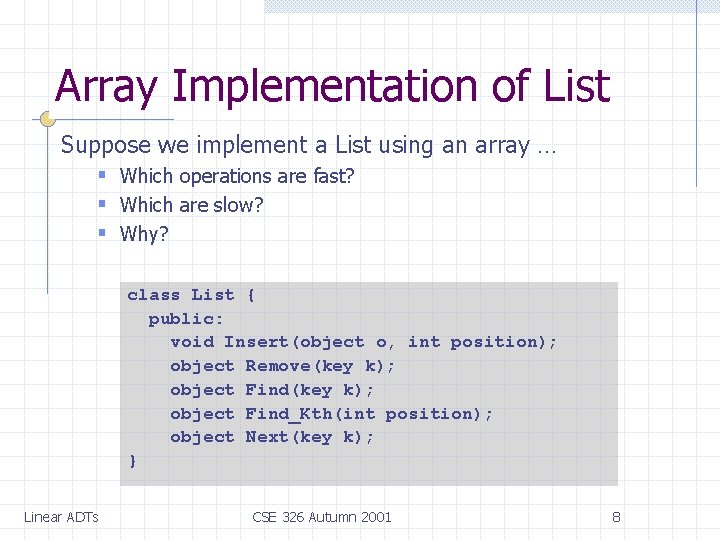
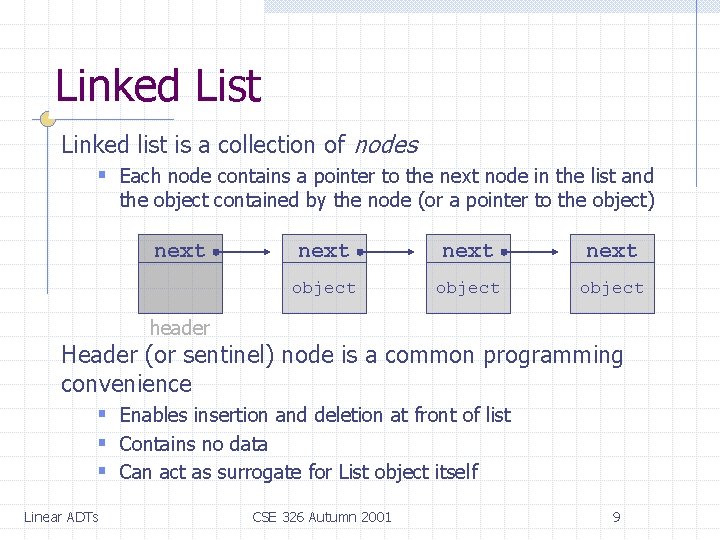
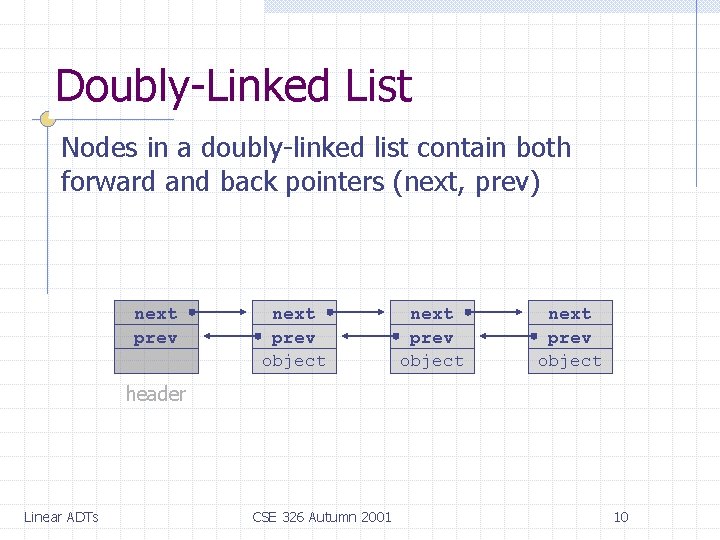
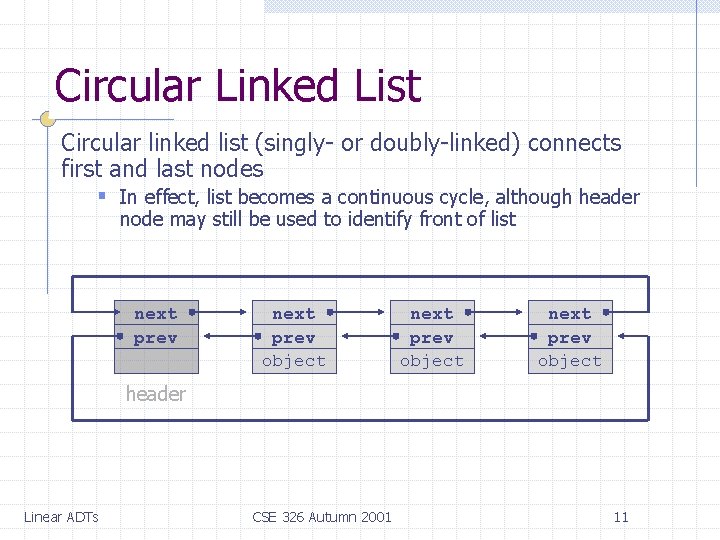
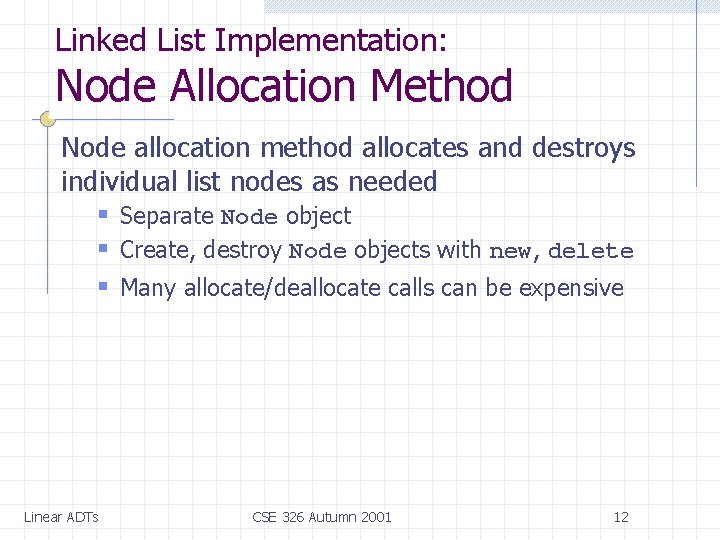
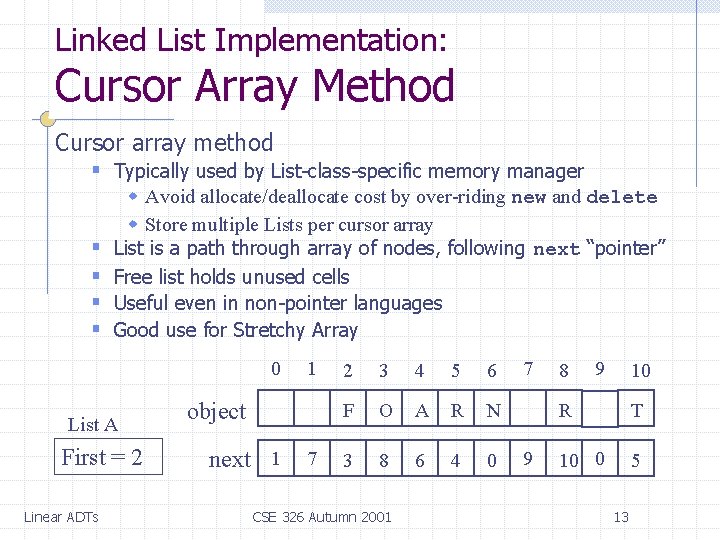
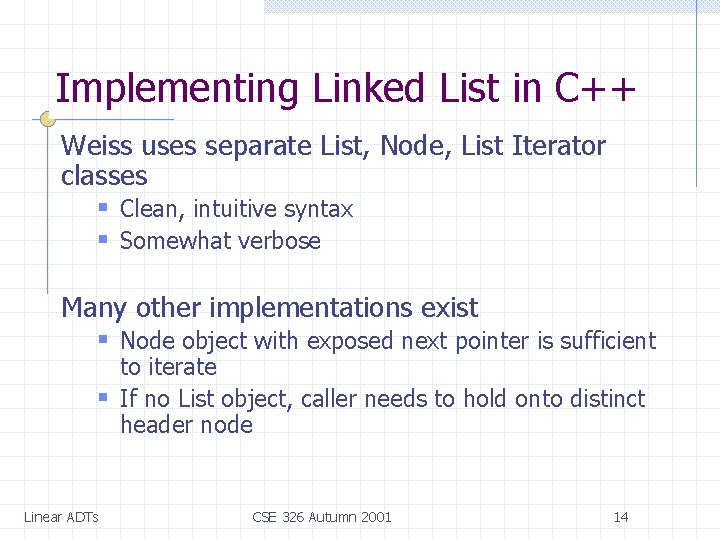
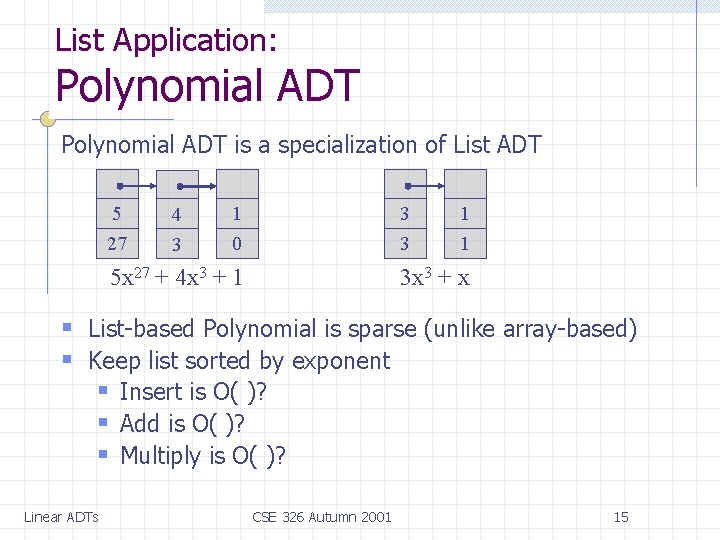
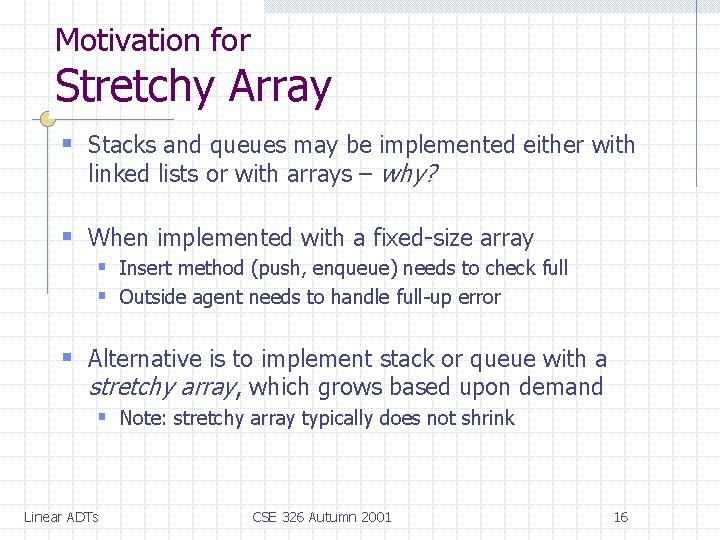
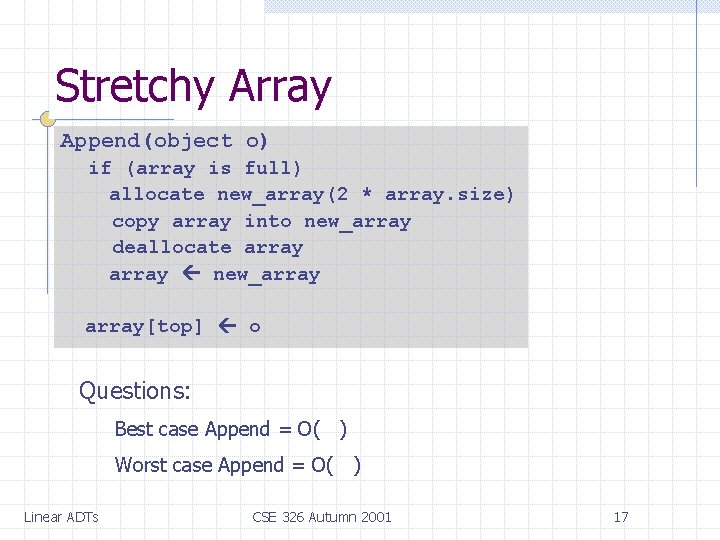
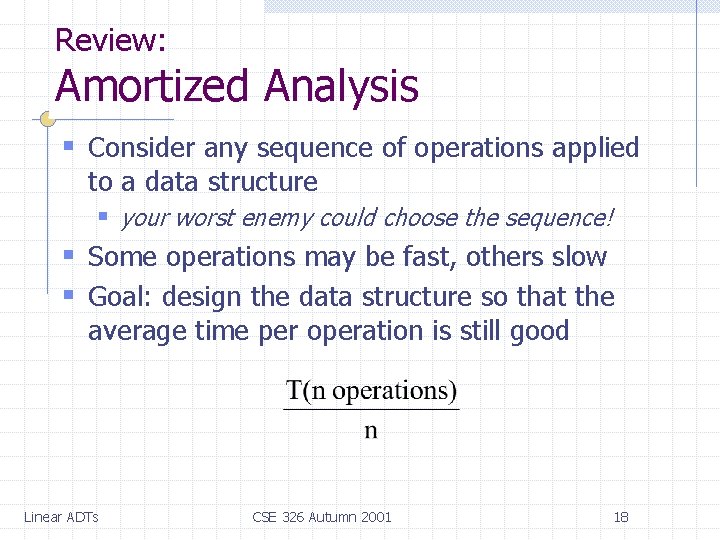
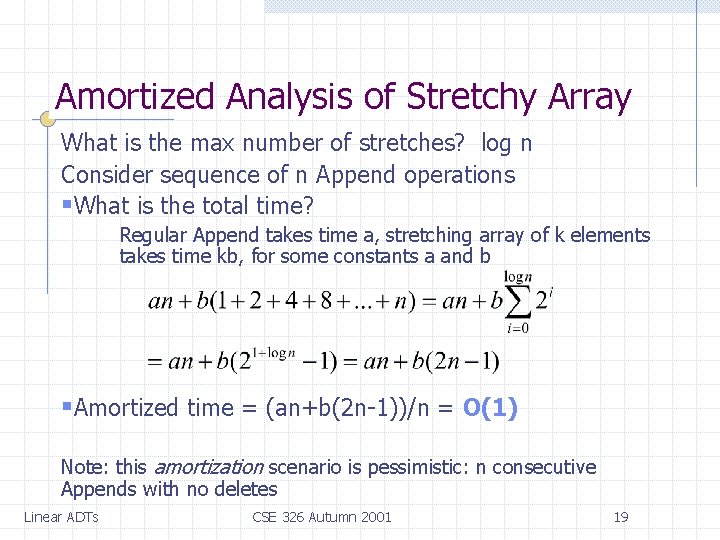
- Slides: 19
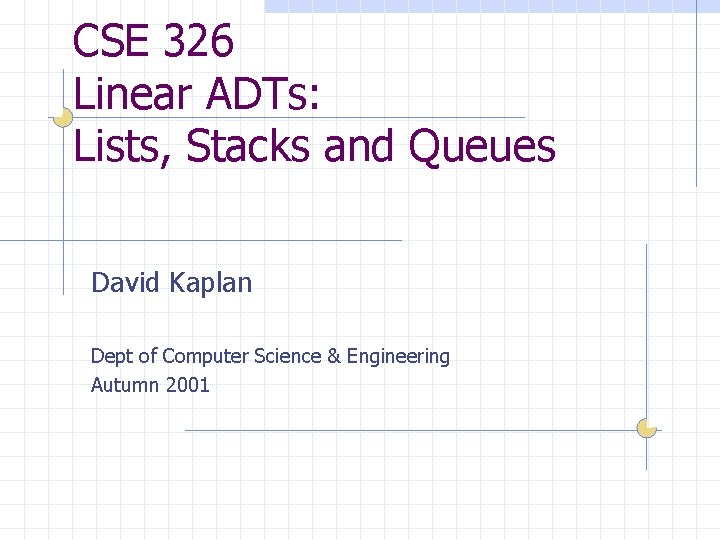
CSE 326 Linear ADTs: Lists, Stacks and Queues David Kaplan Dept of Computer Science & Engineering Autumn 2001
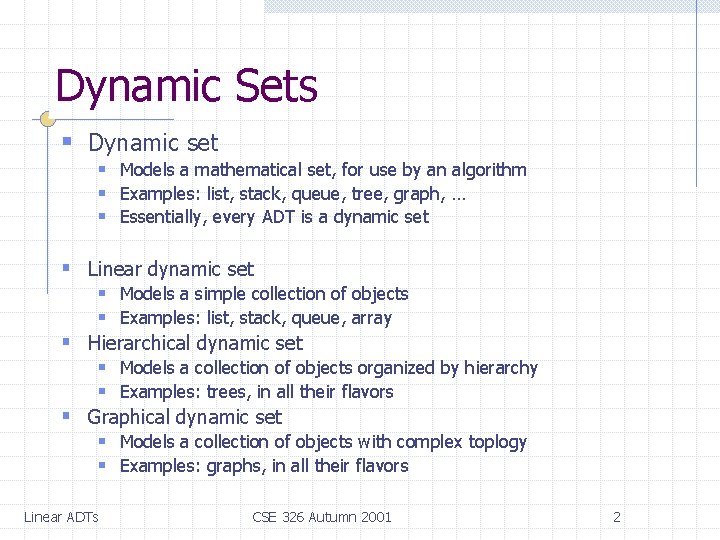
Dynamic Sets § Dynamic set § Models a mathematical set, for use by an algorithm § Examples: list, stack, queue, tree, graph, … § Essentially, every ADT is a dynamic set § Linear dynamic set § Models a simple collection of objects § Examples: list, stack, queue, array § Hierarchical dynamic set § Models a collection of objects organized by hierarchy § Examples: trees, in all their flavors § Graphical dynamic set § Models a collection of objects with complex toplogy § Examples: graphs, in all their flavors Linear ADTs CSE 326 Autumn 2001 2
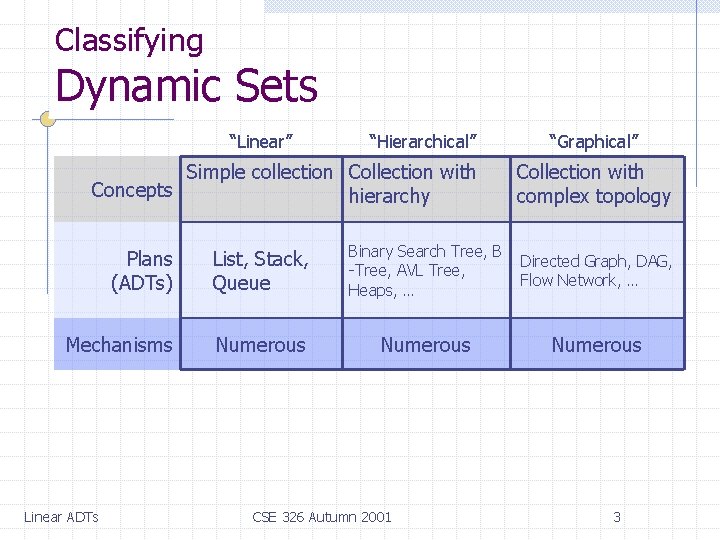
Classifying Dynamic Sets “Linear” “Hierarchical” Simple collection Collection with Concepts hierarchy “Graphical” Collection with complex topology Plans (ADTs) List, Stack, Queue Binary Search Tree, B -Tree, AVL Tree, Heaps, … Directed Graph, DAG, Flow Network, … Mechanisms Numerous Linear ADTs CSE 326 Autumn 2001 3
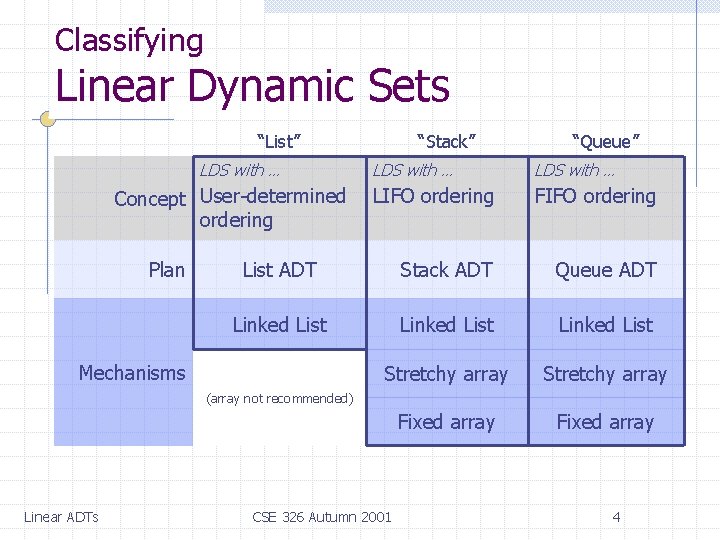
Classifying Linear Dynamic Sets “List” LDS with … “Queue” LDS with … LIFO ordering FIFO ordering List ADT Stack ADT Queue ADT Linked List Stretchy array Fixed array Concept User-determined ordering Plan “Stack” Mechanisms (array not recommended) Linear ADTs CSE 326 Autumn 2001 4
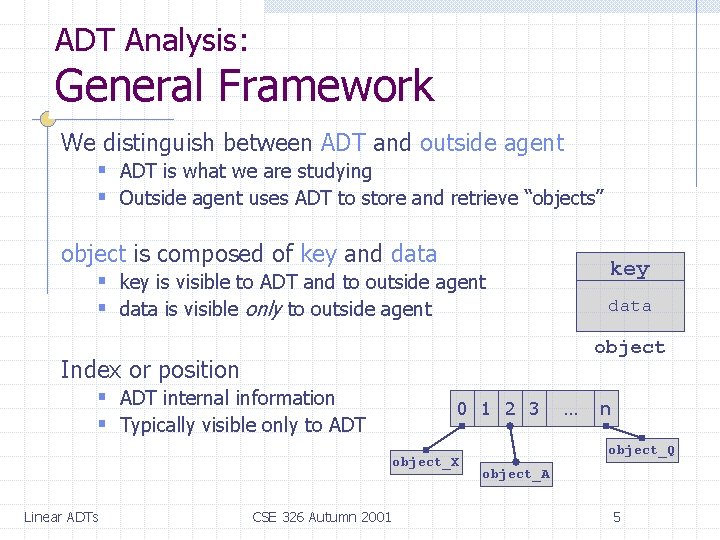
ADT Analysis: General Framework We distinguish between ADT and outside agent § ADT is what we are studying § Outside agent uses ADT to store and retrieve “objects” object is composed of key and data key § key is visible to ADT and to outside agent § data is visible only to outside agent data object Index or position § ADT internal information § Typically visible only to ADT 0 1 2 3 object_X Linear ADTs CSE 326 Autumn 2001 … n object_Q object_A 5
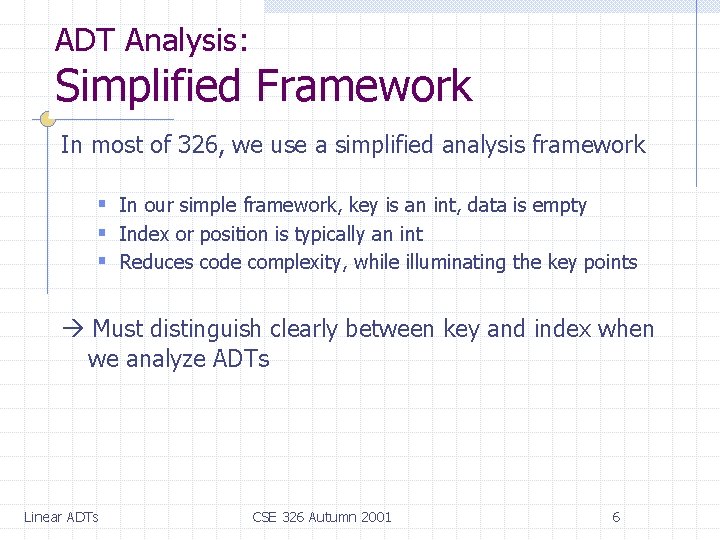
ADT Analysis: Simplified Framework In most of 326, we use a simplified analysis framework § In our simple framework, key is an int, data is empty § Index or position is typically an int § Reduces code complexity, while illuminating the key points Must distinguish clearly between key and index when we analyze ADTs Linear ADTs CSE 326 Autumn 2001 6
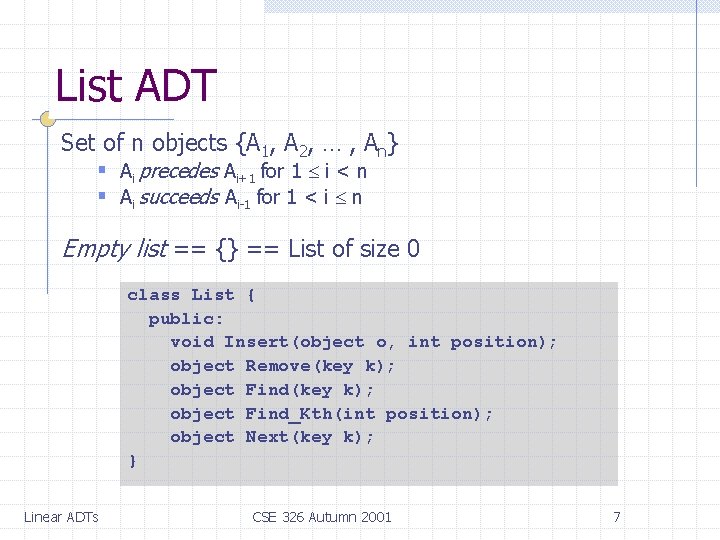
List ADT Set of n objects {A 1, A 2, … , An} § Ai precedes Ai+1 for 1 i < n § Ai succeeds Ai-1 for 1 < i n Empty list == {} == List of size 0 class List { public: void Insert(object o, int position); object Remove(key k); object Find_Kth(int position); object Next(key k); } Linear ADTs CSE 326 Autumn 2001 7
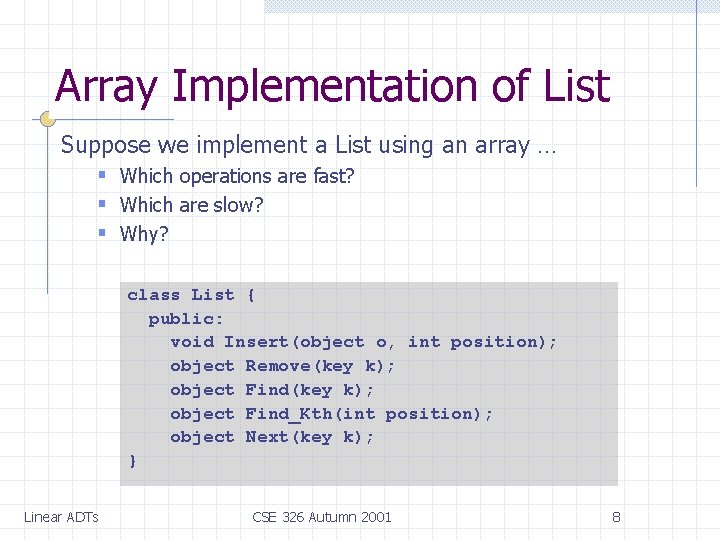
Array Implementation of List Suppose we implement a List using an array … § Which operations are fast? § Which are slow? § Why? class List { public: void Insert(object o, int position); object Remove(key k); object Find_Kth(int position); object Next(key k); } Linear ADTs CSE 326 Autumn 2001 8
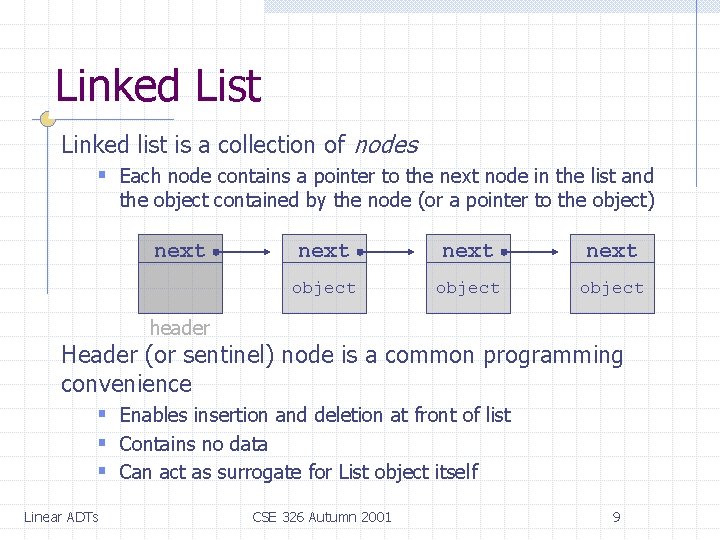
Linked List Linked list is a collection of nodes § Each node contains a pointer to the next node in the list and the object contained by the node (or a pointer to the object) next object header Header (or sentinel) node is a common programming convenience § Enables insertion and deletion at front of list § Contains no data § Can act as surrogate for List object itself Linear ADTs CSE 326 Autumn 2001 9
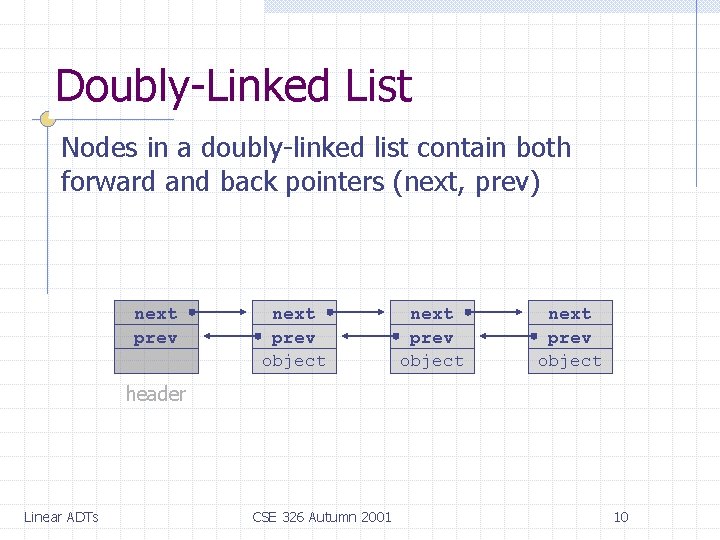
Doubly-Linked List Nodes in a doubly-linked list contain both forward and back pointers (next, prev) next prev object header Linear ADTs CSE 326 Autumn 2001 10
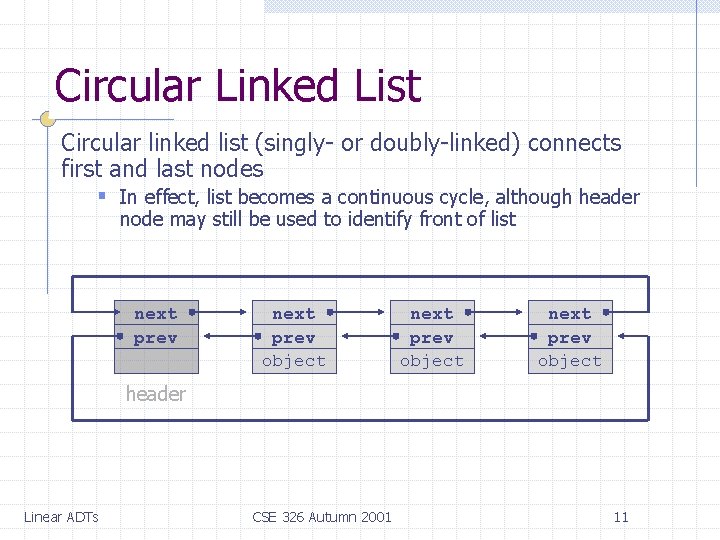
Circular Linked List Circular linked list (singly- or doubly-linked) connects first and last nodes § In effect, list becomes a continuous cycle, although header node may still be used to identify front of list next prev object header Linear ADTs CSE 326 Autumn 2001 11
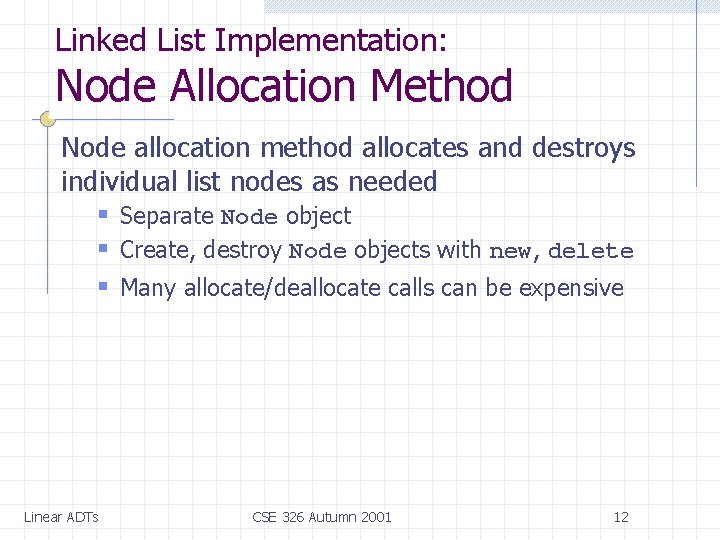
Linked List Implementation: Node Allocation Method Node allocation method allocates and destroys individual list nodes as needed § Separate Node object § Create, destroy Node objects with new, delete § Many allocate/deallocate calls can be expensive Linear ADTs CSE 326 Autumn 2001 12
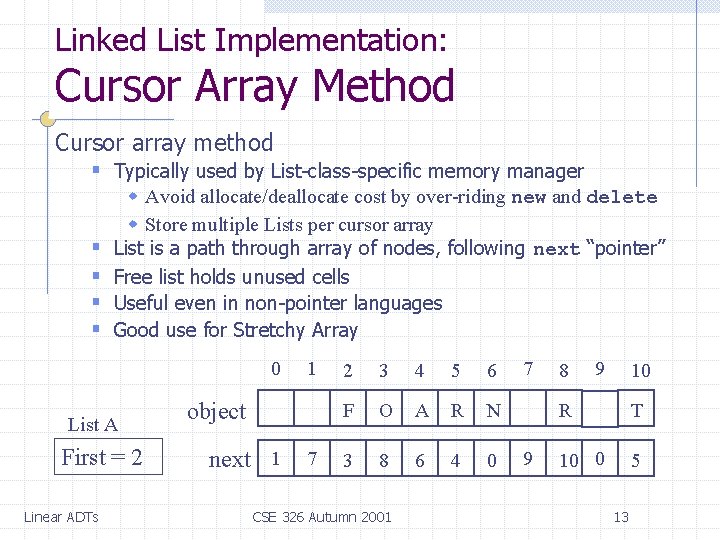
Linked List Implementation: Cursor Array Method Cursor array method § Typically used by List-class-specific memory manager § § w Avoid allocate/deallocate cost by over-riding new and delete w Store multiple Lists per cursor array List is a path through array of nodes, following next “pointer” Free list holds unused cells Useful even in non-pointer languages Good use for Stretchy Array 0 List A First = 2 Linear ADTs 1 object next 1 7 2 3 4 5 6 F O A R N 3 8 6 4 0 CSE 326 Autumn 2001 7 9 8 9 10 R T 10 0 5 13
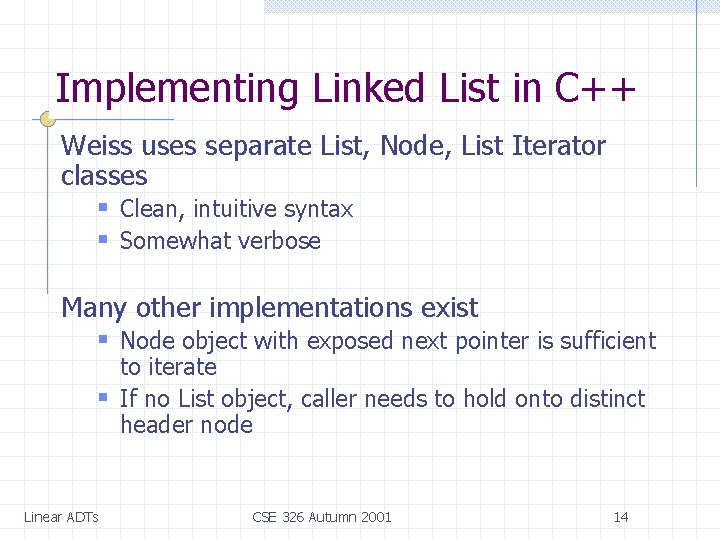
Implementing Linked List in C++ Weiss uses separate List, Node, List Iterator classes § Clean, intuitive syntax § Somewhat verbose Many other implementations exist § Node object with exposed next pointer is sufficient to iterate § If no List object, caller needs to hold onto distinct header node Linear ADTs CSE 326 Autumn 2001 14
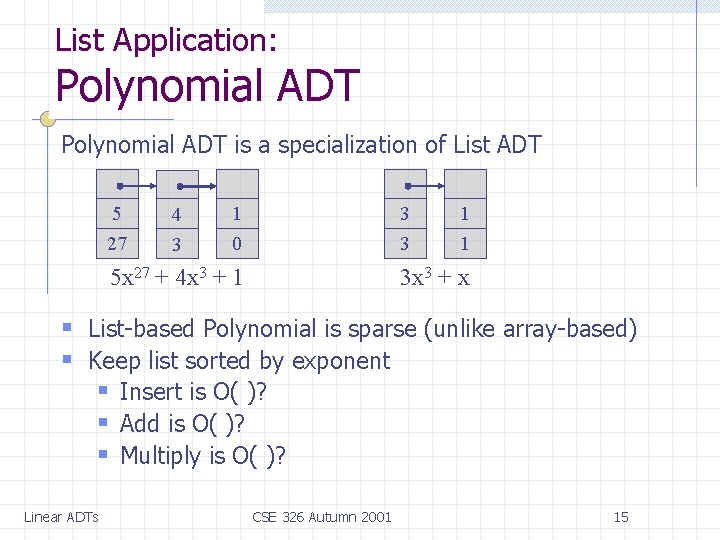
List Application: Polynomial ADT is a specialization of List ADT 5 27 4 3 1 0 3 3 5 x 27 + 4 x 3 + 1 1 1 3 x 3 + x § List-based Polynomial is sparse (unlike array-based) § Keep list sorted by exponent § Insert is O( )? § Add is O( )? § Multiply is O( )? Linear ADTs CSE 326 Autumn 2001 15
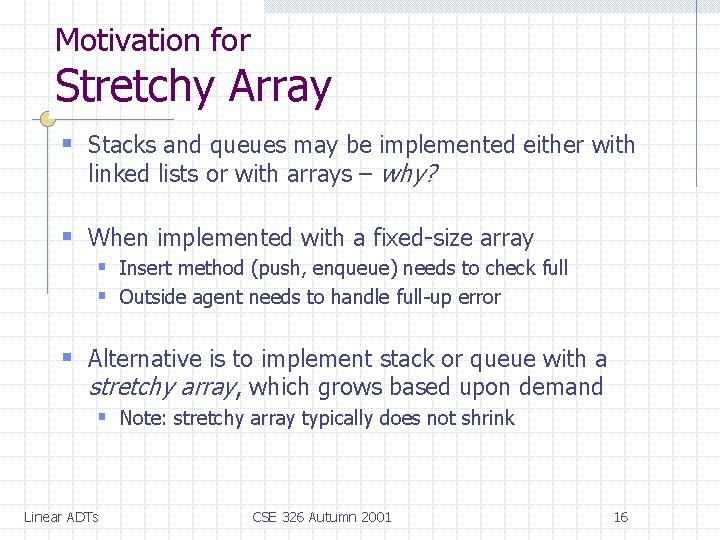
Motivation for Stretchy Array § Stacks and queues may be implemented either with linked lists or with arrays – why? § When implemented with a fixed-size array § Insert method (push, enqueue) needs to check full § Outside agent needs to handle full-up error § Alternative is to implement stack or queue with a stretchy array, which grows based upon demand § Note: stretchy array typically does not shrink Linear ADTs CSE 326 Autumn 2001 16
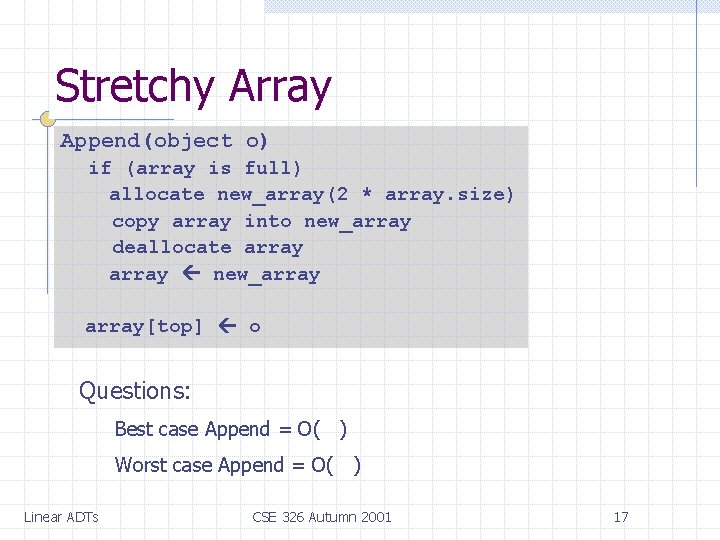
Stretchy Array Append(object o) if (array is full) allocate new_array(2 * array. size) copy array into new_array deallocate array new_array[top] o Questions: Best case Append = O( ) Worst case Append = O( ) Linear ADTs CSE 326 Autumn 2001 17
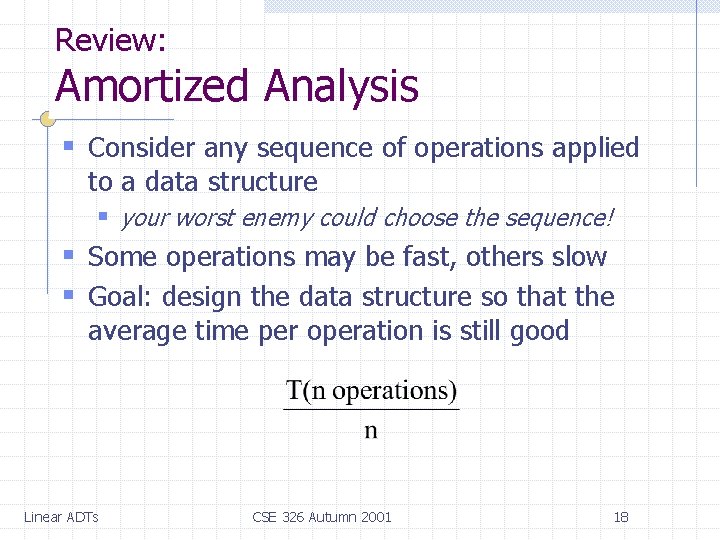
Review: Amortized Analysis § Consider any sequence of operations applied to a data structure § your worst enemy could choose the sequence! § Some operations may be fast, others slow § Goal: design the data structure so that the average time per operation is still good Linear ADTs CSE 326 Autumn 2001 18
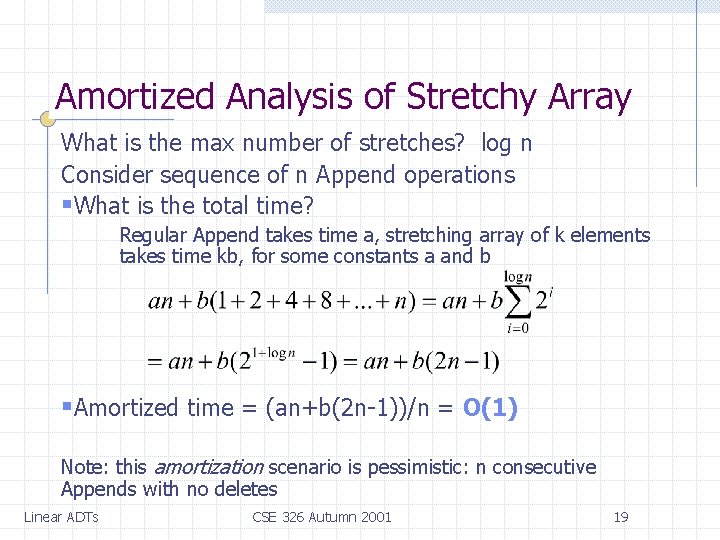
Amortized Analysis of Stretchy Array What is the max number of stretches? log n Consider sequence of n Append operations §What is the total time? Regular Append takes time a, stretching array of k elements takes time kb, for some constants a and b §Amortized time = (an+b(2 n-1))/n = O(1) Note: this amortization scenario is pessimistic: n consecutive Appends with no deletes Linear ADTs CSE 326 Autumn 2001 19
Python stack and queue
Java stacks and queues
Java stack exercises
What are stacks
Cse 326
Cse 326
Cse 326
Adts, data structures, and problem solving with c++
Parameterized abstract data types
Parameterized adts is also known as
Adts ukiah
Coastal features diagram
Queue quiz
Representation of queues
Message queue in unix
Adaptable priority queue
Rtos mailbox
Applications of priority queues
Operasi yang tidak ada pada antrian (queue) adalah
Queue definition