CSE 143 More Collection ADTs Sections 4 6
![CSE 143 More Collection ADTs [Sections 4. 6 -4. 8] 3/30/98 131 CSE 143 More Collection ADTs [Sections 4. 6 -4. 8] 3/30/98 131](https://slidetodoc.com/presentation_image/de75fc91b40923f25843aad80491e77e/image-1.jpg)
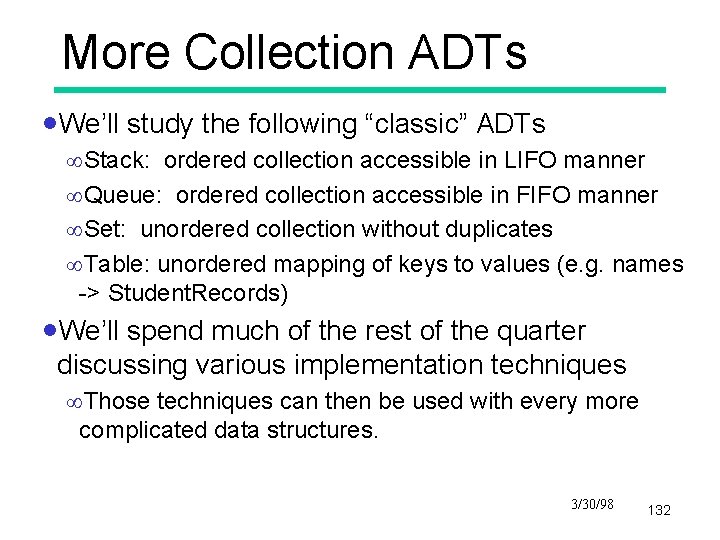
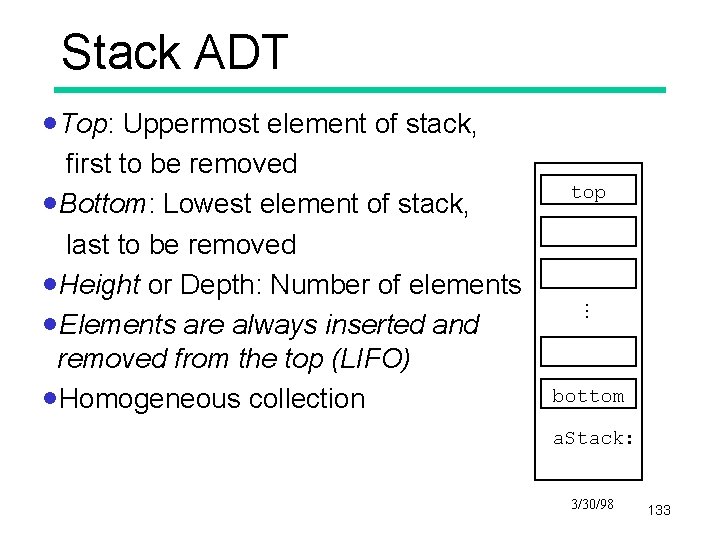
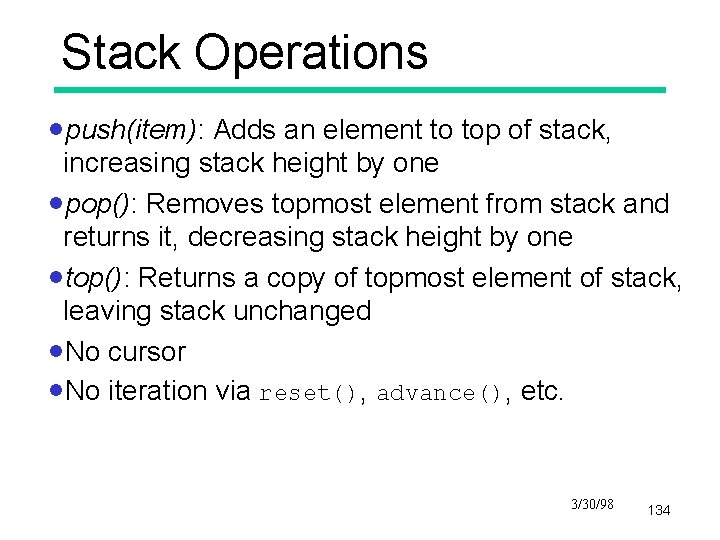
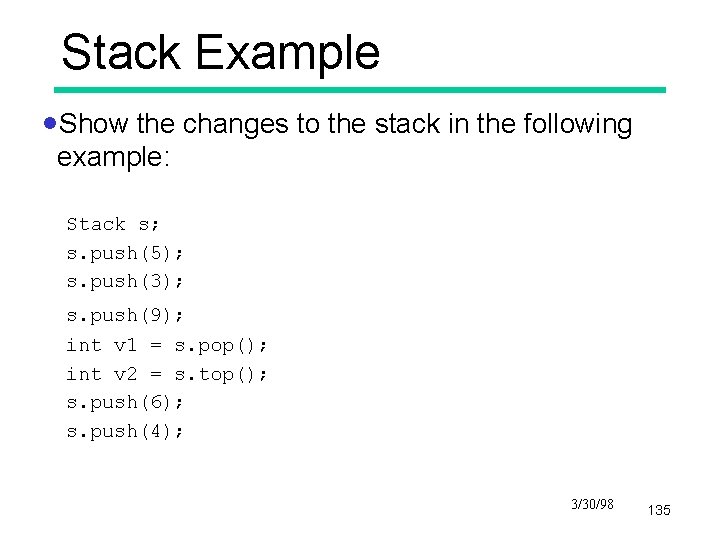
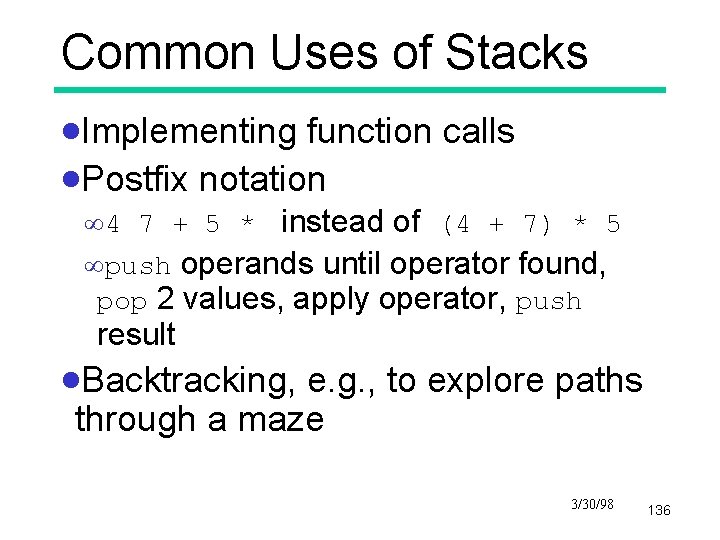
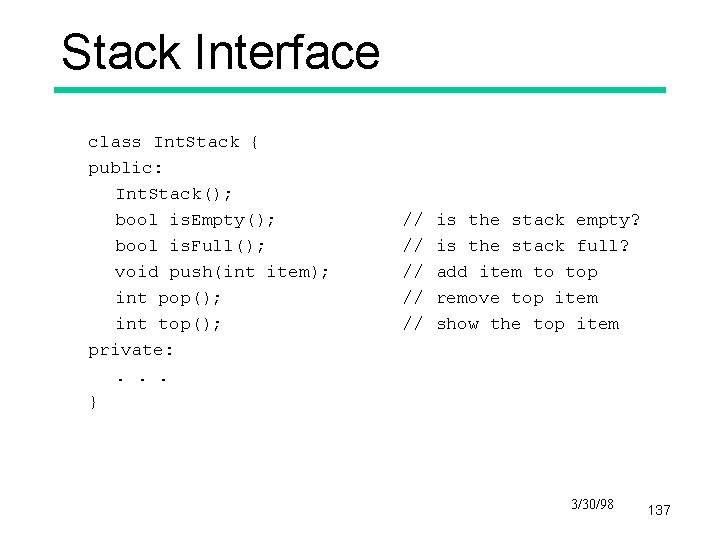
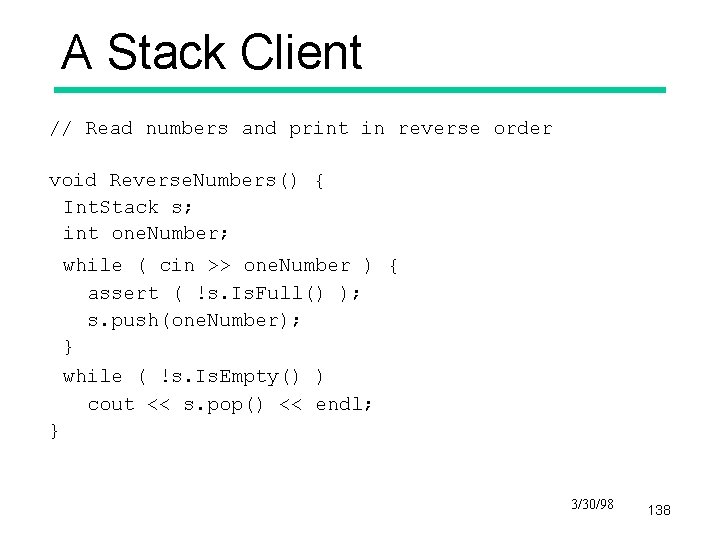
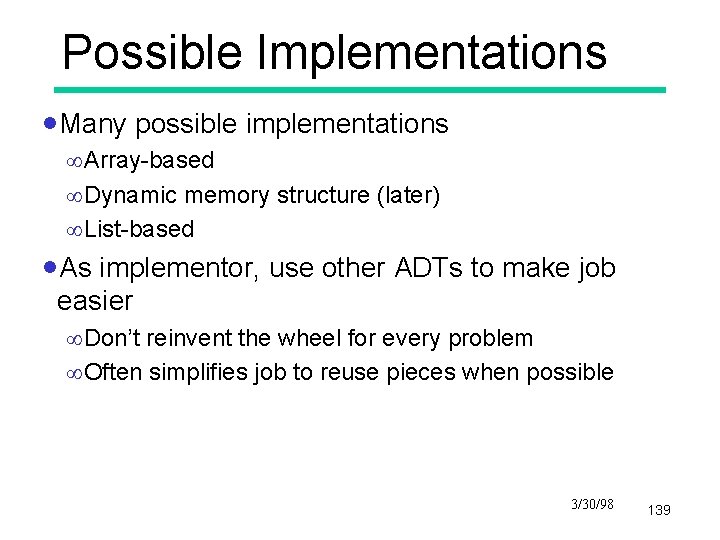
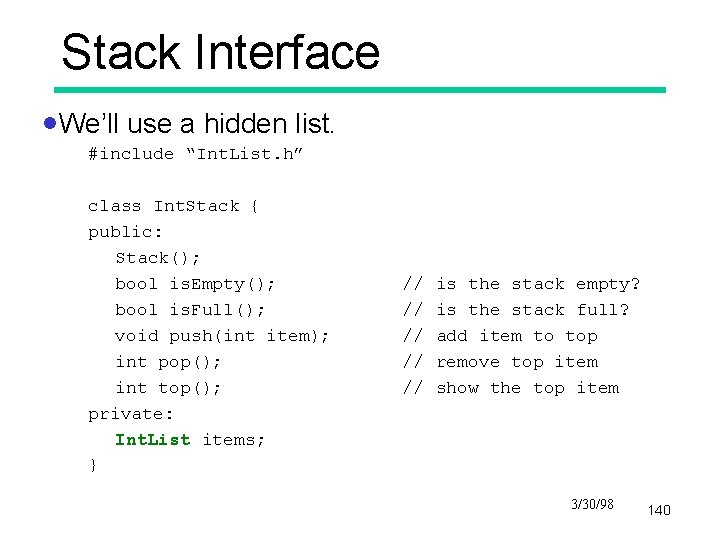
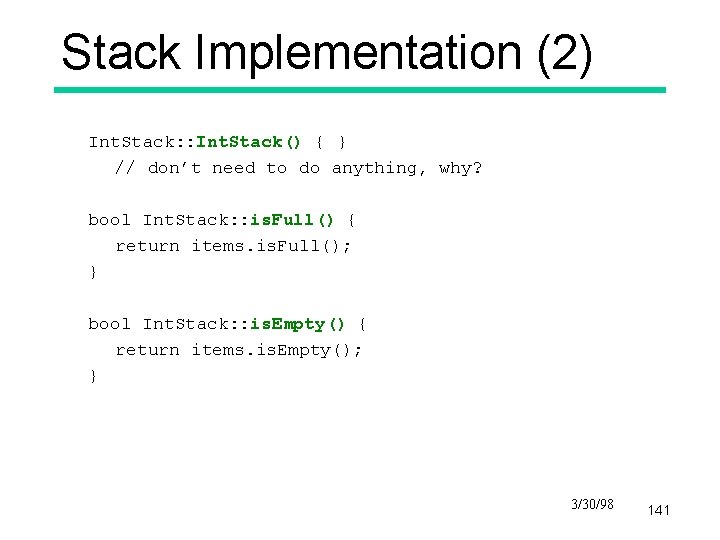
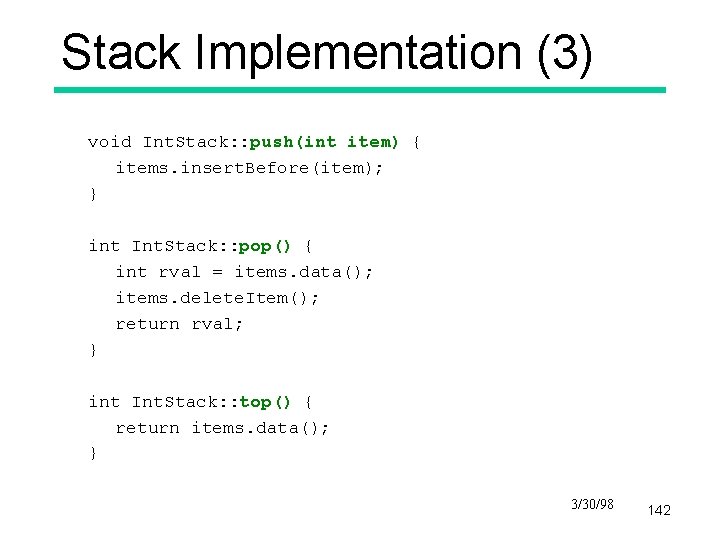
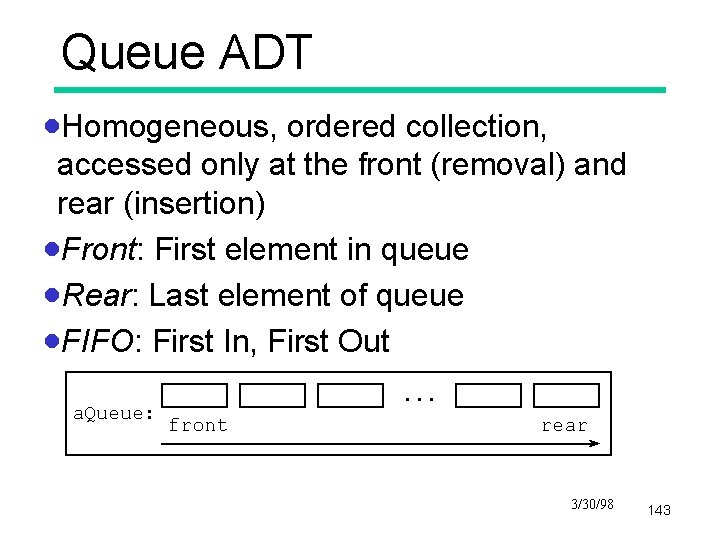
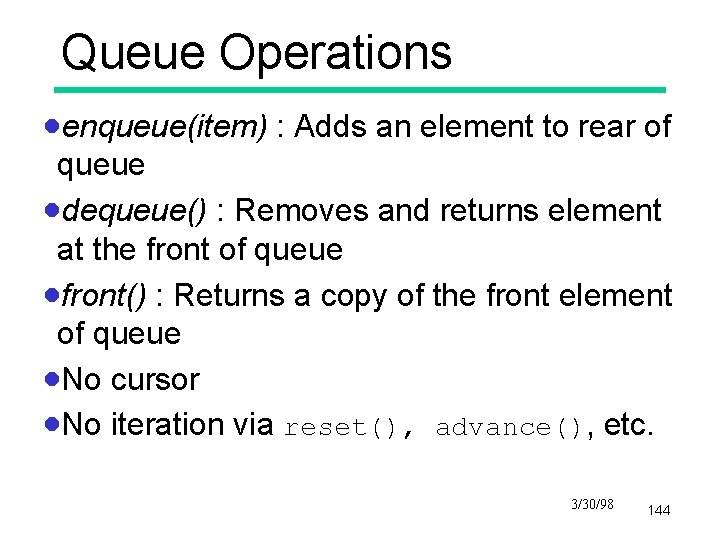
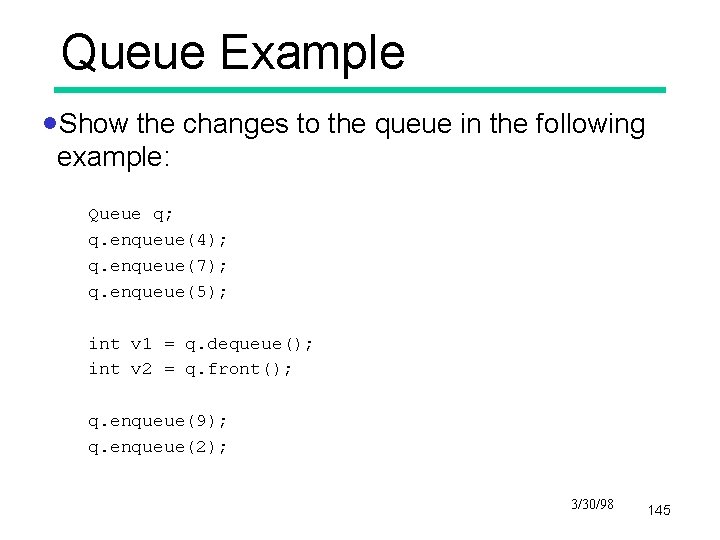
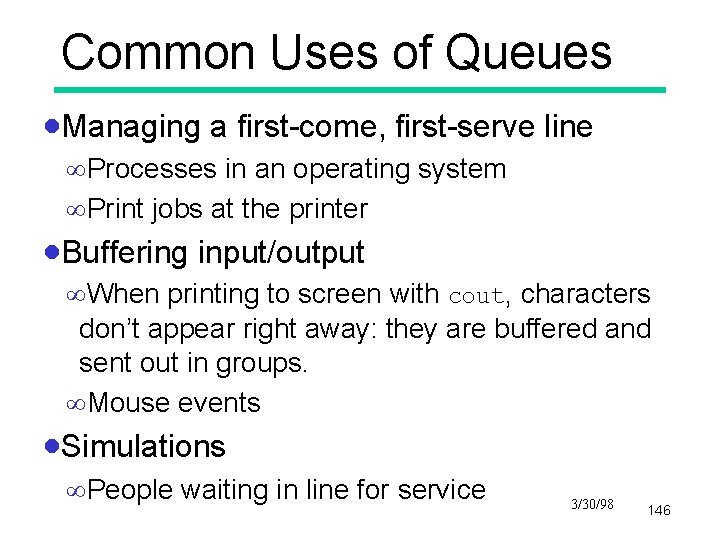
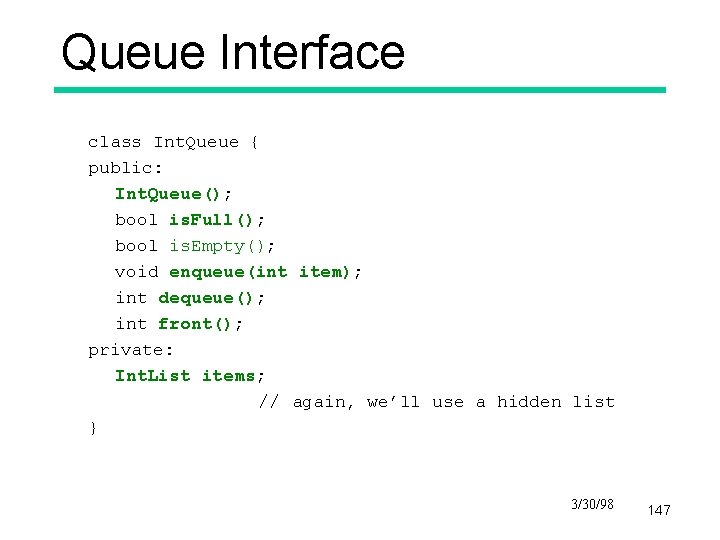
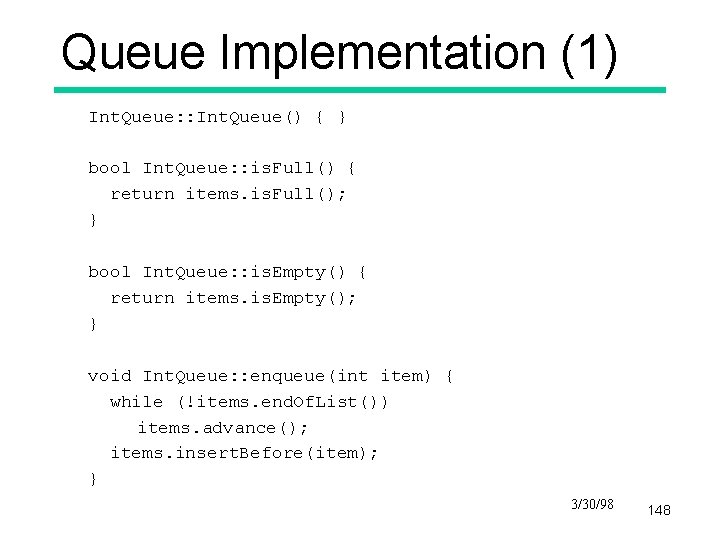
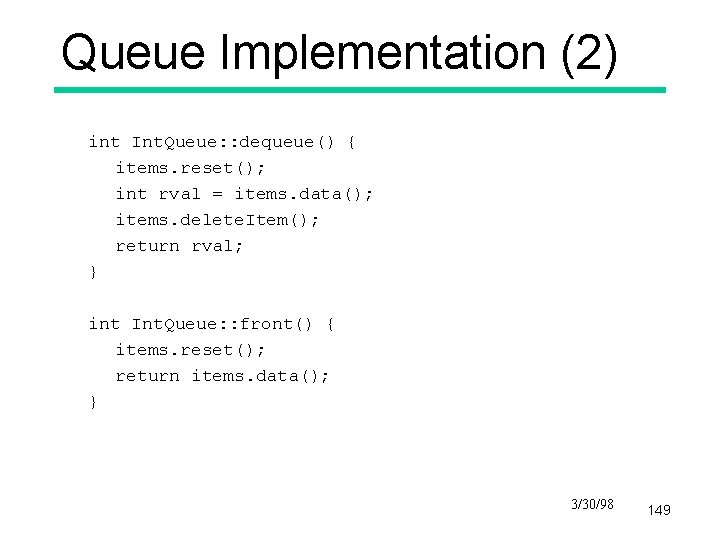
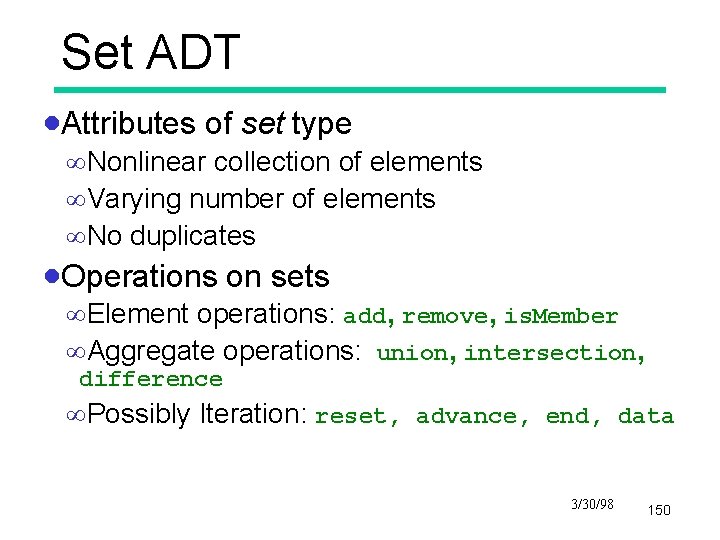
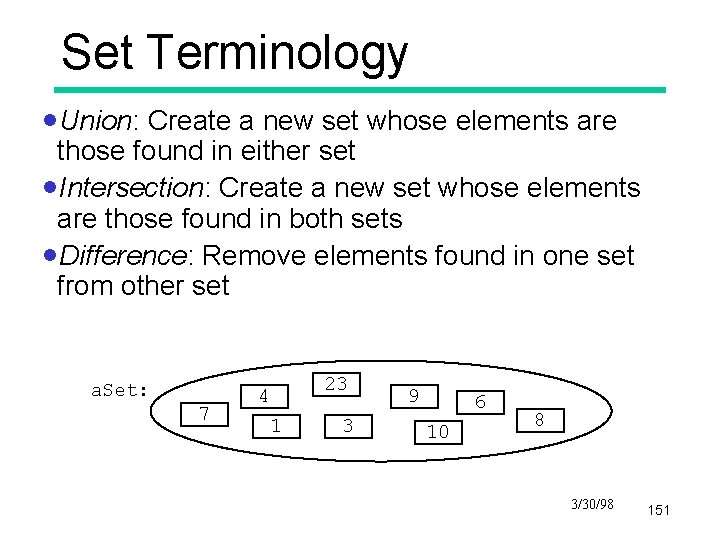
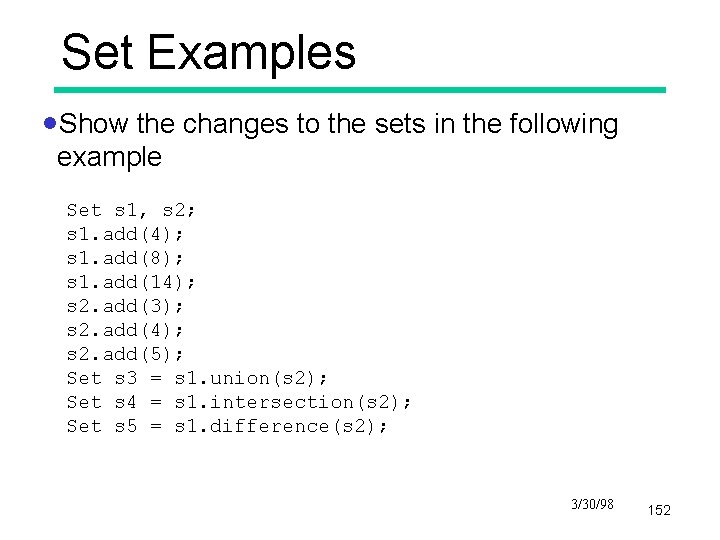
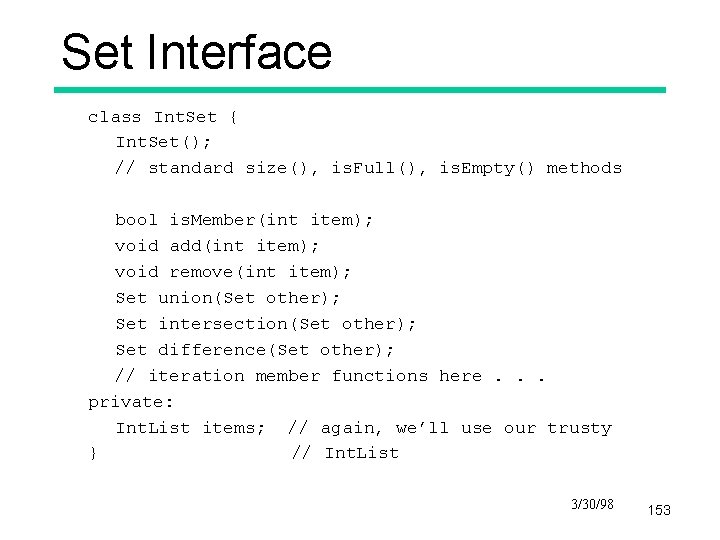
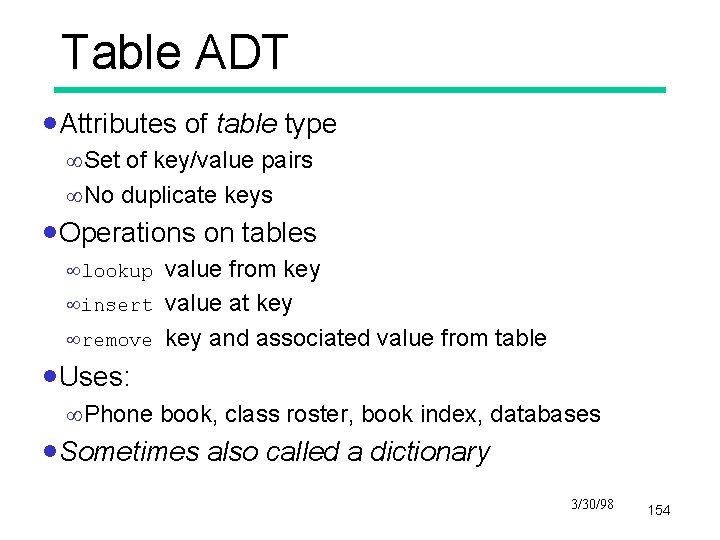
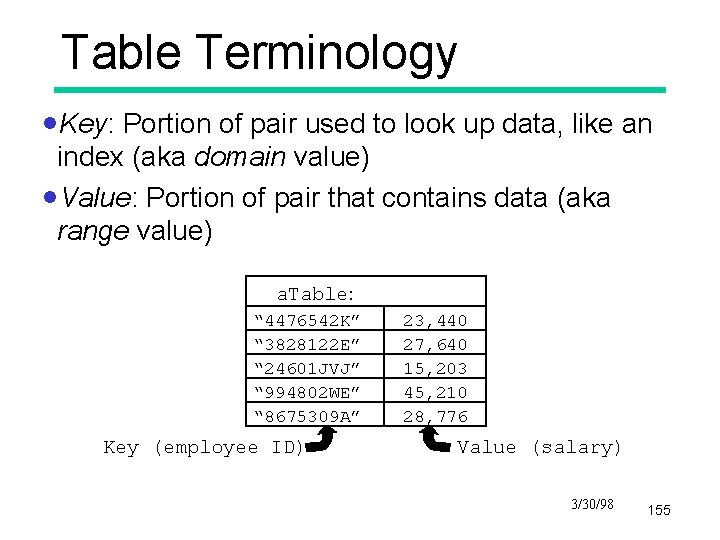
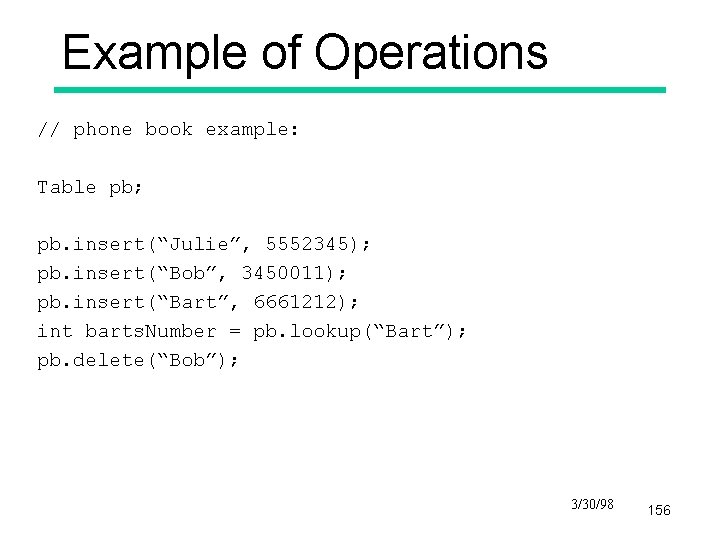
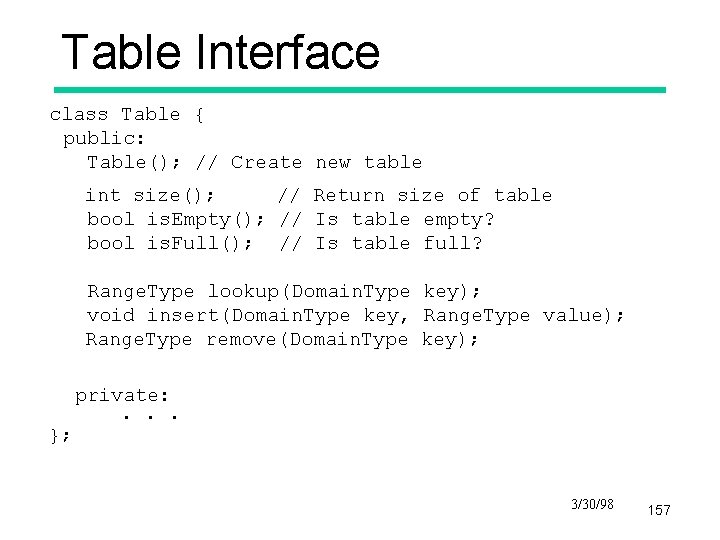
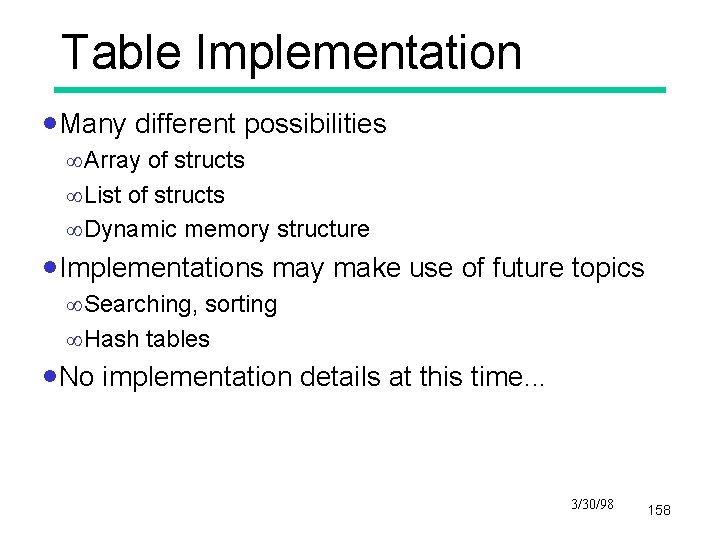
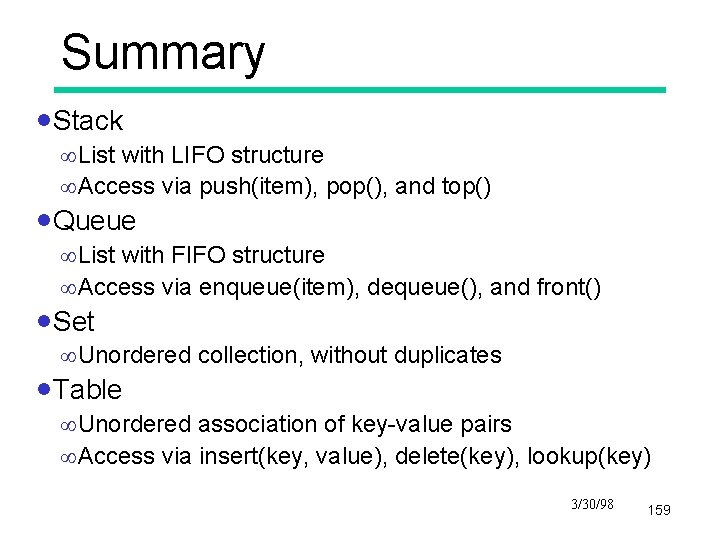
- Slides: 29
![CSE 143 More Collection ADTs Sections 4 6 4 8 33098 131 CSE 143 More Collection ADTs [Sections 4. 6 -4. 8] 3/30/98 131](https://slidetodoc.com/presentation_image/de75fc91b40923f25843aad80491e77e/image-1.jpg)
CSE 143 More Collection ADTs [Sections 4. 6 -4. 8] 3/30/98 131
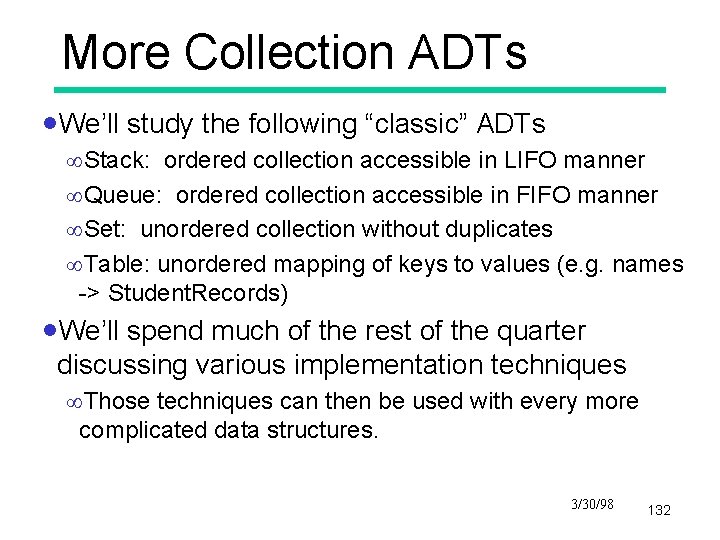
More Collection ADTs ·We’ll study the following “classic” ADTs ¥Stack: ordered collection accessible in LIFO manner ¥Queue: ordered collection accessible in FIFO manner ¥Set: unordered collection without duplicates ¥Table: unordered mapping of keys to values (e. g. names -> Student. Records) ·We’ll spend much of the rest of the quarter discussing various implementation techniques ¥Those techniques can then be used with every more complicated data structures. 3/30/98 132
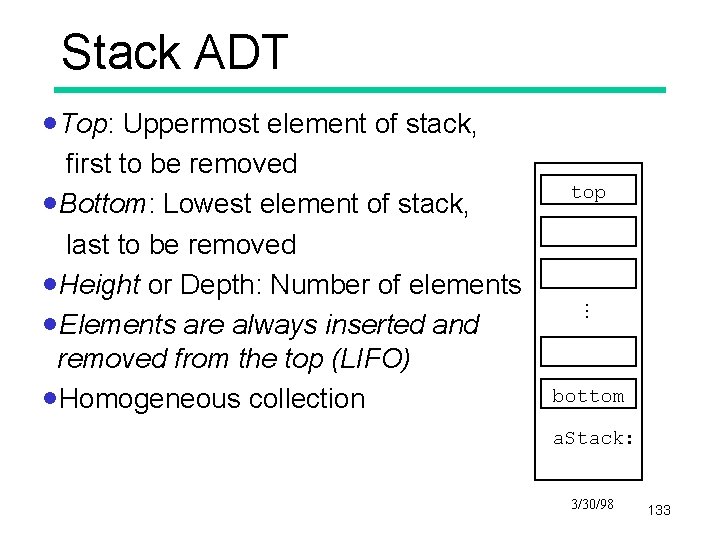
Stack ADT ·Top: Uppermost element of stack, top . . . first to be removed ·Bottom: Lowest element of stack, last to be removed ·Height or Depth: Number of elements ·Elements are always inserted and removed from the top (LIFO) ·Homogeneous collection bottom a. Stack: 3/30/98 133
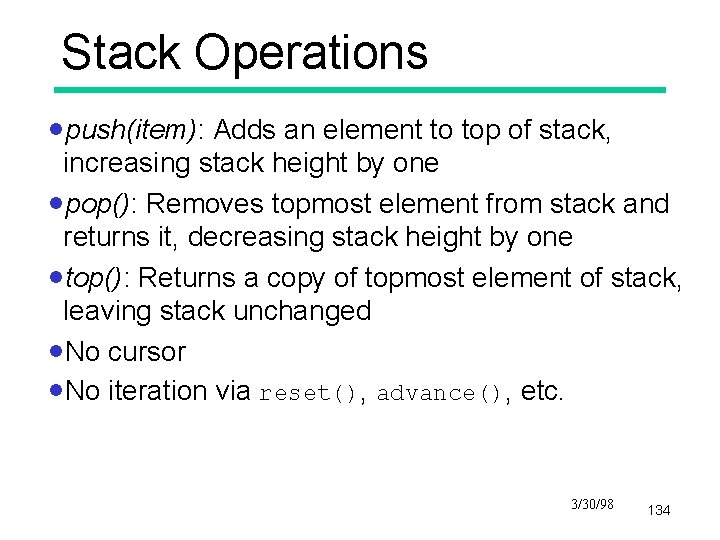
Stack Operations ·push(item): Adds an element to top of stack, increasing stack height by one ·pop(): Removes topmost element from stack and returns it, decreasing stack height by one ·top(): Returns a copy of topmost element of stack, leaving stack unchanged ·No cursor ·No iteration via reset(), advance(), etc. 3/30/98 134
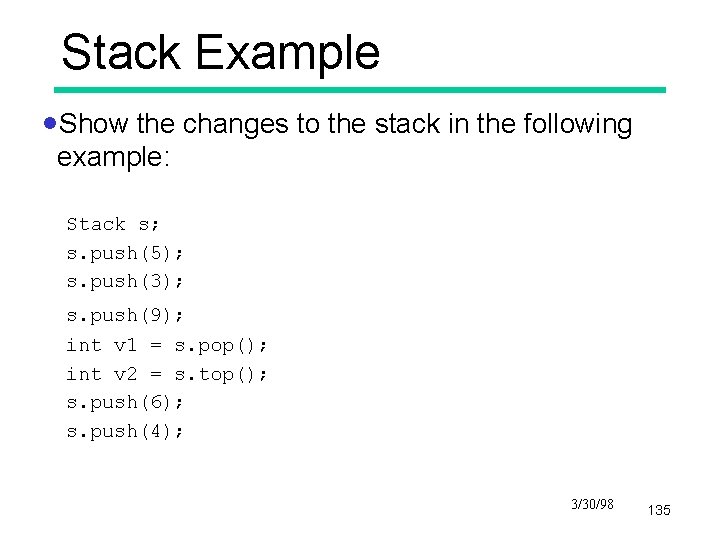
Stack Example ·Show the changes to the stack in the following example: Stack s; s. push(5); s. push(3); s. push(9); int v 1 = s. pop(); int v 2 = s. top(); s. push(6); s. push(4); 3/30/98 135
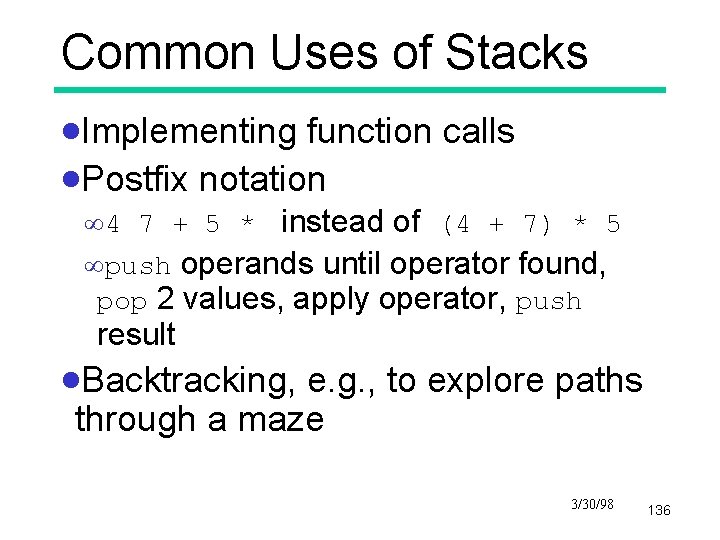
Common Uses of Stacks ·Implementing function calls ·Postfix notation ¥ 4 7 + 5 * instead of (4 + 7) * 5 ¥push operands until operator found, pop 2 values, apply operator, push result ·Backtracking, e. g. , to explore paths through a maze 3/30/98 136
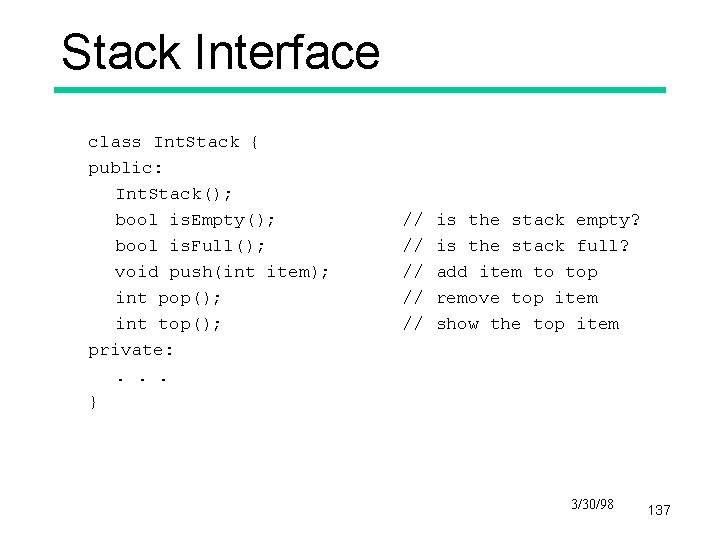
Stack Interface class Int. Stack { public: Int. Stack(); bool is. Empty(); bool is. Full(); void push(int item); int pop(); int top(); private: . . . } // // // is the stack empty? is the stack full? add item to top remove top item show the top item 3/30/98 137
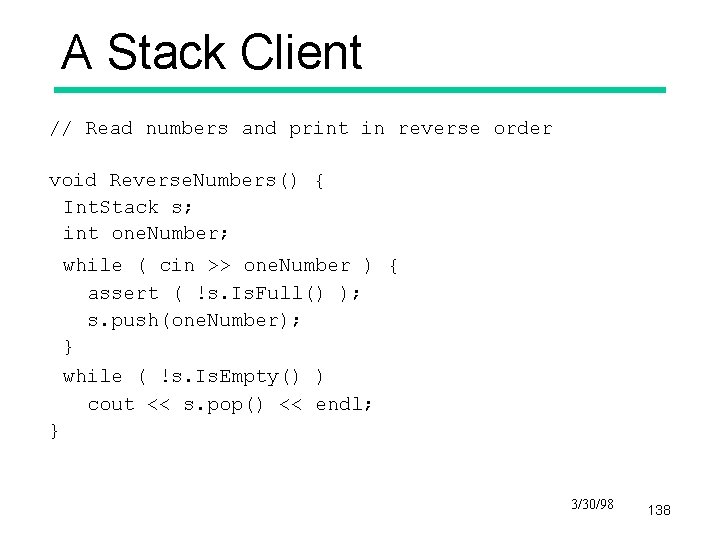
A Stack Client // Read numbers and print in reverse order void Reverse. Numbers() { Int. Stack s; int one. Number; while ( cin >> one. Number ) { assert ( !s. Is. Full() ); s. push(one. Number); } while ( !s. Is. Empty() ) cout << s. pop() << endl; } 3/30/98 138
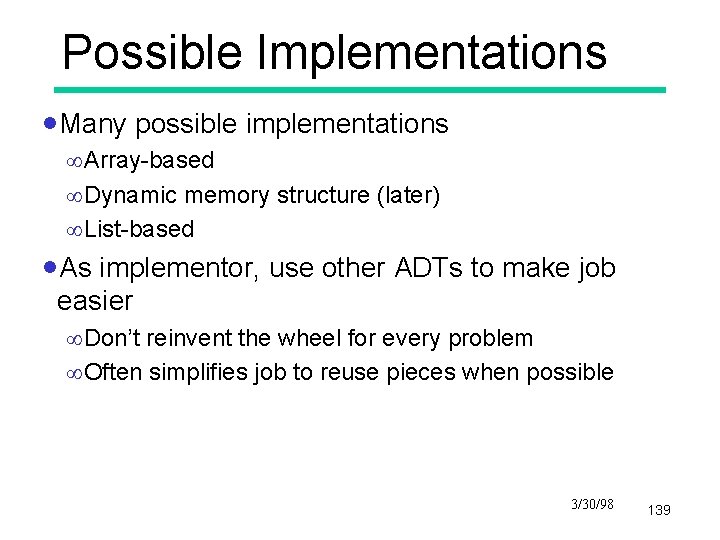
Possible Implementations ·Many possible implementations ¥Array-based ¥Dynamic memory structure (later) ¥List-based ·As implementor, use other ADTs to make job easier ¥Don’t reinvent the wheel for every problem ¥Often simplifies job to reuse pieces when possible 3/30/98 139
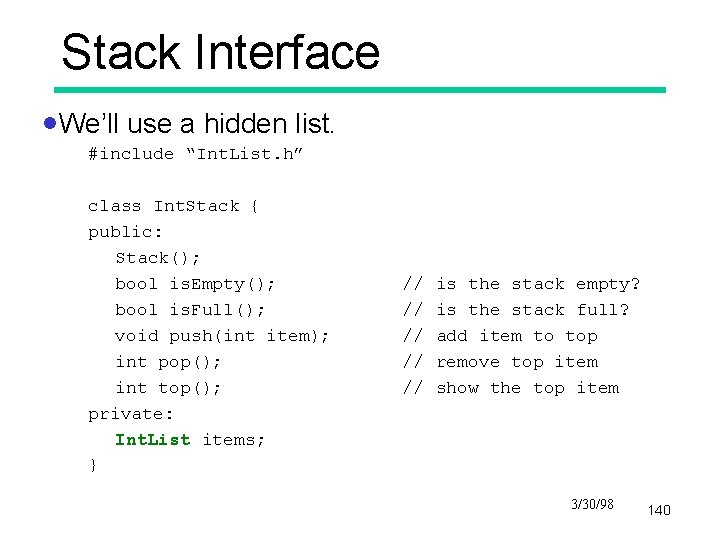
Stack Interface ·We’ll use a hidden list. #include “Int. List. h” class Int. Stack { public: Stack(); bool is. Empty(); bool is. Full(); void push(int item); int pop(); int top(); private: Int. List items; } // // // is the stack empty? is the stack full? add item to top remove top item show the top item 3/30/98 140
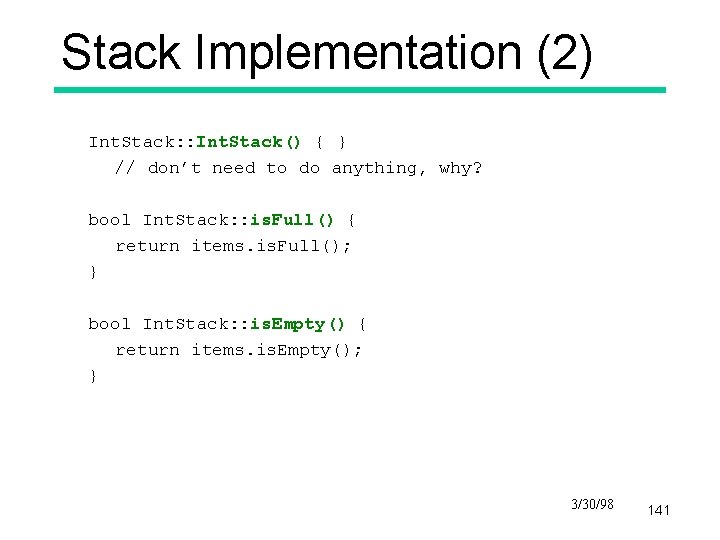
Stack Implementation (2) Int. Stack: : Int. Stack() { } // don’t need to do anything, why? bool Int. Stack: : is. Full() { return items. is. Full(); } bool Int. Stack: : is. Empty() { return items. is. Empty(); } 3/30/98 141
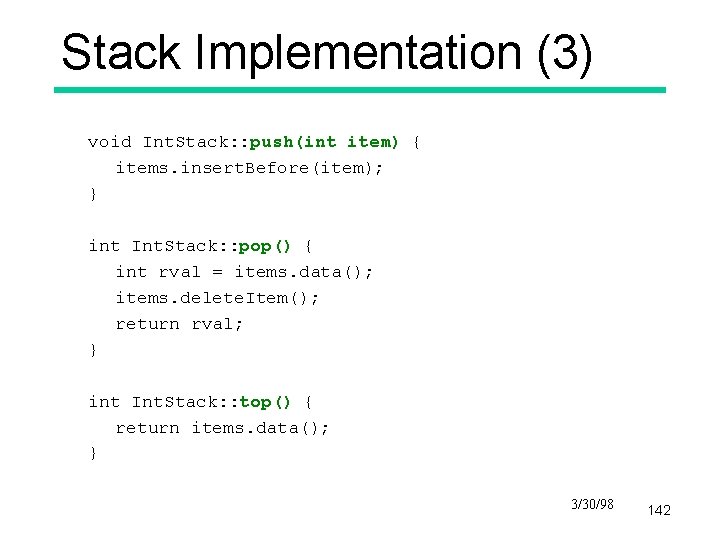
Stack Implementation (3) void Int. Stack: : push(int item) { items. insert. Before(item); } int Int. Stack: : pop() { int rval = items. data(); items. delete. Item(); return rval; } int Int. Stack: : top() { return items. data(); } 3/30/98 142
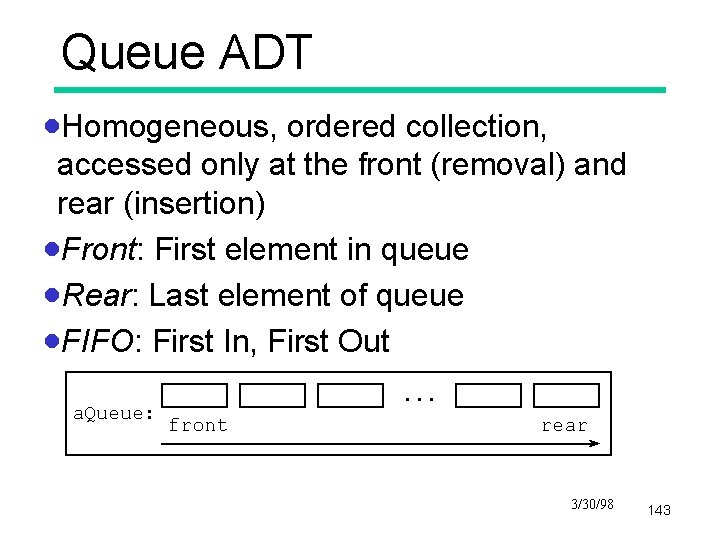
Queue ADT ·Homogeneous, ordered collection, accessed only at the front (removal) and rear (insertion) ·Front: First element in queue ·Rear: Last element of queue ·FIFO: First In, First Out a. Queue: . . . front rear 3/30/98 143
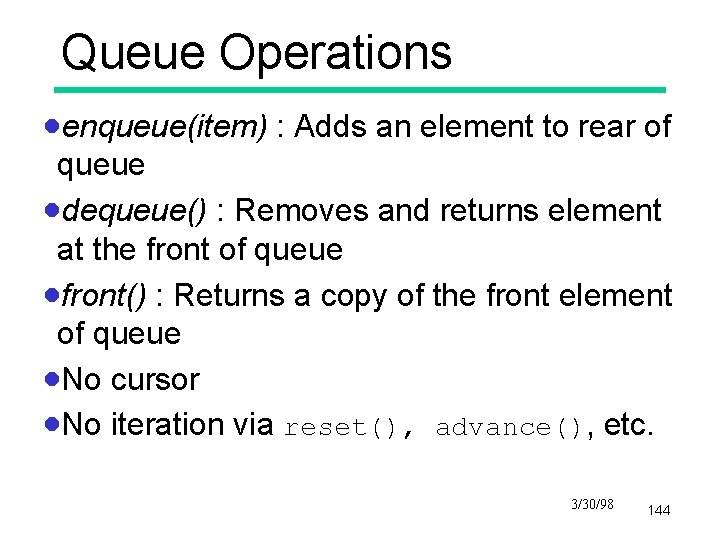
Queue Operations ·enqueue(item) : Adds an element to rear of queue ·dequeue() : Removes and returns element at the front of queue ·front() : Returns a copy of the front element of queue ·No cursor ·No iteration via reset(), advance(), etc. 3/30/98 144
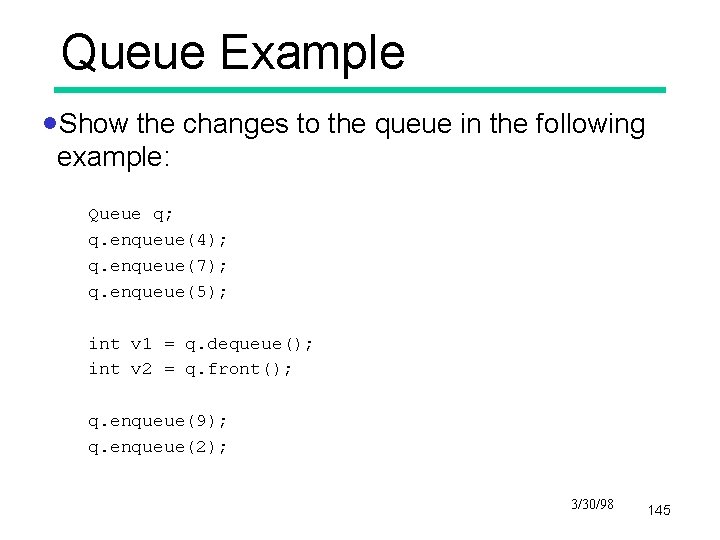
Queue Example ·Show the changes to the queue in the following example: Queue q; q. enqueue(4); q. enqueue(7); q. enqueue(5); int v 1 = q. dequeue(); int v 2 = q. front(); q. enqueue(9); q. enqueue(2); 3/30/98 145
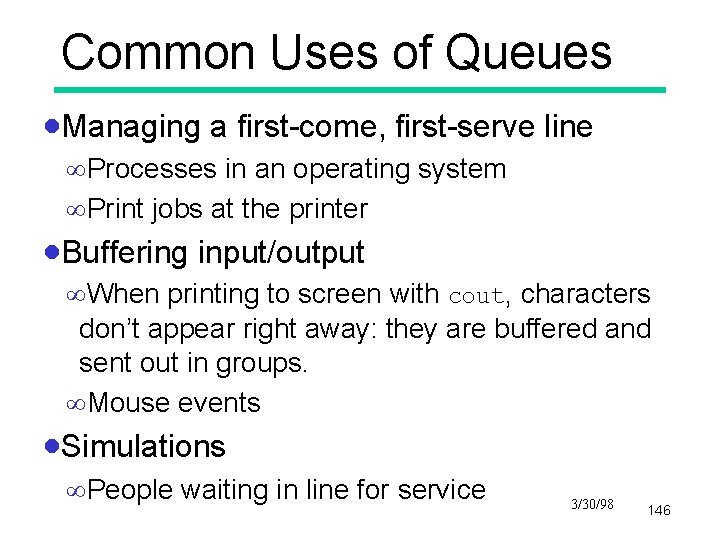
Common Uses of Queues ·Managing a first-come, first-serve line ¥Processes in an operating system ¥Print jobs at the printer ·Buffering input/output ¥When printing to screen with cout, characters don’t appear right away: they are buffered and sent out in groups. ¥Mouse events ·Simulations ¥People waiting in line for service 3/30/98 146
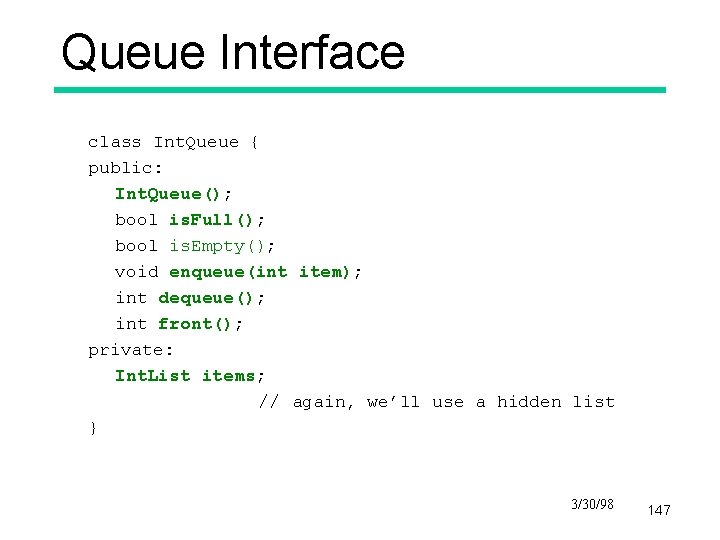
Queue Interface class Int. Queue { public: Int. Queue(); bool is. Full(); bool is. Empty(); void enqueue(int item); int dequeue(); int front(); private: Int. List items; // again, we’ll use a hidden list } 3/30/98 147
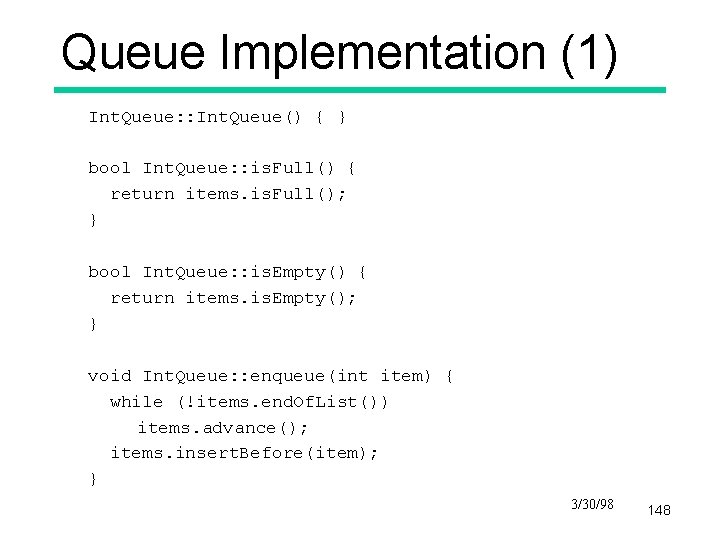
Queue Implementation (1) Int. Queue: : Int. Queue() { } bool Int. Queue: : is. Full() { return items. is. Full(); } bool Int. Queue: : is. Empty() { return items. is. Empty(); } void Int. Queue: : enqueue(int item) { while (!items. end. Of. List()) items. advance(); items. insert. Before(item); } 3/30/98 148
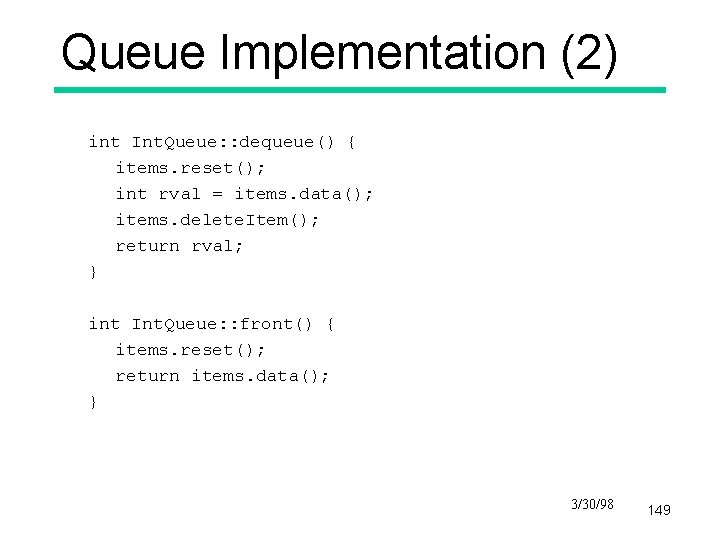
Queue Implementation (2) int Int. Queue: : dequeue() { items. reset(); int rval = items. data(); items. delete. Item(); return rval; } int Int. Queue: : front() { items. reset(); return items. data(); } 3/30/98 149
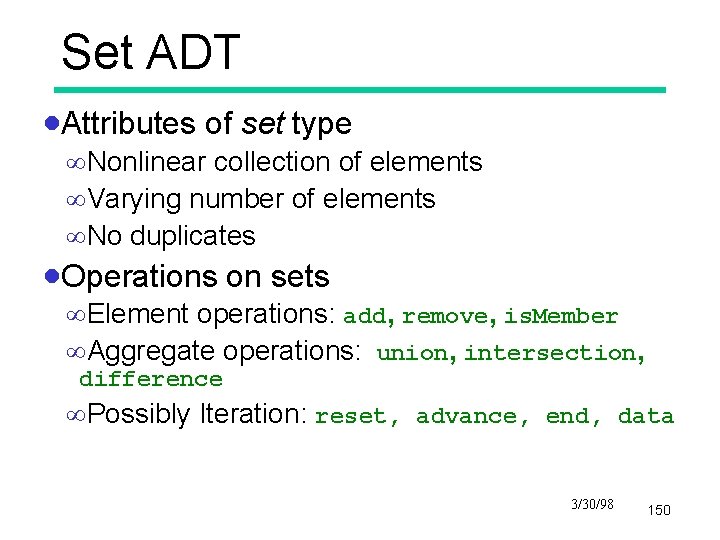
Set ADT ·Attributes of set type ¥Nonlinear collection of elements ¥Varying number of elements ¥No duplicates ·Operations on sets ¥Element operations: add, remove, is. Member ¥Aggregate operations: union, intersection, difference ¥Possibly Iteration: reset, advance, end, data 3/30/98 150
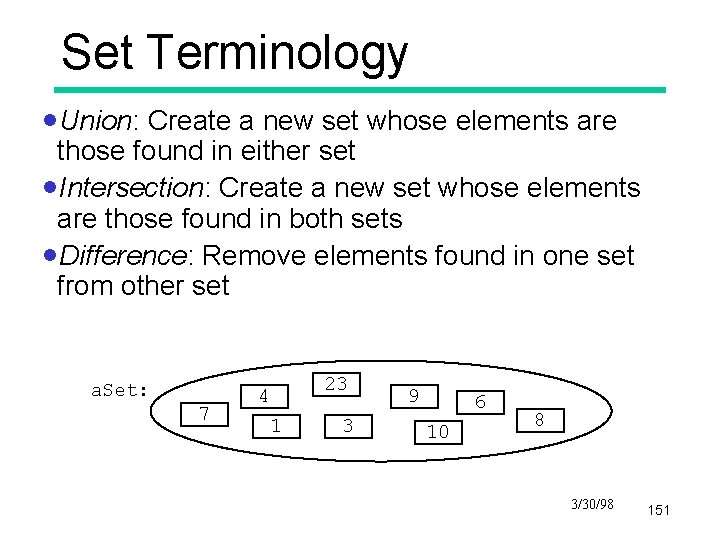
Set Terminology ·Union: Create a new set whose elements are those found in either set ·Intersection: Create a new set whose elements are those found in both sets ·Difference: Remove elements found in one set from other set a. Set: 7 23 4 1 3 9 6 10 8 3/30/98 151
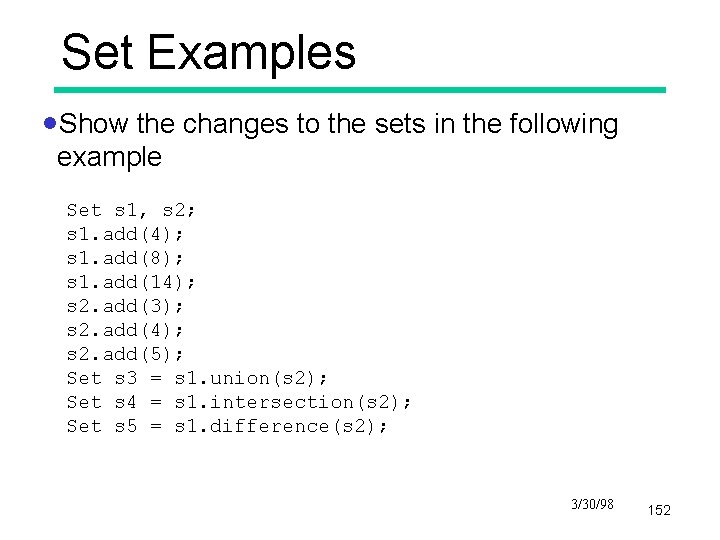
Set Examples ·Show the changes to the sets in the following example Set s 1, s 2; s 1. add(4); s 1. add(8); s 1. add(14); s 2. add(3); s 2. add(4); s 2. add(5); Set s 3 = s 1. union(s 2); Set s 4 = s 1. intersection(s 2); Set s 5 = s 1. difference(s 2); 3/30/98 152
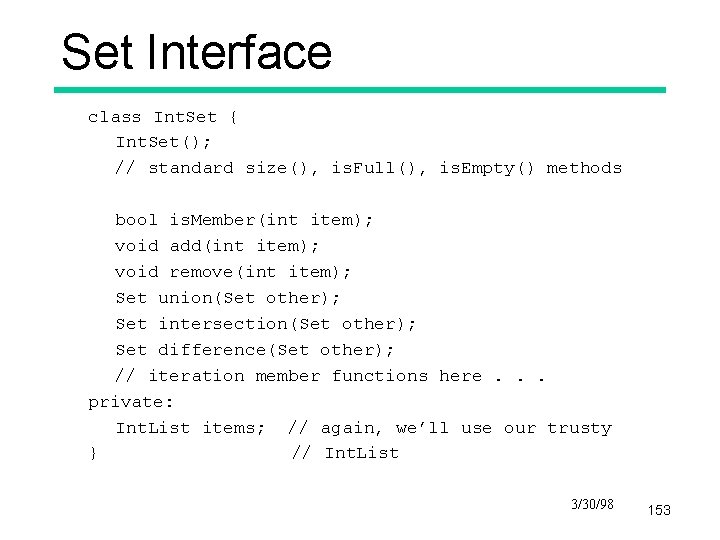
Set Interface class Int. Set { Int. Set(); // standard size(), is. Full(), is. Empty() methods bool is. Member(int item); void add(int item); void remove(int item); Set union(Set other); Set intersection(Set other); Set difference(Set other); // iteration member functions here. . . private: Int. List items; // again, we’ll use our trusty } // Int. List 3/30/98 153
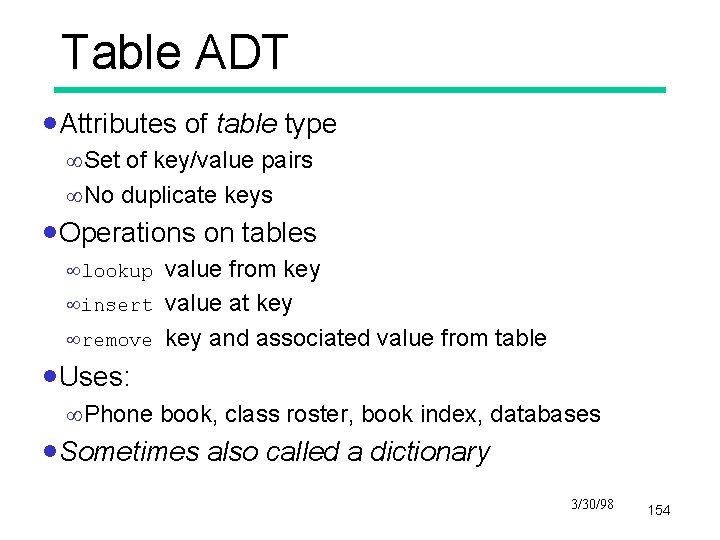
Table ADT ·Attributes of table type ¥Set of key/value pairs ¥No duplicate keys ·Operations on tables ¥lookup value from key ¥insert value at key ¥remove key and associated value from table ·Uses: ¥Phone book, class roster, book index, databases ·Sometimes also called a dictionary 3/30/98 154
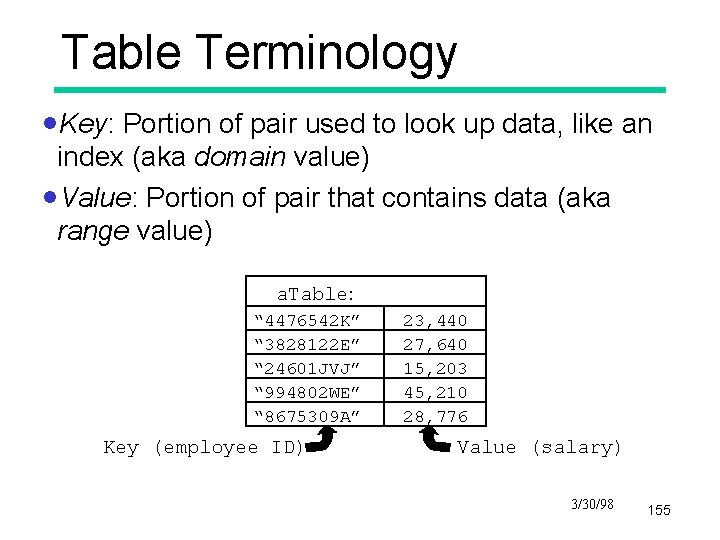
Table Terminology ·Key: Portion of pair used to look up data, like an index (aka domain value) ·Value: Portion of pair that contains data (aka range value) a. Table: “ 4476542 K” “ 3828122 E” “ 24601 JVJ” “ 994802 WE” “ 8675309 A” Key (employee ID) 23, 440 27, 640 15, 203 45, 210 28, 776 Value (salary) 3/30/98 155
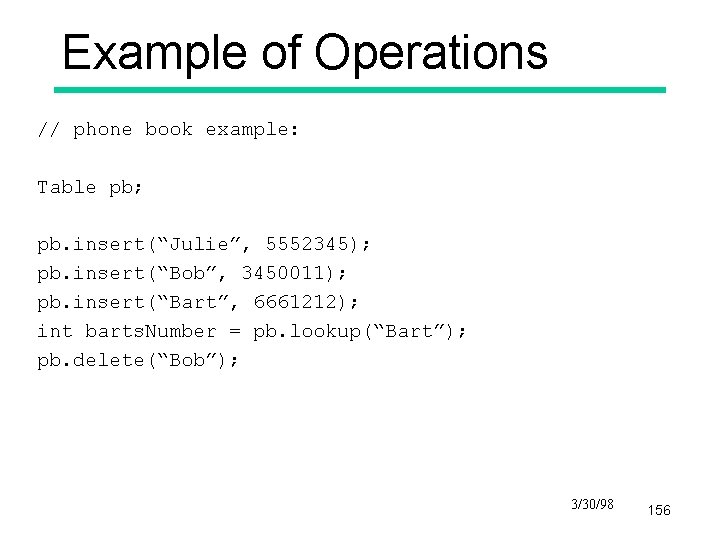
Example of Operations // phone book example: Table pb; pb. insert(“Julie”, 5552345); pb. insert(“Bob”, 3450011); pb. insert(“Bart”, 6661212); int barts. Number = pb. lookup(“Bart”); pb. delete(“Bob”); 3/30/98 156
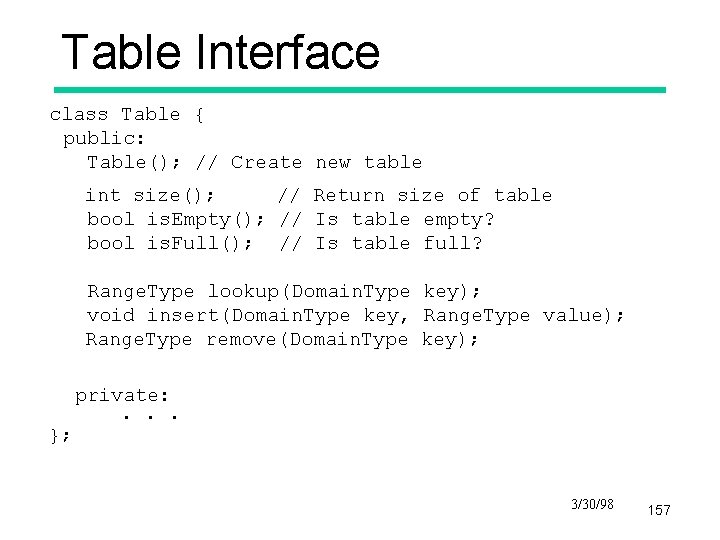
Table Interface class Table { public: Table(); // Create new table int size(); // Return size of table bool is. Empty(); // Is table empty? bool is. Full(); // Is table full? Range. Type lookup(Domain. Type key); void insert(Domain. Type key, Range. Type value); Range. Type remove(Domain. Type key); }; private: . . . 3/30/98 157
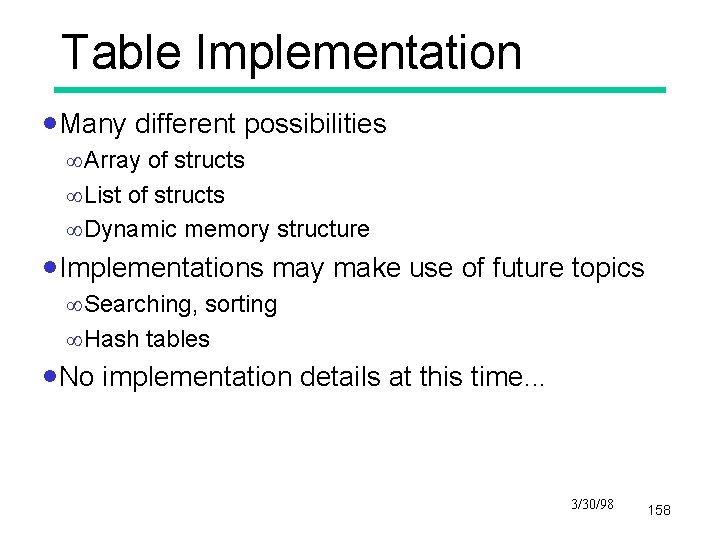
Table Implementation ·Many different possibilities ¥Array of structs ¥List of structs ¥Dynamic memory structure ·Implementations may make use of future topics ¥Searching, sorting ¥Hash tables ·No implementation details at this time. . . 3/30/98 158
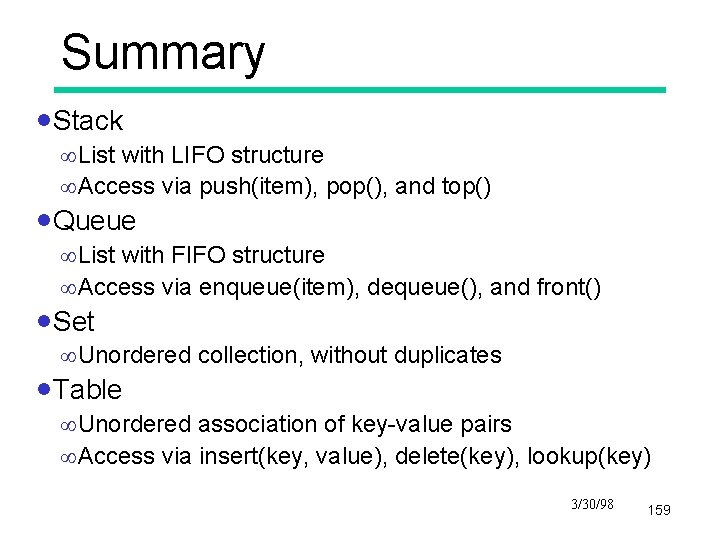
Summary ·Stack ¥List with LIFO structure ¥Access via push(item), pop(), and top() ·Queue ¥List with FIFO structure ¥Access via enqueue(item), dequeue(), and front() ·Set ¥Unordered collection, without duplicates ·Table ¥Unordered association of key-value pairs ¥Access via insert(key, value), delete(key), lookup(key) 3/30/98 159
Lirik lagu more more more we praise you
More more more i want more more more more we praise you
Cse 143
Cse 143
Cse 143
Adts ukiah
Adts, data structures, and problem solving with c++
Parameterized abstract data types
Parameterized abstract data types
Landsat collection 1 vs collection 2
Clean collection vs documentary collection
Air canada flight 143
Anova math
Alan ableson queens
143 000 in scientific notation
143.ent
Biostats usmle
143/100 simplified
427 000 in scientific notation
Salmo 143:2
11-1 area of parallelograms and triangles
143 ent
Melodia do salmo 143 bendito seja o senhor meu rochedo
Pg 143
Hammurabis code 143
Let the holy spirit guide you
Csc 143
Sp 143 warszawa
Accretion expense
Hammurabis code 143