CS 1102 Lec 06 Programming Languages Program Development
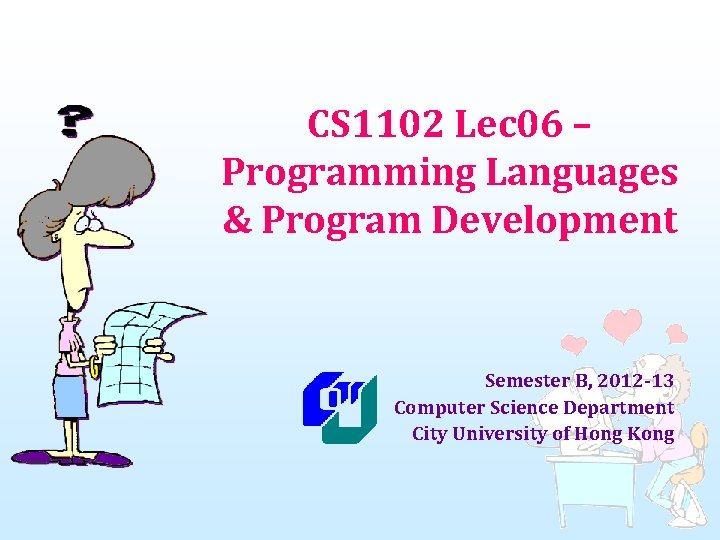
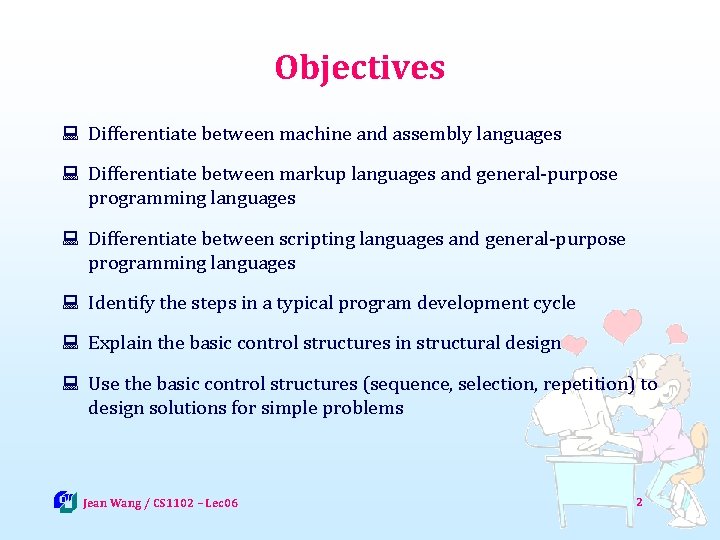
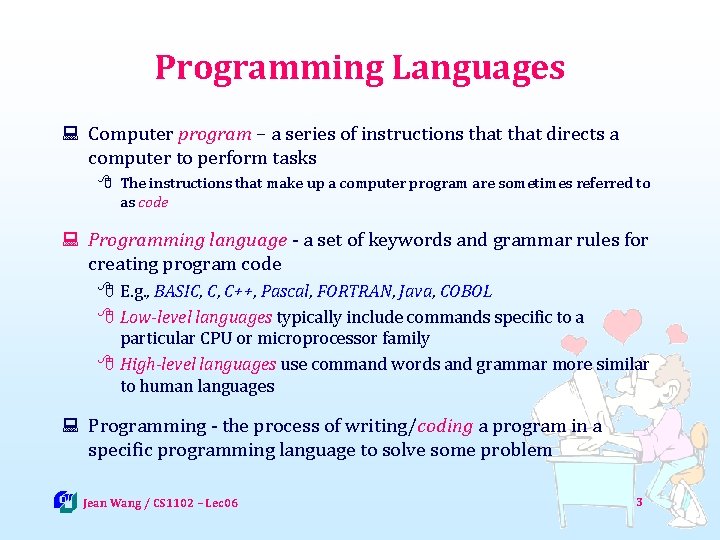
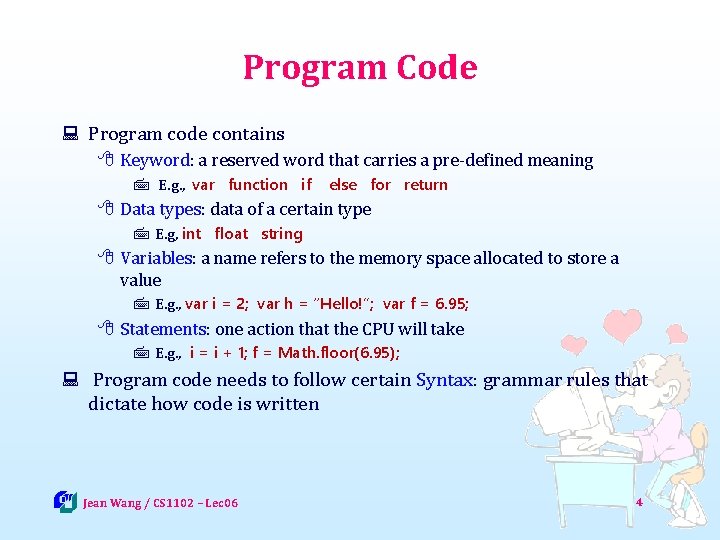
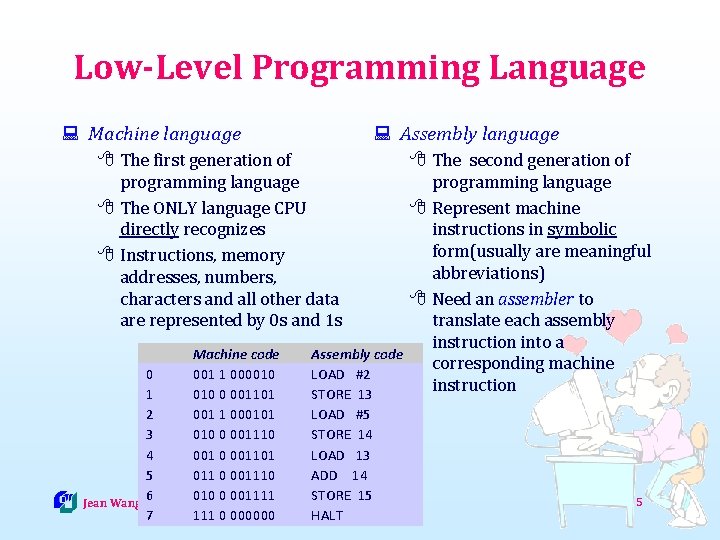
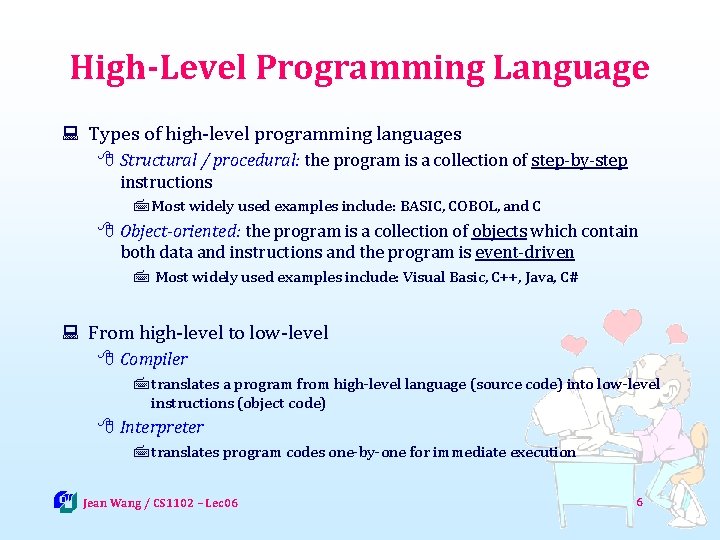
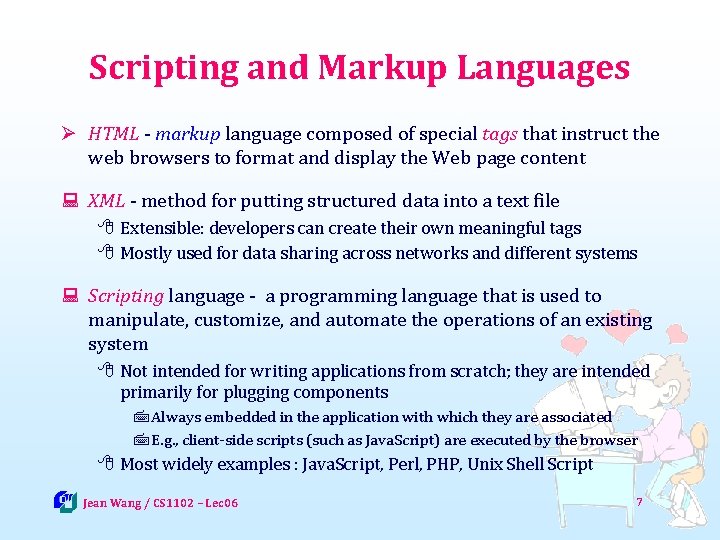
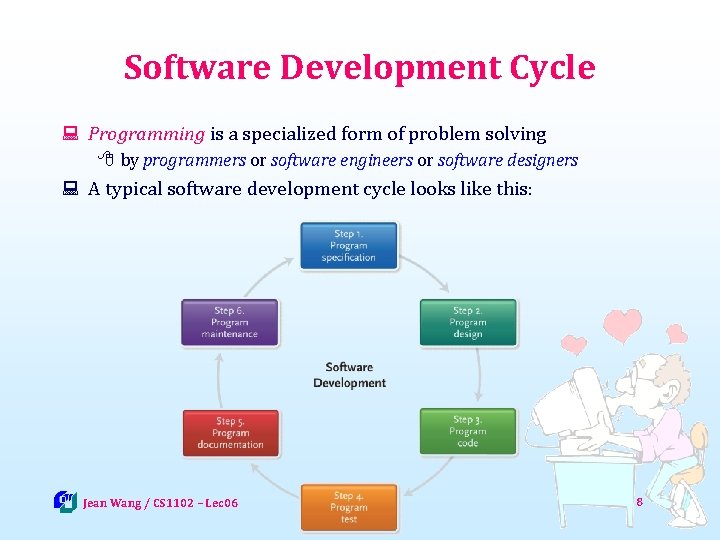
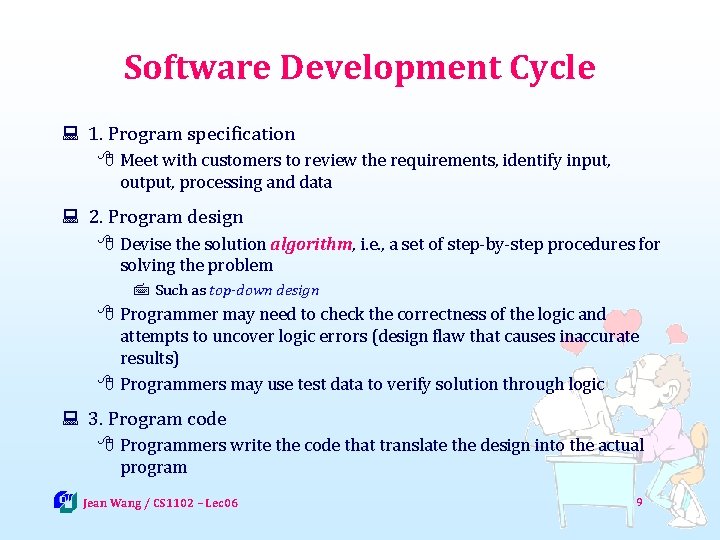
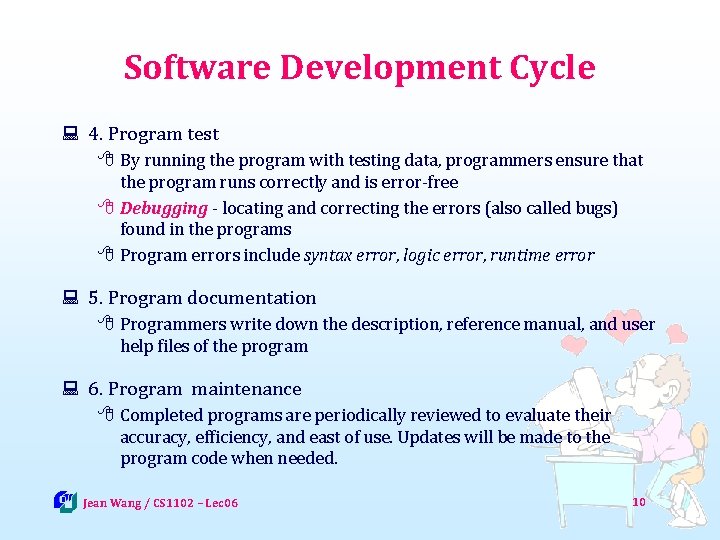
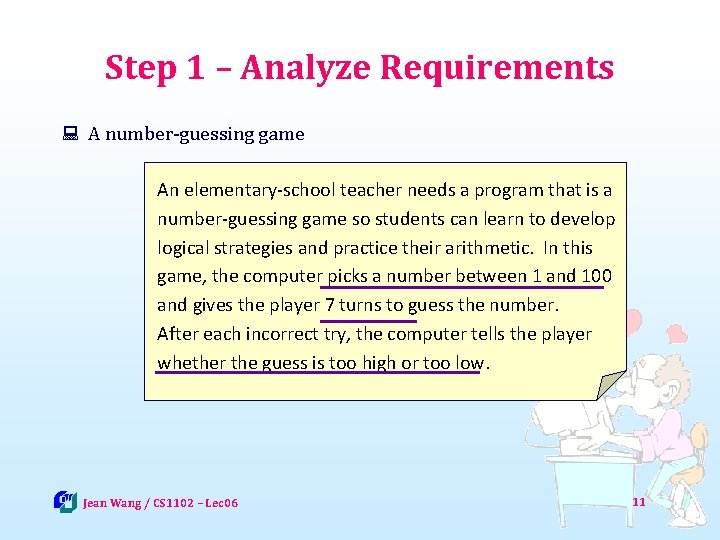
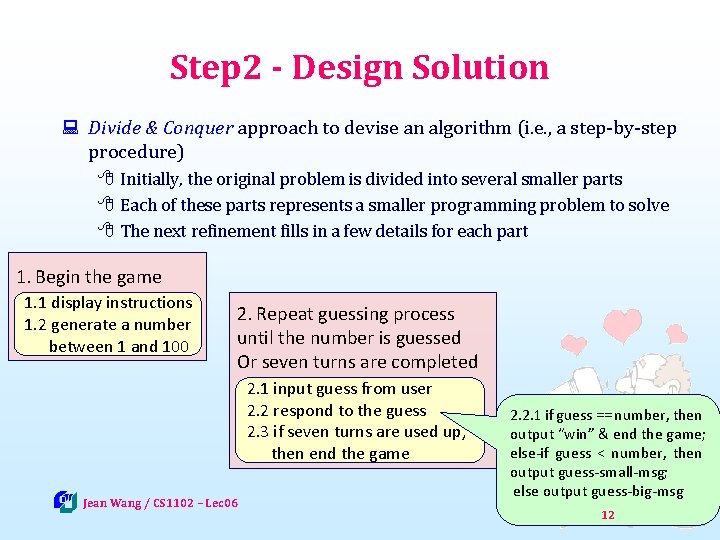
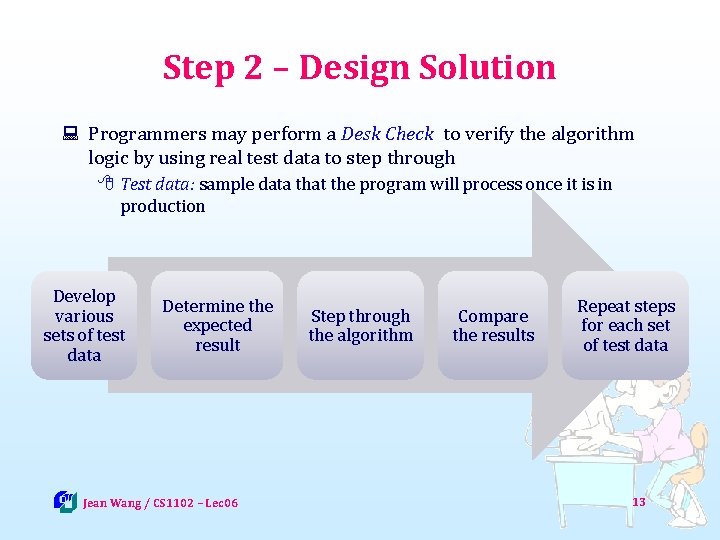
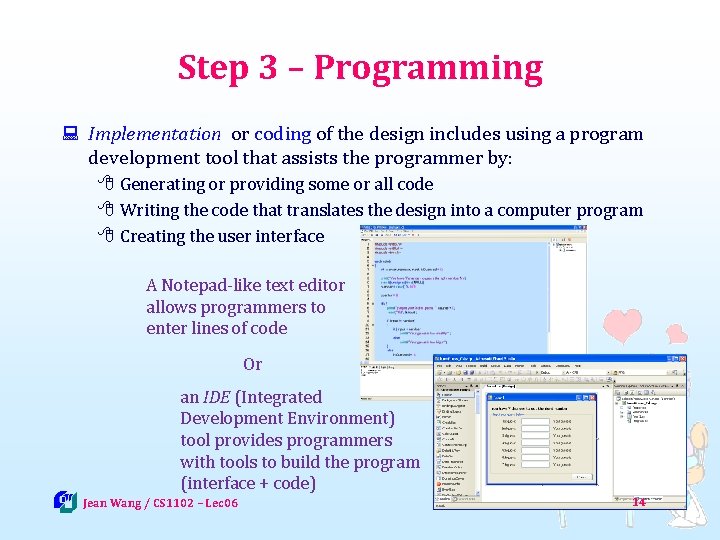
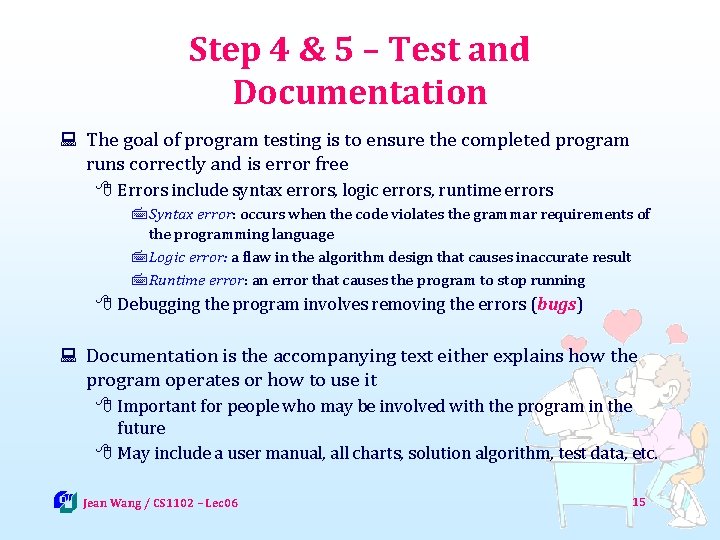
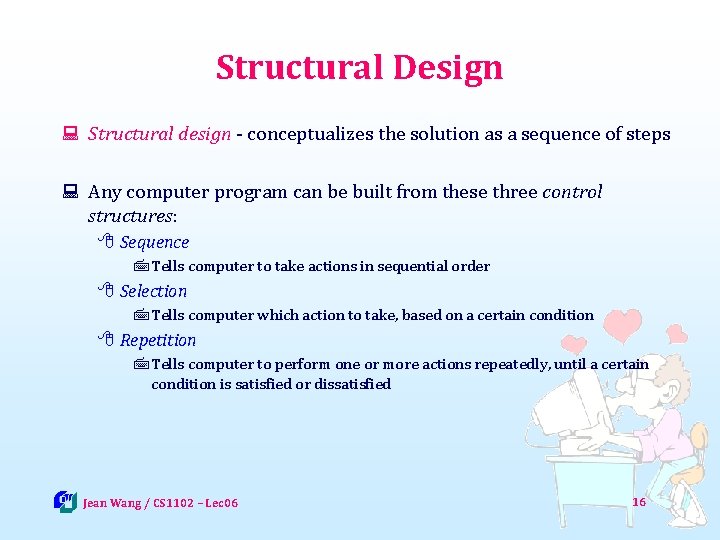
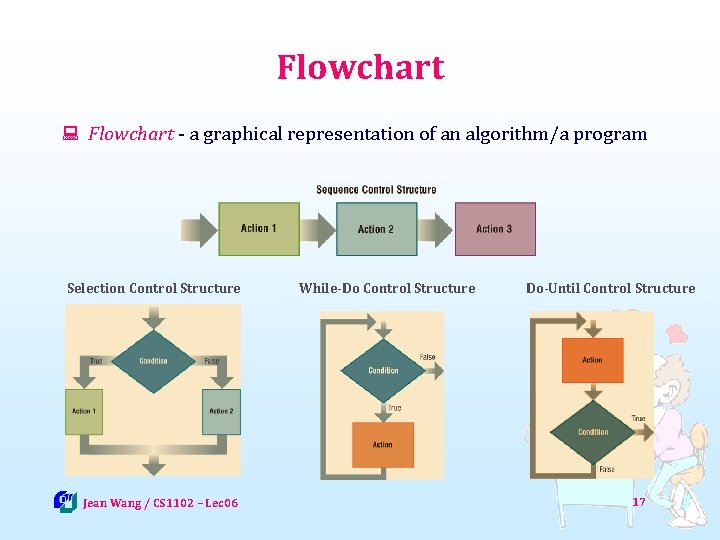
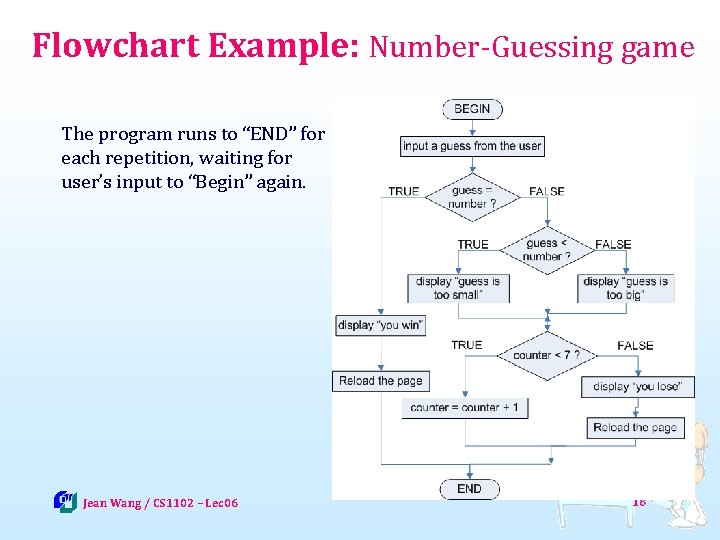
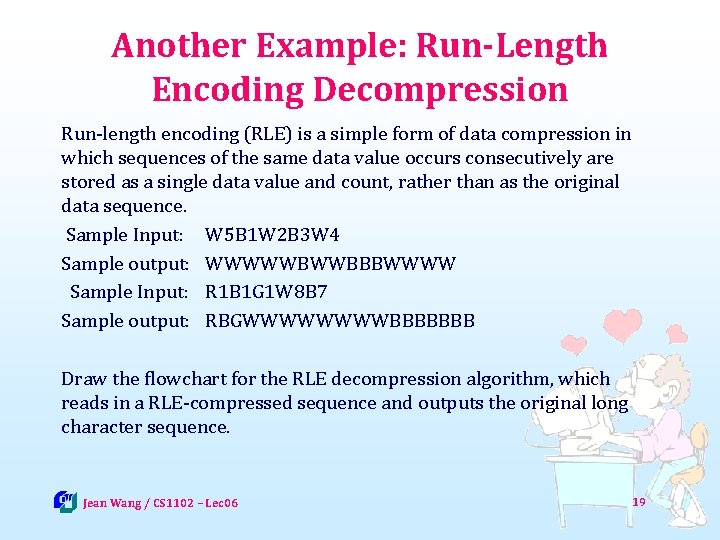
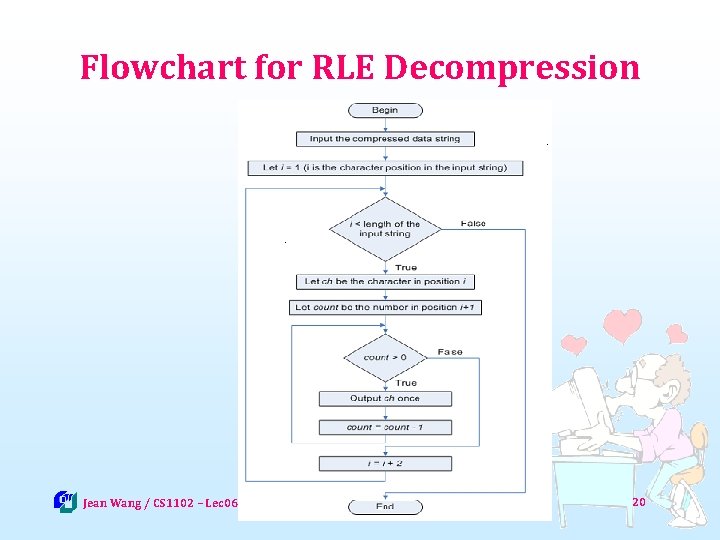
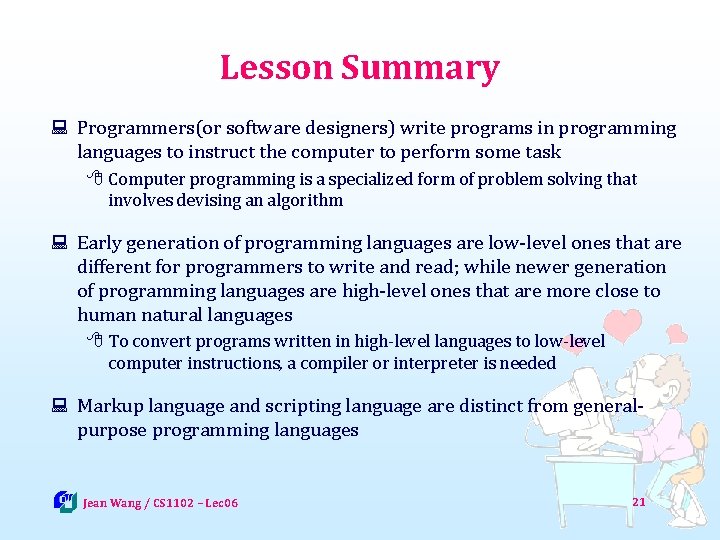
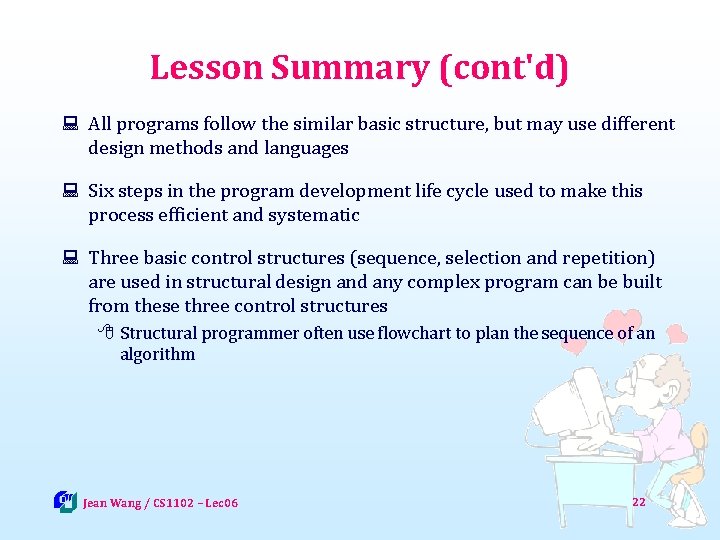
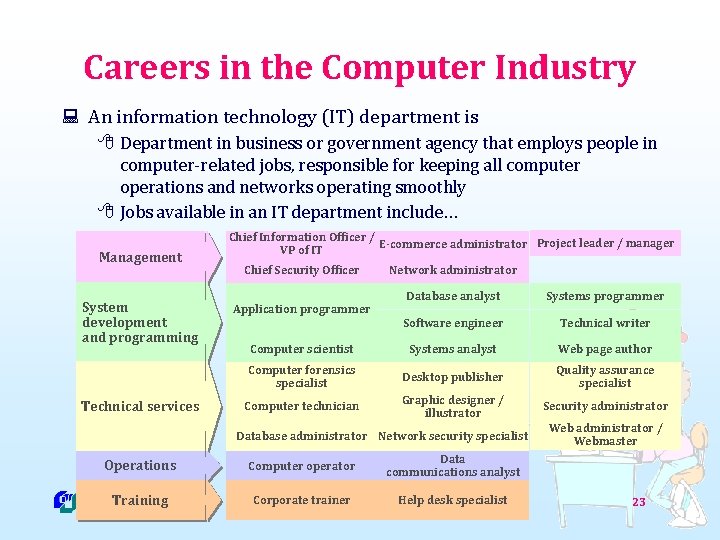
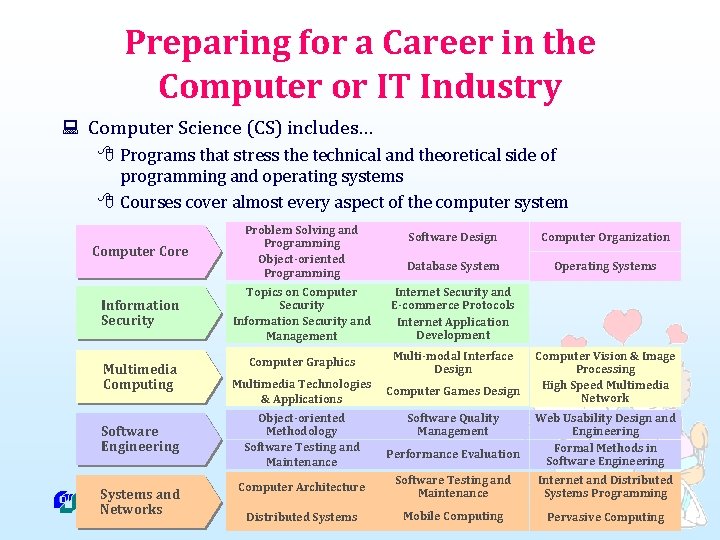
- Slides: 24
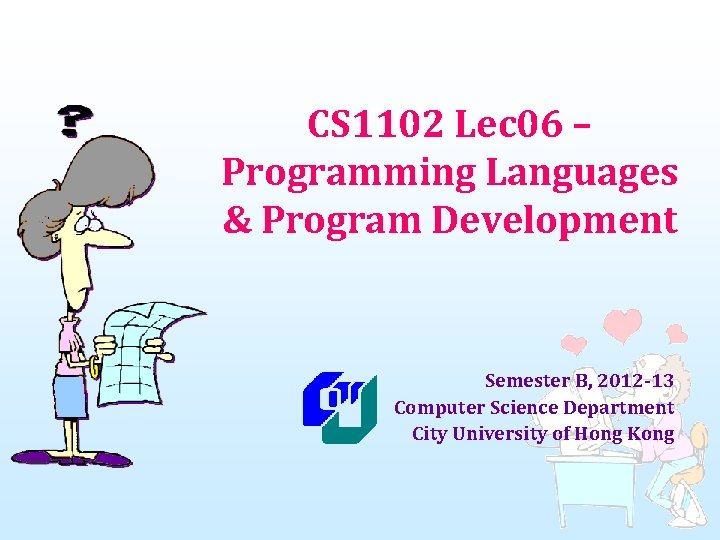
CS 1102 Lec 06 – Programming Languages & Program Development Semester B, 2012 -13 Computer Science Department City University of Hong Kong
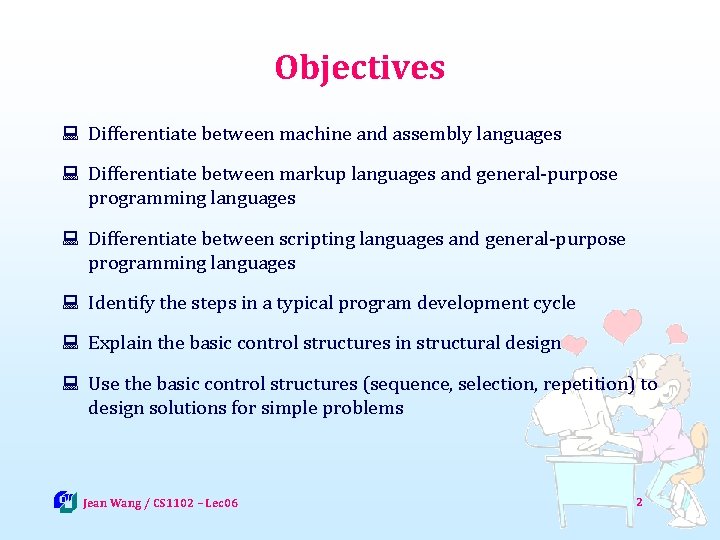
Objectives : Differentiate between machine and assembly languages : Differentiate between markup languages and general-purpose programming languages : Differentiate between scripting languages and general-purpose programming languages : Identify the steps in a typical program development cycle : Explain the basic control structures in structural design : Use the basic control structures (sequence, selection, repetition) to design solutions for simple problems Jean Wang / CS 1102 – Lec 06 2
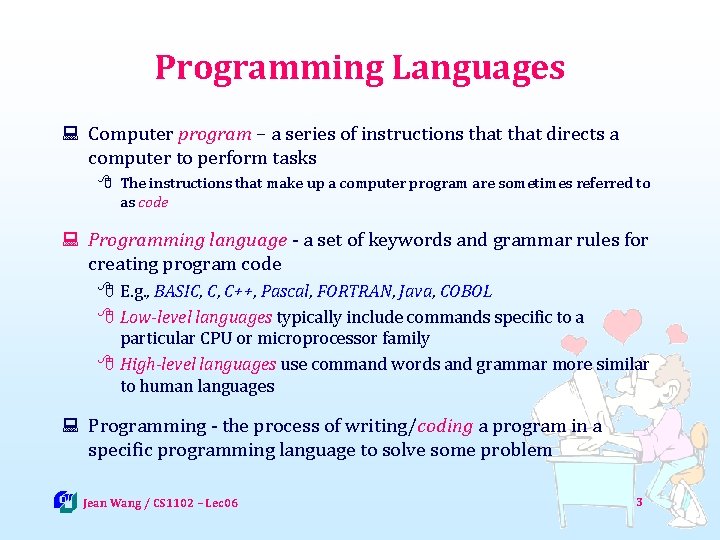
Programming Languages : Computer program – a series of instructions that directs a computer to perform tasks 8 The instructions that make up a computer program are sometimes referred to as code : Programming language - a set of keywords and grammar rules for creating program code 8 E. g. , BASIC, C, C++, Pascal, FORTRAN, Java, COBOL 8 Low-level languages typically include commands specific to a particular CPU or microprocessor family 8 High-level languages use command words and grammar more similar to human languages : Programming - the process of writing/coding a program in a specific programming language to solve some problem Jean Wang / CS 1102 – Lec 06 3
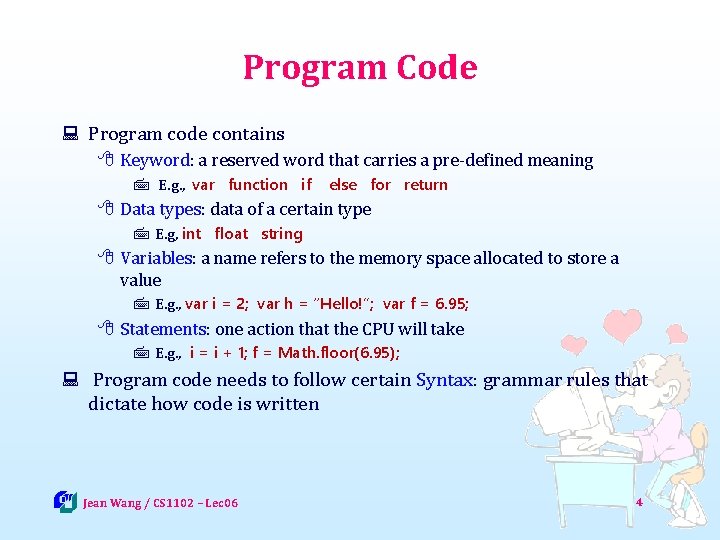
Program Code : Program code contains 8 Keyword: a reserved word that carries a pre-defined meaning 7 E. g. , var function if else for return 8 Data types: data of a certain type 7 E. g, int float string 8 Variables: a name refers to the memory space allocated to store a value 7 E. g. , var i = 2; var h = “Hello!”; var f = 6. 95; 8 Statements: one action that the CPU will take 7 E. g. , i = i + 1; f = Math. floor(6. 95); : Program code needs to follow certain Syntax: grammar rules that dictate how code is written Jean Wang / CS 1102 – Lec 06 4
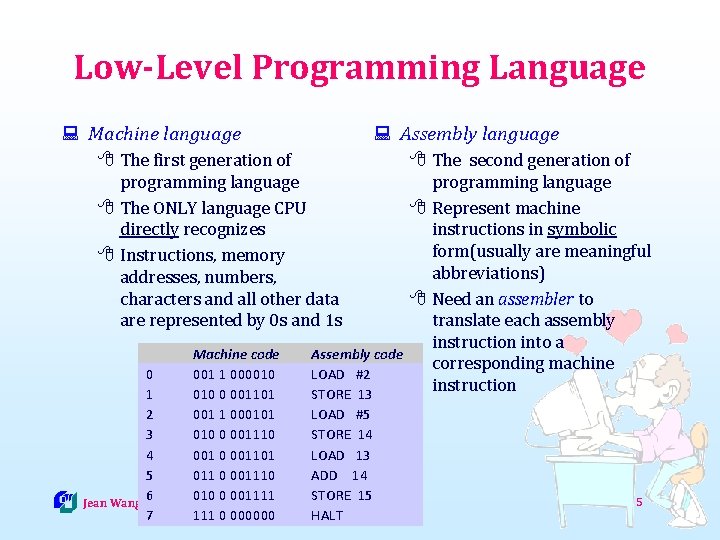
Low-Level Programming Language : Machine language : Assembly language 8 The first generation of programming language 8 The ONLY language CPU directly recognizes 8 Instructions, memory addresses, numbers, characters and all other data are represented by 0 s and 1 s Machine code 0 001 1 000010 1 010 0 001101 2 001 1 000101 3 010 0 001110 4 001 0 001101 5 011 0 001110 6 010 0 001111 Jean Wang / CS 1102 – Lec 06 7 111 0 000000 8 The second generation of programming language 8 Represent machine instructions in symbolic form(usually are meaningful abbreviations) 8 Need an assembler to translate each assembly instruction into a Assembly code corresponding machine LOAD #2 instruction STORE 13 LOAD #5 STORE 14 LOAD 13 ADD 14 STORE 15 HALT 5
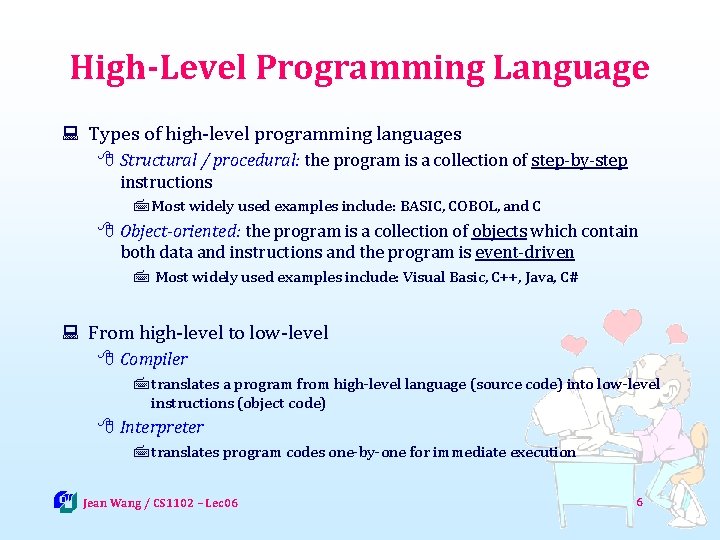
High-Level Programming Language : Types of high-level programming languages 8 Structural / procedural: the program is a collection of step-by-step instructions 7 Most widely used examples include: BASIC, COBOL, and C 8 Object-oriented: the program is a collection of objects which contain both data and instructions and the program is event-driven 7 Most widely used examples include: Visual Basic, C++, Java, C# : From high-level to low-level 8 Compiler 7 translates a program from high-level language (source code) into low-level instructions (object code) 8 Interpreter 7 translates program codes one-by-one for immediate execution Jean Wang / CS 1102 – Lec 06 6
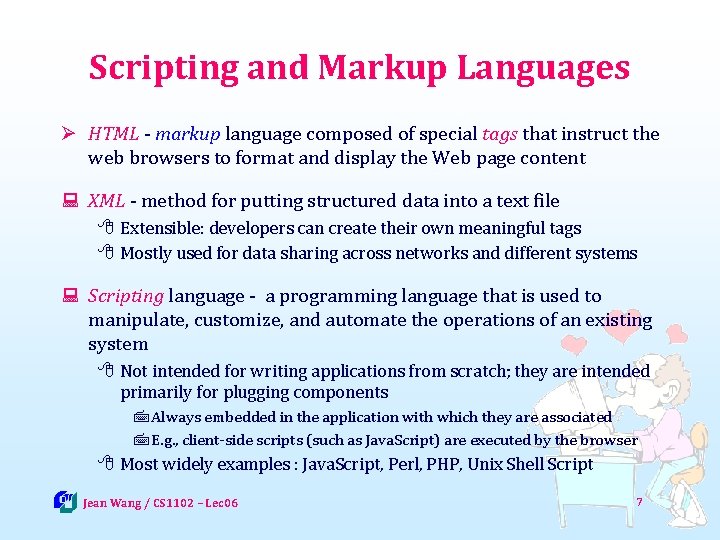
Scripting and Markup Languages Ø HTML - markup language composed of special tags that instruct the web browsers to format and display the Web page content : XML - method for putting structured data into a text file 8 Extensible: developers can create their own meaningful tags 8 Mostly used for data sharing across networks and different systems : Scripting language - a programming language that is used to manipulate, customize, and automate the operations of an existing system 8 Not intended for writing applications from scratch; they are intended primarily for plugging components 7 Always embedded in the application with which they are associated 7 E. g. , client-side scripts (such as Java. Script) are executed by the browser 8 Most widely examples : Java. Script, Perl, PHP, Unix Shell Script Jean Wang / CS 1102 – Lec 06 7
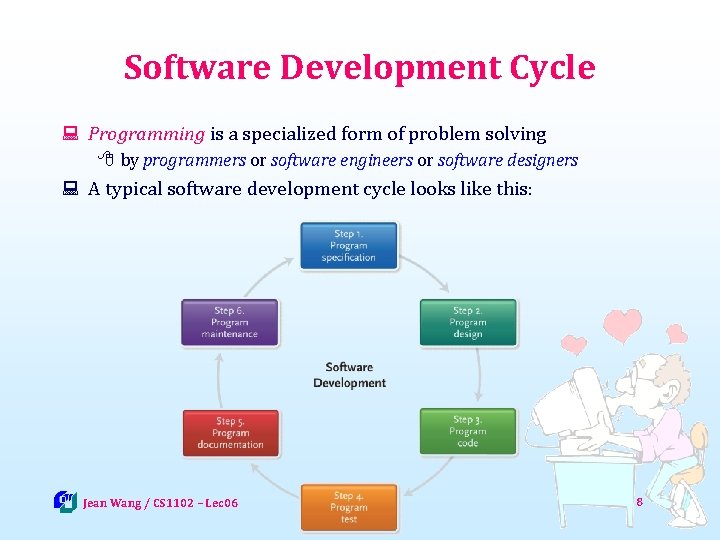
Software Development Cycle : Programming is a specialized form of problem solving 8 by programmers or software engineers or software designers : A typical software development cycle looks like this: Jean Wang / CS 1102 – Lec 06 8
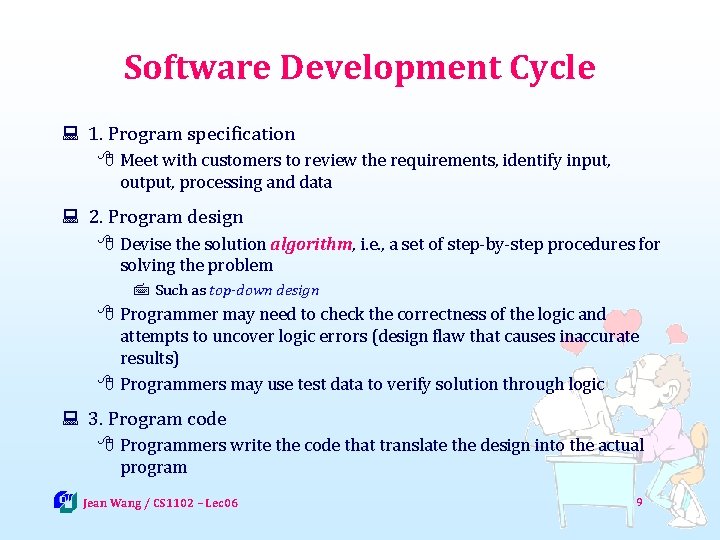
Software Development Cycle : 1. Program specification 8 Meet with customers to review the requirements, identify input, output, processing and data : 2. Program design 8 Devise the solution algorithm, i. e. , a set of step-by-step procedures for solving the problem 7 Such as top-down design 8 Programmer may need to check the correctness of the logic and attempts to uncover logic errors (design flaw that causes inaccurate results) 8 Programmers may use test data to verify solution through logic : 3. Program code 8 Programmers write the code that translate the design into the actual program Jean Wang / CS 1102 – Lec 06 9
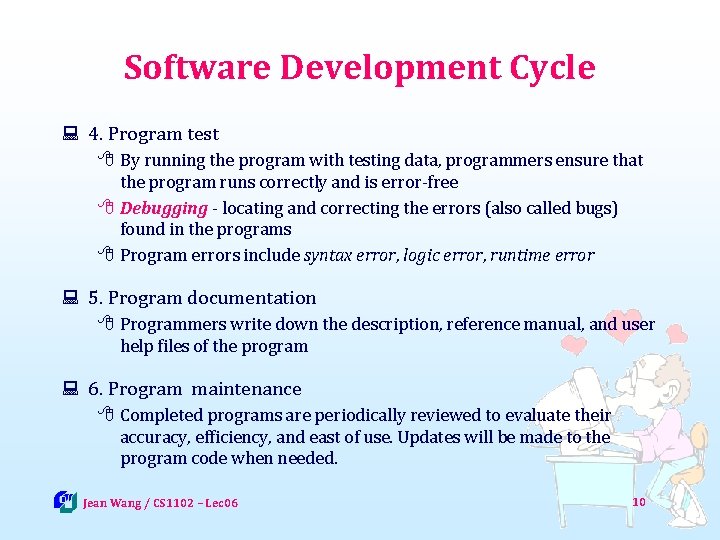
Software Development Cycle : 4. Program test 8 By running the program with testing data, programmers ensure that the program runs correctly and is error-free 8 Debugging - locating and correcting the errors (also called bugs) found in the programs 8 Program errors include syntax error, logic error, runtime error : 5. Program documentation 8 Programmers write down the description, reference manual, and user help files of the program : 6. Program maintenance 8 Completed programs are periodically reviewed to evaluate their accuracy, efficiency, and east of use. Updates will be made to the program code when needed. Jean Wang / CS 1102 – Lec 06 10
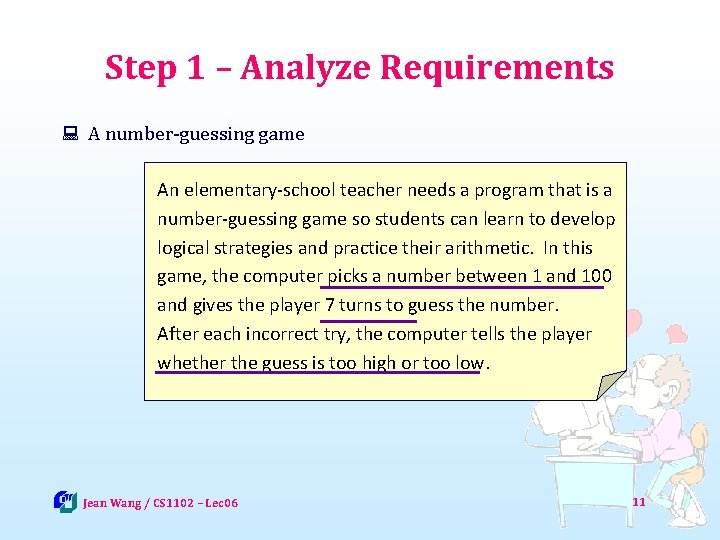
Step 1 – Analyze Requirements : A number-guessing game An elementary-school teacher needs a program that is a number-guessing game so students can learn to develop logical strategies and practice their arithmetic. In this game, the computer picks a number between 1 and 100 and gives the player 7 turns to guess the number. After each incorrect try, the computer tells the player whether the guess is too high or too low. Jean Wang / CS 1102 – Lec 06 11
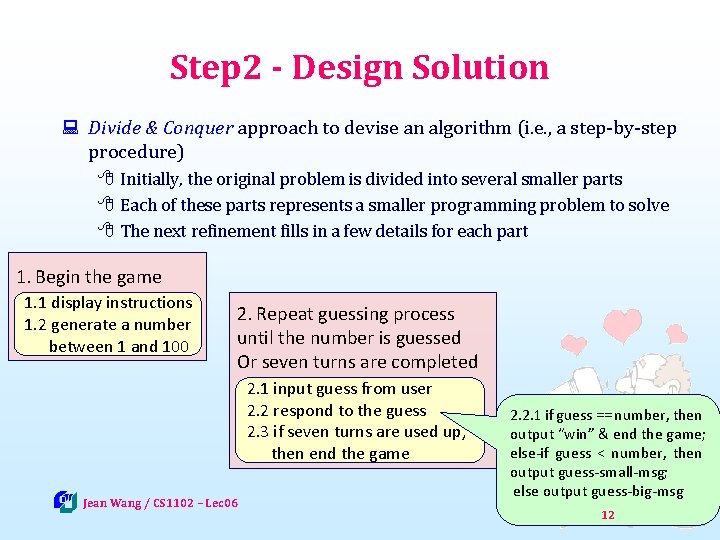
Step 2 - Design Solution : Divide & Conquer approach to devise an algorithm (i. e. , a step-by-step procedure) 8 Initially, the original problem is divided into several smaller parts 8 Each of these parts represents a smaller programming problem to solve 8 The next refinement fills in a few details for each part 1. Begin the game 1. 1 display instructions 1. 2 generate a number between 1 and 100 2. Repeat guessing process until the number is guessed Or seven turns are completed 2. 1 input guess from user 2. 2 respond to the guess 2. 3 if seven turns are used up, then end the game Jean Wang / CS 1102 – Lec 06 2. 2. 1 if guess == number, then output “win” & end the game; else-if guess < number, then output guess-small-msg; else output guess-big-msg 12
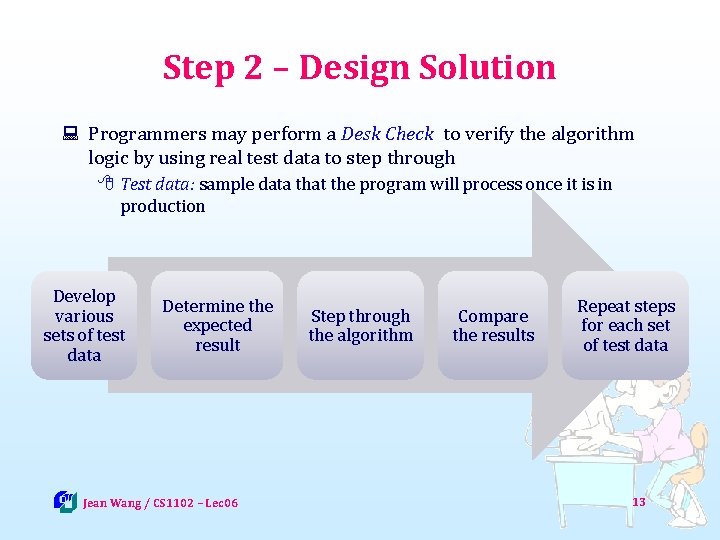
Step 2 – Design Solution : Programmers may perform a Desk Check to verify the algorithm logic by using real test data to step through 8 Test data: sample data that the program will process once it is in production Develop various sets of test data Determine the expected result Jean Wang / CS 1102 – Lec 06 Step through the algorithm Compare the results Repeat steps for each set of test data 13
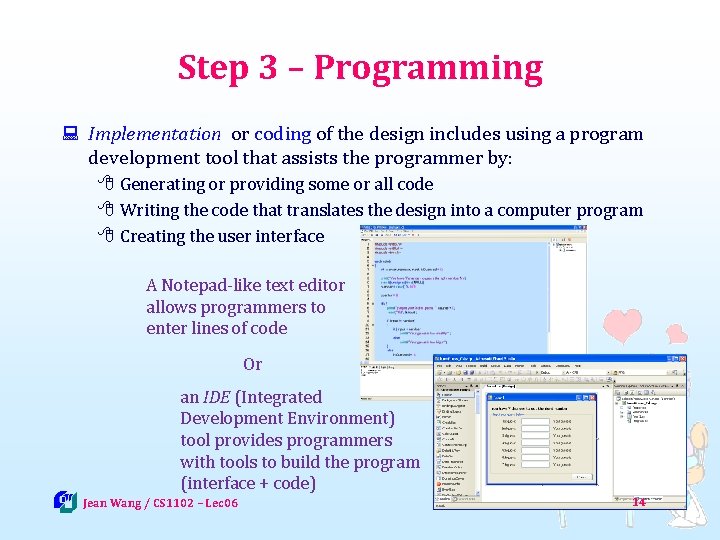
Step 3 – Programming : Implementation or coding of the design includes using a program development tool that assists the programmer by: 8 Generating or providing some or all code 8 Writing the code that translates the design into a computer program 8 Creating the user interface A Notepad-like text editor allows programmers to enter lines of code Or an IDE (Integrated Development Environment) tool provides programmers with tools to build the program (interface + code) Jean Wang / CS 1102 – Lec 06 14
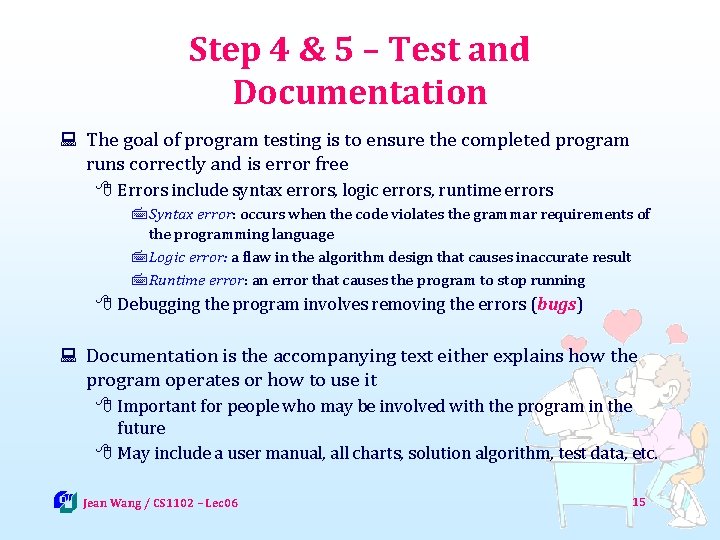
Step 4 & 5 – Test and Documentation : The goal of program testing is to ensure the completed program runs correctly and is error free 8 Errors include syntax errors, logic errors, runtime errors 7 Syntax error: occurs when the code violates the grammar requirements of the programming language 7 Logic error: a flaw in the algorithm design that causes inaccurate result 7 Runtime error: an error that causes the program to stop running 8 Debugging the program involves removing the errors (bugs) : Documentation is the accompanying text either explains how the program operates or how to use it 8 Important for people who may be involved with the program in the future 8 May include a user manual, all charts, solution algorithm, test data, etc. Jean Wang / CS 1102 – Lec 06 15
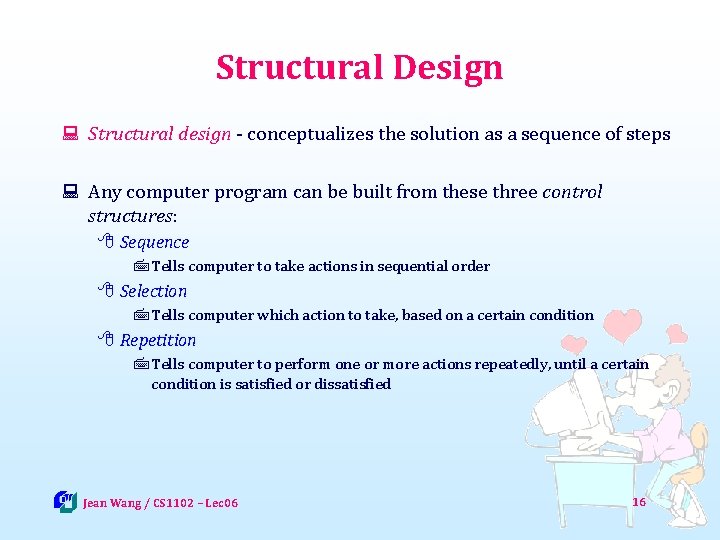
Structural Design : Structural design - conceptualizes the solution as a sequence of steps : Any computer program can be built from these three control structures: 8 Sequence 7 Tells computer to take actions in sequential order 8 Selection 7 Tells computer which action to take, based on a certain condition 8 Repetition 7 Tells computer to perform one or more actions repeatedly, until a certain condition is satisfied or dissatisfied Jean Wang / CS 1102 – Lec 06 16
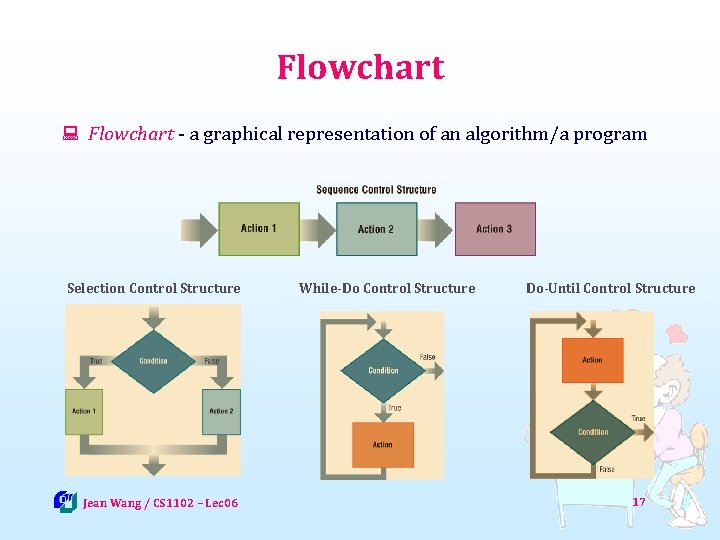
Flowchart : Flowchart - a graphical representation of an algorithm/a program Selection Control Structure Jean Wang / CS 1102 – Lec 06 While-Do Control Structure Do-Until Control Structure 17
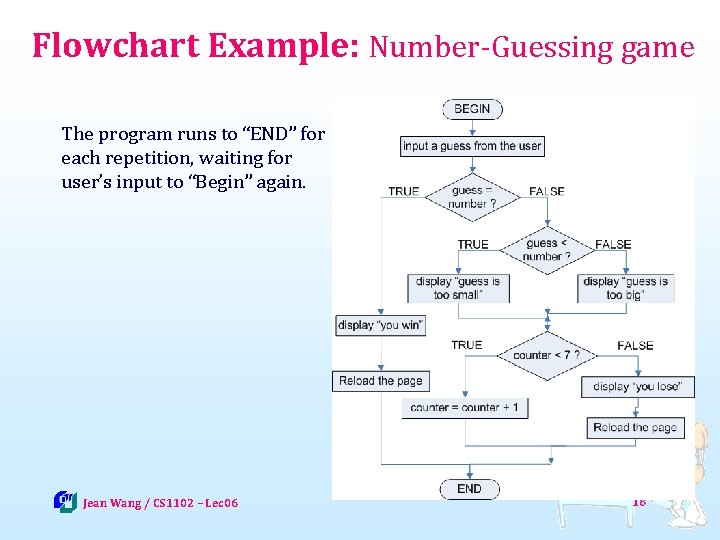
Flowchart Example: Number-Guessing game The program runs to “END” for each repetition, waiting for user’s input to “Begin” again. Jean Wang / CS 1102 – Lec 06 18
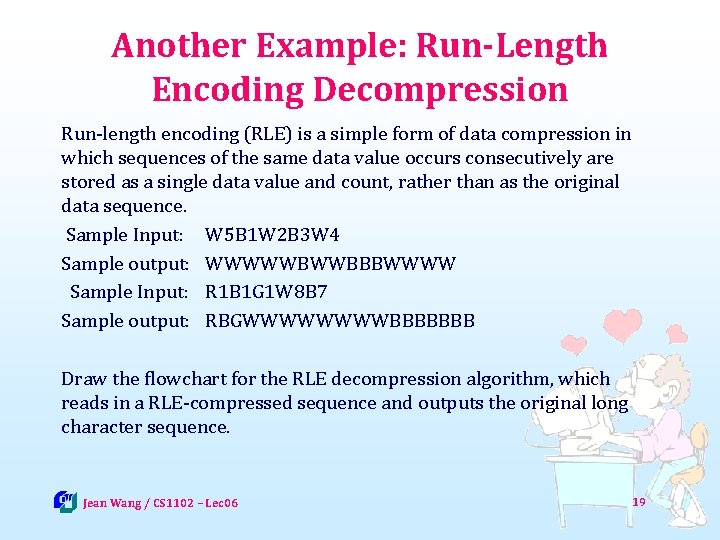
Another Example: Run-Length Encoding Decompression Run-length encoding (RLE) is a simple form of data compression in which sequences of the same data value occurs consecutively are stored as a single data value and count, rather than as the original data sequence. Sample Input: W 5 B 1 W 2 B 3 W 4 Sample output: WWWWWBWWBBBWWWW Sample Input: R 1 B 1 G 1 W 8 B 7 Sample output: RBGWWWWBBBBBBB Draw the flowchart for the RLE decompression algorithm, which reads in a RLE-compressed sequence and outputs the original long character sequence. Jean Wang / CS 1102 – Lec 06 19
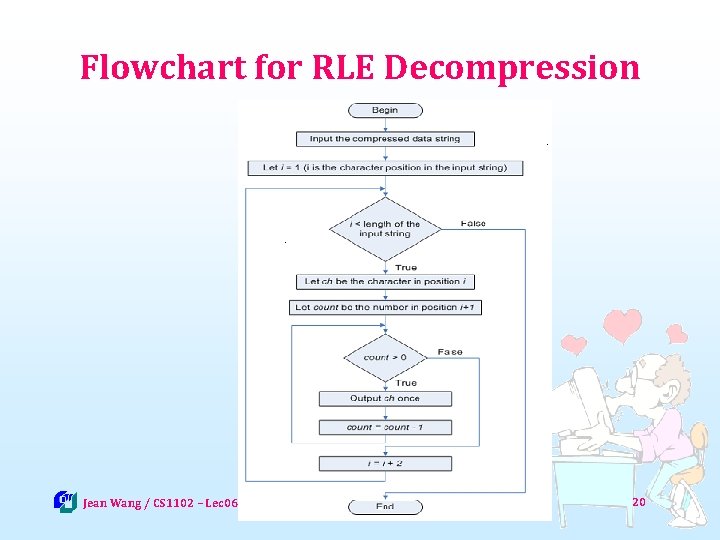
Flowchart for RLE Decompression Jean Wang / CS 1102 – Lec 06 20
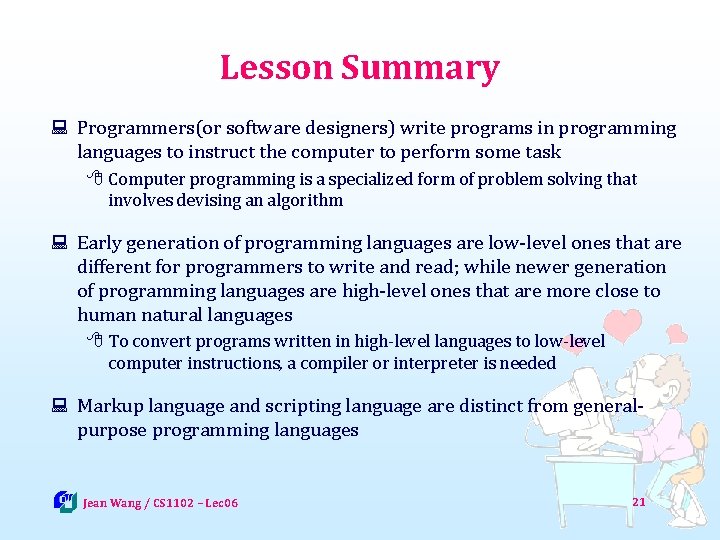
Lesson Summary : Programmers(or software designers) write programs in programming languages to instruct the computer to perform some task 8 Computer programming is a specialized form of problem solving that involves devising an algorithm : Early generation of programming languages are low-level ones that are different for programmers to write and read; while newer generation of programming languages are high-level ones that are more close to human natural languages 8 To convert programs written in high-level languages to low-level computer instructions, a compiler or interpreter is needed : Markup language and scripting language are distinct from generalpurpose programming languages Jean Wang / CS 1102 – Lec 06 21
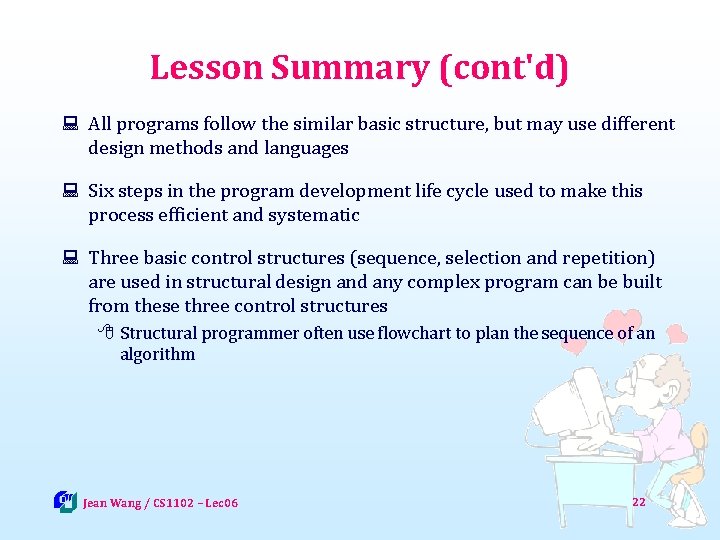
Lesson Summary (cont'd) : All programs follow the similar basic structure, but may use different design methods and languages : Six steps in the program development life cycle used to make this process efficient and systematic : Three basic control structures (sequence, selection and repetition) are used in structural design and any complex program can be built from these three control structures 8 Structural programmer often use flowchart to plan the sequence of an algorithm Jean Wang / CS 1102 – Lec 06 22
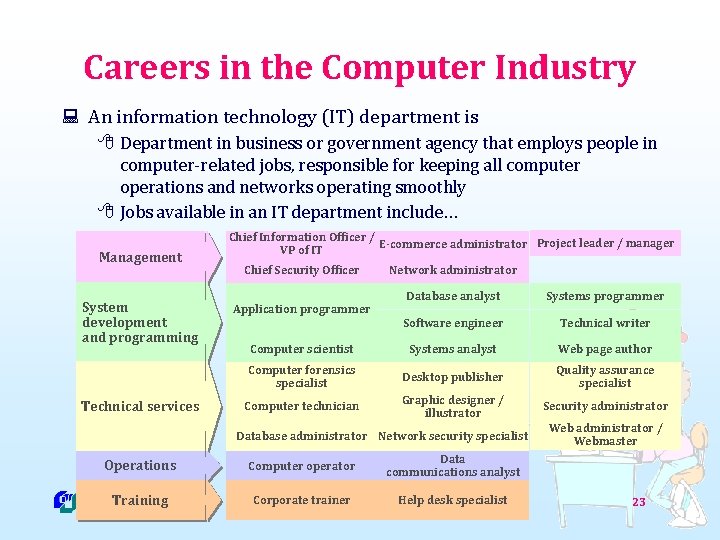
Careers in the Computer Industry : An information technology (IT) department is 8 Department in business or government agency that employs people in computer-related jobs, responsible for keeping all computer operations and networks operating smoothly 8 Jobs available in an IT department include… Management System development and programming Technical services Chief Information Officer / E-commerce administrator Project leader / manager VP of IT Chief Security Officer Network administrator Database analyst Systems programmer Software engineer Technical writer Computer scientist Systems analyst Web page author Computer forensics specialist Desktop publisher Quality assurance specialist Computer technician Graphic designer / illustrator Security administrator Application programmer Database administrator Network security specialist Operations Computer operator Data communications analyst Training Corporate trainer Help desk specialist Web administrator / Webmaster 23
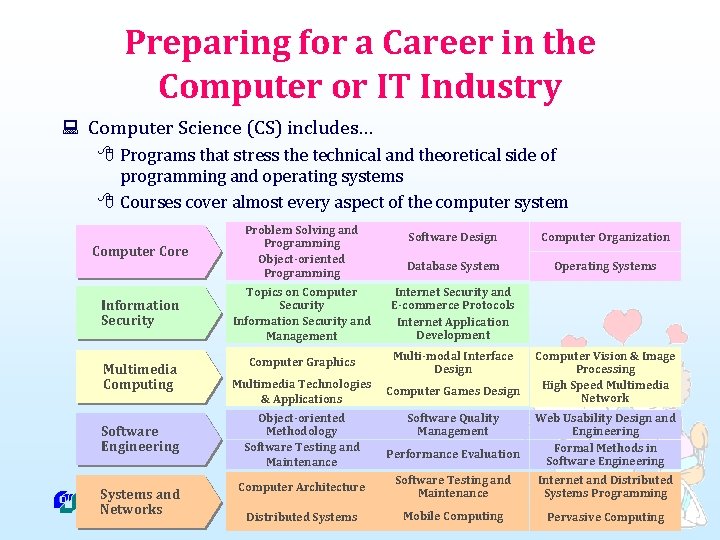
Preparing for a Career in the Computer or IT Industry : Computer Science (CS) includes… 8 Programs that stress the technical and theoretical side of programming and operating systems 8 Courses cover almost every aspect of the computer system Computer Core Information Security Multimedia Computing Software Engineering Systems and Networks Problem Solving and Programming Object-oriented Programming Software Design Computer Organization Database System Operating Systems Topics on Computer Security Information Security and Management Internet Security and E-commerce Protocols Internet Application Development Computer Graphics Multi-modal Interface Design Multimedia Technologies & Applications Computer Games Design Object-oriented Methodology Software Testing and Maintenance Software Quality Management Performance Evaluation Computer Architecture Software Testing and Maintenance Distributed Systems Mobile Computing Computer Vision & Image Processing High Speed Multimedia Network Web Usability Design and Engineering Formal Methods in Software Engineering Internet and Distributed Systems Programming 24 Pervasive Computing
Web res. 1102-404 y res. 785
Comp sci 1102
Amino acids 2 chemsheets answers
Phil 1102
Penjumlahan biner 1012+1102=........2
Real-time systems and programming languages
Cs 421 programming languages and compilers
Real time example of multithreading in java
Programming languages levels
Introduction to programming languages
Plc programming languages
Procedural programming languages
Imperative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Adam doupe cse 340
Types of programming languages
Xenia programming languages
Advantages and disadvantages of programming languages
Mainstream programming languages
Vineeth kashyap
Programing languages
Programming languages
Programming languages