Computer Science 112 Fundamentals of Programming II GUIs
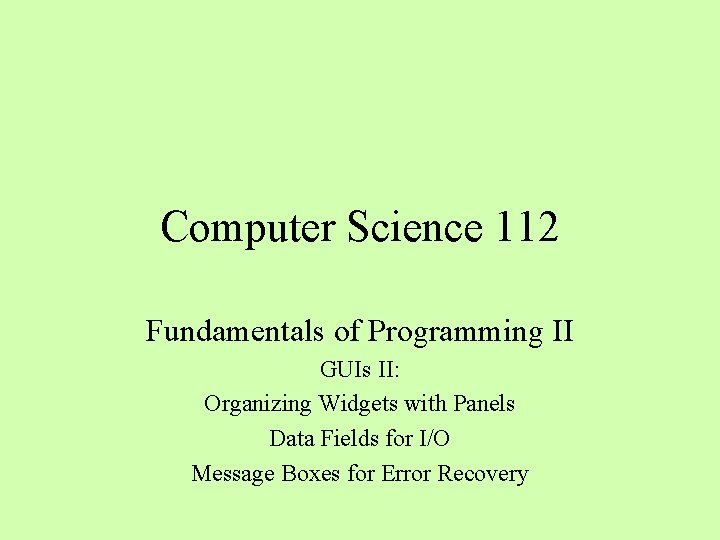
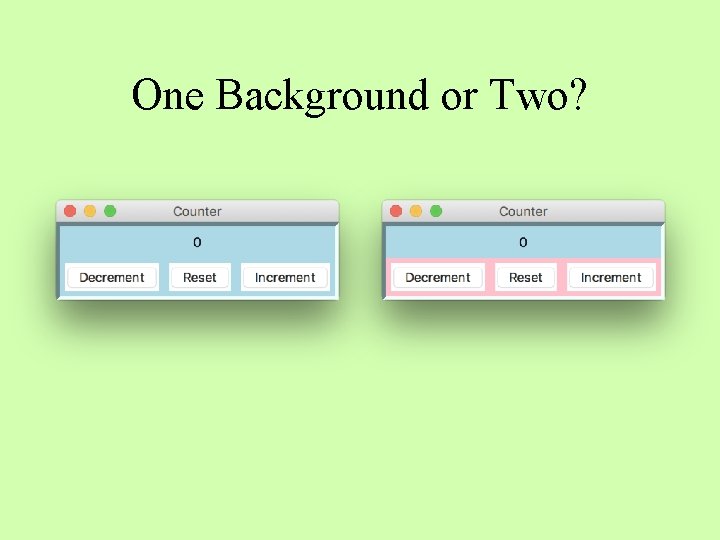
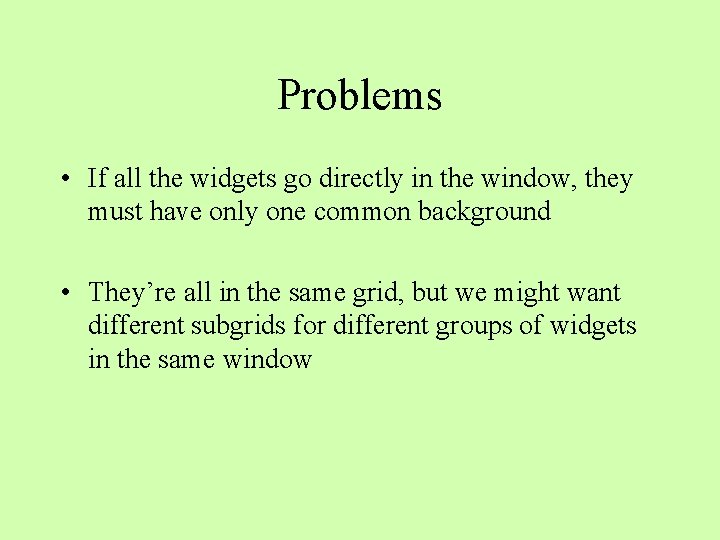
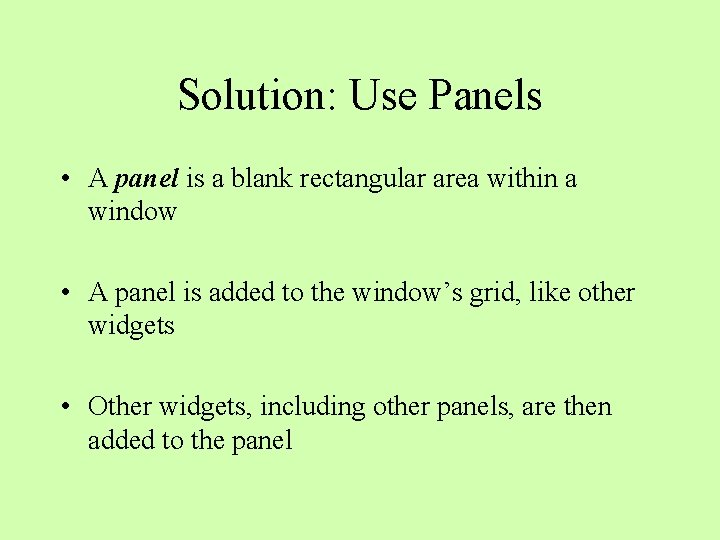
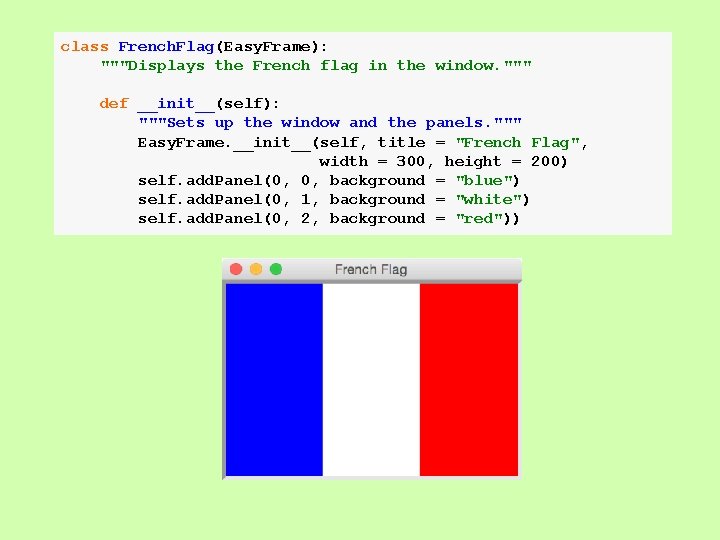
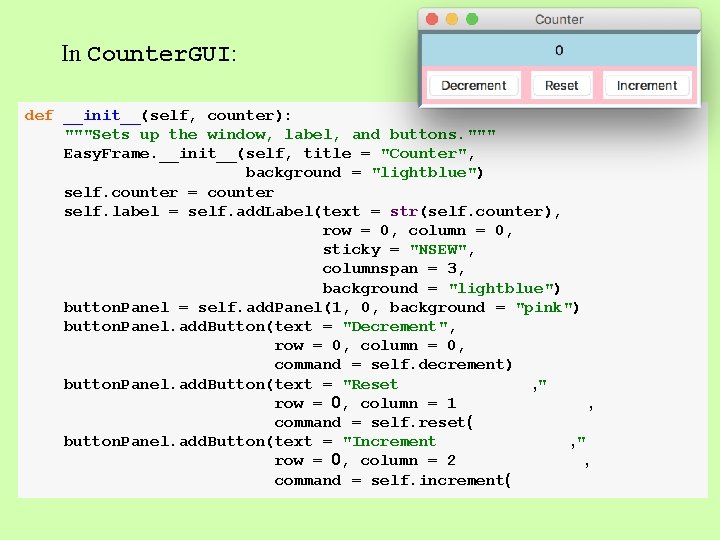
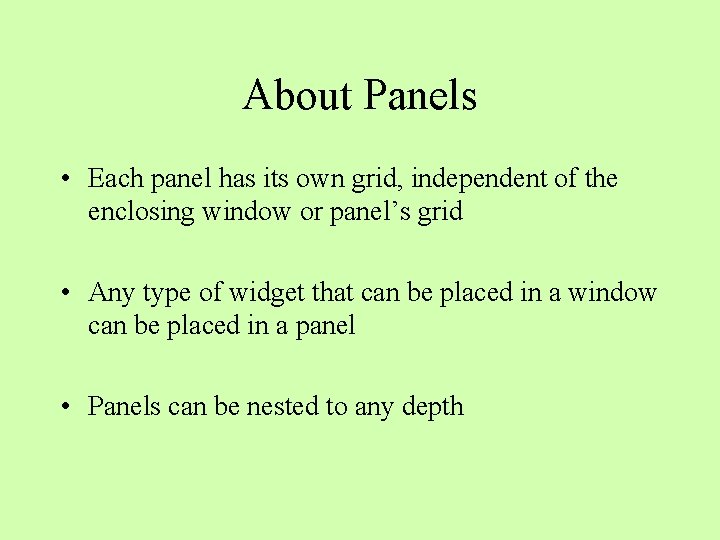
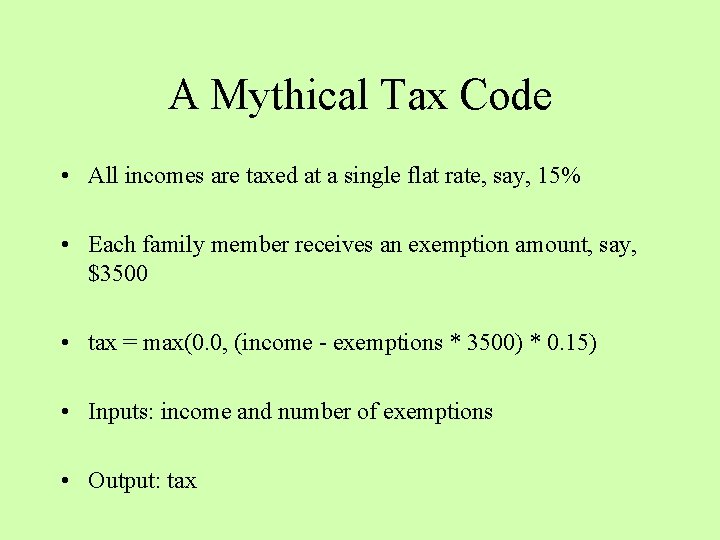
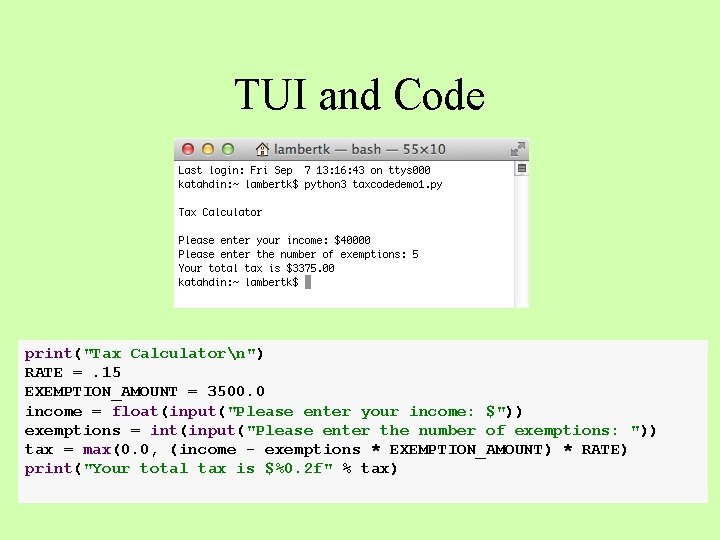
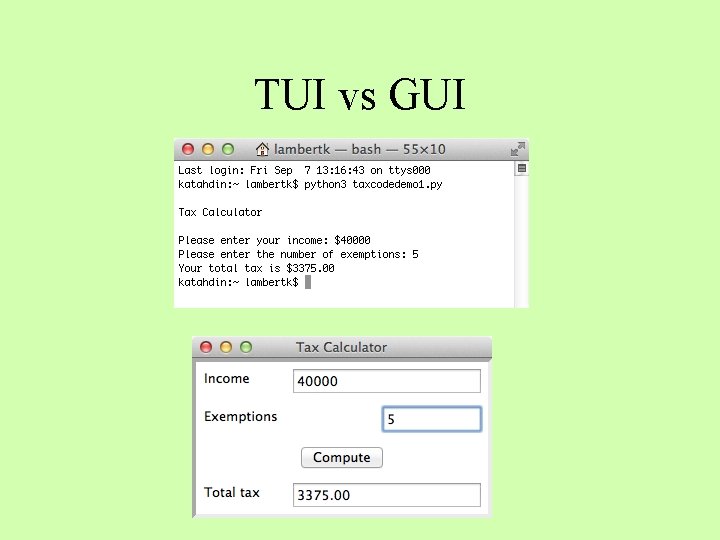
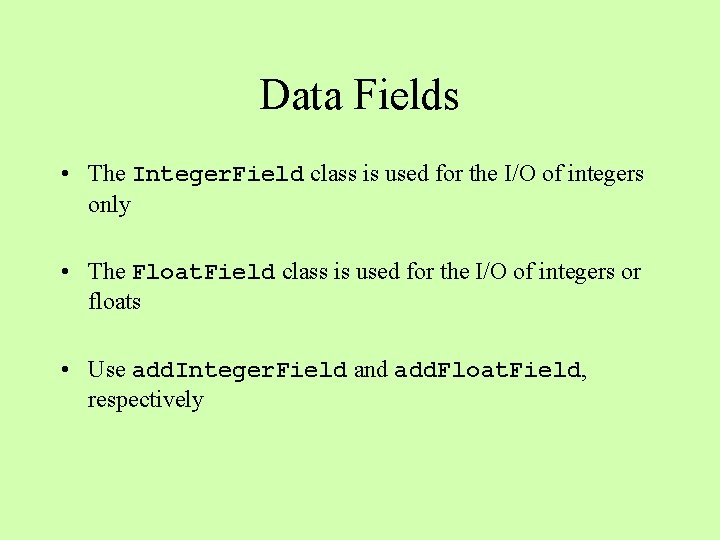
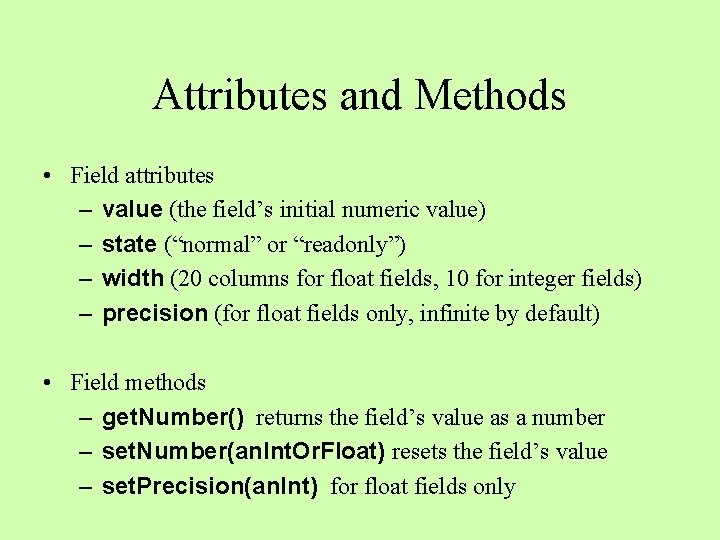
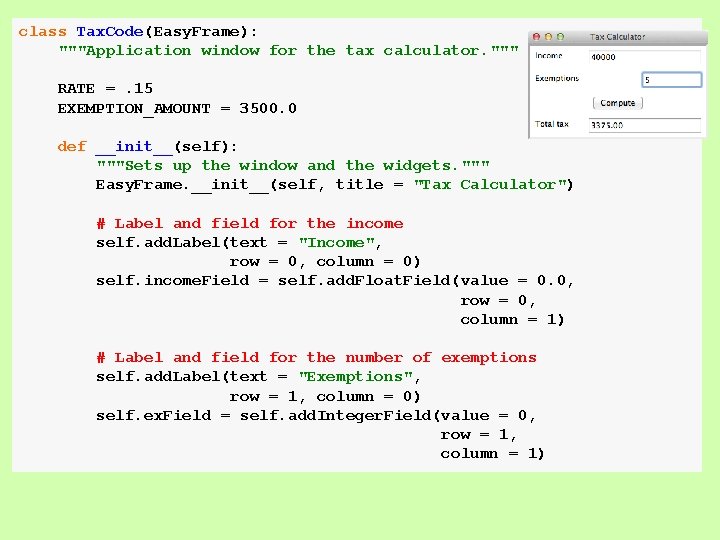
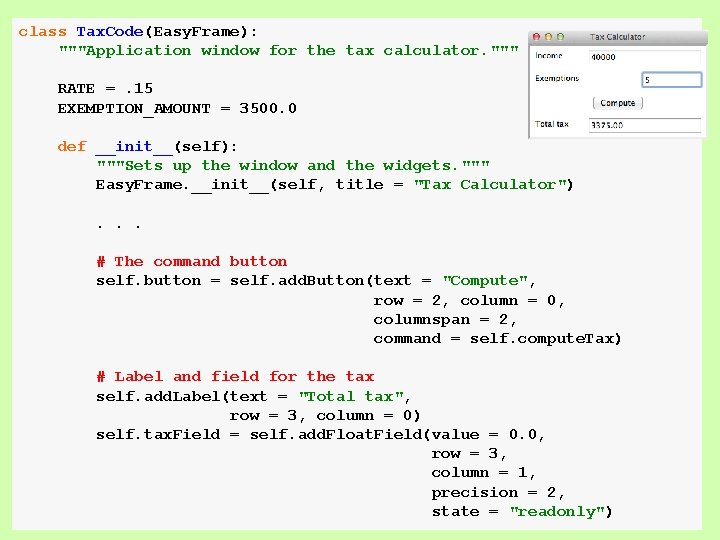
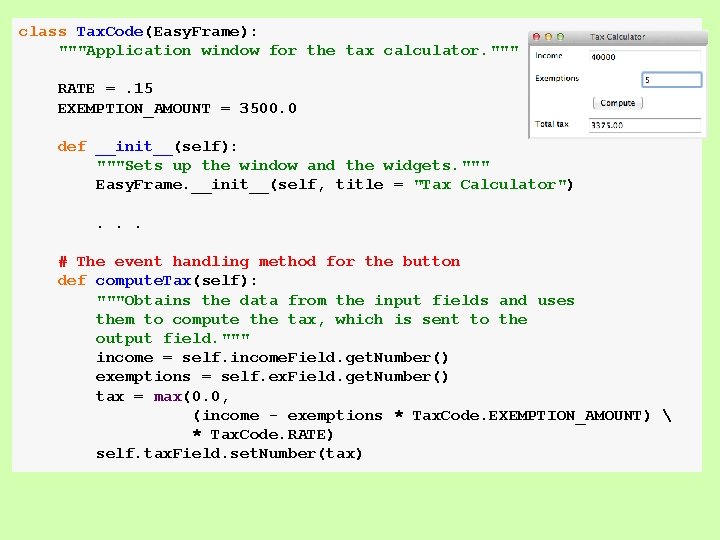
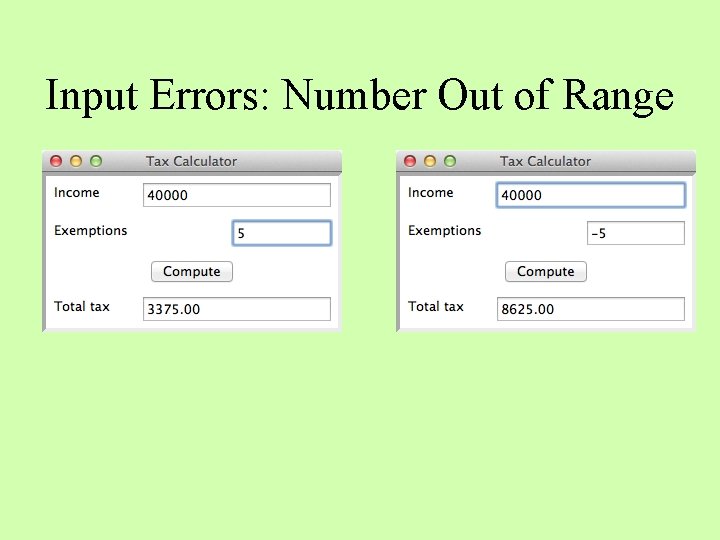
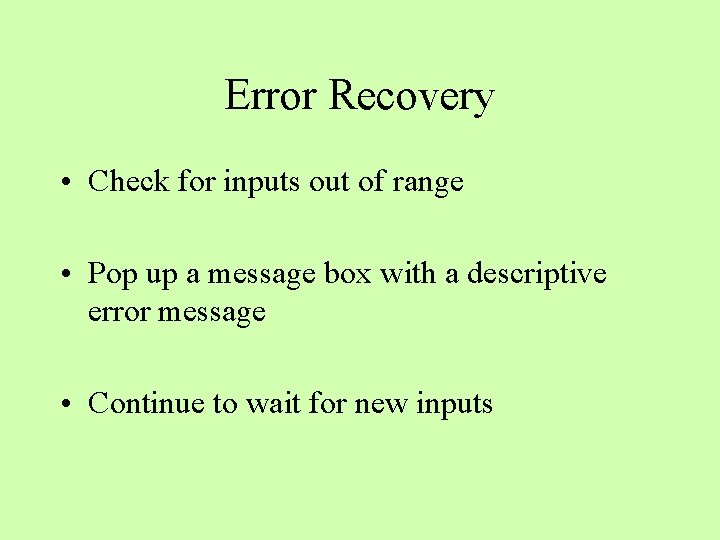
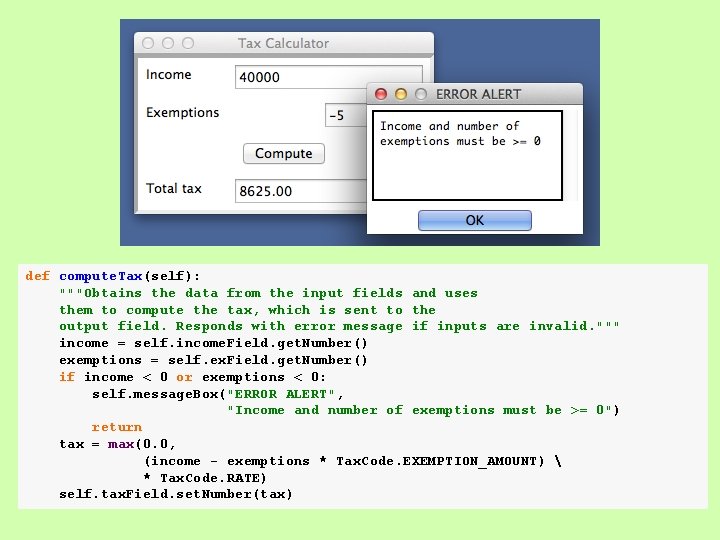
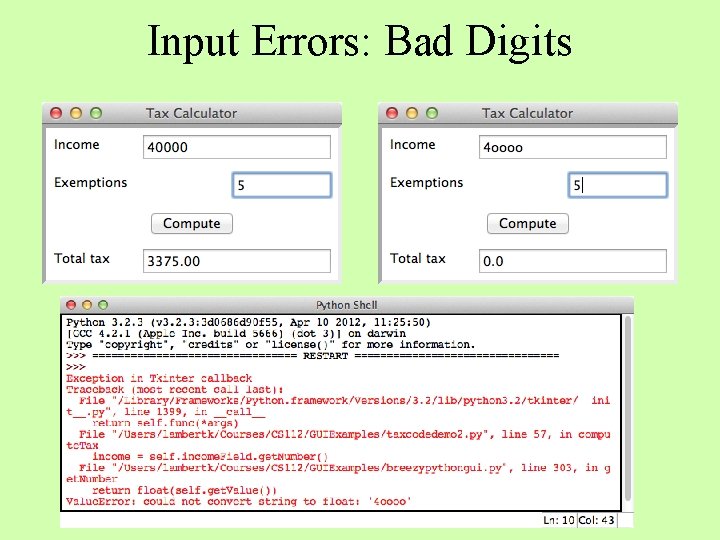
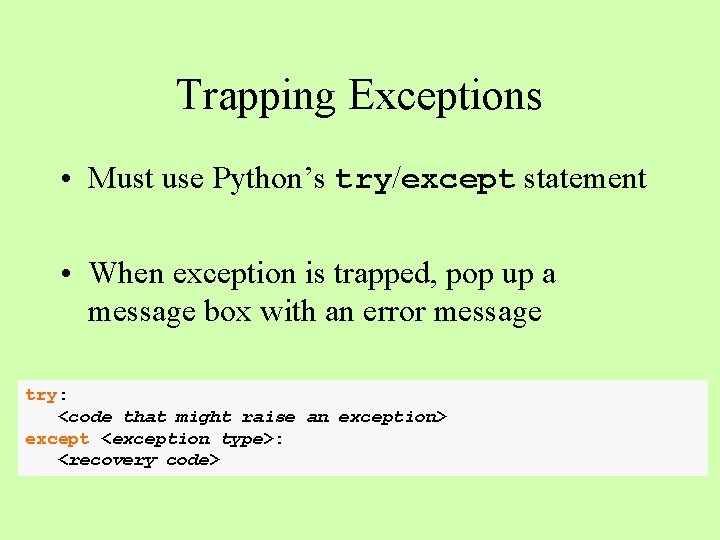
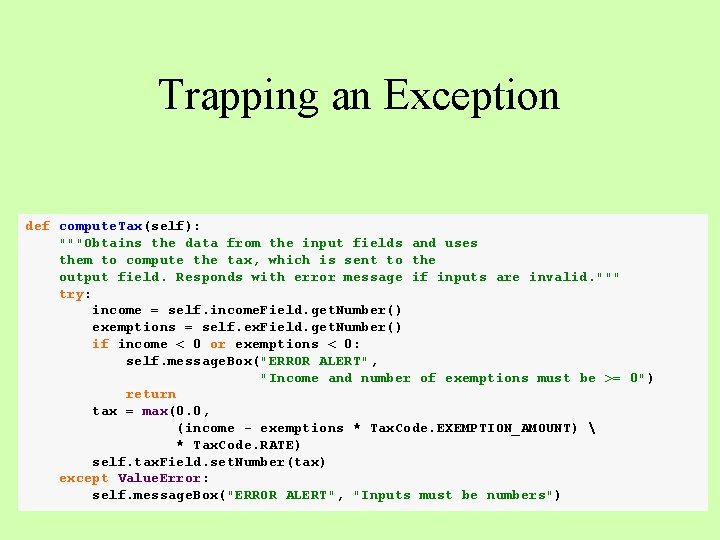
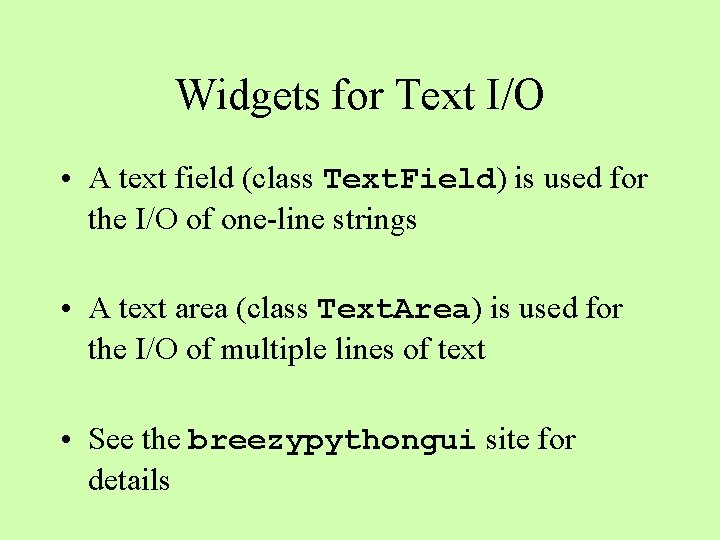
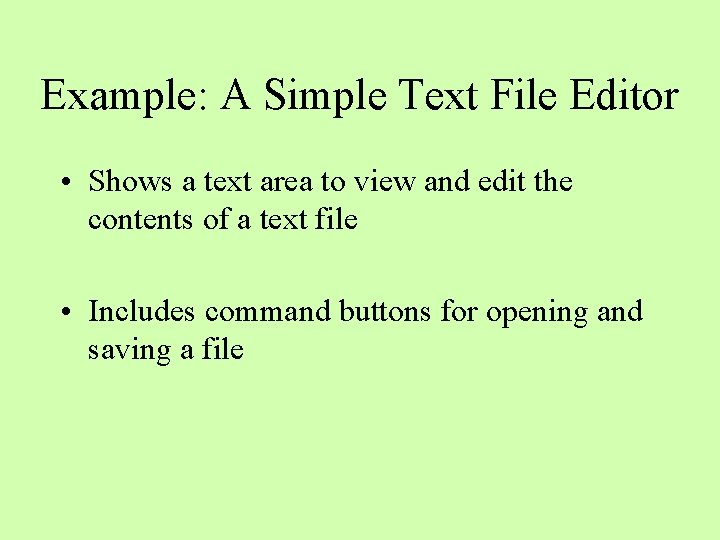
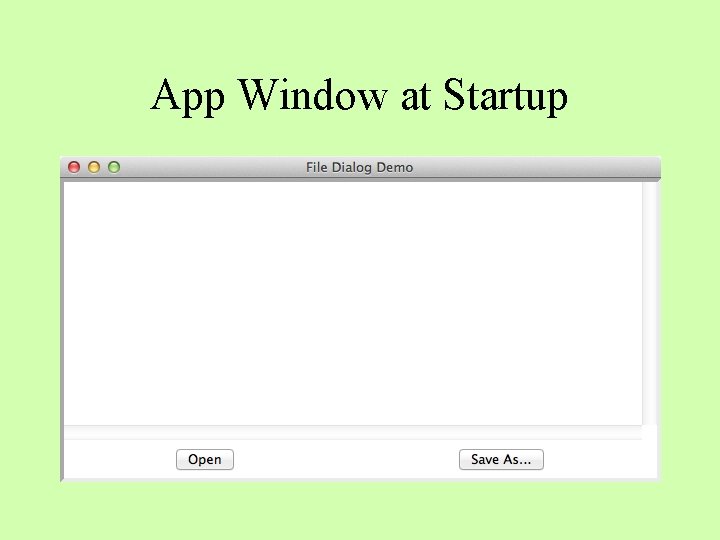
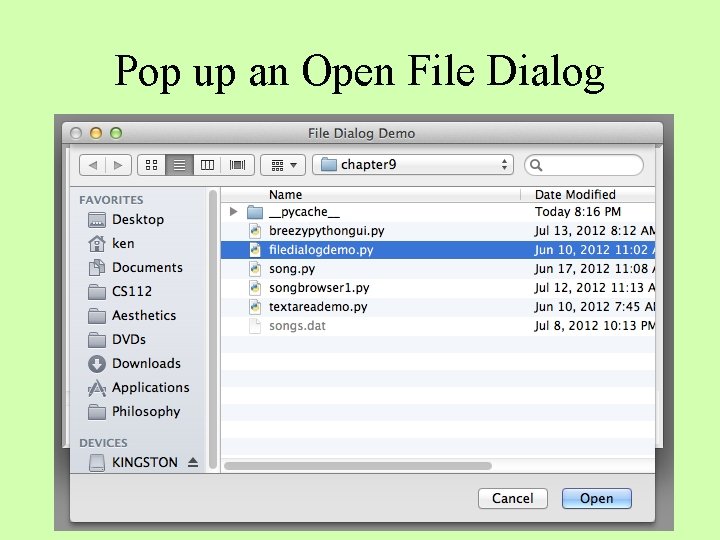
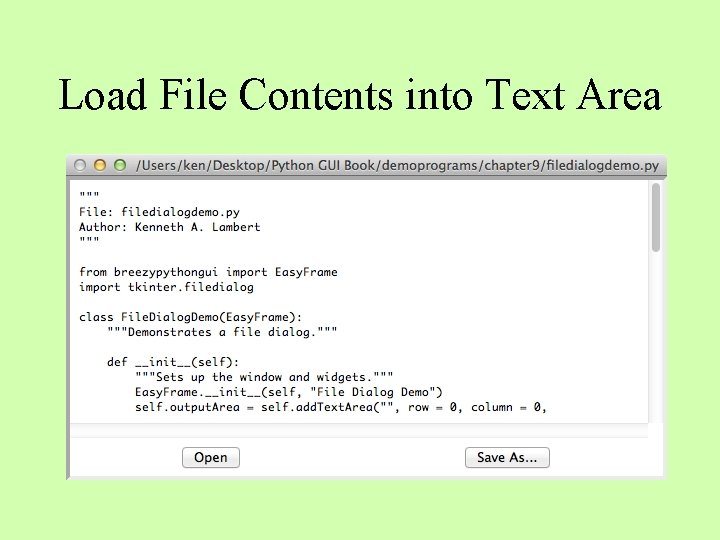
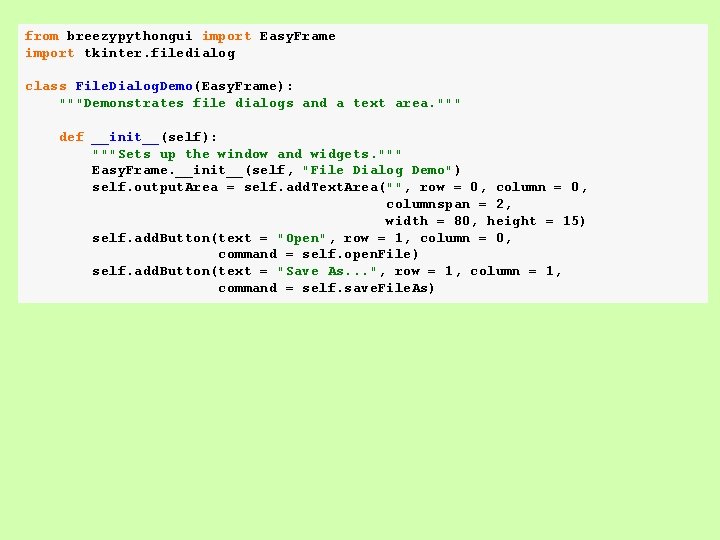
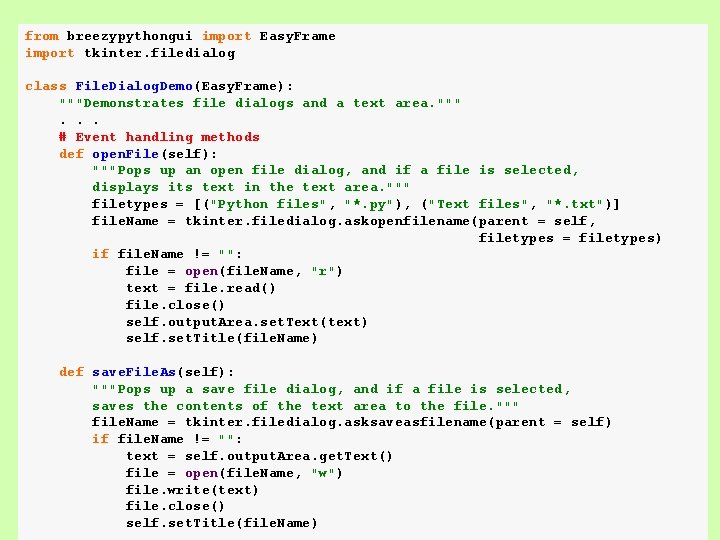
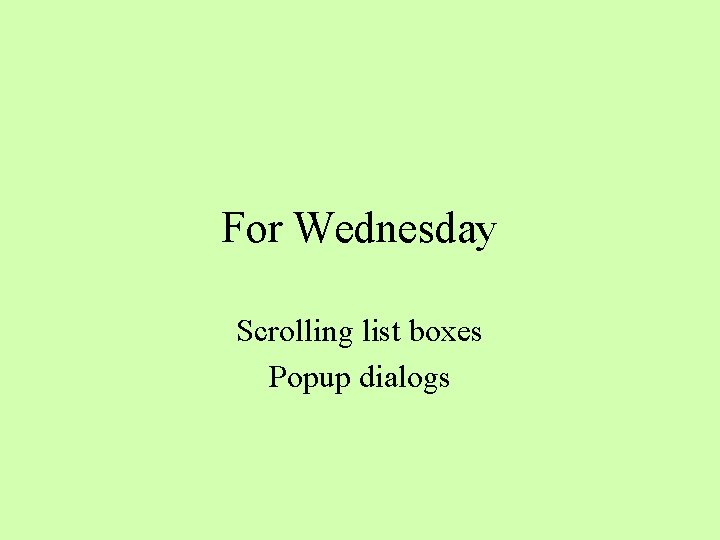
- Slides: 29
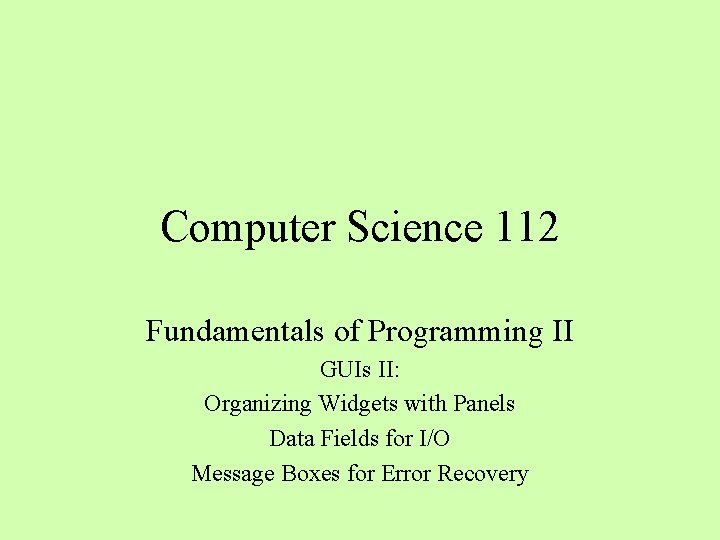
Computer Science 112 Fundamentals of Programming II GUIs II: Organizing Widgets with Panels Data Fields for I/O Message Boxes for Error Recovery
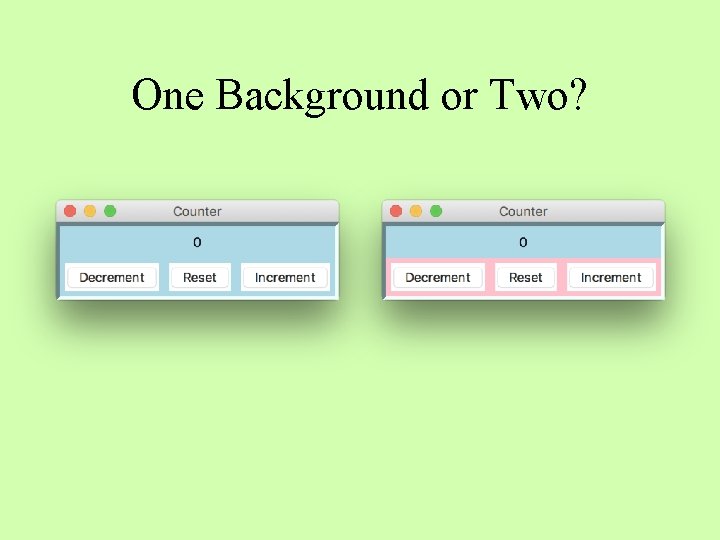
One Background or Two?
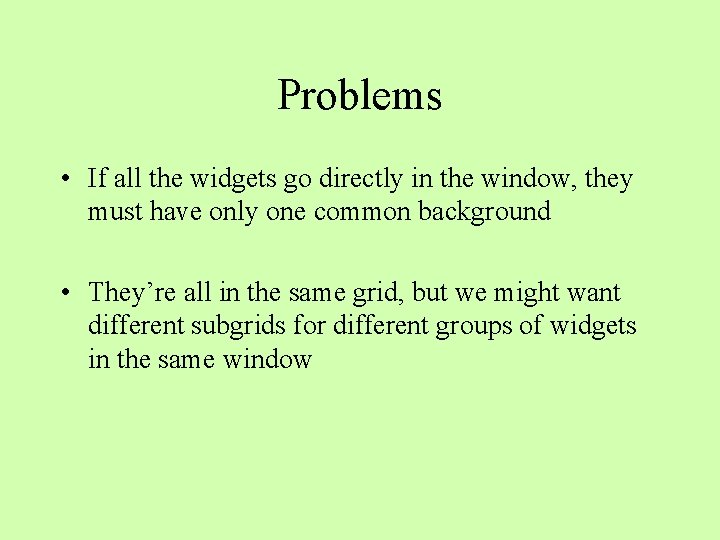
Problems • If all the widgets go directly in the window, they must have only one common background • They’re all in the same grid, but we might want different subgrids for different groups of widgets in the same window
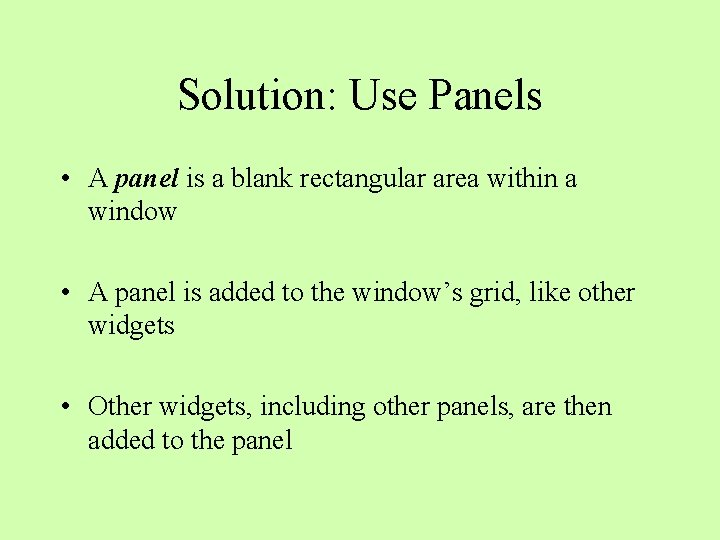
Solution: Use Panels • A panel is a blank rectangular area within a window • A panel is added to the window’s grid, like other widgets • Other widgets, including other panels, are then added to the panel
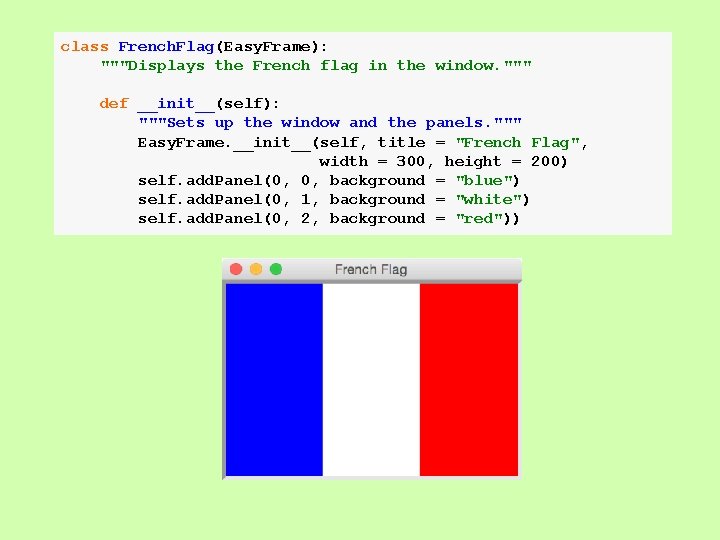
class French. Flag(Easy. Frame): """Displays the French flag in the window. """ def __init__(self): """Sets up the window and the panels. """ Easy. Frame. __init__(self, title = "French Flag", width = 300, height = 200) self. add. Panel(0, 0, background = "blue") self. add. Panel(0, 1, background = "white") self. add. Panel(0, 2, background = "red"))
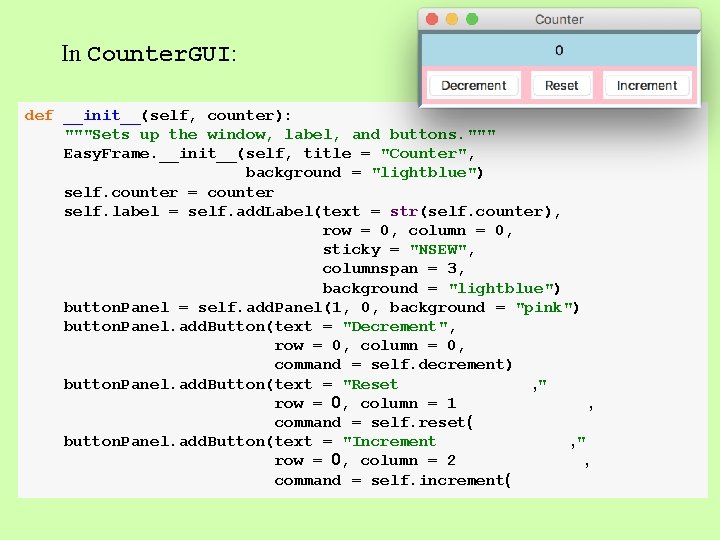
In Counter. GUI: def __init__(self, counter): """Sets up the window, label, and buttons. """ Easy. Frame. __init__(self, title = "Counter", background = "lightblue") self. counter = counter self. label = self. add. Label(text = str(self. counter), row = 0, column = 0, sticky = "NSEW", columnspan = 3, background = "lightblue") button. Panel = self. add. Panel(1, 0, background = "pink") button. Panel. add. Button(text = "Decrement", row = 0, column = 0, command = self. decrement) button. Panel. add. Button(text = "Reset , " row = 0, column = 1 , command = self. reset( button. Panel. add. Button(text = "Increment , " row = 0, column = 2 , command = self. increment(
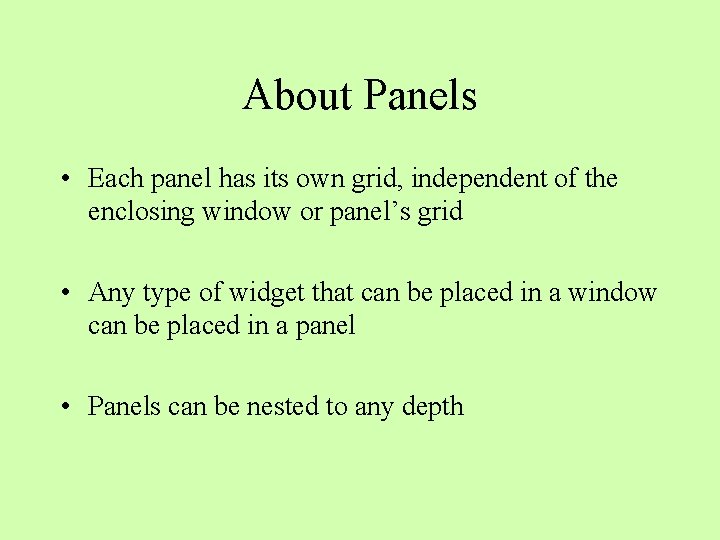
About Panels • Each panel has its own grid, independent of the enclosing window or panel’s grid • Any type of widget that can be placed in a window can be placed in a panel • Panels can be nested to any depth
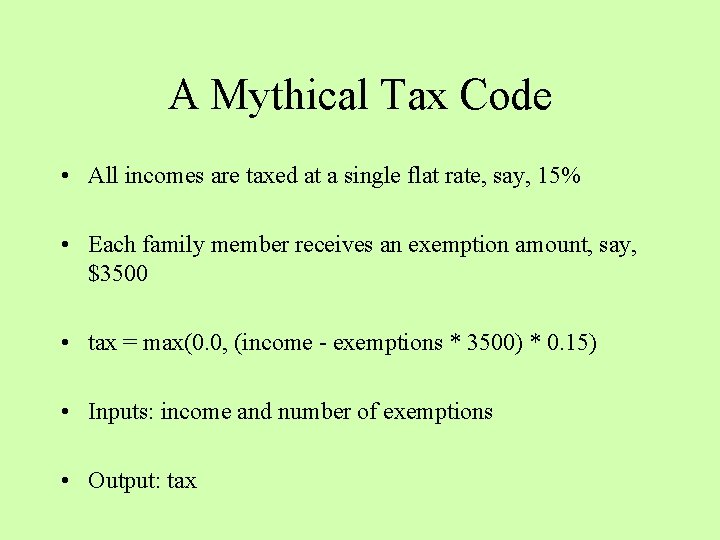
A Mythical Tax Code • All incomes are taxed at a single flat rate, say, 15% • Each family member receives an exemption amount, say, $3500 • tax = max(0. 0, (income - exemptions * 3500) * 0. 15) • Inputs: income and number of exemptions • Output: tax
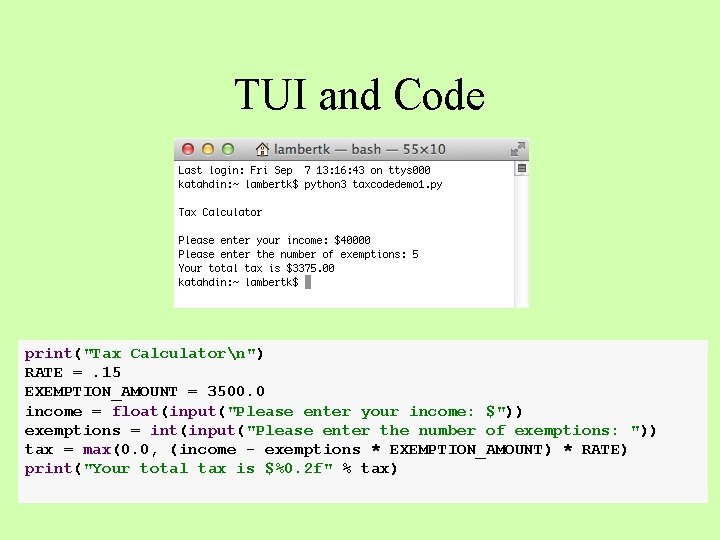
TUI and Code print("Tax Calculatorn") RATE =. 15 EXEMPTION_AMOUNT = 3500. 0 income = float(input("Please enter your income: $")) exemptions = int(input("Please enter the number of exemptions: ")) tax = max(0. 0, (income - exemptions * EXEMPTION_AMOUNT) * RATE) print("Your total tax is $%0. 2 f" % tax)
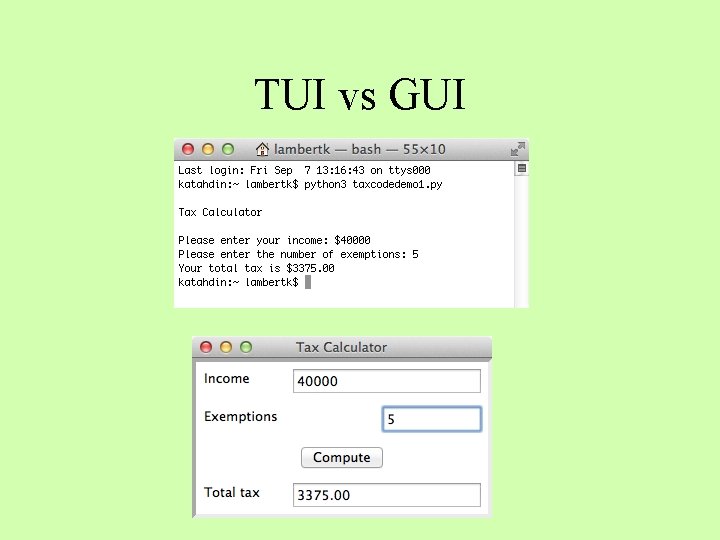
TUI vs GUI
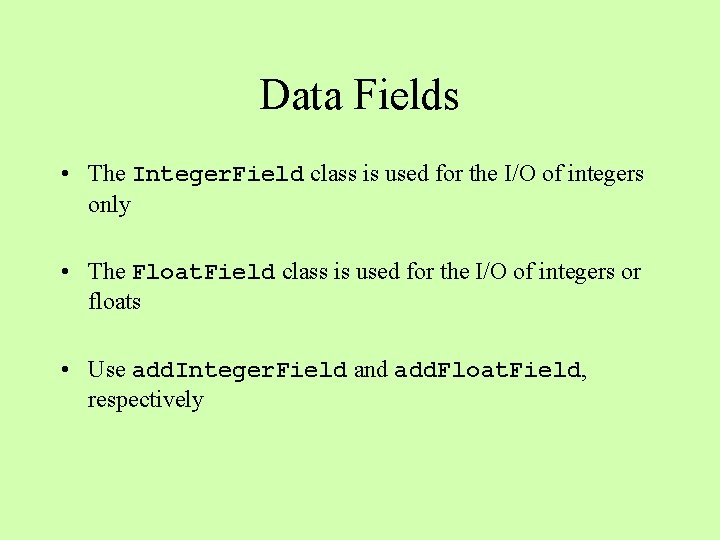
Data Fields • The Integer. Field class is used for the I/O of integers only • The Float. Field class is used for the I/O of integers or floats • Use add. Integer. Field and add. Float. Field, respectively
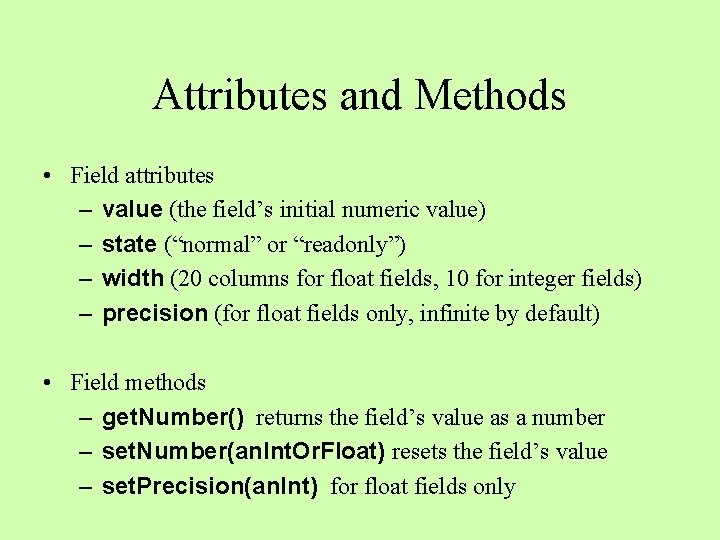
Attributes and Methods • Field attributes – value (the field’s initial numeric value) – state (“normal” or “readonly”) – width (20 columns for float fields, 10 for integer fields) – precision (for float fields only, infinite by default) • Field methods – get. Number() returns the field’s value as a number – set. Number(an. Int. Or. Float) resets the field’s value – set. Precision(an. Int) for float fields only
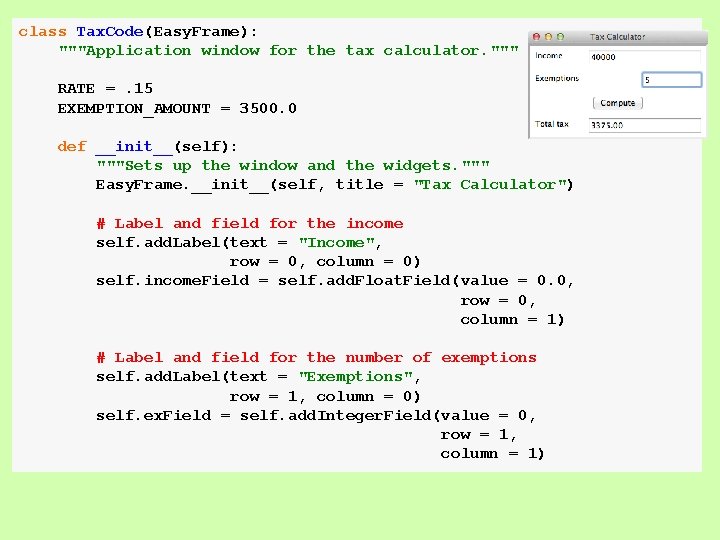
class Tax. Code(Easy. Frame): """Application window for the tax calculator. """ RATE =. 15 EXEMPTION_AMOUNT = 3500. 0 def __init__(self): """Sets up the window and the widgets. """ Easy. Frame. __init__(self, title = "Tax Calculator") # Label and field for the income self. add. Label(text = "Income", row = 0, column = 0) self. income. Field = self. add. Float. Field(value = 0. 0, row = 0, column = 1) # Label and field for the number of exemptions self. add. Label(text = "Exemptions", row = 1, column = 0) self. ex. Field = self. add. Integer. Field(value = 0, row = 1, column = 1)
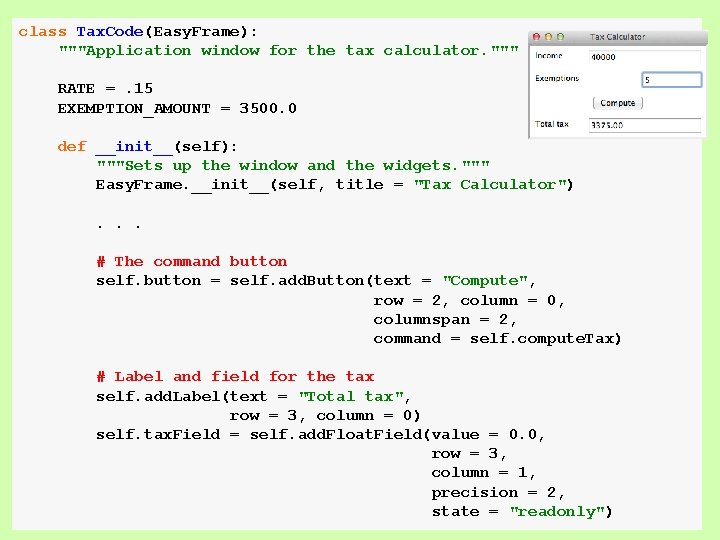
class Tax. Code(Easy. Frame): """Application window for the tax calculator. """ RATE =. 15 EXEMPTION_AMOUNT = 3500. 0 def __init__(self): """Sets up the window and the widgets. """ Easy. Frame. __init__(self, title = "Tax Calculator"). . . # The command button self. button = self. add. Button(text = "Compute", row = 2, column = 0, columnspan = 2, command = self. compute. Tax) # Label and field for the tax self. add. Label(text = "Total tax", row = 3, column = 0) self. tax. Field = self. add. Float. Field(value = 0. 0, row = 3, column = 1, precision = 2, state = "readonly")
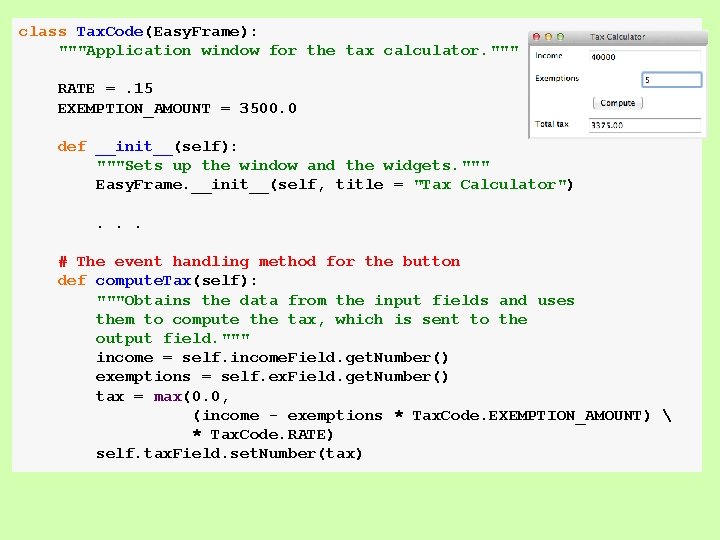
class Tax. Code(Easy. Frame): """Application window for the tax calculator. """ RATE =. 15 EXEMPTION_AMOUNT = 3500. 0 def __init__(self): """Sets up the window and the widgets. """ Easy. Frame. __init__(self, title = "Tax Calculator"). . . # The event handling method for the button def compute. Tax(self): """Obtains the data from the input fields and uses them to compute the tax, which is sent to the output field. """ income = self. income. Field. get. Number() exemptions = self. ex. Field. get. Number() tax = max(0. 0, (income - exemptions * Tax. Code. EXEMPTION_AMOUNT) * Tax. Code. RATE) self. tax. Field. set. Number(tax)
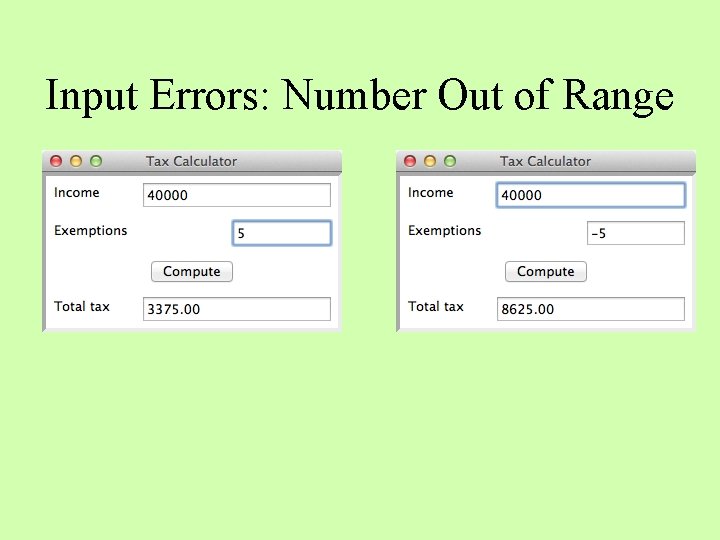
Input Errors: Number Out of Range
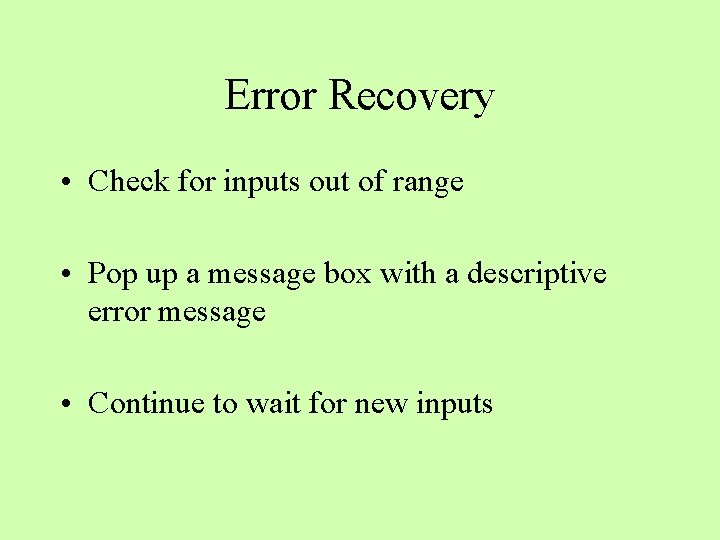
Error Recovery • Check for inputs out of range • Pop up a message box with a descriptive error message • Continue to wait for new inputs
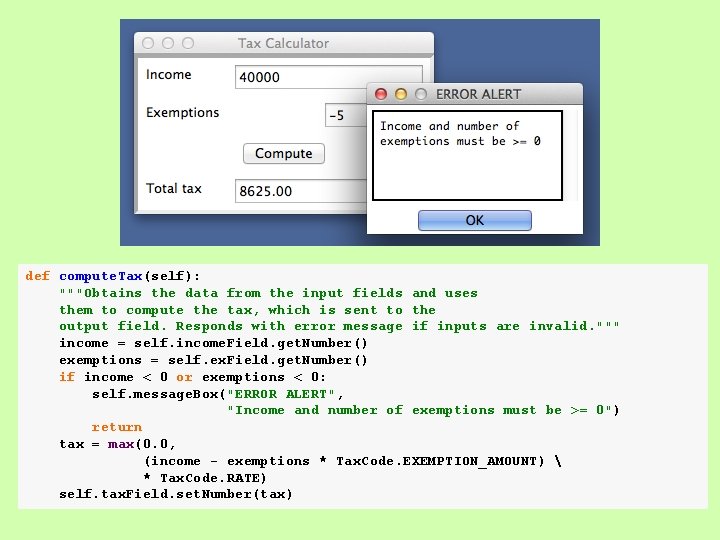
def compute. Tax(self): """Obtains the data from the input fields and uses them to compute the tax, which is sent to the output field. Responds with error message if inputs are invalid. """ income = self. income. Field. get. Number() exemptions = self. ex. Field. get. Number() if income < 0 or exemptions < 0: self. message. Box("ERROR ALERT", "Income and number of exemptions must be >= 0") return tax = max(0. 0, (income - exemptions * Tax. Code. EXEMPTION_AMOUNT) * Tax. Code. RATE) self. tax. Field. set. Number(tax)
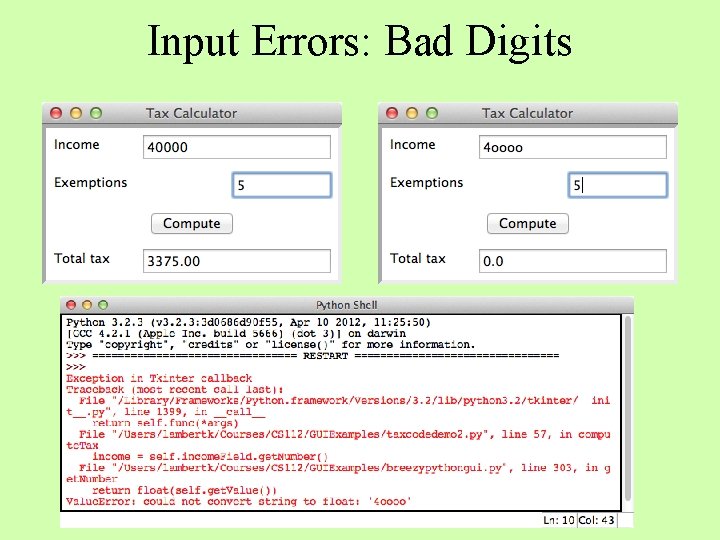
Input Errors: Bad Digits
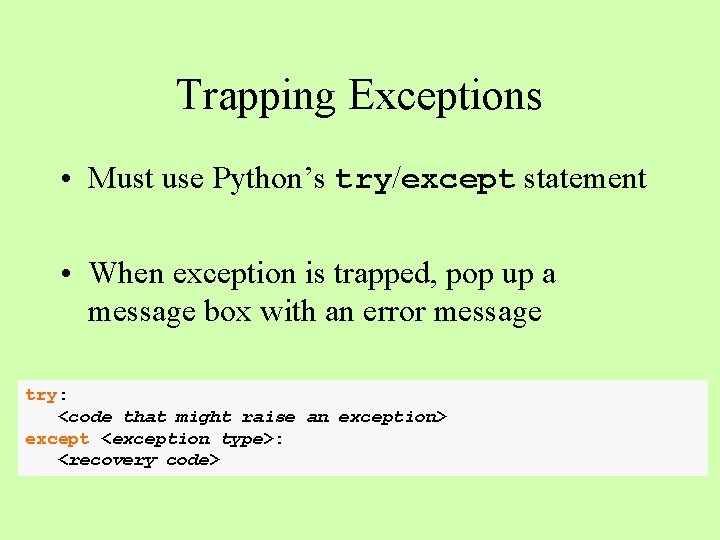
Trapping Exceptions • Must use Python’s try/except statement • When exception is trapped, pop up a message box with an error message try: <code that might raise an exception> except <exception type>: <recovery code>
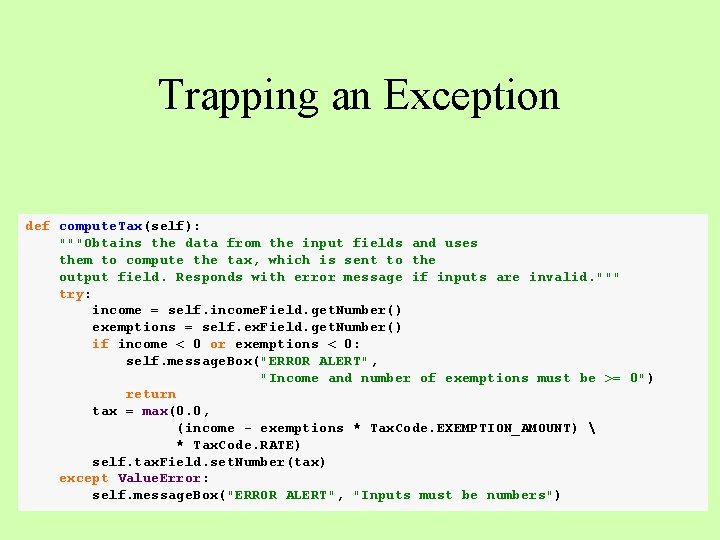
Trapping an Exception def compute. Tax(self): """Obtains the data from the input fields and uses them to compute the tax, which is sent to the output field. Responds with error message if inputs are invalid. """ try: income = self. income. Field. get. Number() exemptions = self. ex. Field. get. Number() if income < 0 or exemptions < 0: self. message. Box("ERROR ALERT", "Income and number of exemptions must be >= 0") return tax = max(0. 0, (income - exemptions * Tax. Code. EXEMPTION_AMOUNT) * Tax. Code. RATE) self. tax. Field. set. Number(tax) except Value. Error: self. message. Box("ERROR ALERT", "Inputs must be numbers")
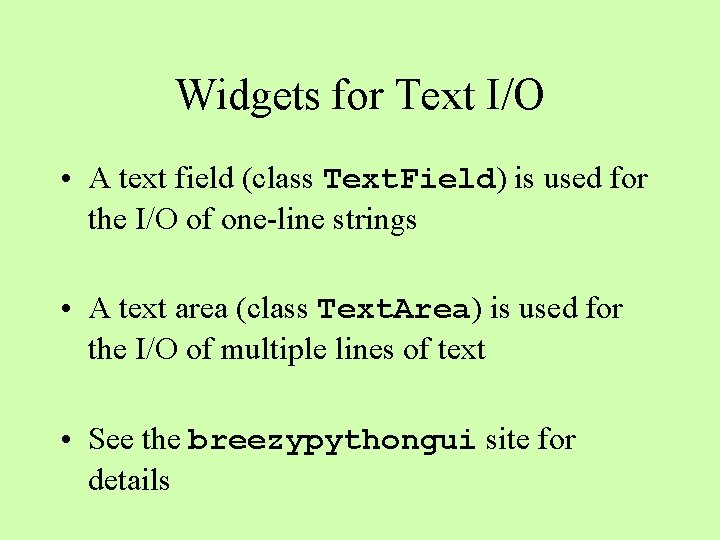
Widgets for Text I/O • A text field (class Text. Field) is used for the I/O of one-line strings • A text area (class Text. Area) is used for the I/O of multiple lines of text • See the breezypythongui site for details
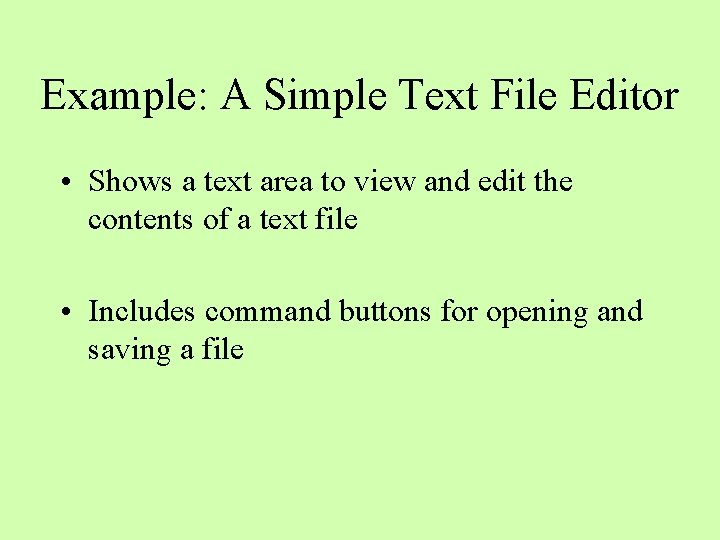
Example: A Simple Text File Editor • Shows a text area to view and edit the contents of a text file • Includes command buttons for opening and saving a file
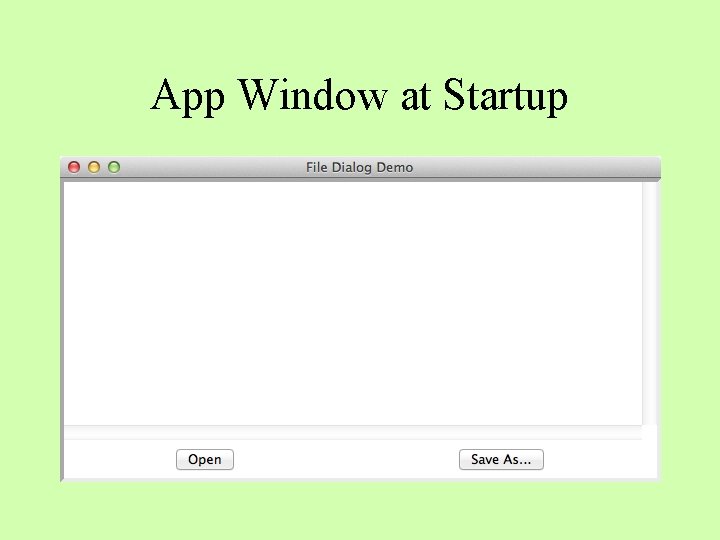
App Window at Startup
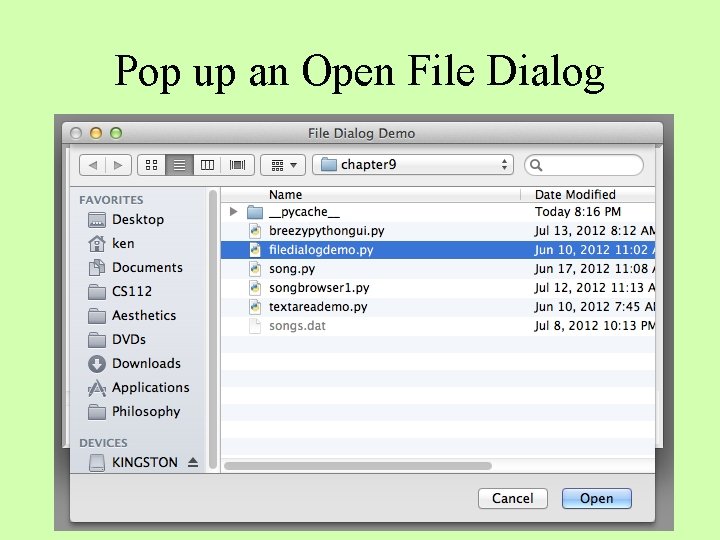
Pop up an Open File Dialog
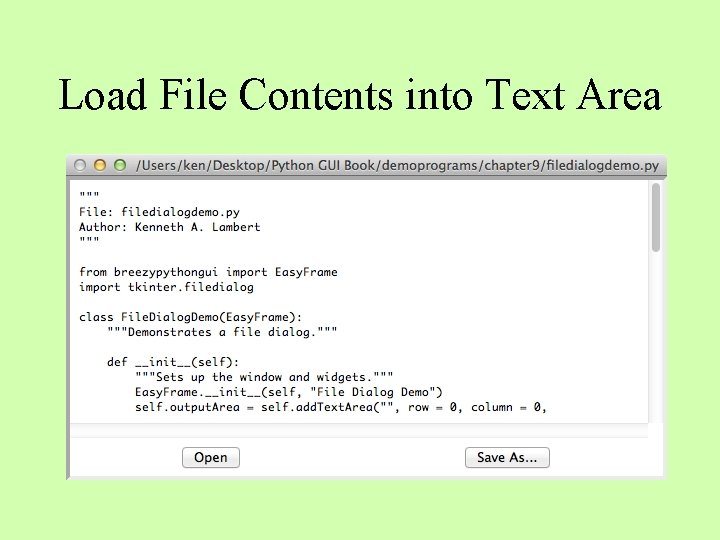
Load File Contents into Text Area
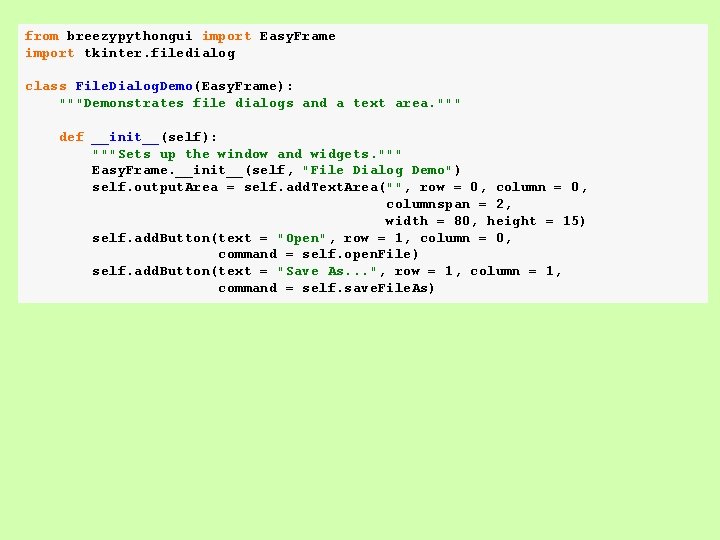
from breezypythongui import Easy. Frame import tkinter. filedialog class File. Dialog. Demo(Easy. Frame): """Demonstrates file dialogs and a text area. """ def __init__(self): """Sets up the window and widgets. """ Easy. Frame. __init__(self, "File Dialog Demo") self. output. Area = self. add. Text. Area("", row = 0, columnspan = 2, width = 80, height = 15) self. add. Button(text = "Open", row = 1, column = 0, command = self. open. File) self. add. Button(text = "Save As. . . ", row = 1, column = 1, command = self. save. File. As)
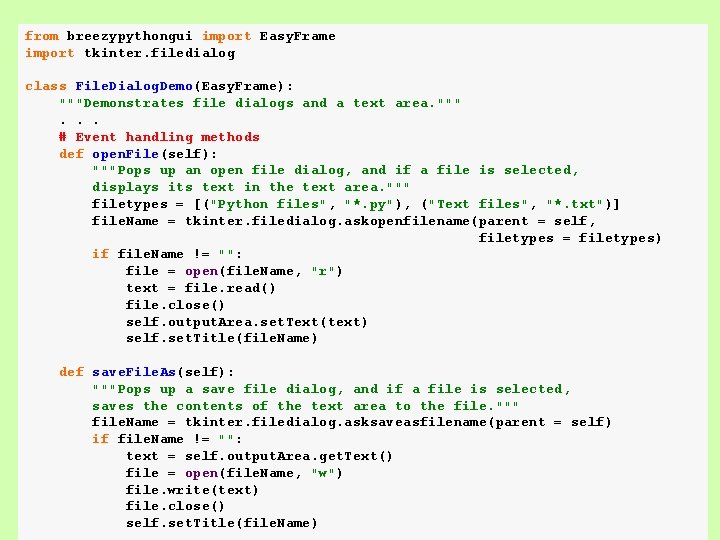
from breezypythongui import Easy. Frame import tkinter. filedialog class File. Dialog. Demo(Easy. Frame): """Demonstrates file dialogs and a text area. """. . . # Event handling methods def open. File(self): """Pops up an open file dialog, and if a file is selected, displays its text in the text area. """ filetypes = [("Python files", "*. py"), ("Text files", "*. txt")] file. Name = tkinter. filedialog. askopenfilename(parent = self, filetypes = filetypes) if file. Name != "": file = open(file. Name, "r") text = file. read() file. close() self. output. Area. set. Text(text) self. set. Title(file. Name) def save. File. As(self): """Pops up a save file dialog, and if a file is selected, saves the contents of the text area to the file. """ file. Name = tkinter. filedialog. asksaveasfilename(parent = self) if file. Name != "": text = self. output. Area. get. Text() file = open(file. Name, "w") file. write(text) file. close() self. set. Title(file. Name)
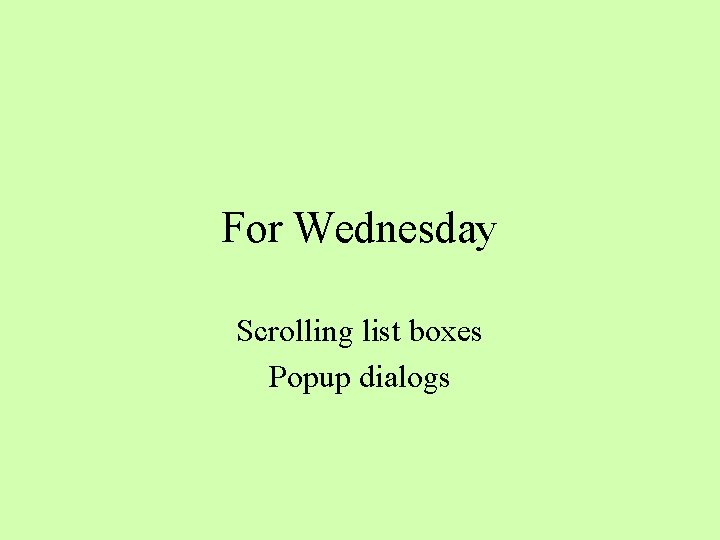
For Wednesday Scrolling list boxes Popup dialogs
Sin 112 1/2
Gui graphics
Characteristics of web user interface
Programming fundamentals 1
American computer science league practice problems
Python programming an introduction to computer science
Functional programming fundamentals
Cs 1101 final exam
Fundamentals of functional programming language
Fundamentals of patrolling
Cs-1101
Cs 1101 programming fundamentals
Cs 1101 programming fundamentals
My favourite subject computer
Chapter 6 fingerprints
The fundamentals of political science research 2nd edition
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
What is system programing
Linear vs integer programming
Definisi linear
Jk flip flop
Logic and computer design fundamentals
Logic and computer design fundamentals
Logic and computer design fundamentals
Orthogonal projection in computer graphics
Sierpinski gasket in computer graphics
Chapter 1 computer fundamentals
Fundamentals of computer design
Computer fundamentals anita goel