COMP 345 Advanced Program Design with C 1
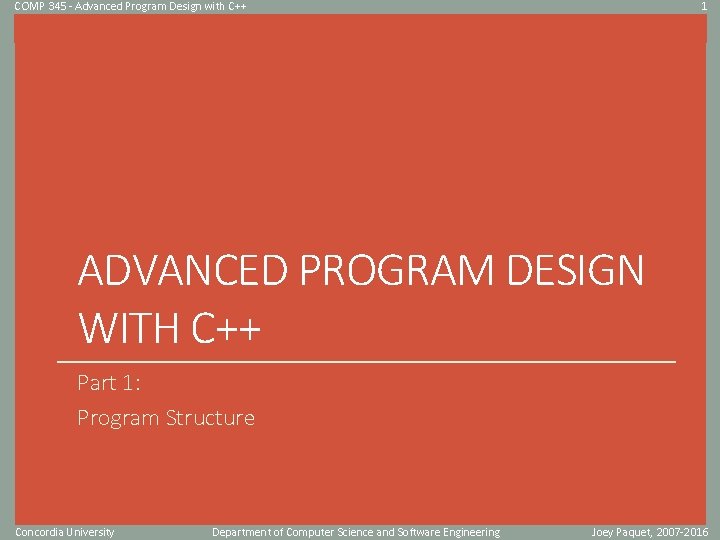
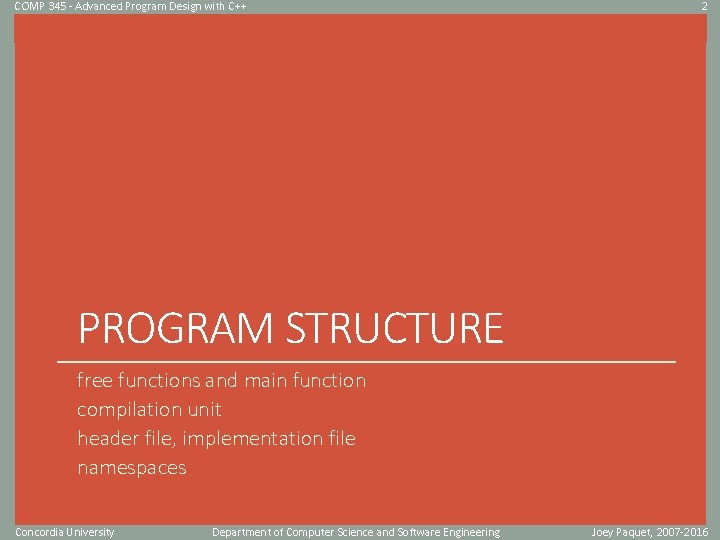
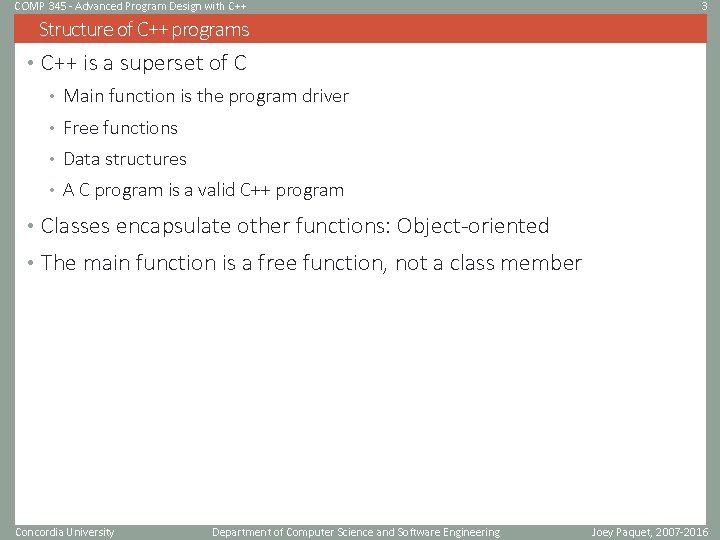
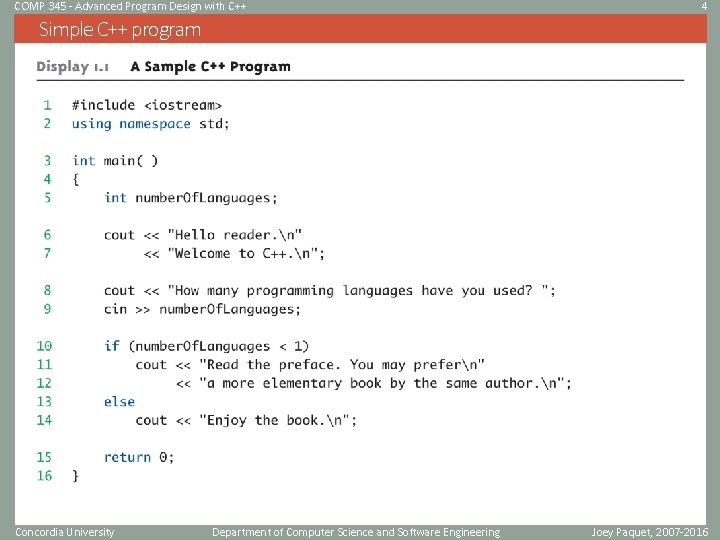
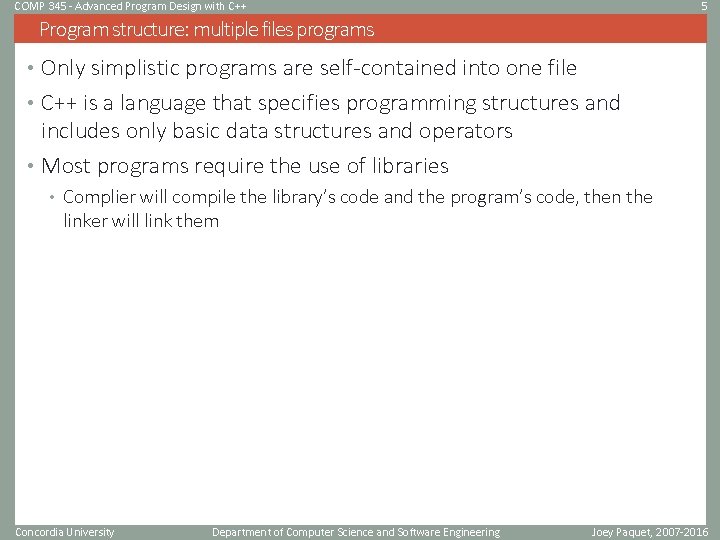
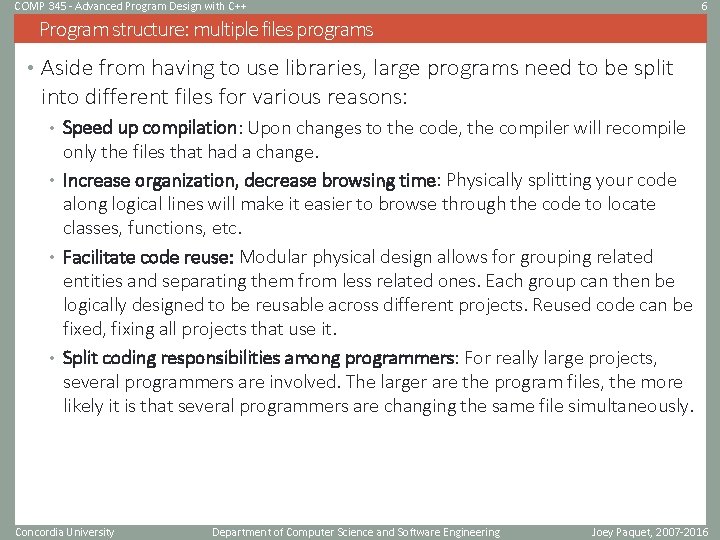
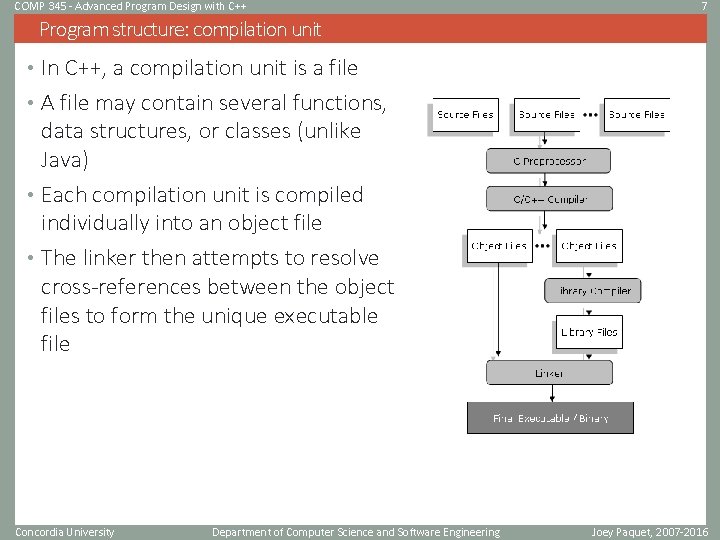
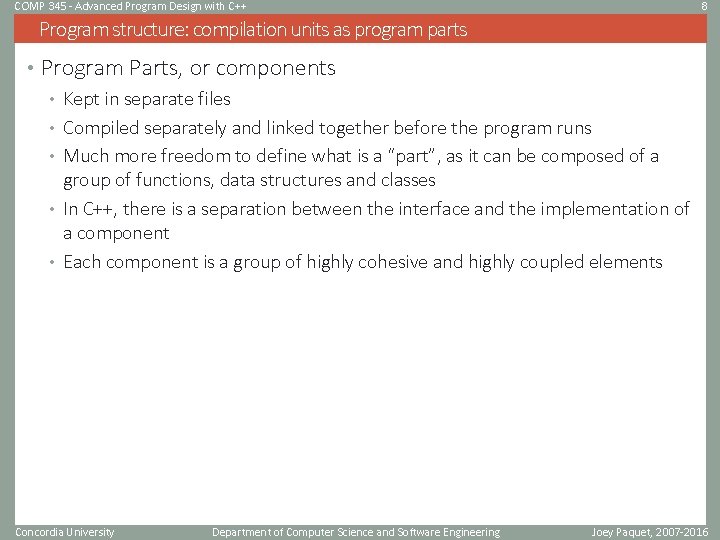
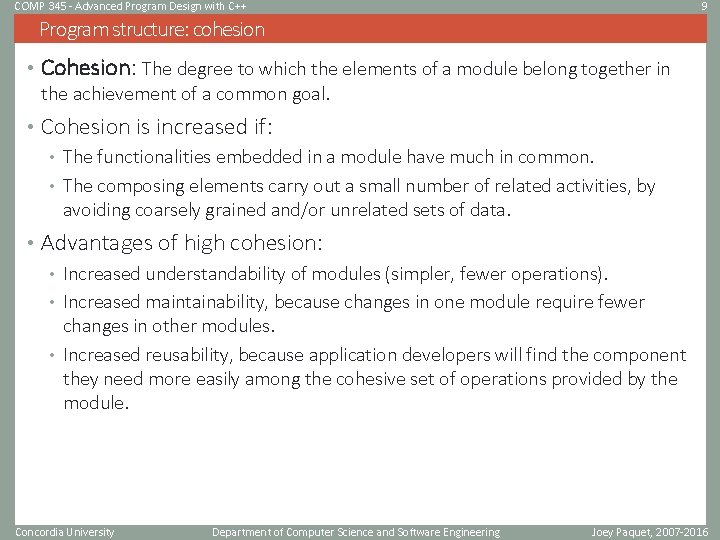
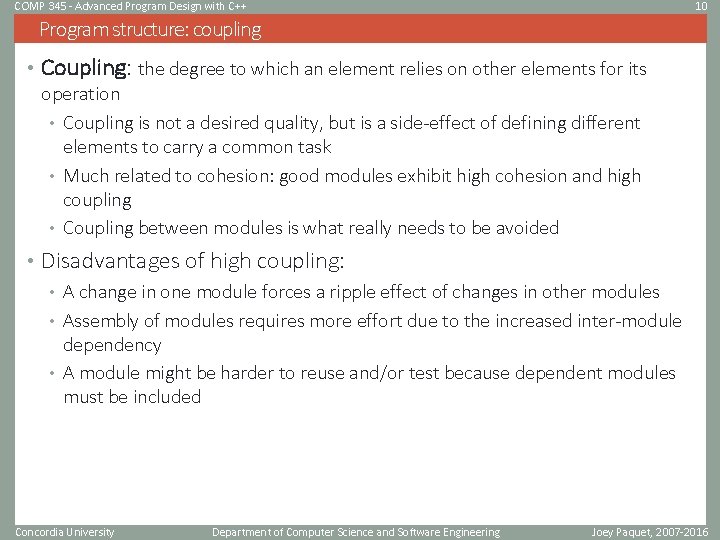
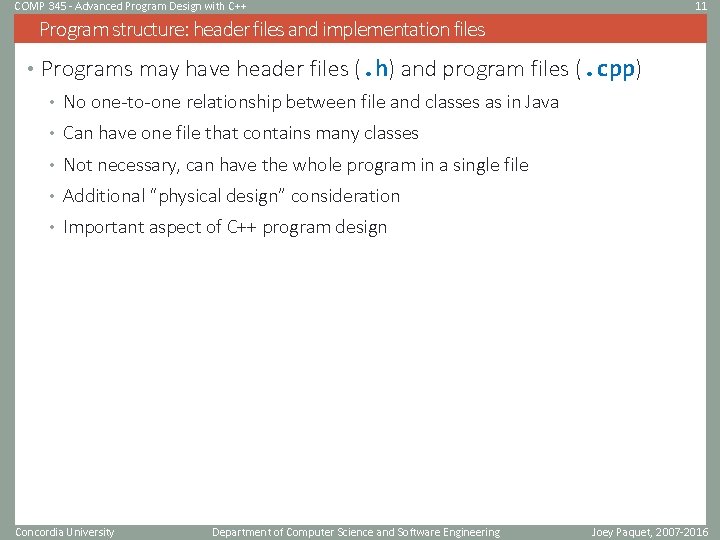
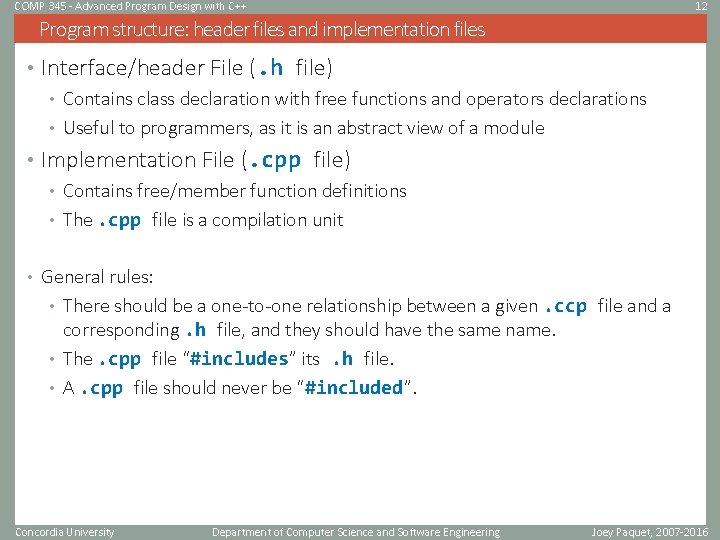
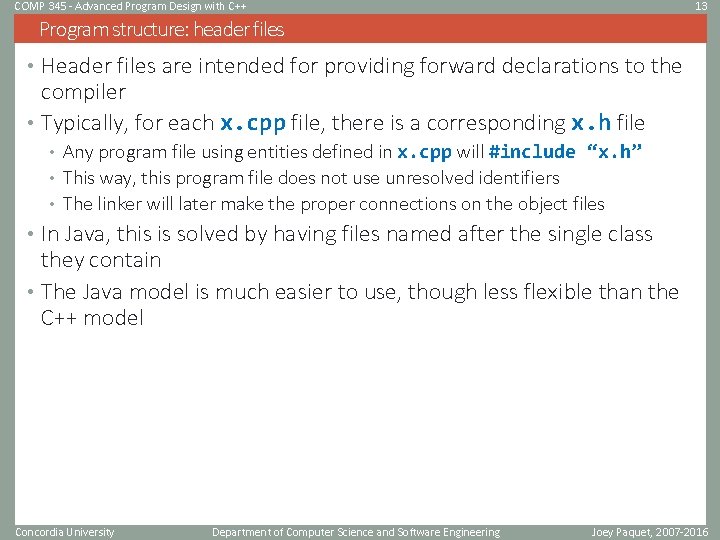
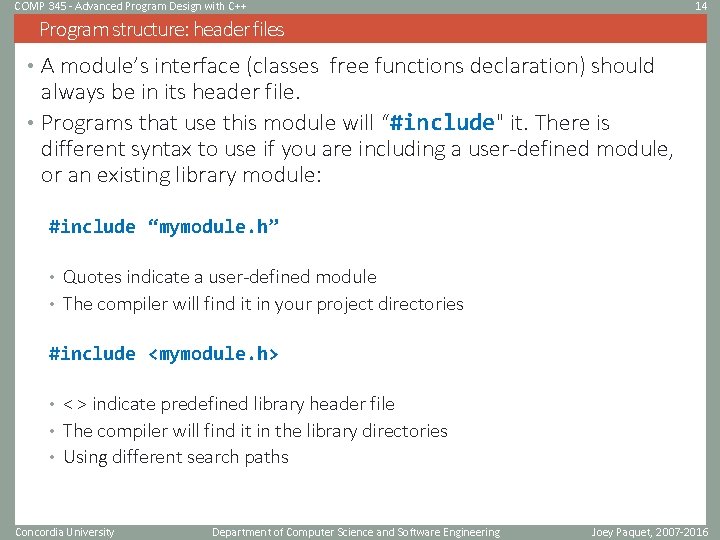
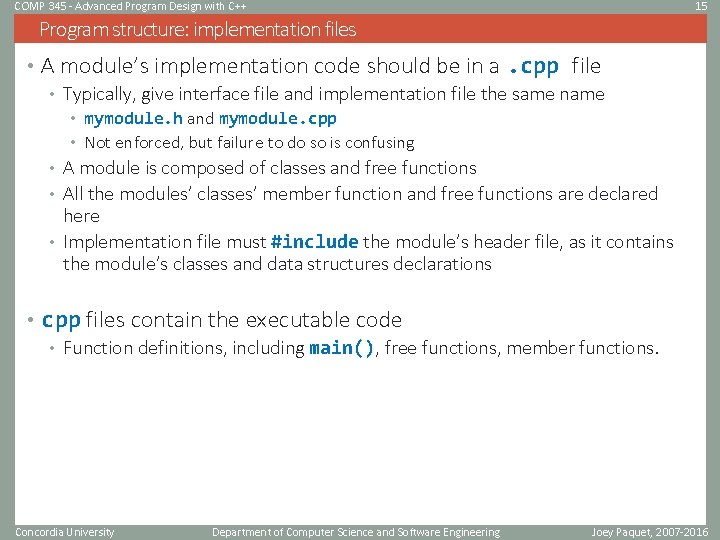
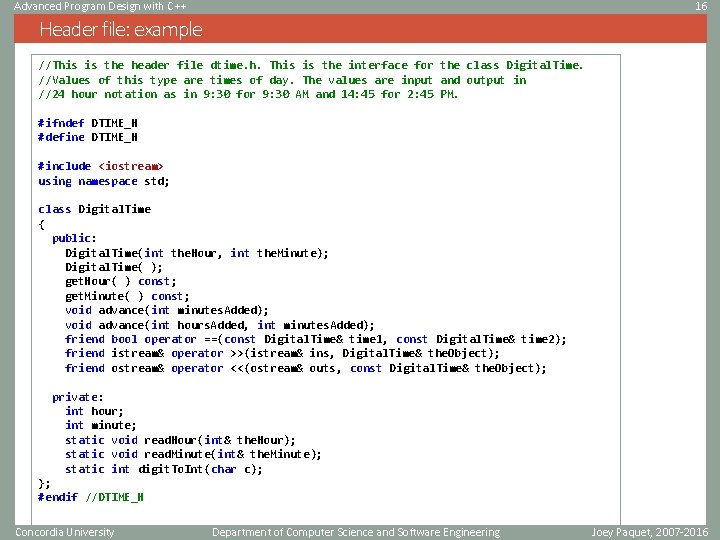
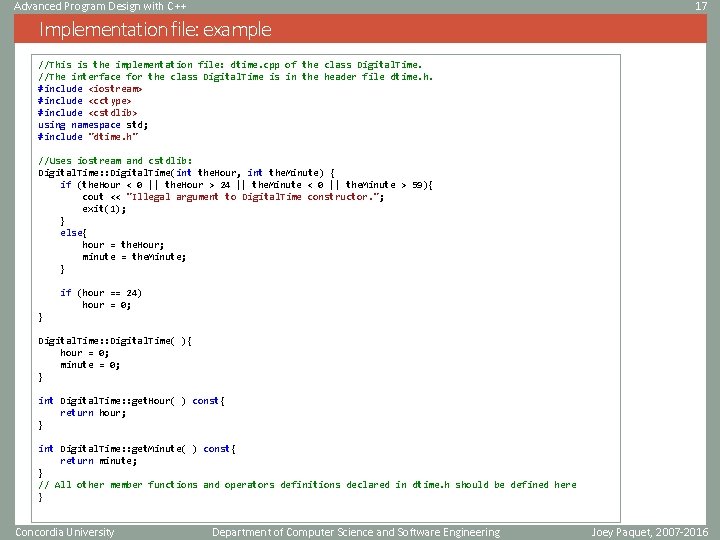
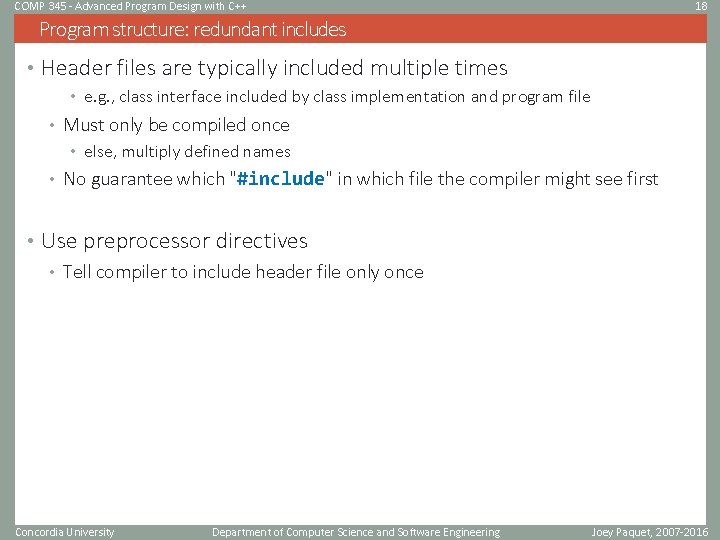
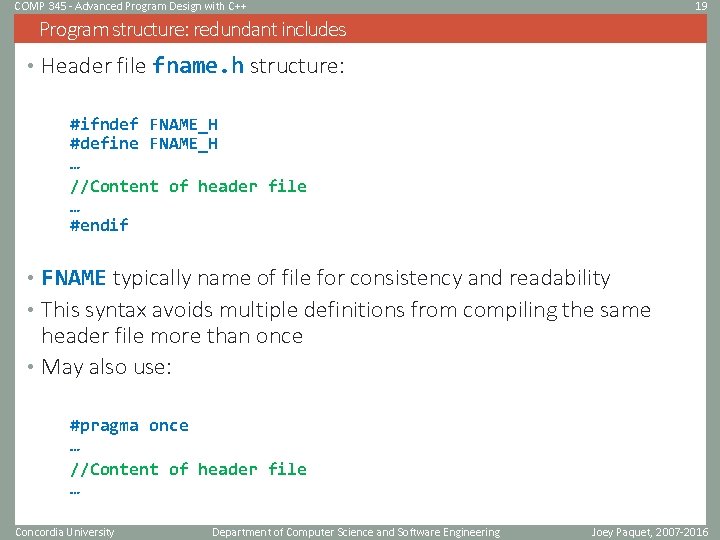
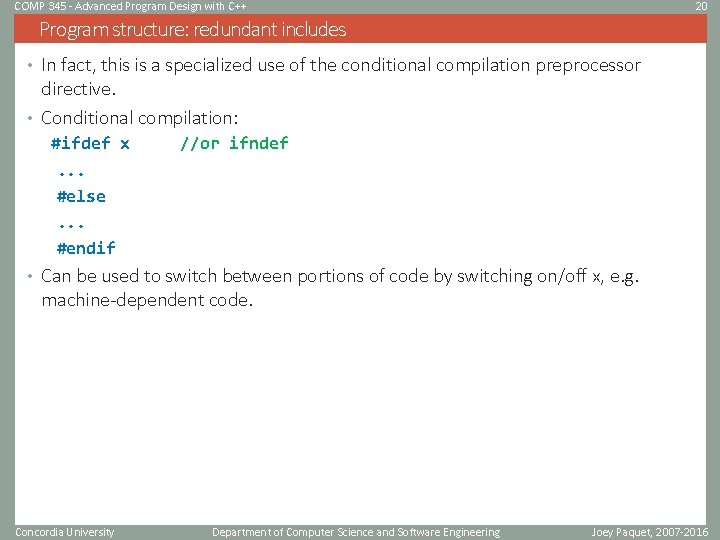
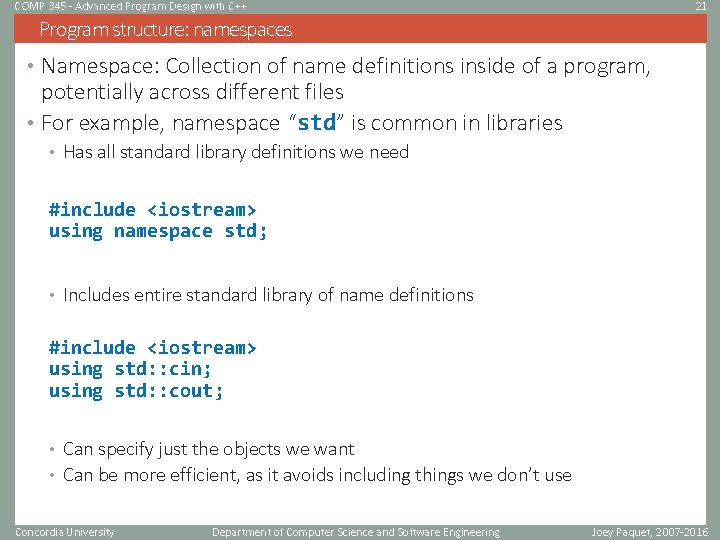
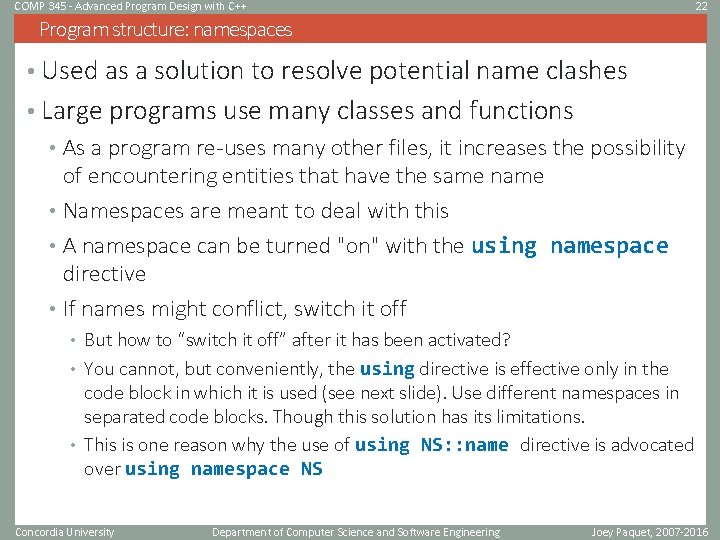
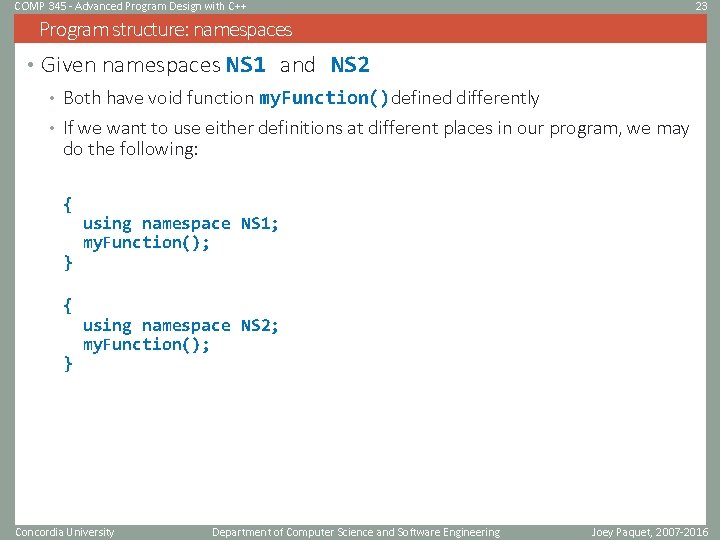
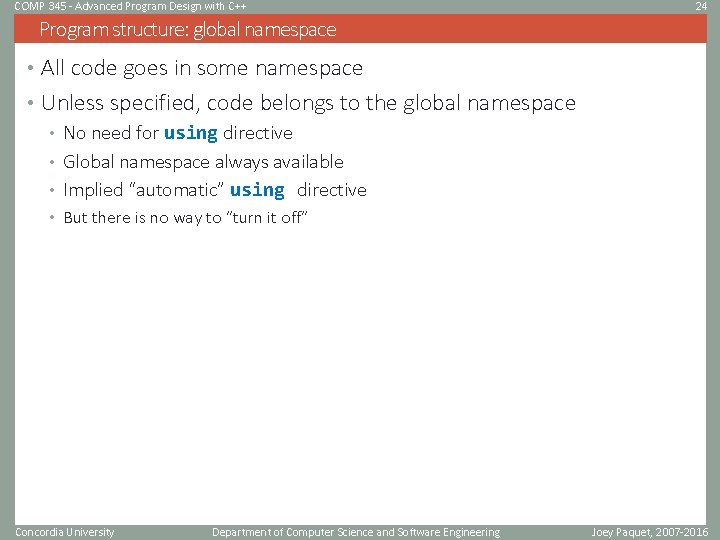
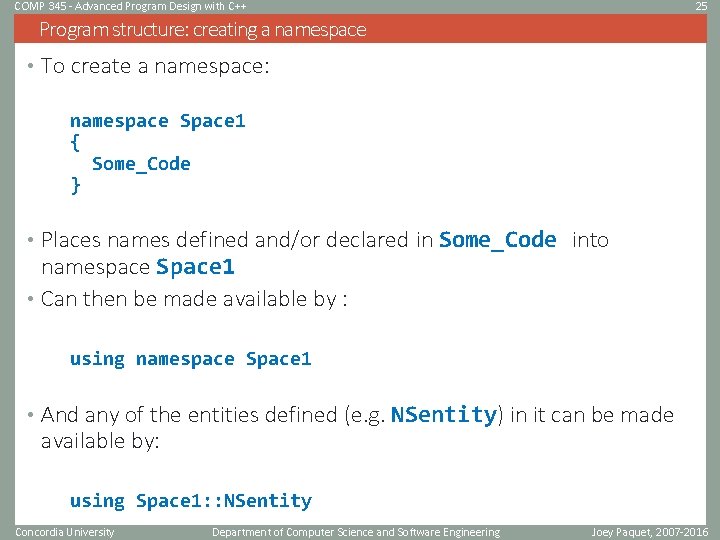
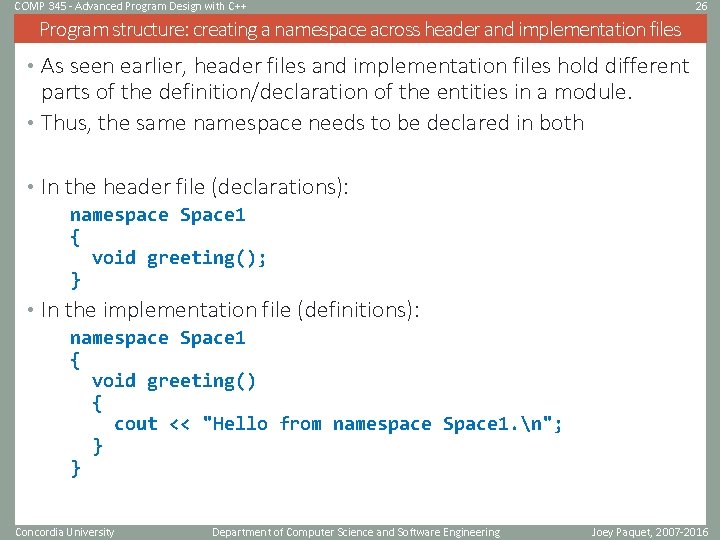
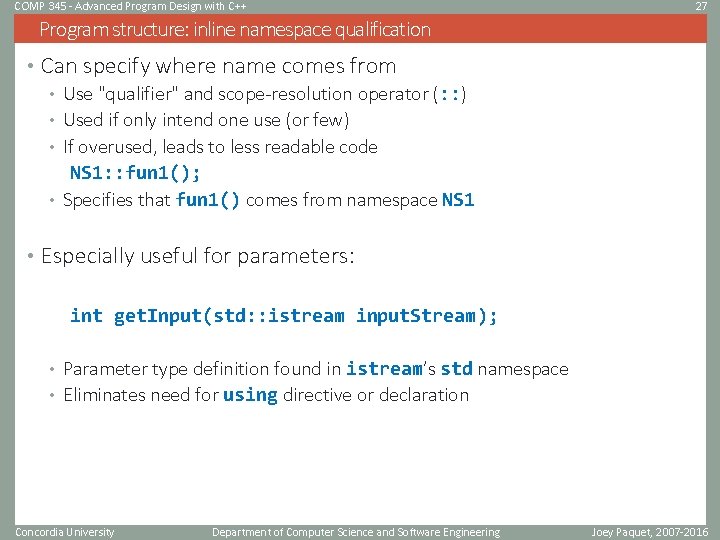
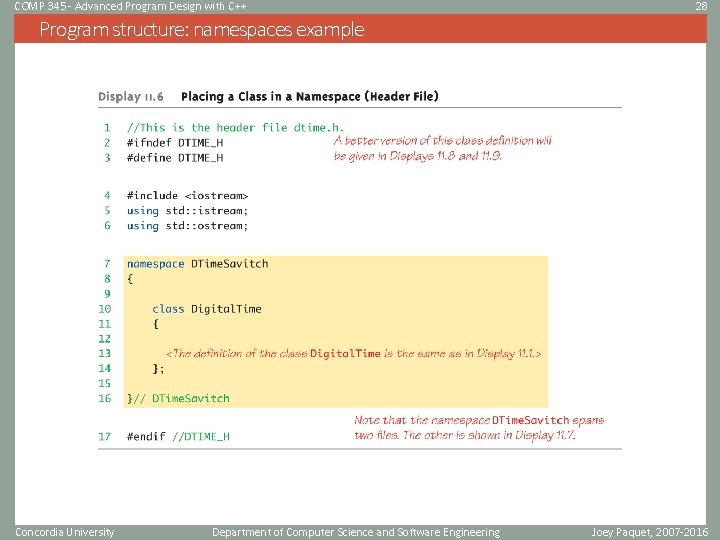
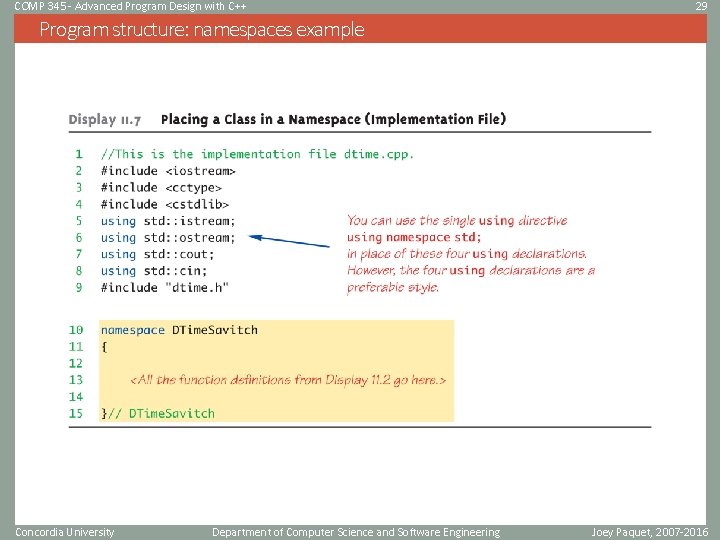
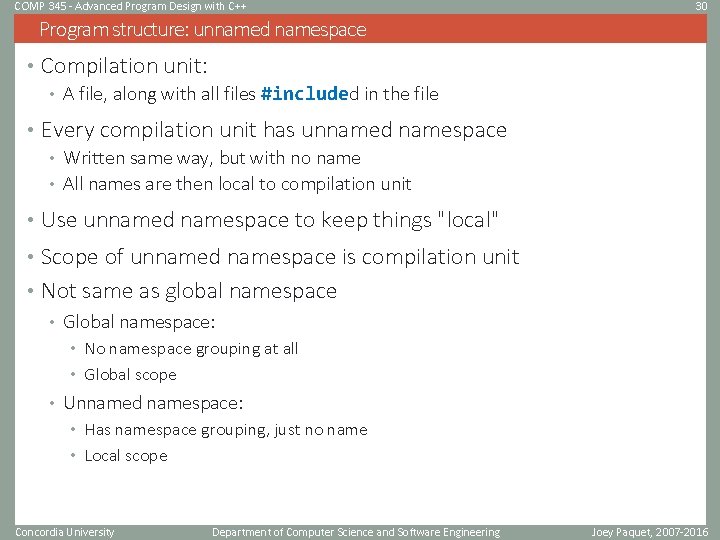
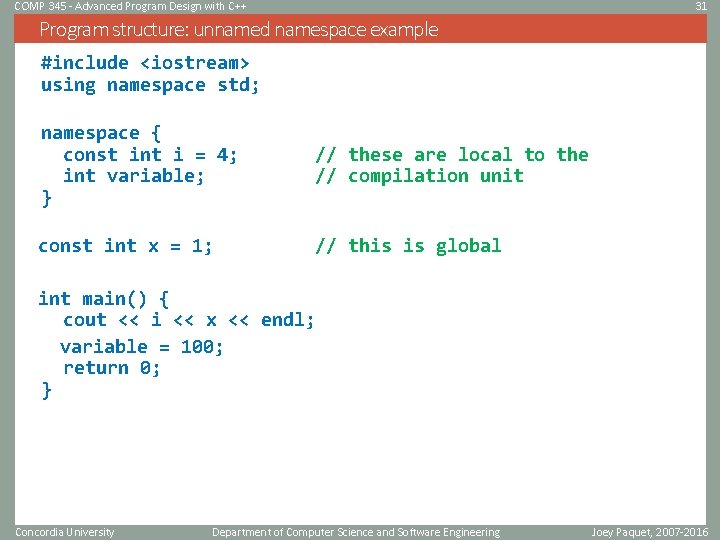
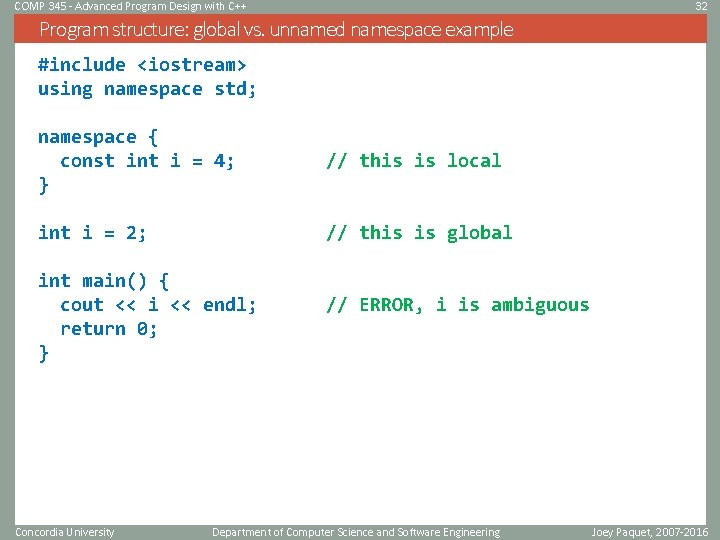
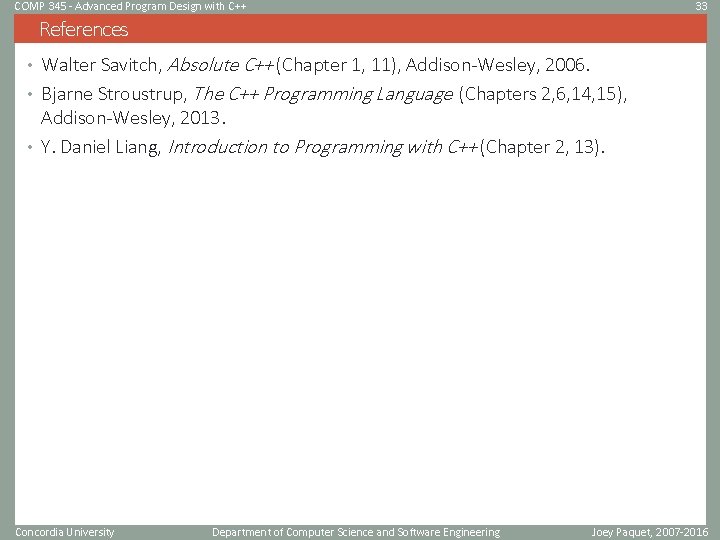
- Slides: 33
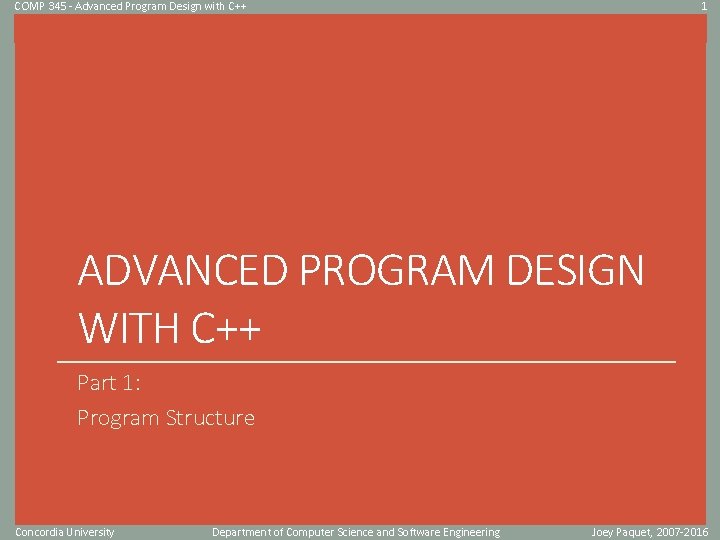
COMP 345 - Advanced Program Design with C++ 1 Click to edit Master title style ADVANCED PROGRAM DESIGN WITH C++ Part 1: Program Structure Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
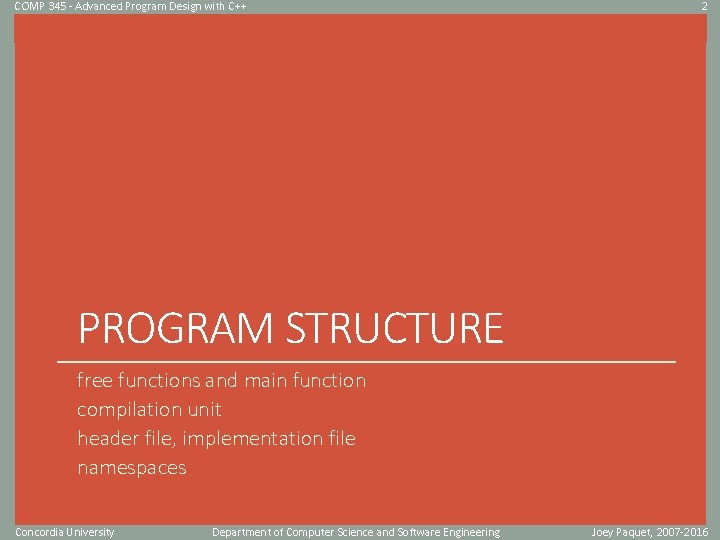
COMP 345 - Advanced Program Design with C++ 2 Click to edit Master title style PROGRAM STRUCTURE free functions and main function compilation unit header file, implementation file namespaces Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
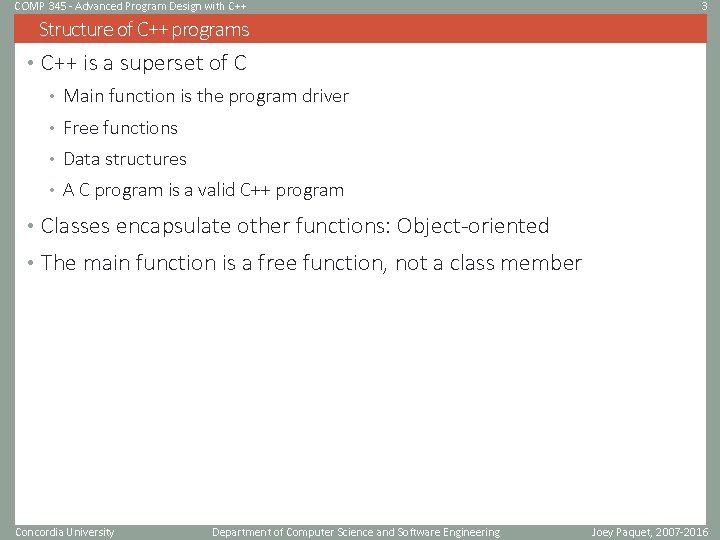
COMP 345 - Advanced Program Design with C++ 3 Structure of C++ programs • C++ is a superset of C • Main function is the program driver • Free functions • Data structures • A C program is a valid C++ program • Classes encapsulate other functions: Object-oriented • The main function is a free function, not a class member Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
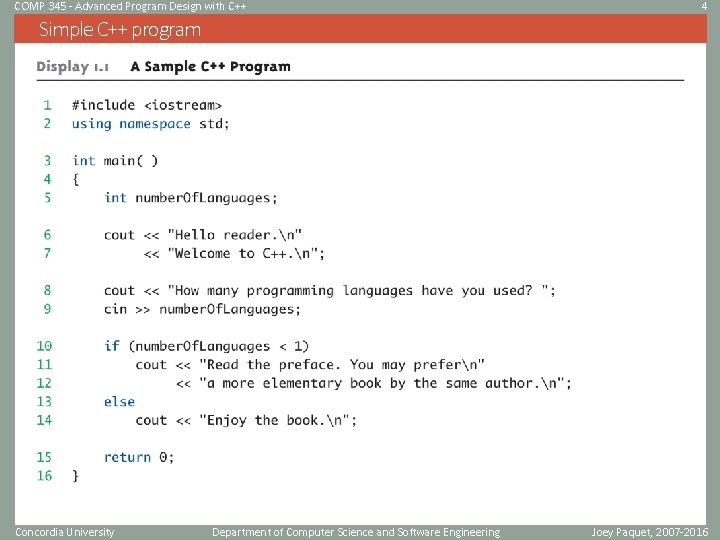
COMP 345 - Advanced Program Design with C++ 4 Simple C++ program Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
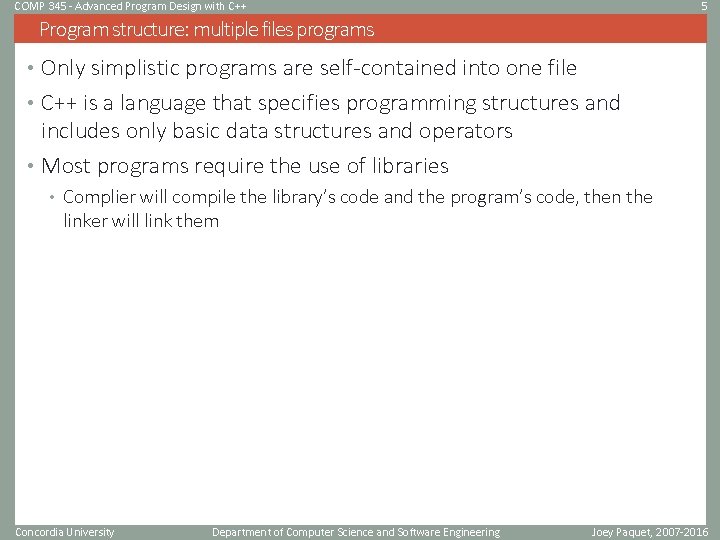
COMP 345 - Advanced Program Design with C++ 5 Program structure: multiple files programs • Only simplistic programs are self-contained into one file • C++ is a language that specifies programming structures and includes only basic data structures and operators • Most programs require the use of libraries • Complier will compile the library’s code and the program’s code, then the linker will link them Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
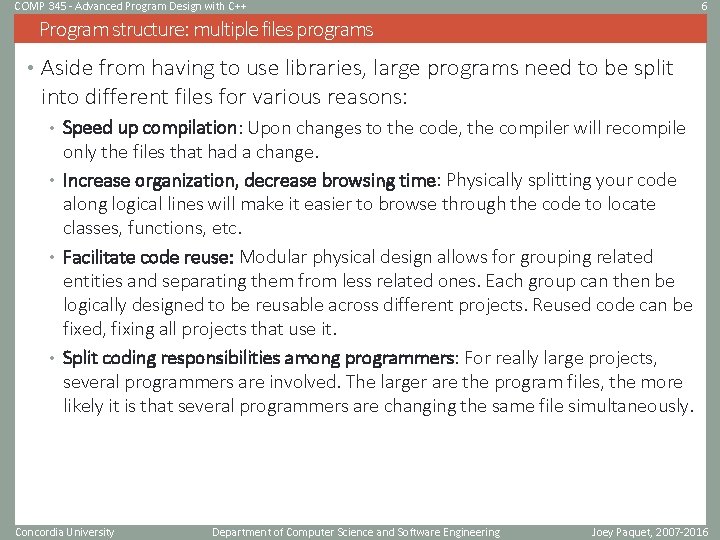
COMP 345 - Advanced Program Design with C++ 6 Program structure: multiple files programs • Aside from having to use libraries, large programs need to be split into different files for various reasons: • Speed up compilation: Upon changes to the code, the compiler will recompile only the files that had a change. • Increase organization, decrease browsing time: Physically splitting your code along logical lines will make it easier to browse through the code to locate classes, functions, etc. • Facilitate code reuse: Modular physical design allows for grouping related entities and separating them from less related ones. Each group can then be logically designed to be reusable across different projects. Reused code can be fixed, fixing all projects that use it. • Split coding responsibilities among programmers: For really large projects, several programmers are involved. The larger are the program files, the more likely it is that several programmers are changing the same file simultaneously. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
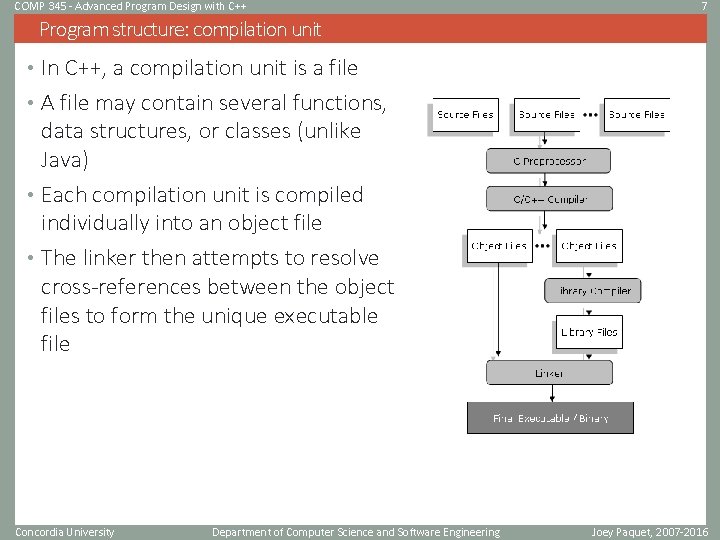
COMP 345 - Advanced Program Design with C++ 7 Program structure: compilation unit • In C++, a compilation unit is a file • A file may contain several functions, data structures, or classes (unlike Java) • Each compilation unit is compiled individually into an object file • The linker then attempts to resolve cross-references between the object files to form the unique executable file Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
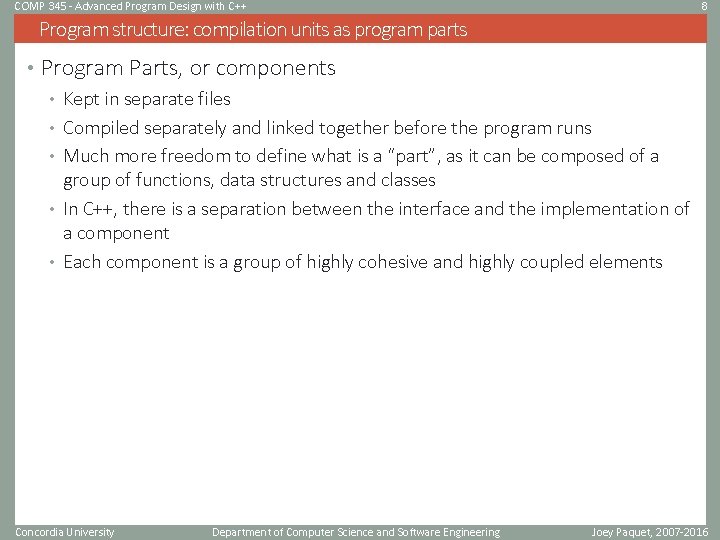
COMP 345 - Advanced Program Design with C++ 8 Program structure: compilation units as program parts • Program Parts, or components • Kept in separate files • Compiled separately and linked together before the program runs • Much more freedom to define what is a “part”, as it can be composed of a group of functions, data structures and classes • In C++, there is a separation between the interface and the implementation of a component • Each component is a group of highly cohesive and highly coupled elements Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
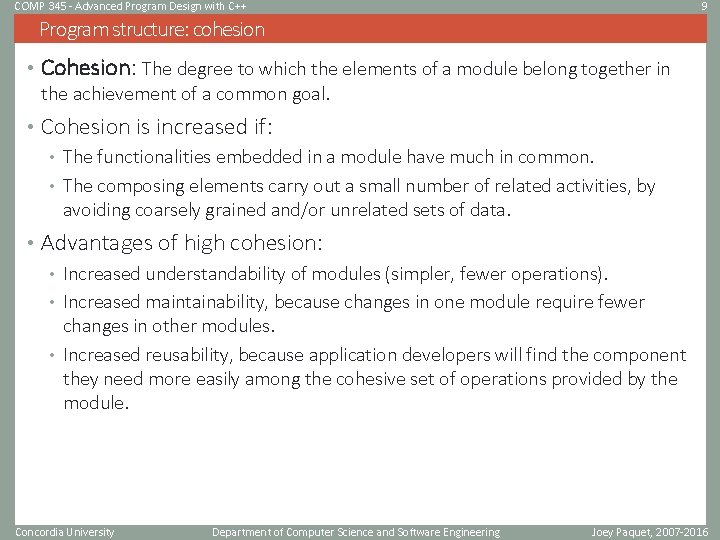
COMP 345 - Advanced Program Design with C++ 9 Program structure: cohesion • Cohesion: The degree to which the elements of a module belong together in the achievement of a common goal. • Cohesion is increased if: • The functionalities embedded in a module have much in common. • The composing elements carry out a small number of related activities, by avoiding coarsely grained and/or unrelated sets of data. • Advantages of high cohesion: • Increased understandability of modules (simpler, fewer operations). • Increased maintainability, because changes in one module require fewer changes in other modules. • Increased reusability, because application developers will find the component they need more easily among the cohesive set of operations provided by the module. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
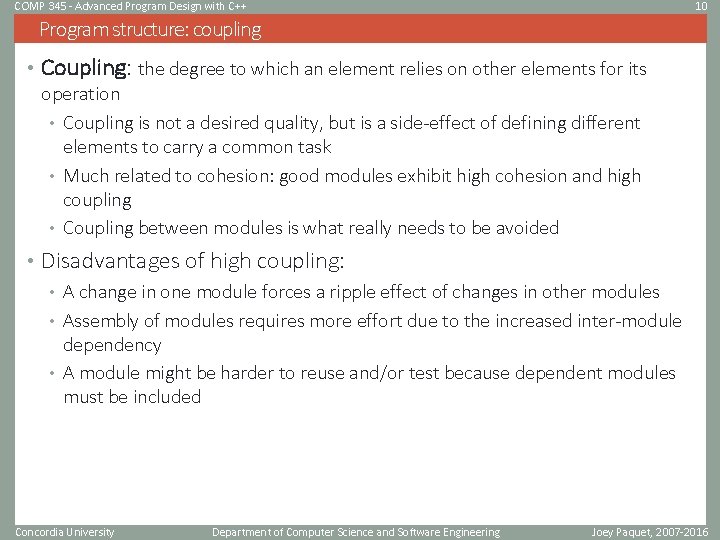
COMP 345 - Advanced Program Design with C++ 10 Program structure: coupling • Coupling: the degree to which an element relies on other elements for its operation • Coupling is not a desired quality, but is a side-effect of defining different elements to carry a common task • Much related to cohesion: good modules exhibit high cohesion and high coupling • Coupling between modules is what really needs to be avoided • Disadvantages of high coupling: • A change in one module forces a ripple effect of changes in other modules • Assembly of modules requires more effort due to the increased inter-module dependency • A module might be harder to reuse and/or test because dependent modules must be included Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
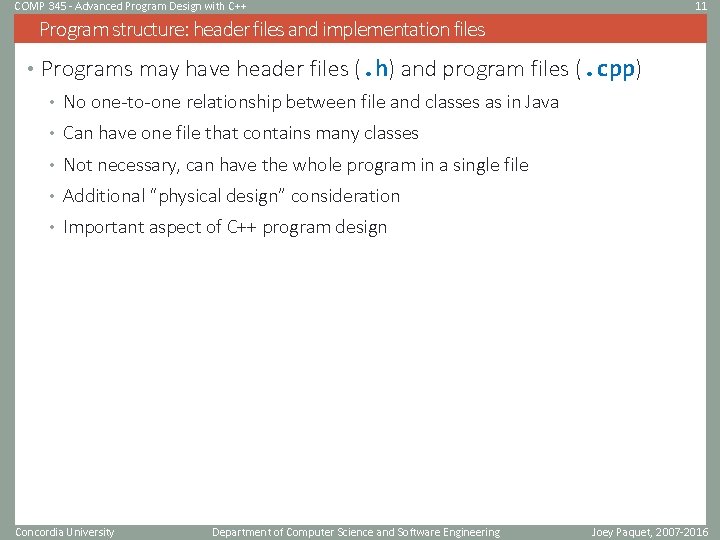
COMP 345 - Advanced Program Design with C++ 11 Program structure: header files and implementation files • Programs may have header files (. h) and program files (. cpp) • No one-to-one relationship between file and classes as in Java • Can have one file that contains many classes • Not necessary, can have the whole program in a single file • Additional “physical design” consideration • Important aspect of C++ program design Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
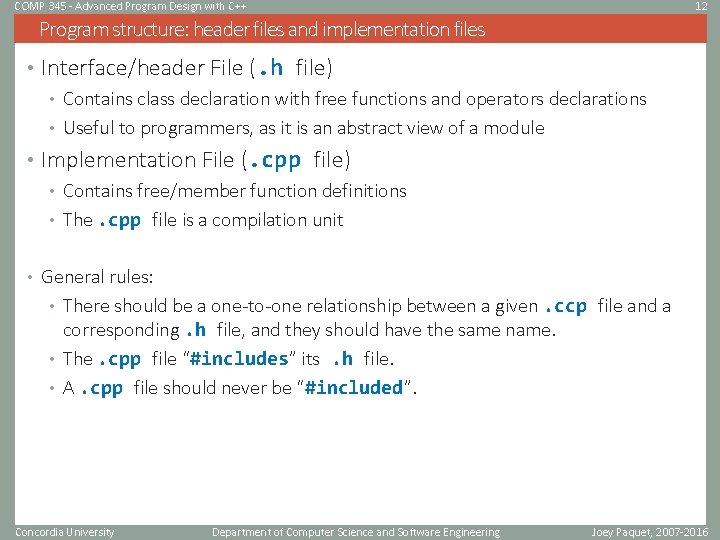
COMP 345 - Advanced Program Design with C++ 12 Program structure: header files and implementation files • Interface/header File (. h file) • Contains class declaration with free functions and operators declarations • Useful to programmers, as it is an abstract view of a module • Implementation File (. cpp file) • Contains free/member function definitions • The. cpp file is a compilation unit • General rules: • There should be a one-to-one relationship between a given. ccp file and a corresponding. h file, and they should have the same name. • The. cpp file “#includes” its. h file. • A. cpp file should never be “#included”. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
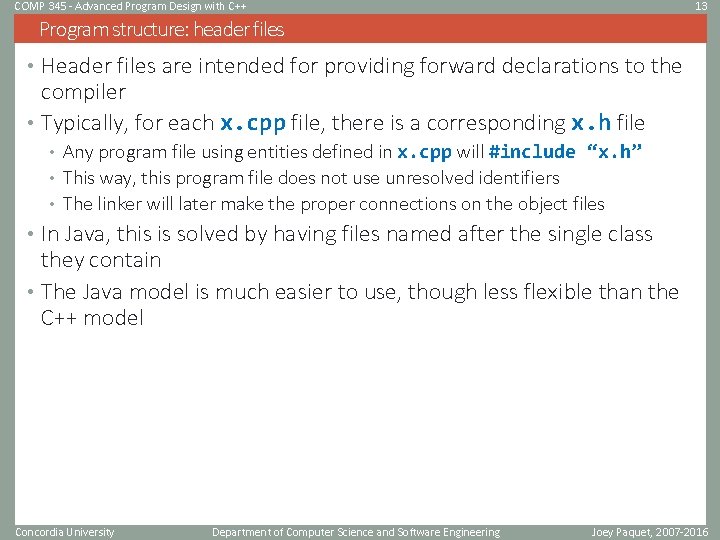
COMP 345 - Advanced Program Design with C++ 13 Program structure: header files • Header files are intended for providing forward declarations to the compiler • Typically, for each x. cpp file, there is a corresponding x. h file • Any program file using entities defined in x. cpp will #include “x. h” • This way, this program file does not use unresolved identifiers • The linker will later make the proper connections on the object files • In Java, this is solved by having files named after the single class they contain • The Java model is much easier to use, though less flexible than the C++ model Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
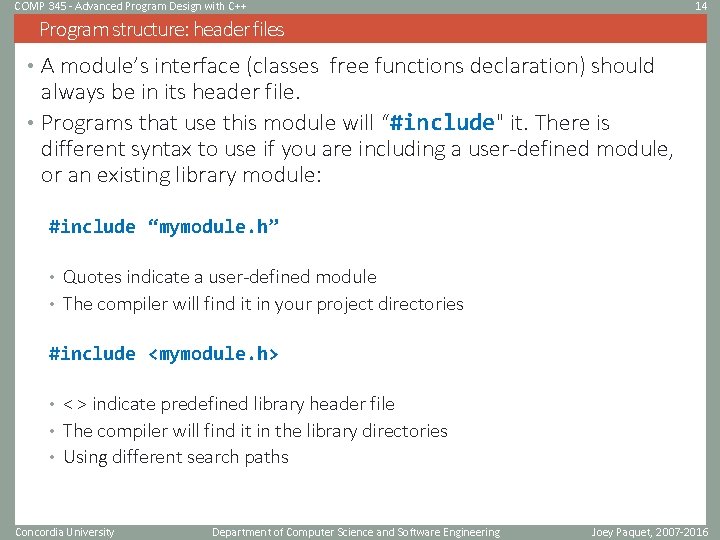
COMP 345 - Advanced Program Design with C++ 14 Program structure: header files • A module’s interface (classes free functions declaration) should always be in its header file. • Programs that use this module will “#include" it. There is different syntax to use if you are including a user-defined module, or an existing library module: #include “mymodule. h” • Quotes indicate a user-defined module • The compiler will find it in your project directories #include <mymodule. h> • < > indicate predefined library header file • The compiler will find it in the library directories • Using different search paths Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
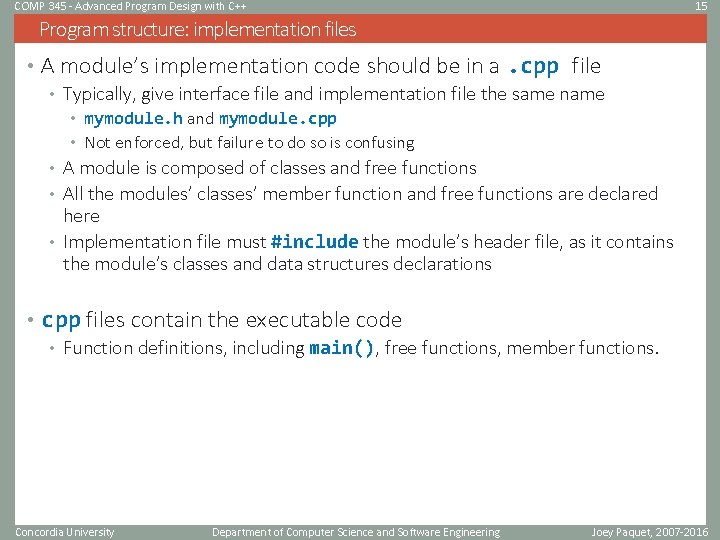
COMP 345 - Advanced Program Design with C++ 15 Program structure: implementation files • A module’s implementation code should be in a. cpp file • Typically, give interface file and implementation file the same name • mymodule. h and mymodule. cpp • Not enforced, but failure to do so is confusing • A module is composed of classes and free functions • All the modules’ classes’ member function and free functions are declared here • Implementation file must #include the module’s header file, as it contains the module’s classes and data structures declarations • cpp files contain the executable code • Function definitions, including main(), free functions, member functions. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
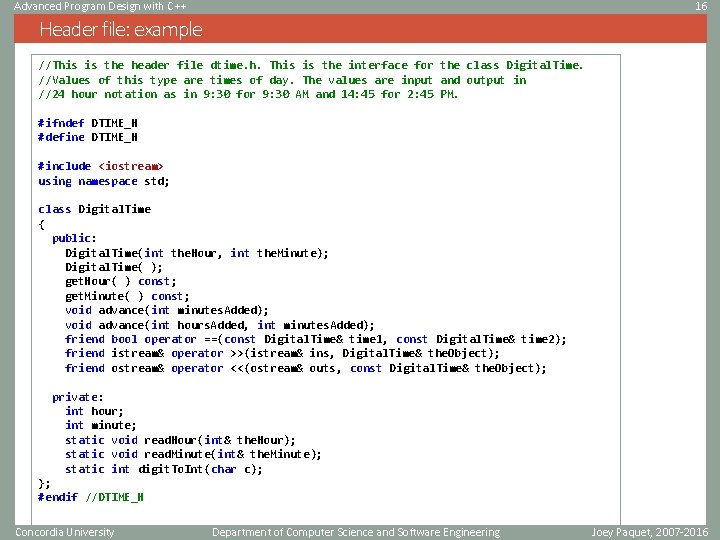
Advanced Program Design with C++ 16 Header file: example //This is the header file dtime. h. This is the interface for the class Digital. Time. //Values of this type are times of day. The values are input and output in //24 hour notation as in 9: 30 for 9: 30 AM and 14: 45 for 2: 45 PM. #ifndef DTIME_H #define DTIME_H #include <iostream> using namespace std; class Digital. Time { public: Digital. Time(int the. Hour, int the. Minute); Digital. Time( ); get. Hour( ) const; get. Minute( ) const; void advance(int minutes. Added); void advance(int hours. Added, int minutes. Added); friend bool operator ==(const Digital. Time& time 1, const Digital. Time& time 2); friend istream& operator >>(istream& ins, Digital. Time& the. Object); friend ostream& operator <<(ostream& outs, const Digital. Time& the. Object); private: int hour; int minute; static void read. Hour(int& the. Hour); static void read. Minute(int& the. Minute); static int digit. To. Int(char c); }; #endif //DTIME_H Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
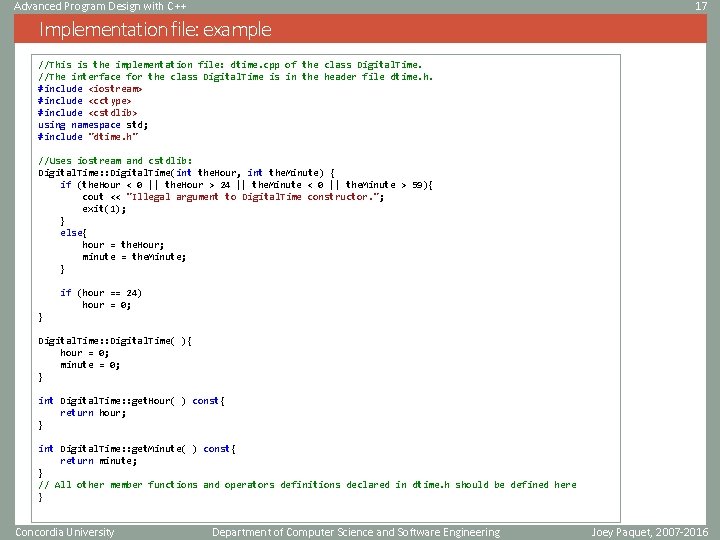
Advanced Program Design with C++ 17 Implementation file: example //This is the implementation file: dtime. cpp of the class Digital. Time. //The interface for the class Digital. Time is in the header file dtime. h. #include <iostream> #include <cctype> #include <cstdlib> using namespace std; #include "dtime. h" //Uses iostream and cstdlib: Digital. Time: : Digital. Time(int the. Hour, int the. Minute) { if (the. Hour < 0 || the. Hour > 24 || the. Minute < 0 || the. Minute > 59){ cout << "Illegal argument to Digital. Time constructor. "; exit(1); } else{ hour = the. Hour; minute = the. Minute; } if (hour == 24) hour = 0; } Digital. Time: : Digital. Time( ){ hour = 0; minute = 0; } int Digital. Time: : get. Hour( ) const{ return hour; } int Digital. Time: : get. Minute( ) const{ return minute; } // All other member functions and operators definitions declared in dtime. h should be defined here } Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
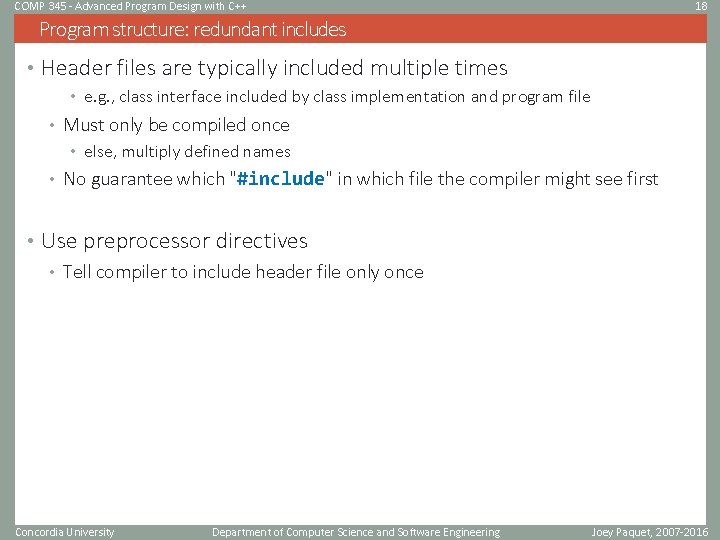
COMP 345 - Advanced Program Design with C++ 18 Program structure: redundant includes • Header files are typically included multiple times • e. g. , class interface included by class implementation and program file • Must only be compiled once • else, multiply defined names • No guarantee which "#include" in which file the compiler might see first • Use preprocessor directives • Tell compiler to include header file only once Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
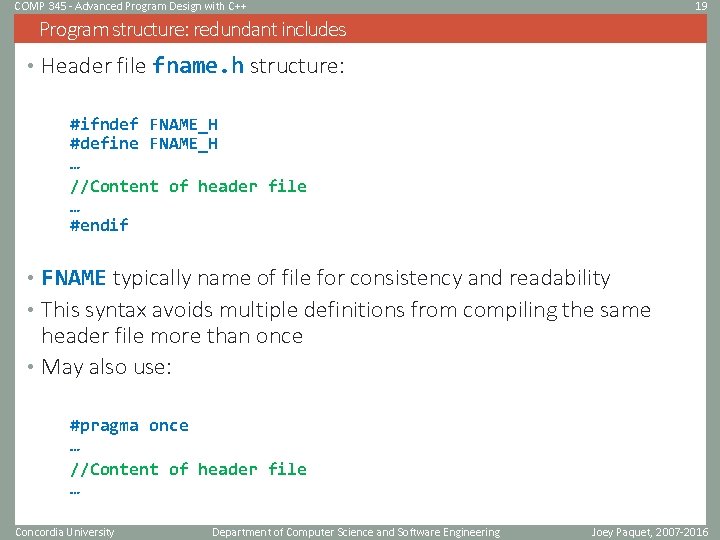
COMP 345 - Advanced Program Design with C++ 19 Program structure: redundant includes • Header file fname. h structure: #ifndef FNAME_H #define FNAME_H … //Content of header file … #endif • FNAME typically name of file for consistency and readability • This syntax avoids multiple definitions from compiling the same header file more than once • May also use: #pragma once … //Content of header file … Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
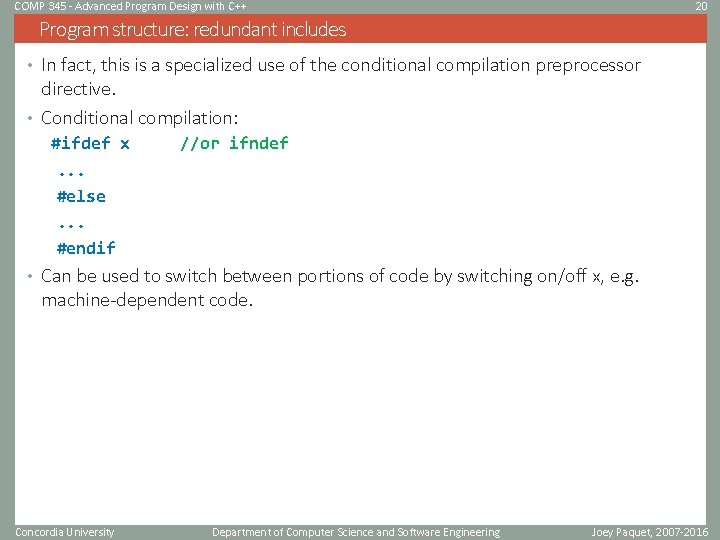
COMP 345 - Advanced Program Design with C++ 20 Program structure: redundant includes • In fact, this is a specialized use of the conditional compilation preprocessor directive. • Conditional compilation: #ifdef x //or ifndef . . . #else. . . #endif • Can be used to switch between portions of code by switching on/off x, e. g. machine-dependent code. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
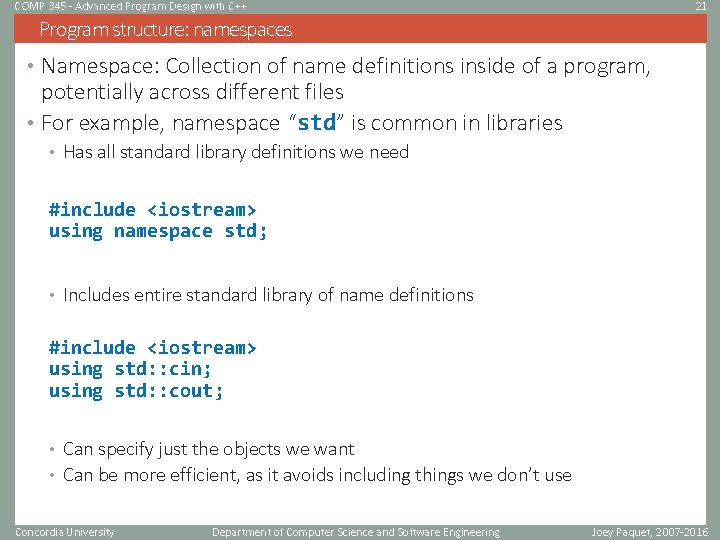
COMP 345 - Advanced Program Design with C++ 21 Program structure: namespaces • Namespace: Collection of name definitions inside of a program, potentially across different files • For example, namespace “std” is common in libraries • Has all standard library definitions we need #include <iostream> using namespace std; • Includes entire standard library of name definitions #include <iostream> using std: : cin; using std: : cout; • Can specify just the objects we want • Can be more efficient, as it avoids including things we don’t use Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
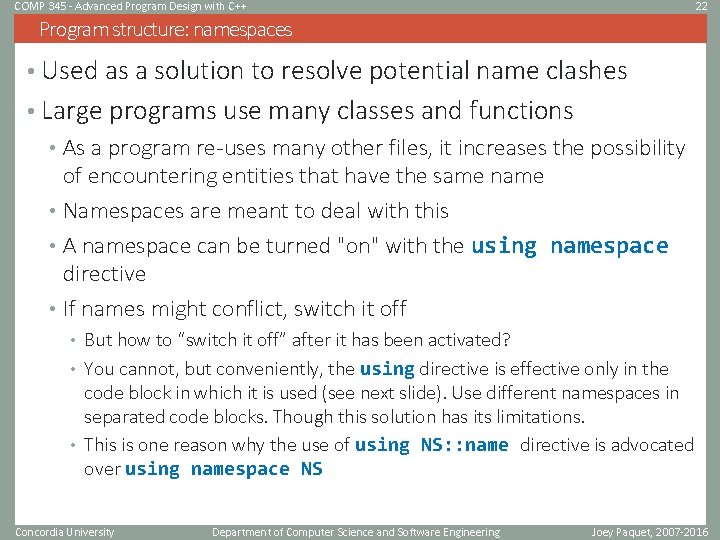
COMP 345 - Advanced Program Design with C++ 22 Program structure: namespaces • Used as a solution to resolve potential name clashes • Large programs use many classes and functions • As a program re-uses many other files, it increases the possibility of encountering entities that have the same name • Namespaces are meant to deal with this • A namespace can be turned "on" with the using namespace directive • If names might conflict, switch it off • But how to “switch it off” after it has been activated? • You cannot, but conveniently, the using directive is effective only in the code block in which it is used (see next slide). Use different namespaces in separated code blocks. Though this solution has its limitations. • This is one reason why the use of using NS: : name directive is advocated over using namespace NS Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
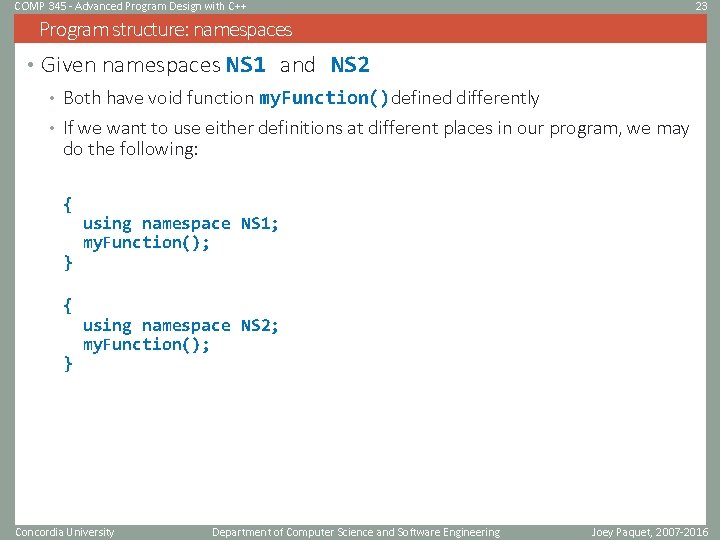
COMP 345 - Advanced Program Design with C++ 23 Program structure: namespaces • Given namespaces NS 1 and NS 2 • Both have void function my. Function()defined differently • If we want to use either definitions at different places in our program, we may do the following: { } using namespace NS 1; my. Function(); using namespace NS 2; my. Function(); Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
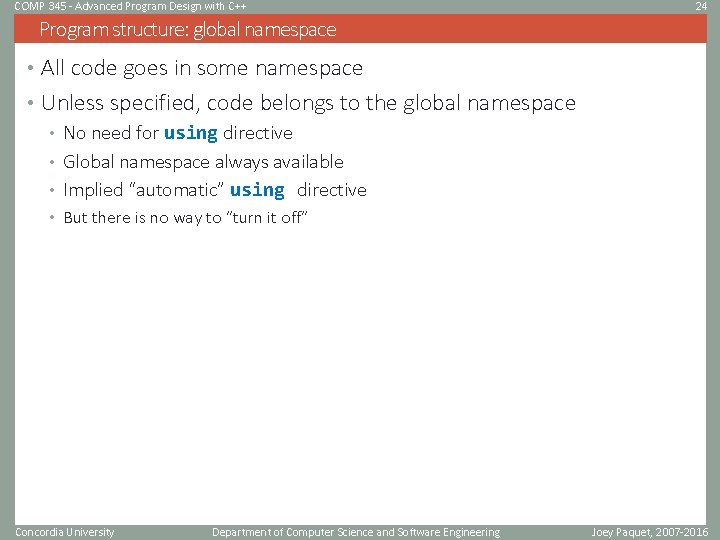
COMP 345 - Advanced Program Design with C++ 24 Program structure: global namespace • All code goes in some namespace • Unless specified, code belongs to the global namespace • No need for using directive • Global namespace always available • Implied “automatic” using directive • But there is no way to “turn it off” Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
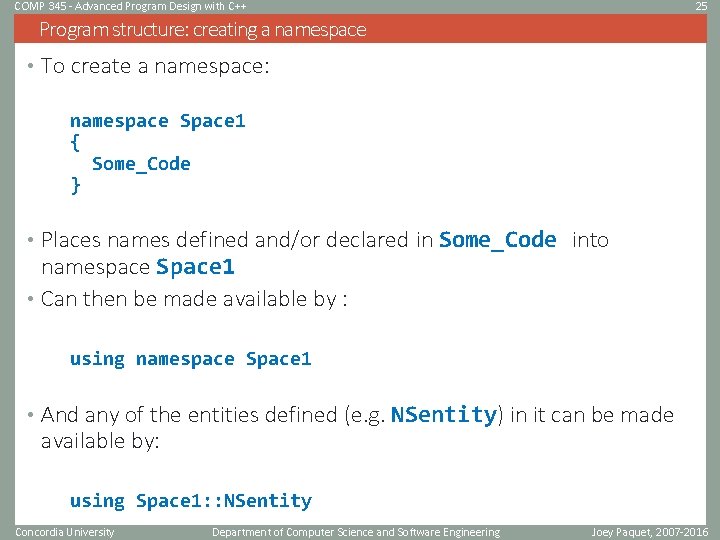
COMP 345 - Advanced Program Design with C++ 25 Program structure: creating a namespace • To create a namespace: namespace Space 1 { Some_Code } • Places names defined and/or declared in Some_Code into namespace Space 1 • Can then be made available by : using namespace Space 1 • And any of the entities defined (e. g. NSentity) in it can be made available by: using Space 1: : NSentity Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
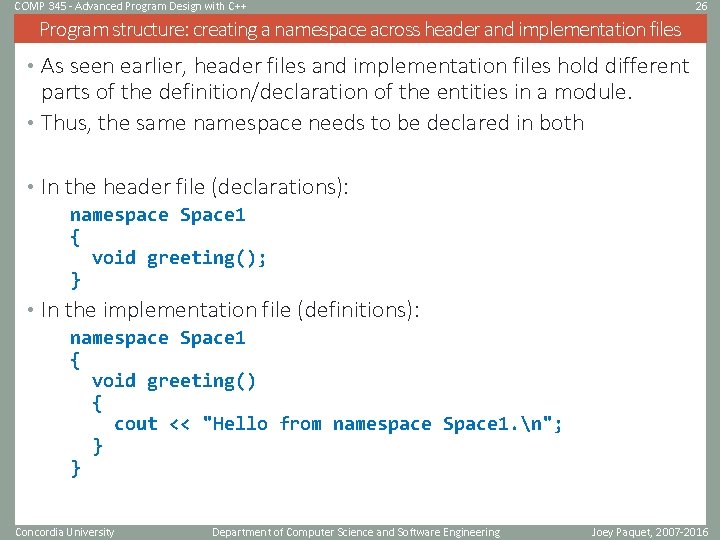
COMP 345 - Advanced Program Design with C++ 26 Program structure: creating a namespace across header and implementation files • As seen earlier, header files and implementation files hold different parts of the definition/declaration of the entities in a module. • Thus, the same namespace needs to be declared in both • In the header file (declarations): namespace Space 1 { void greeting(); } • In the implementation file (definitions): namespace Space 1 { void greeting() { cout << "Hello from namespace Space 1. n"; } } Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
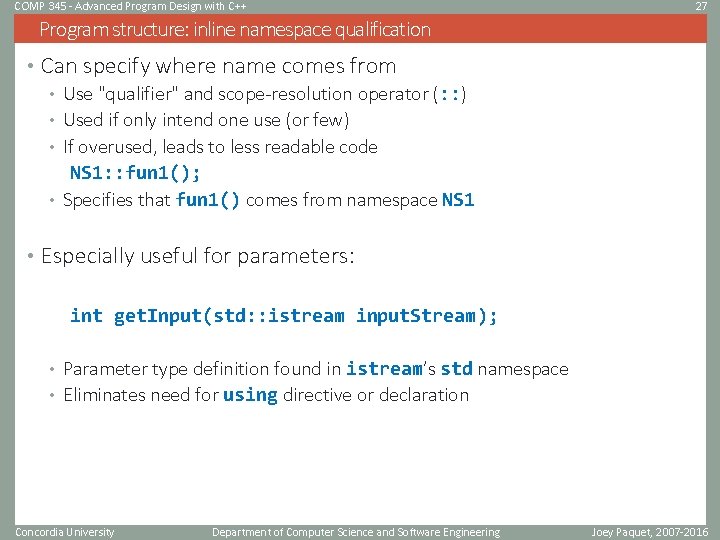
COMP 345 - Advanced Program Design with C++ 27 Program structure: inline namespace qualification • Can specify where name comes from • Use "qualifier" and scope-resolution operator (: : ) • Used if only intend one use (or few) • If overused, leads to less readable code NS 1: : fun 1(); • Specifies that fun 1() comes from namespace NS 1 • Especially useful for parameters: int get. Input(std: : istream input. Stream); • Parameter type definition found in istream’s std namespace • Eliminates need for using directive or declaration Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
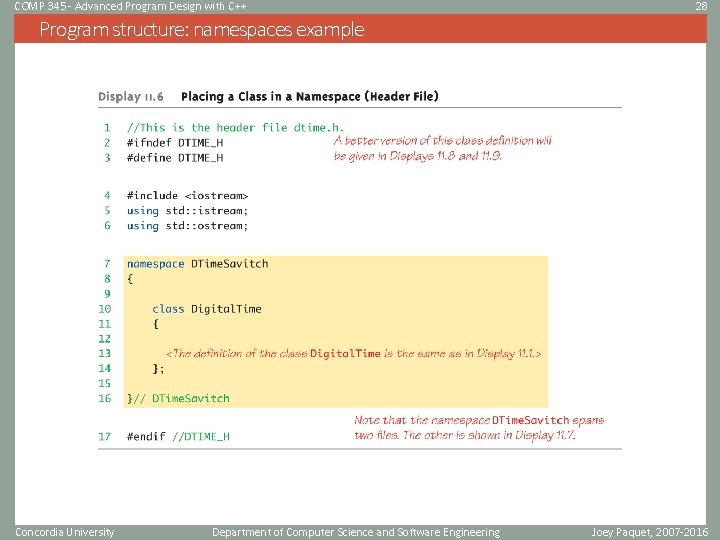
COMP 345 - Advanced Program Design with C++ 28 Program structure: namespaces example Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
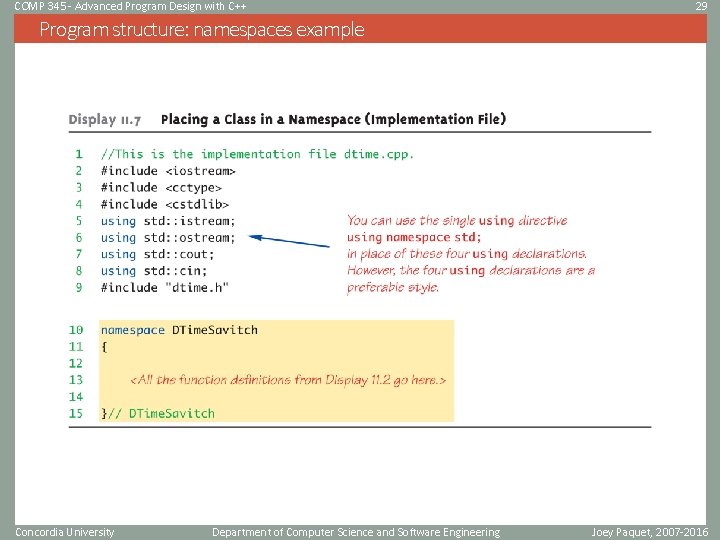
COMP 345 - Advanced Program Design with C++ 29 Program structure: namespaces example Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
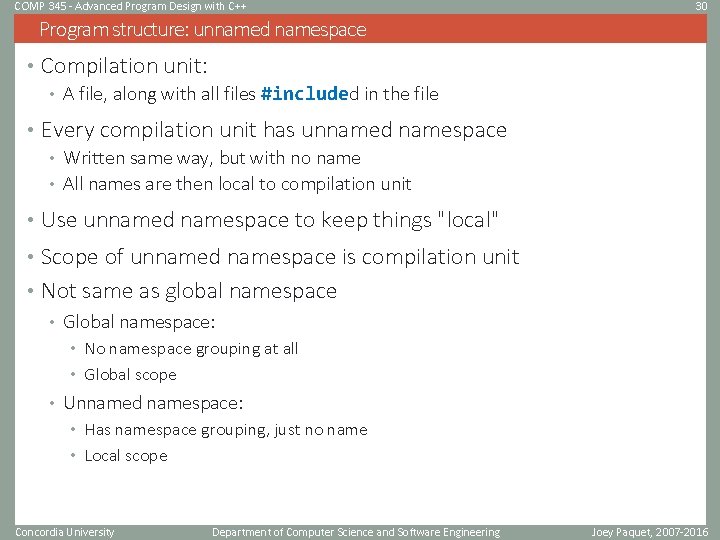
COMP 345 - Advanced Program Design with C++ 30 Program structure: unnamed namespace • Compilation unit: • A file, along with all files #included in the file • Every compilation unit has unnamed namespace • Written same way, but with no name • All names are then local to compilation unit • Use unnamed namespace to keep things "local" • Scope of unnamed namespace is compilation unit • Not same as global namespace • Global namespace: • No namespace grouping at all • Global scope • Unnamed namespace: • Has namespace grouping, just no name • Local scope Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
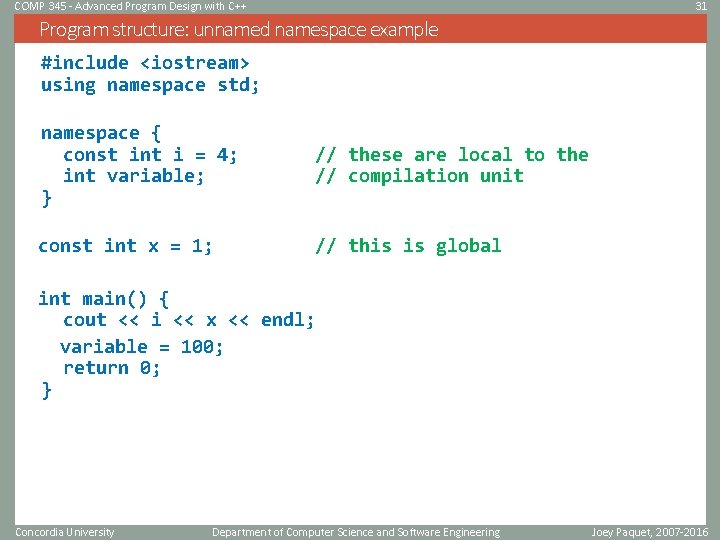
COMP 345 - Advanced Program Design with C++ 31 Program structure: unnamed namespace example #include <iostream> using namespace std; namespace { const int i = 4; int variable; } // these are local to the // compilation unit const int x = 1; // this is global int main() { cout << i << x << endl; variable = 100; return 0; } Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
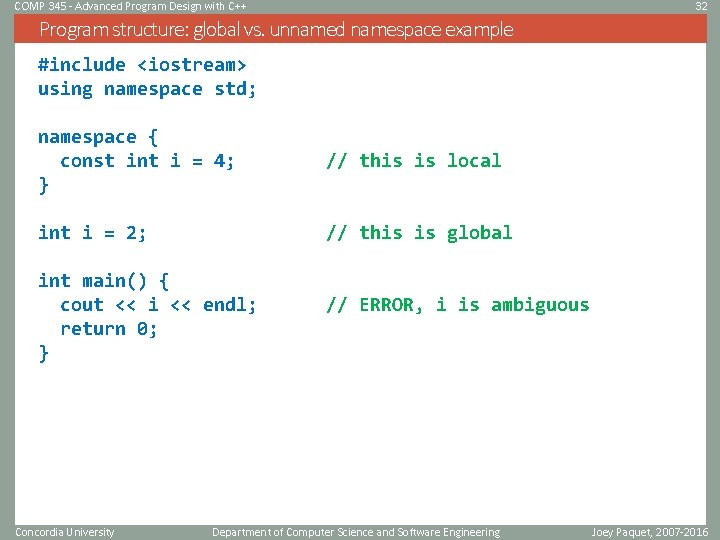
COMP 345 - Advanced Program Design with C++ 32 Program structure: global vs. unnamed namespace example #include <iostream> using namespace std; namespace { const int i = 4; } // this is local int i = 2; // this is global int main() { cout << i << endl; return 0; } Concordia University // ERROR, i is ambiguous Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
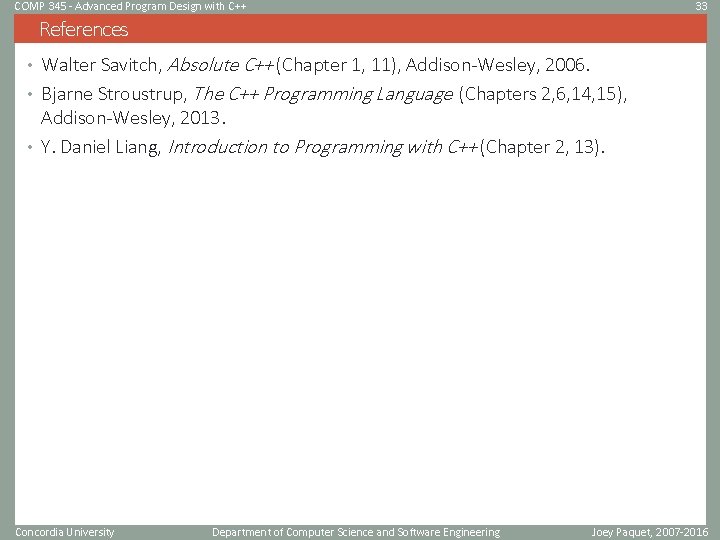
COMP 345 - Advanced Program Design with C++ 33 References • Walter Savitch, Absolute C++ (Chapter 1, 11), Addison-Wesley, 2006. • Bjarne Stroustrup, The C++ Programming Language (Chapters 2, 6, 14, 15), Addison-Wesley, 2013. • Y. Daniel Liang, Introduction to Programming with C++ (Chapter 2, 13). Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2007 -2016
Digital clock in c++ using class
Comp 345
Comp 345 final
Comp345
Comp 345
Comp 345
Softeng 350
Byk345
Bienvenidos himno
Fromme361
345 king william street adelaide
Softeng 350
Deborah amdur
Compsci 345
A-345-k
Compsci 345
Test 345
Compsci 345
Advanced farm management program
Advanced placement incentive program
Unit 18 database design
Advanced machine and engineering
Advanced digital design with the verilog hdl
Advanced compiler design and implementation
Cse 598 advanced software analysis and design
Advanced database design
Cadmim
Advanced algorithm design
Advanced design of steel structures notes
Plexus ranks
Comp tox
Apcs recursion
Comp3007
Hotel comp set data reporting