COMP 121 Week 5 Exceptions and Exception Handling
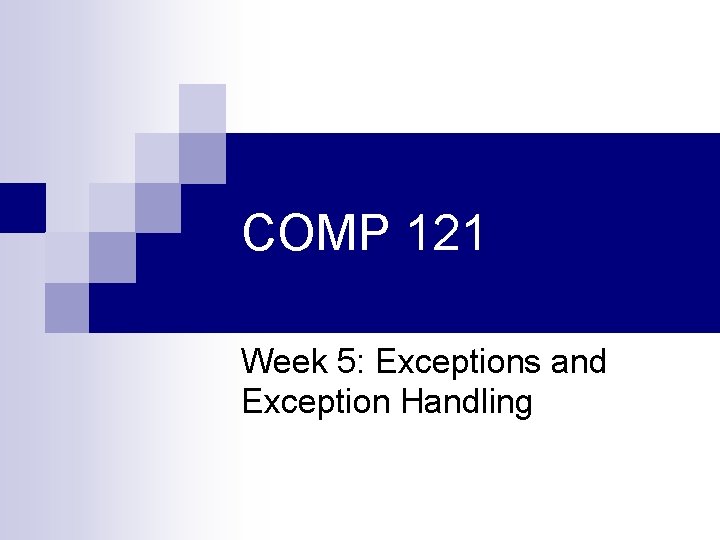
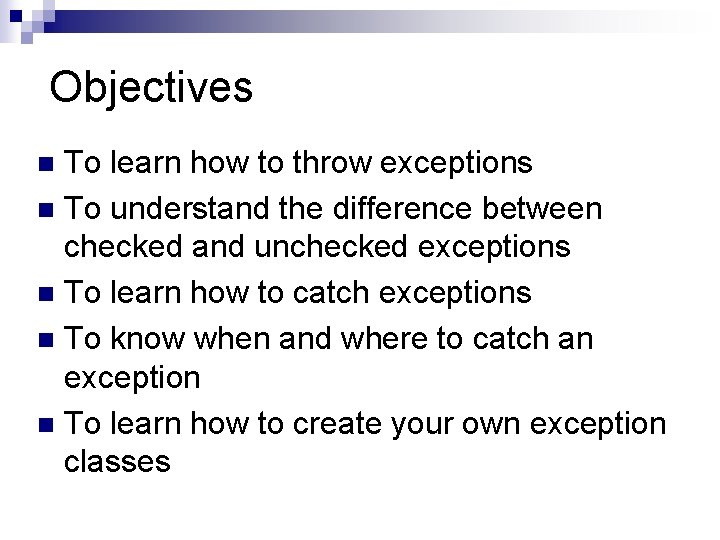
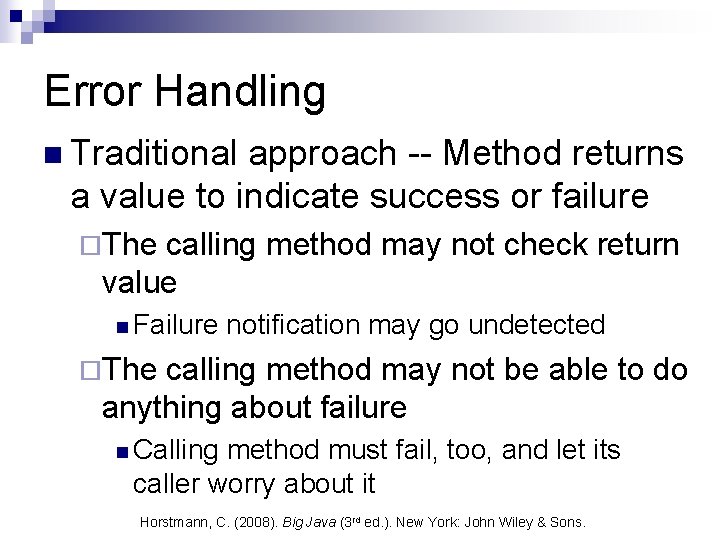
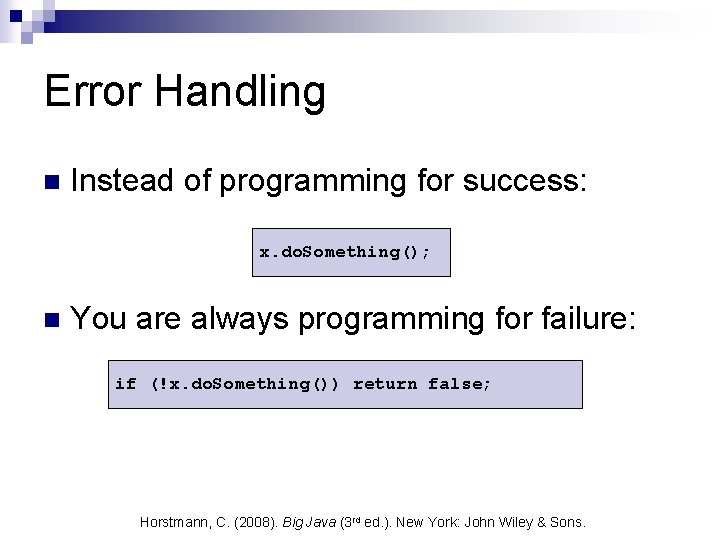
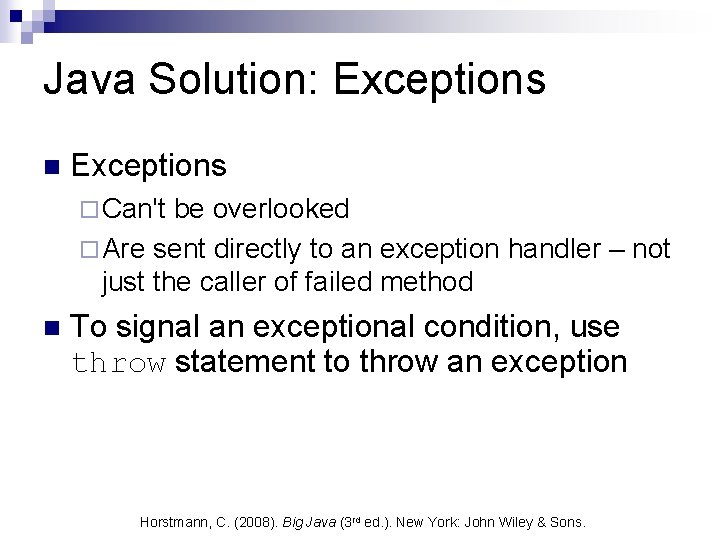
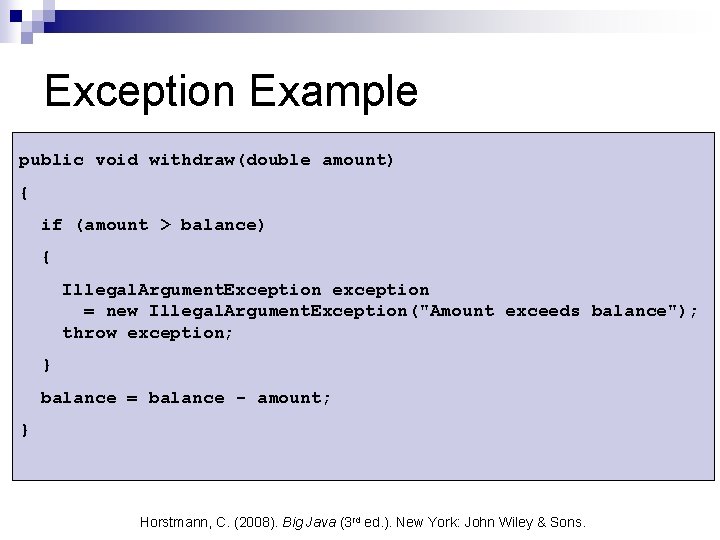
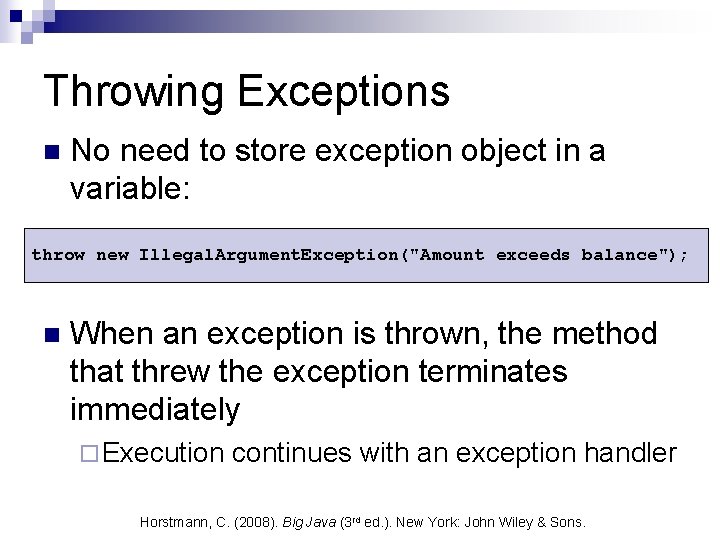
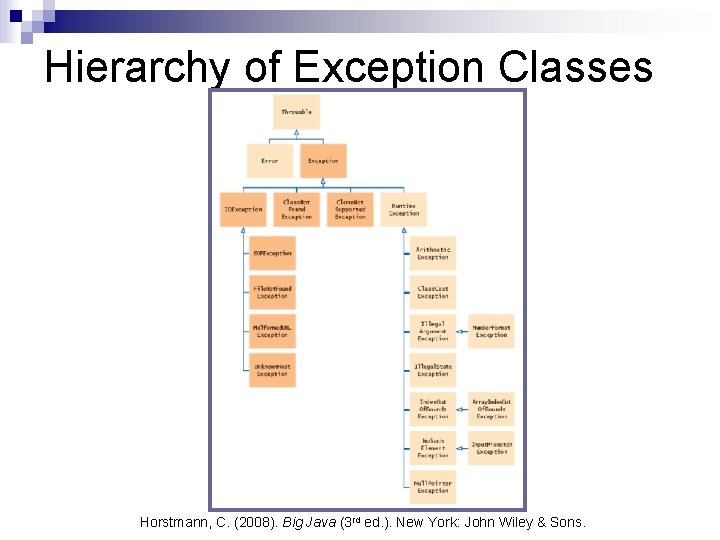
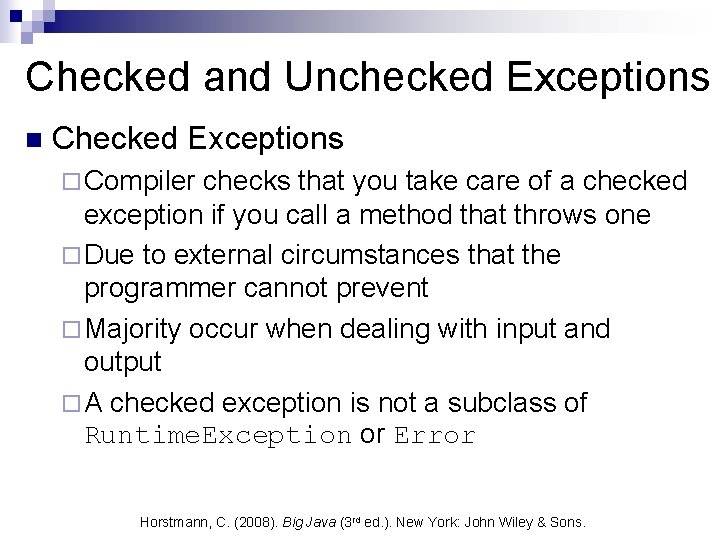
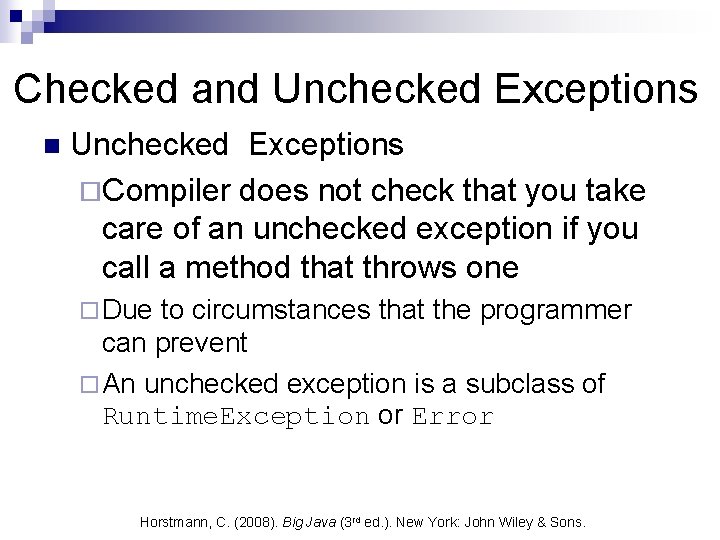
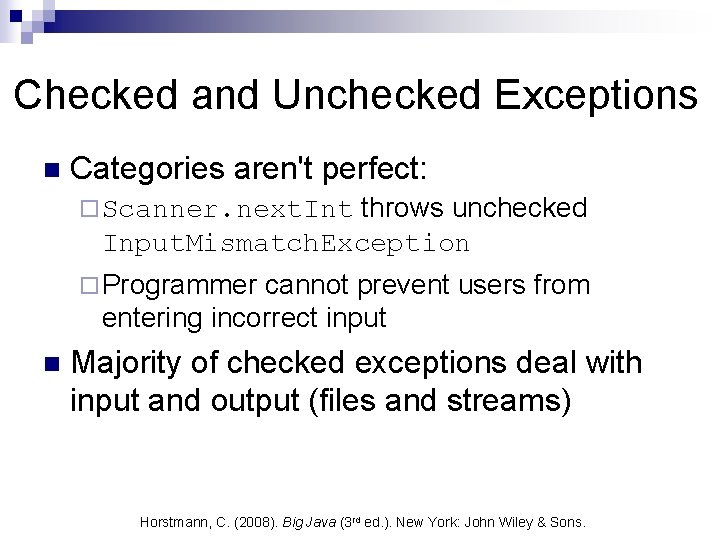
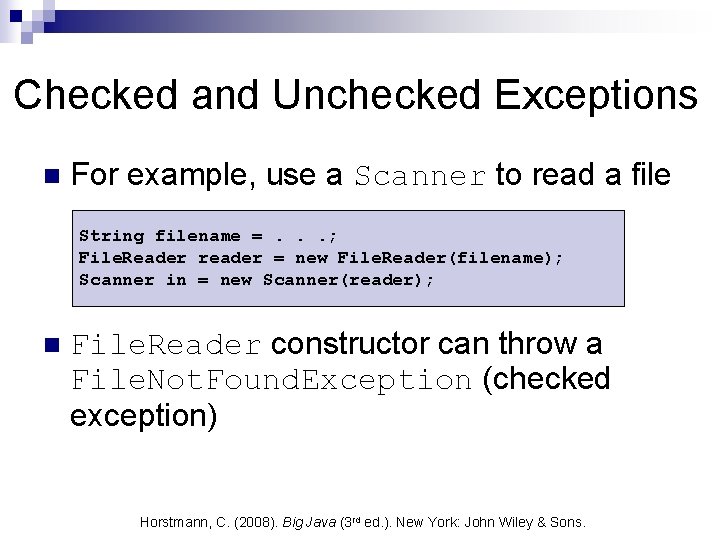
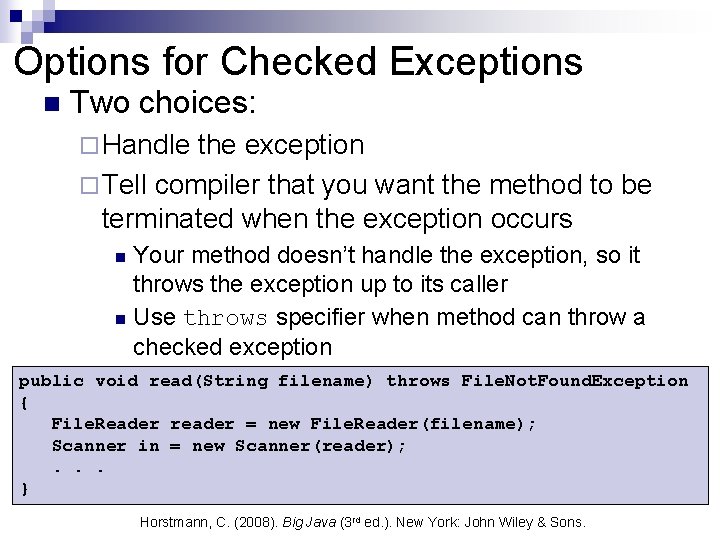
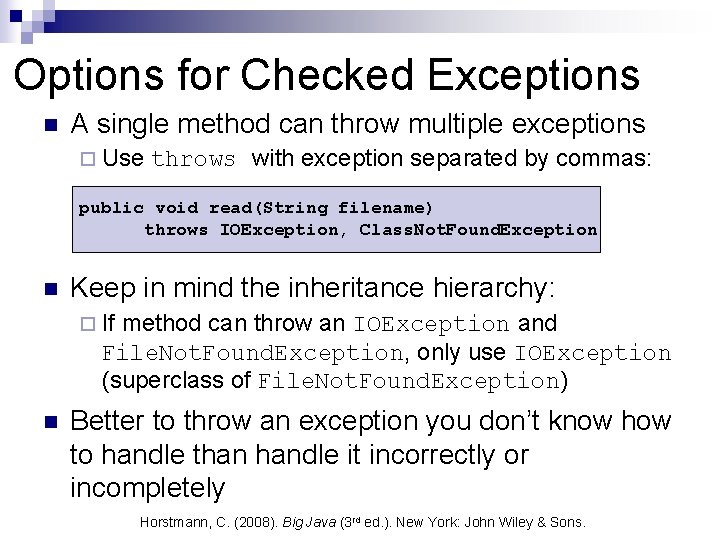
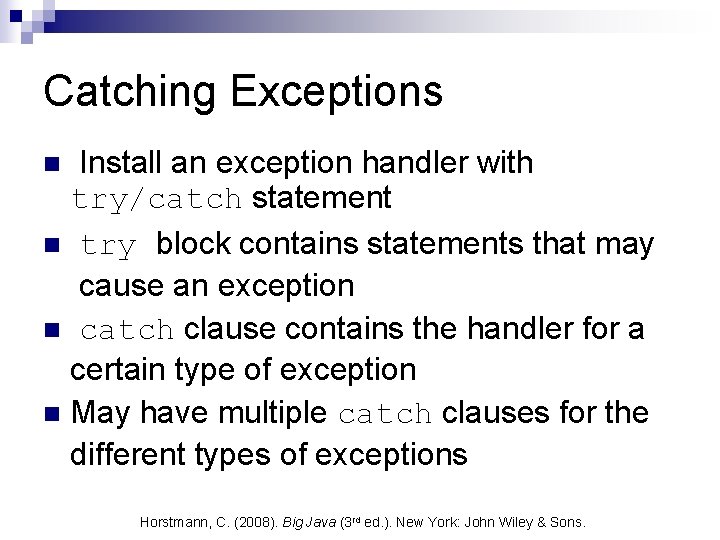
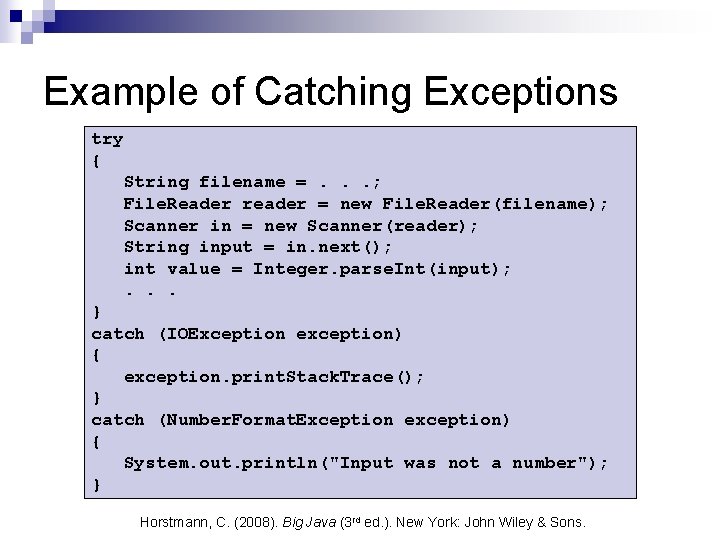
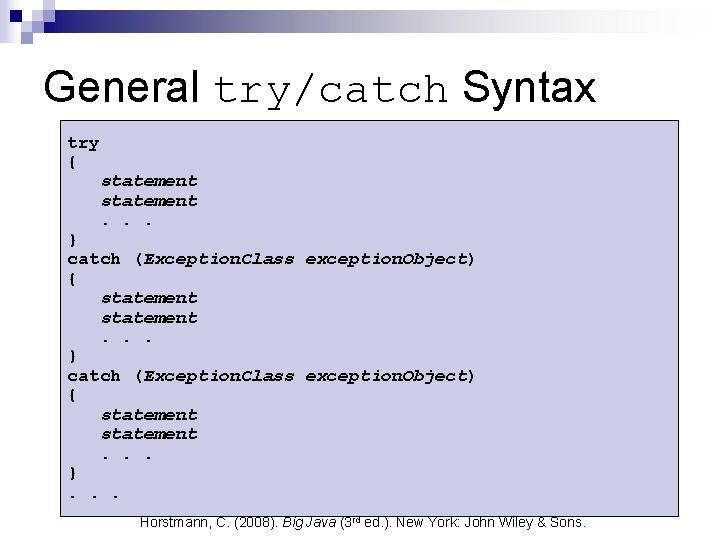
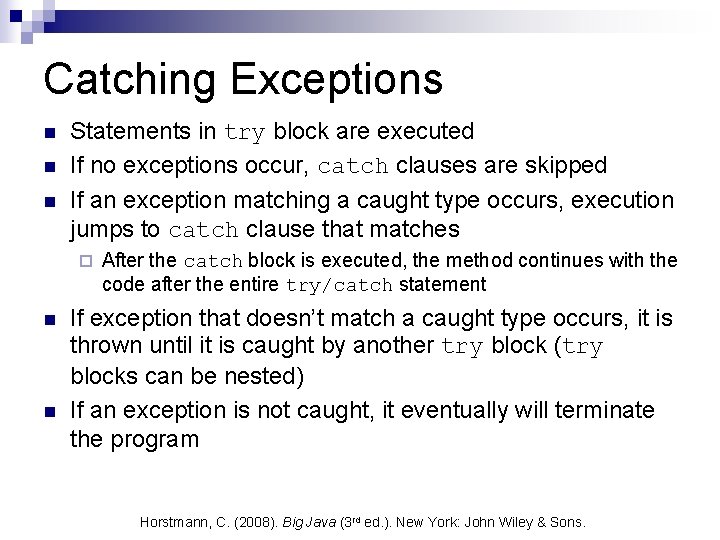
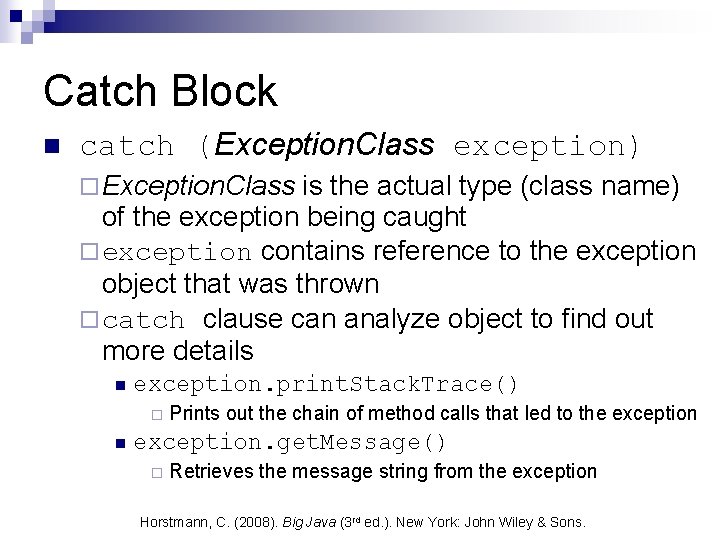
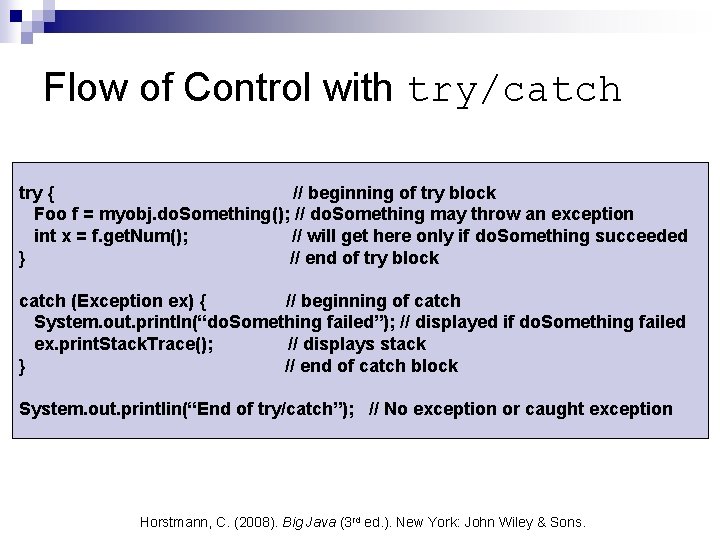
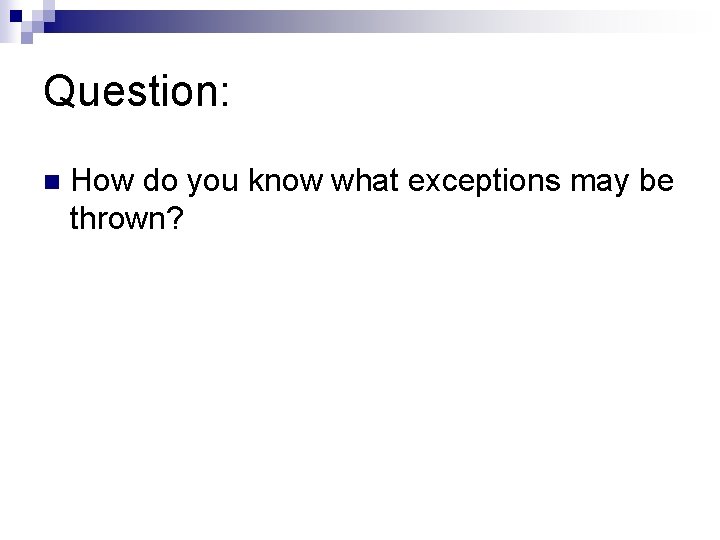
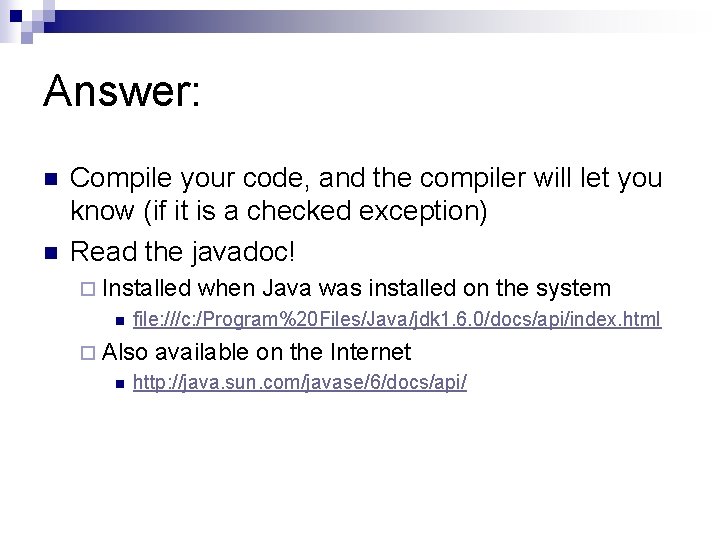
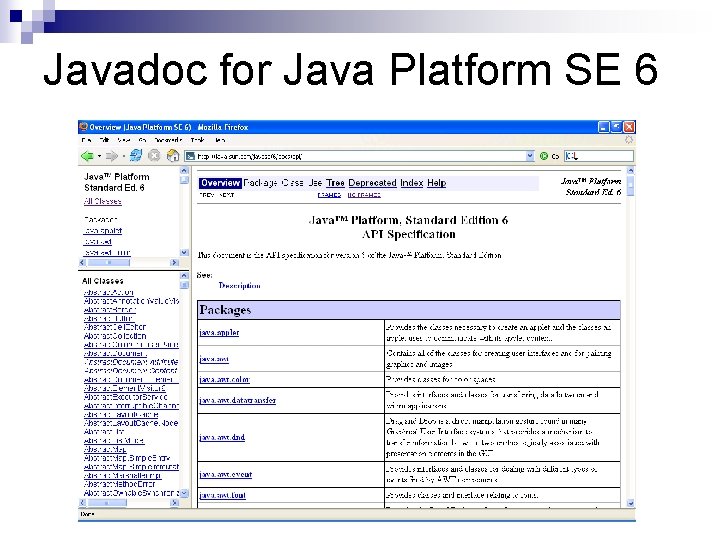
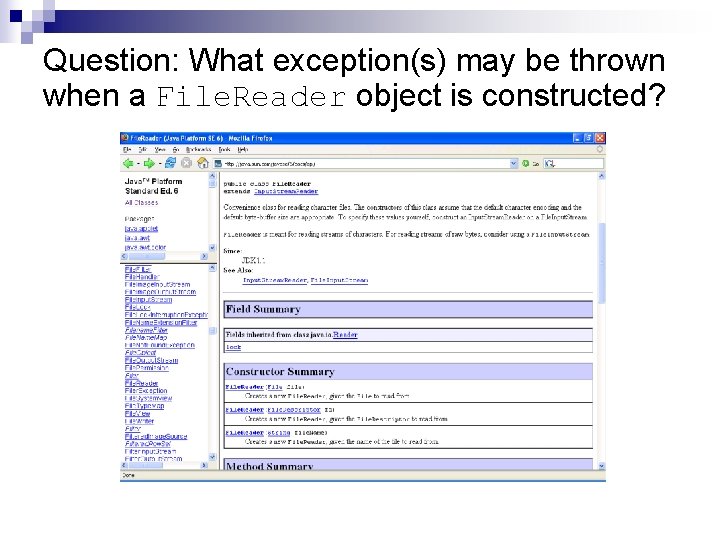
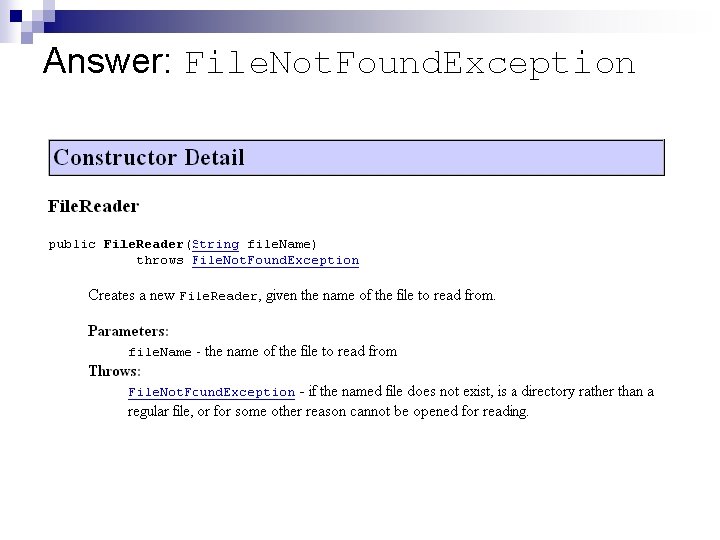
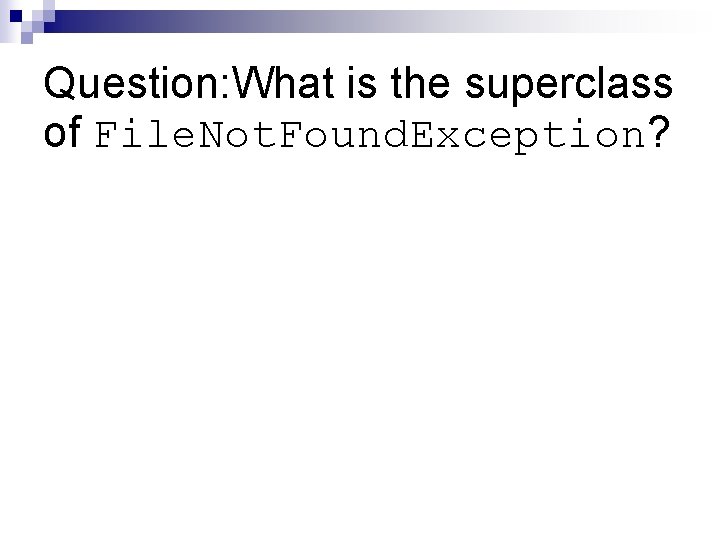
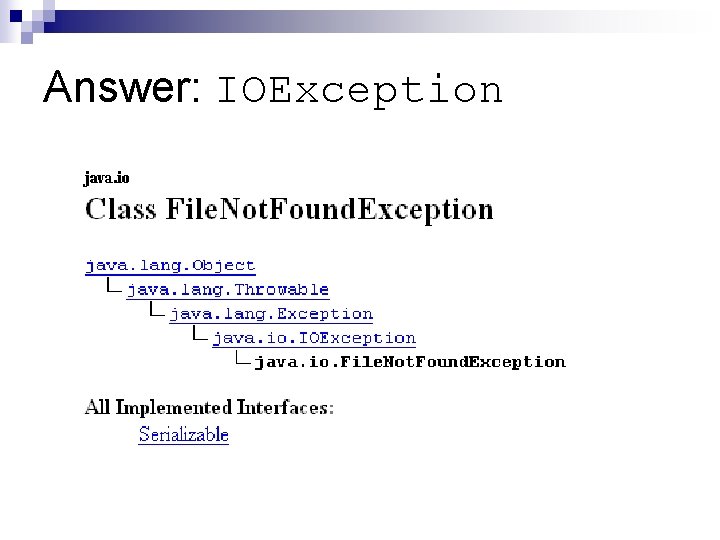
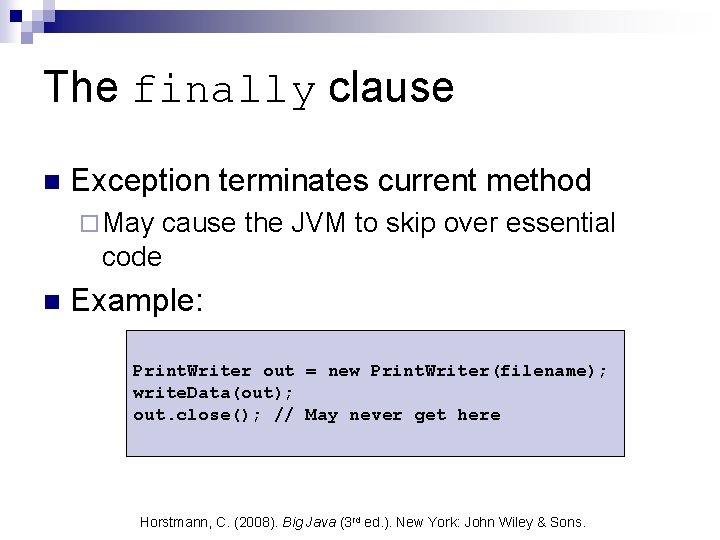
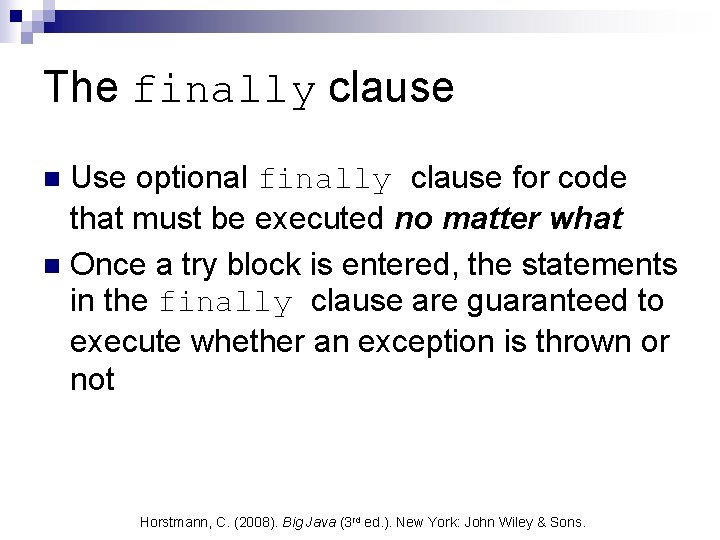
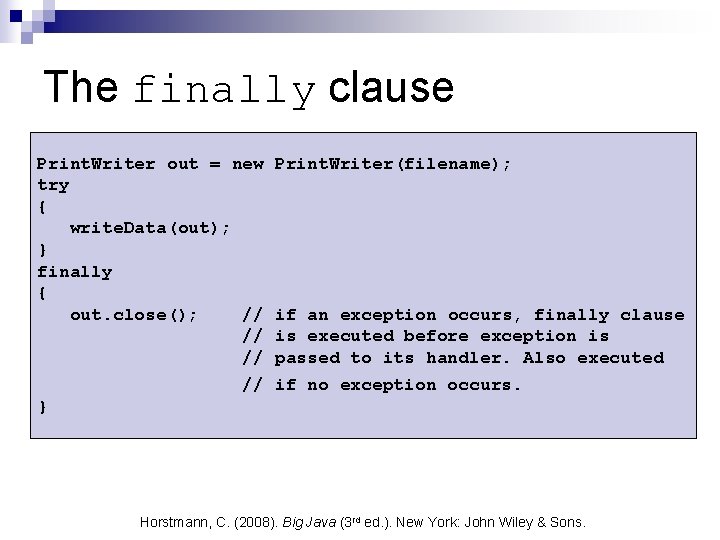
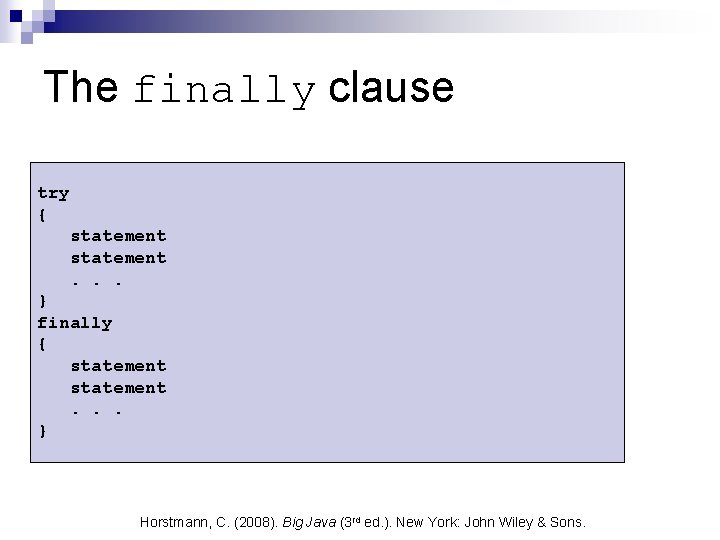
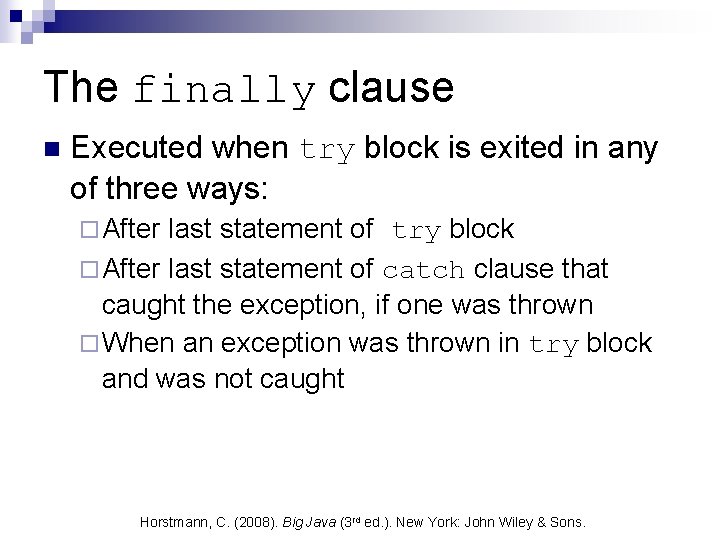
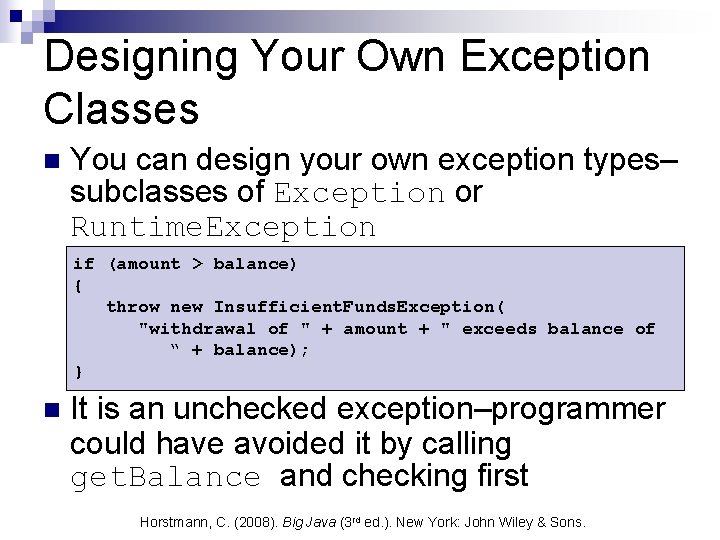
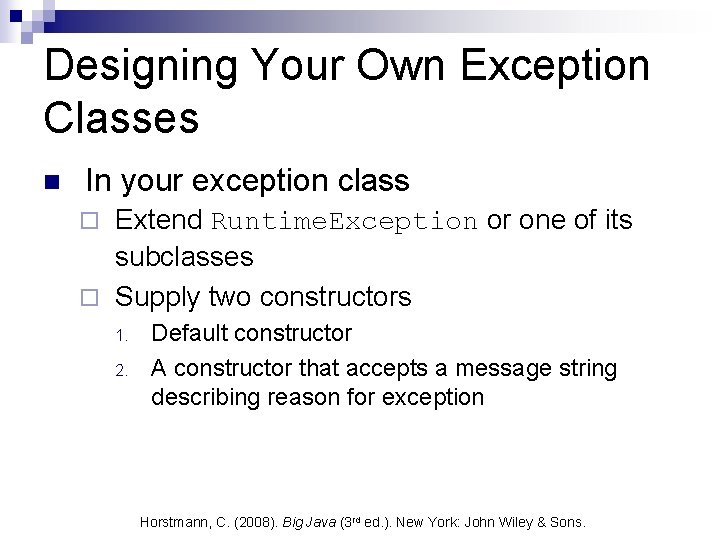
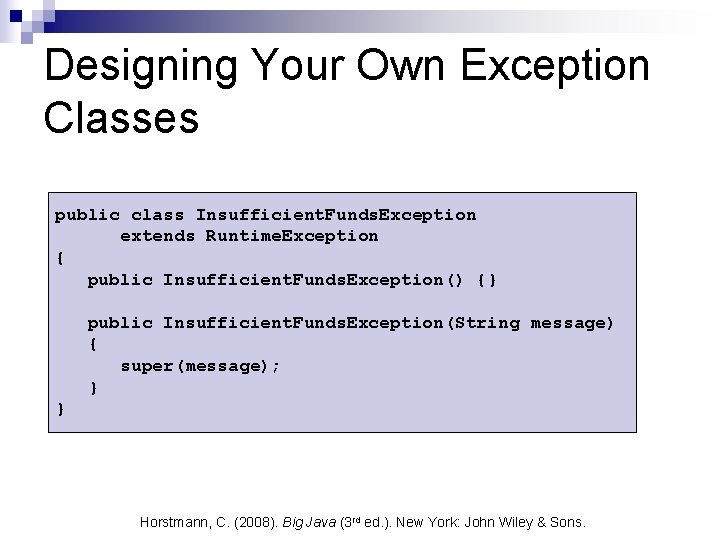
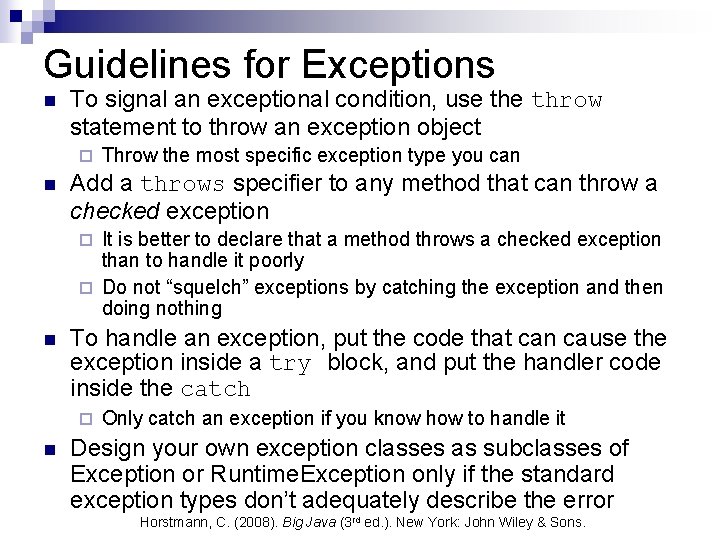
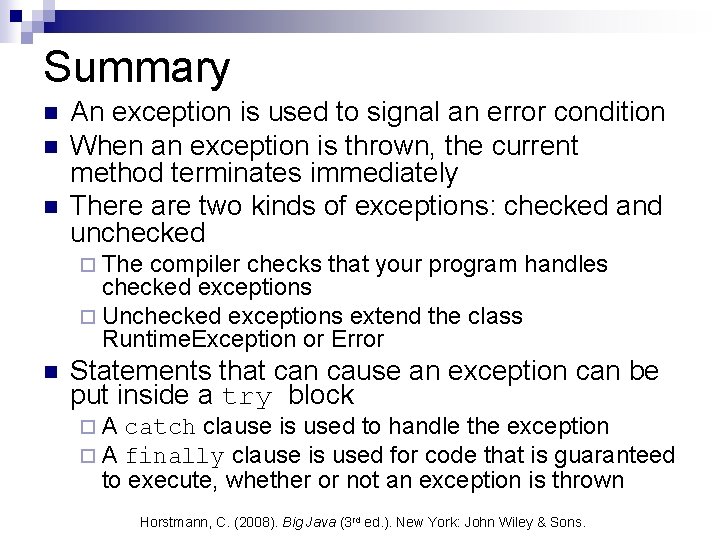
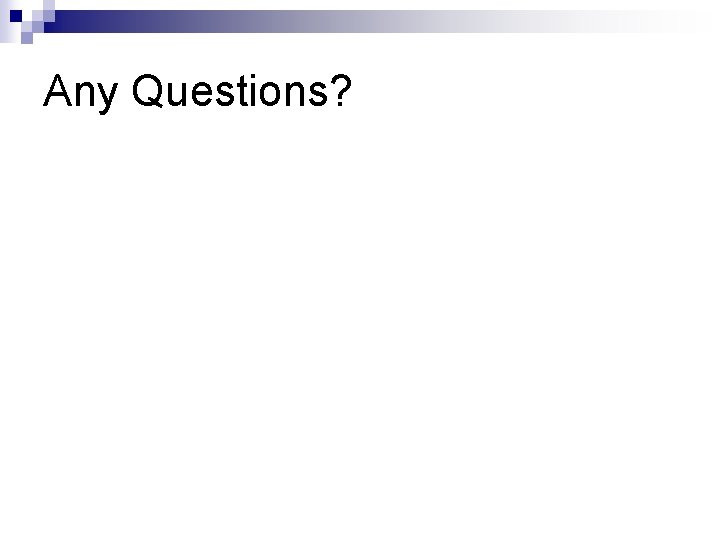
- Slides: 38
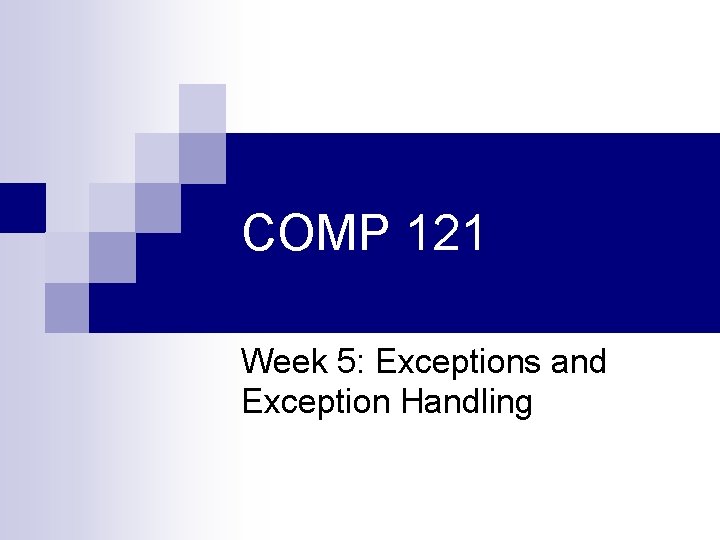
COMP 121 Week 5: Exceptions and Exception Handling
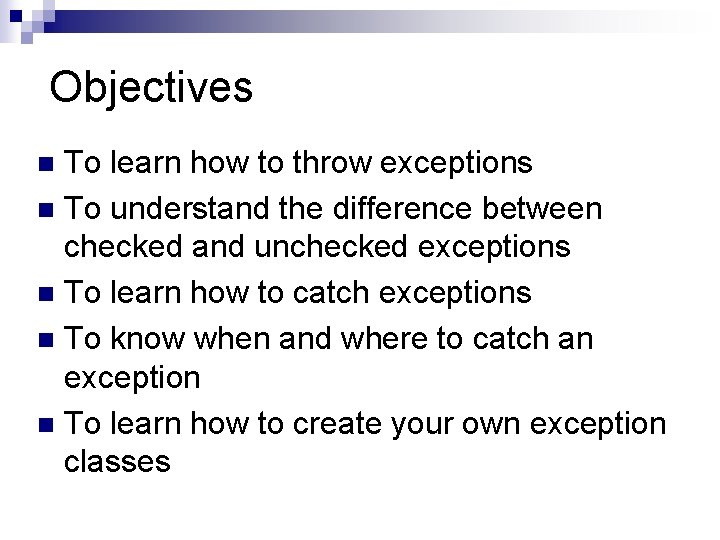
Objectives To learn how to throw exceptions n To understand the difference between checked and unchecked exceptions n To learn how to catch exceptions n To know when and where to catch an exception n To learn how to create your own exception classes n
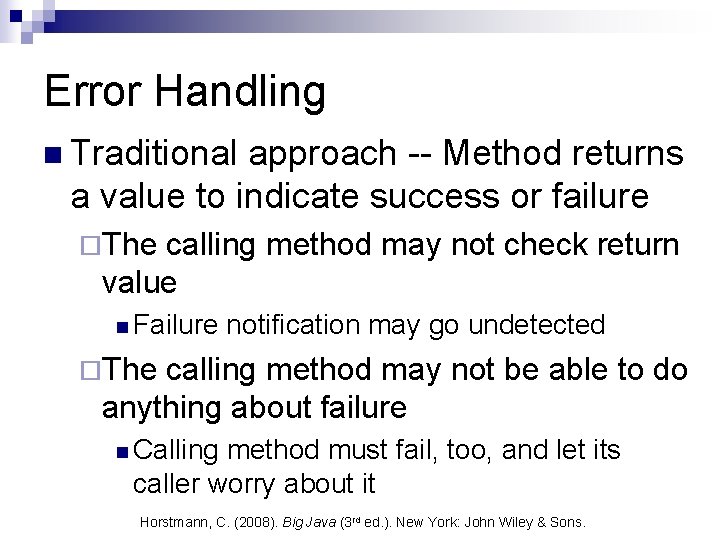
Error Handling n Traditional approach -- Method returns a value to indicate success or failure ¨The calling method may not check return value n Failure notification may go undetected ¨The calling method may not be able to do anything about failure n Calling method must fail, too, and let its caller worry about it Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
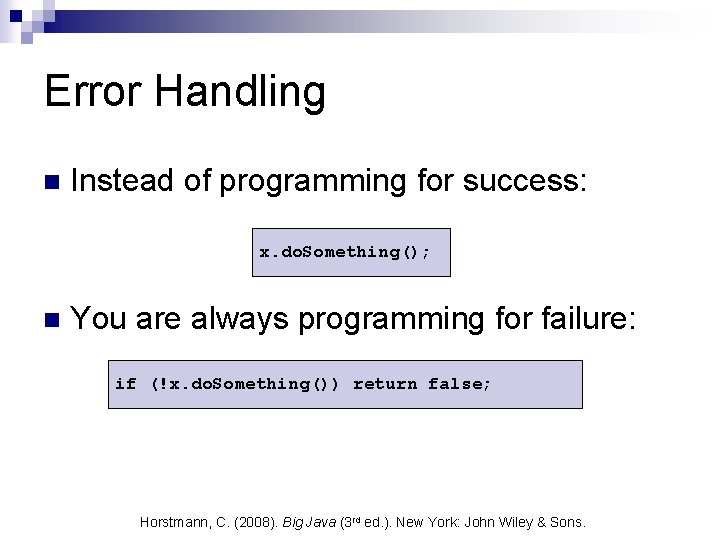
Error Handling n Instead of programming for success: x. do. Something(); n You are always programming for failure: if (!x. do. Something()) return false; Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
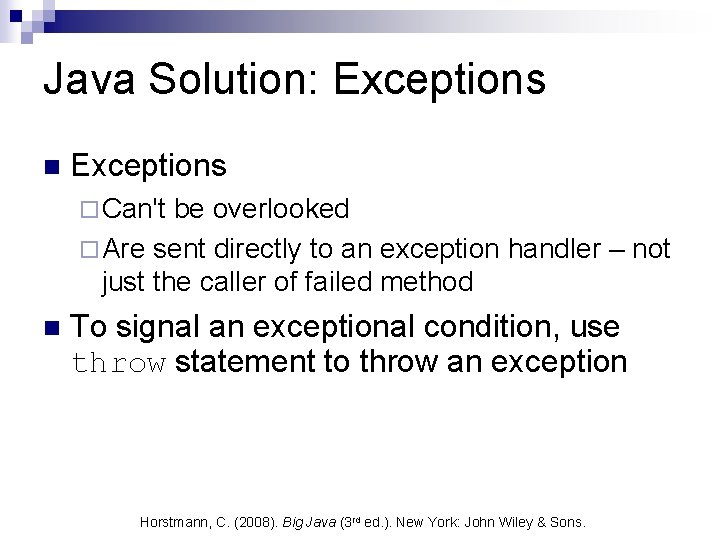
Java Solution: Exceptions n Exceptions ¨ Can't be overlooked ¨ Are sent directly to an exception handler – not just the caller of failed method n To signal an exceptional condition, use throw statement to throw an exception Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
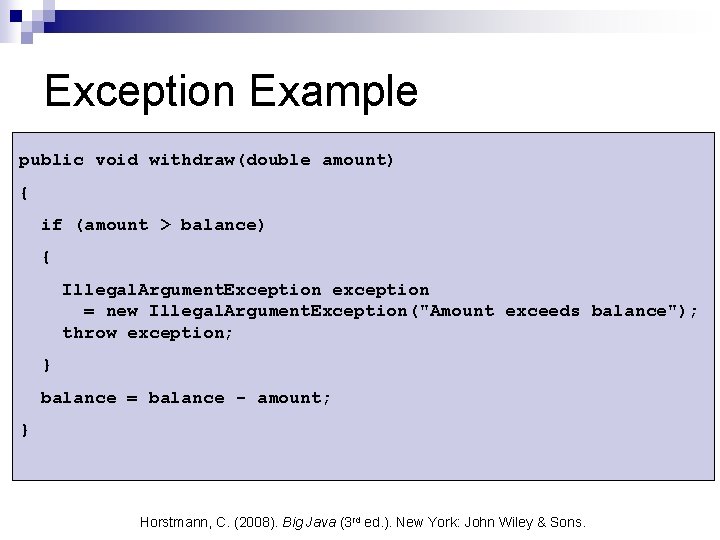
Exception Example public void withdraw(double amount) { if (amount > balance) { Illegal. Argument. Exception exception = new Illegal. Argument. Exception("Amount exceeds balance"); throw exception; } balance = balance - amount; } Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
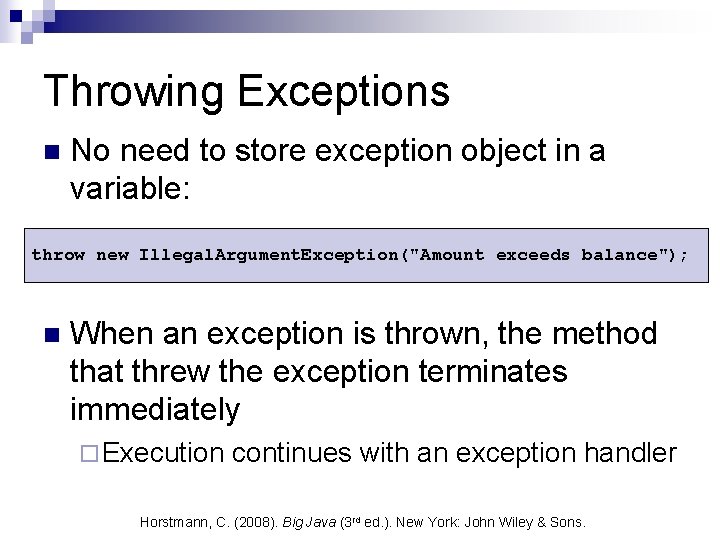
Throwing Exceptions n No need to store exception object in a variable: throw new Illegal. Argument. Exception("Amount exceeds balance"); n When an exception is thrown, the method that threw the exception terminates immediately ¨ Execution continues with an exception handler Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
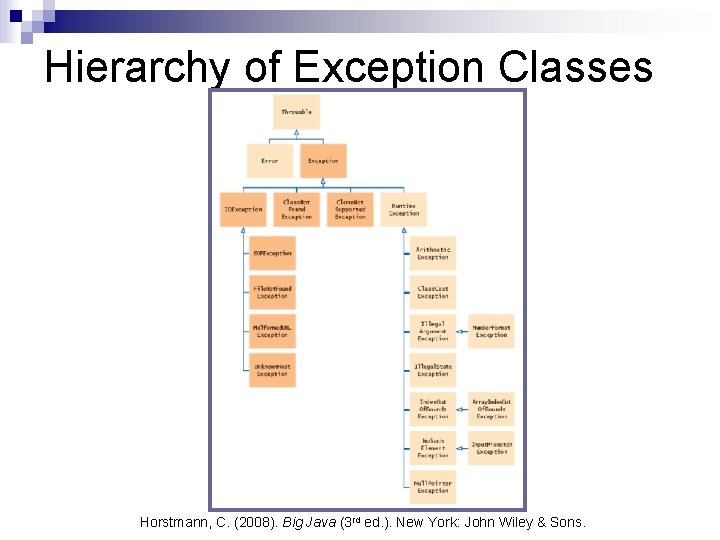
Hierarchy of Exception Classes Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
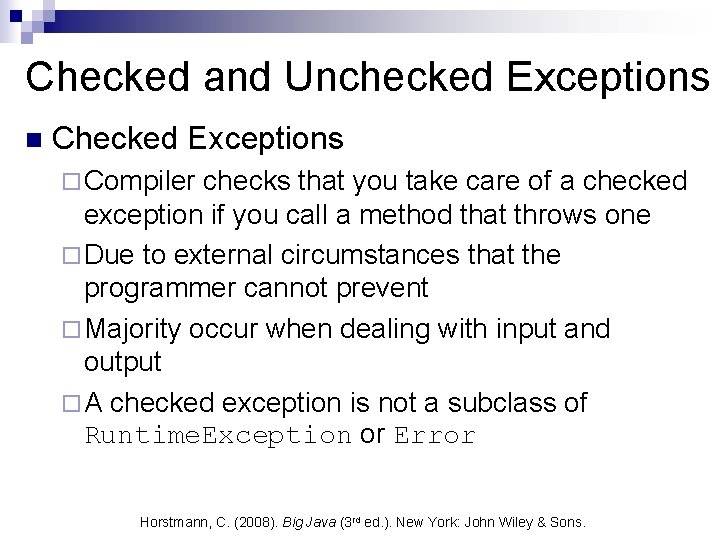
Checked and Unchecked Exceptions n Checked Exceptions ¨ Compiler checks that you take care of a checked exception if you call a method that throws one ¨ Due to external circumstances that the programmer cannot prevent ¨ Majority occur when dealing with input and output ¨ A checked exception is not a subclass of Runtime. Exception or Error Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
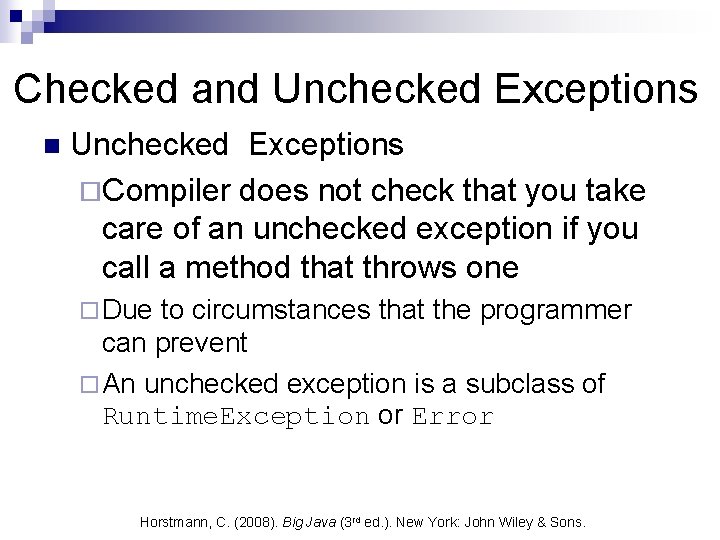
Checked and Unchecked Exceptions n Unchecked Exceptions ¨Compiler does not check that you take care of an unchecked exception if you call a method that throws one ¨ Due to circumstances that the programmer can prevent ¨ An unchecked exception is a subclass of Runtime. Exception or Error Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
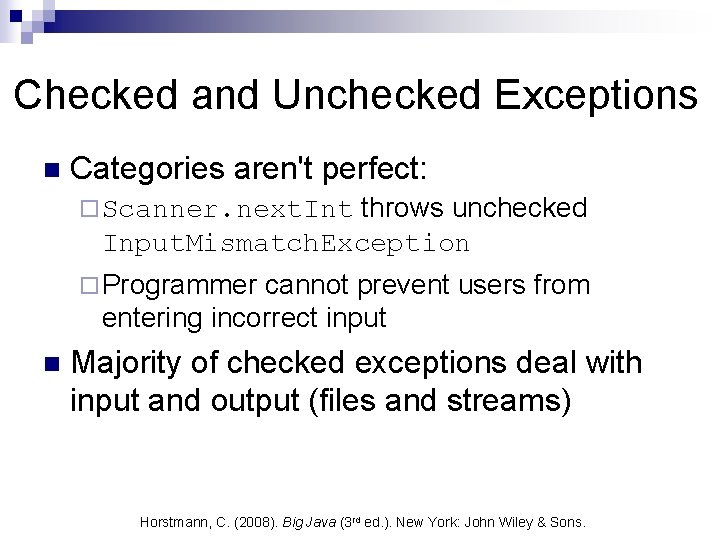
Checked and Unchecked Exceptions n Categories aren't perfect: throws unchecked Input. Mismatch. Exception ¨ Scanner. next. Int ¨ Programmer cannot prevent users from entering incorrect input n Majority of checked exceptions deal with input and output (files and streams) Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
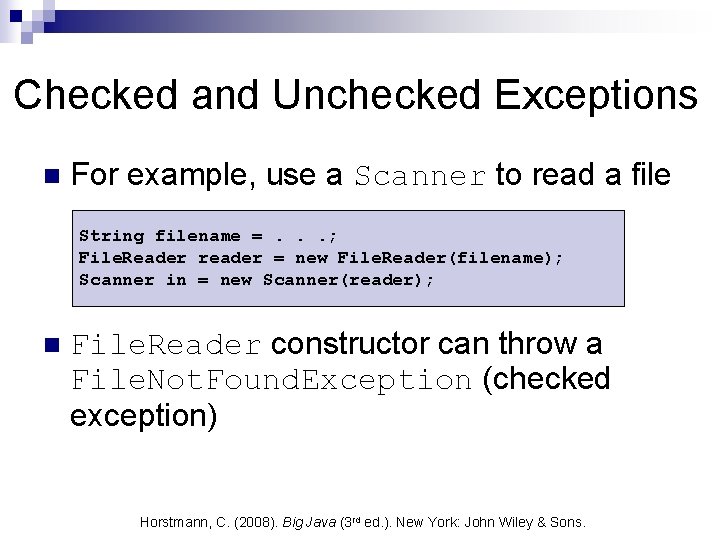
Checked and Unchecked Exceptions n For example, use a Scanner to read a file String filename =. . . ; File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); n File. Reader constructor can throw a File. Not. Found. Exception (checked exception) Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
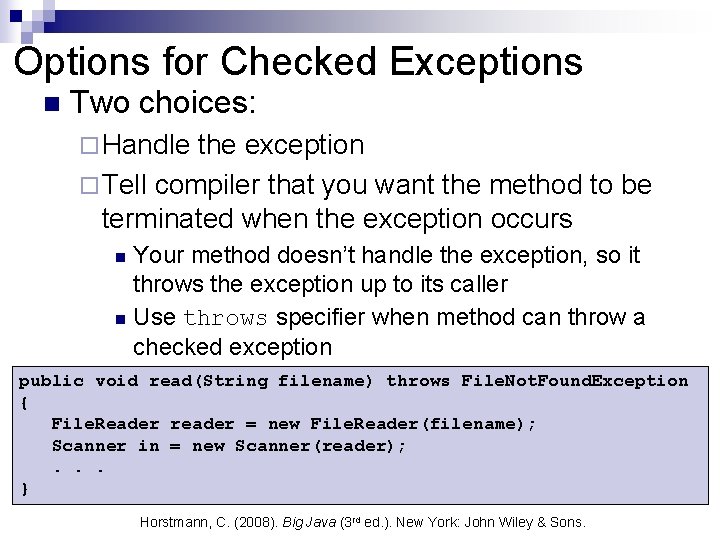
Options for Checked Exceptions n Two choices: ¨ Handle the exception ¨ Tell compiler that you want the method to be terminated when the exception occurs Your method doesn’t handle the exception, so it throws the exception up to its caller n Use throws specifier when method can throw a checked exception n public void read(String filename) throws File. Not. Found. Exception { File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); . . . } Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
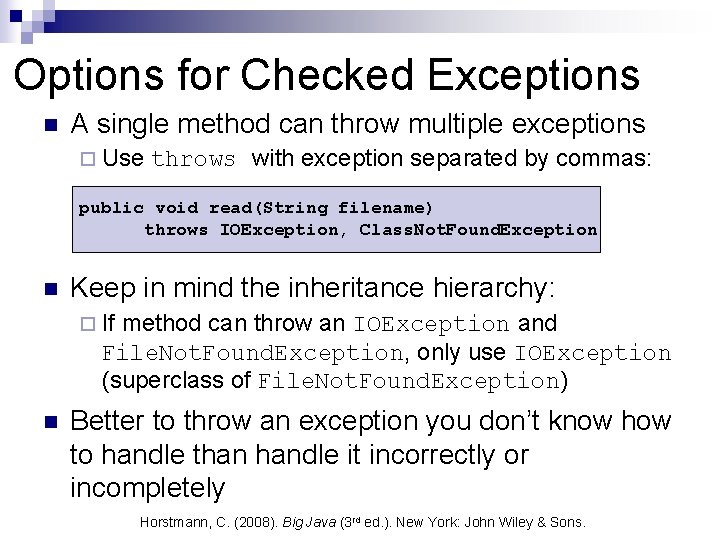
Options for Checked Exceptions n A single method can throw multiple exceptions ¨ Use throws with exception separated by commas: public void read(String filename) throws IOException, Class. Not. Found. Exception n Keep in mind the inheritance hierarchy: ¨ If method can throw an IOException and File. Not. Found. Exception, only use IOException (superclass of File. Not. Found. Exception) n Better to throw an exception you don’t know how to handle than handle it incorrectly or incompletely Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
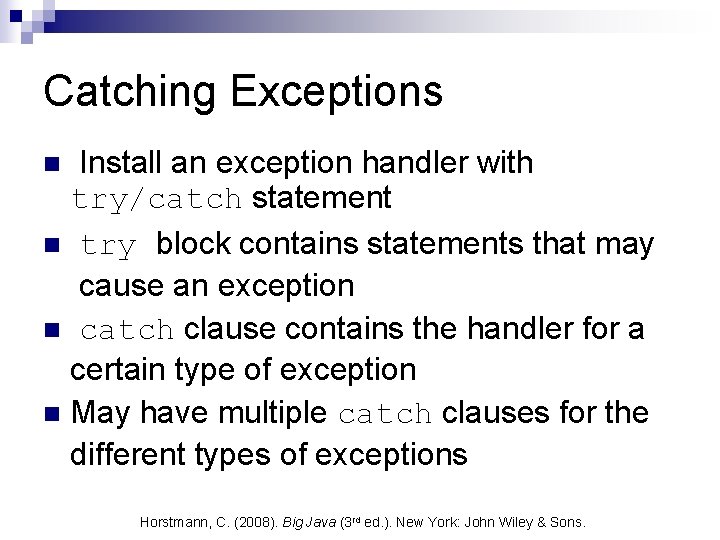
Catching Exceptions Install an exception handler with try/catch statement n try block contains statements that may cause an exception n catch clause contains the handler for a certain type of exception n May have multiple catch clauses for the different types of exceptions n Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
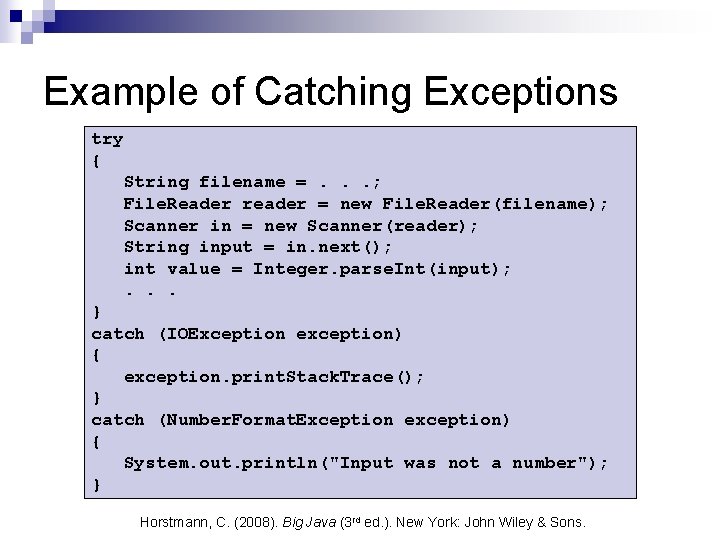
Example of Catching Exceptions try { String filename =. . . ; File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); String input = in. next(); int value = Integer. parse. Int(input); . . . } catch (IOException exception) { exception. print. Stack. Trace(); } catch (Number. Format. Exception exception) { System. out. println("Input was not a number"); } Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
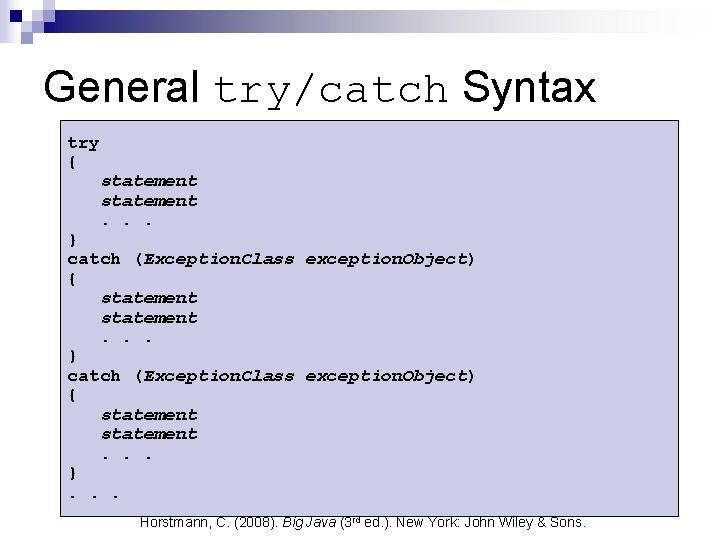
General try/catch Syntax try { statement. . . } catch (Exception. Class exception. Object) { statement. . . }. . . Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
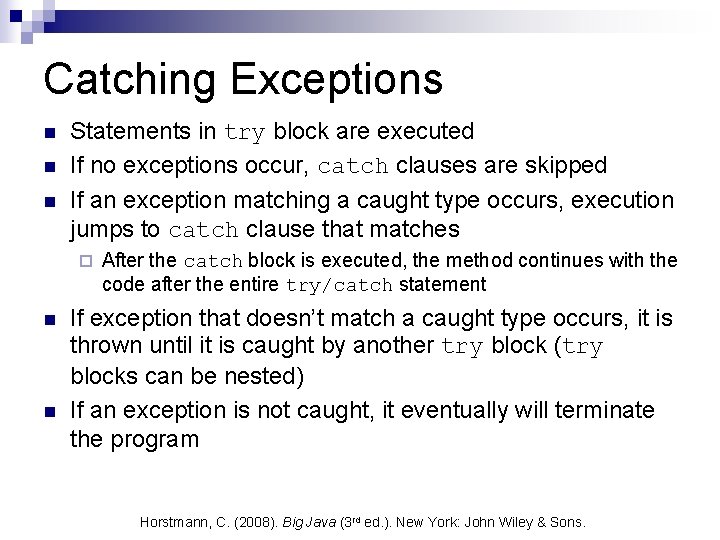
Catching Exceptions n n n Statements in try block are executed If no exceptions occur, catch clauses are skipped If an exception matching a caught type occurs, execution jumps to catch clause that matches ¨ n n After the catch block is executed, the method continues with the code after the entire try/catch statement If exception that doesn’t match a caught type occurs, it is thrown until it is caught by another try block (try blocks can be nested) If an exception is not caught, it eventually will terminate the program Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
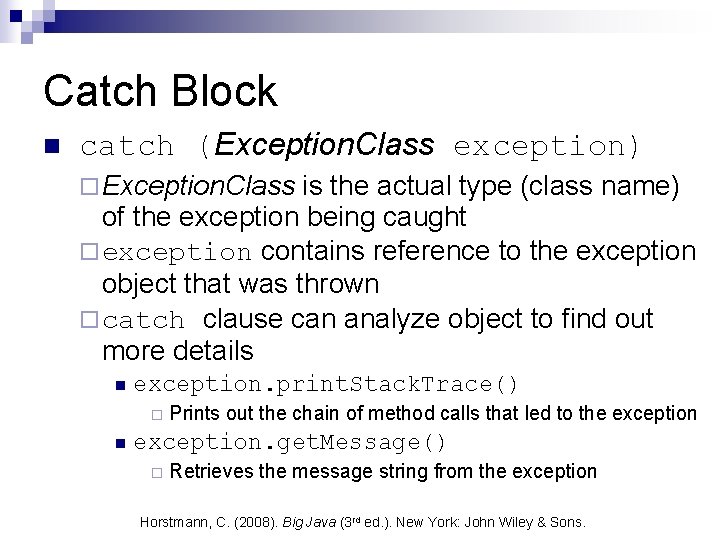
Catch Block n catch (Exception. Class exception) ¨ Exception. Class is the actual type (class name) of the exception being caught ¨ exception contains reference to the exception object that was thrown ¨ catch clause can analyze object to find out more details n exception. print. Stack. Trace() ¨ n Prints out the chain of method calls that led to the exception. get. Message() ¨ Retrieves the message string from the exception Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
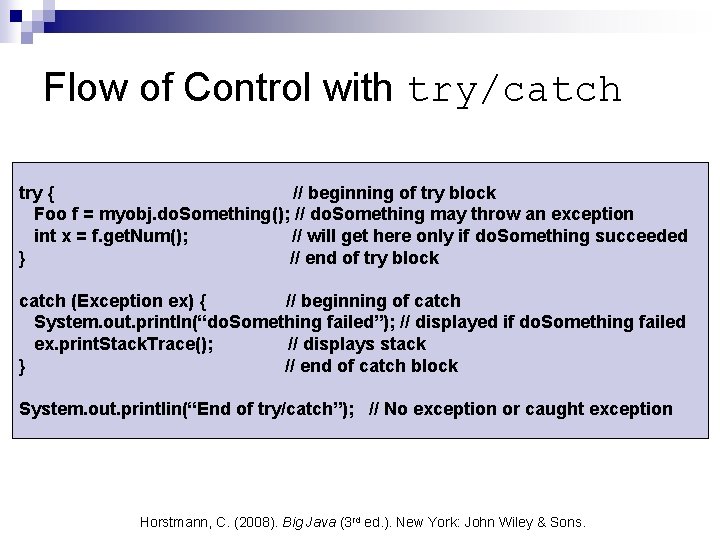
Flow of Control with try/catch try { // beginning of try block Foo f = myobj. do. Something(); // do. Something may throw an exception int x = f. get. Num(); // will get here only if do. Something succeeded } // end of try block catch (Exception ex) { // beginning of catch System. out. println(“do. Something failed”); // displayed if do. Something failed ex. print. Stack. Trace(); // displays stack } // end of catch block System. out. printlin(“End of try/catch”); // No exception or caught exception Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
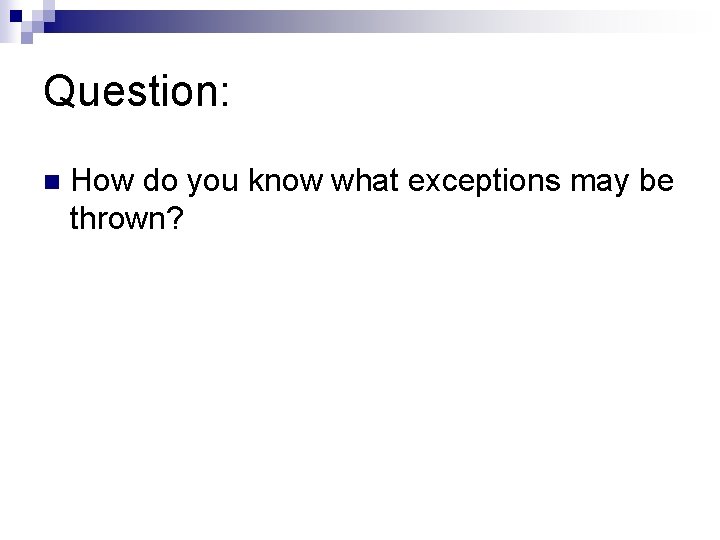
Question: n How do you know what exceptions may be thrown?
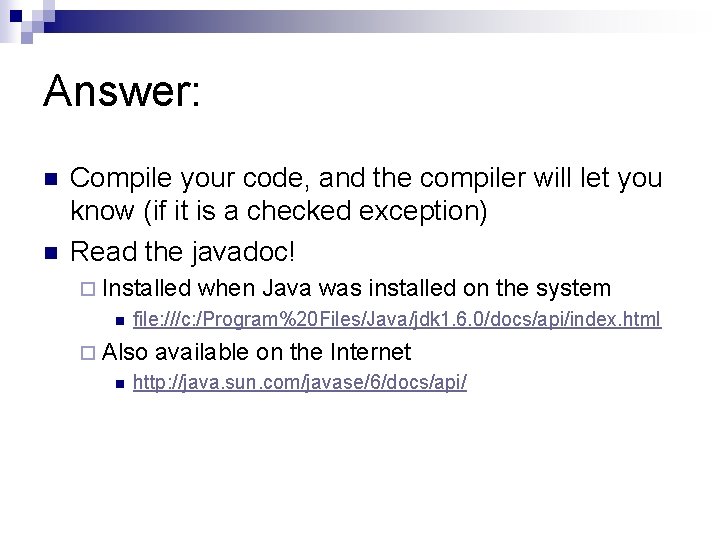
Answer: n n Compile your code, and the compiler will let you know (if it is a checked exception) Read the javadoc! ¨ Installed n file: ///c: /Program%20 Files/Java/jdk 1. 6. 0/docs/api/index. html ¨ Also n when Java was installed on the system available on the Internet http: //java. sun. com/javase/6/docs/api/
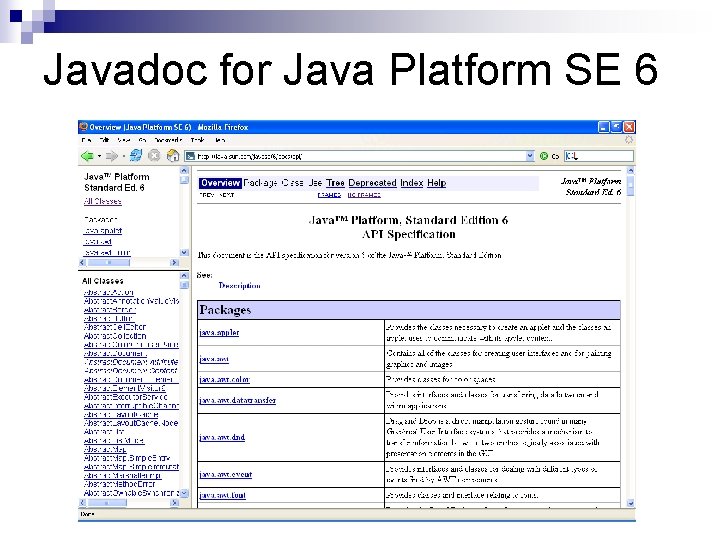
Javadoc for Java Platform SE 6
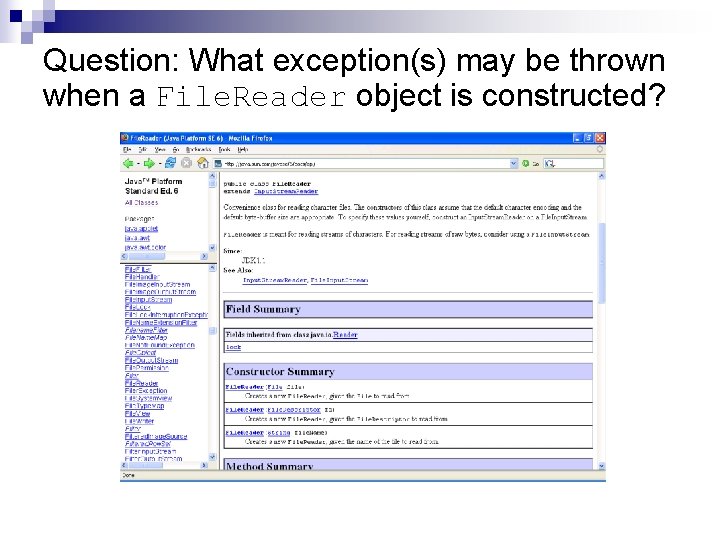
Question: What exception(s) may be thrown when a File. Reader object is constructed?
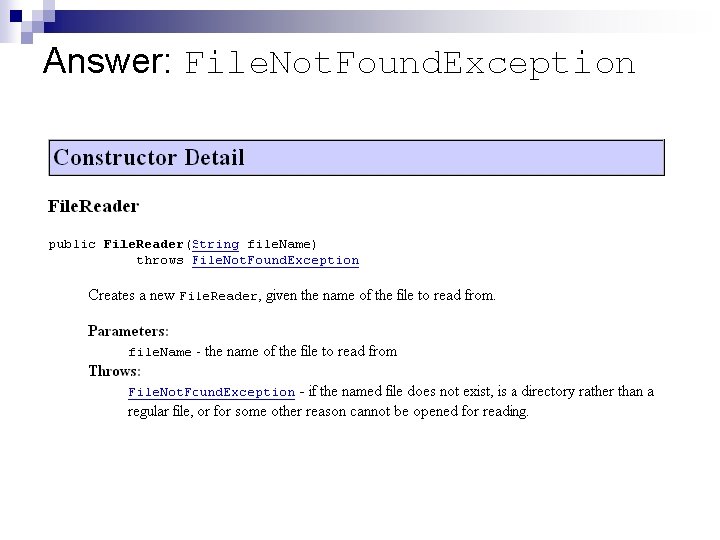
Answer: File. Not. Found. Exception
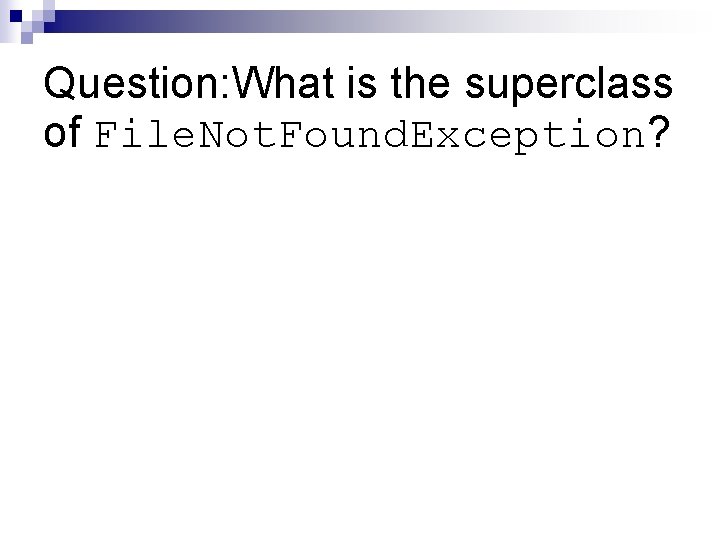
Question: What is the superclass of File. Not. Found. Exception?
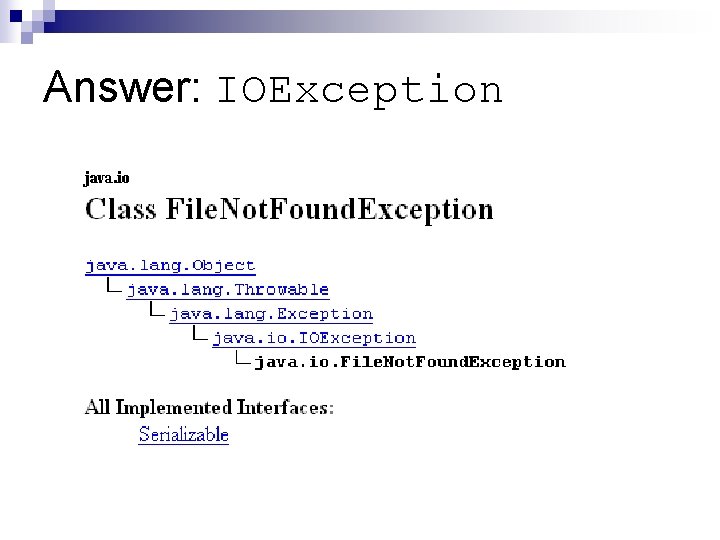
Answer: IOException
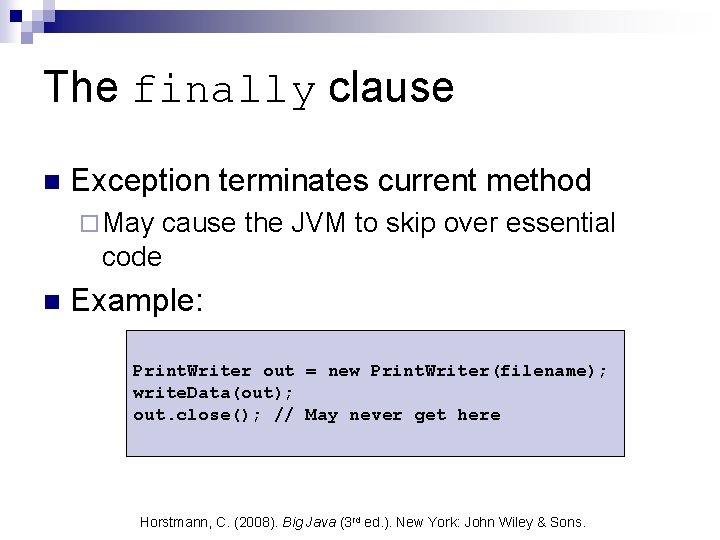
The finally clause n Exception terminates current method ¨ May cause the JVM to skip over essential code n Example: Print. Writer out = new Print. Writer(filename); write. Data(out); out. close(); // May never get here Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
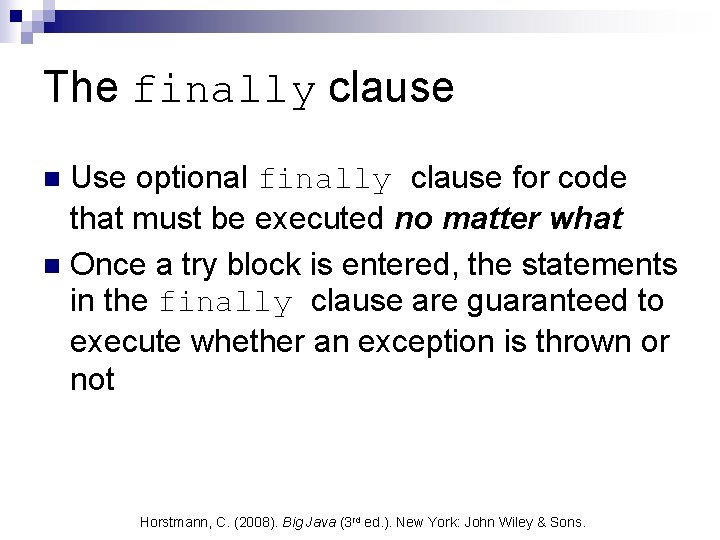
The finally clause Use optional finally clause for code that must be executed no matter what n Once a try block is entered, the statements in the finally clause are guaranteed to execute whether an exception is thrown or not n Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
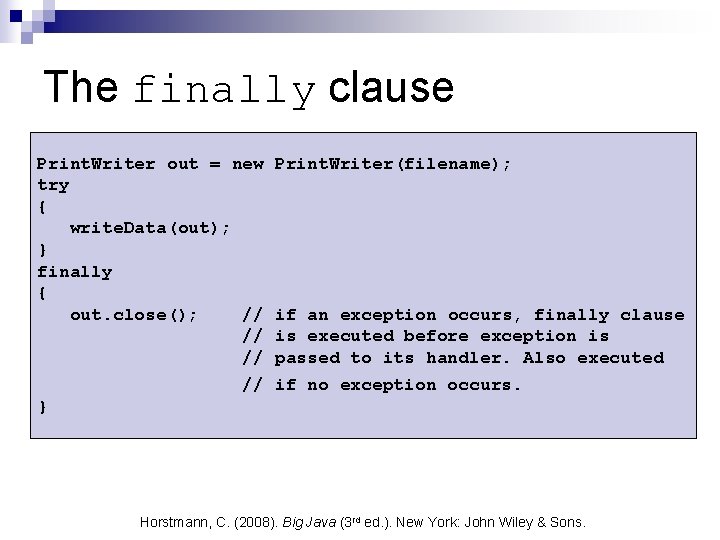
The finally clause Print. Writer out = new try { write. Data(out); } finally { out. close(); // // } Print. Writer(filename); if an exception occurs, finally clause is executed before exception is passed to its handler. Also executed if no exception occurs. Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
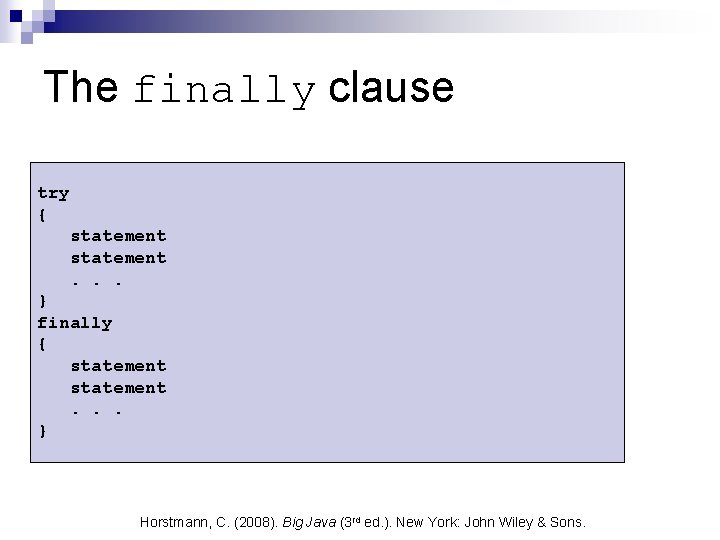
The finally clause try { statement. . . } finally { statement. . . } Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
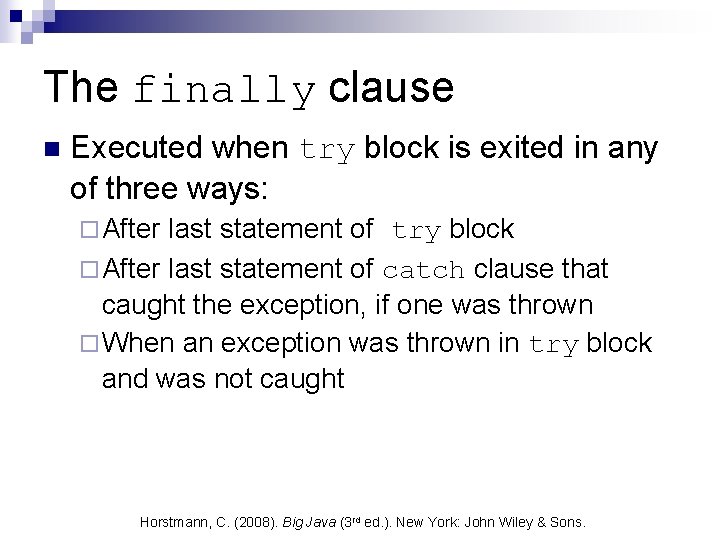
The finally clause n Executed when try block is exited in any of three ways: ¨ After last statement of try block ¨ After last statement of catch clause that caught the exception, if one was thrown ¨ When an exception was thrown in try block and was not caught Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
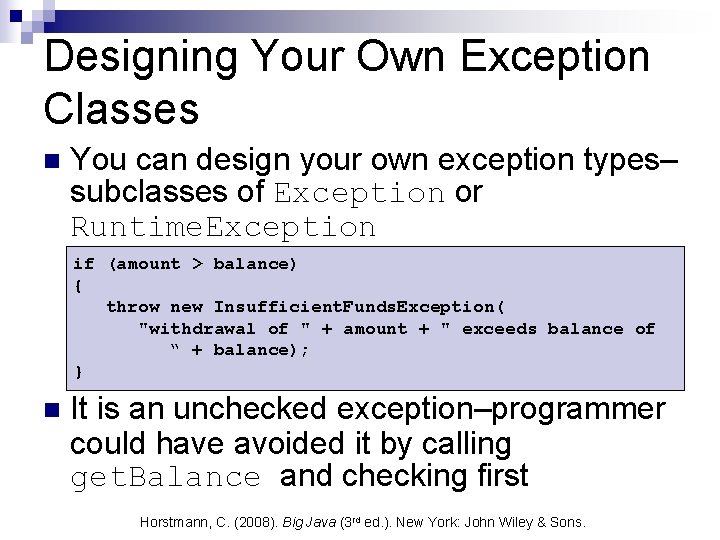
Designing Your Own Exception Classes n You can design your own exception types– subclasses of Exception or Runtime. Exception if (amount > balance) { throw new Insufficient. Funds. Exception( "withdrawal of " + amount + " exceeds balance of “ + balance); } n It is an unchecked exception–programmer could have avoided it by calling get. Balance and checking first Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
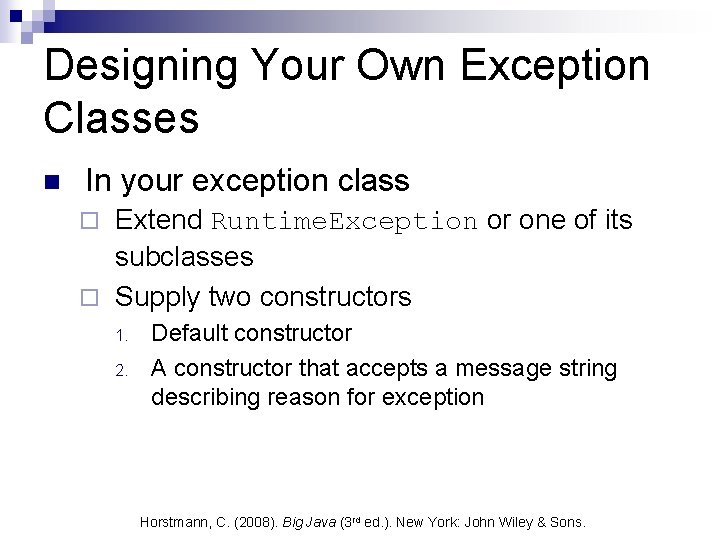
Designing Your Own Exception Classes n In your exception class Extend Runtime. Exception or one of its subclasses ¨ Supply two constructors ¨ 1. 2. Default constructor A constructor that accepts a message string describing reason for exception Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
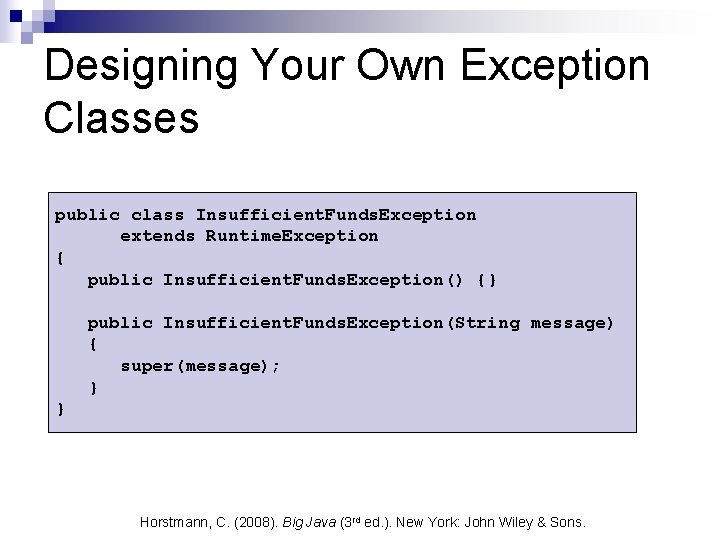
Designing Your Own Exception Classes public class Insufficient. Funds. Exception extends Runtime. Exception { public Insufficient. Funds. Exception() {} public Insufficient. Funds. Exception(String message) { super(message); } } Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
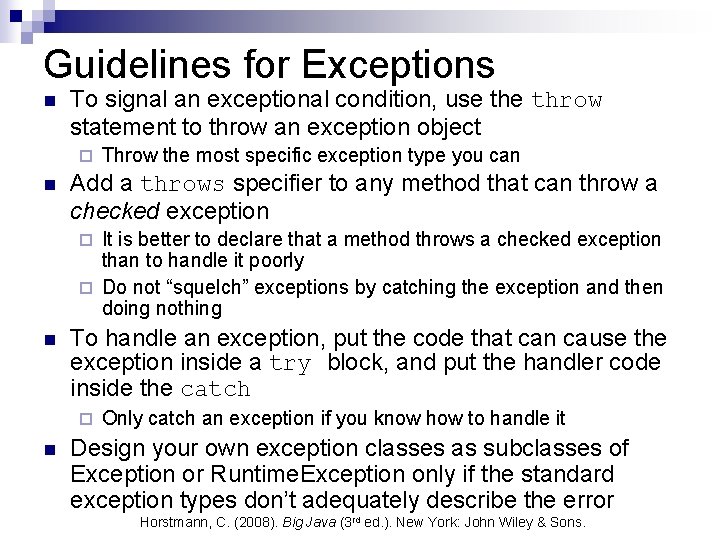
Guidelines for Exceptions n To signal an exceptional condition, use throw statement to throw an exception object ¨ n Throw the most specific exception type you can Add a throws specifier to any method that can throw a checked exception It is better to declare that a method throws a checked exception than to handle it poorly ¨ Do not “squelch” exceptions by catching the exception and then doing nothing ¨ n To handle an exception, put the code that can cause the exception inside a try block, and put the handler code inside the catch ¨ n Only catch an exception if you know how to handle it Design your own exception classes as subclasses of Exception or Runtime. Exception only if the standard exception types don’t adequately describe the error Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
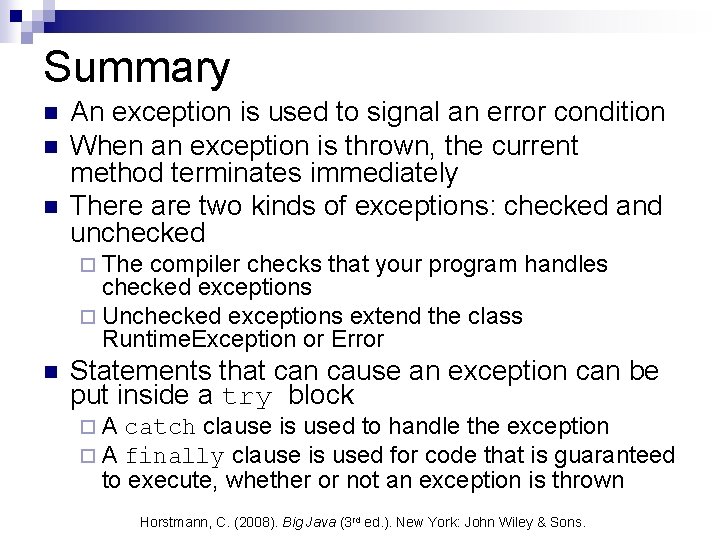
Summary n n n An exception is used to signal an error condition When an exception is thrown, the current method terminates immediately There are two kinds of exceptions: checked and unchecked ¨ The compiler checks that your program handles checked exceptions ¨ Unchecked exceptions extend the class Runtime. Exception or Error n Statements that can cause an exception can be put inside a try block ¨A ¨A catch clause is used to handle the exception finally clause is used for code that is guaranteed to execute, whether or not an exception is thrown Horstmann, C. (2008). Big Java (3 rd ed. ). New York: John Wiley & Sons.
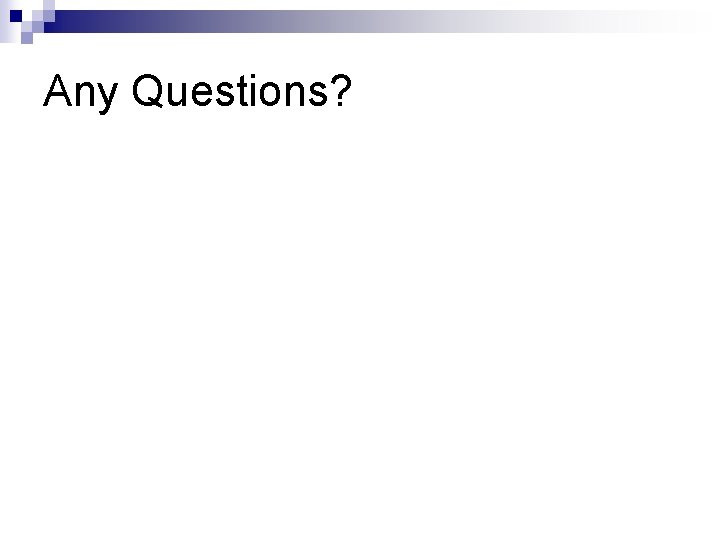
Any Questions?
Contoh error handling
Exception handling in vb net
Exception handling in java
Exception handling pada java
Php exception
Exception handling pada java
Fiq irq
Event handling in ada
Php throw exception
Exception handling in vb.net
Week by week plans for documenting children's development
Fatcomparative and superlative
Predefined and non predefined exceptions are raised
Bad in comparative adjective
Comparatives safe
Spl exceptions
Robustness in java
Stark law exceptions
Present progressive exceptions
Coccus
Koch's postulates exceptions
Koch's postulates exceptions
Stark law exceptions
What is first ionization energy
Octet exceptions
Koch's postulates exceptions
Cave of unreported exceptions
Hors xnxn
Different types of exceptions in c++
Java exceptions list
Don't laugh at the poor question tag
Present continuous exceptions
Invoice exceptions
Octet rule
Pronunciation workshop
Exceptions imparfait
Imparfait exceptions
Phenocopy
Cvc rule