InputOuput and Exception Handling Exceptions An exception is
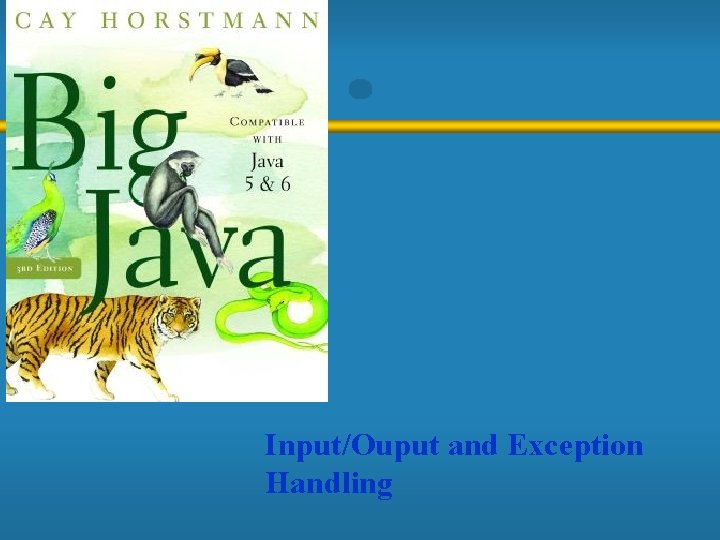
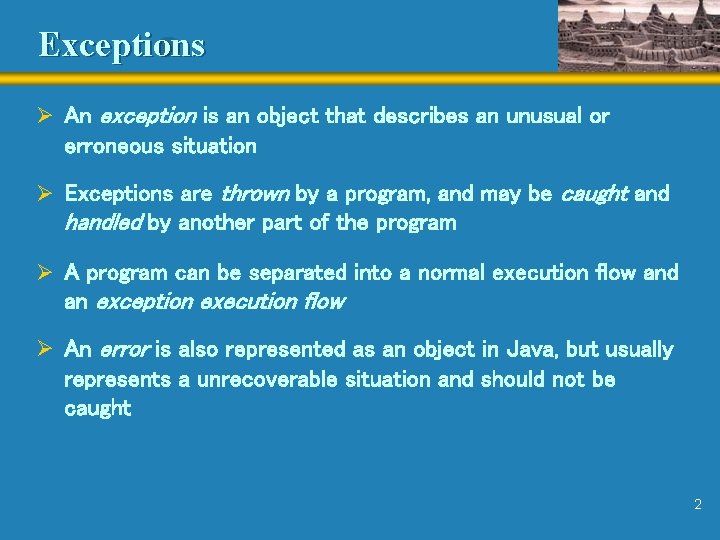
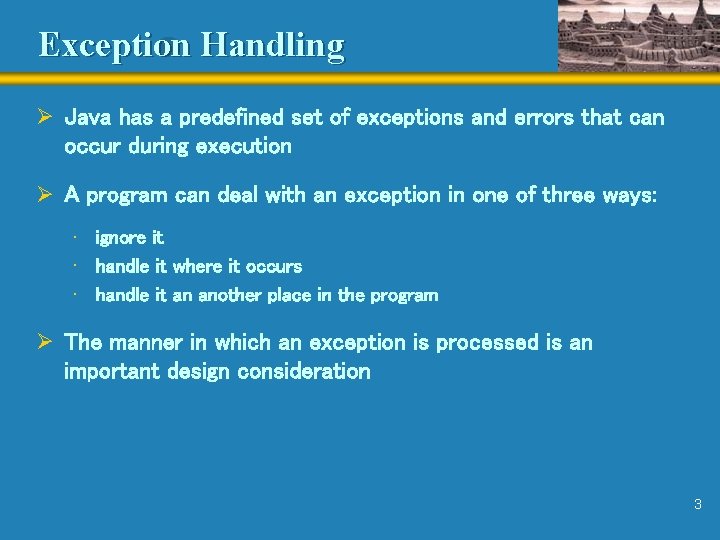
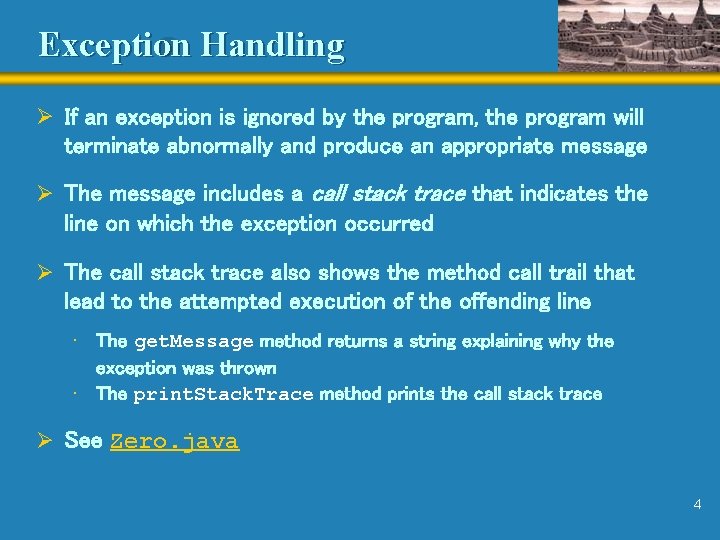
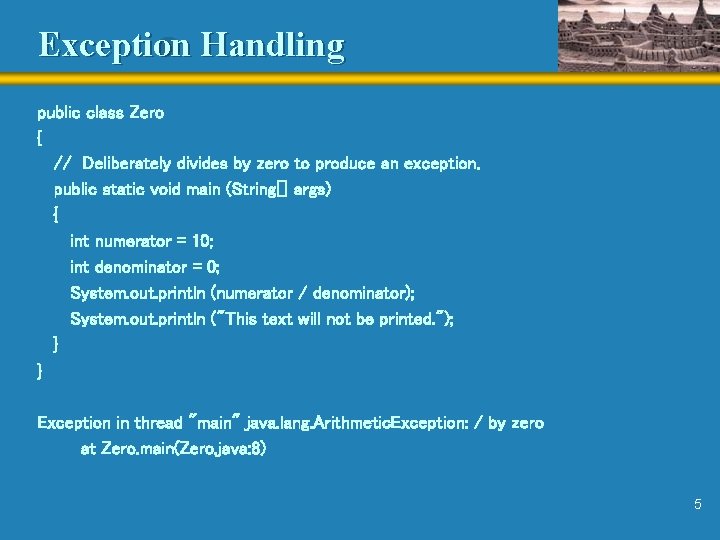
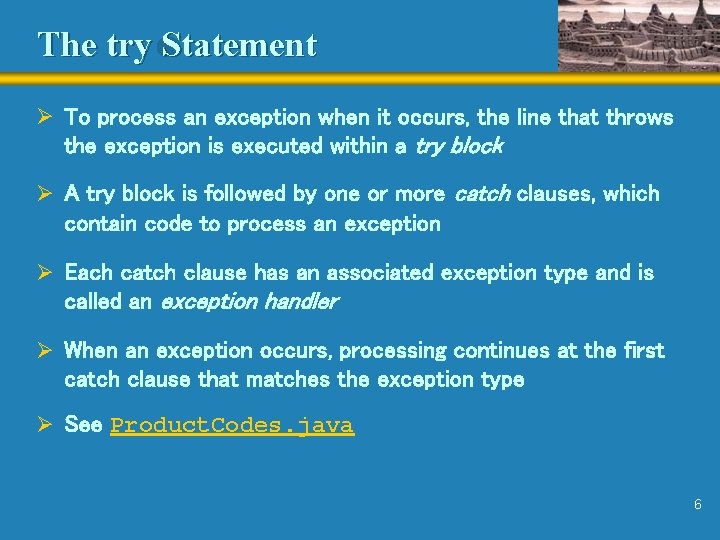
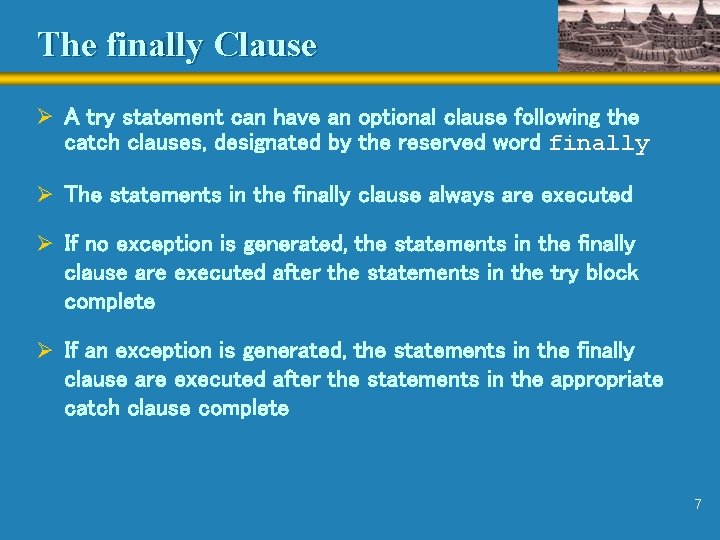
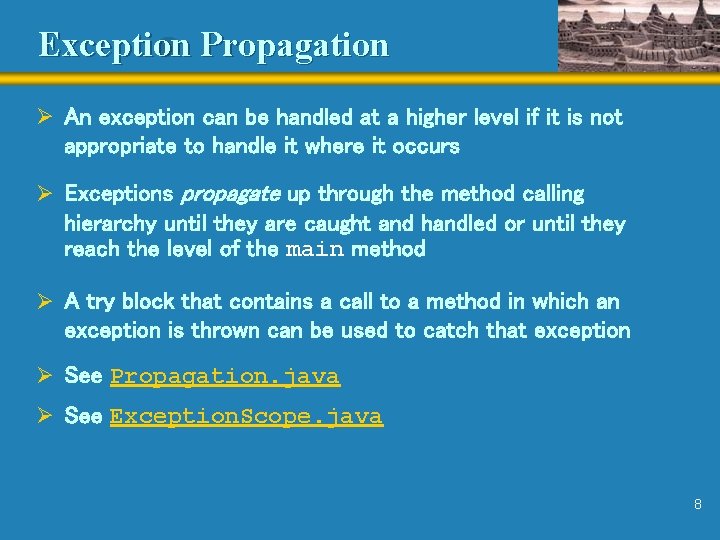
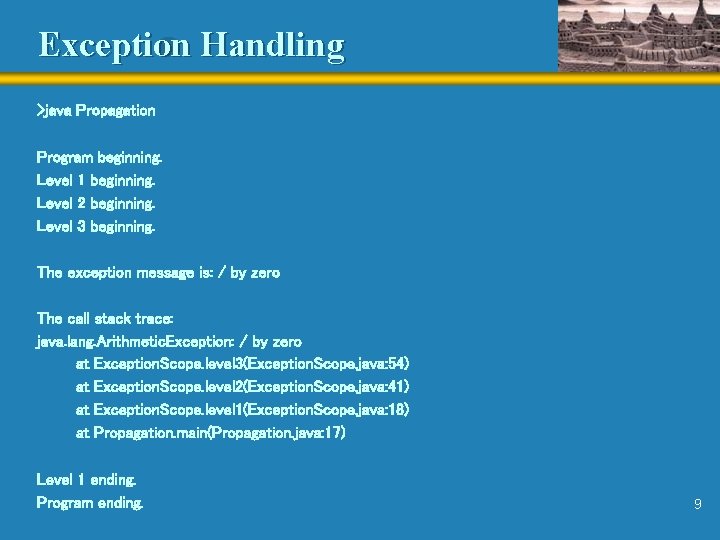
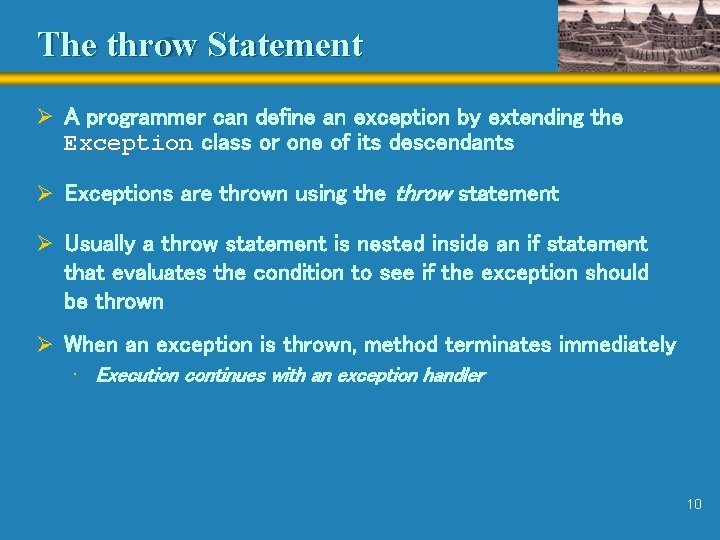
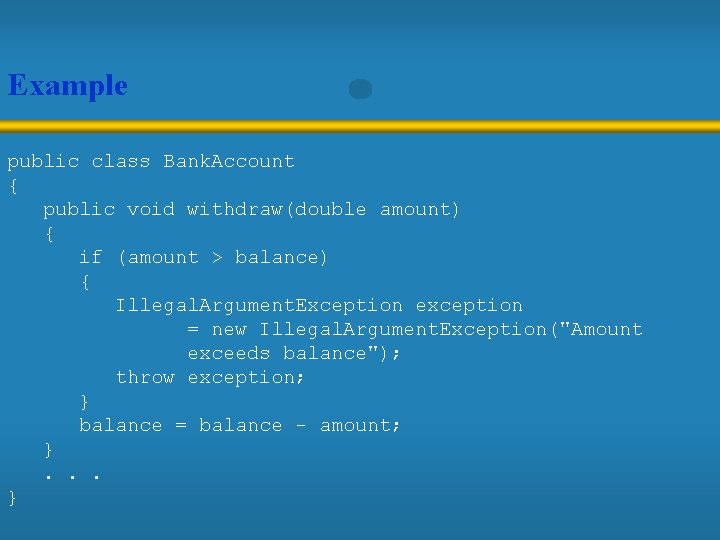
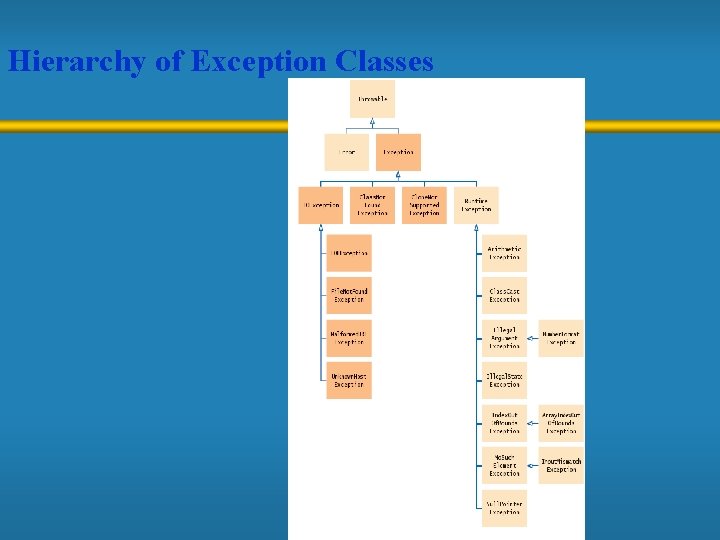
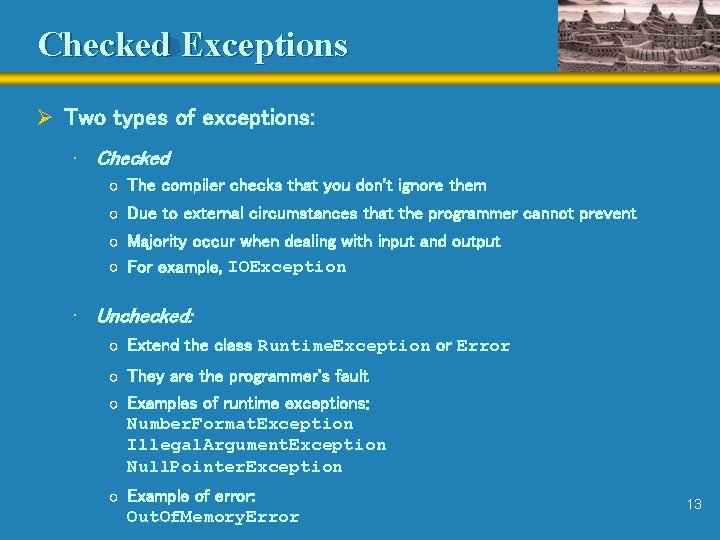
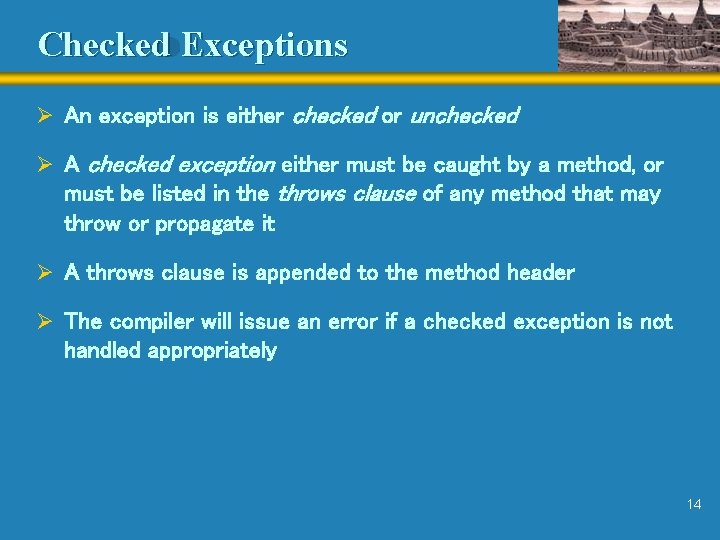
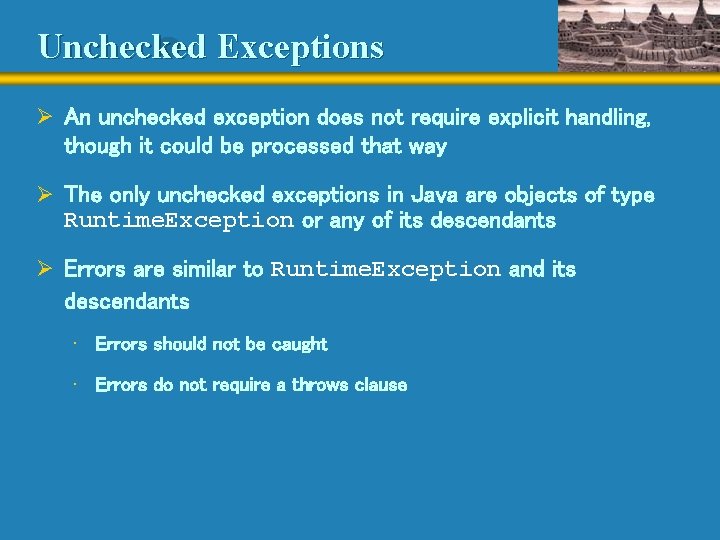
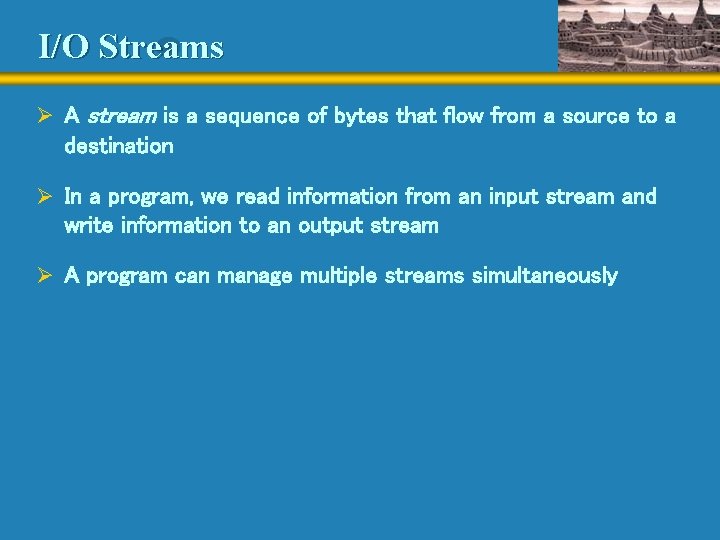
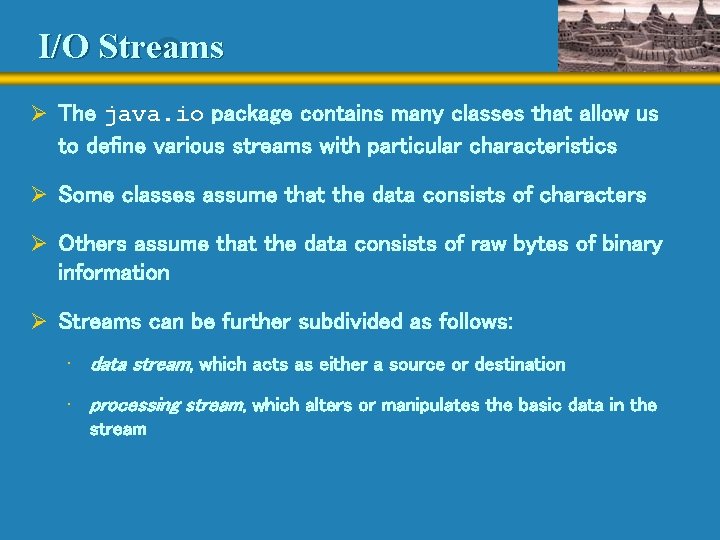
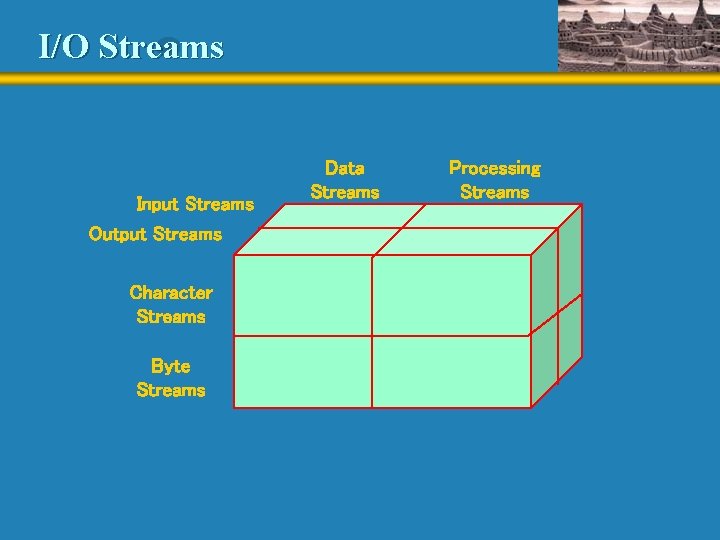
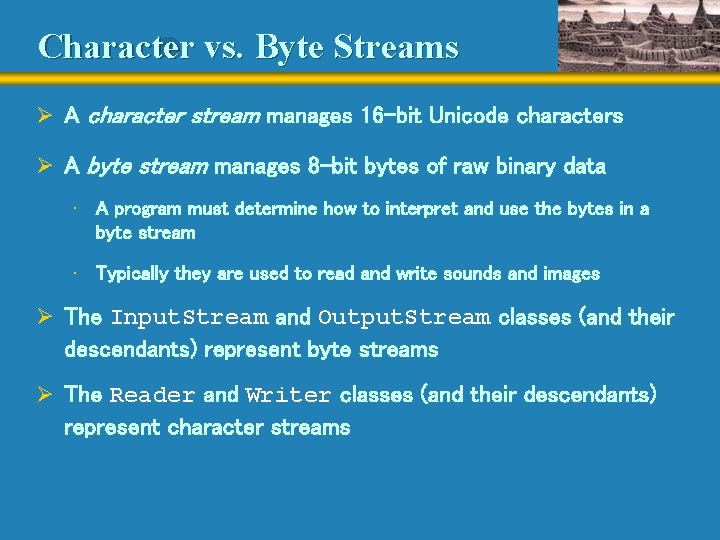
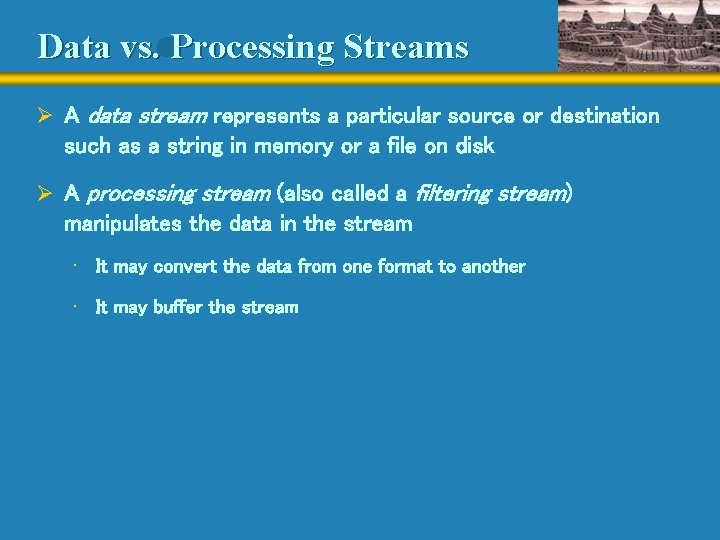
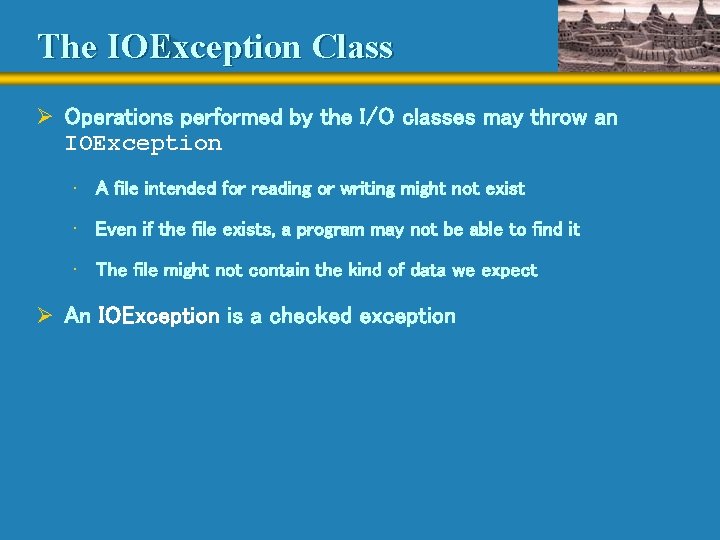
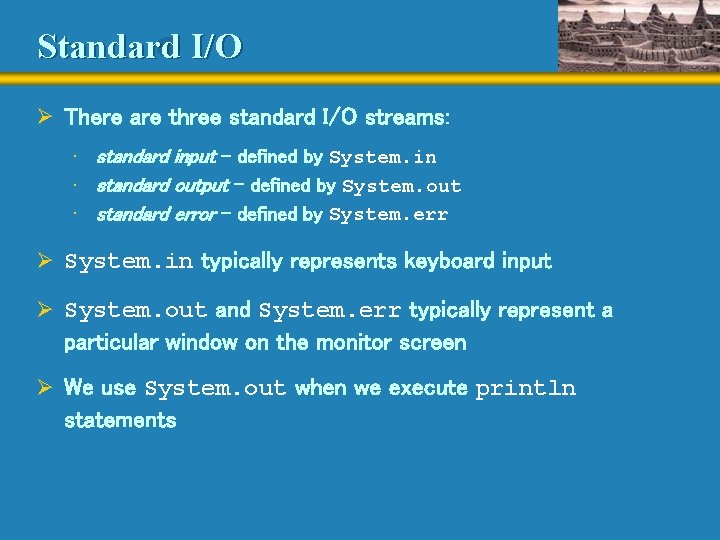
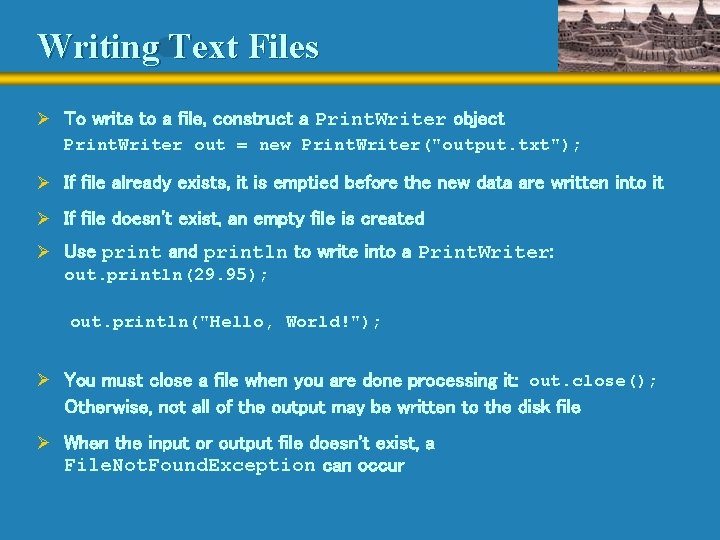
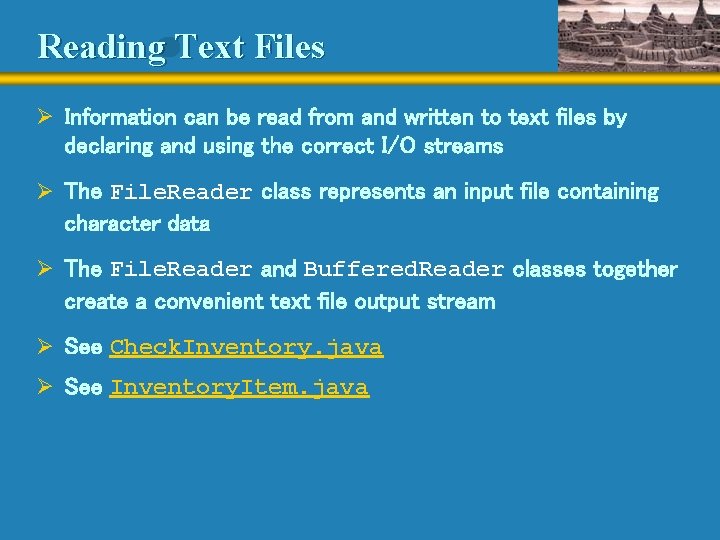
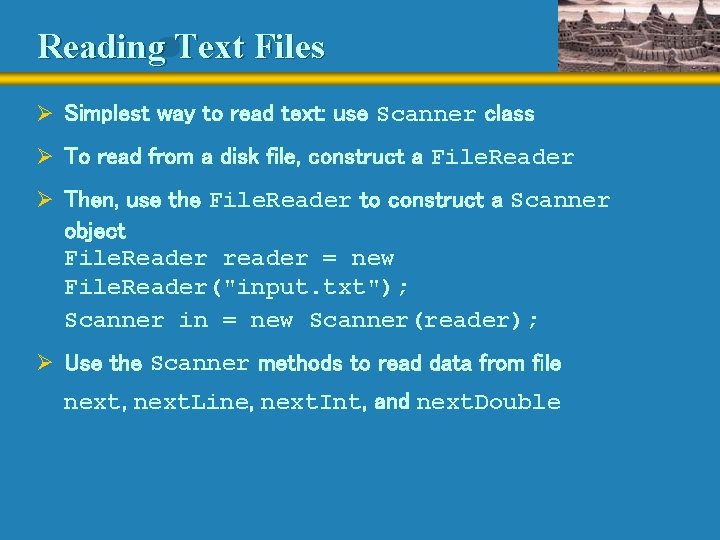
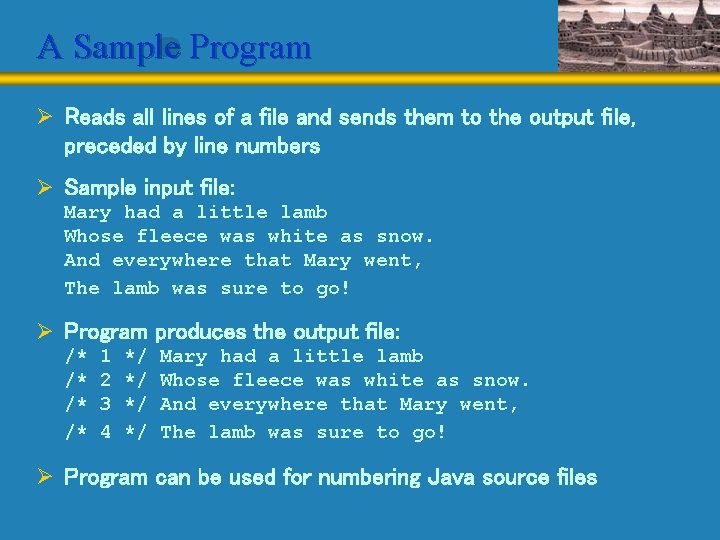
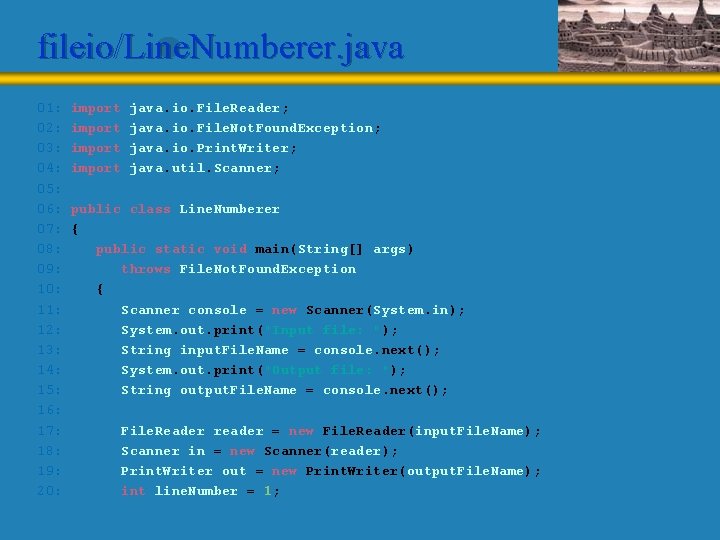
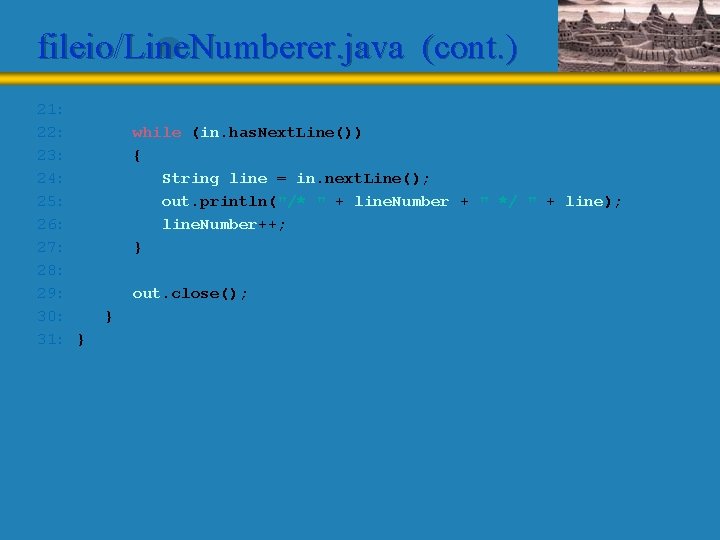
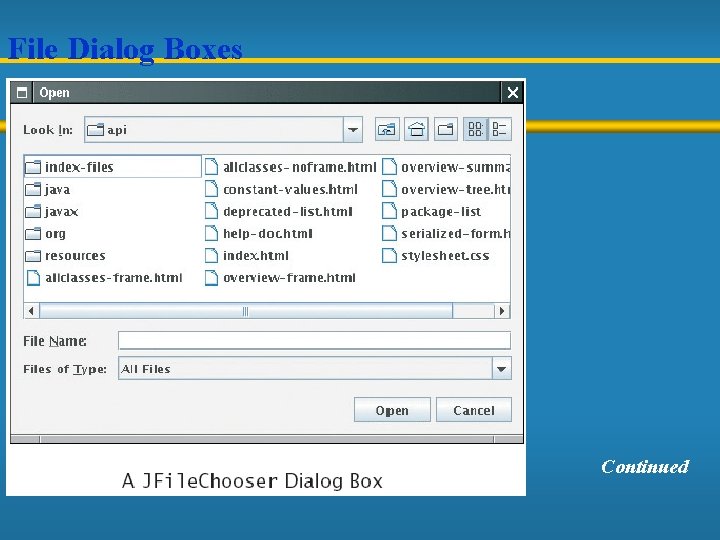
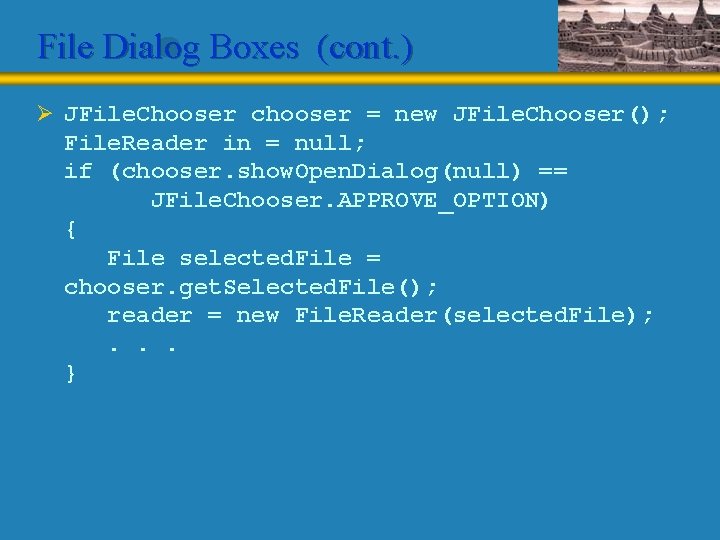
- Slides: 30
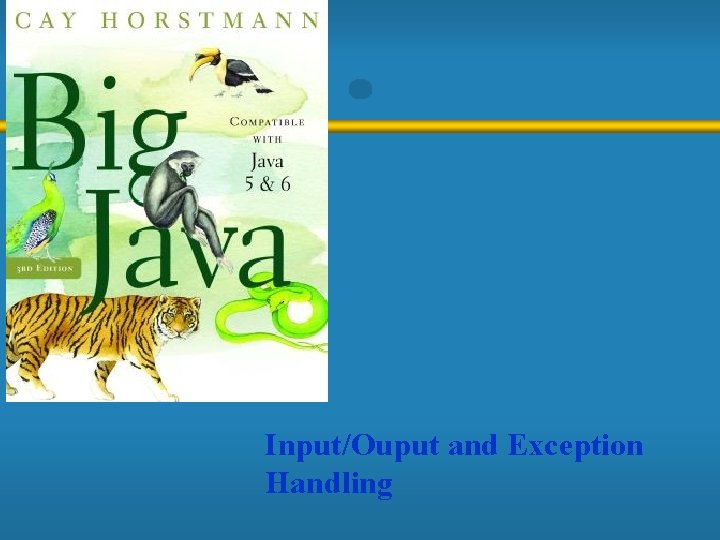
Input/Ouput and Exception Handling
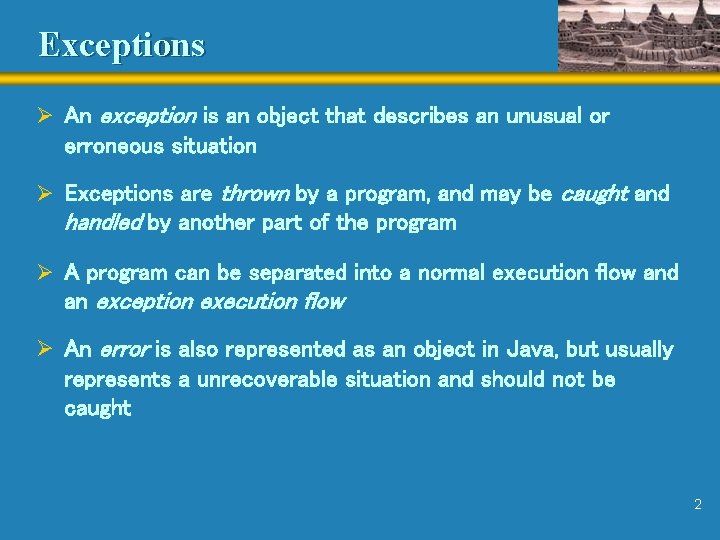
Exceptions Ø An exception is an object that describes an unusual or erroneous situation Ø Exceptions are thrown by a program, and may be caught and handled by another part of the program Ø A program can be separated into a normal execution flow and an exception execution flow Ø An error is also represented as an object in Java, but usually represents a unrecoverable situation and should not be caught 2
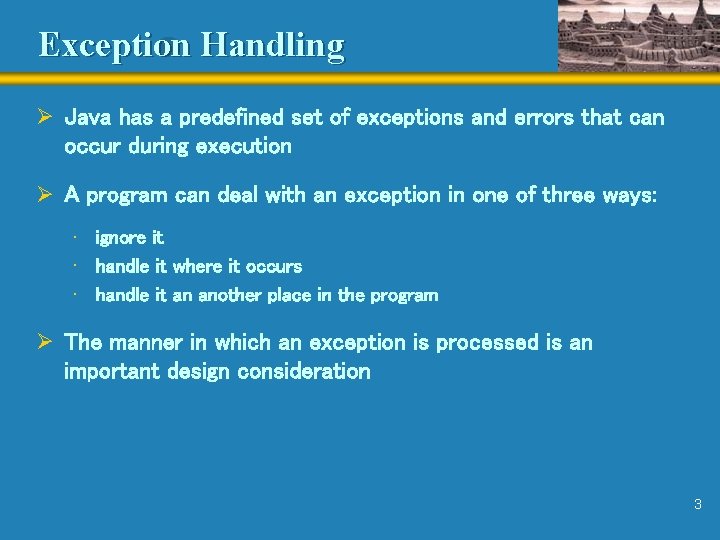
Exception Handling Ø Java has a predefined set of exceptions and errors that can occur during execution Ø A program can deal with an exception in one of three ways: • ignore it • handle it where it occurs • handle it an another place in the program Ø The manner in which an exception is processed is an important design consideration 3
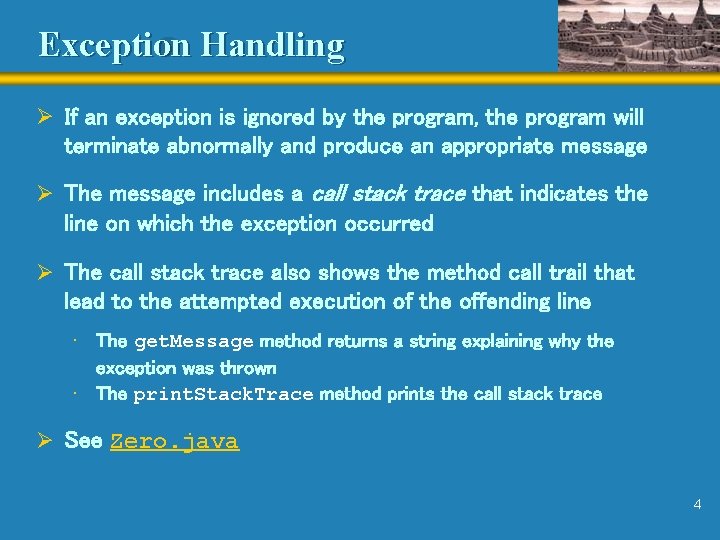
Exception Handling Ø If an exception is ignored by the program, the program will terminate abnormally and produce an appropriate message Ø The message includes a call stack trace that indicates the line on which the exception occurred Ø The call stack trace also shows the method call trail that lead to the attempted execution of the offending line • The get. Message method returns a string explaining why the exception was thrown • The print. Stack. Trace method prints the call stack trace Ø See Zero. java 4
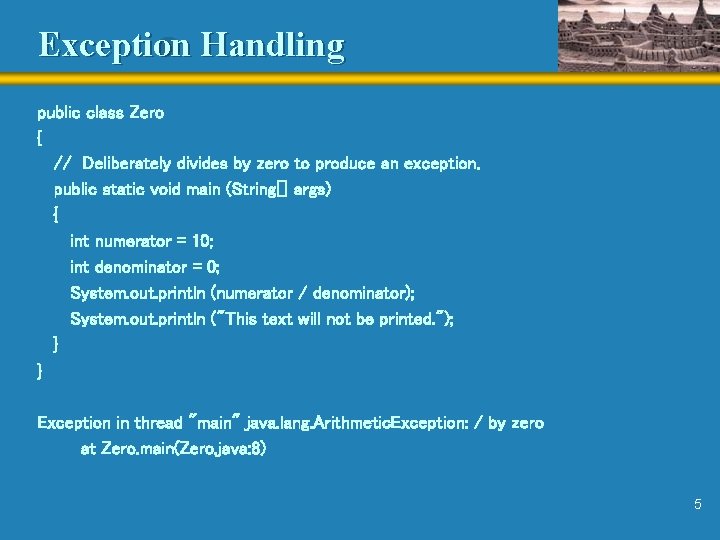
Exception Handling public class Zero { // Deliberately divides by zero to produce an exception. public static void main (String[] args) { int numerator = 10; int denominator = 0; System. out. println (numerator / denominator); System. out. println ("This text will not be printed. "); } } Exception in thread "main" java. lang. Arithmetic. Exception: / by zero at Zero. main(Zero. java: 8) 5
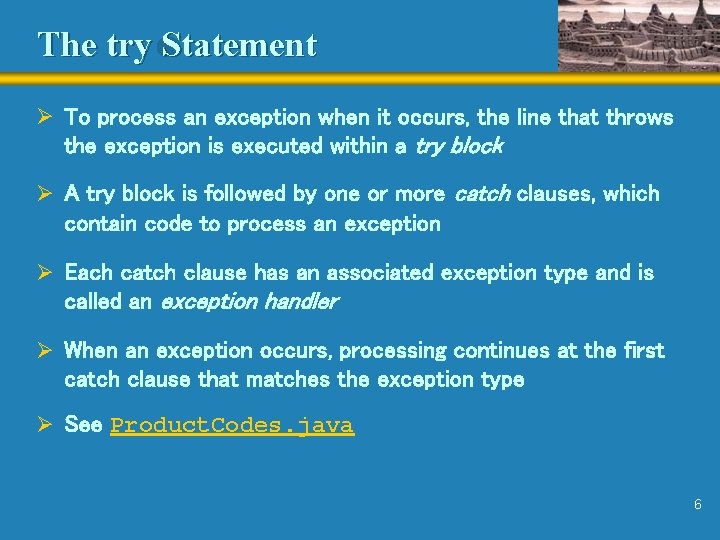
The try Statement Ø To process an exception when it occurs, the line that throws the exception is executed within a try block Ø A try block is followed by one or more catch clauses, which contain code to process an exception Ø Each catch clause has an associated exception type and is called an exception handler Ø When an exception occurs, processing continues at the first catch clause that matches the exception type Ø See Product. Codes. java 6
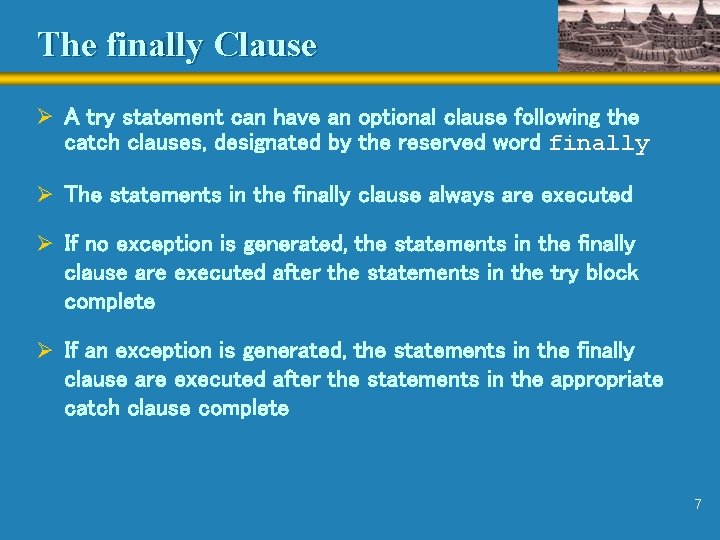
The finally Clause Ø A try statement can have an optional clause following the catch clauses, designated by the reserved word finally Ø The statements in the finally clause always are executed Ø If no exception is generated, the statements in the finally clause are executed after the statements in the try block complete Ø If an exception is generated, the statements in the finally clause are executed after the statements in the appropriate catch clause complete 7
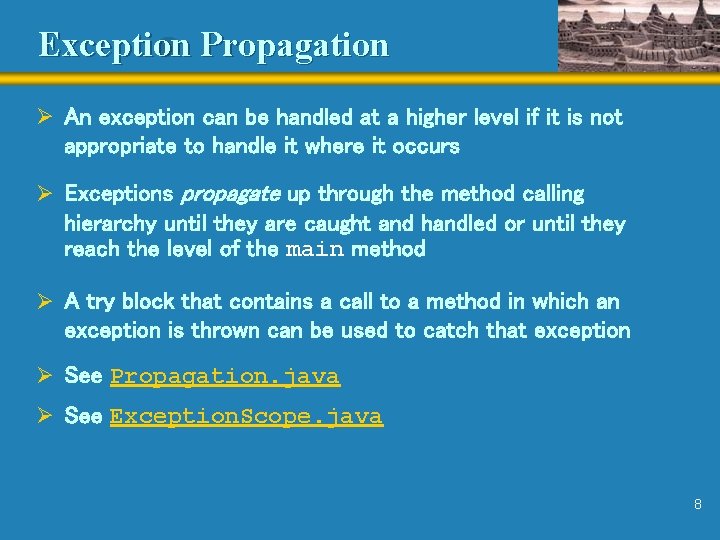
Exception Propagation Ø An exception can be handled at a higher level if it is not appropriate to handle it where it occurs Ø Exceptions propagate up through the method calling hierarchy until they are caught and handled or until they reach the level of the main method Ø A try block that contains a call to a method in which an exception is thrown can be used to catch that exception Ø See Propagation. java Ø See Exception. Scope. java 8
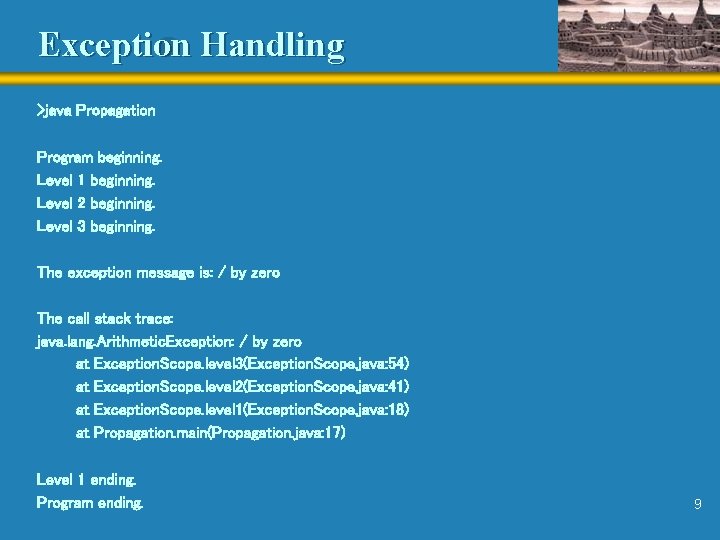
Exception Handling >java Propagation Program beginning. Level 1 beginning. Level 2 beginning. Level 3 beginning. The exception message is: / by zero The call stack trace: java. lang. Arithmetic. Exception: / by zero at Exception. Scope. level 3(Exception. Scope. java: 54) at Exception. Scope. level 2(Exception. Scope. java: 41) at Exception. Scope. level 1(Exception. Scope. java: 18) at Propagation. main(Propagation. java: 17) Level 1 ending. Program ending. 9
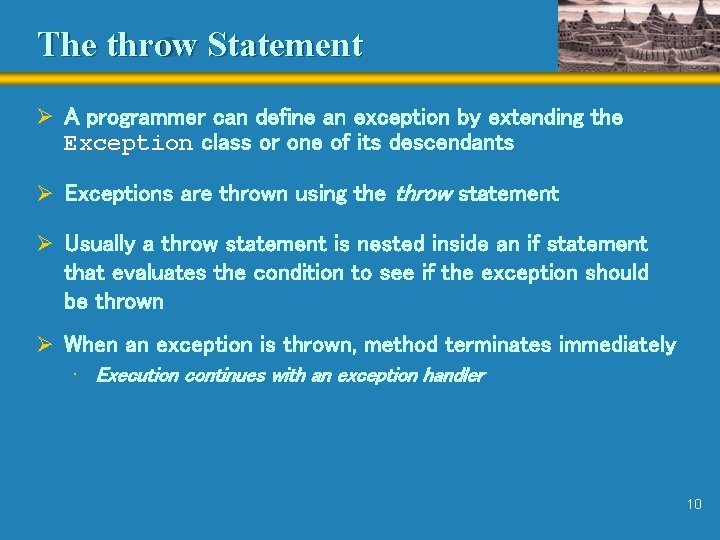
The throw Statement Ø A programmer can define an exception by extending the Exception class or one of its descendants Ø Exceptions are thrown using the throw statement Ø Usually a throw statement is nested inside an if statement that evaluates the condition to see if the exception should be thrown Ø When an exception is thrown, method terminates immediately • Execution continues with an exception handler 10
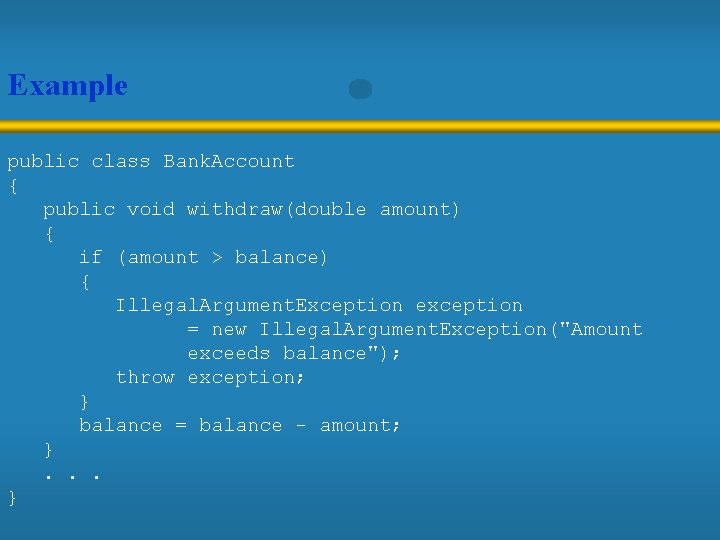
Example public class Bank. Account { public void withdraw(double amount) { if (amount > balance) { Illegal. Argument. Exception exception = new Illegal. Argument. Exception("Amount exceeds balance"); throw exception; } balance = balance - amount; }. . . }
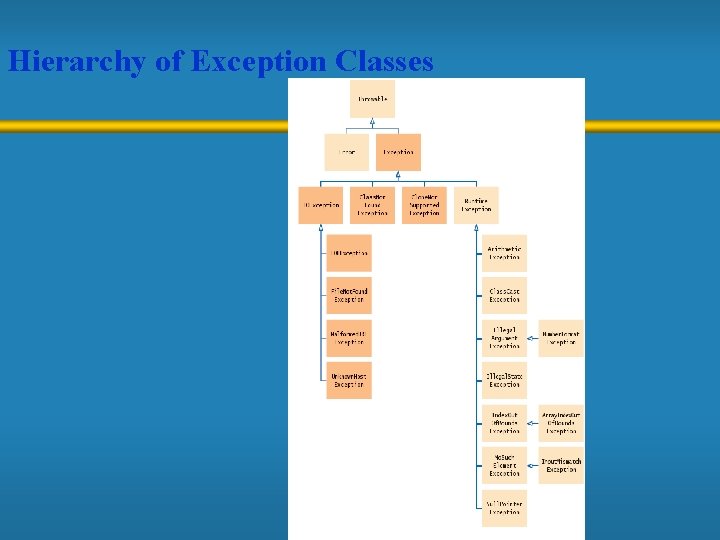
Hierarchy of Exception Classes
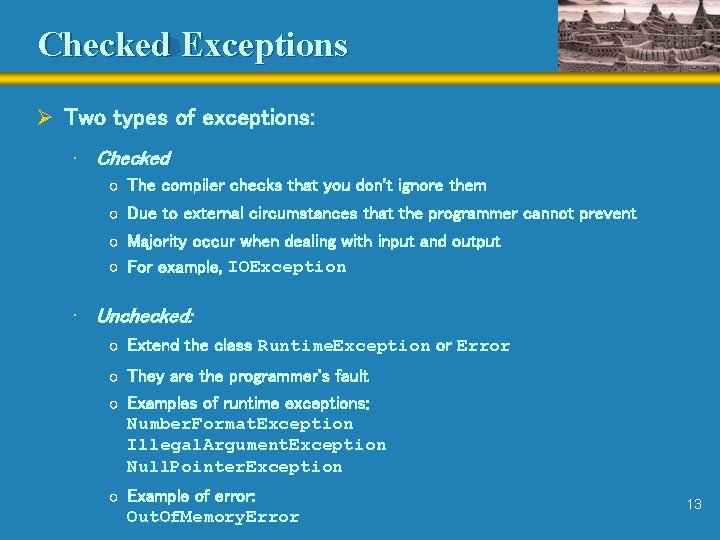
Checked Exceptions Ø Two types of exceptions: • Checked o The compiler checks that you don't ignore them o Due to external circumstances that the programmer cannot prevent o Majority occur when dealing with input and output o For example, IOException • Unchecked: o Extend the class Runtime. Exception or Error o They are the programmer's fault o Examples of runtime exceptions: Number. Format. Exception Illegal. Argument. Exception Null. Pointer. Exception o Example of error: Out. Of. Memory. Error 13
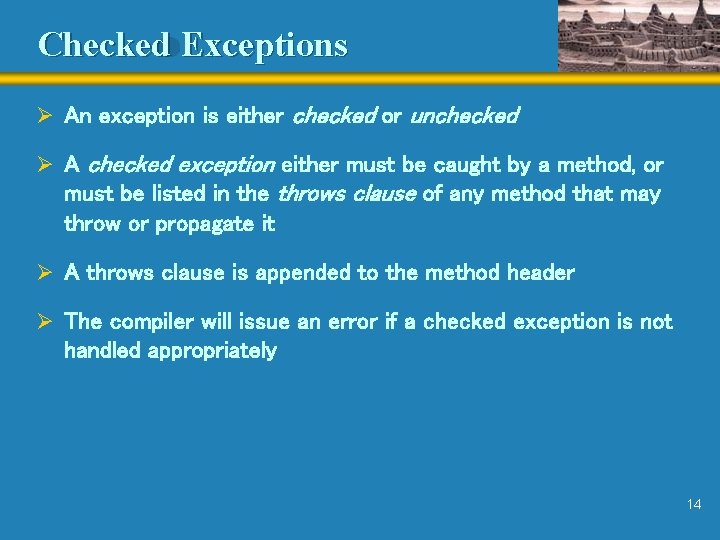
Checked Exceptions Ø An exception is either checked or unchecked Ø A checked exception either must be caught by a method, or must be listed in the throws clause of any method that may throw or propagate it Ø A throws clause is appended to the method header Ø The compiler will issue an error if a checked exception is not handled appropriately 14
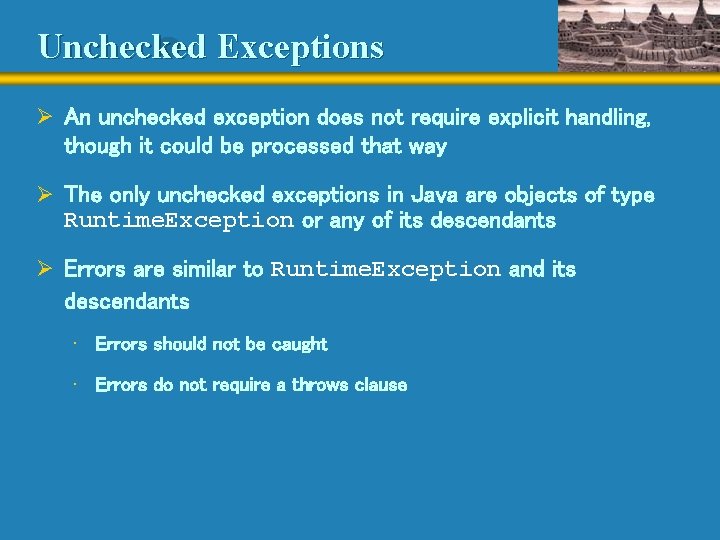
Unchecked Exceptions Ø An unchecked exception does not require explicit handling, though it could be processed that way Ø The only unchecked exceptions in Java are objects of type Runtime. Exception or any of its descendants Ø Errors are similar to Runtime. Exception and its descendants • Errors should not be caught • Errors do not require a throws clause
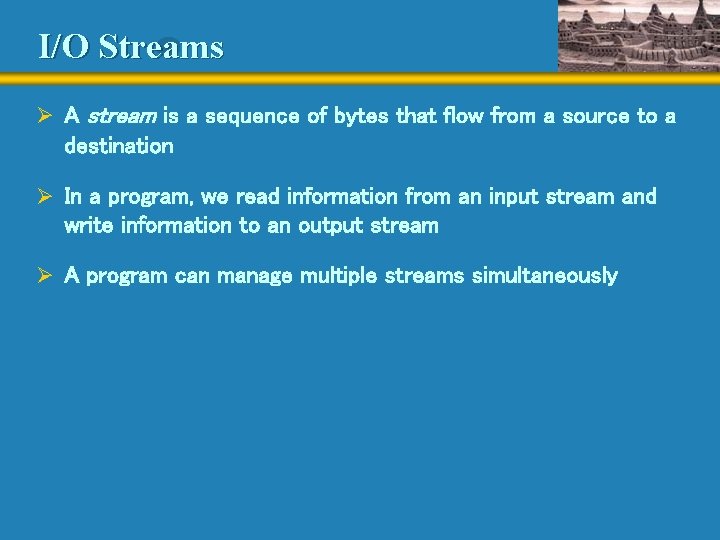
I/O Streams Ø A stream is a sequence of bytes that flow from a source to a destination Ø In a program, we read information from an input stream and write information to an output stream Ø A program can manage multiple streams simultaneously
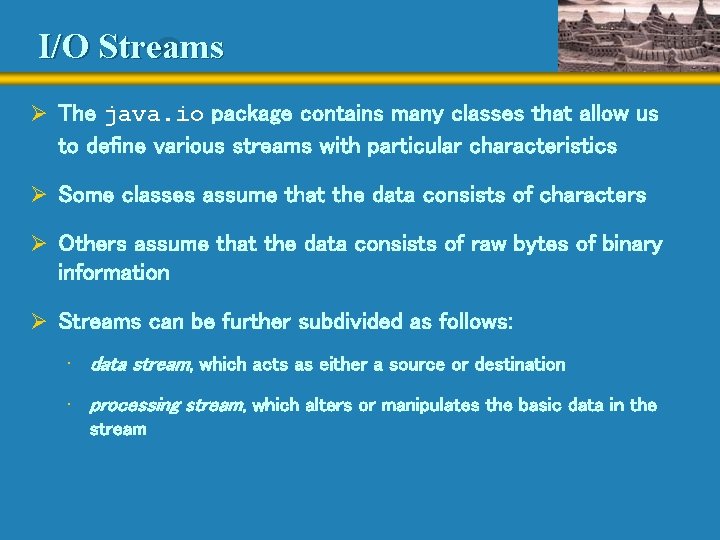
I/O Streams Ø The java. io package contains many classes that allow us to define various streams with particular characteristics Ø Some classes assume that the data consists of characters Ø Others assume that the data consists of raw bytes of binary information Ø Streams can be further subdivided as follows: • data stream, which acts as either a source or destination • processing stream, which alters or manipulates the basic data in the stream
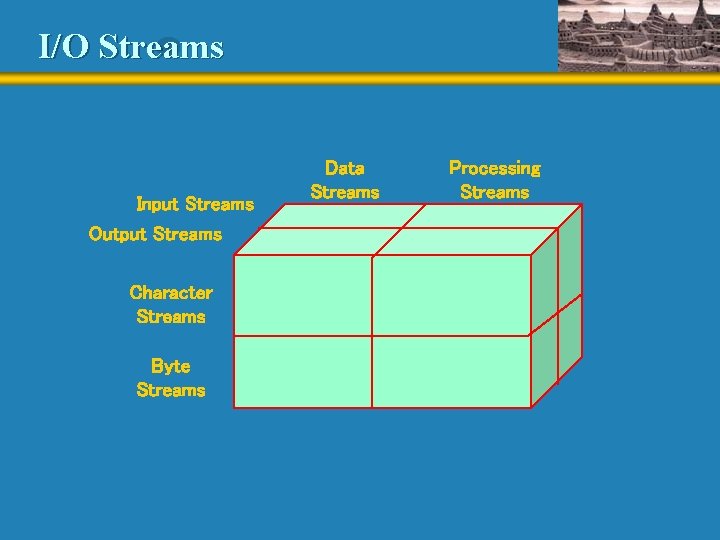
I/O Streams Input Streams Output Streams Character Streams Byte Streams Data Streams Processing Streams
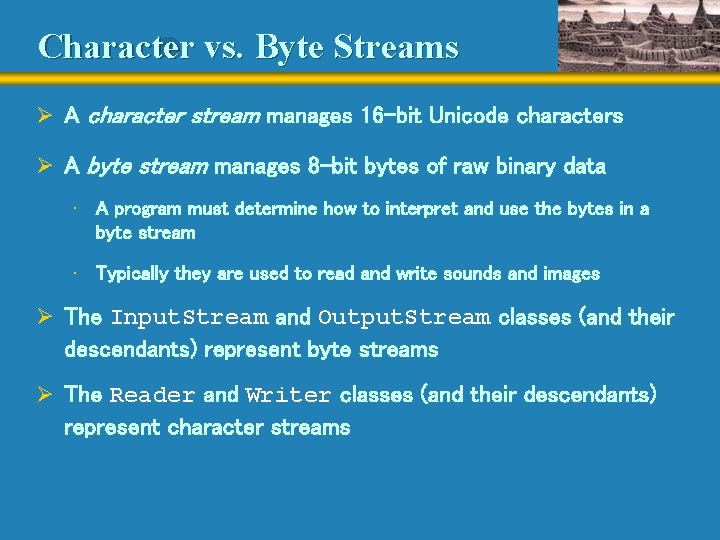
Character vs. Byte Streams Ø A character stream manages 16 -bit Unicode characters Ø A byte stream manages 8 -bit bytes of raw binary data • A program must determine how to interpret and use the bytes in a byte stream • Typically they are used to read and write sounds and images Ø The Input. Stream and Output. Stream classes (and their descendants) represent byte streams Ø The Reader and Writer classes (and their descendants) represent character streams
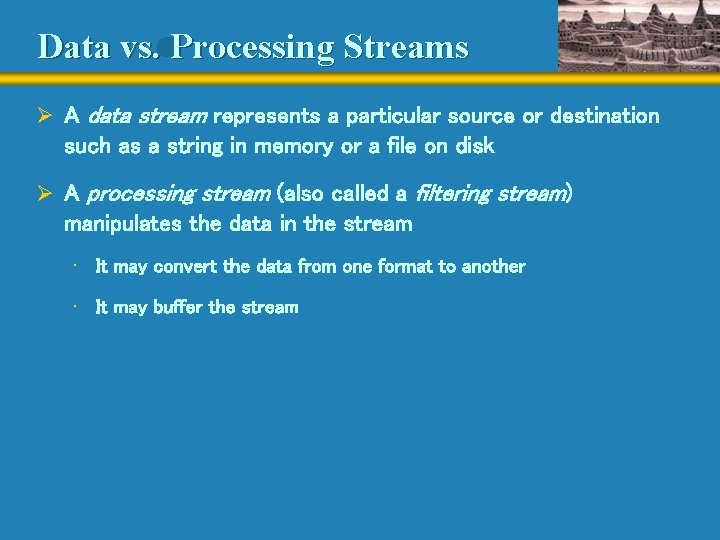
Data vs. Processing Streams Ø A data stream represents a particular source or destination such as a string in memory or a file on disk Ø A processing stream (also called a filtering stream) manipulates the data in the stream • It may convert the data from one format to another • It may buffer the stream
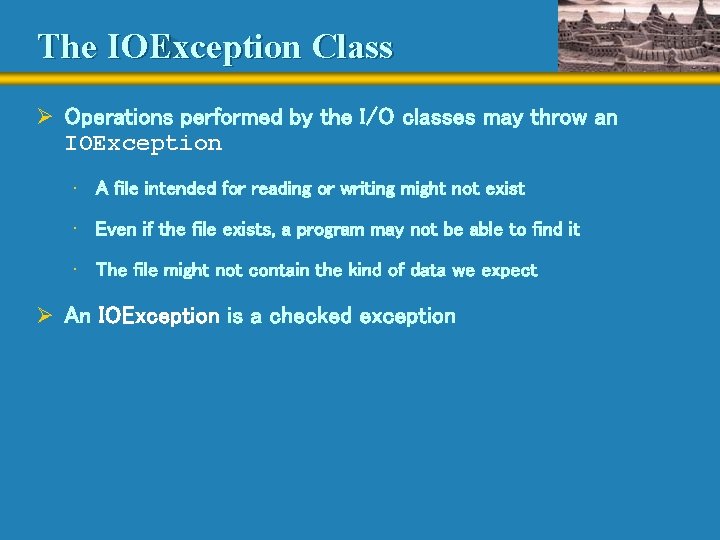
The IOException Class Ø Operations performed by the I/O classes may throw an IOException • A file intended for reading or writing might not exist • Even if the file exists, a program may not be able to find it • The file might not contain the kind of data we expect Ø An IOException is a checked exception
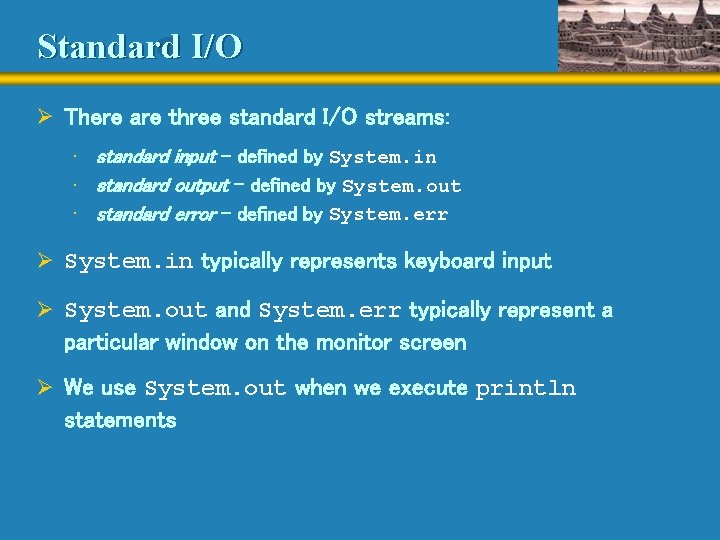
Standard I/O Ø There are three standard I/O streams: • standard input – defined by System. in • standard output – defined by System. out • standard error – defined by System. err Ø System. in typically represents keyboard input Ø System. out and System. err typically represent a particular window on the monitor screen Ø We use System. out when we execute println statements
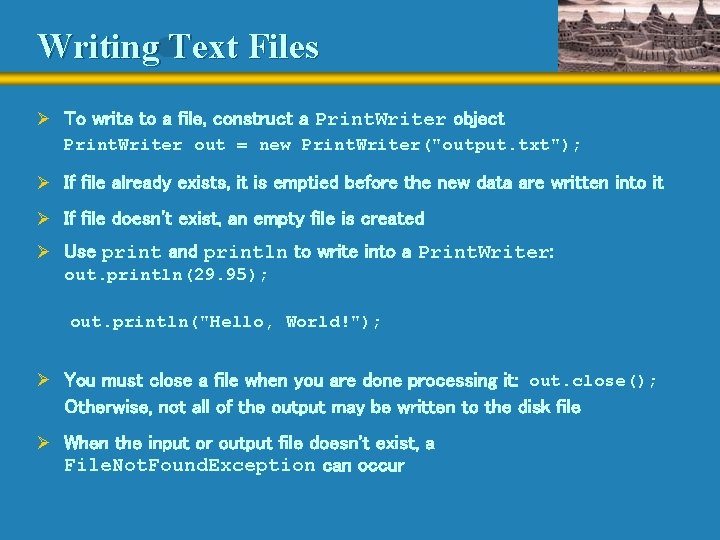
Writing Text Files Ø To write to a file, construct a Print. Writer object Print. Writer out = new Print. Writer("output. txt"); Ø If file already exists, it is emptied before the new data are written into it Ø If file doesn't exist, an empty file is created Ø Use print and println to write into a Print. Writer: out. println(29. 95); out. println("Hello, World!"); Ø You must close a file when you are done processing it: out. close(); Otherwise, not all of the output may be written to the disk file Ø When the input or output file doesn't exist, a File. Not. Found. Exception can occur
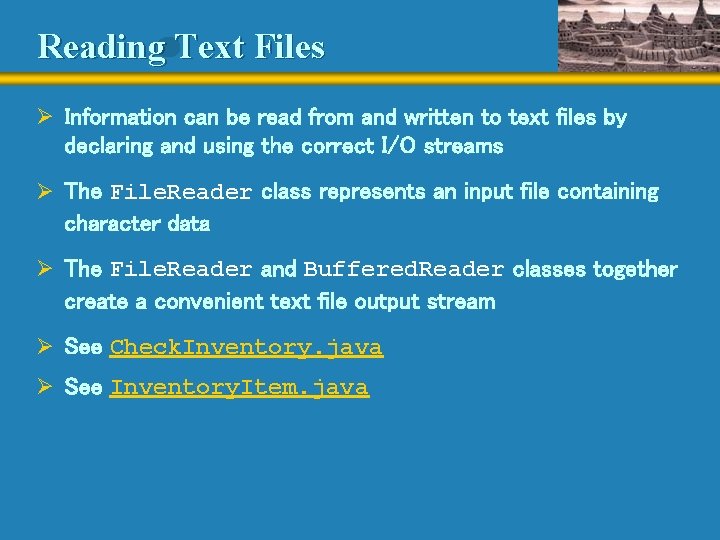
Reading Text Files Ø Information can be read from and written to text files by declaring and using the correct I/O streams Ø The File. Reader class represents an input file containing character data Ø The File. Reader and Buffered. Reader classes together create a convenient text file output stream Ø See Check. Inventory. java Ø See Inventory. Item. java
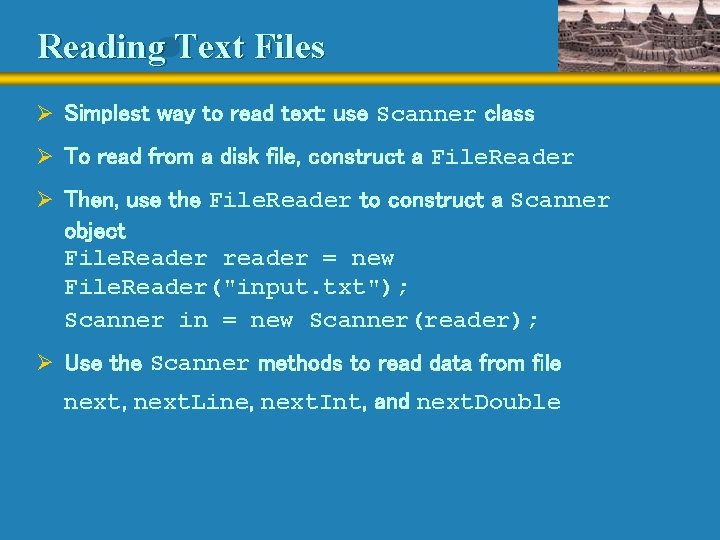
Reading Text Files Ø Simplest way to read text: use Scanner class Ø To read from a disk file, construct a File. Reader Ø Then, use the File. Reader to construct a Scanner object File. Reader reader = new File. Reader("input. txt"); Scanner in = new Scanner(reader); Ø Use the Scanner methods to read data from file next, next. Line, next. Int, and next. Double
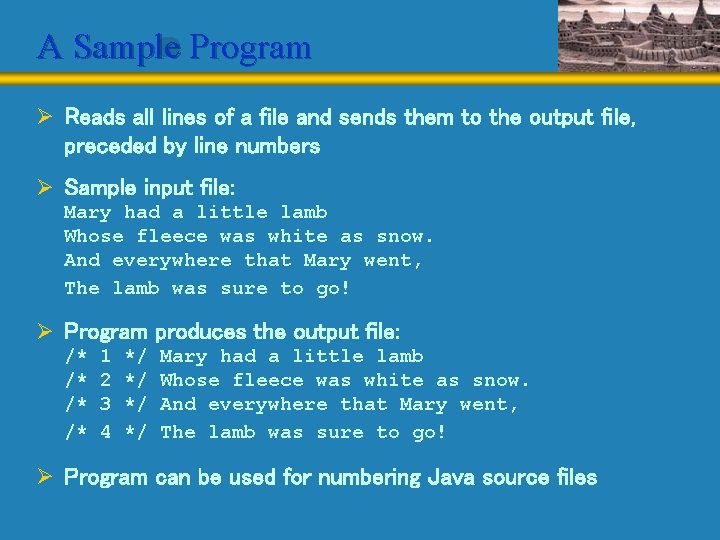
A Sample Program Ø Reads all lines of a file and sends them to the output file, preceded by line numbers Ø Sample input file: Mary had a little lamb Whose fleece was white as snow. And everywhere that Mary went, The lamb was sure to go! Ø Program produces the output file: /* /* 1 2 3 4 */ */ Mary had a little lamb Whose fleece was white as snow. And everywhere that Mary went, The lamb was sure to go! Ø Program can be used for numbering Java source files
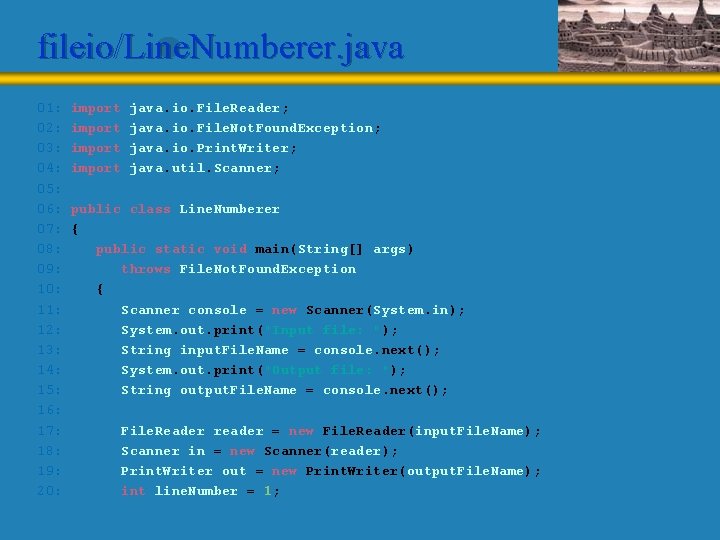
fileio/Line. Numberer. java 01: 02: 03: 04: 05: 06: 07: 08: 09: 10: 11: 12: 13: 14: 15: 16: 17: 18: 19: 20: import java. io. File. Reader; java. io. File. Not. Found. Exception; java. io. Print. Writer; java. util. Scanner; public class Line. Numberer { public static void main(String[] args) throws File. Not. Found. Exception { Scanner console = new Scanner(System. in); System. out. print("Input file: "); String input. File. Name = console. next(); System. out. print("Output file: "); String output. File. Name = console. next(); File. Reader reader = new File. Reader(input. File. Name); Scanner in = new Scanner(reader); Print. Writer out = new Print. Writer(output. File. Name); int line. Number = 1;
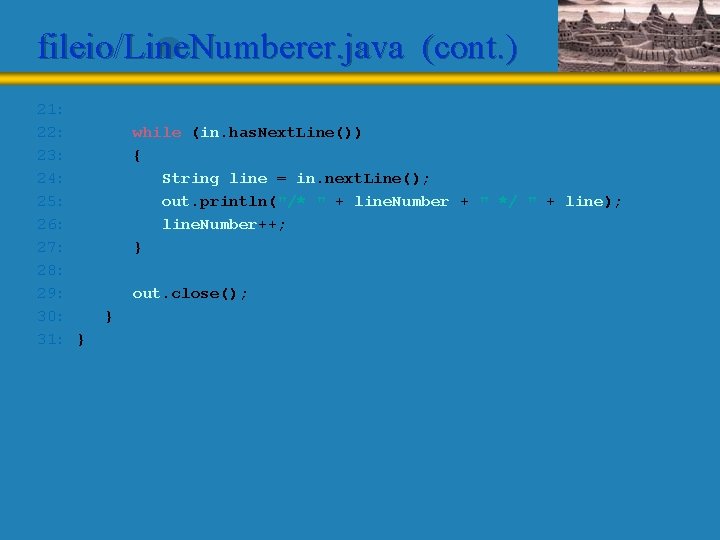
fileio/Line. Numberer. java (cont. ) 21: 22: 23: 24: 25: 26: 27: 28: 29: 30: 31: } while (in. has. Next. Line()) { String line = in. next. Line(); out. println("/* " + line. Number + " */ " + line); line. Number++; } out. close(); }
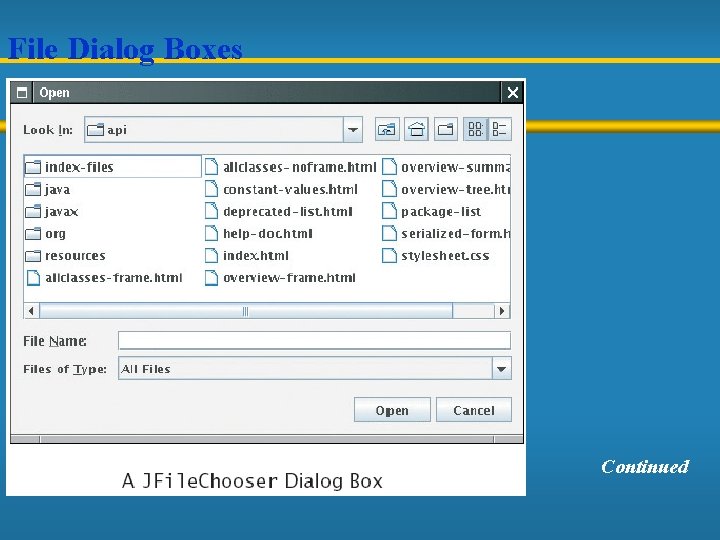
File Dialog Boxes Continued
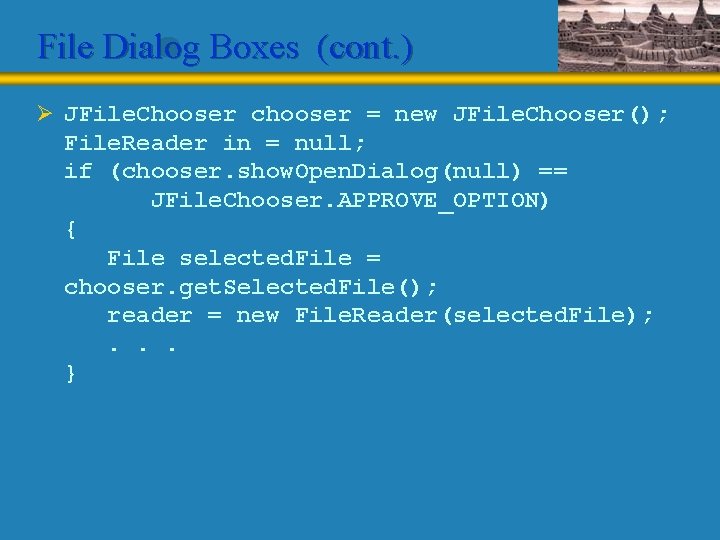
File Dialog Boxes (cont. ) Ø JFile. Chooser chooser = new JFile. Chooser(); File. Reader in = null; if (chooser. show. Open. Dialog(null) == JFile. Chooser. APPROVE_OPTION) { File selected. File = chooser. get. Selected. File(); reader = new File. Reader(selected. File); . . . }