Chapter 7 Arrays 7 1 Creating and Accessing
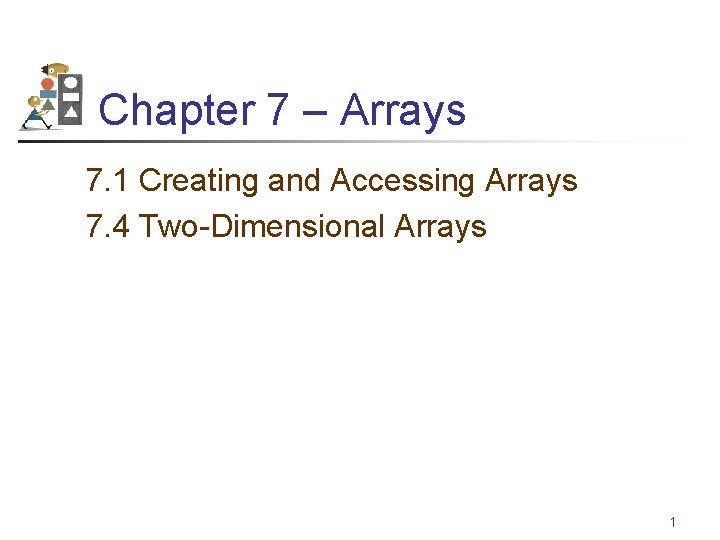
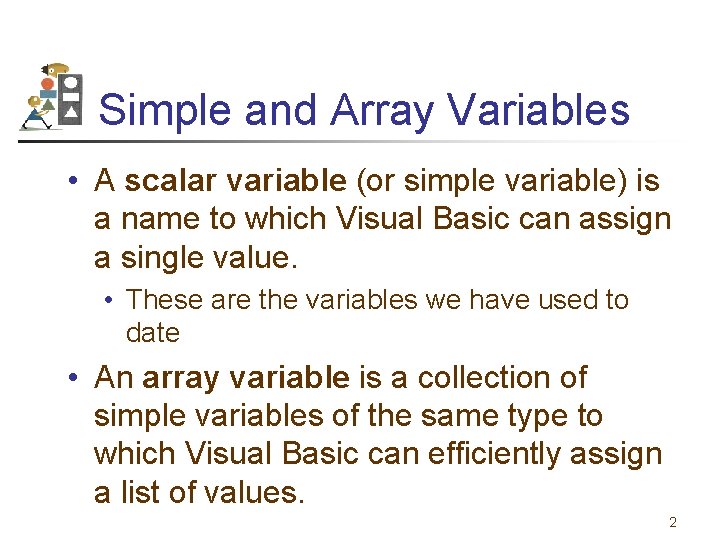
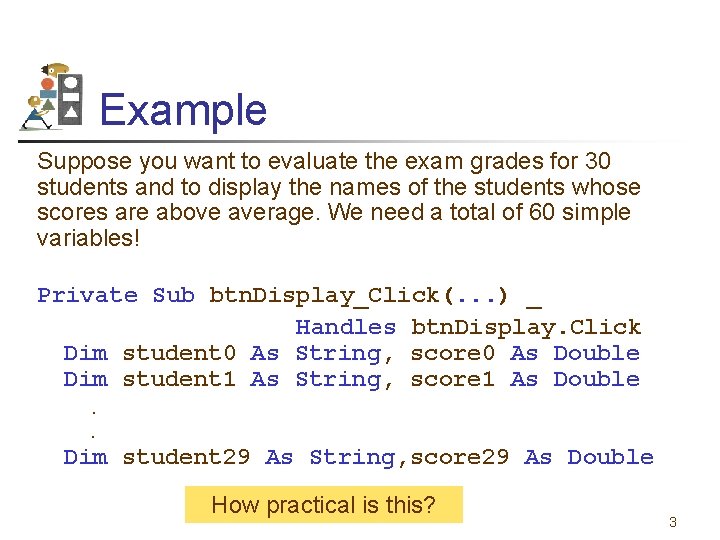
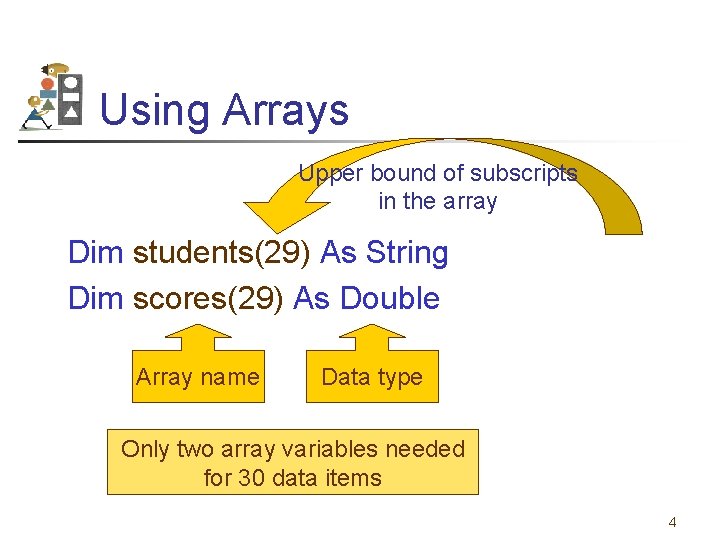
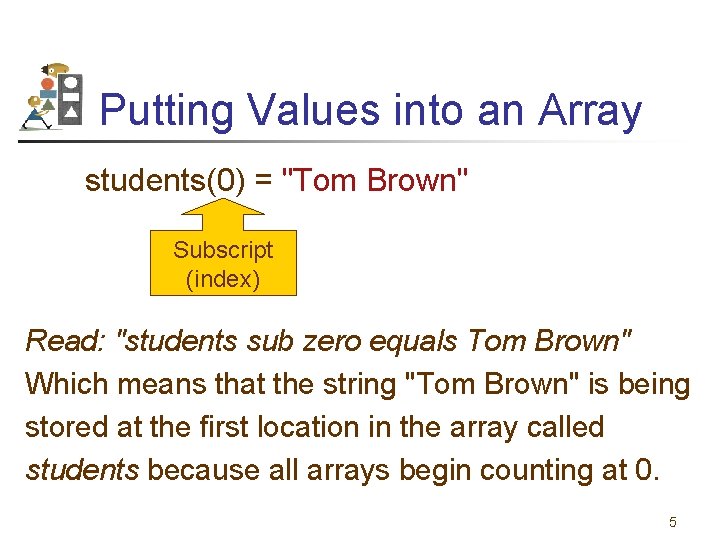
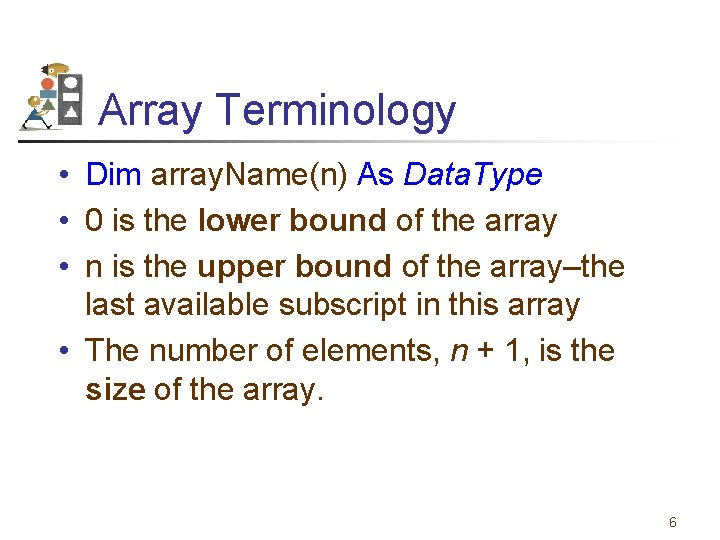
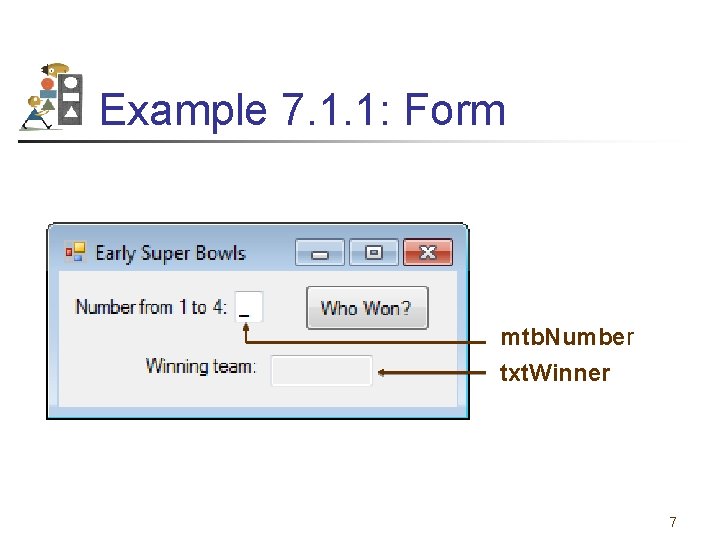
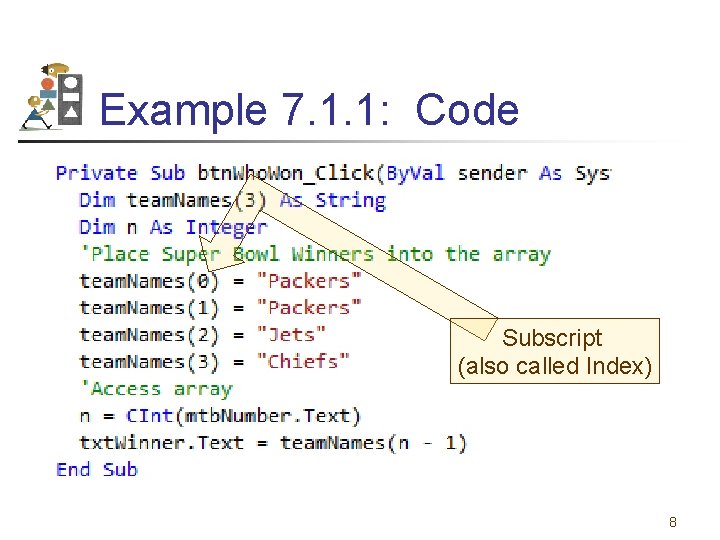
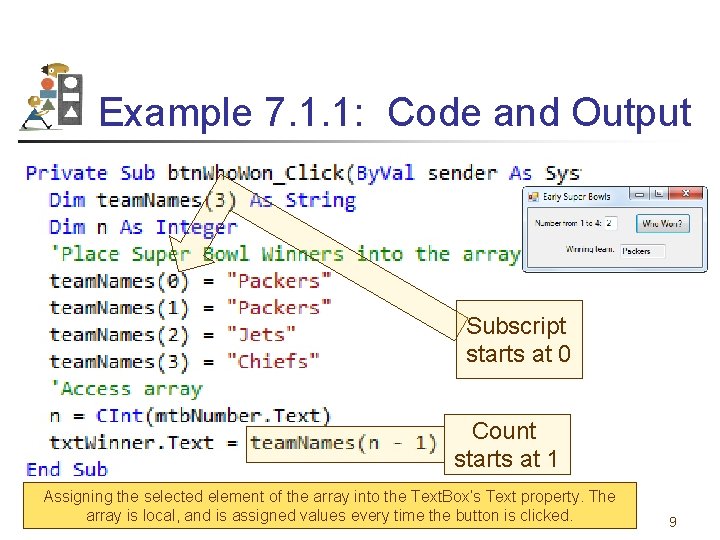
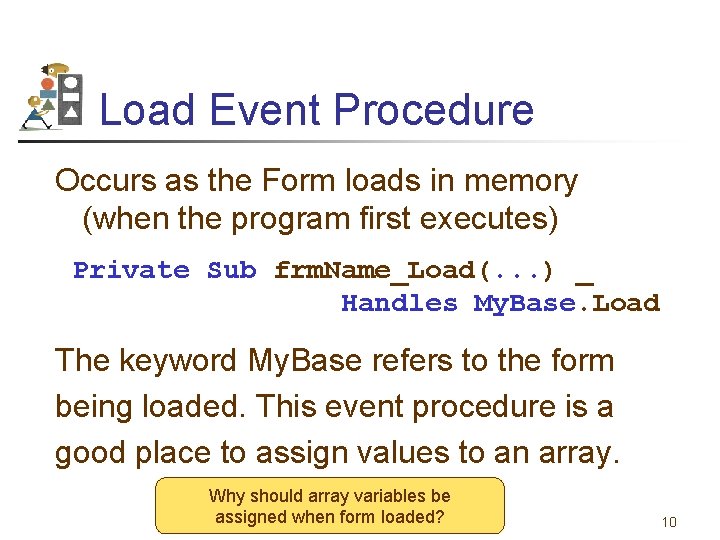
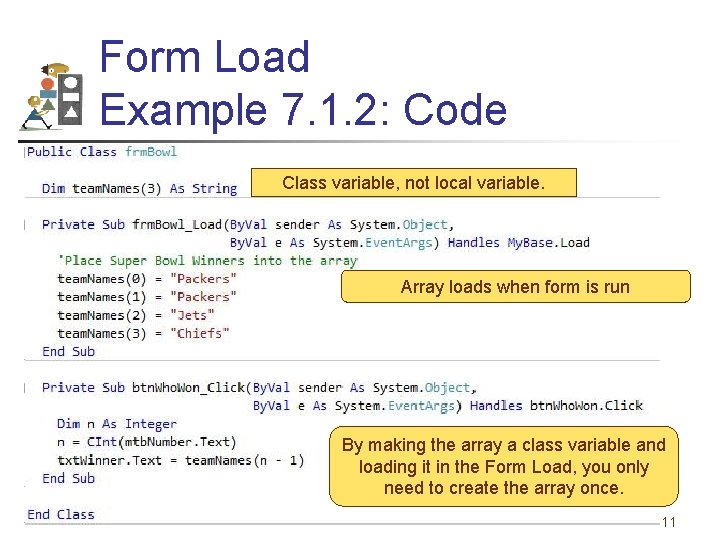
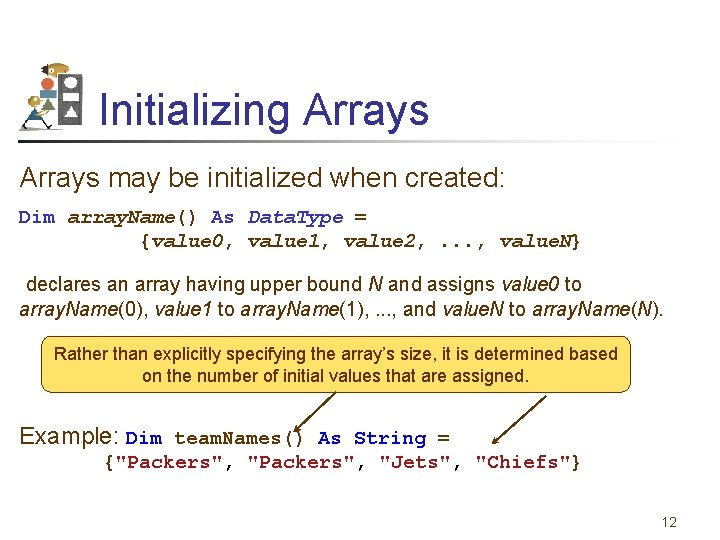
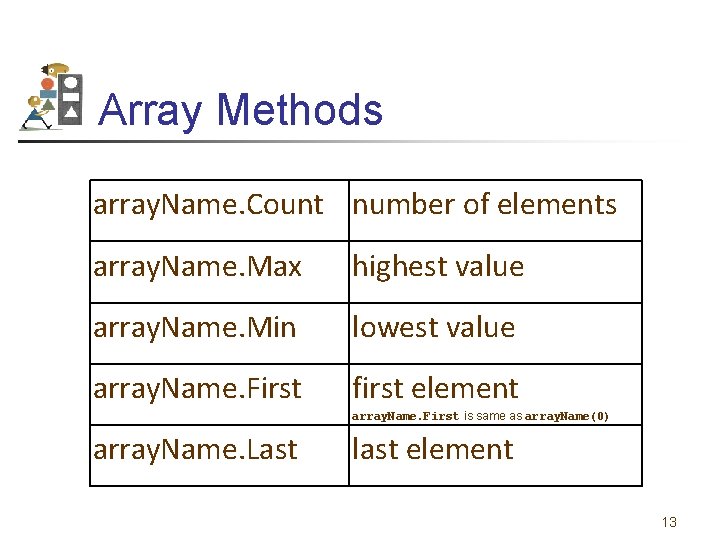
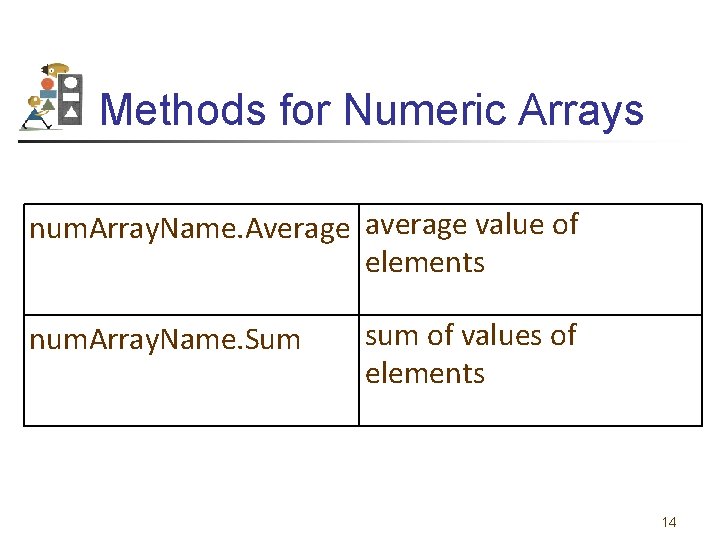
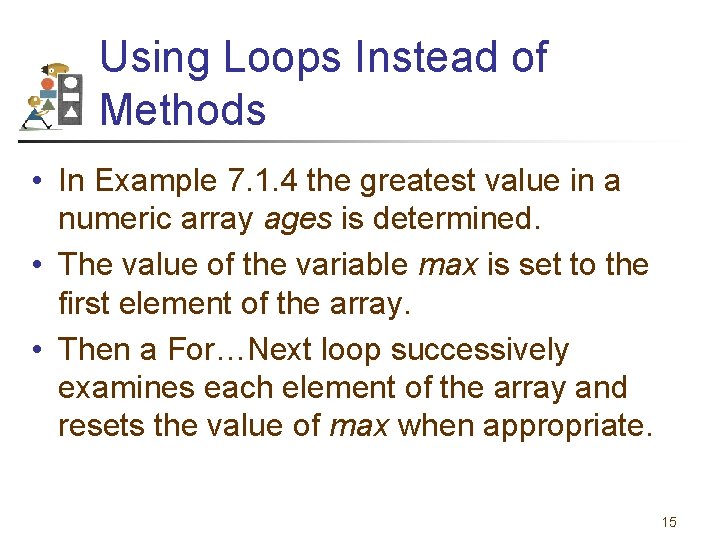
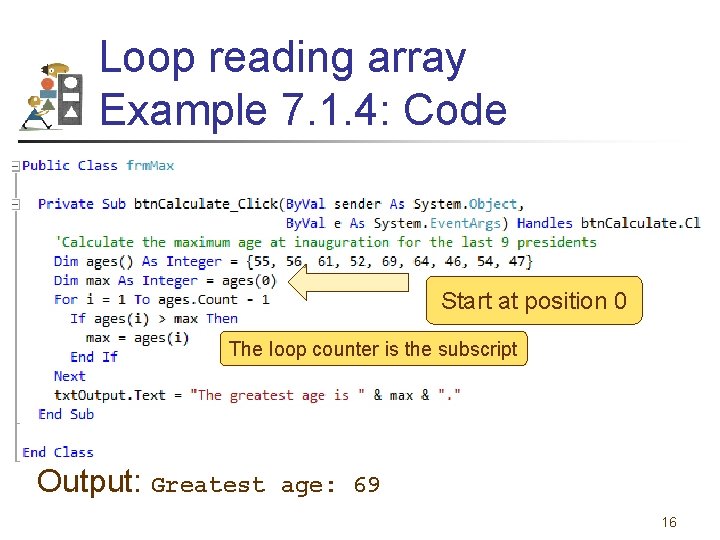
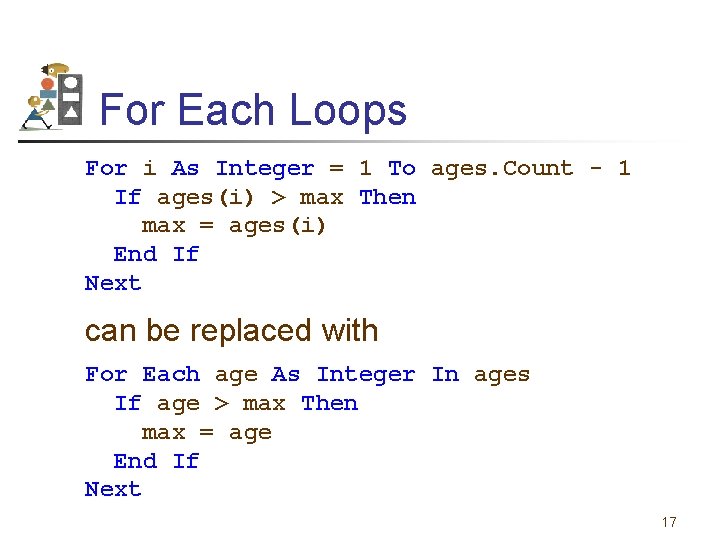
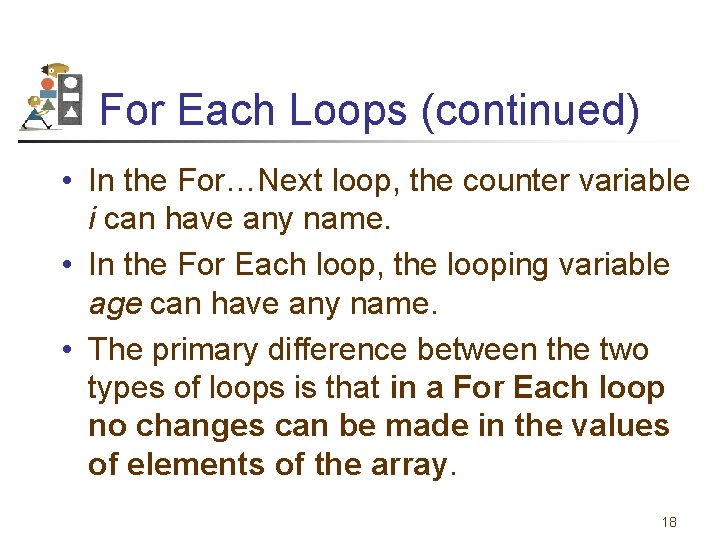
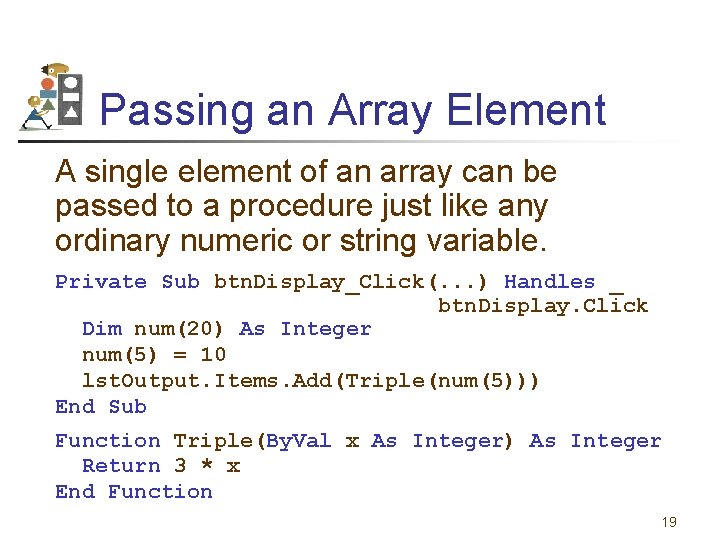
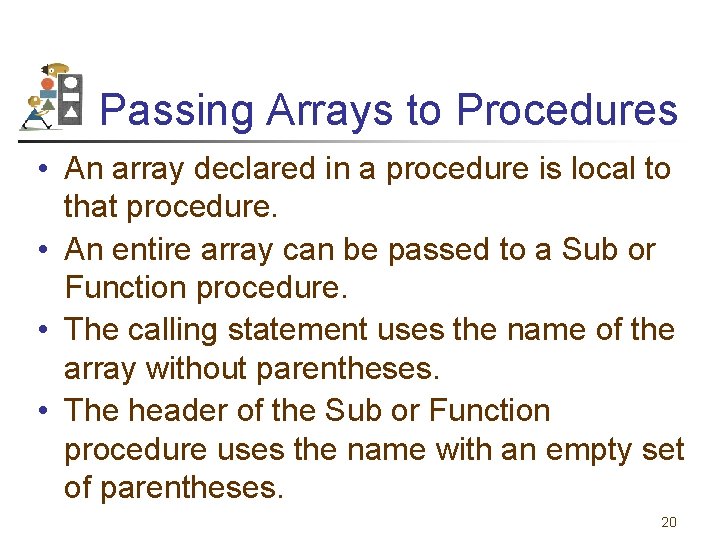
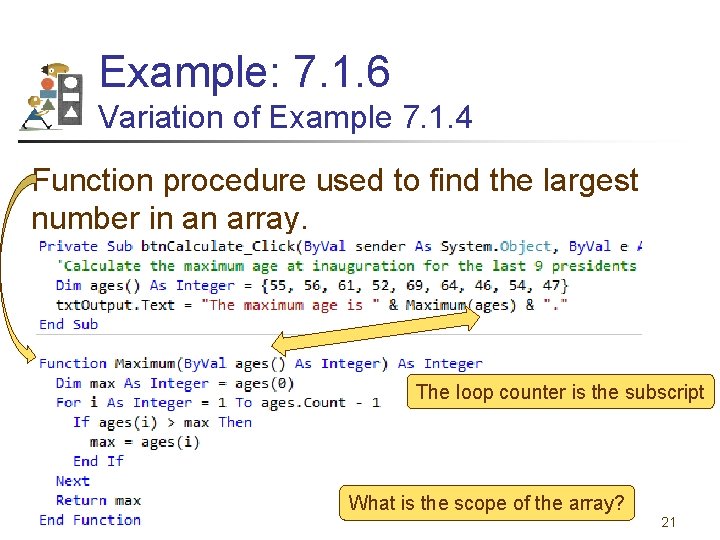
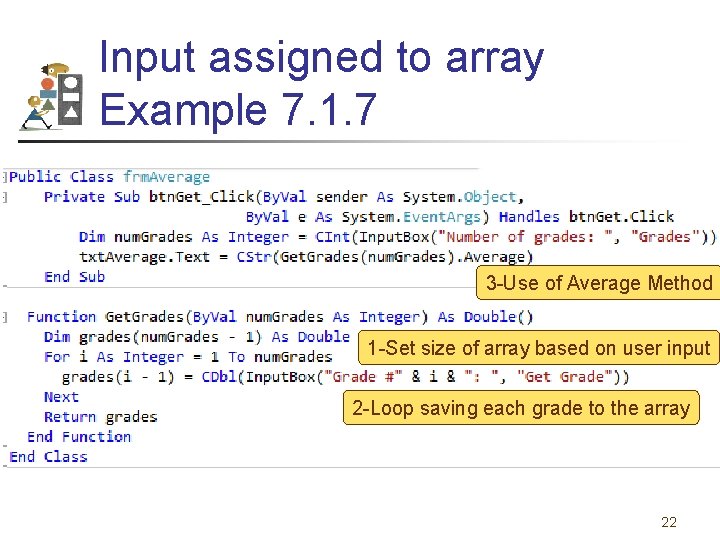
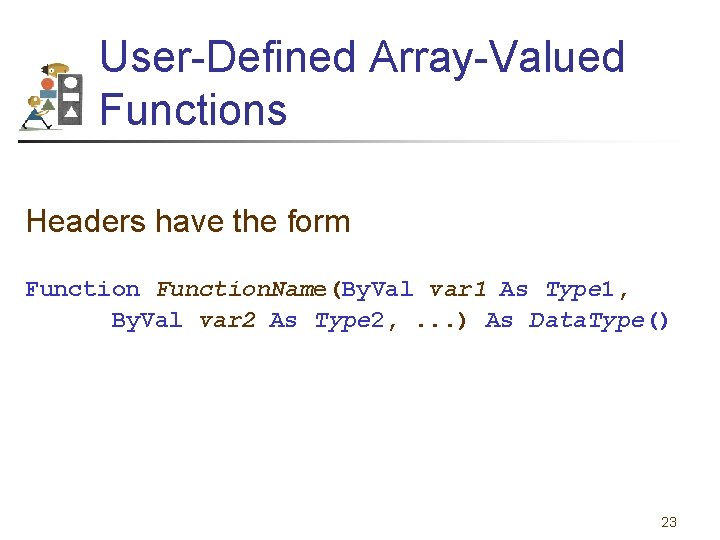
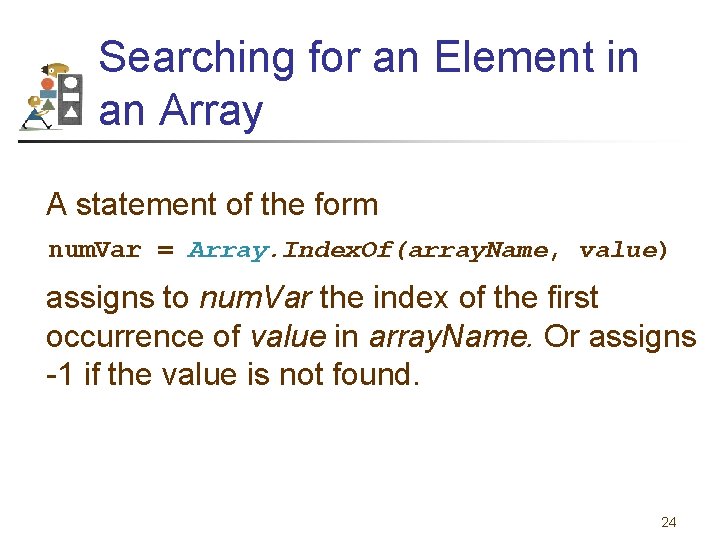
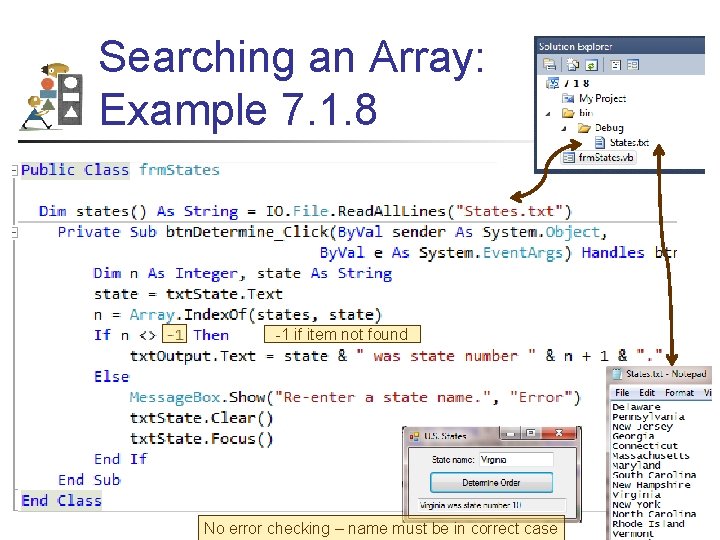
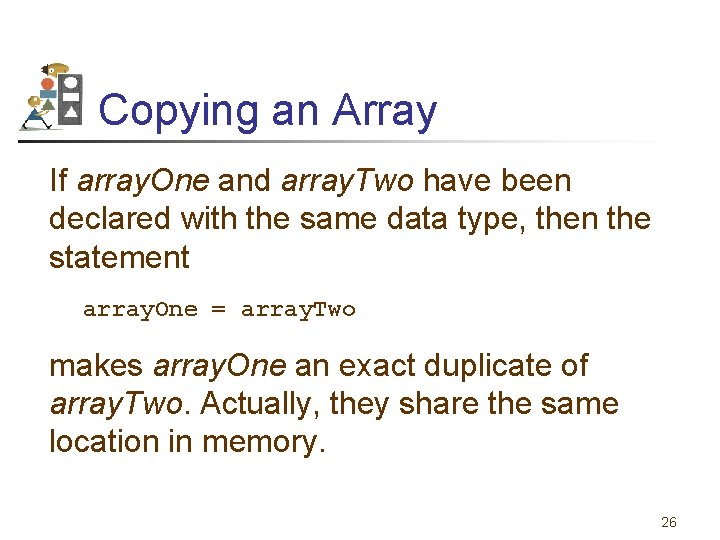
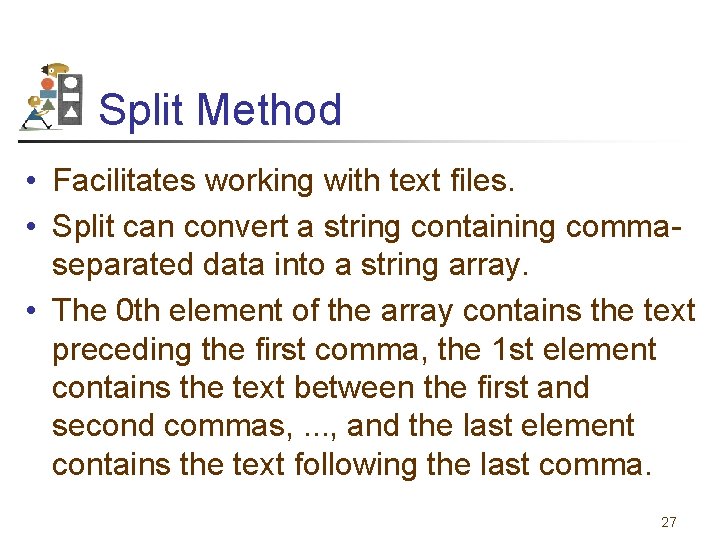
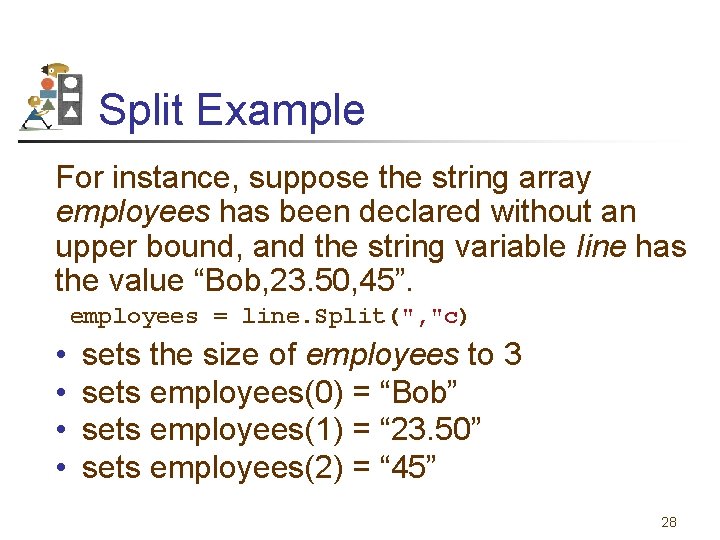
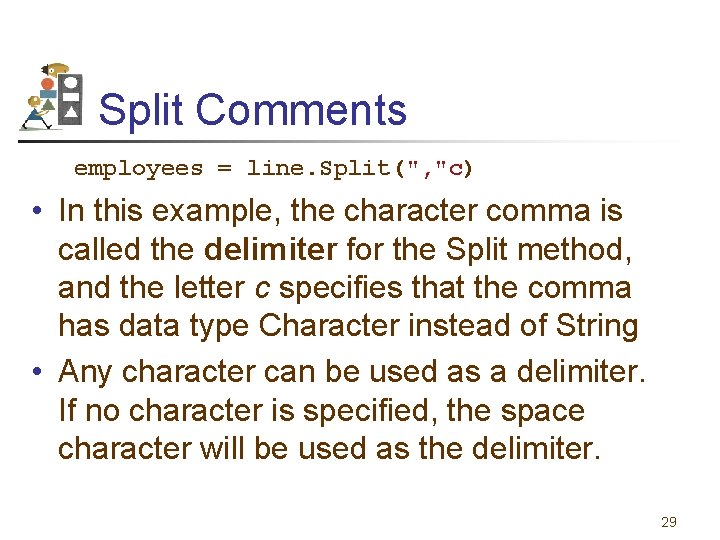
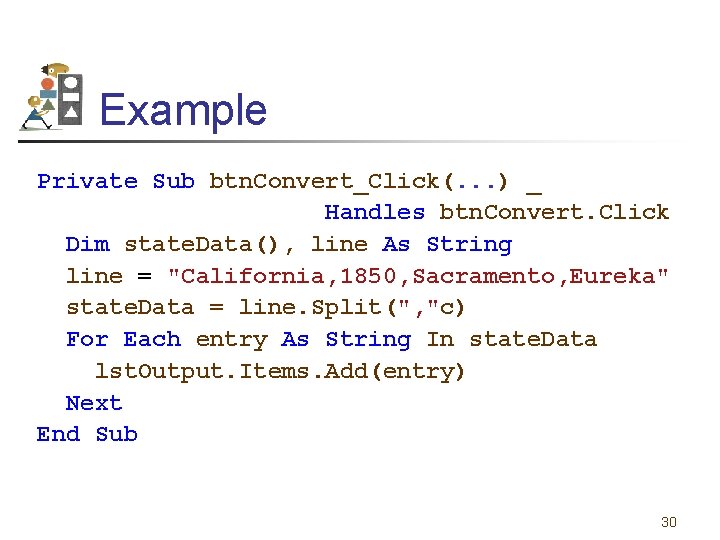
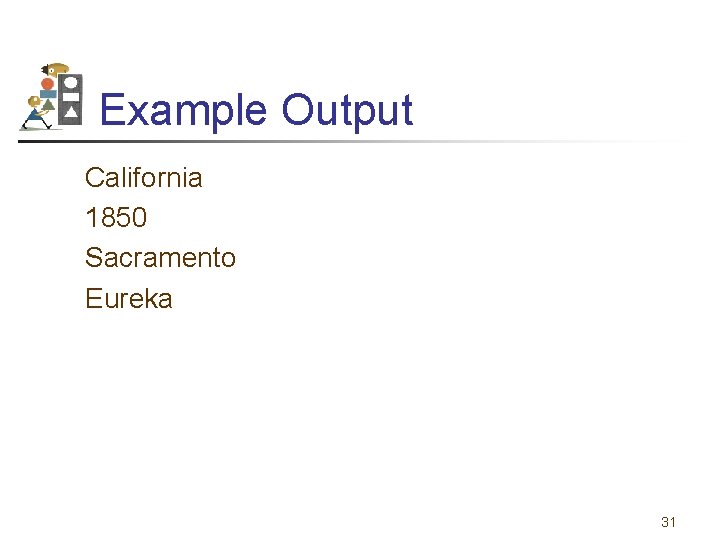
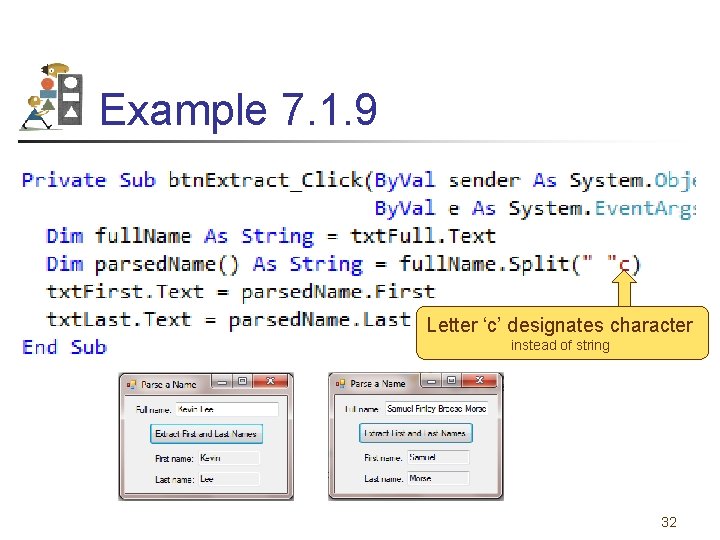
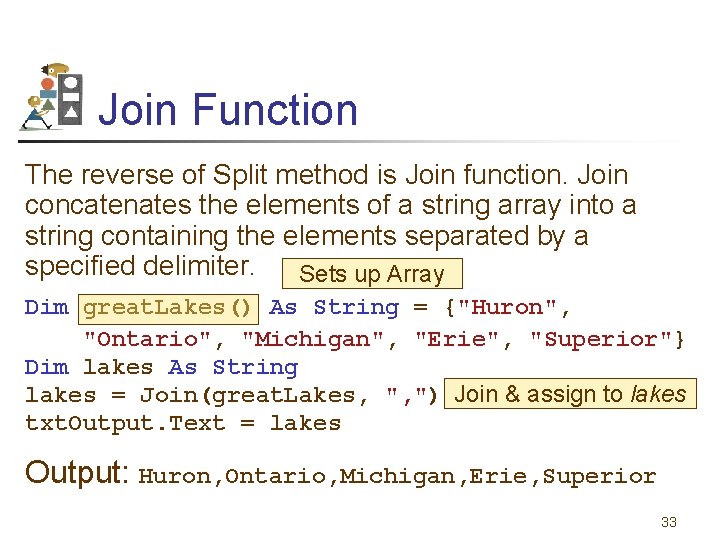
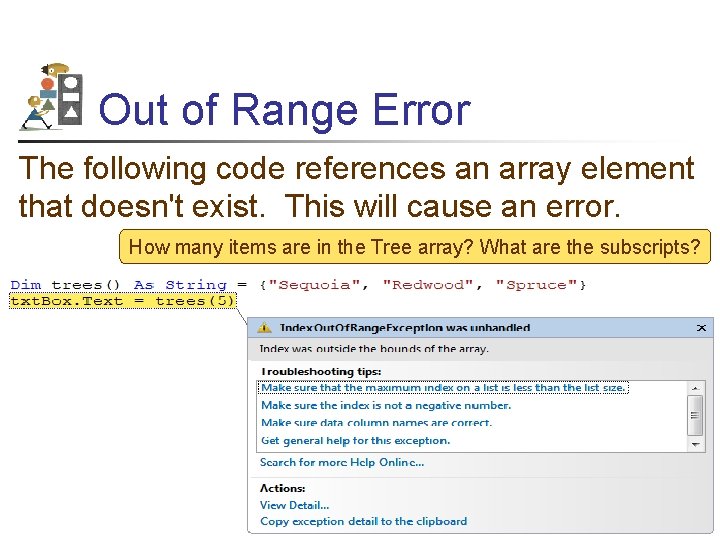
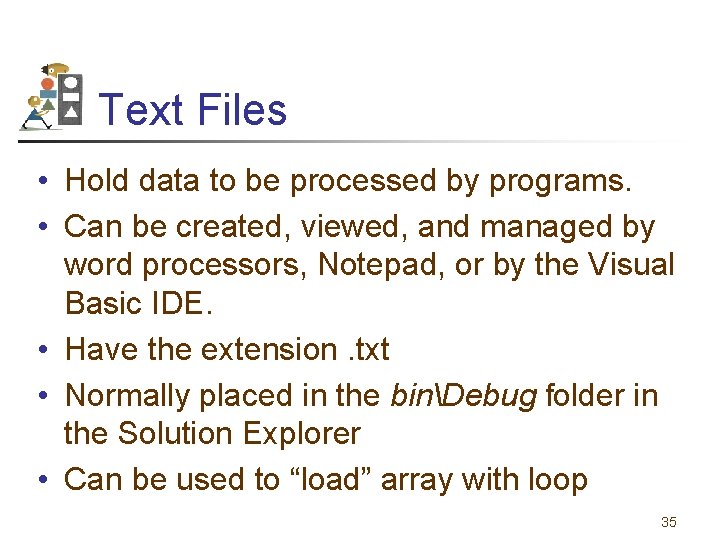
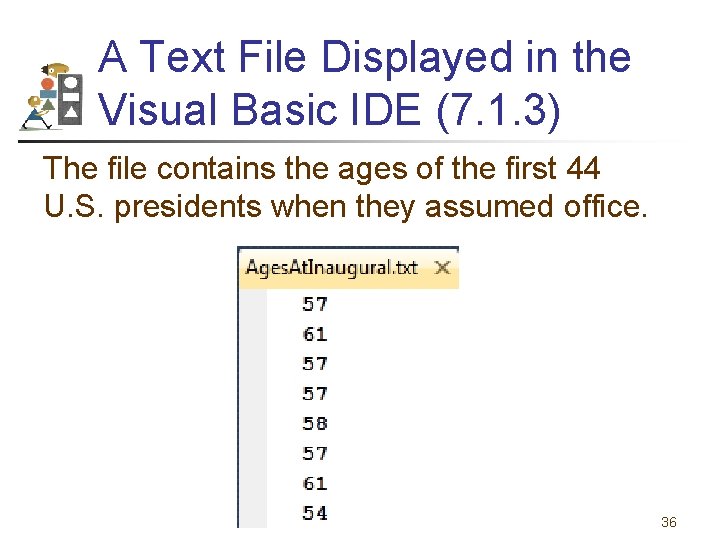
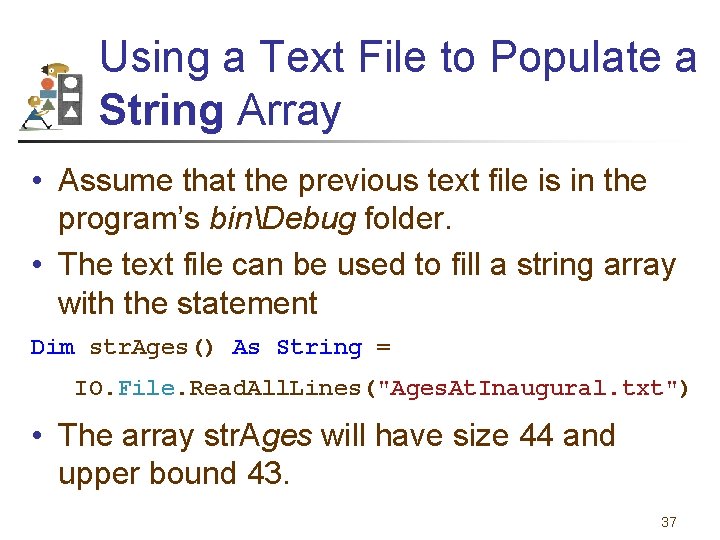
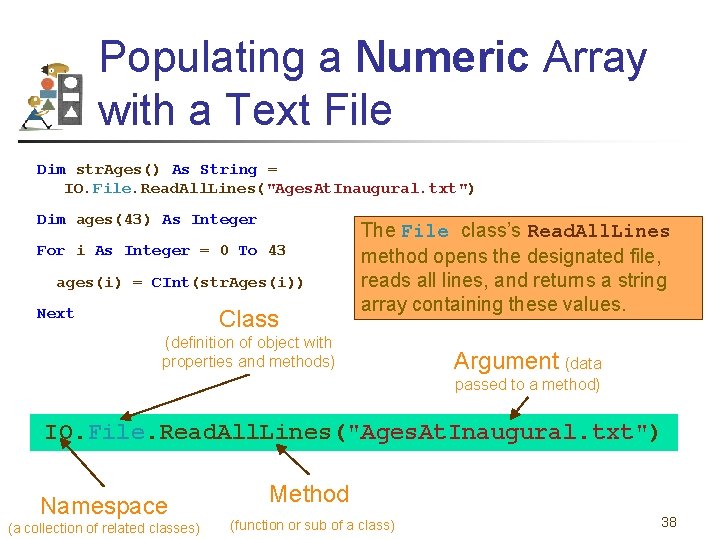
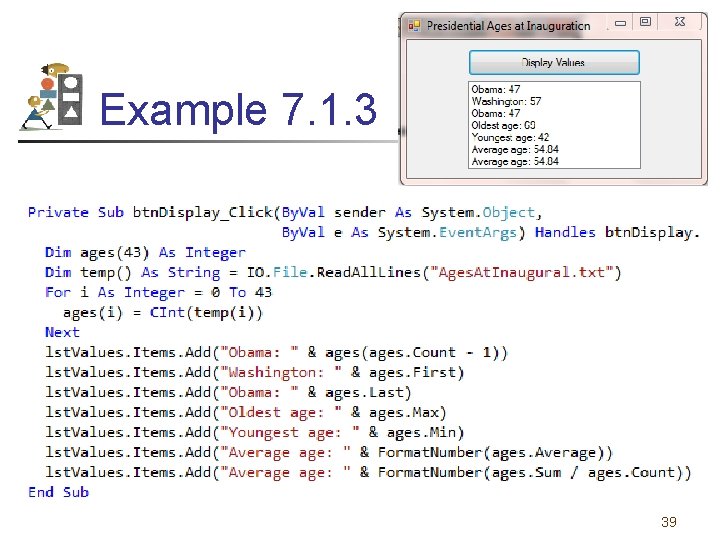
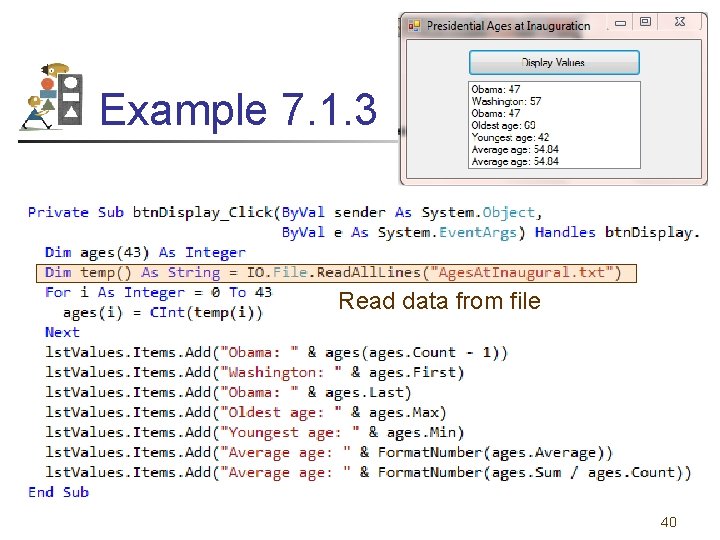
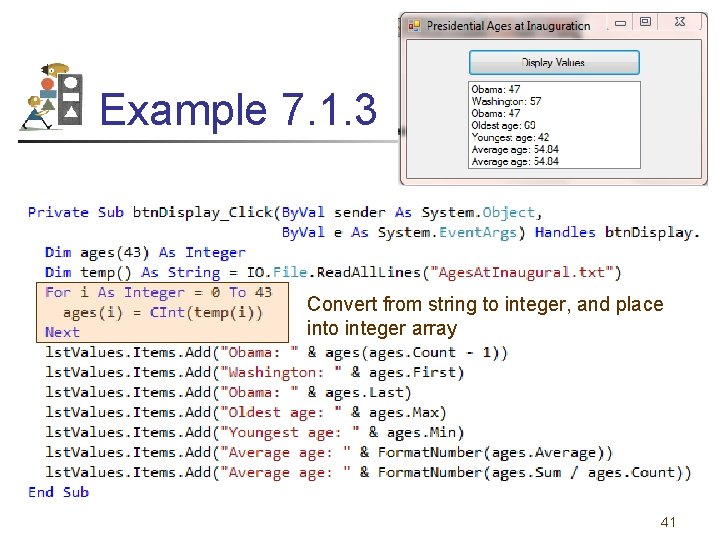
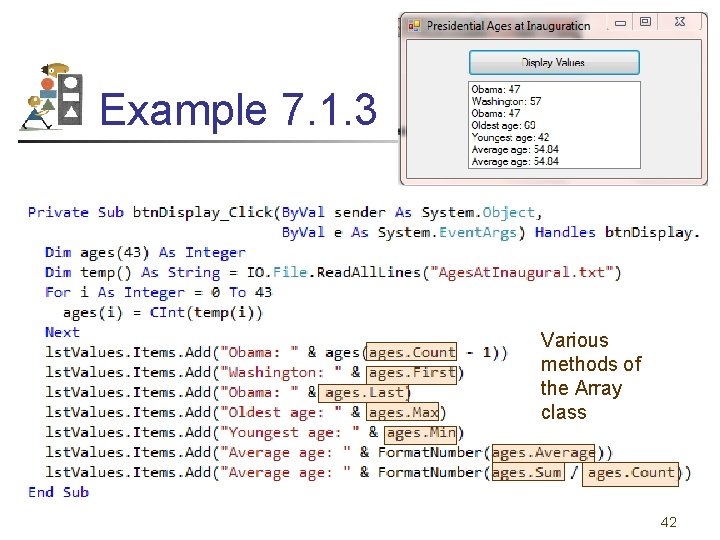
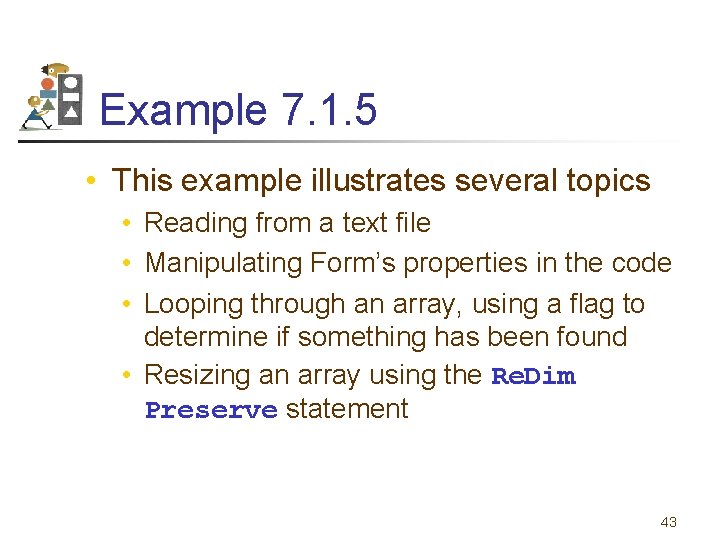
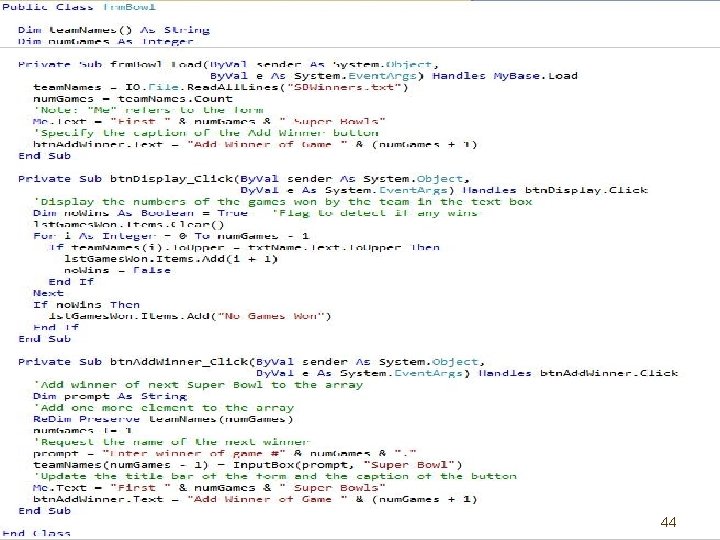
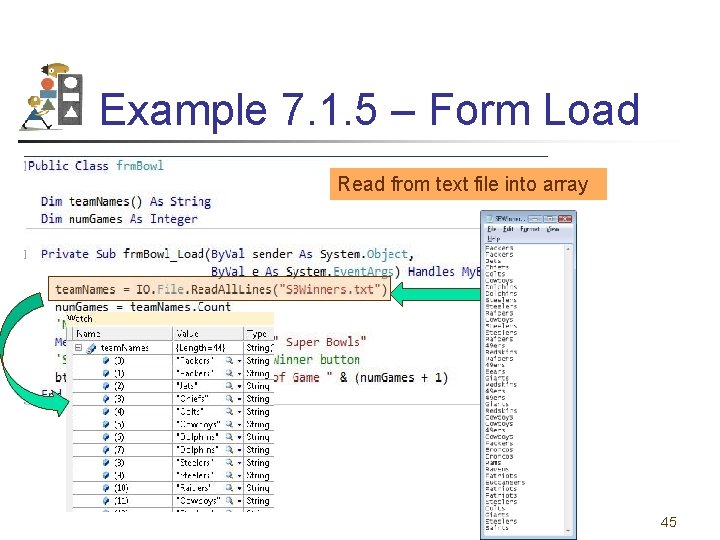
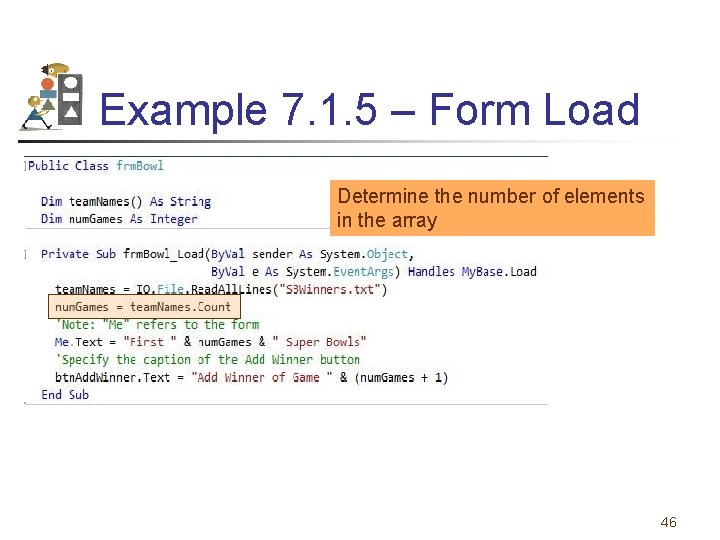
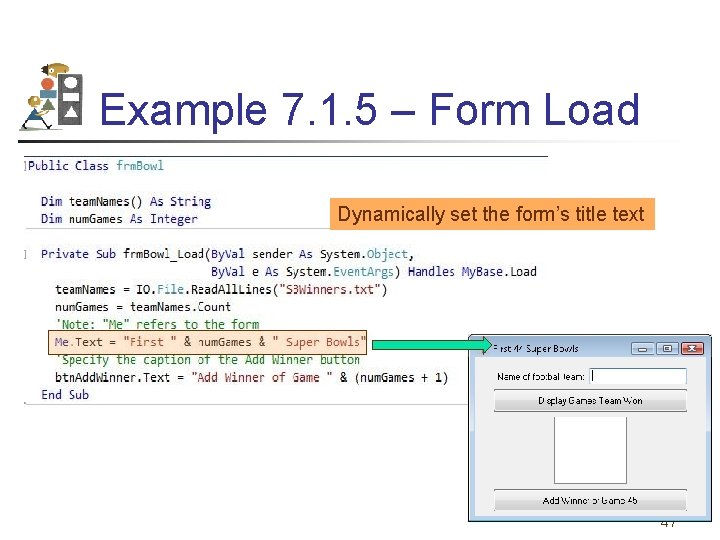
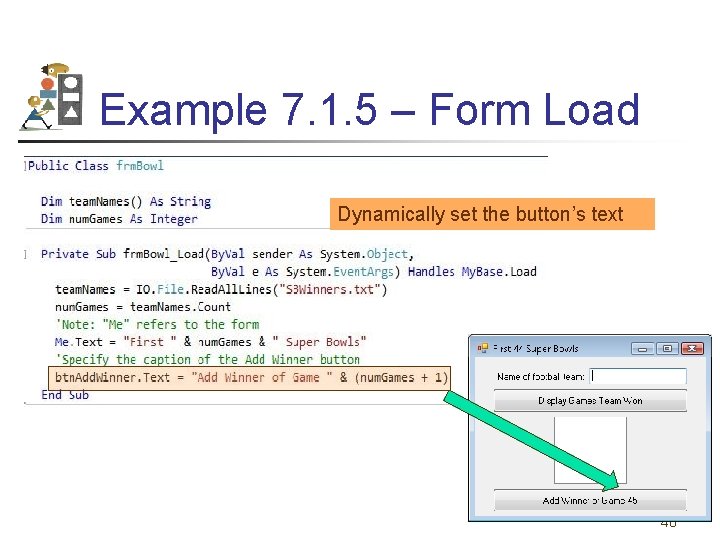
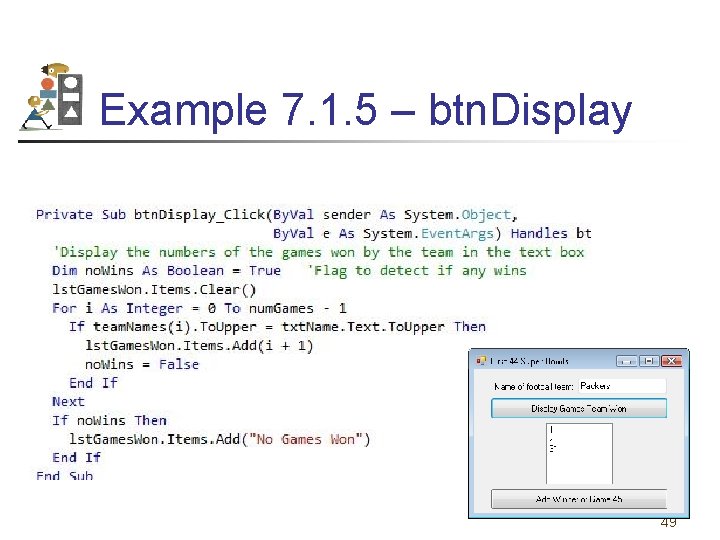
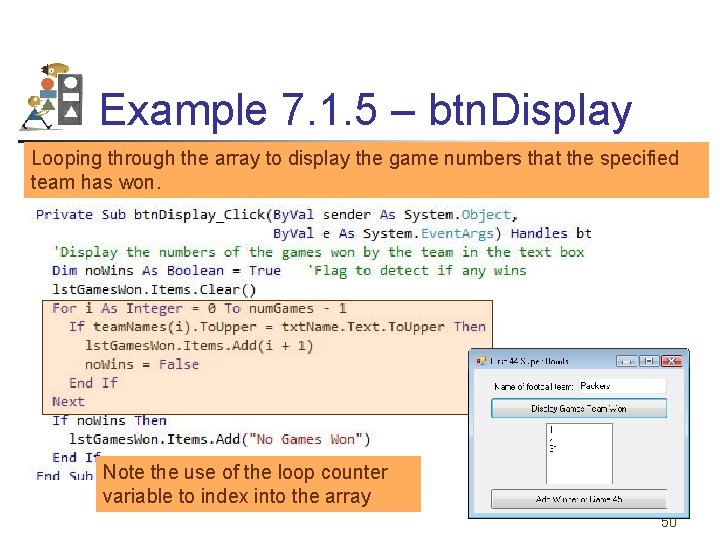
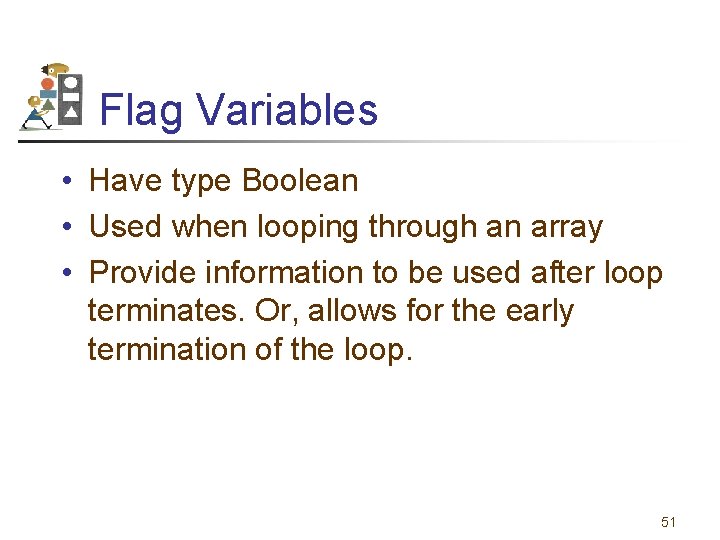
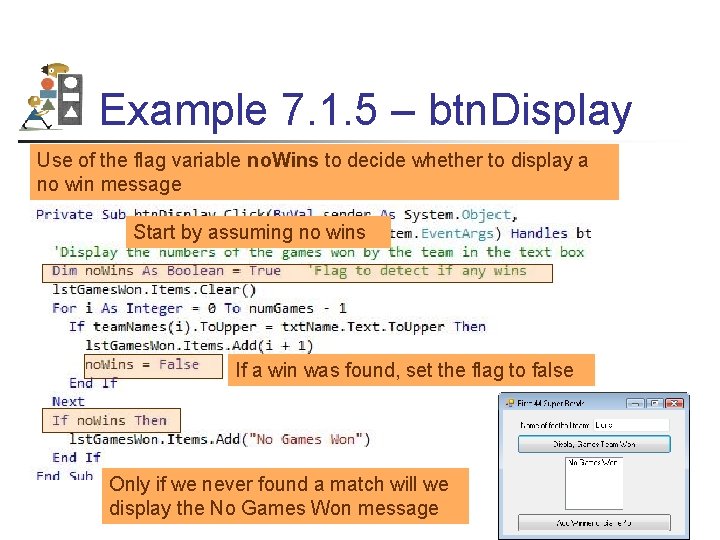
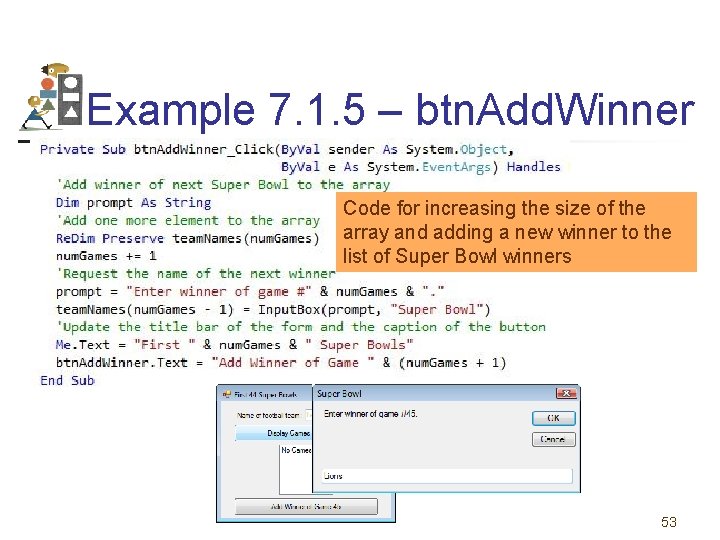
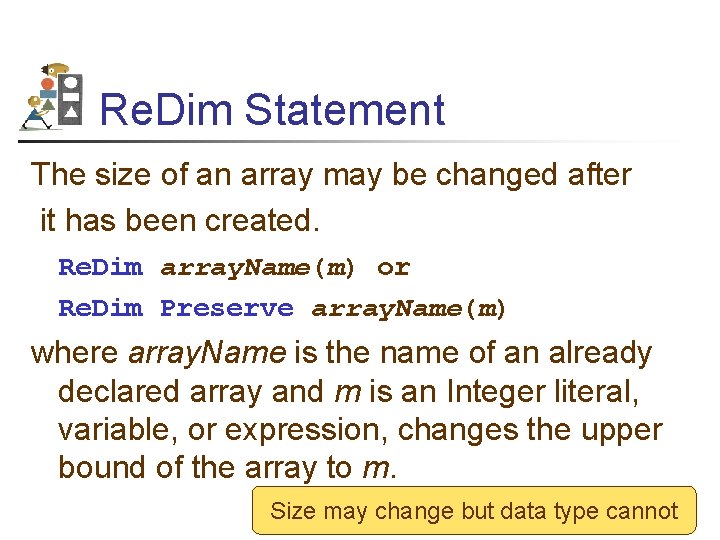
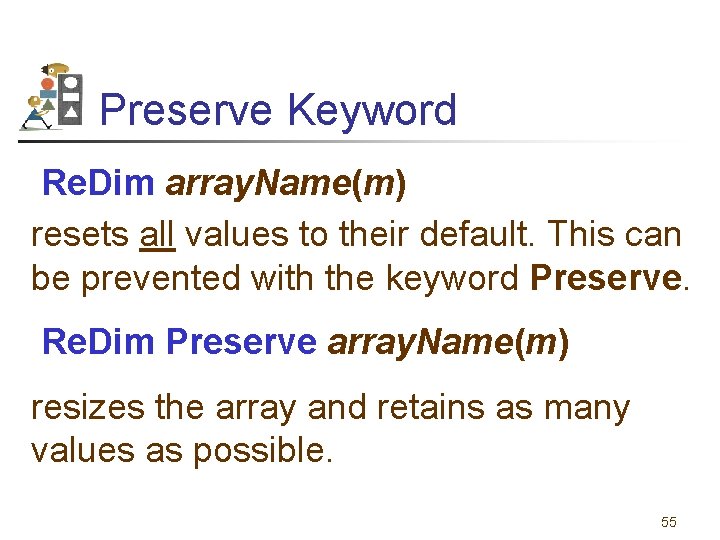
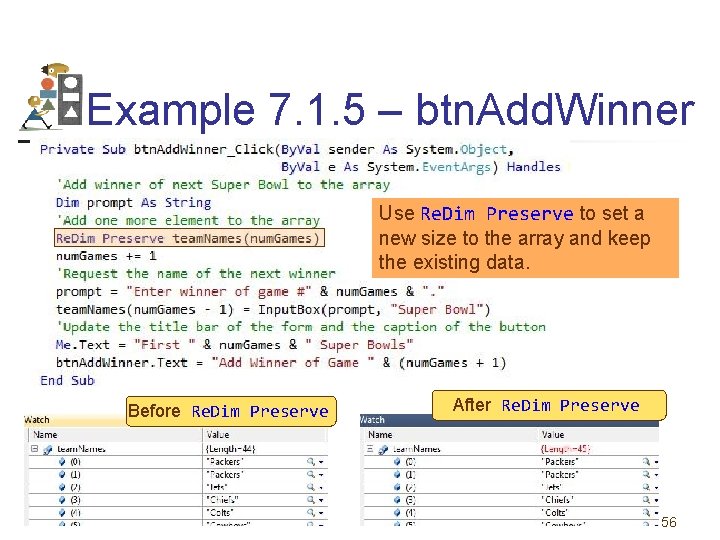
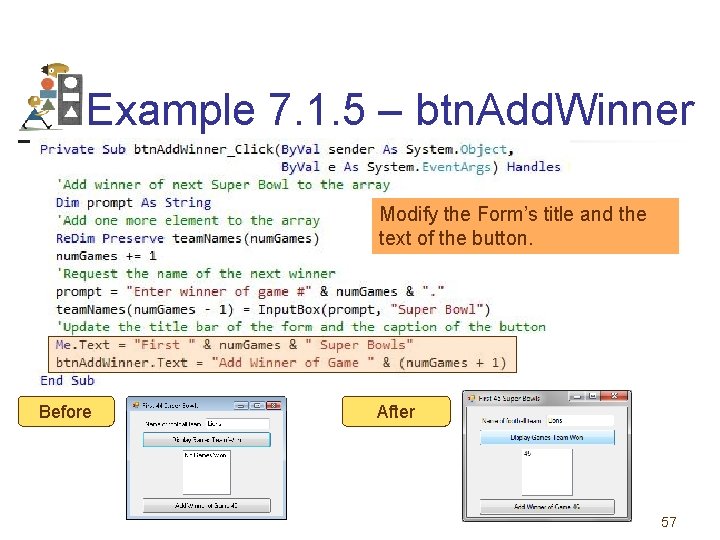
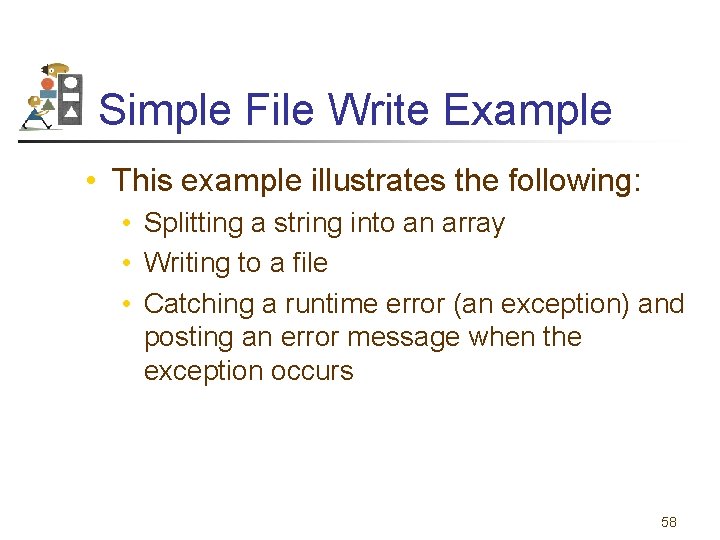
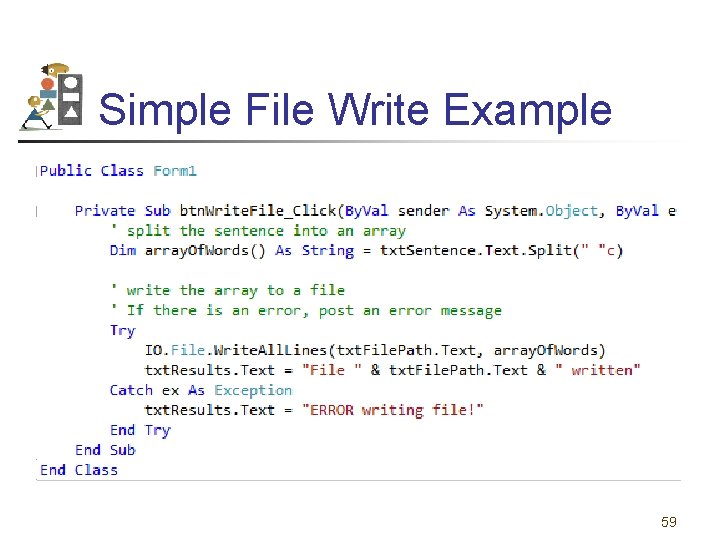
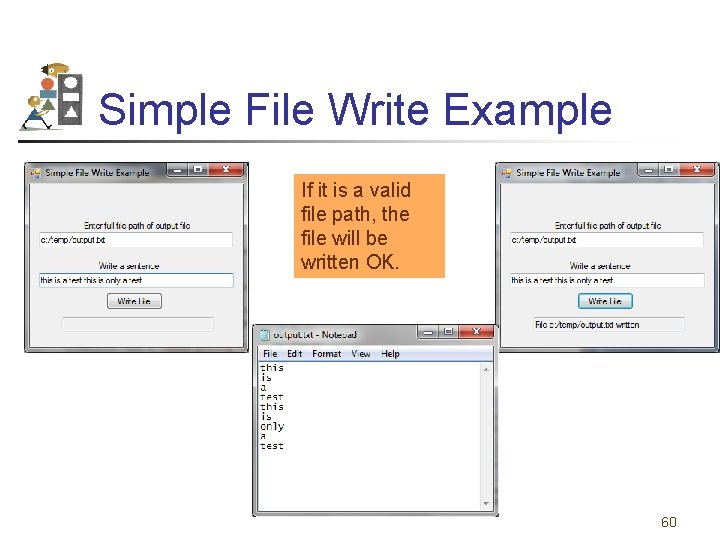
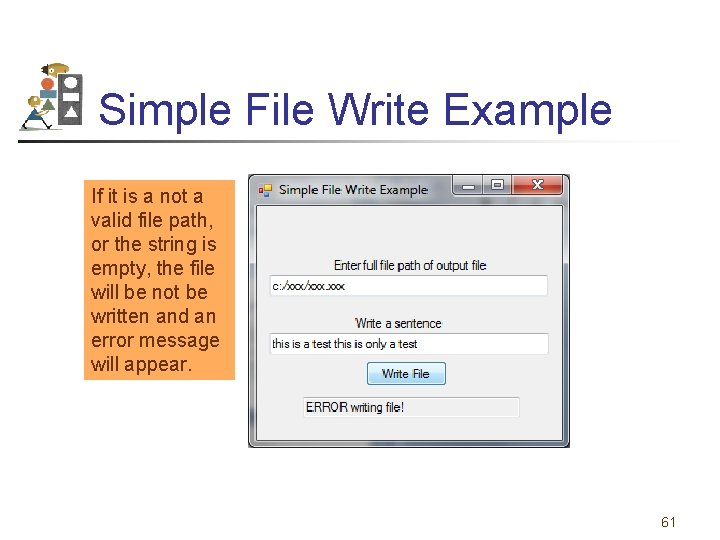
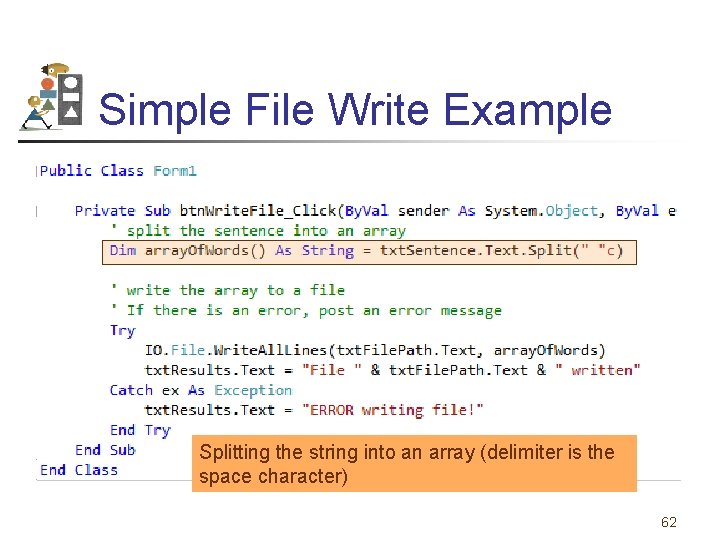
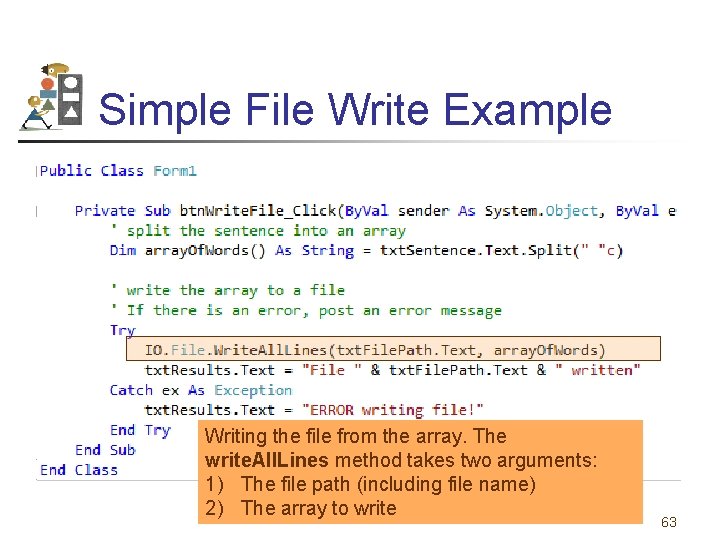
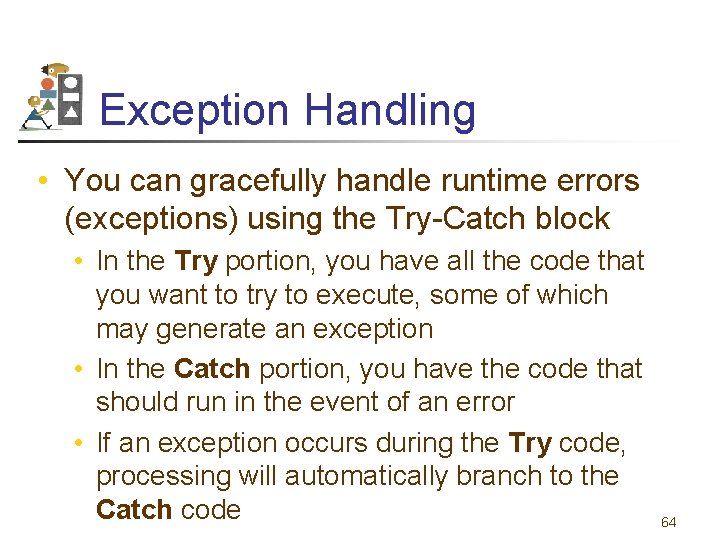
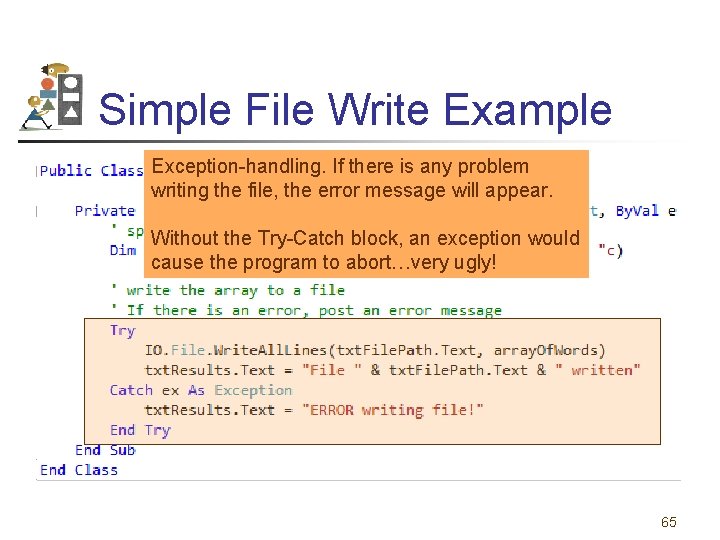
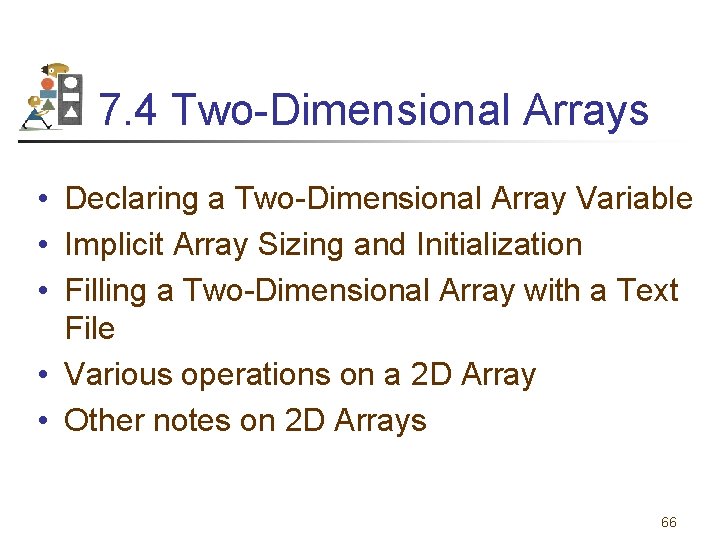
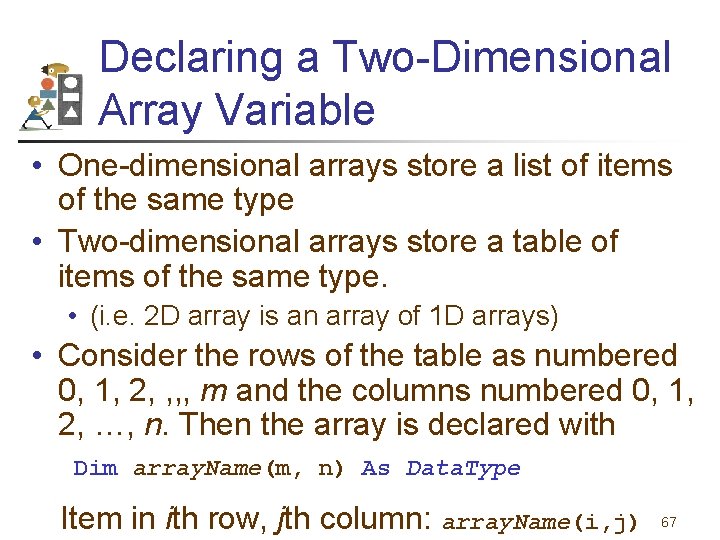
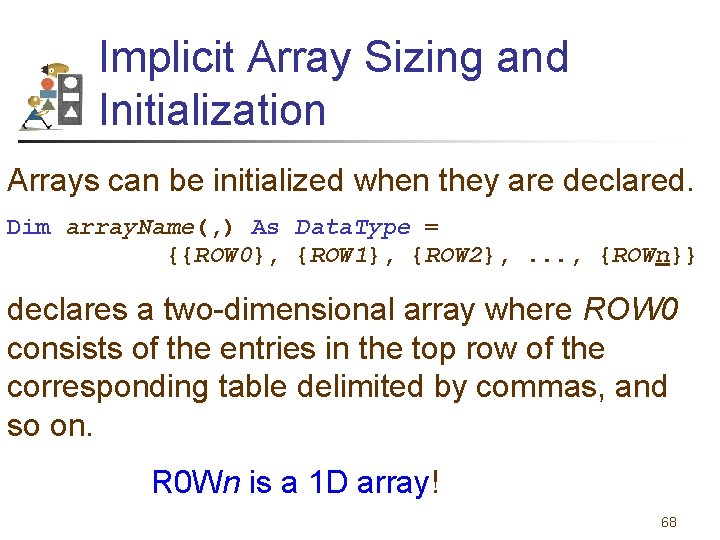
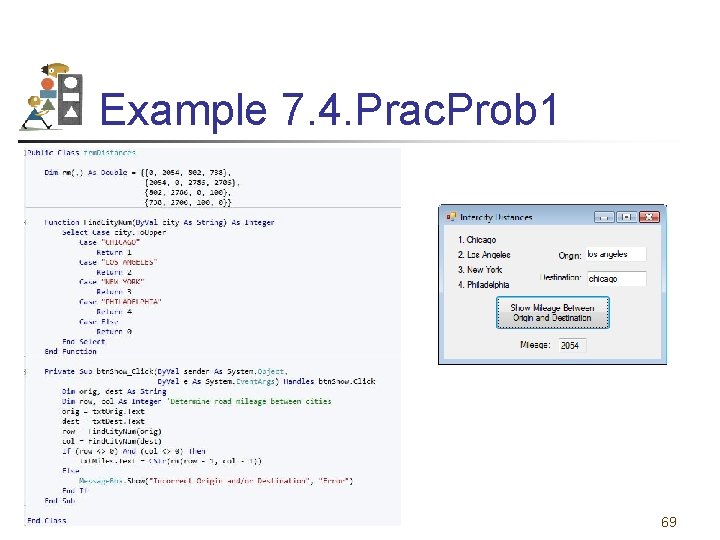
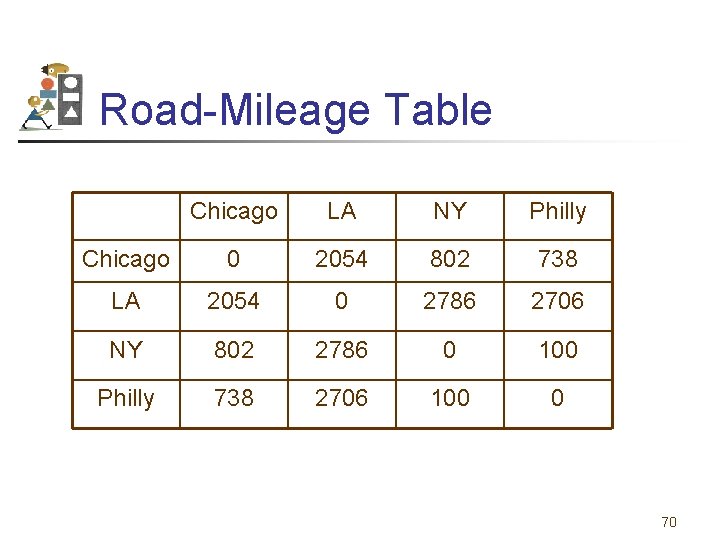
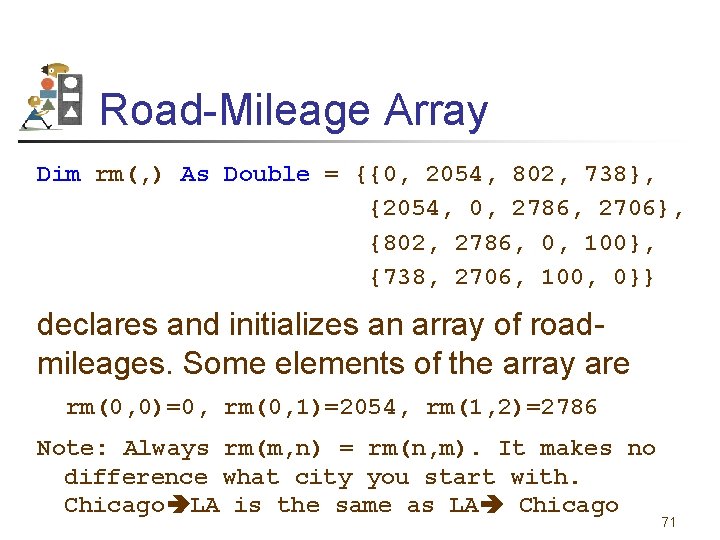
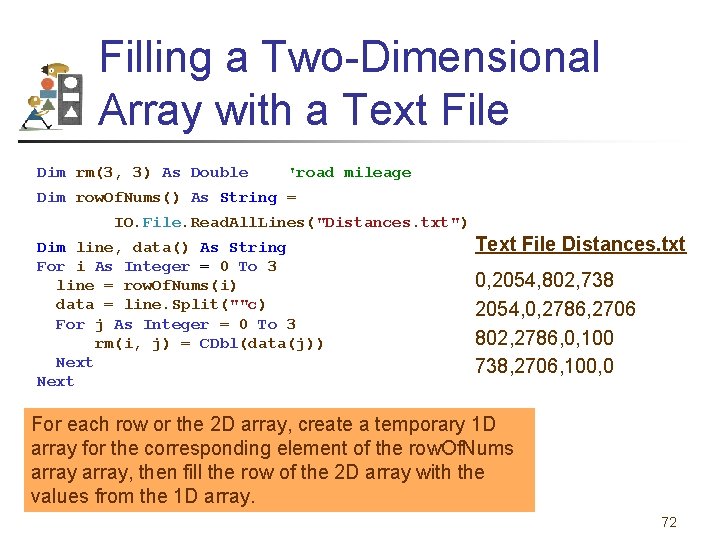
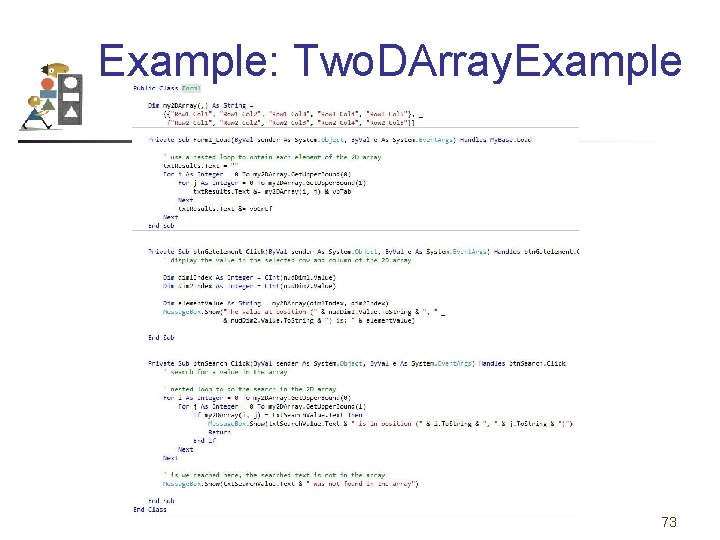
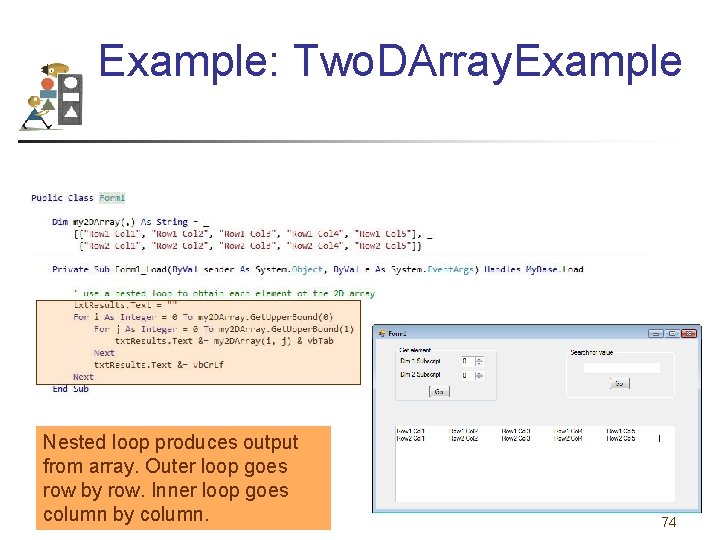
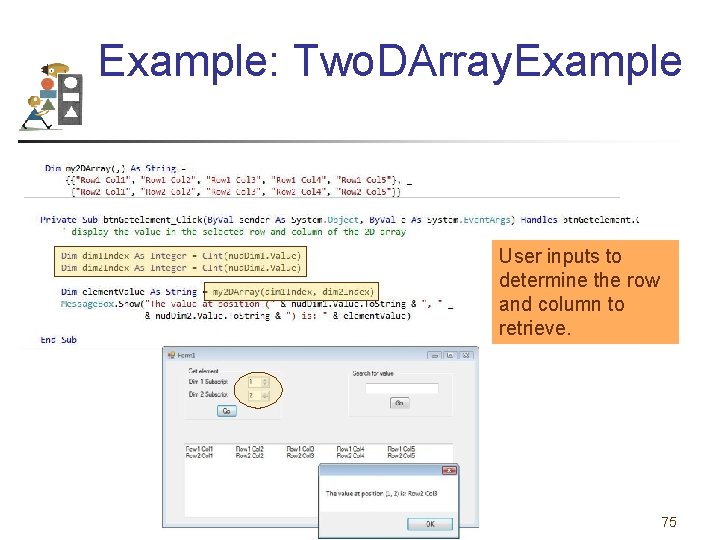
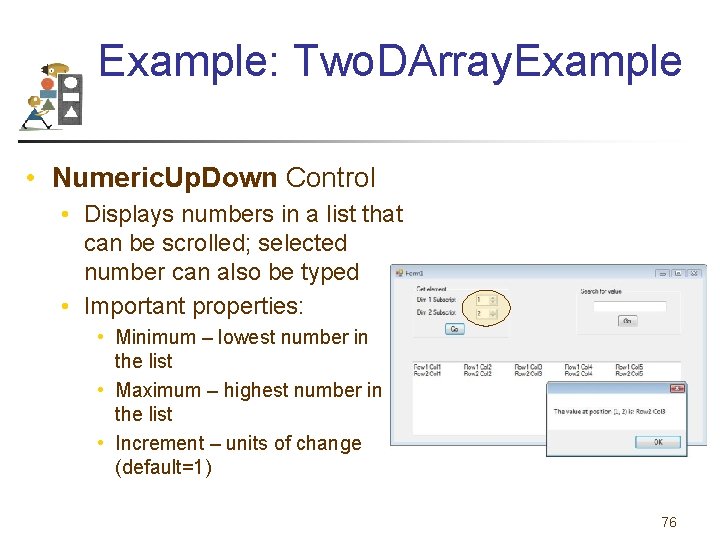
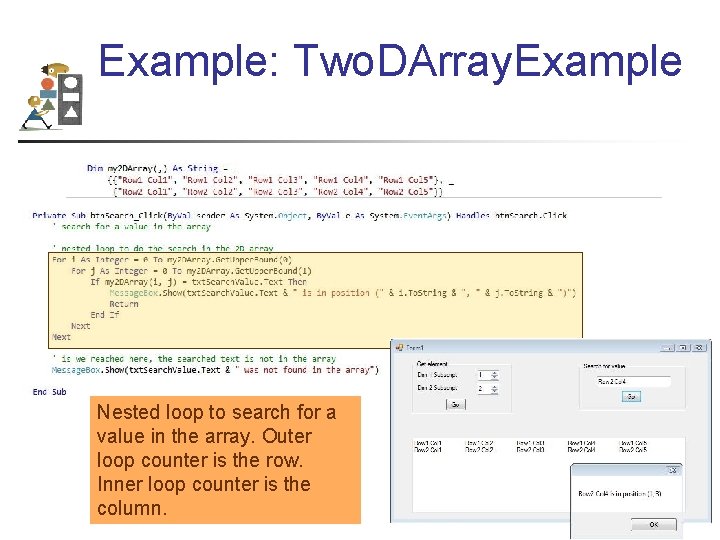
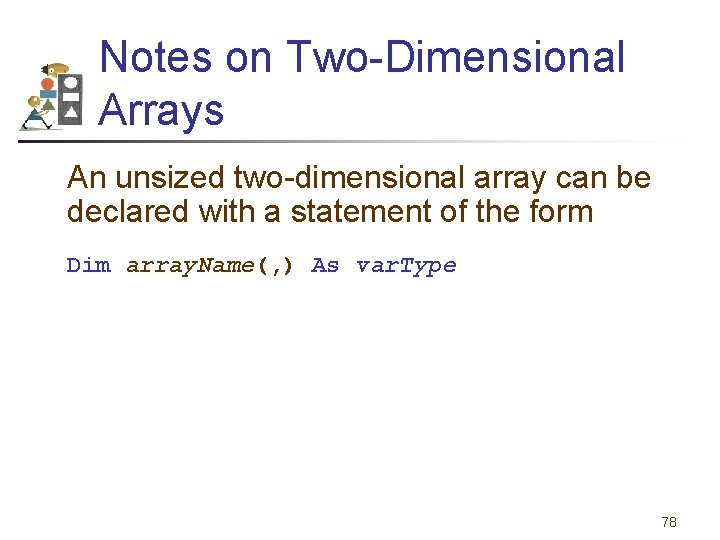
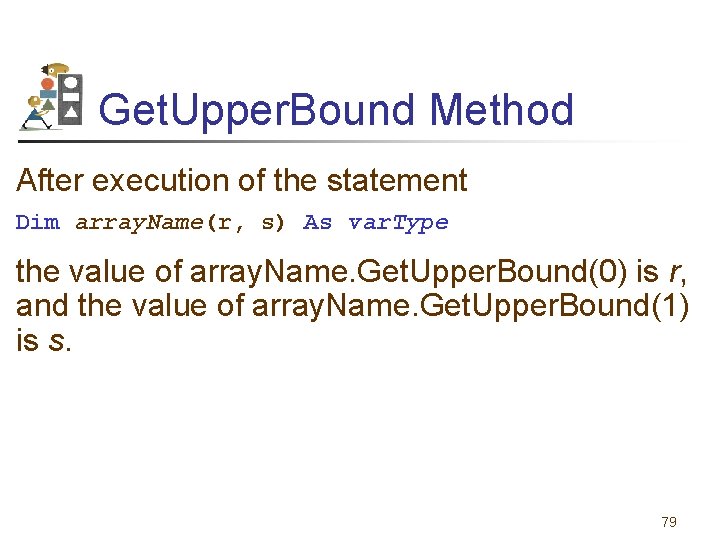
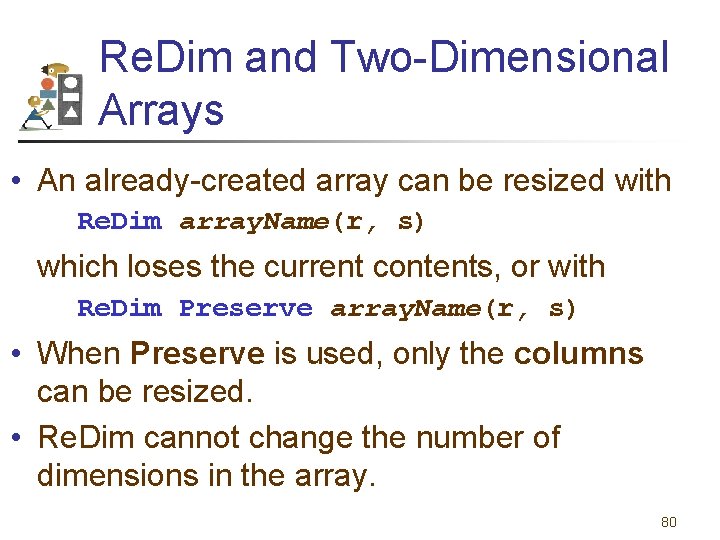
- Slides: 80
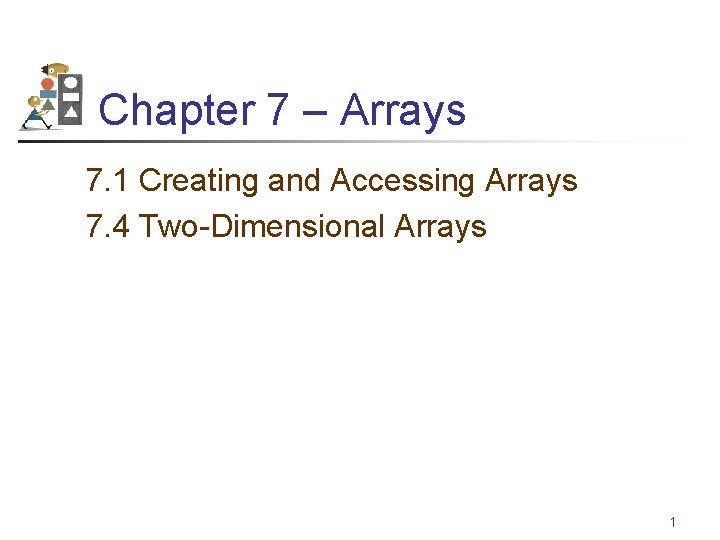
Chapter 7 – Arrays 7. 1 Creating and Accessing Arrays 7. 4 Two-Dimensional Arrays 1
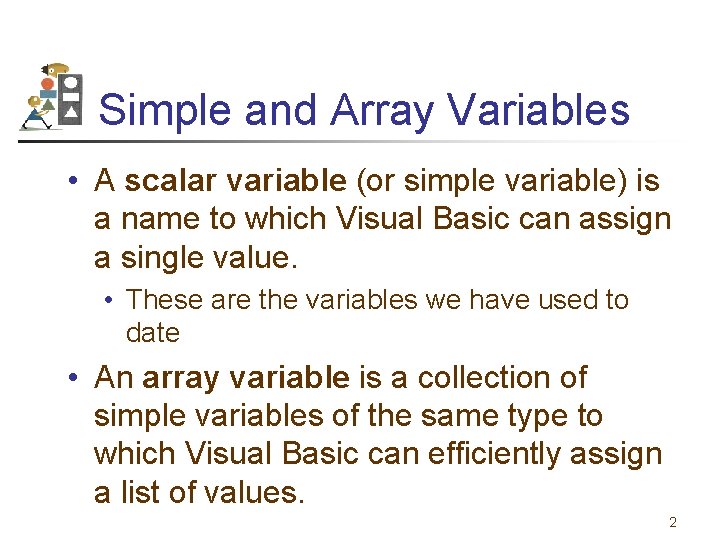
Simple and Array Variables • A scalar variable (or simple variable) is a name to which Visual Basic can assign a single value. • These are the variables we have used to date • An array variable is a collection of simple variables of the same type to which Visual Basic can efficiently assign a list of values. 2
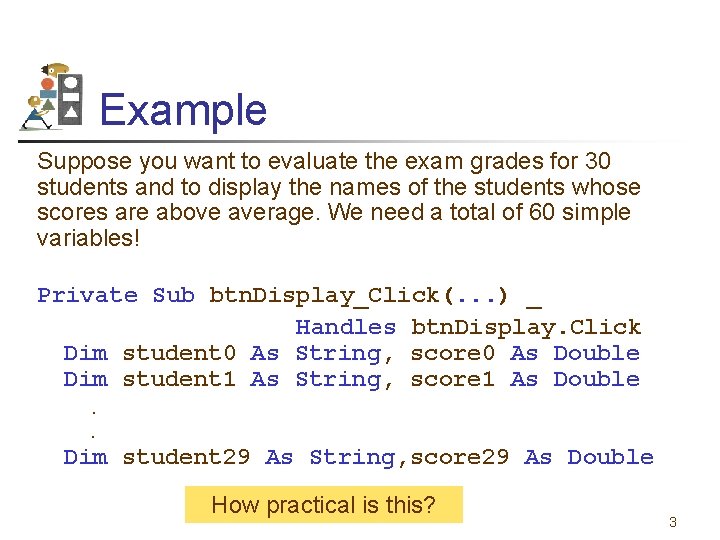
Example Suppose you want to evaluate the exam grades for 30 students and to display the names of the students whose scores are above average. We need a total of 60 simple variables! Private Sub btn. Display_Click(. . . ) _ Handles btn. Display. Click Dim student 0 As String, score 0 As Double Dim student 1 As String, score 1 As Double. . Dim student 29 As String, score 29 As Double How practical is this? 3
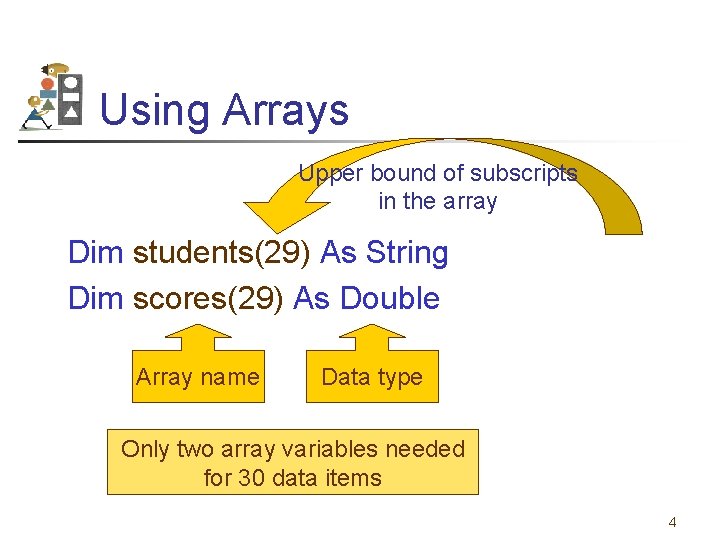
Using Arrays Upper bound of subscripts in the array Dim students(29) As String Dim scores(29) As Double Array name Data type Only two array variables needed for 30 data items 4
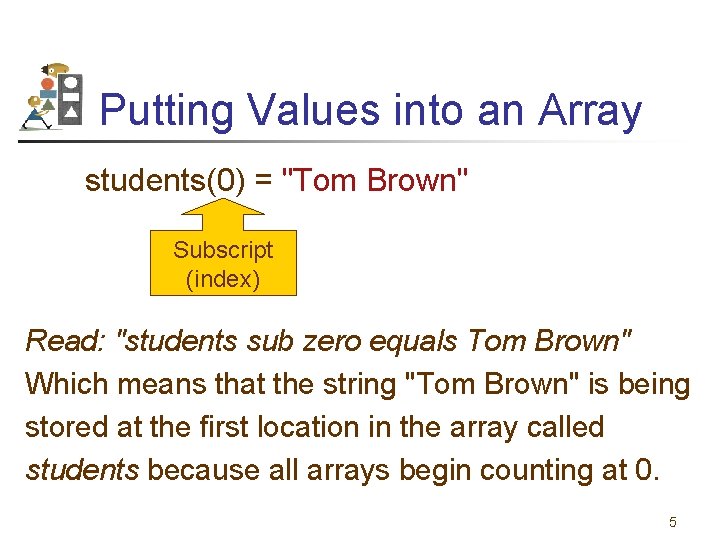
Putting Values into an Array students(0) = "Tom Brown" Subscript (index) Read: "students sub zero equals Tom Brown" Which means that the string "Tom Brown" is being stored at the first location in the array called students because all arrays begin counting at 0. 5
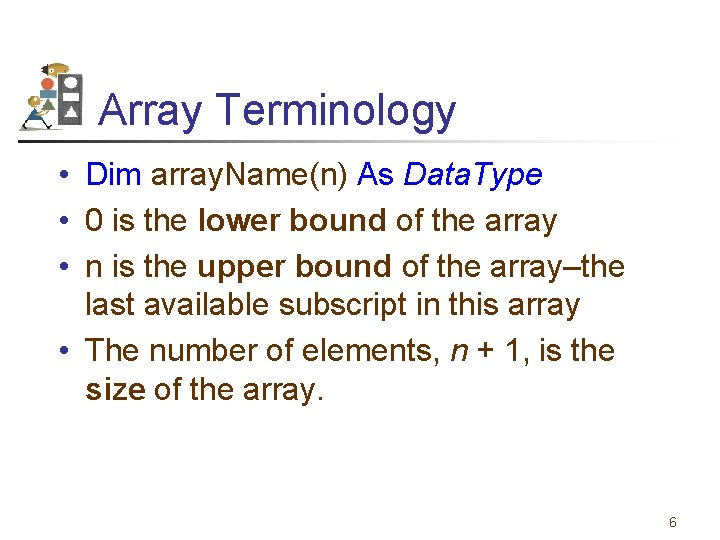
Array Terminology • Dim array. Name(n) As Data. Type • 0 is the lower bound of the array • n is the upper bound of the array–the last available subscript in this array • The number of elements, n + 1, is the size of the array. 6
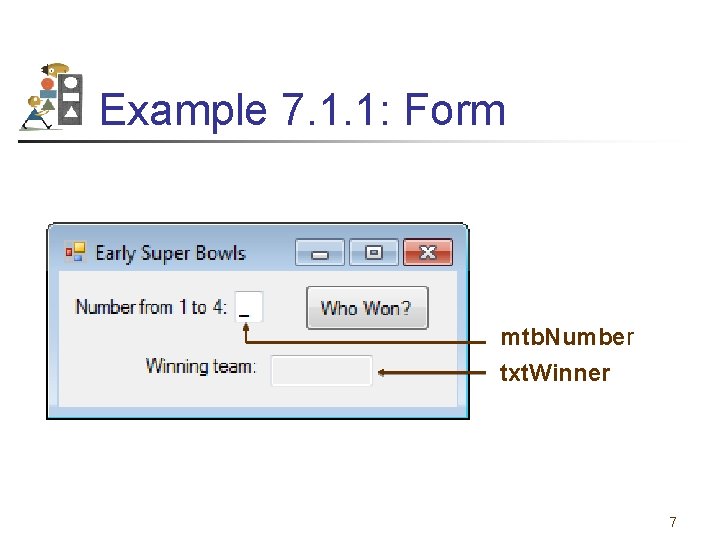
Example 7. 1. 1: Form mtb. Number txt. Winner 7
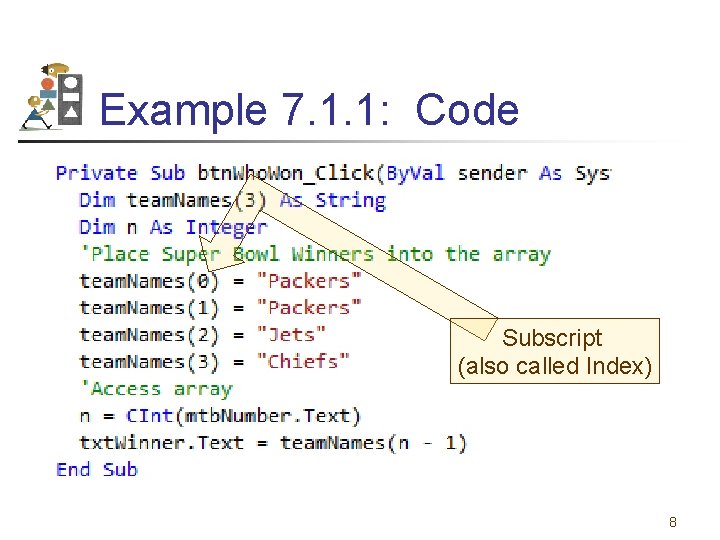
Example 7. 1. 1: Code Subscript (also called Index) 8
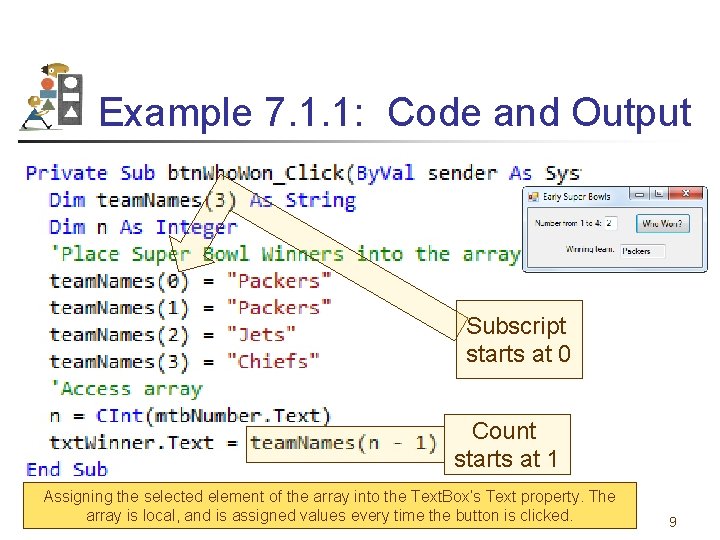
Example 7. 1. 1: Code and Output Subscript starts at 0 Count starts at 1 Assigning the selected element of the array into the Text. Box’s Text property. The array is local, and is assigned values every time the button is clicked. 9
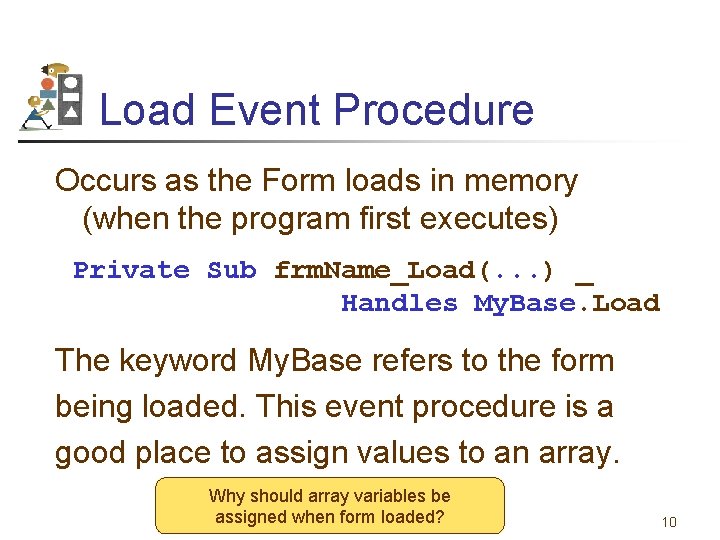
Load Event Procedure Occurs as the Form loads in memory (when the program first executes) Private Sub frm. Name_Load(. . . ) _ Handles My. Base. Load The keyword My. Base refers to the form being loaded. This event procedure is a good place to assign values to an array. Why should array variables be assigned when form loaded? 10
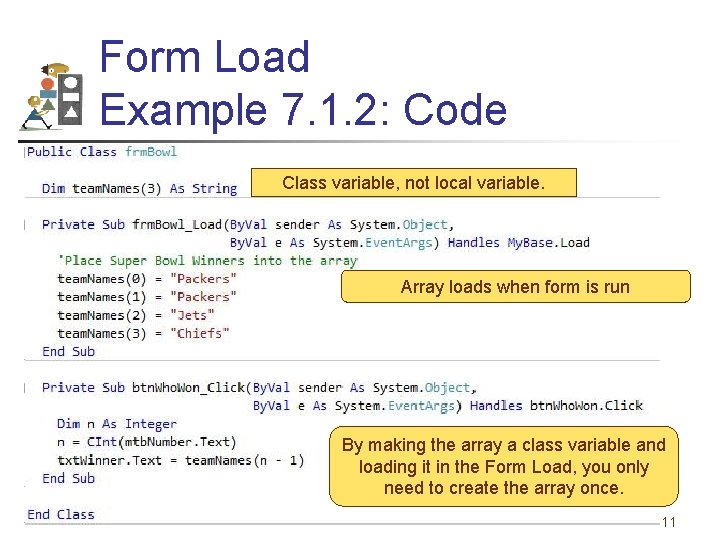
Form Load Example 7. 1. 2: Code Class variable, not local variable. Array loads when form is run By making the array a class variable and loading it in the Form Load, you only need to create the array once. 11
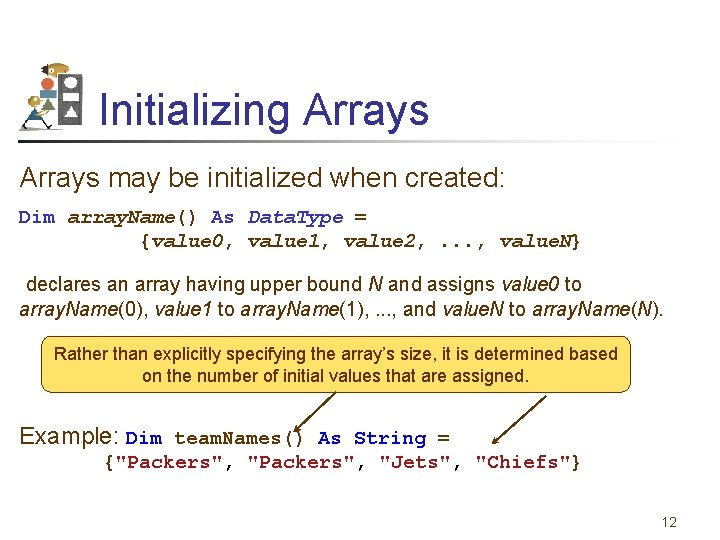
Initializing Arrays may be initialized when created: Dim array. Name() As Data. Type = {value 0, value 1, value 2, . . . , value. N} declares an array having upper bound N and assigns value 0 to array. Name(0), value 1 to array. Name(1), . . . , and value. N to array. Name(N). Rather than explicitly specifying the array’s size, it is determined based on the number of initial values that are assigned. Example: Dim team. Names() As String = {"Packers", "Jets", "Chiefs"} 12
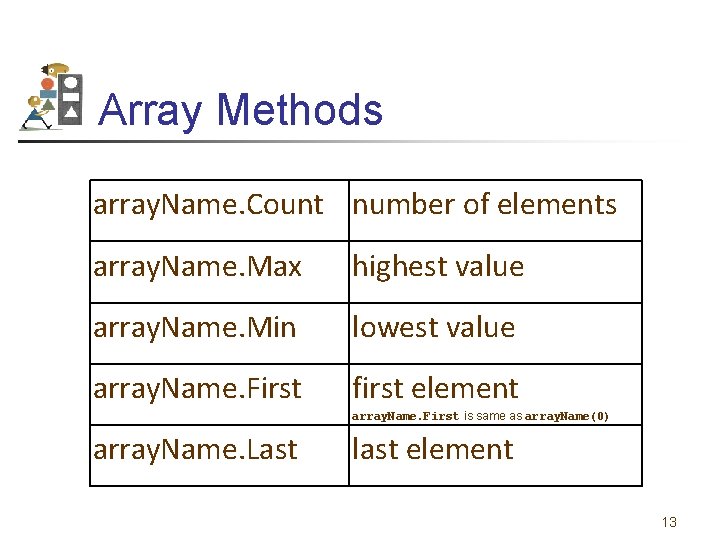
Array Methods array. Name. Count number of elements array. Name. Max highest value array. Name. Min lowest value array. Name. First first element array. Name. First is same as array. Name(0) array. Name. Last last element 13
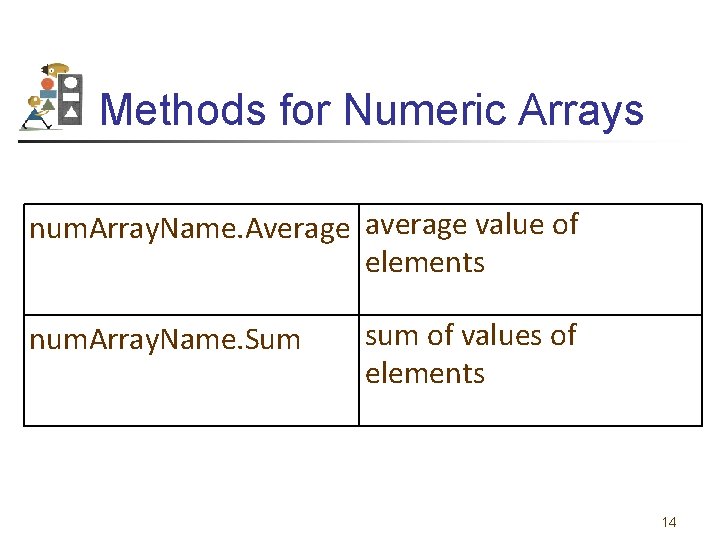
Methods for Numeric Arrays num. Array. Name. Average average value of elements num. Array. Name. Sum sum of values of elements 14
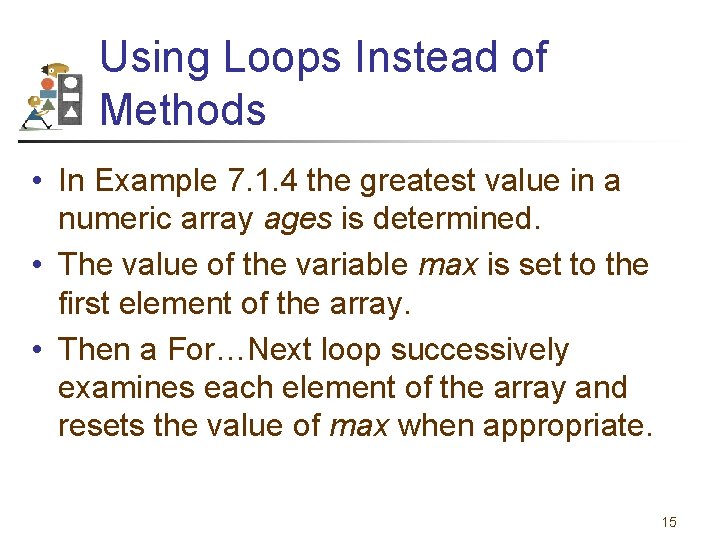
Using Loops Instead of Methods • In Example 7. 1. 4 the greatest value in a numeric array ages is determined. • The value of the variable max is set to the first element of the array. • Then a For…Next loop successively examines each element of the array and resets the value of max when appropriate. 15
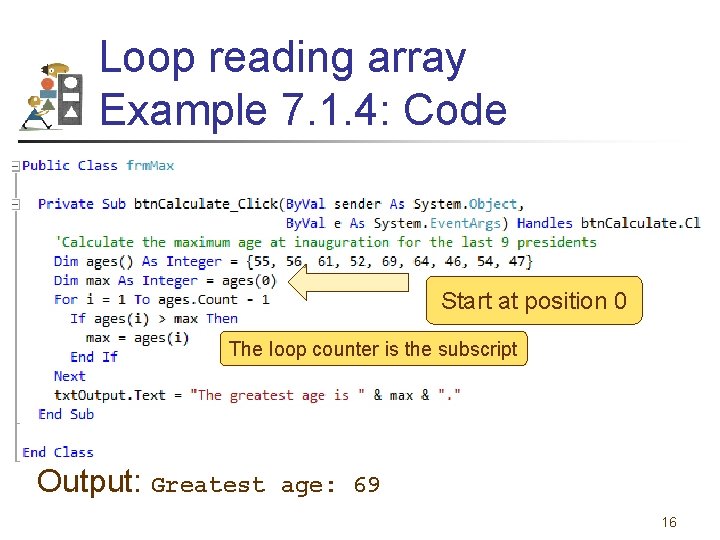
Loop reading array Example 7. 1. 4: Code Dim ages() As Integer = {55, 56, 61, 52, 69, 64, 46, 54, 47} 'last 9 presidents Dim max As Integer = ages(0) For i As Integer = 1 To ages. Count - 1 If ages(i) > max Then Start at position 0 max = ages(i) The loop counter is the subscript End If Next txt. Output. Text = "Greatest age: " & max Output: Greatest age: 69 16
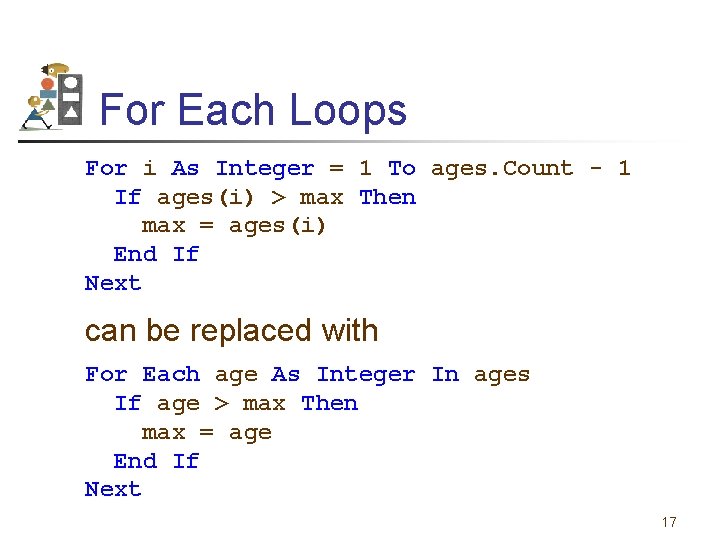
For Each Loops For i As Integer = 1 To ages. Count - 1 If ages(i) > max Then max = ages(i) End If Next can be replaced with For Each age As Integer In ages If age > max Then max = age End If Next 17
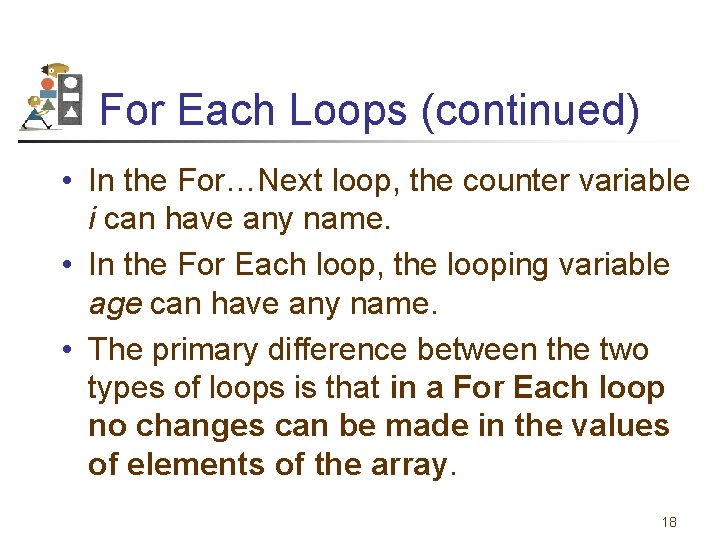
For Each Loops (continued) • In the For…Next loop, the counter variable i can have any name. • In the For Each loop, the looping variable age can have any name. • The primary difference between the two types of loops is that in a For Each loop no changes can be made in the values of elements of the array. 18
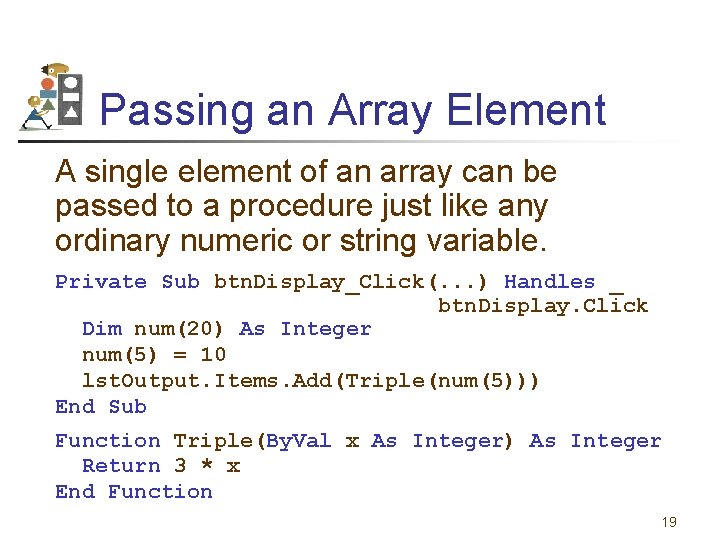
Passing an Array Element A single element of an array can be passed to a procedure just like any ordinary numeric or string variable. Private Sub btn. Display_Click(. . . ) Handles _ btn. Display. Click Dim num(20) As Integer num(5) = 10 lst. Output. Items. Add(Triple(num(5))) End Sub Function Triple(By. Val x As Integer) As Integer Return 3 * x End Function 19
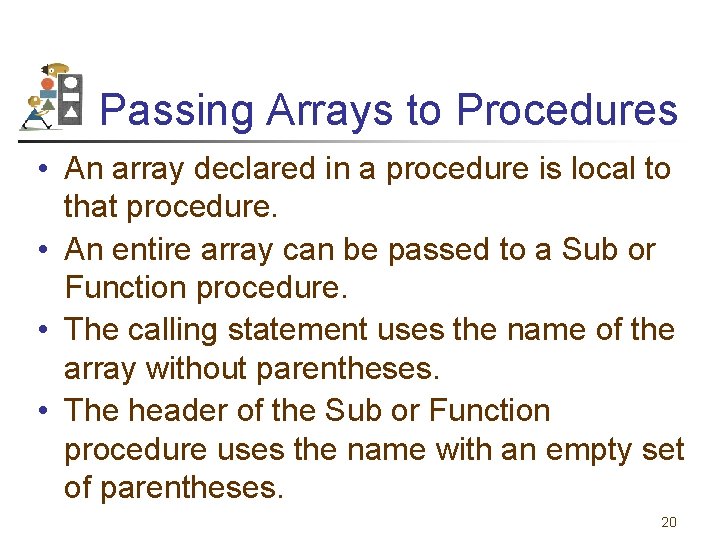
Passing Arrays to Procedures • An array declared in a procedure is local to that procedure. • An entire array can be passed to a Sub or Function procedure. • The calling statement uses the name of the array without parentheses. • The header of the Sub or Function procedure uses the name with an empty set of parentheses. 20
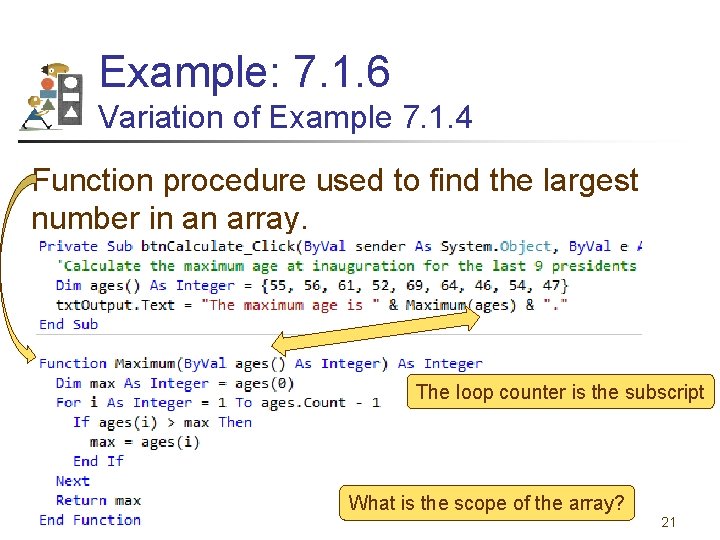
Example: 7. 1. 6 Variation of Example 7. 1. 4 Function procedure used to find the largest number in an array. The loop counter is the subscript What is the scope of the array? 21
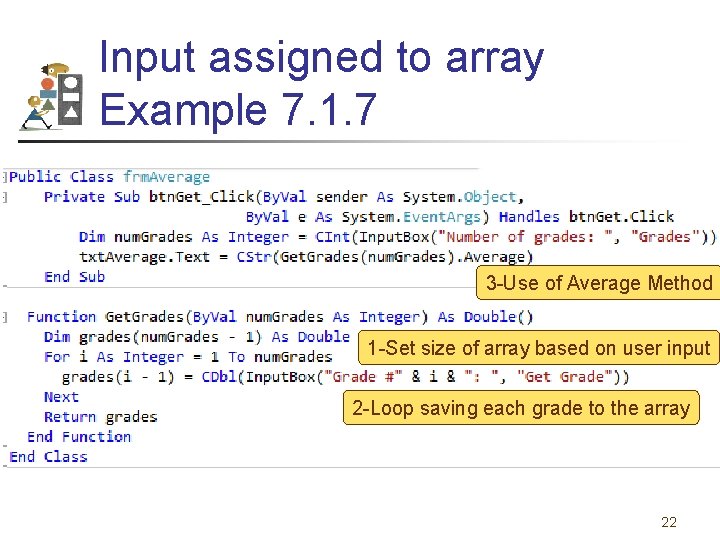
Input assigned to array Example 7. 1. 7 3 -Use of Average Method 1 -Set size of array based on user input 2 -Loop saving each grade to the array 22
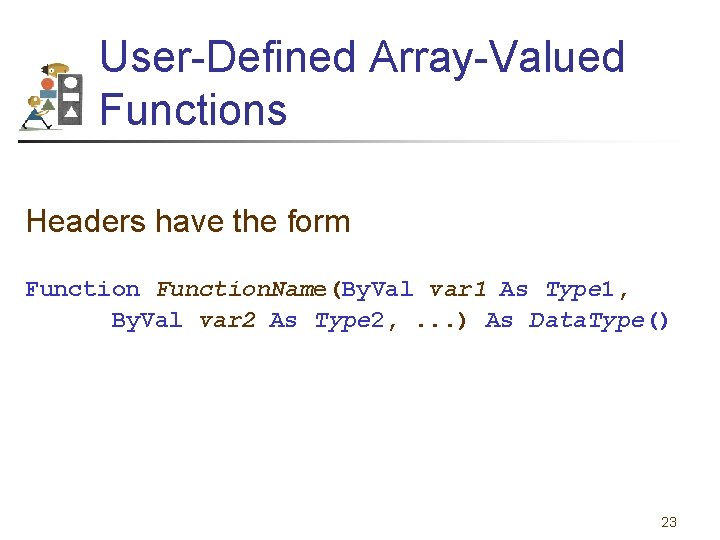
User-Defined Array-Valued Functions Headers have the form Function. Name(By. Val var 1 As Type 1, By. Val var 2 As Type 2, . . . ) As Data. Type() 23
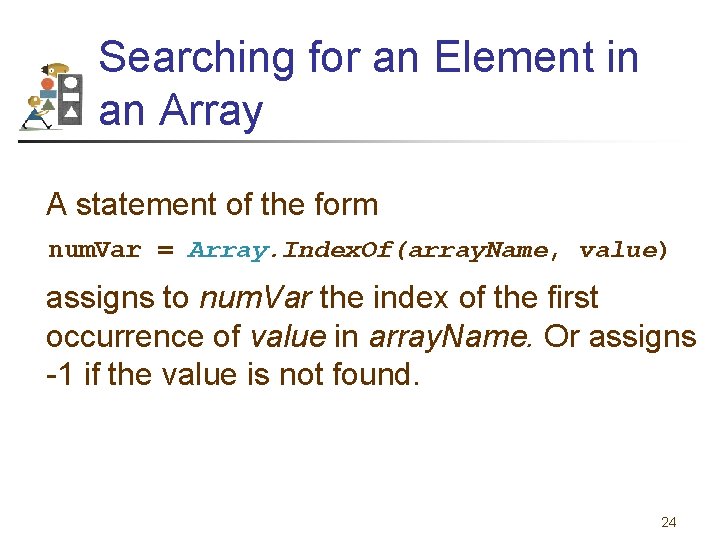
Searching for an Element in an Array A statement of the form num. Var = Array. Index. Of(array. Name, value) assigns to num. Var the index of the first occurrence of value in array. Name. Or assigns -1 if the value is not found. 24
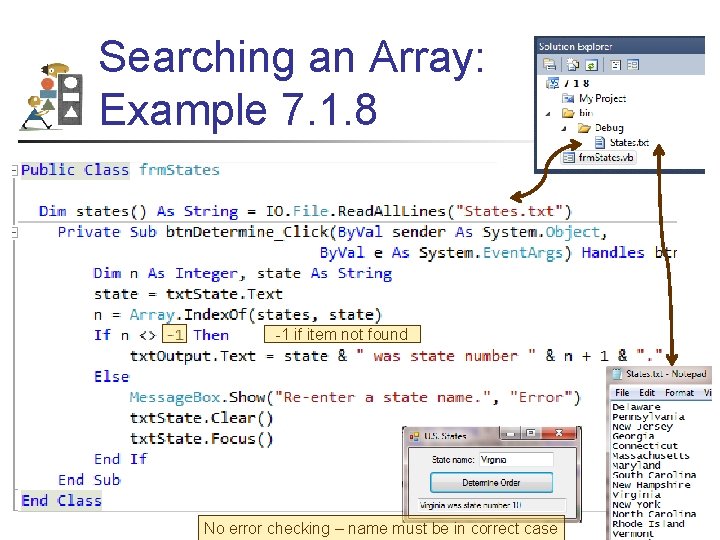
Searching an Array: Example 7. 1. 8 -1 if item not found No error checking – name must be in correct case 25
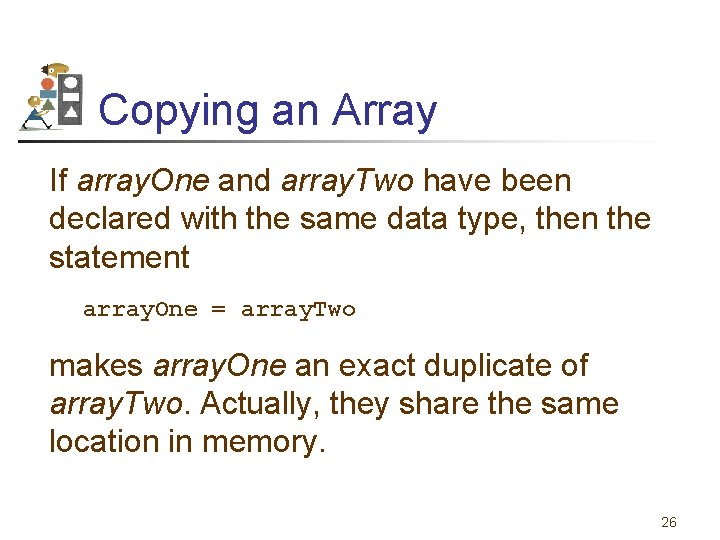
Copying an Array If array. One and array. Two have been declared with the same data type, then the statement array. One = array. Two makes array. One an exact duplicate of array. Two. Actually, they share the same location in memory. 26
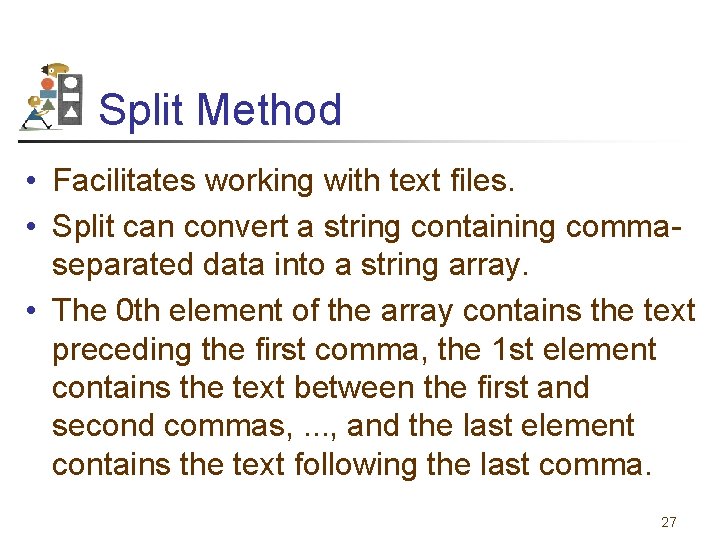
Split Method • Facilitates working with text files. • Split can convert a string containing commaseparated data into a string array. • The 0 th element of the array contains the text preceding the first comma, the 1 st element contains the text between the first and second commas, . . . , and the last element contains the text following the last comma. 27
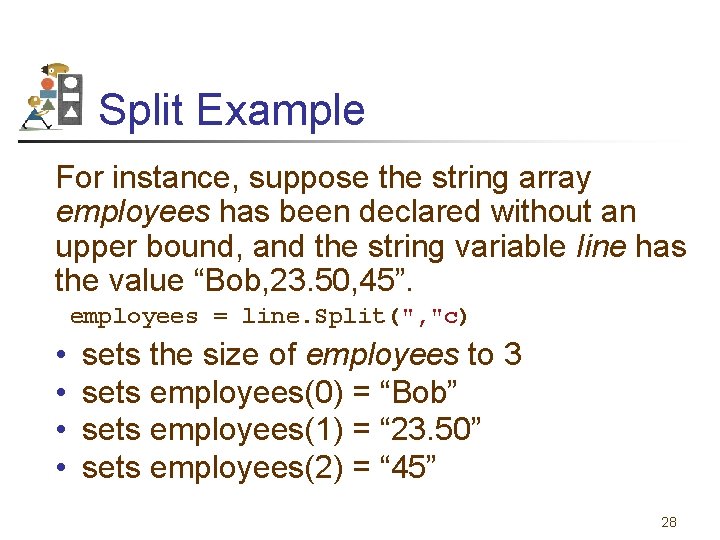
Split Example For instance, suppose the string array employees has been declared without an upper bound, and the string variable line has the value “Bob, 23. 50, 45”. employees = line. Split(", "c) • • sets the size of employees to 3 sets employees(0) = “Bob” sets employees(1) = “ 23. 50” sets employees(2) = “ 45” 28
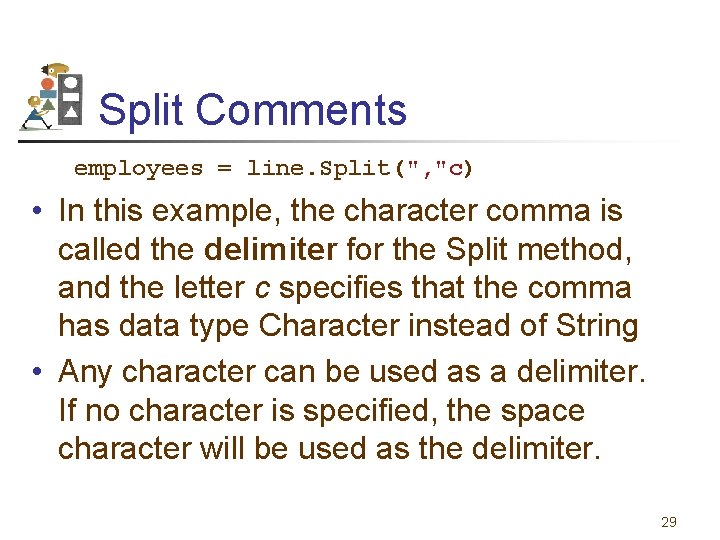
Split Comments employees = line. Split(", "c) • In this example, the character comma is called the delimiter for the Split method, and the letter c specifies that the comma has data type Character instead of String • Any character can be used as a delimiter. If no character is specified, the space character will be used as the delimiter. 29
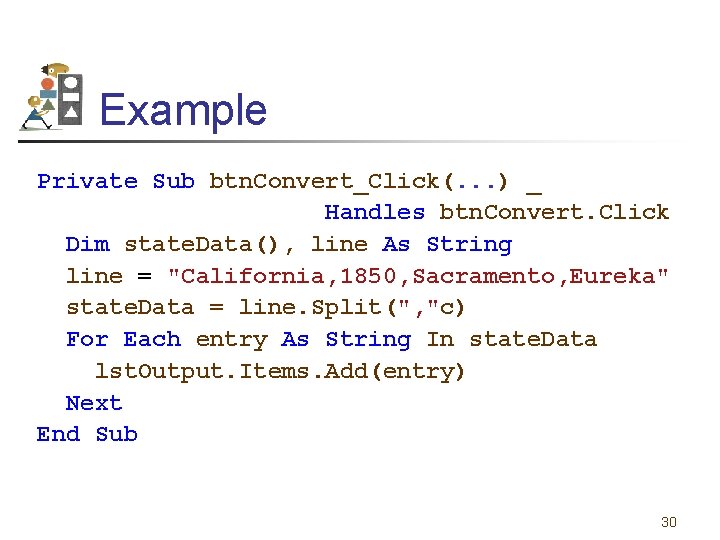
Example Private Sub btn. Convert_Click(. . . ) _ Handles btn. Convert. Click Dim state. Data(), line As String line = "California, 1850, Sacramento, Eureka" state. Data = line. Split(", "c) For Each entry As String In state. Data lst. Output. Items. Add(entry) Next End Sub 30
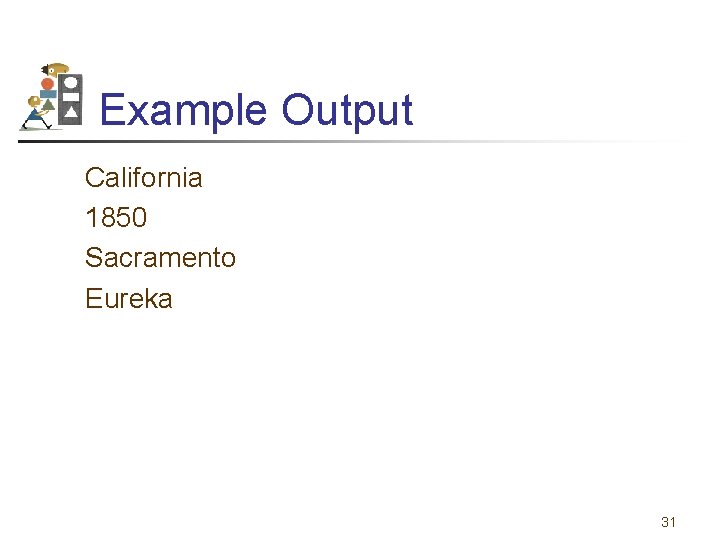
Example Output California 1850 Sacramento Eureka 31
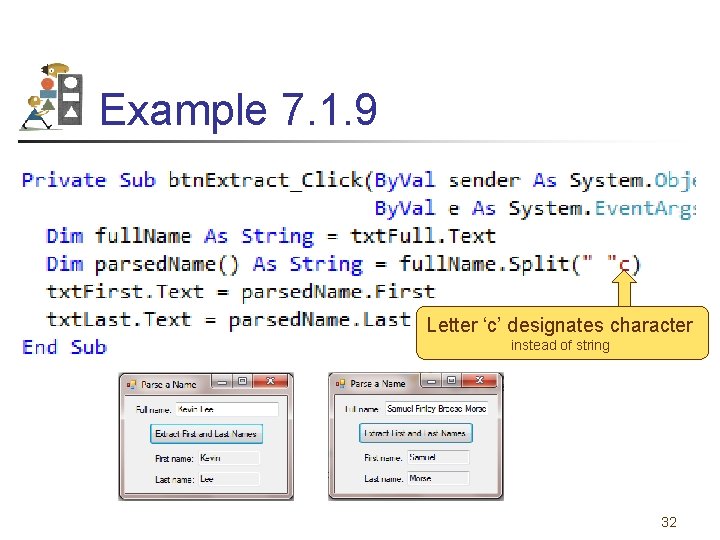
Example 7. 1. 9 Letter ‘c’ designates character instead of string 32
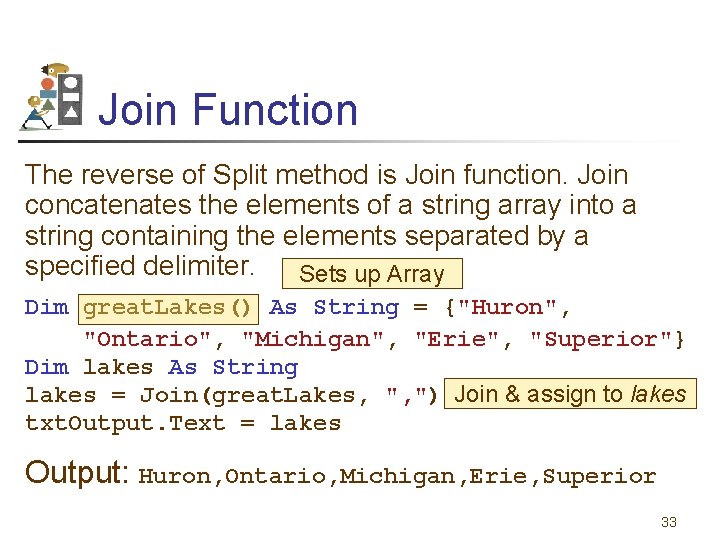
Join Function The reverse of Split method is Join function. Join concatenates the elements of a string array into a string containing the elements separated by a specified delimiter. Sets up Array Dim great. Lakes() As String = {"Huron", "Ontario", "Michigan", "Erie", "Superior"} Dim lakes As String lakes = Join(great. Lakes, ", ") Join & assign to lakes txt. Output. Text = lakes Output: Huron, Ontario, Michigan, Erie, Superior 33
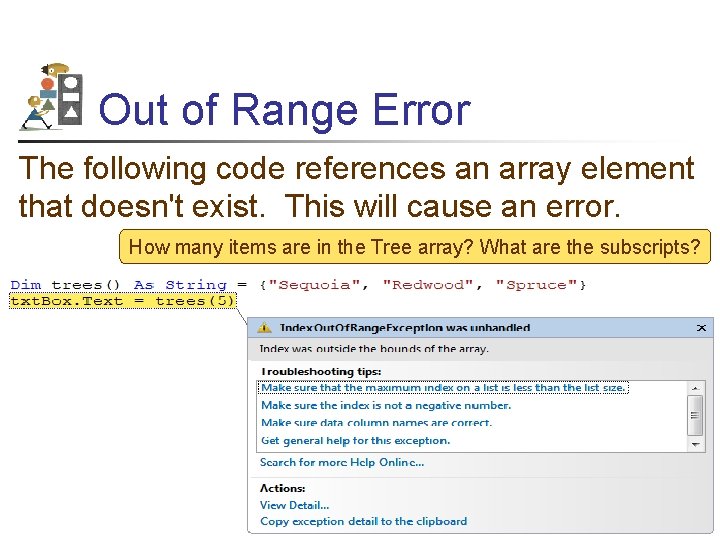
Out of Range Error The following code references an array element that doesn't exist. This will cause an error. How many items are in the Tree array? What are the subscripts? 34
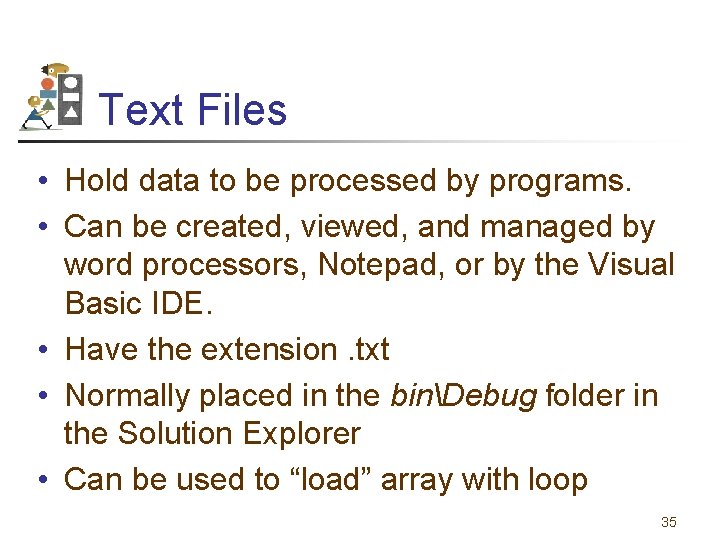
Text Files • Hold data to be processed by programs. • Can be created, viewed, and managed by word processors, Notepad, or by the Visual Basic IDE. • Have the extension. txt • Normally placed in the binDebug folder in the Solution Explorer • Can be used to “load” array with loop 35
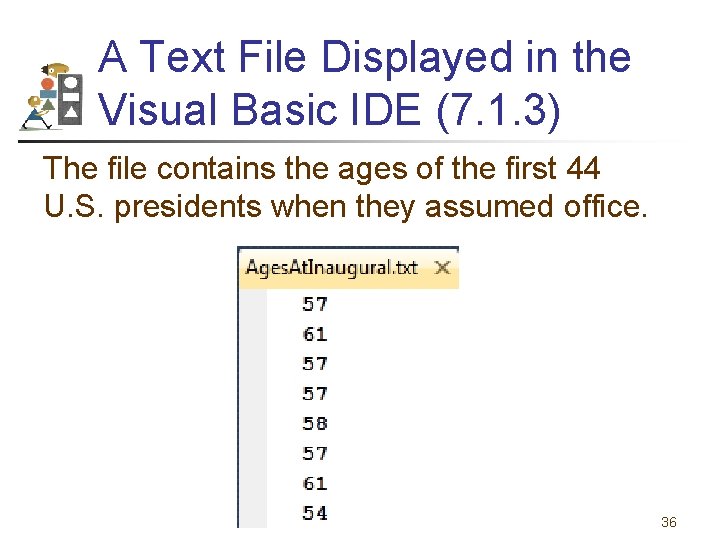
A Text File Displayed in the Visual Basic IDE (7. 1. 3) The file contains the ages of the first 44 U. S. presidents when they assumed office. 36
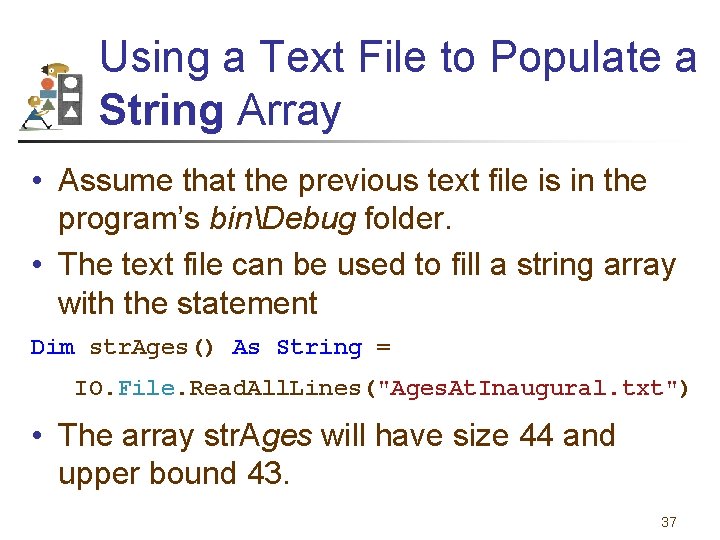
Using a Text File to Populate a String Array • Assume that the previous text file is in the program’s binDebug folder. • The text file can be used to fill a string array with the statement Dim str. Ages() As String = IO. File. Read. All. Lines("Ages. At. Inaugural. txt") • The array str. Ages will have size 44 and upper bound 43. 37
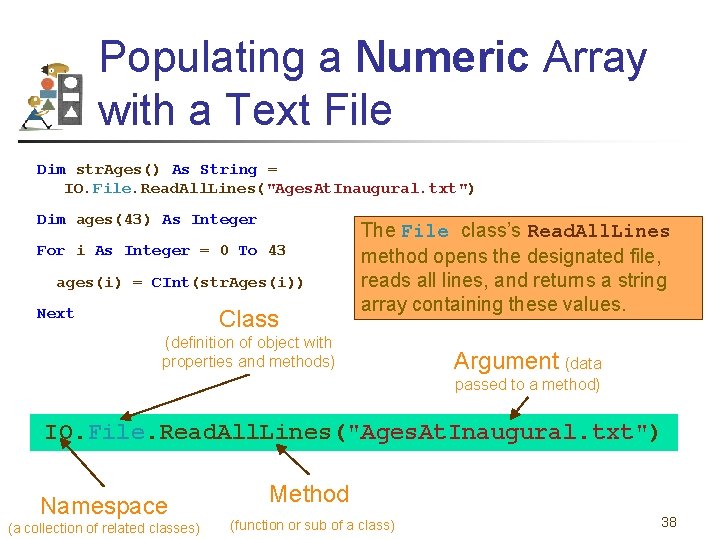
Populating a Numeric Array with a Text File Dim str. Ages() As String = IO. File. Read. All. Lines("Ages. At. Inaugural. txt") Dim ages(43) As Integer For i As Integer = 0 To 43 ages(i) = CInt(str. Ages(i)) Next Class The File class’s Read. All. Lines method opens the designated file, reads all lines, and returns a string array containing these values. (definition of object with properties and methods) Argument (data passed to a method) IO. File. Read. All. Lines("Ages. At. Inaugural. txt") Namespace (a collection of related classes) Method (function or sub of a class) 38
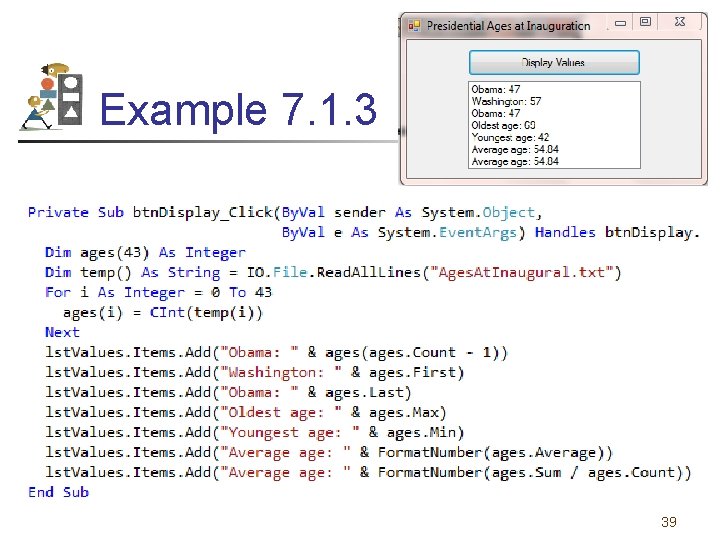
Example 7. 1. 3 39
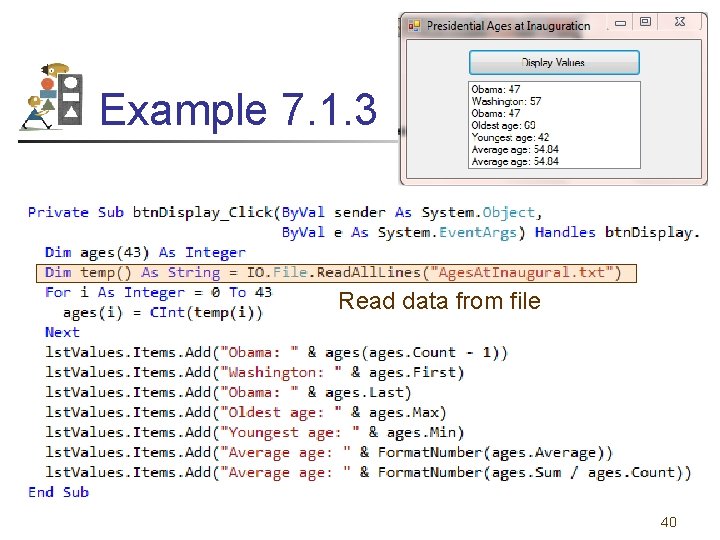
Example 7. 1. 3 Read data from file 40
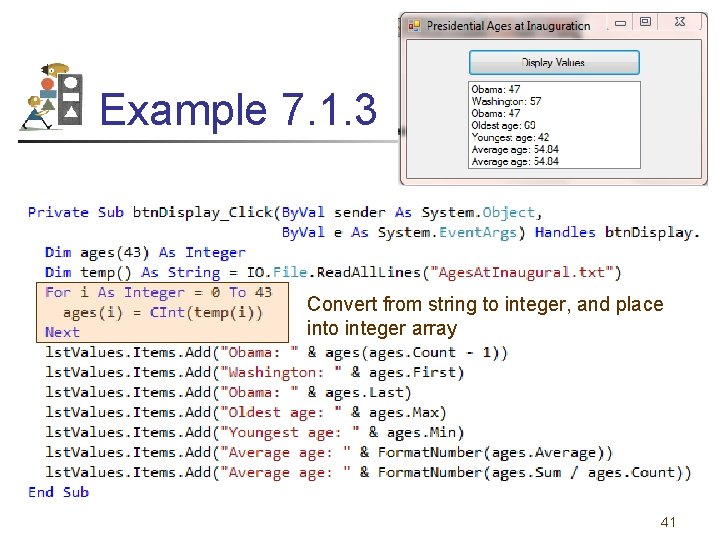
Example 7. 1. 3 Convert from string to integer, and place into integer array 41
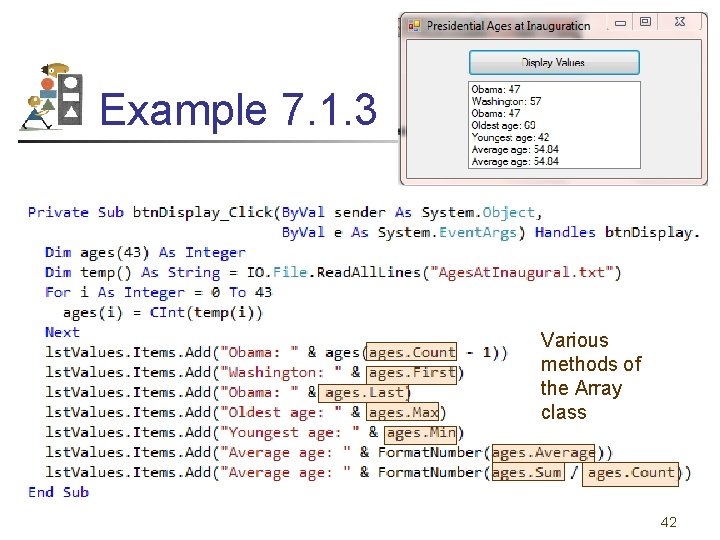
Example 7. 1. 3 Various methods of the Array class 42
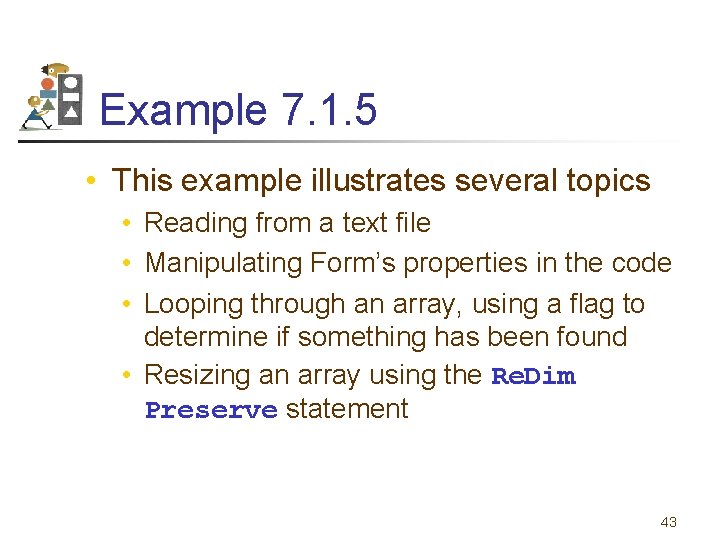
Example 7. 1. 5 • This example illustrates several topics • Reading from a text file • Manipulating Form’s properties in the code • Looping through an array, using a flag to determine if something has been found • Resizing an array using the Re. Dim Preserve statement 43
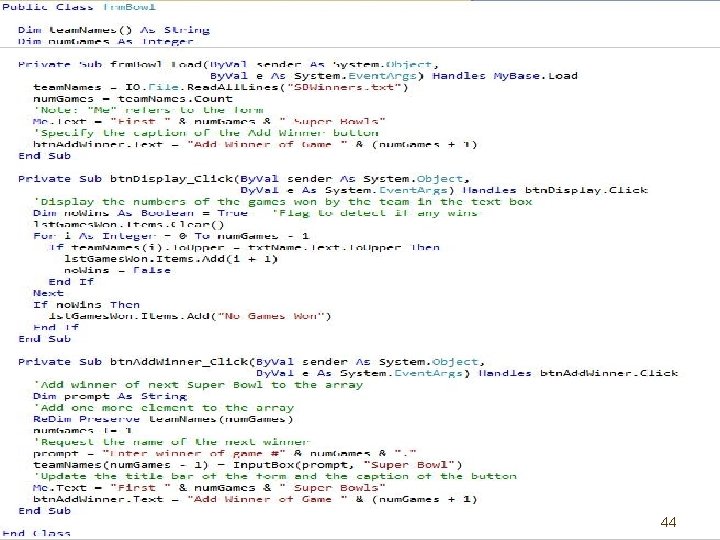
44
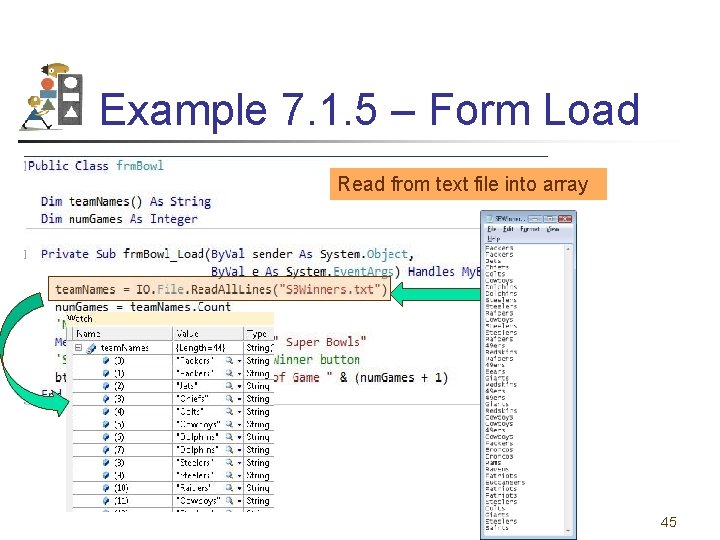
Example 7. 1. 5 – Form Load Read from text file into array 45
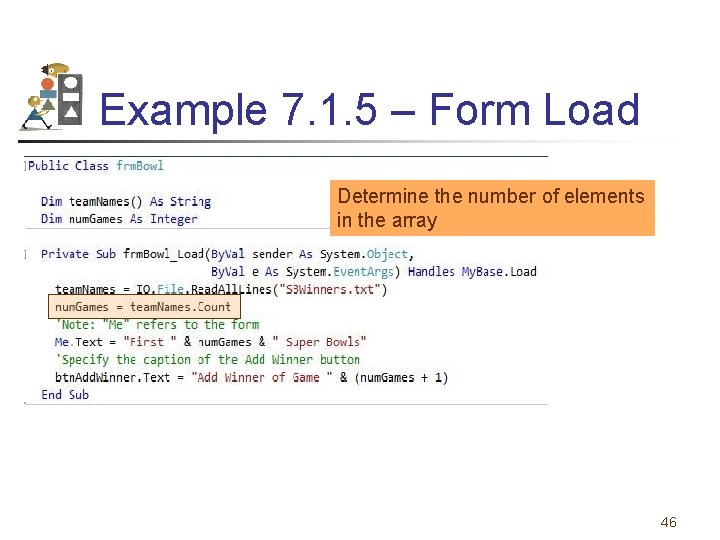
Example 7. 1. 5 – Form Load Determine the number of elements in the array 46
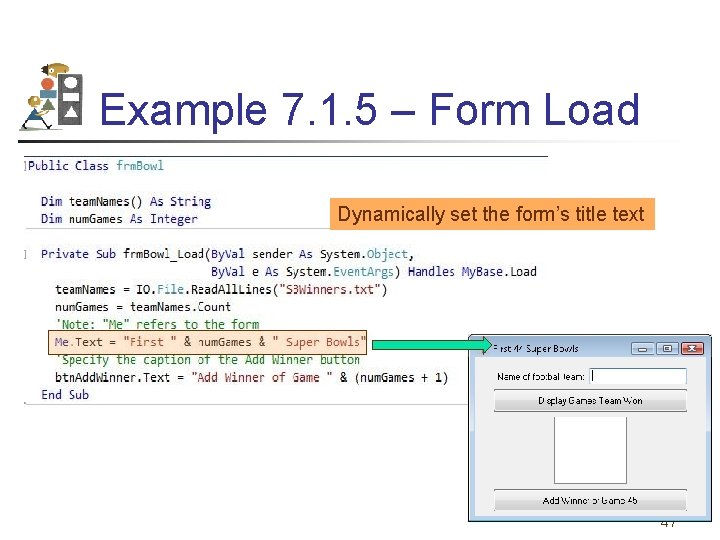
Example 7. 1. 5 – Form Load Dynamically set the form’s title text 47
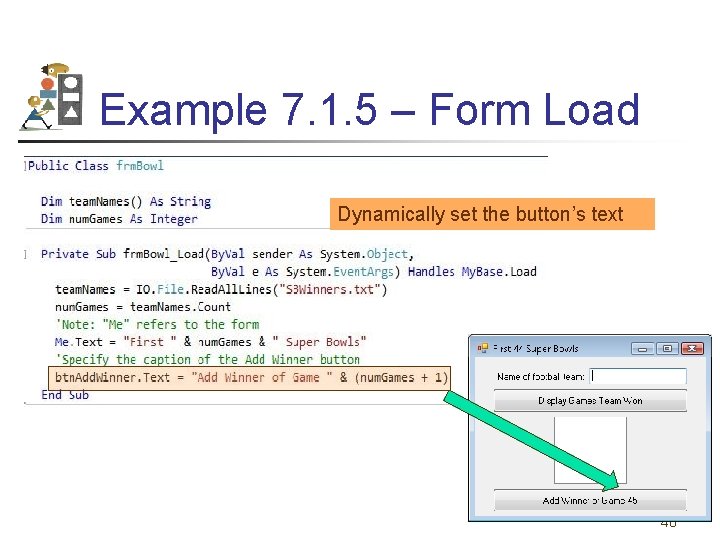
Example 7. 1. 5 – Form Load Dynamically set the button’s text 48
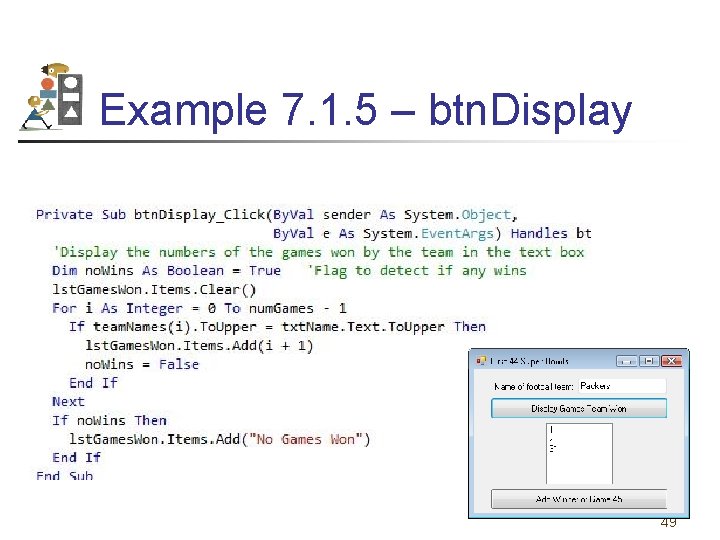
Example 7. 1. 5 – btn. Display 49
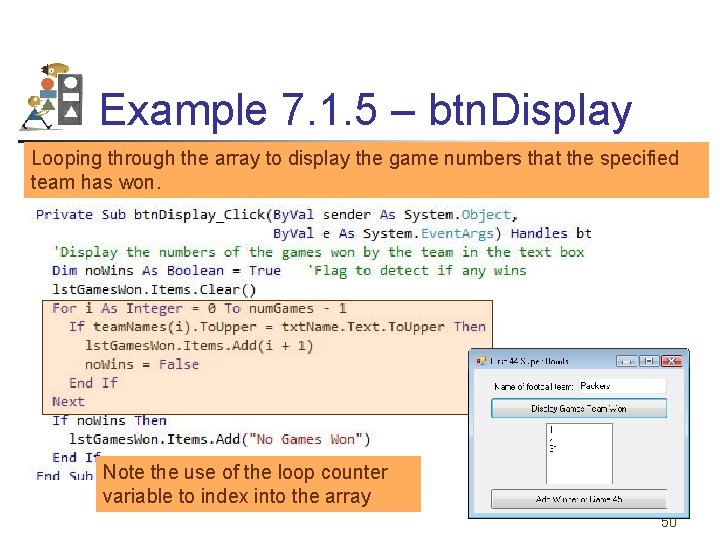
Example 7. 1. 5 – btn. Display Looping through the array to display the game numbers that the specified team has won. Note the use of the loop counter variable to index into the array 50
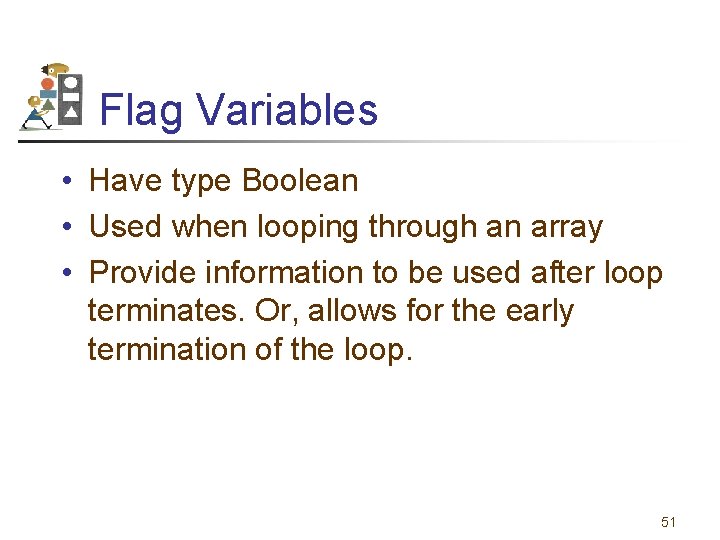
Flag Variables • Have type Boolean • Used when looping through an array • Provide information to be used after loop terminates. Or, allows for the early termination of the loop. 51
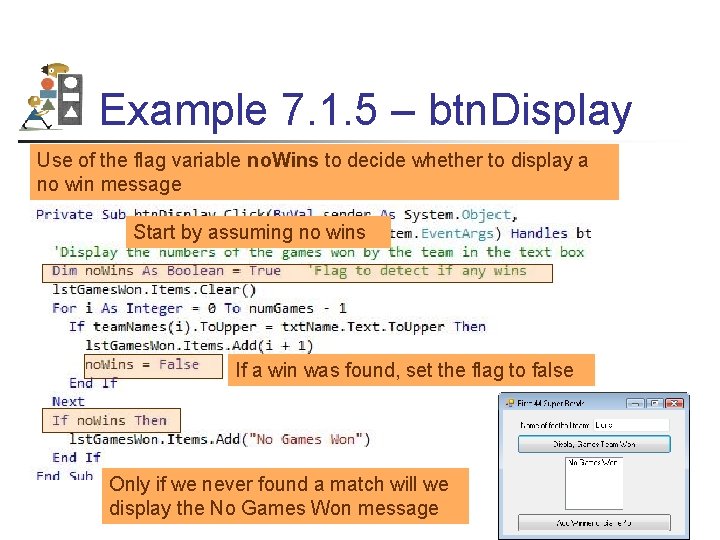
Example 7. 1. 5 – btn. Display Use of the flag variable no. Wins to decide whether to display a no win message Start by assuming no wins If a win was found, set the flag to false Only if we never found a match will we display the No Games Won message 52
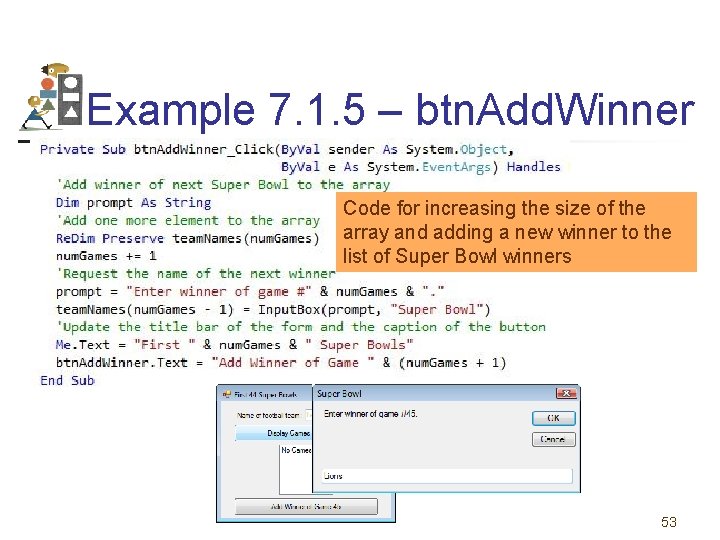
Example 7. 1. 5 – btn. Add. Winner Code for increasing the size of the array and adding a new winner to the list of Super Bowl winners 53
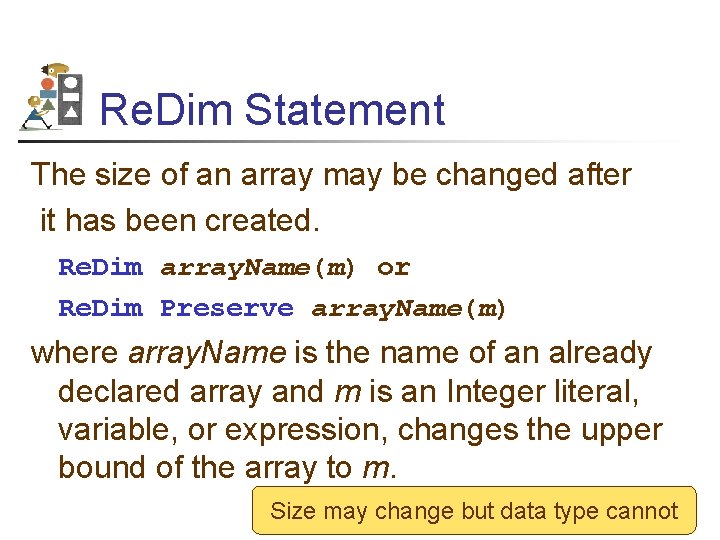
Re. Dim Statement The size of an array may be changed after it has been created. Re. Dim array. Name(m) or Re. Dim Preserve array. Name(m) where array. Name is the name of an already declared array and m is an Integer literal, variable, or expression, changes the upper bound of the array to m. Size may change but data type cannot 54
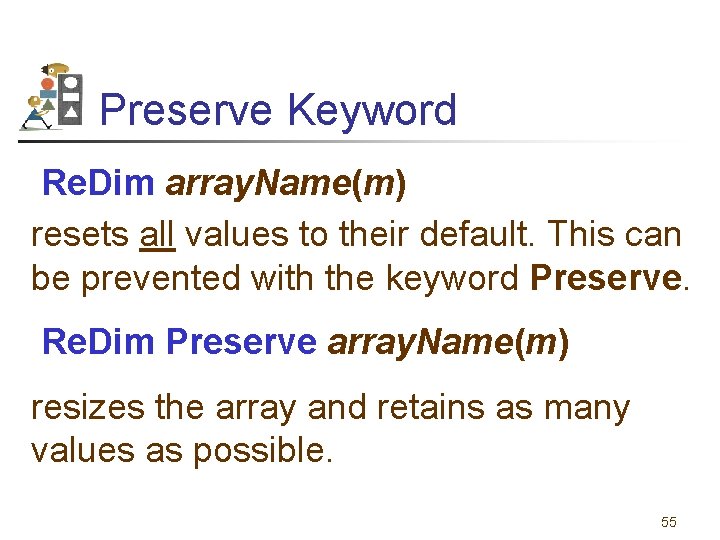
Preserve Keyword Re. Dim array. Name(m) resets all values to their default. This can be prevented with the keyword Preserve. Re. Dim Preserve array. Name(m) resizes the array and retains as many values as possible. 55
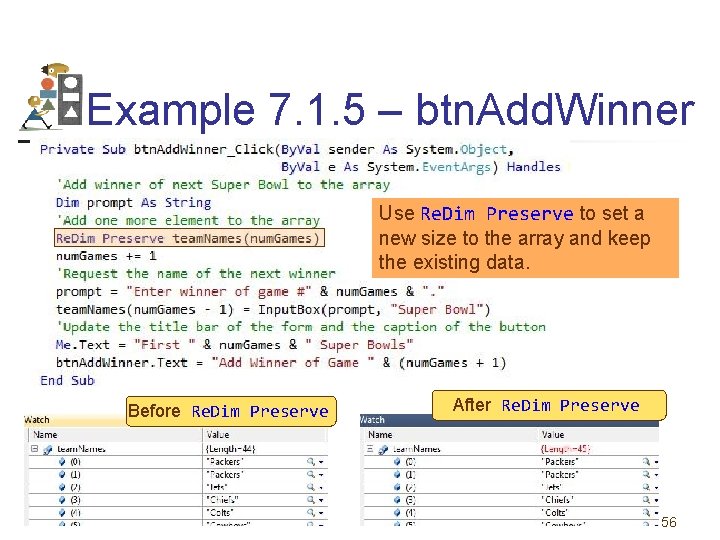
Example 7. 1. 5 – btn. Add. Winner Use Re. Dim Preserve to set a new size to the array and keep the existing data. Before Re. Dim Preserve After Re. Dim Preserve 56
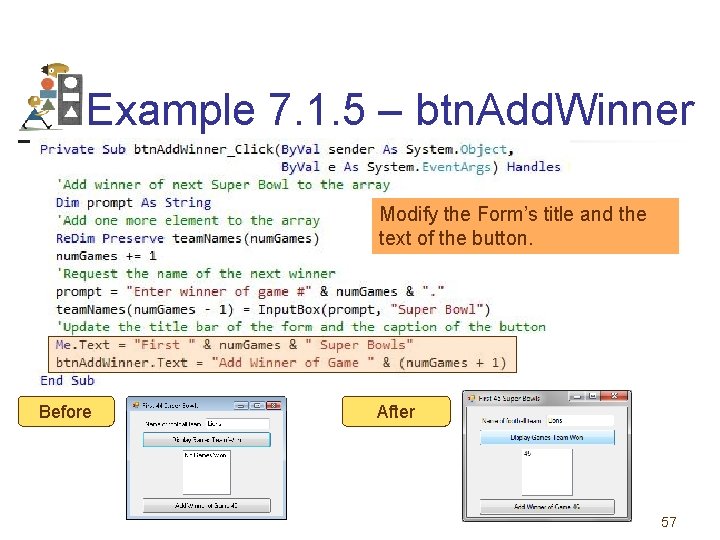
Example 7. 1. 5 – btn. Add. Winner Modify the Form’s title and the text of the button. Before After 57
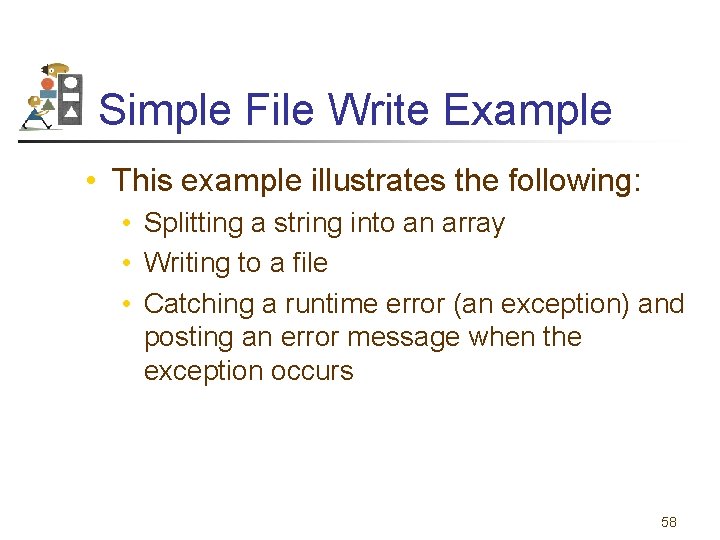
Simple File Write Example • This example illustrates the following: • Splitting a string into an array • Writing to a file • Catching a runtime error (an exception) and posting an error message when the exception occurs 58
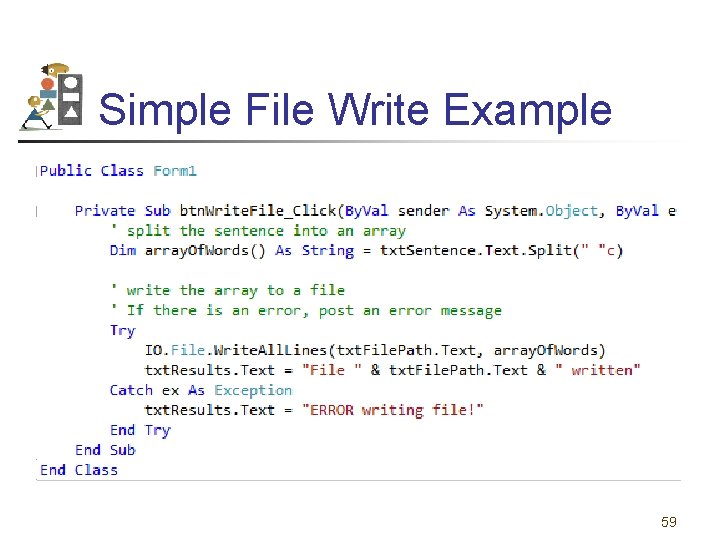
Simple File Write Example 59
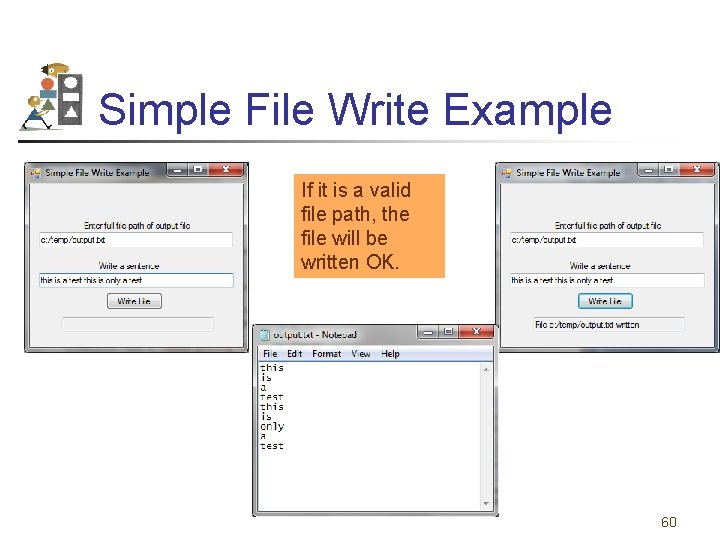
Simple File Write Example If it is a valid file path, the file will be written OK. 60
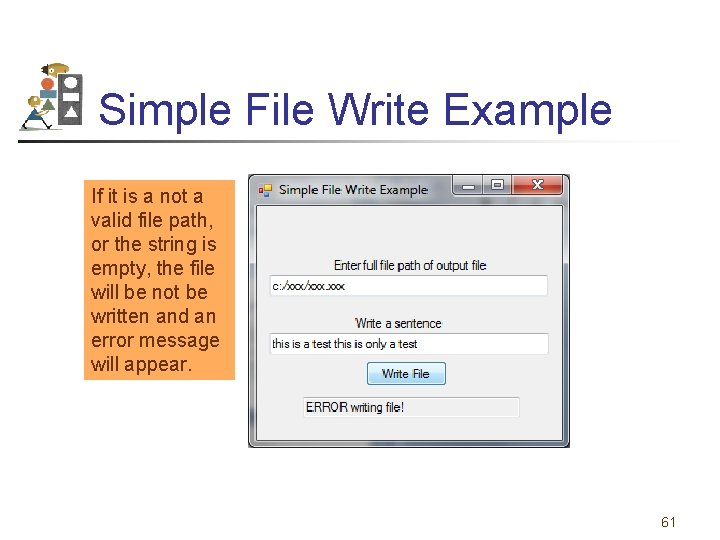
Simple File Write Example If it is a not a valid file path, or the string is empty, the file will be not be written and an error message will appear. 61
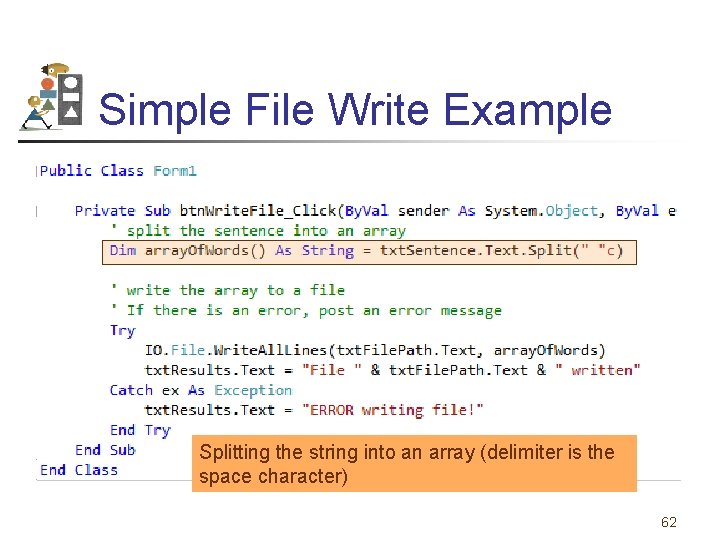
Simple File Write Example Splitting the string into an array (delimiter is the space character) 62
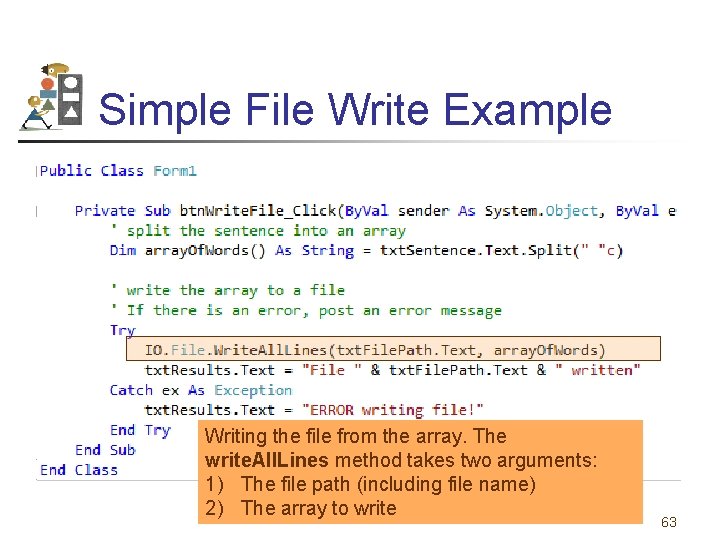
Simple File Write Example Writing the file from the array. The write. All. Lines method takes two arguments: 1) The file path (including file name) 2) The array to write 63
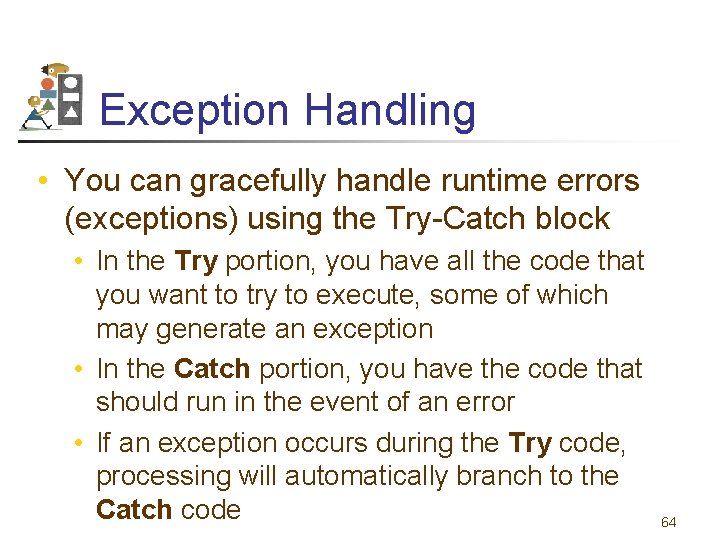
Exception Handling • You can gracefully handle runtime errors (exceptions) using the Try-Catch block • In the Try portion, you have all the code that you want to try to execute, some of which may generate an exception • In the Catch portion, you have the code that should run in the event of an error • If an exception occurs during the Try code, processing will automatically branch to the Catch code 64
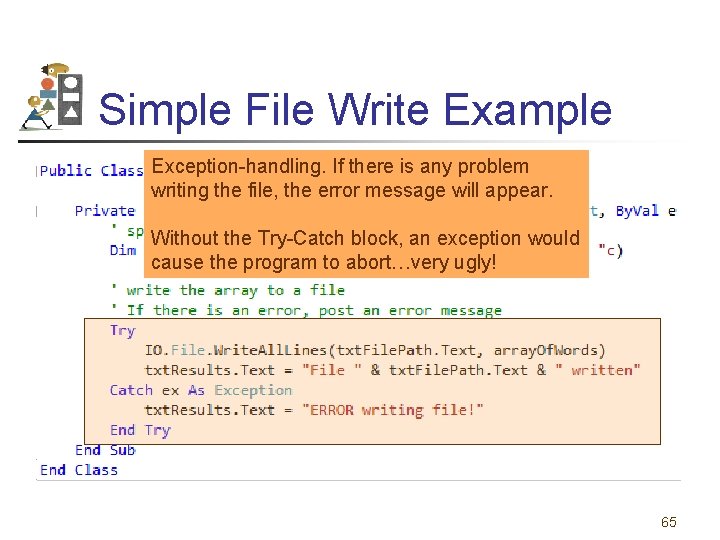
Simple File Write Example Exception-handling. If there is any problem writing the file, the error message will appear. Without the Try-Catch block, an exception would cause the program to abort…very ugly! 65
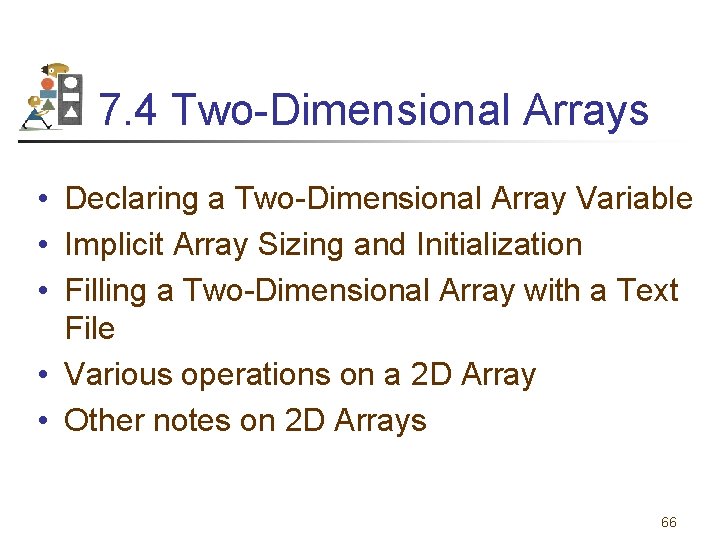
7. 4 Two-Dimensional Arrays • Declaring a Two-Dimensional Array Variable • Implicit Array Sizing and Initialization • Filling a Two-Dimensional Array with a Text File • Various operations on a 2 D Array • Other notes on 2 D Arrays 66
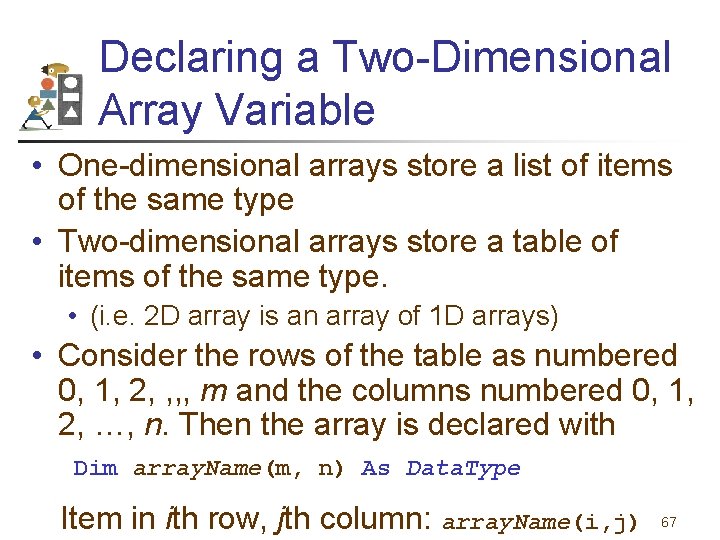
Declaring a Two-Dimensional Array Variable • One-dimensional arrays store a list of items of the same type • Two-dimensional arrays store a table of items of the same type. • (i. e. 2 D array is an array of 1 D arrays) • Consider the rows of the table as numbered 0, 1, 2, , m and the columns numbered 0, 1, 2, …, n. Then the array is declared with Dim array. Name(m, n) As Data. Type Item in ith row, jth column: array. Name(i, j) 67
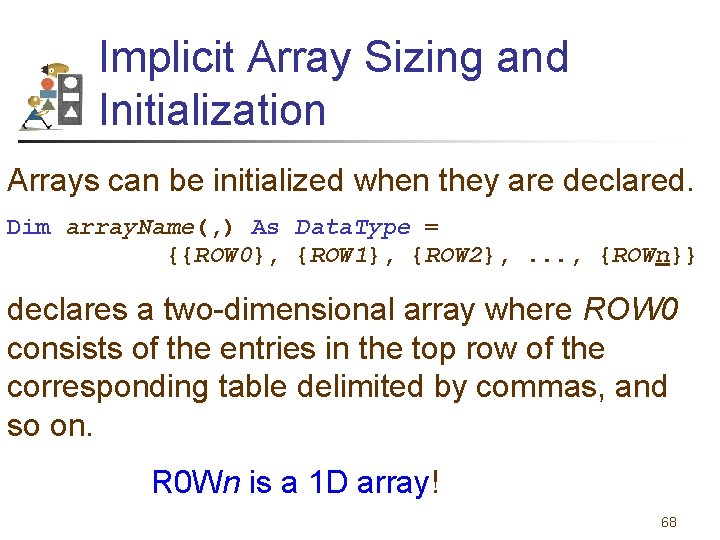
Implicit Array Sizing and Initialization Arrays can be initialized when they are declared. Dim array. Name(, ) As Data. Type = {{ROW 0}, {ROW 1}, {ROW 2}, . . . , {ROWn}} declares a two-dimensional array where ROW 0 consists of the entries in the top row of the corresponding table delimited by commas, and so on. R 0 Wn is a 1 D array! 68
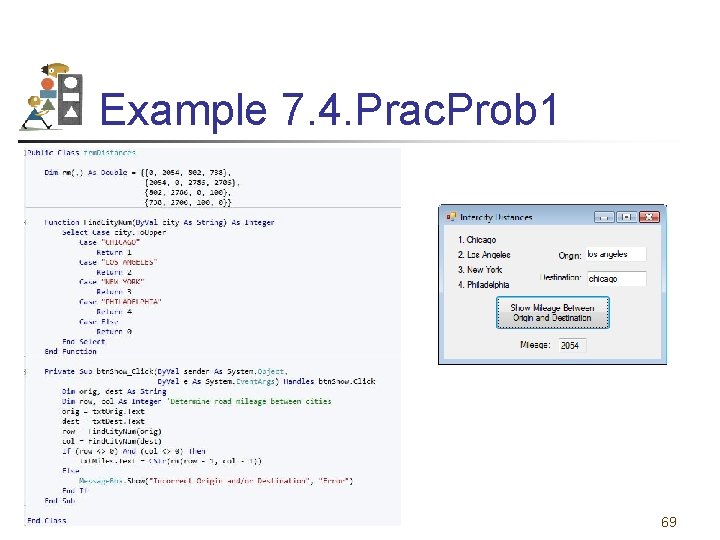
Example 7. 4. Prac. Prob 1 69
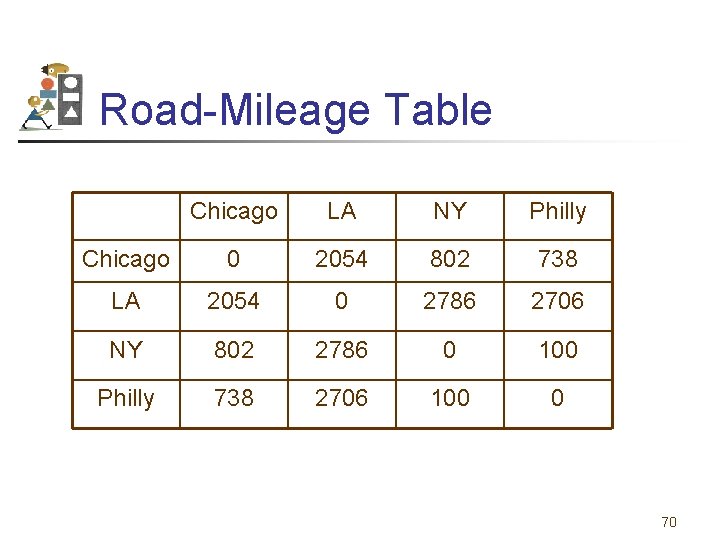
Road-Mileage Table Chicago LA NY Philly Chicago 0 2054 802 738 LA 2054 0 2786 2706 NY 802 2786 0 100 Philly 738 2706 100 0 70
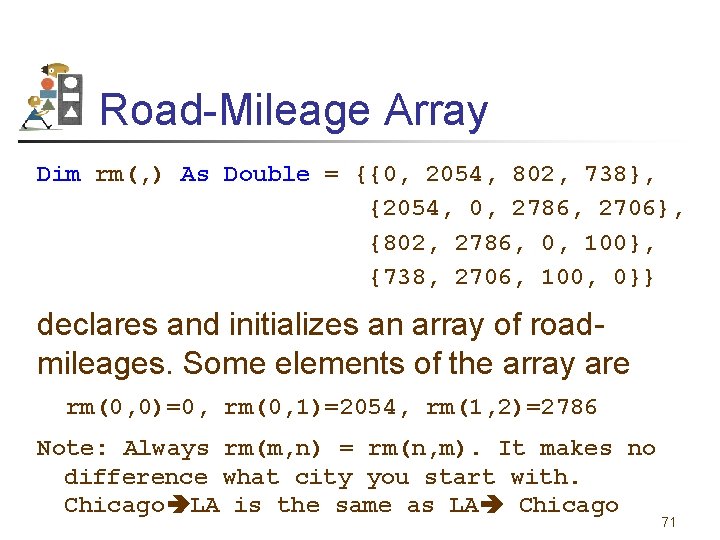
Road-Mileage Array Dim rm(, ) As Double = {{0, 2054, 802, 738}, {2054, 0, 2786, 2706}, {802, 2786, 0, 100}, {738, 2706, 100, 0}} declares and initializes an array of roadmileages. Some elements of the array are rm(0, 0)=0, rm(0, 1)=2054, rm(1, 2)=2786 Note: Always rm(m, n) = rm(n, m). It makes no difference what city you start with. Chicago LA is the same as LA Chicago 71
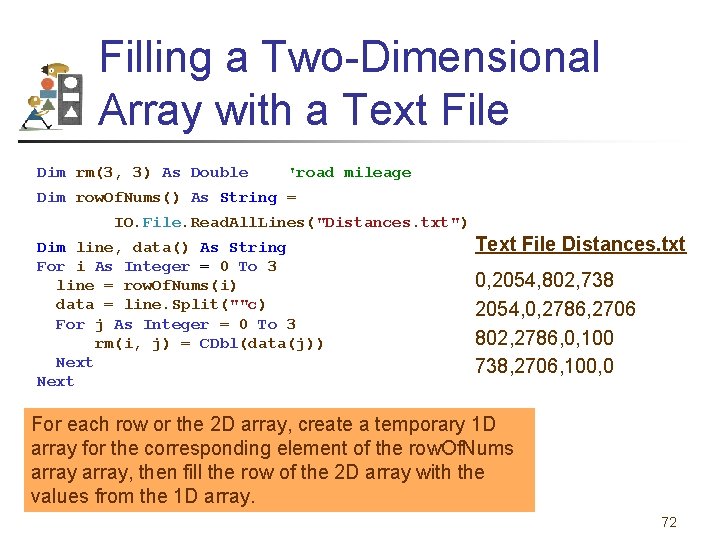
Filling a Two-Dimensional Array with a Text File Dim rm(3, 3) As Double 'road mileage Dim row. Of. Nums() As String = IO. File. Read. All. Lines("Distances. txt") Dim line, data() As String For i As Integer = 0 To 3 line = row. Of. Nums(i) data = line. Split(""c) For j As Integer = 0 To 3 rm(i, j) = CDbl(data(j)) Next Text File Distances. txt 0, 2054, 802, 738 2054, 0, 2786, 2706 802, 2786, 0, 100 738, 2706, 100, 0 For each row or the 2 D array, create a temporary 1 D array for the corresponding element of the row. Of. Nums array, then fill the row of the 2 D array with the values from the 1 D array. 72
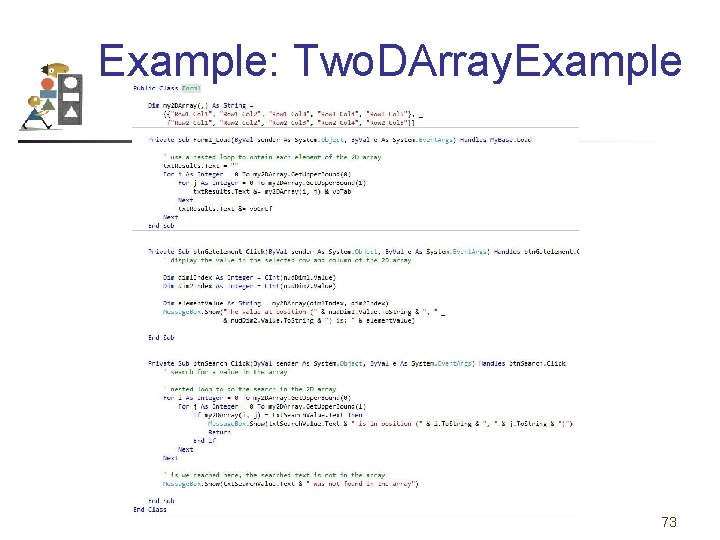
Example: Two. DArray. Example 73
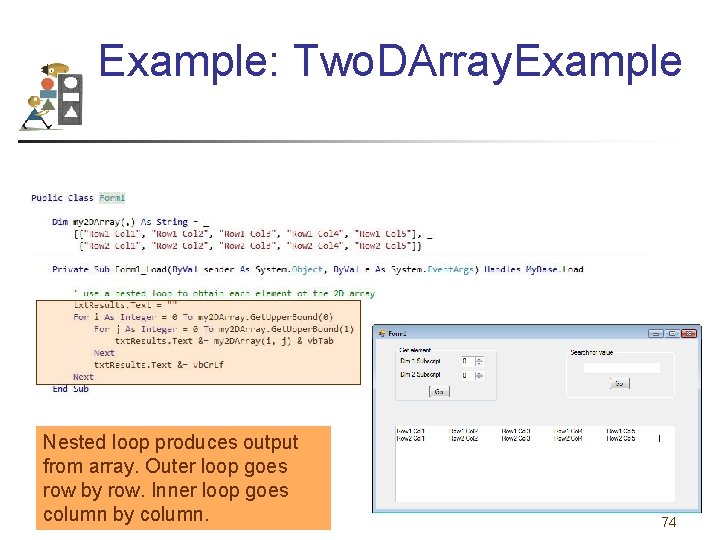
Example: Two. DArray. Example Nested loop produces output from array. Outer loop goes row by row. Inner loop goes column by column. 74
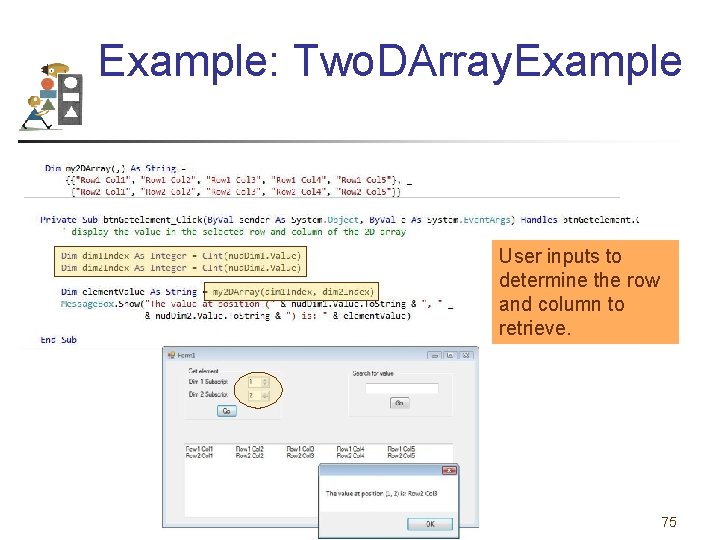
Example: Two. DArray. Example User inputs to determine the row and column to retrieve. 75
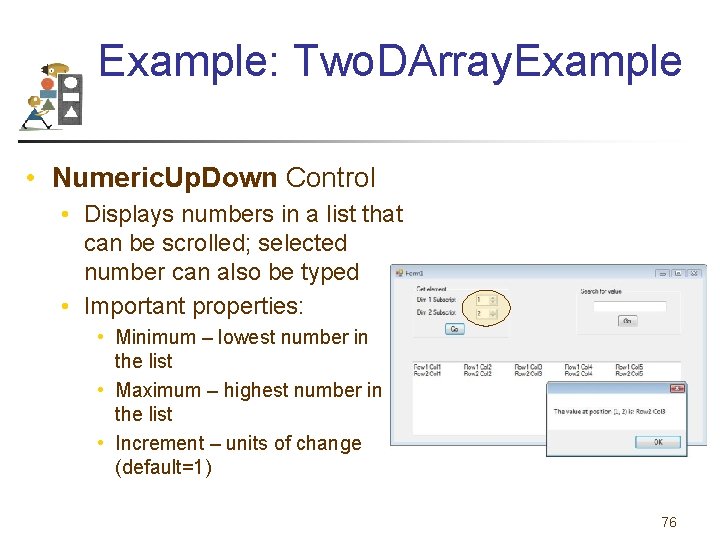
Example: Two. DArray. Example • Numeric. Up. Down Control • Displays numbers in a list that can be scrolled; selected number can also be typed • Important properties: • Minimum – lowest number in the list • Maximum – highest number in the list • Increment – units of change (default=1) 76
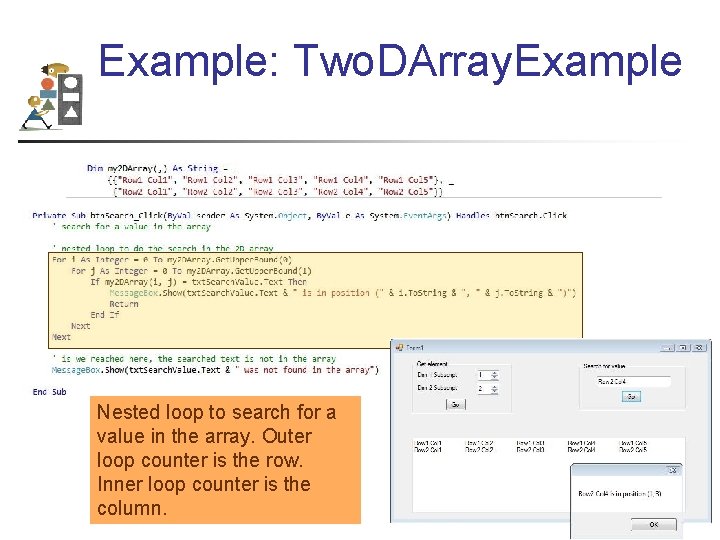
Example: Two. DArray. Example Nested loop to search for a value in the array. Outer loop counter is the row. Inner loop counter is the column. 77
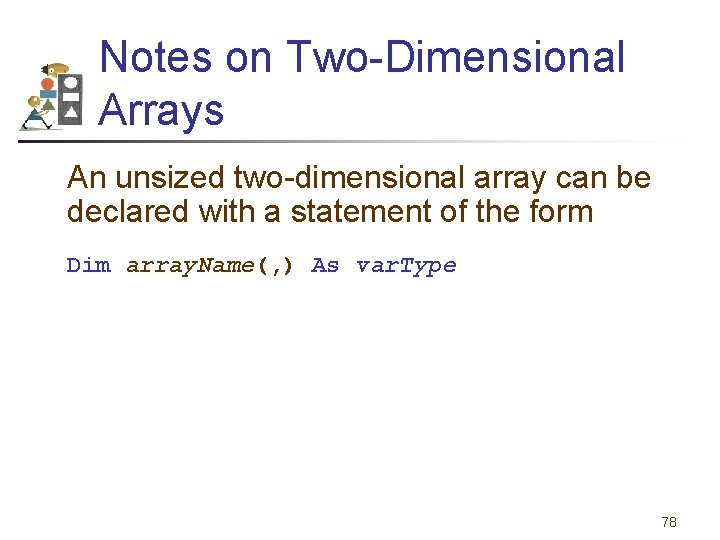
Notes on Two-Dimensional Arrays An unsized two-dimensional array can be declared with a statement of the form Dim array. Name(, ) As var. Type 78
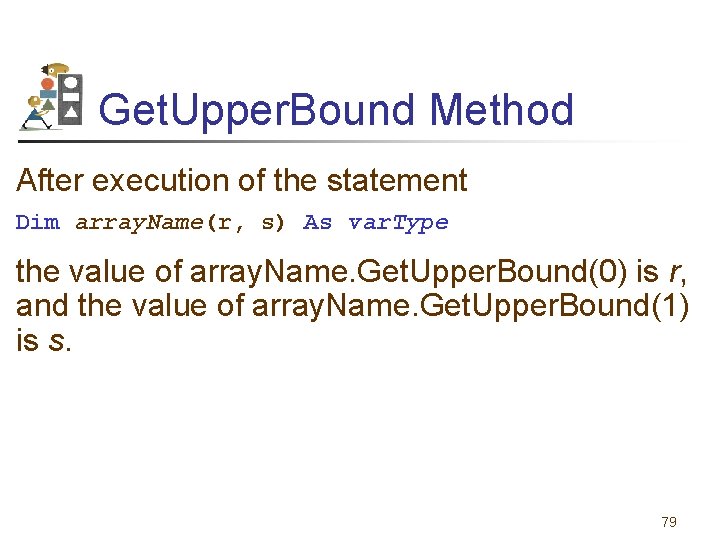
Get. Upper. Bound Method After execution of the statement Dim array. Name(r, s) As var. Type the value of array. Name. Get. Upper. Bound(0) is r, and the value of array. Name. Get. Upper. Bound(1) is s. 79
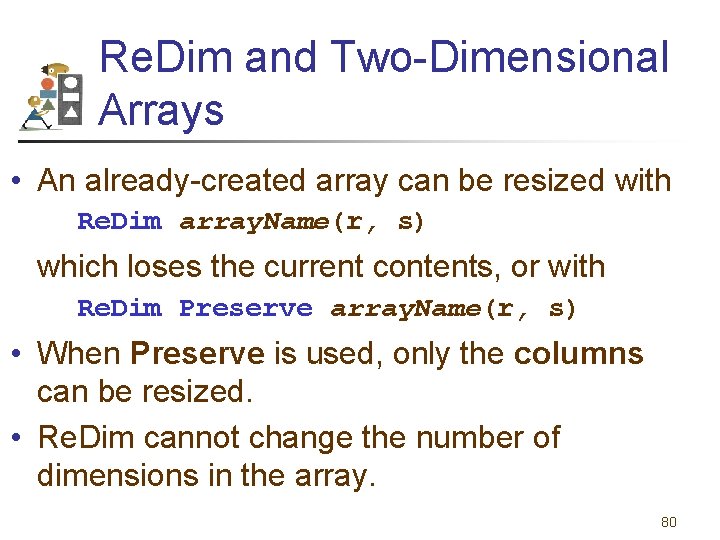
Re. Dim and Two-Dimensional Arrays • An already-created array can be resized with Re. Dim array. Name(r, s) which loses the current contents, or with Re. Dim Preserve array. Name(r, s) • When Preserve is used, only the columns can be resized. • Re. Dim cannot change the number of dimensions in the array. 80
Creating arrays matlab
Downloading and accessing
Accessing i/o devices
Accessing io devices in computer organization
Accessing mainframe data from java
Flipping bits in memory without accessing them
Features of a good distributed file system
Accessing input output devices
Accessing complex texts
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
Disadvantages of random access memory
Parallel arrays
Array of arrays c++
Java array operations
Partially filled arrays
C++ parallel arrays
Why do we need arrays?
Arreglos unidimensionales en java ejemplos
Arrays bidimensionales java
Mips array example
Polynomial representation using array in c
Array of strings assembly
Global arrays in c
Computer science arrays
Arrays visual basic
Python parallel arrays
How many arrays in 24
Arrays in pascal examples
Mips arrays
Arrays as adt
Partially filled array java
Redundancy array of independent disk
Python list of arrays
Arrays
Day 3: arrays
Redundant array of inexpensive disks
Microsoft small basic
Dynamic p table
Microled arrays
Are vectors dynamic arrays
Facts about arrays
Value creation strategy
Chapter 18 creating competitive advantage
Creating the constitution answer key chapter 2 section 4
Lesson 4 creating the constitution answer key
Chapter 18 creating competitive advantage
Tci chapter 6 answers
Chapter 6 creating a nation
Chapter 6 creating a nation
Chapter 18 creating competitive advantage
Chapter 8 creating the constitution
How to understand graphs and charts
Creating production possibilities schedules and curves
Creating customer value and engagement
Porters 3 generic strategy
Creating customer value satisfaction and loyalty
Creating customer value satisfaction and loyalty
Inner defender and inner guide examples
Creating and starting the venture
Creating vision and strategic direction
Mari carlos and amanda collect stamps
Creating and capturing customer value
Marketing concept
Creating customer value satisfaction and loyalty
Excel module 1: creating a worksheet and a chart
5 core customer and marketplace concepts
Interpreting distance time graphs
Graphing speed
The american people creating a nation and a society
Capturing value from customers
William blake elohim creating adam
Creating a dinosaur sculpture
Rubric characteristics
Yas spread to benchmark
Op art 1960
How to build a cloud center of excellence gartner
Creating reports in access
Creating brand equity kotler
Shark dichotomous key answers
Creating extraordinary teams