Chapter 6 Console InputOutput Types Console IO Introduction
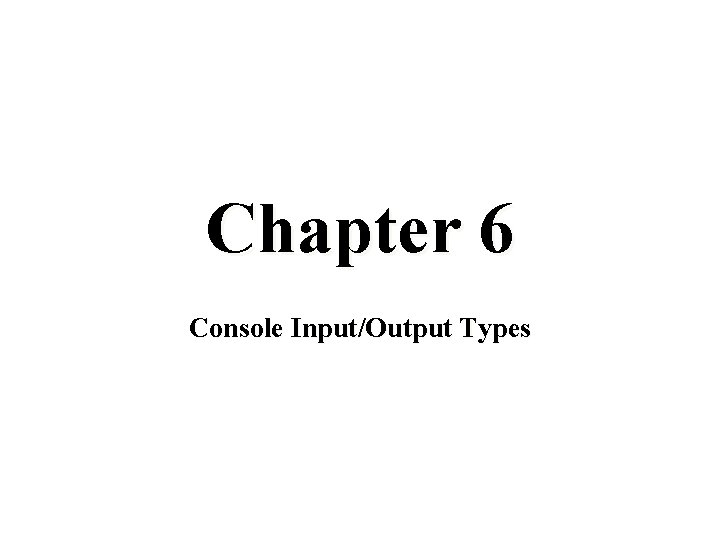
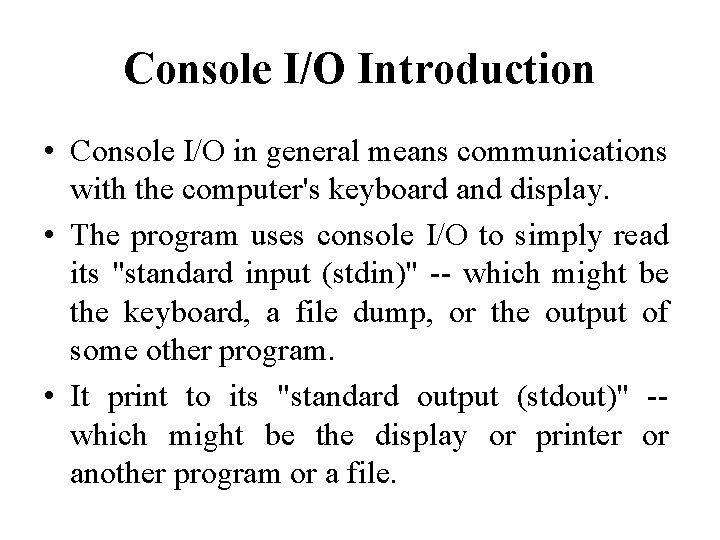
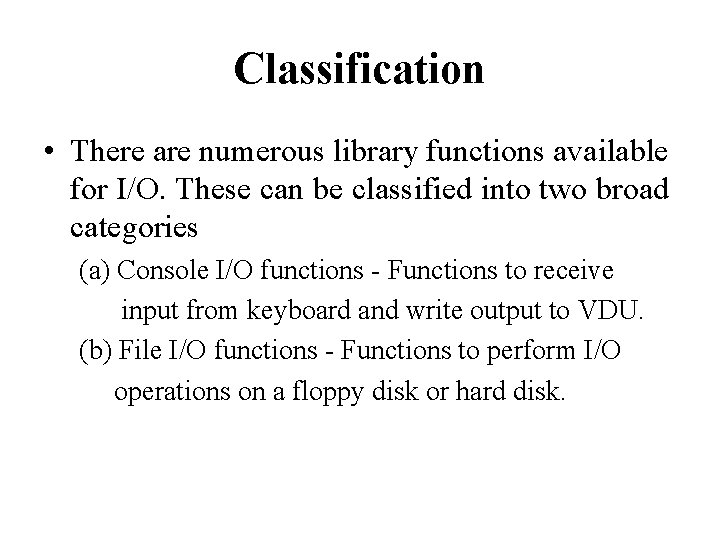
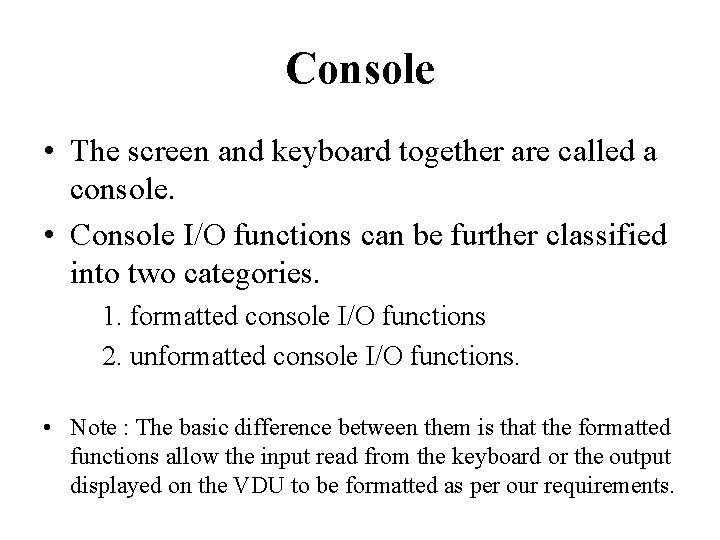
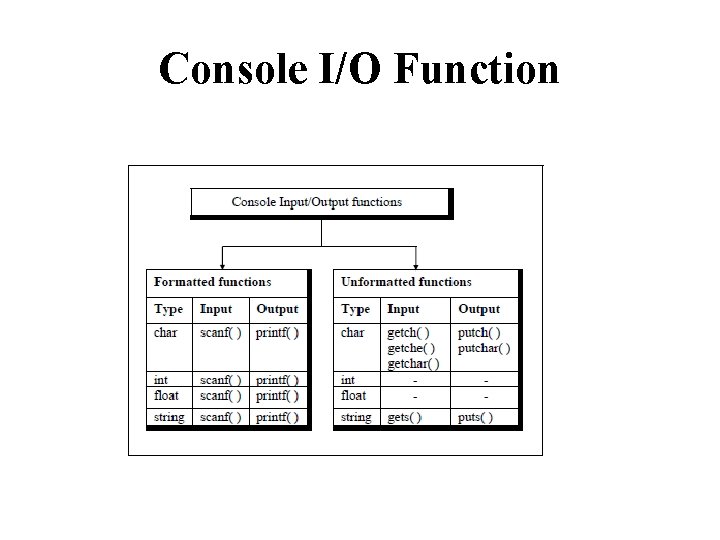
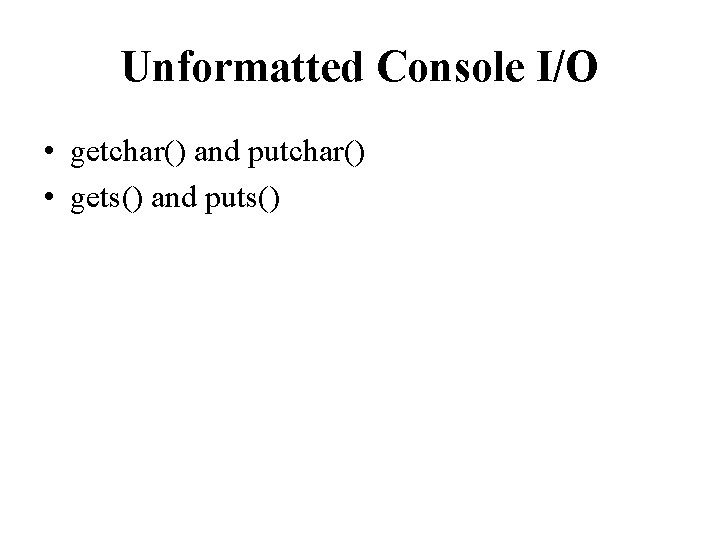
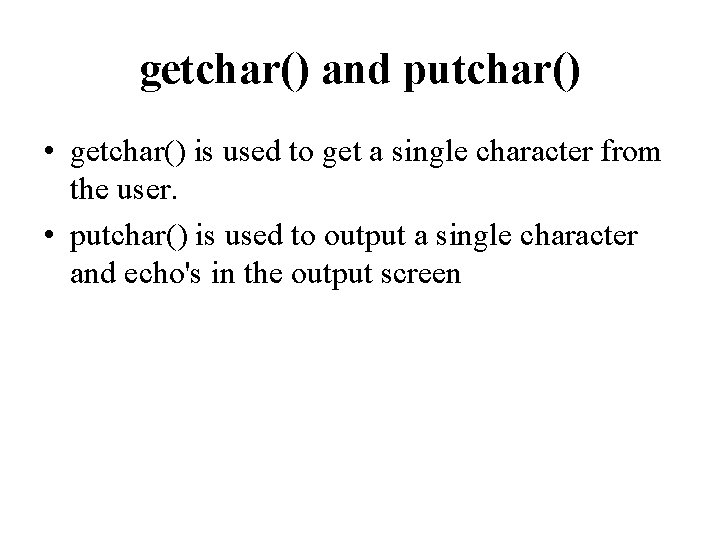
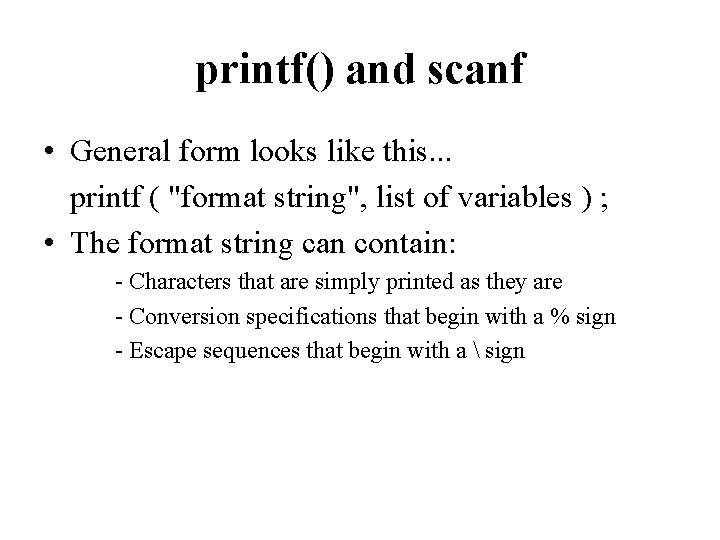
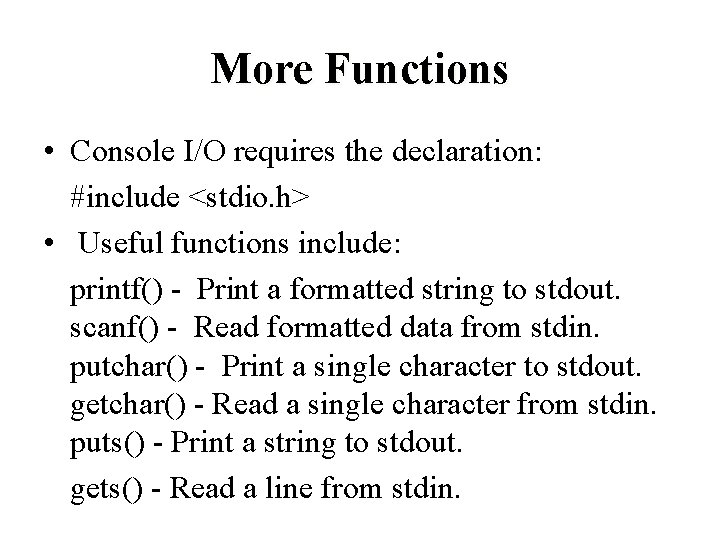
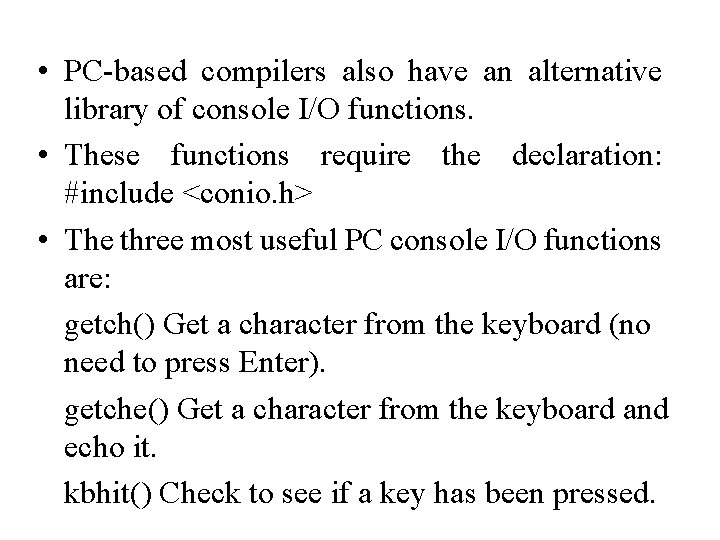
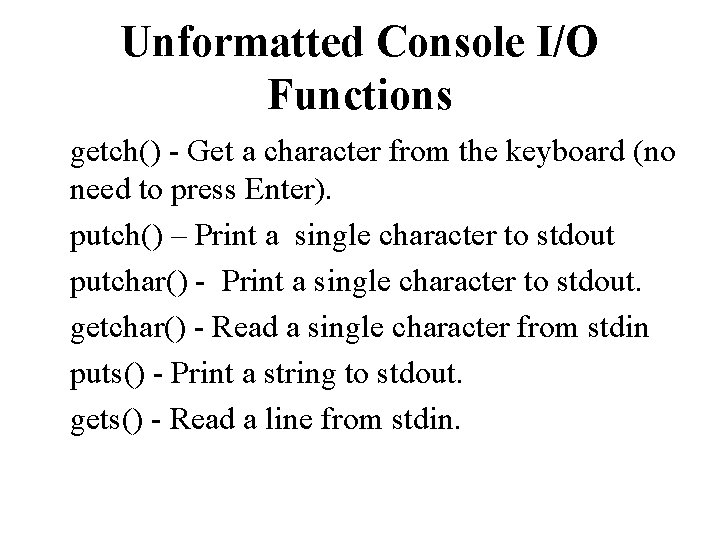
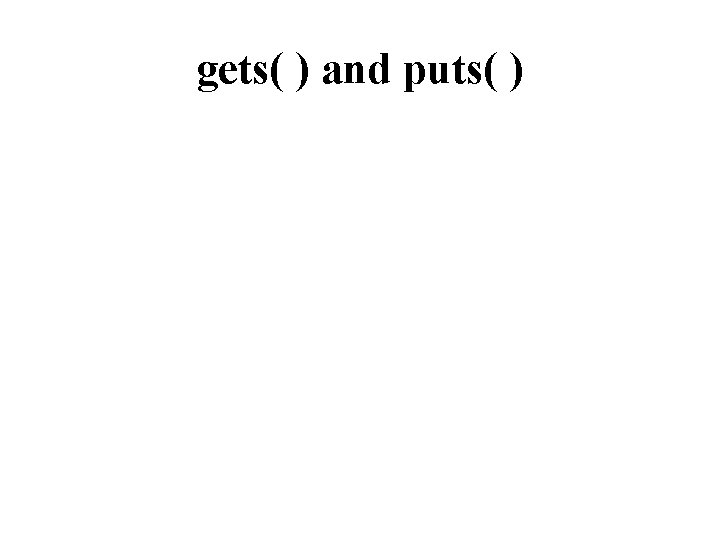
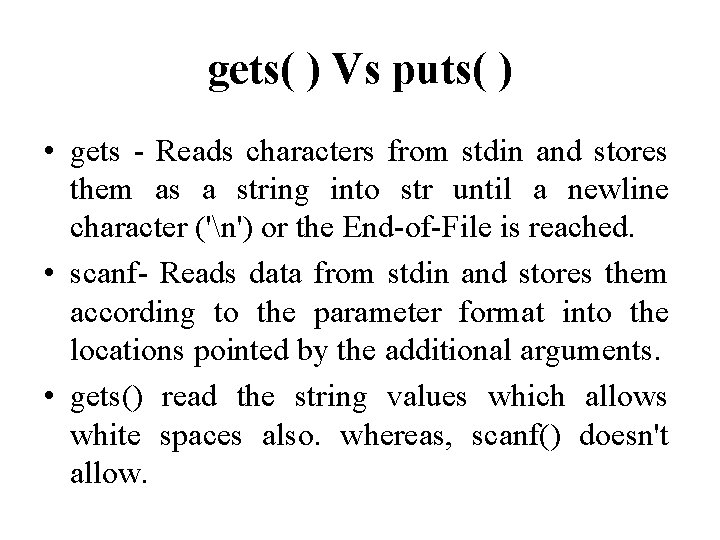
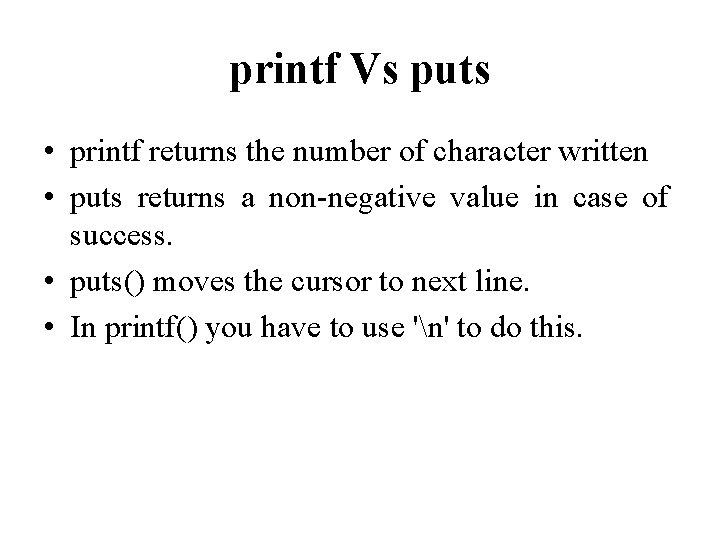
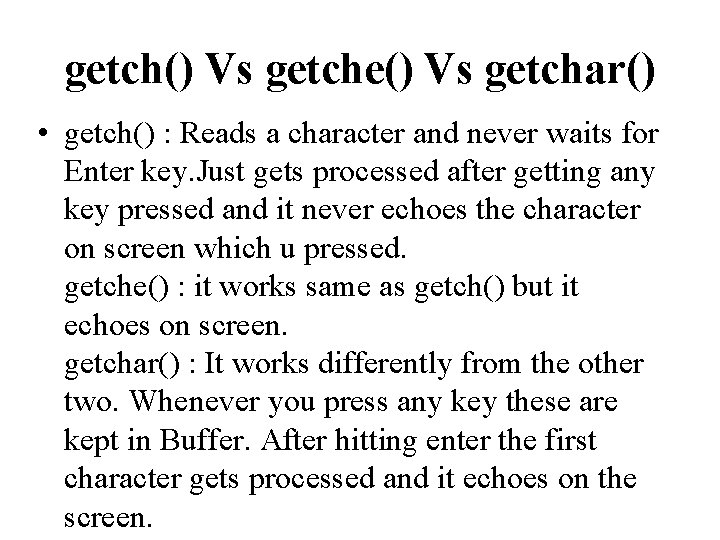
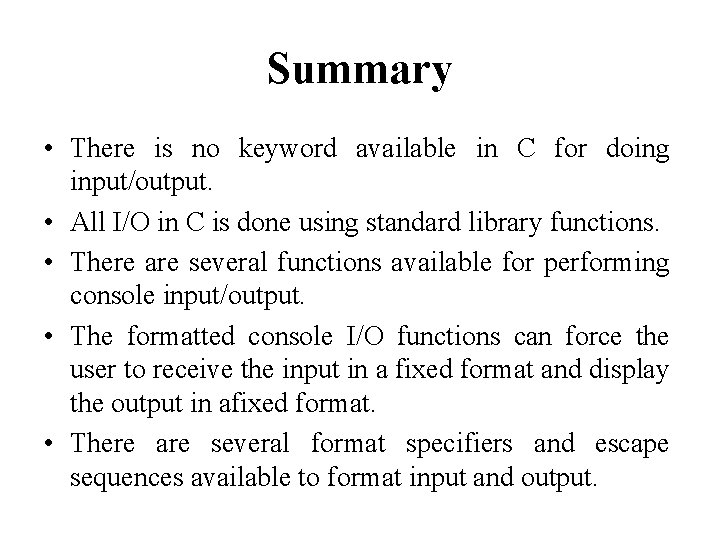
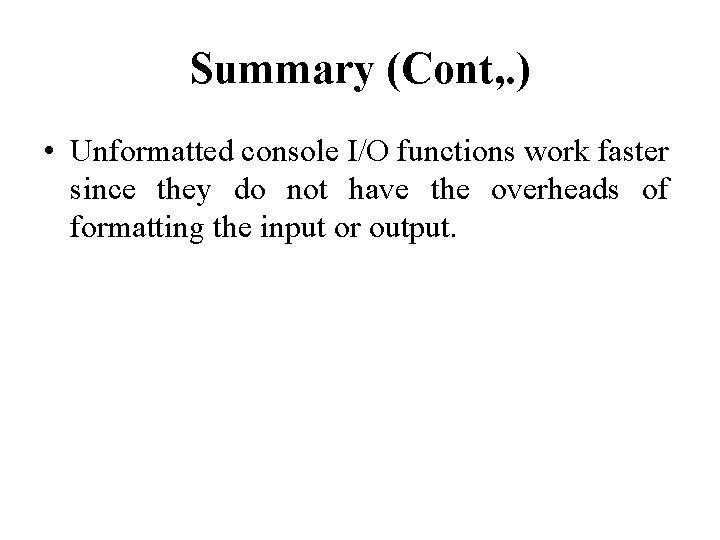
- Slides: 17
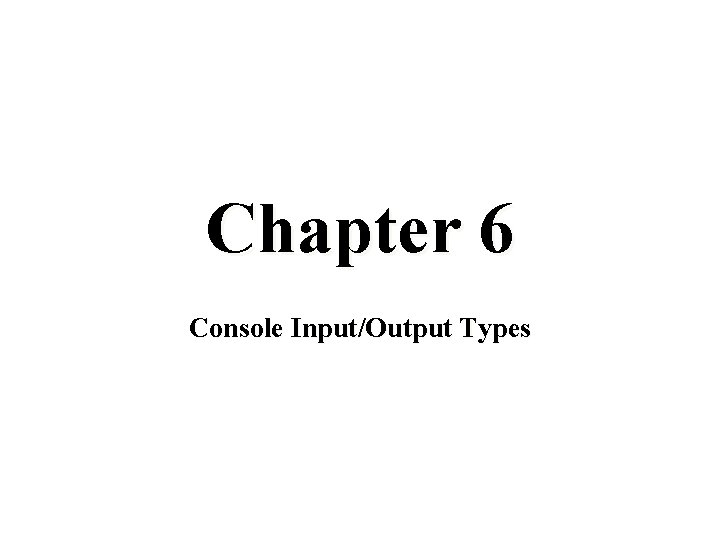
Chapter 6 Console Input/Output Types
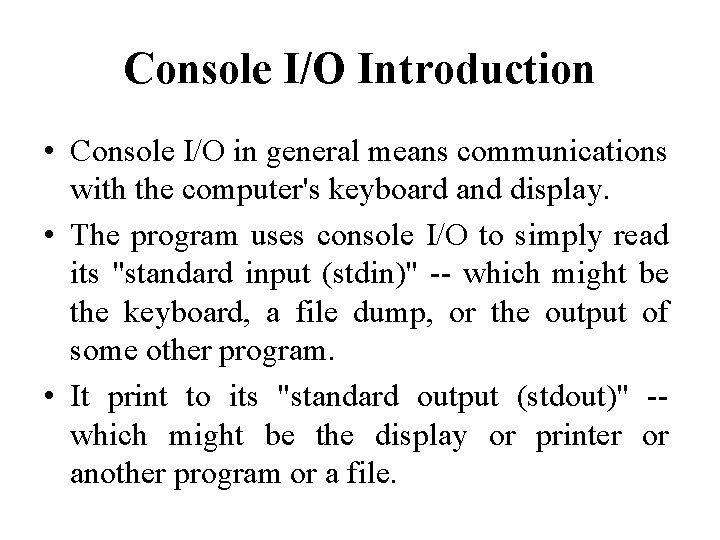
Console I/O Introduction • Console I/O in general means communications with the computer's keyboard and display. • The program uses console I/O to simply read its "standard input (stdin)" -- which might be the keyboard, a file dump, or the output of some other program. • It print to its "standard output (stdout)" -which might be the display or printer or another program or a file.
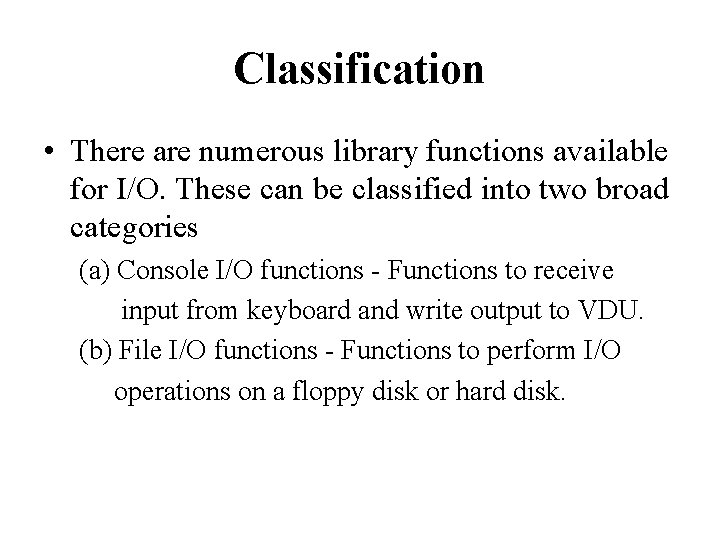
Classification • There are numerous library functions available for I/O. These can be classified into two broad categories (a) Console I/O functions - Functions to receive input from keyboard and write output to VDU. (b) File I/O functions - Functions to perform I/O operations on a floppy disk or hard disk.
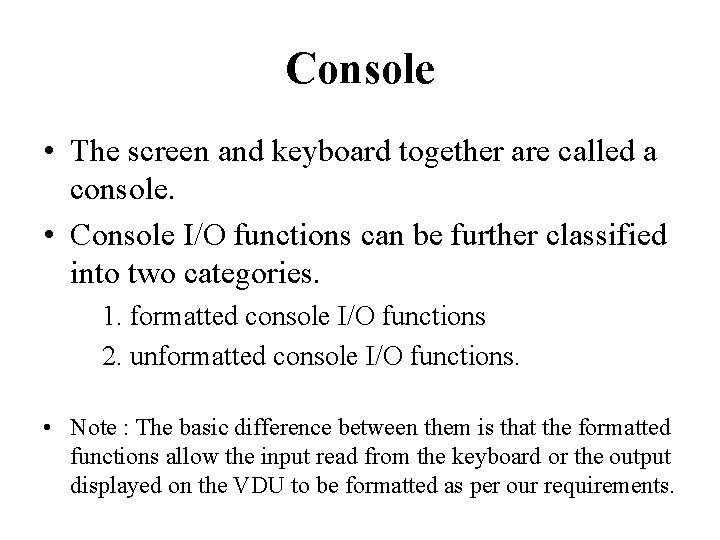
Console • The screen and keyboard together are called a console. • Console I/O functions can be further classified into two categories. 1. formatted console I/O functions 2. unformatted console I/O functions. • Note : The basic difference between them is that the formatted functions allow the input read from the keyboard or the output displayed on the VDU to be formatted as per our requirements.
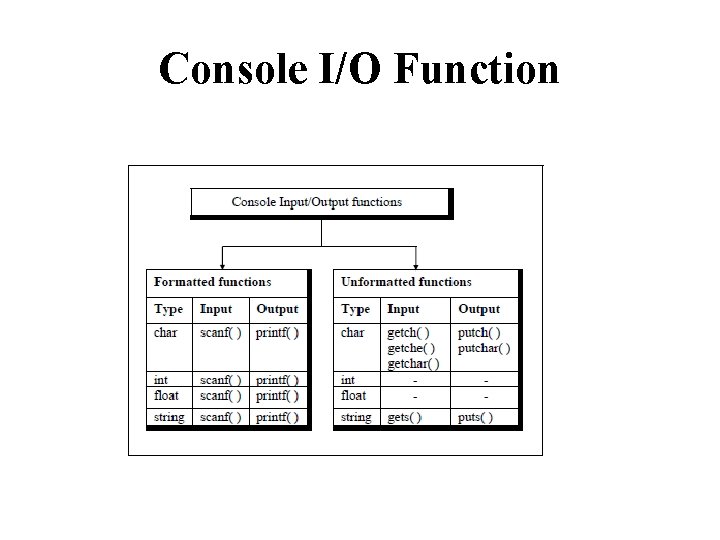
Console I/O Function
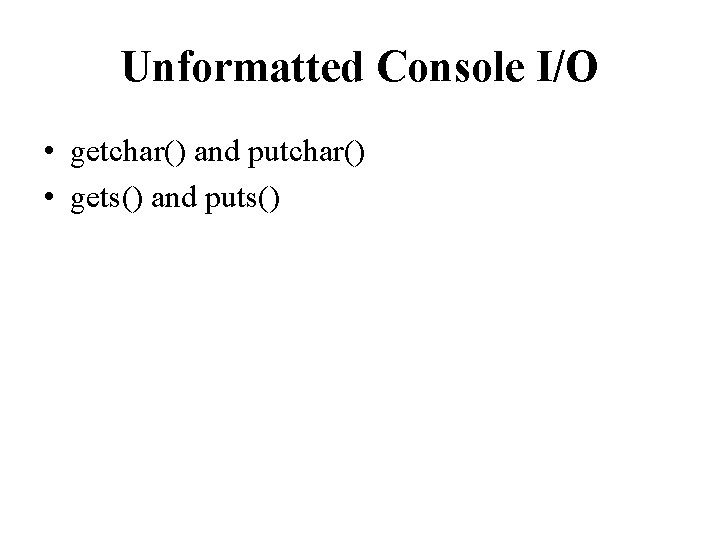
Unformatted Console I/O • getchar() and putchar() • gets() and puts()
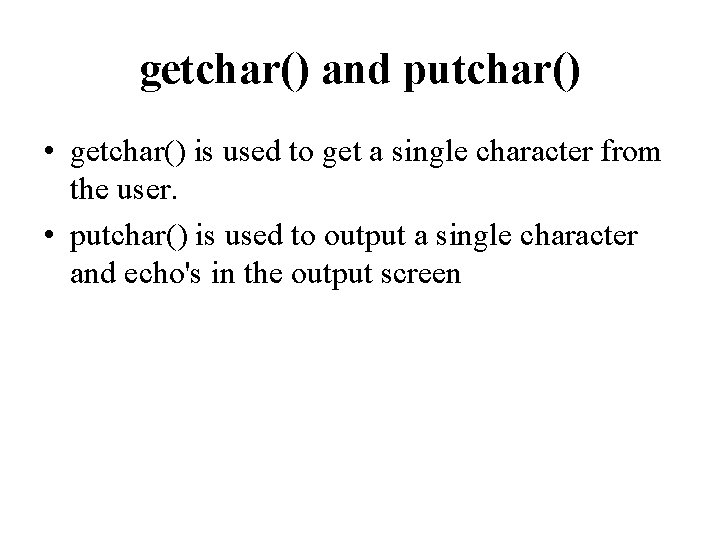
getchar() and putchar() • getchar() is used to get a single character from the user. • putchar() is used to output a single character and echo's in the output screen
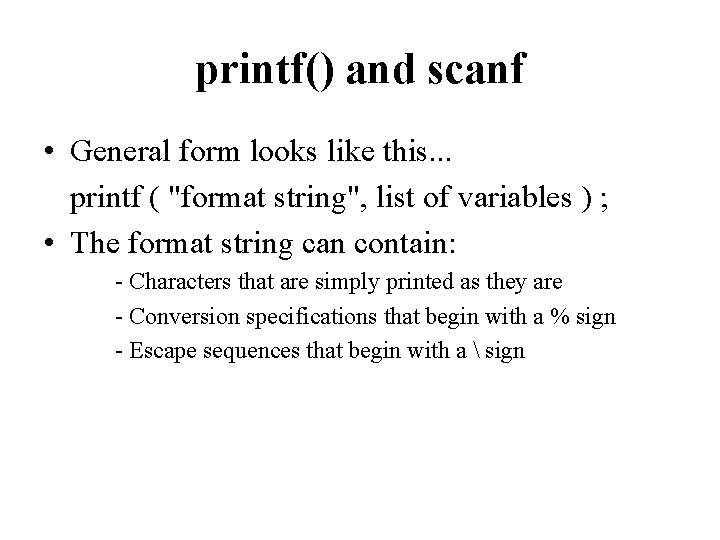
printf() and scanf • General form looks like this. . . printf ( "format string", list of variables ) ; • The format string can contain: - Characters that are simply printed as they are - Conversion specifications that begin with a % sign - Escape sequences that begin with a sign
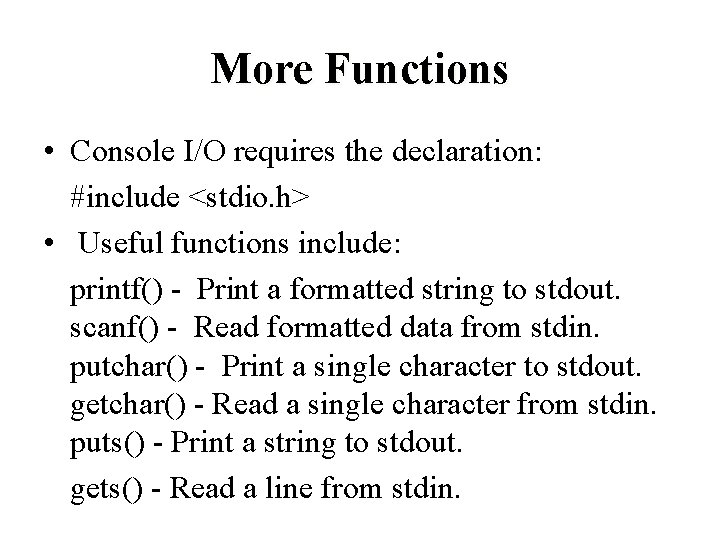
More Functions • Console I/O requires the declaration: #include <stdio. h> • Useful functions include: printf() - Print a formatted string to stdout. scanf() - Read formatted data from stdin. putchar() - Print a single character to stdout. getchar() - Read a single character from stdin. puts() - Print a string to stdout. gets() - Read a line from stdin.
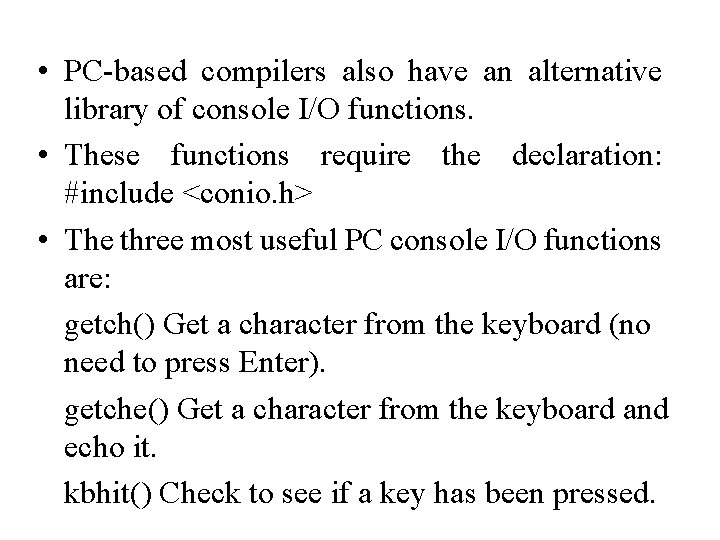
• PC-based compilers also have an alternative library of console I/O functions. • These functions require the declaration: #include <conio. h> • The three most useful PC console I/O functions are: getch() Get a character from the keyboard (no need to press Enter). getche() Get a character from the keyboard and echo it. kbhit() Check to see if a key has been pressed.
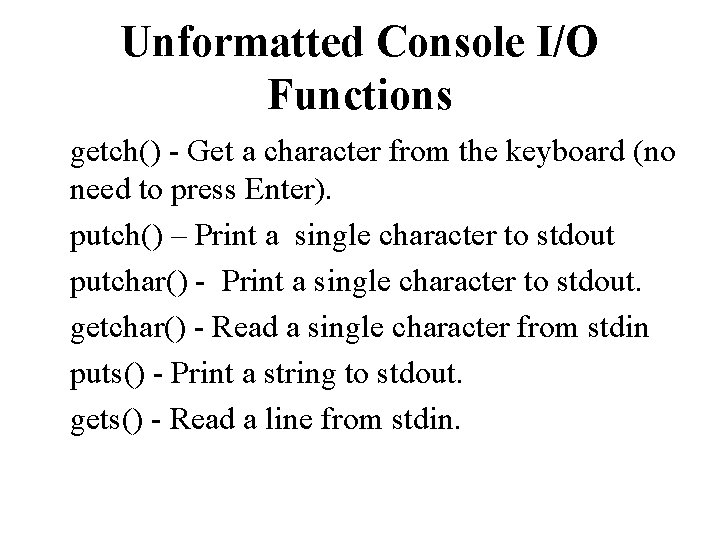
Unformatted Console I/O Functions getch() - Get a character from the keyboard (no need to press Enter). putch() – Print a single character to stdout putchar() - Print a single character to stdout. getchar() - Read a single character from stdin puts() - Print a string to stdout. gets() - Read a line from stdin.
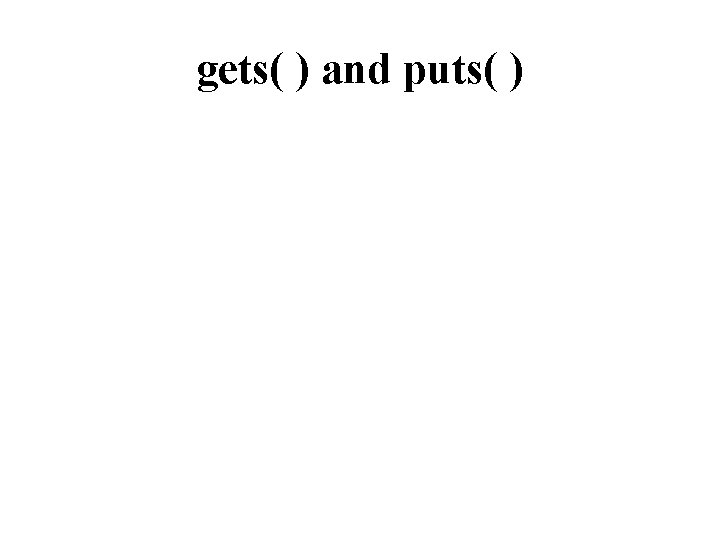
gets( ) and puts( )
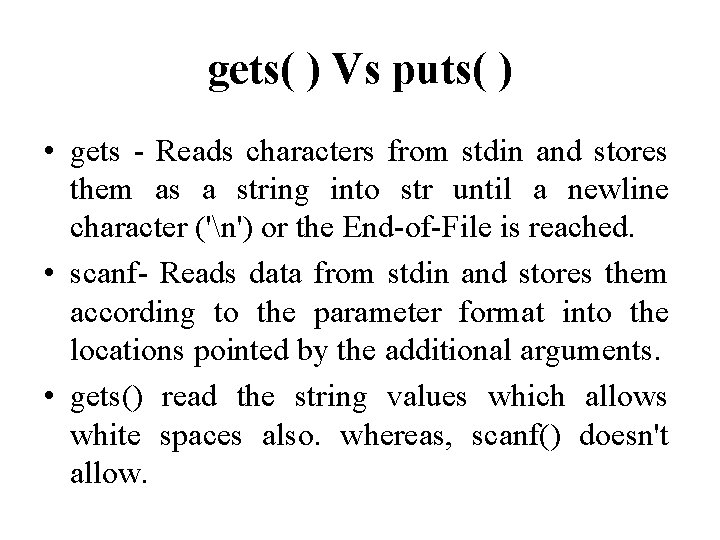
gets( ) Vs puts( ) • gets - Reads characters from stdin and stores them as a string into str until a newline character ('n') or the End-of-File is reached. • scanf- Reads data from stdin and stores them according to the parameter format into the locations pointed by the additional arguments. • gets() read the string values which allows white spaces also. whereas, scanf() doesn't allow.
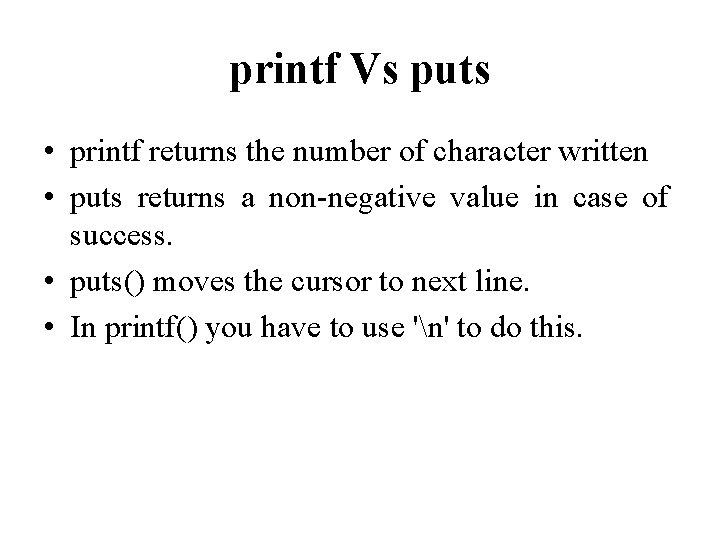
printf Vs puts • printf returns the number of character written • puts returns a non-negative value in case of success. • puts() moves the cursor to next line. • In printf() you have to use 'n' to do this.
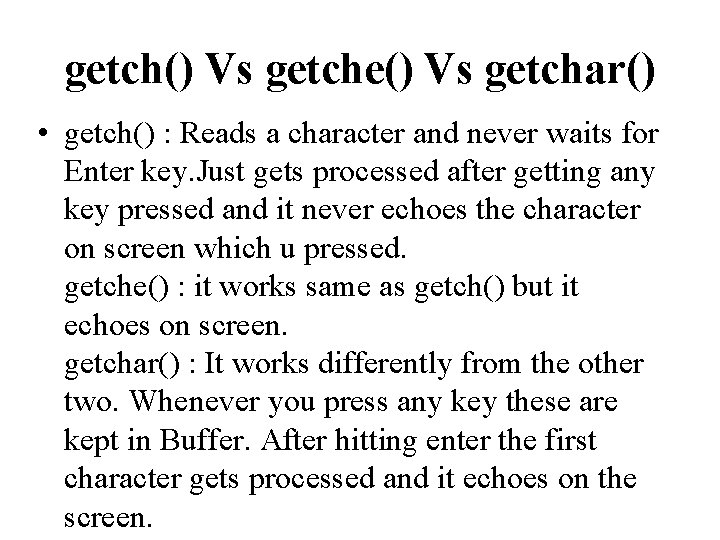
getch() Vs getche() Vs getchar() • getch() : Reads a character and never waits for Enter key. Just gets processed after getting any key pressed and it never echoes the character on screen which u pressed. getche() : it works same as getch() but it echoes on screen. getchar() : It works differently from the other two. Whenever you press any key these are kept in Buffer. After hitting enter the first character gets processed and it echoes on the screen.
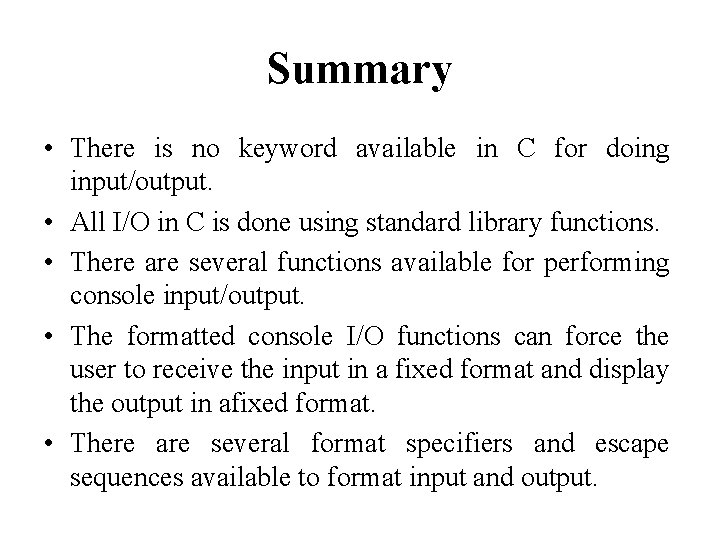
Summary • There is no keyword available in C for doing input/output. • All I/O in C is done using standard library functions. • There are several functions available for performing console input/output. • The formatted console I/O functions can force the user to receive the input in a fixed format and display the output in afixed format. • There are several format specifiers and escape sequences available to format input and output.
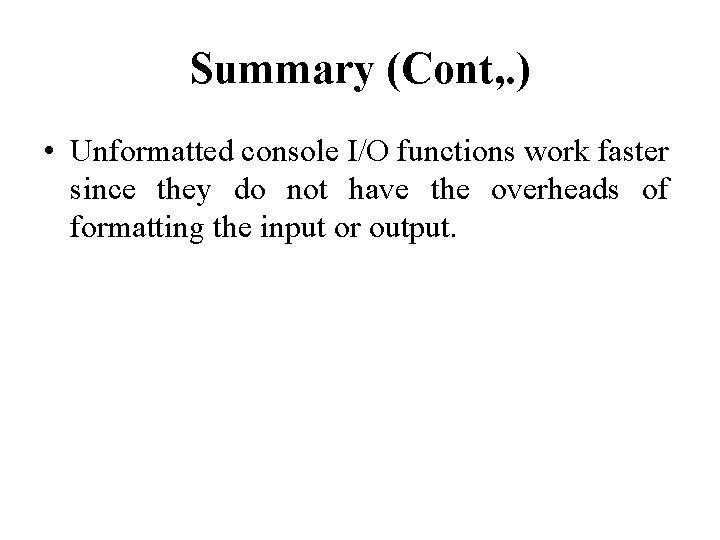
Summary (Cont, . ) • Unformatted console I/O functions work faster since they do not have the overheads of formatting the input or output.
Inputoutput devices
Snmpc management console
Eat, sleep, console scoring sheet
Arc attendant console
Sesta generazione console
Vts console
Nuuo cms
Logitech smartdock av control console
Itrs active console
Igel management interface
Getch vs getchar
Application virtualization client management console
Prestation domatel
Ns unified web maintenance console
Hybris administration console
Input console java
Cisco attendant console standard
Brochage kapandji