Chapter 5 Stacks and Queues 20046142021 Goodrich Tamassia
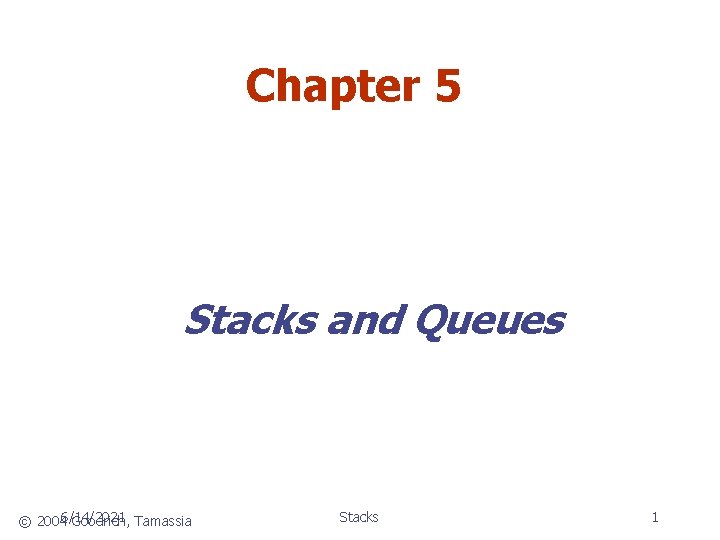
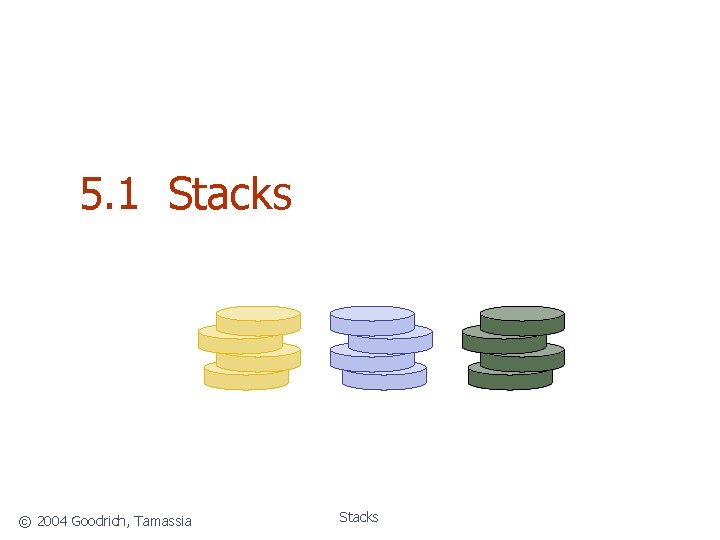
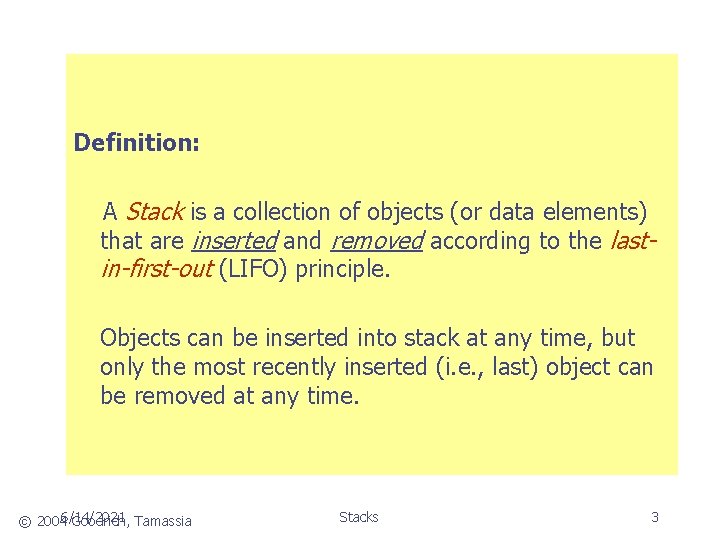
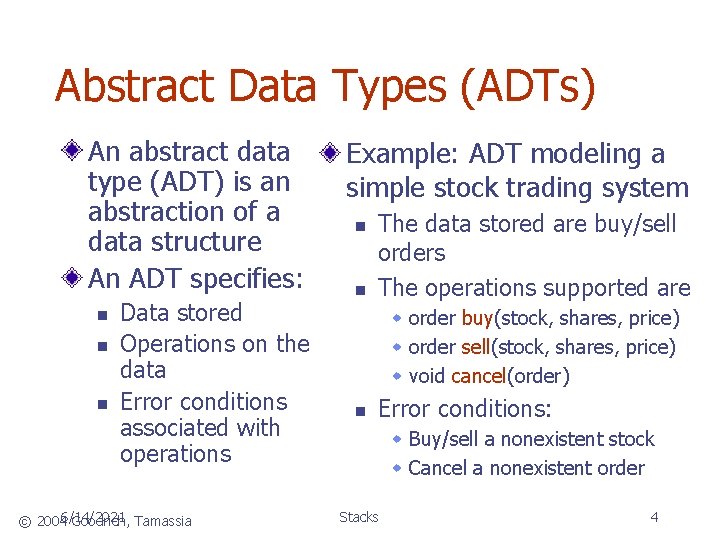
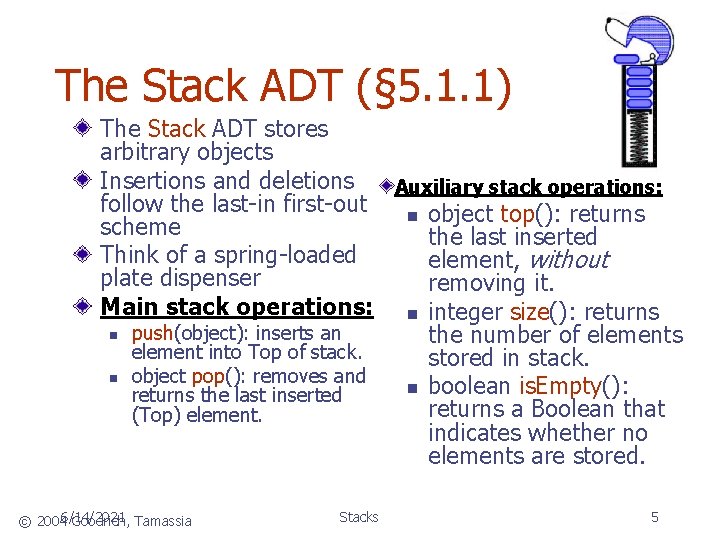
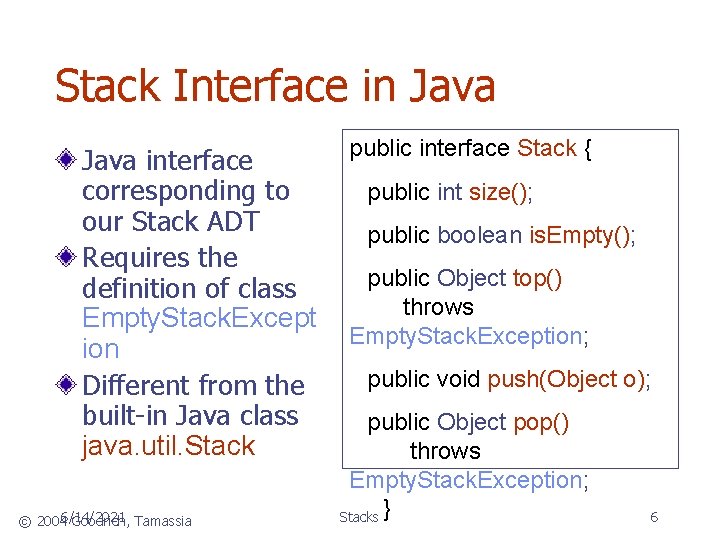
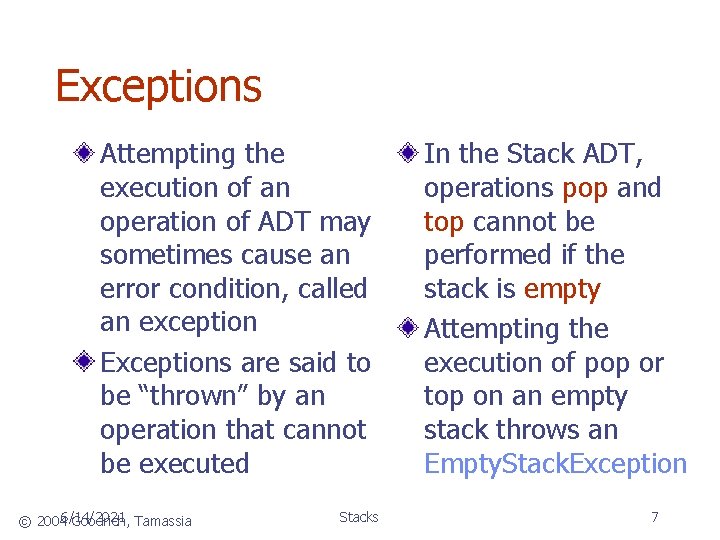
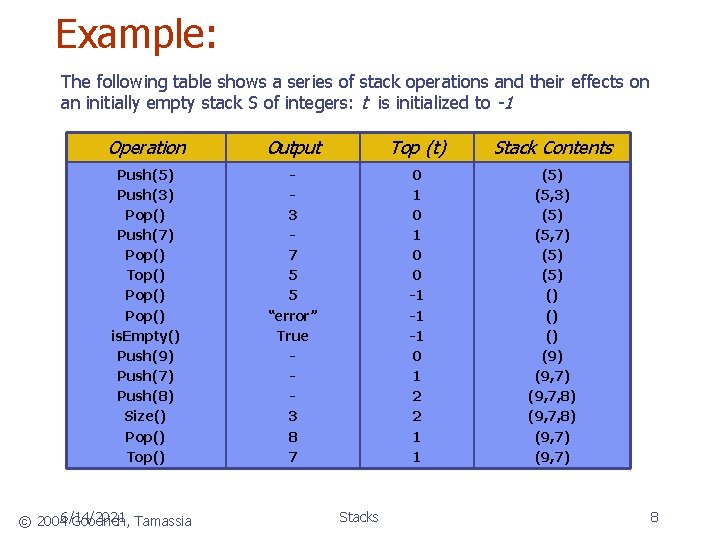
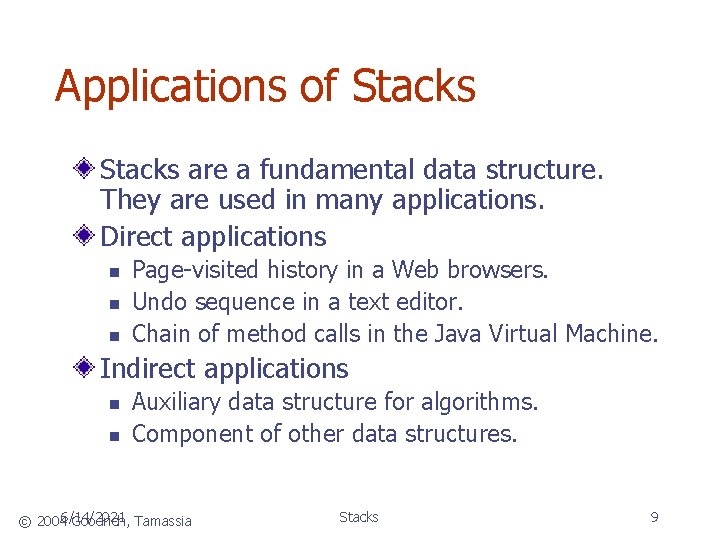
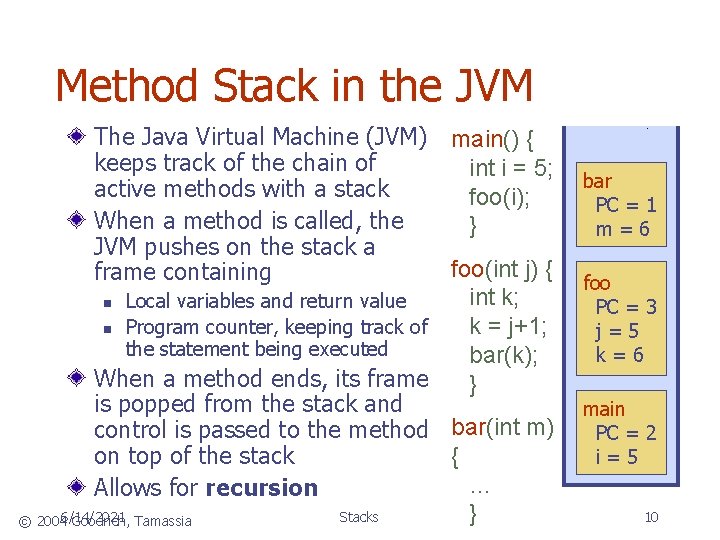
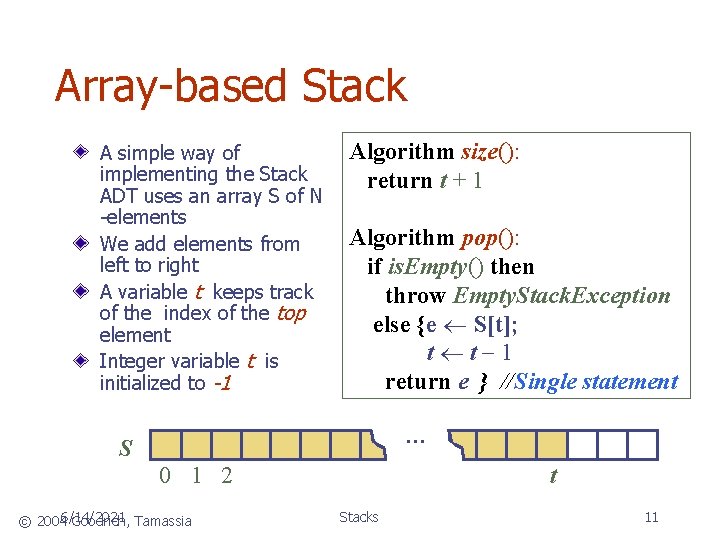
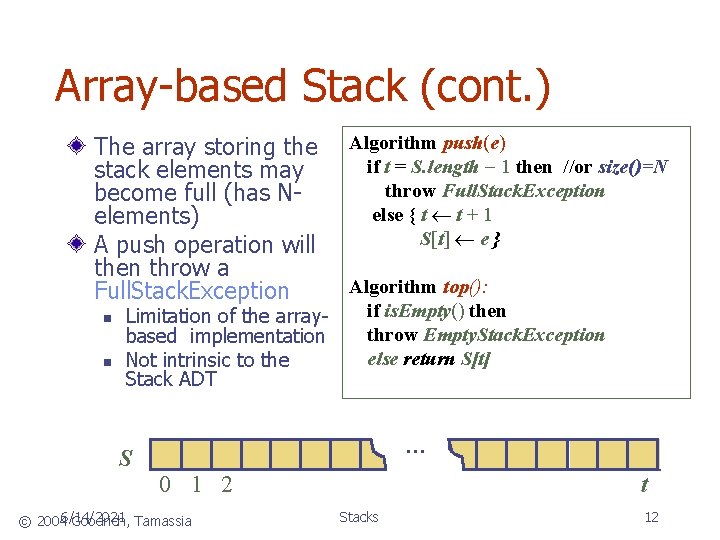
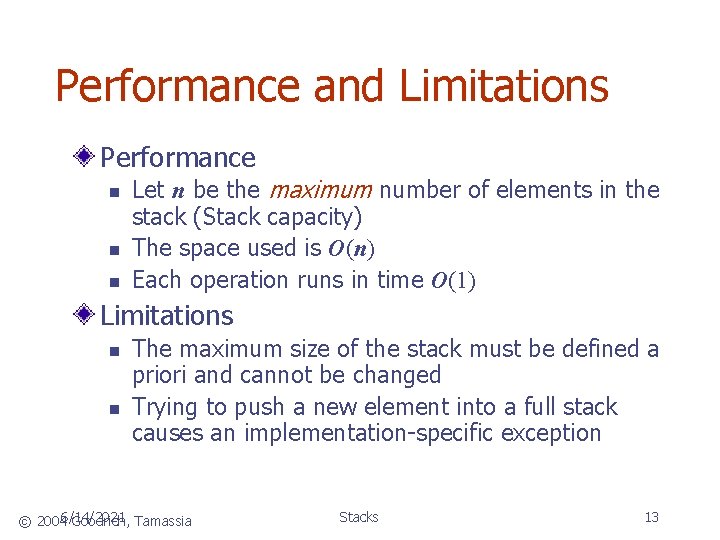
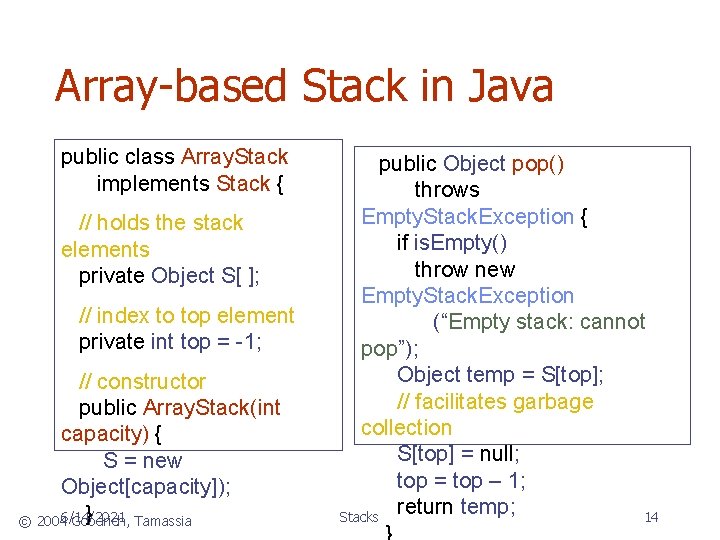
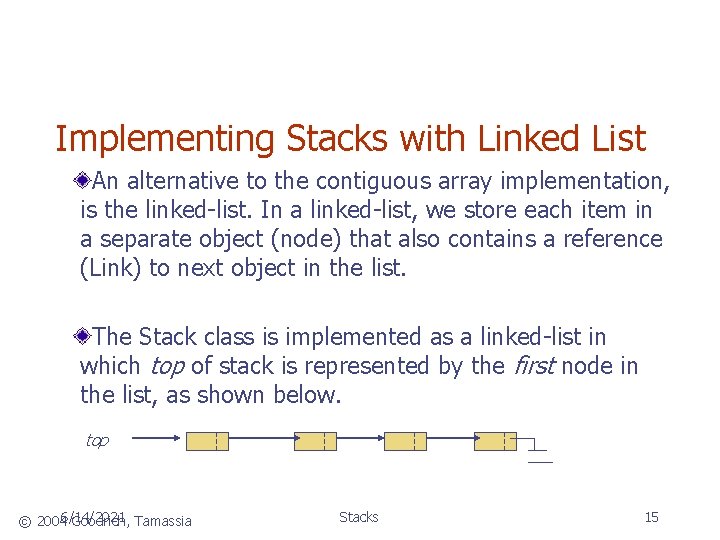
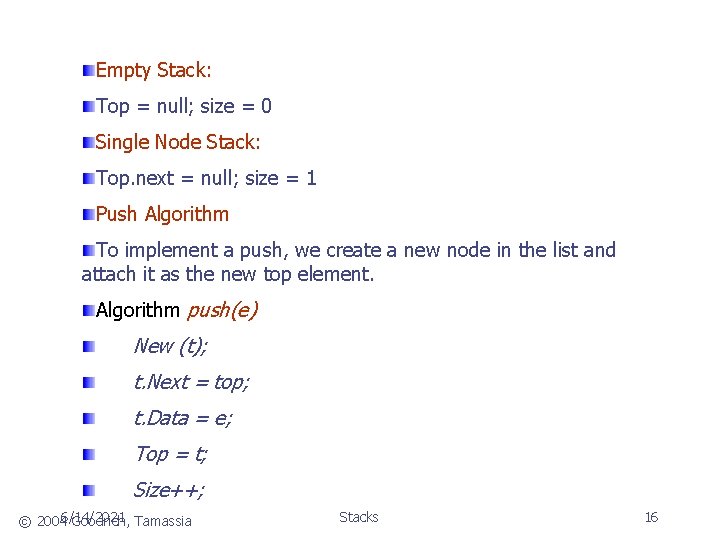
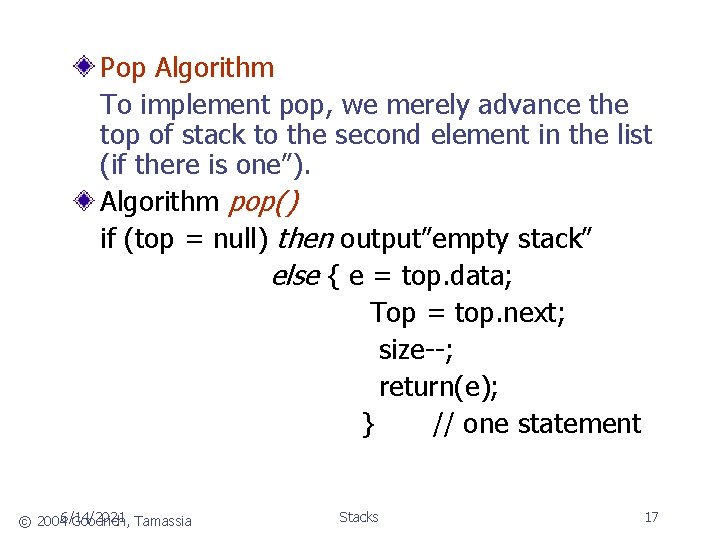
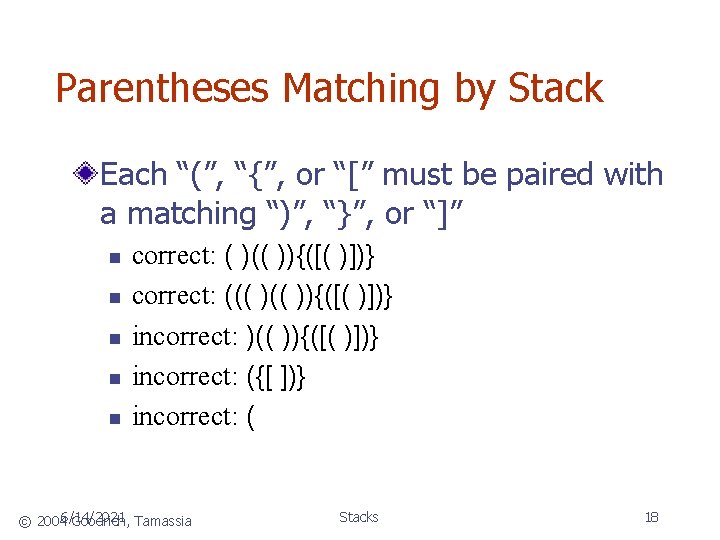
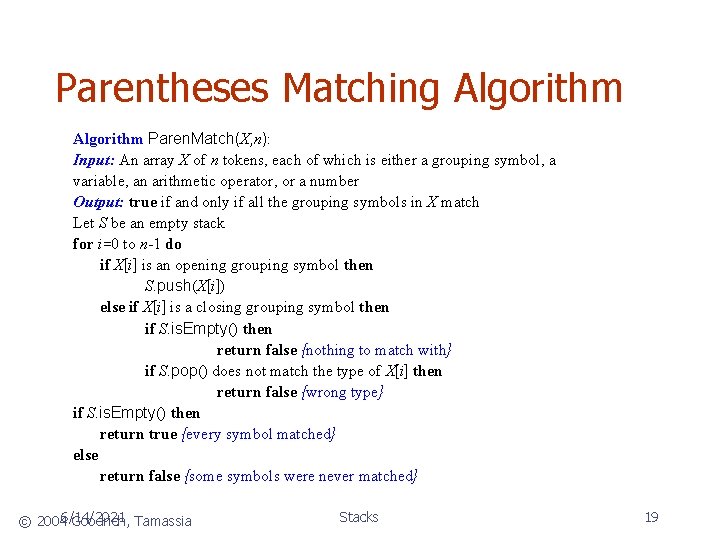
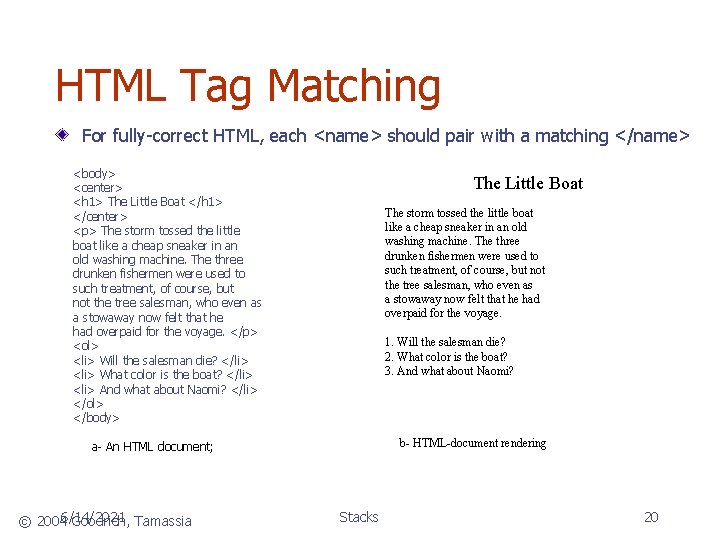
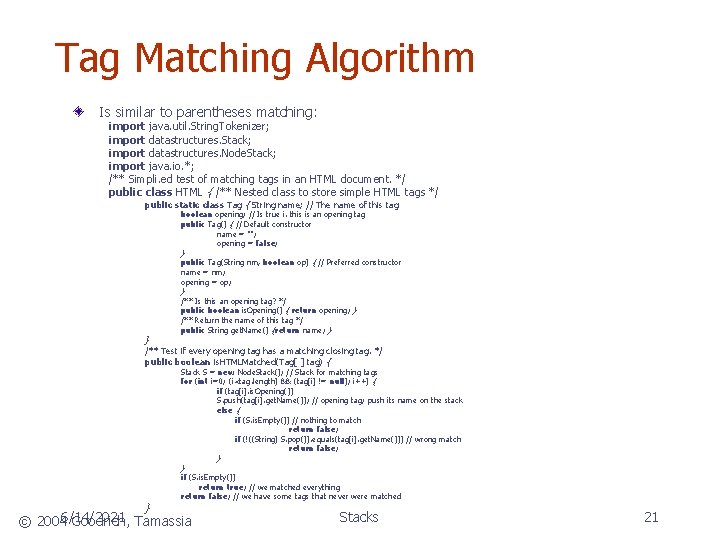
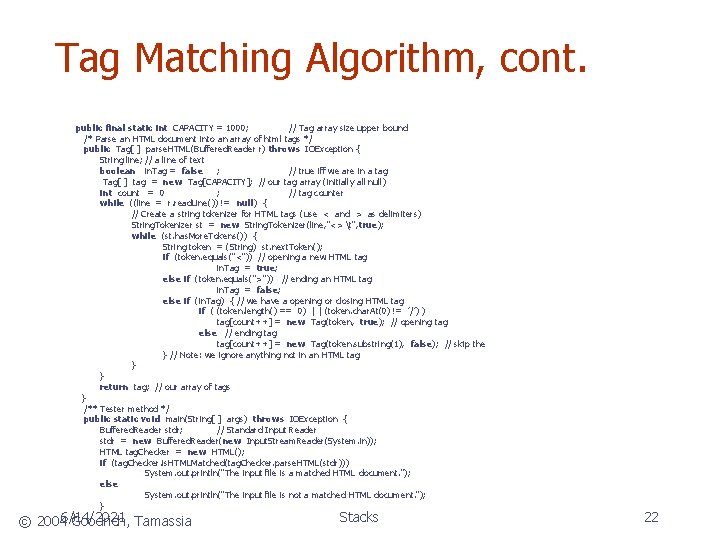
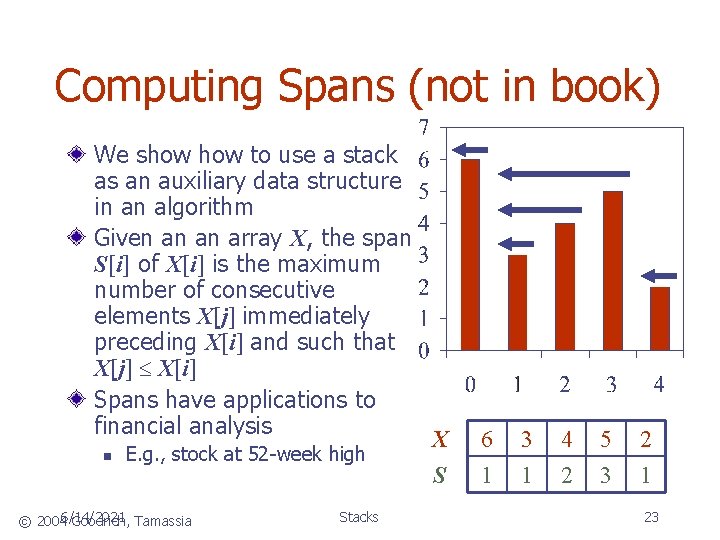
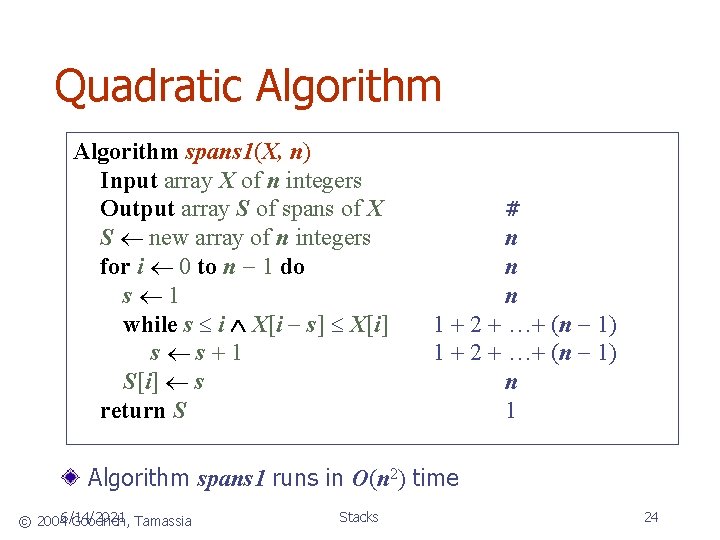
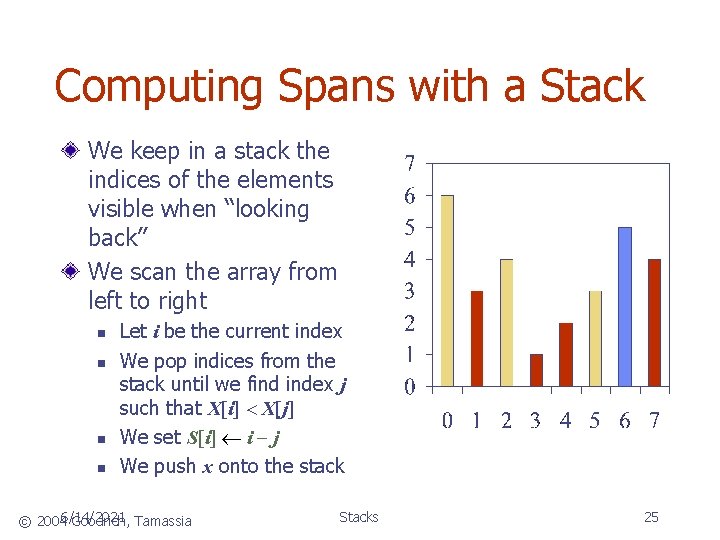
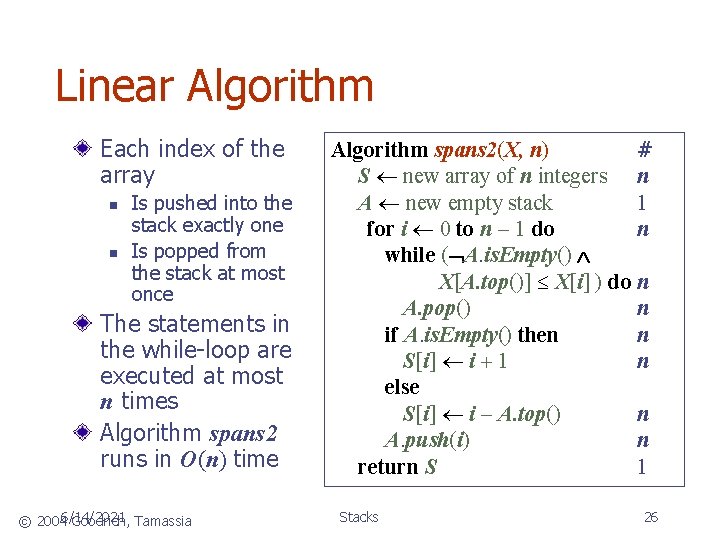
- Slides: 26
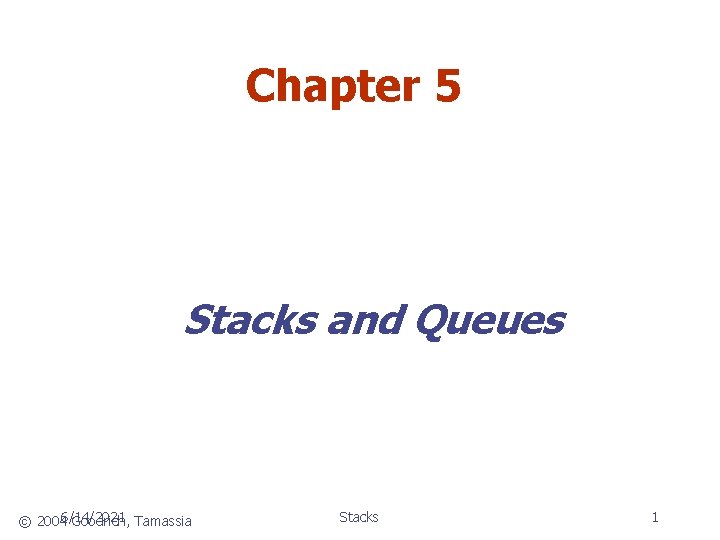
Chapter 5 Stacks and Queues © 20046/14/2021 Goodrich, Tamassia Stacks 1
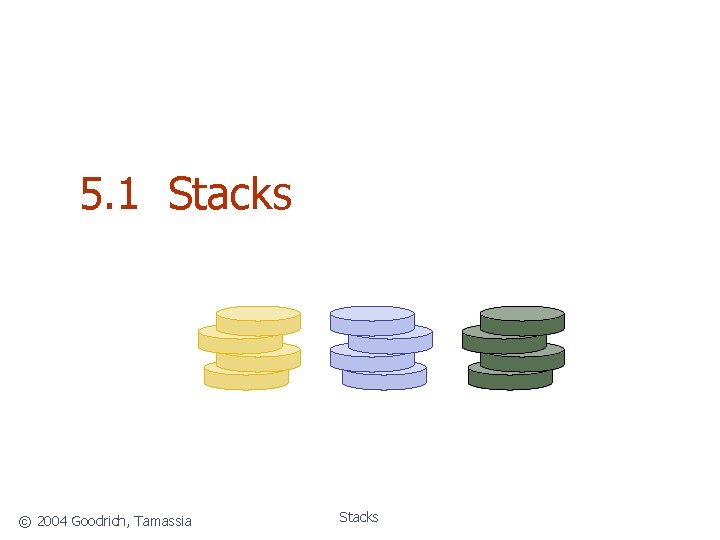
5. 1 Stacks © 2004 Goodrich, Tamassia Stacks
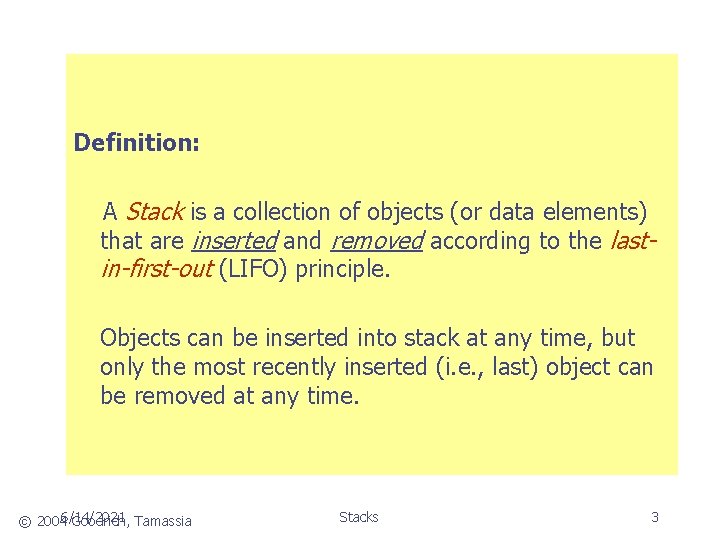
Definition: A Stack is a collection of objects (or data elements) that are inserted and removed according to the lastin-first-out (LIFO) principle. Objects can be inserted into stack at any time, but only the most recently inserted (i. e. , last) object can be removed at any time. © 20046/14/2021 Goodrich, Tamassia Stacks 3
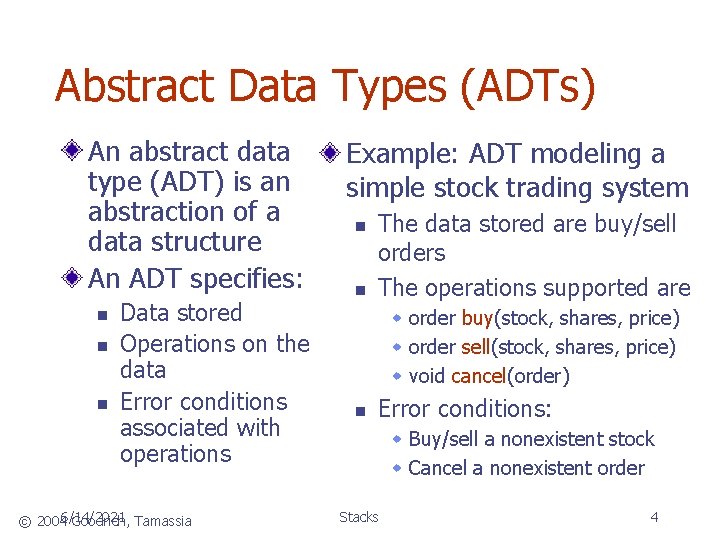
Abstract Data Types (ADTs) An abstract data type (ADT) is an abstraction of a data structure An ADT specifies: n n n Data stored Operations on the data Error conditions associated with operations © 20046/14/2021 Goodrich, Tamassia Example: ADT modeling a simple stock trading system n n The data stored are buy/sell orders The operations supported are w order buy(stock, shares, price) w order sell(stock, shares, price) w void cancel(order) n Error conditions: w Buy/sell a nonexistent stock w Cancel a nonexistent order Stacks 4
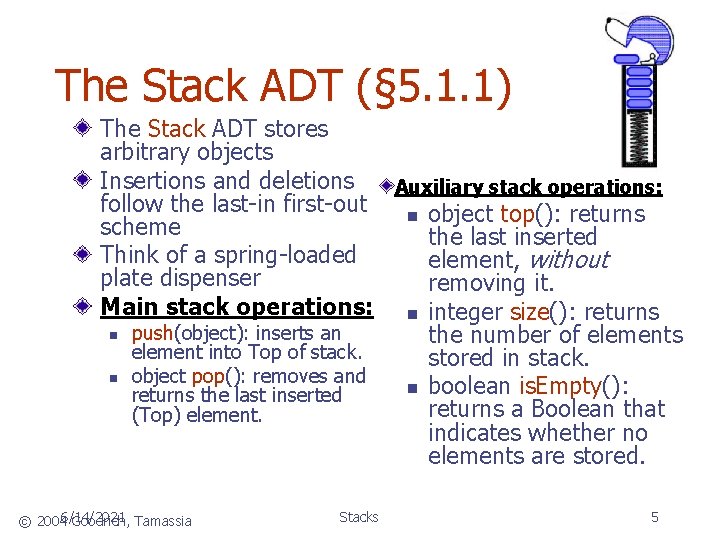
The Stack ADT (§ 5. 1. 1) The Stack ADT stores arbitrary objects Insertions and deletions Auxiliary stack operations: follow the last-in first-out n object top(): returns scheme the last inserted Think of a spring-loaded element, without plate dispenser removing it. Main stack operations: n integer size(): returns n push(object): inserts an the number of elements element into Top of stack. stored in stack. n object pop(): removes and n boolean is. Empty(): returns the last inserted returns a Boolean that (Top) element. indicates whether no elements are stored. © 20046/14/2021 Goodrich, Tamassia Stacks 5
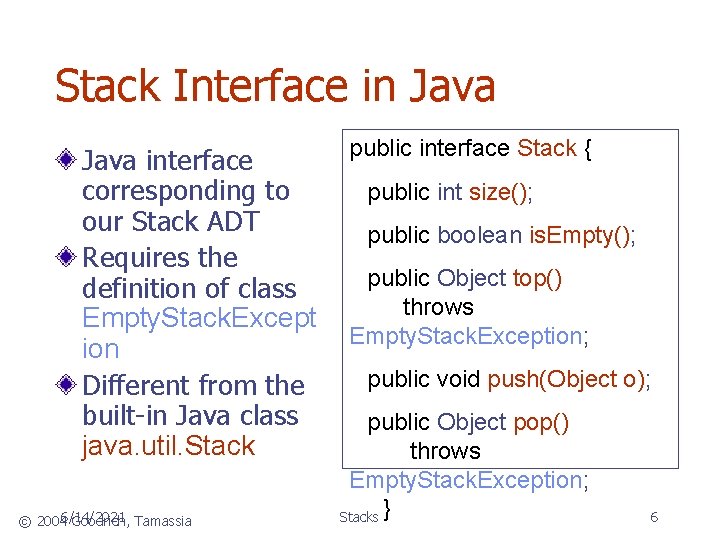
Stack Interface in Java interface corresponding to our Stack ADT Requires the definition of class Empty. Stack. Except ion Different from the built-in Java class java. util. Stack © 20046/14/2021 Goodrich, Tamassia public interface Stack { public int size(); public boolean is. Empty(); public Object top() throws Empty. Stack. Exception; public void push(Object o); public Object pop() throws Empty. Stack. Exception; Stacks } 6
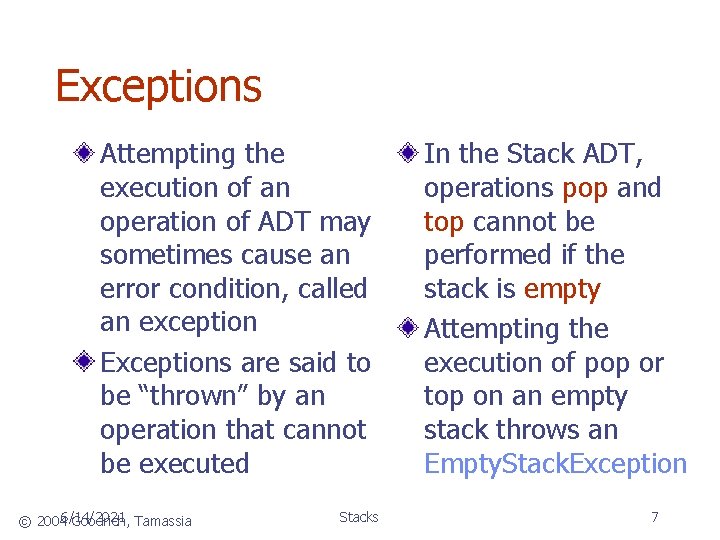
Exceptions Attempting the execution of an operation of ADT may sometimes cause an error condition, called an exception Exceptions are said to be “thrown” by an operation that cannot be executed © 20046/14/2021 Goodrich, Tamassia Stacks In the Stack ADT, operations pop and top cannot be performed if the stack is empty Attempting the execution of pop or top on an empty stack throws an Empty. Stack. Exception 7
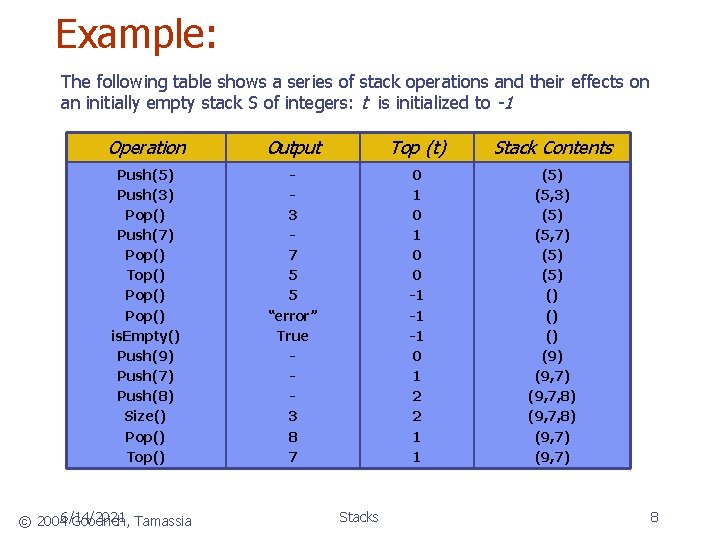
Example: The following table shows a series of stack operations and their effects on an initially empty stack S of integers: t is initialized to -1 Operation Output Top (t) Stack Contents Push(5) Push(3) Pop() Push(7) Pop() Top() Pop() is. Empty() Push(9) Push(7) Push(8) Size() Pop() Top() 3 7 5 5 “error” True 3 8 7 0 1 0 0 -1 -1 -1 0 1 2 2 1 1 (5) (5, 3) (5, 7) (5) () (9) (9, 7, 8) (9, 7) © 20046/14/2021 Goodrich, Tamassia Stacks 8
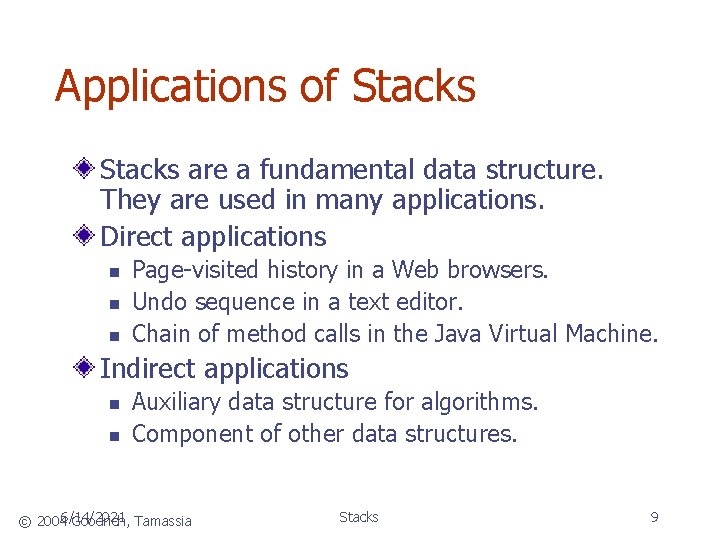
Applications of Stacks are a fundamental data structure. They are used in many applications. Direct applications n n n Page-visited history in a Web browsers. Undo sequence in a text editor. Chain of method calls in the Java Virtual Machine. Indirect applications n n Auxiliary data structure for algorithms. Component of other data structures. © 20046/14/2021 Goodrich, Tamassia Stacks 9
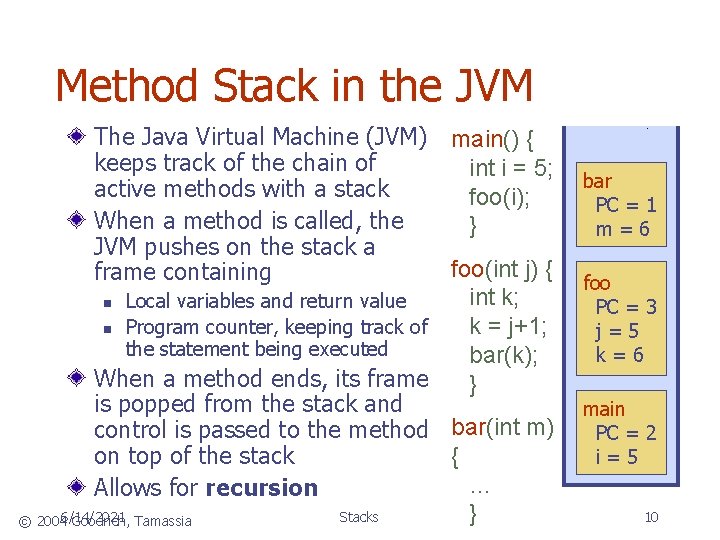
Method Stack in the JVM The Java Virtual Machine (JVM) keeps track of the chain of active methods with a stack When a method is called, the JVM pushes on the stack a frame containing main() { int i = 5; foo(i); } foo(int j) { int k; n Local variables and return value n Program counter, keeping track of k = j+1; the statement being executed bar(k); When a method ends, its frame } is popped from the stack and control is passed to the method bar(int m) { on top of the stack … Allows for recursion } Stacks © 20046/14/2021 Goodrich, Tamassia bar PC = 1 m=6 foo PC = 3 j=5 k=6 main PC = 2 i=5 10
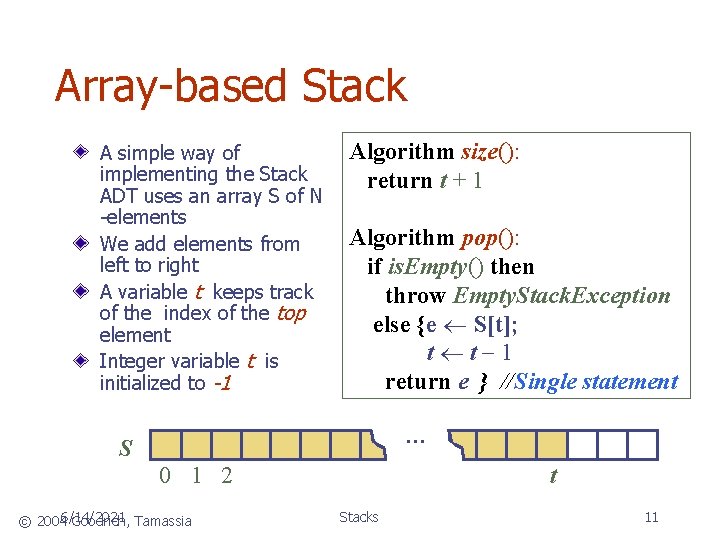
Array-based Stack A simple way of implementing the Stack ADT uses an array S of N -elements We add elements from left to right A variable t keeps track of the index of the top element Integer variable t is initialized to -1 Algorithm size(): return t + 1 Algorithm pop(): if is. Empty() then throw Empty. Stack. Exception else {e S[t]; t t 1 return e } //Single statement … S 0 1 2 © 20046/14/2021 Goodrich, Tamassia t Stacks 11
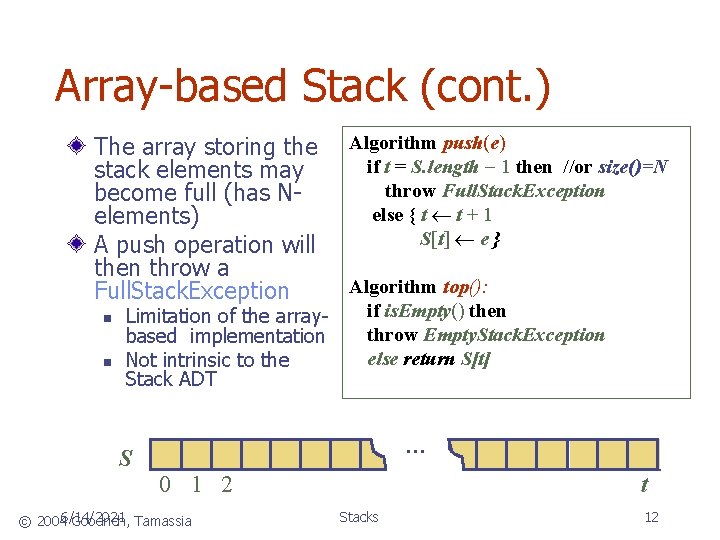
Array-based Stack (cont. ) The array storing the stack elements may become full (has Nelements) A push operation will then throw a Full. Stack. Exception n n Limitation of the arraybased implementation Not intrinsic to the Stack ADT Algorithm push(e) if t = S. length 1 then //or size()=N throw Full. Stack. Exception else { t t + 1 S[t] e } Algorithm top(): if is. Empty() then throw Empty. Stack. Exception else return S[t] … S 0 1 2 © 20046/14/2021 Goodrich, Tamassia t Stacks 12
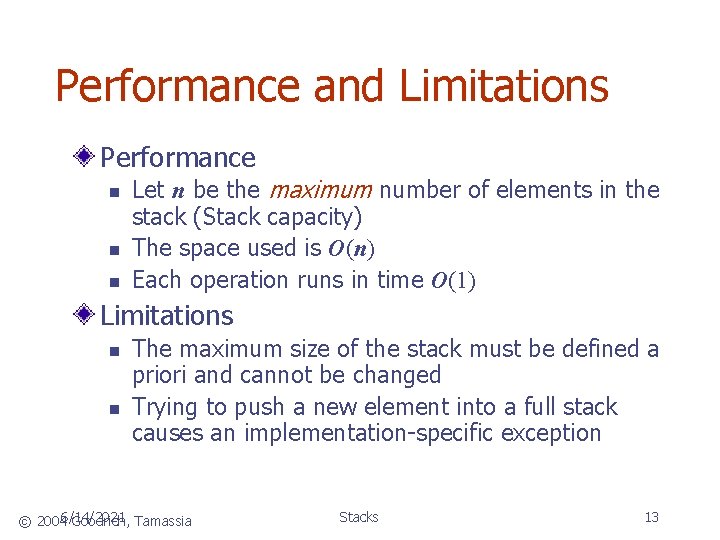
Performance and Limitations Performance n n n Let n be the maximum number of elements in the stack (Stack capacity) The space used is O(n) Each operation runs in time O(1) Limitations n n The maximum size of the stack must be defined a priori and cannot be changed Trying to push a new element into a full stack causes an implementation-specific exception © 20046/14/2021 Goodrich, Tamassia Stacks 13
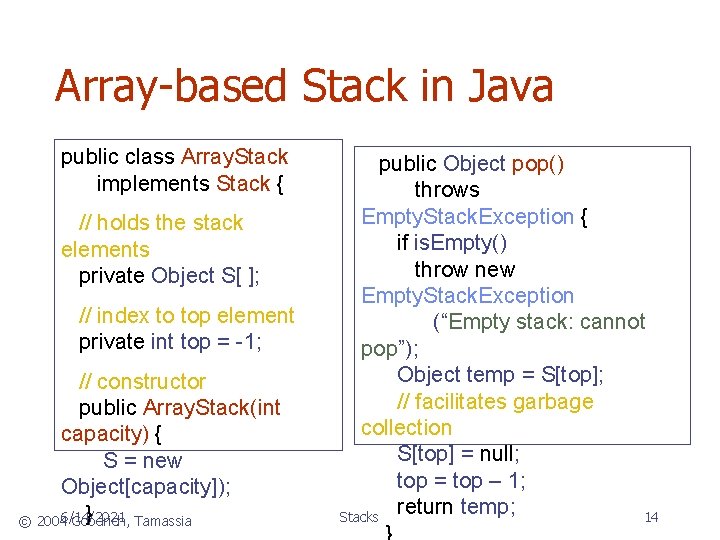
Array-based Stack in Java public class Array. Stack implements Stack { // holds the stack elements private Object S[ ]; // index to top element private int top = -1; // constructor public Array. Stack(int capacity) { S = new Object[capacity]); } © 20046/14/2021 Goodrich, Tamassia public Object pop() throws Empty. Stack. Exception { if is. Empty() throw new Empty. Stack. Exception (“Empty stack: cannot pop”); Object temp = S[top]; // facilitates garbage collection S[top] = null; top = top – 1; return temp; Stacks 14
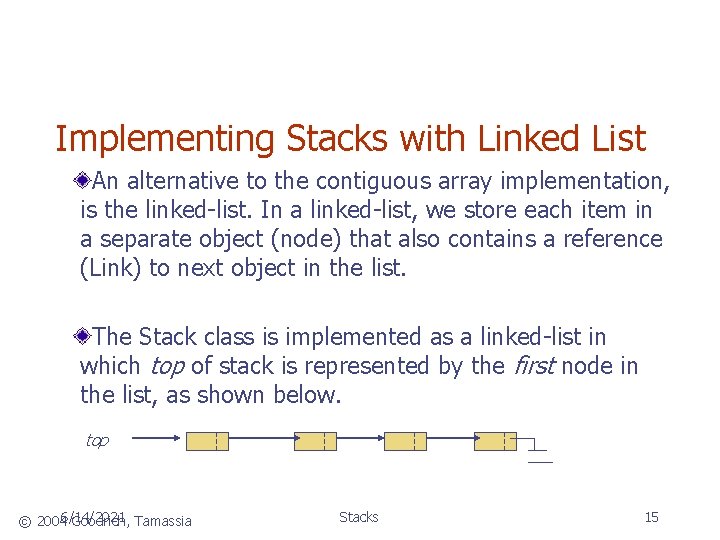
Implementing Stacks with Linked List An alternative to the contiguous array implementation, is the linked-list. In a linked-list, we store each item in a separate object (node) that also contains a reference (Link) to next object in the list. The Stack class is implemented as a linked-list in which top of stack is represented by the first node in the list, as shown below. top © 20046/14/2021 Goodrich, Tamassia Stacks 15
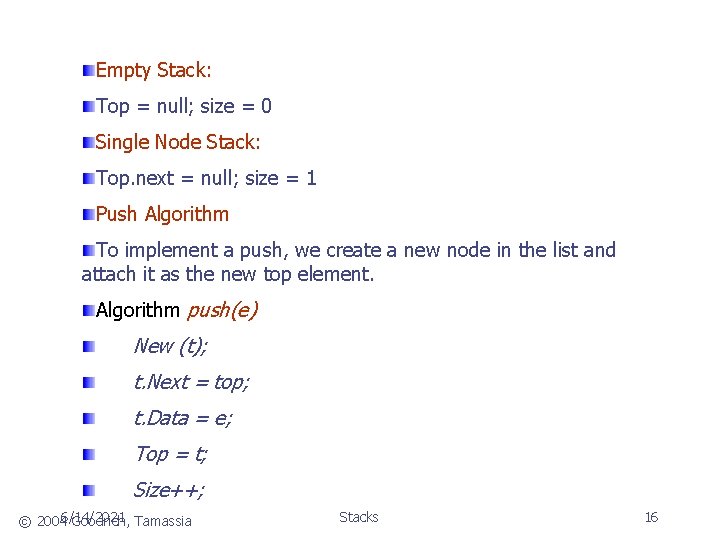
Empty Stack: Top = null; size = 0 Single Node Stack: Top. next = null; size = 1 Push Algorithm To implement a push, we create a new node in the list and attach it as the new top element. Algorithm push(e) New (t); t. Next = top; t. Data = e; Top = t; Size++; © 20046/14/2021 Goodrich, Tamassia Stacks 16
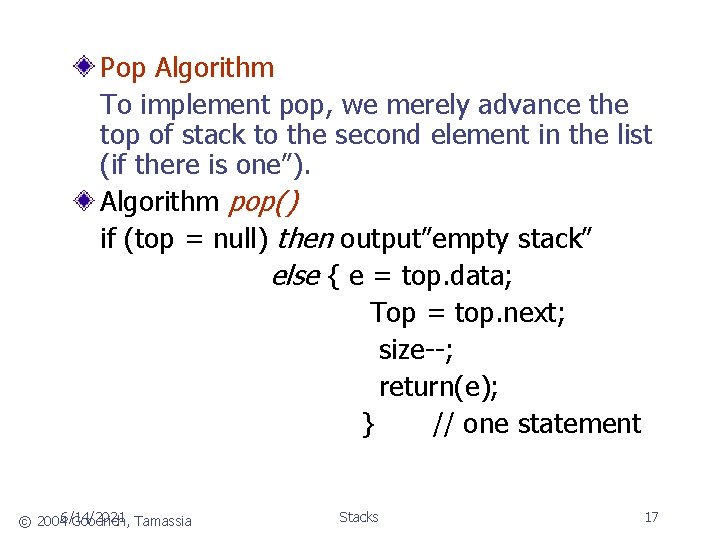
Pop Algorithm To implement pop, we merely advance the top of stack to the second element in the list (if there is one”). Algorithm pop() if (top = null) then output”empty stack” else { e = top. data; Top = top. next; size--; return(e); } // one statement © 20046/14/2021 Goodrich, Tamassia Stacks 17
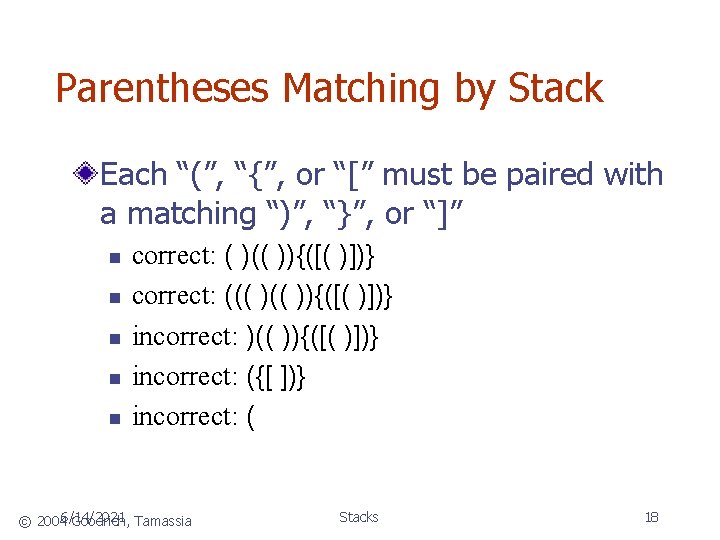
Parentheses Matching by Stack Each “(”, “{”, or “[” must be paired with a matching “)”, “}”, or “]” n n n correct: ( )(( )){([( )])} correct: ((( )){([( )])} incorrect: )(( )){([( )])} incorrect: ({[ ])} incorrect: ( © 20046/14/2021 Goodrich, Tamassia Stacks 18
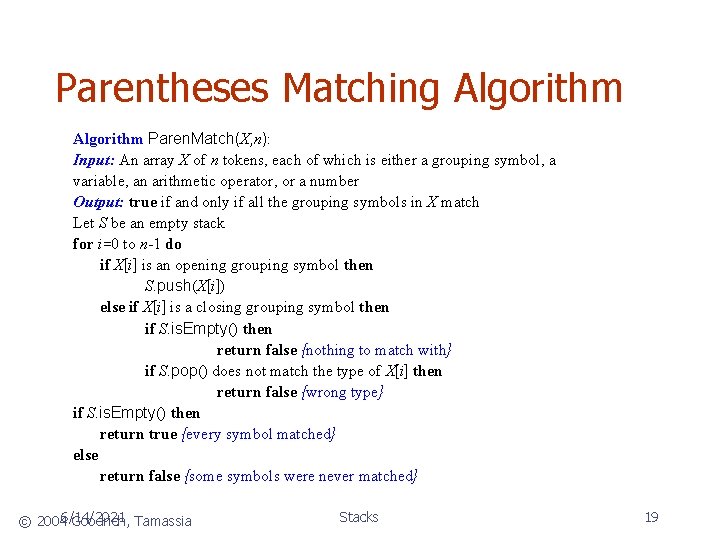
Parentheses Matching Algorithm Paren. Match(X, n): Input: An array X of n tokens, each of which is either a grouping symbol, a variable, an arithmetic operator, or a number Output: true if and only if all the grouping symbols in X match Let S be an empty stack for i=0 to n-1 do if X[i] is an opening grouping symbol then S. push(X[i]) else if X[i] is a closing grouping symbol then if S. is. Empty() then return false {nothing to match with} if S. pop() does not match the type of X[i] then return false {wrong type} if S. is. Empty() then return true {every symbol matched} else return false {some symbols were never matched} © 20046/14/2021 Goodrich, Tamassia Stacks 19
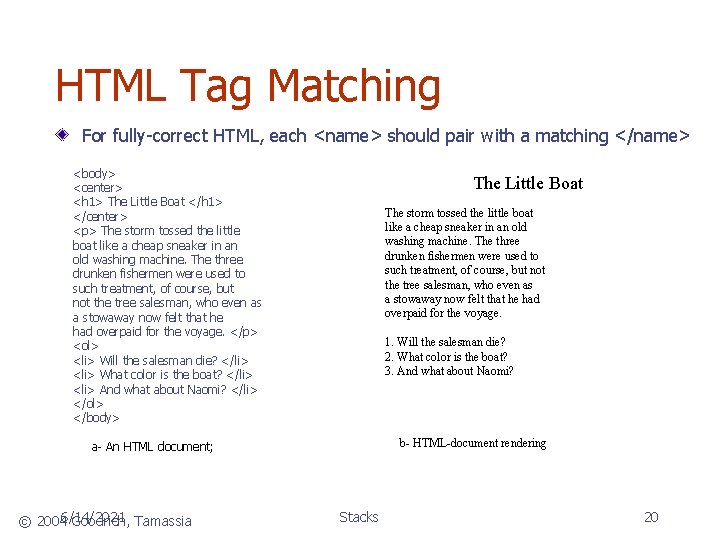
HTML Tag Matching For fully-correct HTML, each <name> should pair with a matching </name> <body> <center> <h 1> The Little Boat </h 1> </center> <p> The storm tossed the little boat like a cheap sneaker in an old washing machine. The three drunken fishermen were used to such treatment, of course, but not the tree salesman, who even as a stowaway now felt that he had overpaid for the voyage. </p> <ol> <li> Will the salesman die? </li> <li> What color is the boat? </li> <li> And what about Naomi? </li> </ol> </body> The Little Boat The storm tossed the little boat like a cheap sneaker in an old washing machine. The three drunken fishermen were used to such treatment, of course, but not the tree salesman, who even as a stowaway now felt that he had overpaid for the voyage. 1. Will the salesman die? 2. What color is the boat? 3. And what about Naomi? b- HTML-document rendering a- An HTML document; © 20046/14/2021 Goodrich, Tamassia Stacks 20
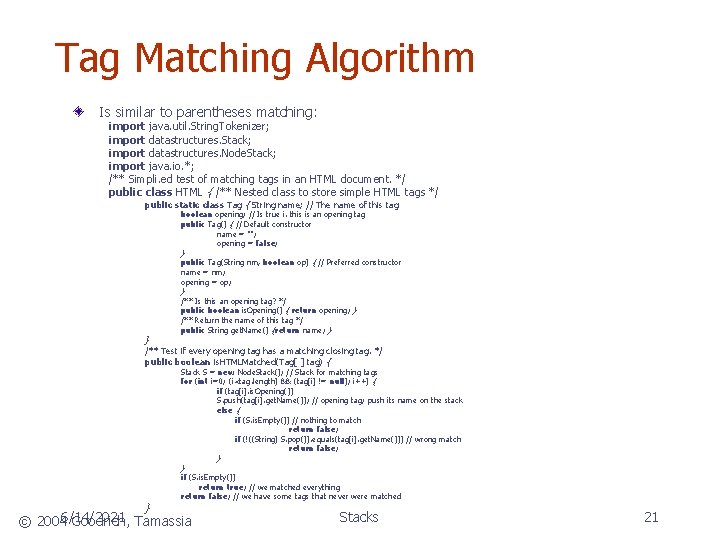
Tag Matching Algorithm Is similar to parentheses matching: import java. util. String. Tokenizer; import datastructures. Stack; import datastructures. Node. Stack; import java. io. *; /** Simpli. ed test of matching tags in an HTML document. */ public class HTML { /** Nested class to store simple HTML tags */ public static class Tag { String name; // The name of this tag boolean opening; // Is true i. this is an opening tag public Tag() { // Default constructor name = ""; opening = false; } public Tag(String nm, boolean op) { // Preferred constructor name = nm; opening = op; } } /** Is this an opening tag? */ public boolean is. Opening() { return opening; } /** Return the name of this tag */ public String get. Name() {return name; } /** Test if every opening tag has a matching closing tag. */ public boolean is. HTMLMatched(Tag[ ] tag) { Stack S = new Node. Stack(); // Stack for matching tags for (int i=0; (i<tag. length) && (tag[i] != null); i++) { if (tag[i]. is. Opening()) S. push(tag[i]. get. Name()); // opening tag; push its name on the stack else { if (S. is. Empty()) // nothing to match return false; if (!((String) S. pop()). equals(tag[i]. get. Name())) // wrong match return false; } } } if (S. is. Empty()) return true; // we matched everything return false; // we have some tags that never were matched © 20046/14/2021 Goodrich, Tamassia Stacks 21
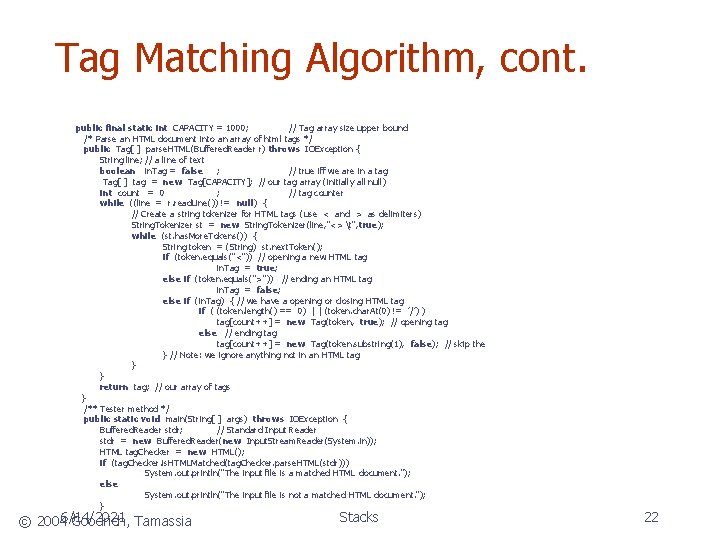
Tag Matching Algorithm, cont. public final static int CAPACITY = 1000; // Tag array size upper bound /* Parse an HTML document into an array of html tags */ public Tag[ ] parse. HTML(Buffered. Reader r) throws IOException { String line; // a line of text boolean in. Tag = false ; // true iff we are in a tag Tag[ ] tag = new Tag[CAPACITY]; // our tag array (initially all null) int count = 0 ; // tag counter while ((line = r. read. Line()) != null) { // Create a string tokenizer for HTML tags (use < and > as delimiters) String. Tokenizer st = new String. Tokenizer(line, "<> t", true); while (st. has. More. Tokens()) { String token = (String) st. next. Token(); if (token. equals("<")) // opening a new HTML tag in. Tag = true; else if (token. equals(">")) // ending an HTML tag in. Tag = false; else if (in. Tag) { // we have a opening or closing HTML tag if ( (token. length() == 0) | | (token. char. At(0) != ’/’) ) tag[count++] = new Tag(token, true); // opening tag else // ending tag[count++] = new Tag(token. substring(1), false); // skip the } // Note: we ignore anything not in an HTML tag } } return tag; // our array of tags } /** Tester method */ public static void main(String[ ] args) throws IOException { Buffered. Reader stdr; // Standard Input Reader stdr = new Buffered. Reader(new Input. Stream. Reader(System. in)); HTML tag. Checker = new HTML(); if (tag. Checker. is. HTMLMatched(tag. Checker. parse. HTML(stdr))) System. out. println("The input file is a matched HTML document. "); else System. out. println("The input file is not a matched HTML document. "); } } 6/14/2021 Stacks © 2004 Goodrich, Tamassia 22
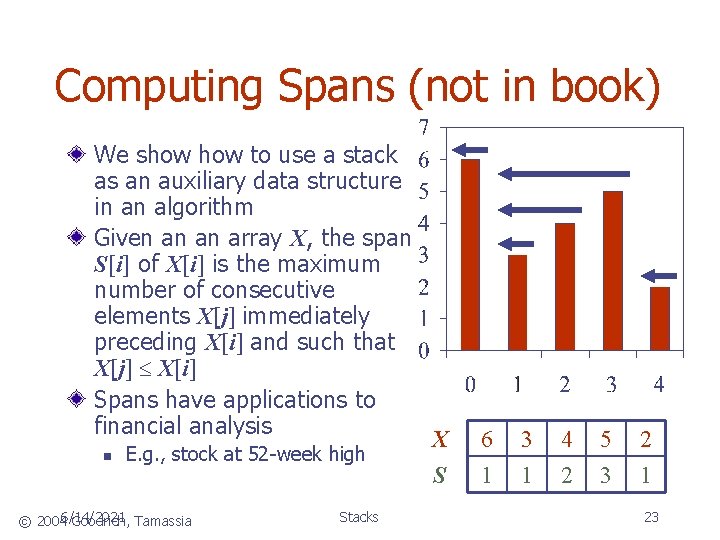
Computing Spans (not in book) We show to use a stack as an auxiliary data structure in an algorithm Given an an array X, the span S[i] of X[i] is the maximum number of consecutive elements X[j] immediately preceding X[i] and such that X[j] X[i] Spans have applications to financial analysis n E. g. , stock at 52 -week high © 20046/14/2021 Goodrich, Tamassia Stacks X S 6 1 3 1 4 2 5 3 2 1 23
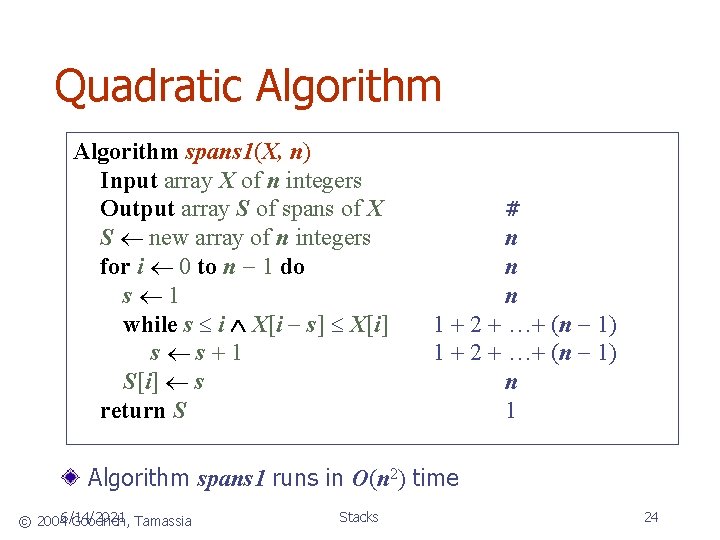
Quadratic Algorithm spans 1(X, n) Input array X of n integers Output array S of spans of X S new array of n integers for i 0 to n 1 do s 1 while s i X[i s] X[i] s s+1 S[i] s return S # n n n 1 + 2 + …+ (n 1) n 1 Algorithm spans 1 runs in O(n 2) time © 20046/14/2021 Goodrich, Tamassia Stacks 24
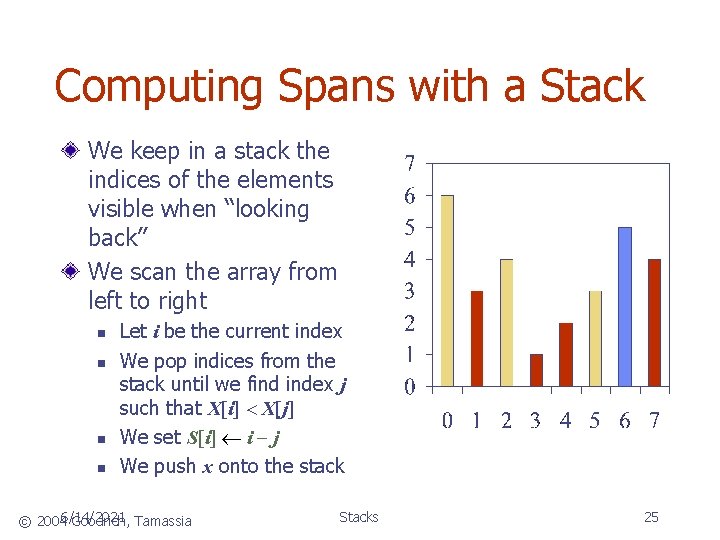
Computing Spans with a Stack We keep in a stack the indices of the elements visible when “looking back” We scan the array from left to right n n Let i be the current index We pop indices from the stack until we find index j such that X[i] X[j] We set S[i] i j We push x onto the stack © 20046/14/2021 Goodrich, Tamassia Stacks 25
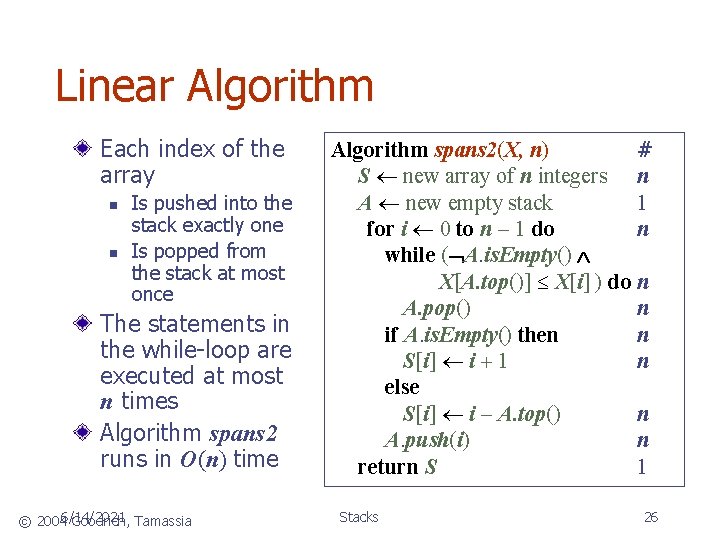
Linear Algorithm Each index of the array n n Is pushed into the stack exactly one Is popped from the stack at most once The statements in the while-loop are executed at most n times Algorithm spans 2 runs in O(n) time © 20046/14/2021 Goodrich, Tamassia Algorithm spans 2(X, n) # S new array of n integers n A new empty stack 1 for i 0 to n 1 do n while ( A. is. Empty() X[A. top()] X[i] ) do n A. pop() n if A. is. Empty() then n S[i] i + 1 n else S[i] i A. top() n A. push(i) n return S 1 Stacks 26
Goodrich tamassia
Goodrich tamassia
Empty python
Java stacks and queues
Exercises on stacks and queues
Java stacks and queues
Laura tamassia
North south east west direction
Wave cut notch and platform diagram
Level pool routing
Heidi goodrich andrade
Jamal goodrich
Flood routing example
3 min quiz
Queue representation
Ipcs unix
Priority queue adt
Pipes in rtos
Applications of priority queues
Struktur data queue sering digunakan untuk
Queue definition
Erisone queues
Pda with two stacks
Cliff terrace caves stacks
Types of stacks
Stacks in data structures
Stacks+routined