Chapter 11 Inheritance and Polymorphism Liang Introduction to
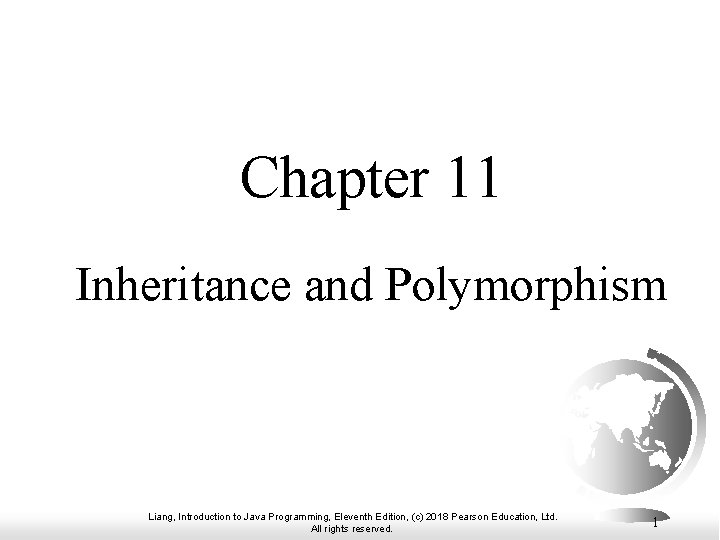
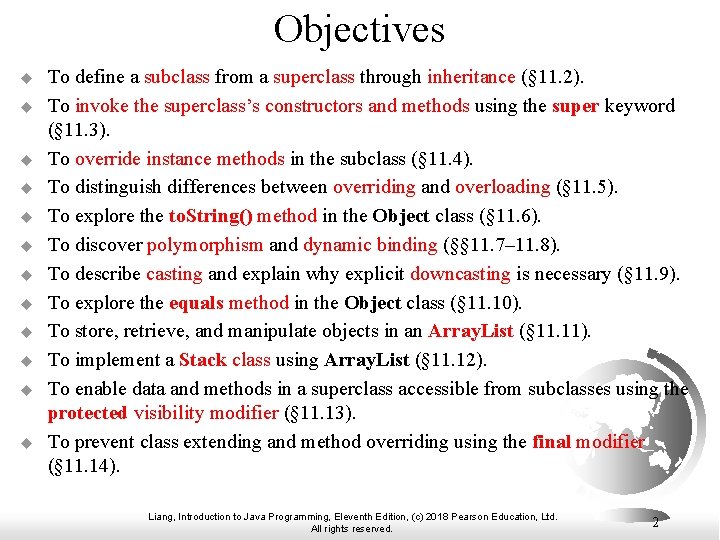
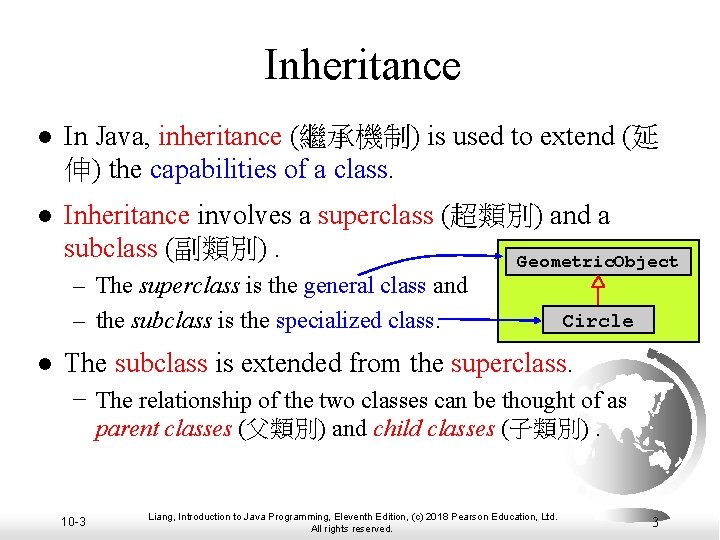
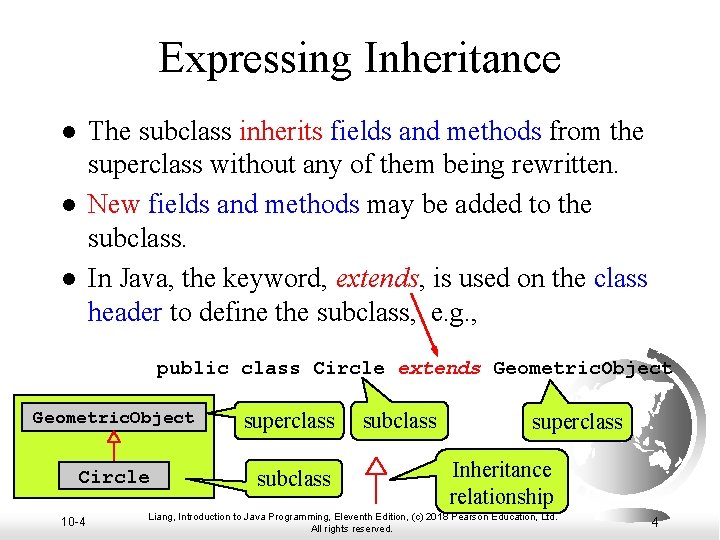
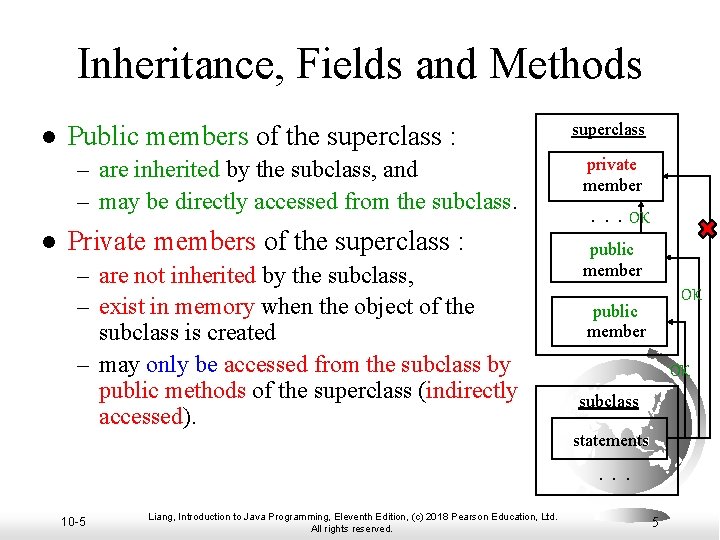
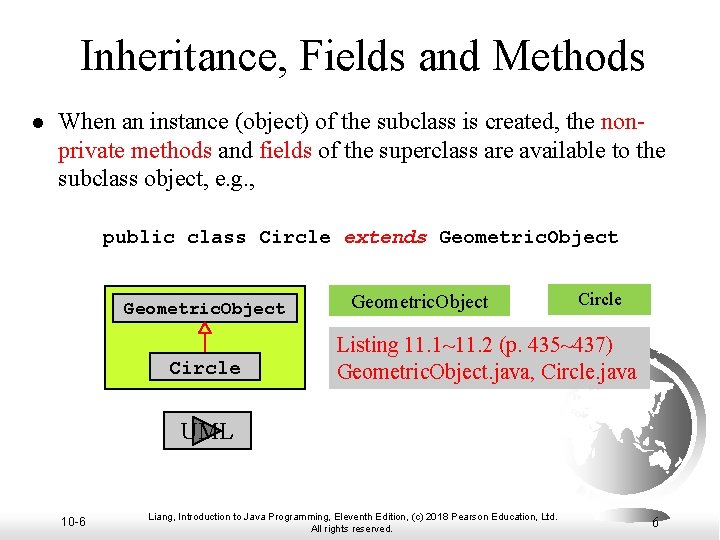
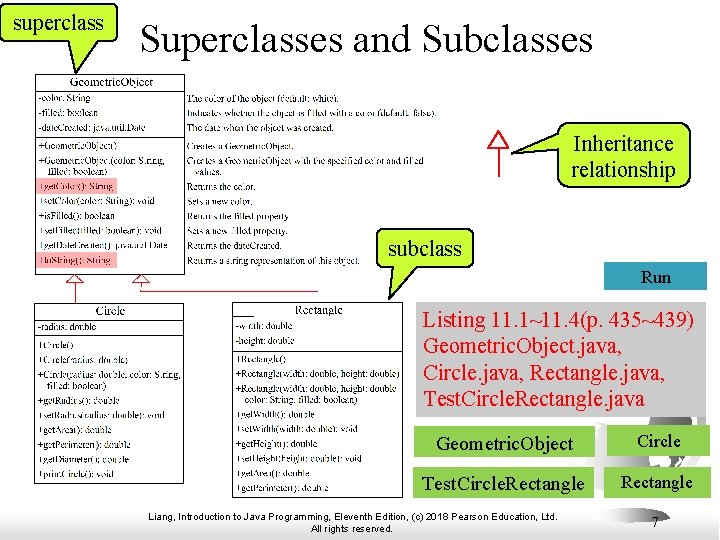
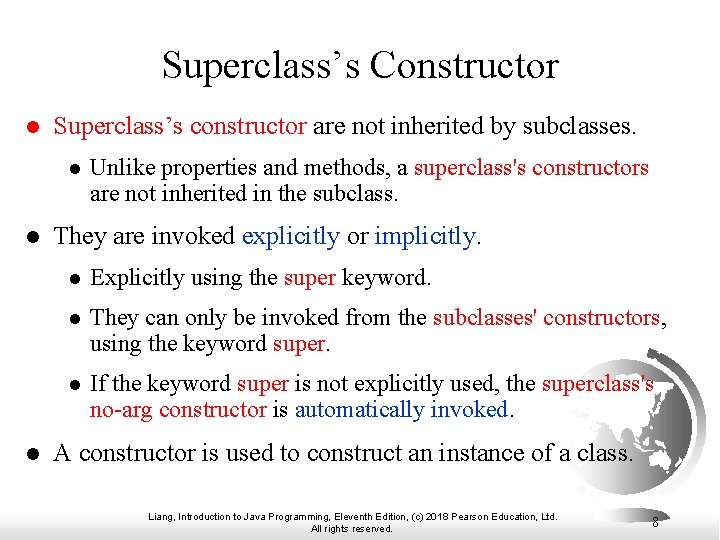
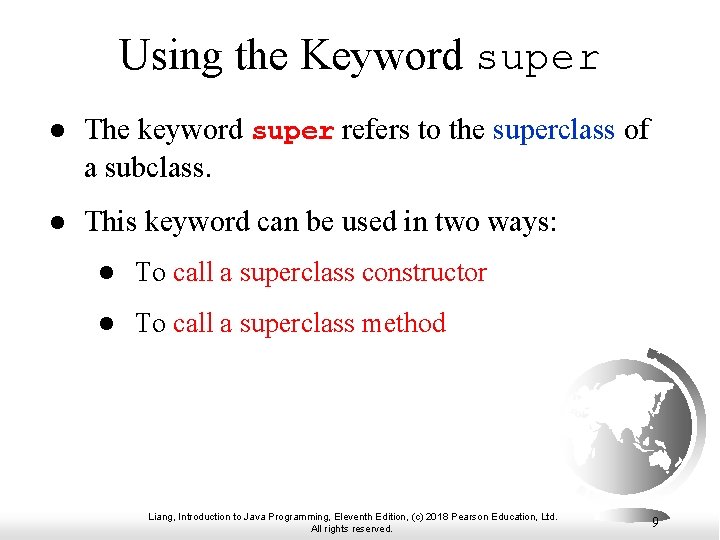
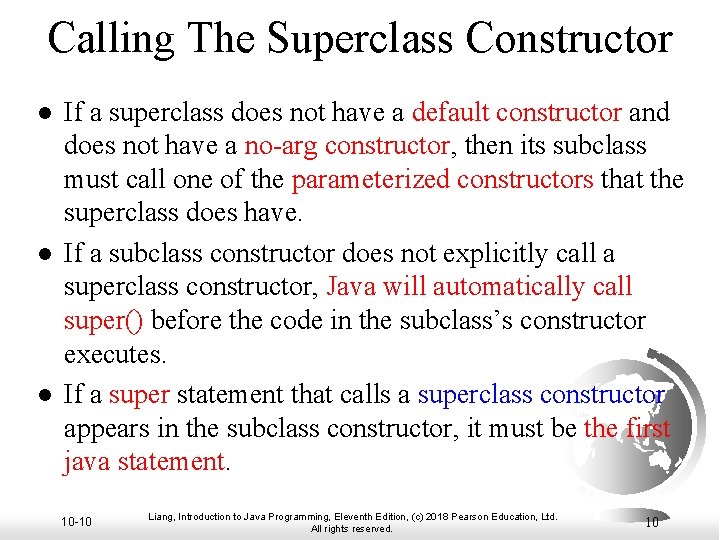
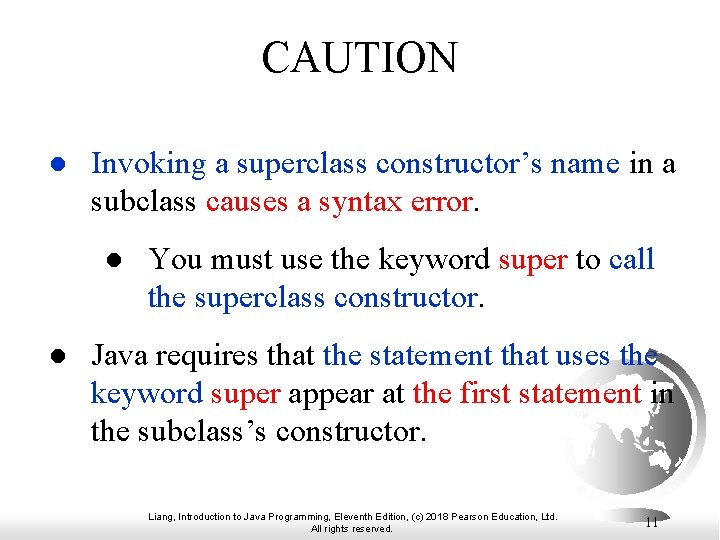
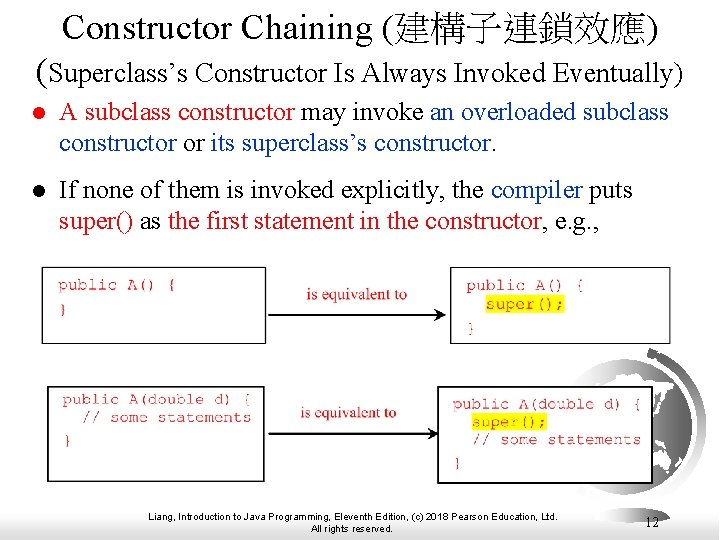
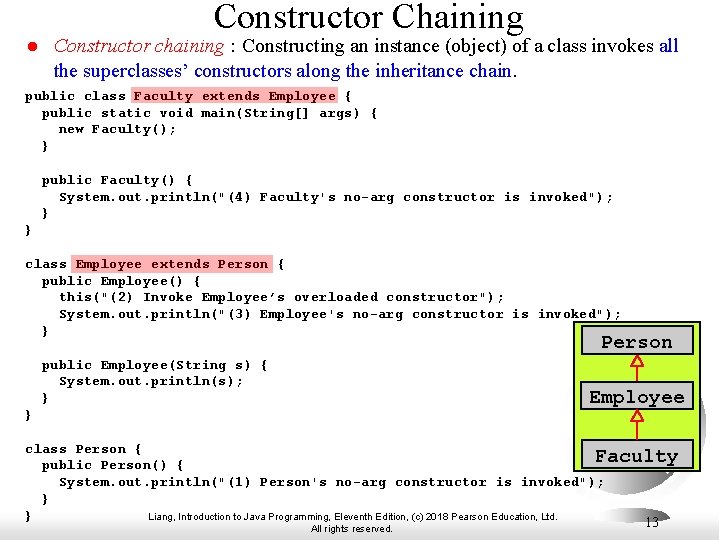
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-14.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-15.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-16.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-17.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-18.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-19.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-20.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-21.jpg)
![animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-22.jpg)
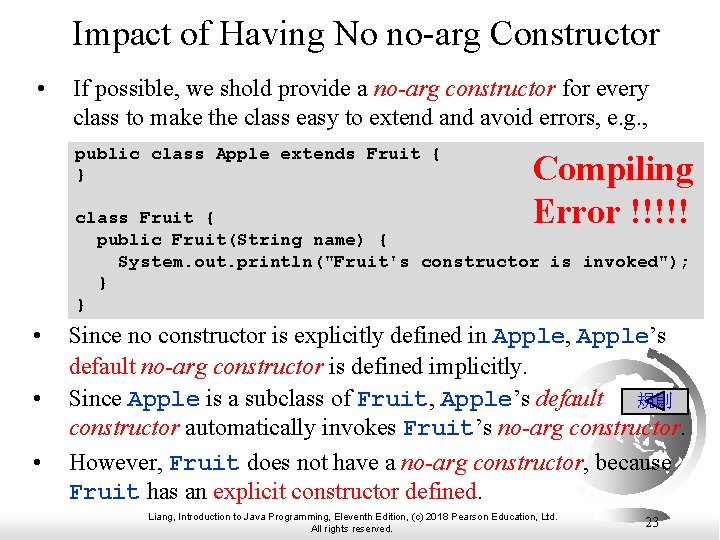
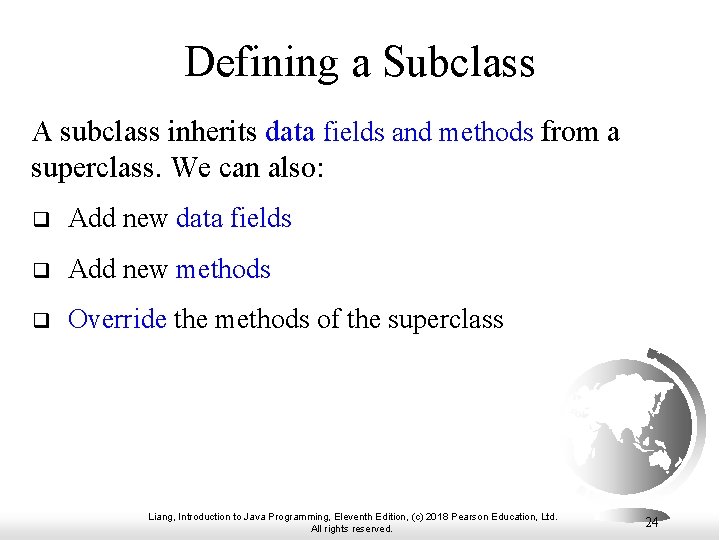
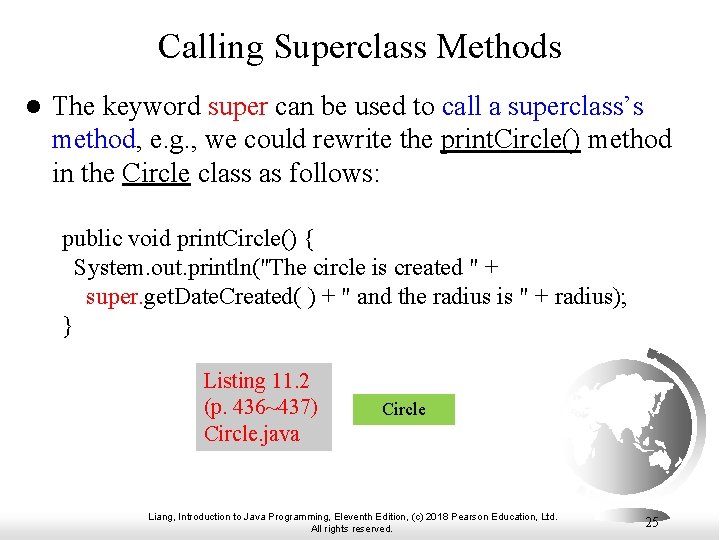
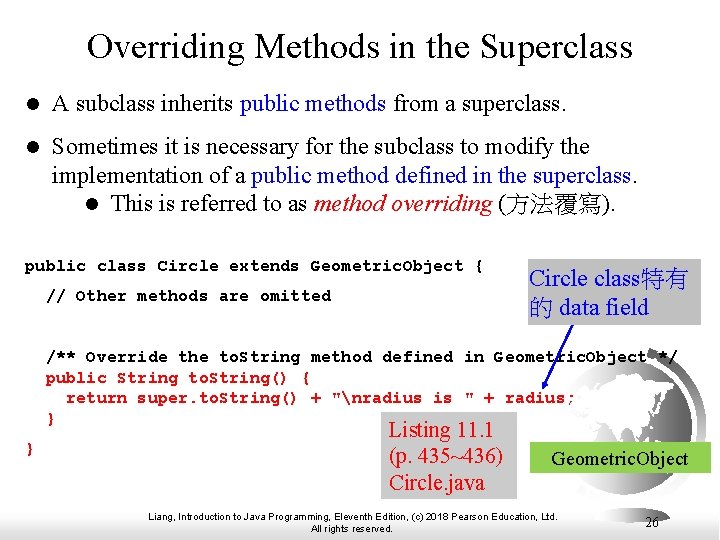
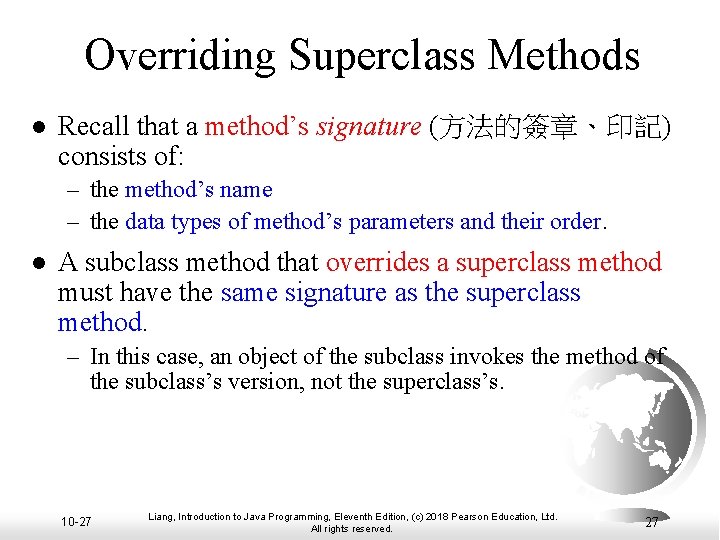
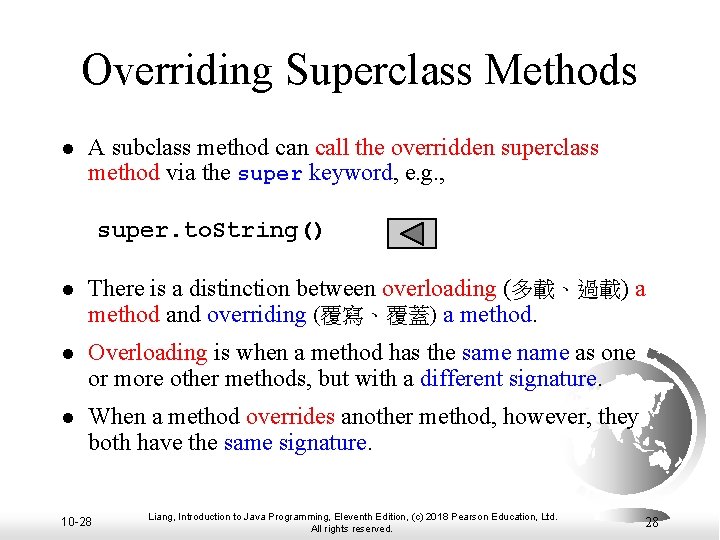
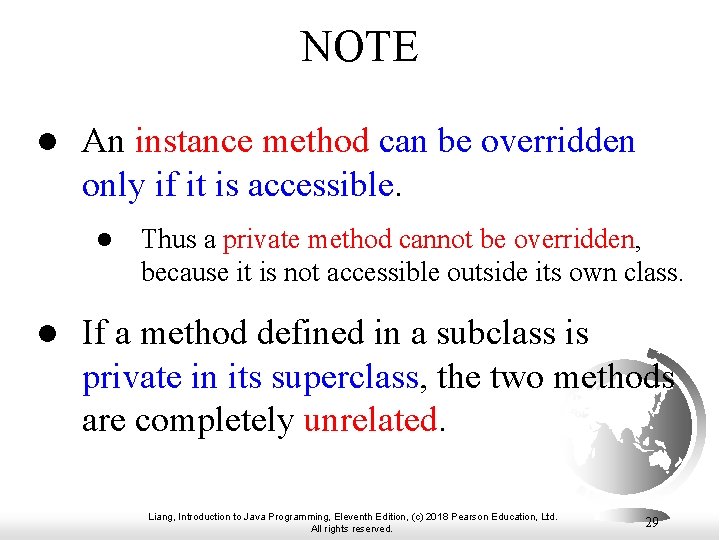
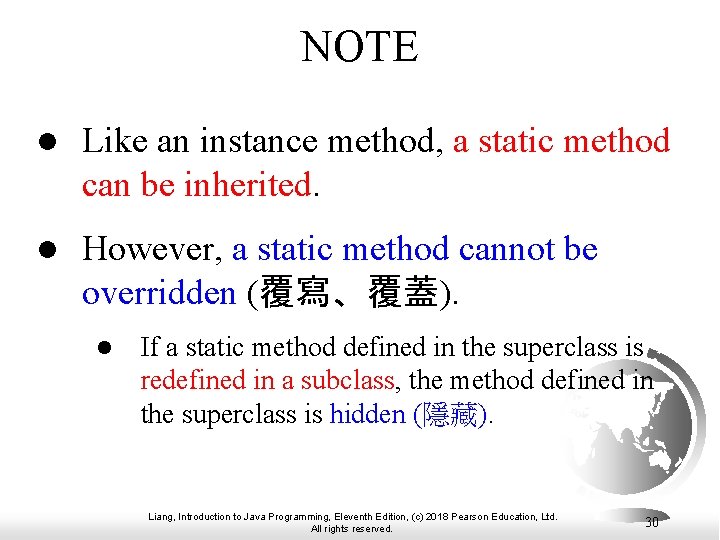
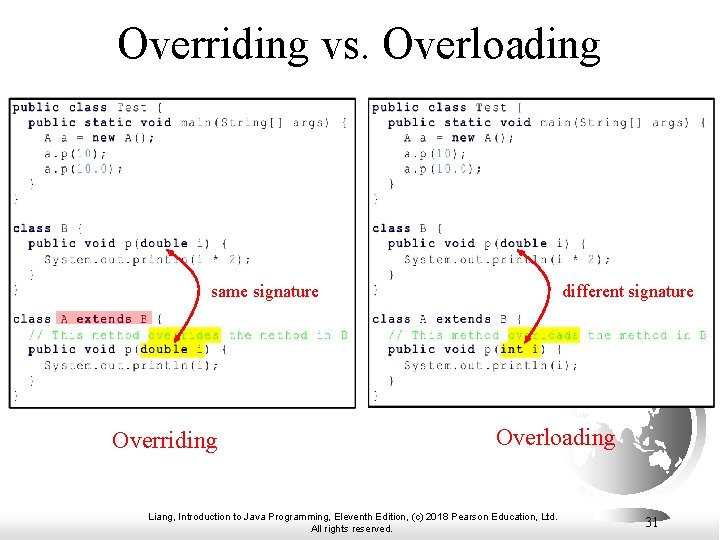
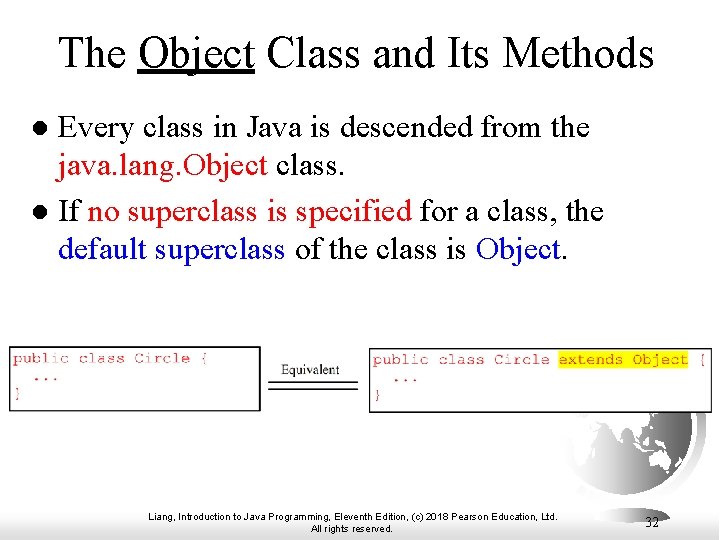
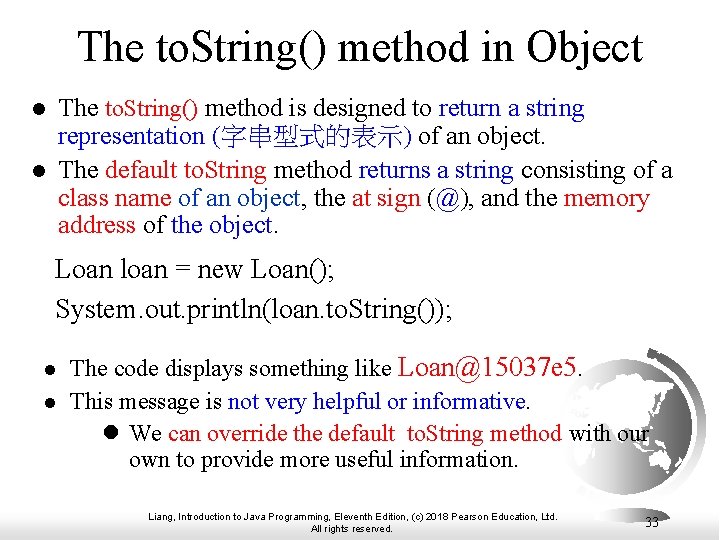
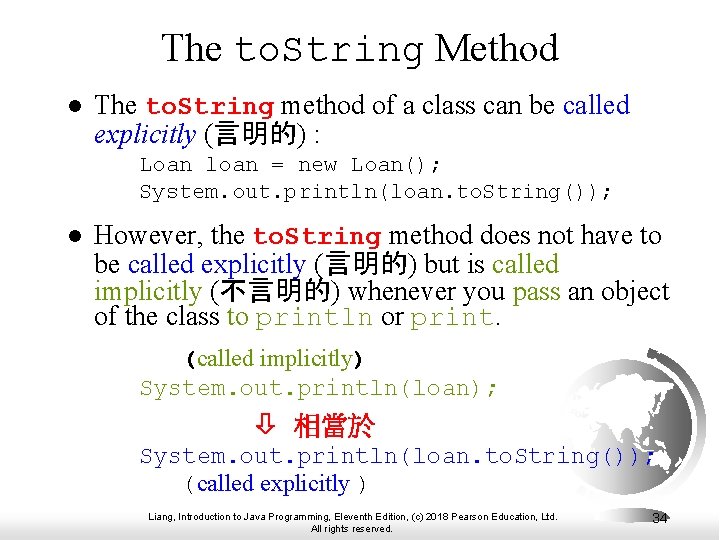
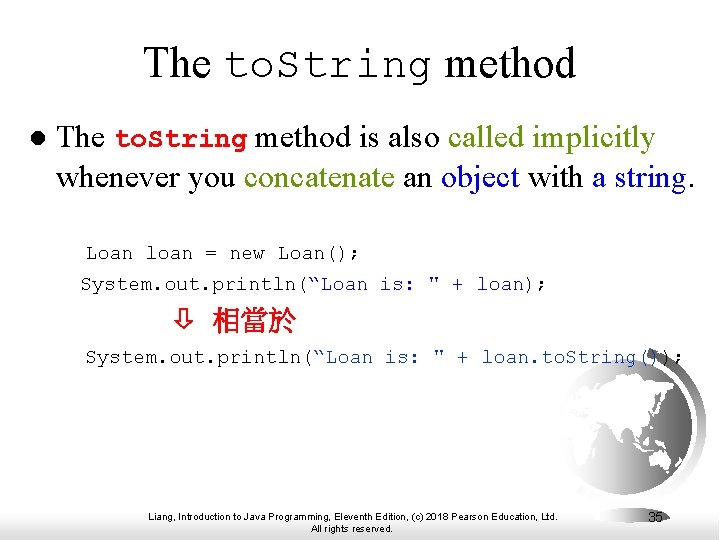
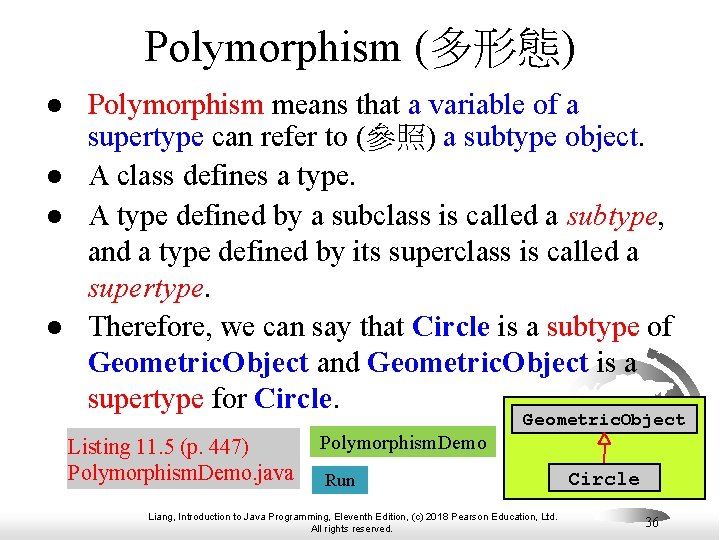
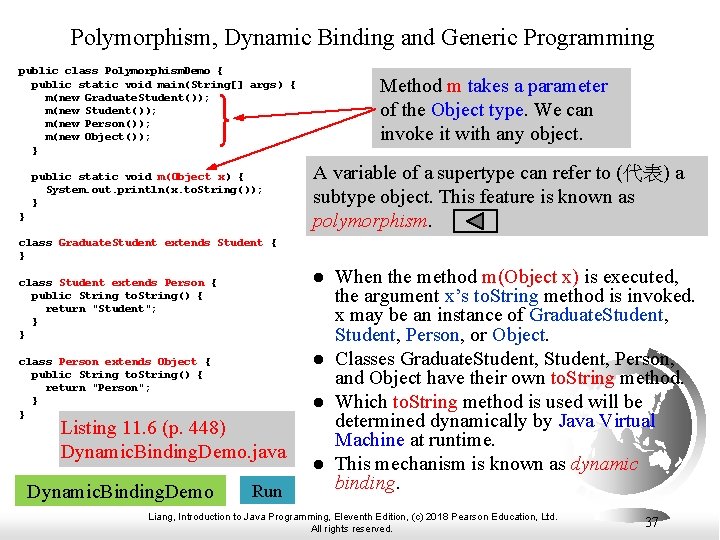
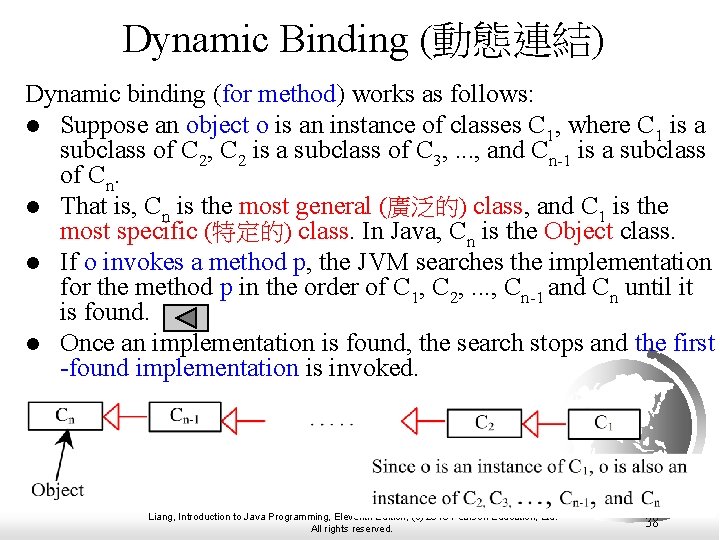
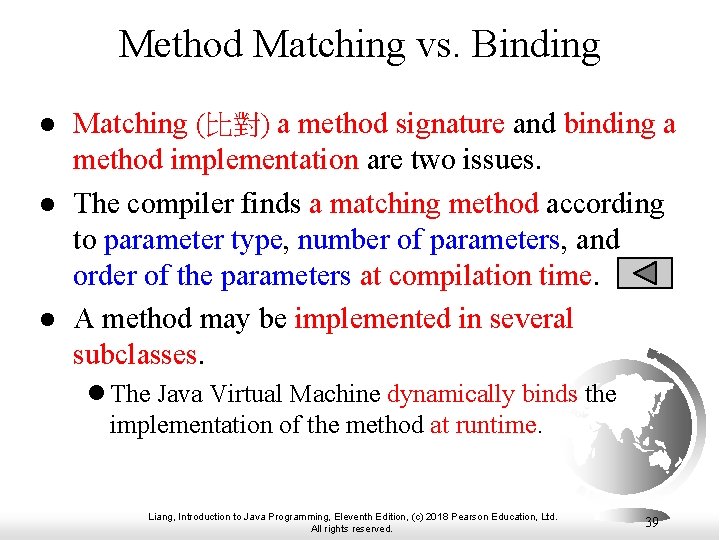
![Generic Programming (泛用的程式設計方式) public class Polymorphism. Demo { public static void main(String[] args) { Generic Programming (泛用的程式設計方式) public class Polymorphism. Demo { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-40.jpg)
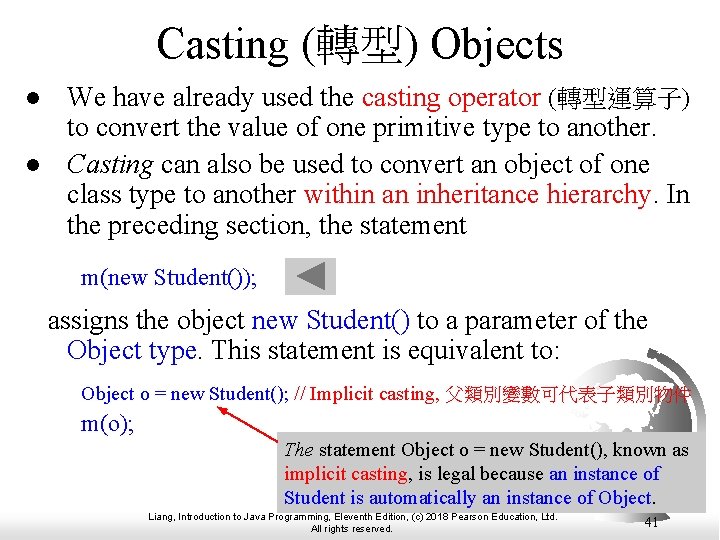
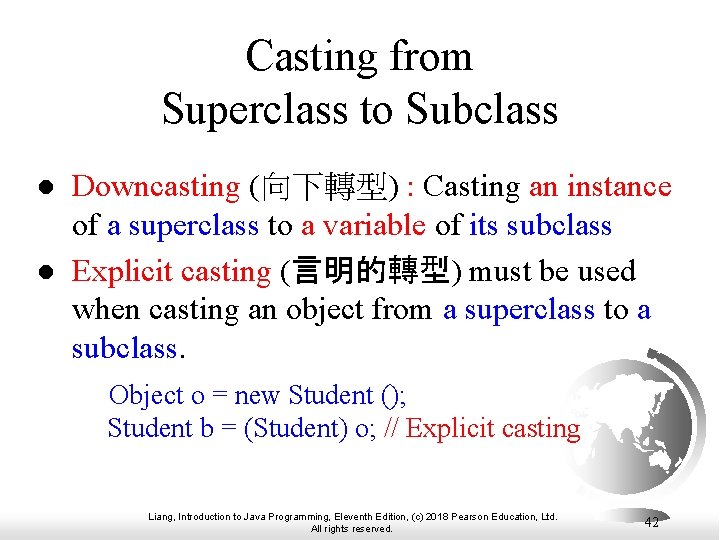
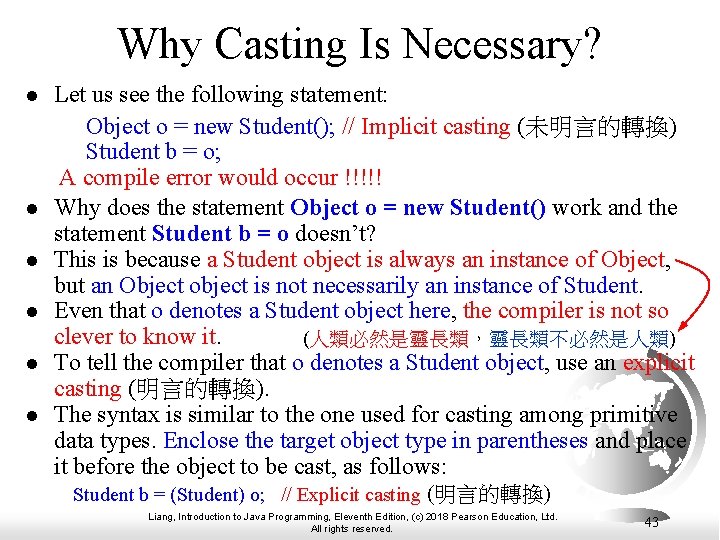
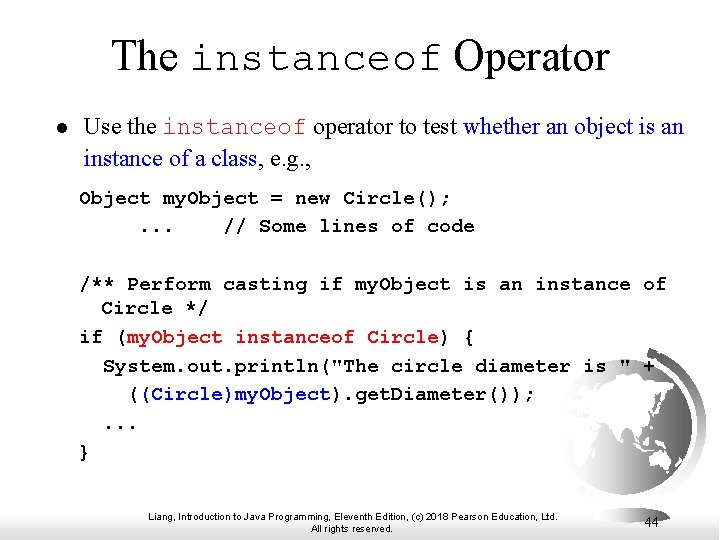
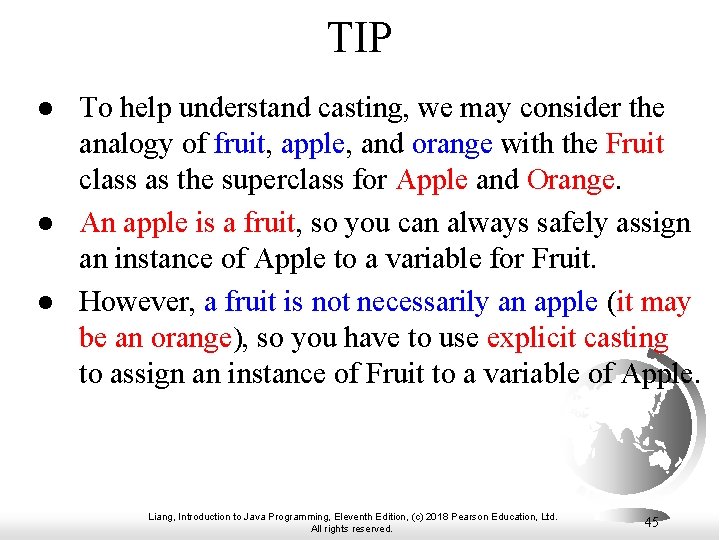
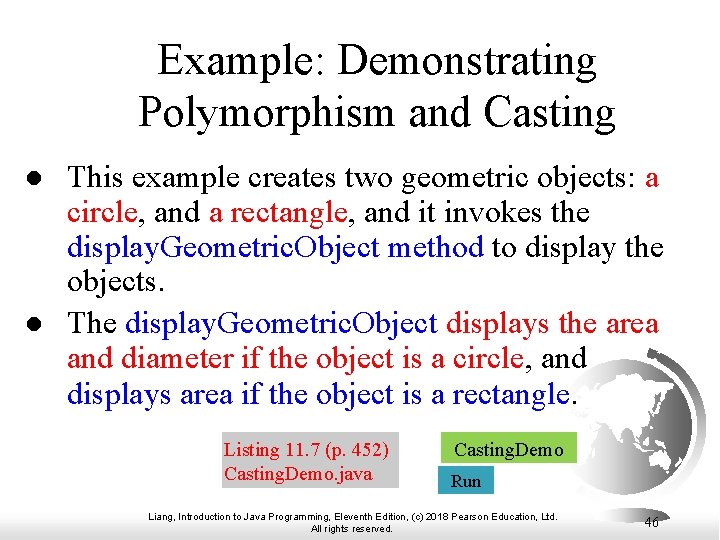
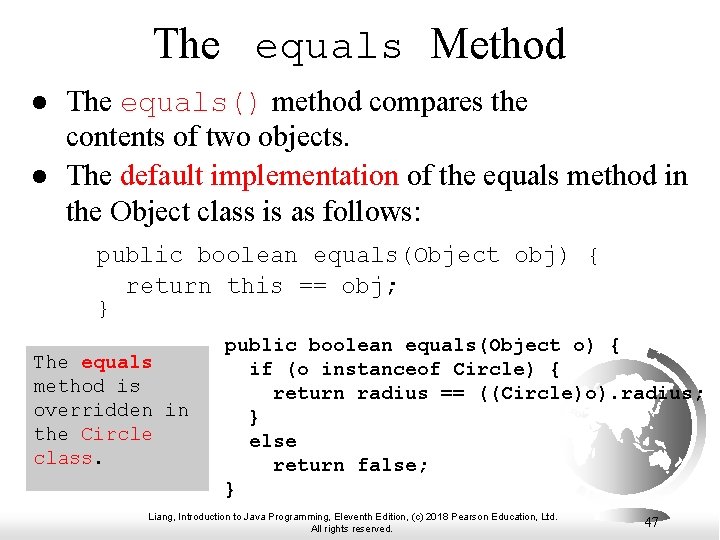
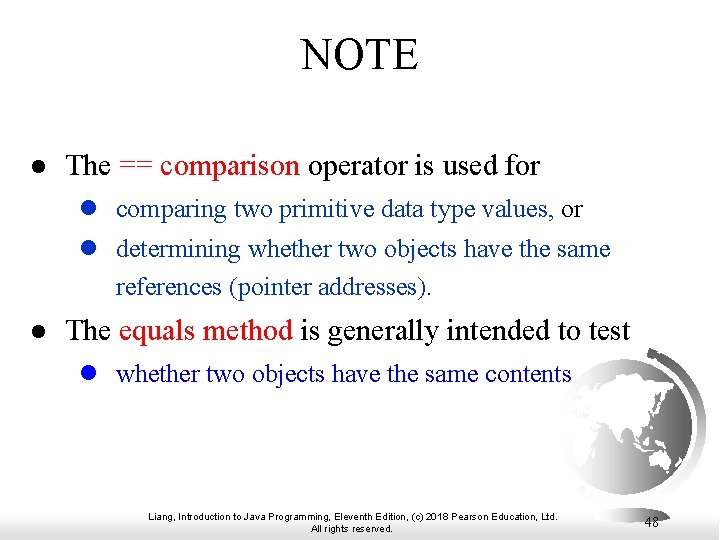
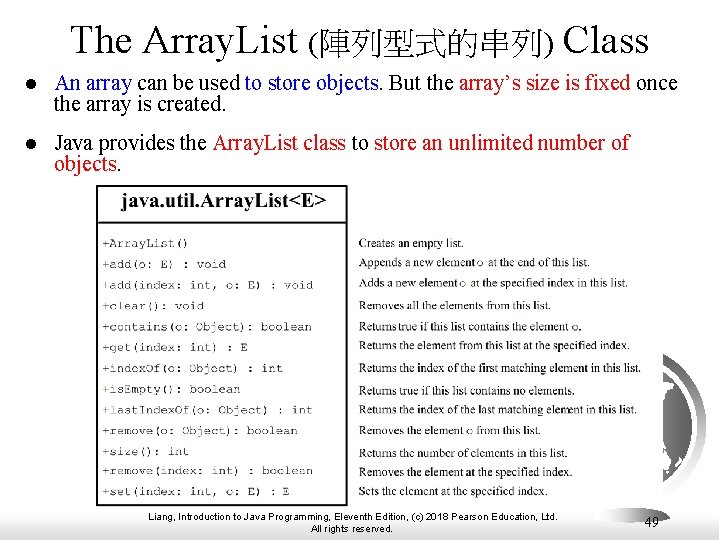
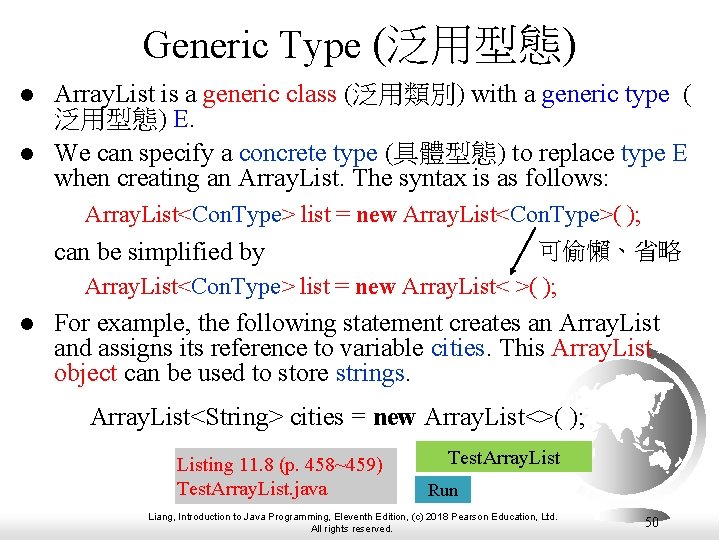
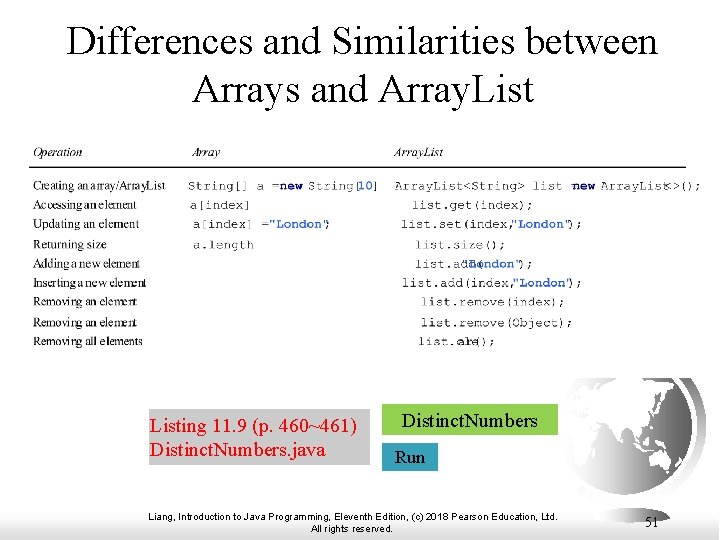
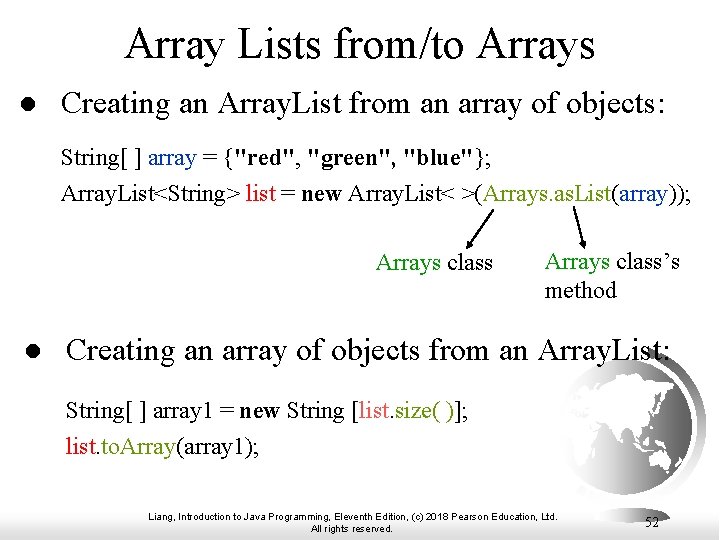
![max and min in an Array List Integer[ ] array = {3, 5, 95, max and min in an Array List Integer[ ] array = {3, 5, 95,](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-53.jpg)
![Shuffling (洗牌) an Array List Integer[ ] array = {3, 5, 95, 4, 15, Shuffling (洗牌) an Array List Integer[ ] array = {3, 5, 95, 4, 15,](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-54.jpg)
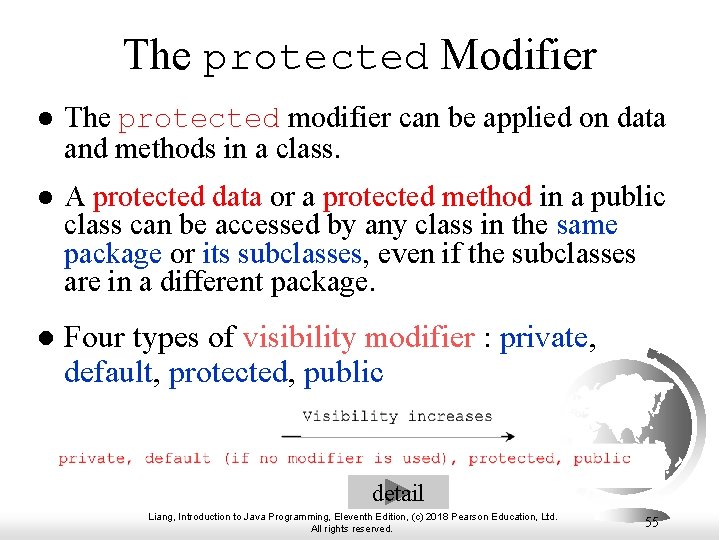
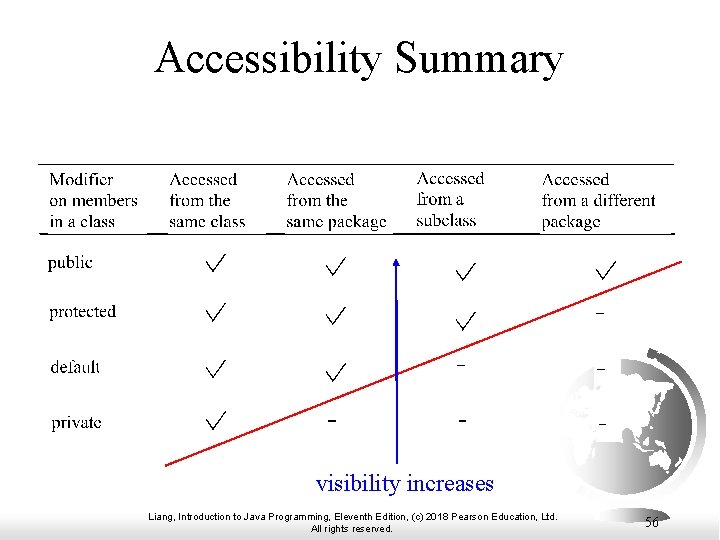
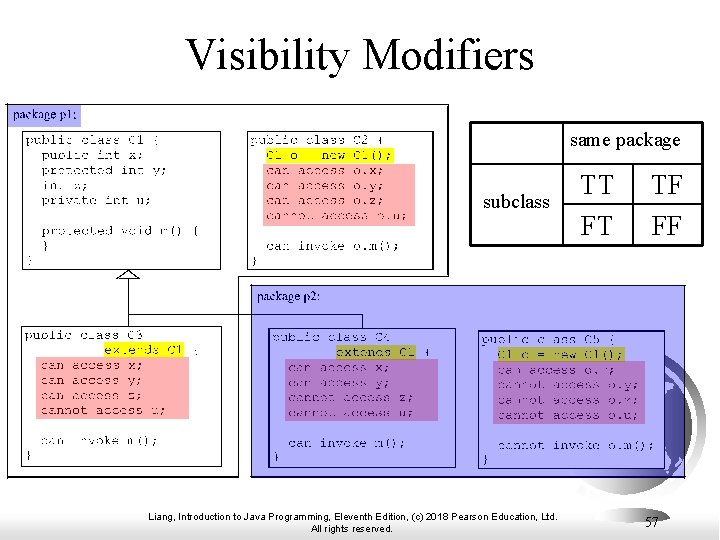
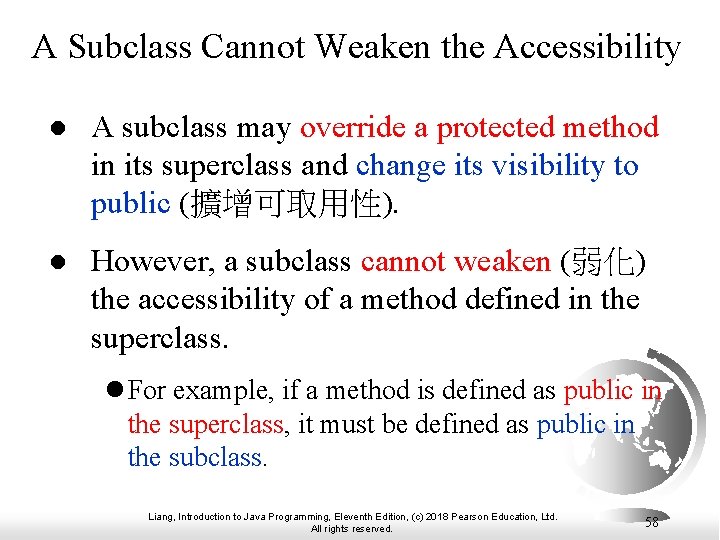
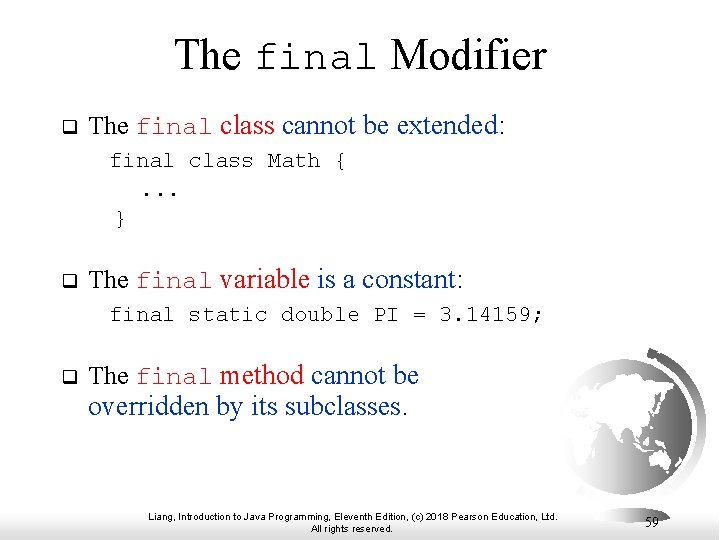
- Slides: 59
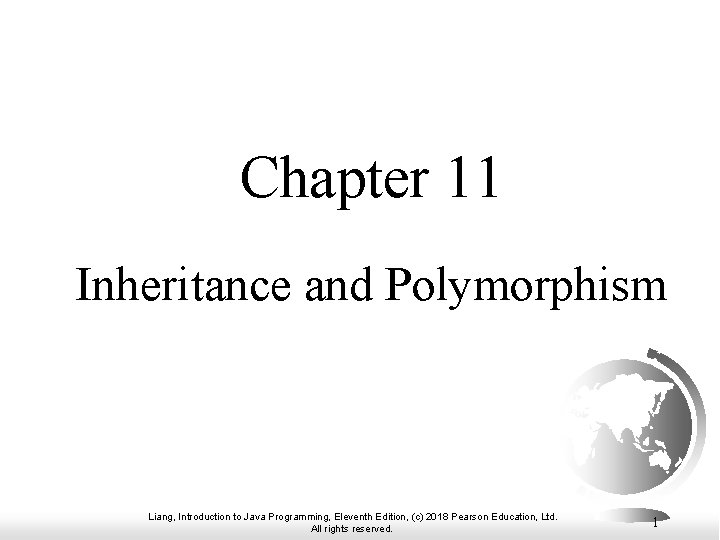
Chapter 11 Inheritance and Polymorphism Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 1
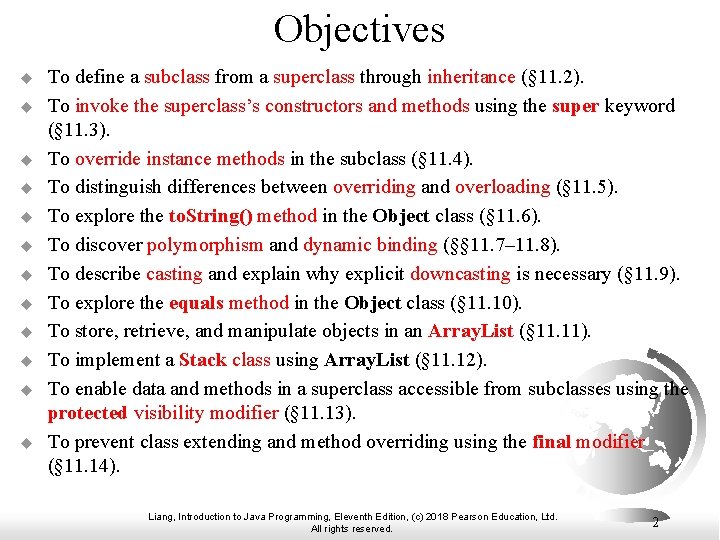
Objectives u u u To define a subclass from a superclass through inheritance (§ 11. 2). To invoke the superclass’s constructors and methods using the super keyword (§ 11. 3). To override instance methods in the subclass (§ 11. 4). To distinguish differences between overriding and overloading (§ 11. 5). To explore the to. String() method in the Object class (§ 11. 6). To discover polymorphism and dynamic binding (§§ 11. 7– 11. 8). To describe casting and explain why explicit downcasting is necessary (§ 11. 9). To explore the equals method in the Object class (§ 11. 10). To store, retrieve, and manipulate objects in an Array. List (§ 11. 11). To implement a Stack class using Array. List (§ 11. 12). To enable data and methods in a superclass accessible from subclasses using the protected visibility modifier (§ 11. 13). To prevent class extending and method overriding using the final modifier (§ 11. 14). Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 2
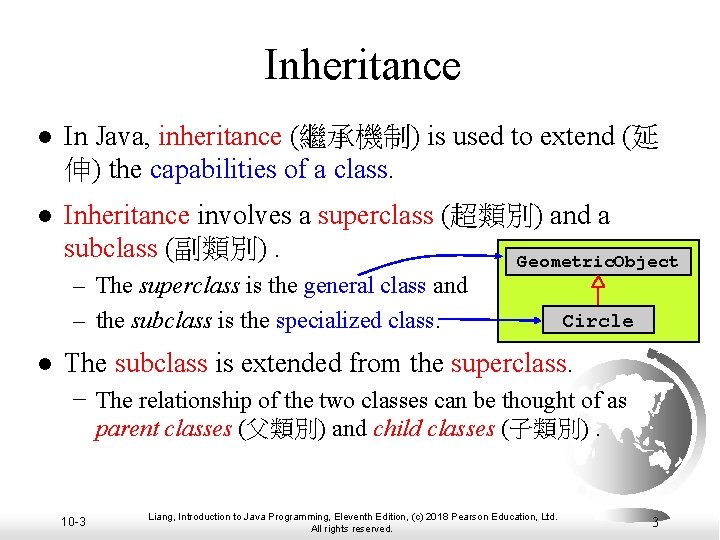
Inheritance l In Java, inheritance (繼承機制) is used to extend (延 伸) the capabilities of a class. l Inheritance involves a superclass (超類別) and a subclass (副類別). Geometric. Object – The superclass is the general class and – the subclass is the specialized class. l Circle The subclass is extended from the superclass. − The relationship of the two classes can be thought of as parent classes (父類別) and child classes (子類別). 10 -3 Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 3
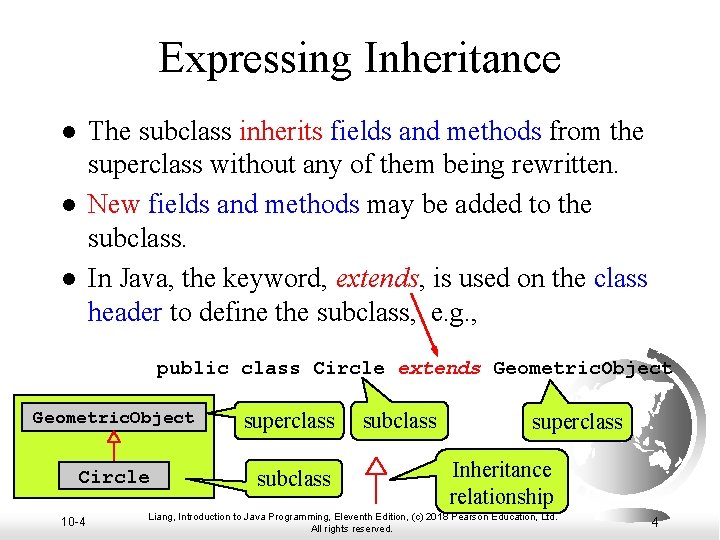
Expressing Inheritance The subclass inherits fields and methods from the superclass without any of them being rewritten. New fields and methods may be added to the subclass. In Java, the keyword, extends, is used on the class header to define the subclass, e. g. , l l l public class Circle extends Geometric. Object superclass Circle subclass 10 -4 subclass superclass Inheritance relationship Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 4
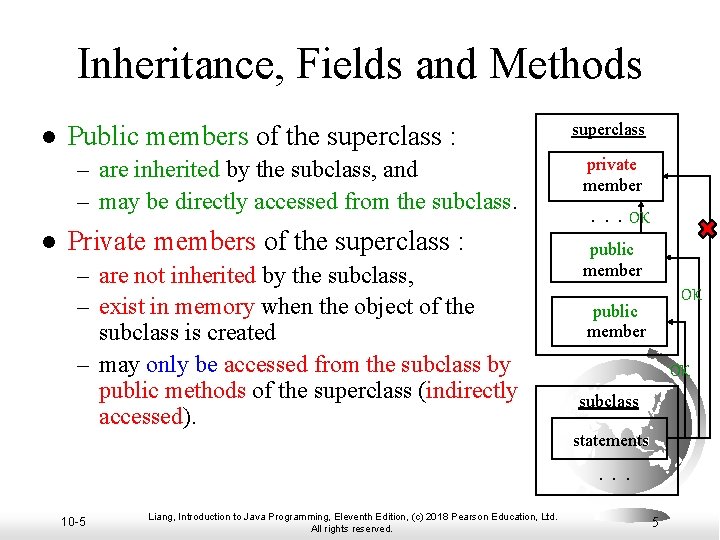
Inheritance, Fields and Methods l Public members of the superclass : – are inherited by the subclass, and – may be directly accessed from the subclass. l Private members of the superclass : – are not inherited by the subclass, – exist in memory when the object of the subclass is created – may only be accessed from the subclass by public methods of the superclass (indirectly accessed). superclass private member. . . OK public member OK subclass statements. . . 10 -5 Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 5
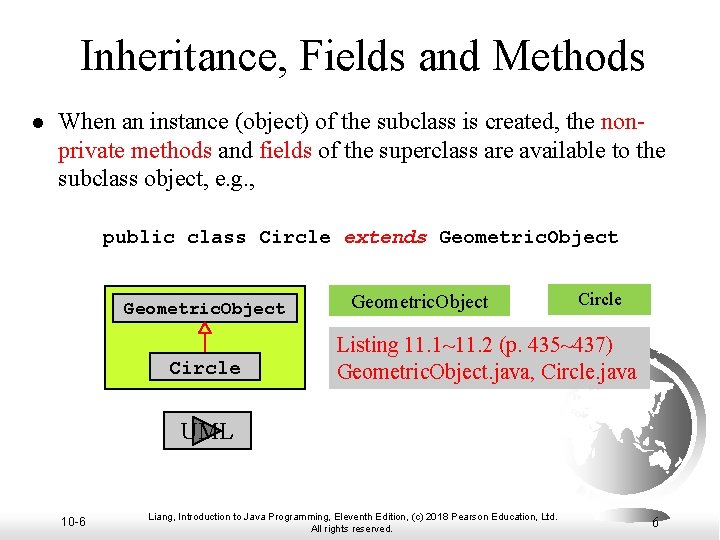
Inheritance, Fields and Methods l When an instance (object) of the subclass is created, the nonprivate methods and fields of the superclass are available to the subclass object, e. g. , public class Circle extends Geometric. Object Circle Listing 11. 1~11. 2 (p. 435~437) Geometric. Object. java, Circle. java UML 10 -6 Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 6
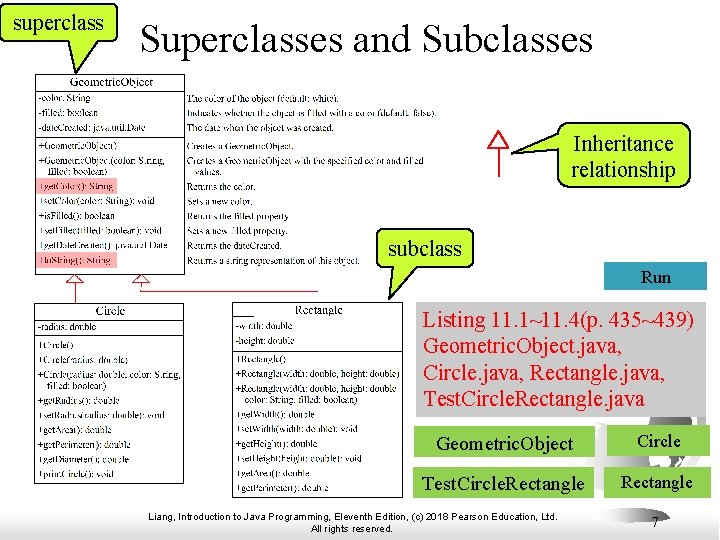
superclass Superclasses and Subclasses Inheritance relationship subclass Run Listing 11. 1~11. 4(p. 435~439) Geometric. Object. java, Circle. java, Rectangle. java, Test. Circle. Rectangle. java Geometric. Object Circle Test. Circle. Rectangle Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 7
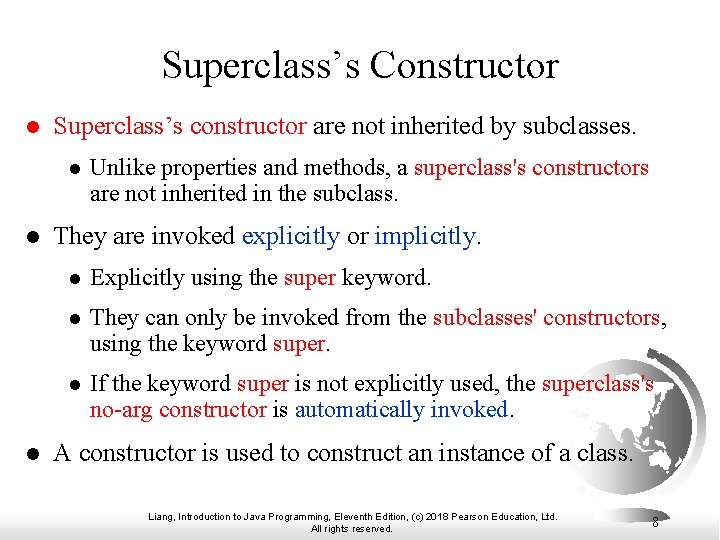
Superclass’s Constructor l Superclass’s constructor are not inherited by subclasses. l l l Unlike properties and methods, a superclass's constructors are not inherited in the subclass. They are invoked explicitly or implicitly. l Explicitly using the super keyword. l They can only be invoked from the subclasses' constructors, using the keyword super. l If the keyword super is not explicitly used, the superclass's no-arg constructor is automatically invoked. A constructor is used to construct an instance of a class. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 8
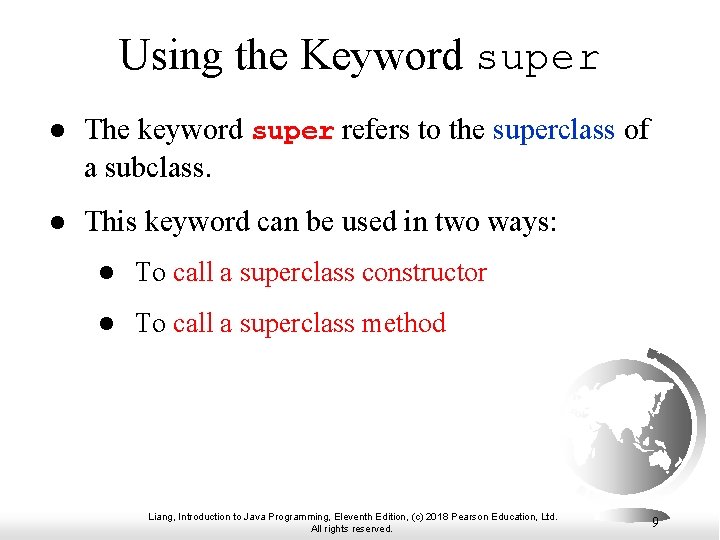
Using the Keyword super l The keyword super refers to the superclass of a subclass. l This keyword can be used in two ways: l To call a superclass constructor l To call a superclass method Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 9
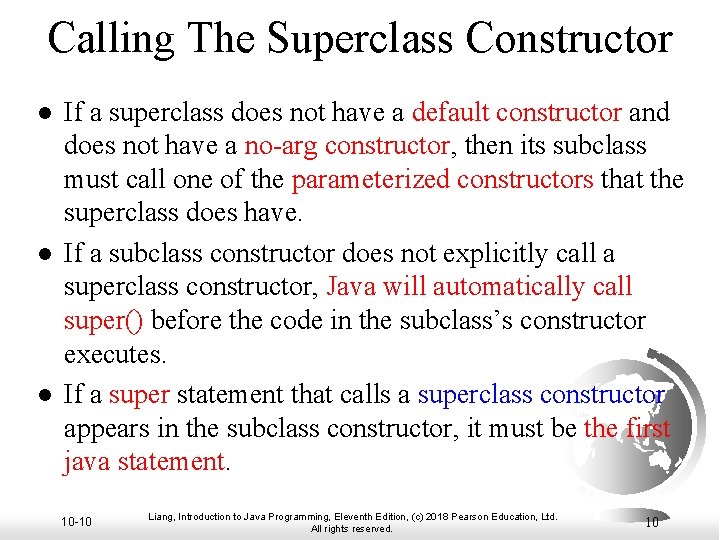
Calling The Superclass Constructor l l l If a superclass does not have a default constructor and does not have a no-arg constructor, then its subclass must call one of the parameterized constructors that the superclass does have. If a subclass constructor does not explicitly call a superclass constructor, Java will automatically call super() before the code in the subclass’s constructor executes. If a super statement that calls a superclass constructor appears in the subclass constructor, it must be the first java statement. 10 -10 Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 10
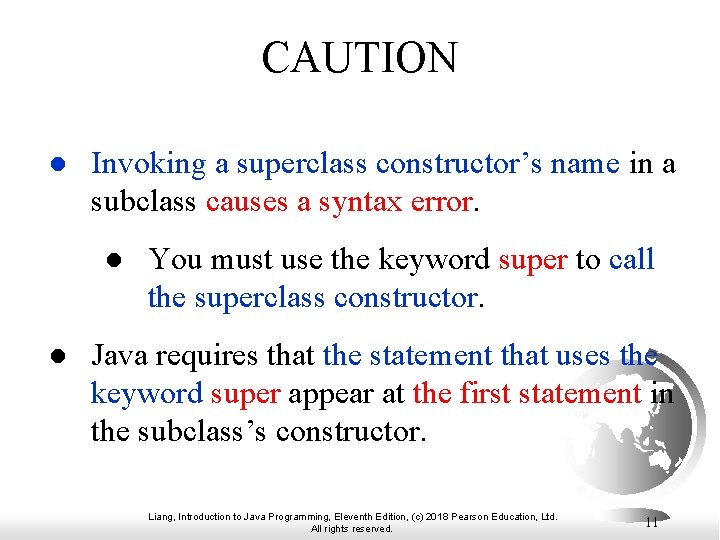
CAUTION l Invoking a superclass constructor’s name in a subclass causes a syntax error. l l You must use the keyword super to call the superclass constructor. Java requires that the statement that uses the keyword super appear at the first statement in the subclass’s constructor. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 11
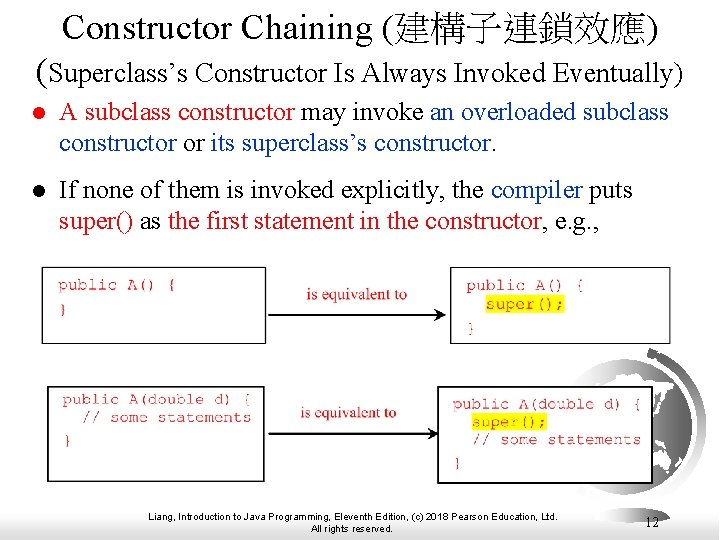
Constructor Chaining (建構子連鎖效應) (Superclass’s Constructor Is Always Invoked Eventually) l A subclass constructor may invoke an overloaded subclass constructor or its superclass’s constructor. l If none of them is invoked explicitly, the compiler puts super() as the first statement in the constructor, e. g. , Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 12
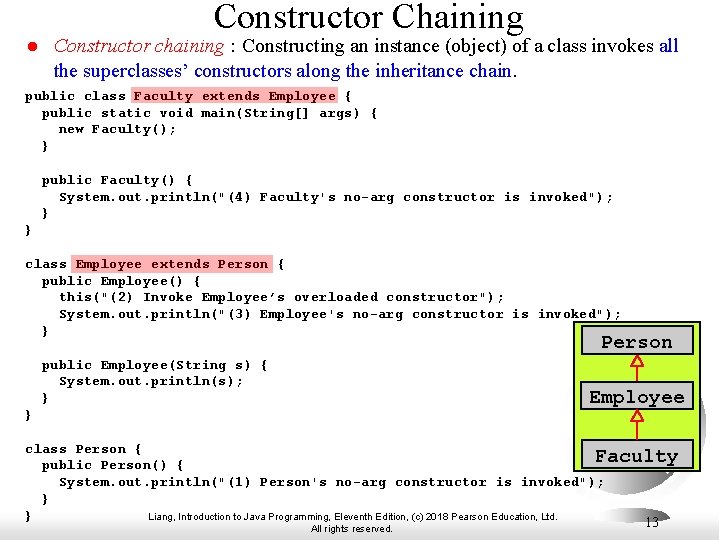
Constructor Chaining l Constructor chaining : Constructing an instance (object) of a class invokes all the superclasses’ constructors along the inheritance chain. public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } Person public Employee(String s) { System. out. println(s); } } Employee class Person { Faculty public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. 13 All rights reserved.
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-14.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } 1. Start from the main method public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 14
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-15.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } 2. Invoke Faculty constructor public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 15
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-16.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } Person Employee Faculty public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } 3. Invoke Employee’s noarg constructor } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } Employee() Faculty( ) main( ) class Person { public Person() { call stack System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 16
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-17.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } 解說 : A constructor may invoke an overloaded constructor or its superclass’s constructor. public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } 4. 1 Invoke Employee(String) constructor class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } 4. 2 Invoke Employee(String) constructor } class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 17
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-18.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } Person Employee Faculty public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } 5. Invoke Person() constructor class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 18
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-19.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } Person( ) Employee( String s) Employee( ) Faculty( ) main( ) call stack public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } 6. Execute println class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 19
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-20.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } Employee( String s) Employee( ) Faculty( ) main( ) call stack public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } 7. Execute println class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 20
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-21.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } Employee() Faculty( ) main( ) call stack public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } 8. Execute println class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 21
![animation Trace Execution public class Faculty extends Employee public static void mainString args animation Trace Execution public class Faculty extends Employee { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-22.jpg)
animation Trace Execution public class Faculty extends Employee { public static void main(String[] args) { new Faculty(); } Faculty() main( ) call stack public Faculty() { System. out. println("(4) Faculty's no-arg constructor is invoked"); } } 9. Execute println class Employee extends Person { public Employee() { this("(2) Invoke Employee’s overloaded constructor"); System. out. println("(3) Employee's no-arg constructor is invoked"); } public Employee(String s) { System. out. println(s); } } class Person { public Person() { System. out. println("(1) Person's no-arg constructor is invoked"); } } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 22
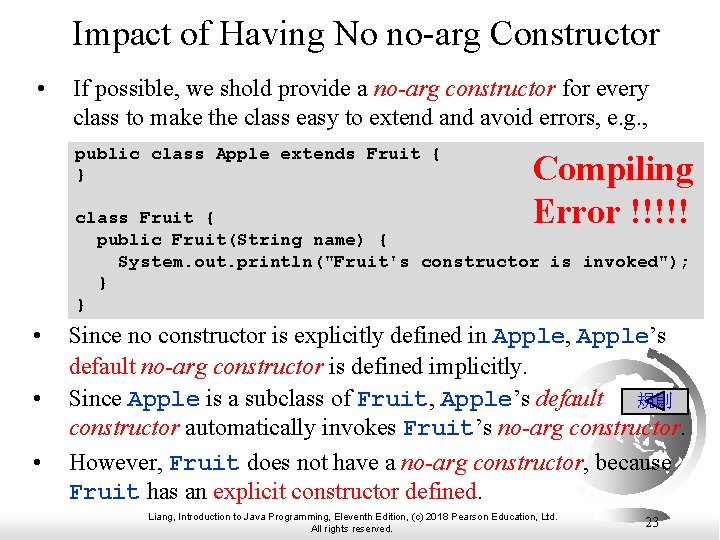
Impact of Having No no-arg Constructor • If possible, we shold provide a no-arg constructor for every class to make the class easy to extend avoid errors, e. g. , public class Apple extends Fruit { } Compiling Error !!!!! class Fruit { public Fruit(String name) { System. out. println("Fruit's constructor is invoked"); } } • • • Since no constructor is explicitly defined in Apple, Apple’s default no-arg constructor is defined implicitly. Since Apple is a subclass of Fruit, Apple’s default 規則 constructor automatically invokes Fruit’s no-arg constructor. However, Fruit does not have a no-arg constructor, because Fruit has an explicit constructor defined. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 23
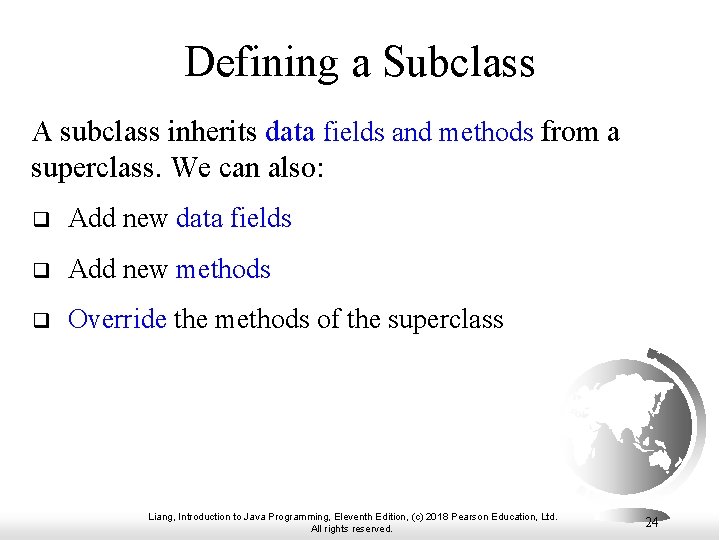
Defining a Subclass A subclass inherits data fields and methods from a superclass. We can also: q Add new data fields q Add new methods q Override the methods of the superclass Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 24
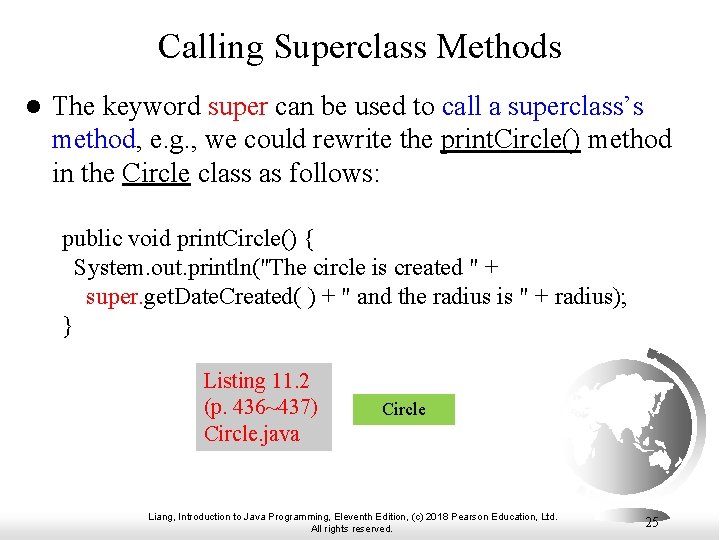
Calling Superclass Methods l The keyword super can be used to call a superclass’s method, e. g. , we could rewrite the print. Circle() method in the Circle class as follows: public void print. Circle() { System. out. println("The circle is created " + super. get. Date. Created( ) + " and the radius is " + radius); } Listing 11. 2 (p. 436~437) Circle. java Circle Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 25
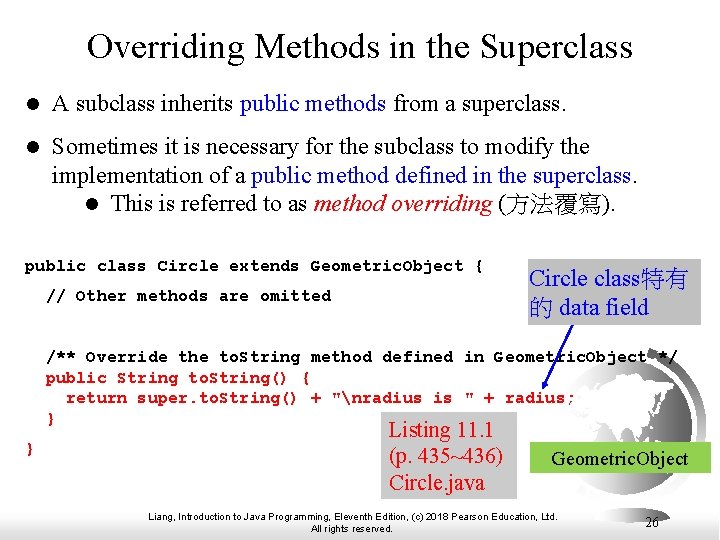
Overriding Methods in the Superclass l A subclass inherits public methods from a superclass. l Sometimes it is necessary for the subclass to modify the implementation of a public method defined in the superclass. l This is referred to as method overriding (方法覆寫). public class Circle extends Geometric. Object { // Other methods are omitted Circle class特有 的 data field /** Override the to. String method defined in Geometric. Object */ public String to. String() { return super. to. String() + "nradius is " + radius; } } Listing 11. 1 (p. 435~436) Circle. java Geometric. Object Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 26
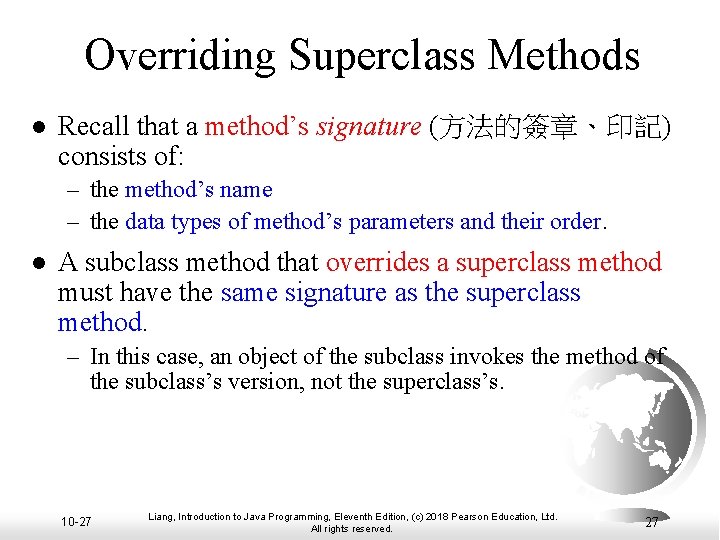
Overriding Superclass Methods l Recall that a method’s signature (方法的簽章、印記) consists of: – the method’s name – the data types of method’s parameters and their order. l A subclass method that overrides a superclass method must have the same signature as the superclass method. – In this case, an object of the subclass invokes the method of the subclass’s version, not the superclass’s. 10 -27 Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 27
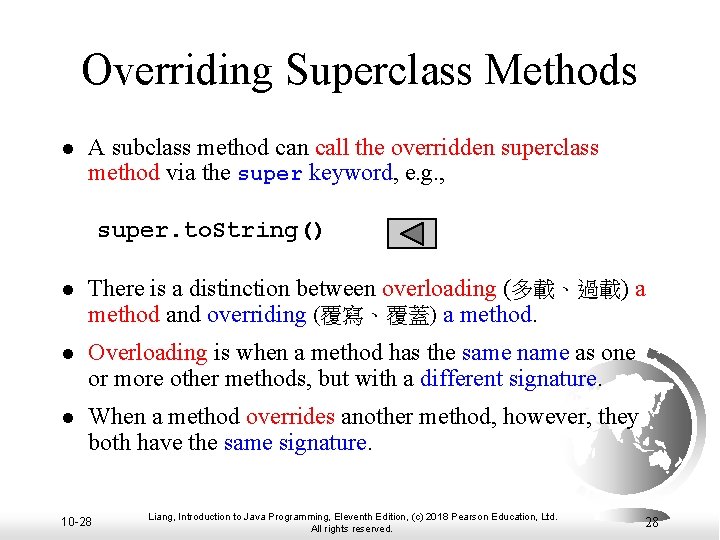
Overriding Superclass Methods l A subclass method can call the overridden superclass method via the super keyword, e. g. , super. to. String() l There is a distinction between overloading (多載、過載) a method and overriding (覆寫、覆蓋) a method. l Overloading is when a method has the same name as one or more other methods, but with a different signature. l When a method overrides another method, however, they both have the same signature. 10 -28 Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 28
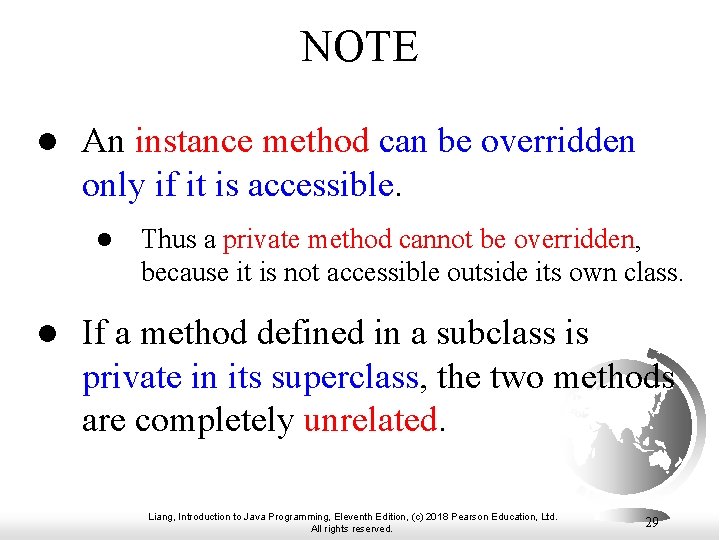
NOTE l An instance method can be overridden only if it is accessible. l l Thus a private method cannot be overridden, because it is not accessible outside its own class. If a method defined in a subclass is private in its superclass, the two methods are completely unrelated. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 29
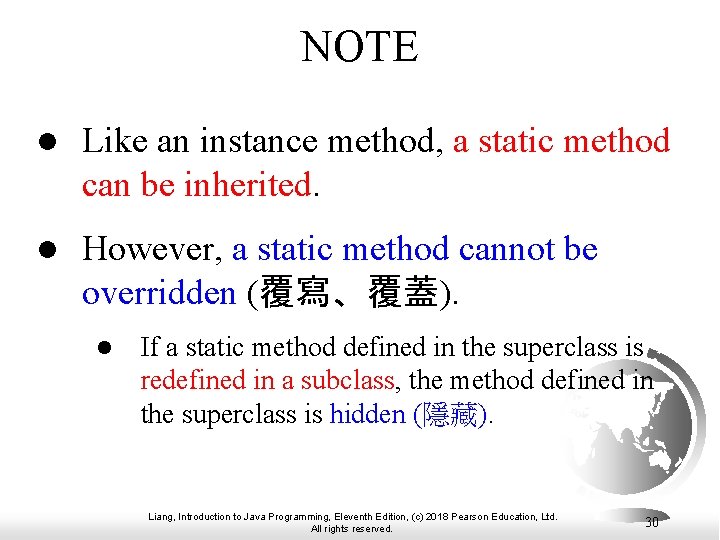
NOTE l Like an instance method, a static method can be inherited. l However, a static method cannot be overridden (覆寫、覆蓋). l If a static method defined in the superclass is redefined in a subclass, the method defined in the superclass is hidden (隱藏). Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 30
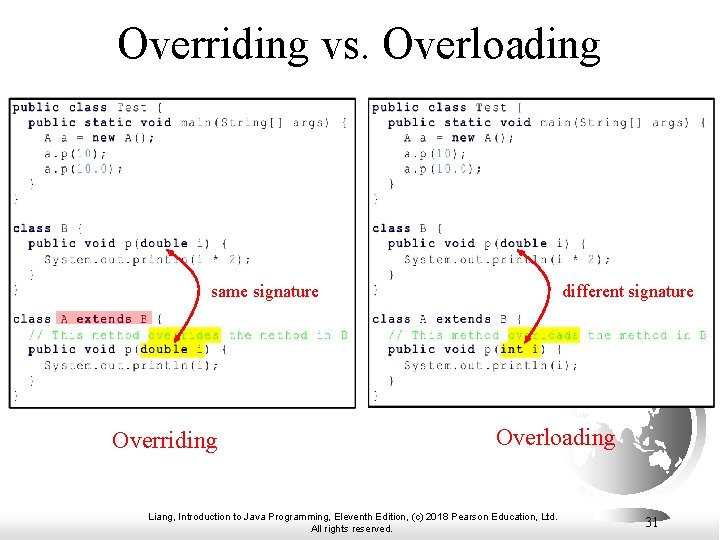
Overriding vs. Overloading same signature Overriding different signature Overloading Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 31
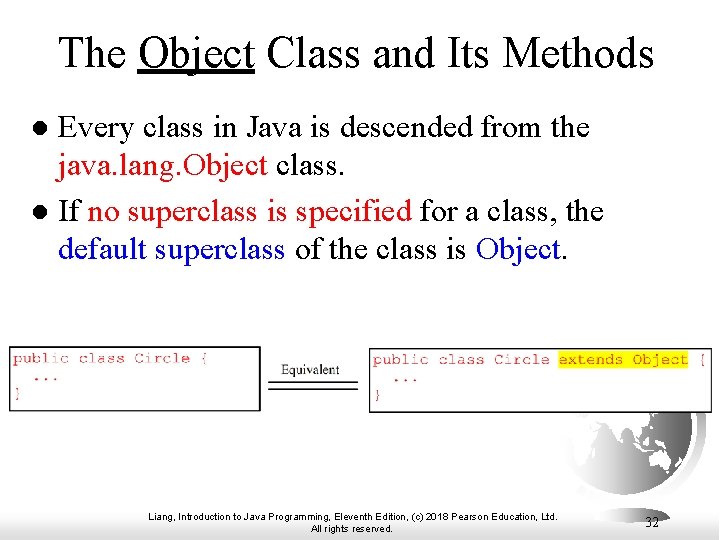
The Object Class and Its Methods Every class in Java is descended from the java. lang. Object class. l If no superclass is specified for a class, the default superclass of the class is Object. l Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 32
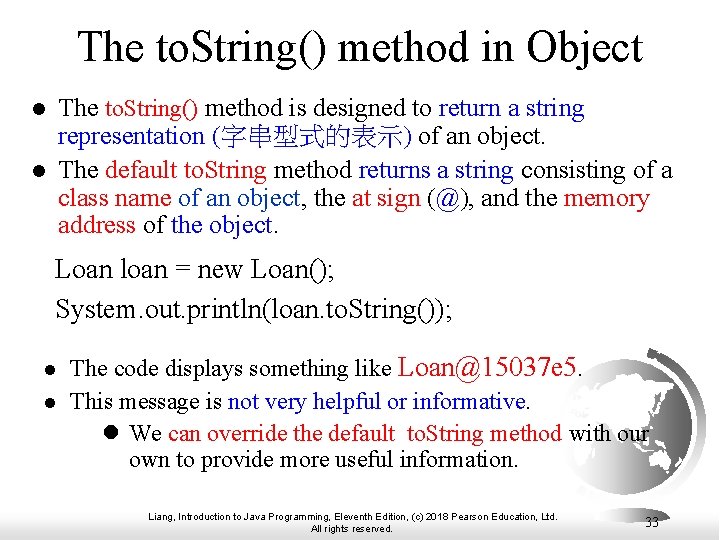
The to. String() method in Object The to. String() method is designed to return a string representation (字串型式的表示) of an object. The default to. String method returns a string consisting of a class name of an object, the at sign (@), and the memory address of the object. l l Loan loan = new Loan(); System. out. println(loan. to. String()); l l The code displays something like Loan@15037 e 5. This message is not very helpful or informative. l We can override the default to. String method with our own to provide more useful information. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 33
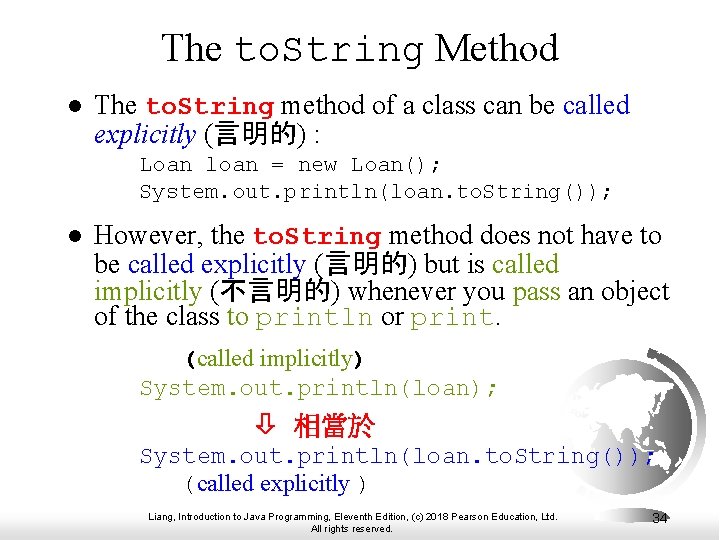
The to. String Method l The to. String method of a class can be called explicitly (言明的) : Loan loan = new Loan(); System. out. println(loan. to. String()); l However, the to. String method does not have to be called explicitly (言明的) but is called implicitly (不言明的) whenever you pass an object of the class to println or print. (called implicitly) System. out. println(loan); 相當於 System. out. println(loan. to. String()); (called explicitly ) Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 34
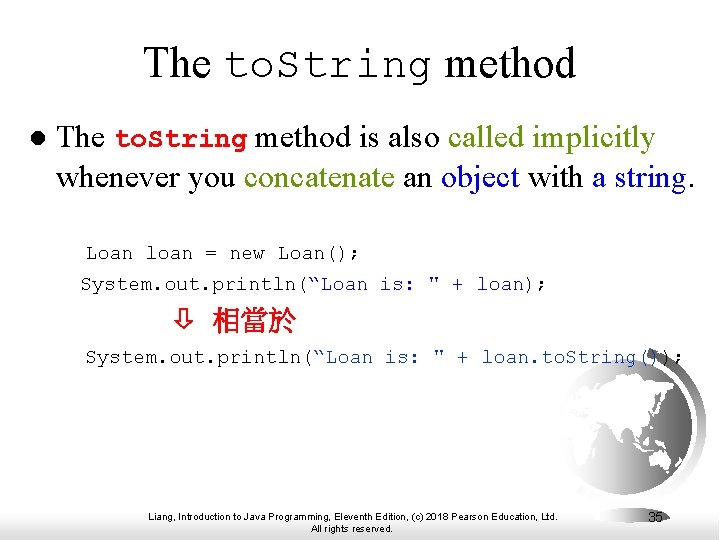
The to. String method l The to. String method is also called implicitly whenever you concatenate an object with a string. Loan loan = new Loan(); System. out. println(“Loan is: " + loan); 相當於 System. out. println(“Loan is: " + loan. to. String()); Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 35
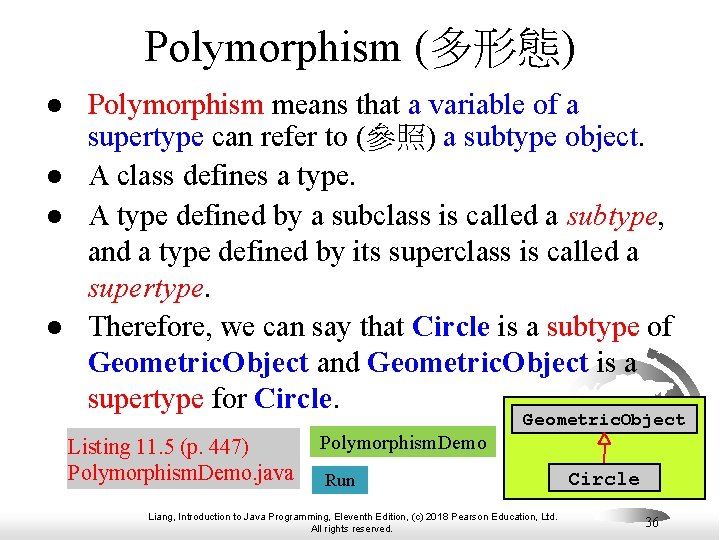
Polymorphism (多形態) l l Polymorphism means that a variable of a supertype can refer to (參照) a subtype object. A class defines a type. A type defined by a subclass is called a subtype, and a type defined by its superclass is called a supertype. Therefore, we can say that Circle is a subtype of Geometric. Object and Geometric. Object is a supertype for Circle. Geometric. Object Listing 11. 5 (p. 447) Polymorphism. Demo. java Polymorphism. Demo Run Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. Circle 36
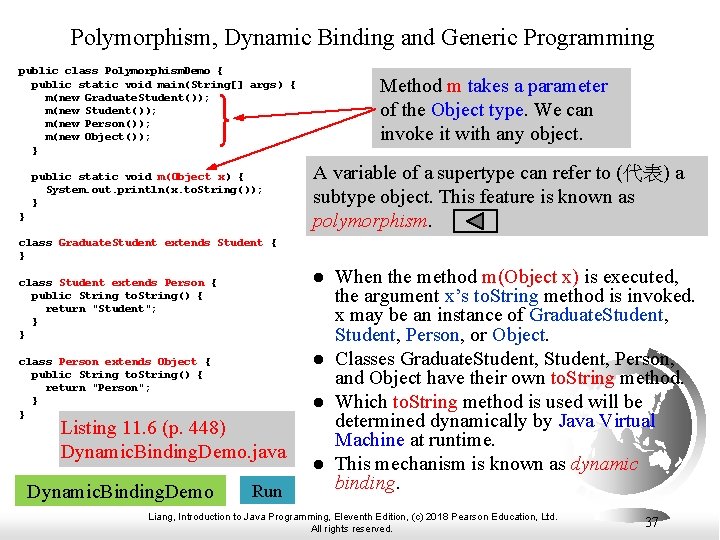
Polymorphism, Dynamic Binding and Generic Programming public class Polymorphism. Demo { public static void main(String[] args) { m(new Graduate. Student()); m(new Person()); m(new Object()); } public static void m(Object x) { System. out. println(x. to. String()); } } Method m takes a parameter of the Object type. We can invoke it with any object. A variable of a supertype can refer to (代表) a subtype object. This feature is known as polymorphism. class Graduate. Student extends Student { } l class Student extends Person { public String to. String() { return "Student"; } } l class Person extends Object { public String to. String() { return "Person"; } } l Listing 11. 6 (p. 448) Dynamic. Binding. Demo. java Dynamic. Binding. Demo Run l When the method m(Object x) is executed, the argument x’s to. String method is invoked. x may be an instance of Graduate. Student, Person, or Object. Classes Graduate. Student, Person, and Object have their own to. String method. Which to. String method is used will be determined dynamically by Java Virtual Machine at runtime. This mechanism is known as dynamic binding. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 37
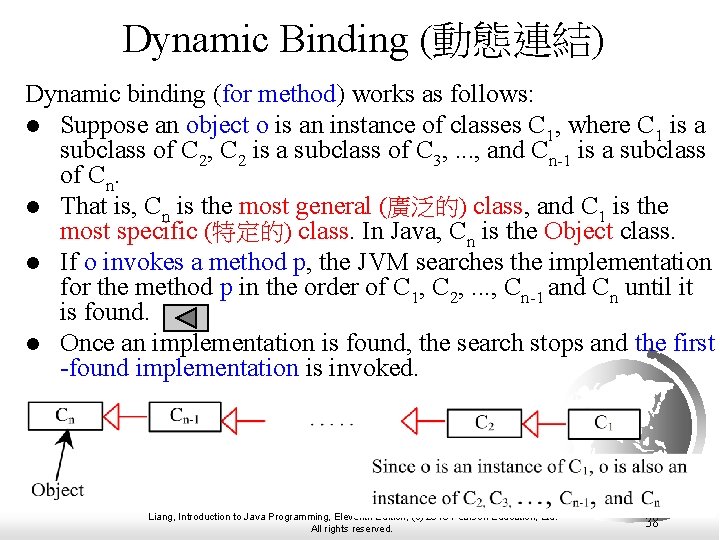
Dynamic Binding (動態連結) Dynamic binding (for method) works as follows: l Suppose an object o is an instance of classes C 1, where C 1 is a subclass of C 2, C 2 is a subclass of C 3, . . . , and Cn-1 is a subclass of Cn. l That is, Cn is the most general (廣泛的) class, and C 1 is the most specific (特定的) class. In Java, Cn is the Object class. l If o invokes a method p, the JVM searches the implementation for the method p in the order of C 1, C 2, . . . , Cn-1 and Cn until it is found. l Once an implementation is found, the search stops and the first -found implementation is invoked. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 38
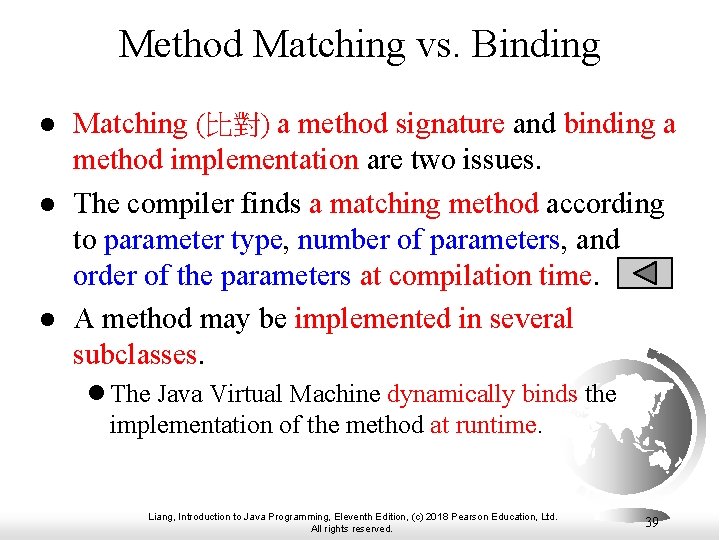
Method Matching vs. Binding l l l Matching (比對) a method signature and binding a method implementation are two issues. The compiler finds a matching method according to parameter type, number of parameters, and order of the parameters at compilation time. A method may be implemented in several subclasses. l The Java Virtual Machine dynamically binds the implementation of the method at runtime. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 39
![Generic Programming 泛用的程式設計方式 public class Polymorphism Demo public static void mainString args Generic Programming (泛用的程式設計方式) public class Polymorphism. Demo { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-40.jpg)
Generic Programming (泛用的程式設計方式) public class Polymorphism. Demo { public static void main(String[] args) { m(new Graduate. Student()); m(new Person()); m(new Object()); } public static void m(Object x) { System. out. println(x. to. String()); } } l l l class Graduate. Student extends Student { } class Student extends Person { public String to. String() { return "Student"; } } class Person extends Object { public String to. String() { return "Person"; } } l Polymorphism allows methods to be used generically (泛用地) for a wide range of object arguments. This is known as generic programming. If a method’s parameter type is a superclass (e. g. , Object), we may pass an object of any of the parameter’s subclasses (e. g. , Student or String). When an object (e. g. , a Student object or a Person object) is used in the method, the particular implementation of the method of the object that is invoked (e. g. , to. String) is determined dynamically. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 40
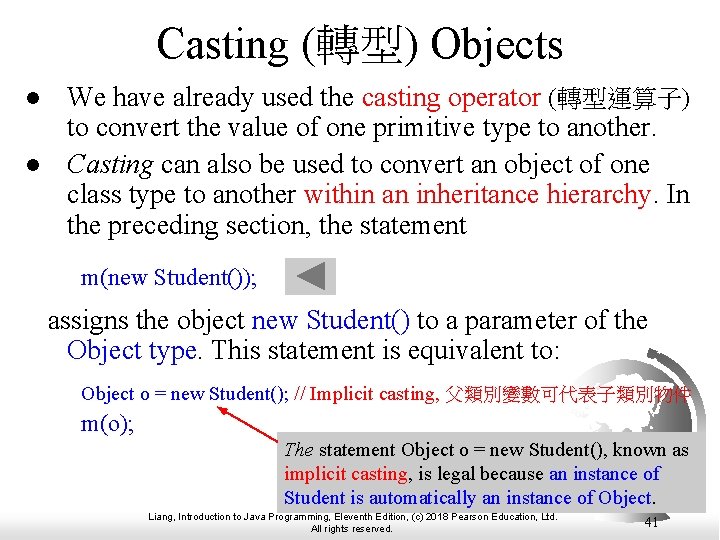
Casting (轉型) Objects l l We have already used the casting operator (轉型運算子) to convert the value of one primitive type to another. Casting can also be used to convert an object of one class type to another within an inheritance hierarchy. In the preceding section, the statement m(new Student()); assigns the object new Student() to a parameter of the Object type. This statement is equivalent to: Object o = new Student(); // Implicit casting, 父類別變數可代表子類別物件 m(o); The statement Object o = new Student(), known as implicit casting, is legal because an instance of Student is automatically an instance of Object. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 41
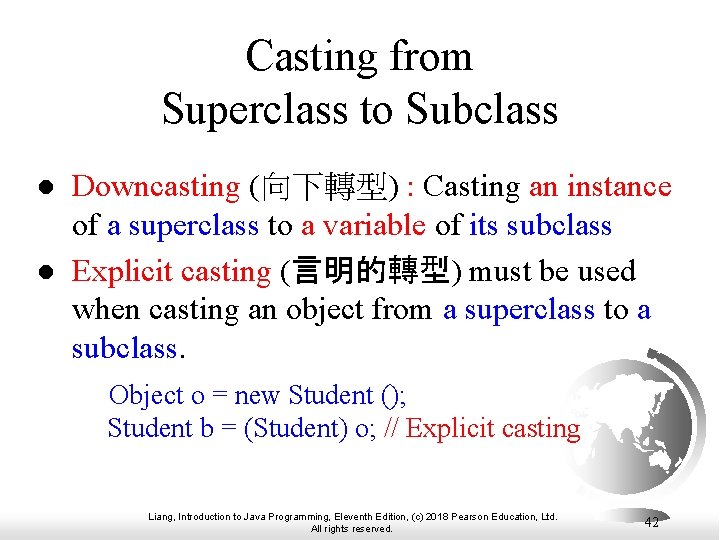
Casting from Superclass to Subclass l l Downcasting (向下轉型) : Casting an instance of a superclass to a variable of its subclass Explicit casting (言明的轉型) must be used when casting an object from a superclass to a subclass. Object o = new Student (); Student b = (Student) o; // Explicit casting Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 42
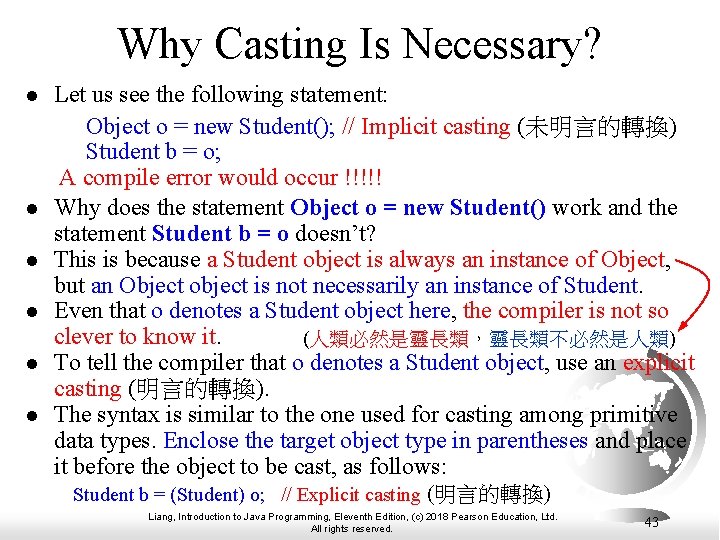
Why Casting Is Necessary? l l l Let us see the following statement: Object o = new Student(); // Implicit casting (未明言的轉換) Student b = o; A compile error would occur !!!!! Why does the statement Object o = new Student() work and the statement Student b = o doesn’t? This is because a Student object is always an instance of Object, but an Object object is not necessarily an instance of Student. Even that o denotes a Student object here, the compiler is not so clever to know it. (人類必然是靈長類,靈長類不必然是人類) To tell the compiler that o denotes a Student object, use an explicit casting (明言的轉換). The syntax is similar to the one used for casting among primitive data types. Enclose the target object type in parentheses and place it before the object to be cast, as follows: Student b = (Student) o; // Explicit casting (明言的轉換) Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 43
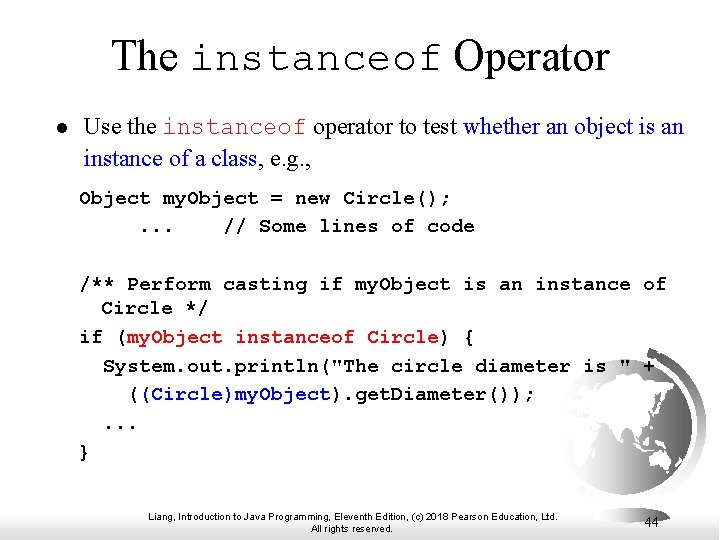
The instanceof Operator l Use the instanceof operator to test whether an object is an instance of a class, e. g. , Object my. Object = new Circle(); . . . // Some lines of code /** Perform casting if my. Object is an instance of Circle */ if (my. Object instanceof Circle) { System. out. println("The circle diameter is " + ((Circle)my. Object). get. Diameter()); . . . } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 44
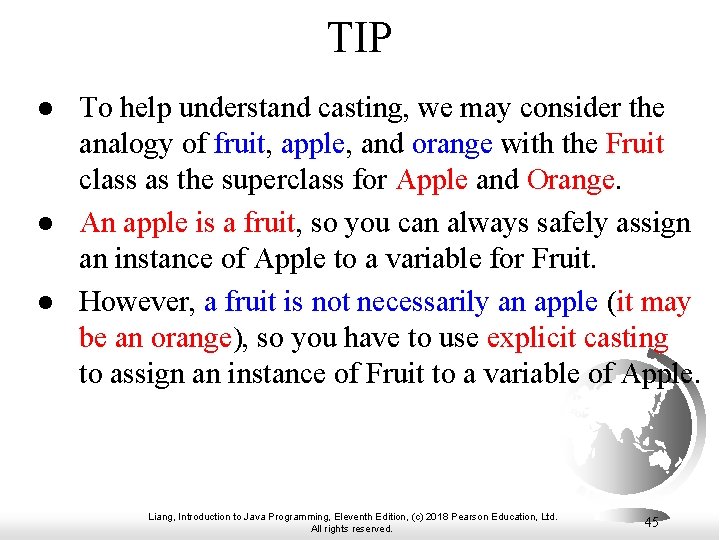
TIP l l l To help understand casting, we may consider the analogy of fruit, apple, and orange with the Fruit class as the superclass for Apple and Orange. An apple is a fruit, so you can always safely assign an instance of Apple to a variable for Fruit. However, a fruit is not necessarily an apple (it may be an orange), so you have to use explicit casting to assign an instance of Fruit to a variable of Apple. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 45
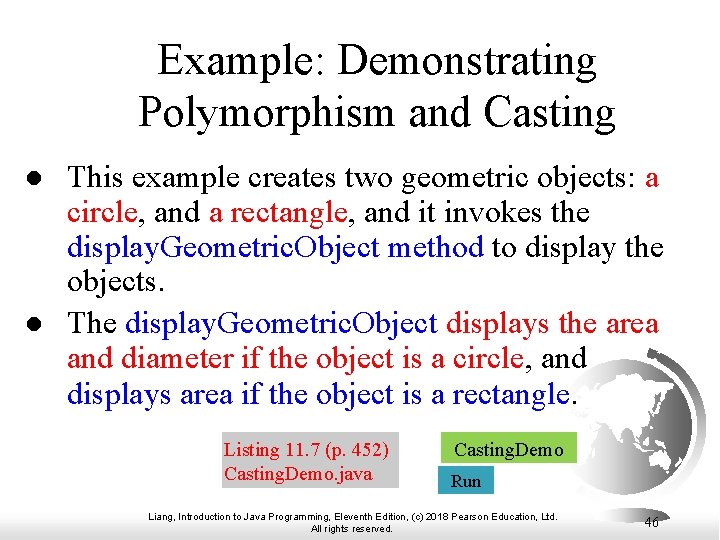
Example: Demonstrating Polymorphism and Casting l l This example creates two geometric objects: a circle, and a rectangle, and it invokes the display. Geometric. Object method to display the objects. The display. Geometric. Object displays the area and diameter if the object is a circle, and displays area if the object is a rectangle. Listing 11. 7 (p. 452) Casting. Demo. java Casting. Demo Run Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 46
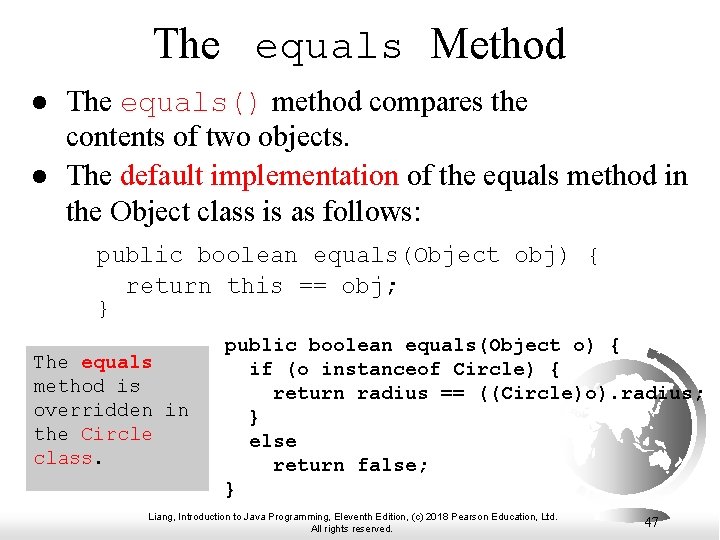
The equals Method l l The equals() method compares the contents of two objects. The default implementation of the equals method in the Object class is as follows: public boolean equals(Object obj) { return this == obj; } The equals method is overridden in the Circle class. public boolean equals(Object o) { if (o instanceof Circle) { return radius == ((Circle)o). radius; } else return false; } Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 47
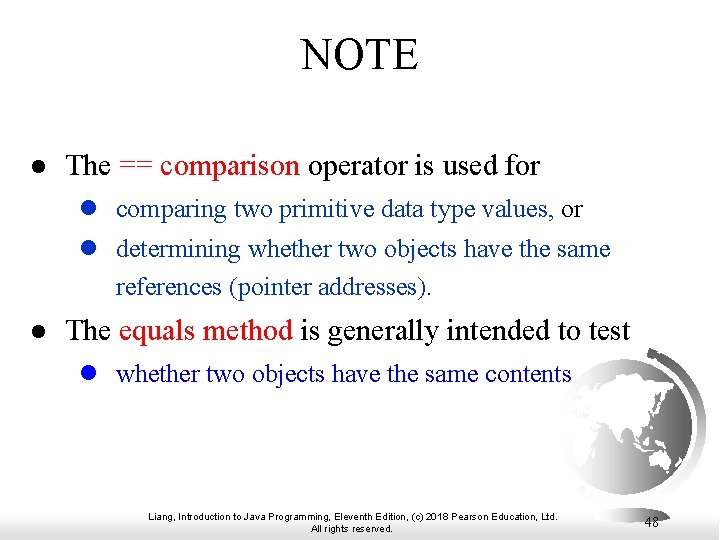
NOTE l The == comparison operator is used for l comparing two primitive data type values, or l determining whether two objects have the same references (pointer addresses). l The equals method is generally intended to test l whether two objects have the same contents Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 48
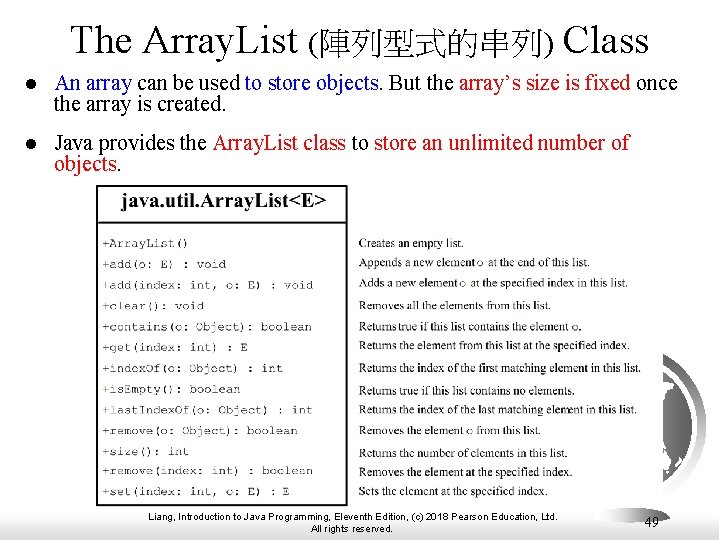
The Array. List (陣列型式的串列) Class l An array can be used to store objects. But the array’s size is fixed once the array is created. l Java provides the Array. List class to store an unlimited number of objects. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 49
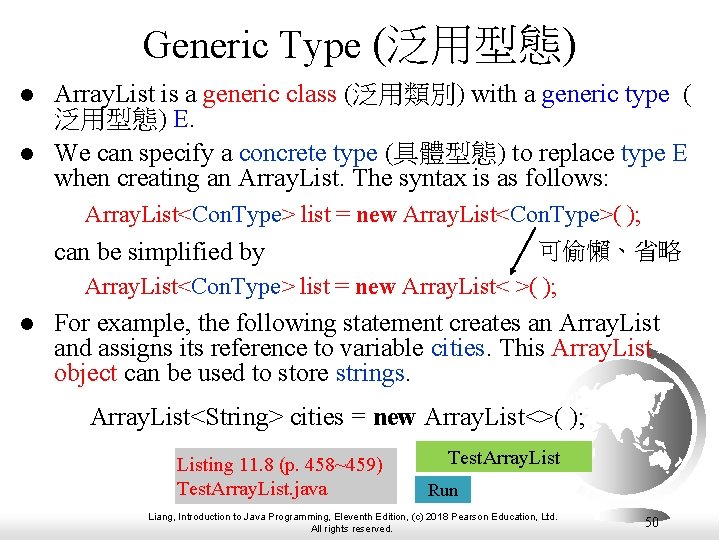
Generic Type (泛用型態) l l Array. List is a generic class (泛用類別) with a generic type ( 泛用型態) E. We can specify a concrete type (具體型態) to replace type E when creating an Array. List. The syntax is as follows: Array. List<Con. Type> list = new Array. List<Con. Type>( ); can be simplified by 可偷懶、省略 Array. List<Con. Type> list = new Array. List< >( ); l For example, the following statement creates an Array. List and assigns its reference to variable cities. This Array. List object can be used to store strings. Array. List<String> cities = new Array. List<>( ); Listing 11. 8 (p. 458~459) Test. Array. List. java Test. Array. List Run Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 50
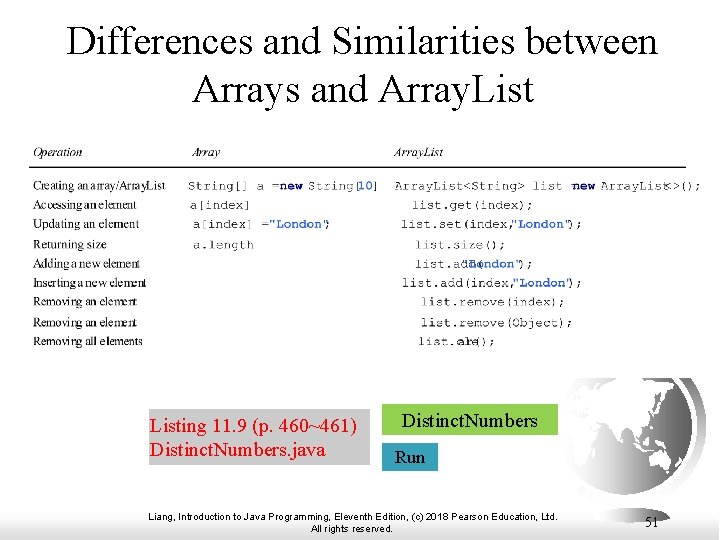
Differences and Similarities between Arrays and Array. Listing 11. 9 (p. 460~461) Distinct. Numbers. java Distinct. Numbers Run Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 51
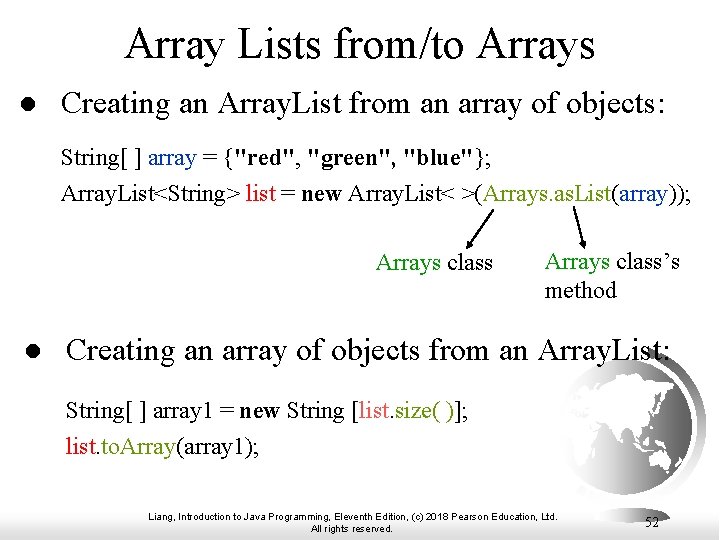
Array Lists from/to Arrays l Creating an Array. List from an array of objects: String[ ] array = {"red", "green", "blue"}; Array. List<String> list = new Array. List< >(Arrays. as. List(array)); Arrays class l Arrays class’s method Creating an array of objects from an Array. List: String[ ] array 1 = new String [list. size( )]; list. to. Array(array 1); Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 52
![max and min in an Array List Integer array 3 5 95 max and min in an Array List Integer[ ] array = {3, 5, 95,](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-53.jpg)
max and min in an Array List Integer[ ] array = {3, 5, 95, 4, 15, 34, 3, 6, 5}; Array. List< Integer > list = new Array. List< Integer > (Arrays. as. List(array)); System. out. pritnln(java. util. Collections. max(list)); System. out. pritnln(java. util. Collections. min(list)); Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 53
![Shuffling 洗牌 an Array List Integer array 3 5 95 4 15 Shuffling (洗牌) an Array List Integer[ ] array = {3, 5, 95, 4, 15,](https://slidetodoc.com/presentation_image_h2/5ee2f8666adc22963d0cc6412412fc9a/image-54.jpg)
Shuffling (洗牌) an Array List Integer[ ] array = {3, 5, 95, 4, 15, 34, 3, 6, 5}; Array. List<Integer> list = new Array. List<>(Arrays. as. List(array)); java. util. Collections. shuffle(list); System. out. println(list); Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 54
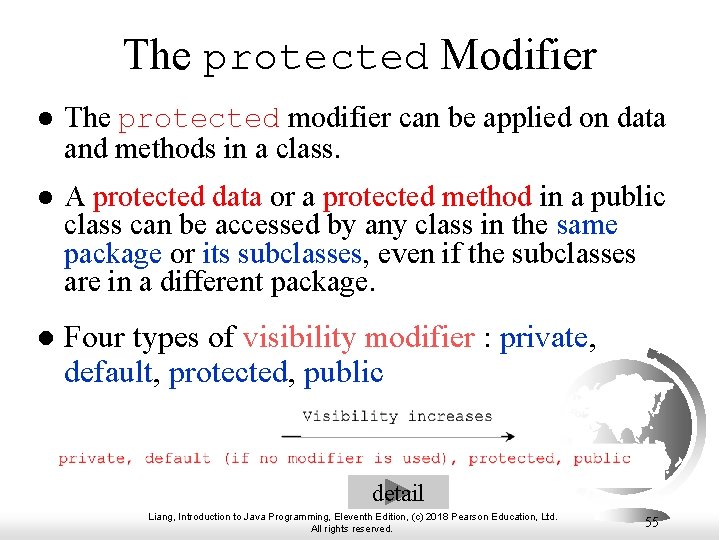
The protected Modifier l The protected modifier can be applied on data and methods in a class. l A protected data or a protected method in a public class can be accessed by any class in the same package or its subclasses, even if the subclasses are in a different package. l Four types of visibility modifier : private, default, protected, public detail Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 55
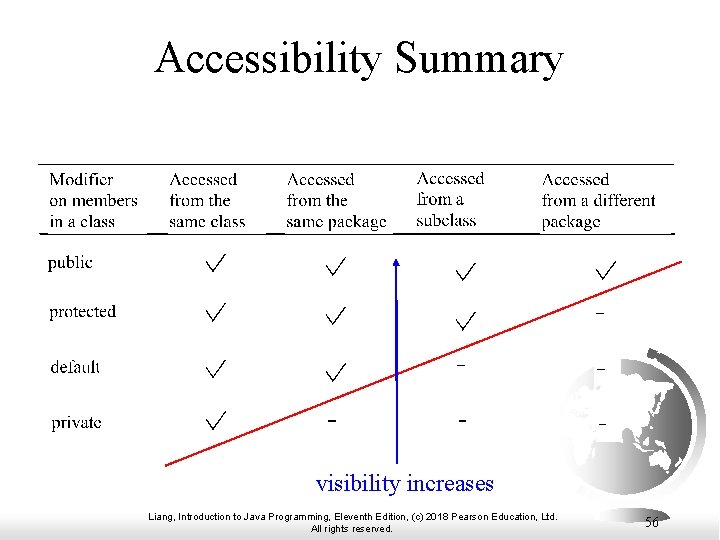
Accessibility Summary visibility increases Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 56
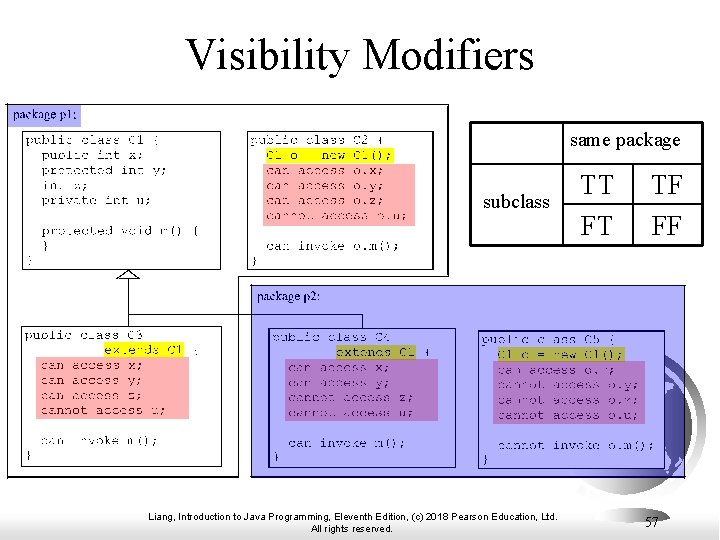
Visibility Modifiers same package subclass Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. TT FT TF FF 57
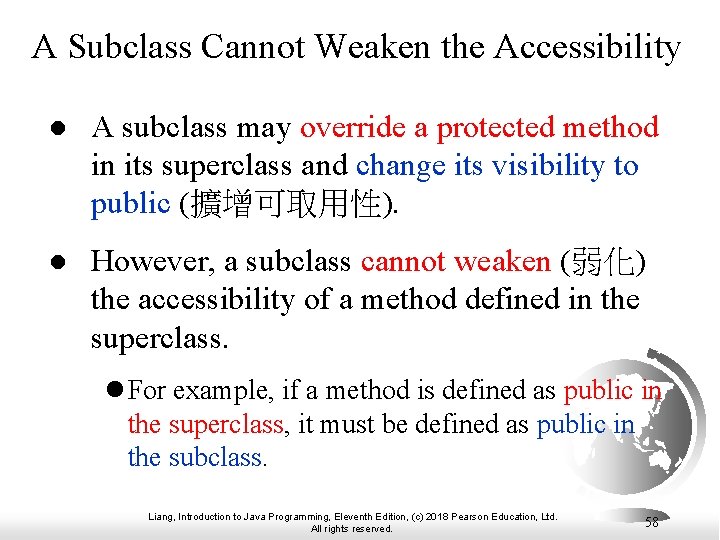
A Subclass Cannot Weaken the Accessibility l A subclass may override a protected method in its superclass and change its visibility to public (擴增可取用性). l However, a subclass cannot weaken (弱化) the accessibility of a method defined in the superclass. l For example, if a method is defined as public in the superclass, it must be defined as public in the subclass. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 58
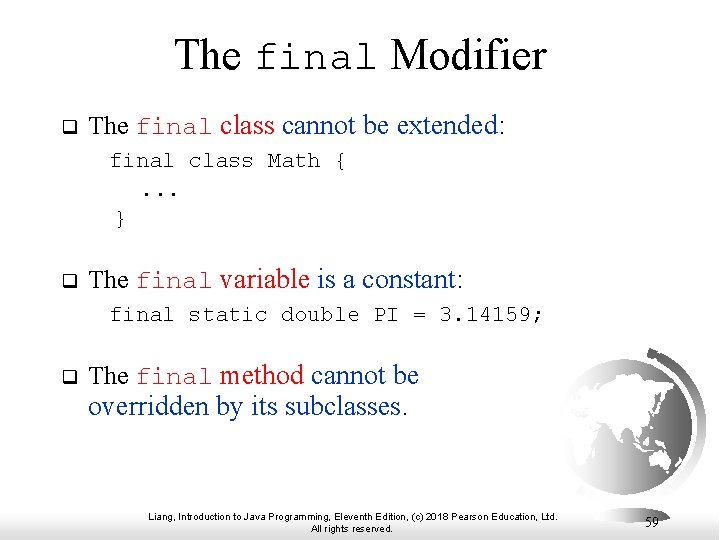
The final Modifier q The final class cannot be extended: final class Math {. . . } q The final variable is a constant: final static double PI = 3. 14159; q The final method cannot be overridden by its subclasses. Liang, Introduction to Java Programming, Eleventh Edition, (c) 2018 Pearson Education, Ltd. All rights reserved. 59
Encapsulation inheritance polymorphism
Oop polymorphism inheritance encapsulation
Encapsulation inheritance polymorphism
Encapsulation inheritance polymorphism
Abstraction encapsulation inheritance polymorphism
Polymophism
Daniel liang introduction to java programming
Richard seow yung liang
Zhuo shi wo li liang
Zhong han liang
Yuanxing liang
Amber liang
Liang fontdemo.java
Liang fontdemo.java
Cara mengindeks surat masuk dan keluar sistem abjad
Randy liang
David teo choon liang
How was ma liang like
Zong-liang yang
Liang jianhui
Zhang liang game
Chia liang cheng
Otk keuangan adalah
Mechanical engineering umbc
Zong-liang yang
Siemens sans bold
Dr liang hao nan
Pengertian arsip menurut the liang gie
Anticorps anti nucléaire moucheté 1/160
Kathleen liang
Edmund liang
Keratan rentas jantung
Sushil dubey intel
Diana liang faa
Foo joon liang
Introduction to inheritance
Chapter 11 complex inheritance and human heredity test
Complex patterns of inheritance
Chapter 16 the molecular basis of inheritance
Chapter 15: the chromosomal basis of inheritance
A gene locus is
Chapter 16 molecular basis of inheritance
Chapter 15 the chromosomal basis of inheritance
The chromosomal basis of inheritance chapter 15
Ap biology chapter 15
Chapter 11 section 1 basic patterns of human inheritance
Chapter 11 section 1 basic patterns of human inheritance
Bioflix dna replication
Chapter 9 patterns of inheritance
Chapter 15: the chromosomal basis of inheritance
Difference between allotropy and polymorphism
Mutation and polymorphism
Physalia is a polymorphic colony
What is polymorphism
Adhoc polymorphism
What is polymorphism in oop
Compile time polymorphism in c++
Subtype polymorphism
Polymorphism computer science
Subtype polymorphism