Arrays Arrays An array is a collection of
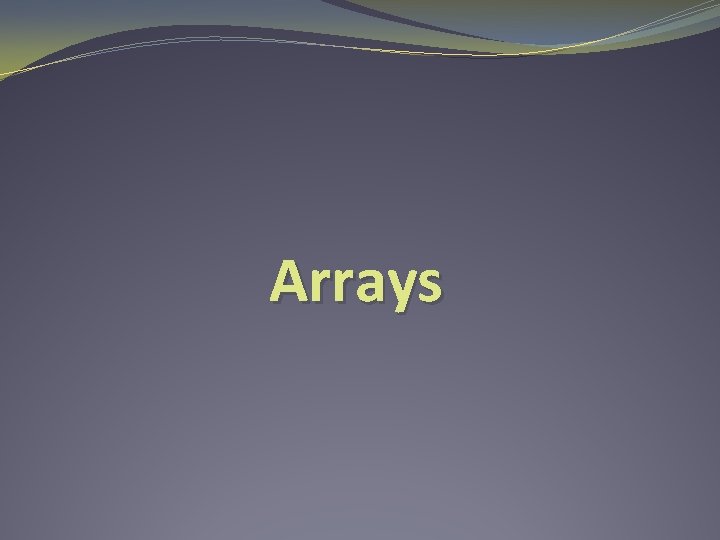
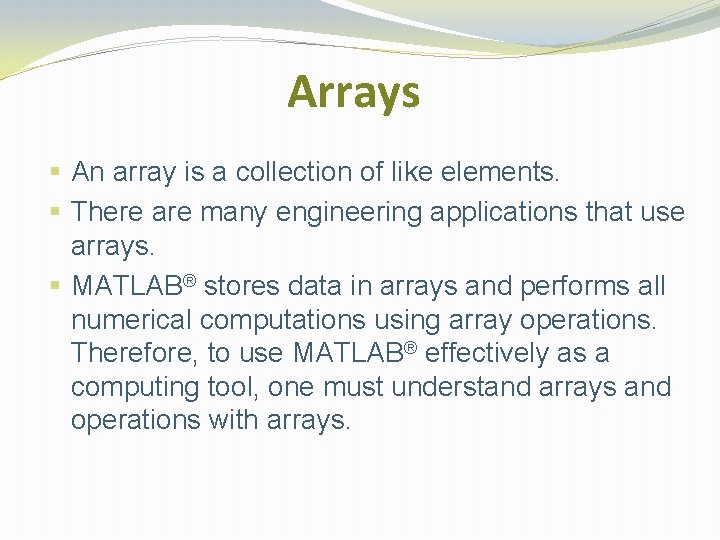
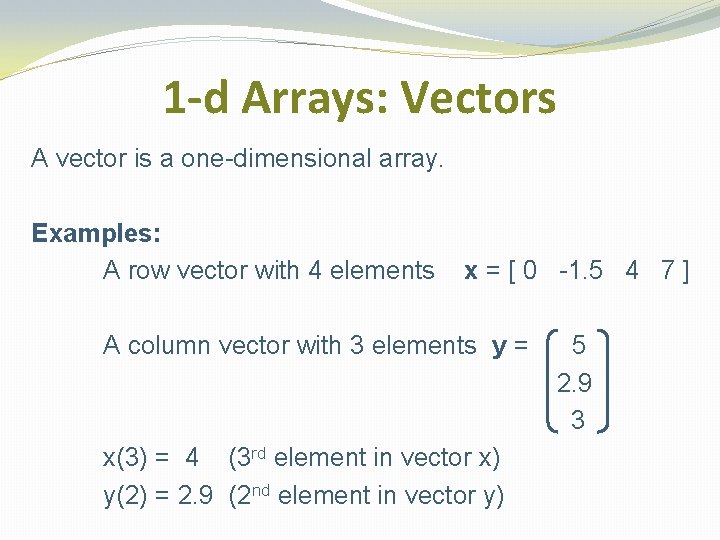
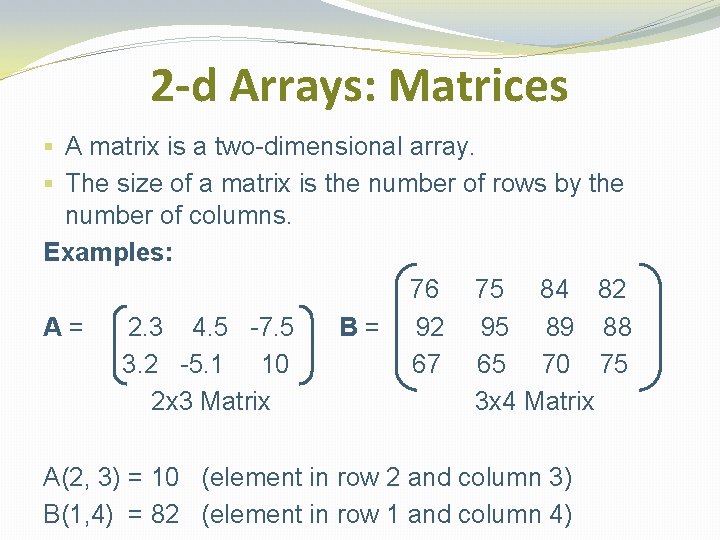
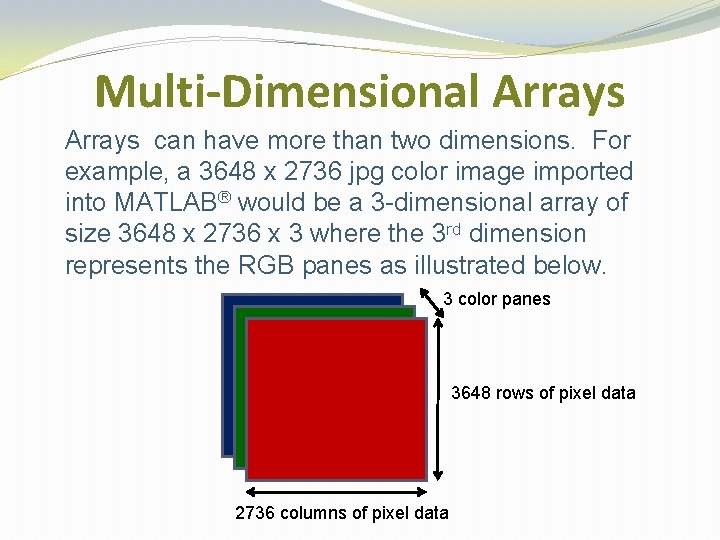
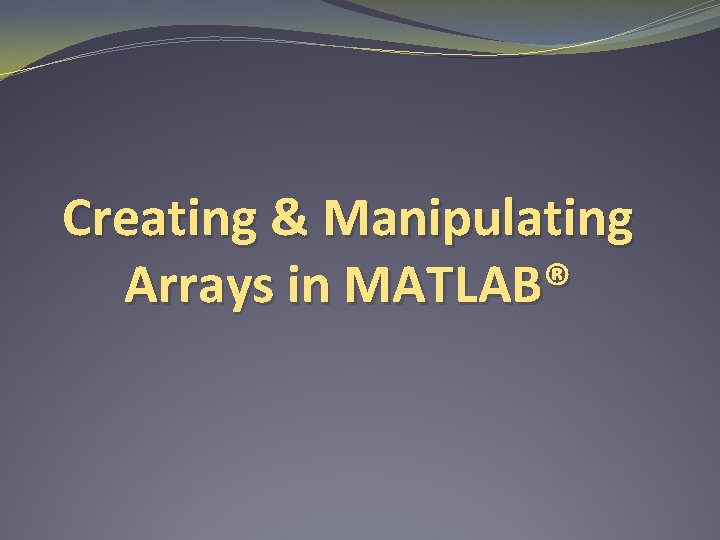
![Creating Arrays in MATLAB® >> a = [2. 3 7. 5 4. 3 6] Creating Arrays in MATLAB® >> a = [2. 3 7. 5 4. 3 6]](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-7.jpg)
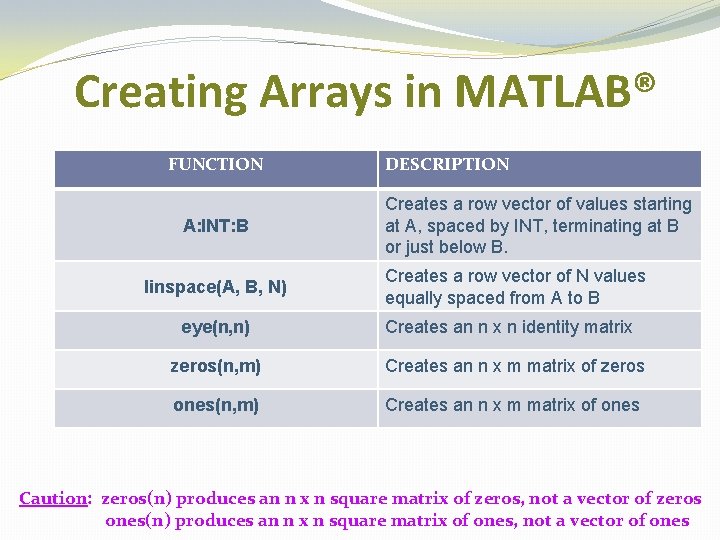
![Creating Arrays in MATLAB® FUNCTION randi([Imin, Imax], [n, m]) DESCRIPTION Creates an n x Creating Arrays in MATLAB® FUNCTION randi([Imin, Imax], [n, m]) DESCRIPTION Creates an n x](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-9.jpg)
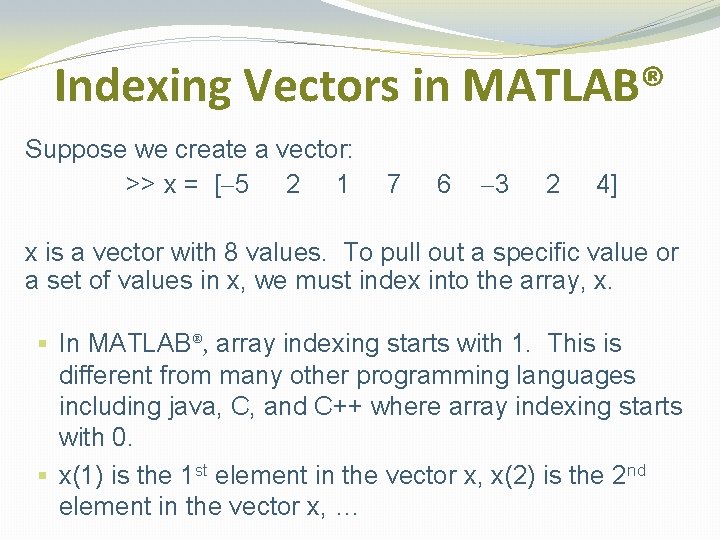
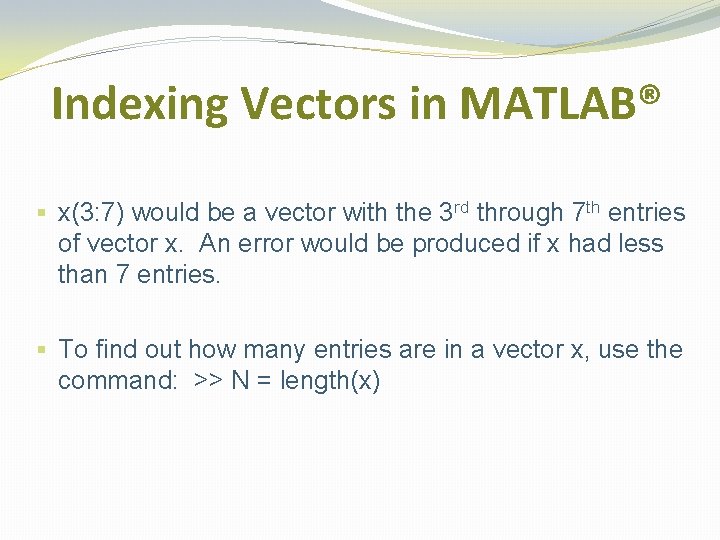
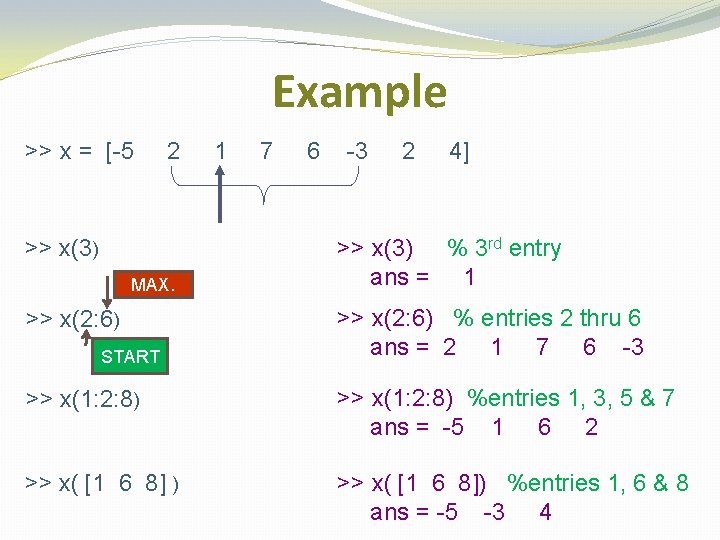
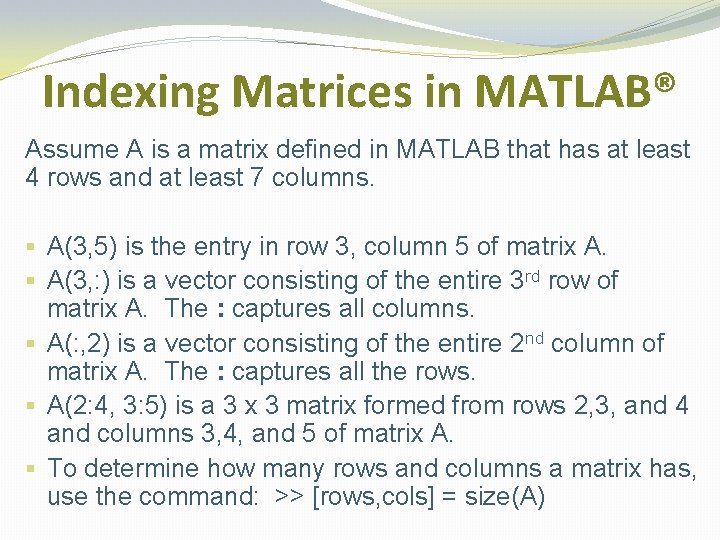
![Matrix Example >> matrix = randi([0 10], [4, 5]) >> matrix(2, 3) ans = Matrix Example >> matrix = randi([0 10], [4, 5]) >> matrix(2, 3) ans =](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-14.jpg)
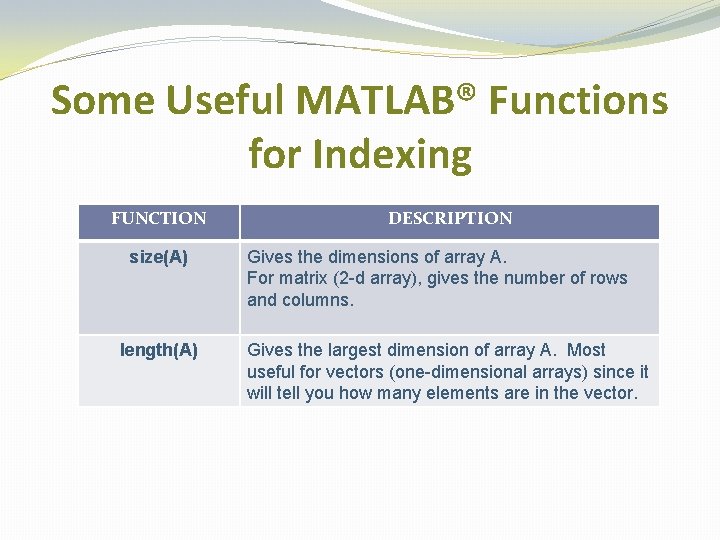
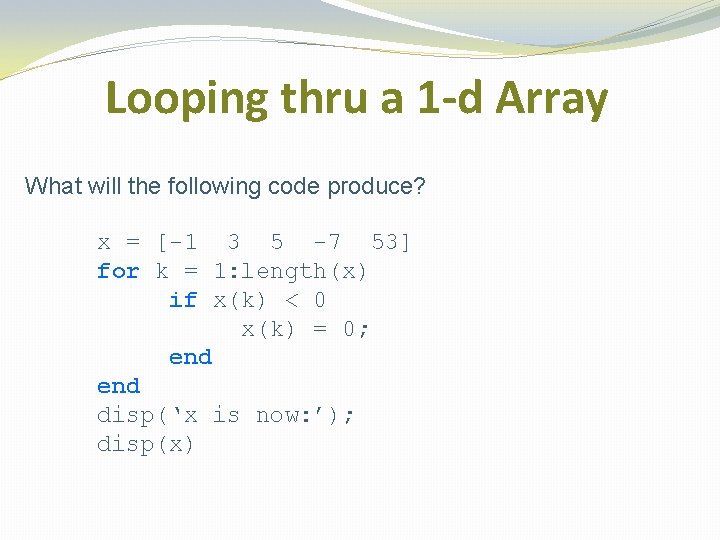
![Looping thru a 1 -d Array x = [-1 3 5 -7 53] for Looping thru a 1 -d Array x = [-1 3 5 -7 53] for](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-17.jpg)
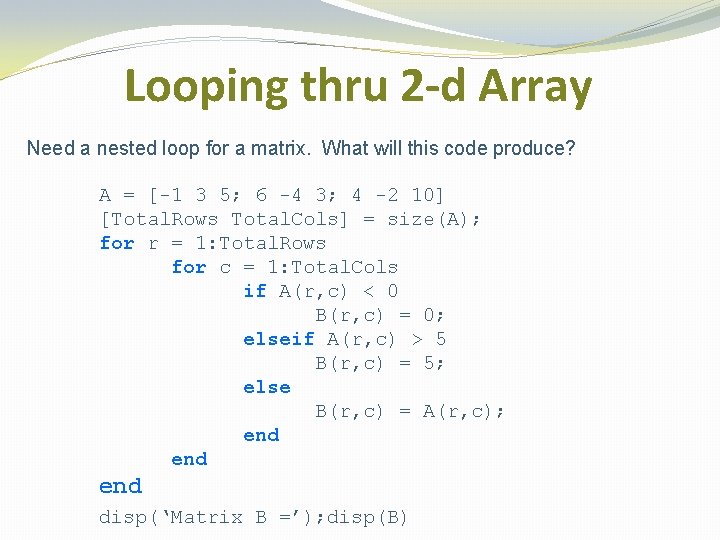
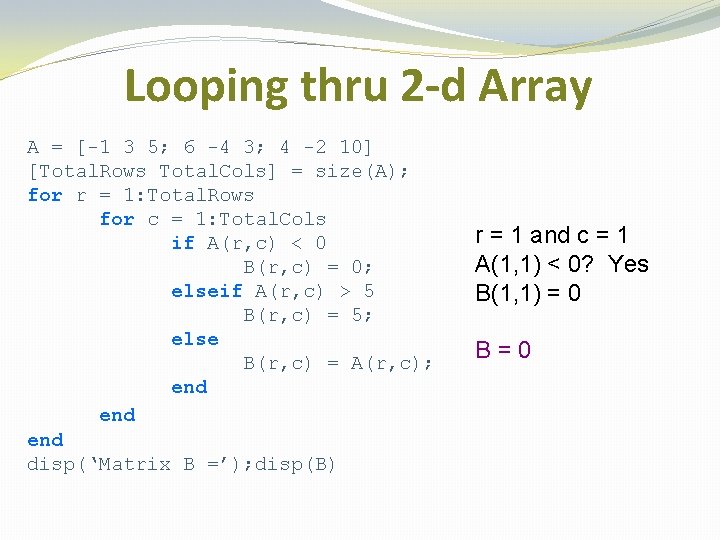
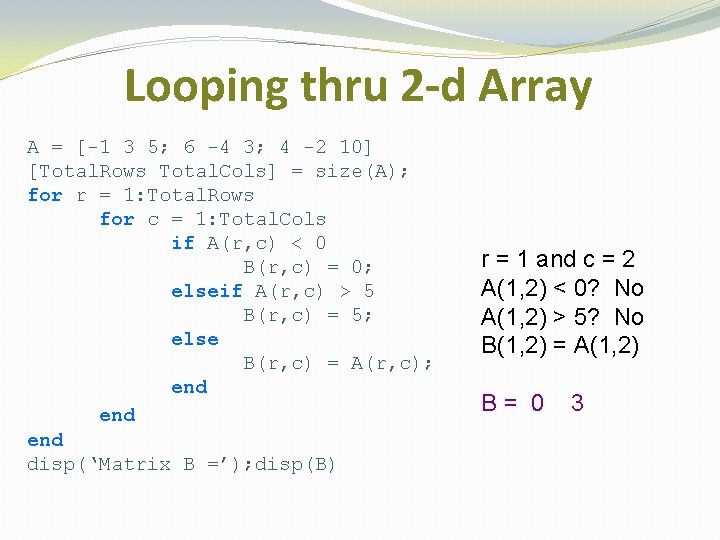
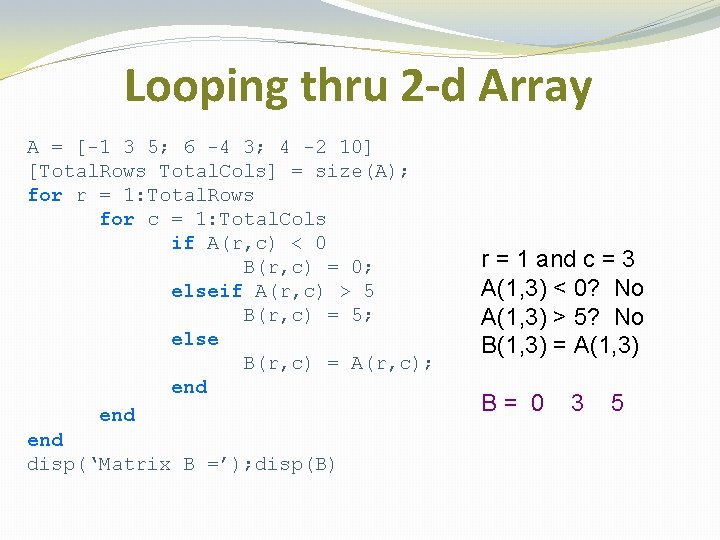
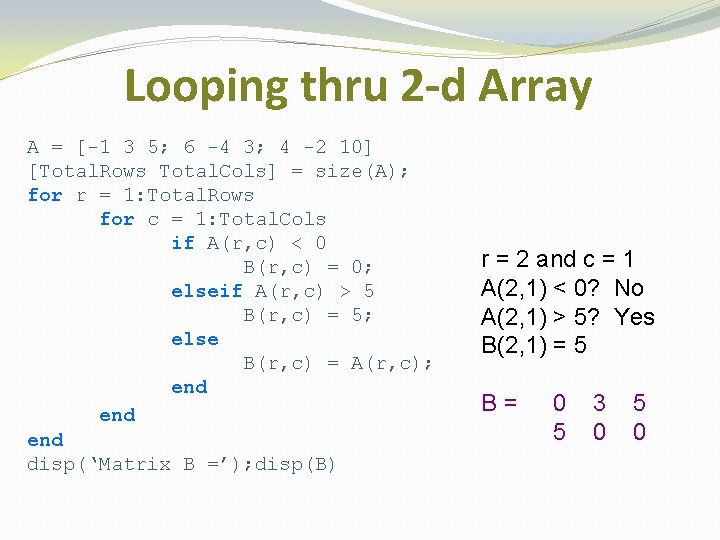
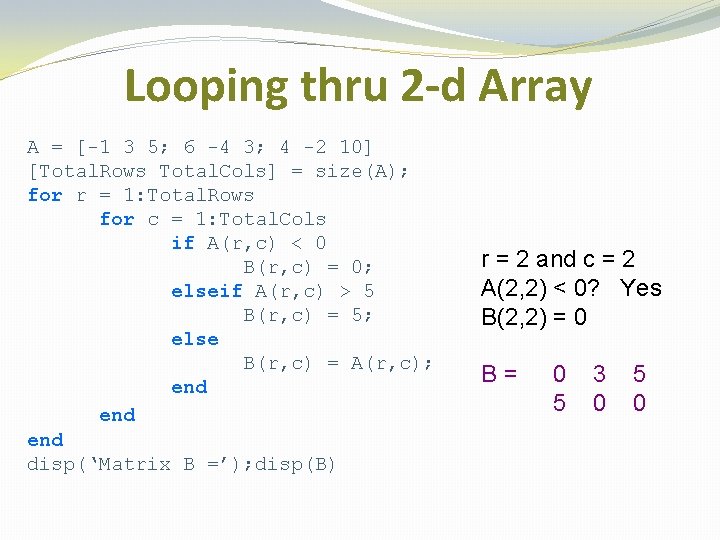
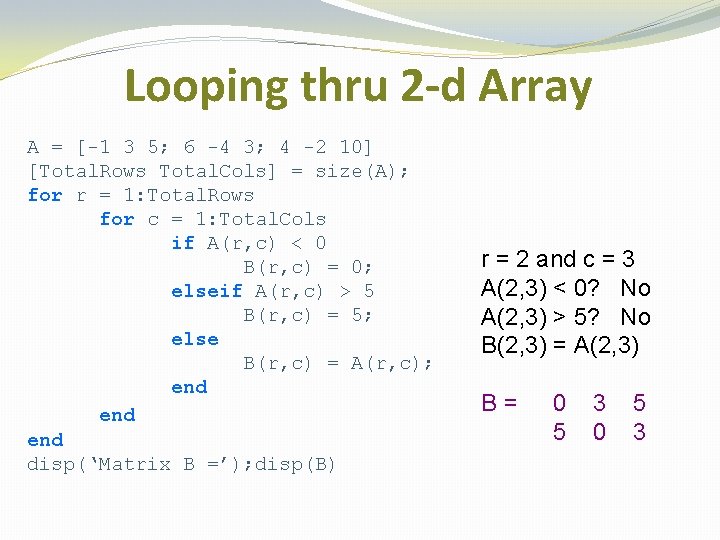
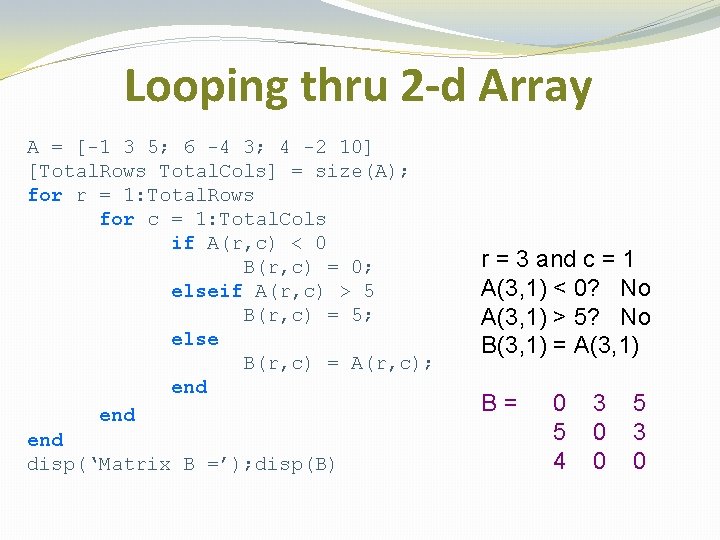
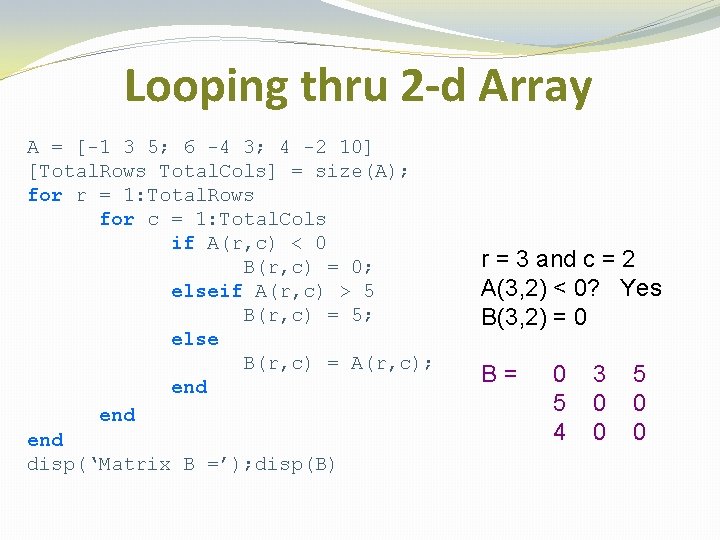
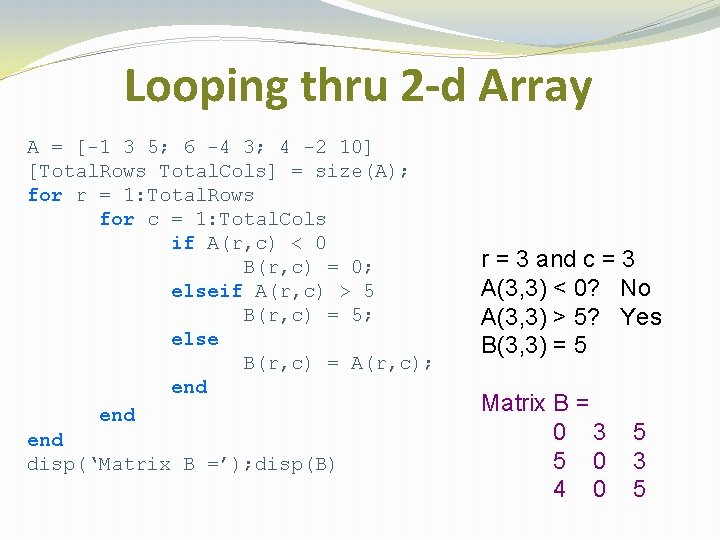
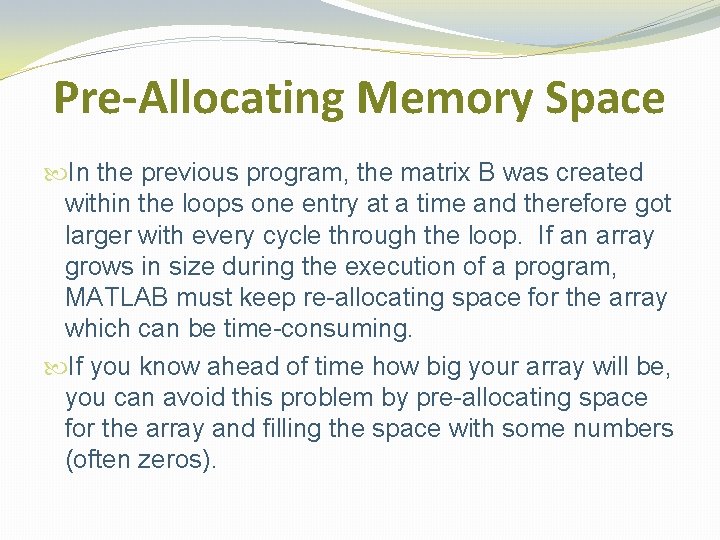
![Pre –Allocating Space A = [-1 3 5; 6 -4 3; 4 -2 5] Pre –Allocating Space A = [-1 3 5; 6 -4 3; 4 -2 5]](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-29.jpg)
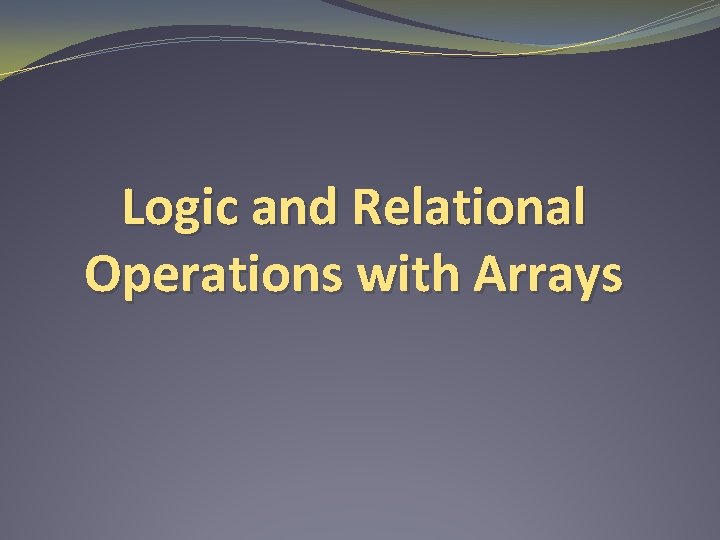
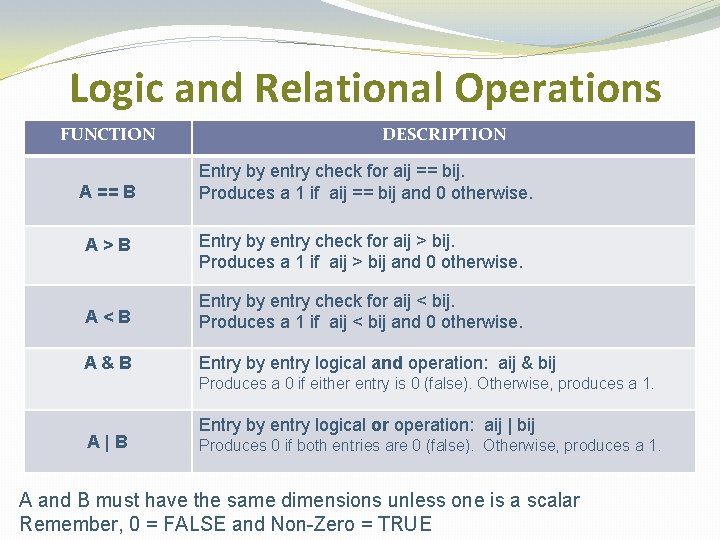
![Examples >> A = randi ( [-10 10] , [4 3] ) A= 7 Examples >> A = randi ( [-10 10] , [4 3] ) A= 7](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-32.jpg)
![Examples >> A = [-1 5; 3 4] A = -1 5 3 4 Examples >> A = [-1 5; 3 4] A = -1 5 3 4](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-33.jpg)
![Examples >> A = [-1 5; 3 4] A = -1 5 3 4 Examples >> A = [-1 5; 3 4] A = -1 5 3 4](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-34.jpg)
![Examples >> A = [-1 5; 3 4] A = -1 5 3 4 Examples >> A = [-1 5; 3 4] A = -1 5 3 4](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-35.jpg)
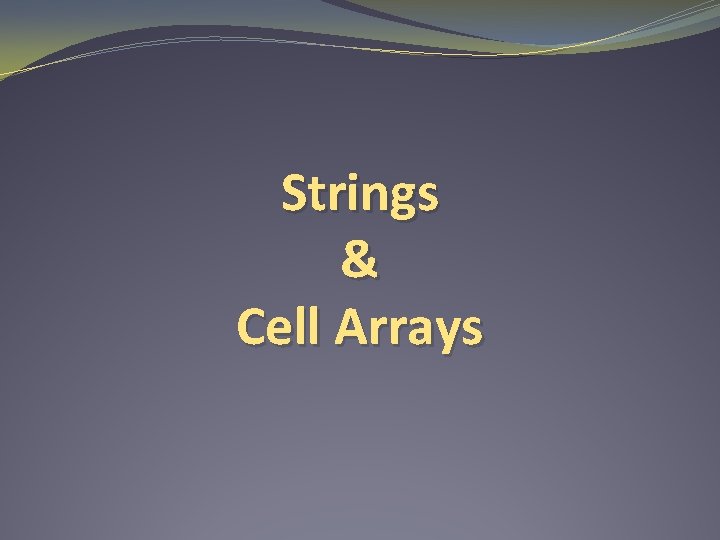
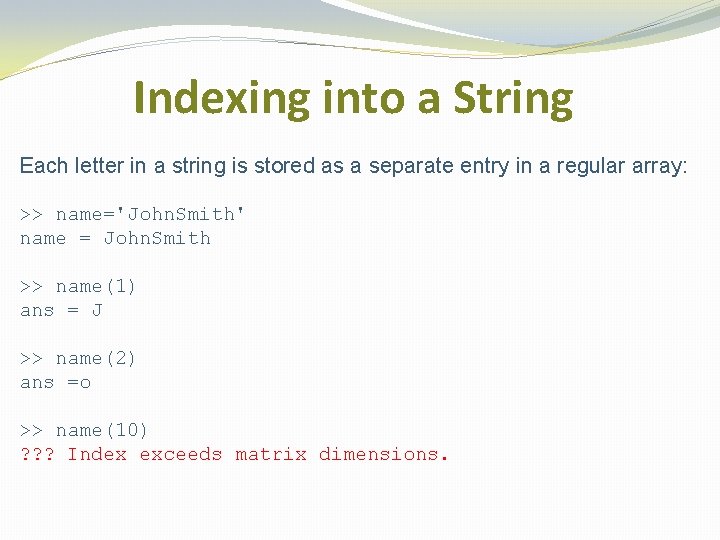
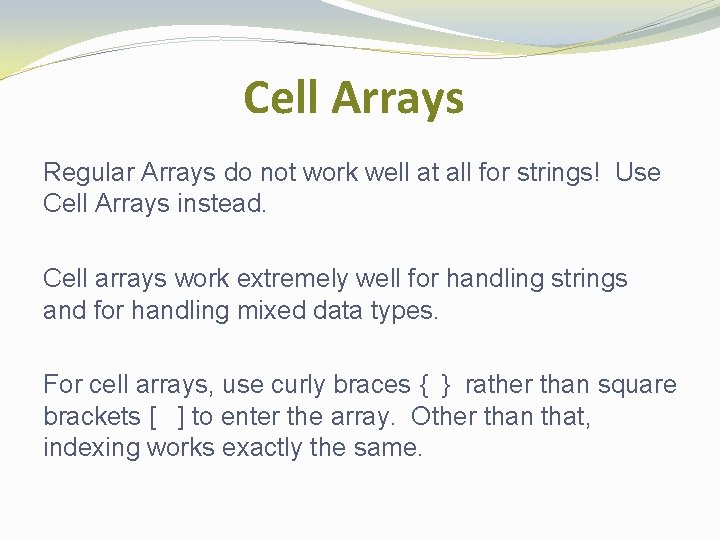
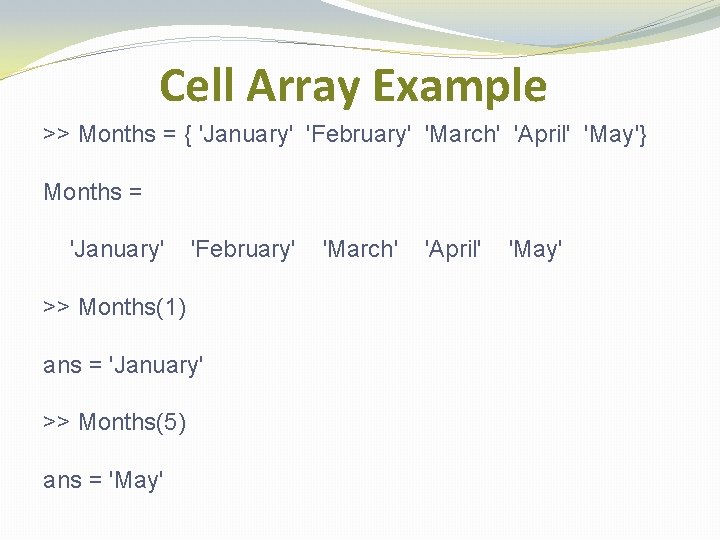
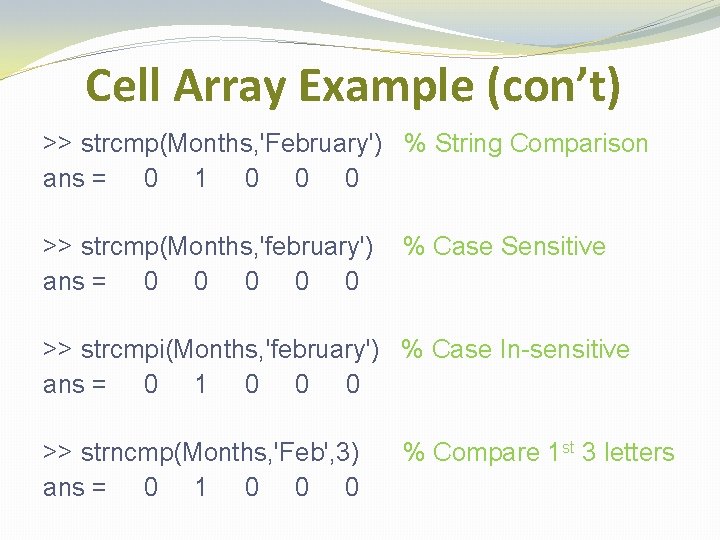
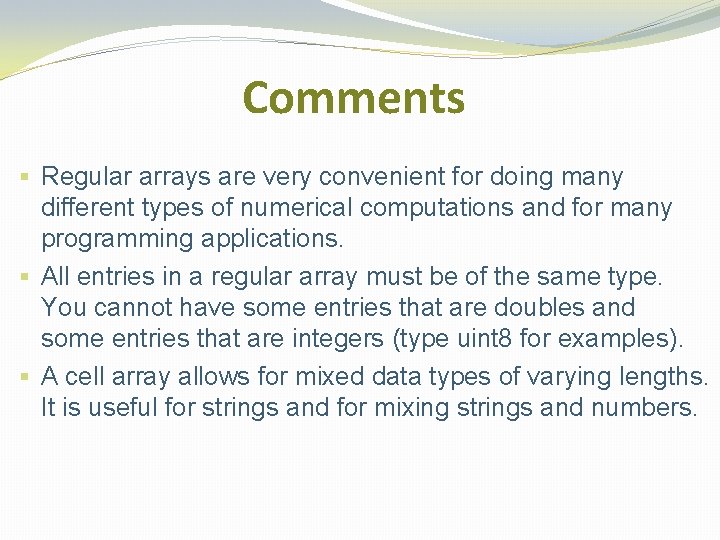
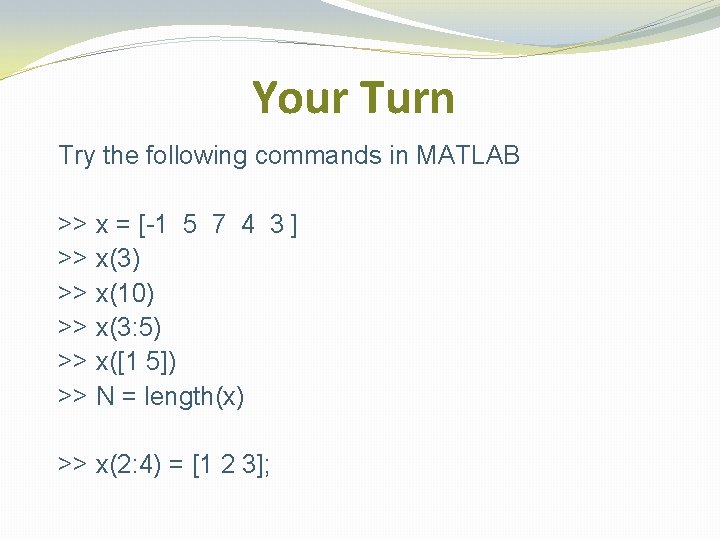
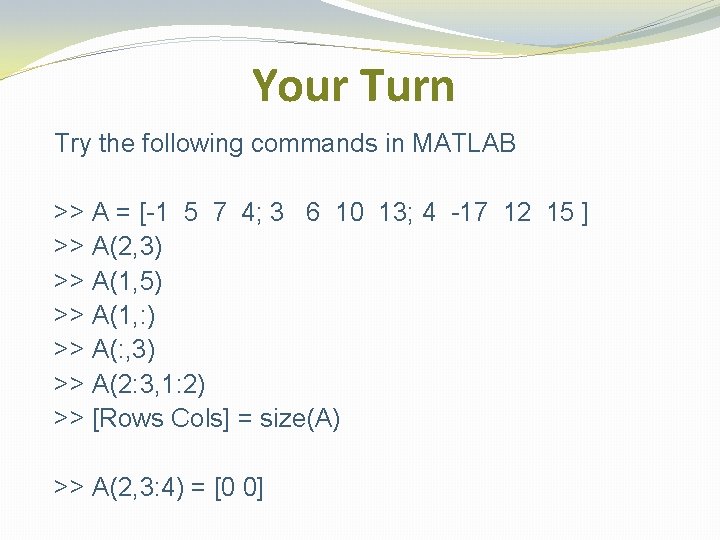
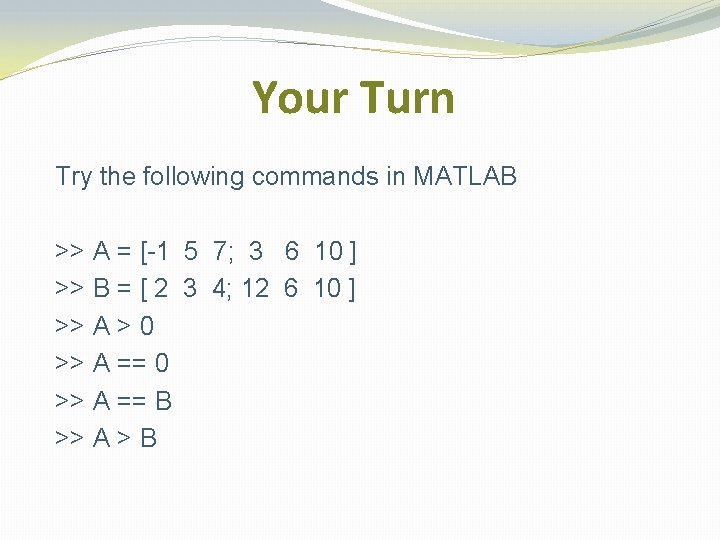
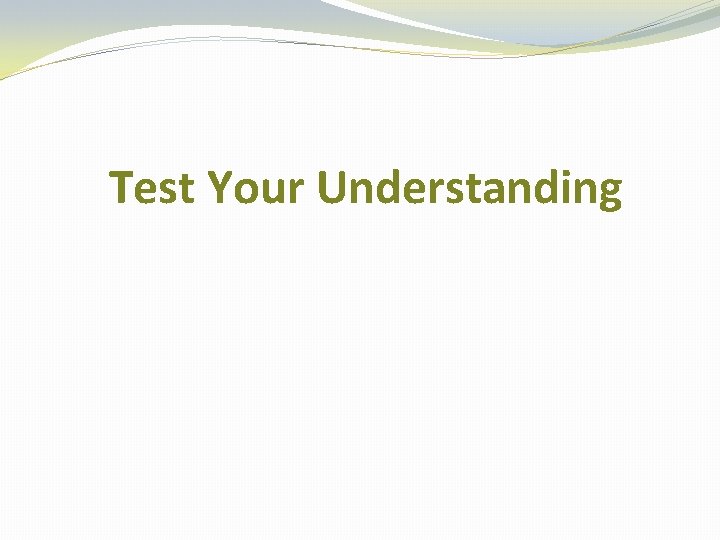
- Slides: 45
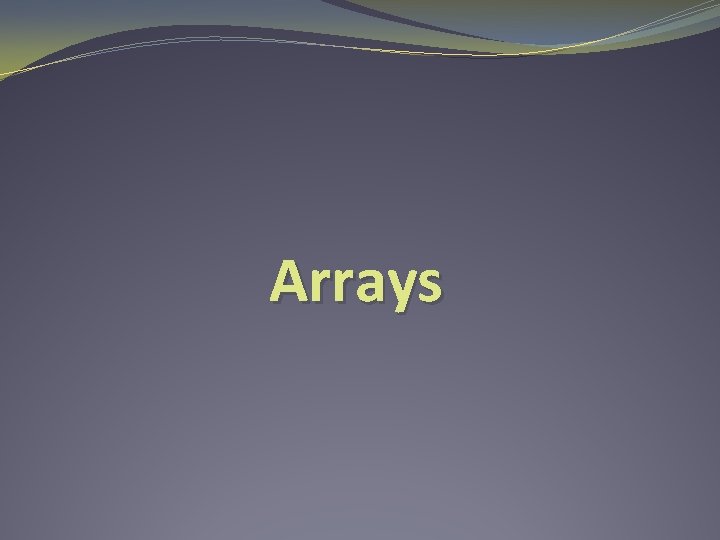
Arrays
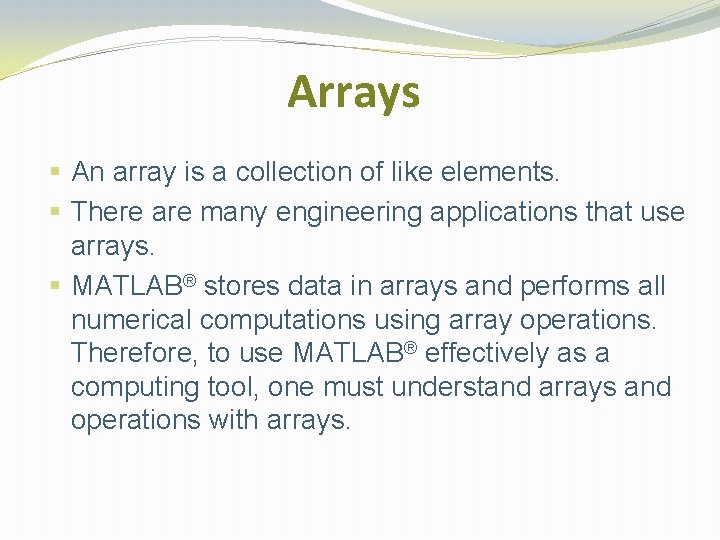
Arrays § An array is a collection of like elements. § There are many engineering applications that use arrays. § MATLAB® stores data in arrays and performs all numerical computations using array operations. Therefore, to use MATLAB® effectively as a computing tool, one must understand arrays and operations with arrays.
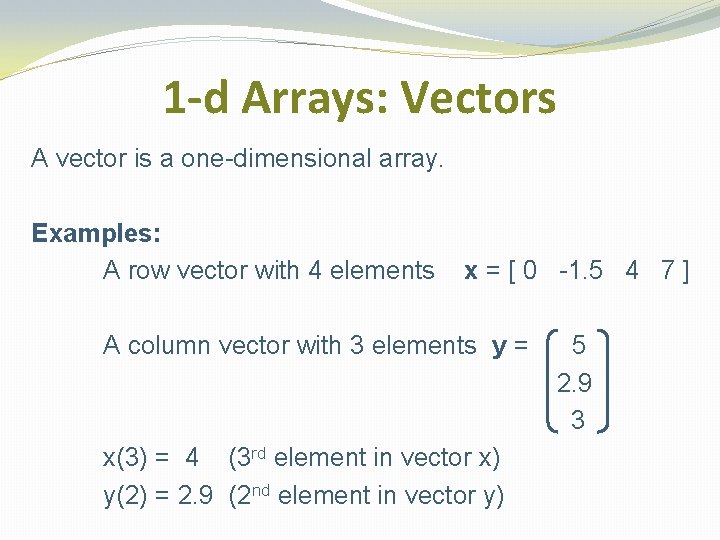
1 -d Arrays: Vectors A vector is a one-dimensional array. Examples: A row vector with 4 elements x = [ 0 -1. 5 4 7 ] A column vector with 3 elements y = x(3) = 4 (3 rd element in vector x) y(2) = 2. 9 (2 nd element in vector y) 5 2. 9 3
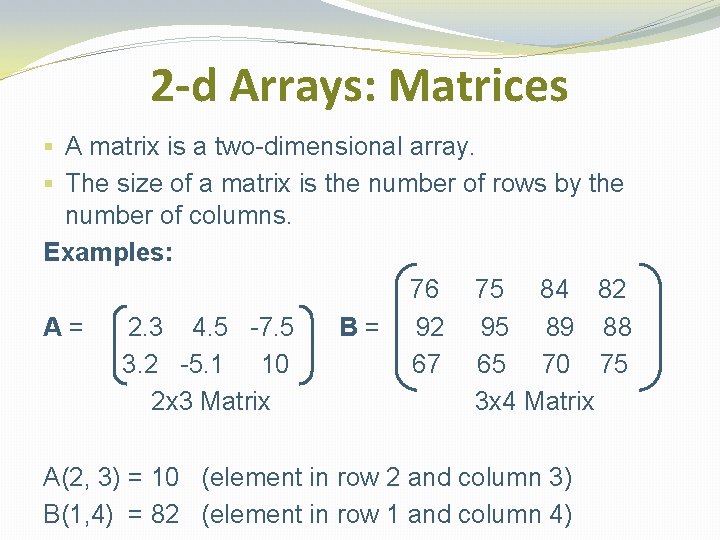
2 -d Arrays: Matrices § A matrix is a two-dimensional array. § The size of a matrix is the number of rows by the number of columns. Examples: 76 75 84 82 A= 2. 3 4. 5 -7. 5 B = 92 95 89 88 3. 2 -5. 1 10 67 65 70 75 2 x 3 Matrix 3 x 4 Matrix A(2, 3) = 10 (element in row 2 and column 3) B(1, 4) = 82 (element in row 1 and column 4)
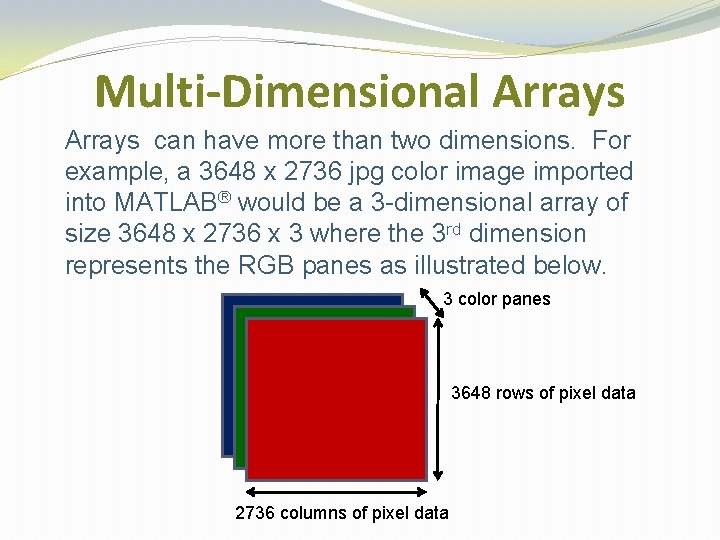
Multi-Dimensional Arrays can have more than two dimensions. For example, a 3648 x 2736 jpg color image imported into MATLAB® would be a 3 -dimensional array of size 3648 x 2736 x 3 where the 3 rd dimension represents the RGB panes as illustrated below. 3 color panes 3648 rows of pixel data 2736 columns of pixel data
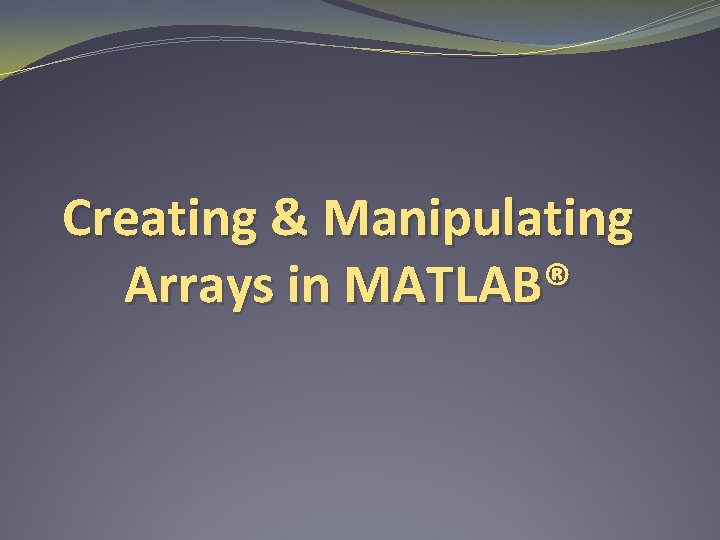
Creating & Manipulating Arrays in MATLAB®
![Creating Arrays in MATLAB a 2 3 7 5 4 3 6 Creating Arrays in MATLAB® >> a = [2. 3 7. 5 4. 3 6]](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-7.jpg)
Creating Arrays in MATLAB® >> a = [2. 3 7. 5 4. 3 6] >> c = [1. 3 7. 2 9. 5 10; 2. 6 5. 1 4. 7 8. 1] a= c= 2. 3000 7. 5000 4. 3000 >> b = [2. 3; 7. 5; 4. 3; 6] b= 2. 3000 7. 5000 4. 3000 6. 0000 1. 3000 2. 6000 7. 2000 5. 1000 9. 5000 10. 0000 4. 7000 8. 1000 >> d = [1. 3 7. 2 9. 5; 2. 6 5. 1 4. 7 8. 1] Error using vertcat Dimensions of matrices being concatenated are not consistent. Note: Commas can be used between values in a row instead of spaces
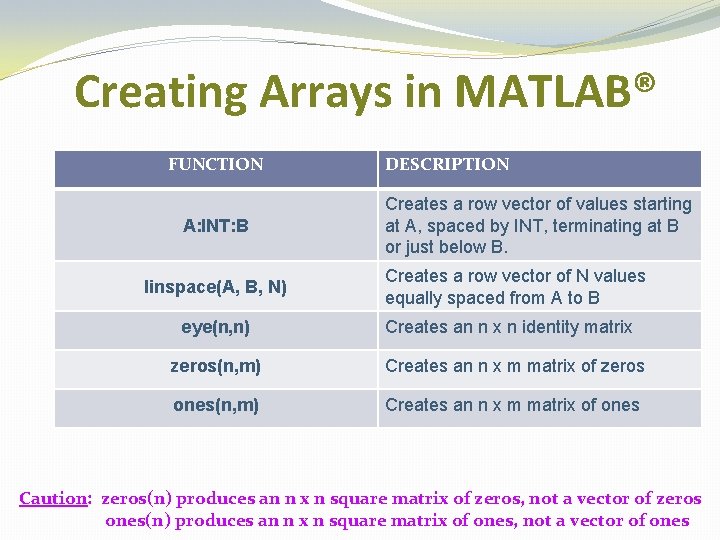
Creating Arrays in MATLAB® FUNCTION A: INT: B linspace(A, B, N) eye(n, n) DESCRIPTION Creates a row vector of values starting at A, spaced by INT, terminating at B or just below B. Creates a row vector of N values equally spaced from A to B Creates an n x n identity matrix zeros(n, m) Creates an n x m matrix of zeros ones(n, m) Creates an n x m matrix of ones Caution: zeros(n) produces an n x n square matrix of zeros, not a vector of zeros ones(n) produces an n x n square matrix of ones, not a vector of ones
![Creating Arrays in MATLAB FUNCTION randiImin Imax n m DESCRIPTION Creates an n x Creating Arrays in MATLAB® FUNCTION randi([Imin, Imax], [n, m]) DESCRIPTION Creates an n x](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-9.jpg)
Creating Arrays in MATLAB® FUNCTION randi([Imin, Imax], [n, m]) DESCRIPTION Creates an n x m matrix of integers uniformly distributed from Imin to Imax randn(n, m) Creates an n x m matrix of random numbers normally distributed with mean 0 and standard deviation of 1. rand(n, m) Creates an n x m matrix of random numbers uniformly distributed on the interval [0 1] randperm(n, m) Creates vector of m unique integers selected from the values 1, 2, … n Note: The MATLAB command: rng('shuffle') seeds the random number generator based on the current time so that RAND, RANDI, and RANDN will produce a different sequence of numbers after each time you call rng.
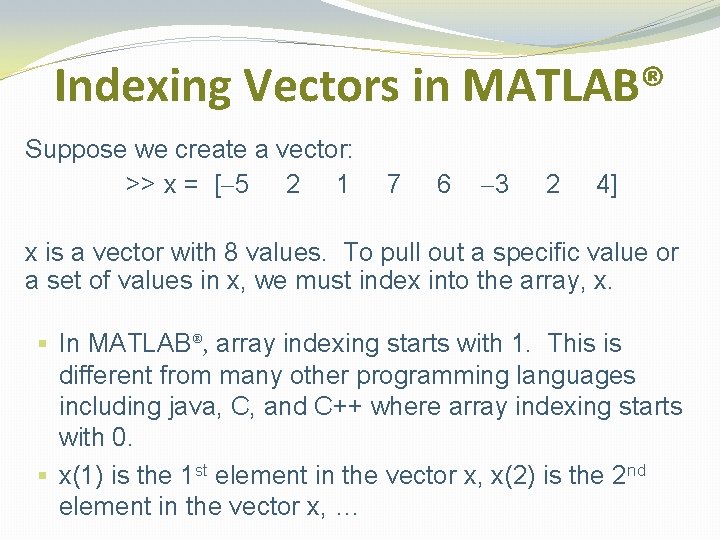
Indexing Vectors in MATLAB® Suppose we create a vector: >> x = [-5 2 1 7 6 -3 2 4] x is a vector with 8 values. To pull out a specific value or a set of values in x, we must index into the array, x. § In MATLAB®, array indexing starts with 1. This is different from many other programming languages including java, C, and C++ where array indexing starts with 0. § x(1) is the 1 st element in the vector x, x(2) is the 2 nd element in the vector x, …
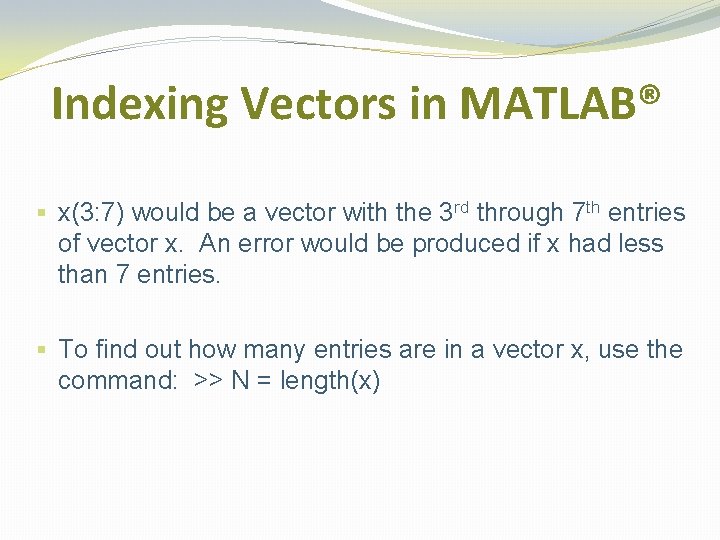
Indexing Vectors in MATLAB® § x(3: 7) would be a vector with the 3 rd through 7 th entries of vector x. An error would be produced if x had less than 7 entries. § To find out how many entries are in a vector x, use the command: >> N = length(x)
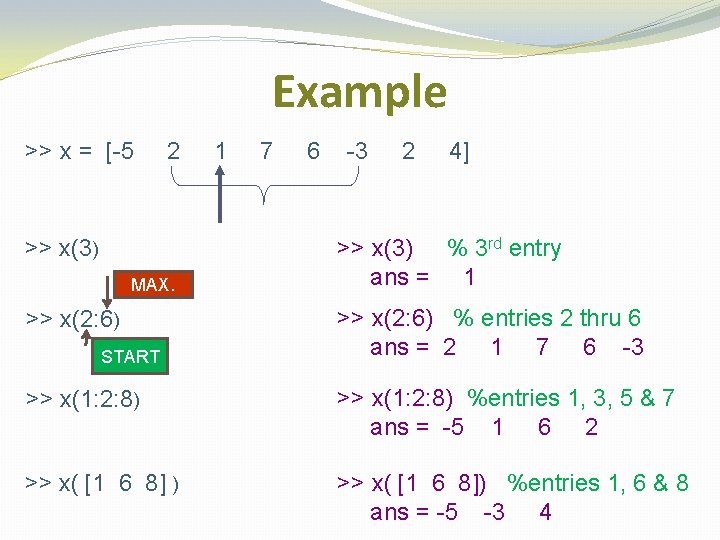
Example >> x = [-5 2 >> x(3) MAX. >> x(2: 6) START 1 7 6 -3 2 4] >> x(3) % 3 rd entry ans = 1 >> x(2: 6) % entries 2 thru 6 ans = 2 1 7 6 -3 >> x(1: 2: 8) %entries 1, 3, 5 & 7 ans = -5 1 6 2 >> x( [1 6 8] ) >> x( [1 6 8]) %entries 1, 6 & 8 ans = -5 -3 4
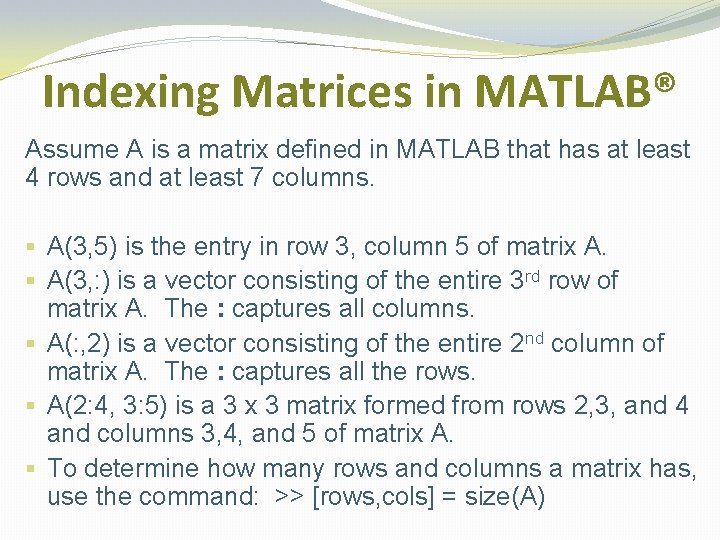
Indexing Matrices in MATLAB® Assume A is a matrix defined in MATLAB that has at least 4 rows and at least 7 columns. § A(3, 5) is the entry in row 3, column 5 of matrix A. § A(3, : ) is a vector consisting of the entire 3 rd row of matrix A. The : captures all columns. § A(: , 2) is a vector consisting of the entire 2 nd column of matrix A. The : captures all the rows. § A(2: 4, 3: 5) is a 3 x 3 matrix formed from rows 2, 3, and 4 and columns 3, 4, and 5 of matrix A. § To determine how many rows and columns a matrix has, use the command: >> [rows, cols] = size(A)
![Matrix Example matrix randi0 10 4 5 matrix2 3 ans Matrix Example >> matrix = randi([0 10], [4, 5]) >> matrix(2, 3) ans =](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-14.jpg)
Matrix Example >> matrix = randi([0 10], [4, 5]) >> matrix(2, 3) ans = 10 matrix = >> matrix(: , 4) ans = 2 8 2 5 7 7 8 3 7 7 1 1 5 10 3 6 2 8 2 5 >> matrix(2, 3) >> matrix(: , 4) >> matrix(3, 2: 4) >> matrix(2: 3, 3: 5) 7 9 10 6 >> matrix(3, 2: 4) ans = 1 3 2 >> matrix(2: 3, 3: 5) ans = 10 8 9 3 2 10
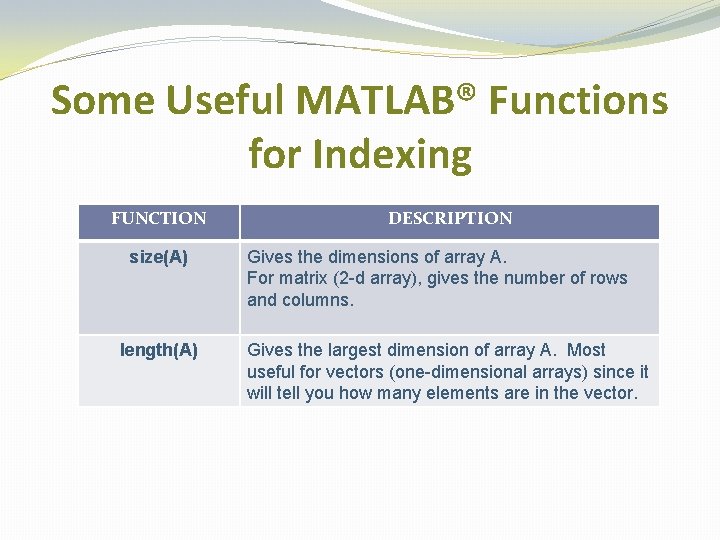
Some Useful MATLAB® Functions for Indexing FUNCTION size(A) length(A) DESCRIPTION Gives the dimensions of array A. For matrix (2 -d array), gives the number of rows and columns. Gives the largest dimension of array A. Most useful for vectors (one-dimensional arrays) since it will tell you how many elements are in the vector.
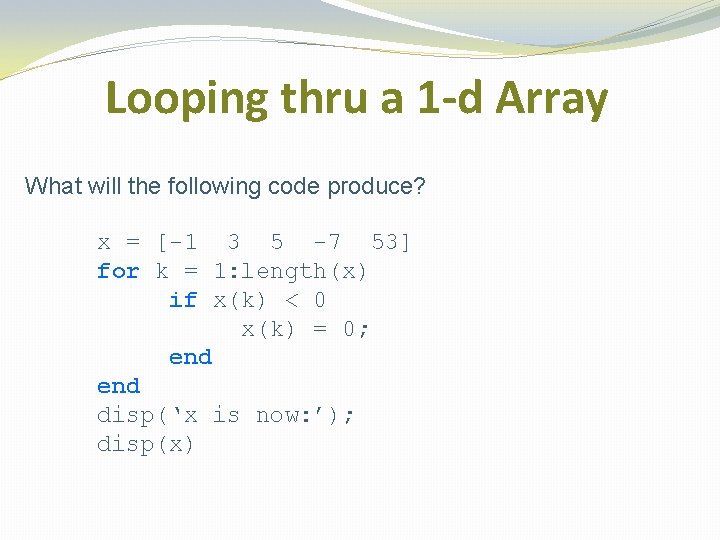
Looping thru a 1 -d Array What will the following code produce? x = [-1 3 5 -7 53] for k = 1: length(x) if x(k) < 0 x(k) = 0; end disp(‘x is now: ’); disp(x)
![Looping thru a 1 d Array x 1 3 5 7 53 for Looping thru a 1 -d Array x = [-1 3 5 -7 53] for](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-17.jpg)
Looping thru a 1 -d Array x = [-1 3 5 -7 53] for k = 1: length(x) if x(k) < 0 x(k) = 0; end disp(‘x is now: ’); disp(x) k=1 x(1) < 0? Yes Set x(1) = 0 x = [0 3 5 -7 53] k = 2 x(2) < 0? No x = [0 3 5 -7 53] k = 3 x(3) < 0? No x = [0 3 5 -7 53] k=4 x(4) < 0? Yes Set x(4) = 0 x = [0 3 5 0 53] k = 5 x(5) < 0? x is now: 0 3 5 0 No 53
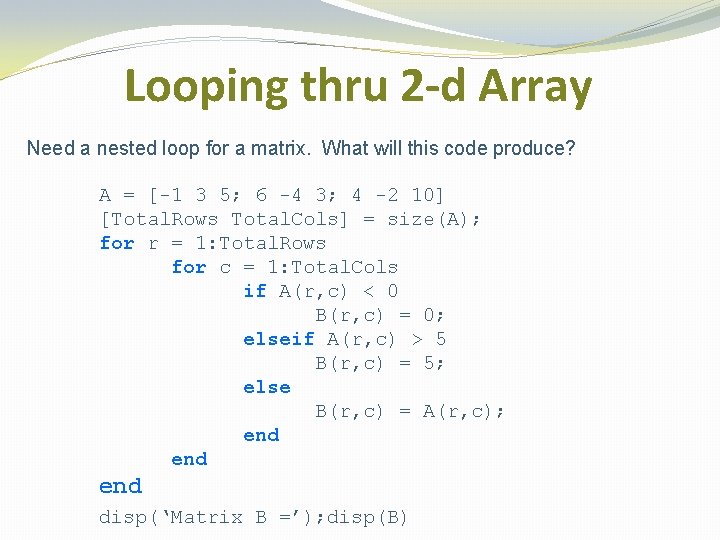
Looping thru 2 -d Array Need a nested loop for a matrix. What will this code produce? A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B)
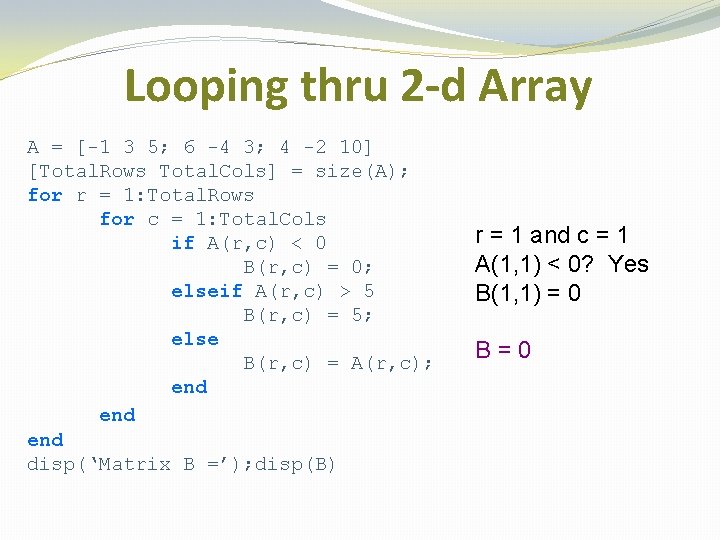
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 1 and c = 1 A(1, 1) < 0? Yes B(1, 1) = 0 B=0
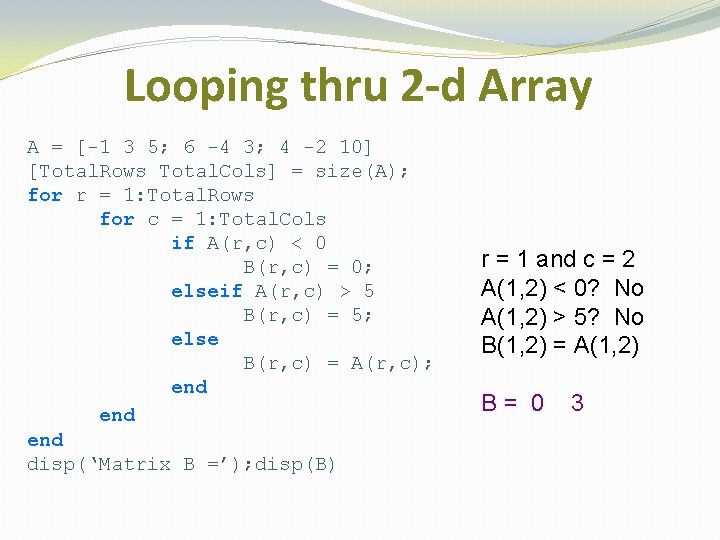
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 1 and c = 2 A(1, 2) < 0? No A(1, 2) > 5? No B(1, 2) = A(1, 2) B= 0 3
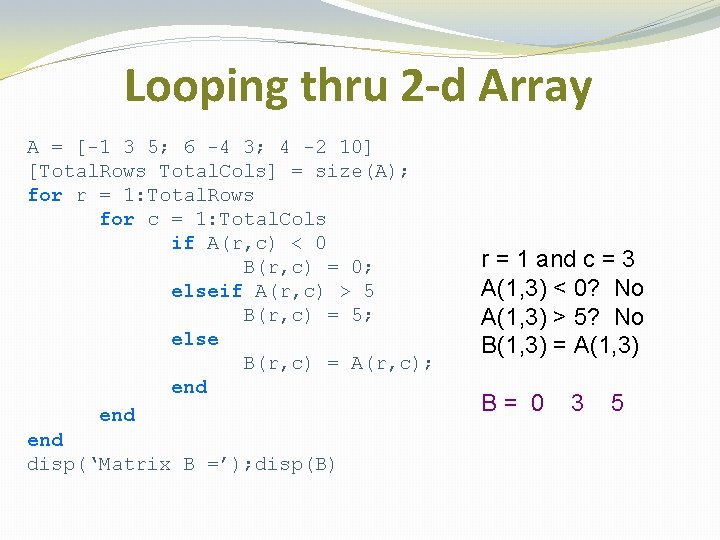
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 1 and c = 3 A(1, 3) < 0? No A(1, 3) > 5? No B(1, 3) = A(1, 3) B= 0 3 5
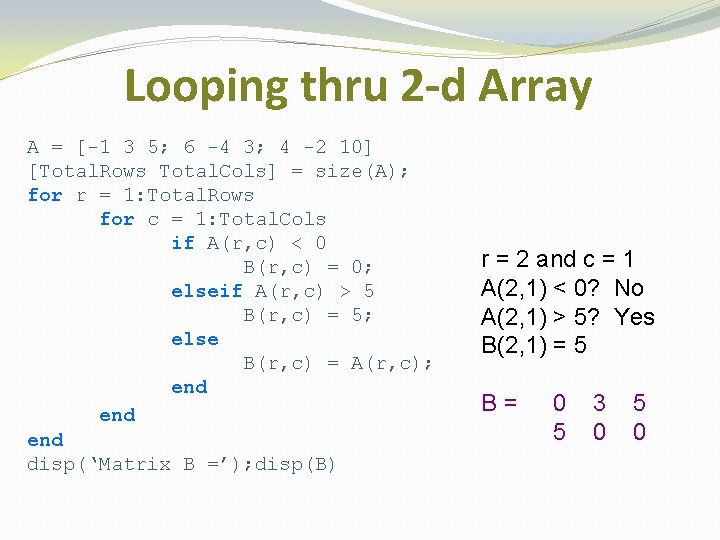
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 2 and c = 1 A(2, 1) < 0? No A(2, 1) > 5? Yes B(2, 1) = 5 B= 0 5 3 0 5 0
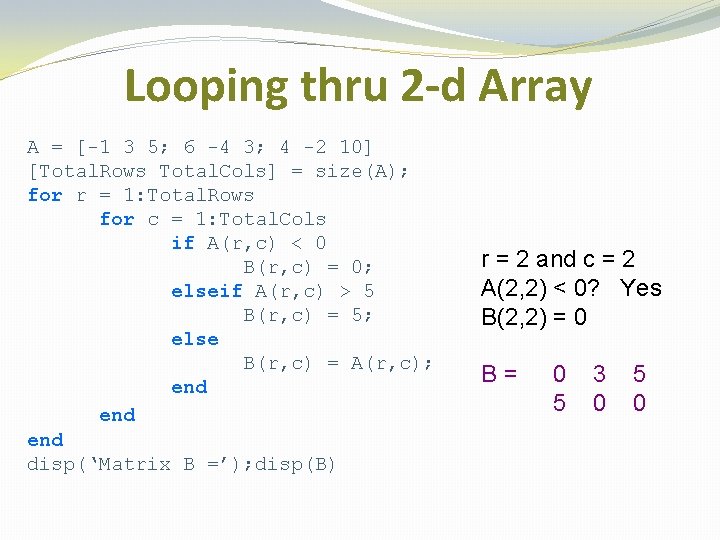
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 2 and c = 2 A(2, 2) < 0? Yes B(2, 2) = 0 B= 0 5 3 0 5 0
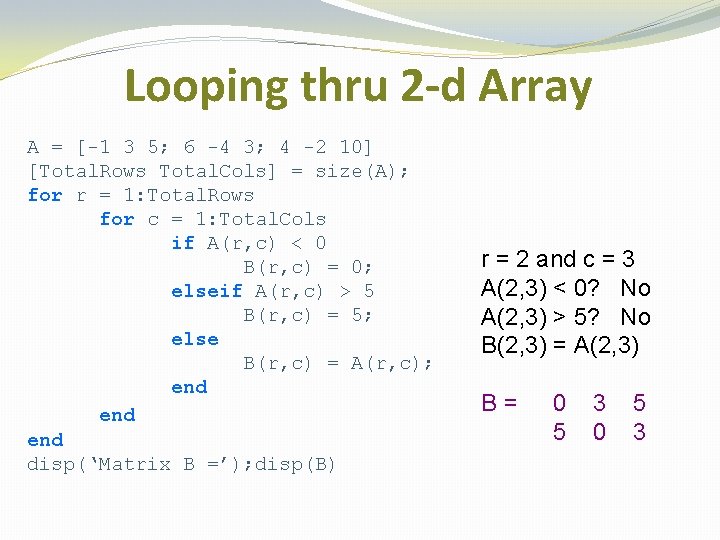
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 2 and c = 3 A(2, 3) < 0? No A(2, 3) > 5? No B(2, 3) = A(2, 3) B= 0 5 3
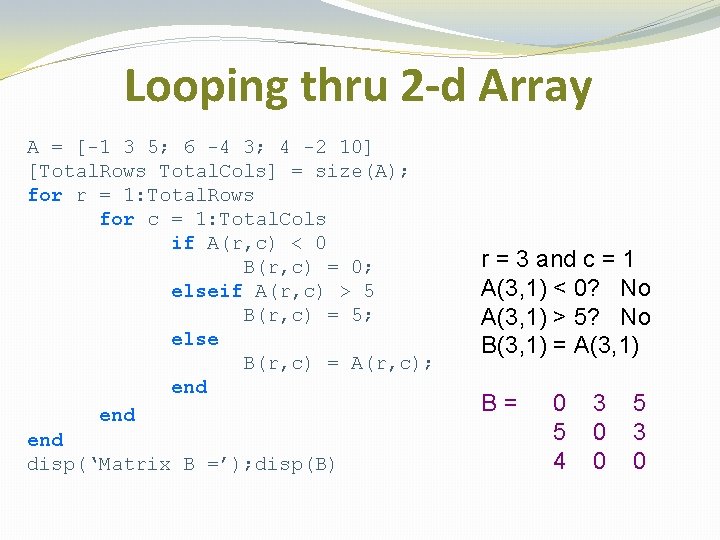
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 3 and c = 1 A(3, 1) < 0? No A(3, 1) > 5? No B(3, 1) = A(3, 1) B= 0 5 4 3 0 0 5 3 0
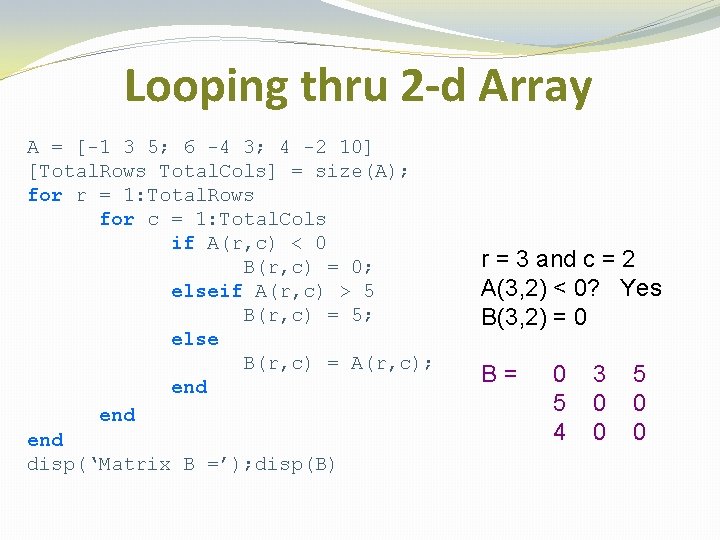
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 3 and c = 2 A(3, 2) < 0? Yes B(3, 2) = 0 B= 0 5 4 3 0 0 5 0 0
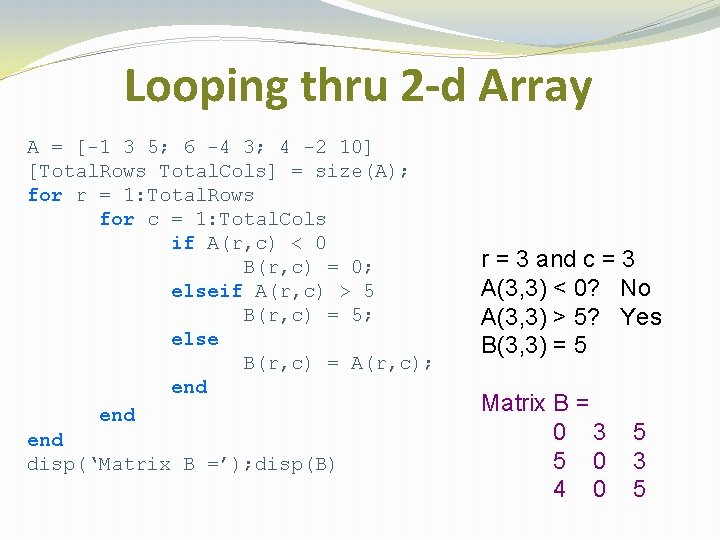
Looping thru 2 -d Array A = [-1 3 5; 6 -4 3; 4 -2 10] [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B) r = 3 and c = 3 A(3, 3) < 0? No A(3, 3) > 5? Yes B(3, 3) = 5 Matrix B = 0 3 5 0 4 0 5 3 5
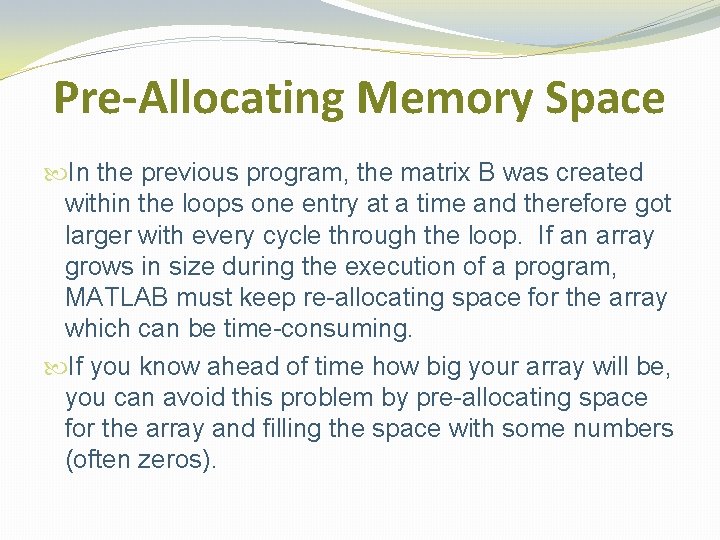
Pre-Allocating Memory Space In the previous program, the matrix B was created within the loops one entry at a time and therefore got larger with every cycle through the loop. If an array grows in size during the execution of a program, MATLAB must keep re-allocating space for the array which can be time-consuming. If you know ahead of time how big your array will be, you can avoid this problem by pre-allocating space for the array and filling the space with some numbers (often zeros).
![Pre Allocating Space A 1 3 5 6 4 3 4 2 5 Pre –Allocating Space A = [-1 3 5; 6 -4 3; 4 -2 5]](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-29.jpg)
Pre –Allocating Space A = [-1 3 5; 6 -4 3; 4 -2 5] B = zeros(size(A)); % Create a matrix B of 0 s [Total. Rows Total. Cols] = size(A); for r = 1: Total. Rows for c = 1: Total. Cols if A(r, c) < 0 B(r, c) = 0; elseif A(r, c) > 5 B(r, c) = 5; else B(r, c) = A(r, c); end end disp(‘Matrix B =’); disp(B)
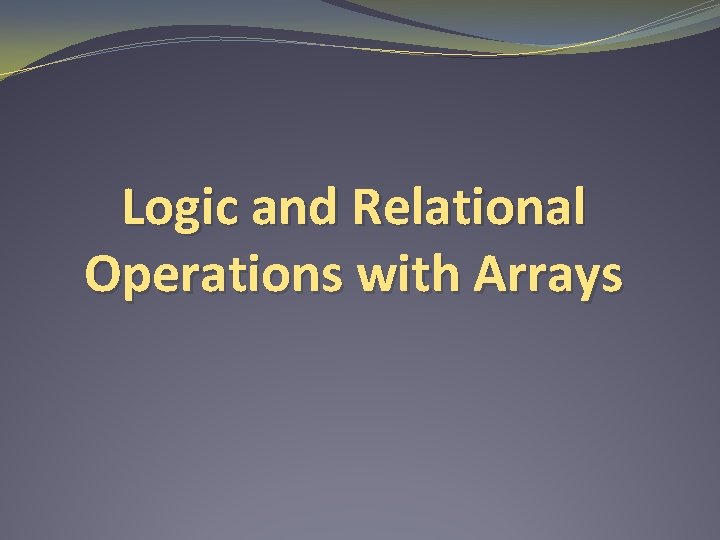
Logic and Relational Operations with Arrays
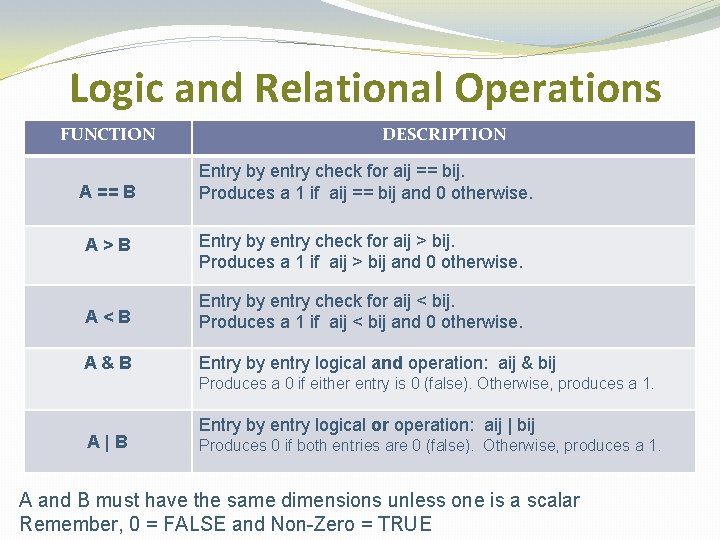
Logic and Relational Operations FUNCTION A == B DESCRIPTION Entry by entry check for aij == bij. Produces a 1 if aij == bij and 0 otherwise. A>B Entry by entry check for aij > bij. Produces a 1 if aij > bij and 0 otherwise. A<B Entry by entry check for aij < bij. Produces a 1 if aij < bij and 0 otherwise. A&B Entry by entry logical and operation: aij & bij Produces a 0 if either entry is 0 (false). Otherwise, produces a 1. A|B Entry by entry logical or operation: aij | bij Produces 0 if both entries are 0 (false). Otherwise, produces a 1. A and B must have the same dimensions unless one is a scalar Remember, 0 = FALSE and Non-Zero = TRUE
![Examples A randi 10 10 4 3 A 7 Examples >> A = randi ( [-10 10] , [4 3] ) A= 7](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-32.jpg)
Examples >> A = randi ( [-10 10] , [4 3] ) A= 7 9 -8 9 3 -8 -5 1 10 10 -7 10 >> A > 5 ans = 1 1 0 0 0 0 1 1 0 1
![Examples A 1 5 3 4 A 1 5 3 4 Examples >> A = [-1 5; 3 4] A = -1 5 3 4](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-33.jpg)
Examples >> A = [-1 5; 3 4] A = -1 5 3 4 >> B = [2 10; 3 1] B = 2 10 3 1 >> A == B ans = 0 1 0 0
![Examples A 1 5 3 4 A 1 5 3 4 Examples >> A = [-1 5; 3 4] A = -1 5 3 4](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-34.jpg)
Examples >> A = [-1 5; 3 4] A = -1 5 3 4 >> B = [2 10; 3 1] B = 2 10 3 1 >> A > B ans = 0 0 0 1
![Examples A 1 5 3 4 A 1 5 3 4 Examples >> A = [-1 5; 3 4] A = -1 5 3 4](https://slidetodoc.com/presentation_image_h2/8383d4ea3810d1e56c13f609c11949f1/image-35.jpg)
Examples >> A = [-1 5; 3 4] A = -1 5 3 4 >> B = [2 10; 3 1] B = 2 10 3 1 >> A >= B ans = 0 1
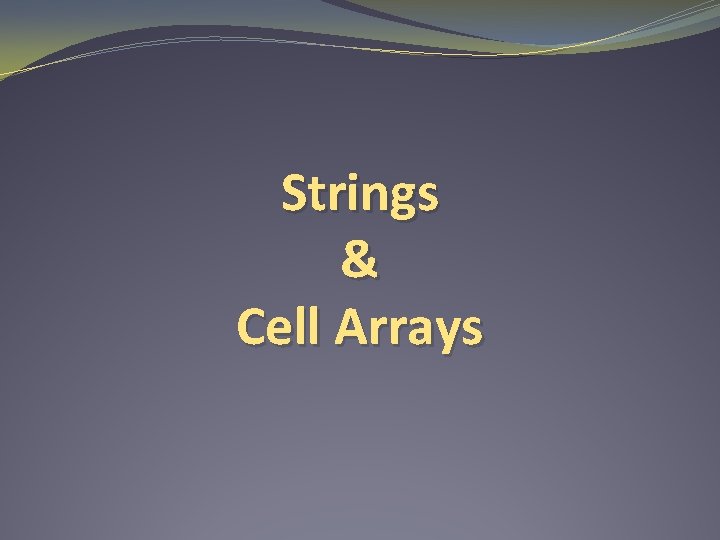
Strings & Cell Arrays
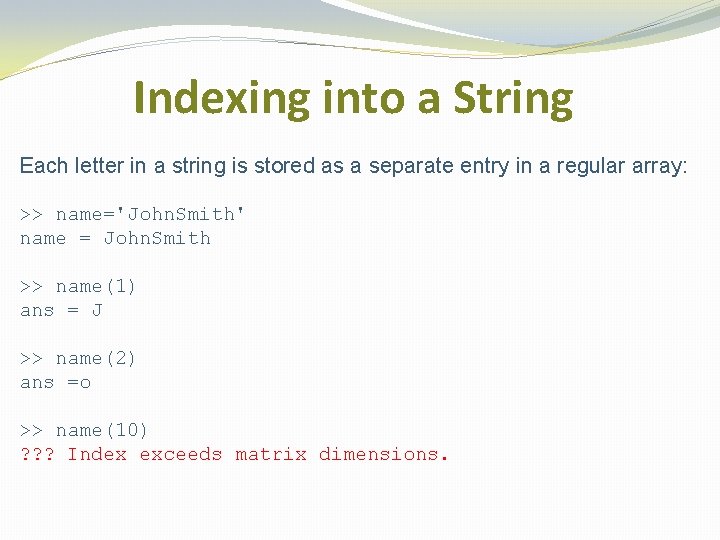
Indexing into a String Each letter in a string is stored as a separate entry in a regular array: >> name='John. Smith' name = John. Smith >> name(1) ans = J >> name(2) ans =o >> name(10) ? ? ? Index exceeds matrix dimensions.
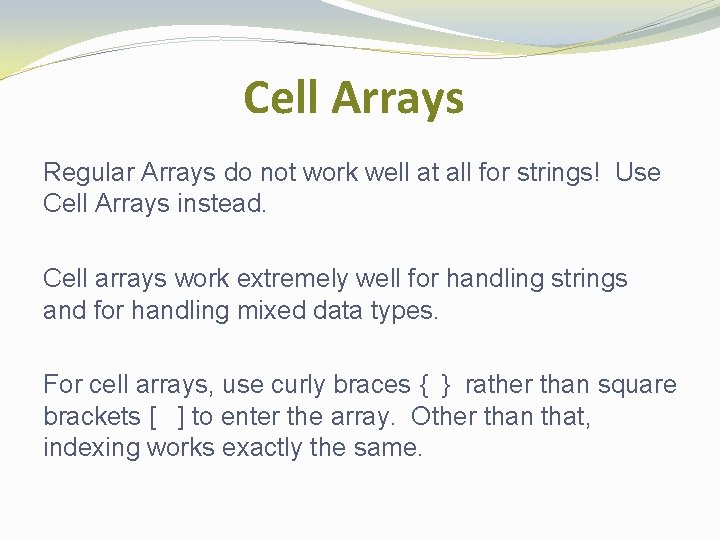
Cell Arrays Regular Arrays do not work well at all for strings! Use Cell Arrays instead. Cell arrays work extremely well for handling strings and for handling mixed data types. For cell arrays, use curly braces { } rather than square brackets [ ] to enter the array. Other than that, indexing works exactly the same.
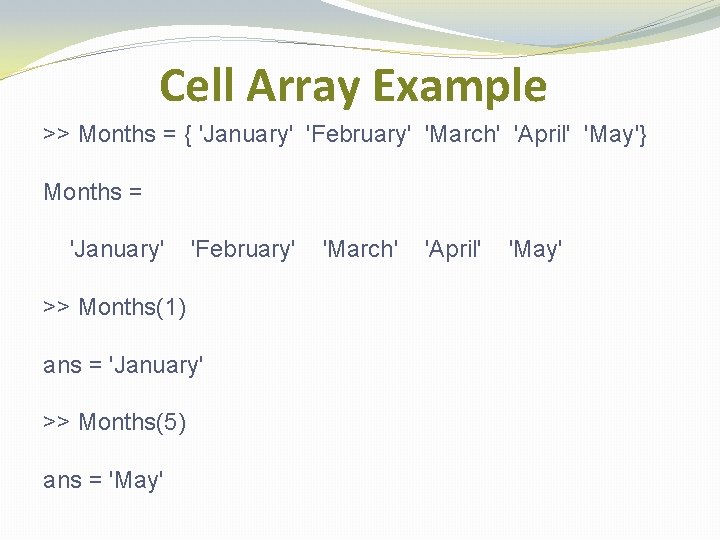
Cell Array Example >> Months = { 'January' 'February' 'March' 'April' 'May'} Months = 'January' 'February' >> Months(1) ans = 'January' >> Months(5) ans = 'May' 'March' 'April' 'May'
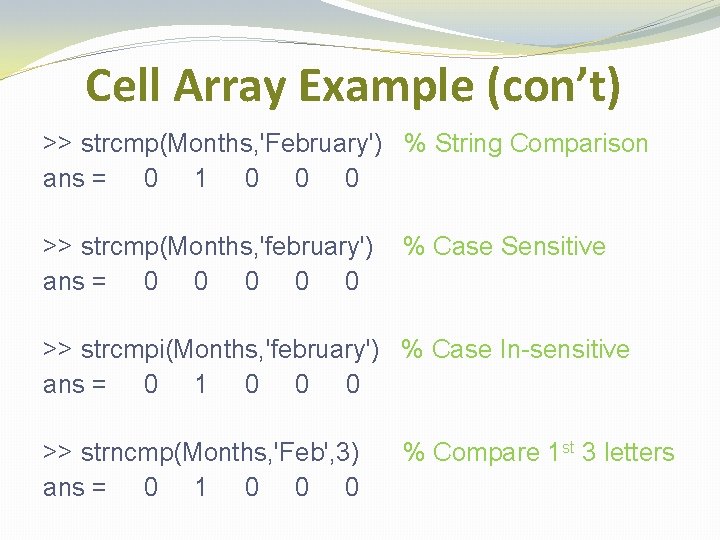
Cell Array Example (con’t) >> strcmp(Months, 'February') % String Comparison ans = 0 1 0 0 0 >> strcmp(Months, 'february') ans = 0 0 0 % Case Sensitive >> strcmpi(Months, 'february') % Case In-sensitive ans = 0 1 0 0 0 >> strncmp(Months, 'Feb', 3) ans = 0 1 0 0 0 % Compare 1 st 3 letters
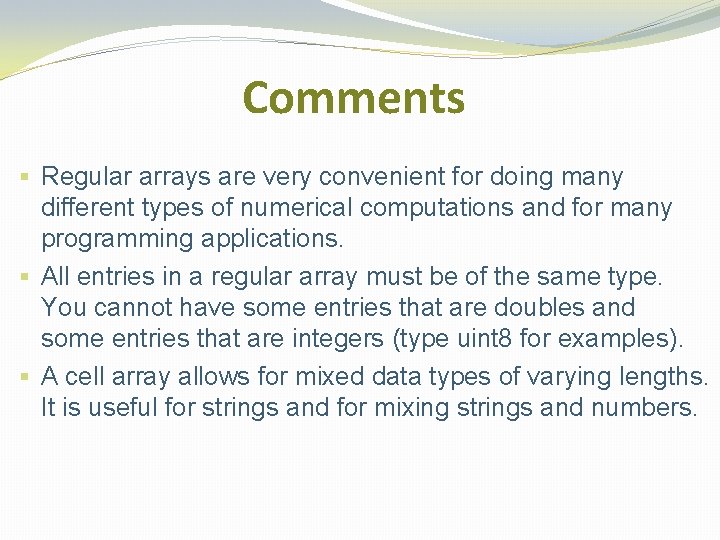
Comments § Regular arrays are very convenient for doing many different types of numerical computations and for many programming applications. § All entries in a regular array must be of the same type. You cannot have some entries that are doubles and some entries that are integers (type uint 8 for examples). § A cell array allows for mixed data types of varying lengths. It is useful for strings and for mixing strings and numbers.
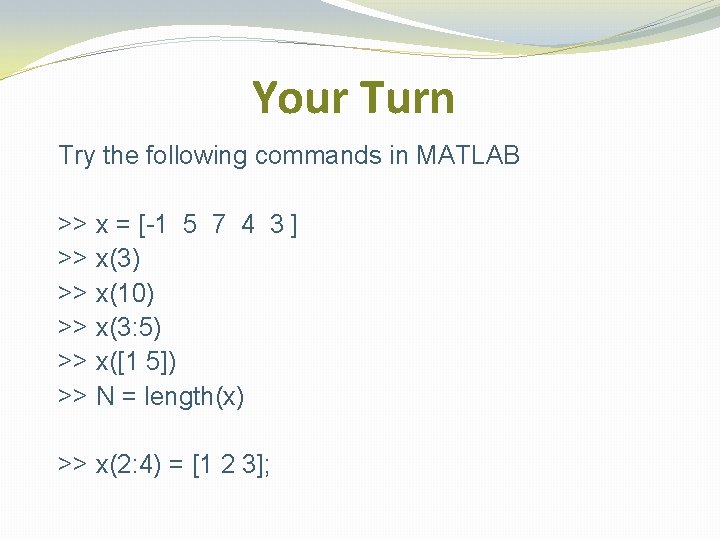
Your Turn Try the following commands in MATLAB >> x = [-1 5 7 4 3 ] >> x(3) >> x(10) >> x(3: 5) >> x([1 5]) >> N = length(x) >> x(2: 4) = [1 2 3];
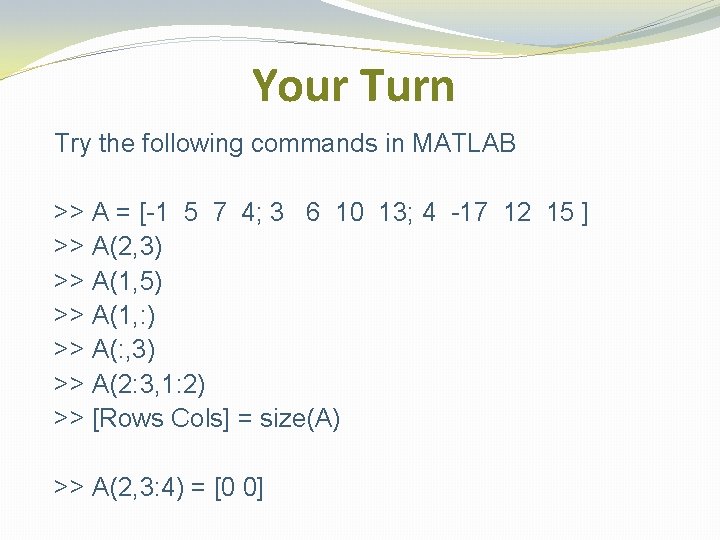
Your Turn Try the following commands in MATLAB >> A = [-1 5 7 4; 3 6 10 13; 4 -17 12 15 ] >> A(2, 3) >> A(1, 5) >> A(1, : ) >> A(: , 3) >> A(2: 3, 1: 2) >> [Rows Cols] = size(A) >> A(2, 3: 4) = [0 0]
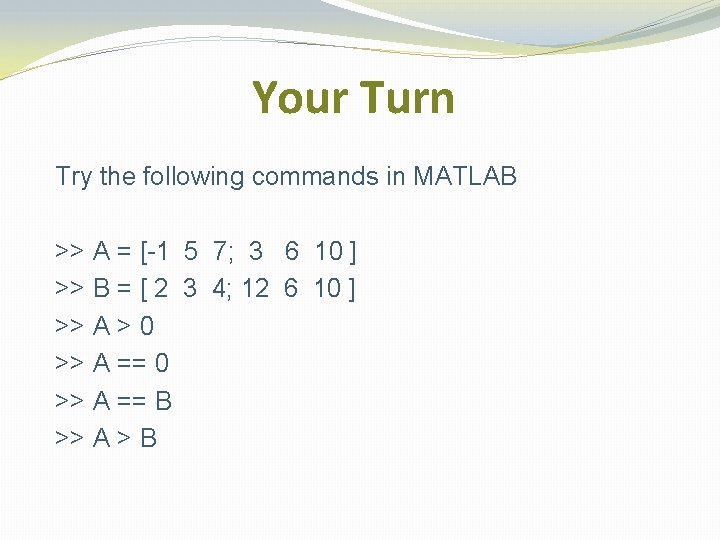
Your Turn Try the following commands in MATLAB >> A = [-1 5 7; 3 6 10 ] >> B = [ 2 3 4; 12 6 10 ] >> A > 0 >> A == B >> A > B
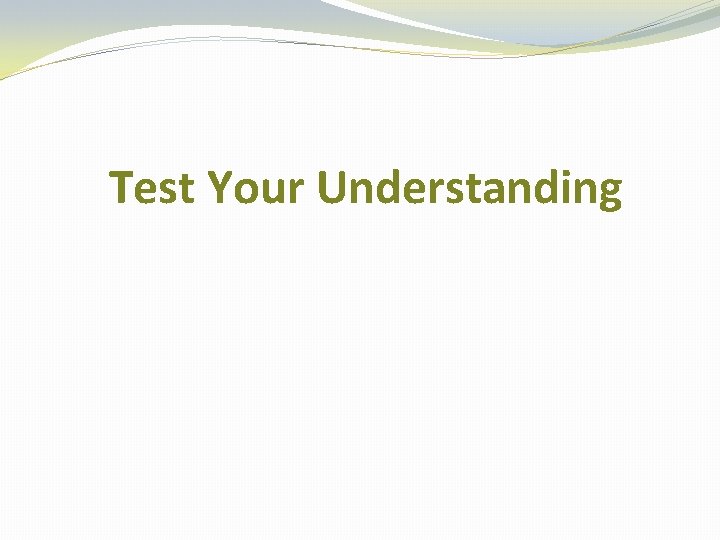
Test Your Understanding
Array of arrays c++
Jagged array vs multidimensional array
Sparse array adalah
Associative array vs indexed array
Array 2 dimension
Pin grid array
Endfire array
Photovoltaic array maximum power point tracking array
Deklarasi array x adalah
Larik adalah
Array is a collection of
Landsat collection 1 vs collection 2
Sightd
Day 3: arrays
Disadvantages of random access files
Dynamic array mips
Polynomial representation using arrays
潘仁義
Microled arrays
Redundant arrays of independent disks
Ragged array
Searching and sorting arrays in c++
Arreglos en java ejemplos
Raid redundant array of independent disks
Creating arrays matlab
Arrays in arm assembly
C++ parallel arrays
Are vectors dynamic arrays
Python list of arrays
How many arrays in 24
Arrays visual basic
Java arreglos bidimensionales
Small basic arrays
Array adt
Global arrays in c
Why do we need arrays?
Arrays
Arrays in pascal
Python parallel arrays
Array mips
Facts about arrays
Advantage of dynamic memory allocation
Parallel arrays
Java partially filled array
Computer science arrays
Dynamic arrays and amortized analysis