Algorithm simulation activity Dijkstras alg Single Source Shortest
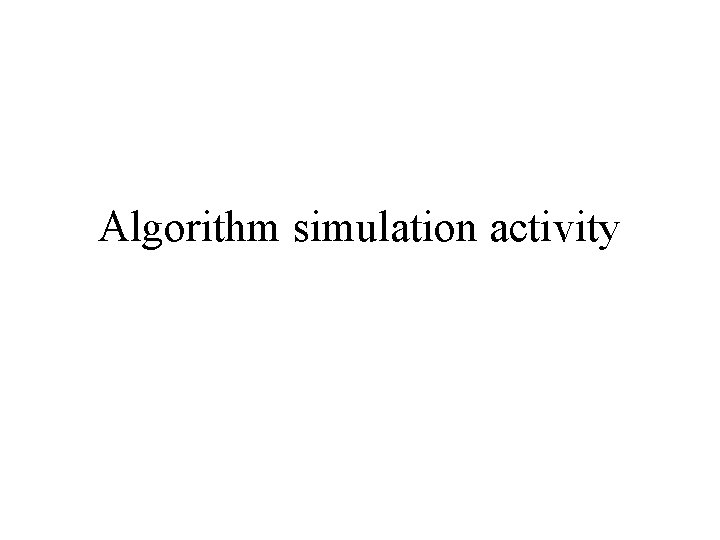
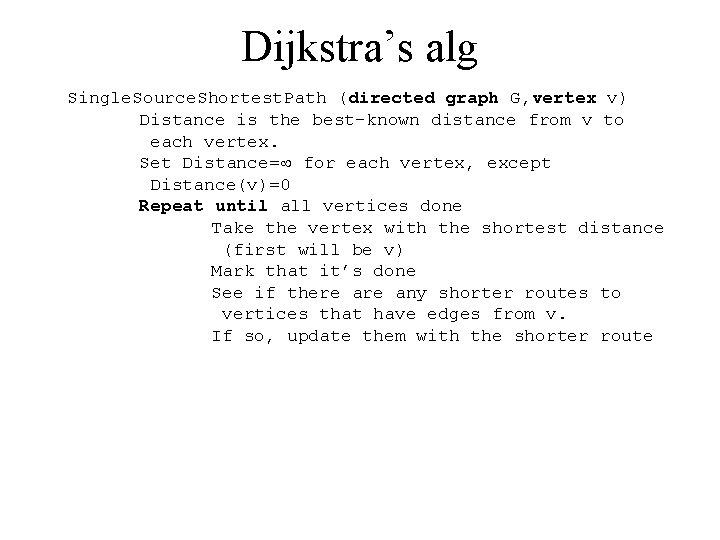
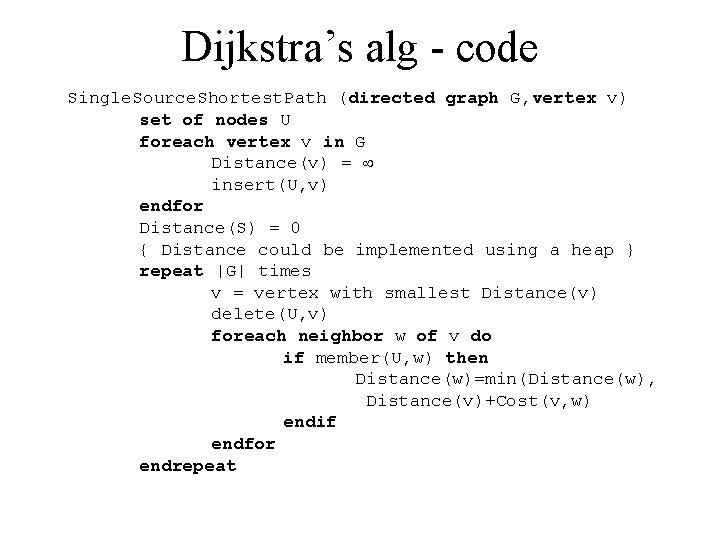
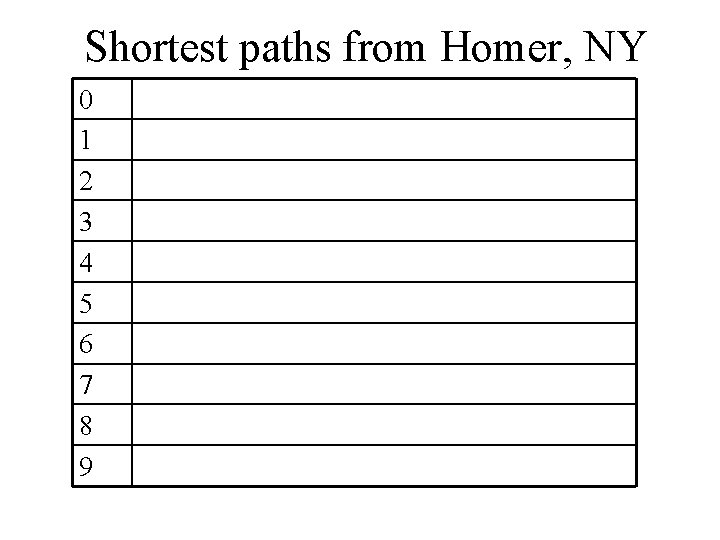
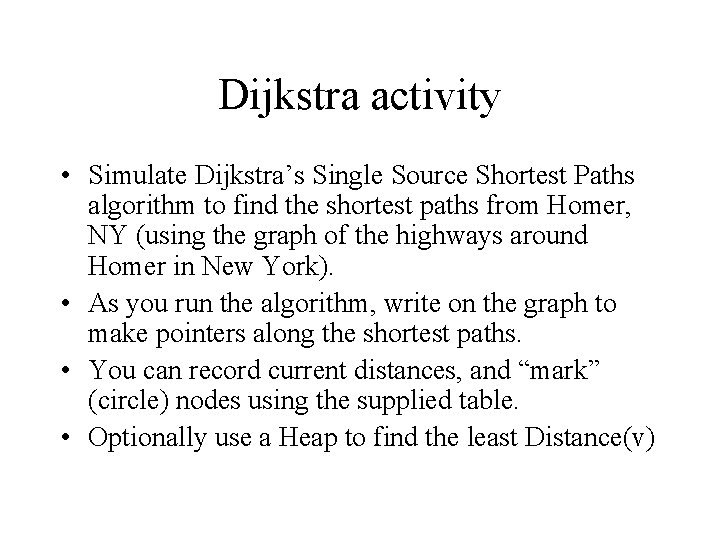
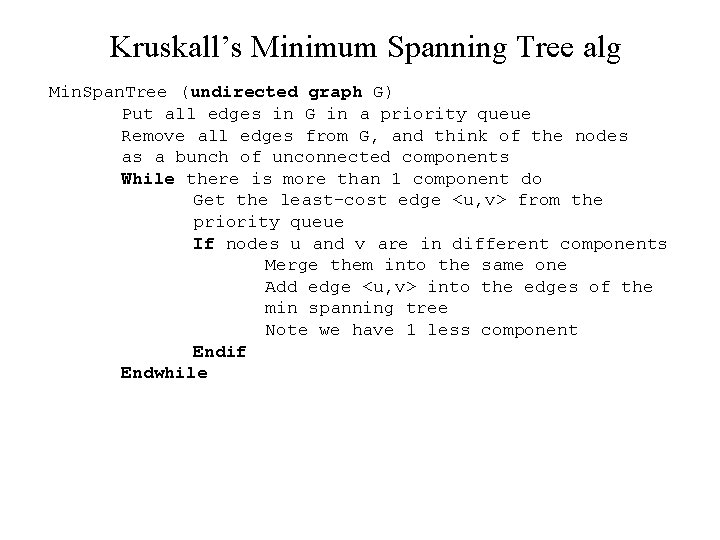
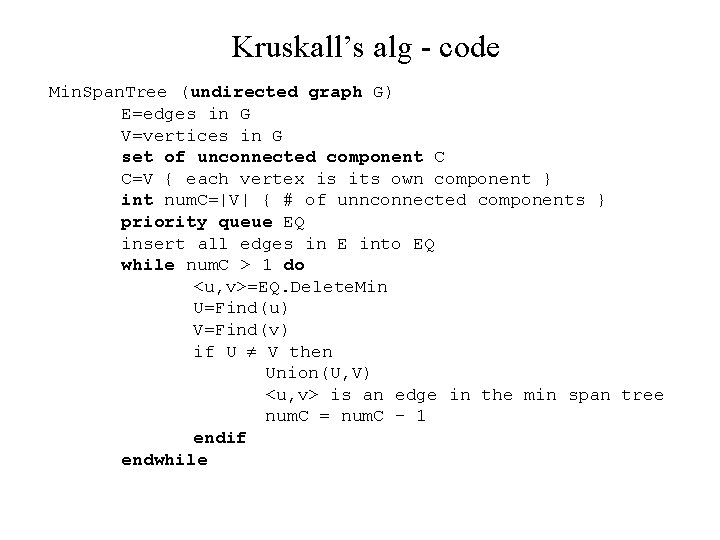
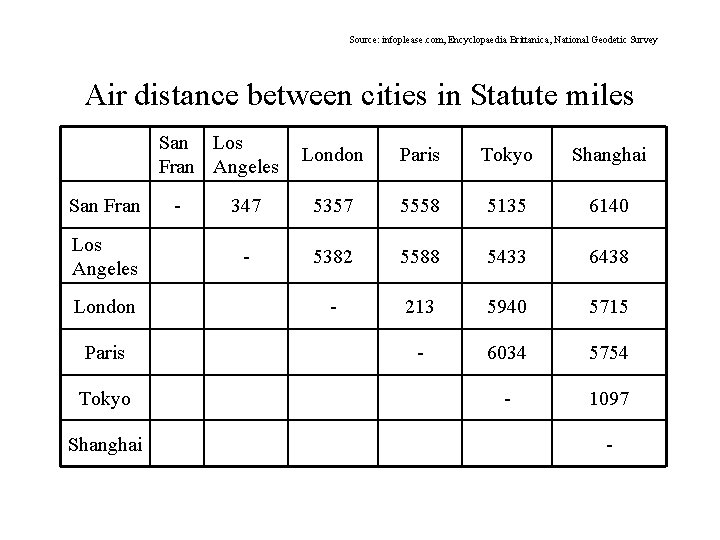
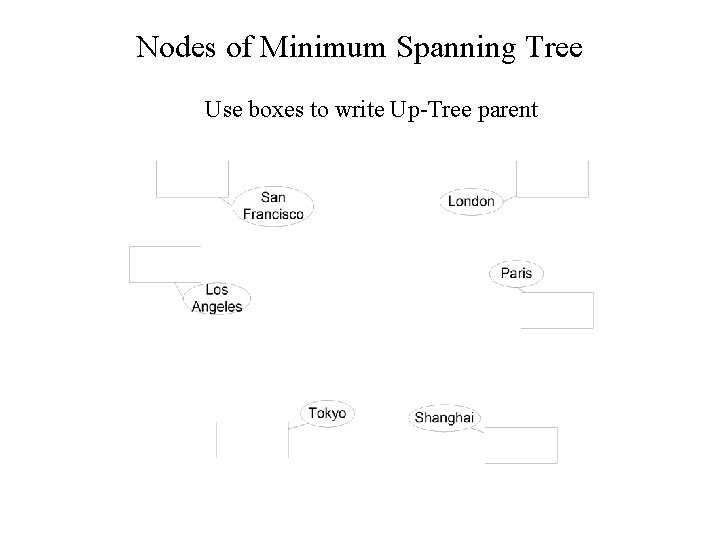
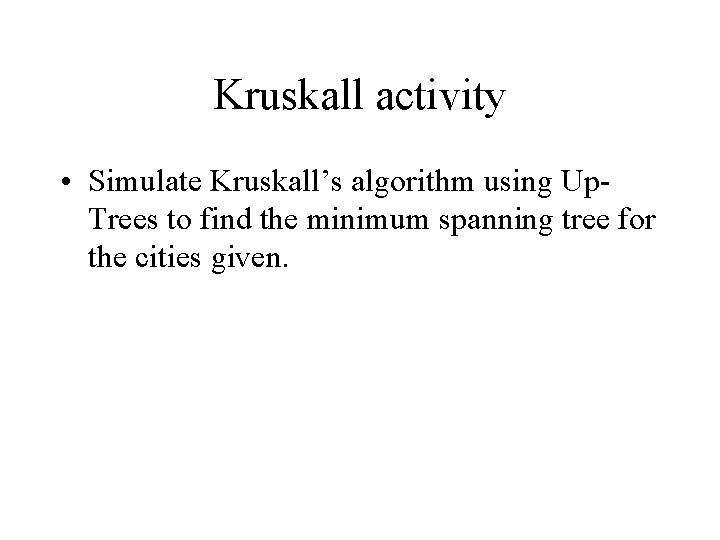
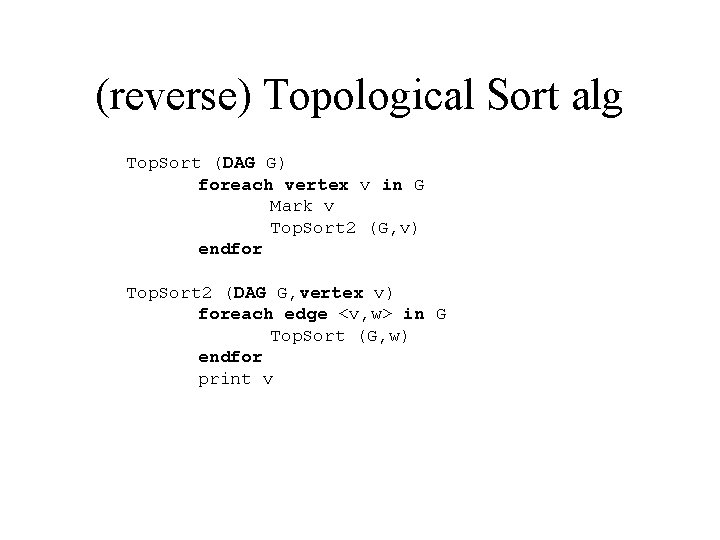
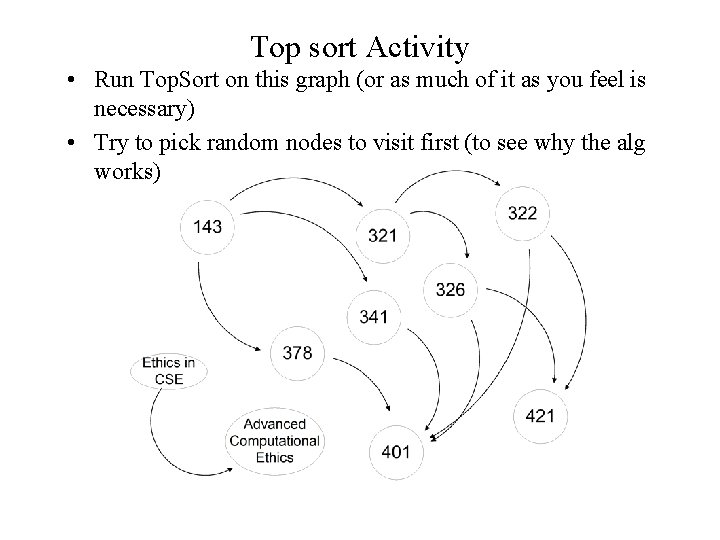
- Slides: 12
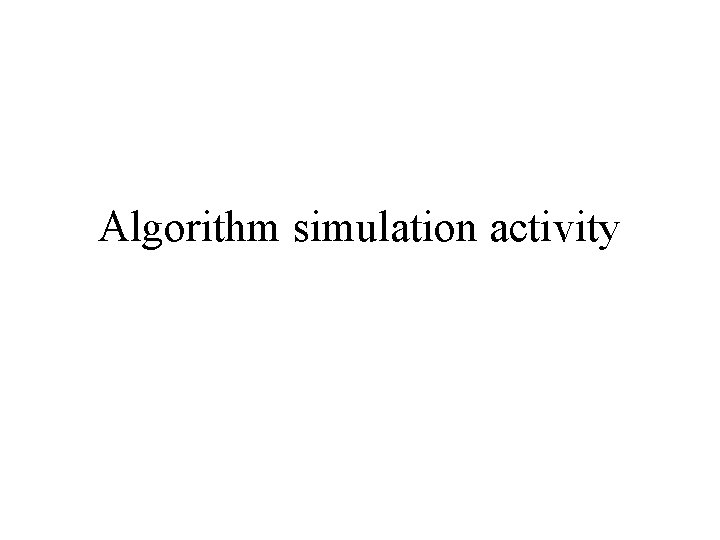
Algorithm simulation activity
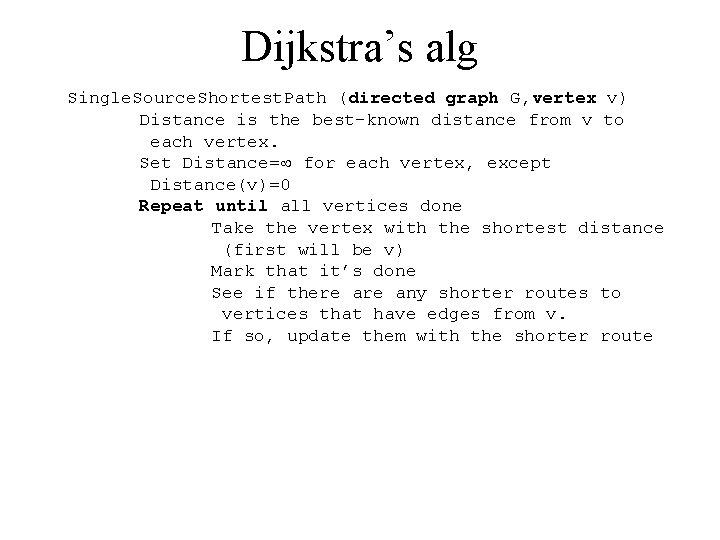
Dijkstra’s alg Single. Source. Shortest. Path (directed graph G, vertex v) Distance is the best-known distance from v to each vertex. Set Distance= for each vertex, except Distance(v)=0 Repeat until all vertices done Take the vertex with the shortest distance (first will be v) Mark that it’s done See if there any shorter routes to vertices that have edges from v. If so, update them with the shorter route
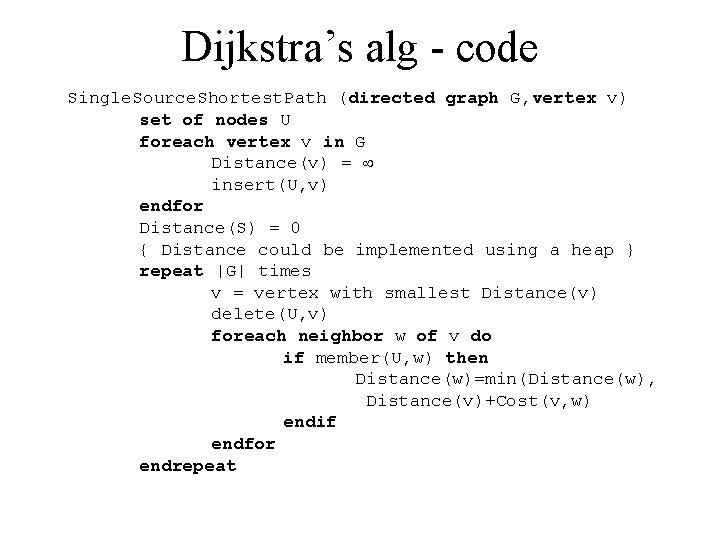
Dijkstra’s alg - code Single. Source. Shortest. Path (directed graph G, vertex v) set of nodes U foreach vertex v in G Distance(v) = insert(U, v) endfor Distance(S) = 0 { Distance could be implemented using a heap } repeat |G| times v = vertex with smallest Distance(v) delete(U, v) foreach neighbor w of v do if member(U, w) then Distance(w)=min(Distance(w), Distance(v)+Cost(v, w) endif endfor endrepeat
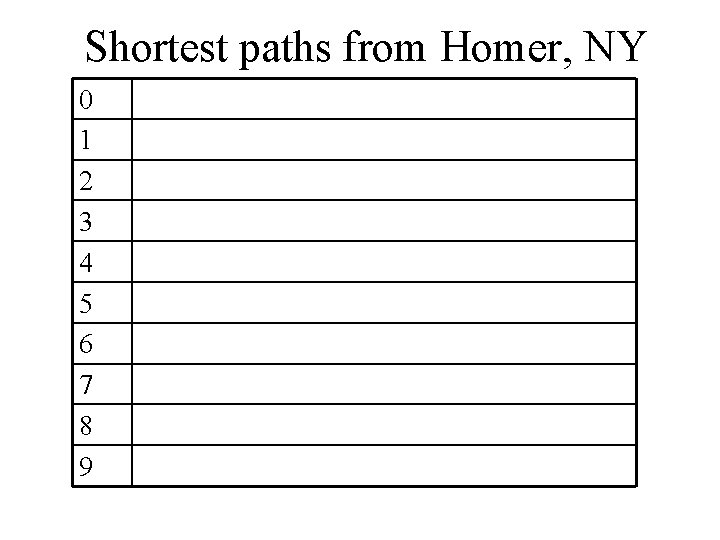
Shortest paths from Homer, NY 0 1 2 3 4 5 6 7 8 9
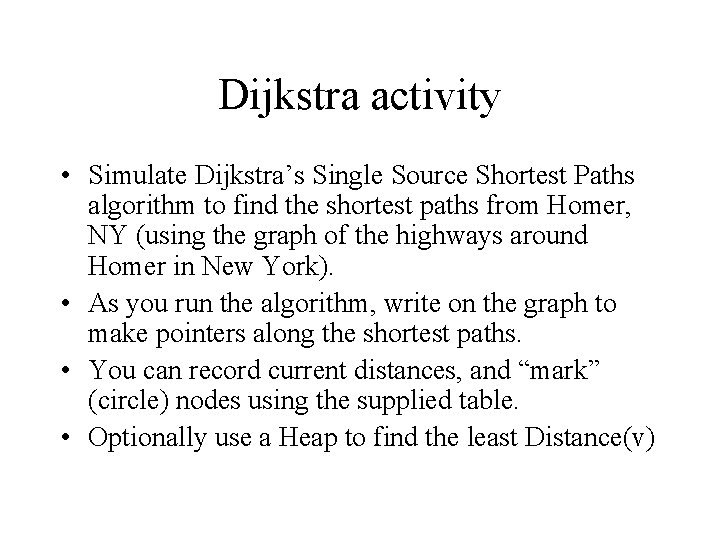
Dijkstra activity • Simulate Dijkstra’s Single Source Shortest Paths algorithm to find the shortest paths from Homer, NY (using the graph of the highways around Homer in New York). • As you run the algorithm, write on the graph to make pointers along the shortest paths. • You can record current distances, and “mark” (circle) nodes using the supplied table. • Optionally use a Heap to find the least Distance(v)
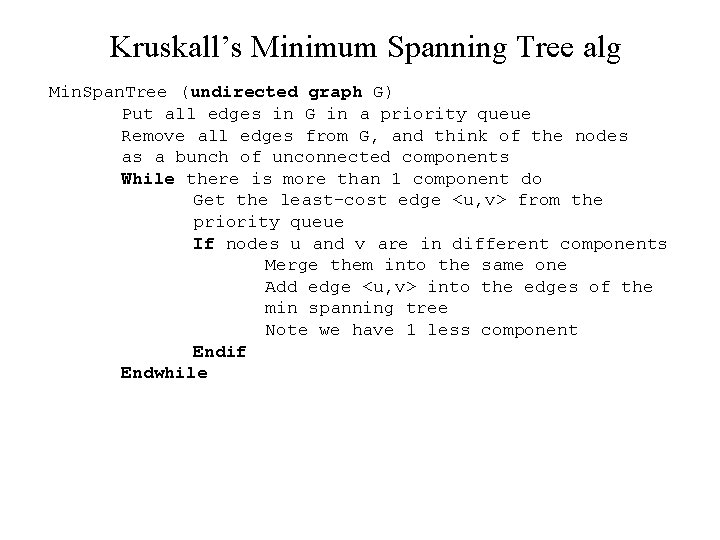
Kruskall’s Minimum Spanning Tree alg Min. Span. Tree (undirected graph G) Put all edges in G in a priority queue Remove all edges from G, and think of the nodes as a bunch of unconnected components While there is more than 1 component do Get the least-cost edge <u, v> from the priority queue If nodes u and v are in different components Merge them into the same one Add edge <u, v> into the edges of the min spanning tree Note we have 1 less component Endif Endwhile
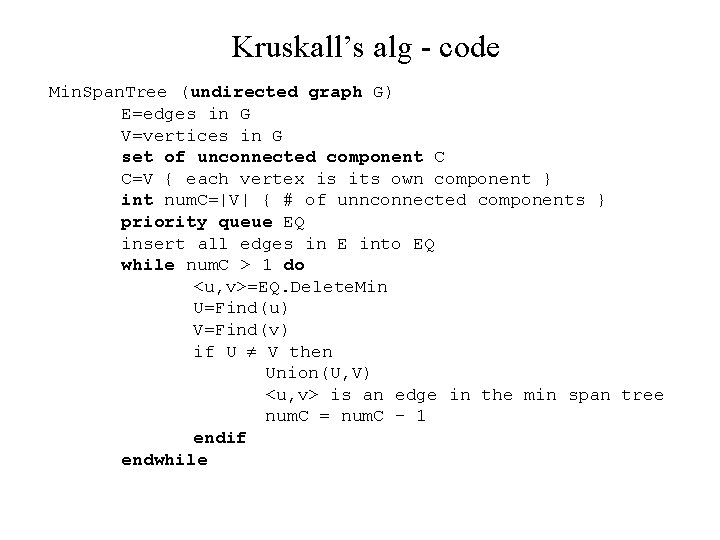
Kruskall’s alg - code Min. Span. Tree (undirected graph G) E=edges in G V=vertices in G set of unconnected component C C=V { each vertex is its own component } int num. C=|V| { # of unnconnected components } priority queue EQ insert all edges in E into EQ while num. C > 1 do <u, v>=EQ. Delete. Min U=Find(u) V=Find(v) if U V then Union(U, V) <u, v> is an edge in the min span tree num. C = num. C - 1 endif endwhile
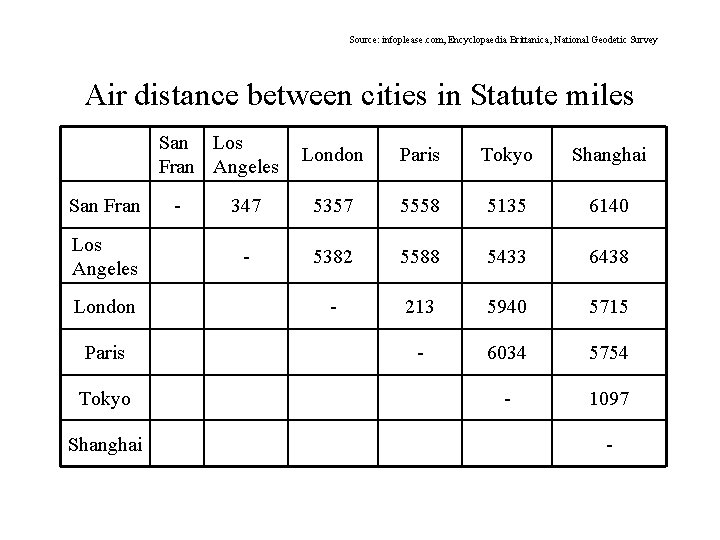
Source: infoplease. com, Encyclopaedia Brittanica, National Geodetic Survey Air distance between cities in Statute miles San Los Fran Angeles San Fran Los Angeles London Paris Tokyo Shanghai - London Paris Tokyo Shanghai 347 5357 5558 5135 6140 - 5382 5588 5433 6438 - 213 5940 5715 - 6034 5754 - 1097 -
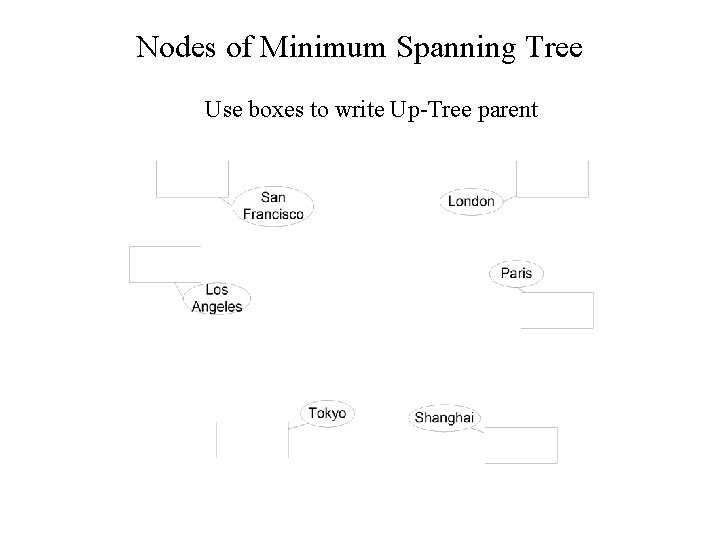
Nodes of Minimum Spanning Tree Use boxes to write Up-Tree parent
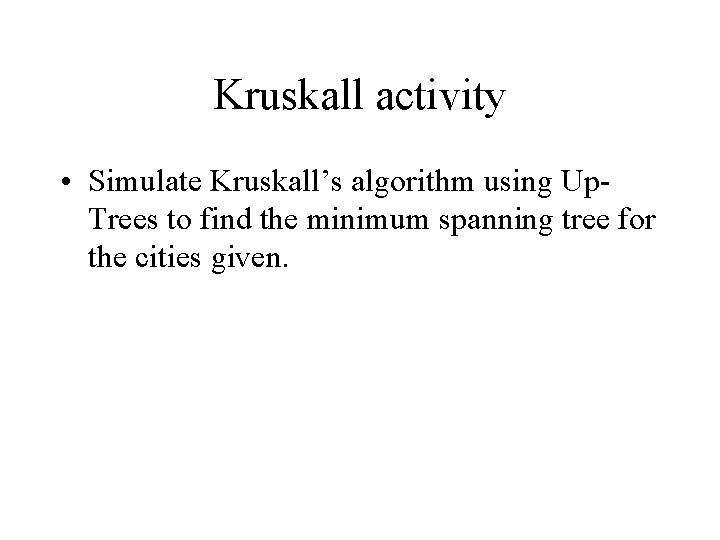
Kruskall activity • Simulate Kruskall’s algorithm using Up. Trees to find the minimum spanning tree for the cities given.
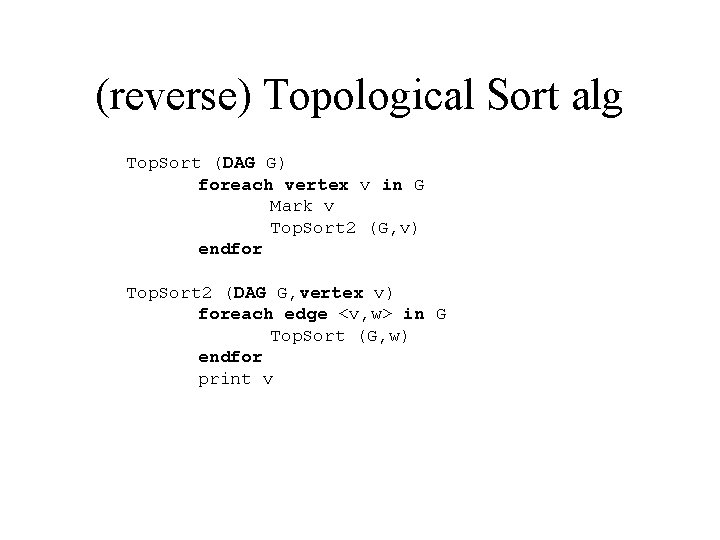
(reverse) Topological Sort alg Top. Sort (DAG G) foreach vertex v in G Mark v Top. Sort 2 (G, v) endfor Top. Sort 2 (DAG G, vertex v) foreach edge <v, w> in G Top. Sort (G, w) endfor print v
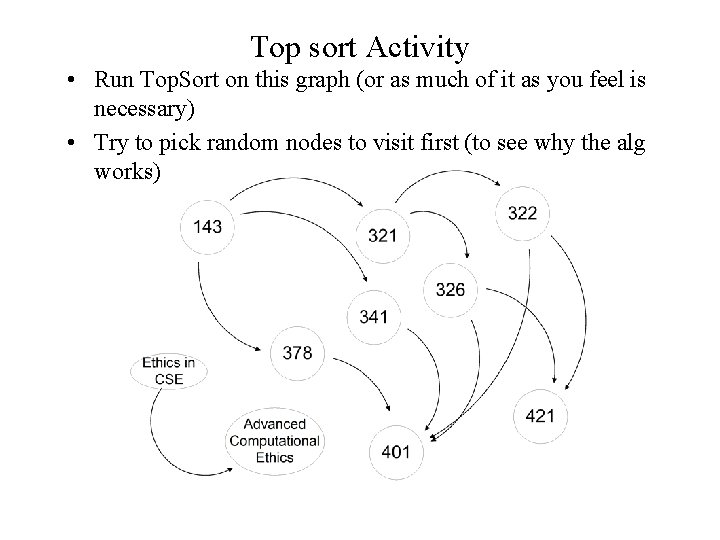
Top sort Activity • Run Top. Sort on this graph (or as much of it as you feel is necessary) • Try to pick random nodes to visit first (to see why the alg works)
Single-source shortest paths
Undirected
Single-source shortest paths
Single source shortest path in c
Shortest path from source to destination in weighted graph
Dijkstra's shortest path algorithm pseudocode
Define shortest path
Shortest path problems in discrete mathematics
Widest path problem dijkstra
Successive shortest path algorithm
Length of path
Shortest path algorithm in mapreduce
Sca 2021 calendar