Parallel Programming AllPairs Shortest Path CS 599 David
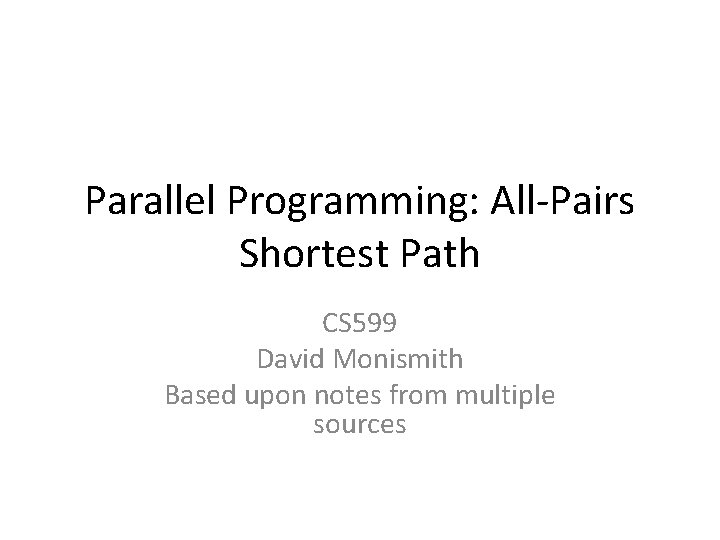
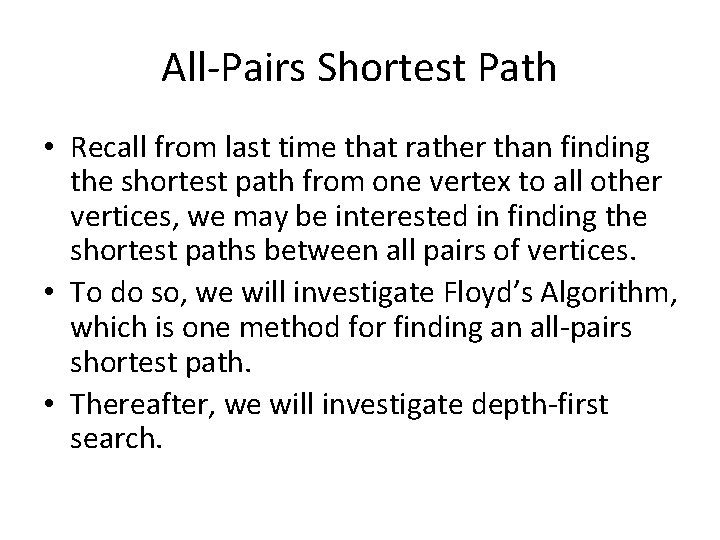
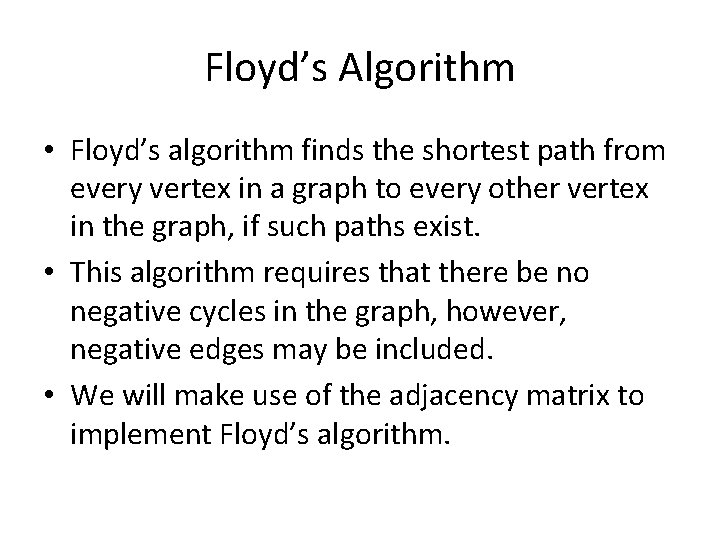
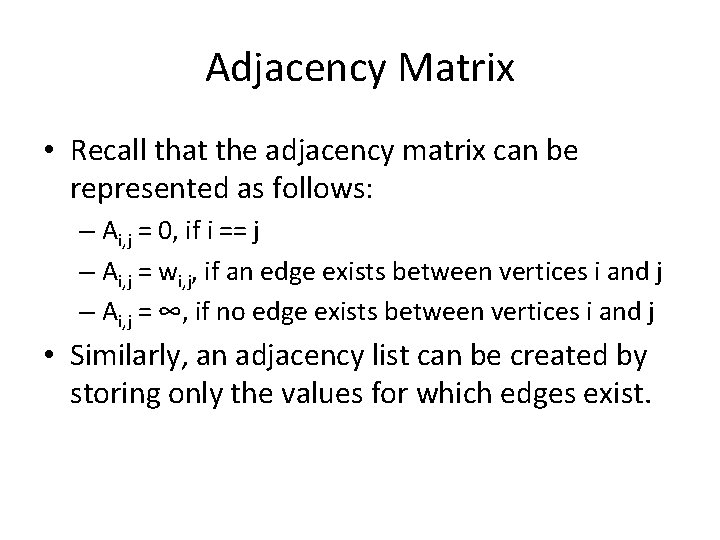
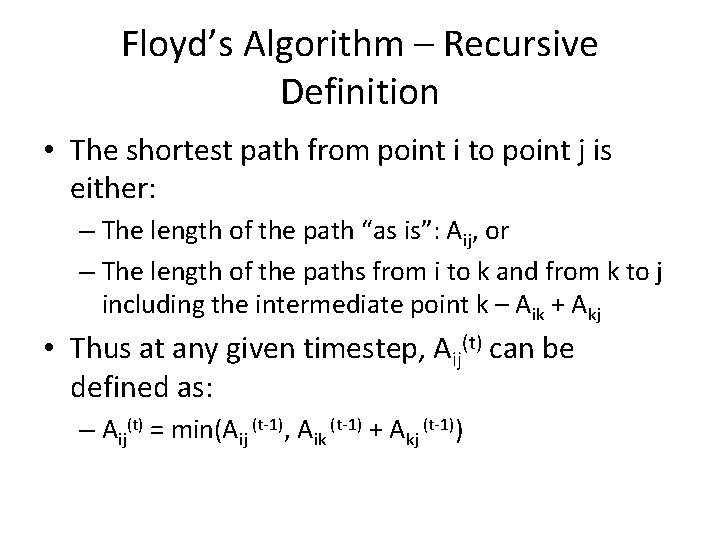
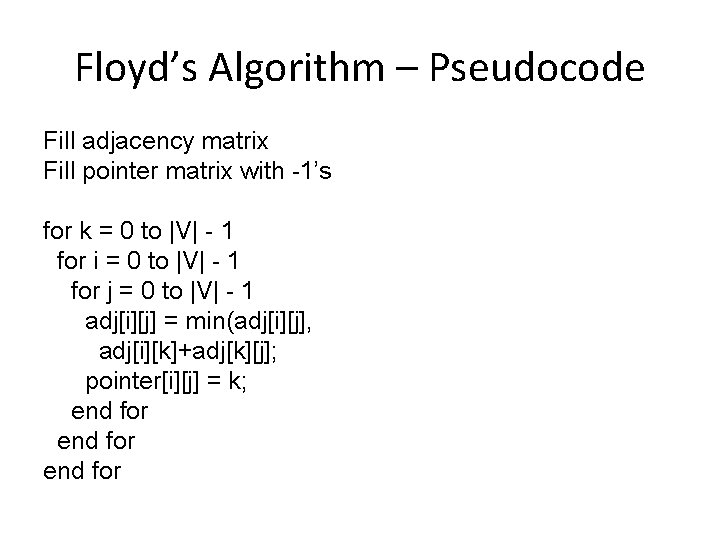
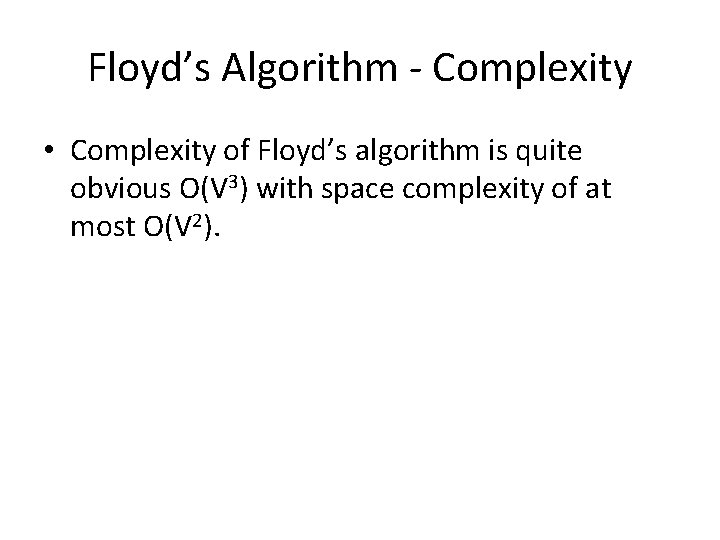
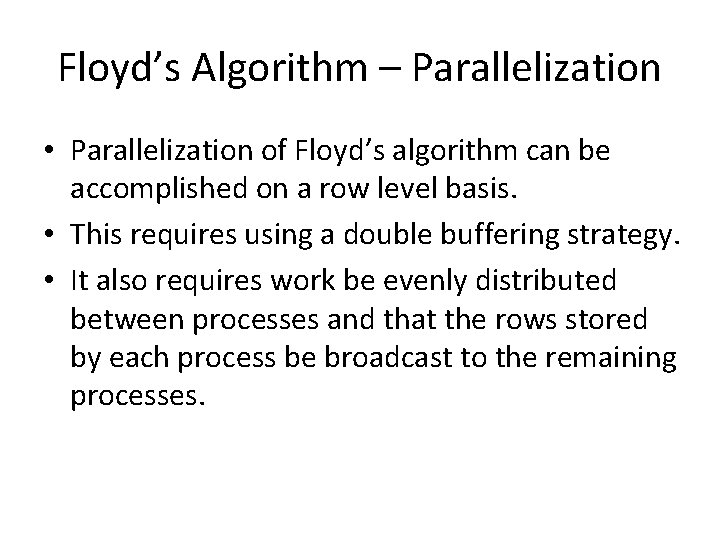
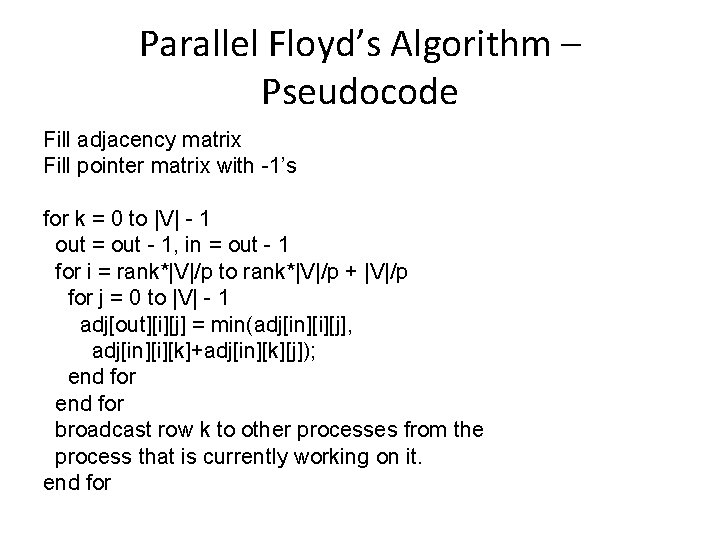
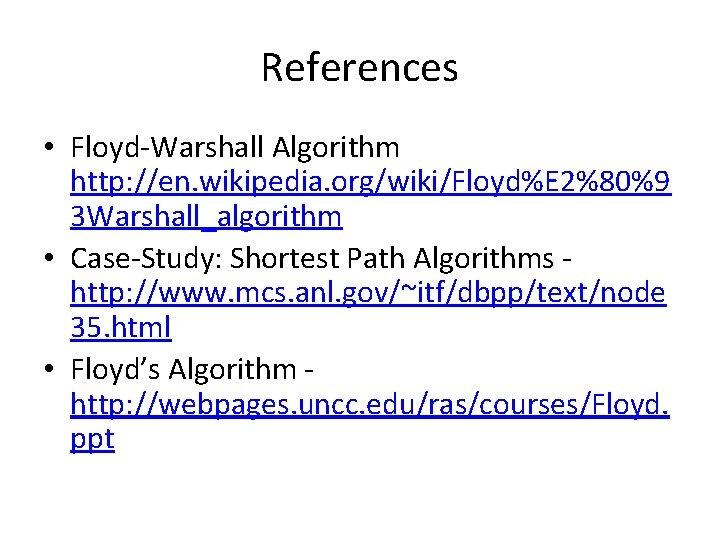
- Slides: 10
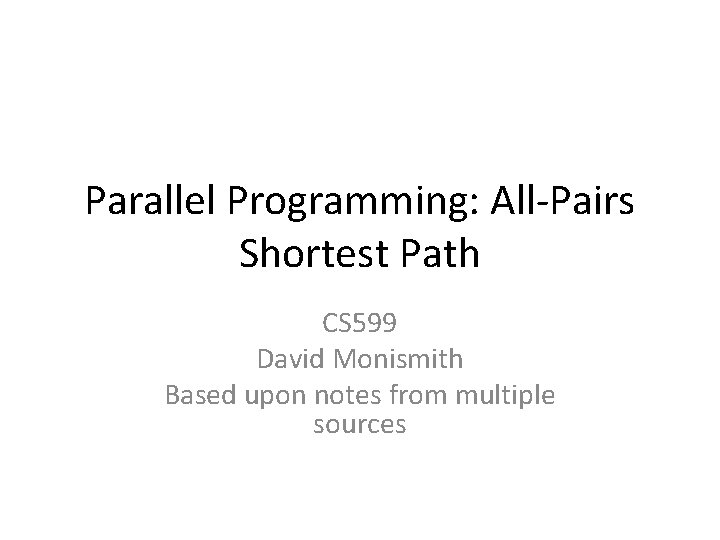
Parallel Programming: All-Pairs Shortest Path CS 599 David Monismith Based upon notes from multiple sources
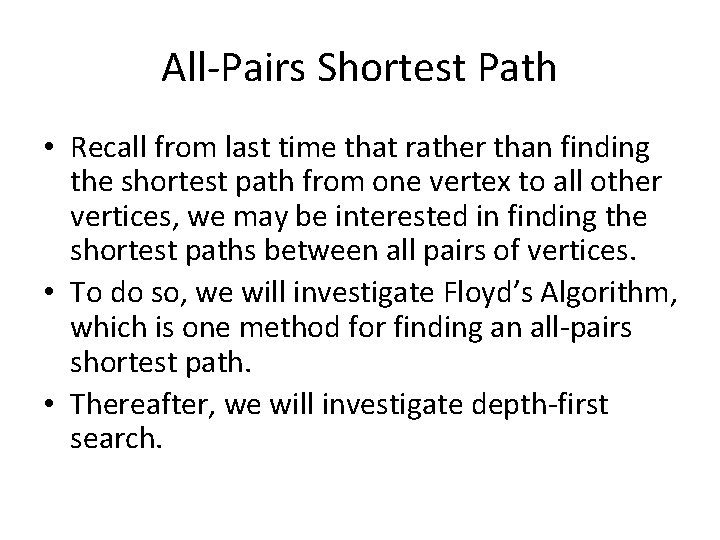
All-Pairs Shortest Path • Recall from last time that rather than finding the shortest path from one vertex to all other vertices, we may be interested in finding the shortest paths between all pairs of vertices. • To do so, we will investigate Floyd’s Algorithm, which is one method for finding an all-pairs shortest path. • Thereafter, we will investigate depth-first search.
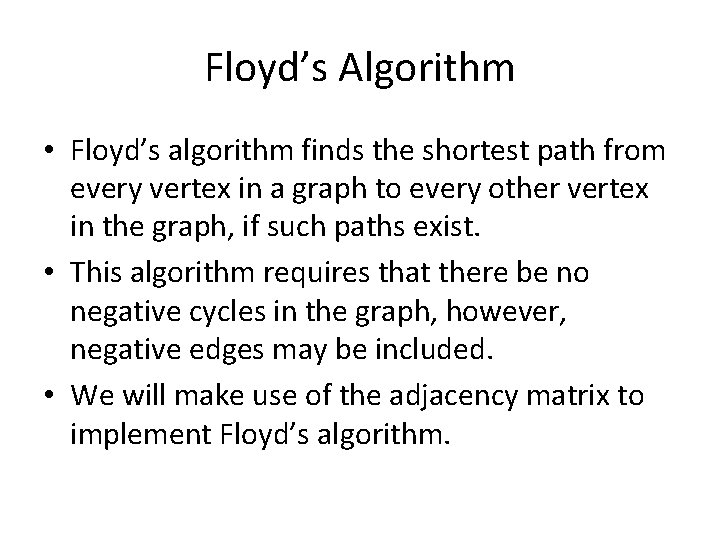
Floyd’s Algorithm • Floyd’s algorithm finds the shortest path from every vertex in a graph to every other vertex in the graph, if such paths exist. • This algorithm requires that there be no negative cycles in the graph, however, negative edges may be included. • We will make use of the adjacency matrix to implement Floyd’s algorithm.
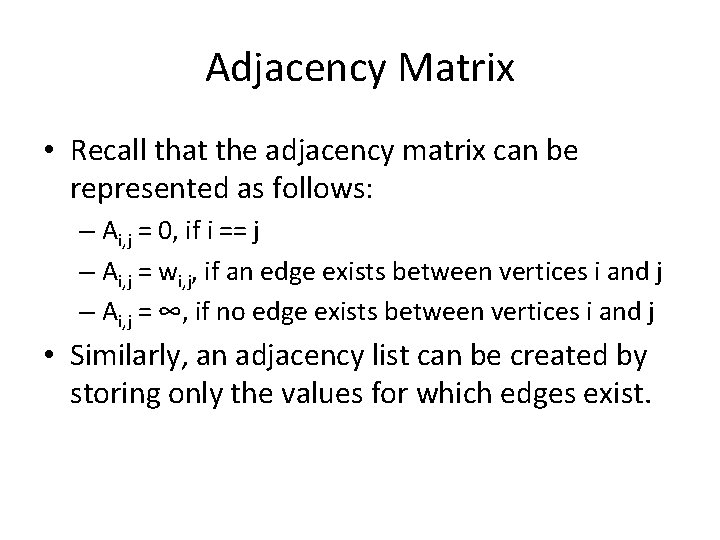
Adjacency Matrix • Recall that the adjacency matrix can be represented as follows: – Ai, j = 0, if i == j – Ai, j = wi, j, if an edge exists between vertices i and j – Ai, j = ∞, if no edge exists between vertices i and j • Similarly, an adjacency list can be created by storing only the values for which edges exist.
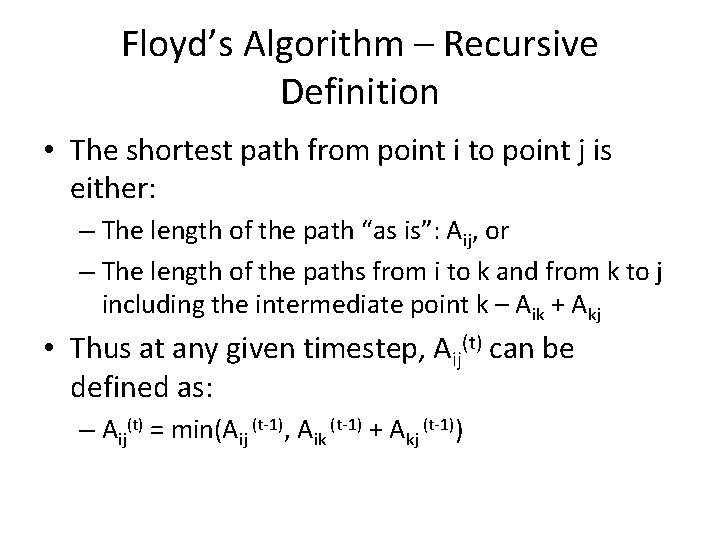
Floyd’s Algorithm – Recursive Definition • The shortest path from point i to point j is either: – The length of the path “as is”: Aij, or – The length of the paths from i to k and from k to j including the intermediate point k – Aik + Akj • Thus at any given timestep, Aij(t) can be defined as: – Aij(t) = min(Aij (t-1), Aik (t-1) + Akj (t-1))
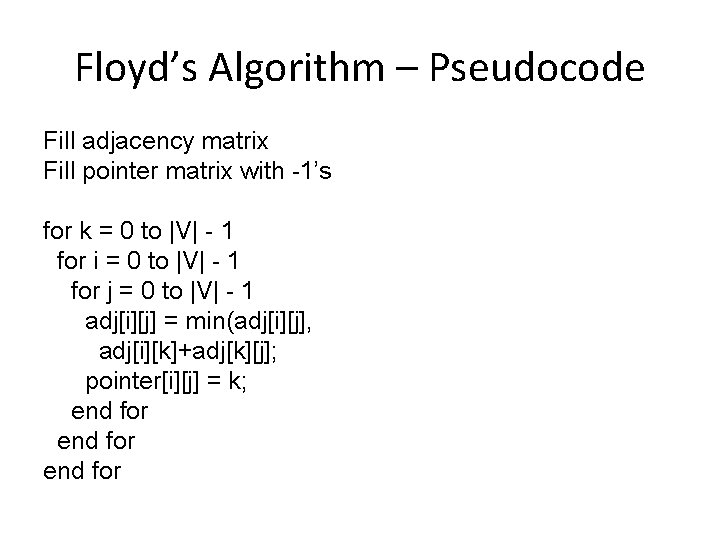
Floyd’s Algorithm – Pseudocode Fill adjacency matrix Fill pointer matrix with -1’s for k = 0 to |V| - 1 for i = 0 to |V| - 1 for j = 0 to |V| - 1 adj[i][j] = min(adj[i][j], adj[i][k]+adj[k][j]; pointer[i][j] = k; end for
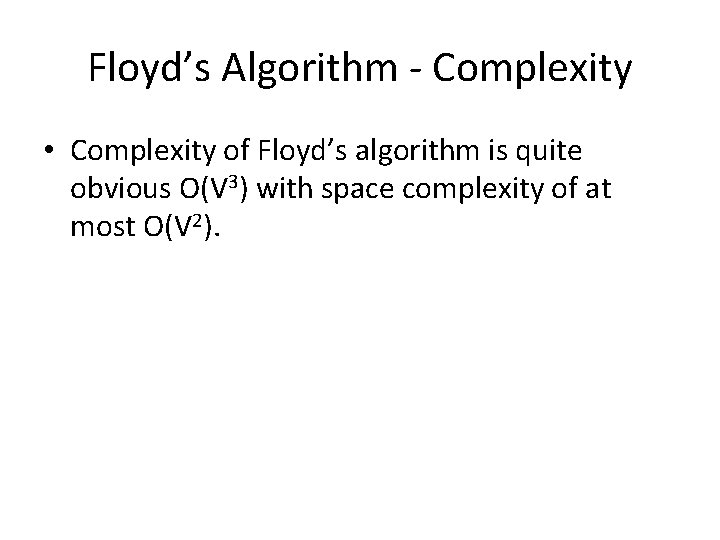
Floyd’s Algorithm - Complexity • Complexity of Floyd’s algorithm is quite obvious O(V 3) with space complexity of at most O(V 2).
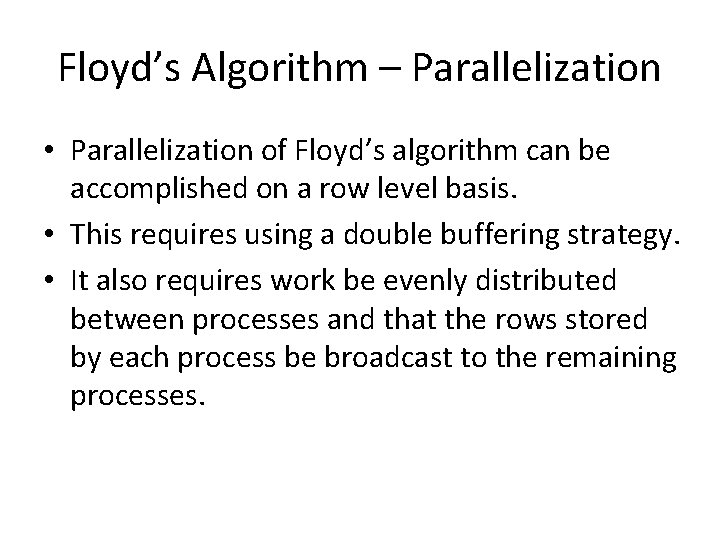
Floyd’s Algorithm – Parallelization • Parallelization of Floyd’s algorithm can be accomplished on a row level basis. • This requires using a double buffering strategy. • It also requires work be evenly distributed between processes and that the rows stored by each process be broadcast to the remaining processes.
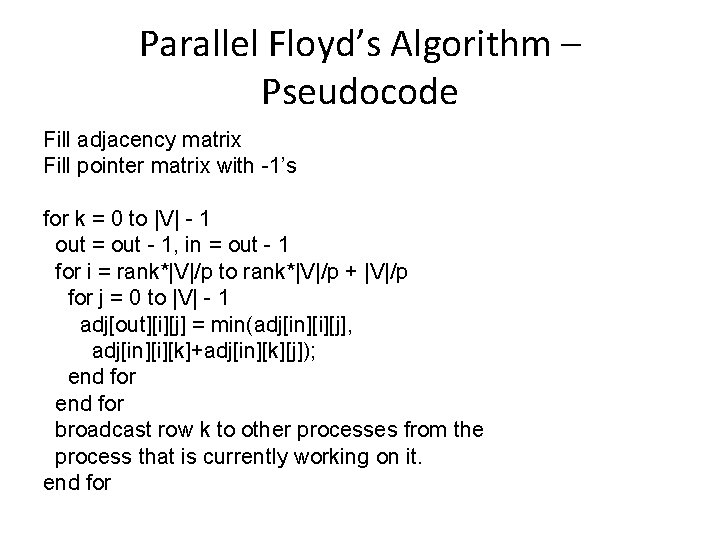
Parallel Floyd’s Algorithm – Pseudocode Fill adjacency matrix Fill pointer matrix with -1’s for k = 0 to |V| - 1 out = out - 1, in = out - 1 for i = rank*|V|/p to rank*|V|/p + |V|/p for j = 0 to |V| - 1 adj[out][i][j] = min(adj[in][i][j], adj[in][i][k]+adj[in][k][j]); end for broadcast row k to other processes from the process that is currently working on it. end for
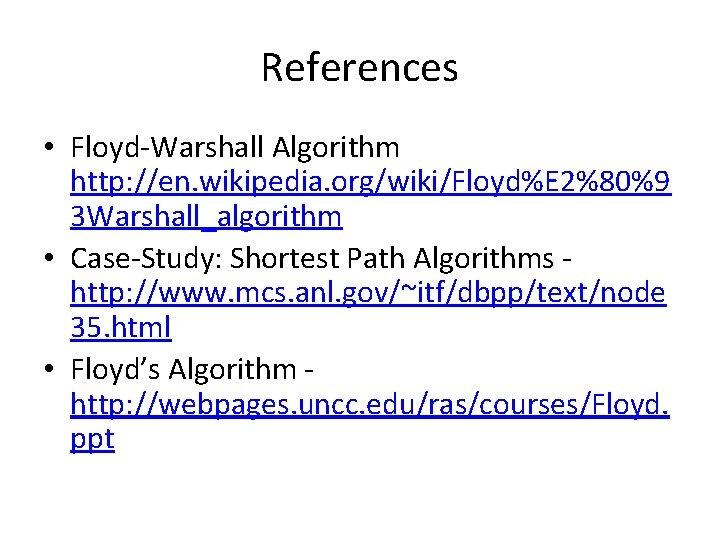
References • Floyd-Warshall Algorithm http: //en. wikipedia. org/wiki/Floyd%E 2%80%9 3 Warshall_algorithm • Case-Study: Shortest Path Algorithms http: //www. mcs. anl. gov/~itf/dbpp/text/node 35. html • Floyd’s Algorithm http: //webpages. uncc. edu/ras/courses/Floyd. ppt