Dijkstras Algorithm Shortest Path Single Source Comparison to
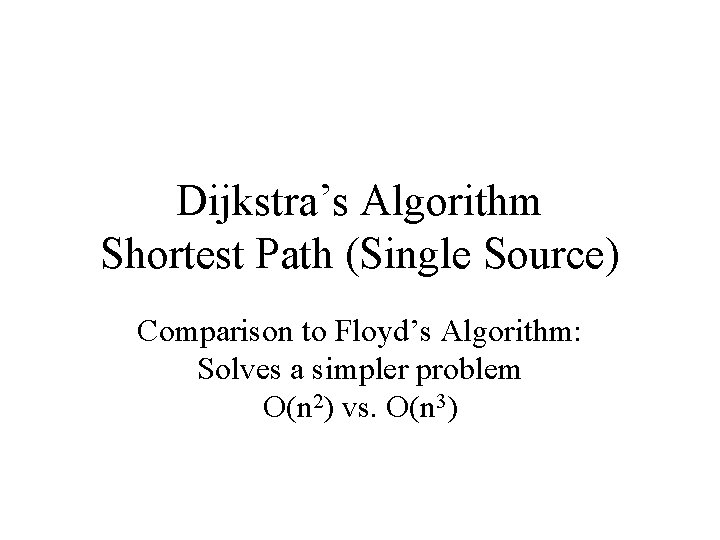
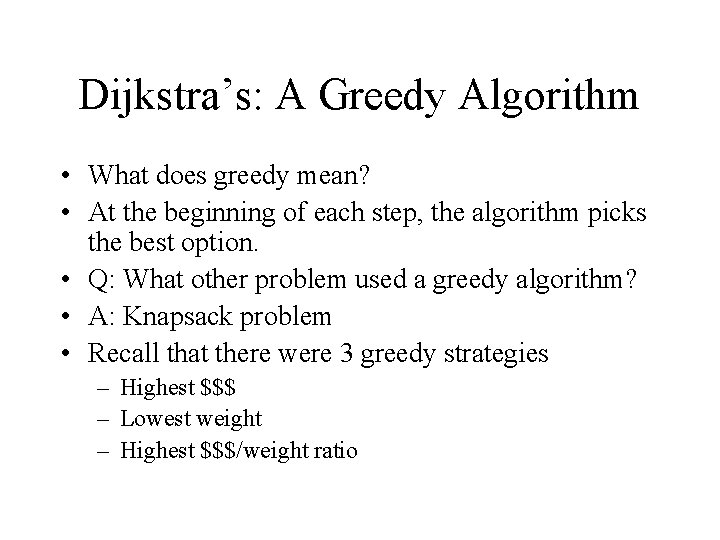
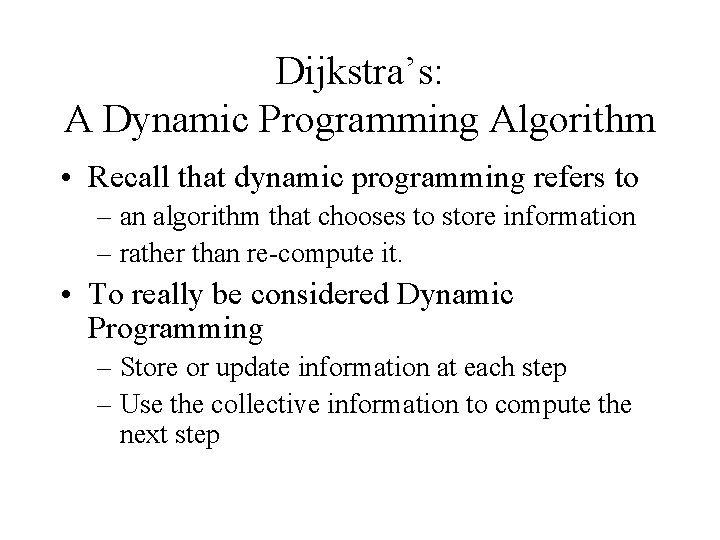
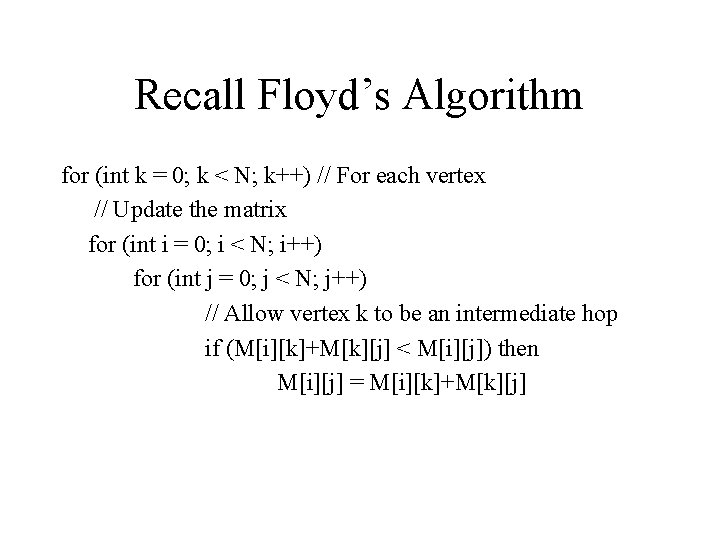
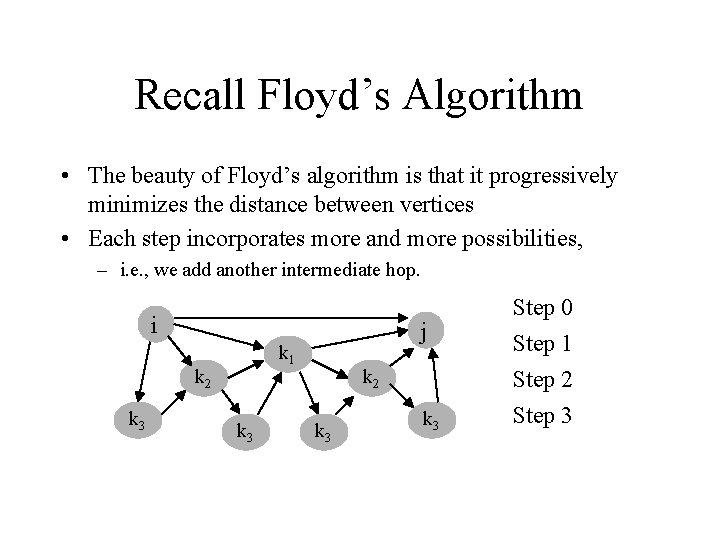
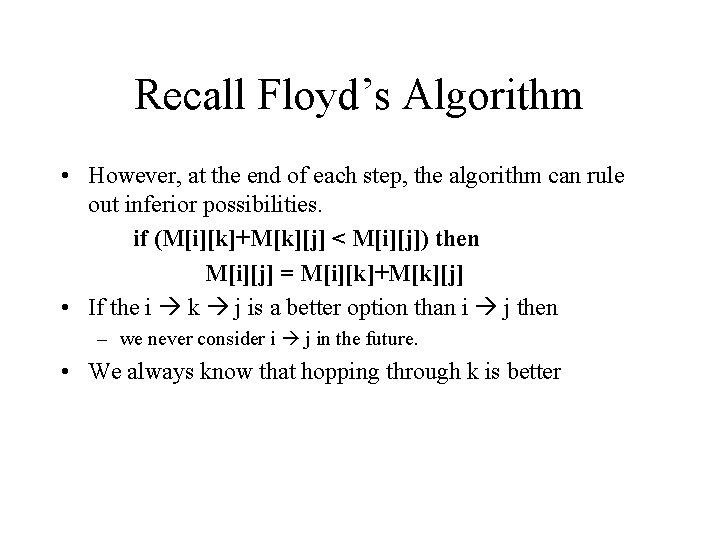
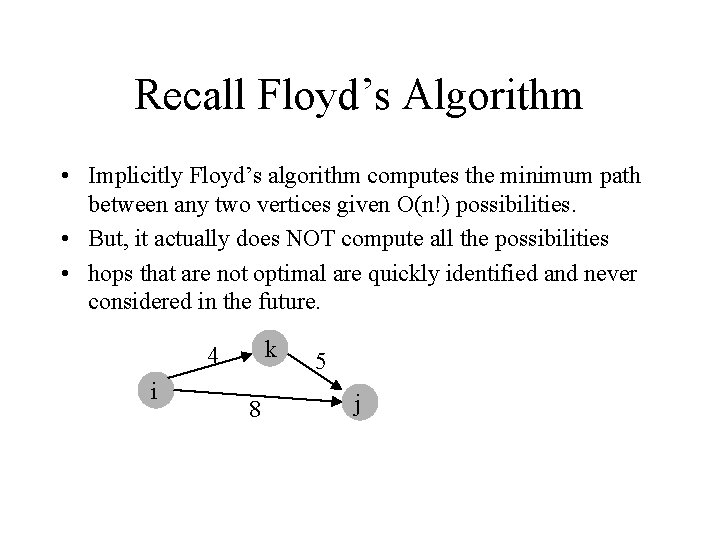
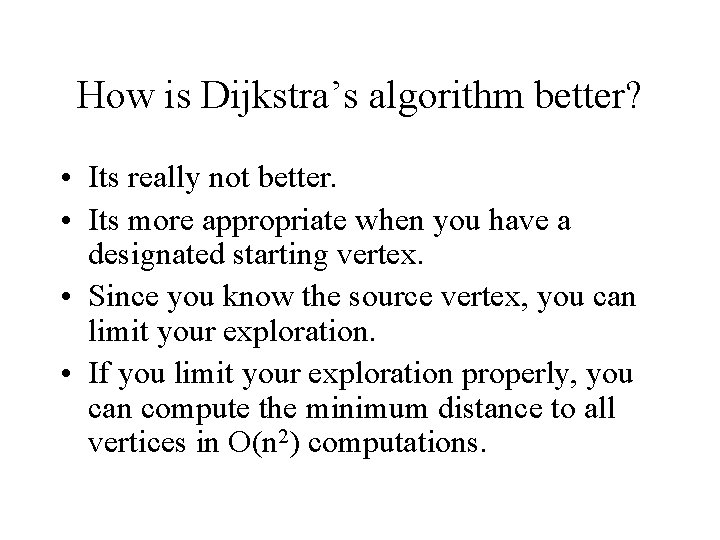
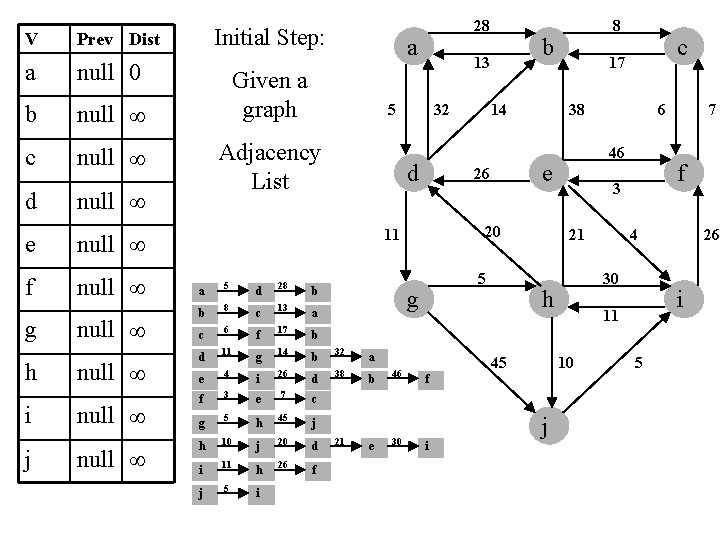
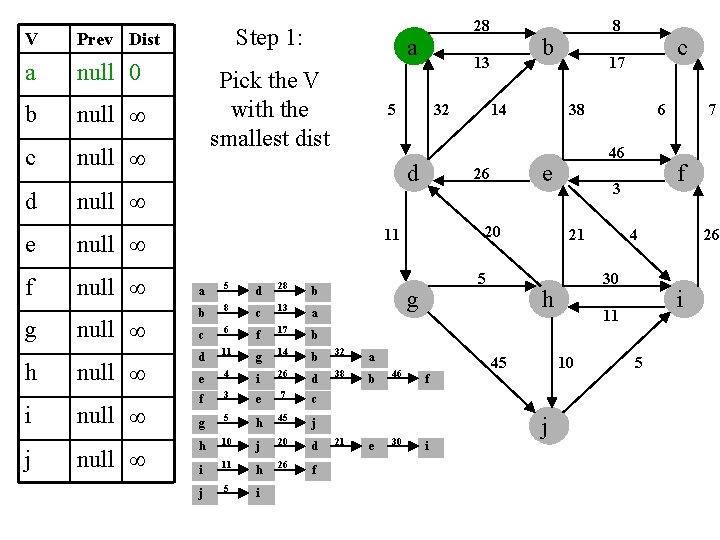
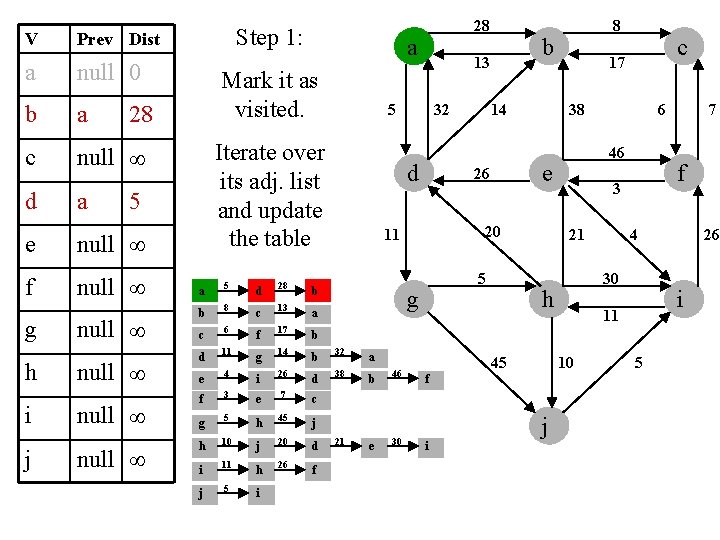
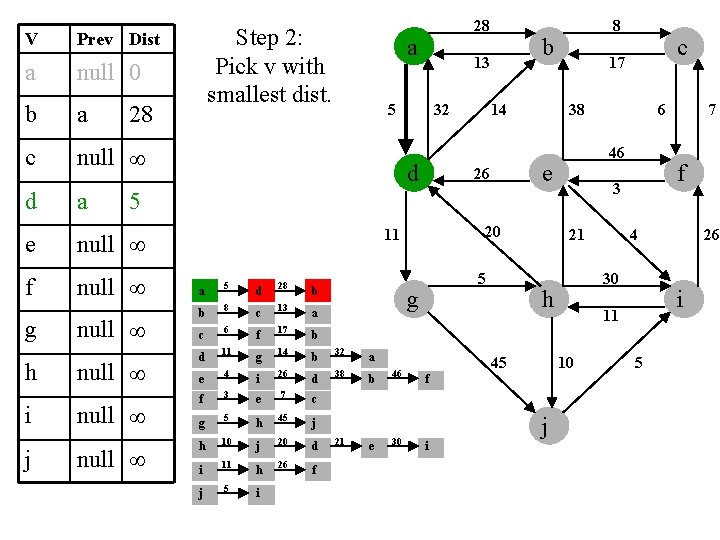
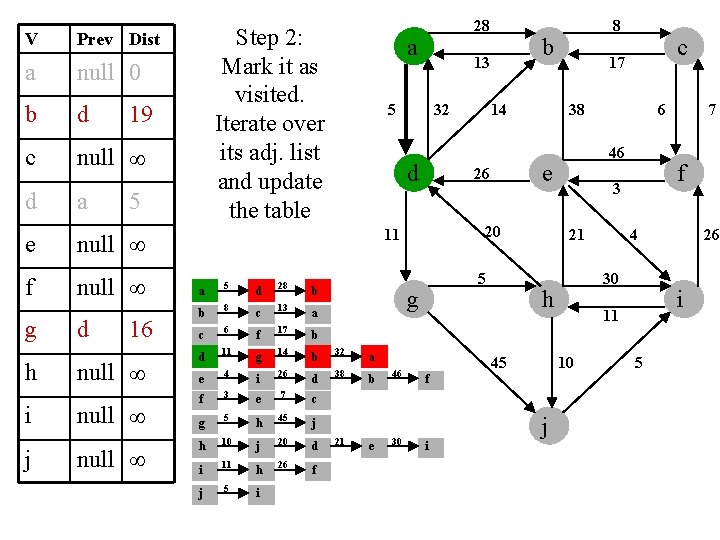
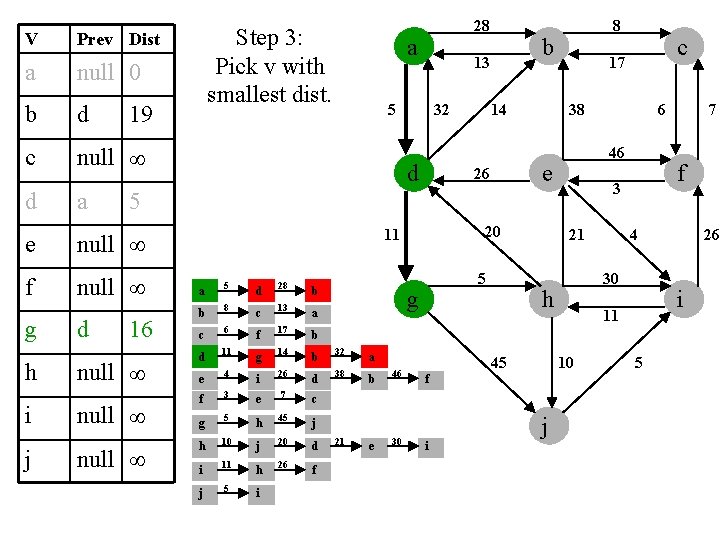
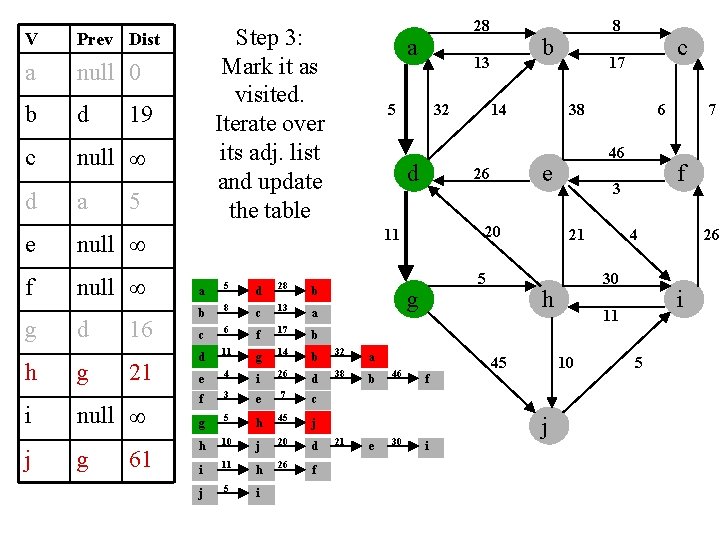
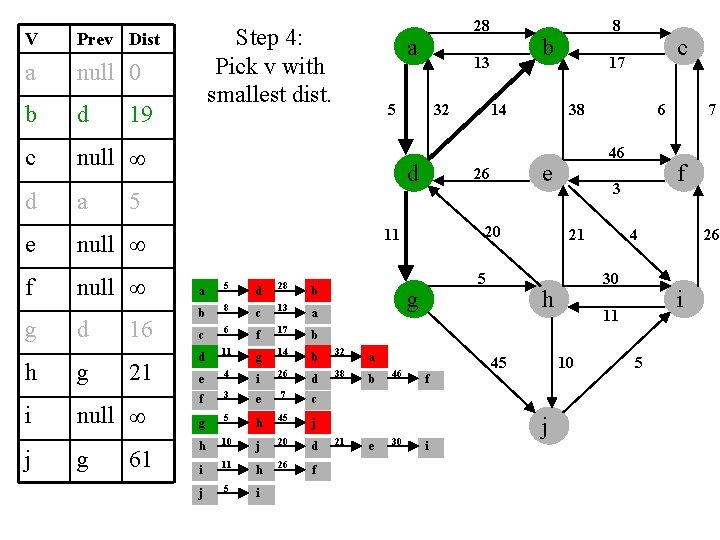
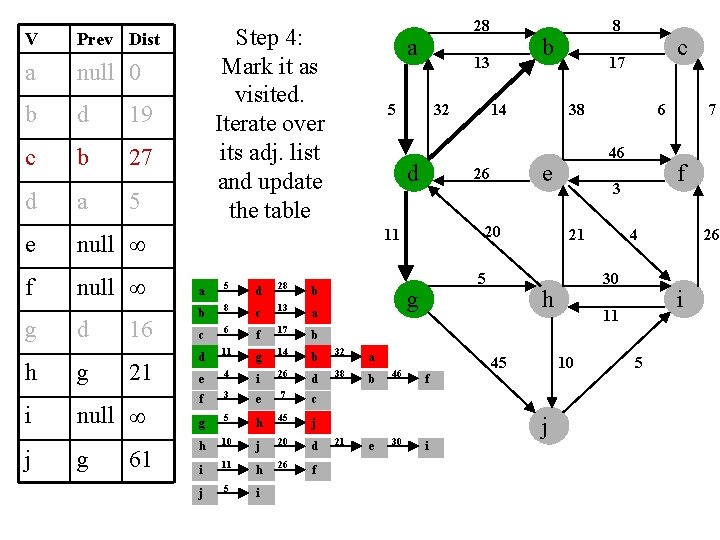
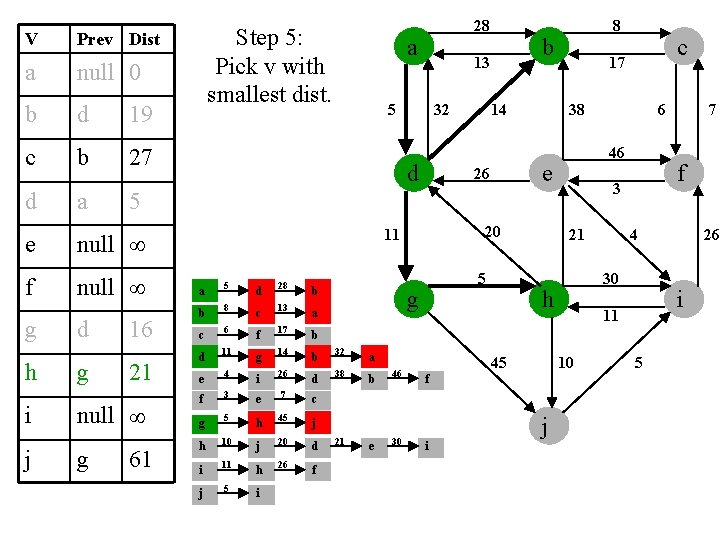
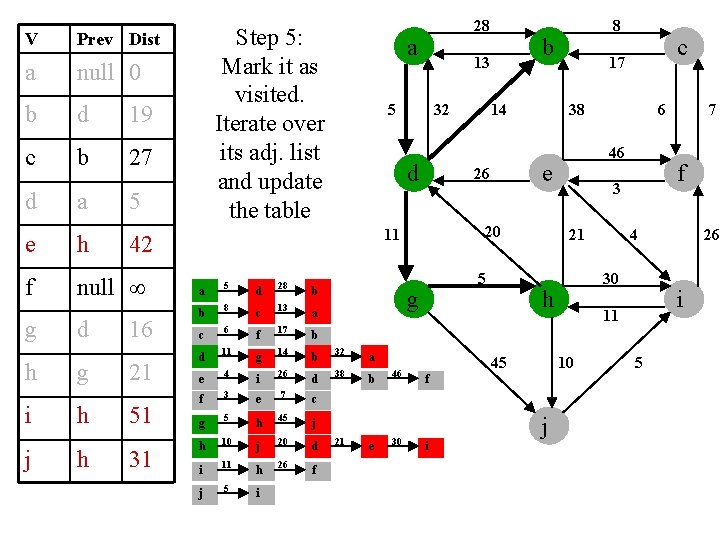
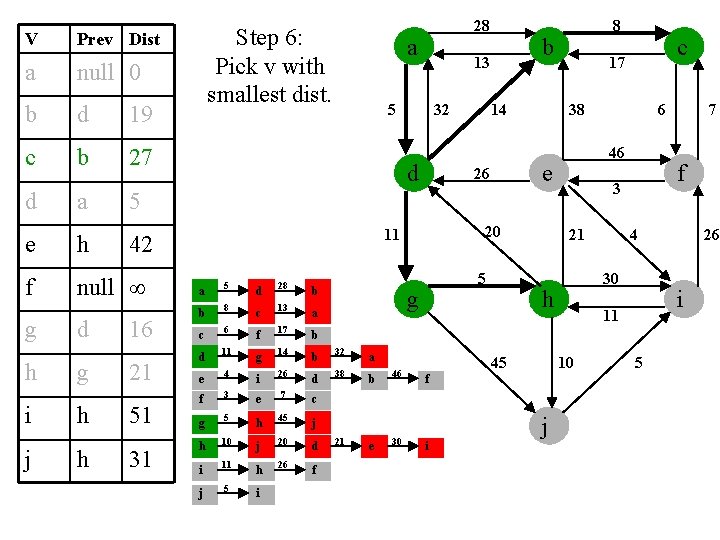
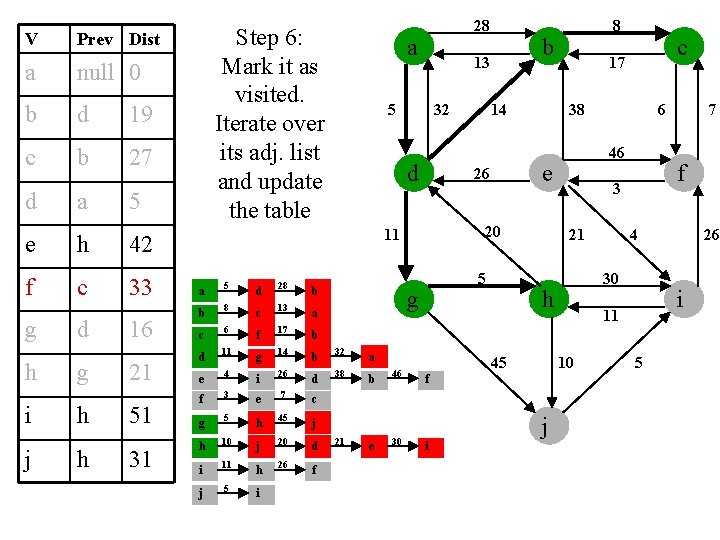
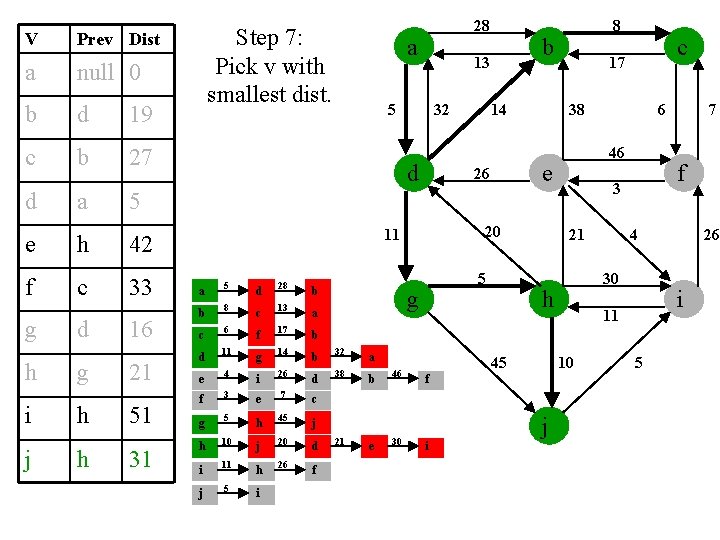
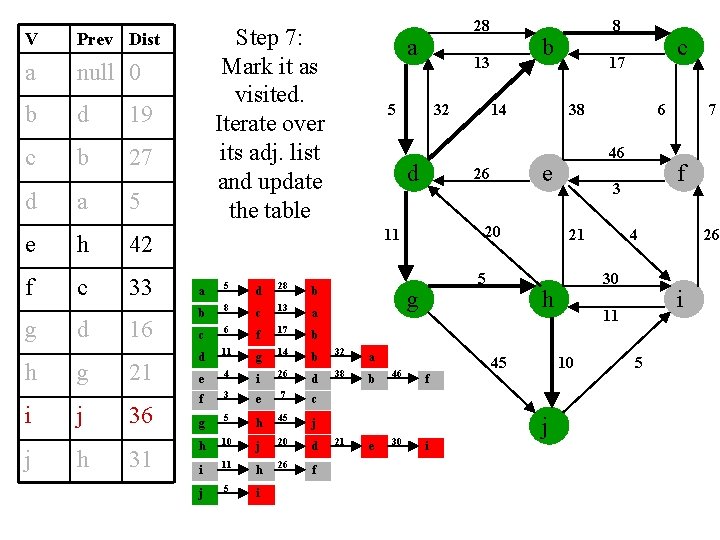
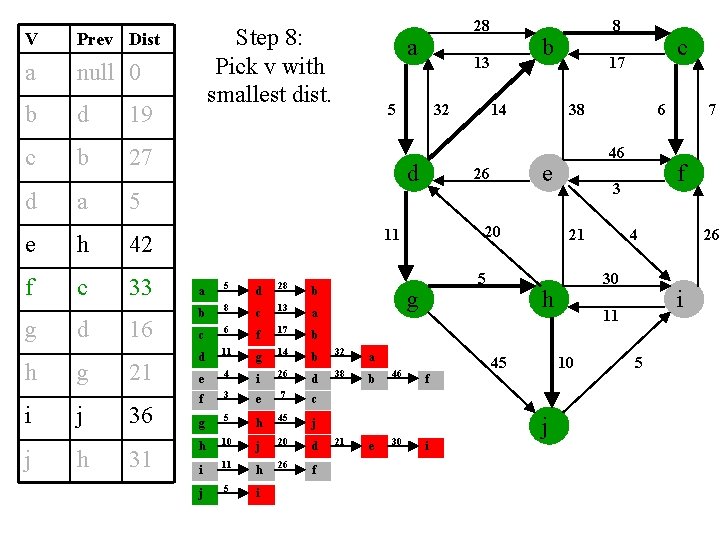
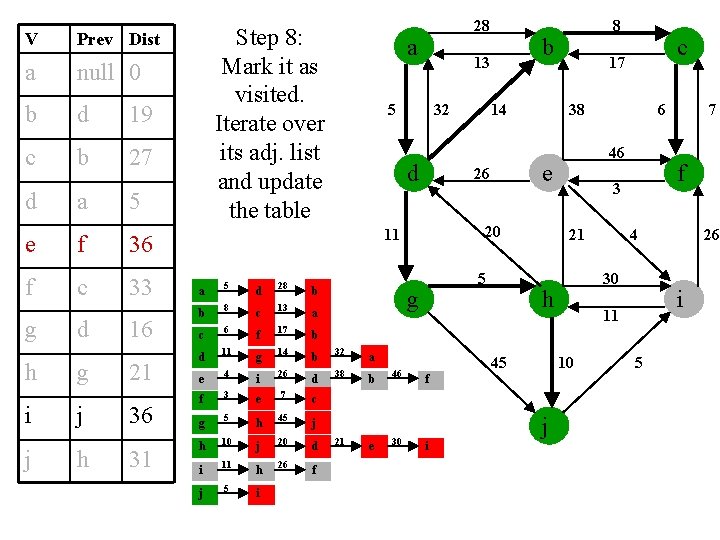
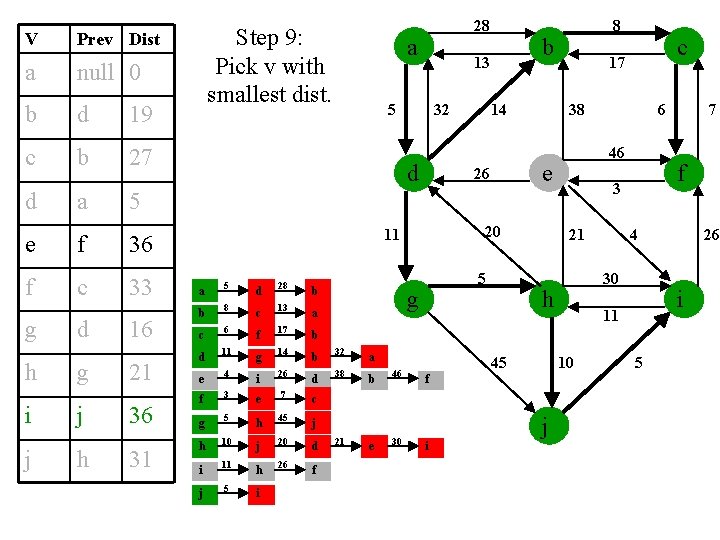
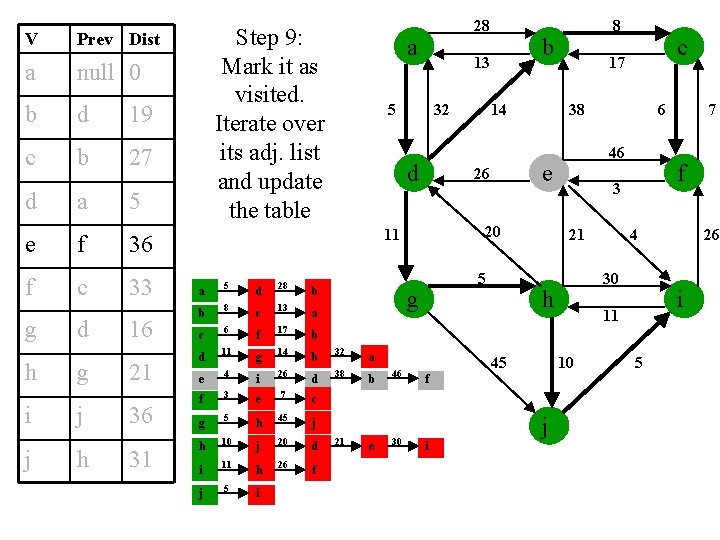
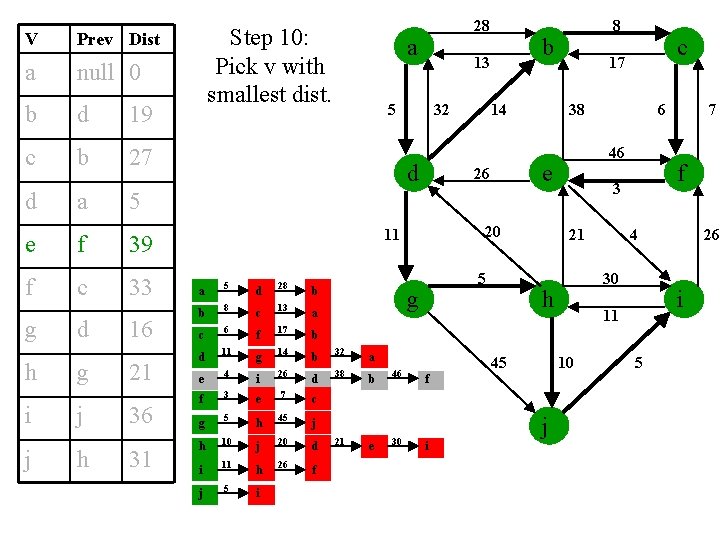
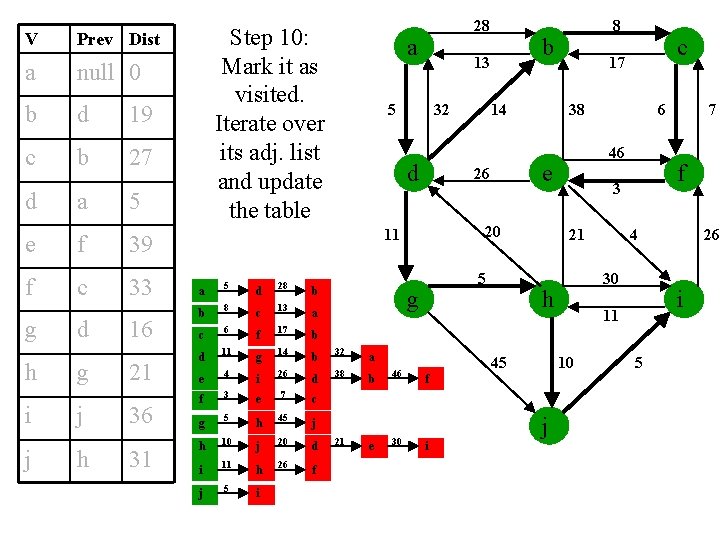
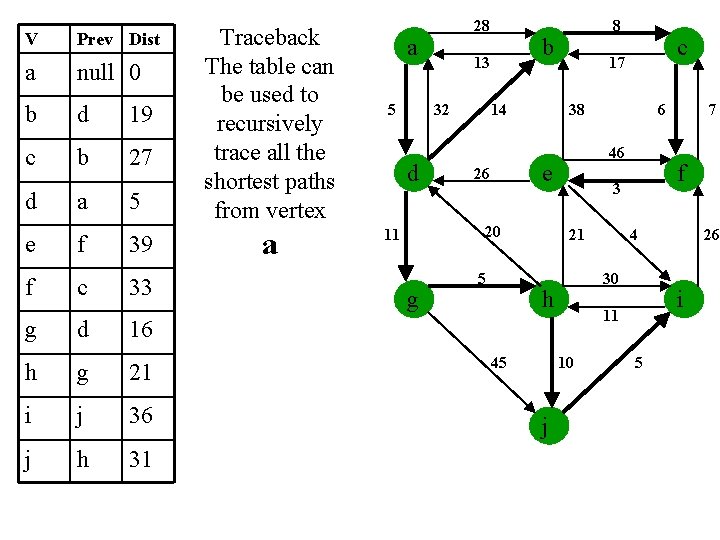
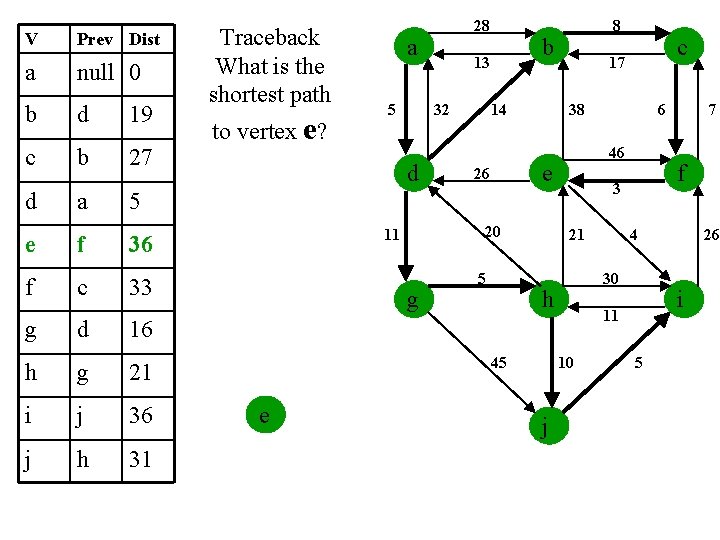
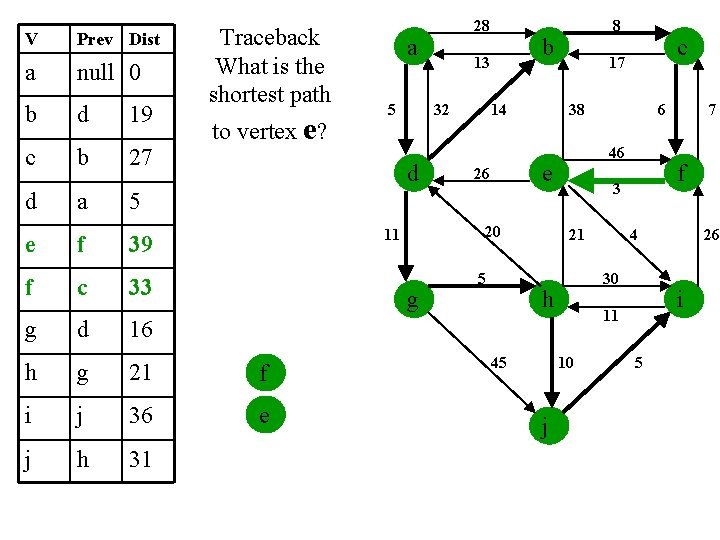
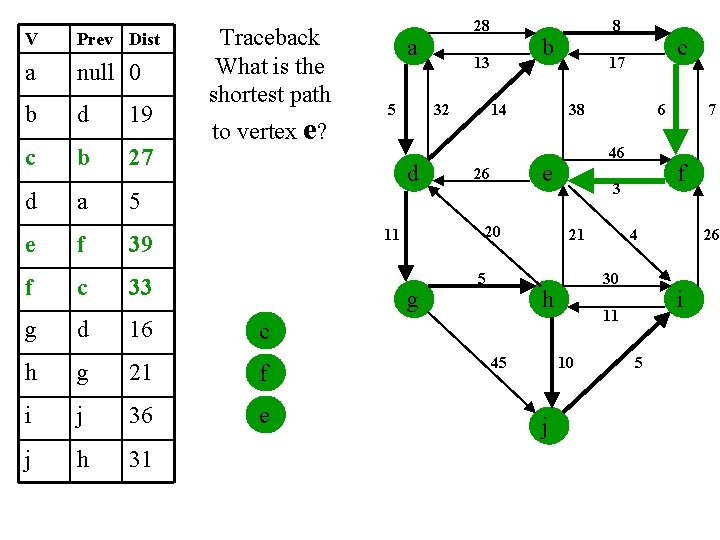
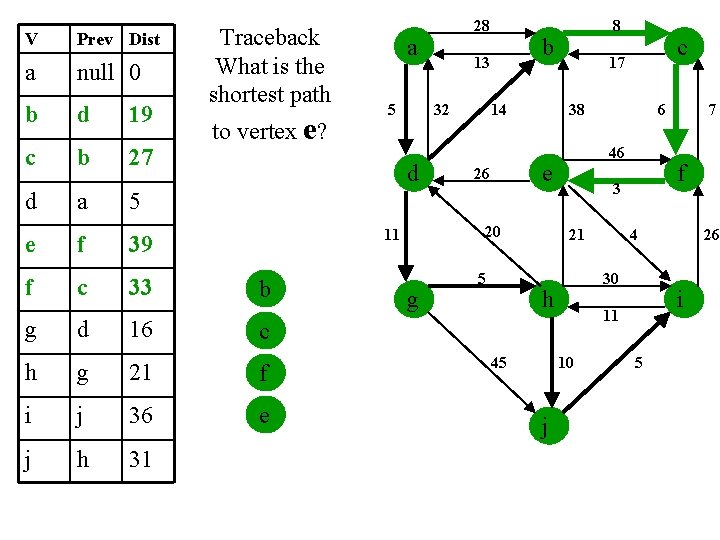
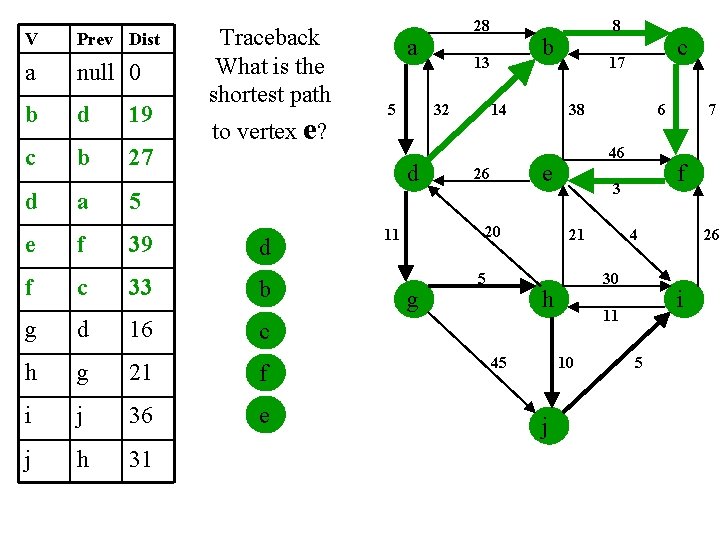
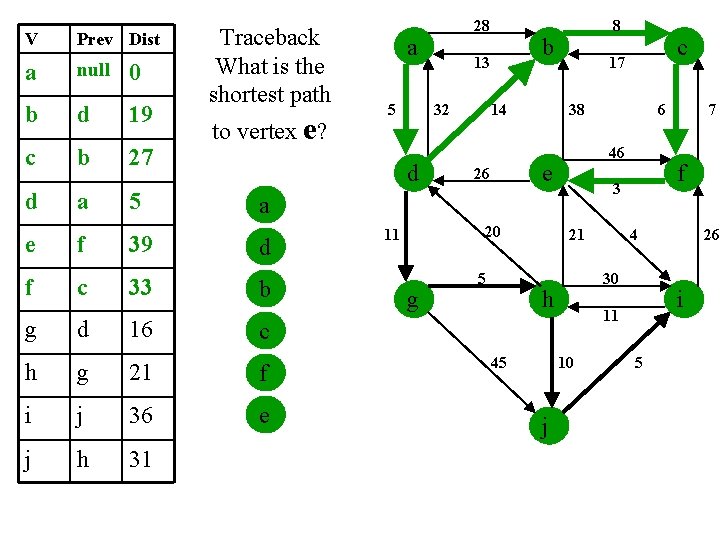
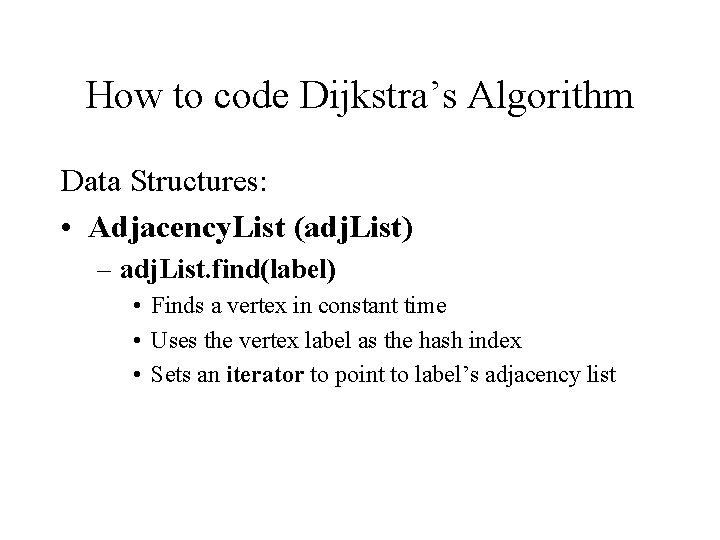
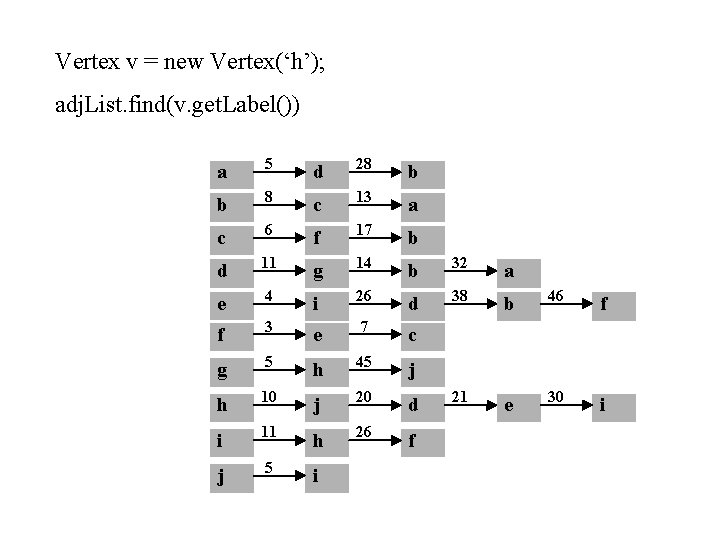
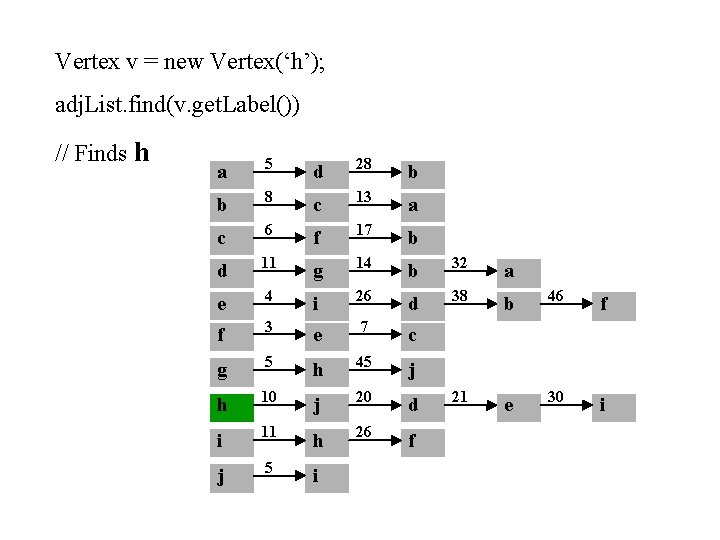
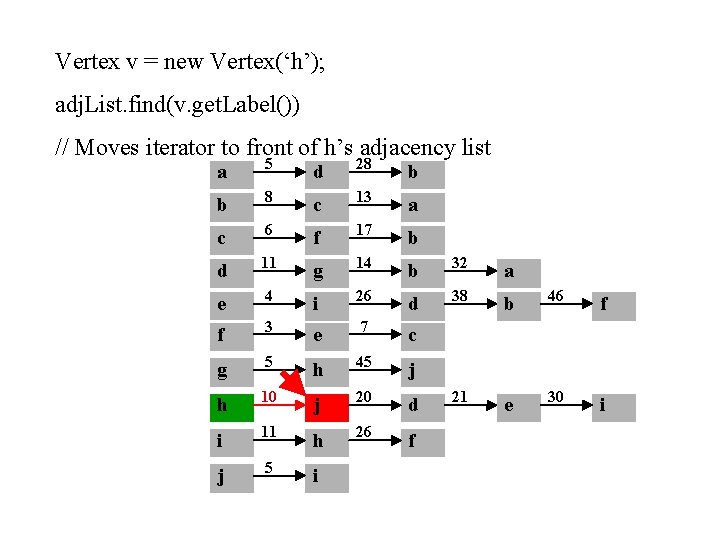
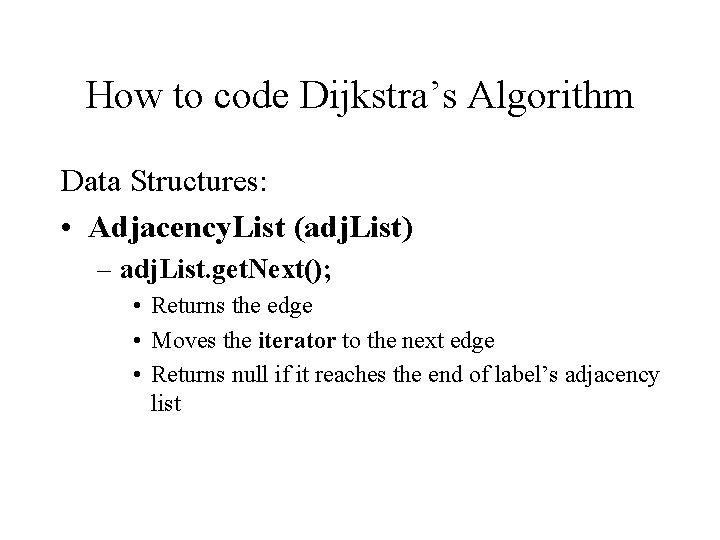
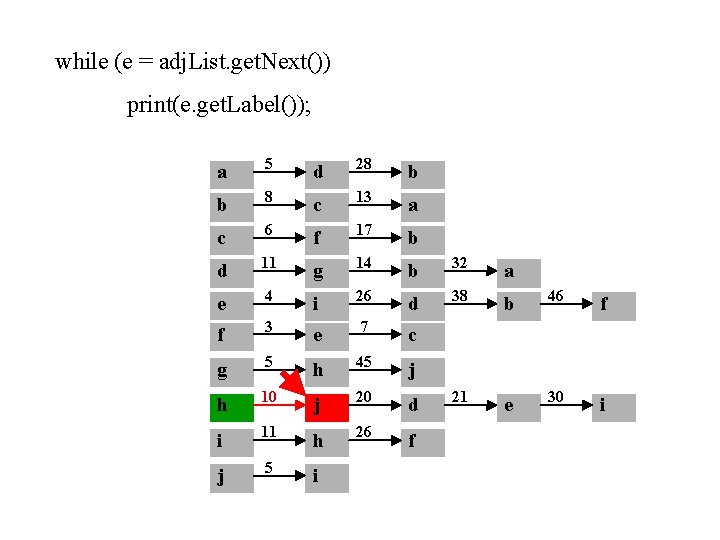
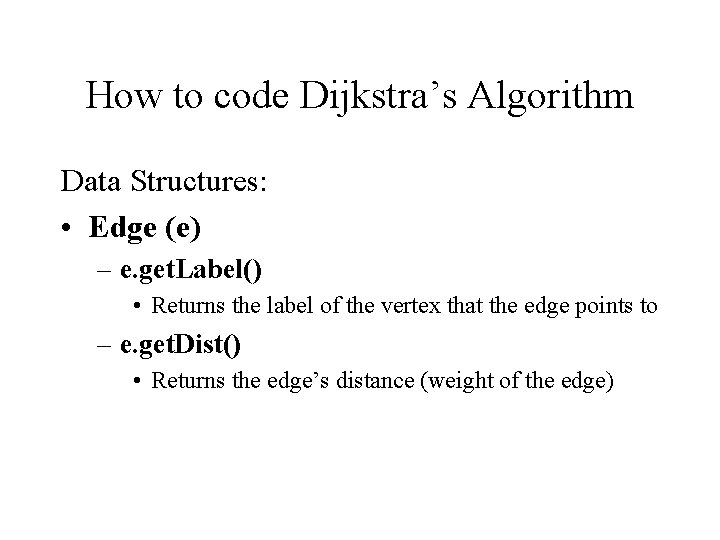
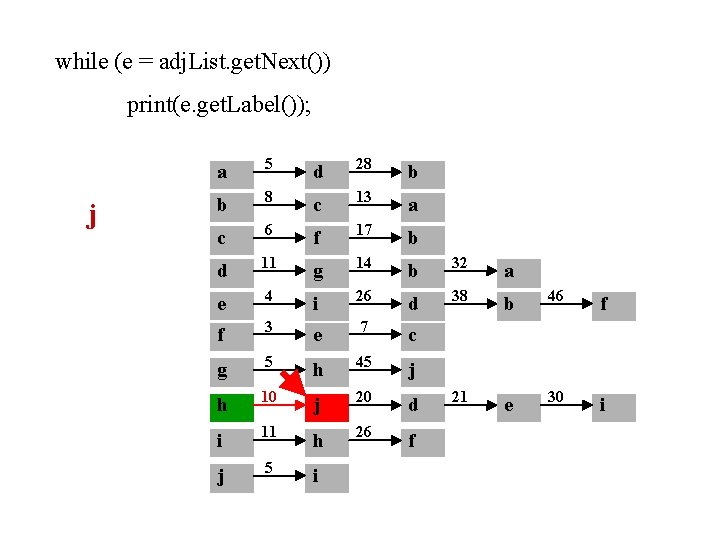
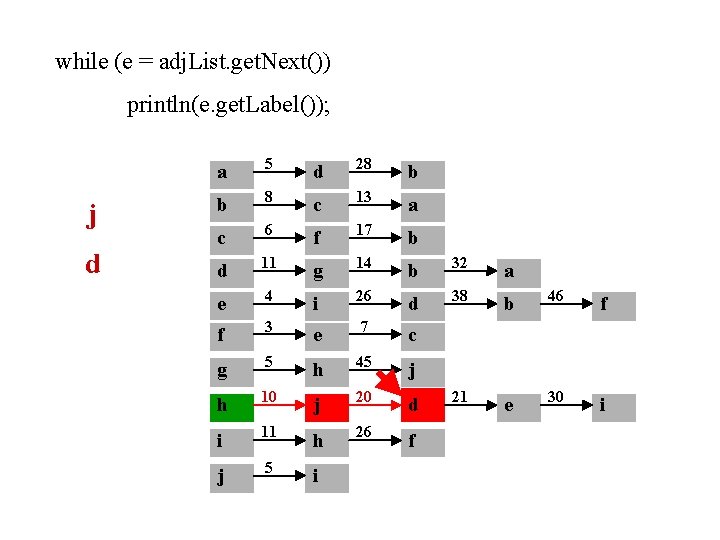
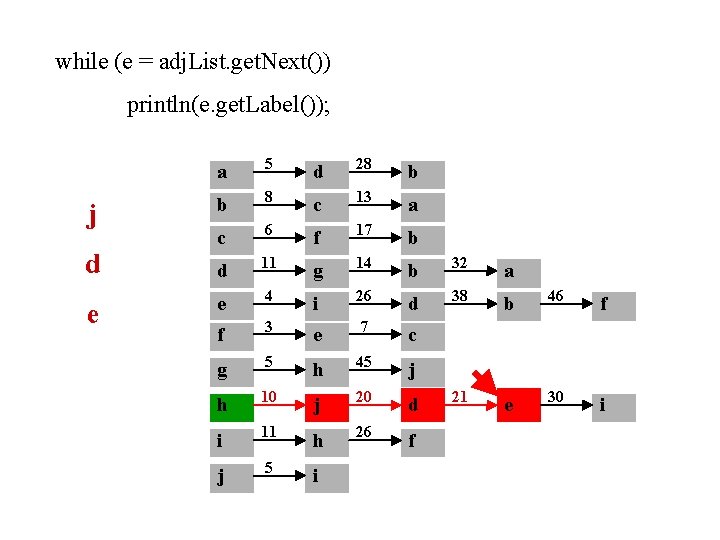
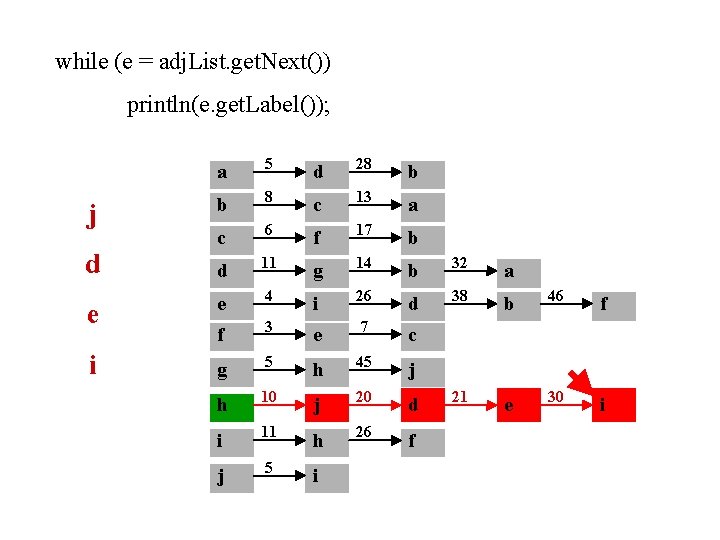
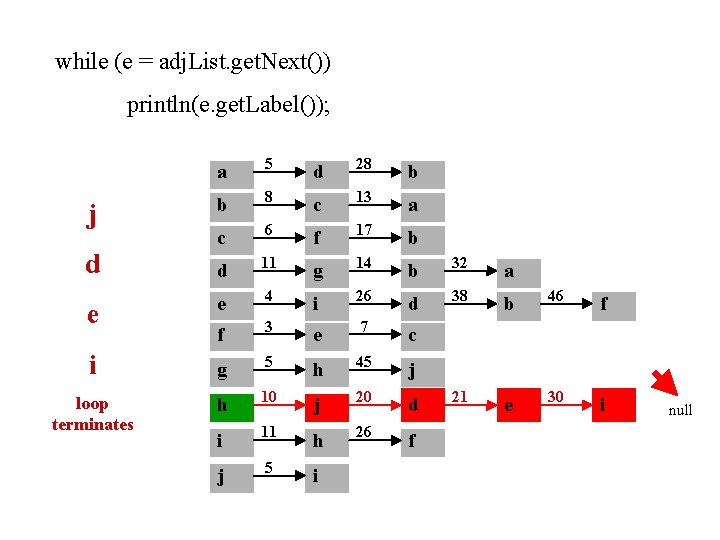
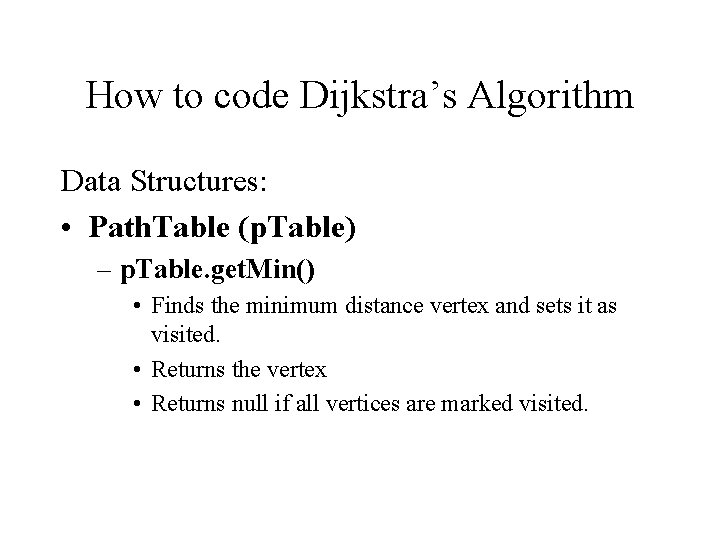
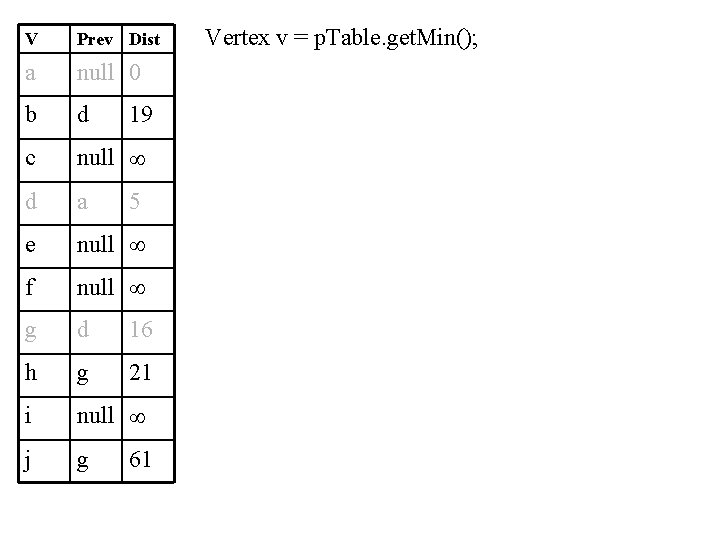
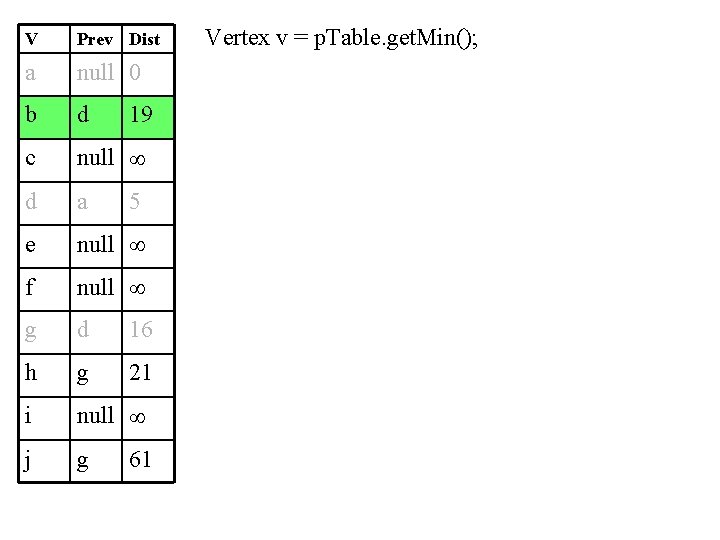
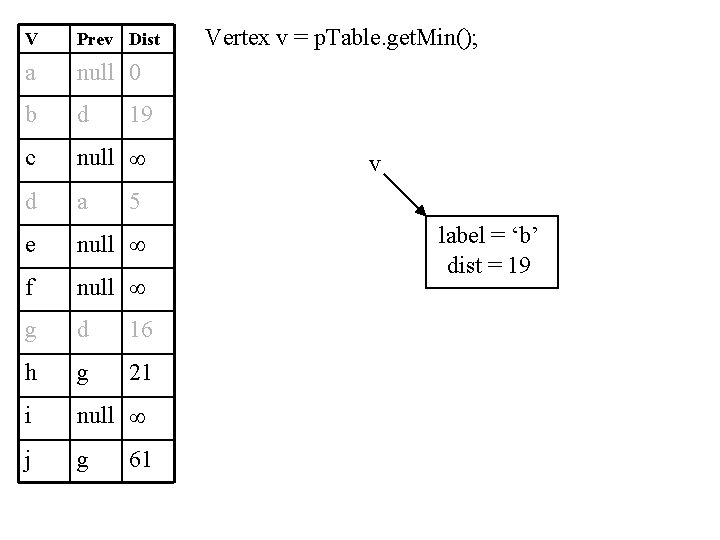
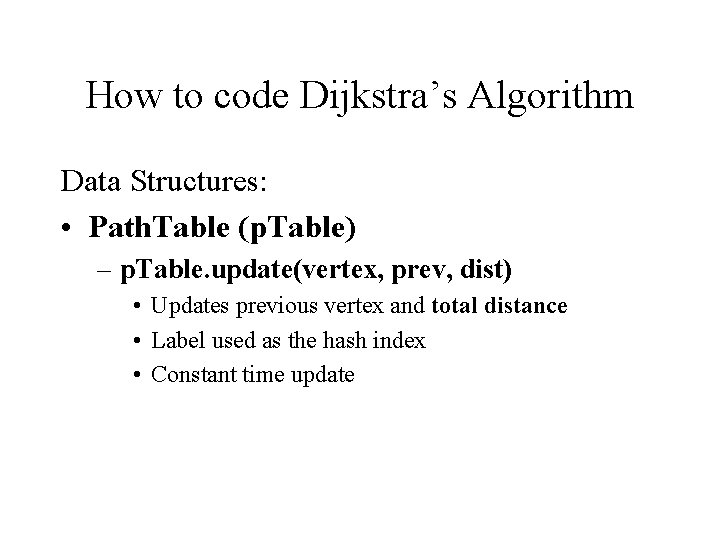
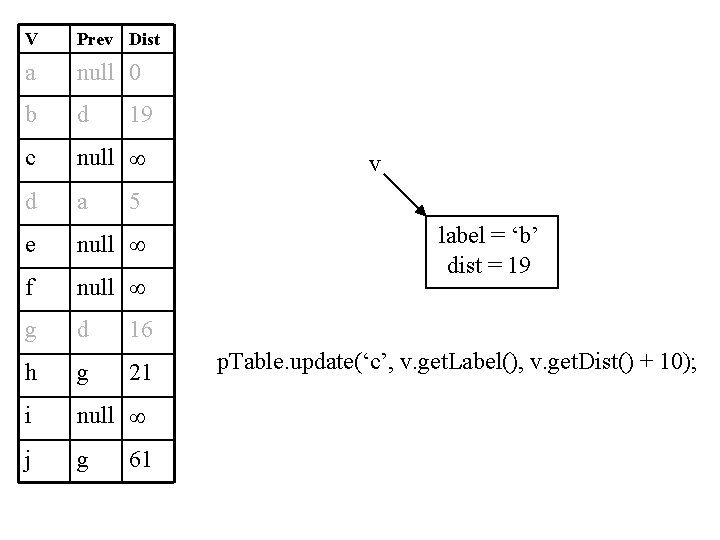
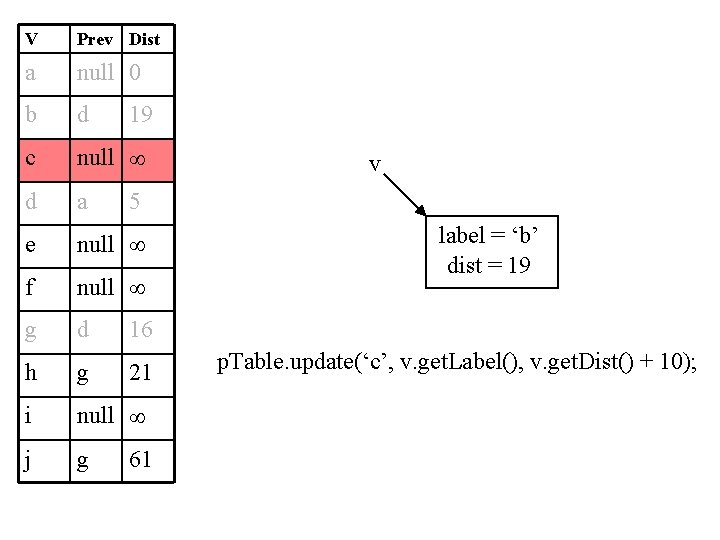
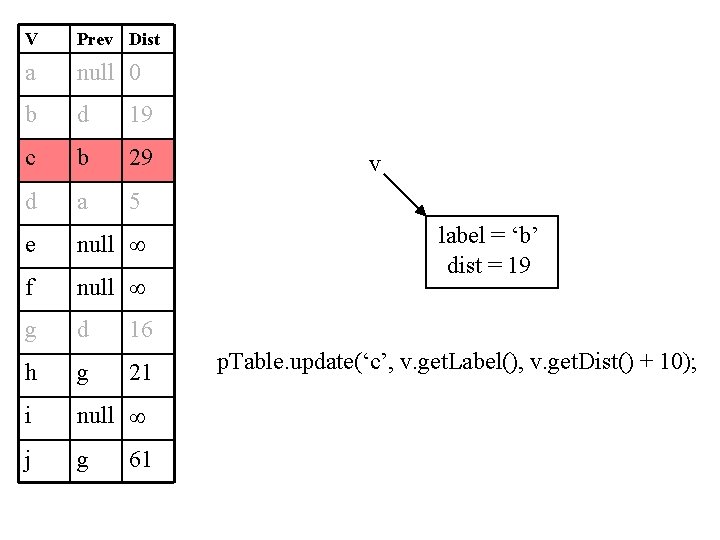
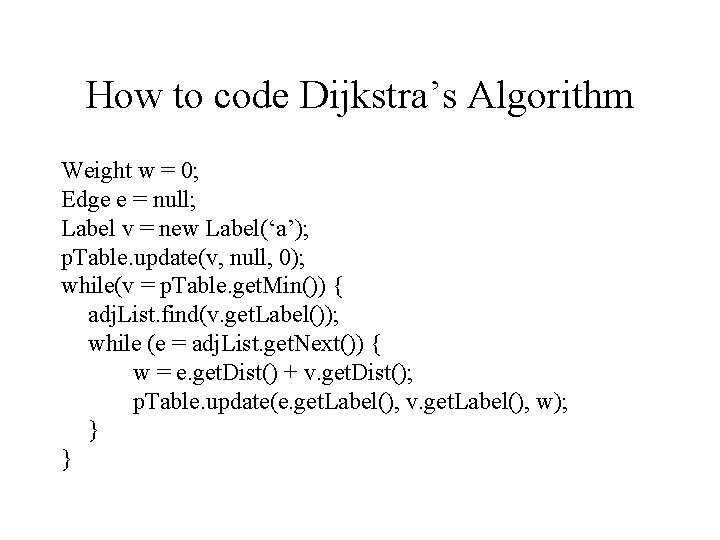
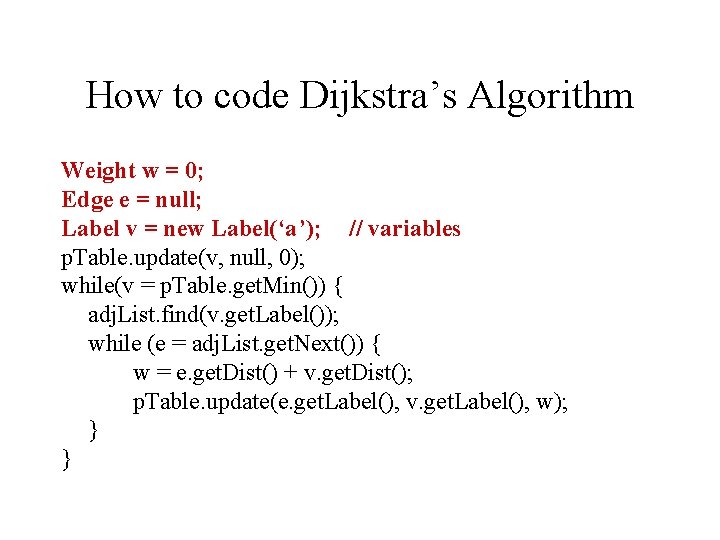
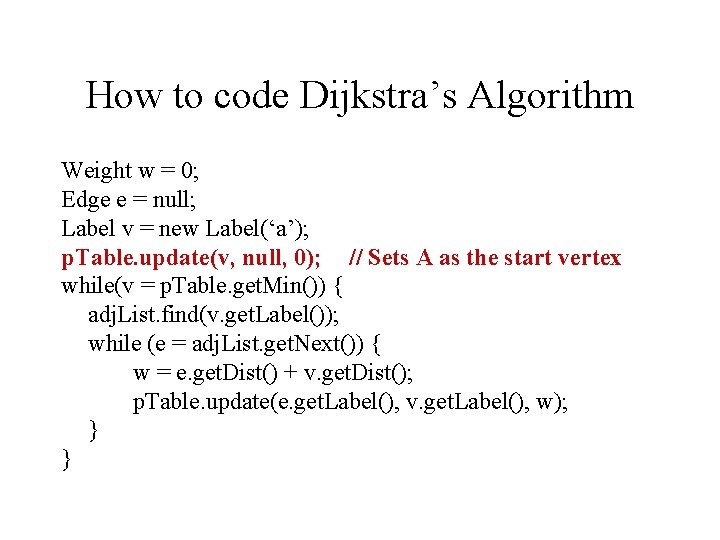
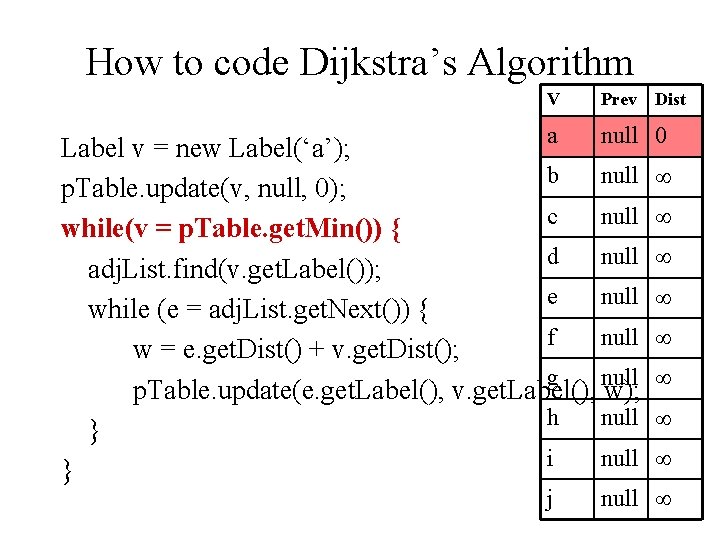
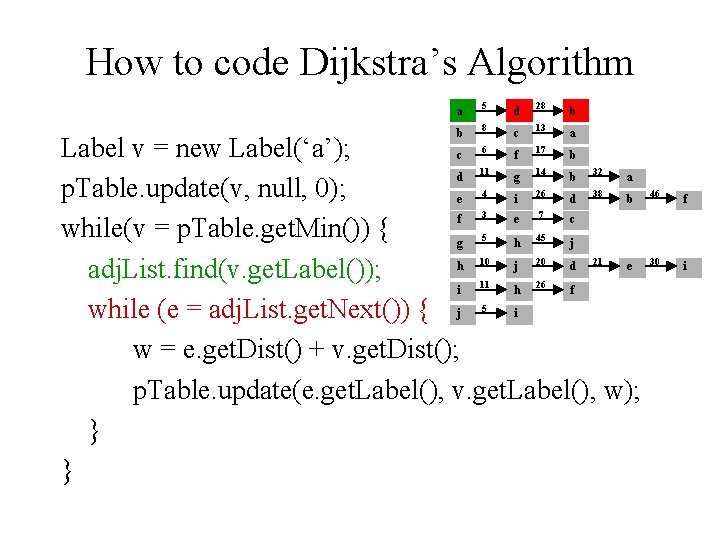
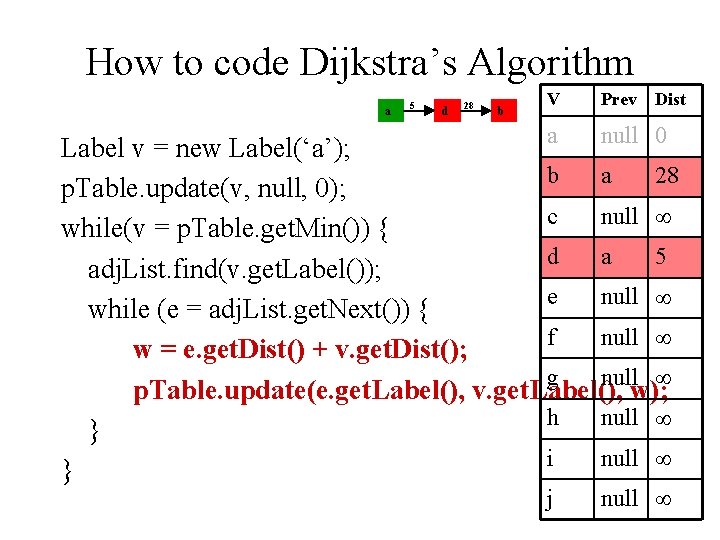
- Slides: 62
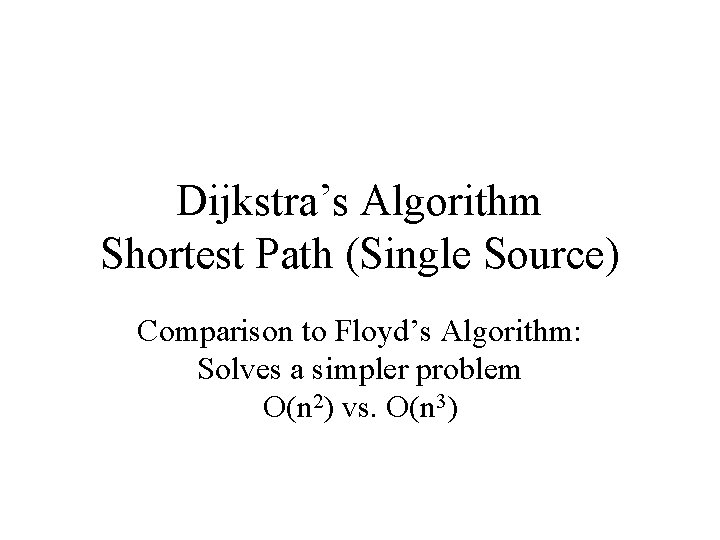
Dijkstra’s Algorithm Shortest Path (Single Source) Comparison to Floyd’s Algorithm: Solves a simpler problem O(n 2) vs. O(n 3)
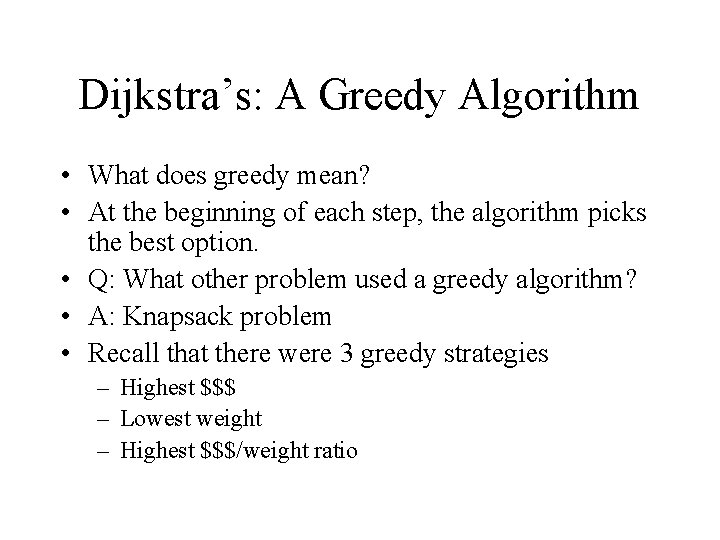
Dijkstra’s: A Greedy Algorithm • What does greedy mean? • At the beginning of each step, the algorithm picks the best option. • Q: What other problem used a greedy algorithm? • A: Knapsack problem • Recall that there were 3 greedy strategies – Highest $$$ – Lowest weight – Highest $$$/weight ratio
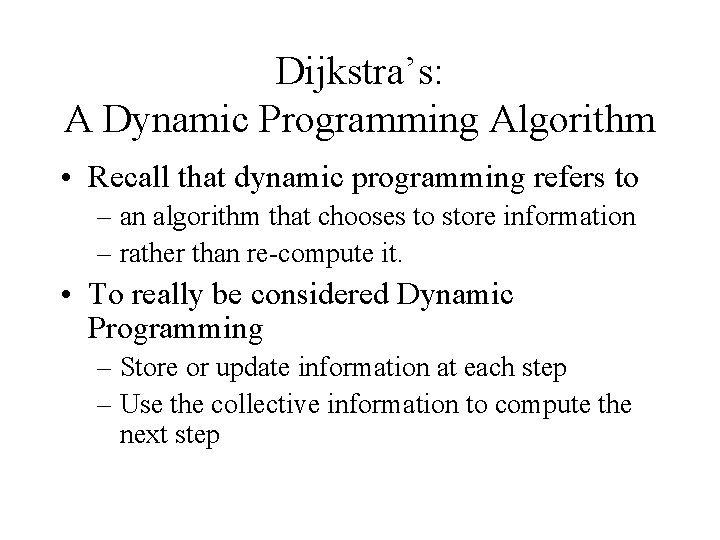
Dijkstra’s: A Dynamic Programming Algorithm • Recall that dynamic programming refers to – an algorithm that chooses to store information – rather than re-compute it. • To really be considered Dynamic Programming – Store or update information at each step – Use the collective information to compute the next step
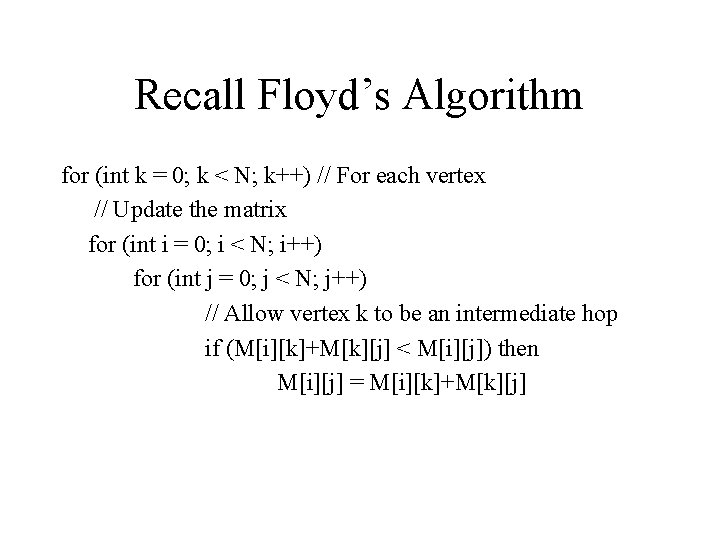
Recall Floyd’s Algorithm for (int k = 0; k < N; k++) // For each vertex // Update the matrix for (int i = 0; i < N; i++) for (int j = 0; j < N; j++) // Allow vertex k to be an intermediate hop if (M[i][k]+M[k][j] < M[i][j]) then M[i][j] = M[i][k]+M[k][j]
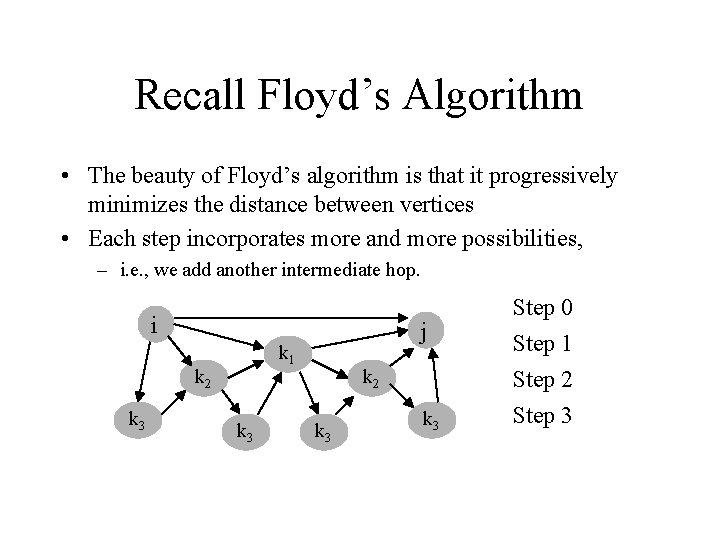
Recall Floyd’s Algorithm • The beauty of Floyd’s algorithm is that it progressively minimizes the distance between vertices • Each step incorporates more and more possibilities, – i. e. , we add another intermediate hop. i k 1 k 2 k 3 j k 3 k 2 k 3 Step 0 Step 1 Step 2 Step 3
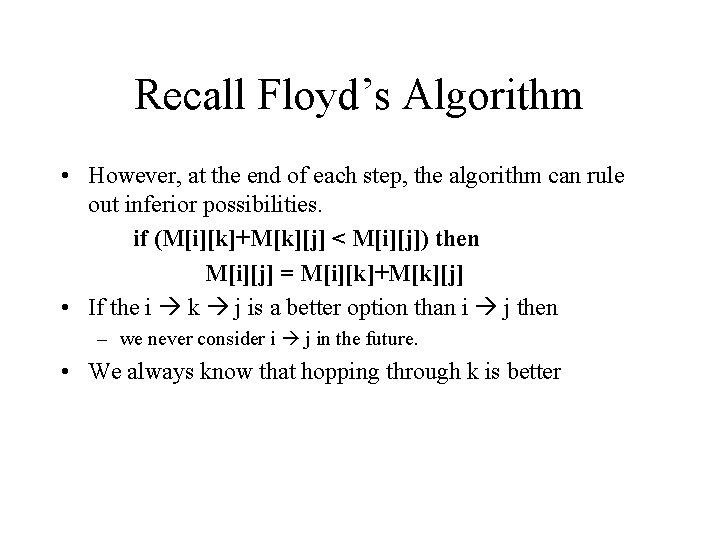
Recall Floyd’s Algorithm • However, at the end of each step, the algorithm can rule out inferior possibilities. if (M[i][k]+M[k][j] < M[i][j]) then M[i][j] = M[i][k]+M[k][j] • If the i k j is a better option than i j then – we never consider i j in the future. • We always know that hopping through k is better
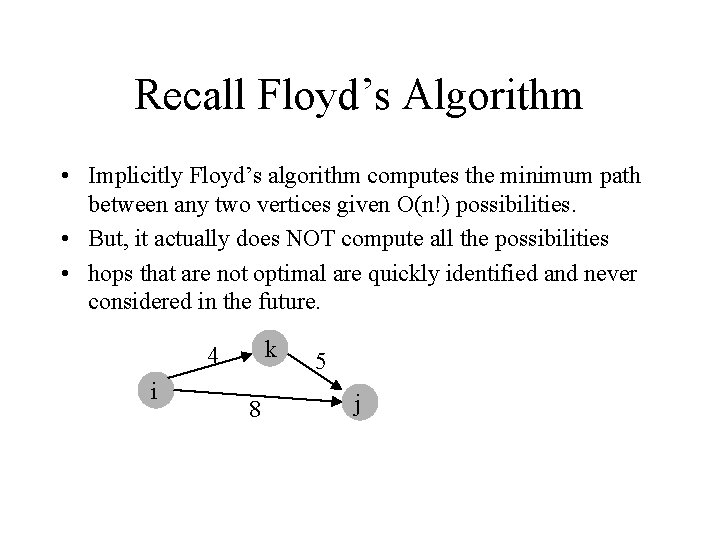
Recall Floyd’s Algorithm • Implicitly Floyd’s algorithm computes the minimum path between any two vertices given O(n!) possibilities. • But, it actually does NOT compute all the possibilities • hops that are not optimal are quickly identified and never considered in the future. k 4 i 8 5 j
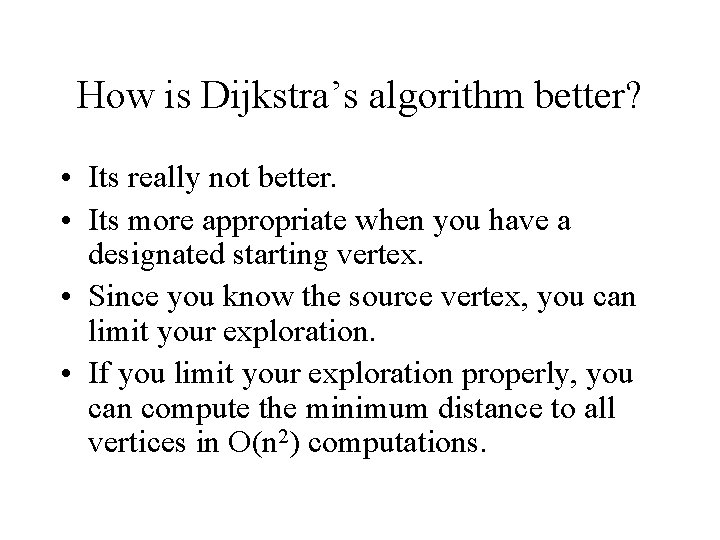
How is Dijkstra’s algorithm better? • Its really not better. • Its more appropriate when you have a designated starting vertex. • Since you know the source vertex, you can limit your exploration. • If you limit your exploration properly, you can compute the minimum distance to all vertices in O(n 2) computations.
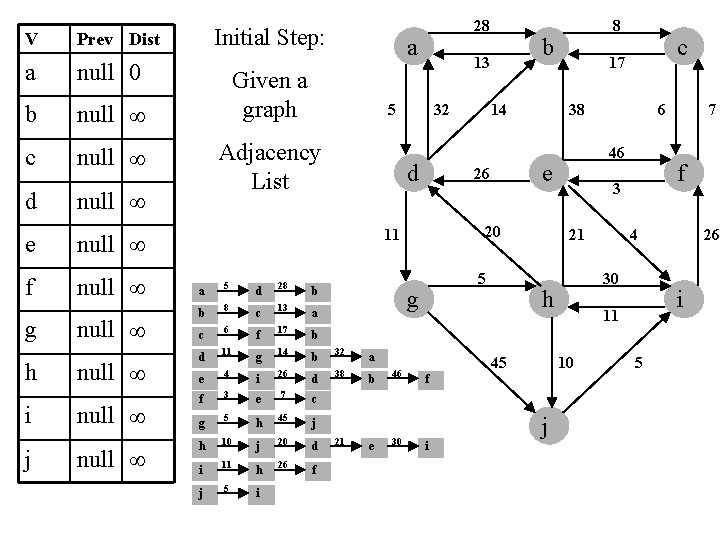
V a null 0 b null c null d null e null f null g h i j Initial Step: Prev Dist null 28 a Given a graph Adjacency List 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
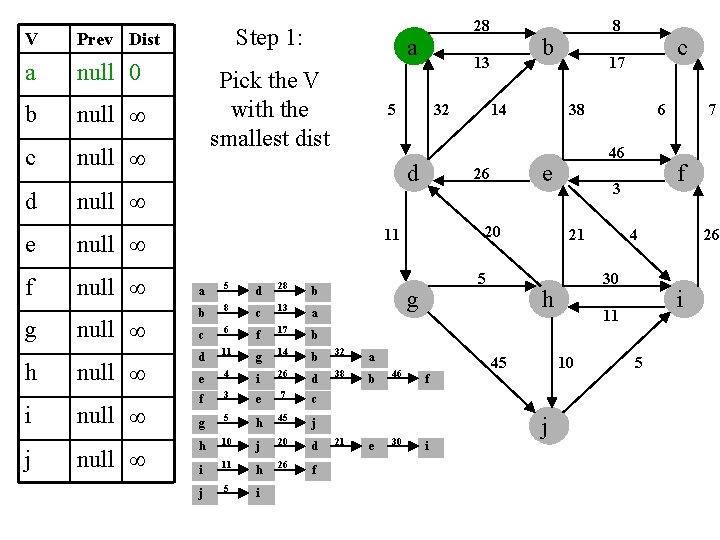
V a null 0 b null c null d null e null f null g h i j Step 1: Prev Dist null 28 a Pick the V with the smallest dist 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
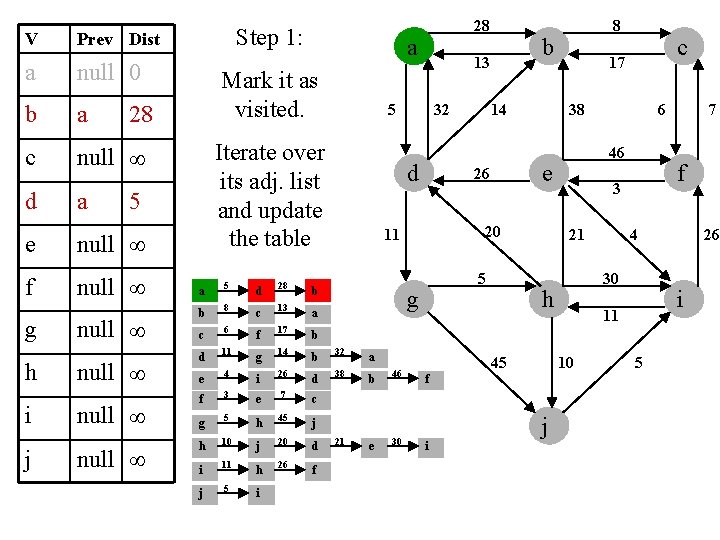
V a null 0 b a c null a e null f null h i j null 32 d a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 8 b 13 5 Iterate over its adj. list and update the table 5 null a Mark it as visited. 28 d g Step 1: Prev Dist 28 5
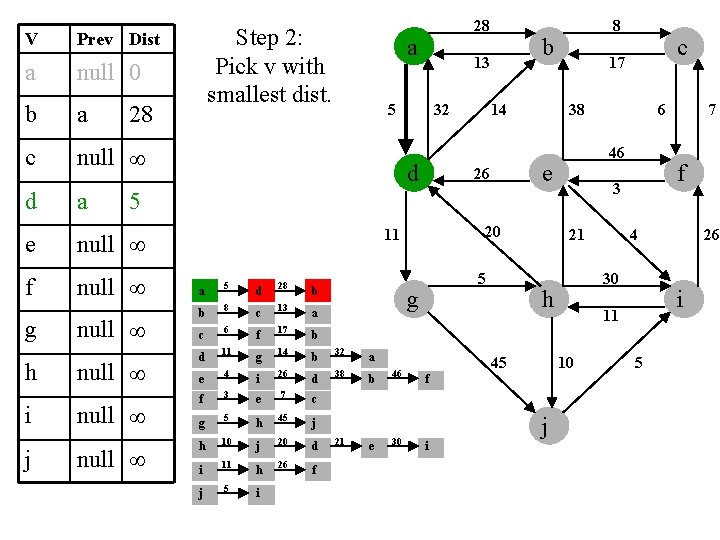
V a null 0 b a c null 28 d a e null f null g h i j Step 2: Pick v with smallest dist. Prev Dist 28 a b 13 5 32 d 8 14 38 null 20 a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 5 g 21 21 45 j i 26 i 11 10 f 4 30 h 7 f 3 5 11 6 46 e 26 c 17 5
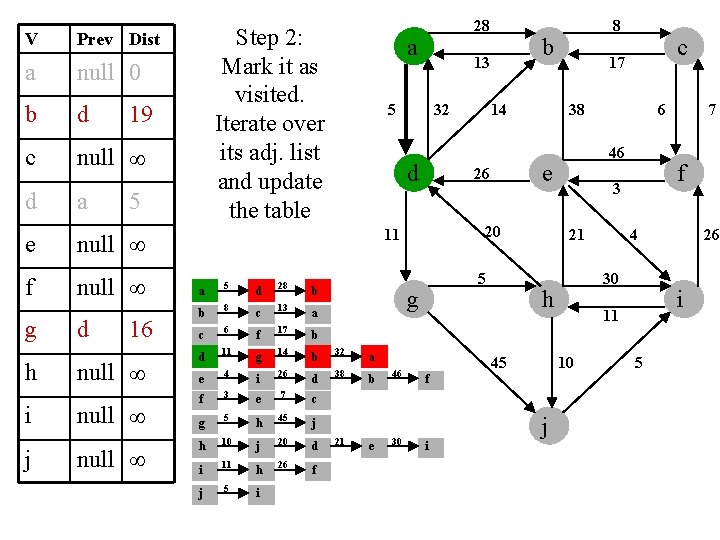
V a null 0 b d c null 19 d a e null f null g h i j Step 2: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d 5 16 null 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
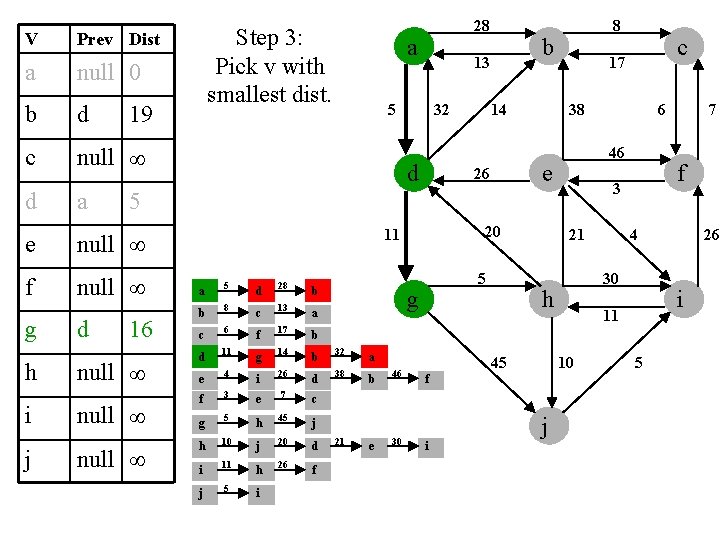
V a null 0 b d c null 19 d a e null f null g h i j Step 3: Pick v with smallest dist. Prev Dist d 28 a b 13 5 32 d 8 14 38 16 null 20 a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 5 g 21 21 45 j i 26 i 11 10 f 4 30 h 7 f 3 5 11 6 46 e 26 c 17 5
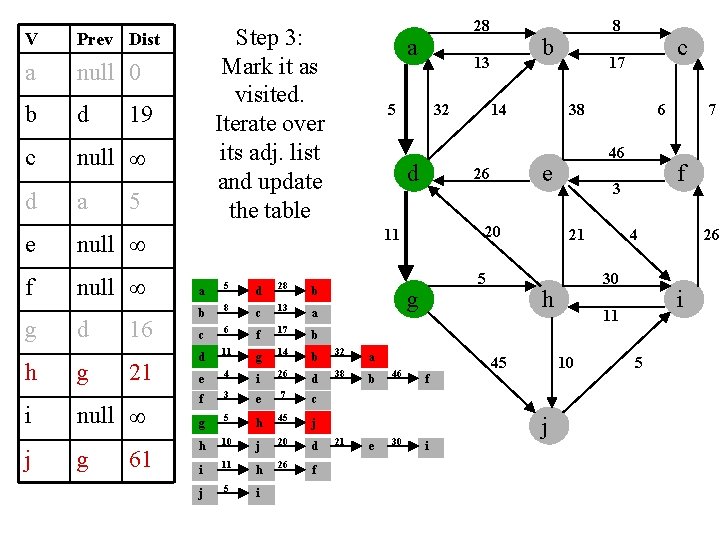
V a null 0 b d c null 19 d a e null f null g h i j Step 3: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g 5 16 21 null g 61 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
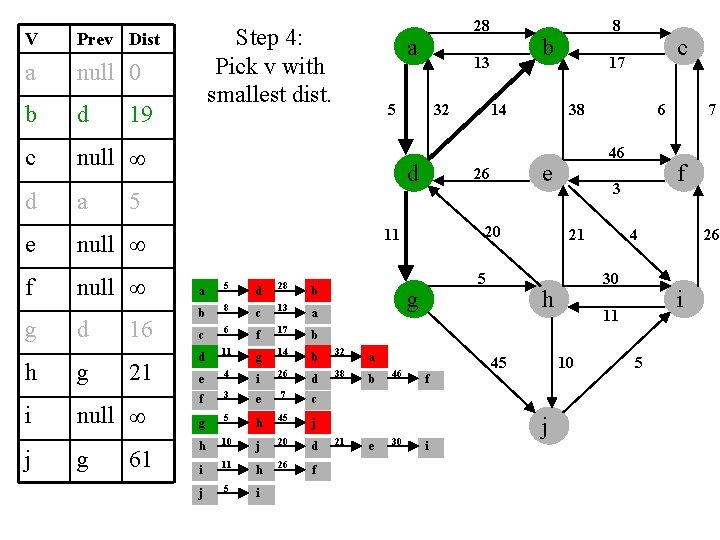
V a null 0 b d c null 19 d a e null f null g h i j Step 4: Pick v with smallest dist. Prev Dist d g a b 13 5 32 d 8 14 38 16 21 61 20 11 a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 5 g 21 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 26 c 17 5 null g 28 5
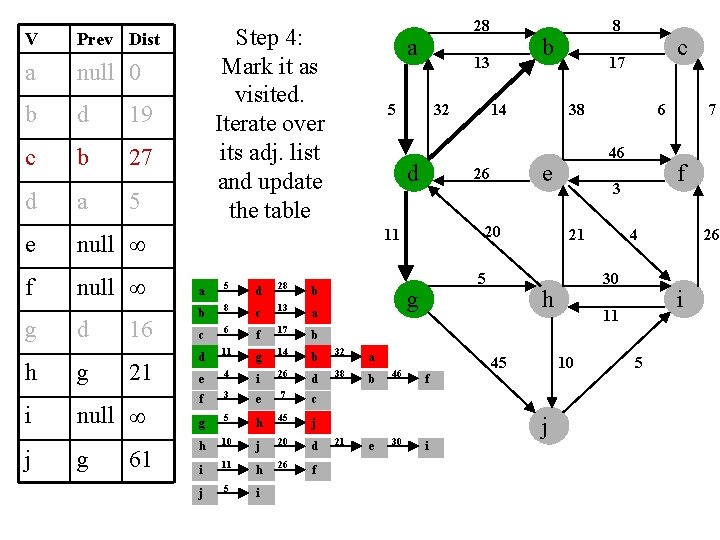
V a null 0 b d 19 c b 27 d a e null f null g h i j Step 4: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g 5 16 21 null g 61 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
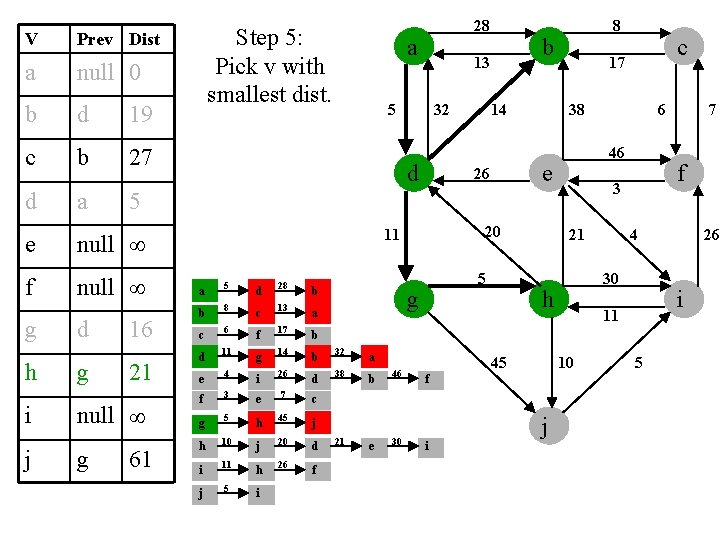
V a null 0 b d 19 c b 27 d a e null f null g h i j Step 5: Pick v with smallest dist. Prev Dist d g a b 13 5 32 d 8 14 38 16 21 61 20 11 a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 5 g 21 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 26 c 17 5 null g 28 5
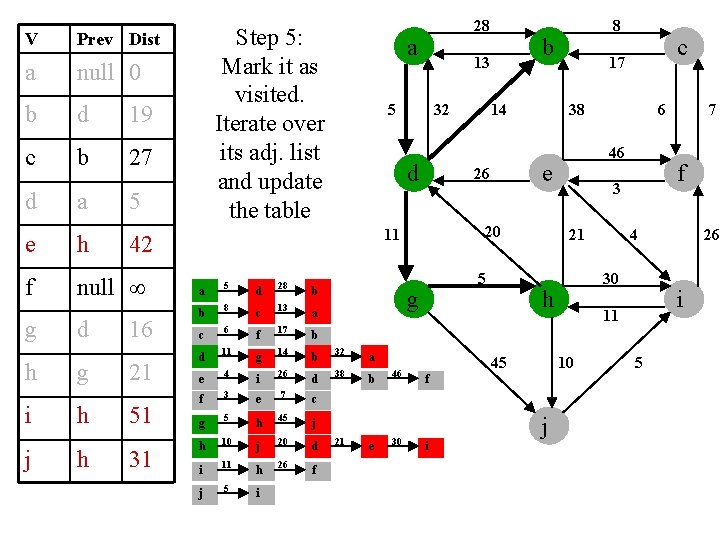
V a null 0 b d 19 c b 27 d a 5 e h 42 f null g h i j Step 5: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g h h 16 21 51 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
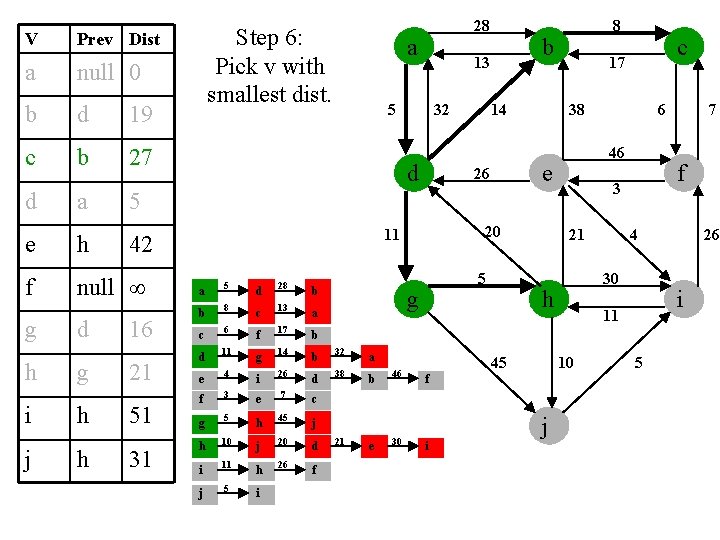
V a null 0 b d 19 c b 27 d a 5 e h 42 f null g h i j Step 6: Pick v with smallest dist. Prev Dist d g h h 16 21 51 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
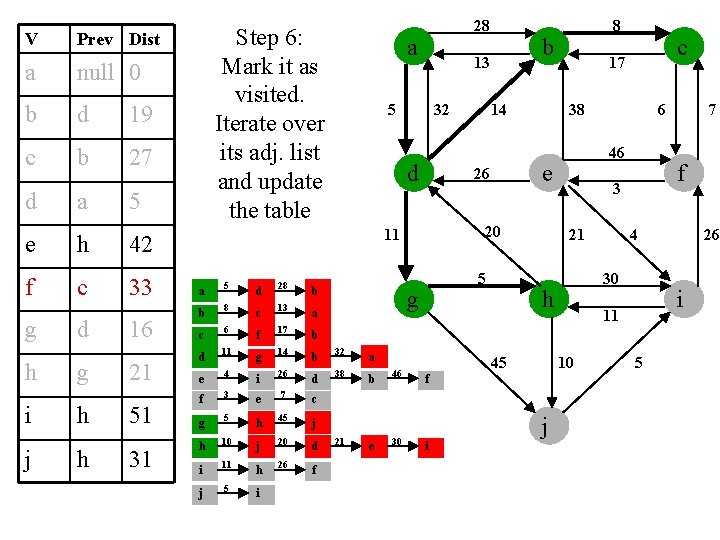
V a null 0 b d 19 c b 27 d a 5 e h 42 f c 33 g h i j Step 6: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g h h 16 21 51 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
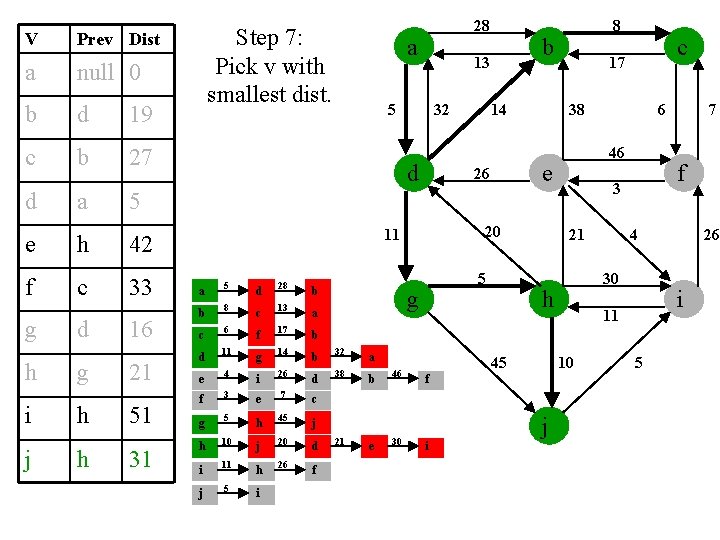
V a null 0 b d 19 c b 27 d a 5 e h 42 f c 33 g h i j Step 7: Pick v with smallest dist. Prev Dist d g h h 16 21 51 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
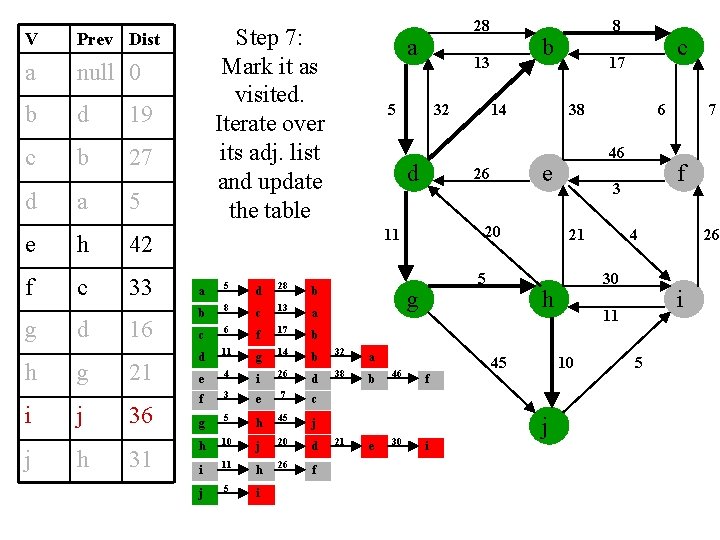
V a null 0 b d 19 c b 27 d a 5 e h 42 f c 33 g h i j Step 7: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g j h 16 21 36 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
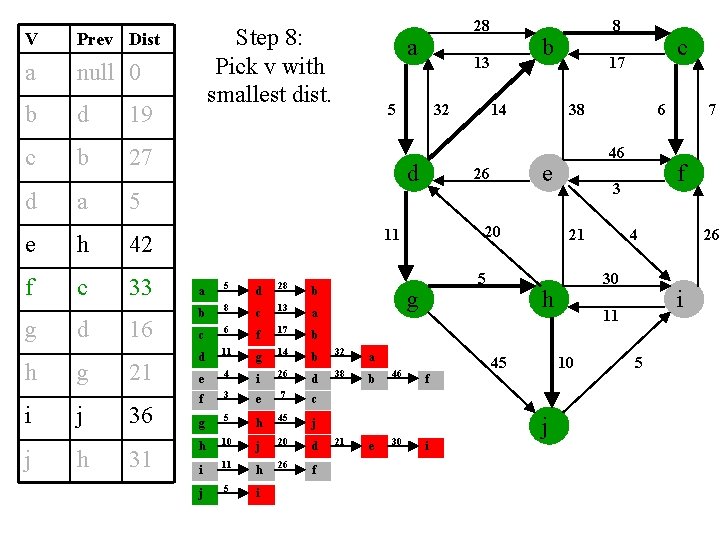
V a null 0 b d 19 c b 27 d a 5 e h 42 f c 33 g h i j Step 8: Pick v with smallest dist. Prev Dist d g j h 16 21 36 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
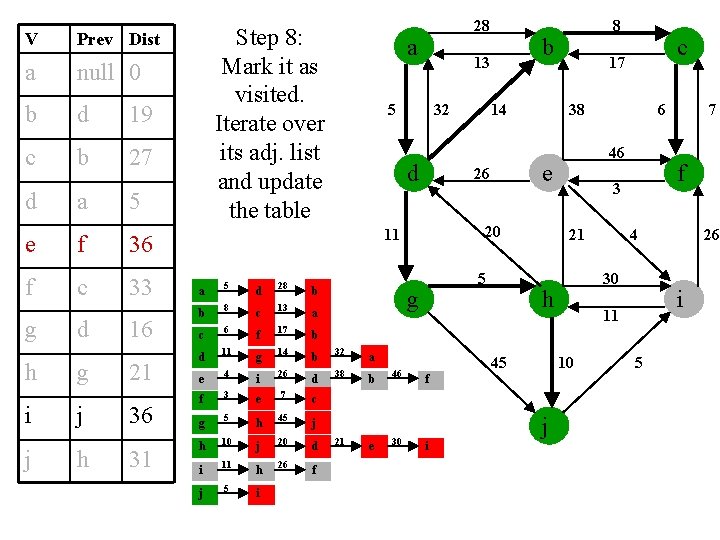
V a null 0 b d 19 c b 27 d a 5 e f 36 f c 33 g h i j Step 8: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g j h 16 21 36 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
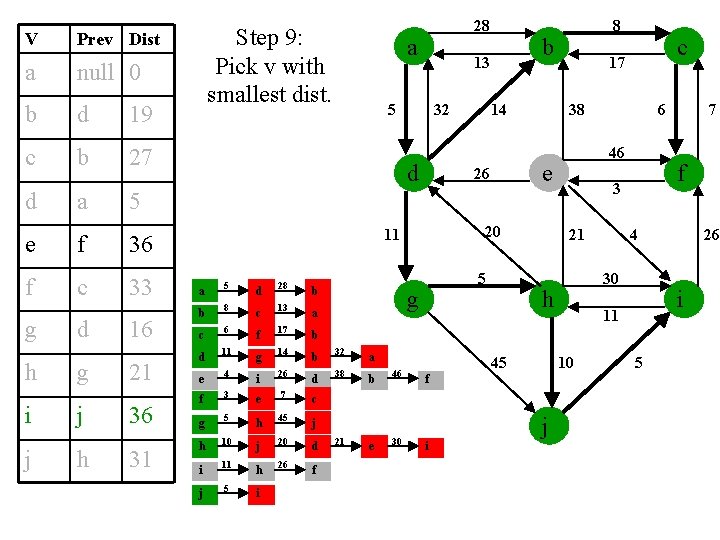
V a null 0 b d 19 c b 27 d a 5 e f 36 f c 33 g h i j Step 9: Pick v with smallest dist. Prev Dist d g j h 16 21 36 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
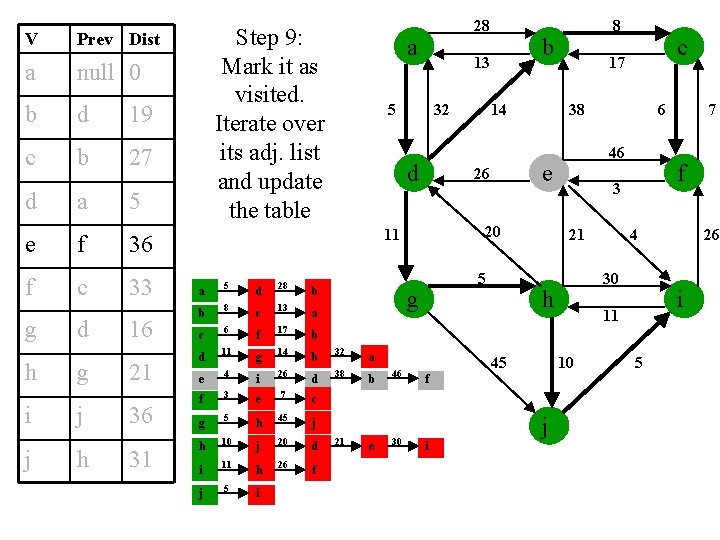
V a null 0 b d 19 c b 27 d a 5 e f 36 f c 33 g h i j Step 9: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g j h 16 21 36 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
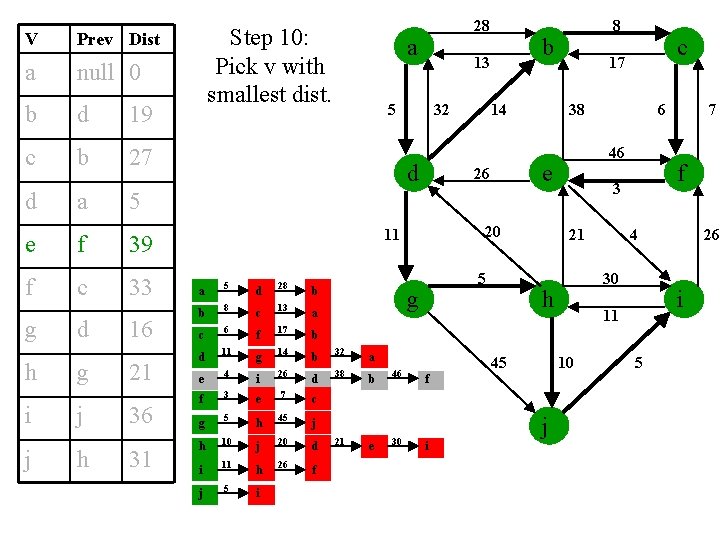
V a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 g h i j Step 10: Pick v with smallest dist. Prev Dist d g j h 16 21 36 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
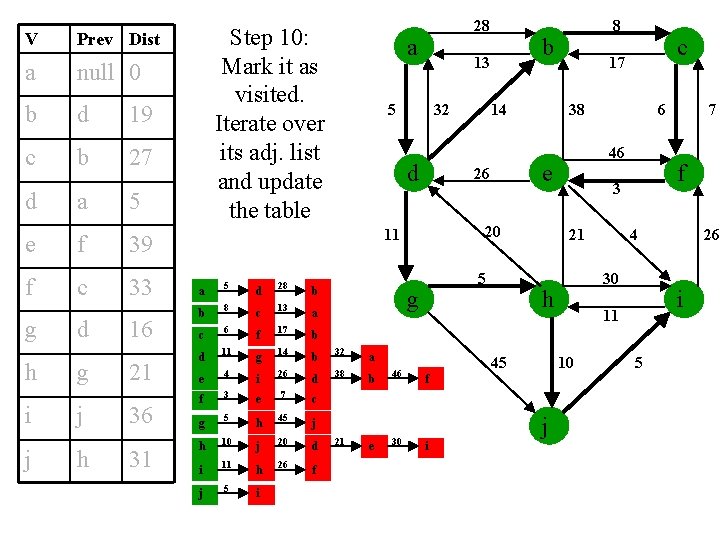
V a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 g h i j Step 10: Mark it as visited. Iterate over its adj. list and update the table Prev Dist d g j h 16 21 36 31 28 a 32 d 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f 3 e 7 c g 5 h 45 j h 10 j 20 d e 30 i 11 h 26 f j 5 i 45 26 i 11 10 j 4 30 h 7 f 3 21 f i 6 46 e 5 c 17 38 20 g 21 14 26 11 a b 13 5 8 5
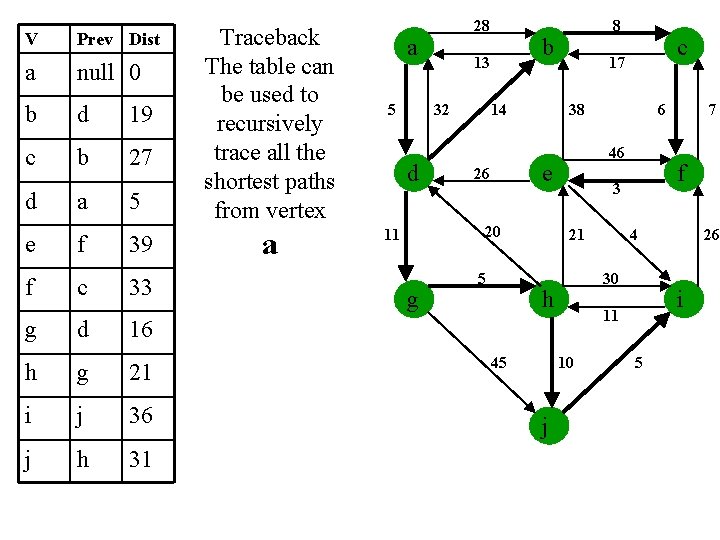
V Prev Dist a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 g d 16 h g 21 i j 36 j h 31 Traceback The table can be used to recursively trace all the shortest paths from vertex a 28 a 5 d b 13 32 14 g 5 6 46 e 26 c 17 38 20 11 8 26 i 11 10 j 4 30 h 45 f 3 21 7 5
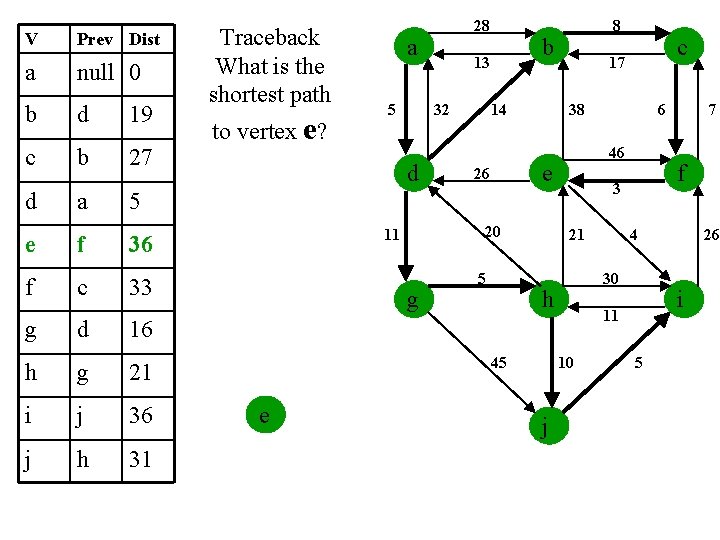
V Prev Dist a null 0 b d 19 c b 27 d a 5 e f 36 f c 33 g d 16 h g 21 i j 36 j h 31 Traceback What is the shortest path to vertex e? 28 a 5 d b 13 32 14 g 5 26 i 11 10 j 4 30 h 7 f 3 21 45 e 6 46 e 26 c 17 38 20 11 8 5
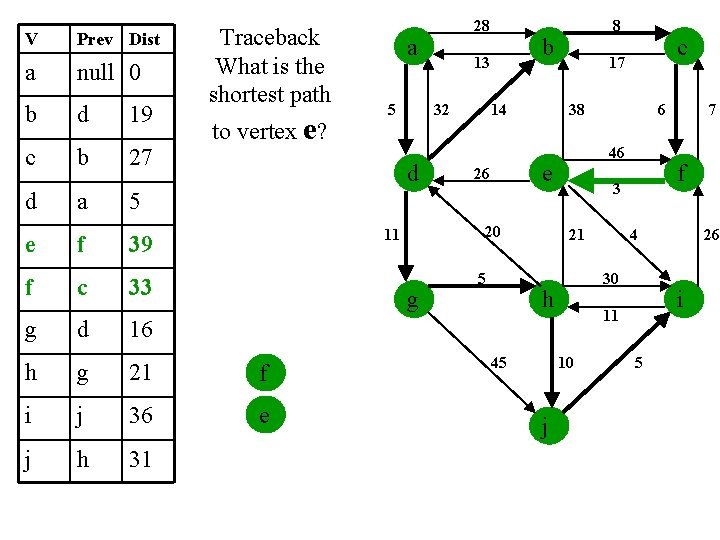
V Prev Dist a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 Traceback What is the shortest path to vertex e? a 5 g d 16 h g 21 f i j 36 e j h 31 14 5 6 46 e 26 c 17 38 20 11 8 b 13 32 d g 28 26 i 11 10 j 4 30 h 45 f 3 21 7 5
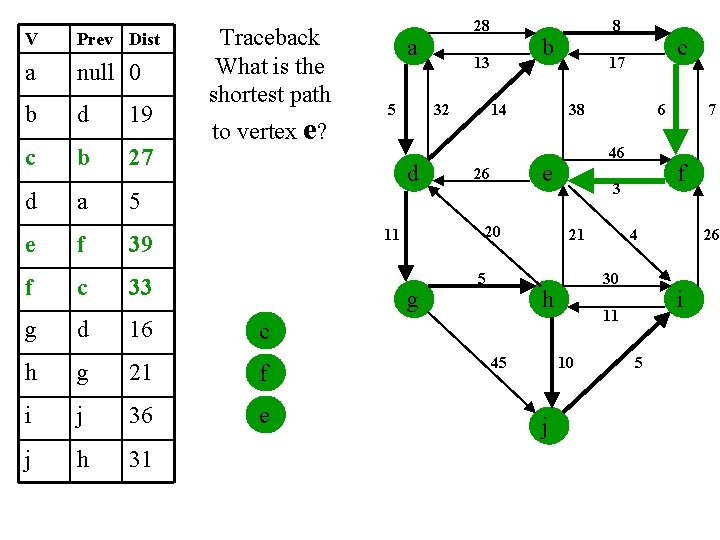
V Prev Dist a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 Traceback What is the shortest path to vertex e? a 5 g d 16 c h g 21 f i j 36 e j h 31 14 5 6 46 e 26 c 17 38 20 11 8 b 13 32 d g 28 26 i 11 10 j 4 30 h 45 f 3 21 7 5
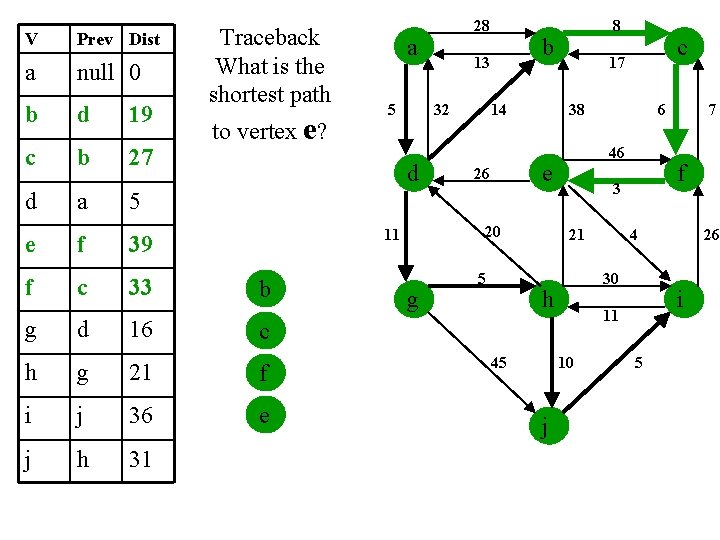
V Prev Dist a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 Traceback What is the shortest path to vertex e? a 5 g d 16 c h g 21 f i j 36 e j h 31 14 g 5 6 46 e 26 c 17 38 20 11 8 b 13 32 d b 28 26 i 11 10 j 4 30 h 45 f 3 21 7 5
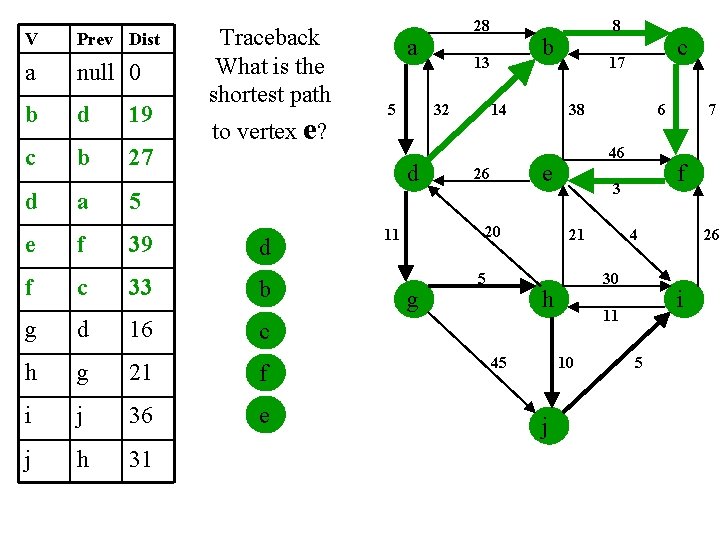
V Prev Dist a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 Traceback What is the shortest path to vertex e? a 5 b g d 16 c h g 21 f i j 36 e j h 31 14 g 5 6 46 e 26 c 17 38 20 11 8 b 13 32 d d 28 26 i 11 10 j 4 30 h 45 f 3 21 7 5
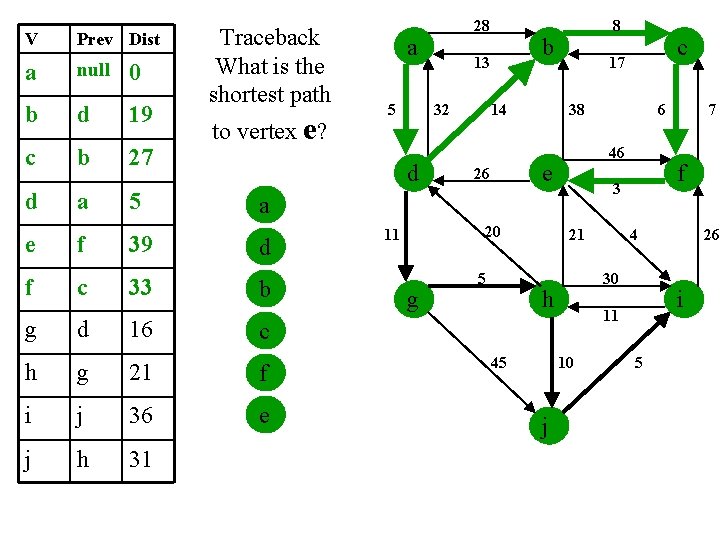
V Prev Dist a null 0 b d 19 c b 27 d a 5 e f 39 f c 33 Traceback What is the shortest path to vertex e? 28 a 5 d b 13 32 8 14 38 d b g d 16 c h g 21 f i j 36 e j h 31 20 g 5 21 45 26 i 11 10 j 4 30 h 7 f 3 a 11 6 46 e 26 c 17 5
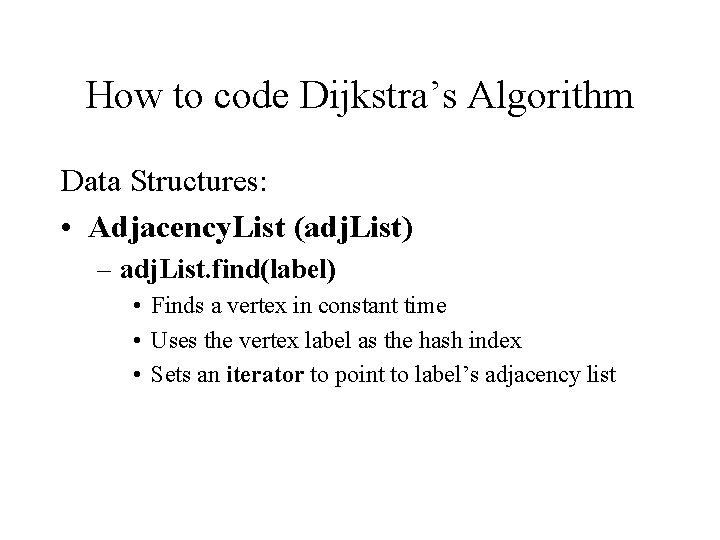
How to code Dijkstra’s Algorithm Data Structures: • Adjacency. List (adj. List) – adj. List. find(label) • Finds a vertex in constant time • Uses the vertex label as the hash index • Sets an iterator to point to label’s adjacency list
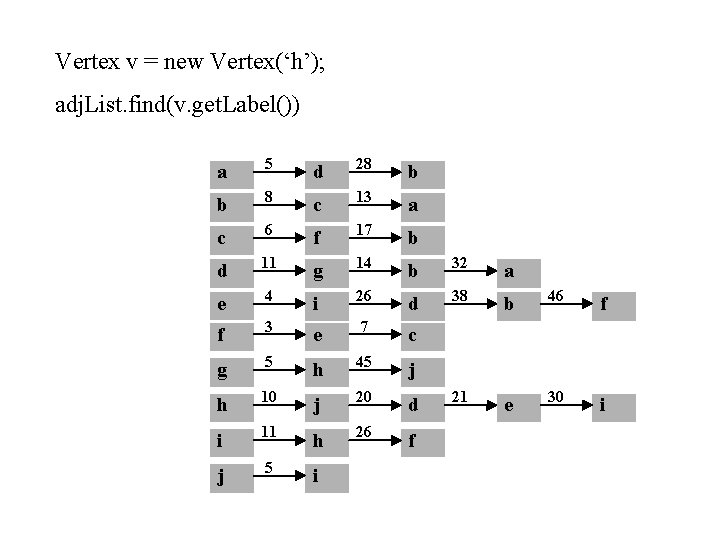
Vertex v = new Vertex(‘h’); adj. List. find(v. get. Label()) a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f f 3 e 7 c g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i
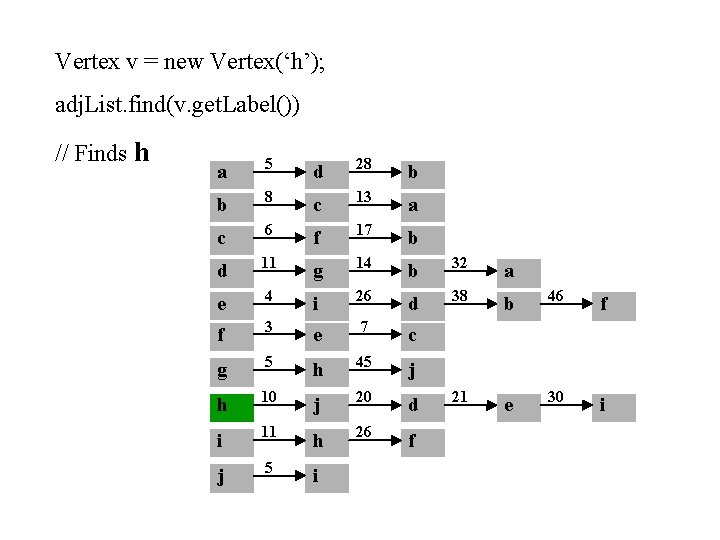
Vertex v = new Vertex(‘h’); adj. List. find(v. get. Label()) // Finds h a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f f 3 e 7 c g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i
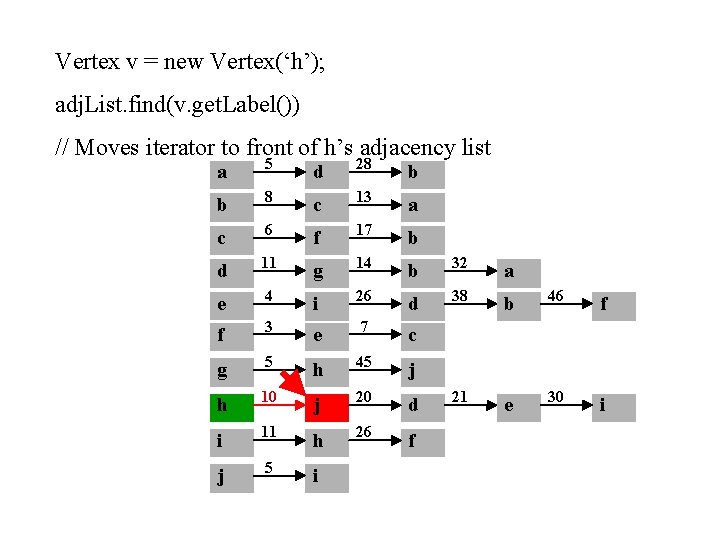
Vertex v = new Vertex(‘h’); adj. List. find(v. get. Label()) // Moves iterator to front of h’s adjacency list a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f f 3 e 7 c g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i
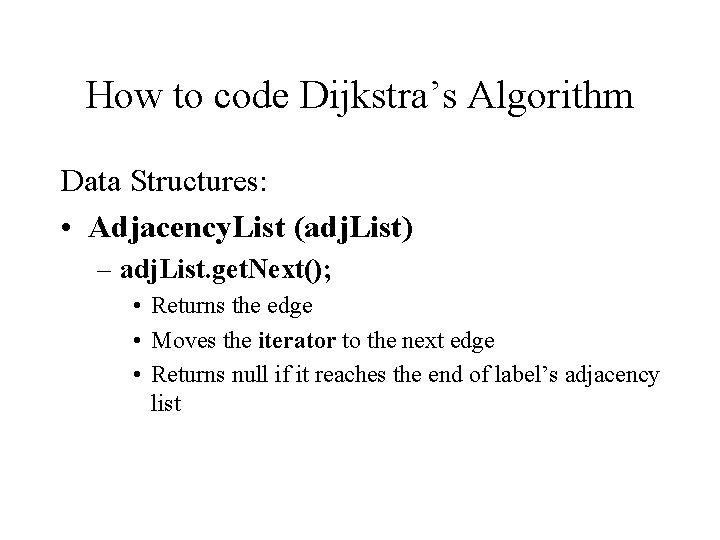
How to code Dijkstra’s Algorithm Data Structures: • Adjacency. List (adj. List) – adj. List. get. Next(); • Returns the edge • Moves the iterator to the next edge • Returns null if it reaches the end of label’s adjacency list
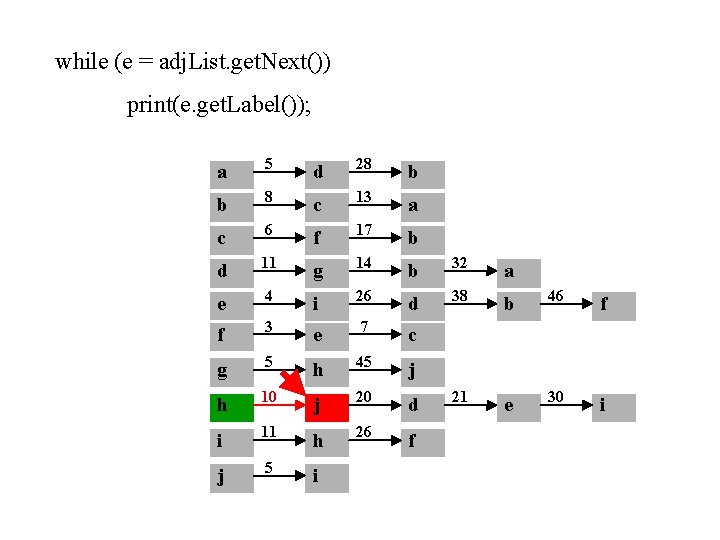
while (e = adj. List. get. Next()) print(e. get. Label()); a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f f 3 e 7 c g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i
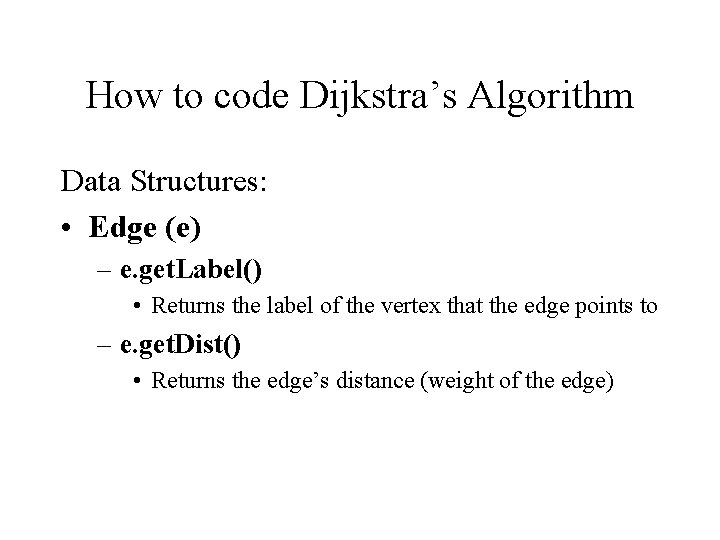
How to code Dijkstra’s Algorithm Data Structures: • Edge (e) – e. get. Label() • Returns the label of the vertex that the edge points to – e. get. Dist() • Returns the edge’s distance (weight of the edge)
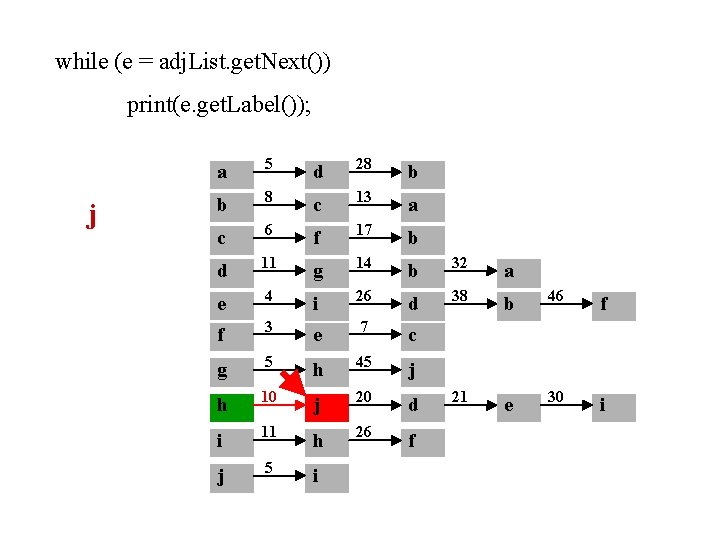
while (e = adj. List. get. Next()) print(e. get. Label()); j a 5 d 28 b b 8 c 13 a c 6 f 17 b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f f 3 e 7 c g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i
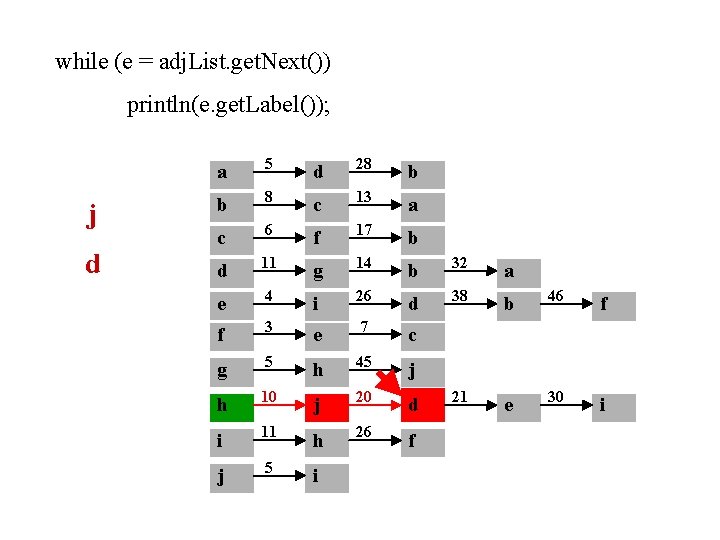
while (e = adj. List. get. Next()) println(e. get. Label()); a 5 d 28 b j b 8 c 13 a 6 f 17 d c b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f f 3 e 7 c g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i
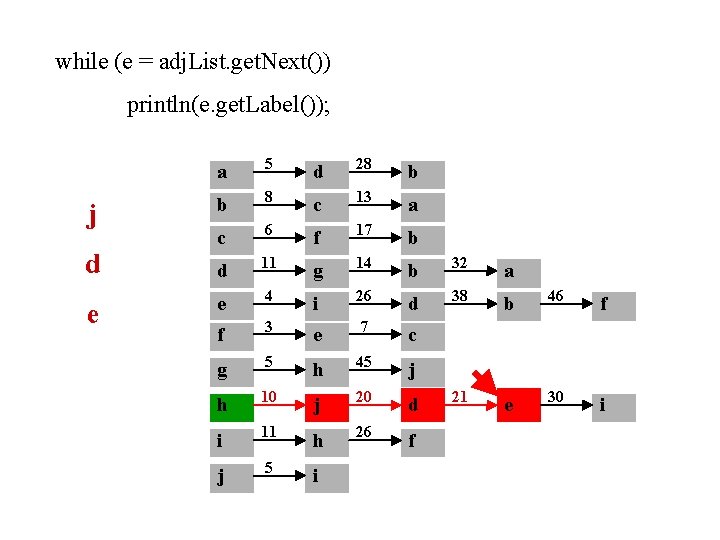
while (e = adj. List. get. Next()) println(e. get. Label()); a 5 d 28 b j b 8 c 13 a 6 f 17 d c b d 11 g 14 b 32 a e 4 i 26 d 38 b 46 f f 3 e 7 c g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i e
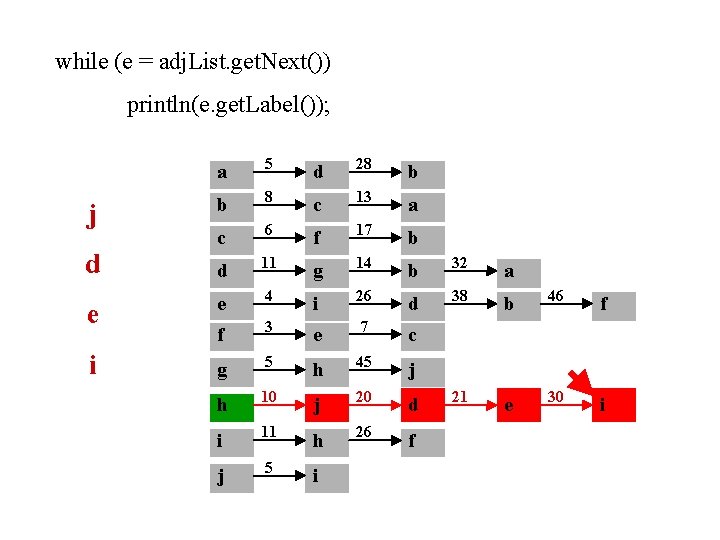
while (e = adj. List. get. Next()) println(e. get. Label()); a 5 d 28 b j b 8 c 13 a 6 f 17 d c b d 11 g 14 b 32 a e e 4 i 26 d 38 b 46 f f 3 e 7 c i g 5 h 45 j h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i
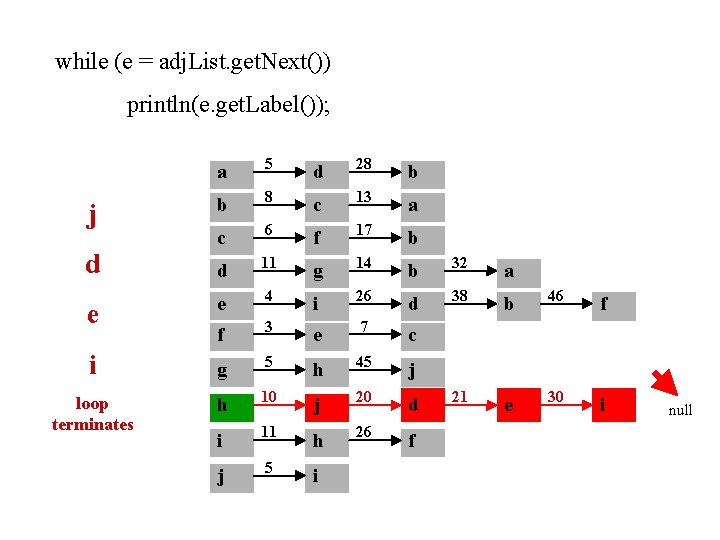
while (e = adj. List. get. Next()) println(e. get. Label()); a 5 d 28 b j b 8 c 13 a 6 f 17 d c b d 11 g 14 b 32 a e e 4 i 26 d 38 b 46 f f 3 e 7 c i g 5 h 45 j loop terminates h 10 j 20 d 21 e 30 i i 11 h 26 f j 5 i null
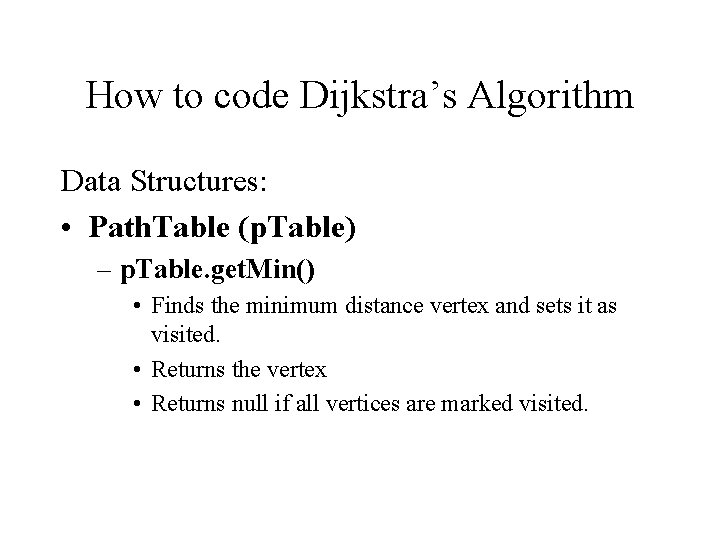
How to code Dijkstra’s Algorithm Data Structures: • Path. Table (p. Table) – p. Table. get. Min() • Finds the minimum distance vertex and sets it as visited. • Returns the vertex • Returns null if all vertices are marked visited.
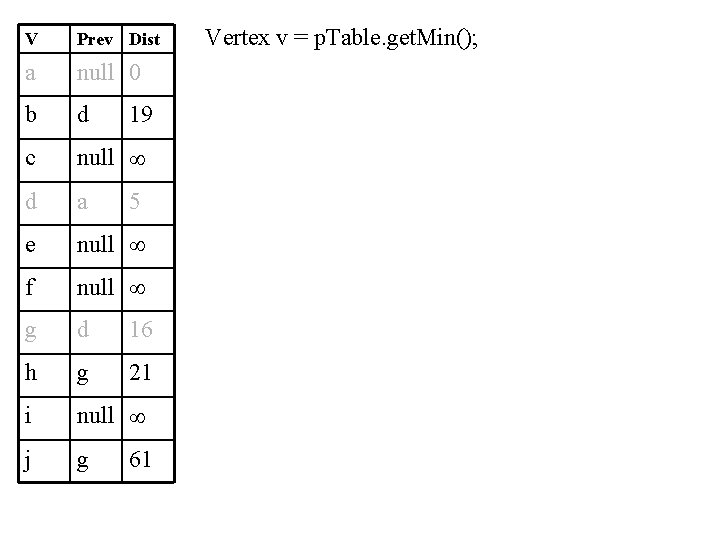
V Prev Dist a null 0 b d c null d a e null f null g d 16 h g 21 i null j g 19 5 61 Vertex v = p. Table. get. Min();
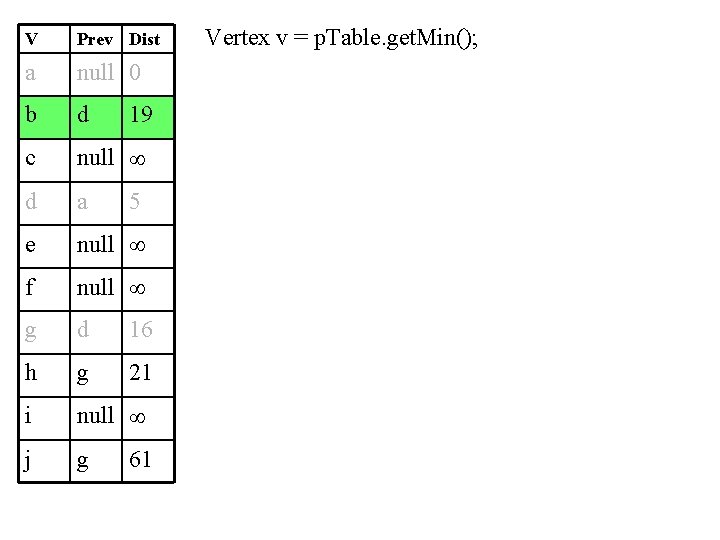
V Prev Dist a null 0 b d c null d a e null f null g d 16 h g 21 i null j g 19 5 61 Vertex v = p. Table. get. Min();
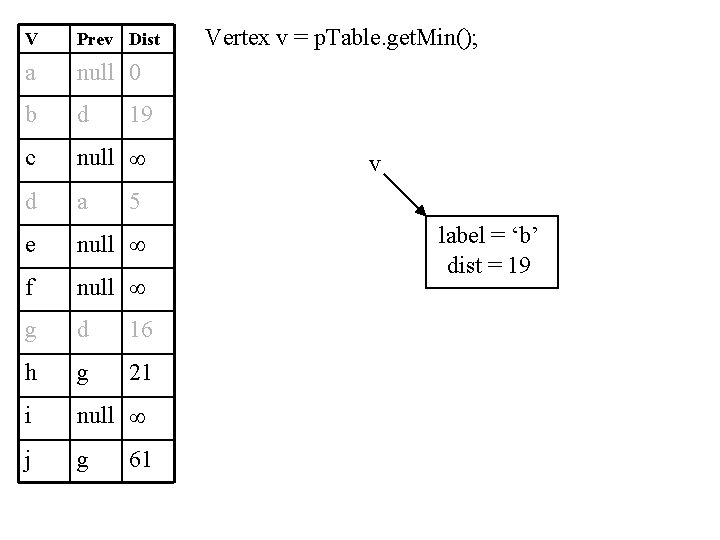
V Prev Dist a null 0 b d c null d a e null f null g d 16 h g 21 i null j g Vertex v = p. Table. get. Min(); 19 v 5 61 label = ‘b’ dist = 19
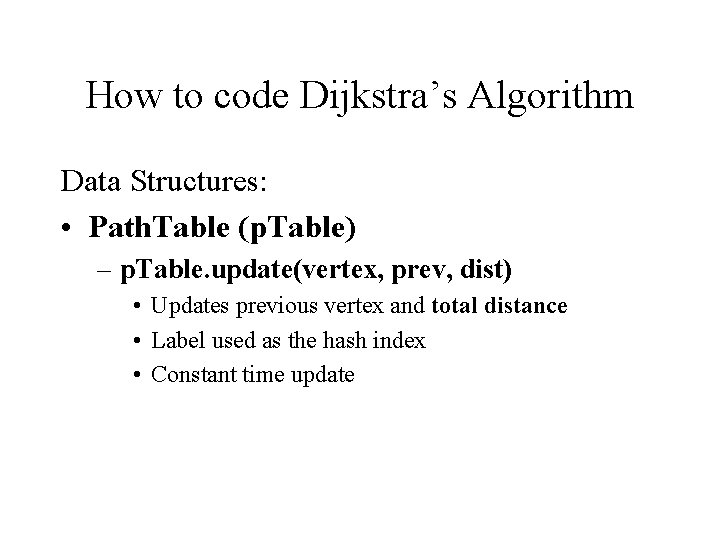
How to code Dijkstra’s Algorithm Data Structures: • Path. Table (p. Table) – p. Table. update(vertex, prev, dist) • Updates previous vertex and total distance • Label used as the hash index • Constant time update
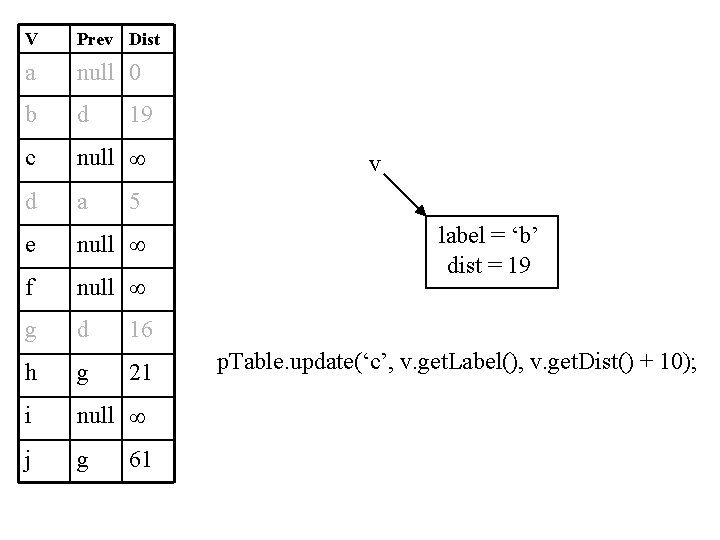
V Prev Dist a null 0 b d c null d a e null f null g d 16 h g 21 i null j g 19 v 5 61 label = ‘b’ dist = 19 p. Table. update(‘c’, v. get. Label(), v. get. Dist() + 10);
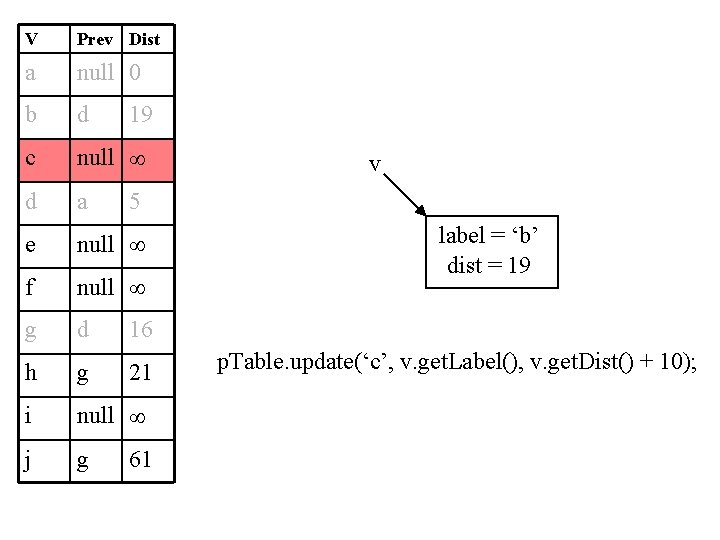
V Prev Dist a null 0 b d c null d a e null f null g d 16 h g 21 i null j g 19 v 5 61 label = ‘b’ dist = 19 p. Table. update(‘c’, v. get. Label(), v. get. Dist() + 10);
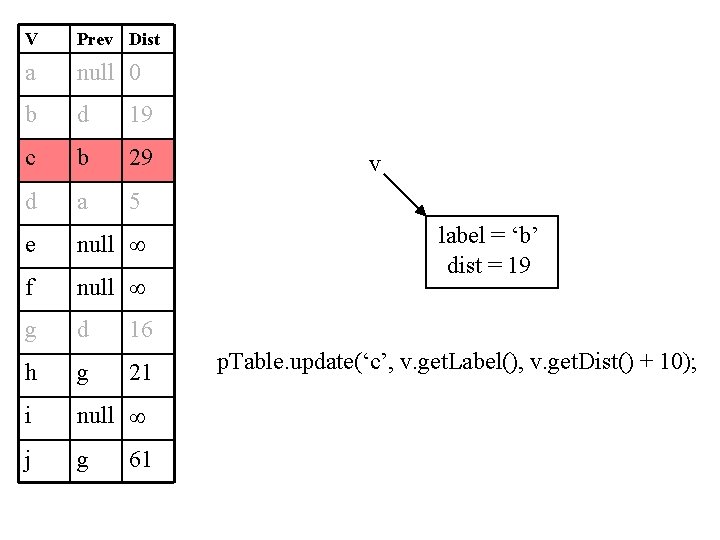
V Prev Dist a null 0 b d 19 c b 29 d a 5 e null f null g d 16 h g 21 i null j g 61 v label = ‘b’ dist = 19 p. Table. update(‘c’, v. get. Label(), v. get. Dist() + 10);
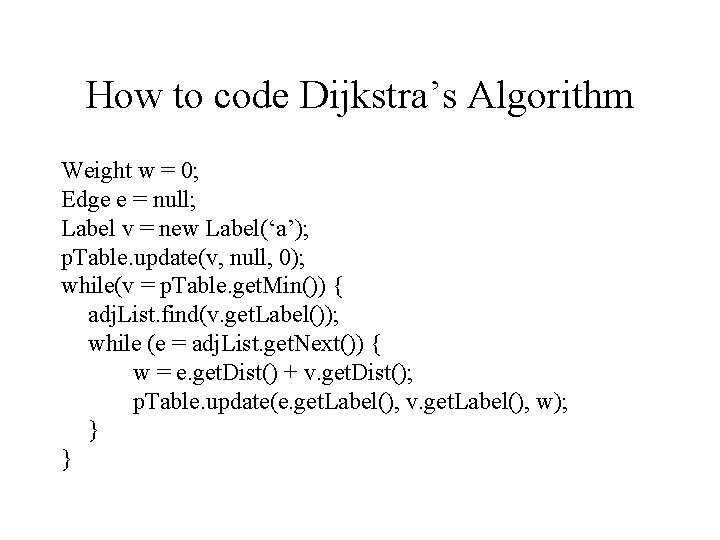
How to code Dijkstra’s Algorithm Weight w = 0; Edge e = null; Label v = new Label(‘a’); p. Table. update(v, null, 0); while(v = p. Table. get. Min()) { adj. List. find(v. get. Label()); while (e = adj. List. get. Next()) { w = e. get. Dist() + v. get. Dist(); p. Table. update(e. get. Label(), v. get. Label(), w); } }
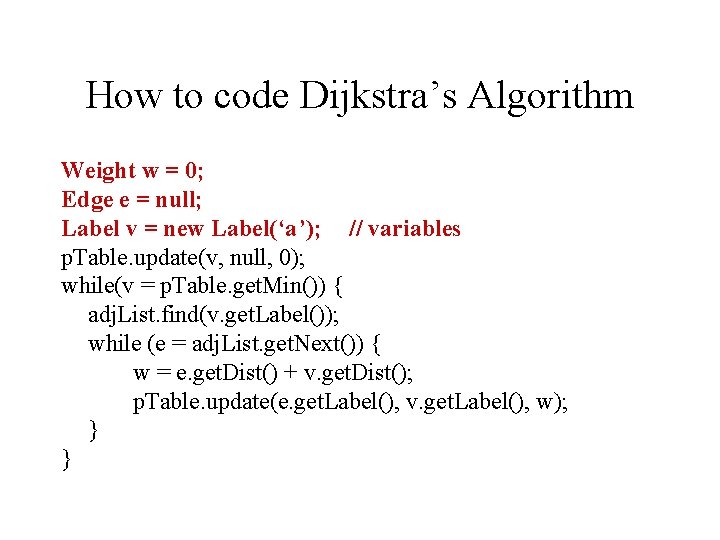
How to code Dijkstra’s Algorithm Weight w = 0; Edge e = null; Label v = new Label(‘a’); // variables p. Table. update(v, null, 0); while(v = p. Table. get. Min()) { adj. List. find(v. get. Label()); while (e = adj. List. get. Next()) { w = e. get. Dist() + v. get. Dist(); p. Table. update(e. get. Label(), v. get. Label(), w); } }
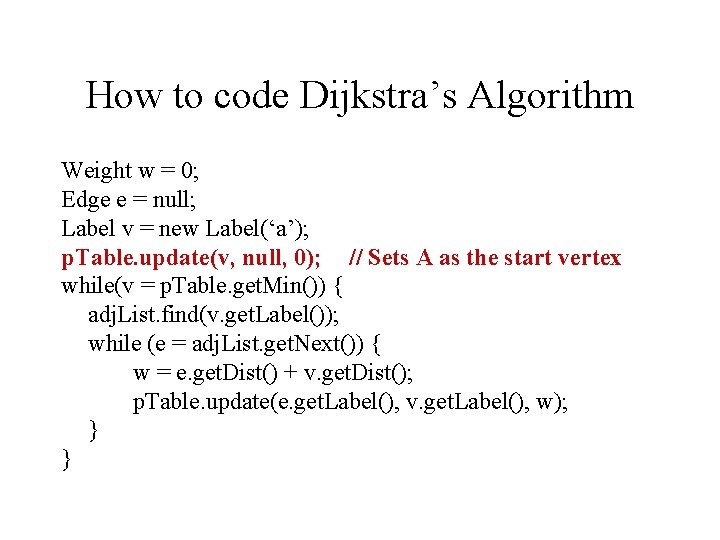
How to code Dijkstra’s Algorithm Weight w = 0; Edge e = null; Label v = new Label(‘a’); p. Table. update(v, null, 0); // Sets A as the start vertex while(v = p. Table. get. Min()) { adj. List. find(v. get. Label()); while (e = adj. List. get. Next()) { w = e. get. Dist() + v. get. Dist(); p. Table. update(e. get. Label(), v. get. Label(), w); } }
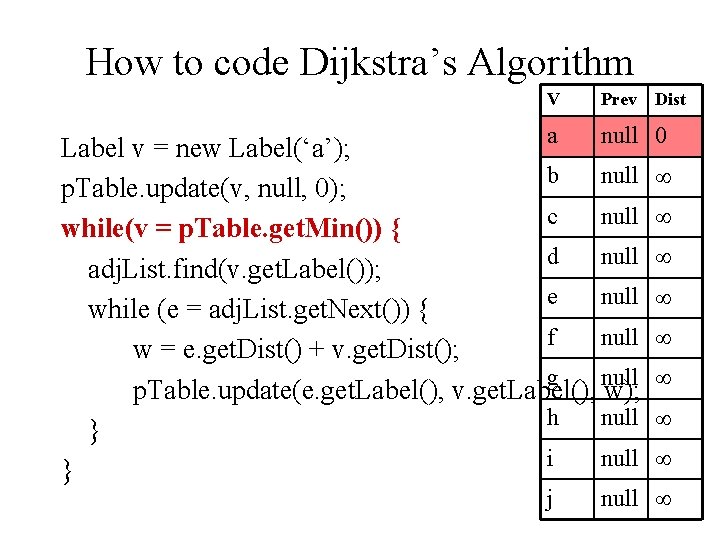
How to code Dijkstra’s Algorithm V Prev a null 0 Label v = new Label(‘a’); b null p. Table. update(v, null, 0); c null while(v = p. Table. get. Min()) { d null adj. List. find(v. get. Label()); e null while (e = adj. List. get. Next()) { f null w = e. get. Dist() + v. get. Dist(); g null p. Table. update(e. get. Label(), v. get. Label(), w); h null } i null } j Dist null
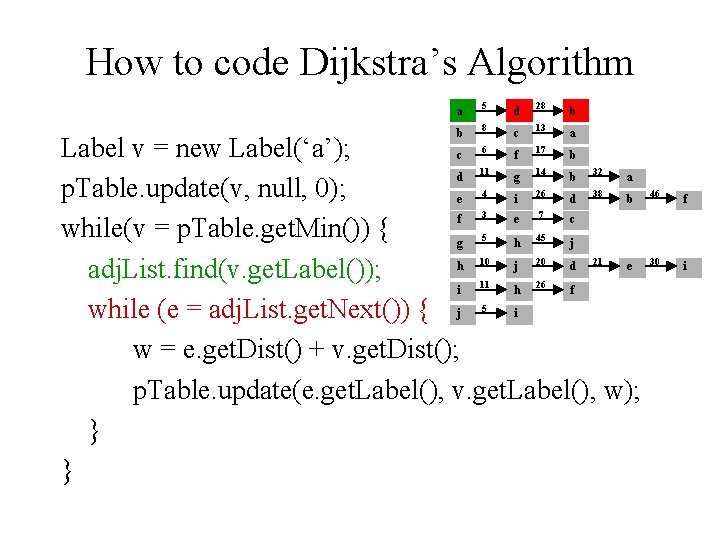
How to code Dijkstra’s Algorithm a 5 d 28 b b 8 c 13 a Label v = new Label(‘a’); c f b b a d g p. Table. update(v, null, 0); b e i d f e c while(v = p. Table. get. Min()) { g h j d e adj. List. find(v. get. Label()); i h f i while (e = adj. List. get. Next()) { j w = e. get. Dist() + v. get. Dist(); p. Table. update(e. get. Label(), v. get. Label(), w); } } 6 17 11 14 32 4 26 38 46 f 3 7 5 45 10 20 21 30 i 11 26 5
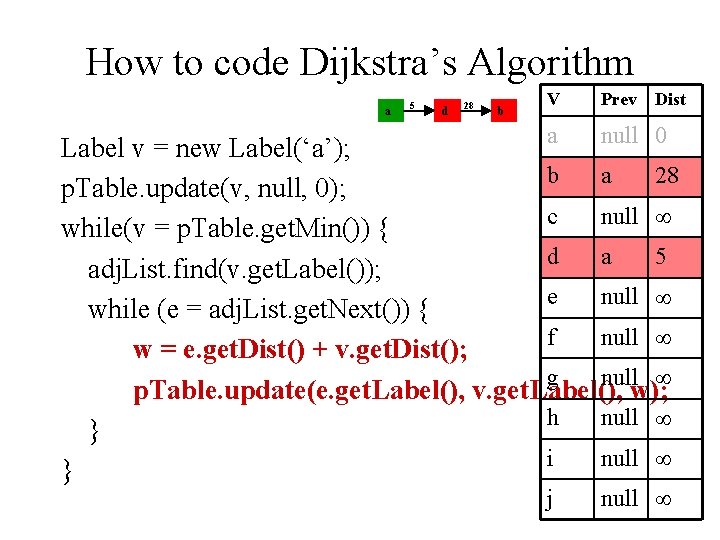
How to code Dijkstra’s Algorithm a 5 d 28 b V Prev Dist a null 0 j null Label v = new Label(‘a’); b a 28 p. Table. update(v, null, 0); c null while(v = p. Table. get. Min()) { d a 5 adj. List. find(v. get. Label()); e null while (e = adj. List. get. Next()) { f null w = e. get. Dist() + v. get. Dist(); g null p. Table. update(e. get. Label(), v. get. Label(), w); h null } i null }