Advanced Flow of Control Joe Komar 1292022 Komar
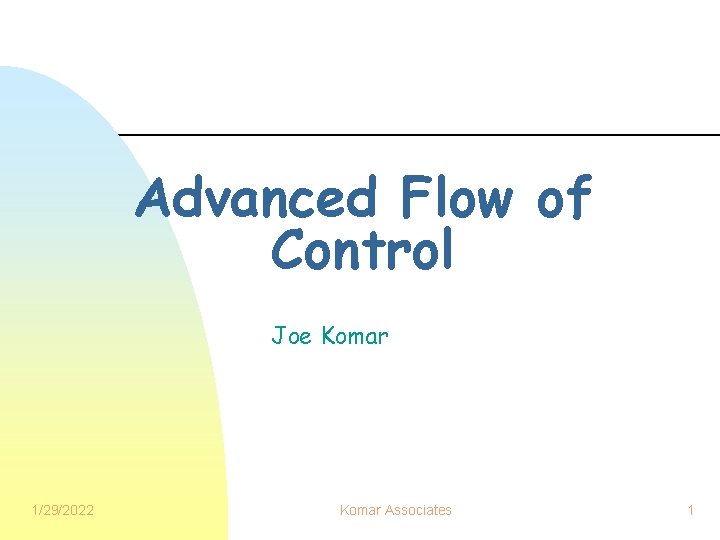
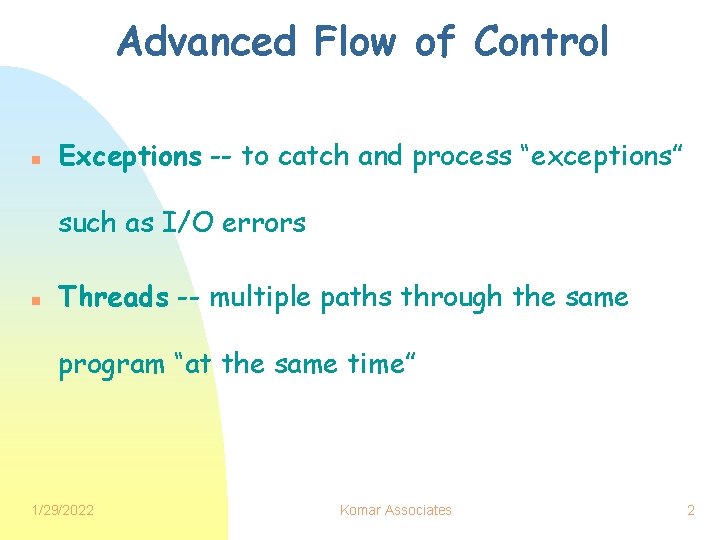
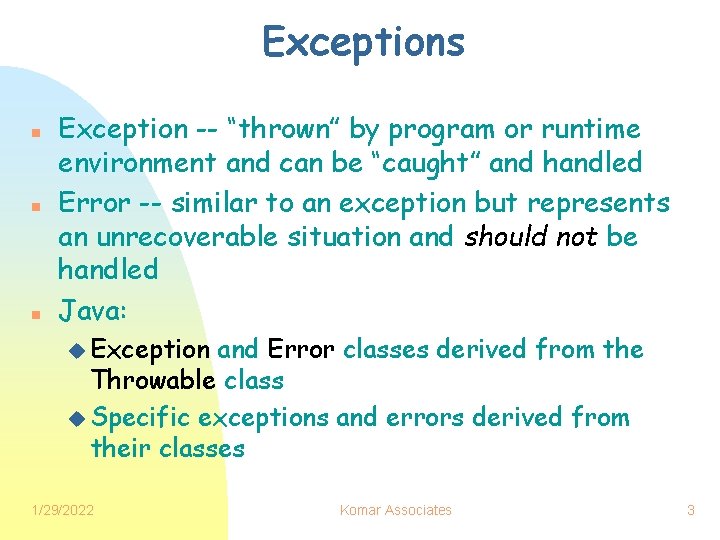
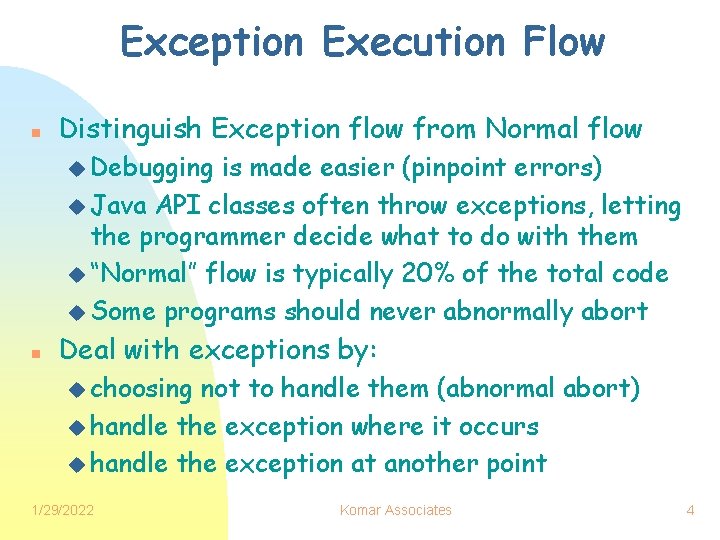
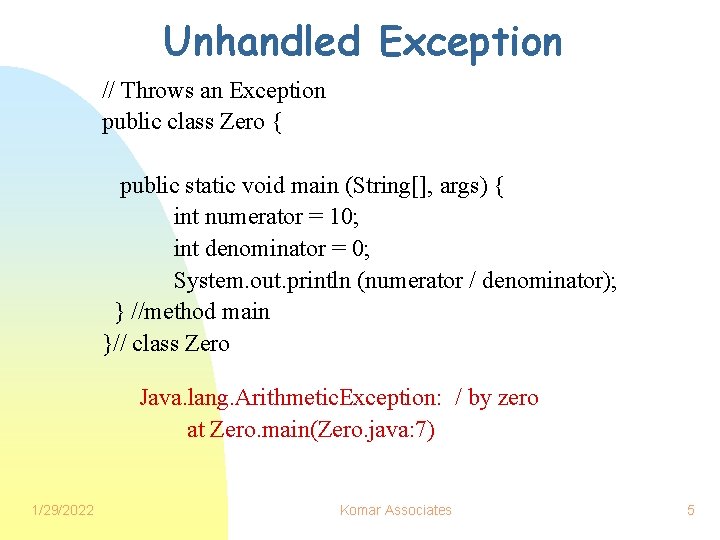
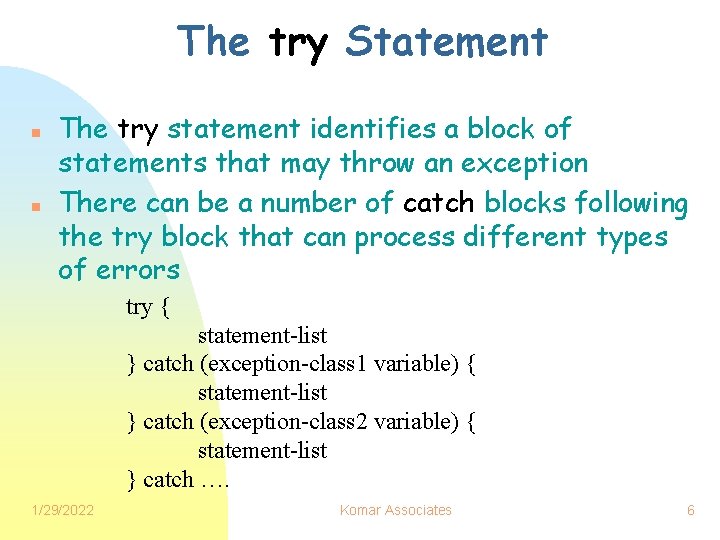
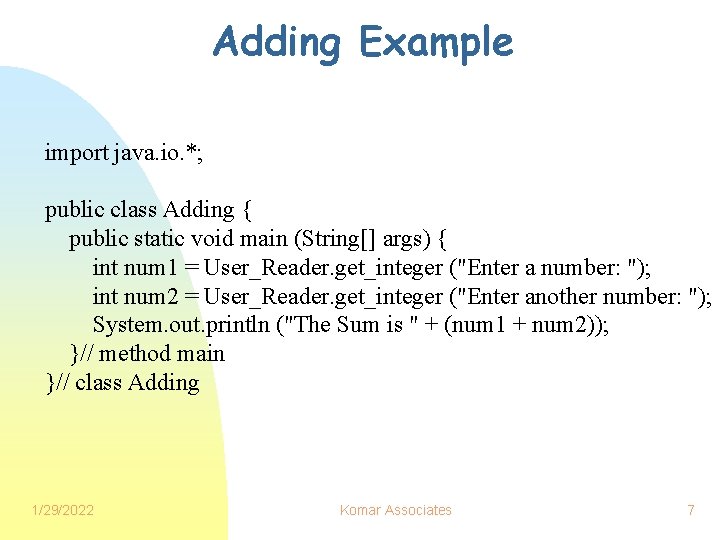
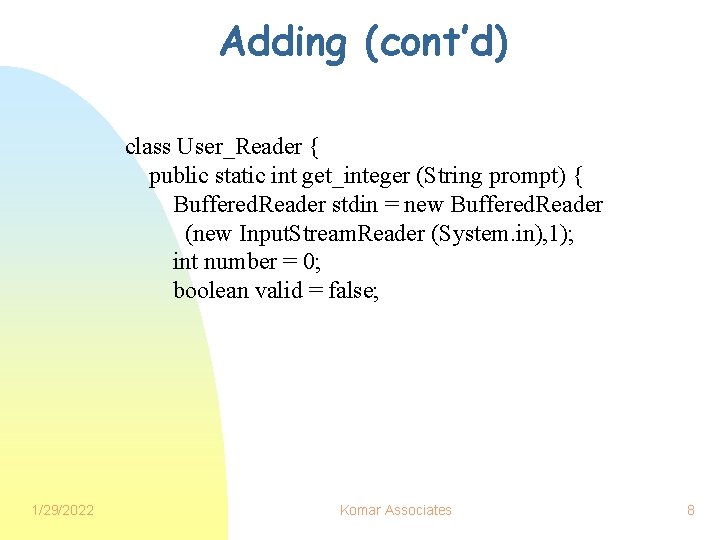
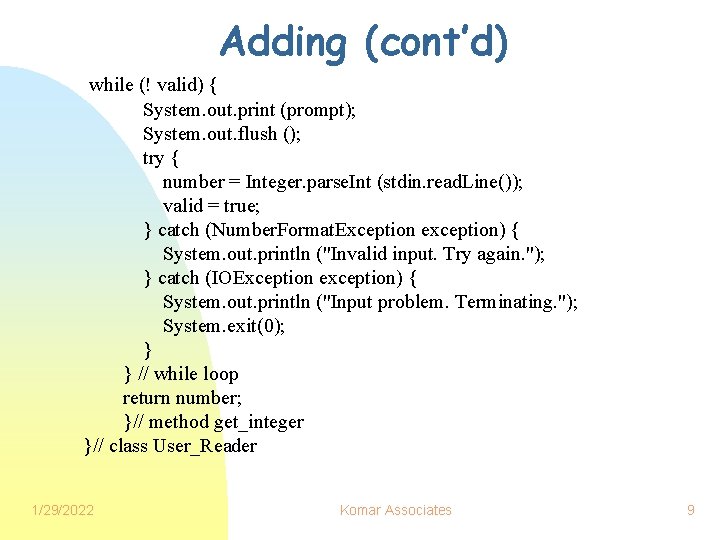
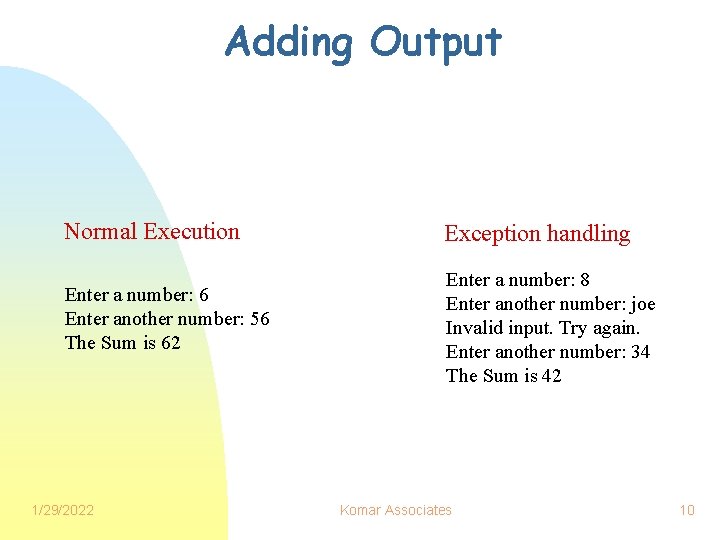
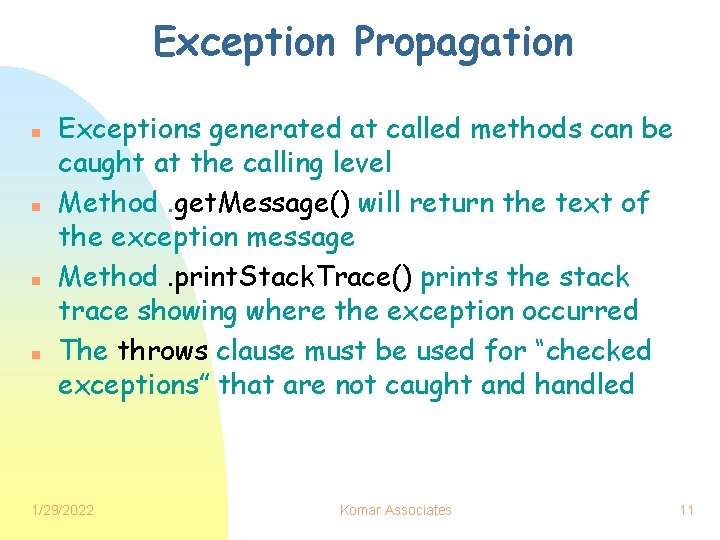
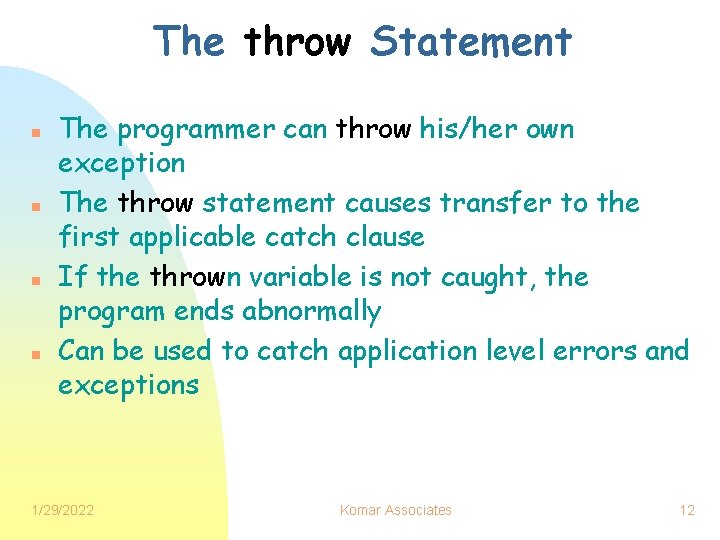
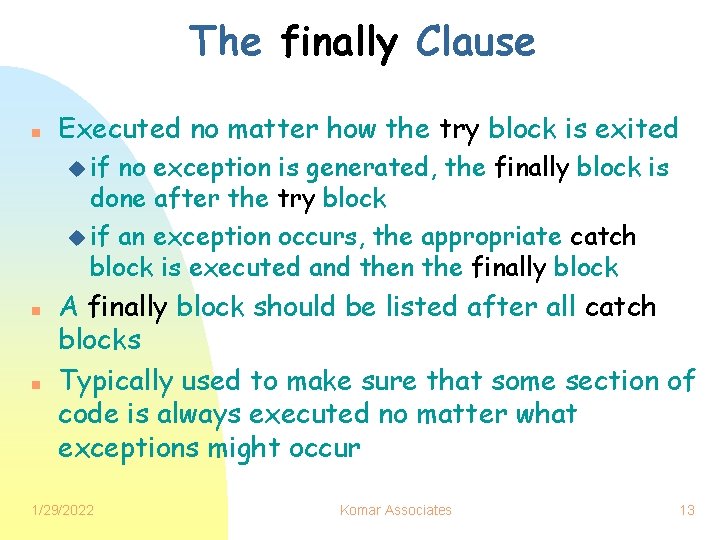
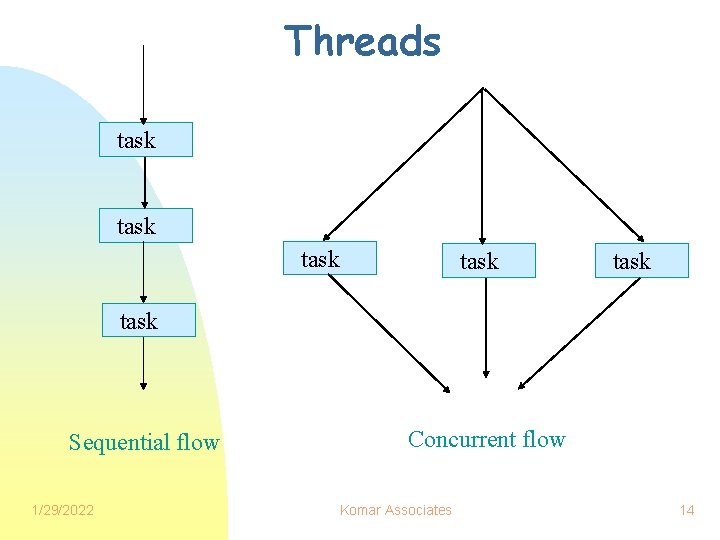
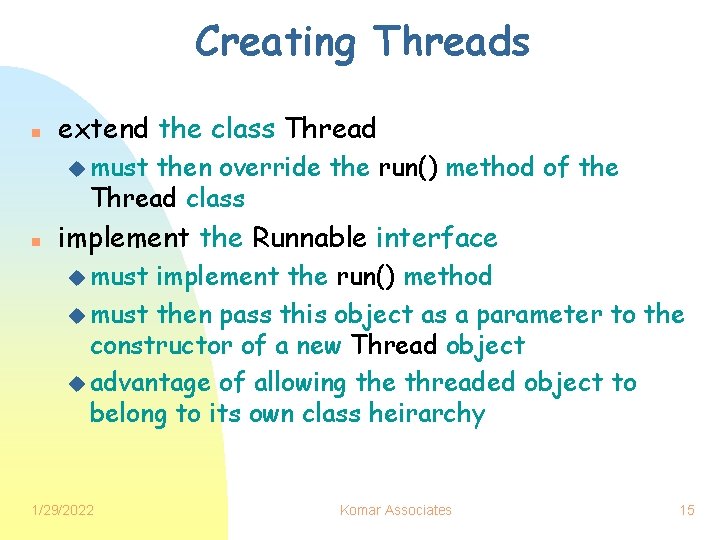
![Simultaneous public class Simultaneous { public static void main (String[]args) { Soda one = Simultaneous public class Simultaneous { public static void main (String[]args) { Soda one =](https://slidetodoc.com/presentation_image_h2/95b86dd13c880c7d4558ff010de94a89/image-16.jpg)
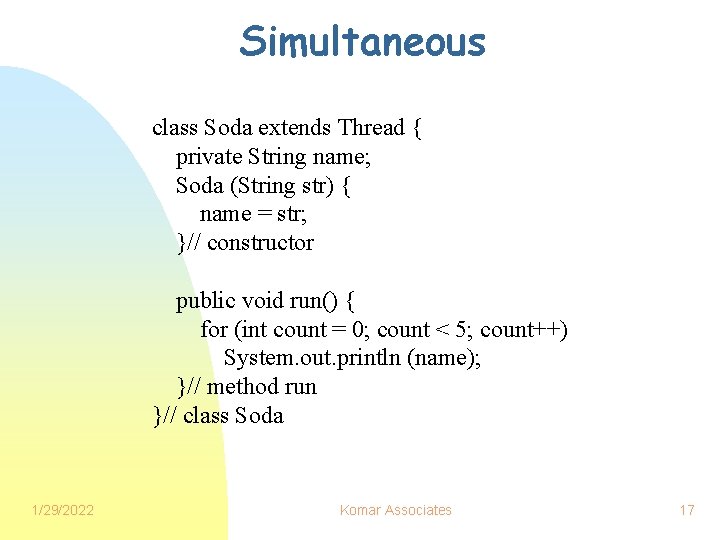
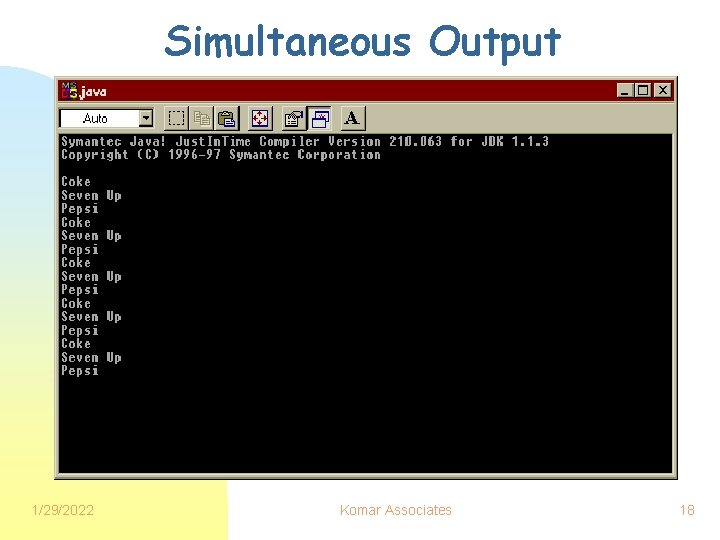
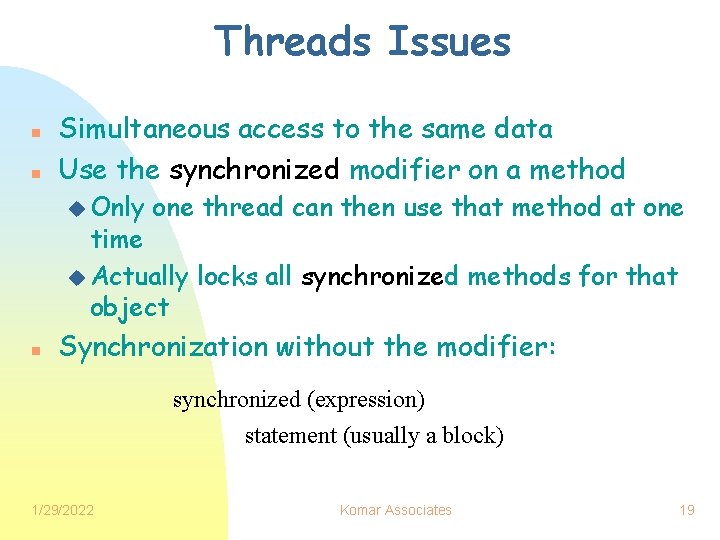
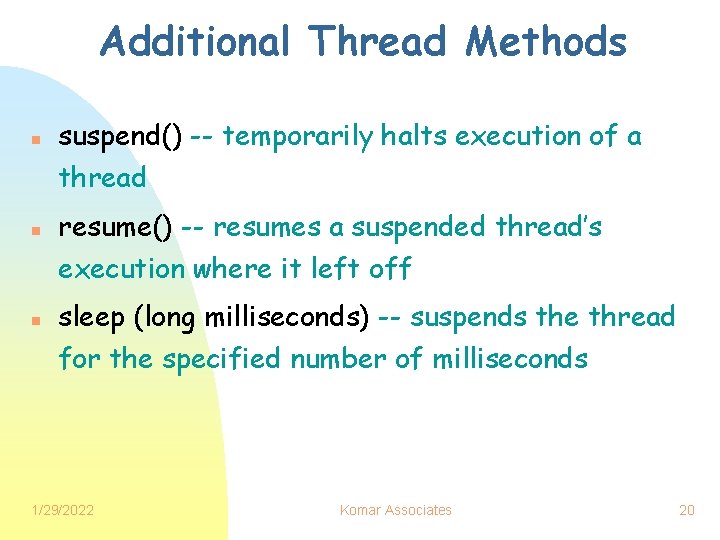
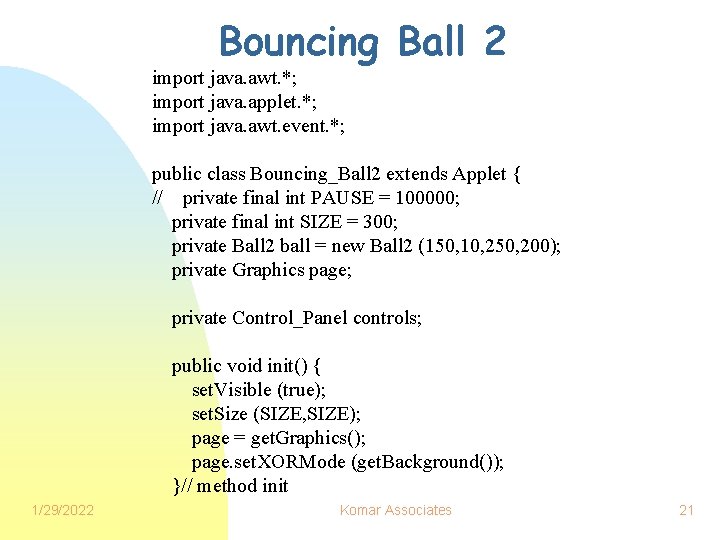
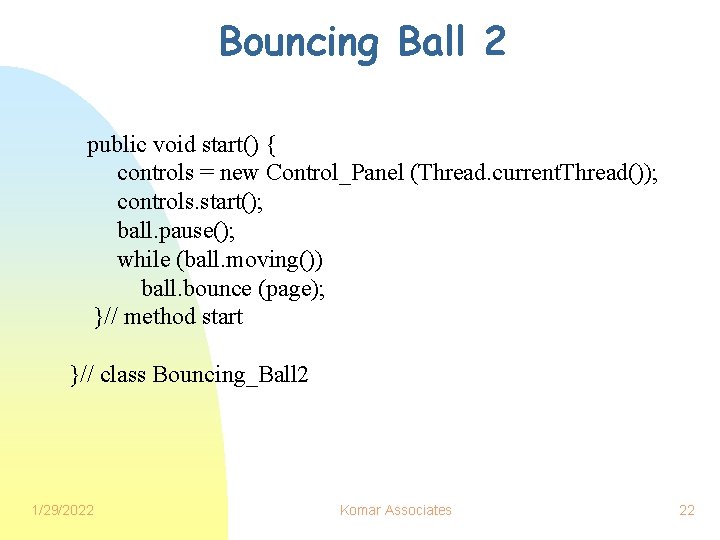
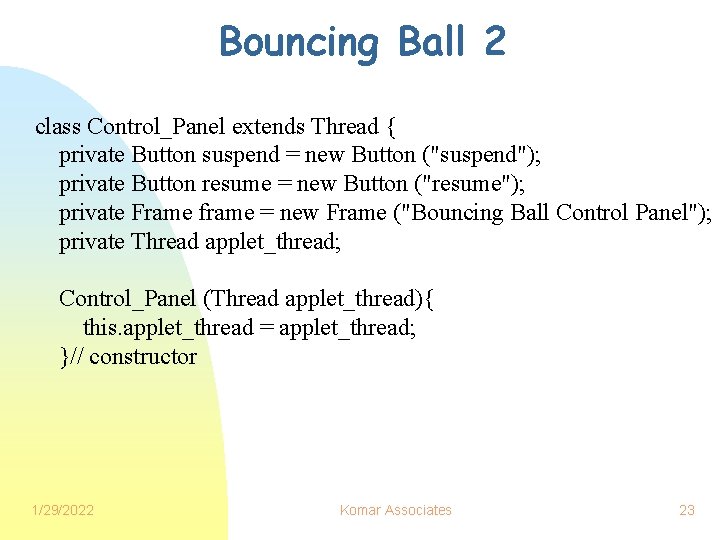
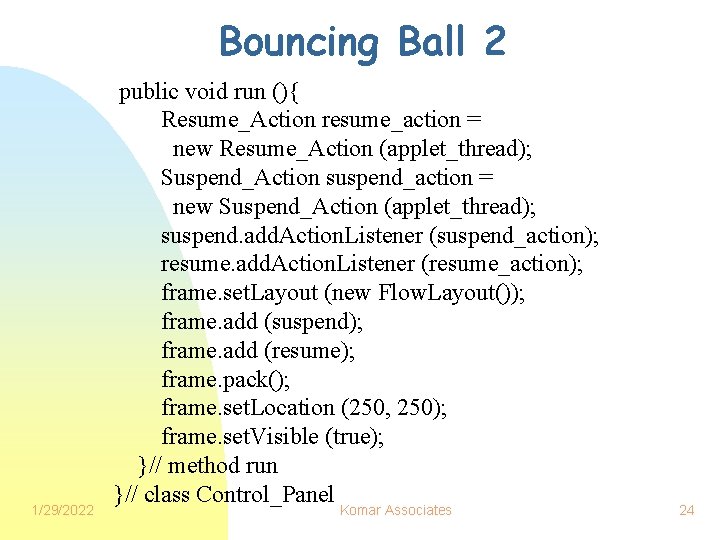
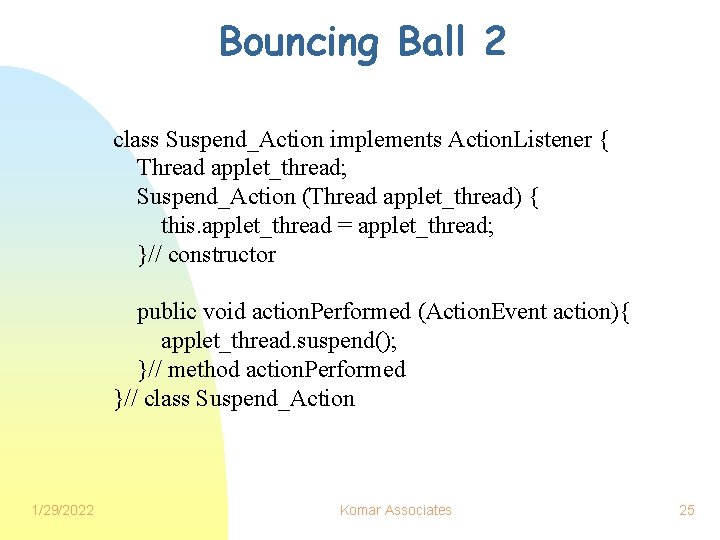
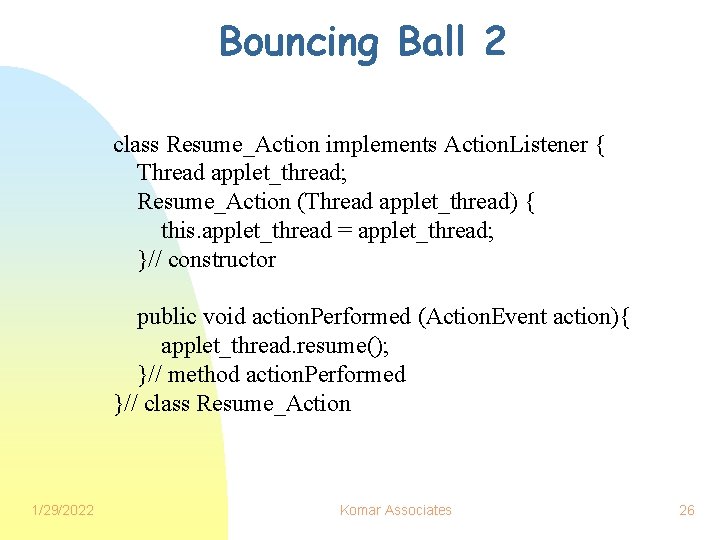
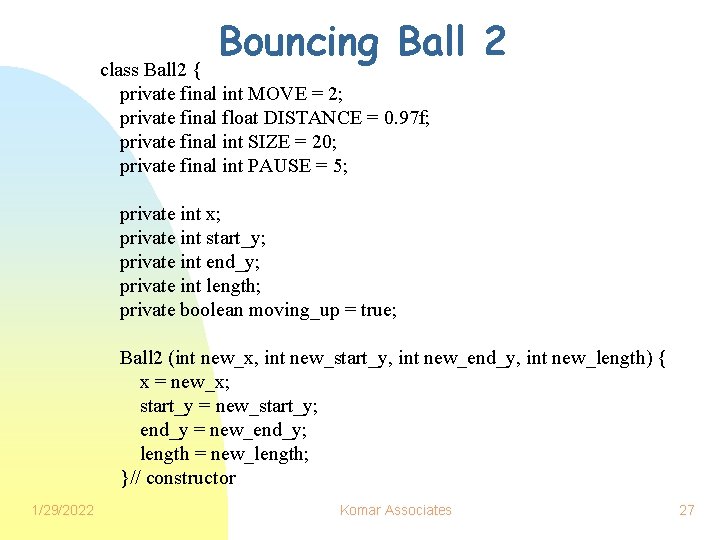
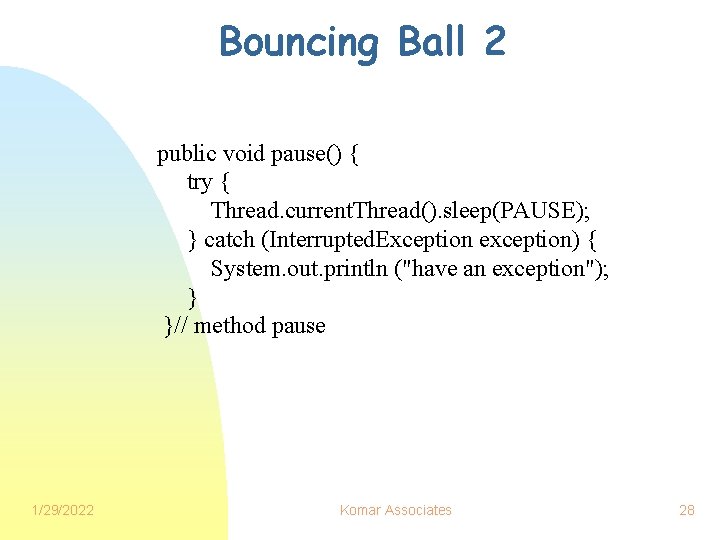
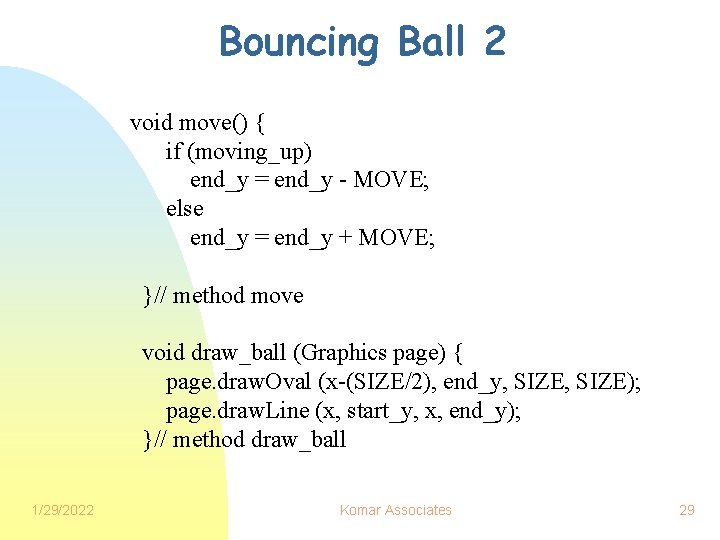
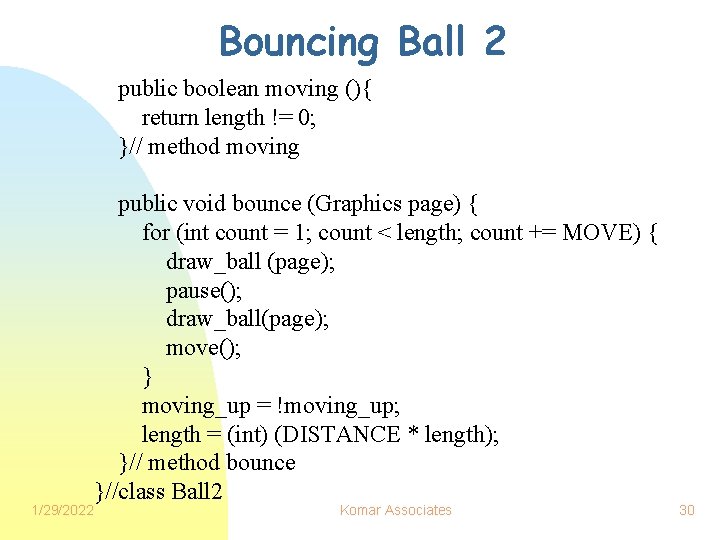
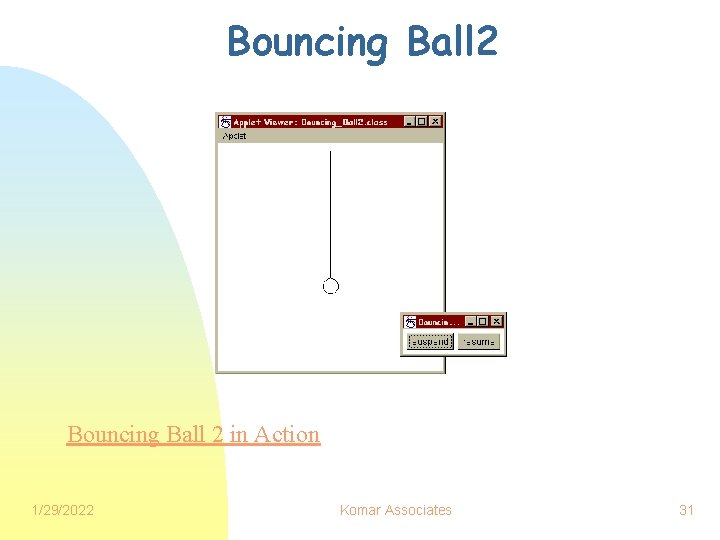
- Slides: 31
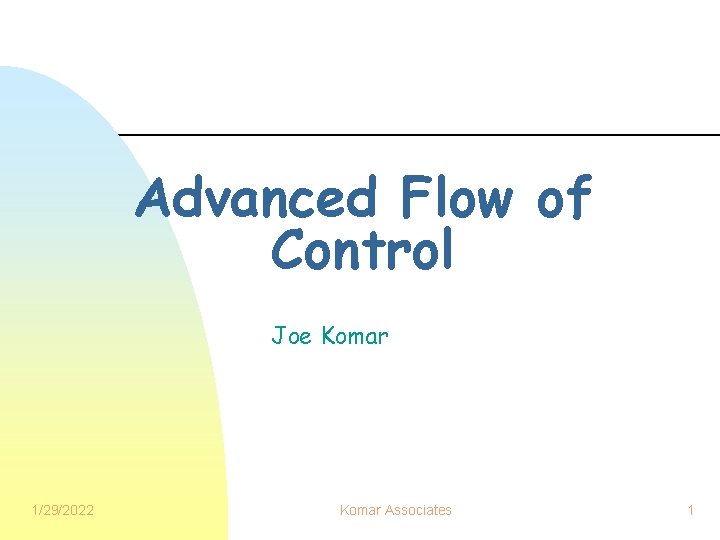
Advanced Flow of Control Joe Komar 1/29/2022 Komar Associates 1
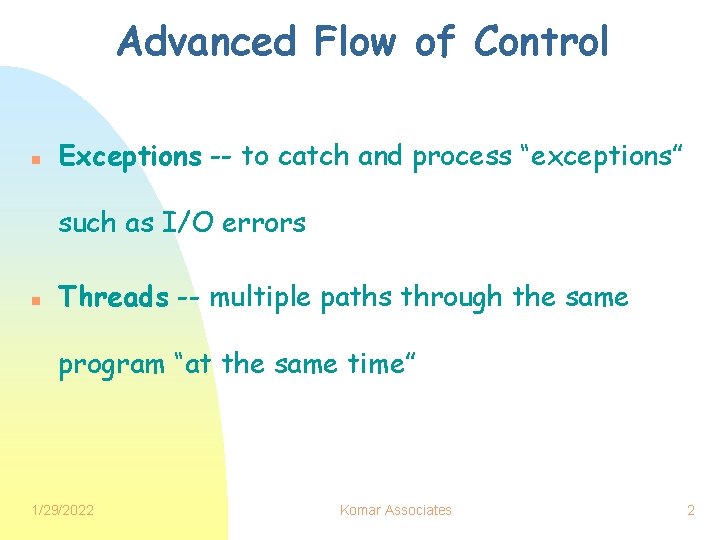
Advanced Flow of Control n Exceptions -- to catch and process “exceptions” such as I/O errors n Threads -- multiple paths through the same program “at the same time” 1/29/2022 Komar Associates 2
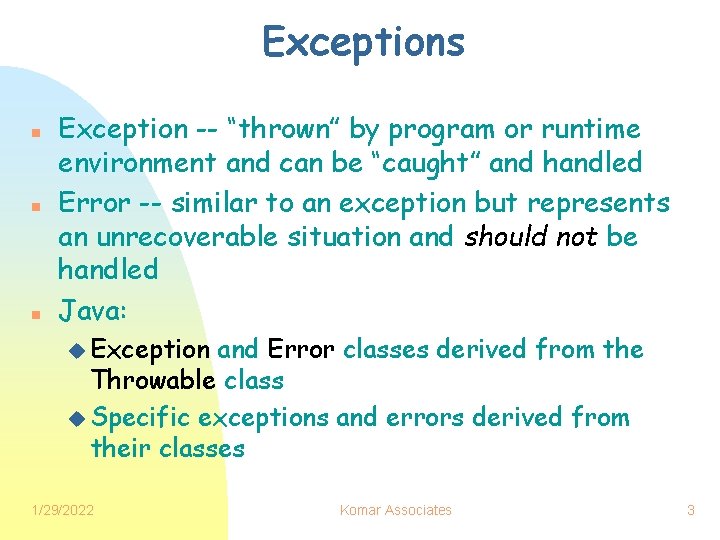
Exceptions n n n Exception -- “thrown” by program or runtime environment and can be “caught” and handled Error -- similar to an exception but represents an unrecoverable situation and should not be handled Java: u Exception and Error classes derived from the Throwable class u Specific exceptions and errors derived from their classes 1/29/2022 Komar Associates 3
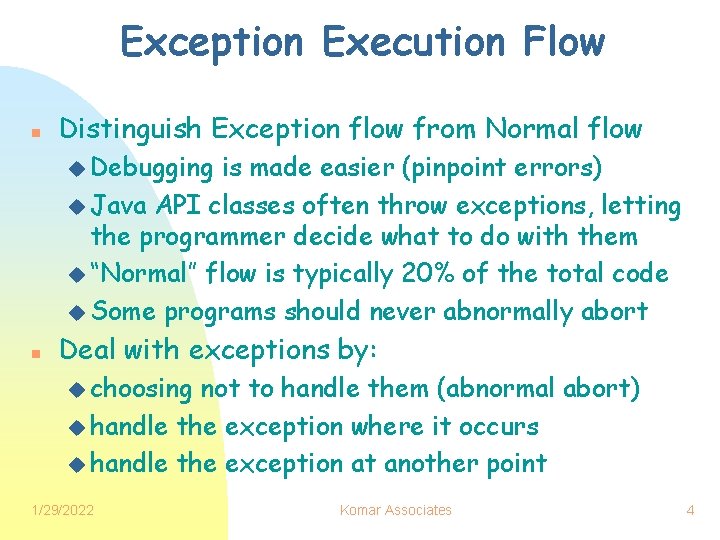
Exception Execution Flow n Distinguish Exception flow from Normal flow u Debugging is made easier (pinpoint errors) u Java API classes often throw exceptions, letting the programmer decide what to do with them u “Normal” flow is typically 20% of the total code u Some programs should never abnormally abort n Deal with exceptions by: u choosing not to handle them (abnormal abort) u handle the exception where it occurs u handle the exception at another point 1/29/2022 Komar Associates 4
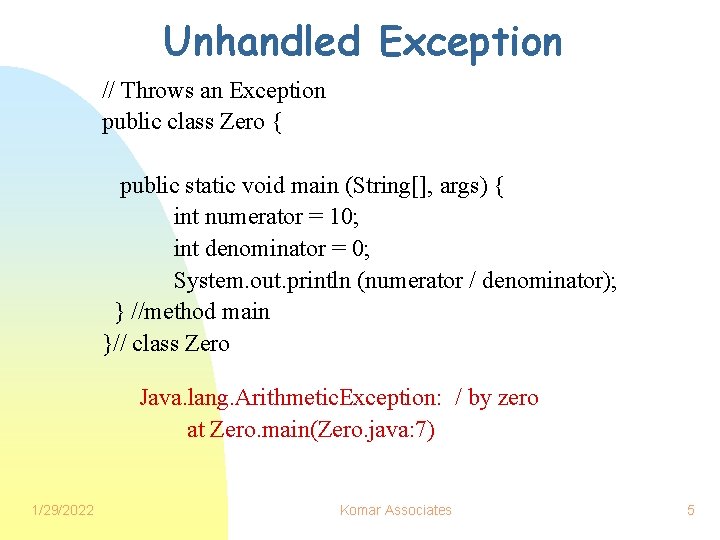
Unhandled Exception // Throws an Exception public class Zero { public static void main (String[], args) { int numerator = 10; int denominator = 0; System. out. println (numerator / denominator); } //method main }// class Zero Java. lang. Arithmetic. Exception: / by zero at Zero. main(Zero. java: 7) 1/29/2022 Komar Associates 5
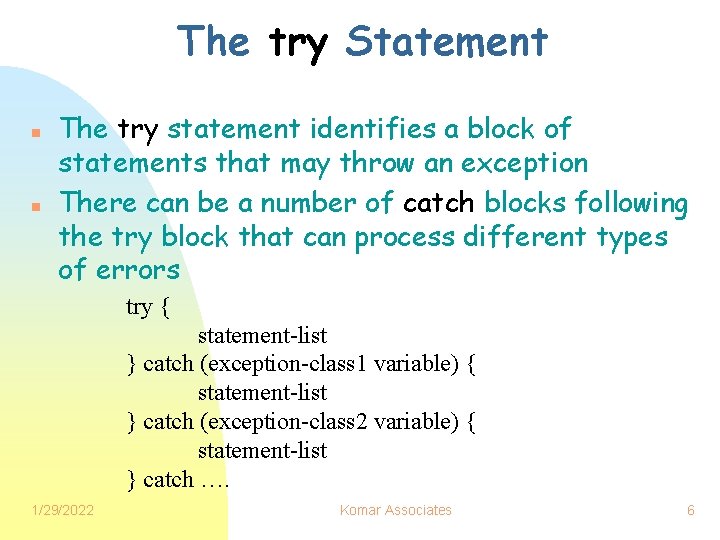
The try Statement n n The try statement identifies a block of statements that may throw an exception There can be a number of catch blocks following the try block that can process different types of errors try { statement-list } catch (exception-class 1 variable) { statement-list } catch (exception-class 2 variable) { statement-list } catch …. 1/29/2022 Komar Associates 6
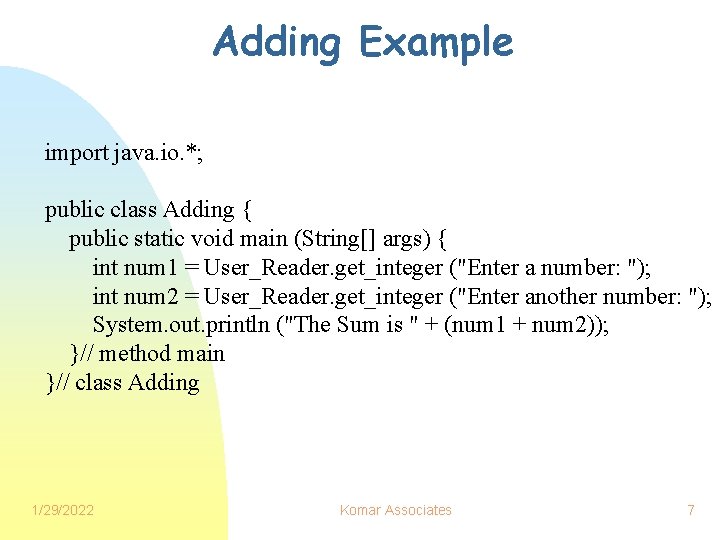
Adding Example import java. io. *; public class Adding { public static void main (String[] args) { int num 1 = User_Reader. get_integer ("Enter a number: "); int num 2 = User_Reader. get_integer ("Enter another number: "); System. out. println ("The Sum is " + (num 1 + num 2)); }// method main }// class Adding 1/29/2022 Komar Associates 7
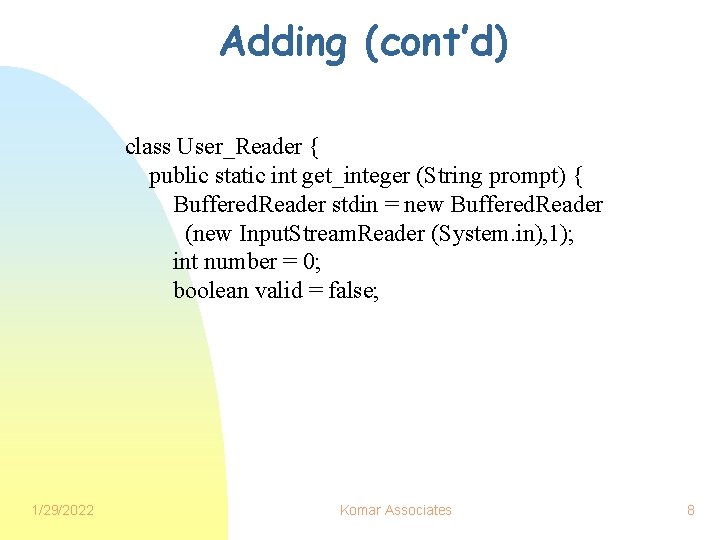
Adding (cont’d) class User_Reader { public static int get_integer (String prompt) { Buffered. Reader stdin = new Buffered. Reader (new Input. Stream. Reader (System. in), 1); int number = 0; boolean valid = false; 1/29/2022 Komar Associates 8
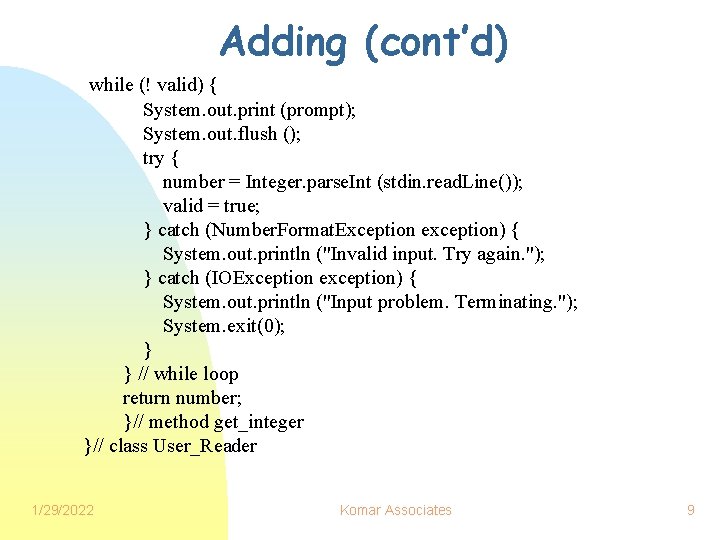
Adding (cont’d) while (! valid) { System. out. print (prompt); System. out. flush (); try { number = Integer. parse. Int (stdin. read. Line()); valid = true; } catch (Number. Format. Exception exception) { System. out. println ("Invalid input. Try again. "); } catch (IOException exception) { System. out. println ("Input problem. Terminating. "); System. exit(0); } } // while loop return number; }// method get_integer }// class User_Reader 1/29/2022 Komar Associates 9
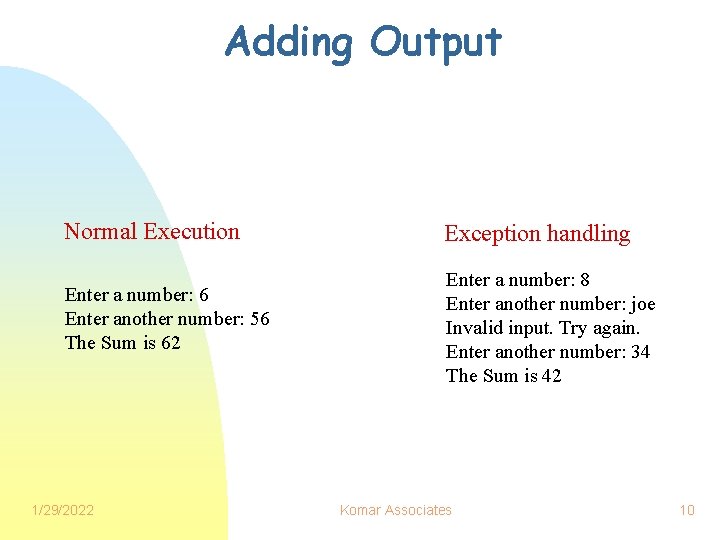
Adding Output Normal Execution Exception handling Enter a number: 6 Enter another number: 56 The Sum is 62 Enter a number: 8 Enter another number: joe Invalid input. Try again. Enter another number: 34 The Sum is 42 1/29/2022 Komar Associates 10
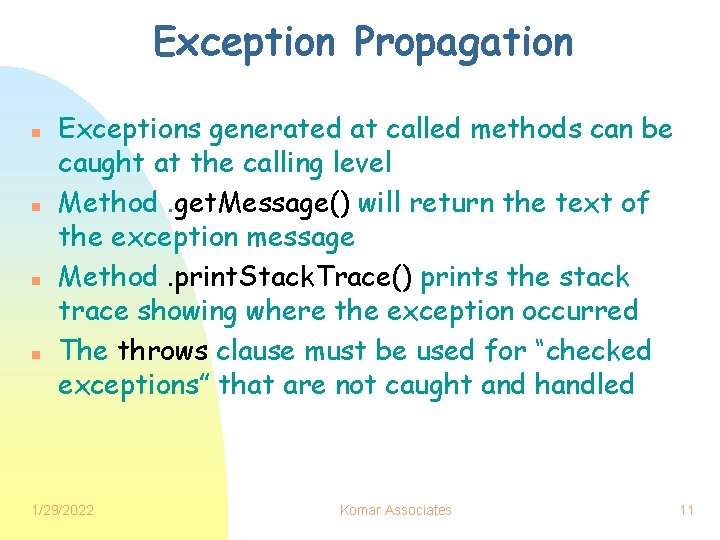
Exception Propagation n n Exceptions generated at called methods can be caught at the calling level Method. get. Message() will return the text of the exception message Method. print. Stack. Trace() prints the stack trace showing where the exception occurred The throws clause must be used for “checked exceptions” that are not caught and handled 1/29/2022 Komar Associates 11
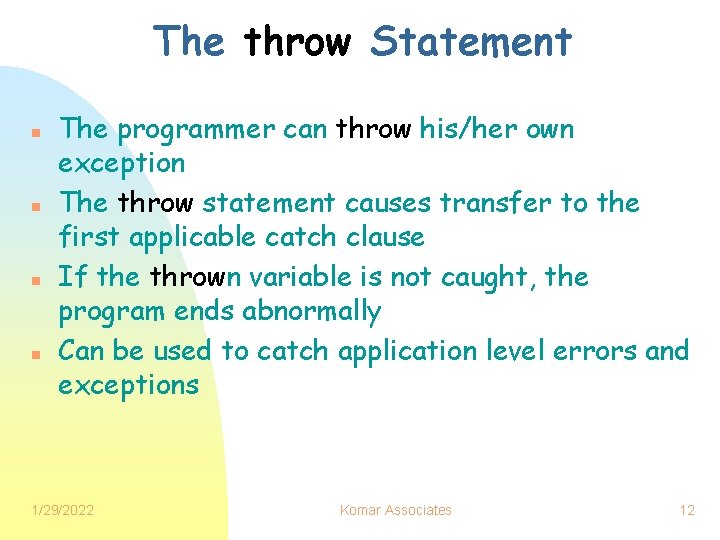
The throw Statement n n The programmer can throw his/her own exception The throw statement causes transfer to the first applicable catch clause If the thrown variable is not caught, the program ends abnormally Can be used to catch application level errors and exceptions 1/29/2022 Komar Associates 12
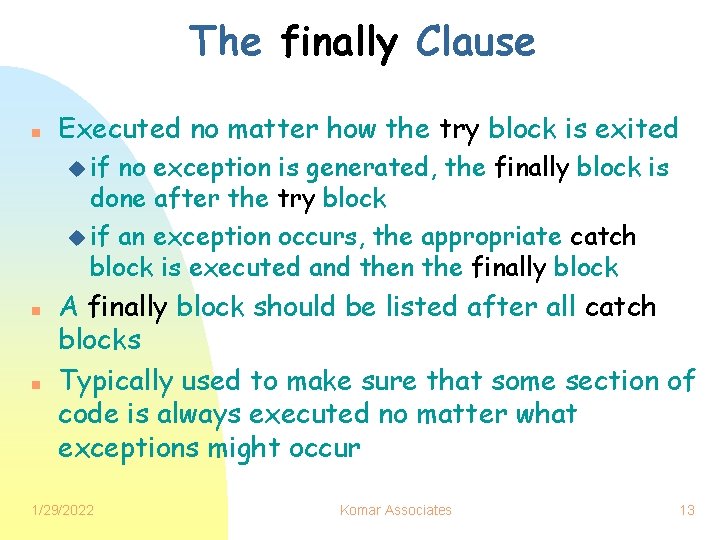
The finally Clause n Executed no matter how the try block is exited u if no exception is generated, the finally block is done after the try block u if an exception occurs, the appropriate catch block is executed and then the finally block n n A finally block should be listed after all catch blocks Typically used to make sure that some section of code is always executed no matter what exceptions might occur 1/29/2022 Komar Associates 13
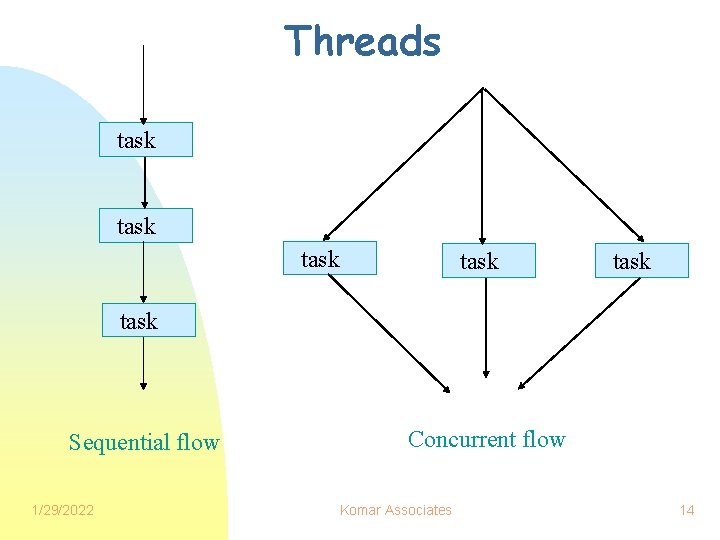
Threads task task Sequential flow 1/29/2022 Concurrent flow Komar Associates 14
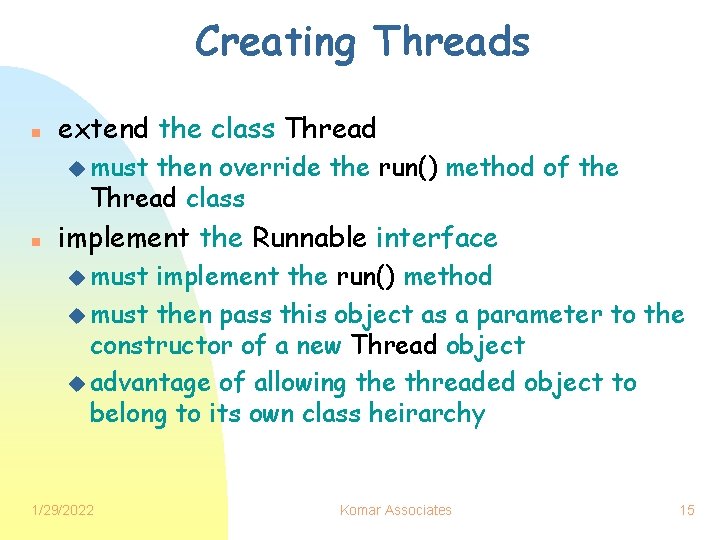
Creating Threads n extend the class Thread u must then override the run() method of the Thread class n implement the Runnable interface u must implement the run() method u must then pass this object as a parameter to the constructor of a new Thread object u advantage of allowing the threaded object to belong to its own class heirarchy 1/29/2022 Komar Associates 15
![Simultaneous public class Simultaneous public static void main Stringargs Soda one Simultaneous public class Simultaneous { public static void main (String[]args) { Soda one =](https://slidetodoc.com/presentation_image_h2/95b86dd13c880c7d4558ff010de94a89/image-16.jpg)
Simultaneous public class Simultaneous { public static void main (String[]args) { Soda one = new Soda ("Coke"); Soda two = new Soda ("Pepsi"); Soda three = new Soda ("Seven Up"); one. start(); two. start(); three. start(); try { System. in. read(); } catch (Exception e) {} }// method main }// class Simultaneous 1/29/2022 Komar Associates 16
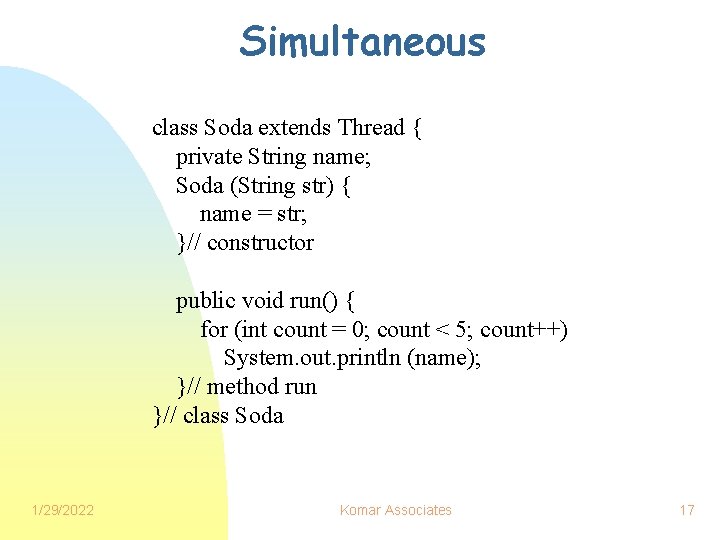
Simultaneous class Soda extends Thread { private String name; Soda (String str) { name = str; }// constructor public void run() { for (int count = 0; count < 5; count++) System. out. println (name); }// method run }// class Soda 1/29/2022 Komar Associates 17
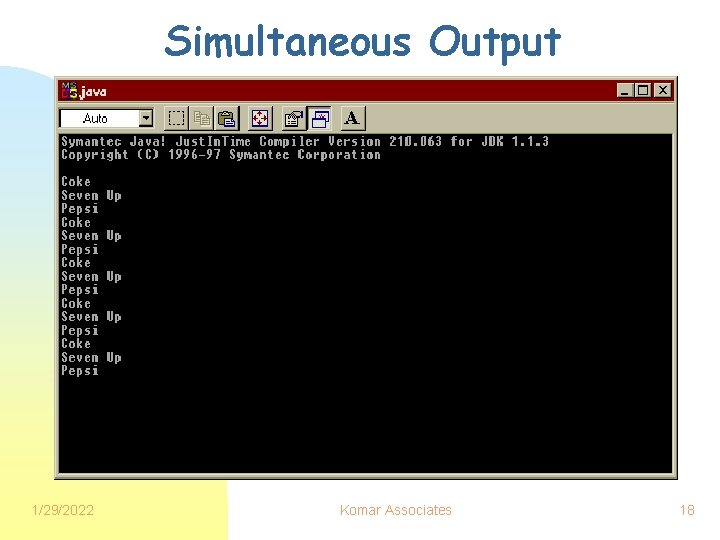
Simultaneous Output 1/29/2022 Komar Associates 18
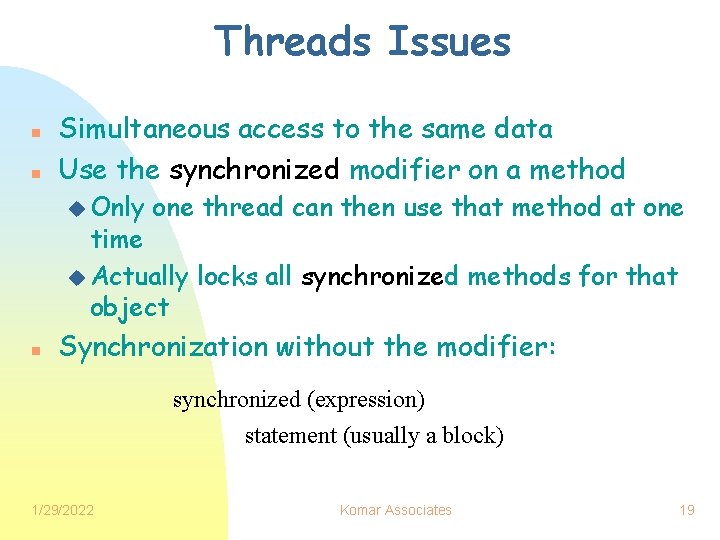
Threads Issues n n Simultaneous access to the same data Use the synchronized modifier on a method u Only one thread can then use that method at one time u Actually locks all synchronized methods for that object n Synchronization without the modifier: synchronized (expression) statement (usually a block) 1/29/2022 Komar Associates 19
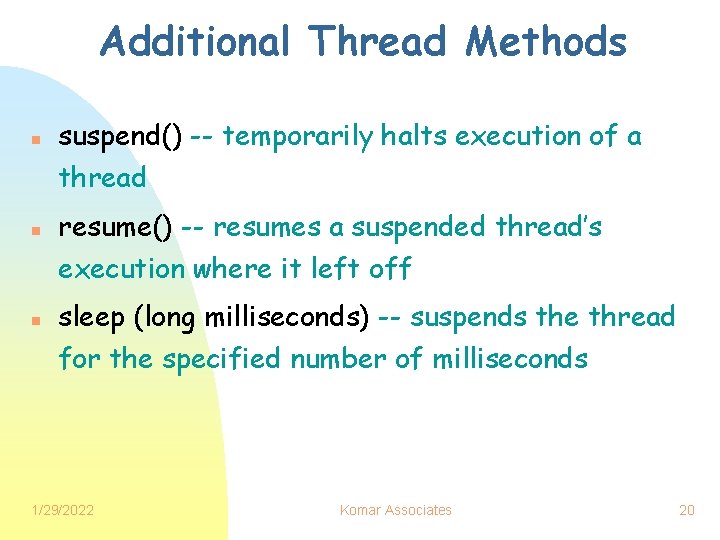
Additional Thread Methods n suspend() -- temporarily halts execution of a thread n n resume() -- resumes a suspended thread’s execution where it left off sleep (long milliseconds) -- suspends the thread for the specified number of milliseconds 1/29/2022 Komar Associates 20
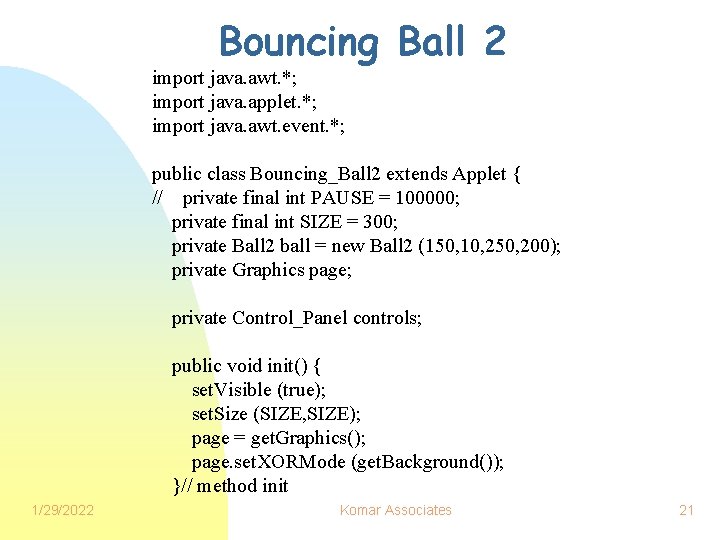
Bouncing Ball 2 import java. awt. *; import java. applet. *; import java. awt. event. *; public class Bouncing_Ball 2 extends Applet { // private final int PAUSE = 100000; private final int SIZE = 300; private Ball 2 ball = new Ball 2 (150, 10, 250, 200); private Graphics page; private Control_Panel controls; public void init() { set. Visible (true); set. Size (SIZE, SIZE); page = get. Graphics(); page. set. XORMode (get. Background()); }// method init 1/29/2022 Komar Associates 21
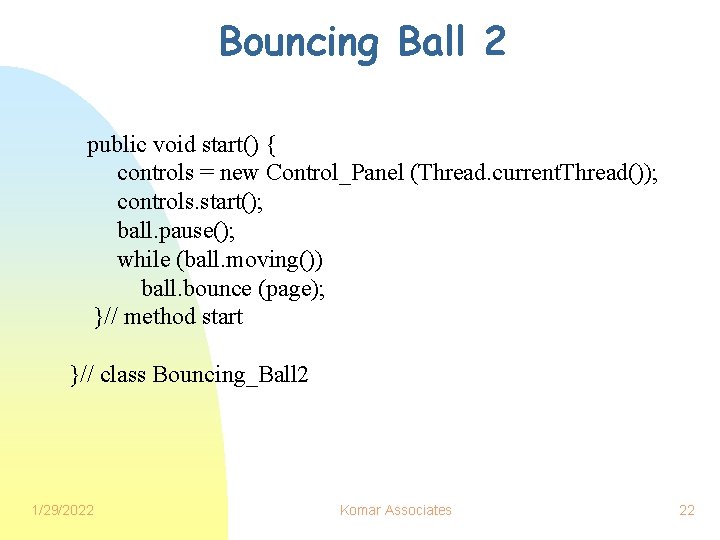
Bouncing Ball 2 public void start() { controls = new Control_Panel (Thread. current. Thread()); controls. start(); ball. pause(); while (ball. moving()) ball. bounce (page); }// method start }// class Bouncing_Ball 2 1/29/2022 Komar Associates 22
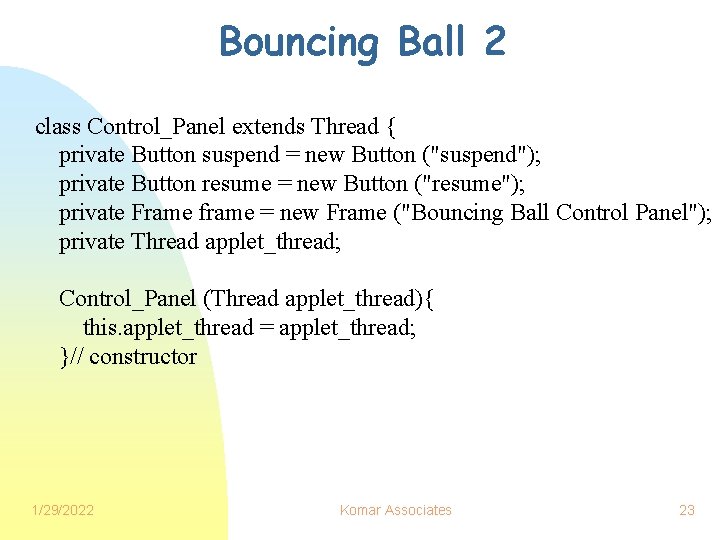
Bouncing Ball 2 class Control_Panel extends Thread { private Button suspend = new Button ("suspend"); private Button resume = new Button ("resume"); private Frame frame = new Frame ("Bouncing Ball Control Panel"); private Thread applet_thread; Control_Panel (Thread applet_thread){ this. applet_thread = applet_thread; }// constructor 1/29/2022 Komar Associates 23
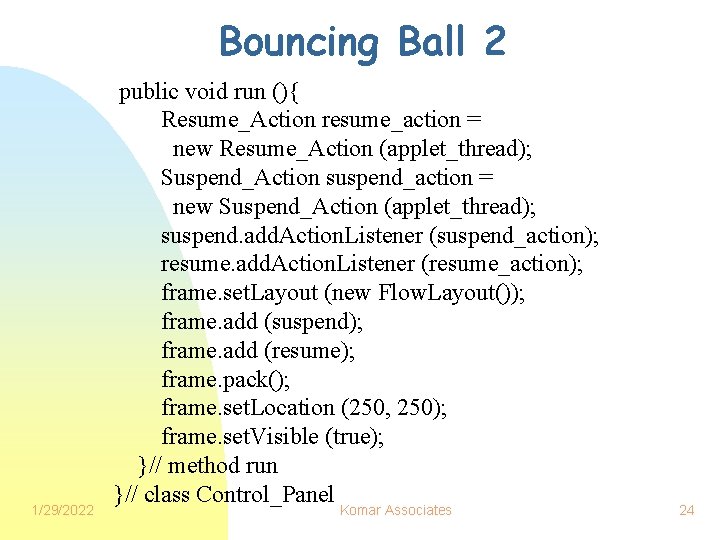
Bouncing Ball 2 1/29/2022 public void run (){ Resume_Action resume_action = new Resume_Action (applet_thread); Suspend_Action suspend_action = new Suspend_Action (applet_thread); suspend. add. Action. Listener (suspend_action); resume. add. Action. Listener (resume_action); frame. set. Layout (new Flow. Layout()); frame. add (suspend); frame. add (resume); frame. pack(); frame. set. Location (250, 250); frame. set. Visible (true); }// method run }// class Control_Panel Komar Associates 24
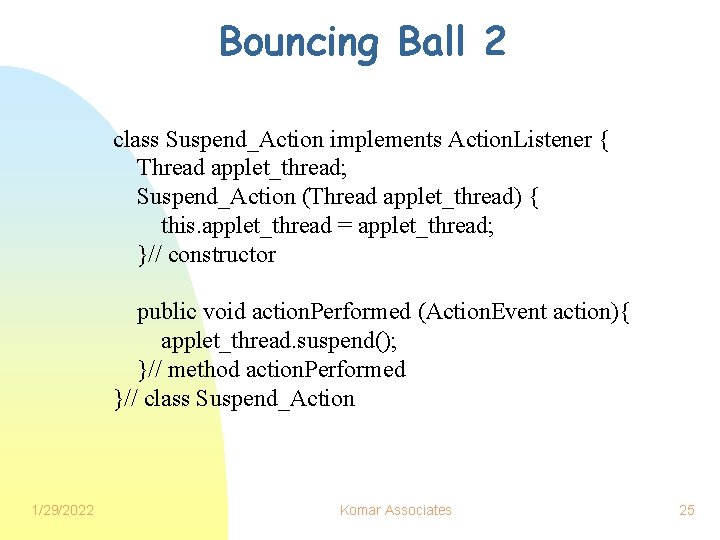
Bouncing Ball 2 class Suspend_Action implements Action. Listener { Thread applet_thread; Suspend_Action (Thread applet_thread) { this. applet_thread = applet_thread; }// constructor public void action. Performed (Action. Event action){ applet_thread. suspend(); }// method action. Performed }// class Suspend_Action 1/29/2022 Komar Associates 25
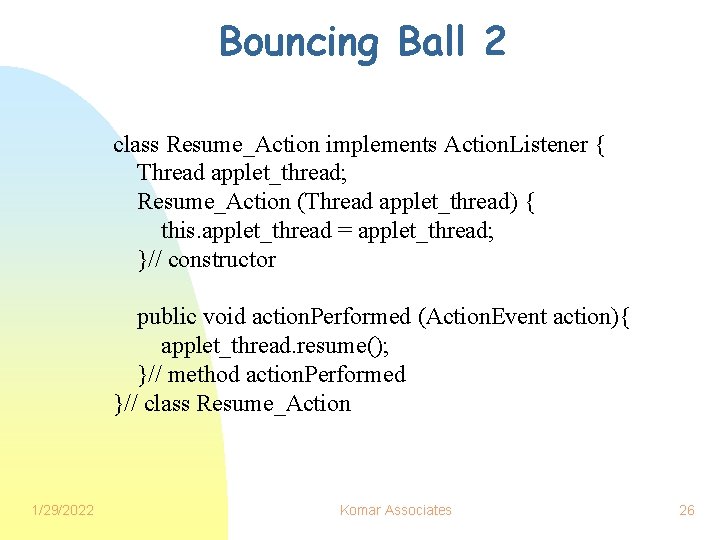
Bouncing Ball 2 class Resume_Action implements Action. Listener { Thread applet_thread; Resume_Action (Thread applet_thread) { this. applet_thread = applet_thread; }// constructor public void action. Performed (Action. Event action){ applet_thread. resume(); }// method action. Performed }// class Resume_Action 1/29/2022 Komar Associates 26
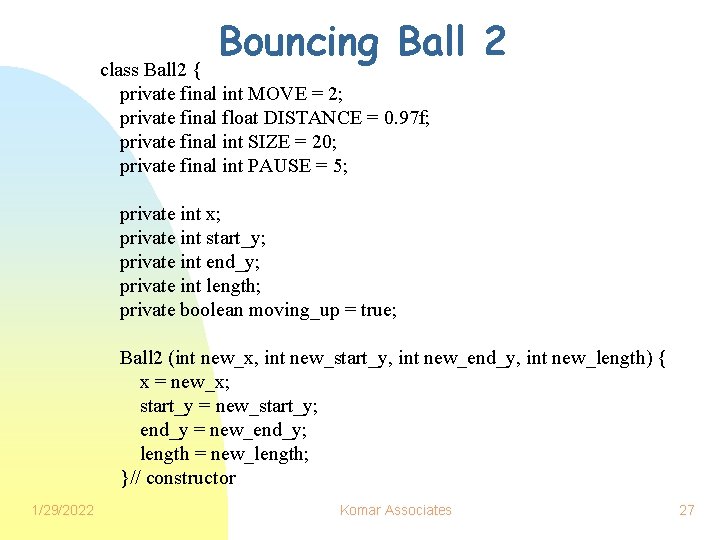
Bouncing Ball 2 class Ball 2 { private final int MOVE = 2; private final float DISTANCE = 0. 97 f; private final int SIZE = 20; private final int PAUSE = 5; private int x; private int start_y; private int end_y; private int length; private boolean moving_up = true; Ball 2 (int new_x, int new_start_y, int new_end_y, int new_length) { x = new_x; start_y = new_start_y; end_y = new_end_y; length = new_length; }// constructor 1/29/2022 Komar Associates 27
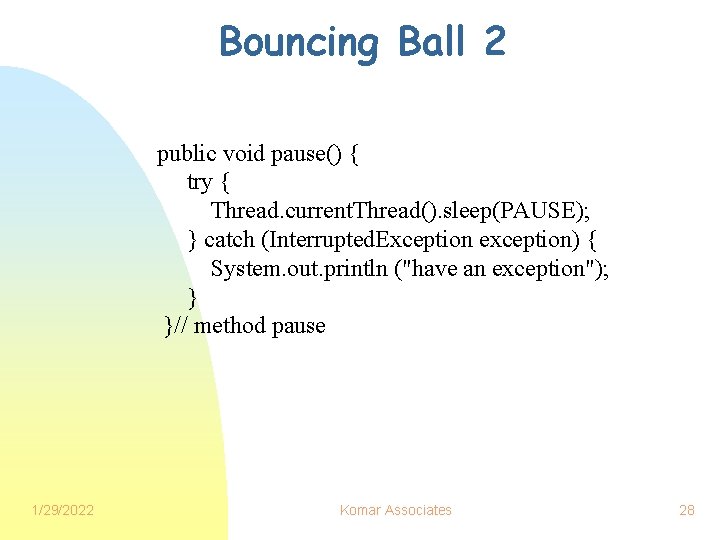
Bouncing Ball 2 public void pause() { try { Thread. current. Thread(). sleep(PAUSE); } catch (Interrupted. Exception exception) { System. out. println ("have an exception"); } }// method pause 1/29/2022 Komar Associates 28
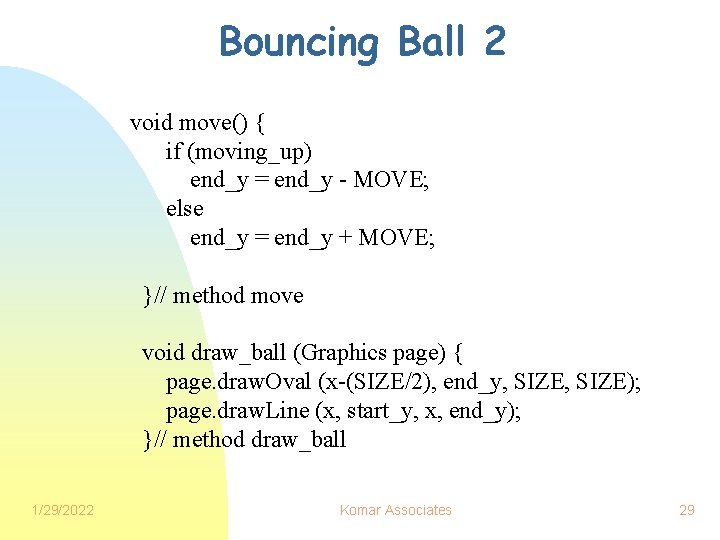
Bouncing Ball 2 void move() { if (moving_up) end_y = end_y - MOVE; else end_y = end_y + MOVE; }// method move void draw_ball (Graphics page) { page. draw. Oval (x-(SIZE/2), end_y, SIZE); page. draw. Line (x, start_y, x, end_y); }// method draw_ball 1/29/2022 Komar Associates 29
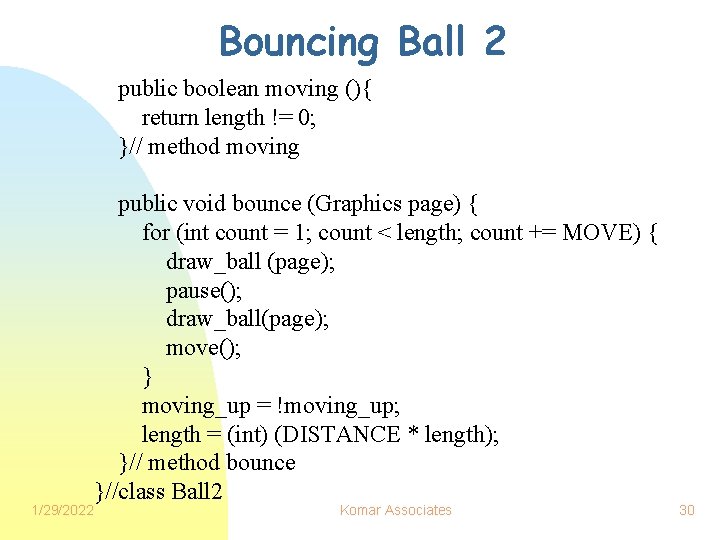
Bouncing Ball 2 public boolean moving (){ return length != 0; }// method moving 1/29/2022 public void bounce (Graphics page) { for (int count = 1; count < length; count += MOVE) { draw_ball (page); pause(); draw_ball(page); move(); } moving_up = !moving_up; length = (int) (DISTANCE * length); }// method bounce }//class Ball 2 Komar Associates 30
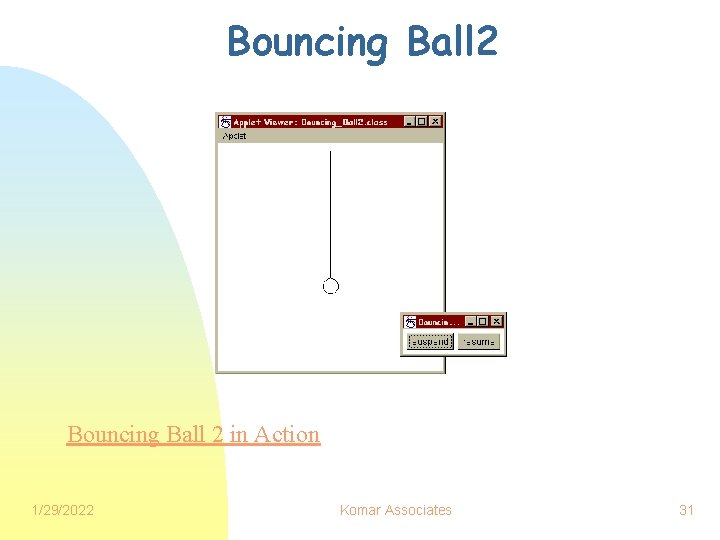
Bouncing Ball 2 in Action 1/29/2022 Komar Associates 31
Komar and melamid
Budowa pszczoly
Meir komar
Data flow vs control flow
Control flow and data flow computers
Transaction flow graph
Stock control e flow control
Control flow errors
Hijacking attacks
Advanced vehicle control
Richa jain md
Venturi mask vs face mask
Venturi mask flow rate
Lumina flow
Internal vs external flow
Ecological succession
Oikos meaning
Transform flow and transaction flow
Transform flow and transaction flow
Rotational irrotational flow
Internal and external flow
Cheese making flow chart
Symbol of flow control valve
Flow control udp
Flow control instructions in assembly language
Seoul national university events
Repl capture/apply: memory
Material flow control
Control flow graph for bubble sort
Relief valve
Flow graph unity
Database security definition