308 203 A Introduction to Computing II Lecture
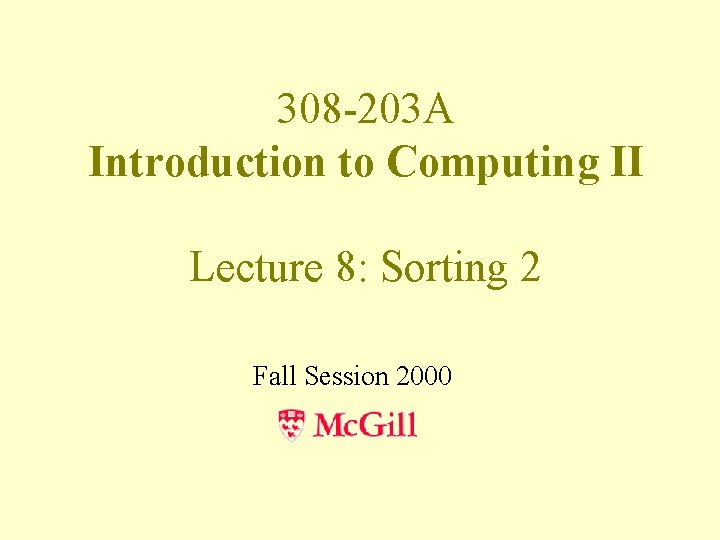
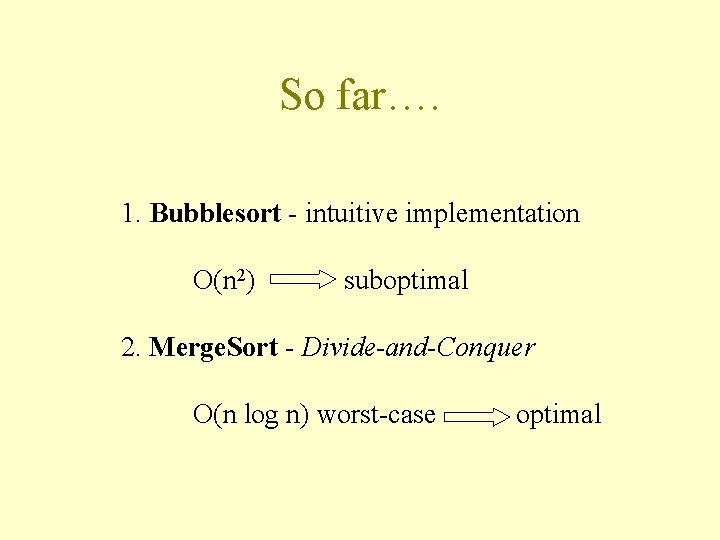
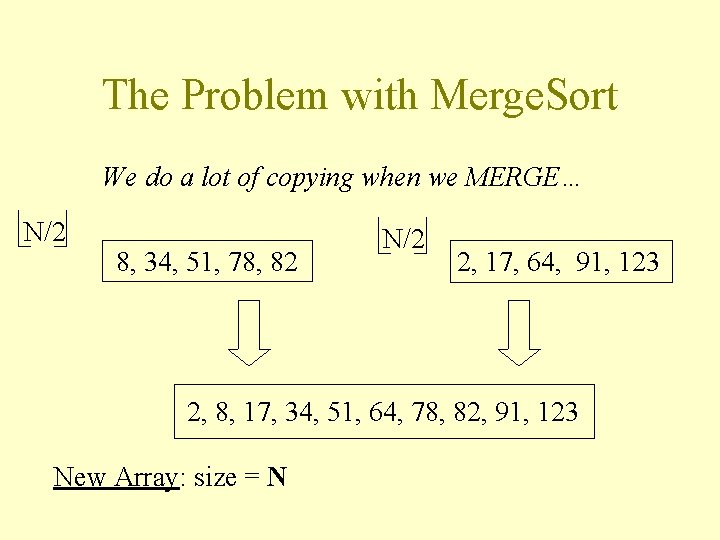
![In Java, for example. . . int [] merge(int [] a, int [] b) In Java, for example. . . int [] merge(int [] a, int [] b)](https://slidetodoc.com/presentation_image_h2/a921f8b850d9cfb52b88c6a6db38ffee/image-4.jpg)
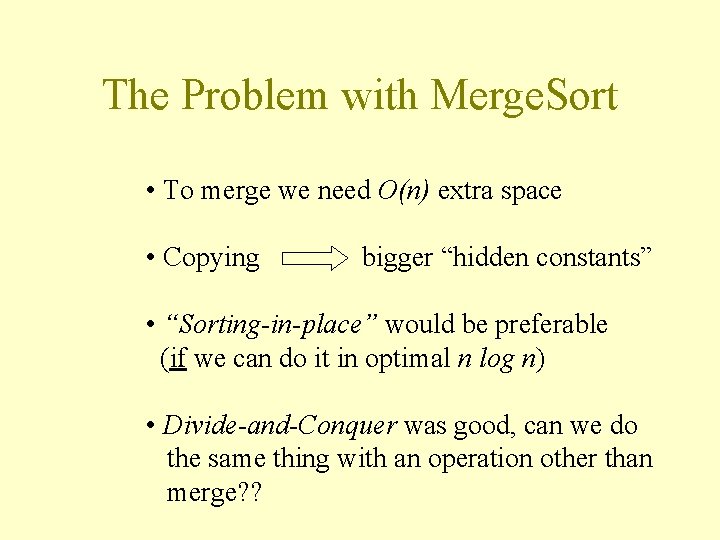
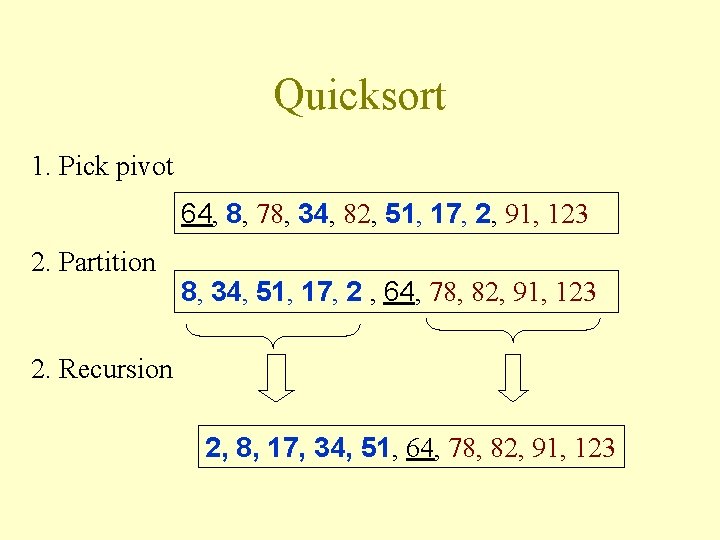
![Quicksort(int [] A, int start, int end) { int pivot = A[0]; int midpoint Quicksort(int [] A, int start, int end) { int pivot = A[0]; int midpoint](https://slidetodoc.com/presentation_image_h2/a921f8b850d9cfb52b88c6a6db38ffee/image-7.jpg)
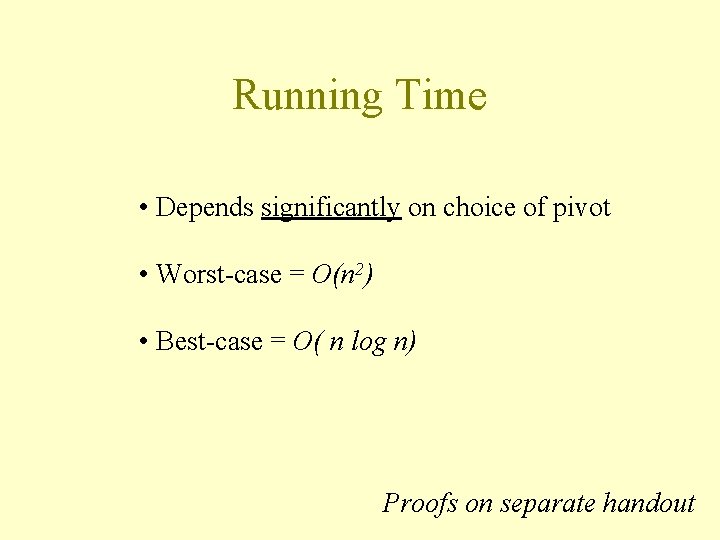
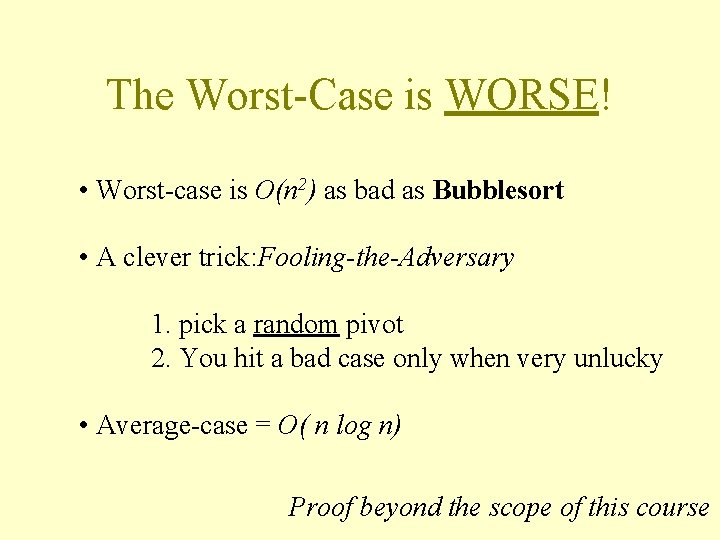
![Randomized Quicksort(int [] A, int start, int end) { int pivot = A[ random. Randomized Quicksort(int [] A, int start, int end) { int pivot = A[ random.](https://slidetodoc.com/presentation_image_h2/a921f8b850d9cfb52b88c6a6db38ffee/image-10.jpg)
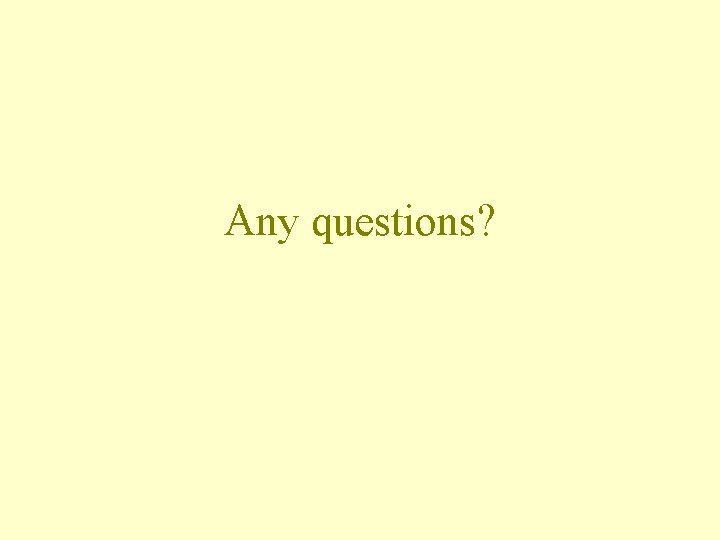
- Slides: 11
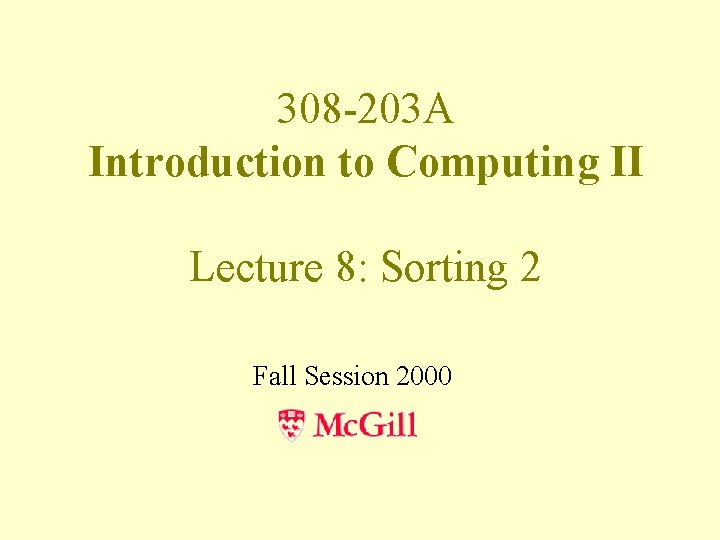
308 -203 A Introduction to Computing II Lecture 8: Sorting 2 Fall Session 2000
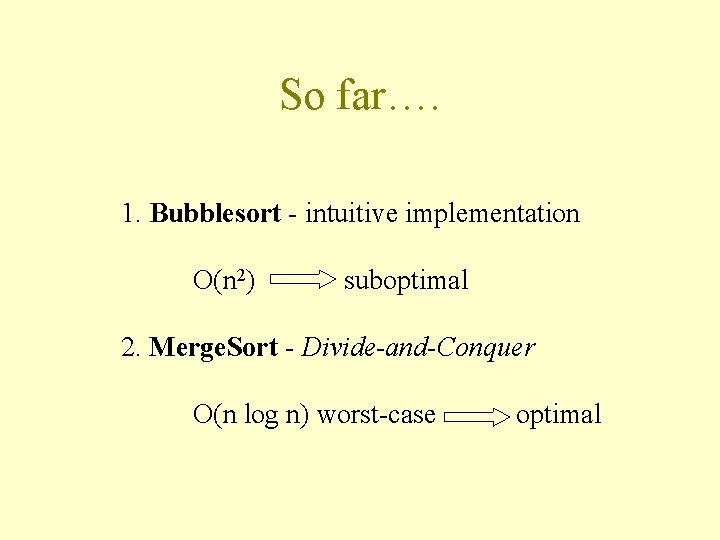
So far…. 1. Bubblesort - intuitive implementation O(n 2) suboptimal 2. Merge. Sort - Divide-and-Conquer O(n log n) worst-case optimal
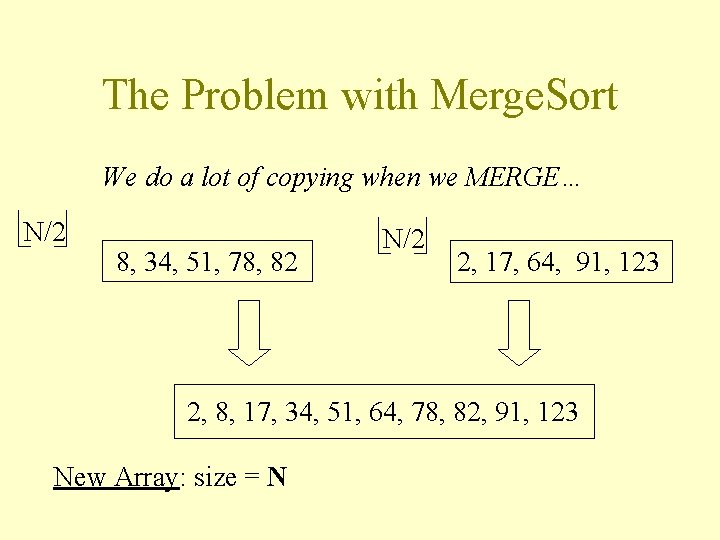
The Problem with Merge. Sort We do a lot of copying when we MERGE… N/2 8, 34, 51, 78, 82 N/2 2, 17, 64, 91, 123 2, 8, 17, 34, 51, 64, 78, 82, 91, 123 New Array: size = N
![In Java for example int mergeint a int b In Java, for example. . . int [] merge(int [] a, int [] b)](https://slidetodoc.com/presentation_image_h2/a921f8b850d9cfb52b88c6a6db38ffee/image-4.jpg)
In Java, for example. . . int [] merge(int [] a, int [] b) { int [] c = new int [a. length + b. length]; // merge a and b into c …. return c; }
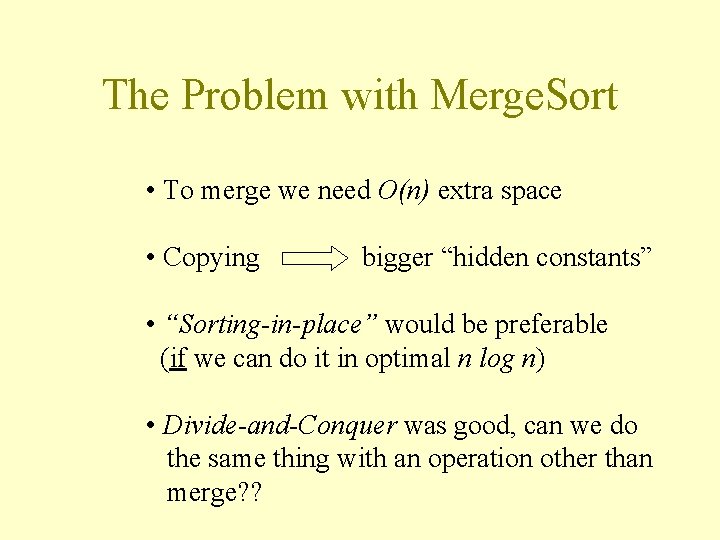
The Problem with Merge. Sort • To merge we need O(n) extra space • Copying bigger “hidden constants” • “Sorting-in-place” would be preferable (if we can do it in optimal n log n) • Divide-and-Conquer was good, can we do the same thing with an operation other than merge? ?
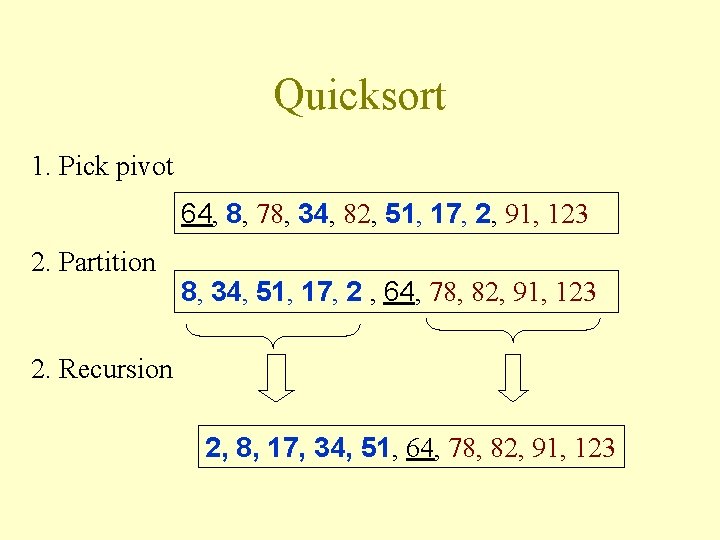
Quicksort 1. Pick pivot 64, 8, 78, 34, 82, 51, 17, 2, 91, 123 2. Partition 8, 34, 51, 17, 2 , 64, 78, 82, 91, 123 2. Recursion 2, 8, 17, 34, 51, 64, 78, 82, 91, 123
![Quicksortint A int start int end int pivot A0 int midpoint Quicksort(int [] A, int start, int end) { int pivot = A[0]; int midpoint](https://slidetodoc.com/presentation_image_h2/a921f8b850d9cfb52b88c6a6db38ffee/image-7.jpg)
Quicksort(int [] A, int start, int end) { int pivot = A[0]; int midpoint = Partition(pivot, A); Quicksort(A, start, midpoint); Quicksort(A, midpoint+1, end); }
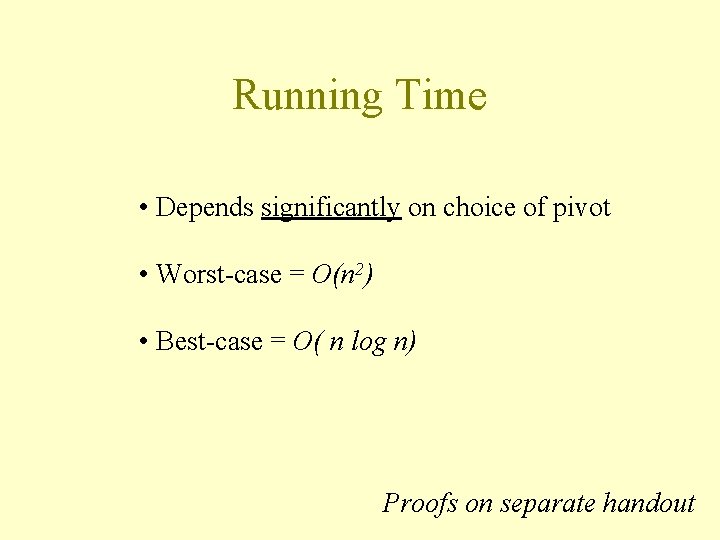
Running Time • Depends significantly on choice of pivot • Worst-case = O(n 2) • Best-case = O( n log n) Proofs on separate handout
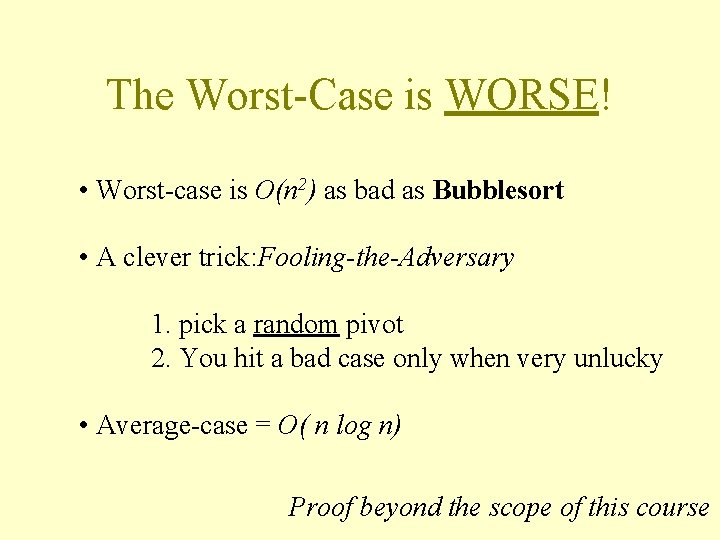
The Worst-Case is WORSE! • Worst-case is O(n 2) as bad as Bubblesort • A clever trick: Fooling-the-Adversary 1. pick a random pivot 2. You hit a bad case only when very unlucky • Average-case = O( n log n) Proof beyond the scope of this course
![Randomized Quicksortint A int start int end int pivot A random Randomized Quicksort(int [] A, int start, int end) { int pivot = A[ random.](https://slidetodoc.com/presentation_image_h2/a921f8b850d9cfb52b88c6a6db38ffee/image-10.jpg)
Randomized Quicksort(int [] A, int start, int end) { int pivot = A[ random. Int(start, end) ]; int midpoint = partition(pivot, A); Quicksort(A, start, midpoint); Quicksort(A, midpoint+1, end); }
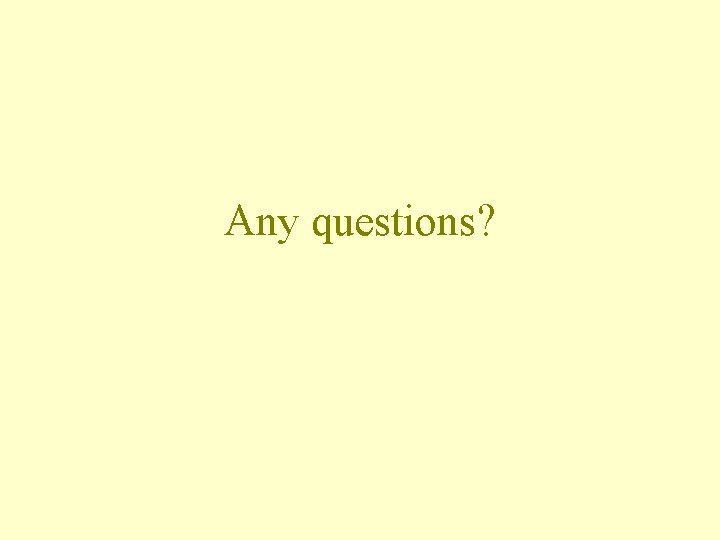
Any questions?