14 1 ch 1401 cpp include iostream using
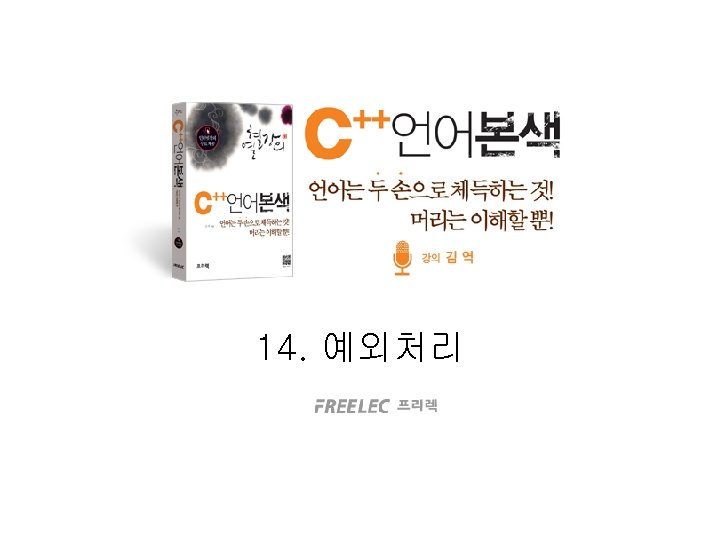
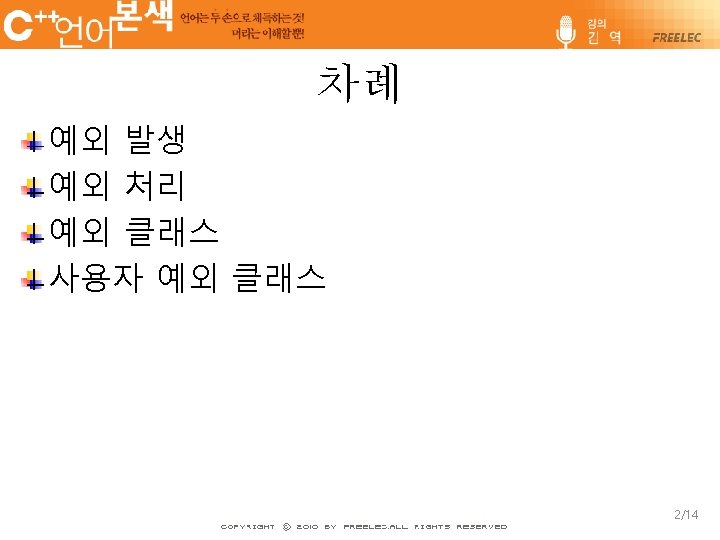
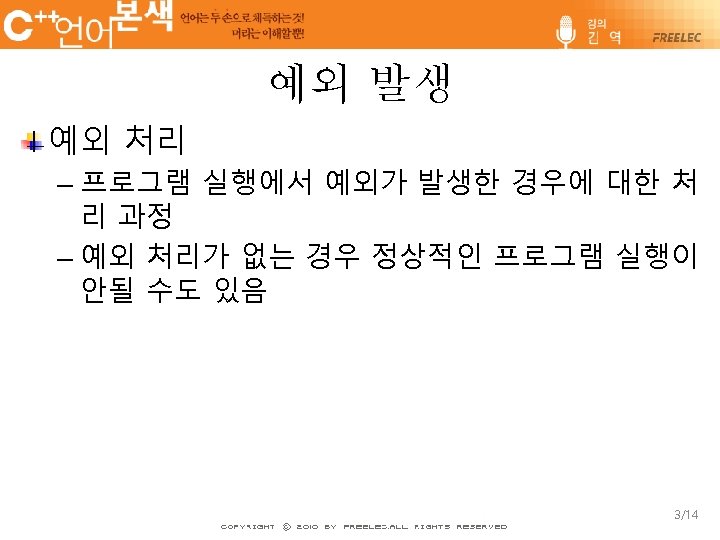
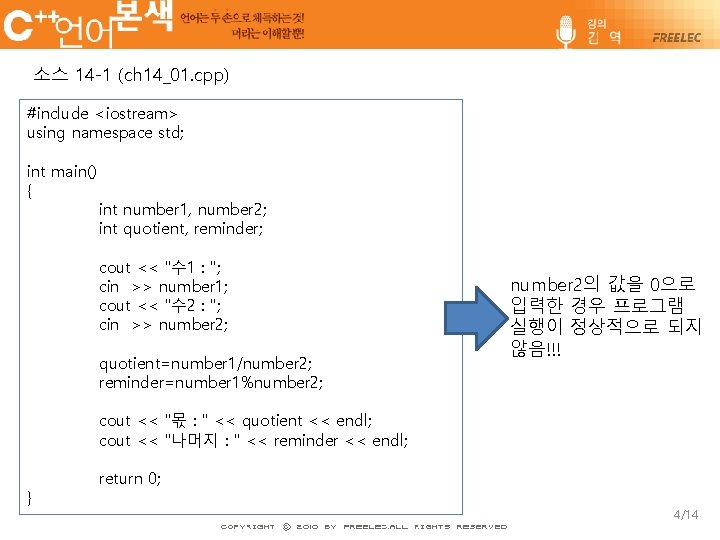
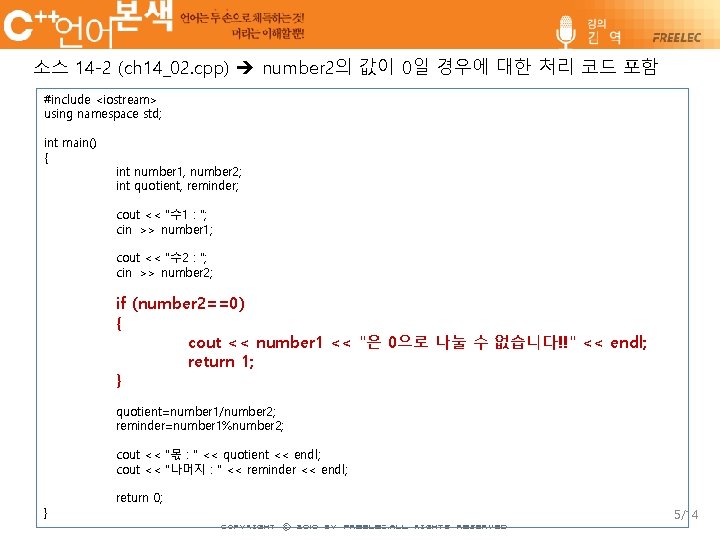
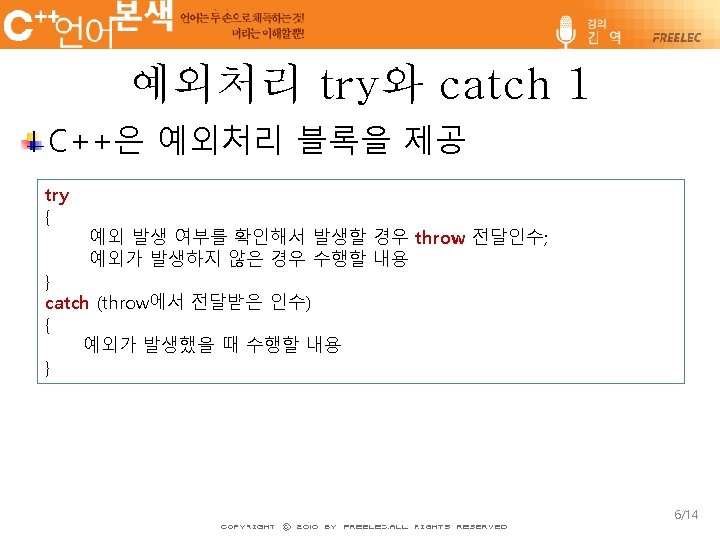
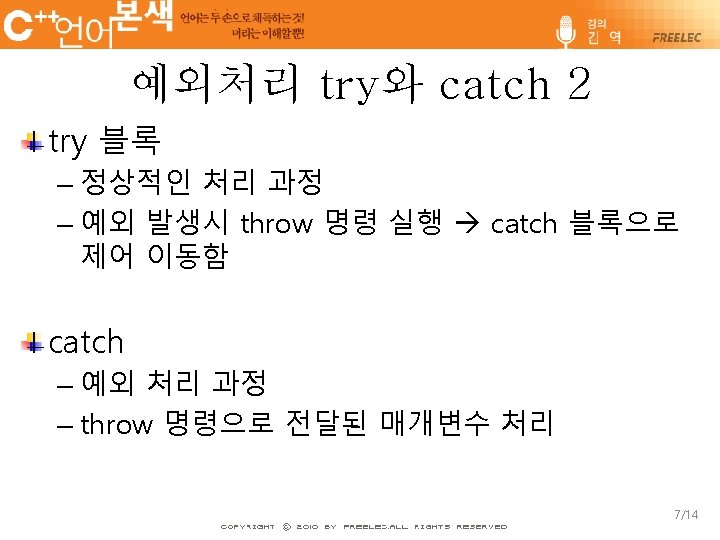
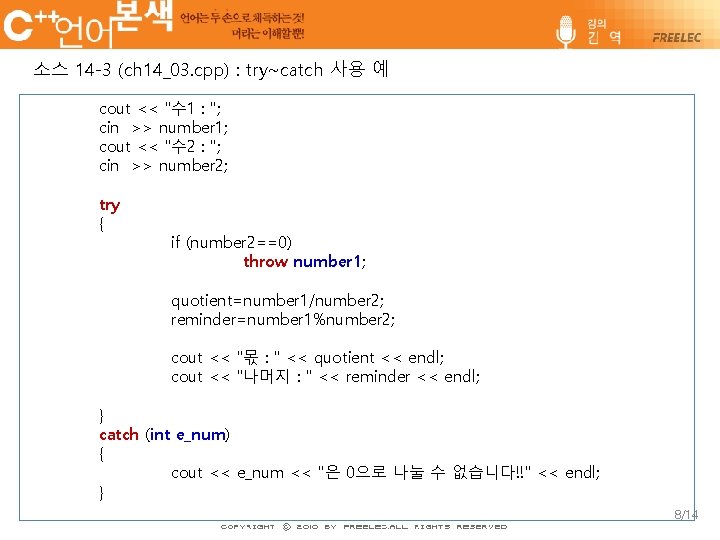
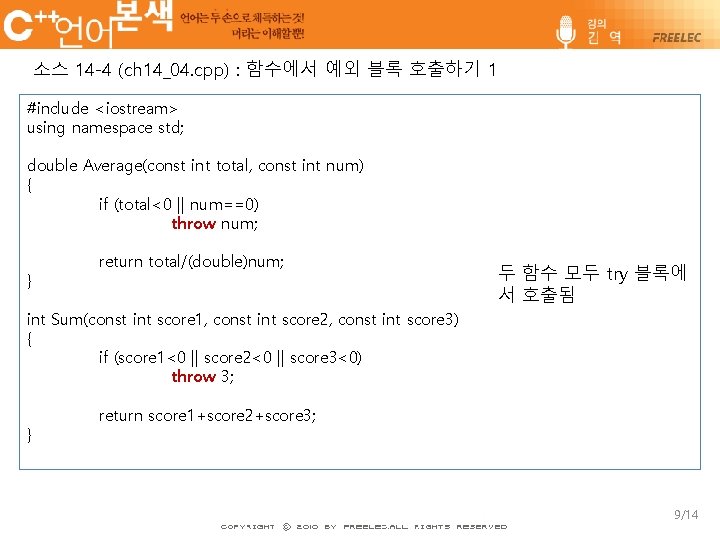
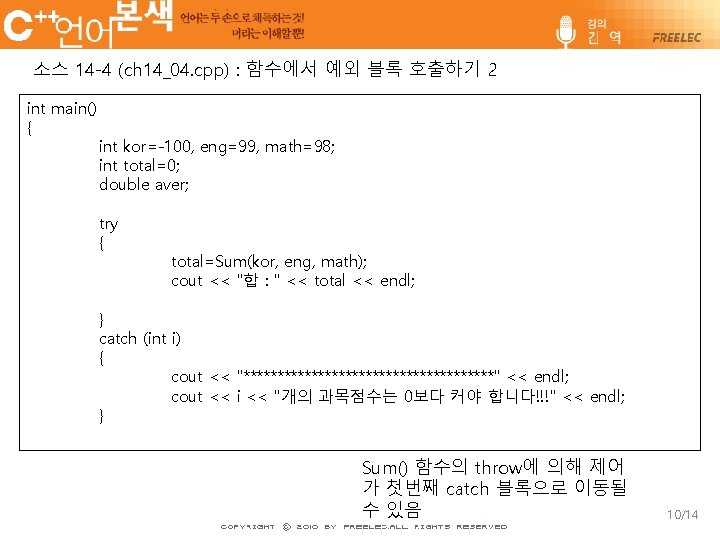
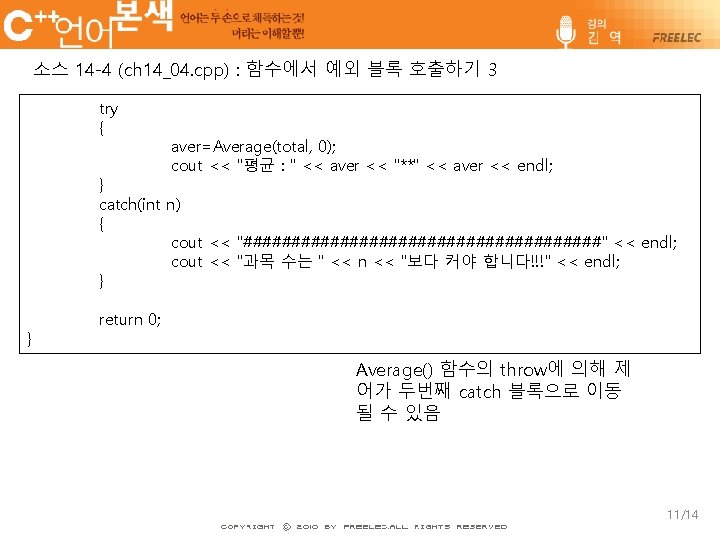
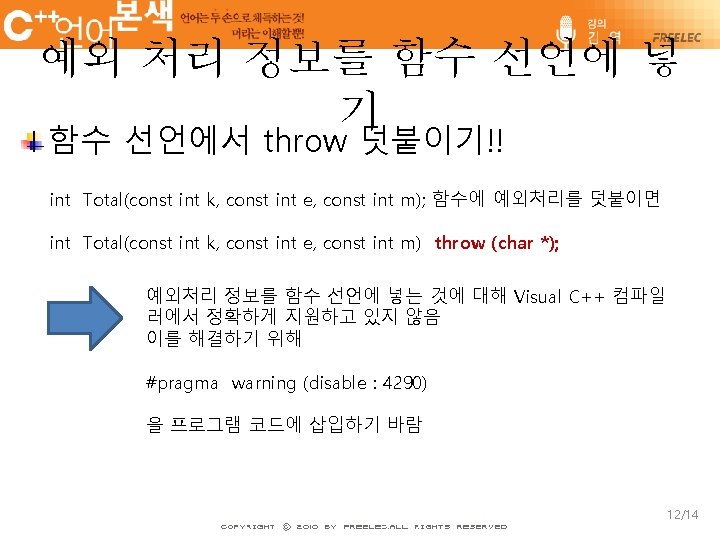
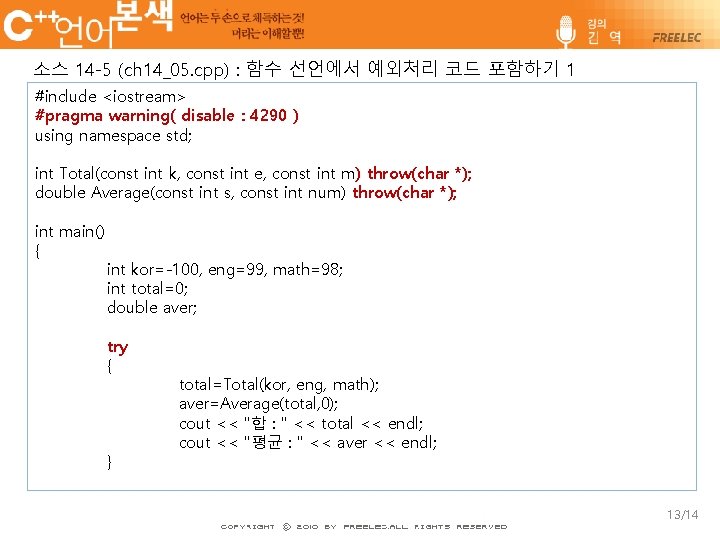
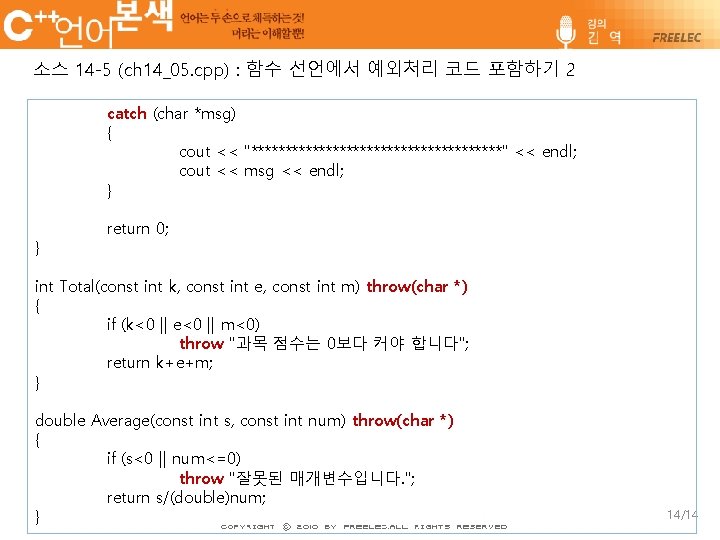
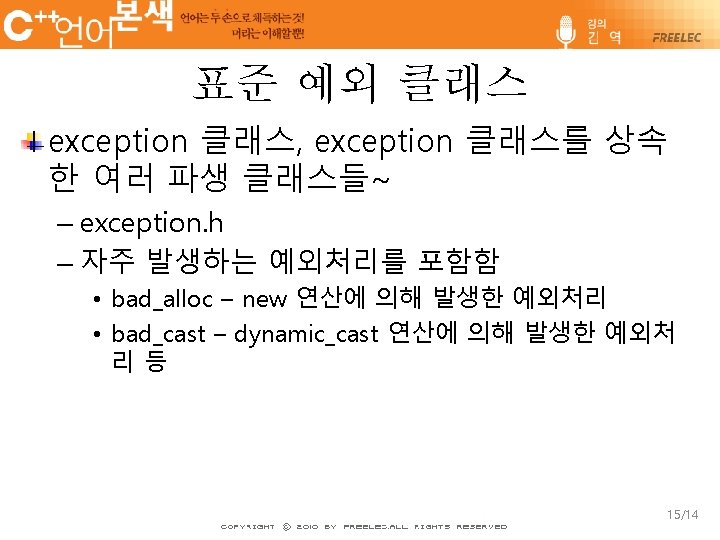
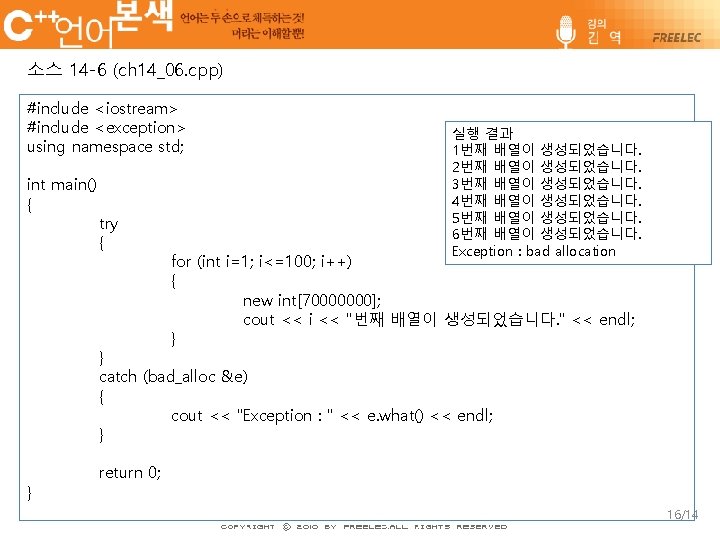
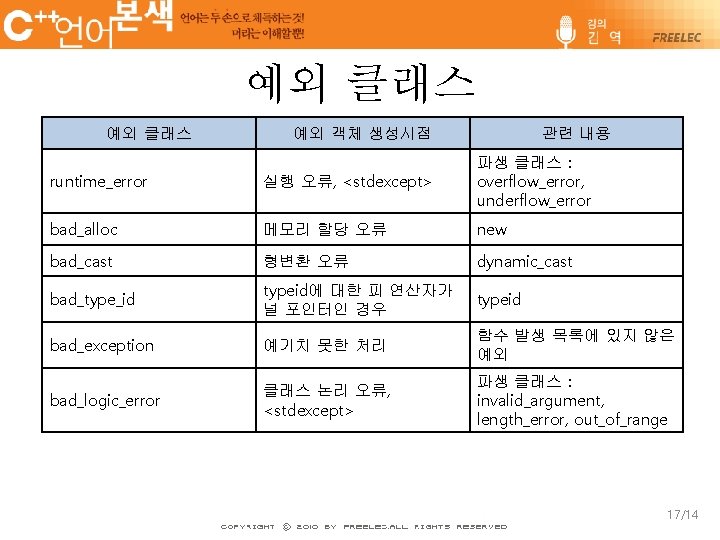
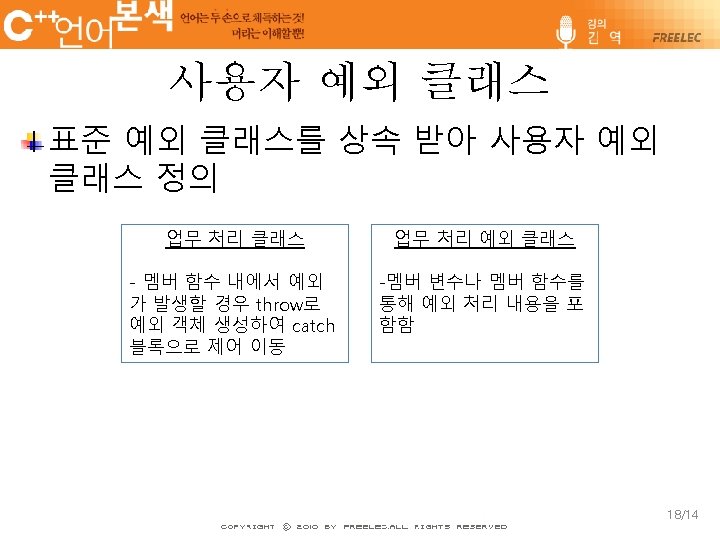
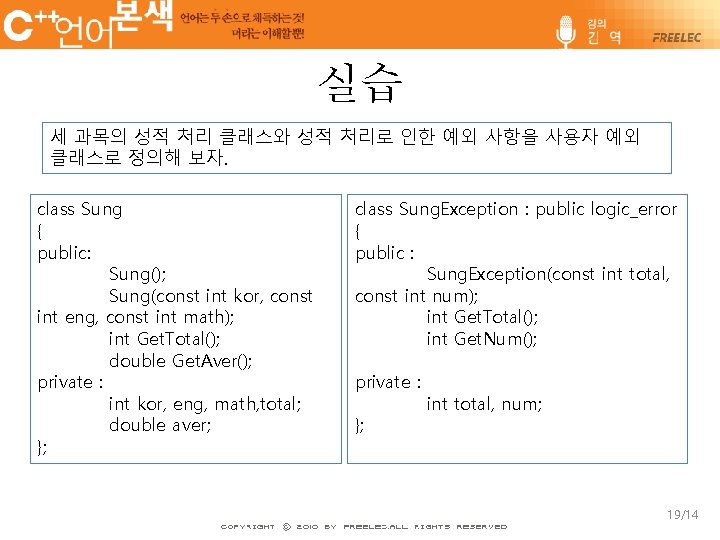
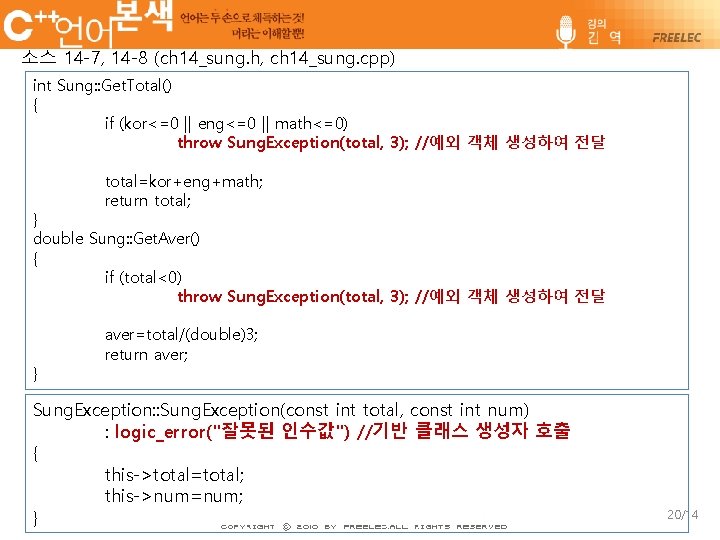
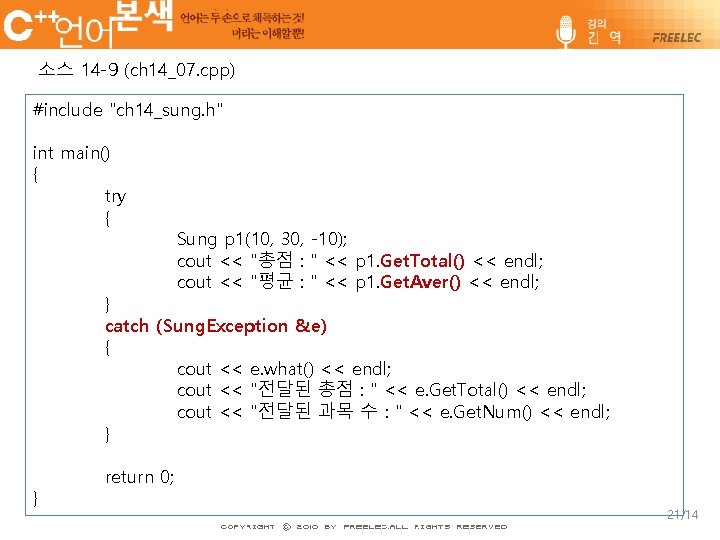
- Slides: 21
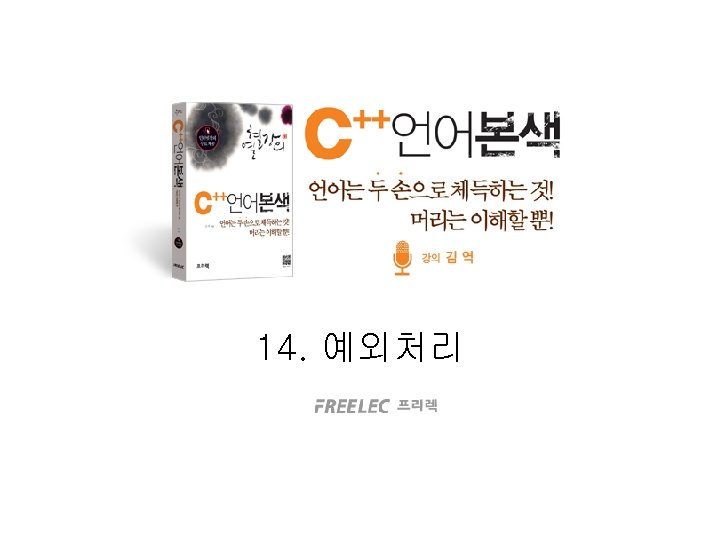
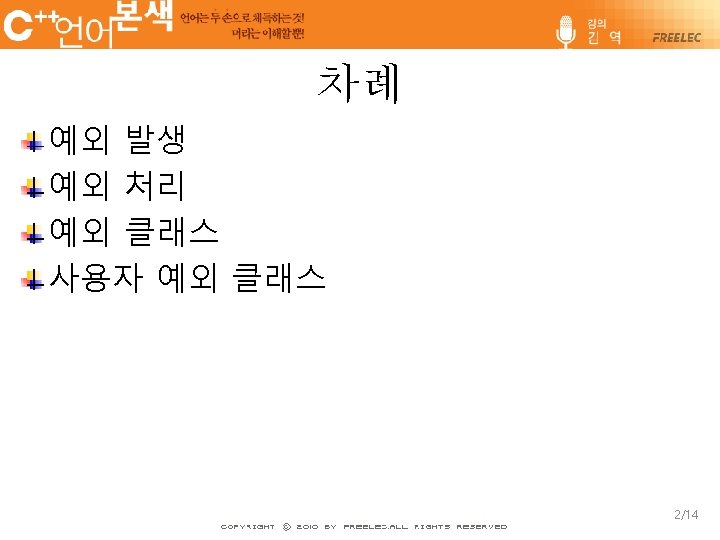
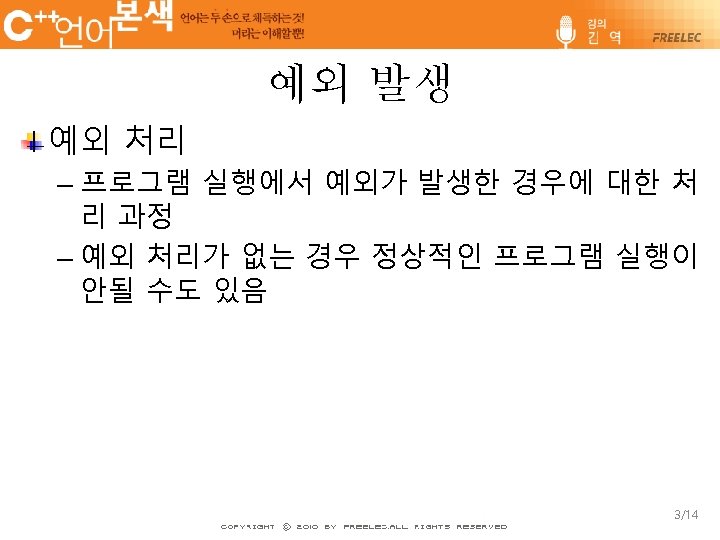
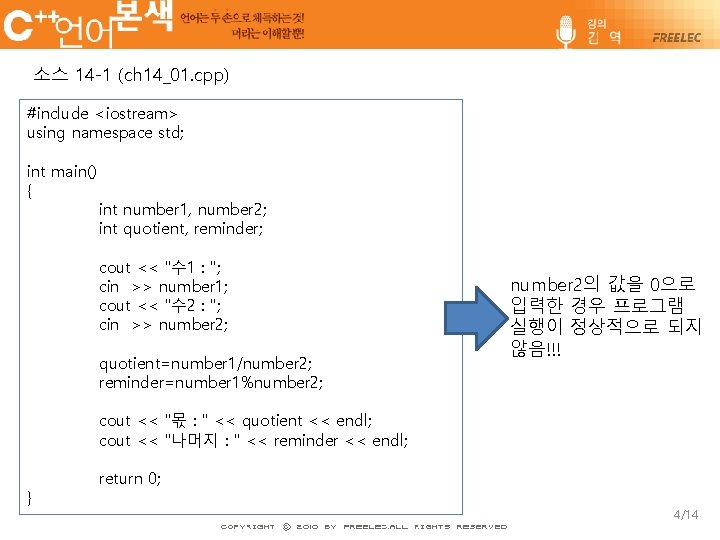
소스 14 -1 (ch 14_01. cpp) #include <iostream> using namespace std; int main() { int number 1, number 2; int quotient, reminder; cout << "수 1 : "; cin >> number 1; cout << "수 2 : "; cin >> number 2; quotient=number 1/number 2; reminder=number 1%number 2; number 2의 값을 0으로 입력한 경우 프로그램 실행이 정상적으로 되지 않음!!! cout << "몫 : " << quotient << endl; cout << "나머지 : " << reminder << endl; } return 0; 4/14
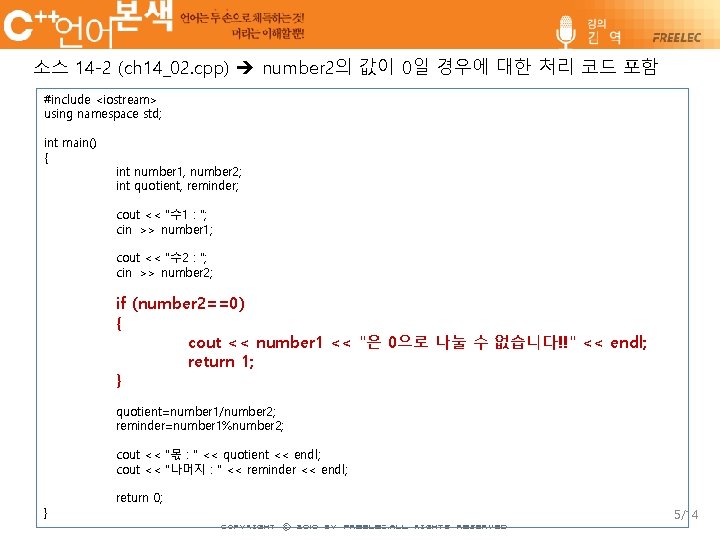
소스 14 -2 (ch 14_02. cpp) number 2의 값이 0일 경우에 대한 처리 코드 포함 #include <iostream> using namespace std; int main() { int number 1, number 2; int quotient, reminder; cout << "수 1 : "; cin >> number 1; cout << "수 2 : "; cin >> number 2; if (number 2==0) { cout << number 1 << "은 0으로 나눌 수 없습니다!!" << endl; return 1; } quotient=number 1/number 2; reminder=number 1%number 2; cout << "몫 : " << quotient << endl; cout << "나머지 : " << reminder << endl; } return 0; 5/14
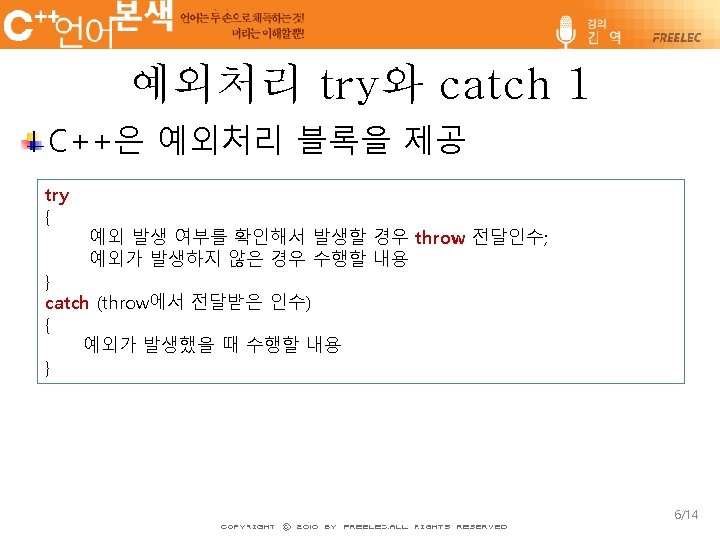
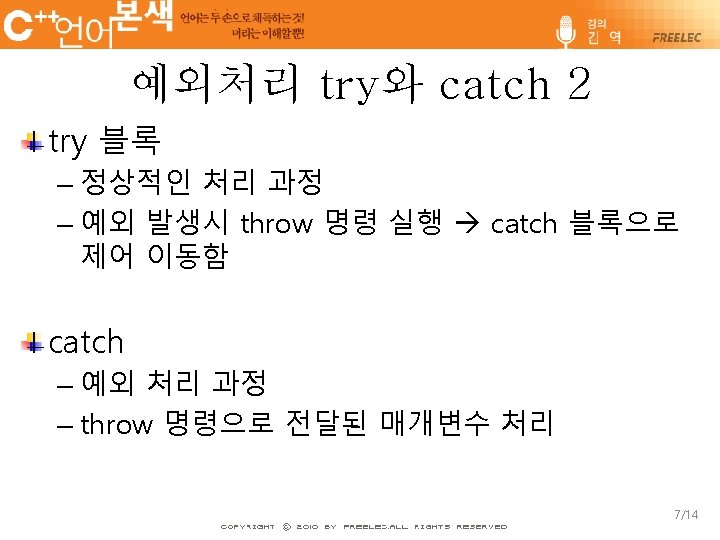
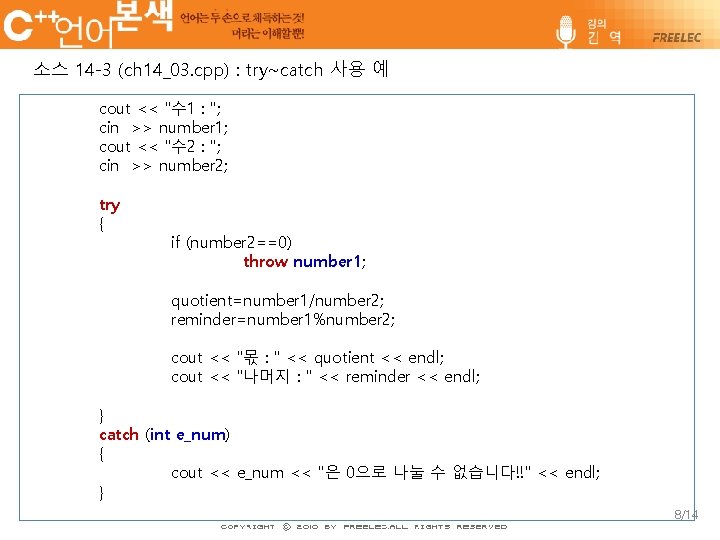
소스 14 -3 (ch 14_03. cpp) : try~catch 사용 예 cout << "수 1 : "; cin >> number 1; cout << "수 2 : "; cin >> number 2; try { if (number 2==0) throw number 1; quotient=number 1/number 2; reminder=number 1%number 2; cout << "몫 : " << quotient << endl; cout << "나머지 : " << reminder << endl; } catch (int e_num) { cout << e_num << "은 0으로 나눌 수 없습니다!!" << endl; } 8/14
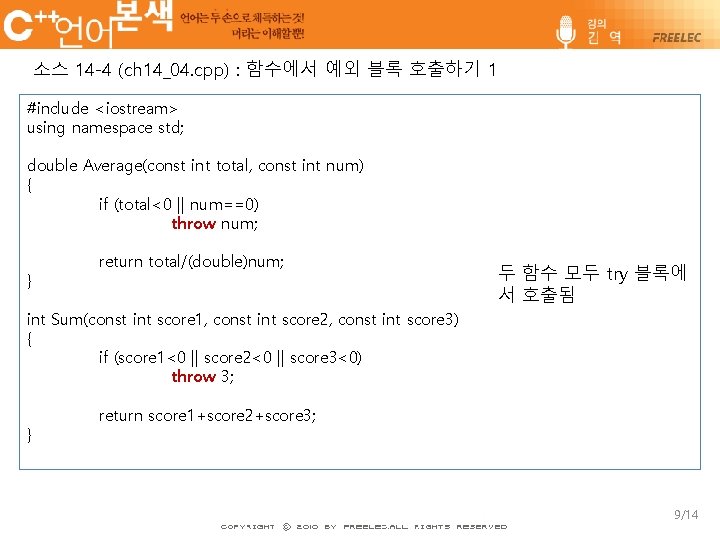
소스 14 -4 (ch 14_04. cpp) : 함수에서 예외 블록 호출하기 1 #include <iostream> using namespace std; double Average(const int total, const int num) { if (total<0 || num==0) throw num; } return total/(double)num; 두 함수 모두 try 블록에 서 호출됨 int Sum(const int score 1, const int score 2, const int score 3) { if (score 1<0 || score 2<0 || score 3<0) throw 3; } return score 1+score 2+score 3; 9/14
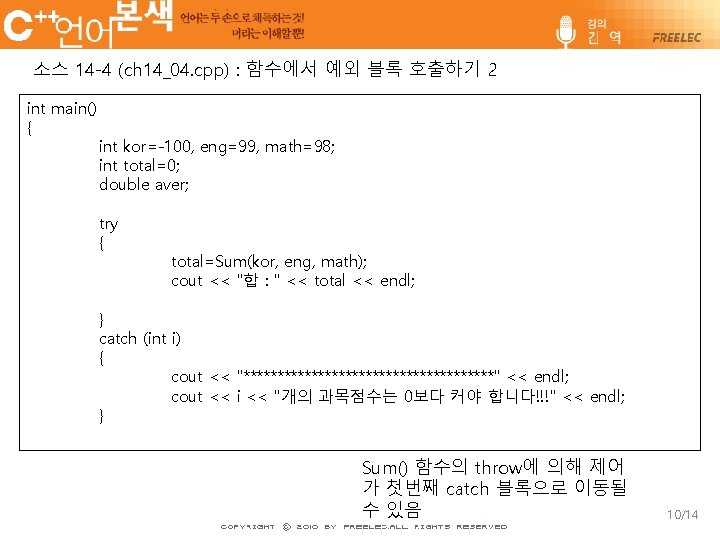
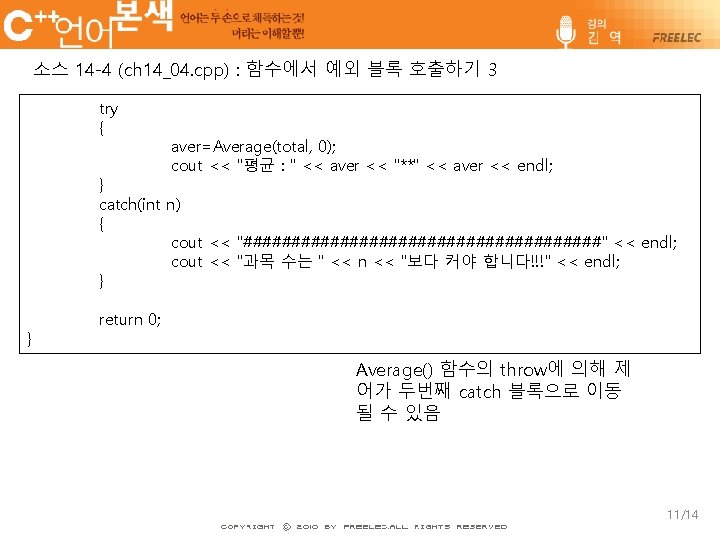
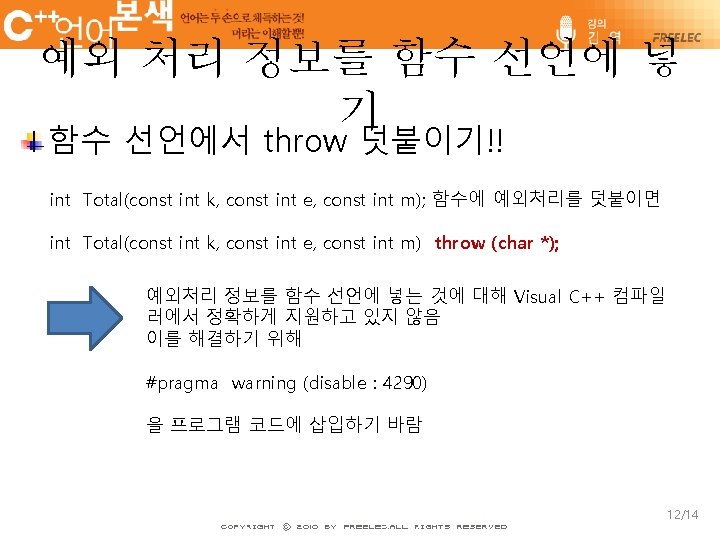
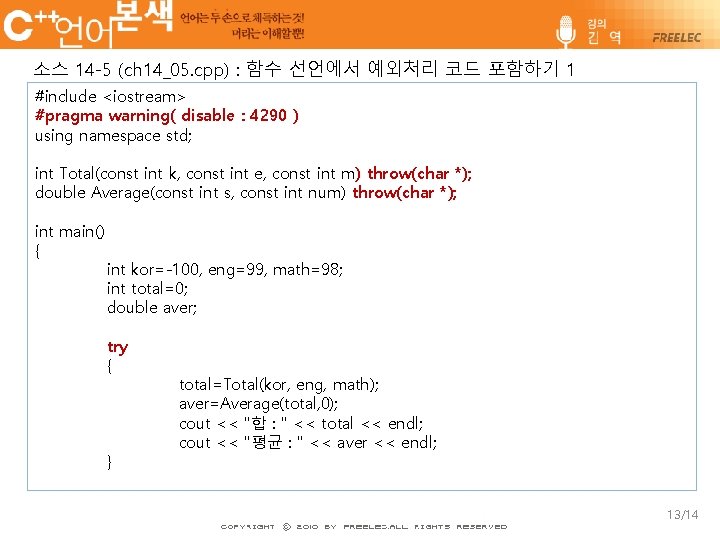
소스 14 -5 (ch 14_05. cpp) : 함수 선언에서 예외처리 코드 포함하기 1 #include <iostream> #pragma warning( disable : 4290 ) using namespace std; int Total(const int k, const int e, const int m) throw(char *); double Average(const int s, const int num) throw(char *); int main() { int kor=-100, eng=99, math=98; int total=0; double aver; try { } total=Total(kor, eng, math); aver=Average(total, 0); cout << "합 : " << total << endl; cout << "평균 : " << aver << endl; 13/14
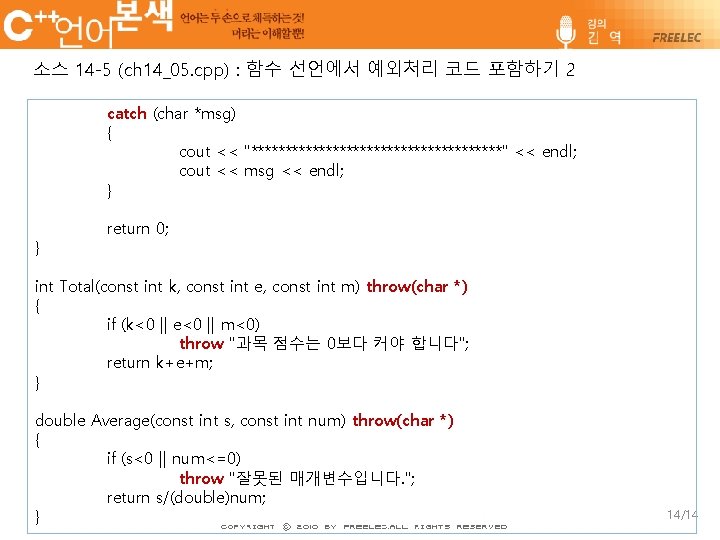
소스 14 -5 (ch 14_05. cpp) : 함수 선언에서 예외처리 코드 포함하기 2 catch (char *msg) { cout << "*******************" << endl; cout << msg << endl; } } return 0; int Total(const int k, const int e, const int m) throw(char *) { if (k<0 || e<0 || m<0) throw "과목 점수는 0보다 커야 합니다"; return k+e+m; } double Average(const int s, const int num) throw(char *) { if (s<0 || num<=0) throw "잘못된 매개변수입니다. "; return s/(double)num; } 14/14
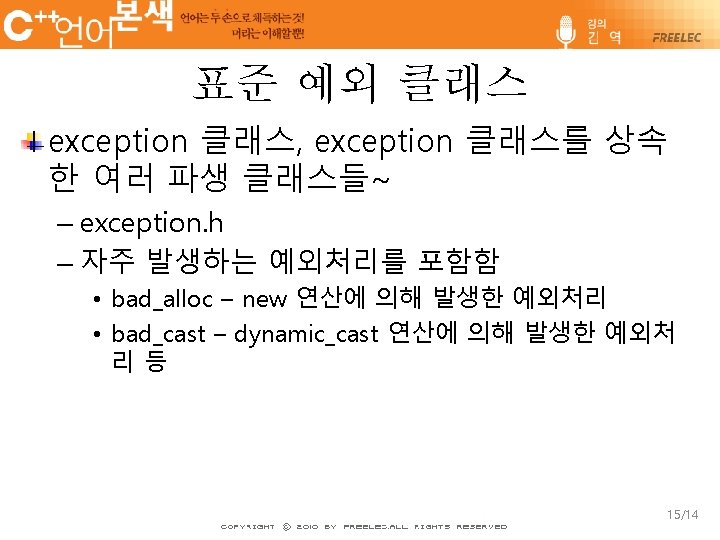
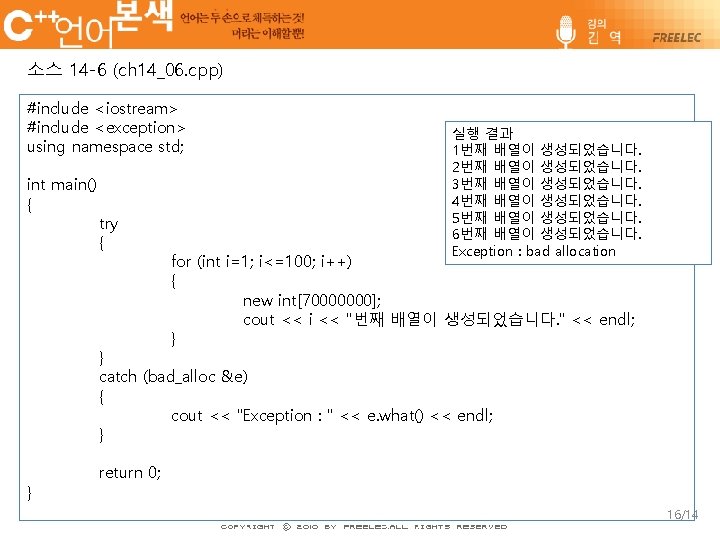
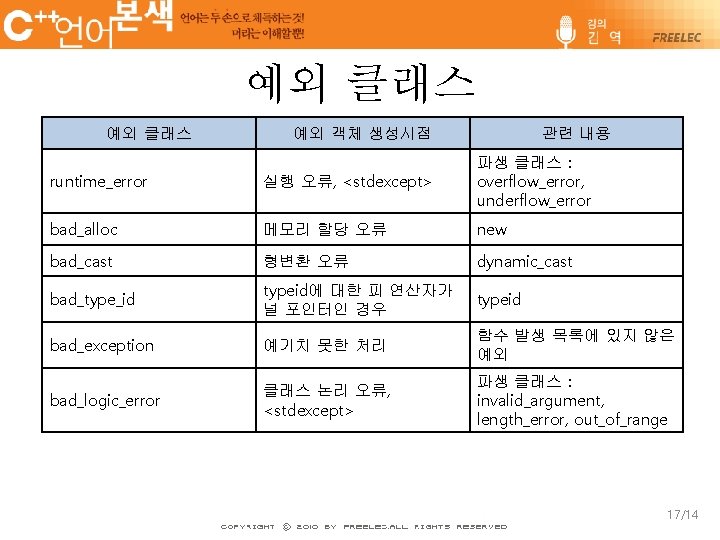
예외 클래스 예외 객체 생성시점 관련 내용 runtime_error 실행 오류, <stdexcept> 파생 클래스 : overflow_error, underflow_error bad_alloc 메모리 할당 오류 new bad_cast 형변환 오류 dynamic_cast bad_type_id typeid에 대한 피 연산자가 널 포인터인 경우 typeid bad_exception 예기치 못한 처리 함수 발생 목록에 있지 않은 예외 bad_logic_error 클래스 논리 오류, <stdexcept> 파생 클래스 : invalid_argument, length_error, out_of_range 17/14
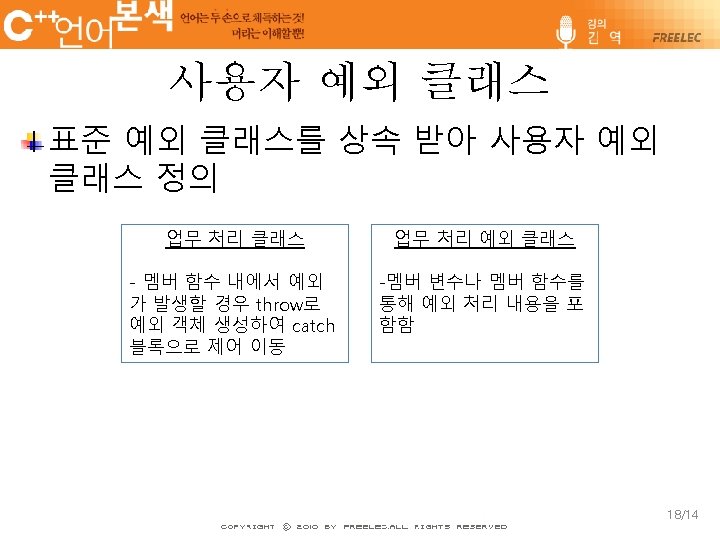
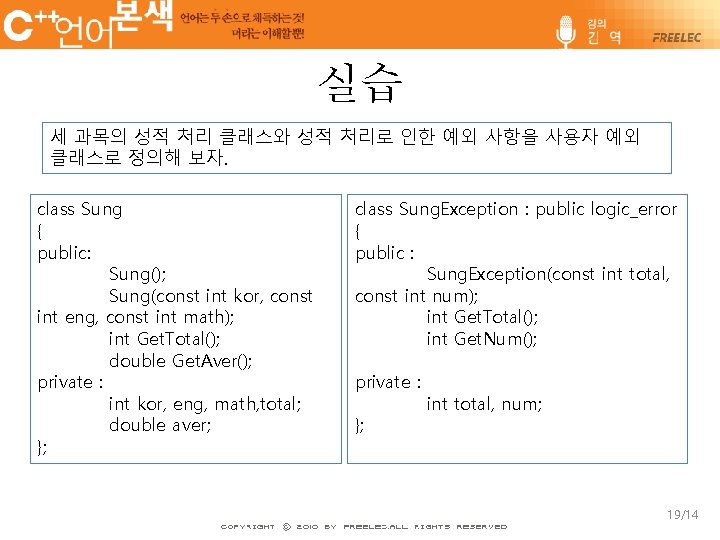
실습 세 과목의 성적 처리 클래스와 성적 처리로 인한 예외 사항을 사용자 예외 클래스로 정의해 보자. class Sung { public: Sung(); Sung(const int kor, const int eng, const int math); int Get. Total(); double Get. Aver(); private : int kor, eng, math, total; double aver; }; class Sung. Exception : public logic_error { public : Sung. Exception(const int total, const int num); int Get. Total(); int Get. Num(); private : }; int total, num; 19/14
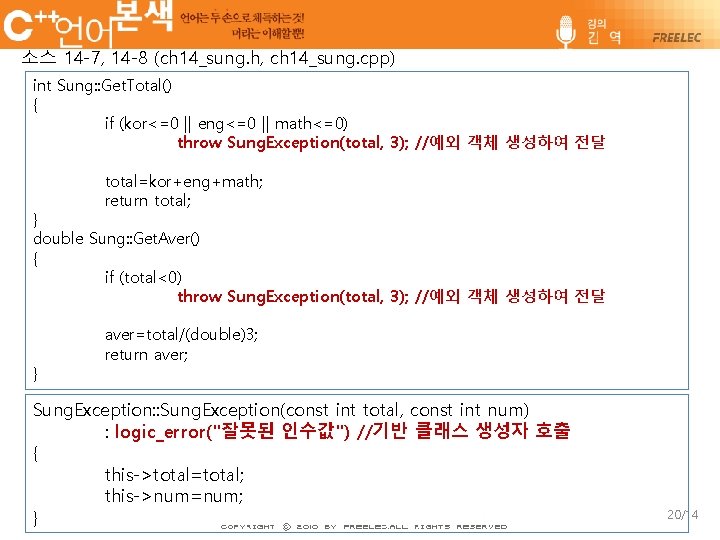
소스 14 -7, 14 -8 (ch 14_sung. h, ch 14_sung. cpp) int Sung: : Get. Total() { if (kor<=0 || eng<=0 || math<=0) throw Sung. Exception(total, 3); //예외 객체 생성하여 전달 total=kor+eng+math; return total; } double Sung: : Get. Aver() { if (total<0) throw Sung. Exception(total, 3); //예외 객체 생성하여 전달 } aver=total/(double)3; return aver; Sung. Exception: : Sung. Exception(const int total, const int num) : logic_error("잘못된 인수값") //기반 클래스 생성자 호출 { this->total=total; this->num=num; } 20/14
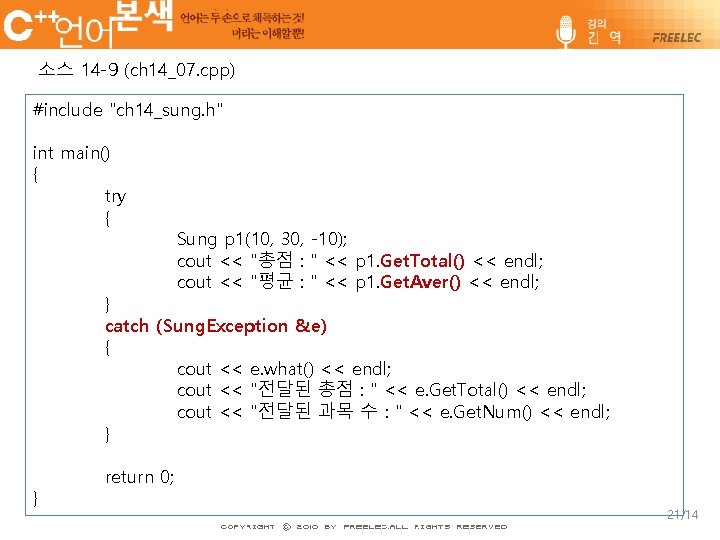
소스 14 -9 (ch 14_07. cpp) #include "ch 14_sung. h" int main() { try { Sung p 1(10, 30, -10); cout << "총점 : " << p 1. Get. Total() << endl; cout << "평균 : " << p 1. Get. Aver() << endl; } catch (Sung. Exception &e) { cout << e. what() << endl; cout << "전달된 총점 : " << e. Get. Total() << endl; cout << "전달된 과목 수 : " << e. Get. Num() << endl; } } return 0; 21/14
#include iostream using namespace std
#include iostream #include string using namespace std
#include iostream #include string using namespace std
#include string
#include iostream #include string
Iostream en c
#include iostream #include cmath
윤년 계산
Include iostream using namespace std
Using namespace std class base
#include iostream using namespace std
#include iostream using namespace std
#include iostream using namespace std int main()
#include stdio.h #include conio.h #include stdlib.h
#include stdio.h #include stdlib.h #include string.h