UDP vs TCP l UDP l TCP Lowlevel
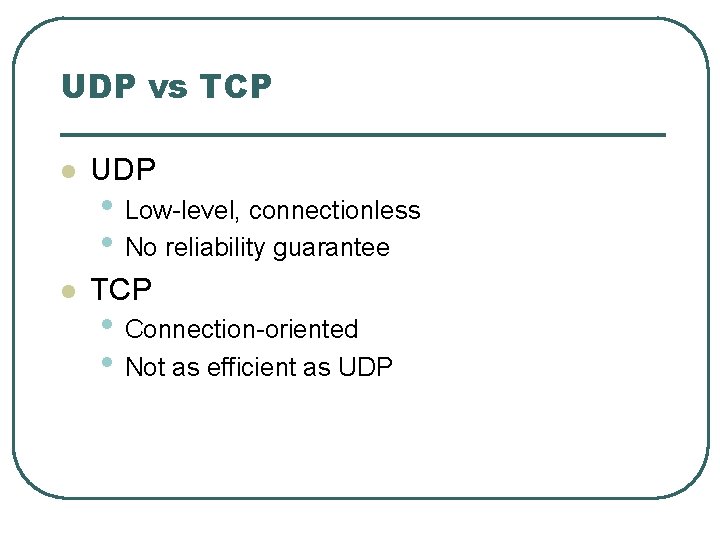
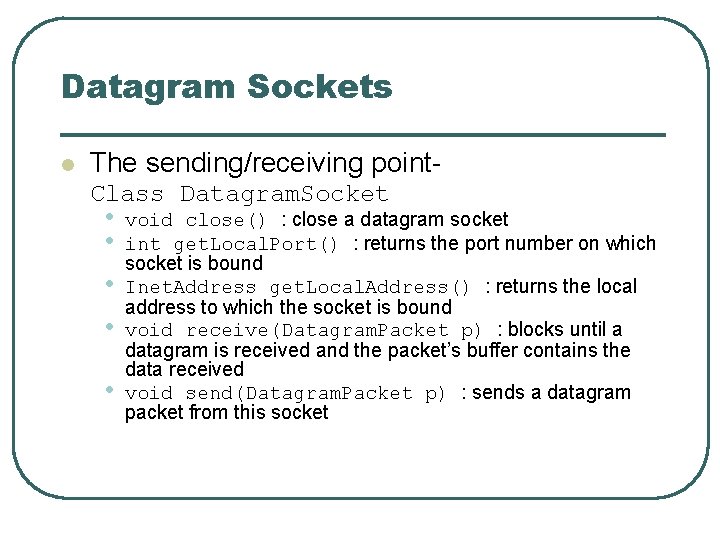
![//Datagram Server public class Datagram. Server { public static void main(String[] args) { Datagram. //Datagram Server public class Datagram. Server { public static void main(String[] args) { Datagram.](https://slidetodoc.com/presentation_image_h2/18e3952c9816d52fa57823e28bd0b61f/image-3.jpg)
![//Datagram Client public class Datagram. Client { public static void main(String[] args) { String //Datagram Client public class Datagram. Client { public static void main(String[] args) { String](https://slidetodoc.com/presentation_image_h2/18e3952c9816d52fa57823e28bd0b61f/image-4.jpg)
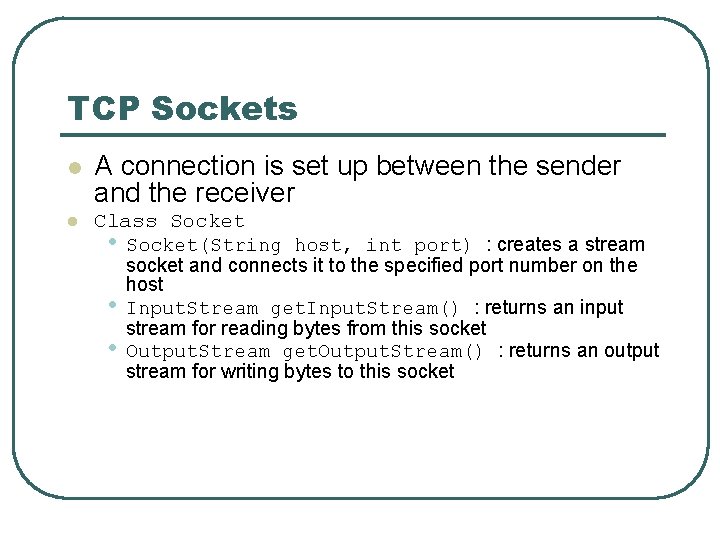
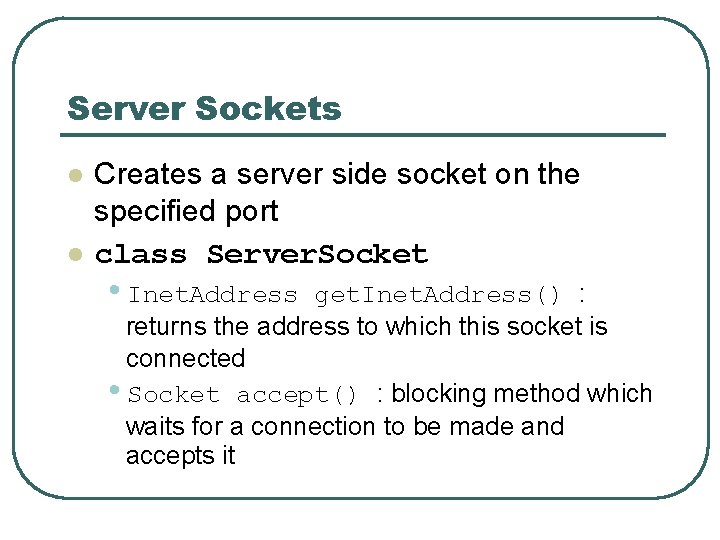
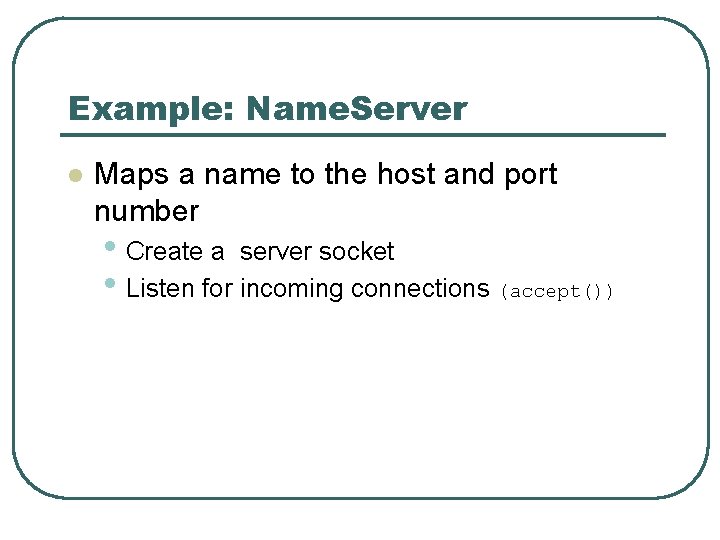
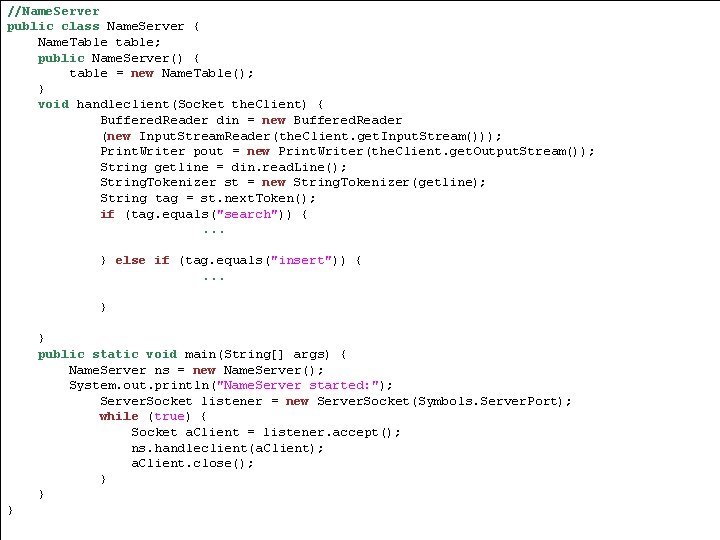
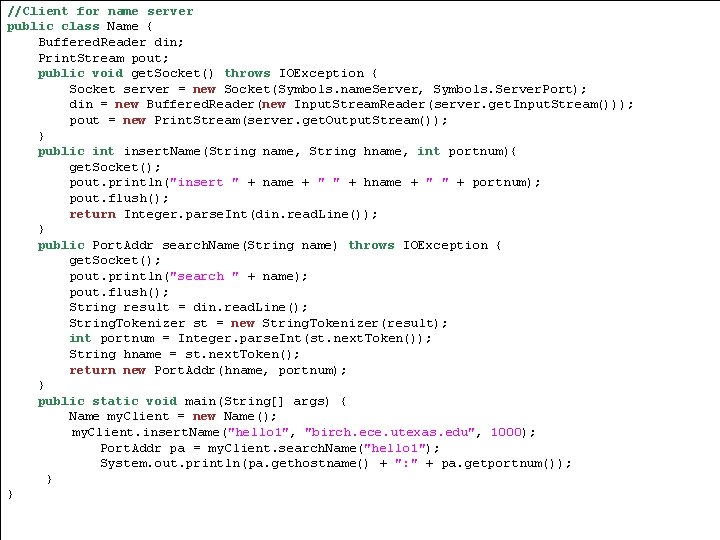
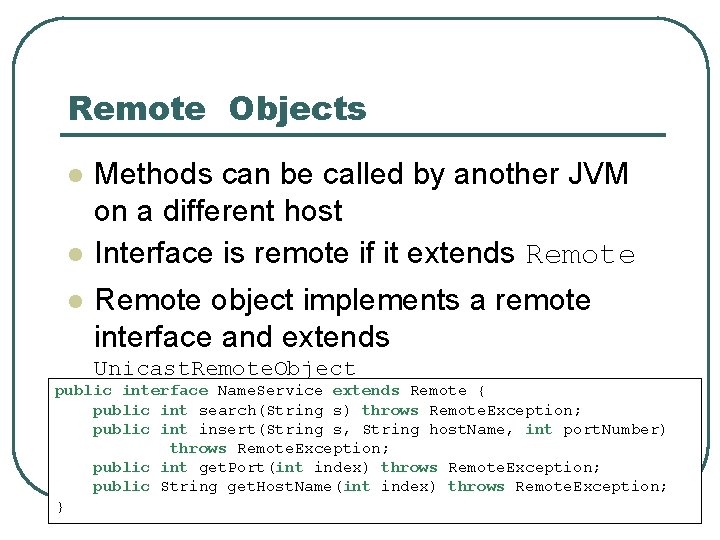
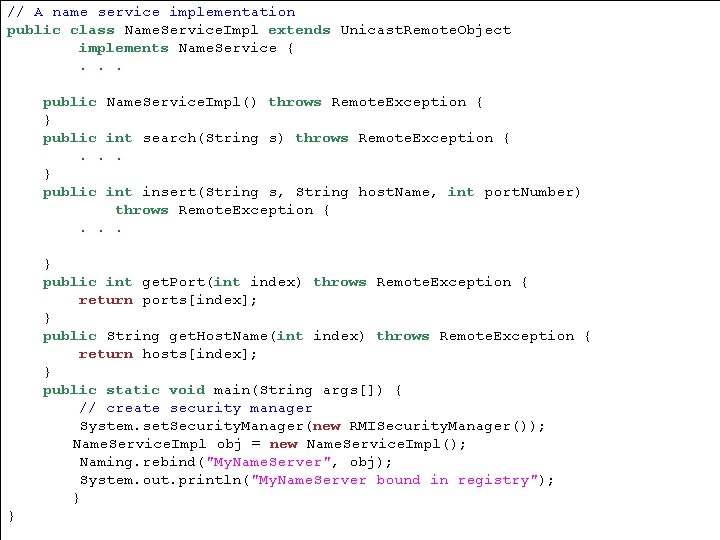
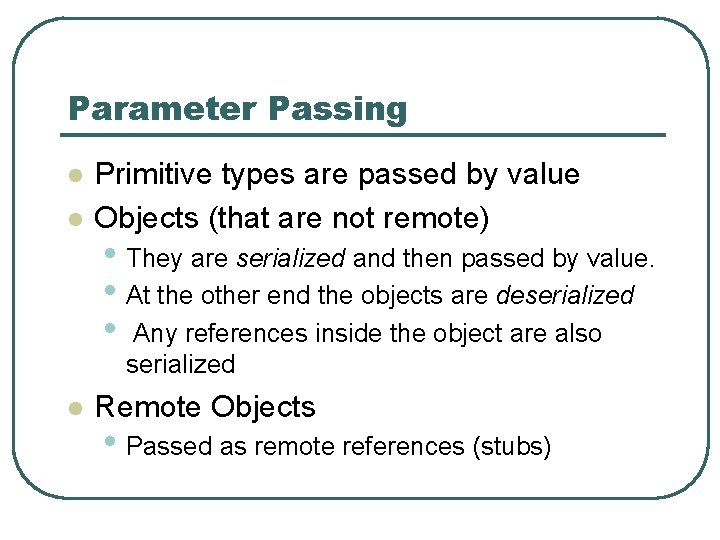
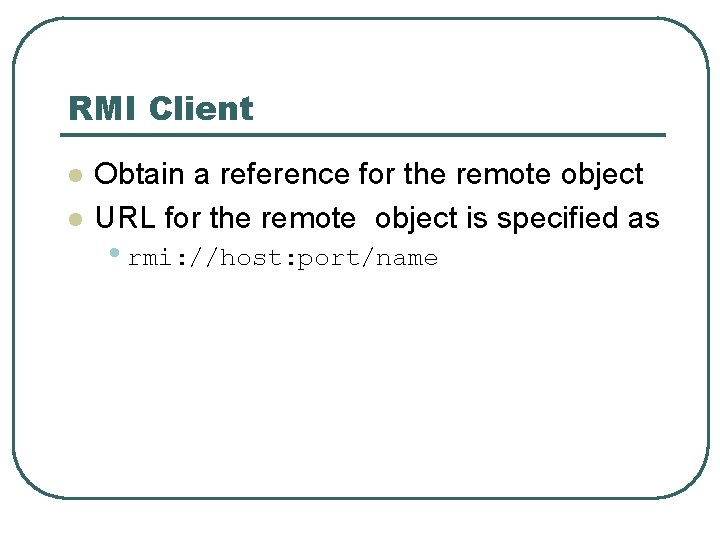
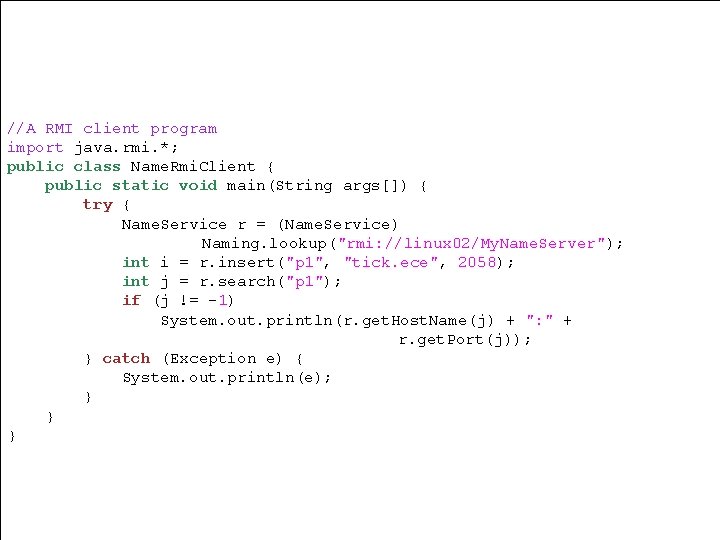
- Slides: 14
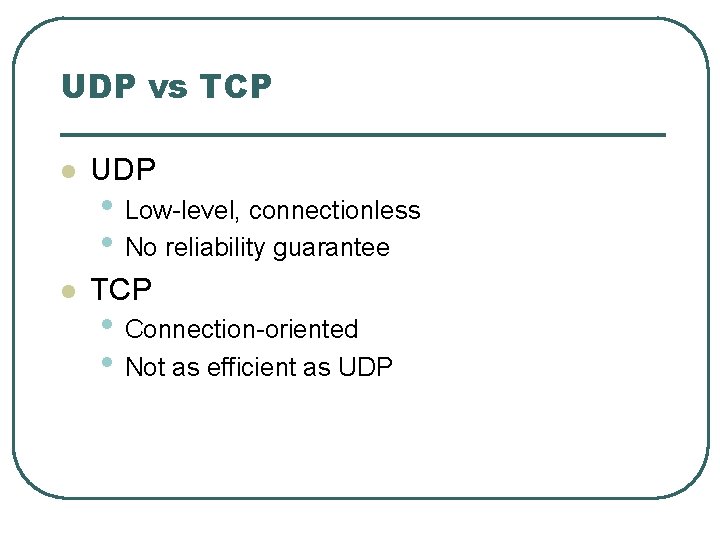
UDP vs TCP l UDP l TCP • Low-level, connectionless • No reliability guarantee • Connection-oriented • Not as efficient as UDP
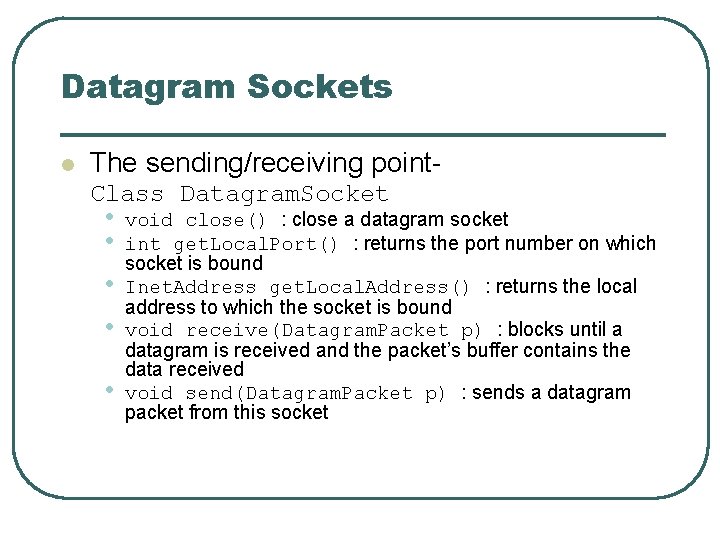
Datagram Sockets l The sending/receiving point. Class Datagram. Socket • void close() : close a datagram socket • int get. Local. Port() : returns the port number on which socket is bound • Inet. Address get. Local. Address() : returns the local address to which the socket is bound • void receive(Datagram. Packet p) : blocks until a • datagram is received and the packet’s buffer contains the data received void send(Datagram. Packet p) : sends a datagram packet from this socket
![Datagram Server public class Datagram Server public static void mainString args Datagram //Datagram Server public class Datagram. Server { public static void main(String[] args) { Datagram.](https://slidetodoc.com/presentation_image_h2/18e3952c9816d52fa57823e28bd0b61f/image-3.jpg)
//Datagram Server public class Datagram. Server { public static void main(String[] args) { Datagram. Packet datapacket, returnpacket; int port = 2018; int len = 1024; try { Datagram. Socket datasocket = new Datagram. Socket(port); byte[] buf = new byte[len]; datapacket = new Datagram. Packet(buf, buf. length); while (true) { try { datasocket. receive(datapacket); returnpacket = new Datagram. Packet( datapacket. get. Data(), datapacket. get. Length(), datapacket. get. Address(), datapacket. get. Port()); datasocket. send(returnpacket); } catch (IOException e) { System. err. println(e); } } } catch (Socket. Exception se) { System. err. println(se); } } }
![Datagram Client public class Datagram Client public static void mainString args String //Datagram Client public class Datagram. Client { public static void main(String[] args) { String](https://slidetodoc.com/presentation_image_h2/18e3952c9816d52fa57823e28bd0b61f/image-4.jpg)
//Datagram Client public class Datagram. Client { public static void main(String[] args) { String hostname; int port = 2018; int len = 1024; Datagram. Packet s. Packet, r. Packet; Inet. Address ia = Inet. Address. get. By. Name(hostname); Datagram. Socket datasocket = new Datagram. Socket(); Buffered. Reader stdinp = new Buffered. Reader( new Input. Stream. Reader(System. in)); while (true) { String echoline = stdinp. read. Line(); if (echoline. equals("done")) break; byte[] buffer = new byte[echoline. length()]; buffer = echoline. get. Bytes(); s. Packet = new Datagram. Packet(buffer, buffer. length, ia, port); datasocket. send(s. Packet); byte[] rbuffer = new byte[len]; r. Packet = new Datagram. Packet(rbuffer, rbuffer. length); datasocket. receive(r. Packet); String retstring = new String(r. Packet. get. Data()); System. out. println(retstring); } catch (IOException e) { System. err. println(e); } } // while } } // end main
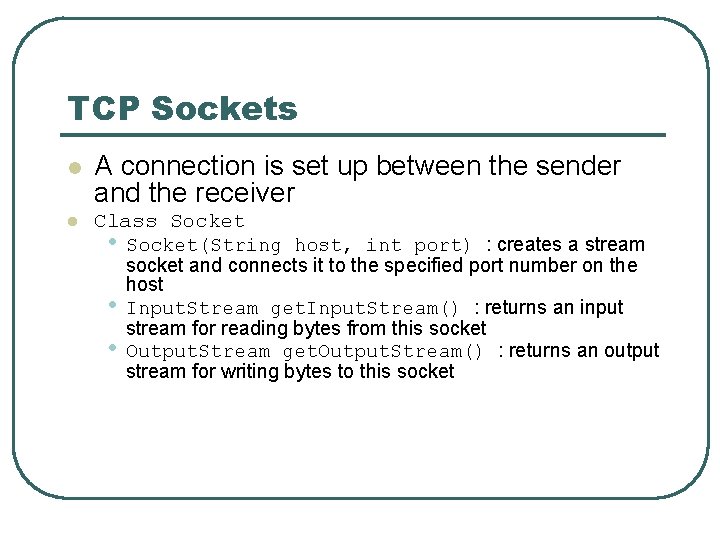
TCP Sockets l A connection is set up between the sender and the receiver l Class Socket • Socket(String host, int port) : creates a stream socket and connects it to the specified port number on the host • Input. Stream get. Input. Stream() : returns an input stream for reading bytes from this socket • Output. Stream get. Output. Stream() : returns an output stream for writing bytes to this socket
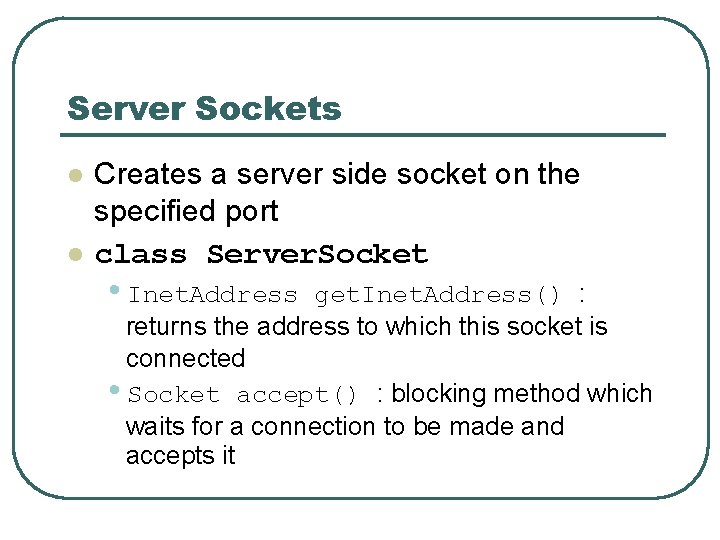
Server Sockets l l Creates a server side socket on the specified port class Server. Socket • Inet. Address get. Inet. Address() : returns the address to which this socket is connected • Socket accept() : blocking method which waits for a connection to be made and accepts it
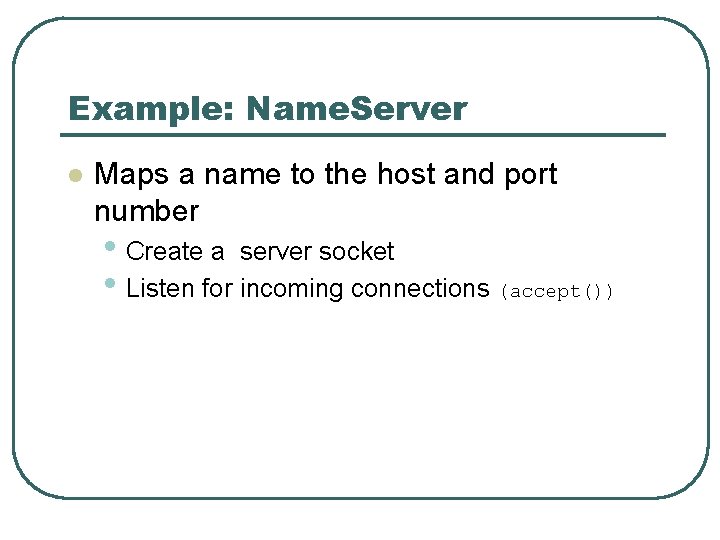
Example: Name. Server l Maps a name to the host and port number • Create a server socket • Listen for incoming connections (accept())
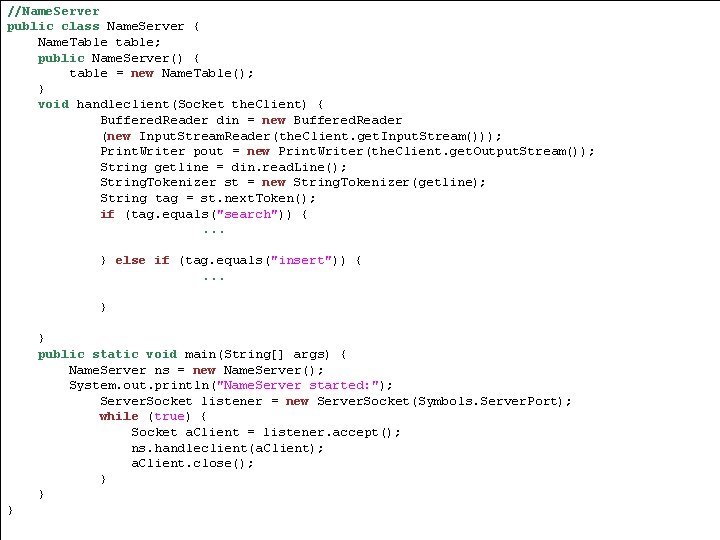
//Name. Server public class Name. Server { Name. Table table; public Name. Server() { table = new Name. Table(); } void handleclient(Socket the. Client) { Buffered. Reader din = new Buffered. Reader (new Input. Stream. Reader(the. Client. get. Input. Stream())); Print. Writer pout = new Print. Writer(the. Client. get. Output. Stream()); String getline = din. read. Line(); String. Tokenizer st = new String. Tokenizer(getline); String tag = st. next. Token(); if (tag. equals("search")) {. . . } else if (tag. equals("insert")) {. . . } } public static void main(String[] args) { Name. Server ns = new Name. Server(); System. out. println("Name. Server started: "); Server. Socket listener = new Server. Socket(Symbols. Server. Port); while (true) { Socket a. Client = listener. accept(); ns. handleclient(a. Client); a. Client. close(); } } }
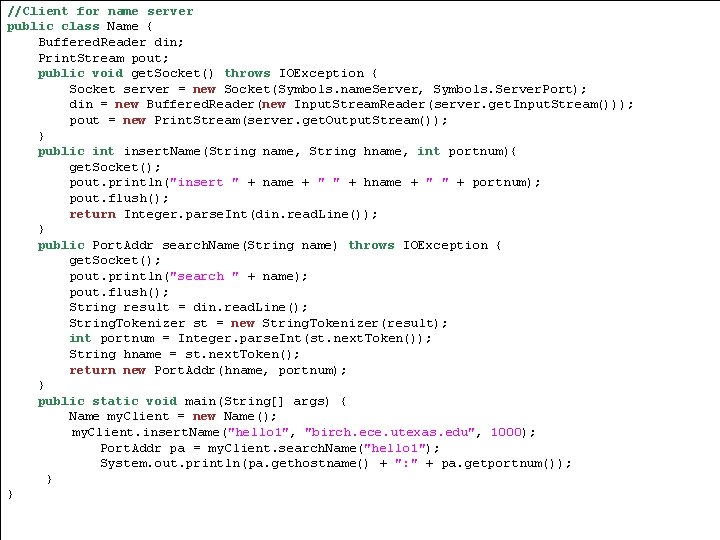
//Client for name server public class Name { Buffered. Reader din; Print. Stream pout; public void get. Socket() throws IOException { Socket server = new Socket(Symbols. name. Server, Symbols. Server. Port); din = new Buffered. Reader(new Input. Stream. Reader(server. get. Input. Stream())); pout = new Print. Stream(server. get. Output. Stream()); } public int insert. Name(String name, String hname, int portnum){ get. Socket(); pout. println("insert " + name + " " + hname + " " + portnum); pout. flush(); return Integer. parse. Int(din. read. Line()); } public Port. Addr search. Name(String name) throws IOException { get. Socket(); pout. println("search " + name); pout. flush(); String result = din. read. Line(); String. Tokenizer st = new String. Tokenizer(result); int portnum = Integer. parse. Int(st. next. Token()); String hname = st. next. Token(); return new Port. Addr(hname, portnum); } public static void main(String[] args) { Name my. Client = new Name(); my. Client. insert. Name("hello 1", "birch. ece. utexas. edu", 1000); Port. Addr pa = my. Client. search. Name("hello 1"); System. out. println(pa. gethostname() + ": " + pa. getportnum()); } }
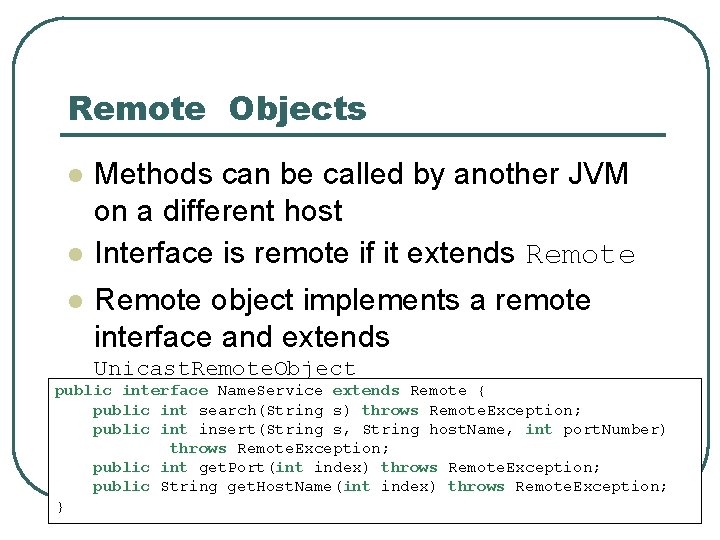
Remote Objects l l l Methods can be called by another JVM on a different host Interface is remote if it extends Remote object implements a remote interface and extends Unicast. Remote. Object public interface Name. Service extends Remote { public int search(String s) throws Remote. Exception; public int insert(String s, String host. Name, int port. Number) throws Remote. Exception; public int get. Port(int index) throws Remote. Exception; public String get. Host. Name(int index) throws Remote. Exception; }
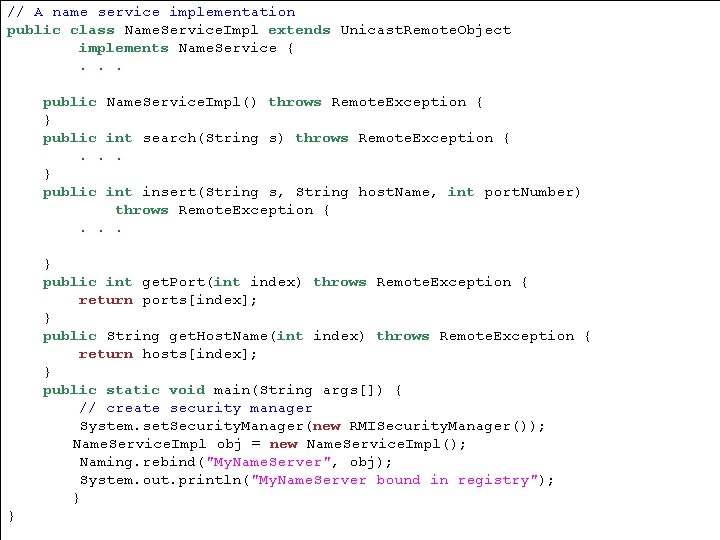
// A name service implementation public class Name. Service. Impl extends Unicast. Remote. Object implements Name. Service {. . . public Name. Service. Impl() throws Remote. Exception { } public int search(String s) throws Remote. Exception {. . . } public int insert(String s, String host. Name, int port. Number) throws Remote. Exception {. . . } public int get. Port(int index) throws Remote. Exception { return ports[index]; } public String get. Host. Name(int index) throws Remote. Exception { return hosts[index]; } public static void main(String args[]) { // create security manager System. set. Security. Manager(new RMISecurity. Manager()); Name. Service. Impl obj = new Name. Service. Impl(); Naming. rebind("My. Name. Server", obj); System. out. println("My. Name. Server bound in registry"); } }
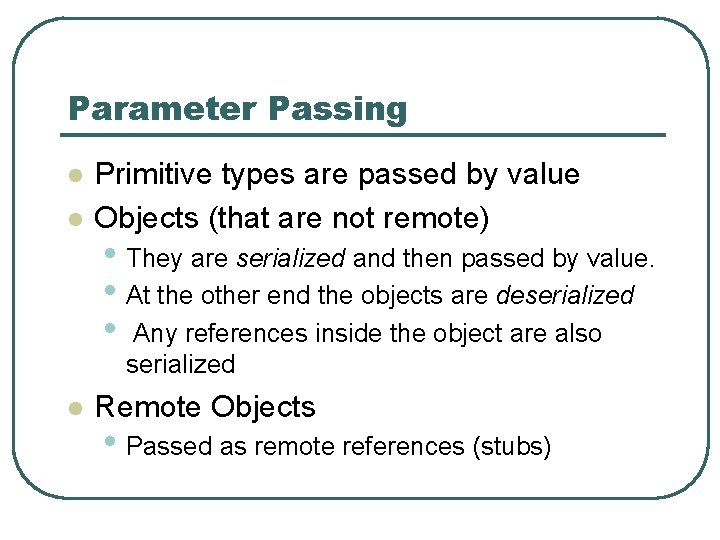
Parameter Passing l l Primitive types are passed by value Objects (that are not remote) • They are serialized and then passed by value. • At the other end the objects are deserialized • Any references inside the object are also serialized l Remote Objects • Passed as remote references (stubs)
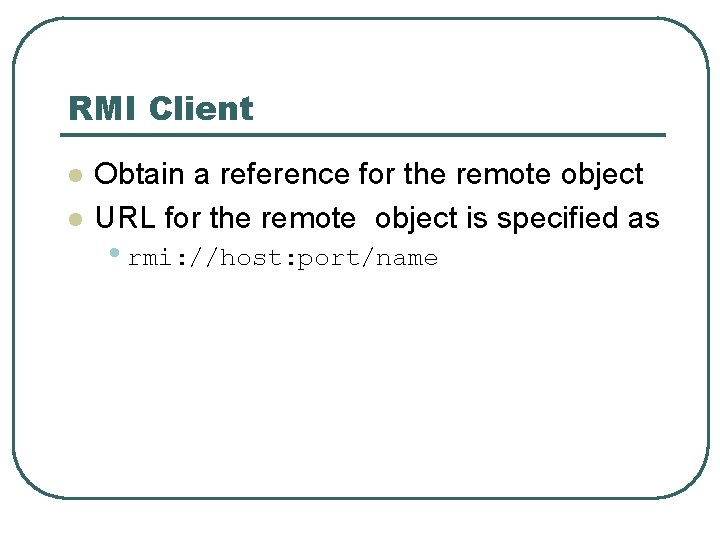
RMI Client l l Obtain a reference for the remote object URL for the remote object is specified as • rmi: //host: port/name
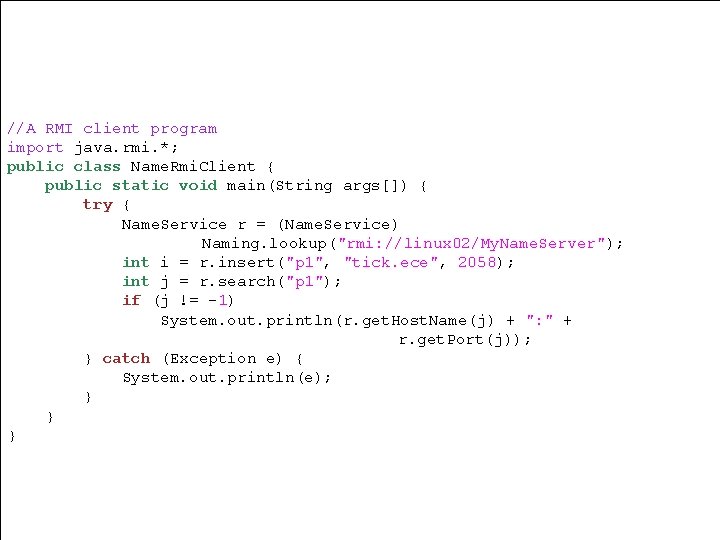
//A RMI client program import java. rmi. *; public class Name. Rmi. Client { public static void main(String args[]) { try { Name. Service r = (Name. Service) Naming. lookup("rmi: //linux 02/My. Name. Server"); int i = r. insert("p 1", "tick. ece", 2058); int j = r. search("p 1"); if (j != -1) System. out. println(r. get. Host. Name(j) + ": " + r. get. Port(j)); } catch (Exception e) { System. out. println(e); } } }