Tutorial 3 In this tutorial well see n
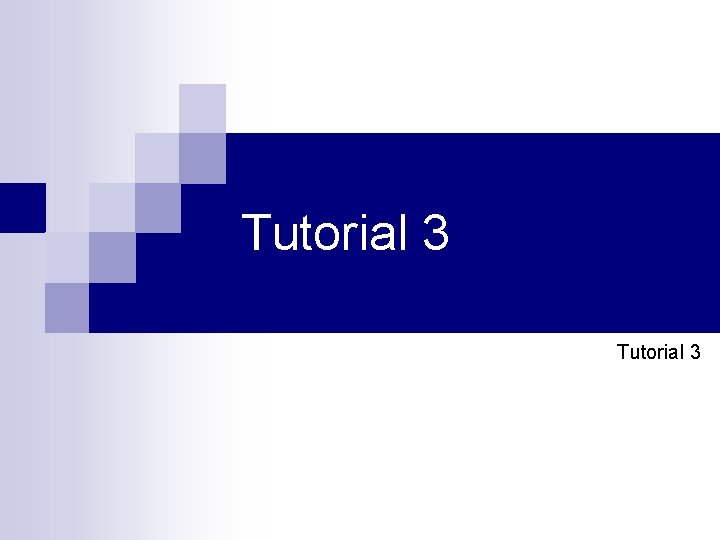
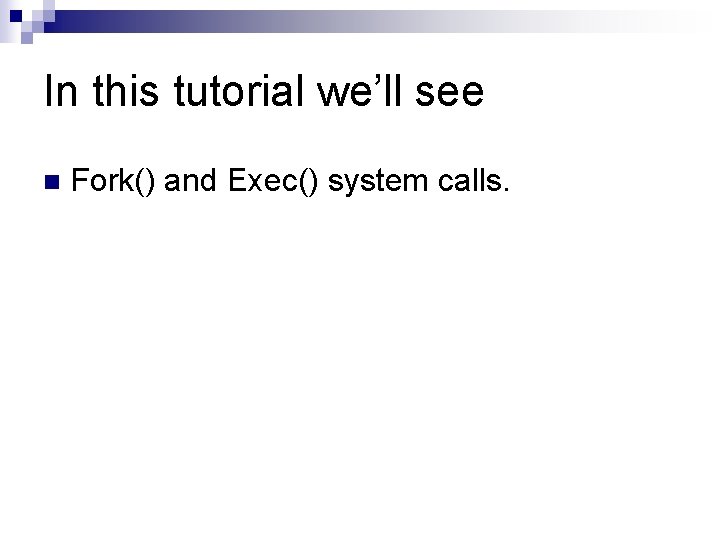
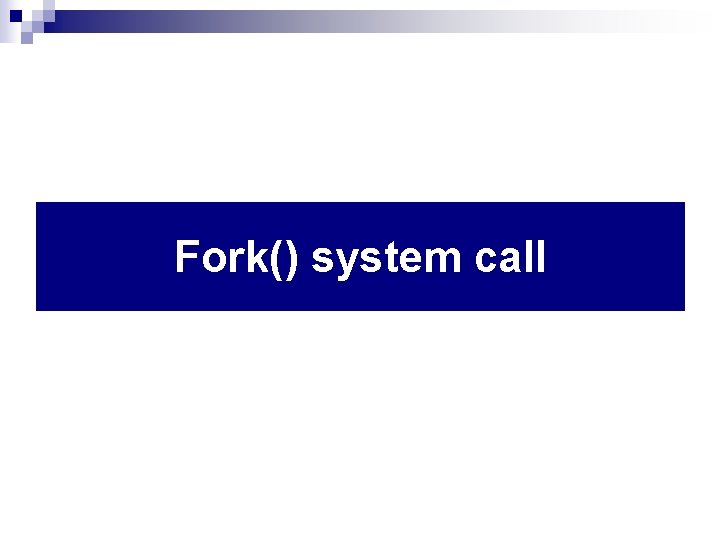
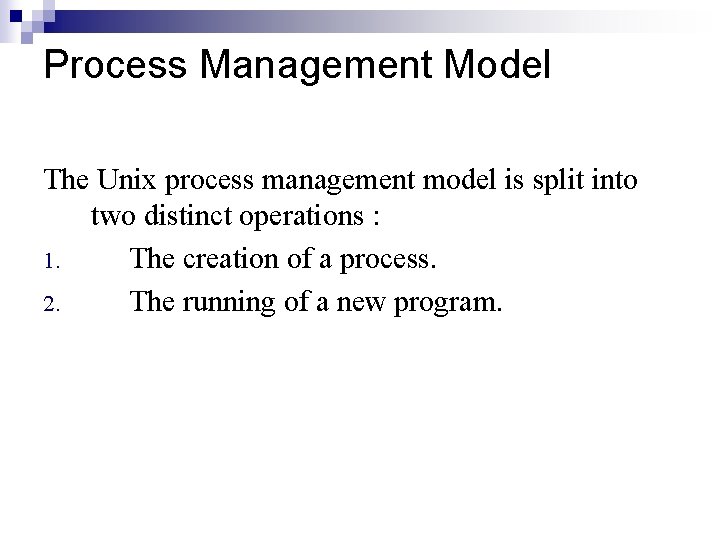
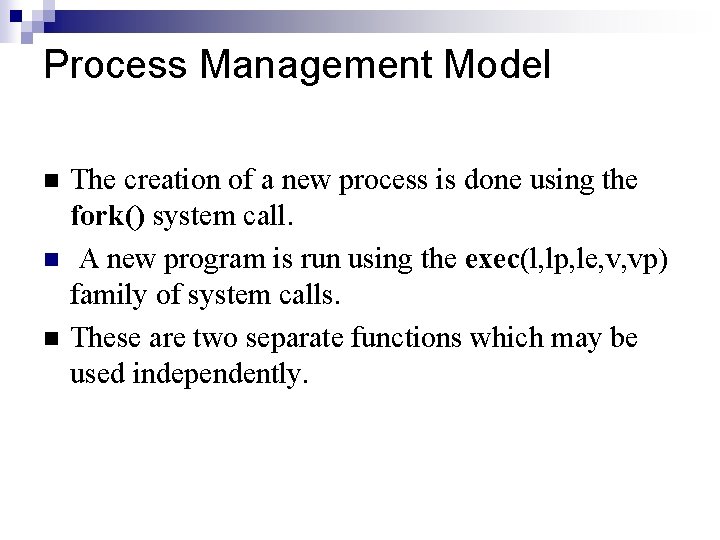
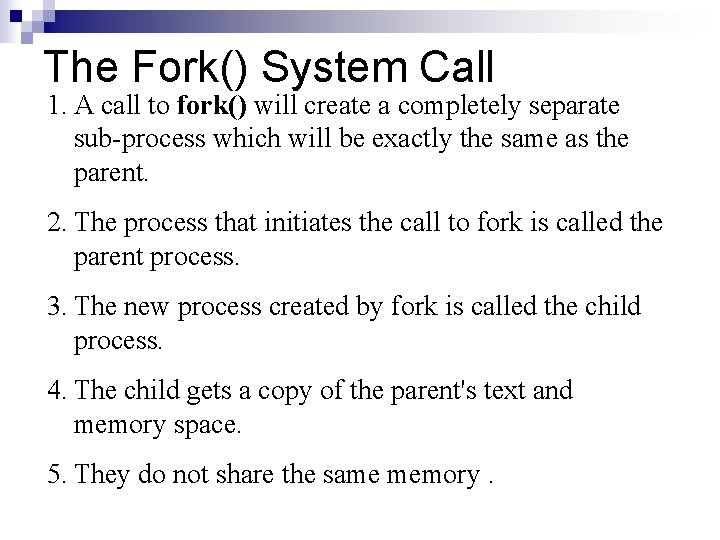
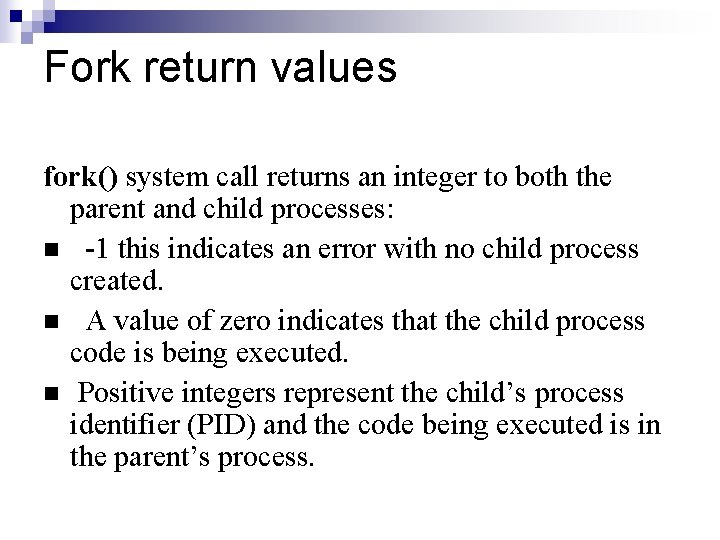
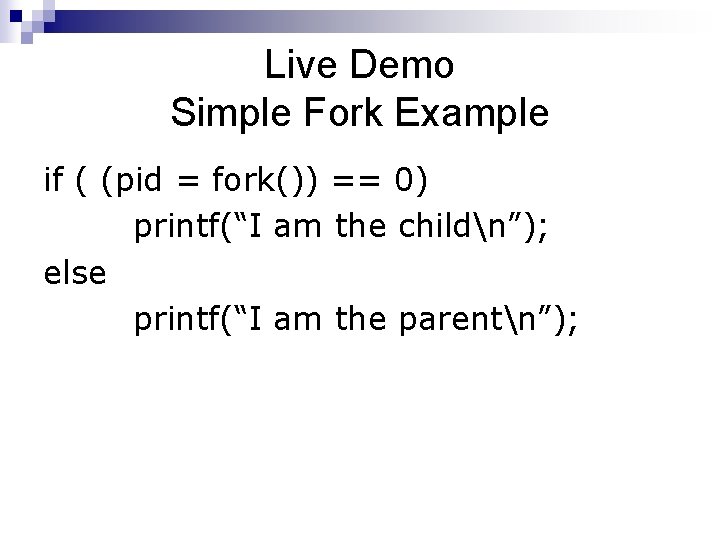
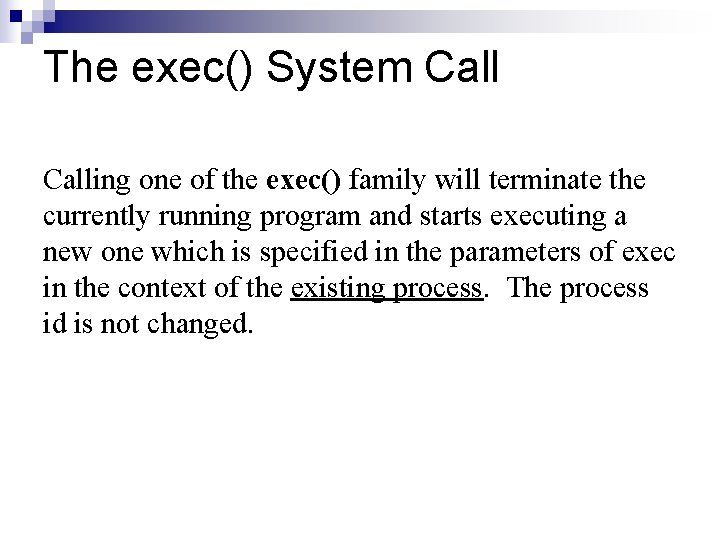
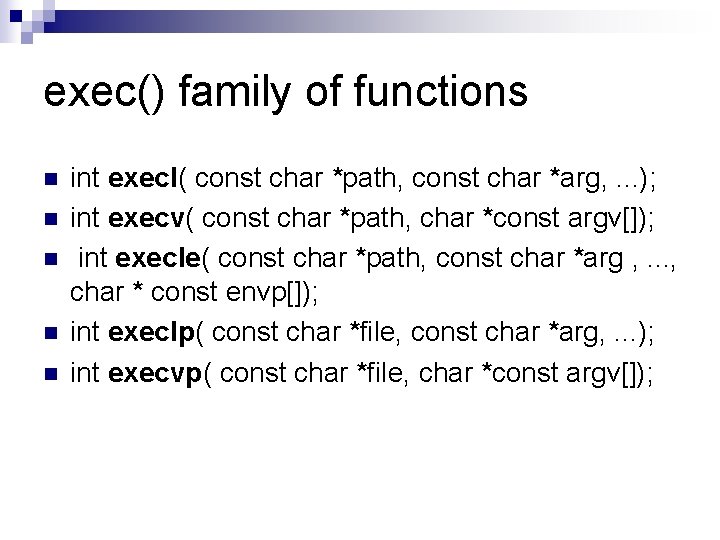
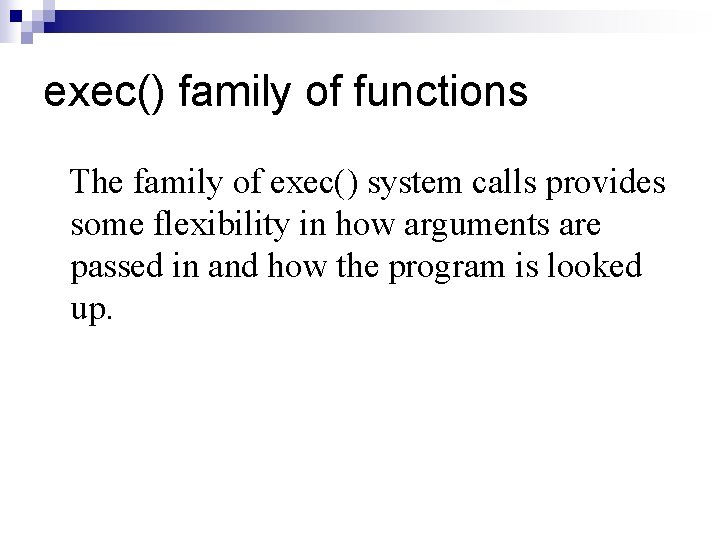
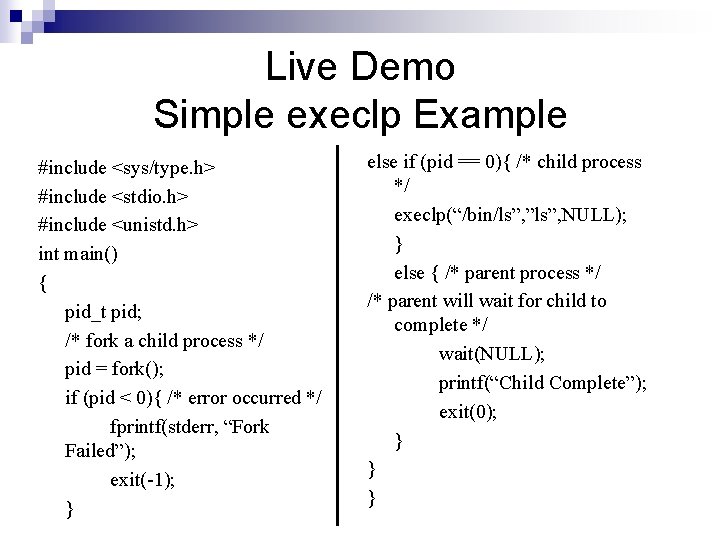
- Slides: 12
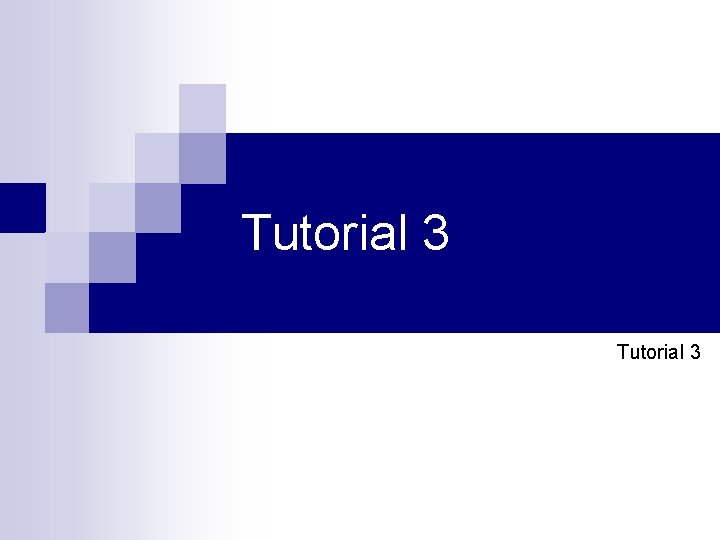
Tutorial 3
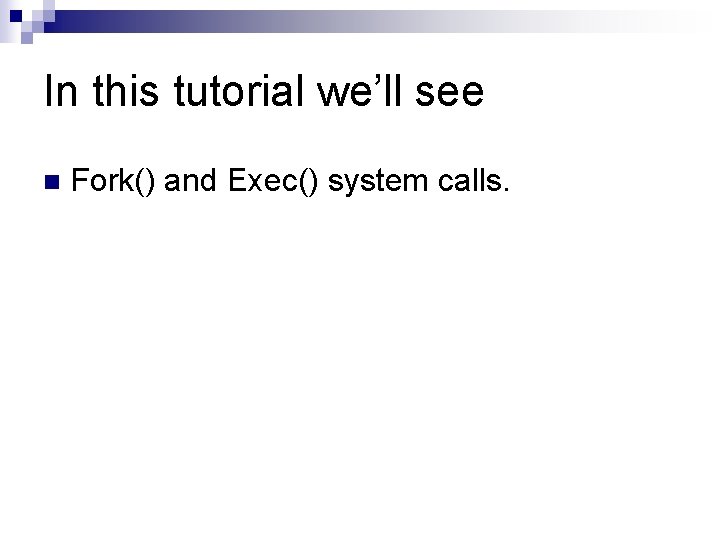
In this tutorial we’ll see n Fork() and Exec() system calls.
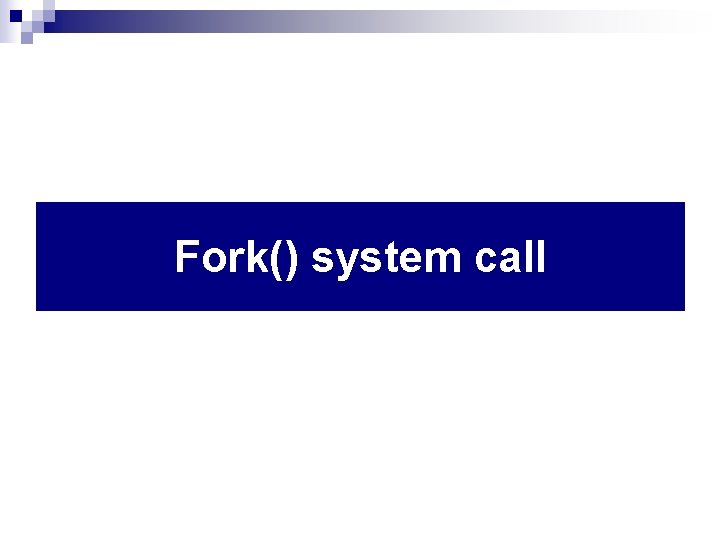
Fork() system call
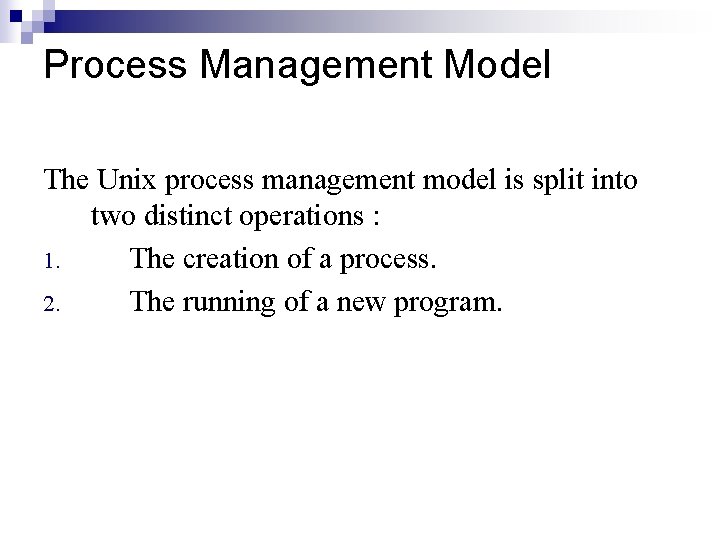
Process Management Model The Unix process management model is split into two distinct operations : 1. The creation of a process. 2. The running of a new program.
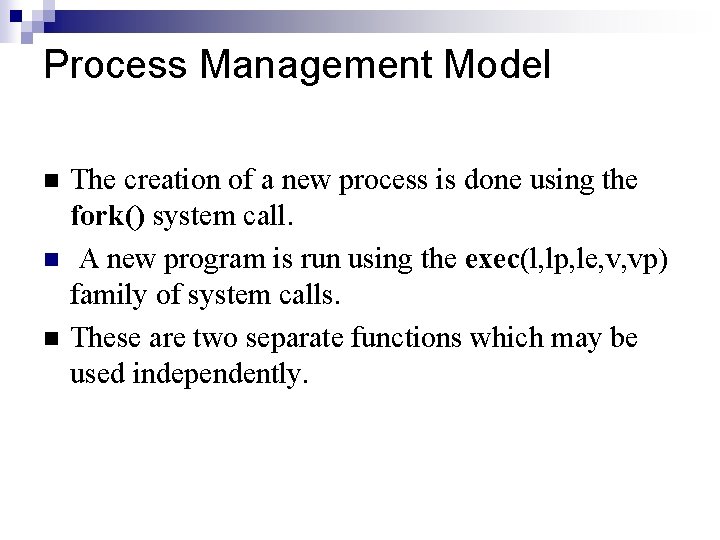
Process Management Model n n n The creation of a new process is done using the fork() system call. A new program is run using the exec(l, lp, le, v, vp) family of system calls. These are two separate functions which may be used independently.
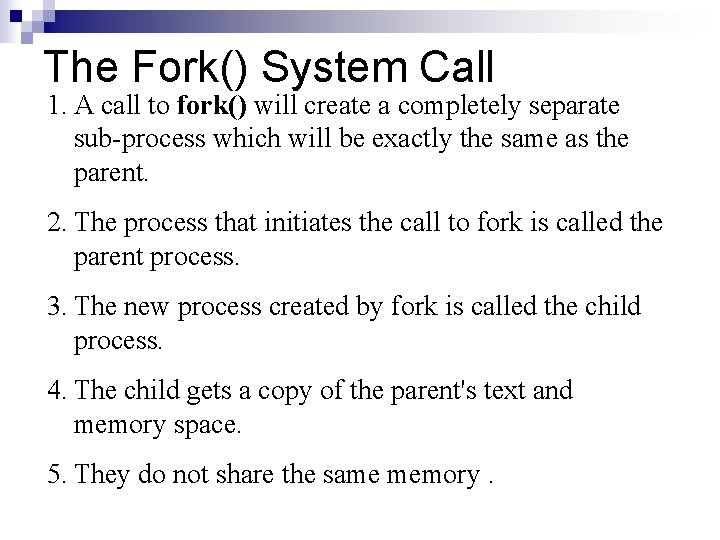
The Fork() System Call 1. A call to fork() will create a completely separate sub-process which will be exactly the same as the parent. 2. The process that initiates the call to fork is called the parent process. 3. The new process created by fork is called the child process. 4. The child gets a copy of the parent's text and memory space. 5. They do not share the same memory.
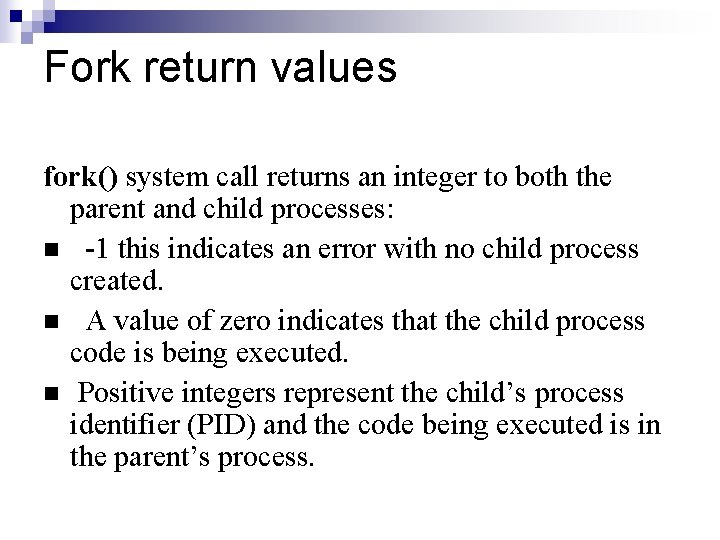
Fork return values fork() system call returns an integer to both the parent and child processes: n -1 this indicates an error with no child process created. n A value of zero indicates that the child process code is being executed. n Positive integers represent the child’s process identifier (PID) and the code being executed is in the parent’s process.
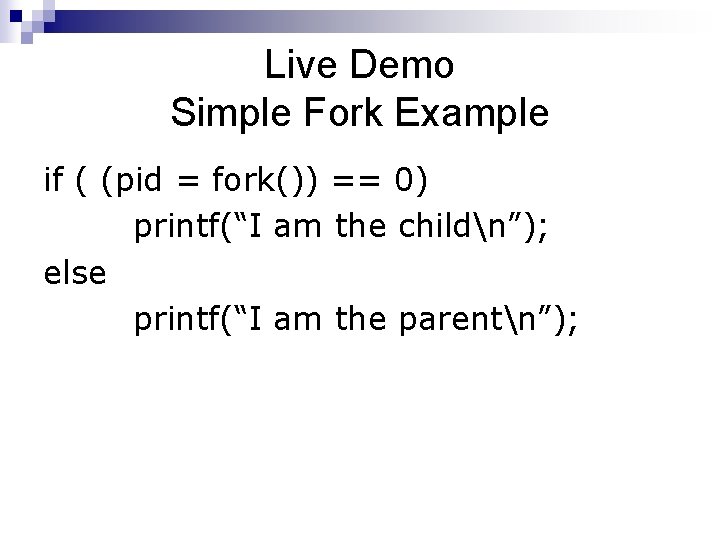
Live Demo Simple Fork Example if ( (pid = fork()) == 0) printf(“I am the childn”); else printf(“I am the parentn”);
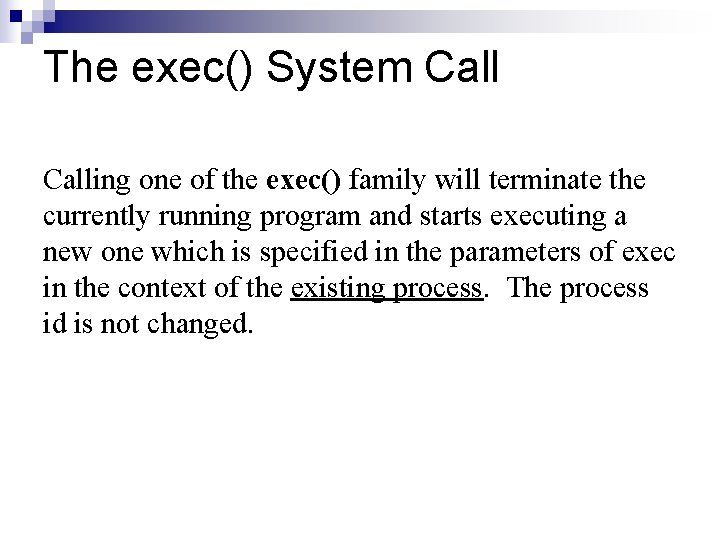
The exec() System Calling one of the exec() family will terminate the currently running program and starts executing a new one which is specified in the parameters of exec in the context of the existing process. The process id is not changed.
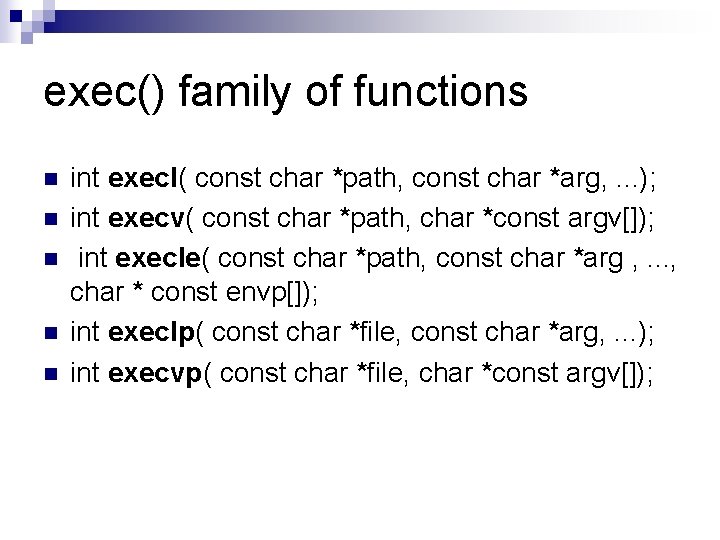
exec() family of functions n n n int execl( const char *path, const char *arg, . . . ); int execv( const char *path, char *const argv[]); int execle( const char *path, const char *arg , . . . , char * const envp[]); int execlp( const char *file, const char *arg, . . . ); int execvp( const char *file, char *const argv[]);
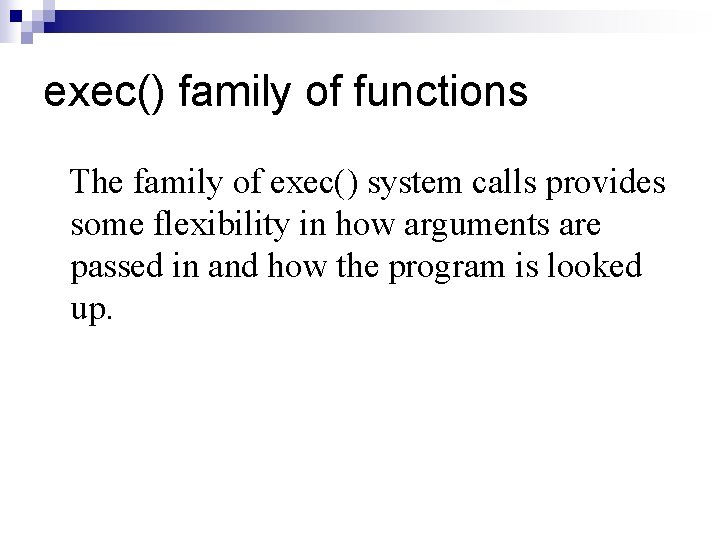
exec() family of functions The family of exec() system calls provides some flexibility in how arguments are passed in and how the program is looked up.
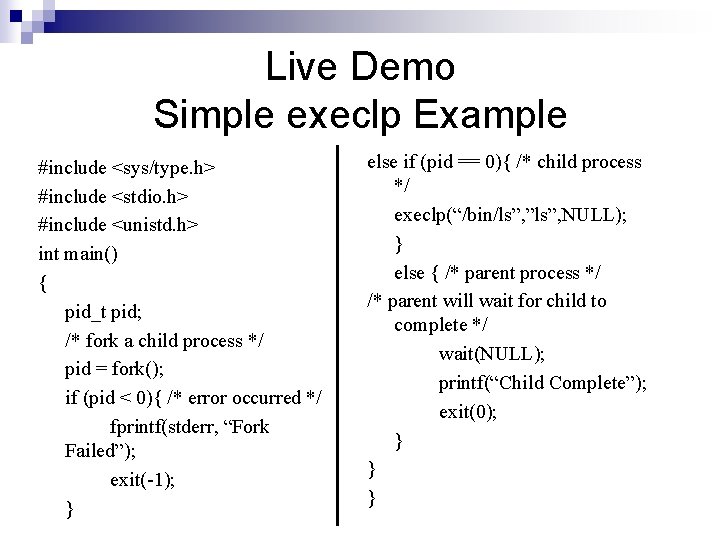
Live Demo Simple execlp Example #include <sys/type. h> #include <stdio. h> #include <unistd. h> int main() { pid_t pid; /* fork a child process */ pid = fork(); if (pid < 0){ /* error occurred */ fprintf(stderr, “Fork Failed”); exit(-1); } else if (pid == 0){ /* child process */ execlp(“/bin/ls”, ”ls”, NULL); } else { /* parent process */ /* parent will wait for child to complete */ wait(NULL); printf(“Child Complete”); exit(0); } } }
The part can never be well
Sanitary well parapet wall
What do you call a collection of well-defined objects
Eat well stay well
"to be happy is to live well and to do well."
Diet for spinal cord injury patient
How to write a conclusio
What microscope is used to see mitochondria
Major prophets
Nahrungspyramide see
Verb of identical
What comes to your mind when you see this logo?
How do you know that beowulf is an honorable man?