TUPLES IN PYTHON Python Tuples A tuple is
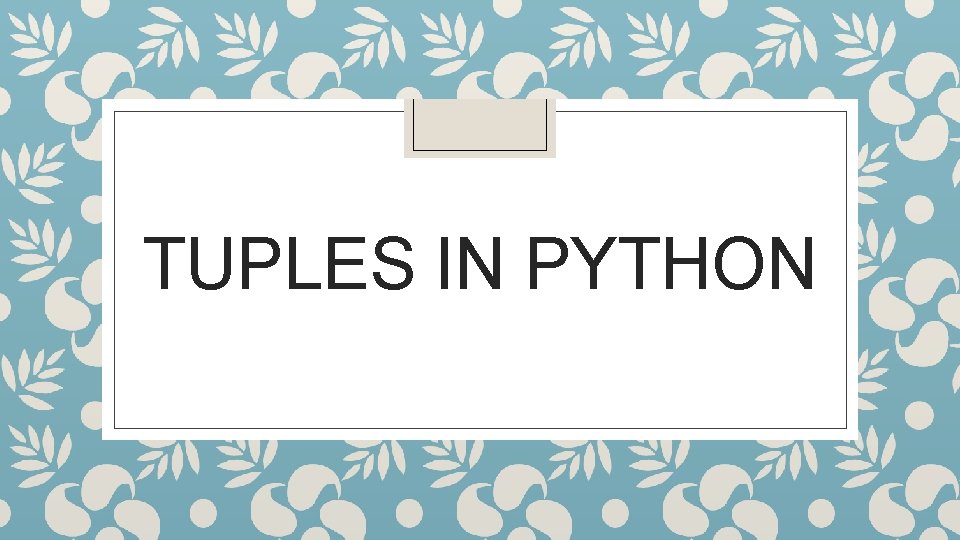
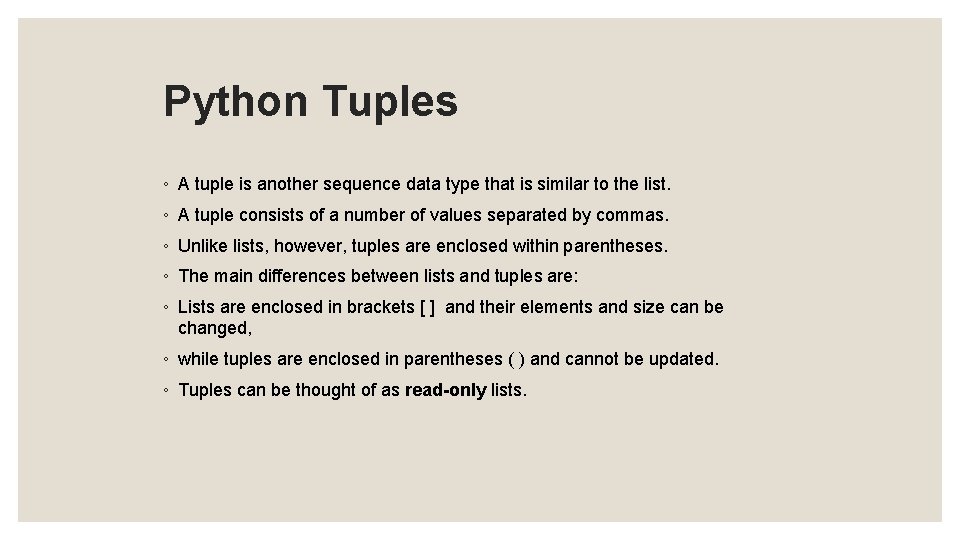
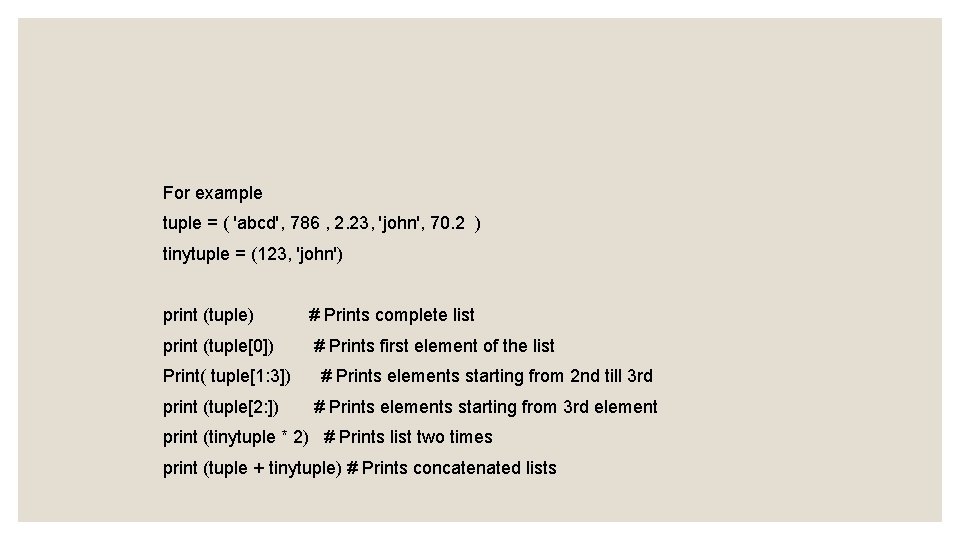
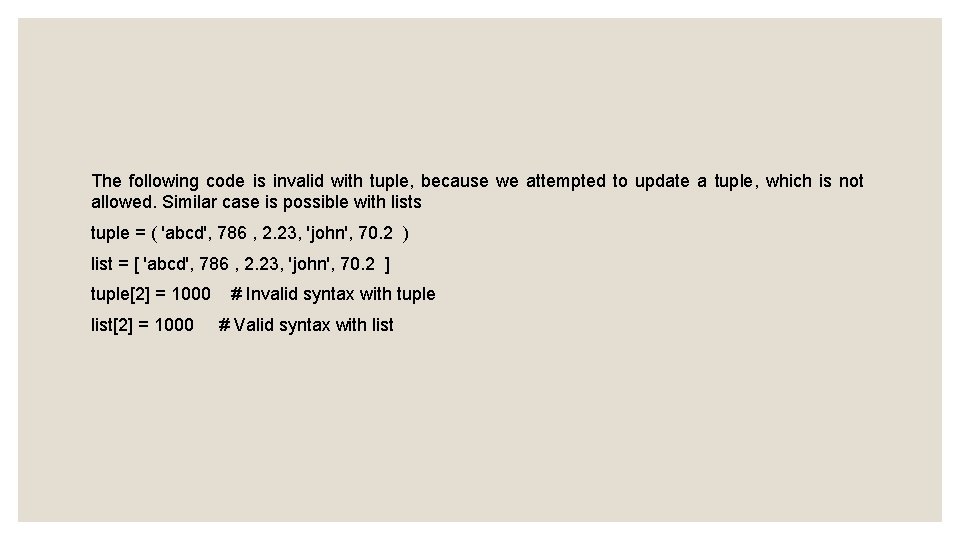
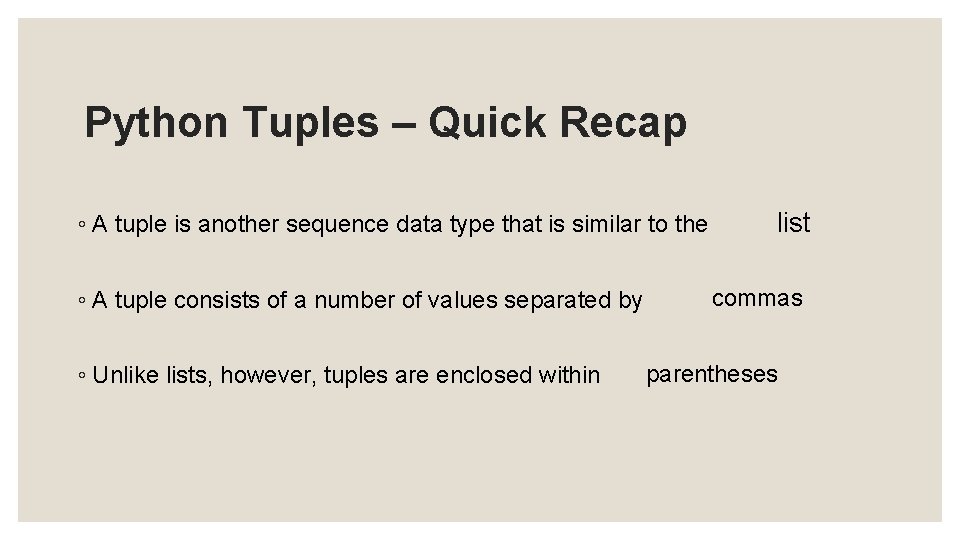
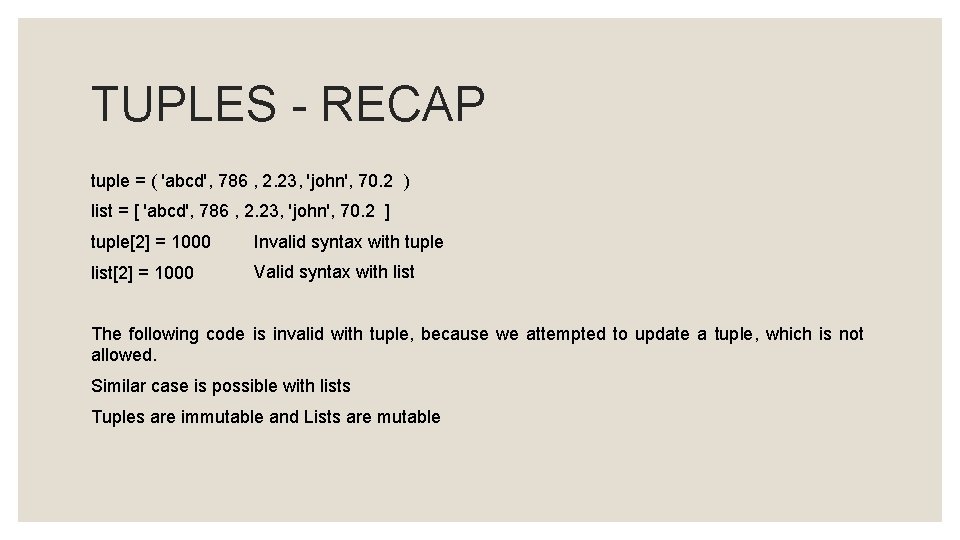
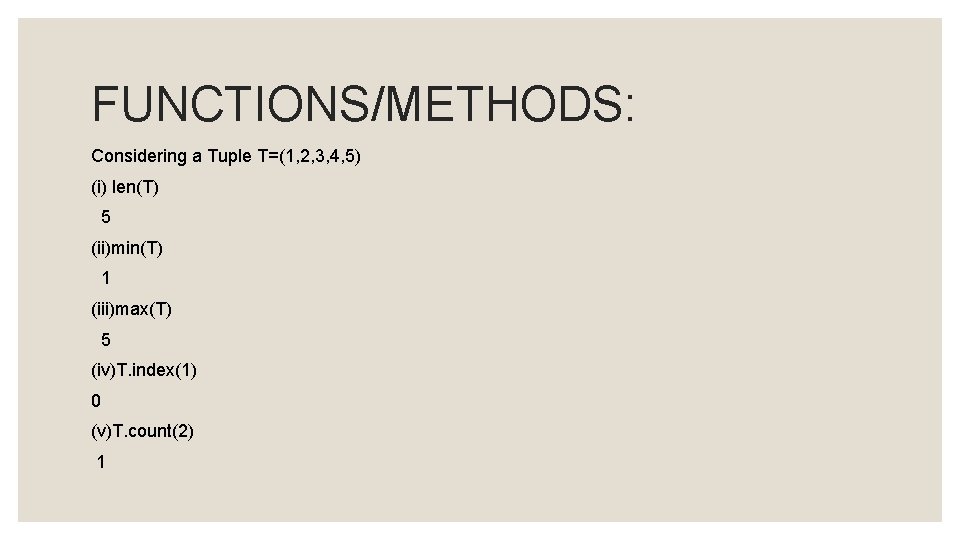
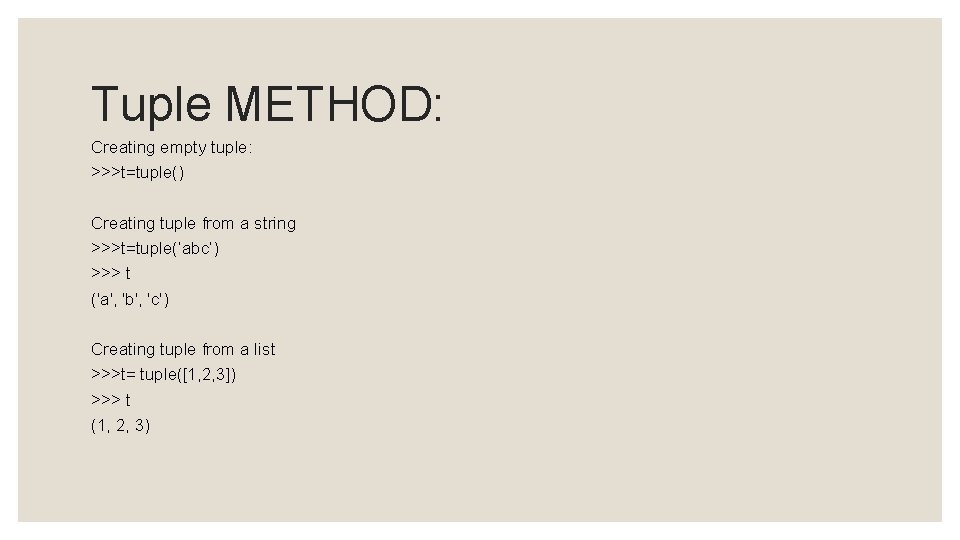
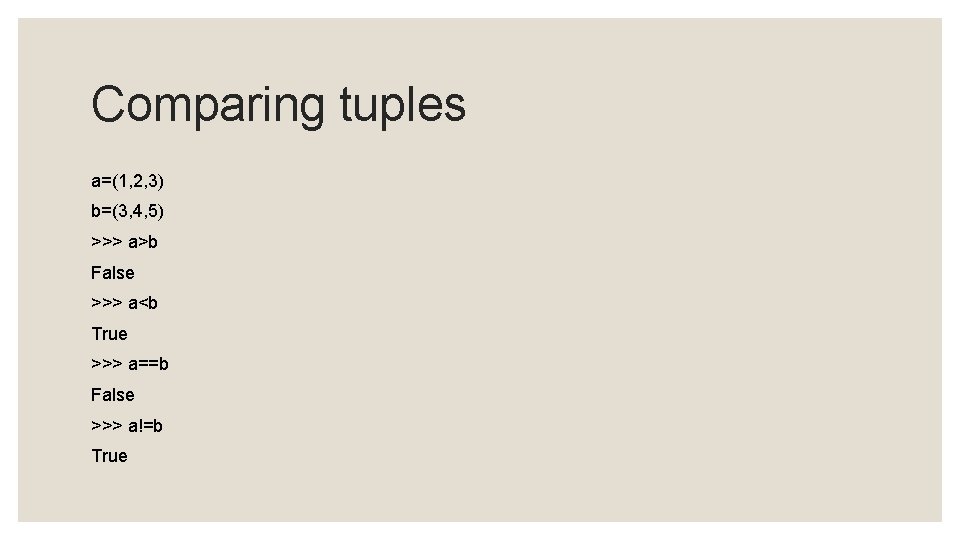
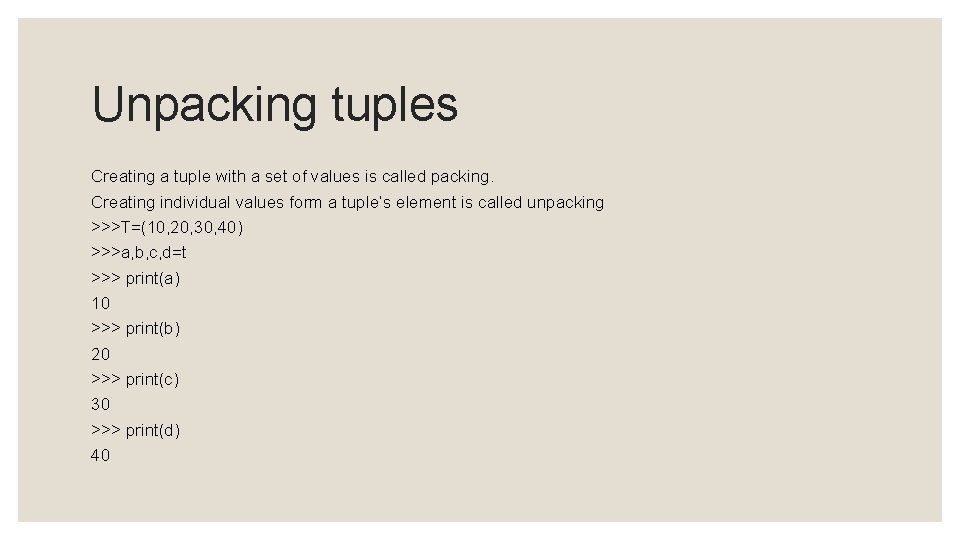
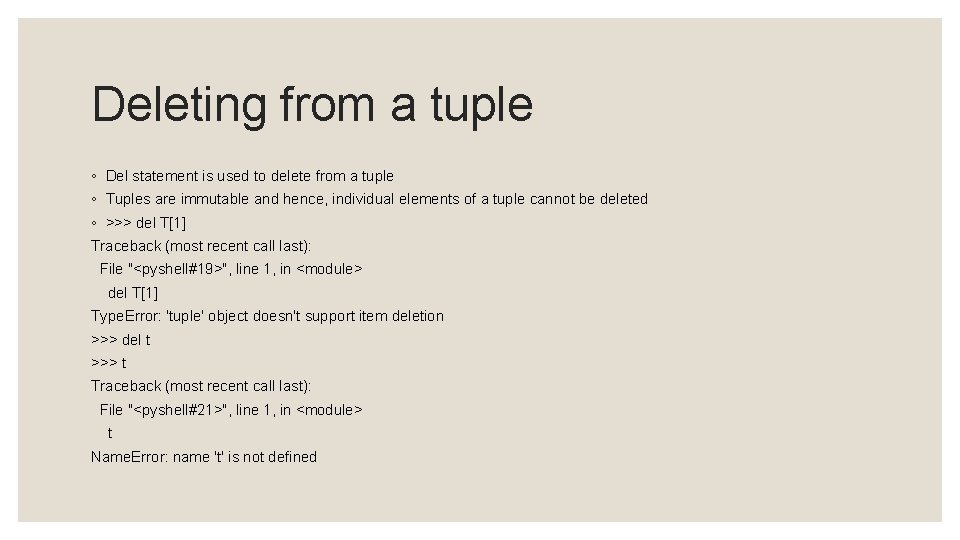
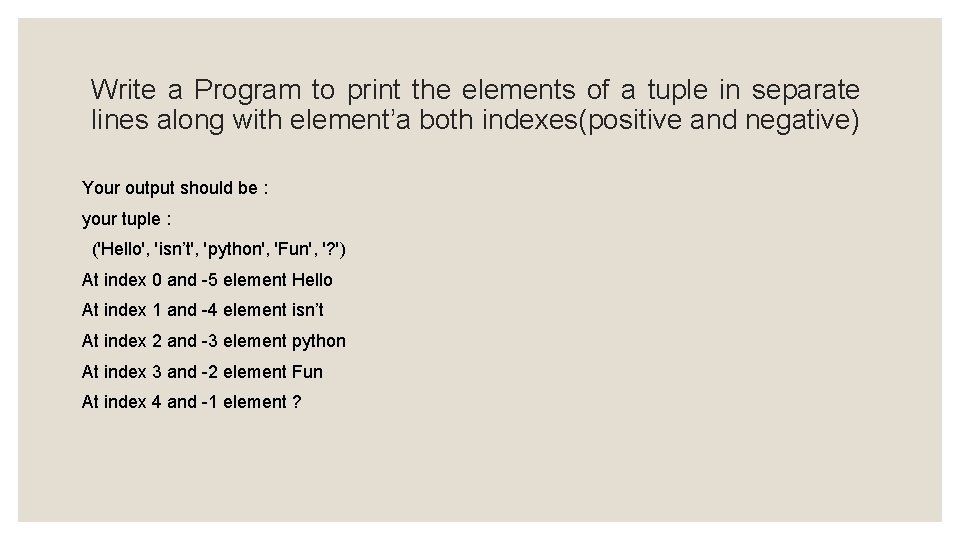
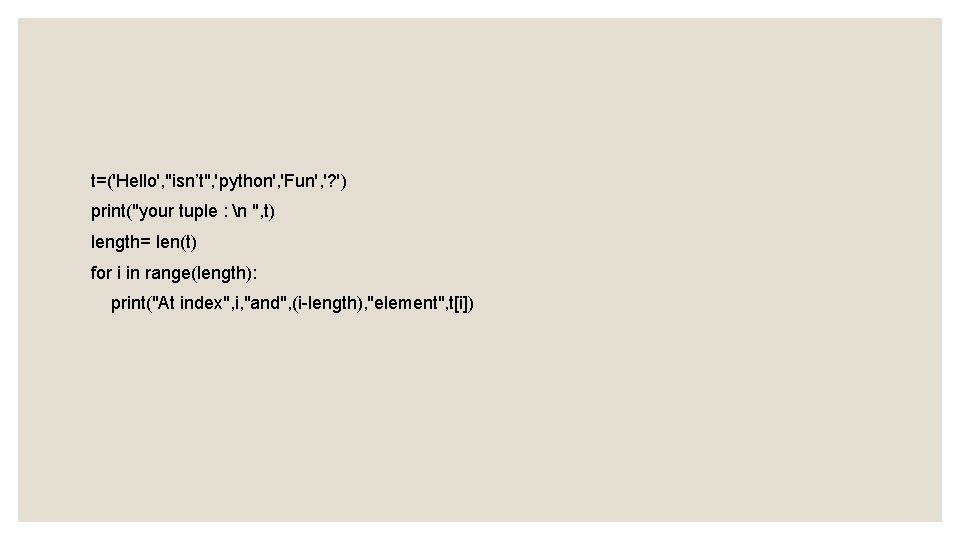
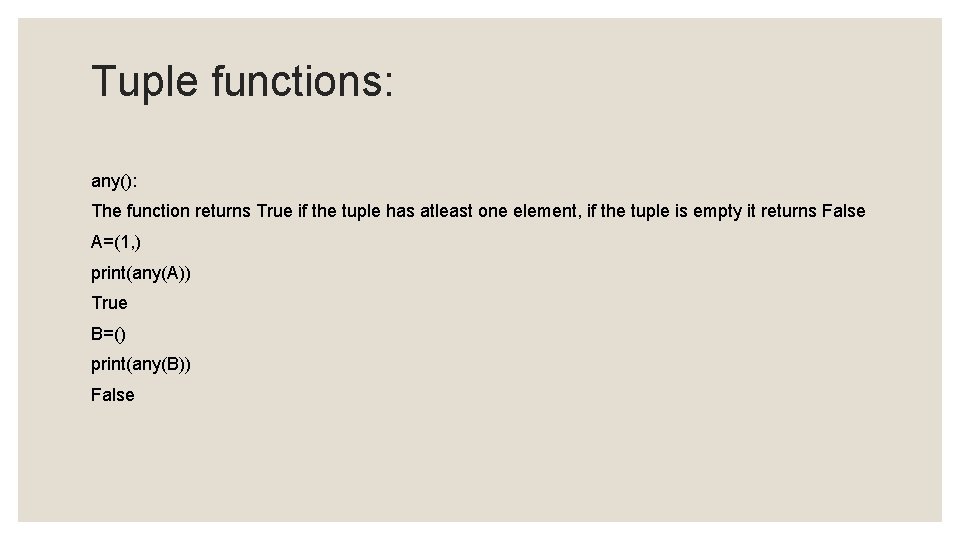
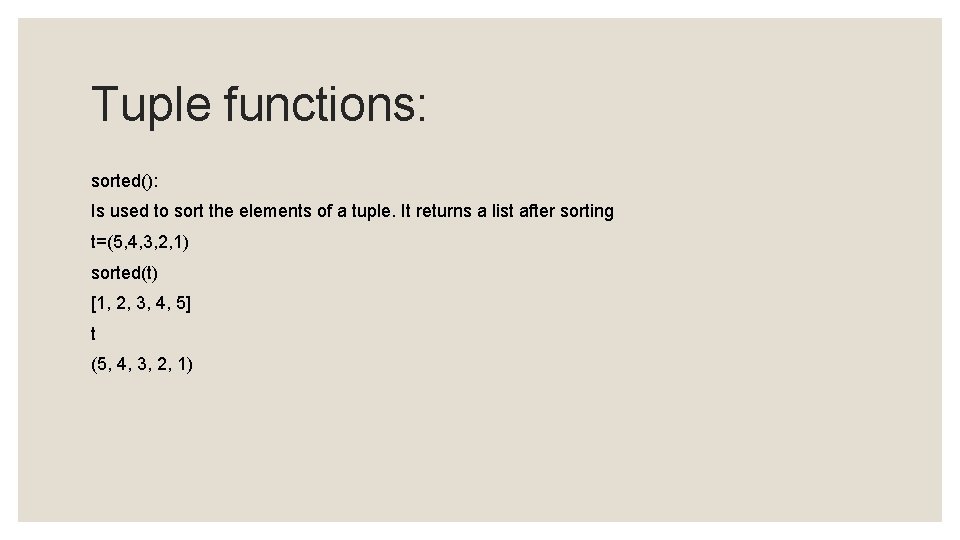
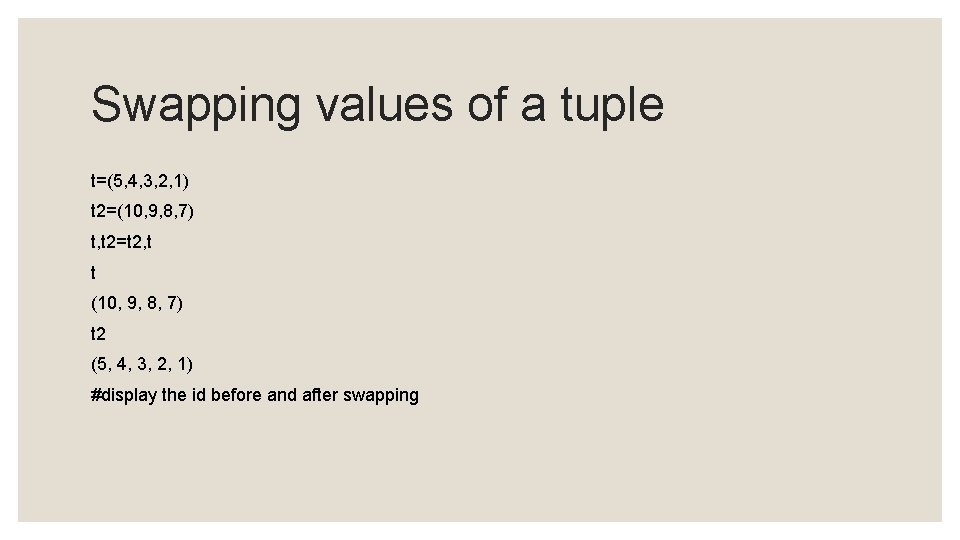
- Slides: 16
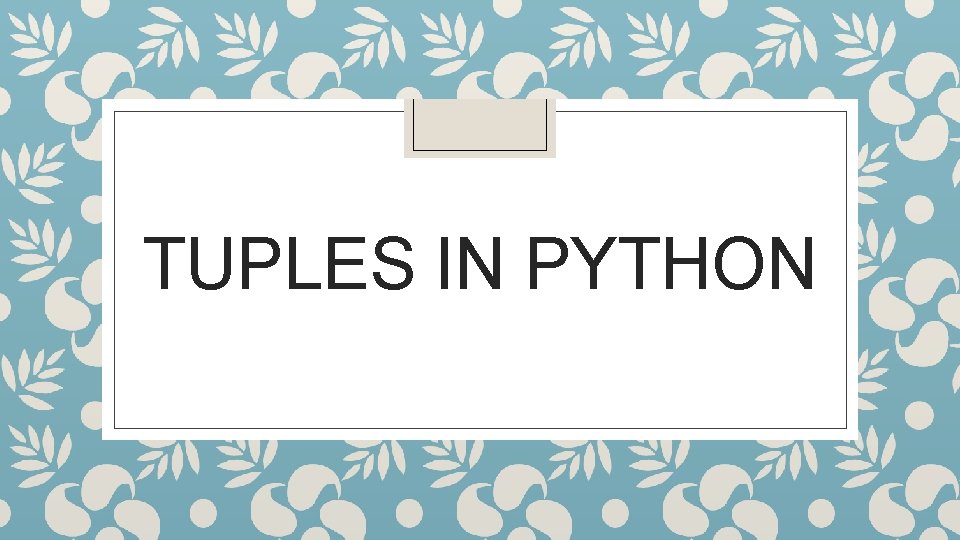
TUPLES IN PYTHON
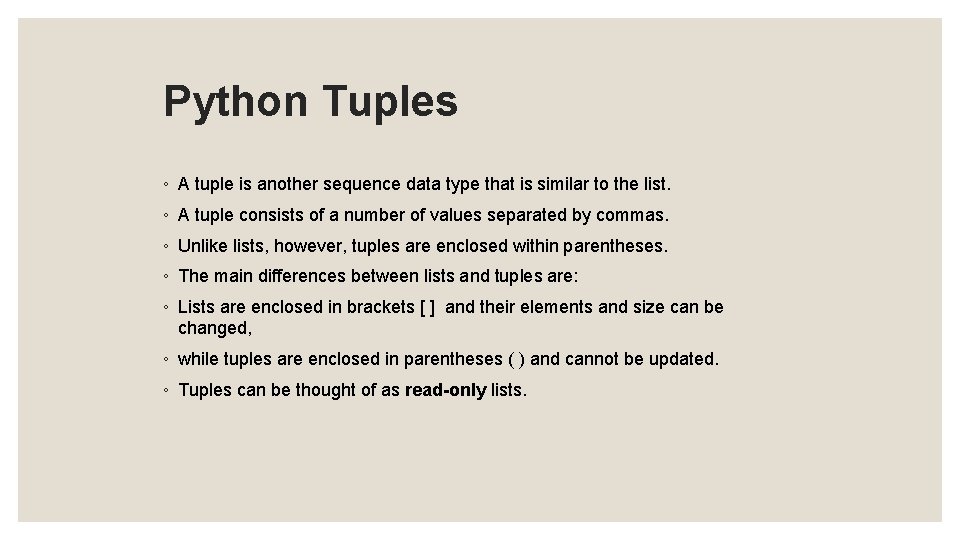
Python Tuples ◦ A tuple is another sequence data type that is similar to the list. ◦ A tuple consists of a number of values separated by commas. ◦ Unlike lists, however, tuples are enclosed within parentheses. ◦ The main differences between lists and tuples are: ◦ Lists are enclosed in brackets [ ] and their elements and size can be changed, ◦ while tuples are enclosed in parentheses ( ) and cannot be updated. ◦ Tuples can be thought of as read-only lists.
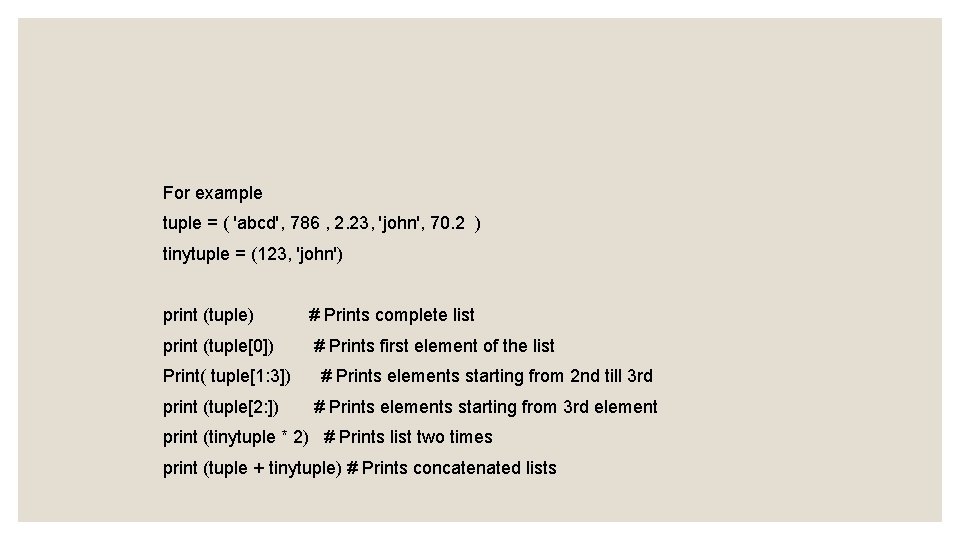
For example tuple = ( 'abcd', 786 , 2. 23, 'john', 70. 2 ) tinytuple = (123, 'john') print (tuple) # Prints complete list print (tuple[0]) # Prints first element of the list Print( tuple[1: 3]) print (tuple[2: ]) # Prints elements starting from 2 nd till 3 rd # Prints elements starting from 3 rd element print (tinytuple * 2) # Prints list two times print (tuple + tinytuple) # Prints concatenated lists
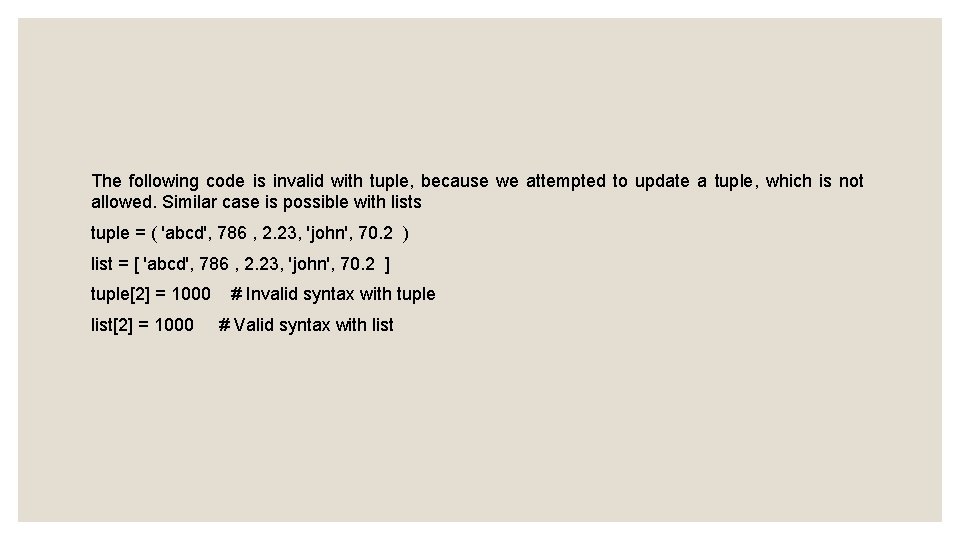
The following code is invalid with tuple, because we attempted to update a tuple, which is not allowed. Similar case is possible with lists tuple = ( 'abcd', 786 , 2. 23, 'john', 70. 2 ) list = [ 'abcd', 786 , 2. 23, 'john', 70. 2 ] tuple[2] = 1000 list[2] = 1000 # Invalid syntax with tuple # Valid syntax with list
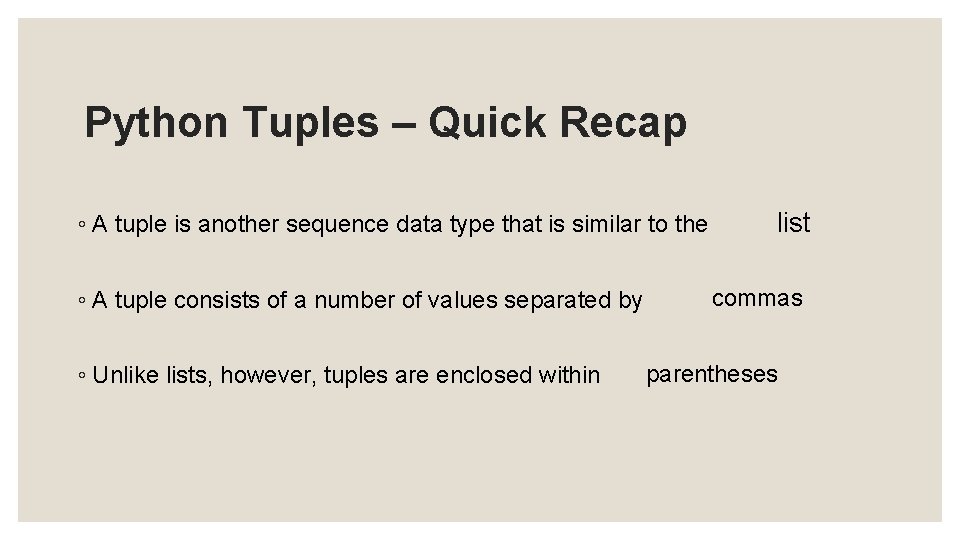
Python Tuples – Quick Recap ◦ A tuple is another sequence data type that is similar to the ◦ A tuple consists of a number of values separated by ◦ Unlike lists, however, tuples are enclosed within list commas parentheses
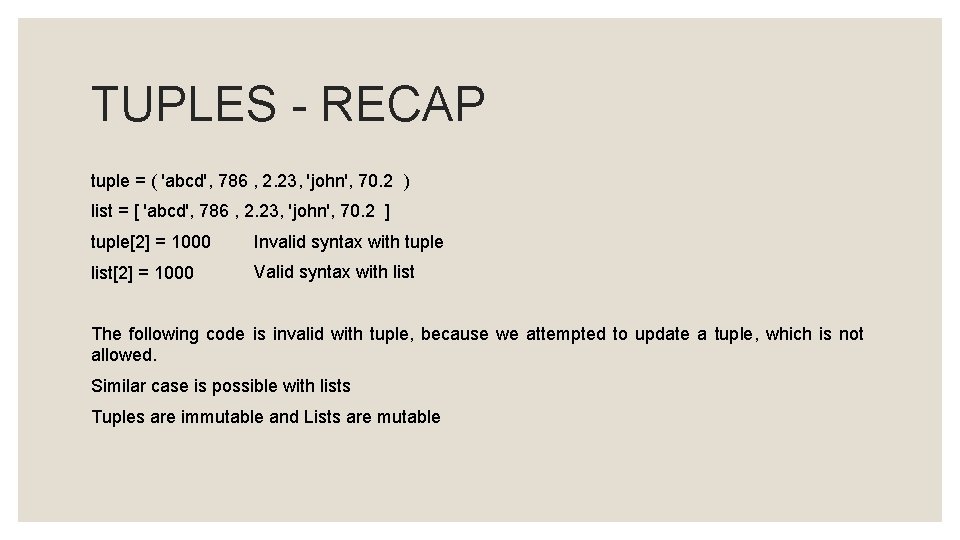
TUPLES - RECAP tuple = ( 'abcd', 786 , 2. 23, 'john', 70. 2 ) list = [ 'abcd', 786 , 2. 23, 'john', 70. 2 ] tuple[2] = 1000 Invalid syntax with tuple list[2] = 1000 Valid syntax with list The following code is invalid with tuple, because we attempted to update a tuple, which is not allowed. Similar case is possible with lists Tuples are immutable and Lists are mutable
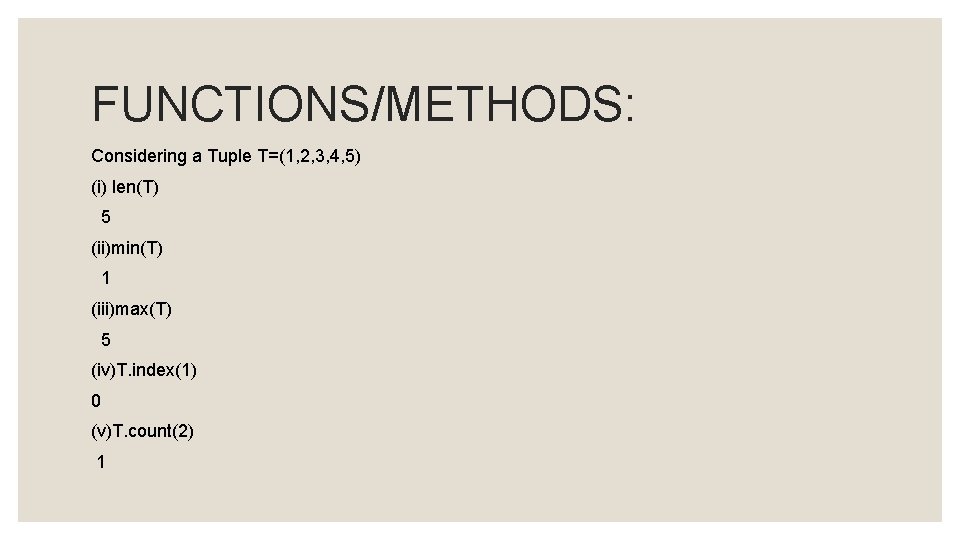
FUNCTIONS/METHODS: Considering a Tuple T=(1, 2, 3, 4, 5) (i) len(T) 5 (ii)min(T) 1 (iii)max(T) 5 (iv)T. index(1) 0 (v)T. count(2) 1
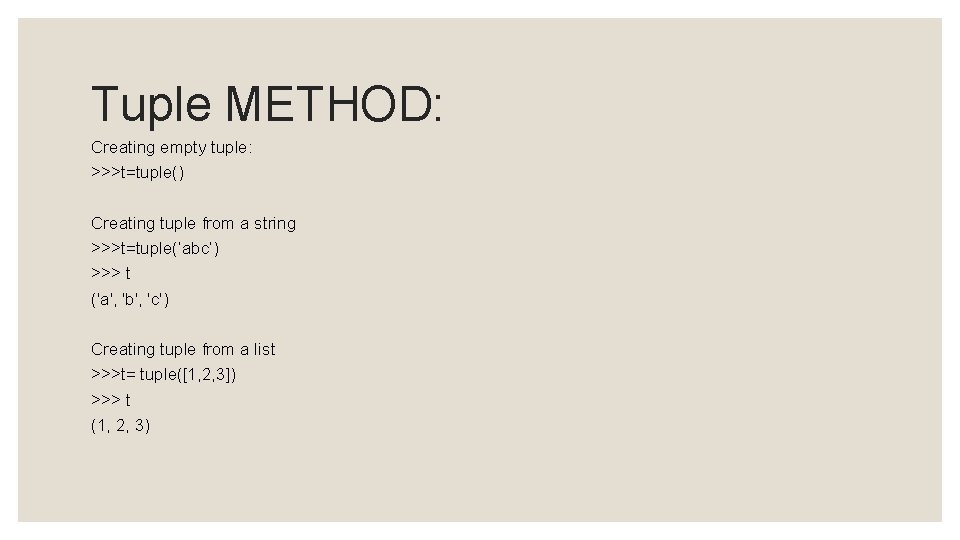
Tuple METHOD: Creating empty tuple: >>>t=tuple() Creating tuple from a string >>>t=tuple(‘abc’) >>> t ('a', 'b', 'c') Creating tuple from a list >>>t= tuple([1, 2, 3]) >>> t (1, 2, 3)
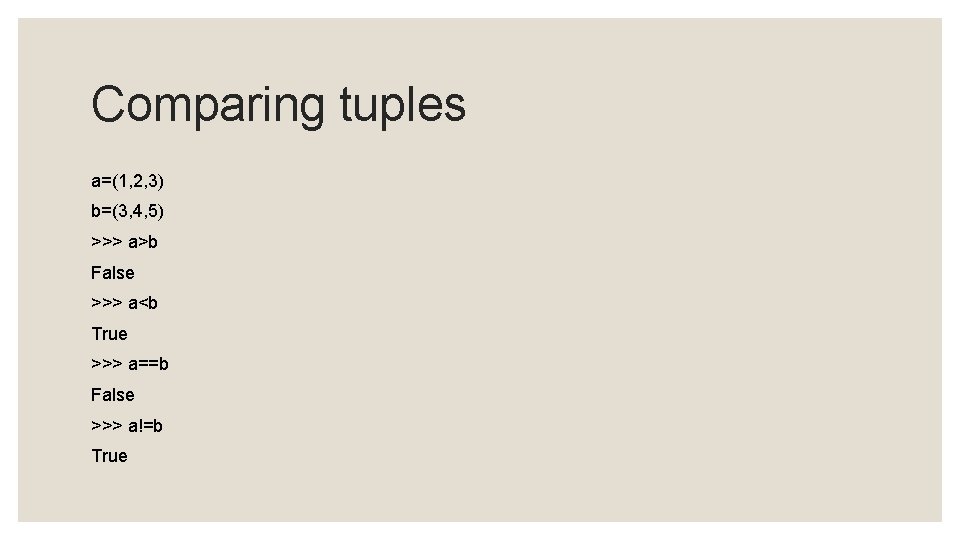
Comparing tuples a=(1, 2, 3) b=(3, 4, 5) >>> a>b False >>> a<b True >>> a==b False >>> a!=b True
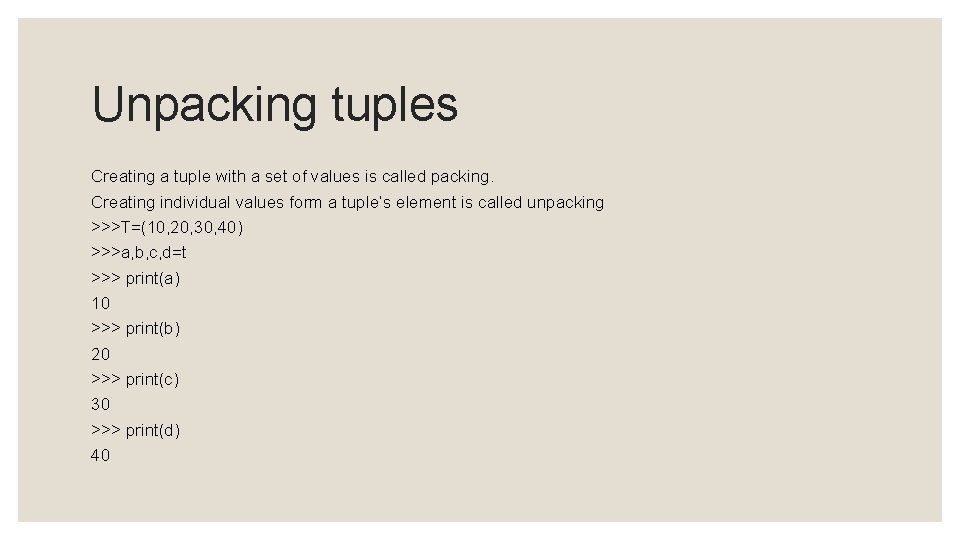
Unpacking tuples Creating a tuple with a set of values is called packing. Creating individual values form a tuple’s element is called unpacking >>>T=(10, 20, 30, 40) >>>a, b, c, d=t >>> print(a) 10 >>> print(b) 20 >>> print(c) 30 >>> print(d) 40
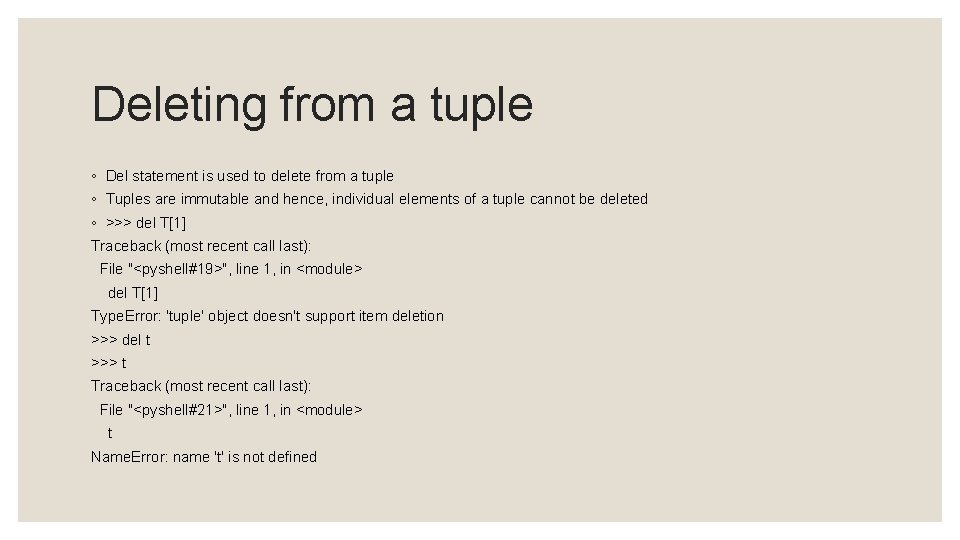
Deleting from a tuple ◦ Del statement is used to delete from a tuple ◦ Tuples are immutable and hence, individual elements of a tuple cannot be deleted ◦ >>> del T[1] Traceback (most recent call last): File "<pyshell#19>", line 1, in <module> del T[1] Type. Error: 'tuple' object doesn't support item deletion >>> del t >>> t Traceback (most recent call last): File "<pyshell#21>", line 1, in <module> t Name. Error: name 't' is not defined
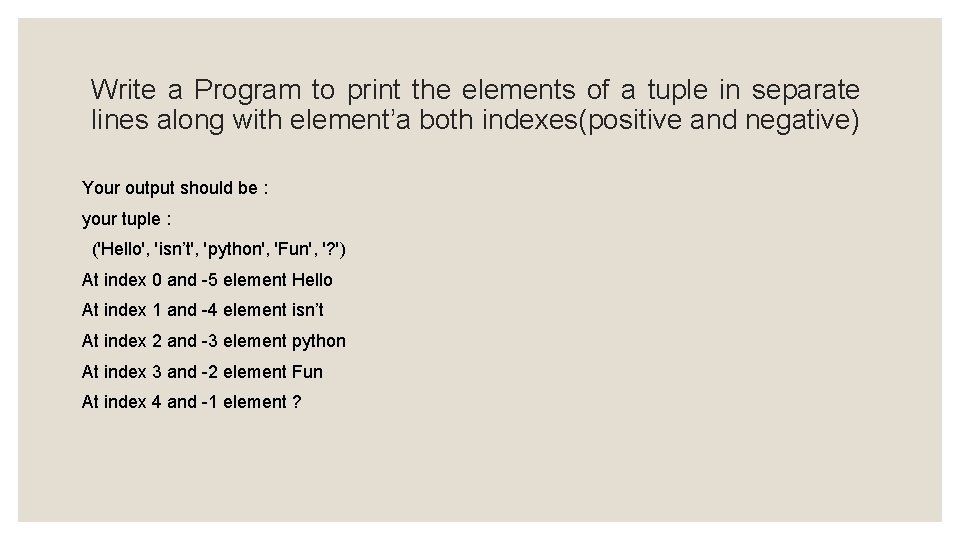
Write a Program to print the elements of a tuple in separate lines along with element’a both indexes(positive and negative) Your output should be : your tuple : ('Hello', 'isn’t', 'python', 'Fun', '? ') At index 0 and -5 element Hello At index 1 and -4 element isn’t At index 2 and -3 element python At index 3 and -2 element Fun At index 4 and -1 element ?
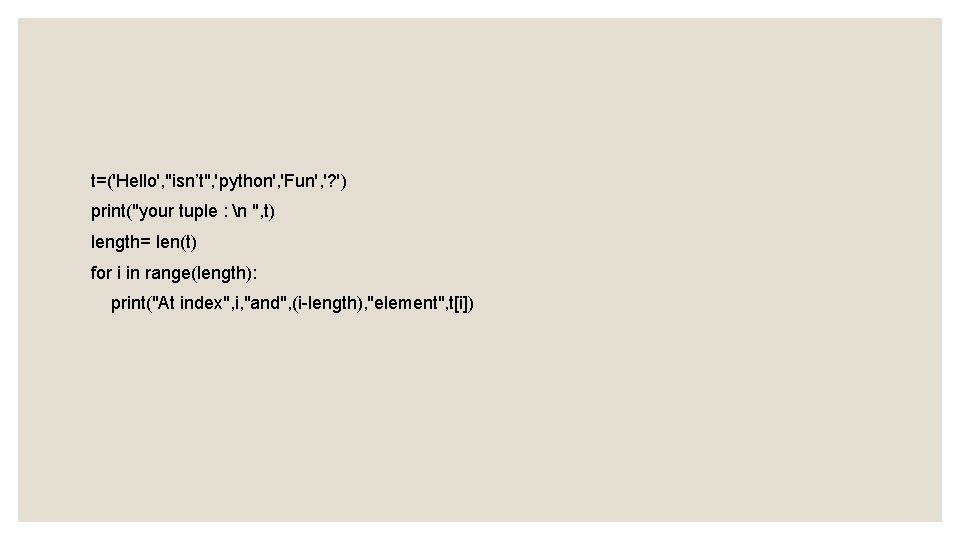
t=('Hello', "isn’t", 'python', 'Fun', '? ') print("your tuple : n ", t) length= len(t) for i in range(length): print("At index", i, "and", (i-length), "element", t[i])
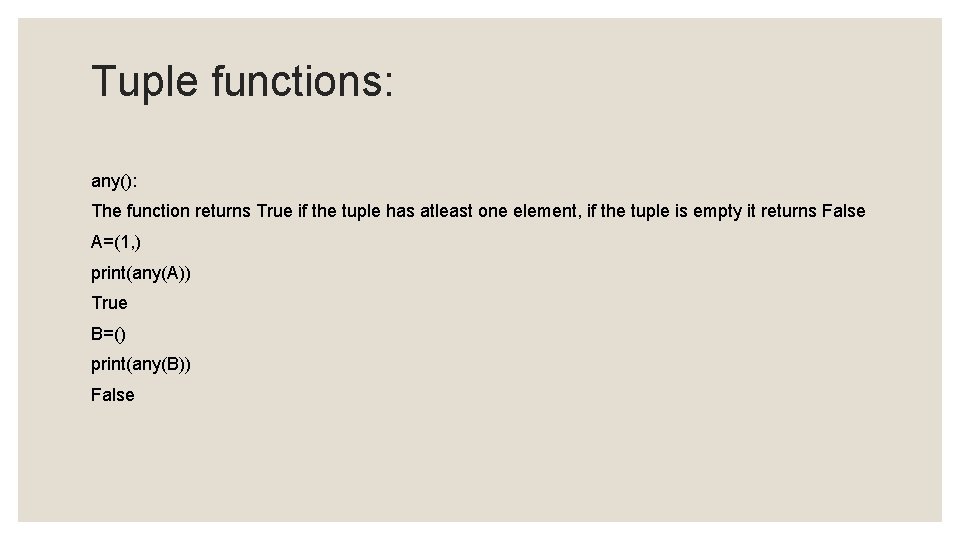
Tuple functions: any(): The function returns True if the tuple has atleast one element, if the tuple is empty it returns False A=(1, ) print(any(A)) True B=() print(any(B)) False
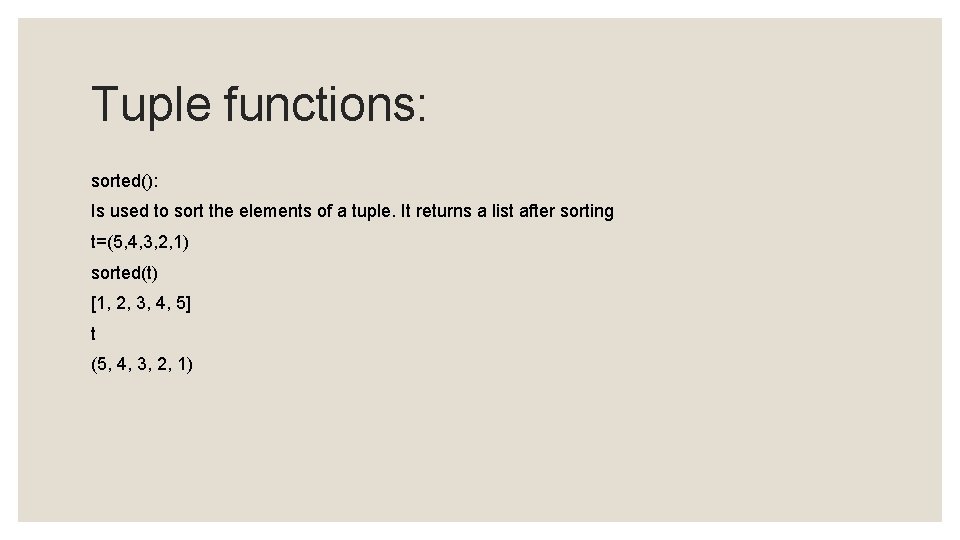
Tuple functions: sorted(): Is used to sort the elements of a tuple. It returns a list after sorting t=(5, 4, 3, 2, 1) sorted(t) [1, 2, 3, 4, 5] t (5, 4, 3, 2, 1)
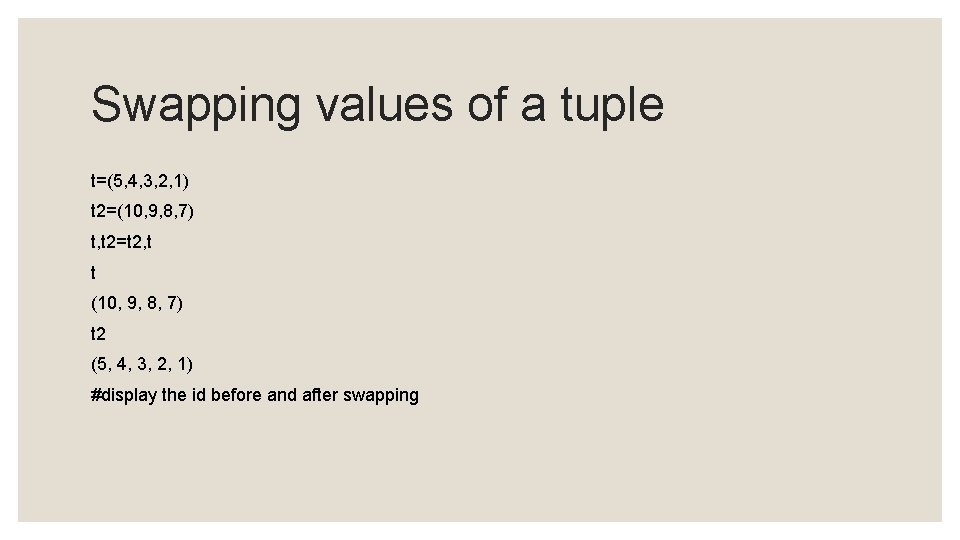
Swapping values of a tuple t=(5, 4, 3, 2, 1) t 2=(10, 9, 8, 7) t, t 2=t 2, t t (10, 9, 8, 7) t 2 (5, 4, 3, 2, 1) #display the id before and after swapping
Difference between list and tuple in python
Python sort list of tuples
Lists of tuples python
Relational calculus
Universal computational device
5 tuple
Markov decision process merupakan tuple dari
Tuple based check constraints
Prolog tuples
Kepan harris benedict
Sürekli infüzyon enteral beslenme
Tuple and domain calculus collectively known as
Tuple meaning
Robustness of turing machine
Turing machine 7 tuple
Tuple relational calculus
Relational algebra to tuple relational calculus