TUPLES Module 11 1 COP 4020 Programming Language
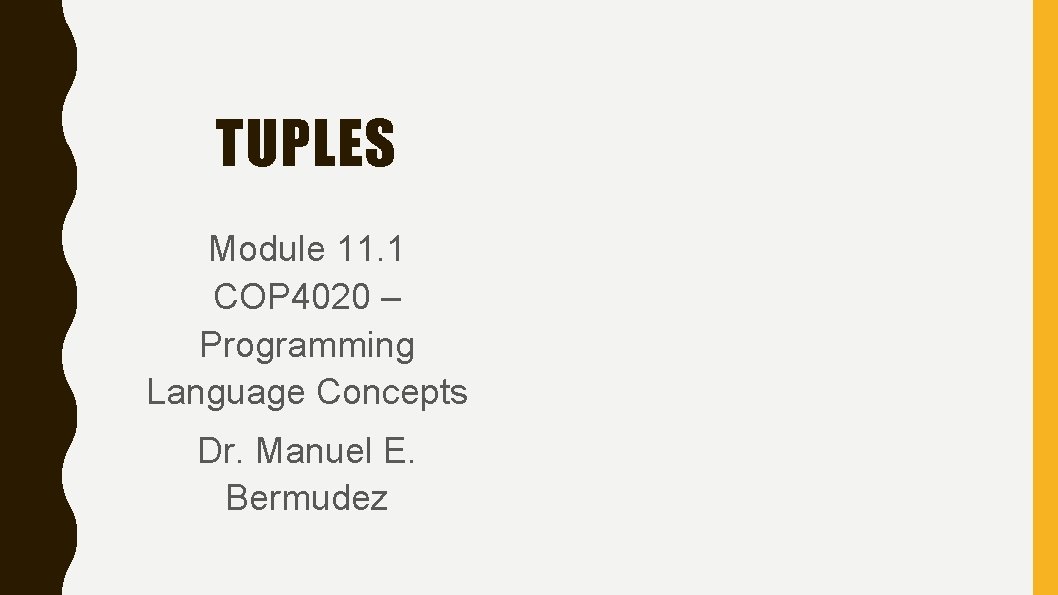
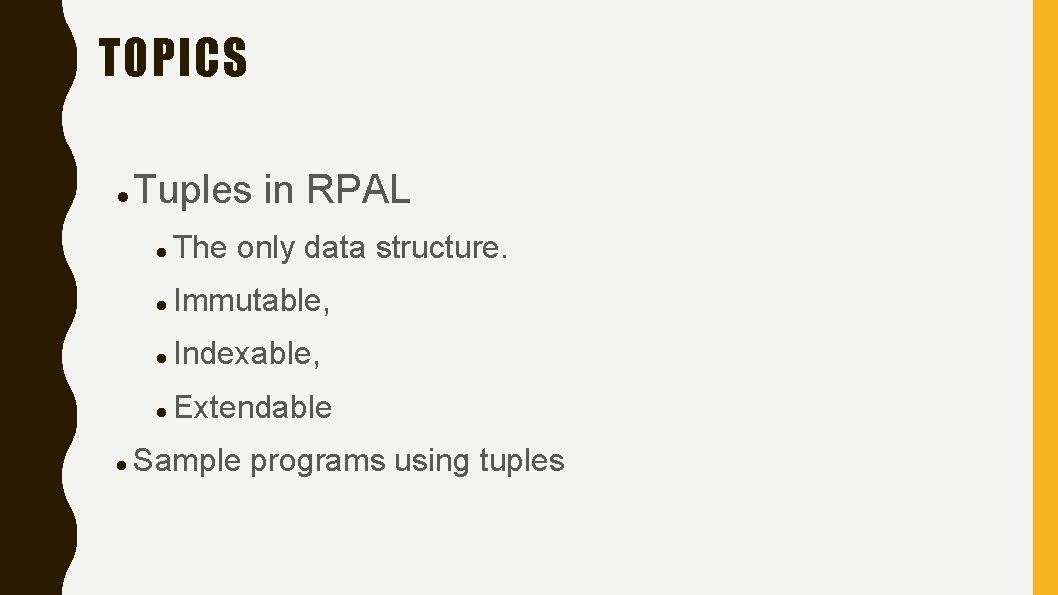
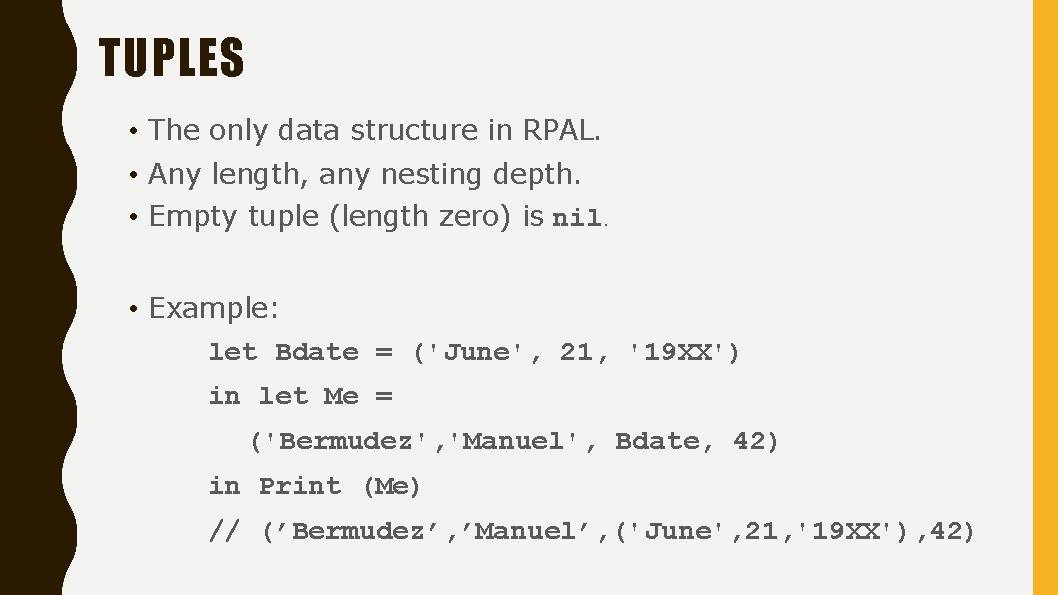
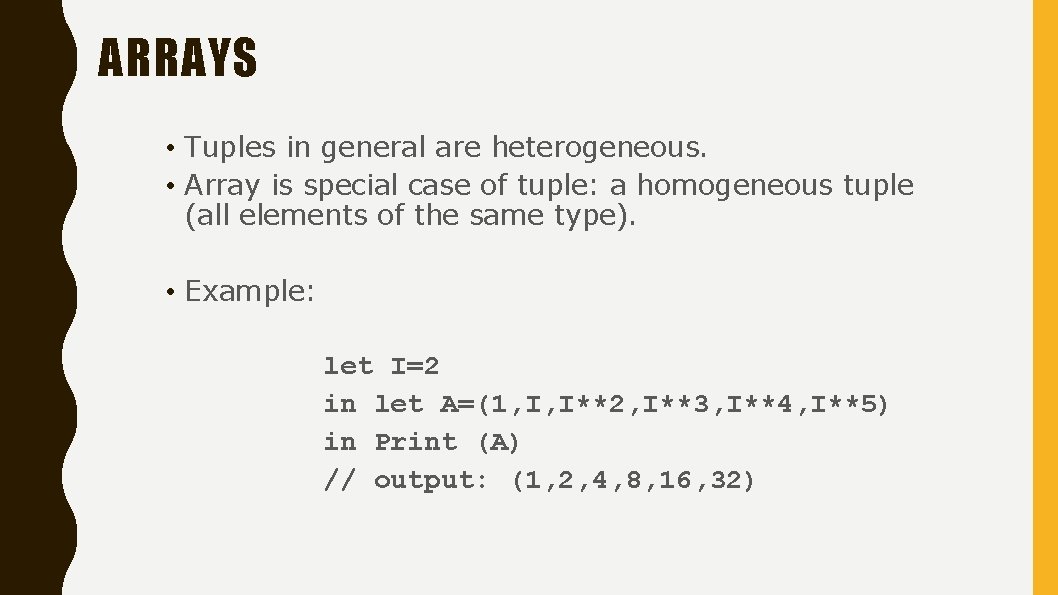
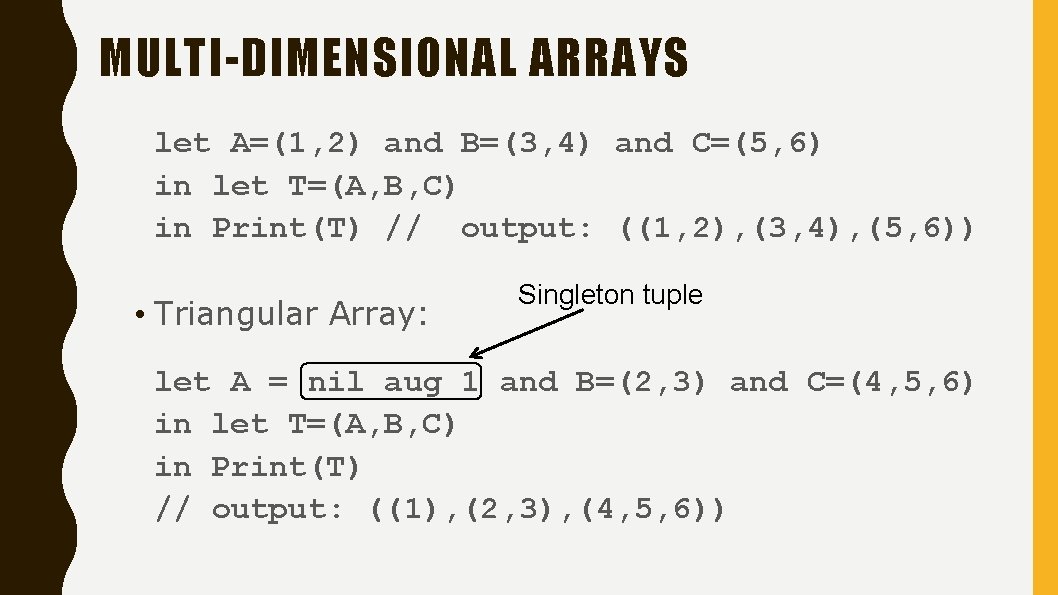
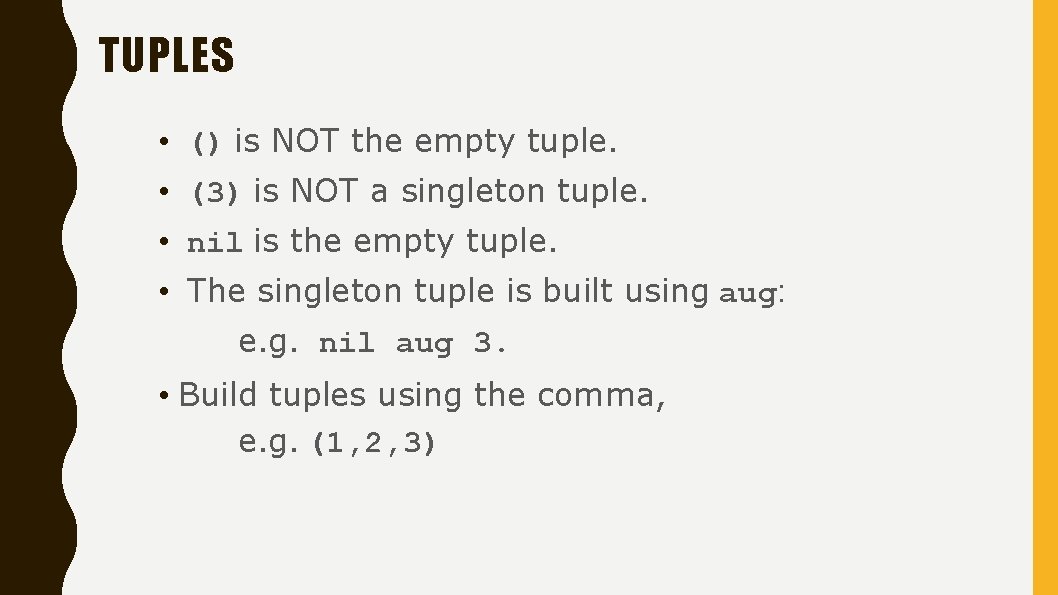
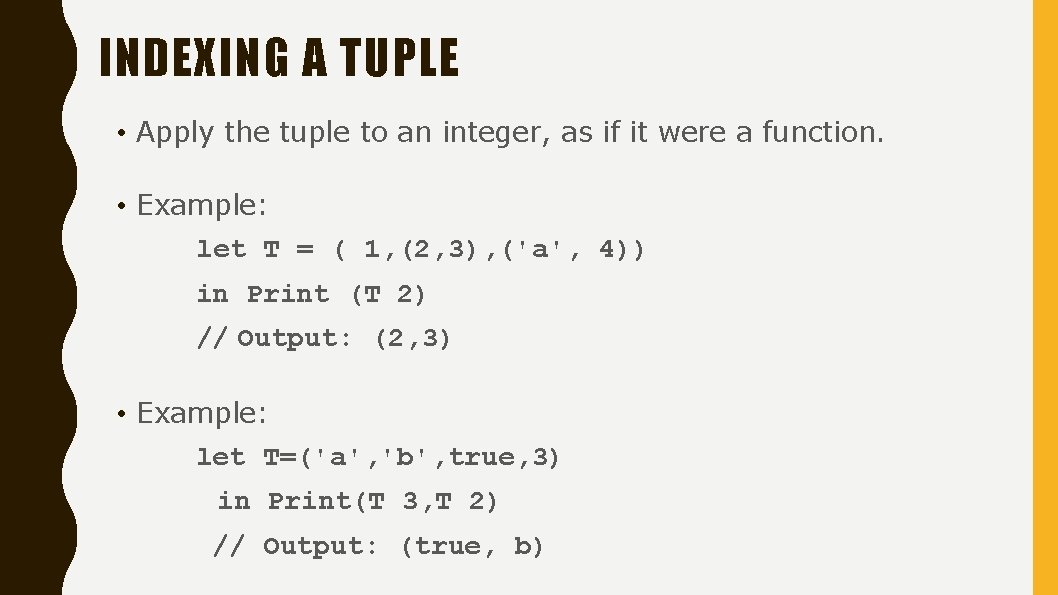
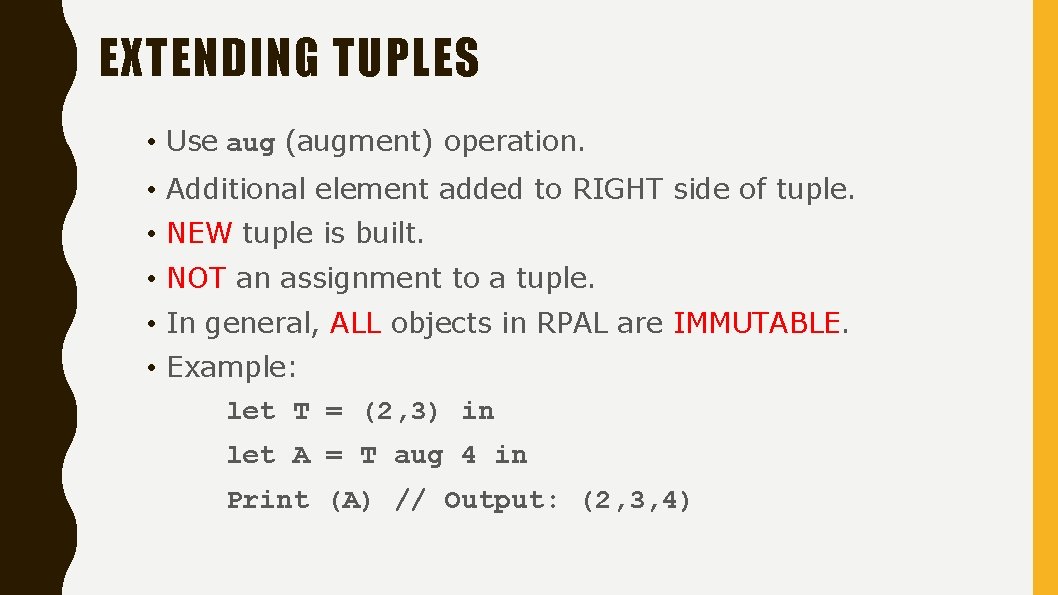
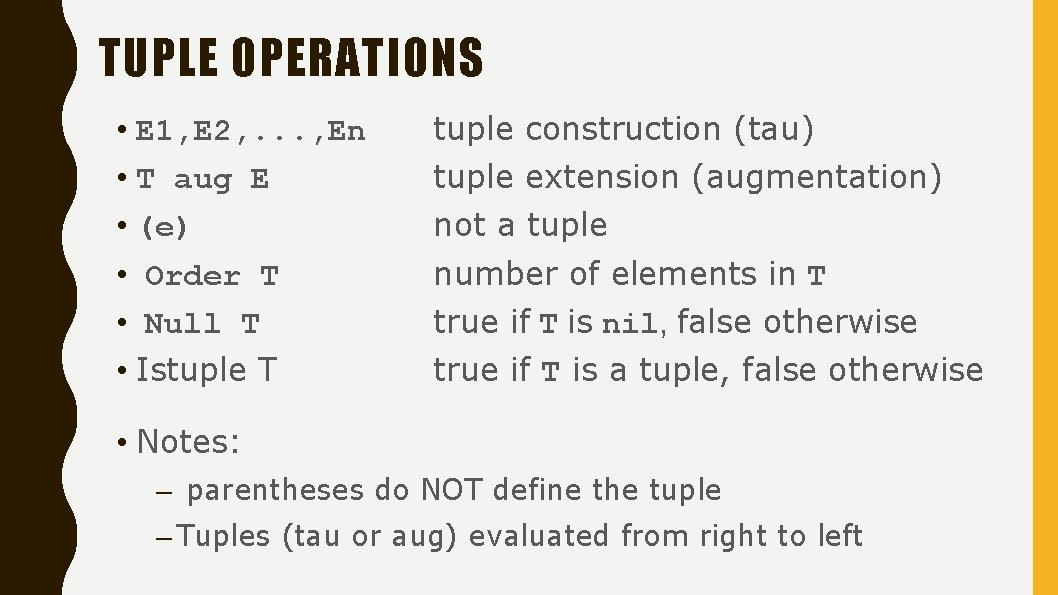
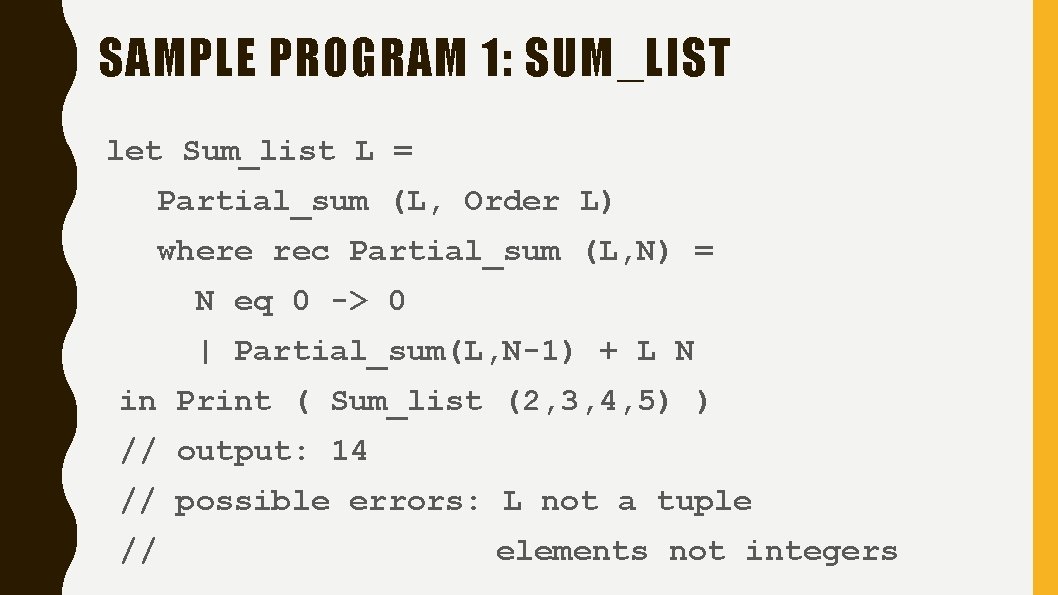
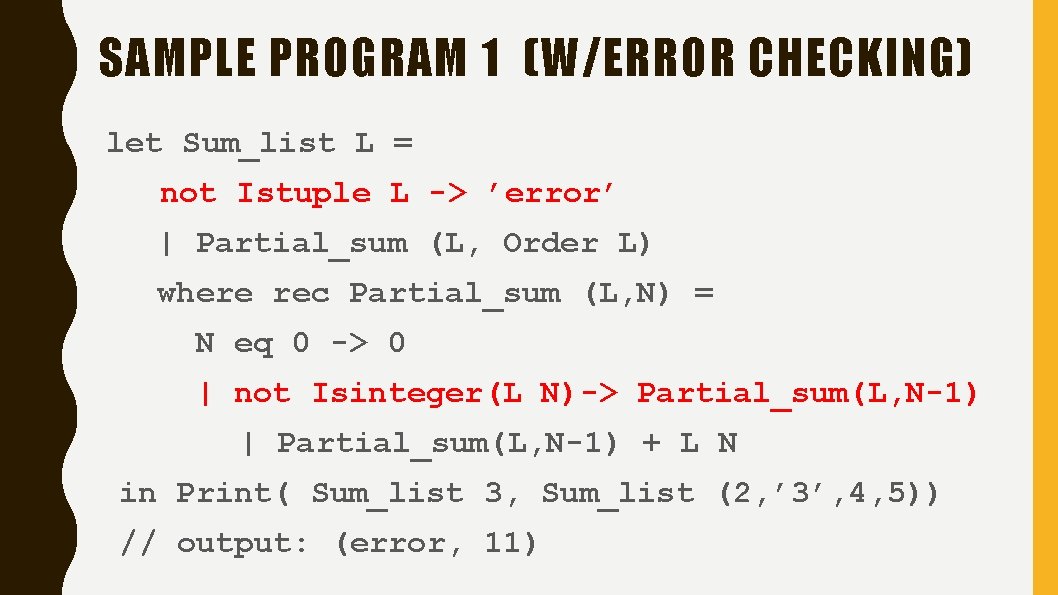
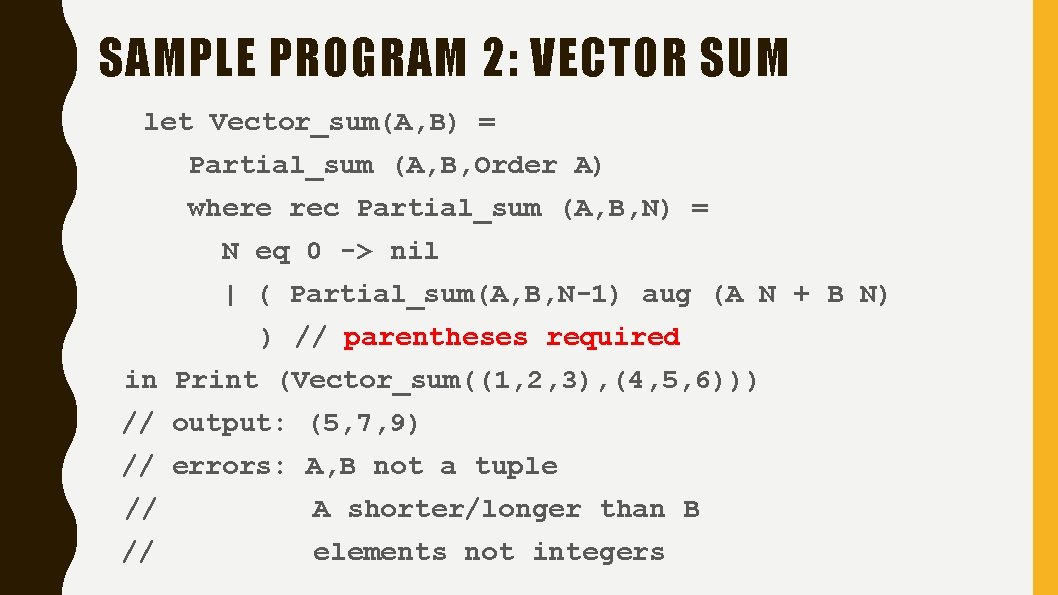
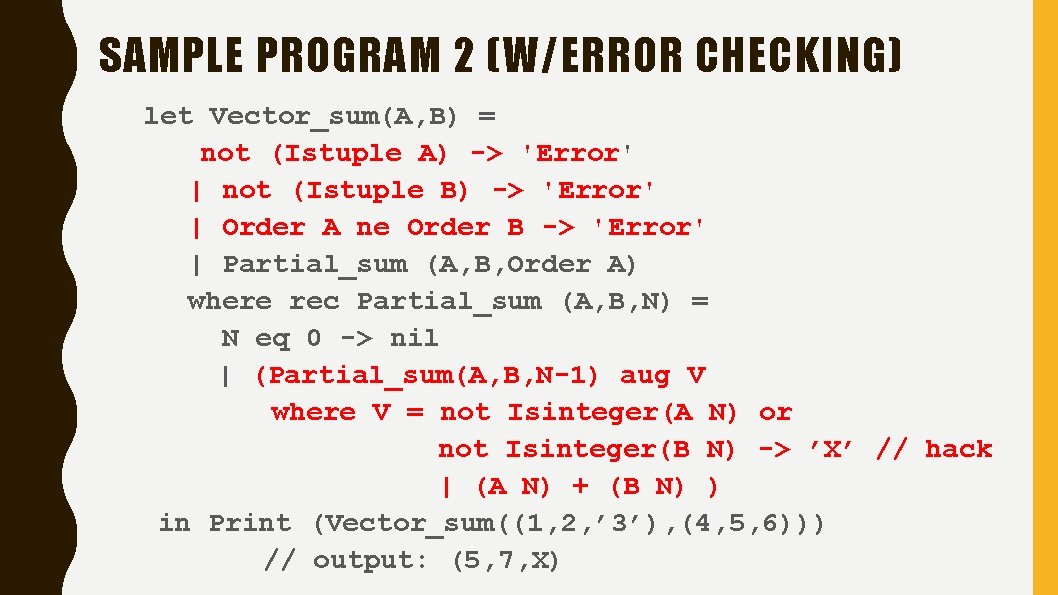
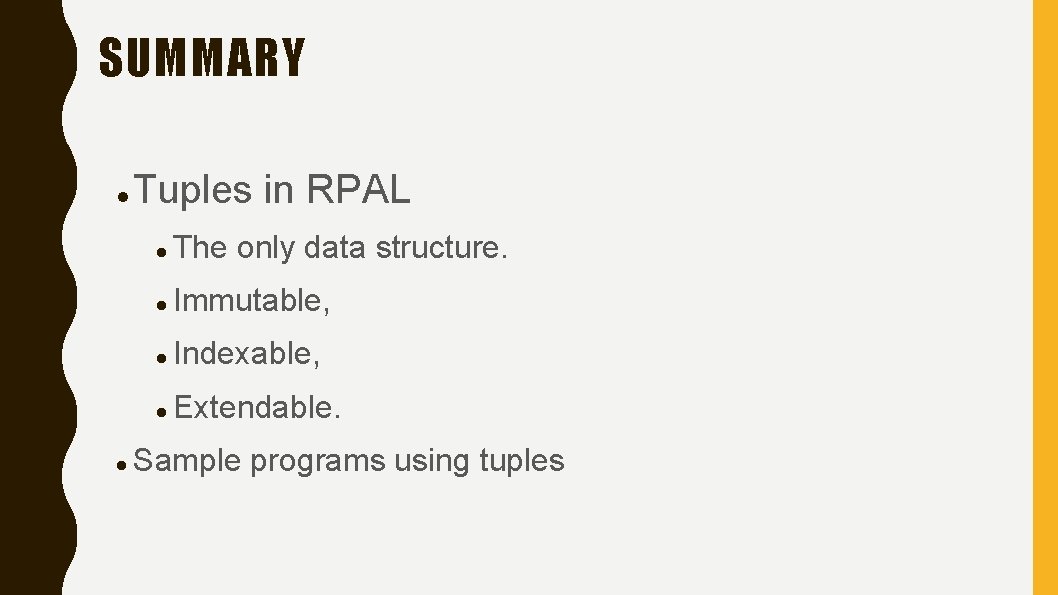
- Slides: 14
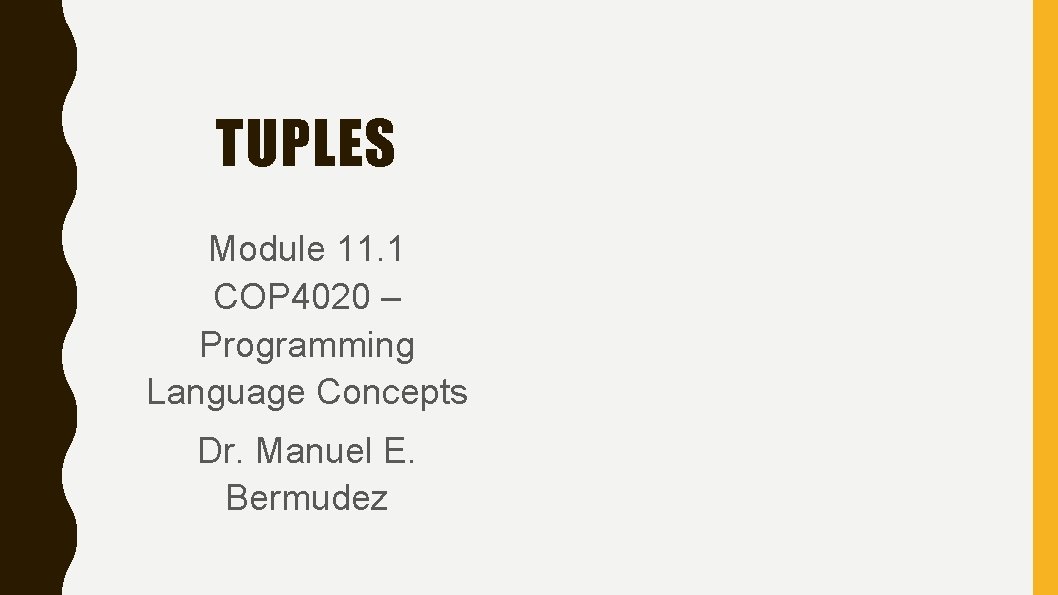
TUPLES Module 11. 1 COP 4020 – Programming Language Concepts Dr. Manuel E. Bermudez
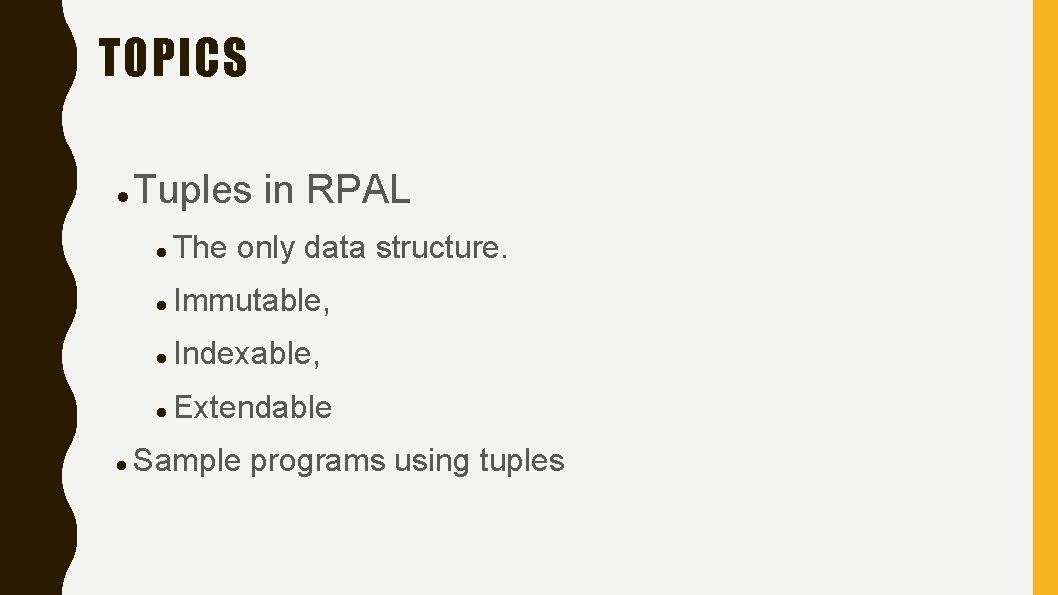
TOPICS Tuples in RPAL The only data structure. Immutable, Indexable, Extendable Sample programs using tuples
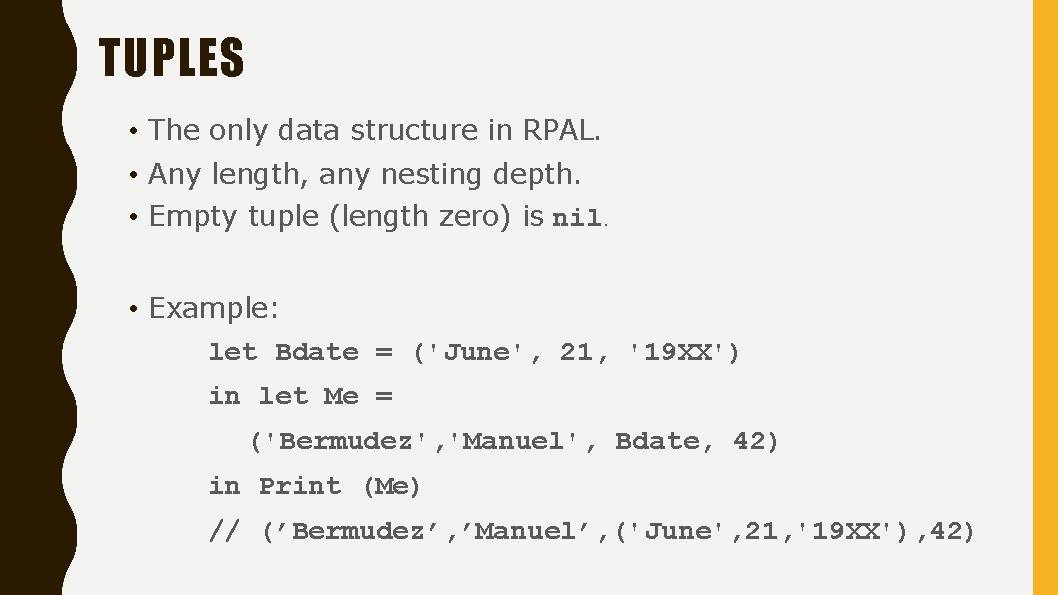
TUPLES • The only data structure in RPAL. • Any length, any nesting depth. • Empty tuple (length zero) is nil. • Example: let Bdate = ('June', 21, '19 XX') in let Me = ('Bermudez', 'Manuel', Bdate, 42) in Print (Me) // (’Bermudez’, ’Manuel’, ('June', 21, '19 XX'), 42)
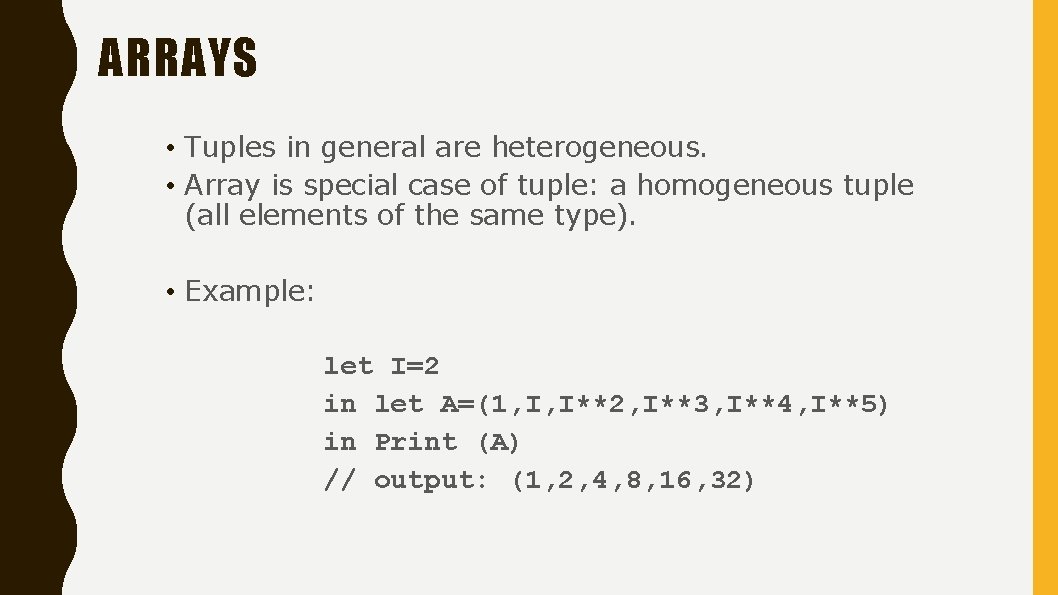
ARRAYS • Tuples in general are heterogeneous. • Array is special case of tuple: a homogeneous tuple (all elements of the same type). • Example: let I=2 in let A=(1, I, I**2, I**3, I**4, I**5) in Print (A) // output: (1, 2, 4, 8, 16, 32)
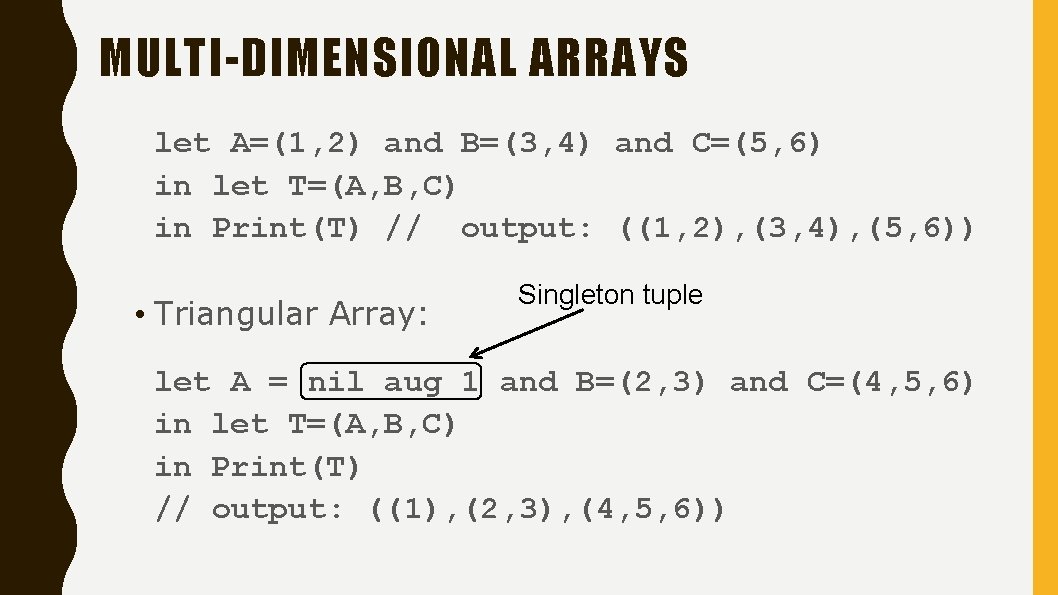
MULTI-DIMENSIONAL ARRAYS let A=(1, 2) and B=(3, 4) and C=(5, 6) in let T=(A, B, C) in Print(T) // output: ((1, 2), (3, 4), (5, 6)) • Triangular Array: Singleton tuple let A = nil aug 1 and B=(2, 3) and C=(4, 5, 6) in let T=(A, B, C) in Print(T) // output: ((1), (2, 3), (4, 5, 6))
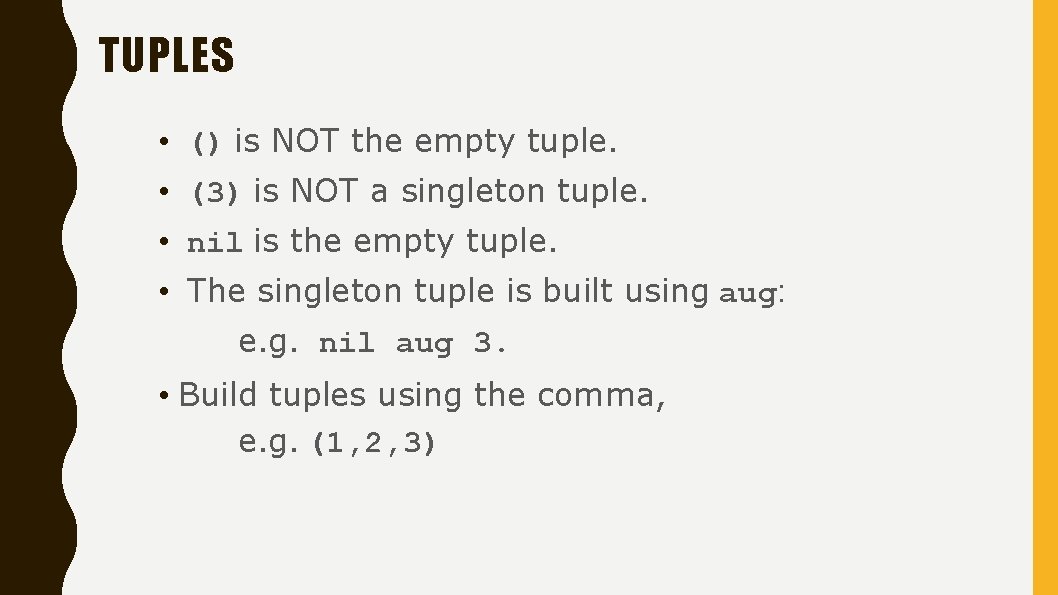
TUPLES • () is NOT the empty tuple. • (3) is NOT a singleton tuple. • nil is the empty tuple. • The singleton tuple is built using aug: e. g. nil aug 3. • Build tuples using the comma, e. g. (1, 2, 3)
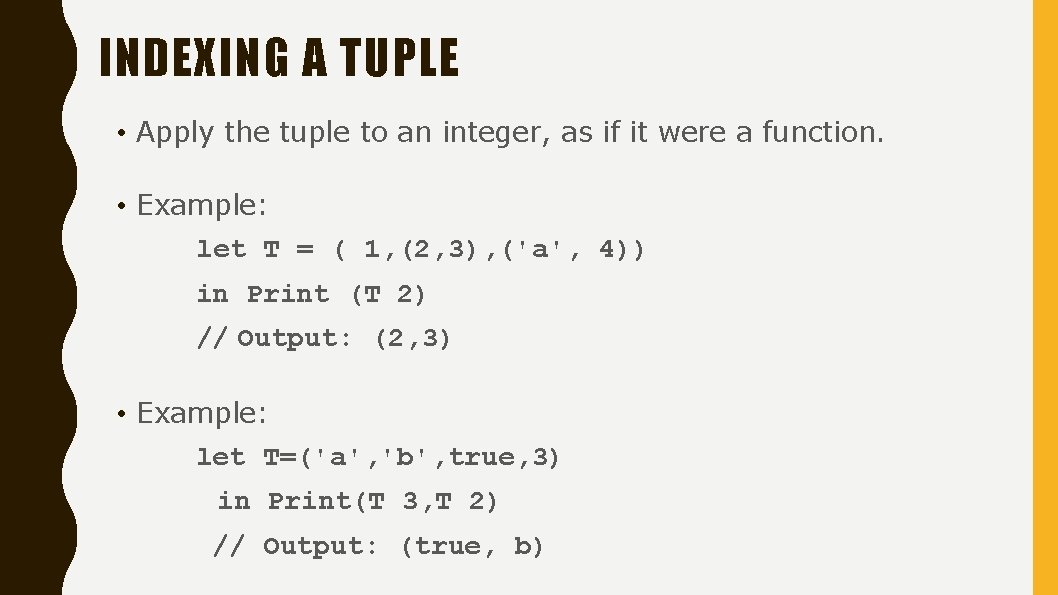
INDEXING A TUPLE • Apply the tuple to an integer, as if it were a function. • Example: let T = ( 1, (2, 3), ('a', 4)) in Print (T 2) // Output: (2, 3) • Example: let T=('a', 'b', true, 3) in Print(T 3, T 2) // Output: (true, b)
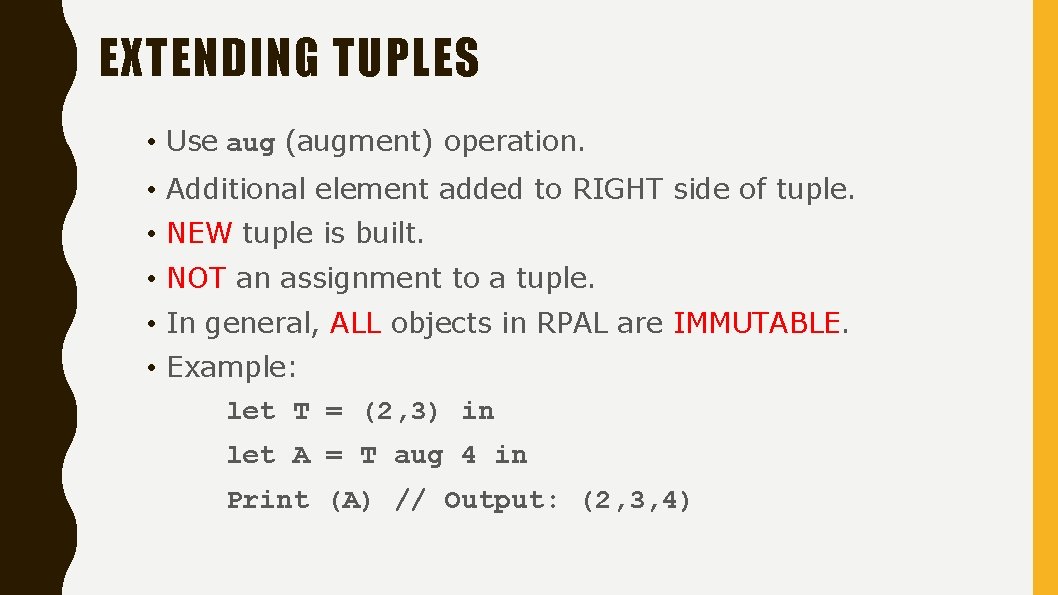
EXTENDING TUPLES • Use aug (augment) operation. • Additional element added to RIGHT side of tuple. • NEW tuple is built. • NOT an assignment to a tuple. • In general, ALL objects in RPAL are IMMUTABLE. • Example: let T = (2, 3) in let A = T aug 4 in Print (A) // Output: (2, 3, 4)
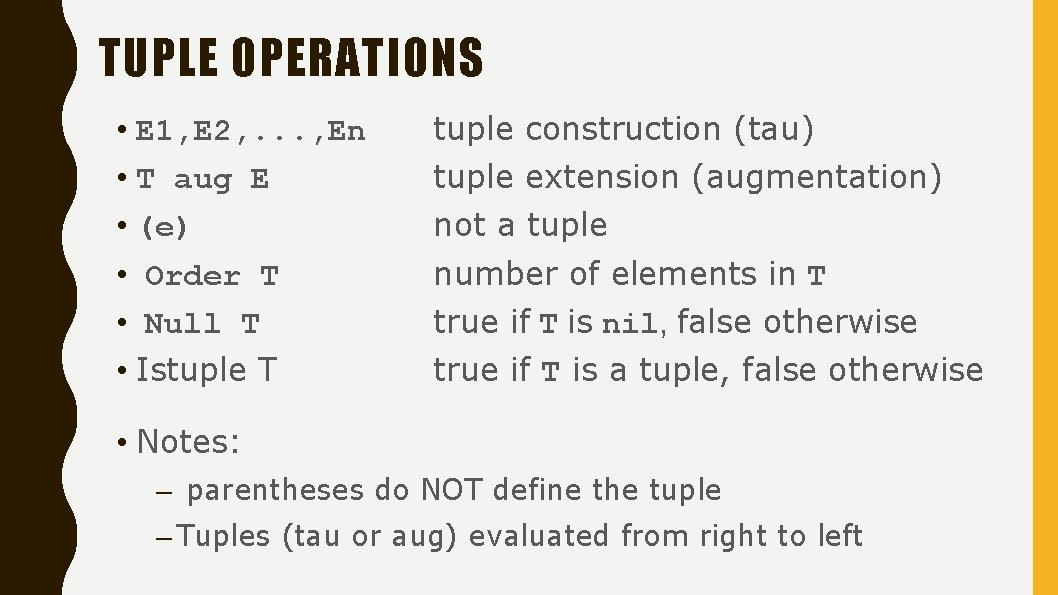
TUPLE OPERATIONS • E 1, E 2, . . . , En • T aug E • (e) • Order T • Null T • Istuple T tuple construction (tau) tuple extension (augmentation) not a tuple number of elements in T true if T is nil, false otherwise true if T is a tuple, false otherwise • Notes: – parentheses do NOT define the tuple – Tuples (tau or aug) evaluated from right to left
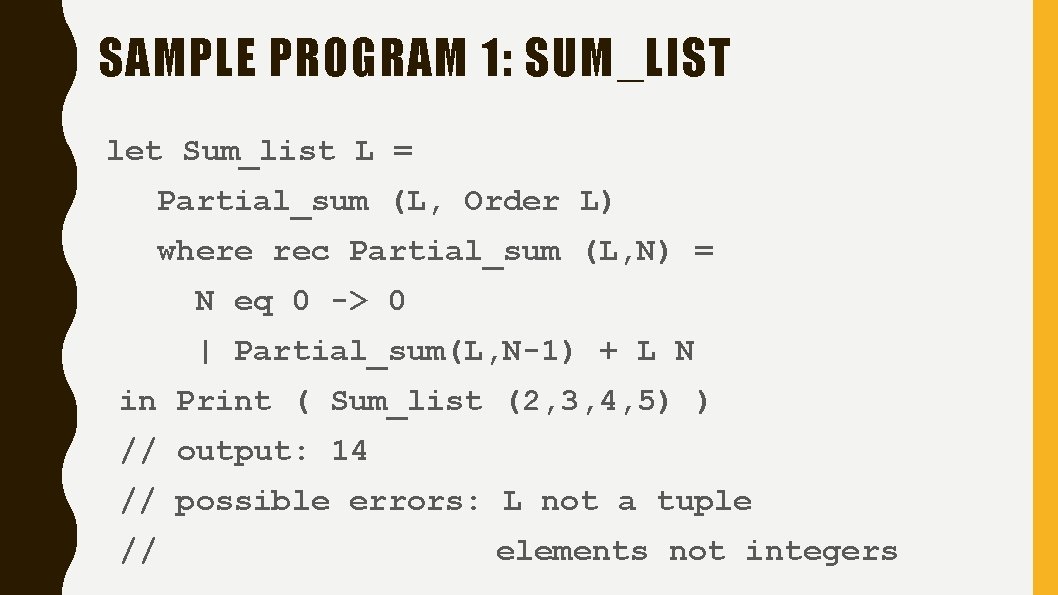
SAMPLE PROGRAM 1: SUM_LIST let Sum_list L = Partial_sum (L, Order L) where rec Partial_sum (L, N) = N eq 0 -> 0 | Partial_sum(L, N-1) + L N in Print ( Sum_list (2, 3, 4, 5) ) // output: 14 // possible errors: L not a tuple // elements not integers
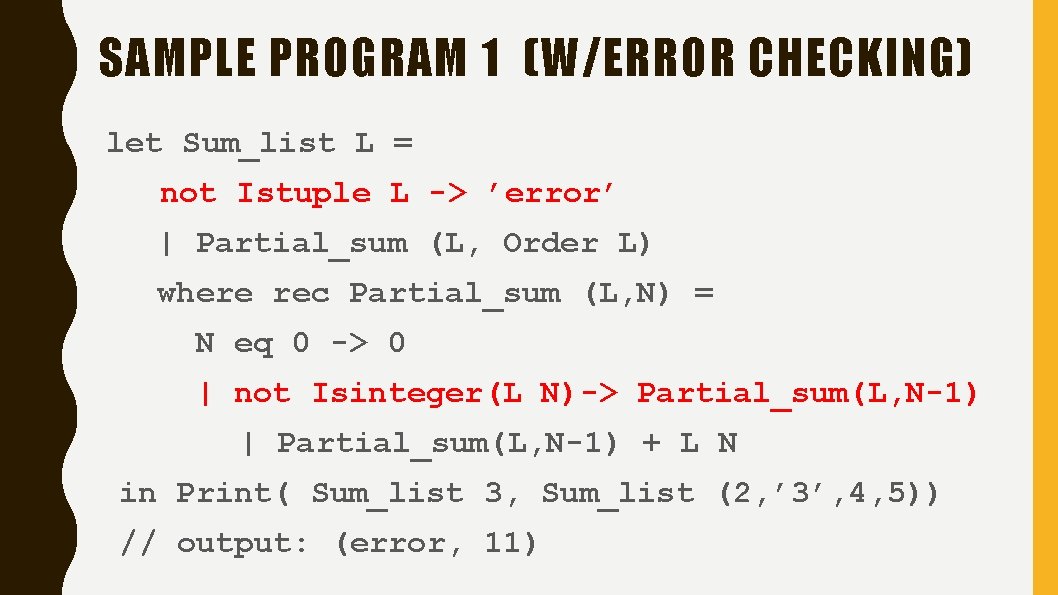
SAMPLE PROGRAM 1 (W/ERROR CHECKING) let Sum_list L = not Istuple L -> ’error’ | Partial_sum (L, Order L) where rec Partial_sum (L, N) = N eq 0 -> 0 | not Isinteger(L N)-> Partial_sum(L, N-1) | Partial_sum(L, N-1) + L N in Print( Sum_list 3, Sum_list (2, ’ 3’, 4, 5)) // output: (error, 11)
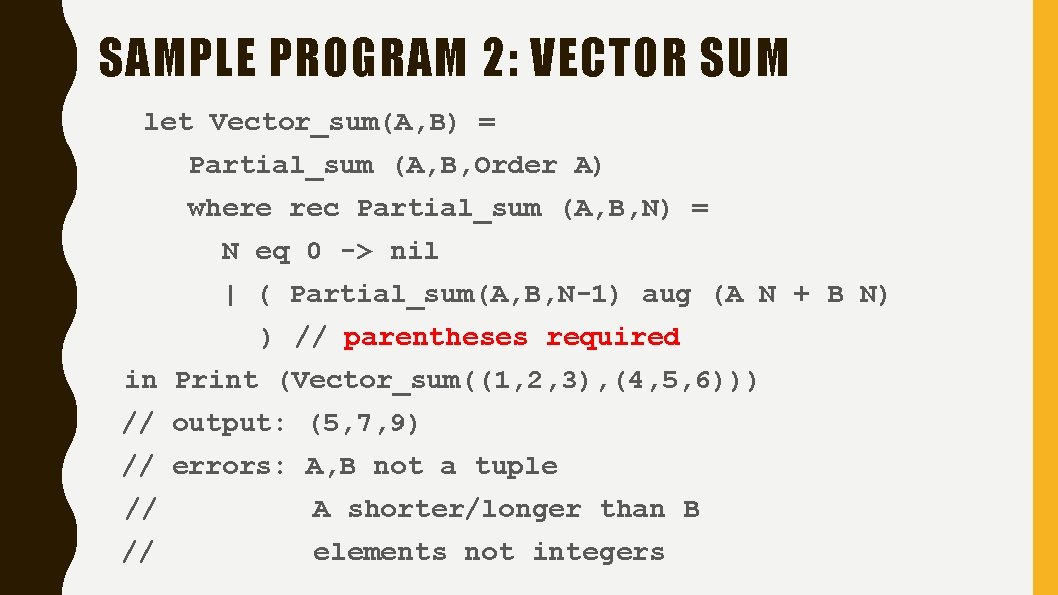
SAMPLE PROGRAM 2: VECTOR SUM let Vector_sum(A, B) = Partial_sum (A, B, Order A) where rec Partial_sum (A, B, N) = N eq 0 -> nil | ( Partial_sum(A, B, N-1) aug (A N + B N) ) // parentheses required in Print (Vector_sum((1, 2, 3), (4, 5, 6))) // output: (5, 7, 9) // errors: A, B not a tuple // A shorter/longer than B // elements not integers
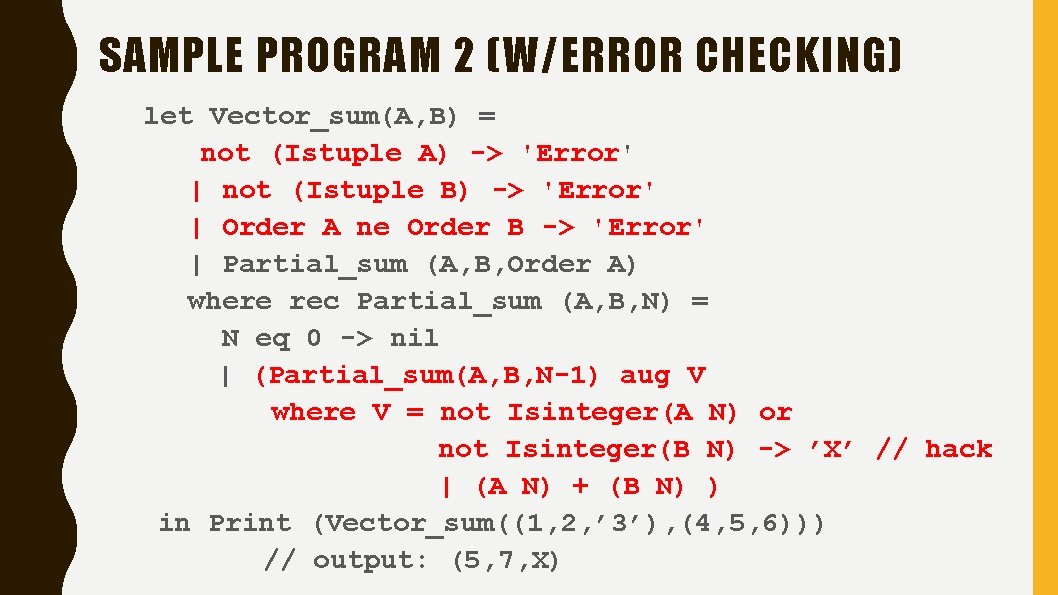
SAMPLE PROGRAM 2 (W/ERROR CHECKING) let Vector_sum(A, B) = not (Istuple A) -> 'Error' | not (Istuple B) -> 'Error' | Order A ne Order B -> 'Error' | Partial_sum (A, B, Order A) where rec Partial_sum (A, B, N) = N eq 0 -> nil | (Partial_sum(A, B, N-1) aug V where V = not Isinteger(A N) or not Isinteger(B N) -> ’X’ // hack | (A N) + (B N) ) in Print (Vector_sum((1, 2, ’ 3’), (4, 5, 6))) // output: (5, 7, X)
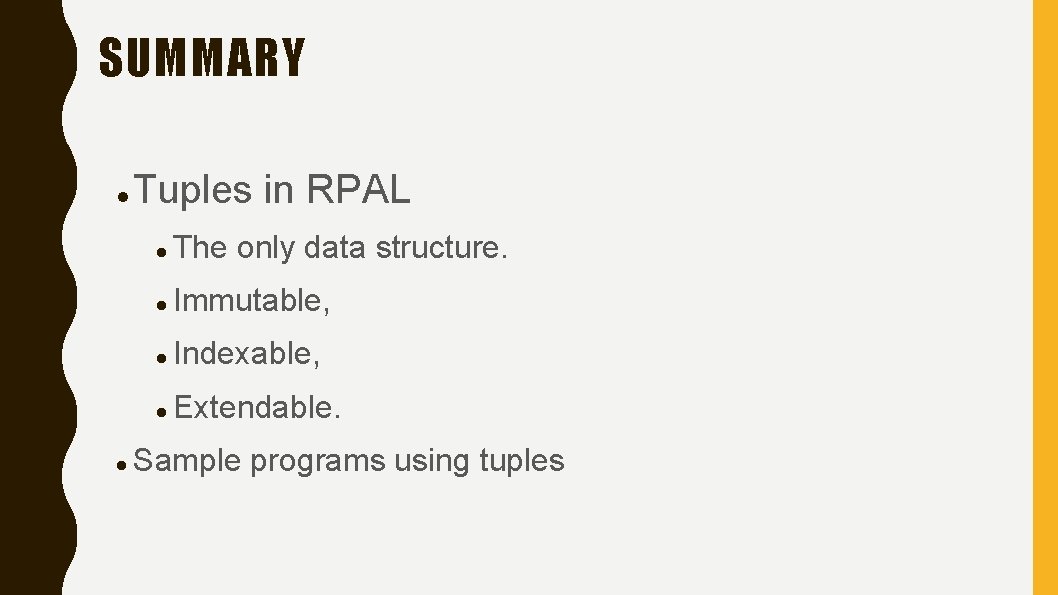
SUMMARY Tuples in RPAL The only data structure. Immutable, Indexable, Extendable. Sample programs using tuples