Systems Architecture I CS 281 001 Lecture 6
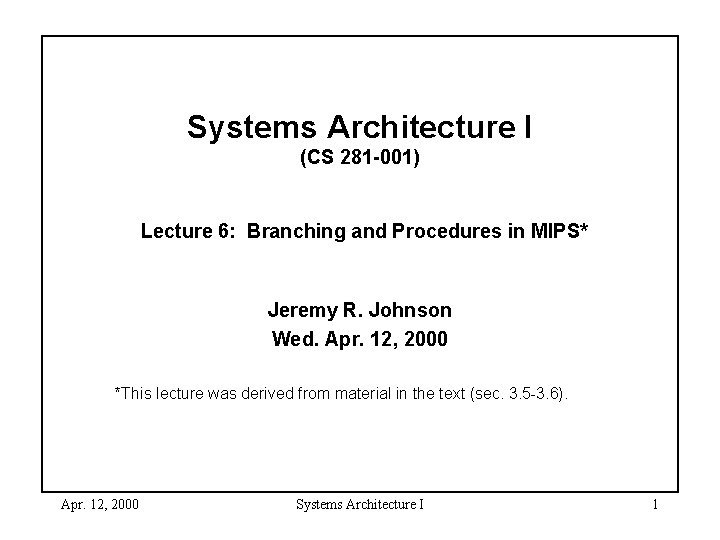
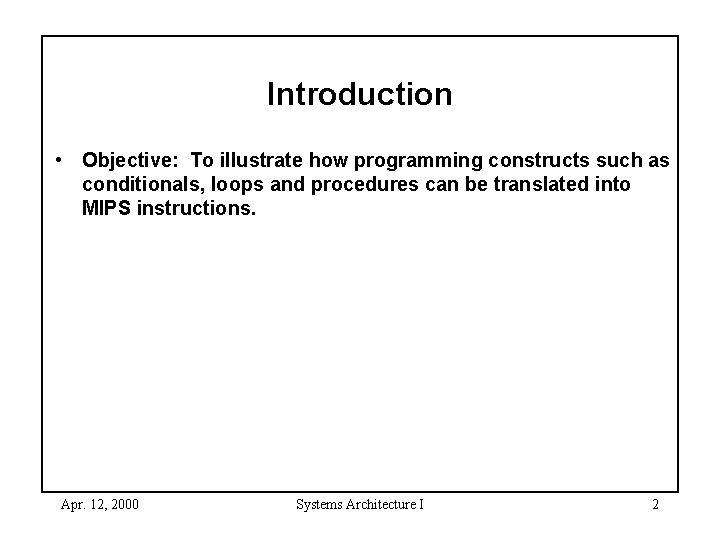
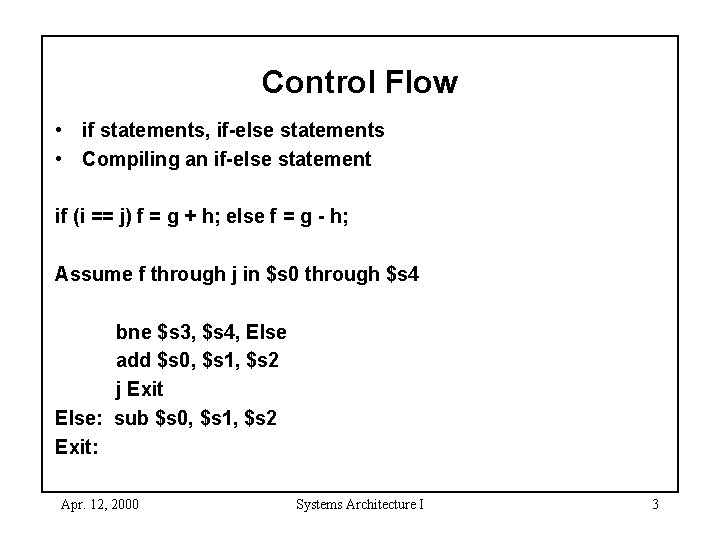
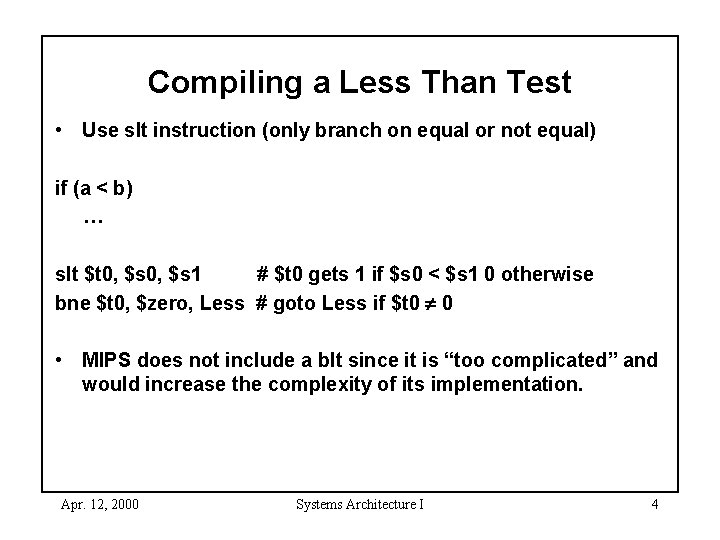
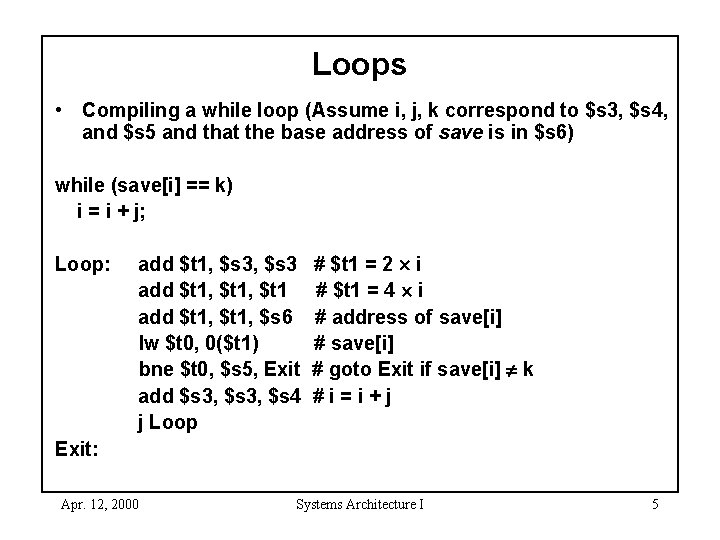
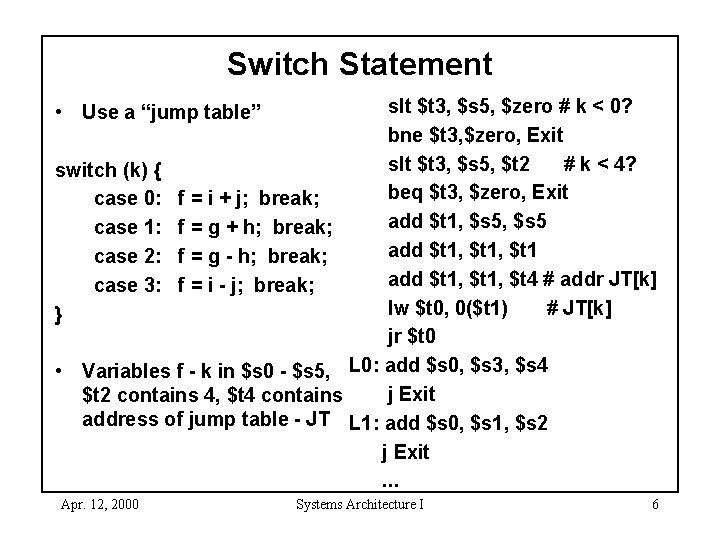
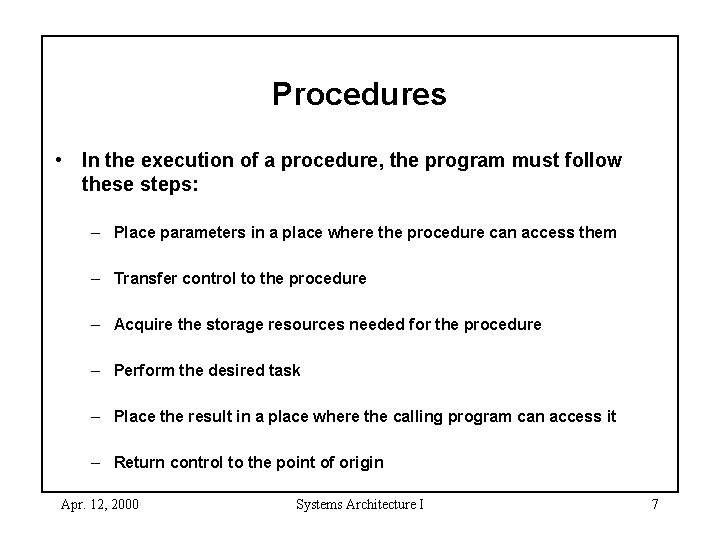
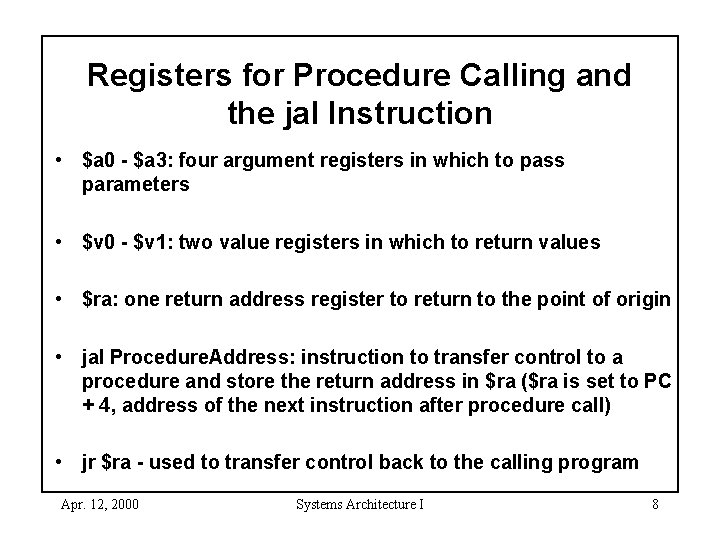
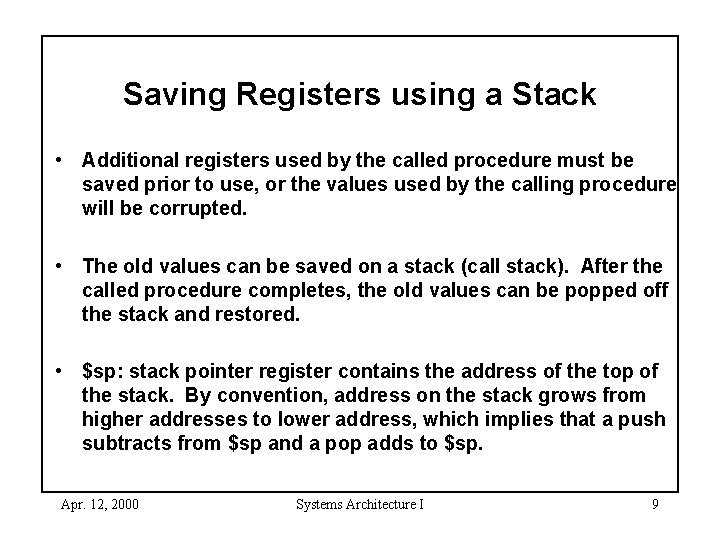
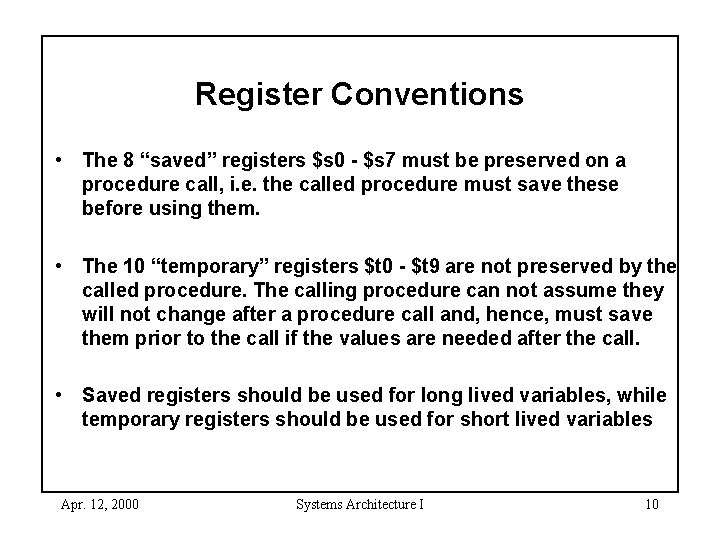
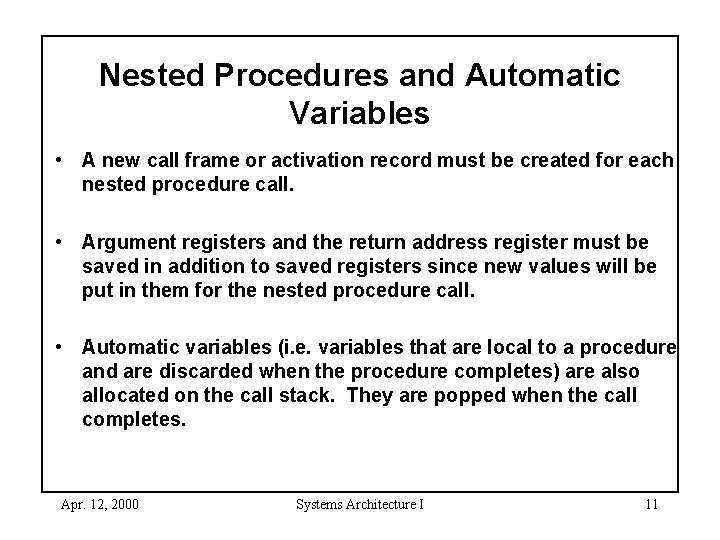
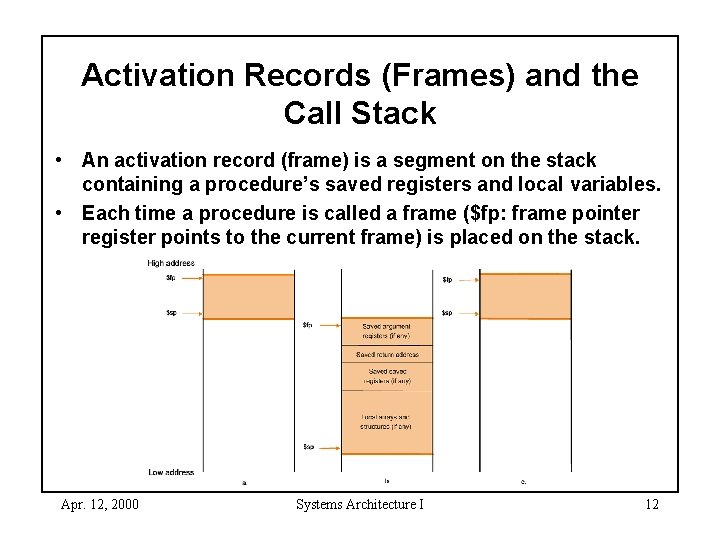
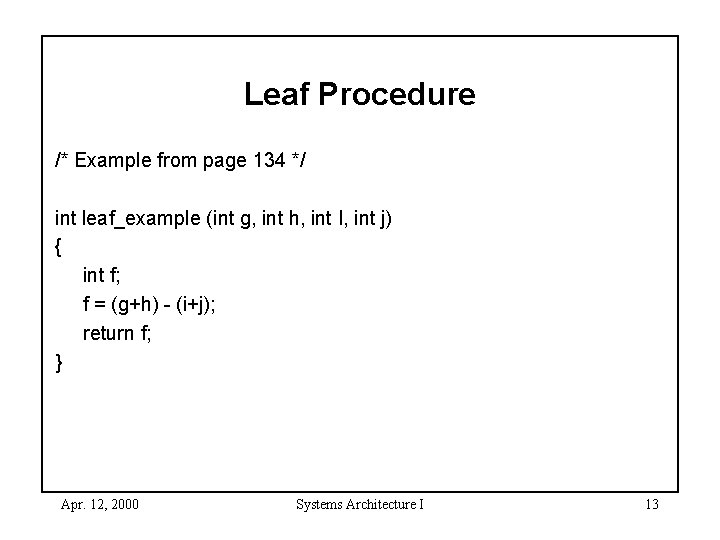
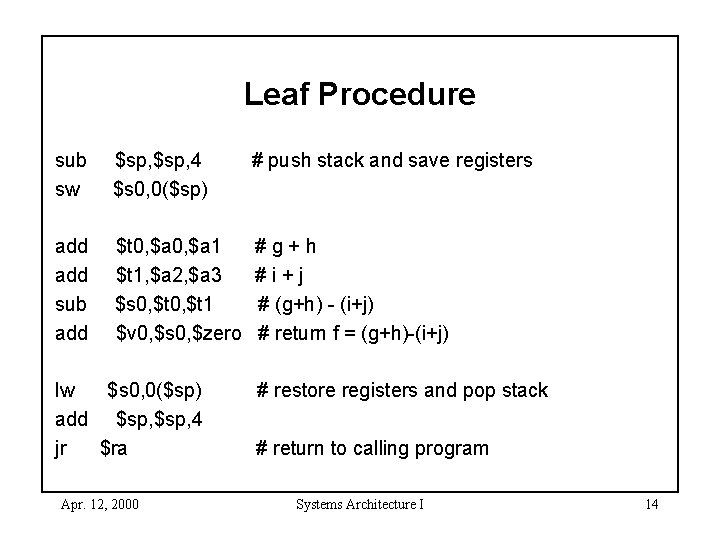
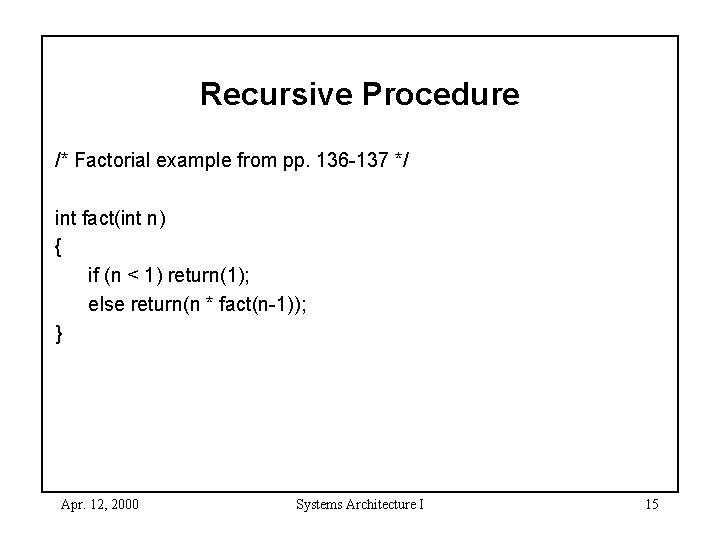
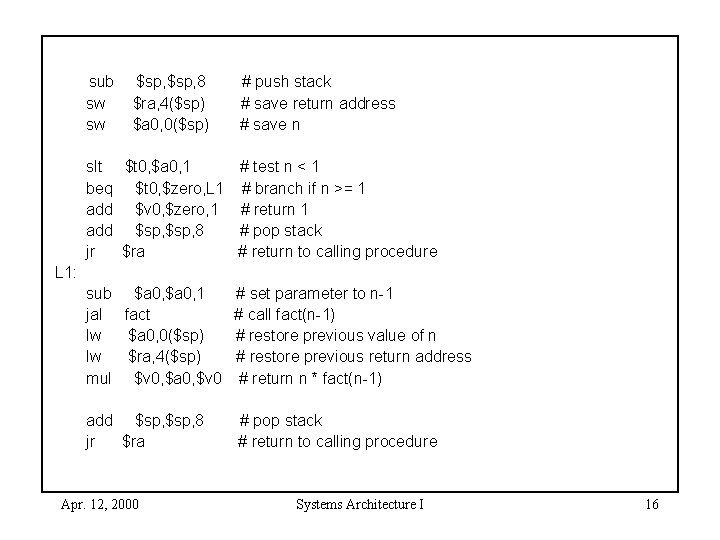
- Slides: 16
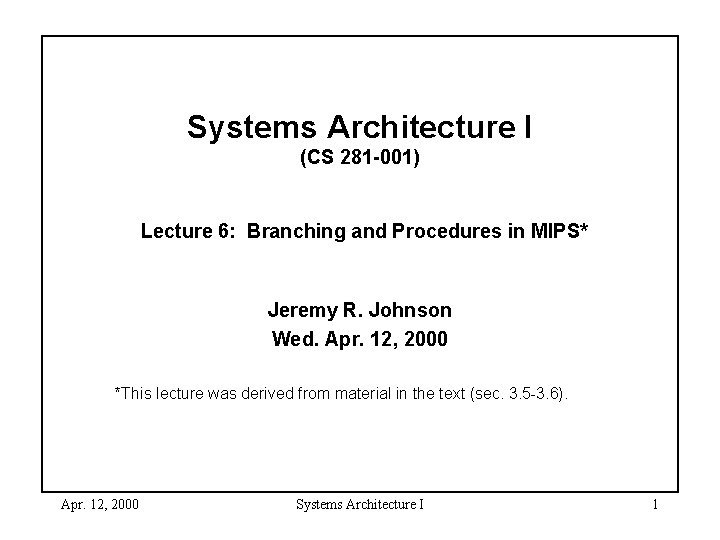
Systems Architecture I (CS 281 -001) Lecture 6: Branching and Procedures in MIPS* Jeremy R. Johnson Wed. Apr. 12, 2000 *This lecture was derived from material in the text (sec. 3. 5 -3. 6). Apr. 12, 2000 Systems Architecture I 1
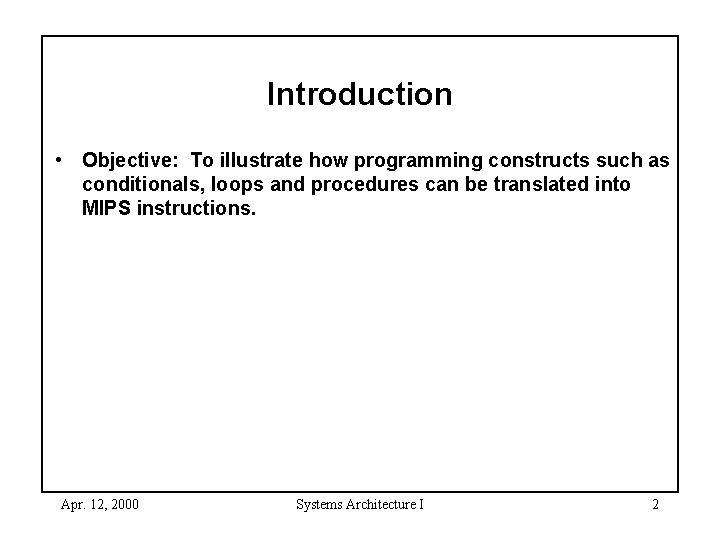
Introduction • Objective: To illustrate how programming constructs such as conditionals, loops and procedures can be translated into MIPS instructions. Apr. 12, 2000 Systems Architecture I 2
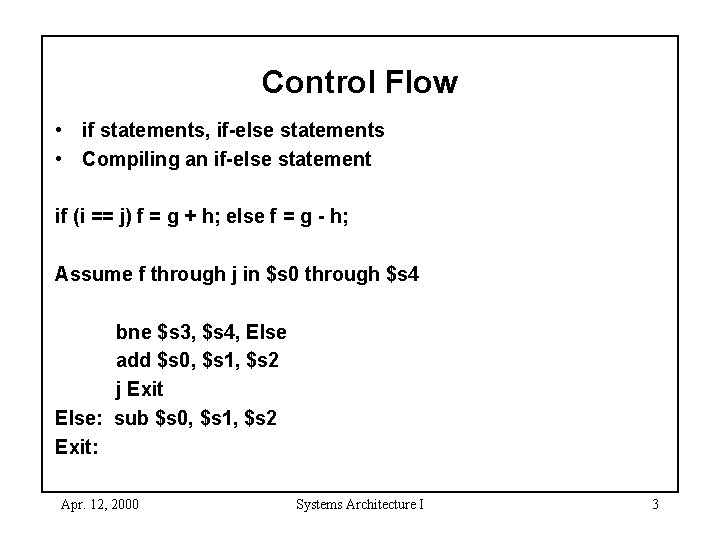
Control Flow • if statements, if-else statements • Compiling an if-else statement if (i == j) f = g + h; else f = g - h; Assume f through j in $s 0 through $s 4 bne $s 3, $s 4, Else add $s 0, $s 1, $s 2 j Exit Else: sub $s 0, $s 1, $s 2 Exit: Apr. 12, 2000 Systems Architecture I 3
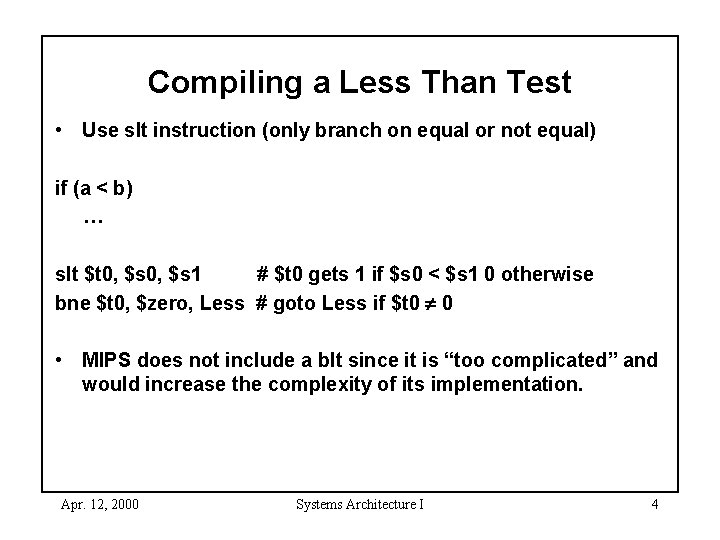
Compiling a Less Than Test • Use slt instruction (only branch on equal or not equal) if (a < b) … slt $t 0, $s 1 # $t 0 gets 1 if $s 0 < $s 1 0 otherwise bne $t 0, $zero, Less # goto Less if $t 0 0 • MIPS does not include a blt since it is “too complicated” and would increase the complexity of its implementation. Apr. 12, 2000 Systems Architecture I 4
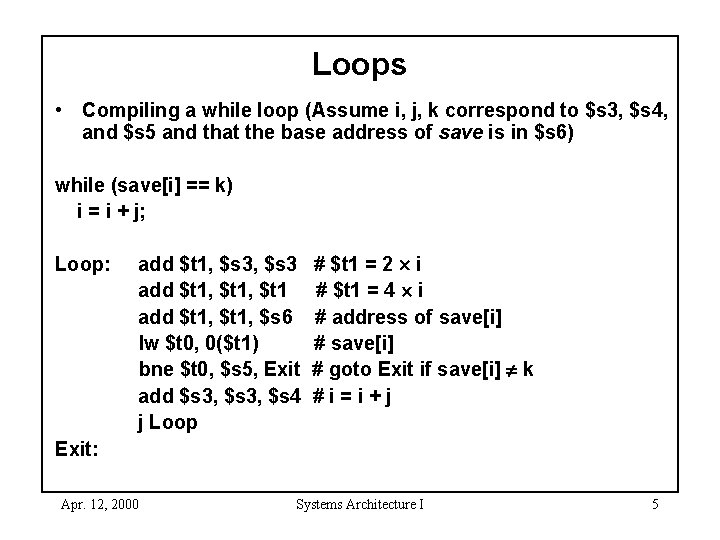
Loops • Compiling a while loop (Assume i, j, k correspond to $s 3, $s 4, and $s 5 and that the base address of save is in $s 6) while (save[i] == k) i = i + j; Loop: add $t 1, $s 3 add $t 1, $t 1 add $t 1, $s 6 lw $t 0, 0($t 1) bne $t 0, $s 5, Exit add $s 3, $s 4 j Loop # $t 1 = 2 i # $t 1 = 4 i # address of save[i] # goto Exit if save[i] k #i=i+j Exit: Apr. 12, 2000 Systems Architecture I 5
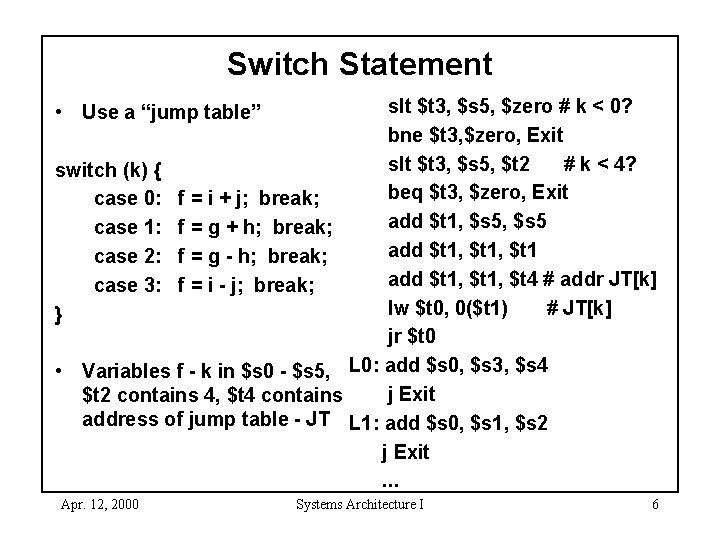
Switch Statement • Use a “jump table” switch (k) { case 0: case 1: case 2: case 3: } f = i + j; break; f = g + h; break; f = g - h; break; f = i - j; break; • Variables f - k in $s 0 - $s 5, $t 2 contains 4, $t 4 contains address of jump table - JT Apr. 12, 2000 slt $t 3, $s 5, $zero # k < 0? bne $t 3, $zero, Exit slt $t 3, $s 5, $t 2 # k < 4? beq $t 3, $zero, Exit add $t 1, $s 5 add $t 1, $t 1 add $t 1, $t 4 # addr JT[k] lw $t 0, 0($t 1) # JT[k] jr $t 0 L 0: add $s 0, $s 3, $s 4 j Exit L 1: add $s 0, $s 1, $s 2 j Exit. . . Systems Architecture I 6
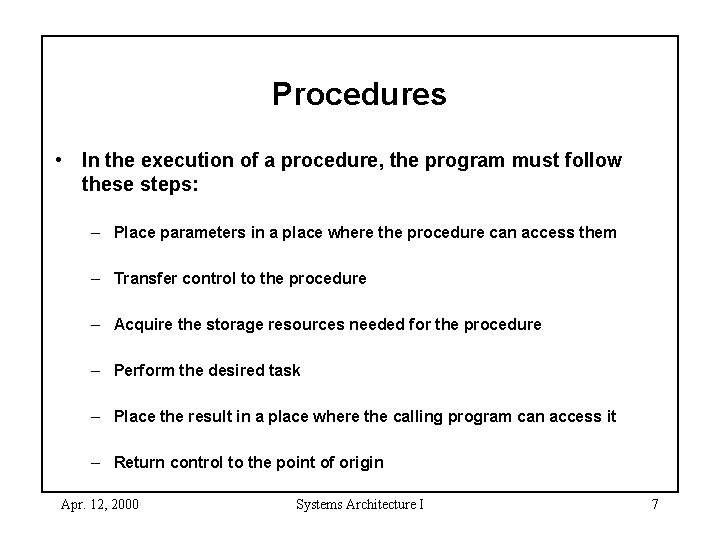
Procedures • In the execution of a procedure, the program must follow these steps: – Place parameters in a place where the procedure can access them – Transfer control to the procedure – Acquire the storage resources needed for the procedure – Perform the desired task – Place the result in a place where the calling program can access it – Return control to the point of origin Apr. 12, 2000 Systems Architecture I 7
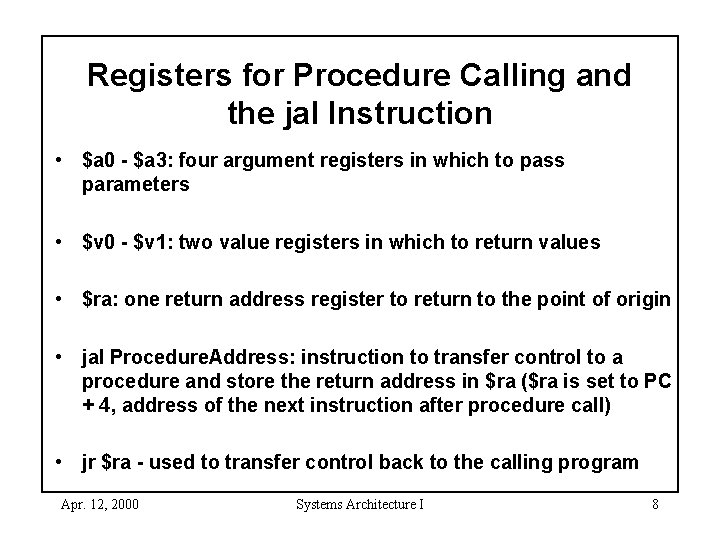
Registers for Procedure Calling and the jal Instruction • $a 0 - $a 3: four argument registers in which to pass parameters • $v 0 - $v 1: two value registers in which to return values • $ra: one return address register to return to the point of origin • jal Procedure. Address: instruction to transfer control to a procedure and store the return address in $ra ($ra is set to PC + 4, address of the next instruction after procedure call) • jr $ra - used to transfer control back to the calling program Apr. 12, 2000 Systems Architecture I 8
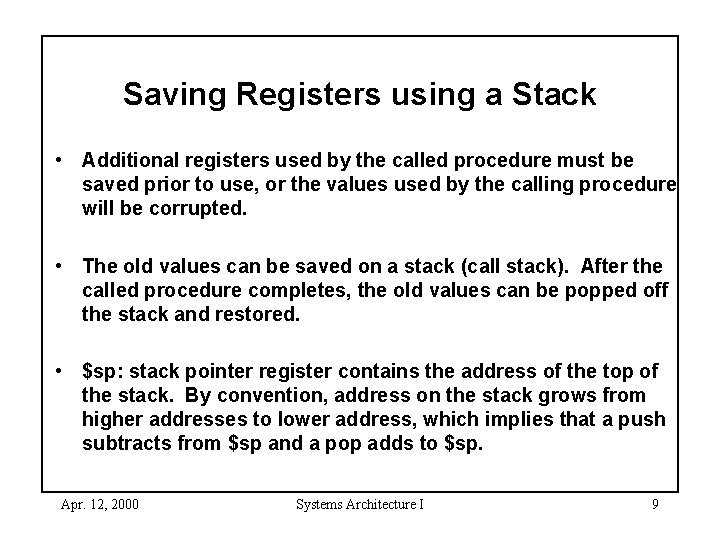
Saving Registers using a Stack • Additional registers used by the called procedure must be saved prior to use, or the values used by the calling procedure will be corrupted. • The old values can be saved on a stack (call stack). After the called procedure completes, the old values can be popped off the stack and restored. • $sp: stack pointer register contains the address of the top of the stack. By convention, address on the stack grows from higher addresses to lower address, which implies that a push subtracts from $sp and a pop adds to $sp. Apr. 12, 2000 Systems Architecture I 9
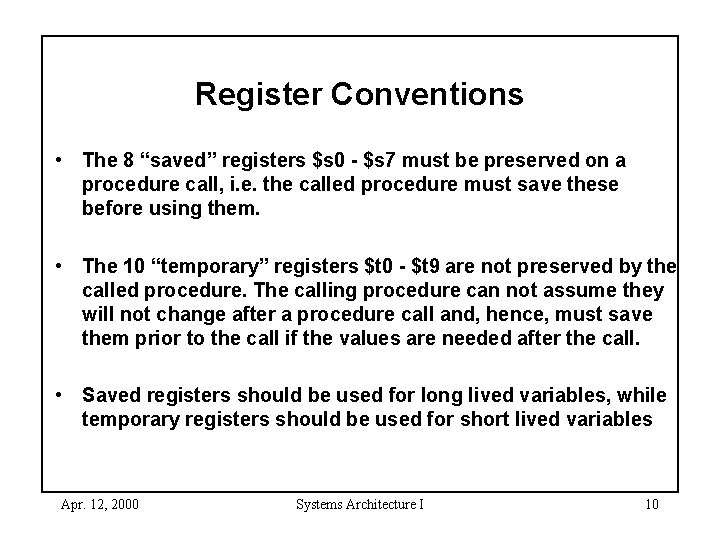
Register Conventions • The 8 “saved” registers $s 0 - $s 7 must be preserved on a procedure call, i. e. the called procedure must save these before using them. • The 10 “temporary” registers $t 0 - $t 9 are not preserved by the called procedure. The calling procedure can not assume they will not change after a procedure call and, hence, must save them prior to the call if the values are needed after the call. • Saved registers should be used for long lived variables, while temporary registers should be used for short lived variables Apr. 12, 2000 Systems Architecture I 10
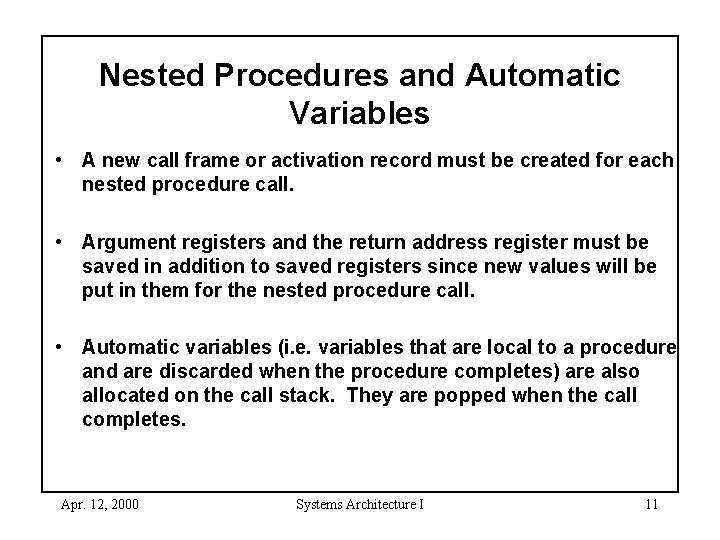
Nested Procedures and Automatic Variables • A new call frame or activation record must be created for each nested procedure call. • Argument registers and the return address register must be saved in addition to saved registers since new values will be put in them for the nested procedure call. • Automatic variables (i. e. variables that are local to a procedure and are discarded when the procedure completes) are also allocated on the call stack. They are popped when the call completes. Apr. 12, 2000 Systems Architecture I 11
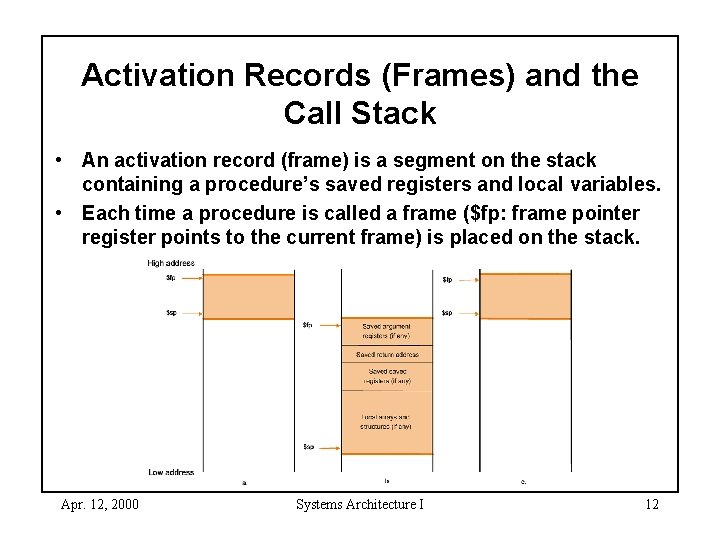
Activation Records (Frames) and the Call Stack • An activation record (frame) is a segment on the stack containing a procedure’s saved registers and local variables. • Each time a procedure is called a frame ($fp: frame pointer register points to the current frame) is placed on the stack. Apr. 12, 2000 Systems Architecture I 12
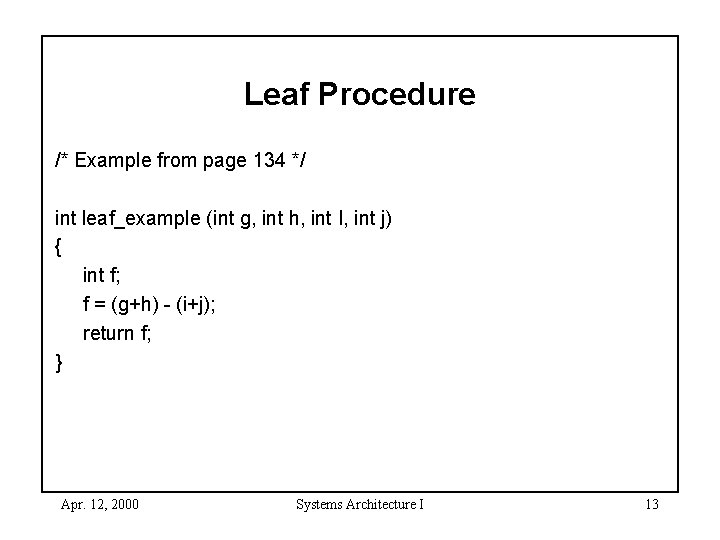
Leaf Procedure /* Example from page 134 */ int leaf_example (int g, int h, int I, int j) { int f; f = (g+h) - (i+j); return f; } Apr. 12, 2000 Systems Architecture I 13
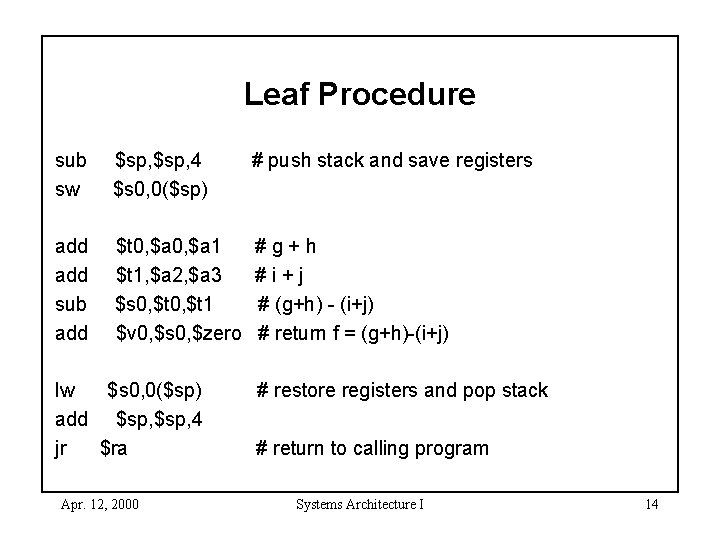
Leaf Procedure sub sw $sp, 4 $s 0, 0($sp) # push stack and save registers add sub add $t 0, $a 1 $t 1, $a 2, $a 3 $s 0, $t 1 $v 0, $s 0, $zero #g+h #i+j # (g+h) - (i+j) # return f = (g+h)-(i+j) lw $s 0, 0($sp) add $sp, 4 jr $ra Apr. 12, 2000 # restore registers and pop stack # return to calling program Systems Architecture I 14
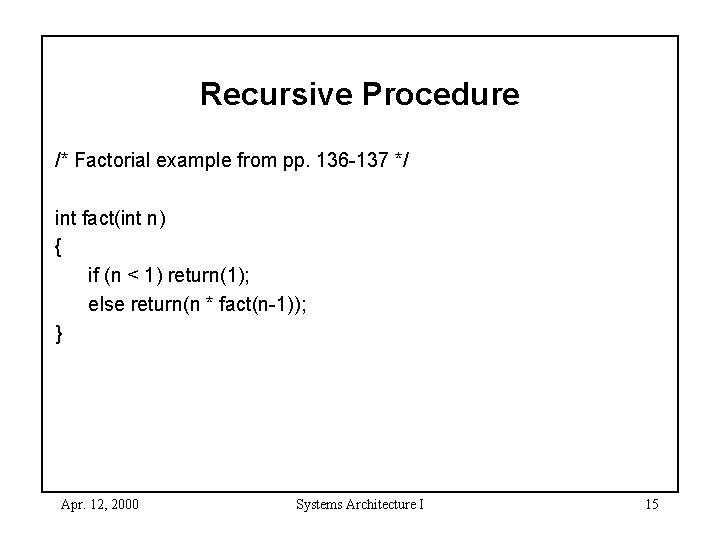
Recursive Procedure /* Factorial example from pp. 136 -137 */ int fact(int n) { if (n < 1) return(1); else return(n * fact(n-1)); } Apr. 12, 2000 Systems Architecture I 15
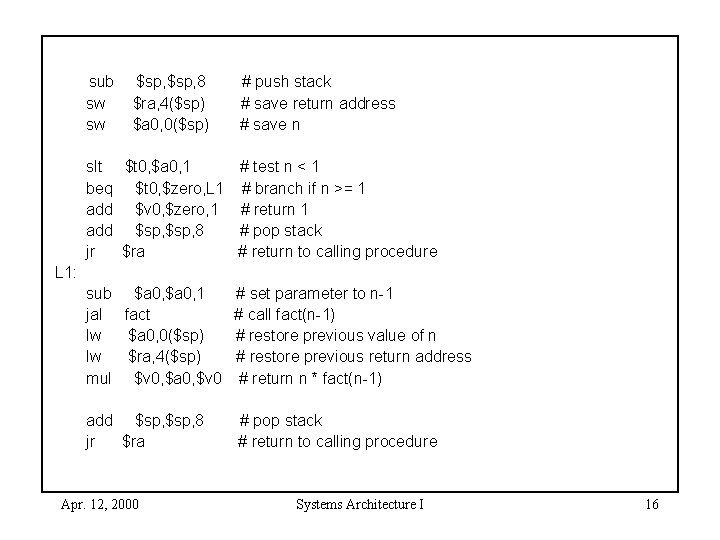
sub sw sw $sp, 8 $ra, 4($sp) $a 0, 0($sp) # push stack # save return address # save n slt $t 0, $a 0, 1 # test n < 1 beq $t 0, $zero, L 1 # branch if n >= 1 add $v 0, $zero, 1 # return 1 add $sp, 8 # pop stack jr $ra # return to calling procedure L 1: sub $a 0, 1 jal fact lw $a 0, 0($sp) lw $ra, 4($sp) mul $v 0, $a 0, $v 0 # set parameter to n-1 # call fact(n-1) # restore previous value of n # restore previous return address # return n * fact(n-1) add $sp, 8 jr $ra # pop stack # return to calling procedure Apr. 12, 2000 Systems Architecture I 16
Ipx 281
Cs281 wordpress
Cpr e 281
It is a circular or elliptical anticlinal structure
Granite weathering
281-284-0027
Cs 281
Numeros romanos del 1 al 100
Stoytchev 281
Stoytchev 281
18 gelecek aylara ait giderler ve gelir tahakkukları
Dönem ayirici hesaplar 180 181 280 281 380 381
01:640:244 lecture notes - lecture 15: plat, idah, farad
Computer architecture lecture notes
Isa definition computer
Operating systems lecture notes
Lecture sound systems