Software Tools Perl Arrays and Lists Slide 2
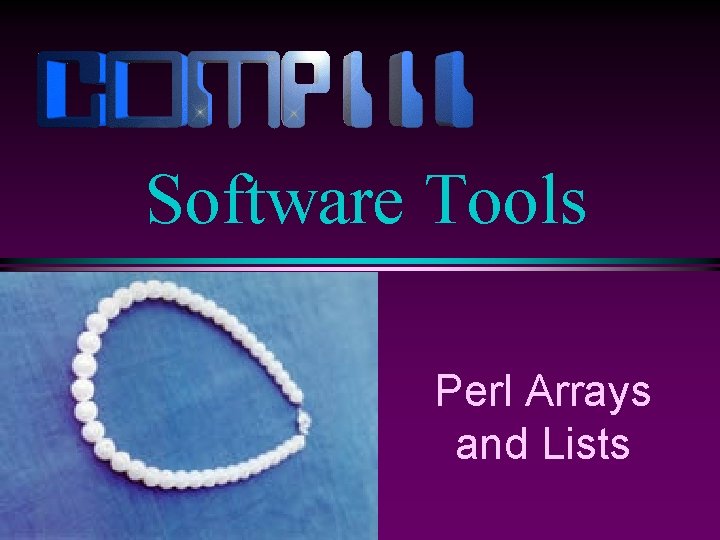
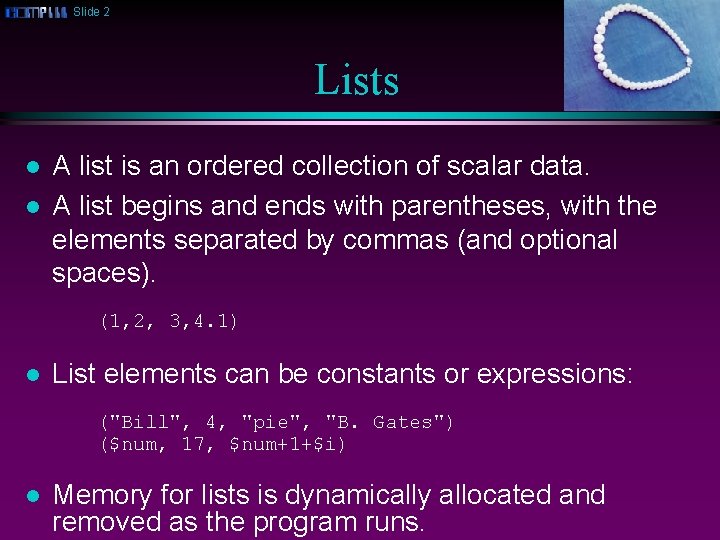
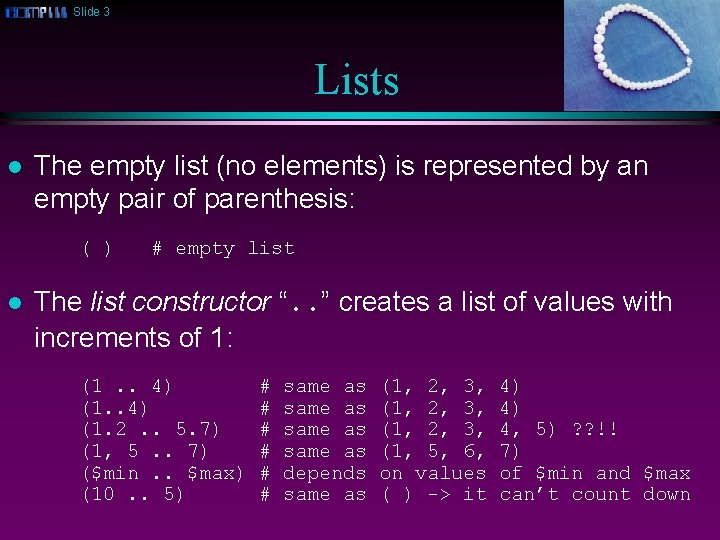
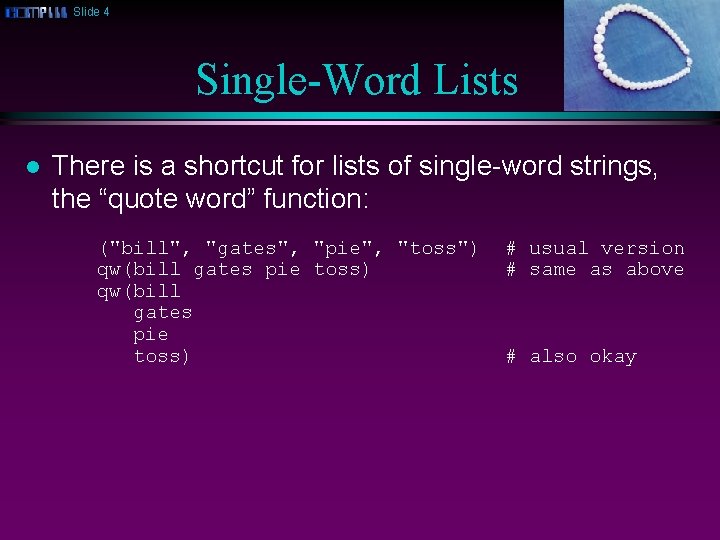
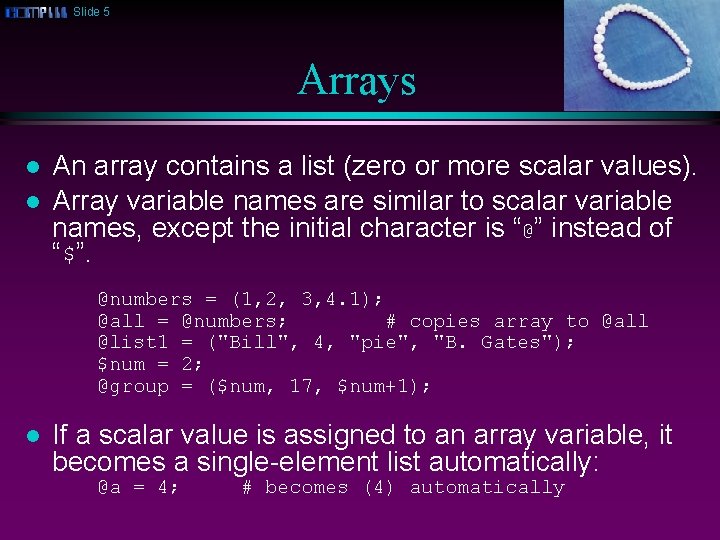
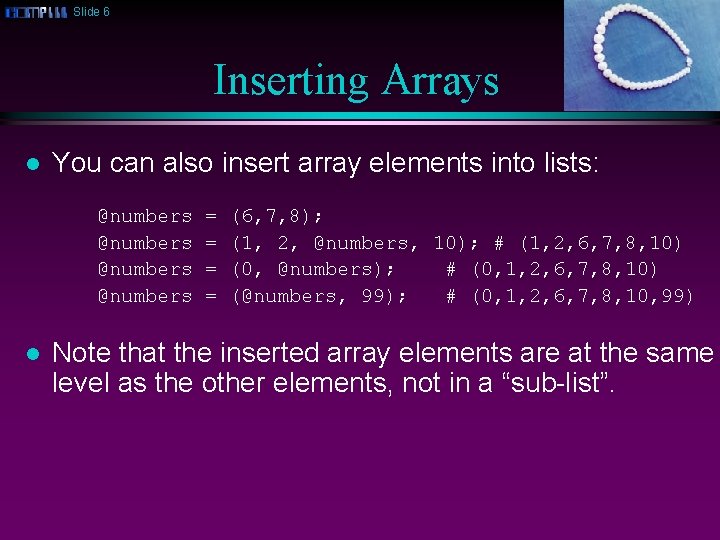
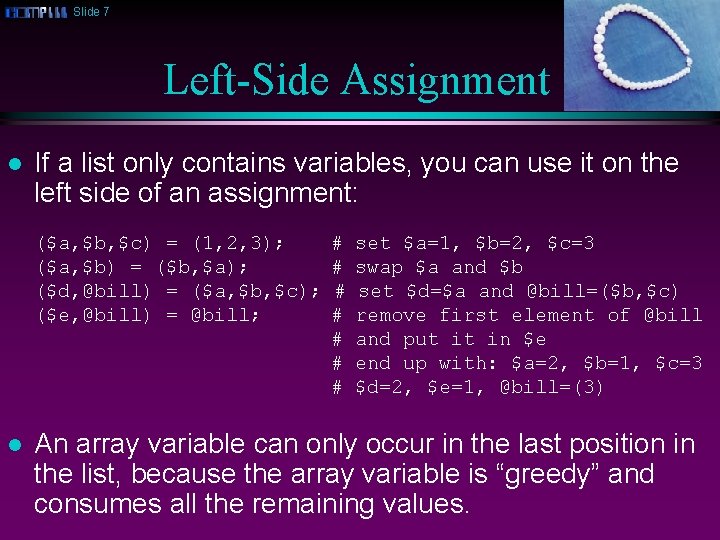
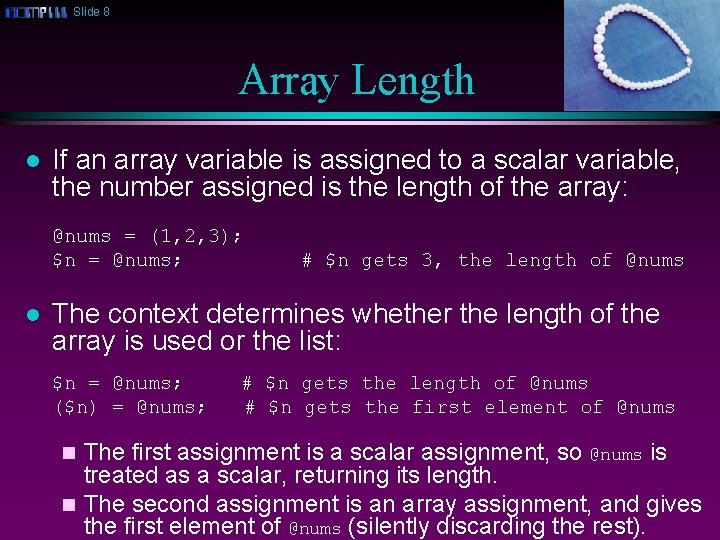
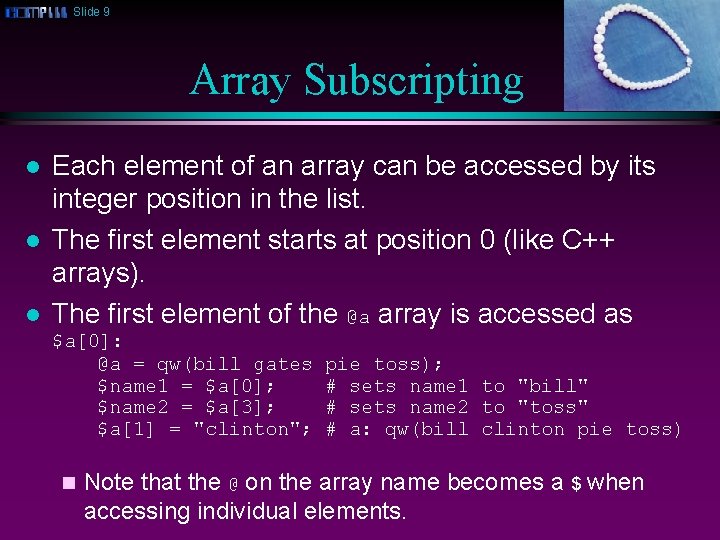
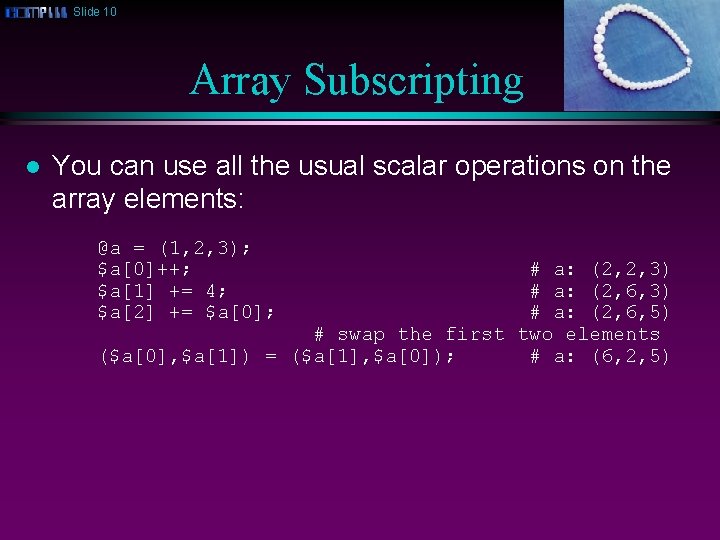
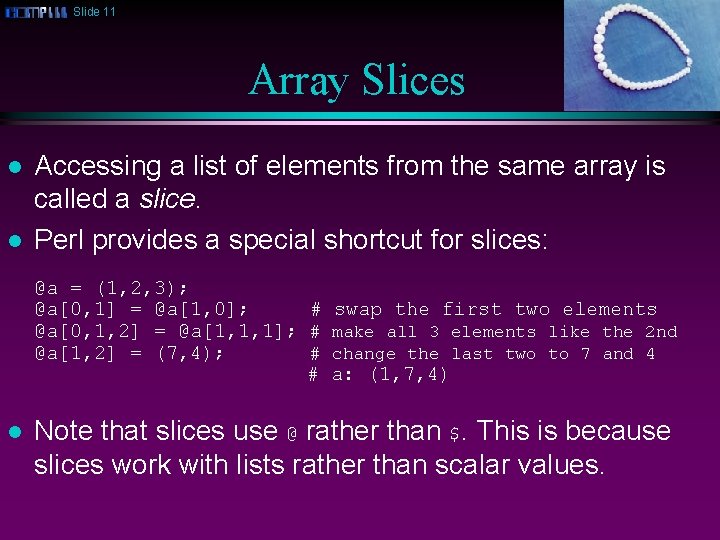
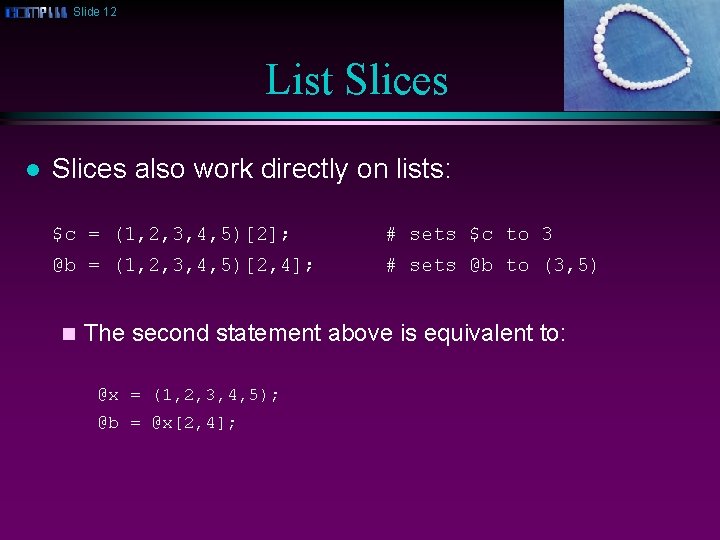
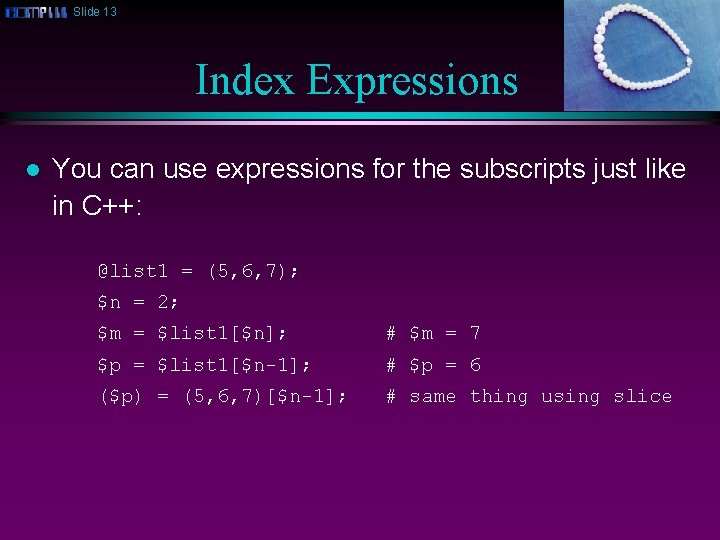
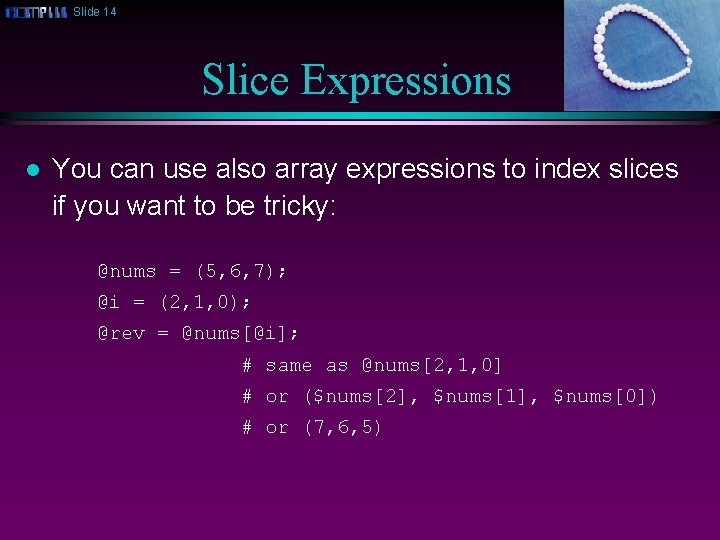
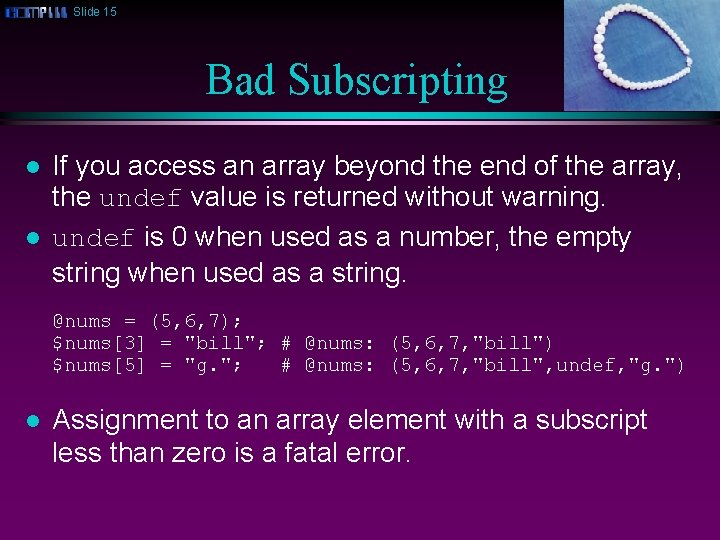
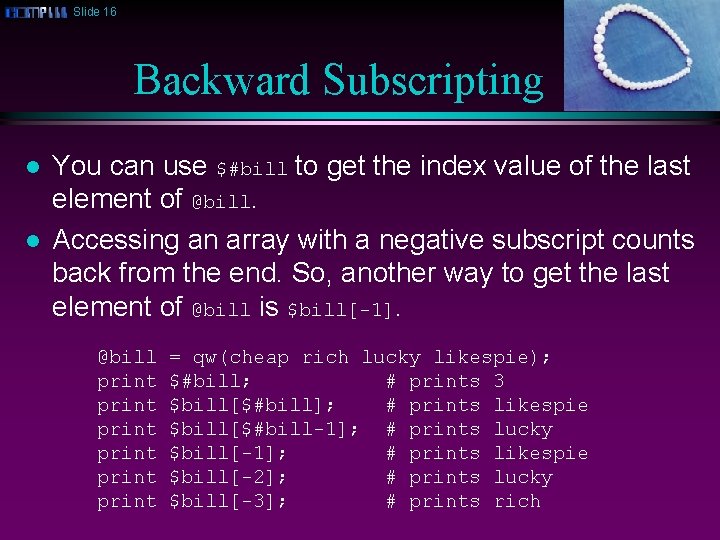
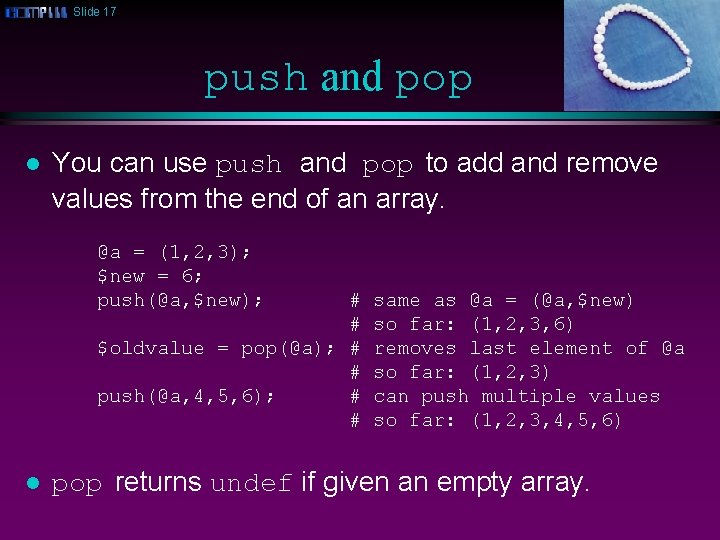
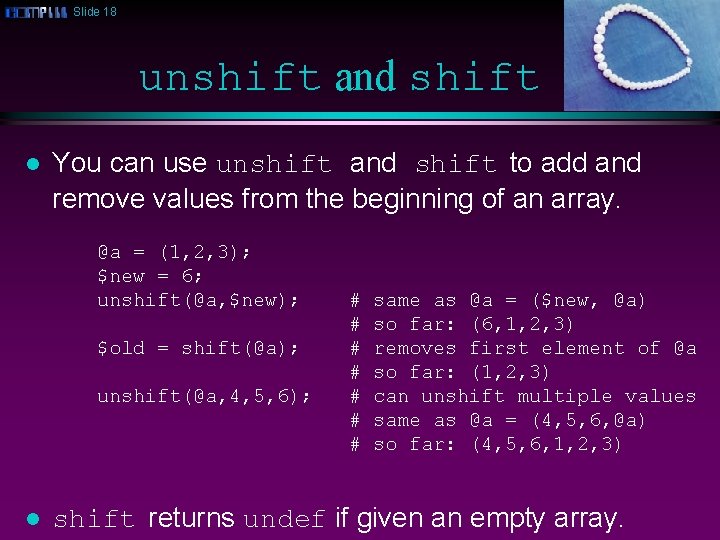
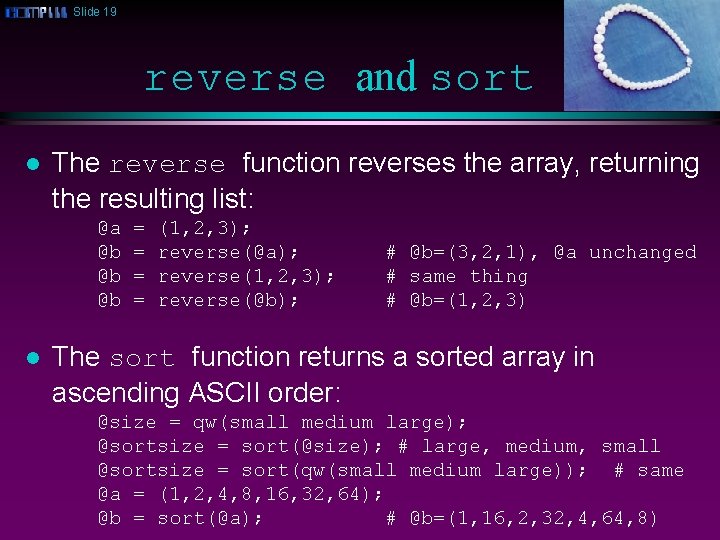
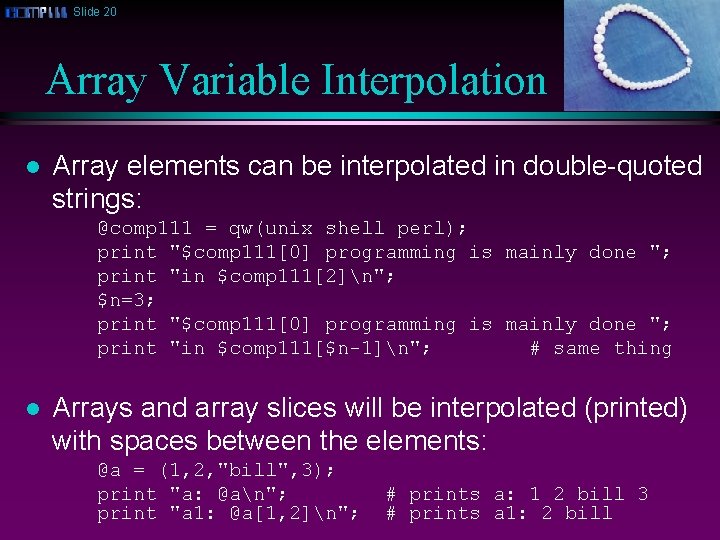
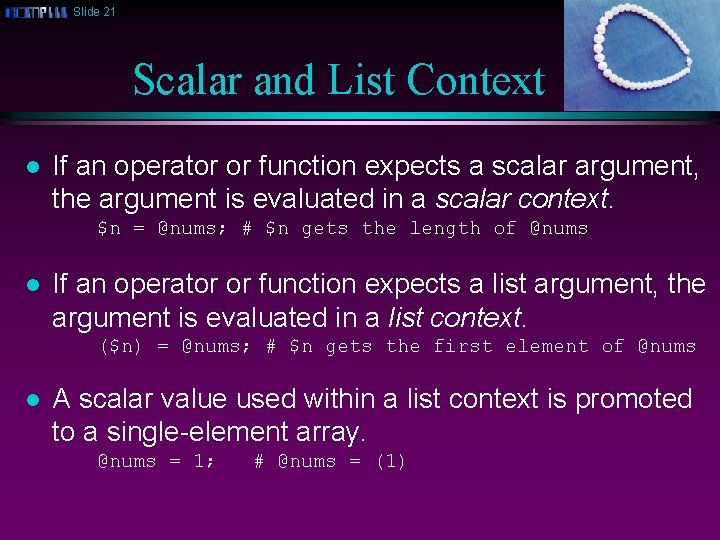
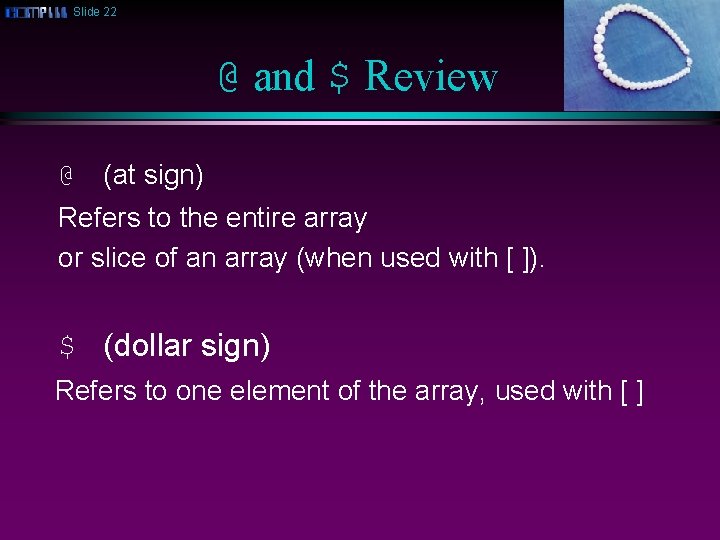
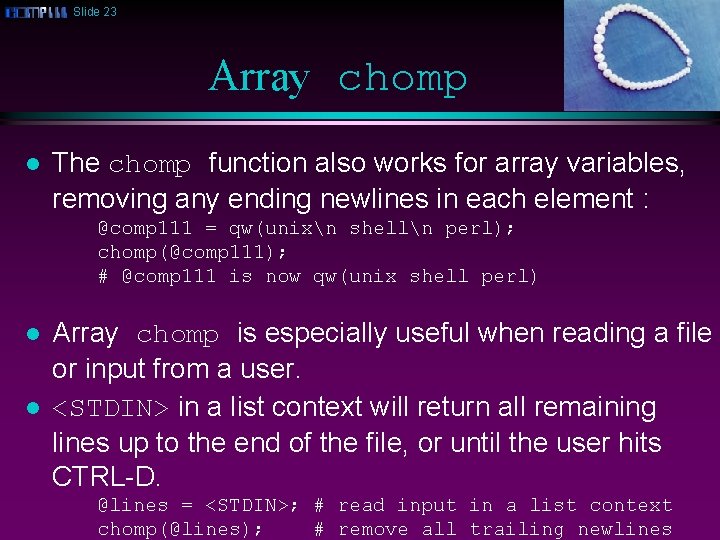
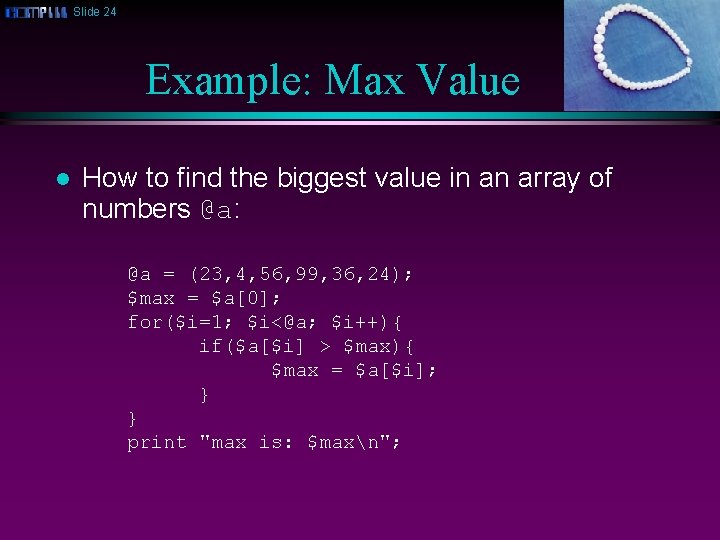
- Slides: 24
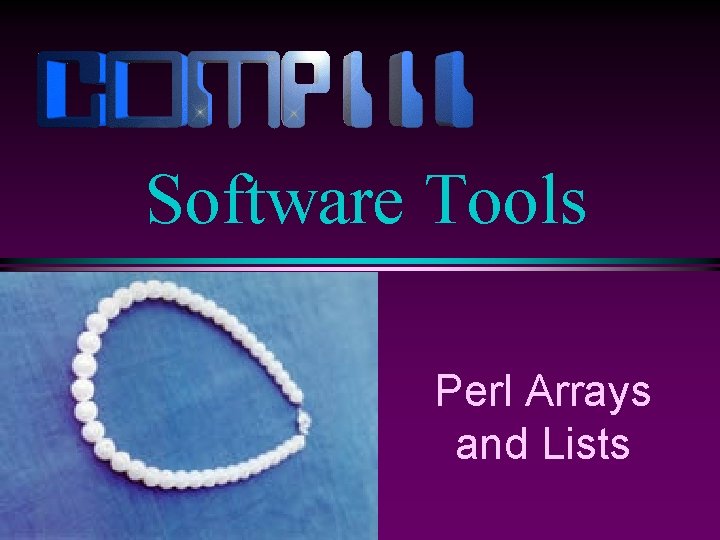
Software Tools Perl Arrays and Lists
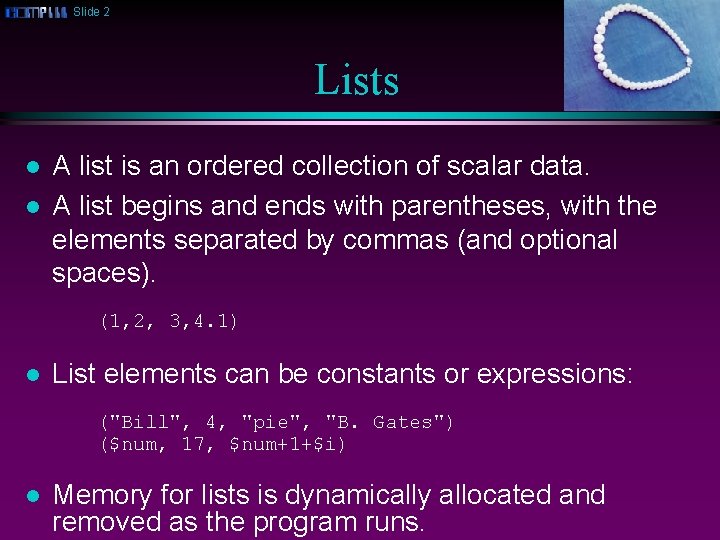
Slide 2 Lists l l A list is an ordered collection of scalar data. A list begins and ends with parentheses, with the elements separated by commas (and optional spaces). (1, 2, 3, 4. 1) l List elements can be constants or expressions: ("Bill", 4, "pie", "B. Gates") ($num, 17, $num+1+$i) l Memory for lists is dynamically allocated and removed as the program runs.
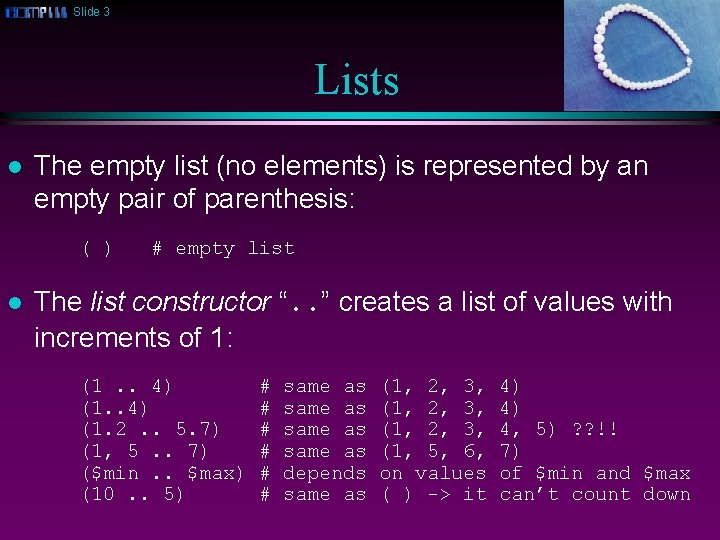
Slide 3 Lists l The empty list (no elements) is represented by an empty pair of parenthesis: ( ) l # empty list The list constructor “. . ” creates a list of values with increments of 1: (1. . 4) (1. 2. . 5. 7) (1, 5. . 7) ($min. . $max) (10. . 5) # # # same as depends same as (1, 2, 3, (1, 5, 6, on values ( ) -> it 4) 4) 4, 5) ? ? !! 7) of $min and $max can’t count down
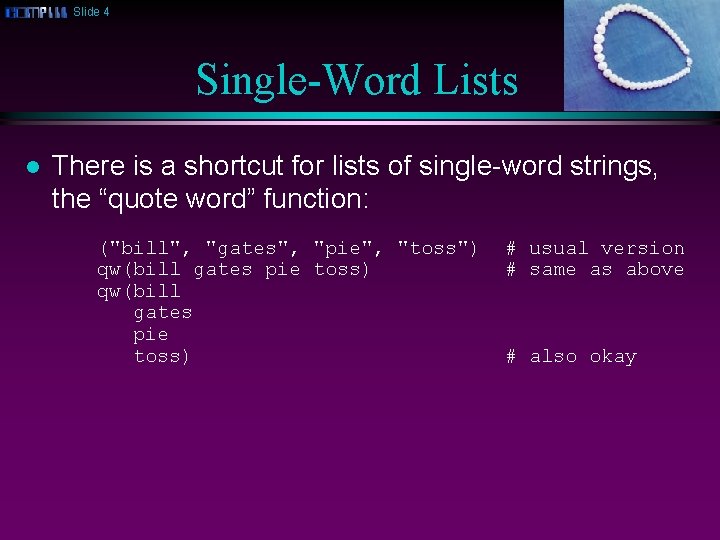
Slide 4 Single-Word Lists l There is a shortcut for lists of single-word strings, the “quote word” function: ("bill", "gates", "pie", "toss") qw(bill gates pie toss) # usual version # same as above # also okay
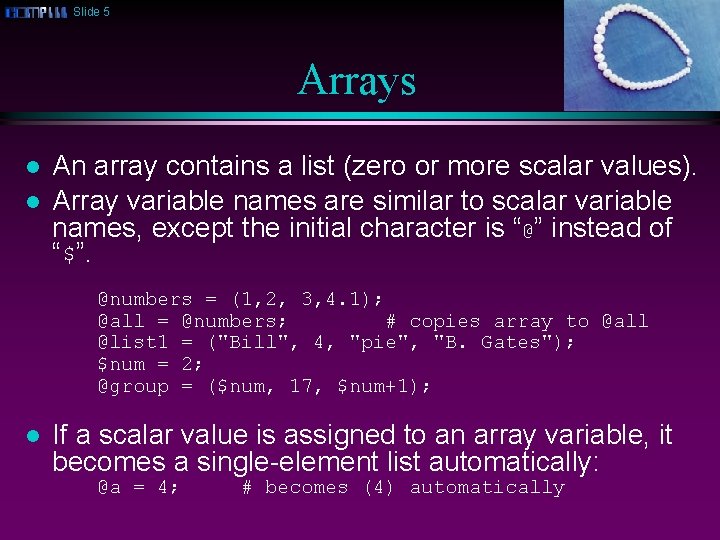
Slide 5 Arrays l l An array contains a list (zero or more scalar values). Array variable names are similar to scalar variable names, except the initial character is “@” instead of “$”. @numbers = (1, 2, 3, 4. 1); @all = @numbers; # copies array to @all @list 1 = ("Bill", 4, "pie", "B. Gates"); $num = 2; @group = ($num, 17, $num+1); l If a scalar value is assigned to an array variable, it becomes a single-element list automatically: @a = 4; # becomes (4) automatically
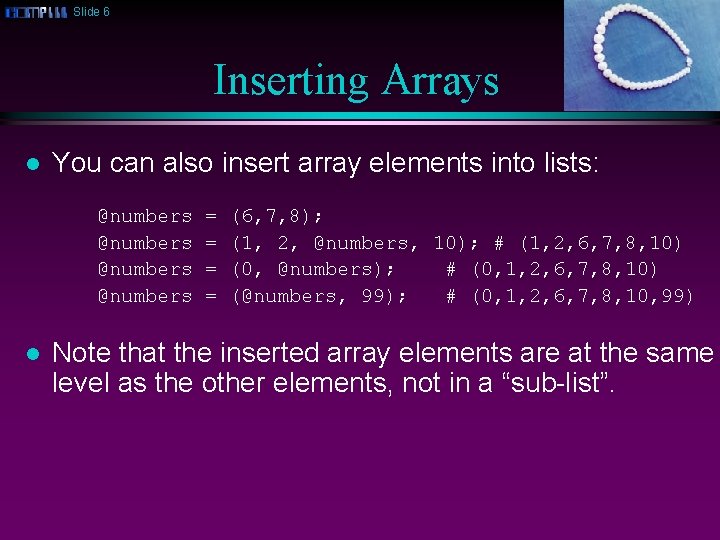
Slide 6 Inserting Arrays l You can also insert array elements into lists: @numbers l = = (6, 7, 8); (1, 2, @numbers, 10); # (1, 2, 6, 7, 8, 10) (0, @numbers); # (0, 1, 2, 6, 7, 8, 10) (@numbers, 99); # (0, 1, 2, 6, 7, 8, 10, 99) Note that the inserted array elements are at the same level as the other elements, not in a “sub-list”.
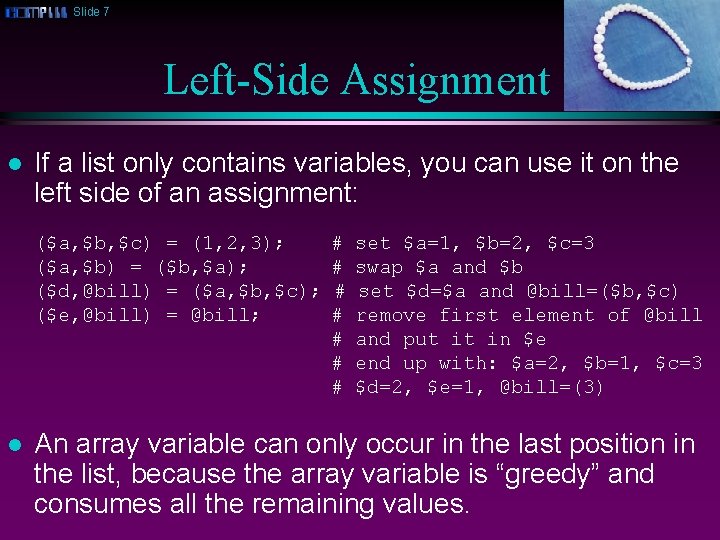
Slide 7 Left-Side Assignment l If a list only contains variables, you can use it on the left side of an assignment: ($a, $b, $c) = (1, 2, 3); ($a, $b) = ($b, $a); ($d, @bill) = ($a, $b, $c); ($e, @bill) = @bill; l # # # # set $a=1, $b=2, $c=3 swap $a and $b set $d=$a and @bill=($b, $c) remove first element of @bill and put it in $e end up with: $a=2, $b=1, $c=3 $d=2, $e=1, @bill=(3) An array variable can only occur in the last position in the list, because the array variable is “greedy” and consumes all the remaining values.
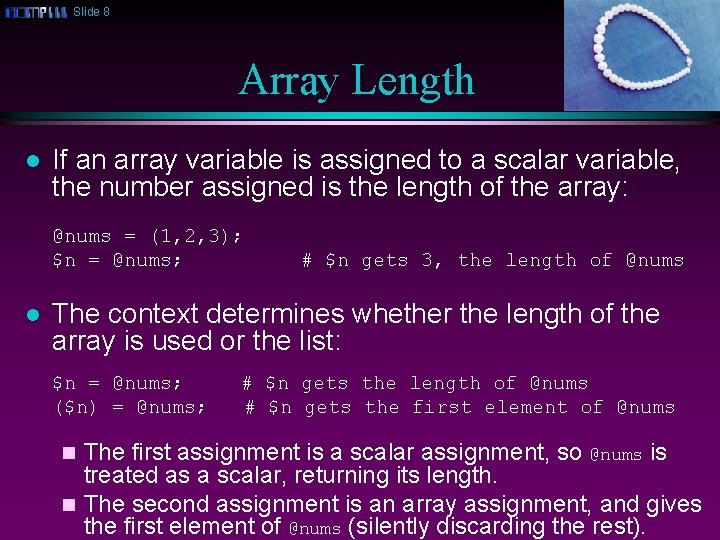
Slide 8 Array Length l If an array variable is assigned to a scalar variable, the number assigned is the length of the array: @nums = (1, 2, 3); $n = @nums; l # $n gets 3, the length of @nums The context determines whether the length of the array is used or the list: $n = @nums; ($n) = @nums; # $n gets the length of @nums # $n gets the first element of @nums The first assignment is a scalar assignment, so @nums is treated as a scalar, returning its length. n The second assignment is an array assignment, and gives the first element of @nums (silently discarding the rest). n
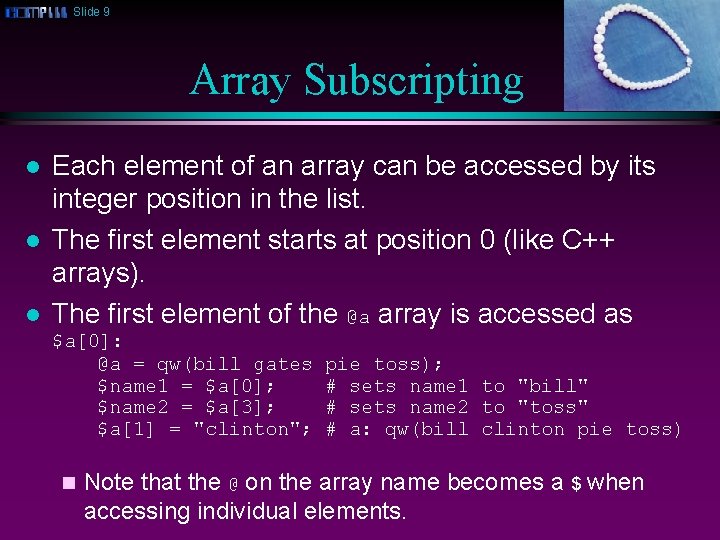
Slide 9 Array Subscripting l l l Each element of an array can be accessed by its integer position in the list. The first element starts at position 0 (like C++ arrays). The first element of the @a array is accessed as $a[0]: @a = qw(bill gates $name 1 = $a[0]; $name 2 = $a[3]; $a[1] = "clinton"; n pie toss); # sets name 1 to "bill" # sets name 2 to "toss" # a: qw(bill clinton pie toss) Note that the @ on the array name becomes a $ when accessing individual elements.
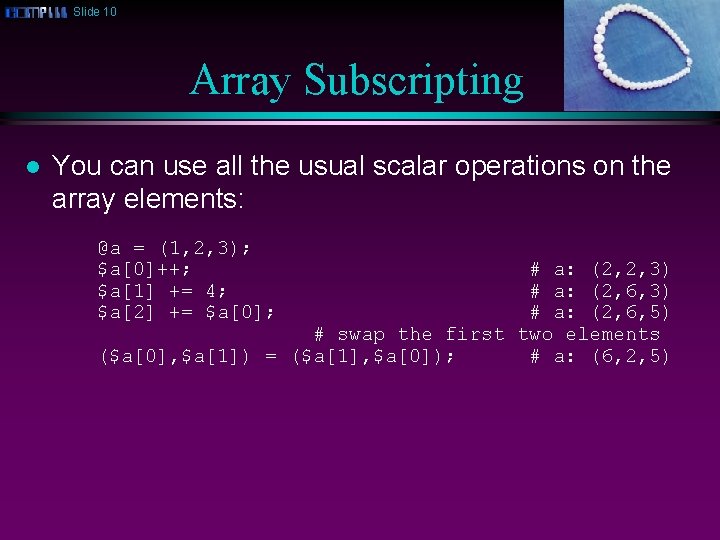
Slide 10 Array Subscripting l You can use all the usual scalar operations on the array elements: @a = (1, 2, 3); $a[0]++; $a[1] += 4; $a[2] += $a[0]; # a: (2, 2, 3) # a: (2, 6, 5) # swap the first two elements ($a[0], $a[1]) = ($a[1], $a[0]); # a: (6, 2, 5)
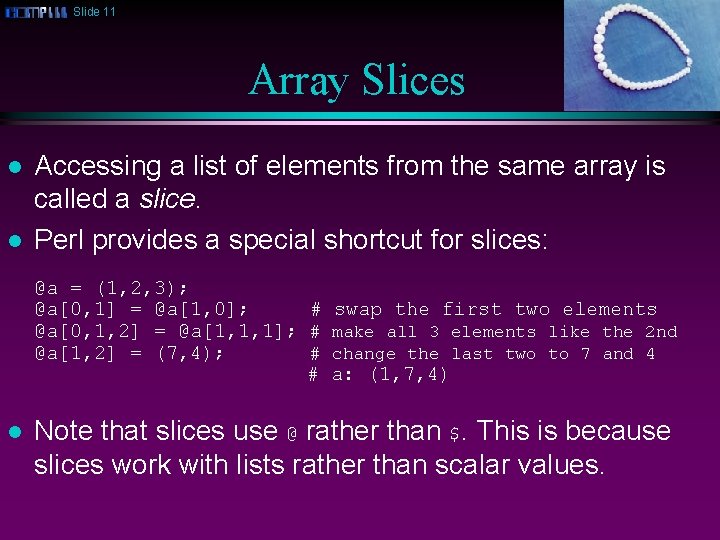
Slide 11 Array Slices l l Accessing a list of elements from the same array is called a slice. Perl provides a special shortcut for slices: @a = (1, 2, 3); @a[0, 1] = @a[1, 0]; # swap the first two elements @a[0, 1, 2] = @a[1, 1, 1]; # make all 3 elements like the 2 nd @a[1, 2] = (7, 4); # change the last two to 7 and 4 # a: (1, 7, 4) l Note that slices use @ rather than $. This is because slices work with lists rather than scalar values.
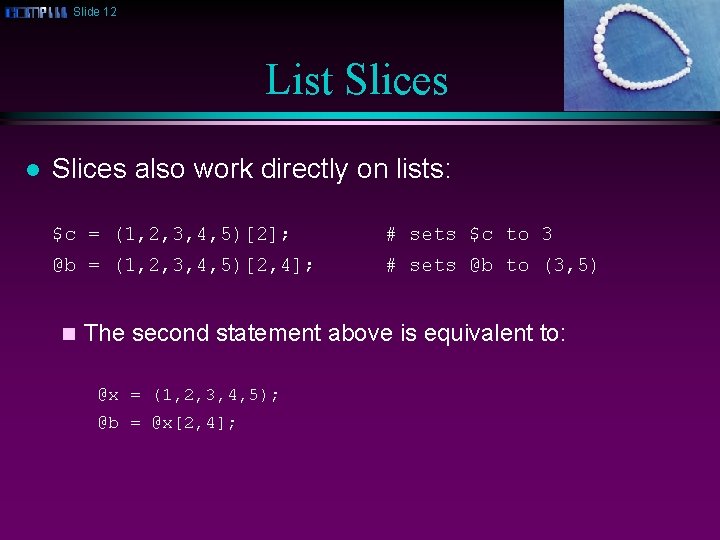
Slide 12 List Slices l Slices also work directly on lists: $c = (1, 2, 3, 4, 5)[2]; # sets $c to 3 @b = (1, 2, 3, 4, 5)[2, 4]; # sets @b to (3, 5) n The second statement above is equivalent to: @x = (1, 2, 3, 4, 5); @b = @x[2, 4];
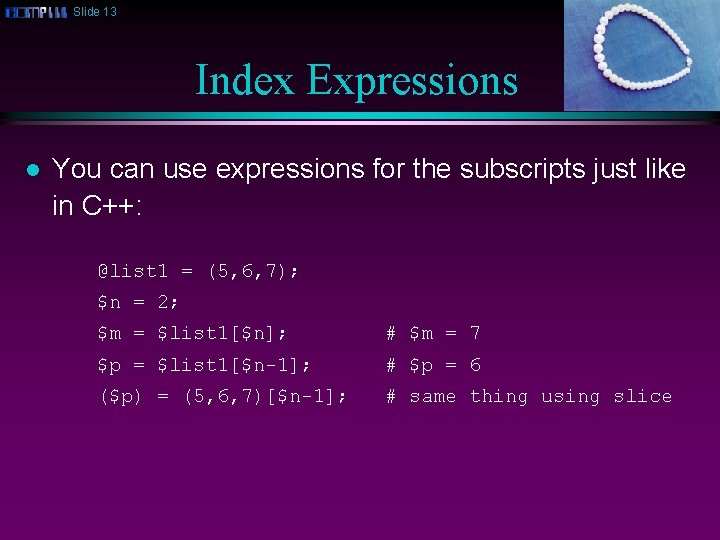
Slide 13 Index Expressions l You can use expressions for the subscripts just like in C++: @list 1 = (5, 6, 7); $n = 2; $m = $list 1[$n]; # $m = 7 $p = $list 1[$n-1]; # $p = 6 ($p) = (5, 6, 7)[$n-1]; # same thing using slice
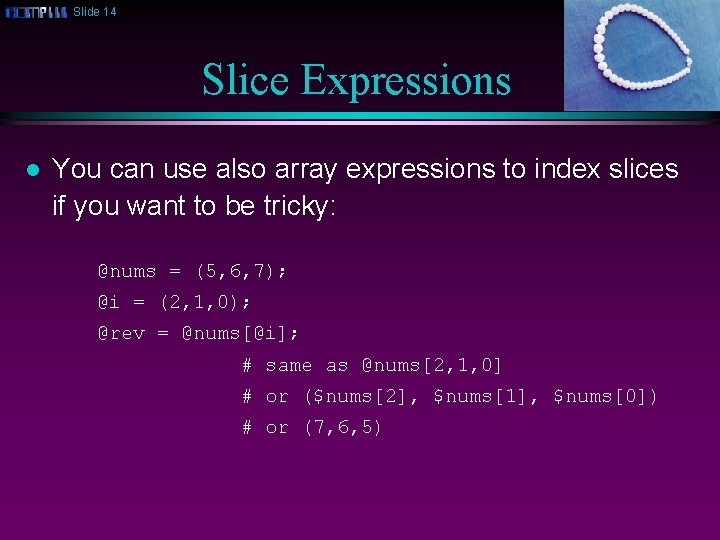
Slide 14 Slice Expressions l You can use also array expressions to index slices if you want to be tricky: @nums = (5, 6, 7); @i = (2, 1, 0); @rev = @nums[@i]; # same as @nums[2, 1, 0] # or ($nums[2], $nums[1], $nums[0]) # or (7, 6, 5)
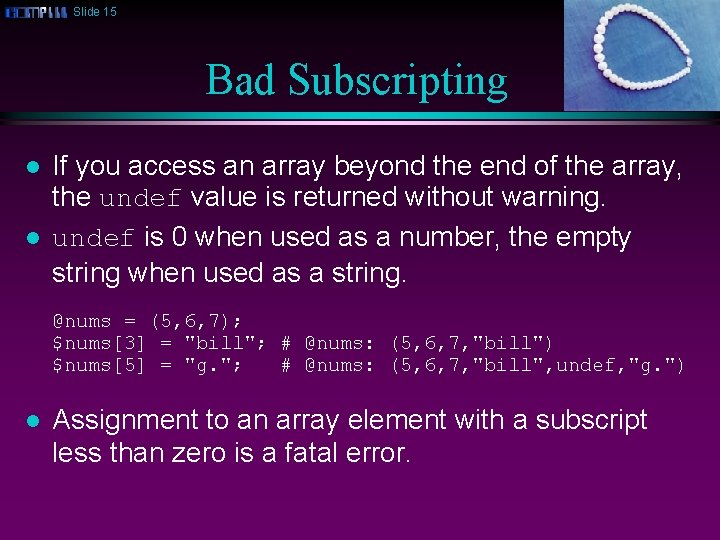
Slide 15 Bad Subscripting l l If you access an array beyond the end of the array, the undef value is returned without warning. undef is 0 when used as a number, the empty string when used as a string. @nums = (5, 6, 7); $nums[3] = "bill"; # @nums: (5, 6, 7, "bill") $nums[5] = "g. "; # @nums: (5, 6, 7, "bill", undef, "g. ") l Assignment to an array element with a subscript less than zero is a fatal error.
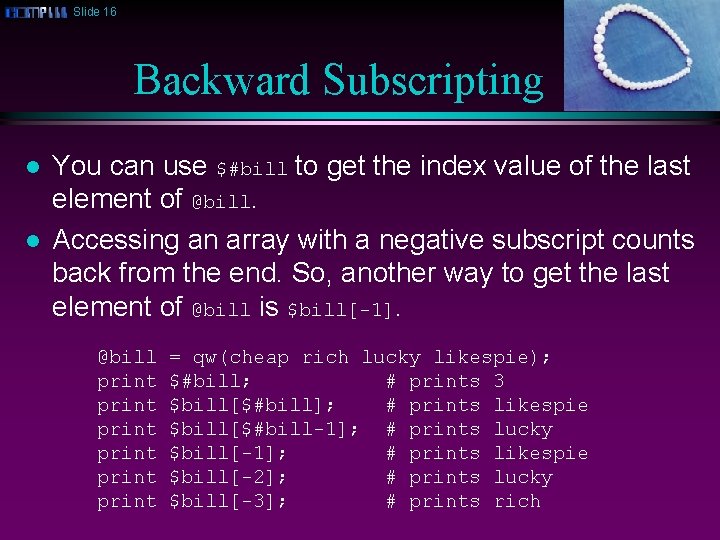
Slide 16 Backward Subscripting l l You can use $#bill to get the index value of the last element of @bill. Accessing an array with a negative subscript counts back from the end. So, another way to get the last element of @bill is $bill[-1]. @bill print print = qw(cheap rich lucky likespie); $#bill; # prints 3 $bill[$#bill]; # prints likespie $bill[$#bill-1]; # prints lucky $bill[-1]; # prints likespie $bill[-2]; # prints lucky $bill[-3]; # prints rich
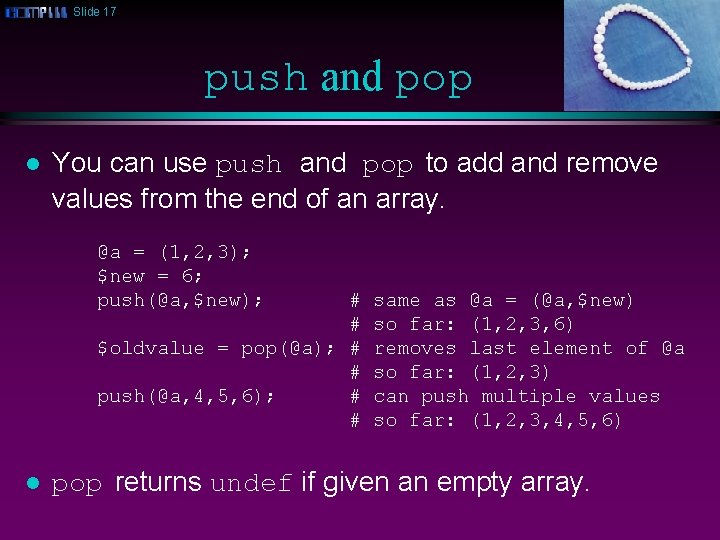
Slide 17 push and pop l You can use push and pop to add and remove values from the end of an array. @a = (1, 2, 3); $new = 6; push(@a, $new); # # $oldvalue = pop(@a); # # push(@a, 4, 5, 6); # # l same as @a = (@a, $new) so far: (1, 2, 3, 6) removes last element of @a so far: (1, 2, 3) can push multiple values so far: (1, 2, 3, 4, 5, 6) pop returns undef if given an empty array.
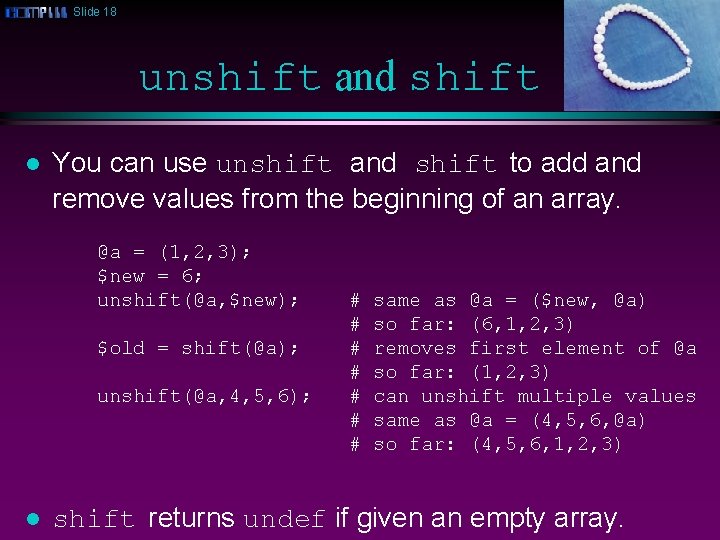
Slide 18 unshift and shift l You can use unshift and shift to add and remove values from the beginning of an array. @a = (1, 2, 3); $new = 6; unshift(@a, $new); $old = shift(@a); unshift(@a, 4, 5, 6); l # # # # same as @a = ($new, @a) so far: (6, 1, 2, 3) removes first element of @a so far: (1, 2, 3) can unshift multiple values same as @a = (4, 5, 6, @a) so far: (4, 5, 6, 1, 2, 3) shift returns undef if given an empty array.
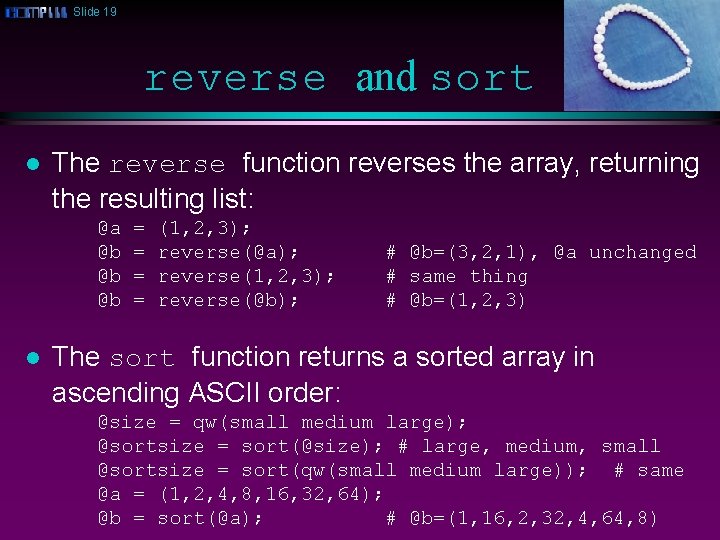
Slide 19 reverse and sort l The reverse function reverses the array, returning the resulting list: @a @b @b @b l = = (1, 2, 3); reverse(@a); reverse(1, 2, 3); reverse(@b); # @b=(3, 2, 1), @a unchanged # same thing # @b=(1, 2, 3) The sort function returns a sorted array in ascending ASCII order: @size = qw(small medium large); @sortsize = sort(@size); # large, medium, small @sortsize = sort(qw(small medium large)); # same @a = (1, 2, 4, 8, 16, 32, 64); @b = sort(@a); # @b=(1, 16, 2, 32, 4, 64, 8)
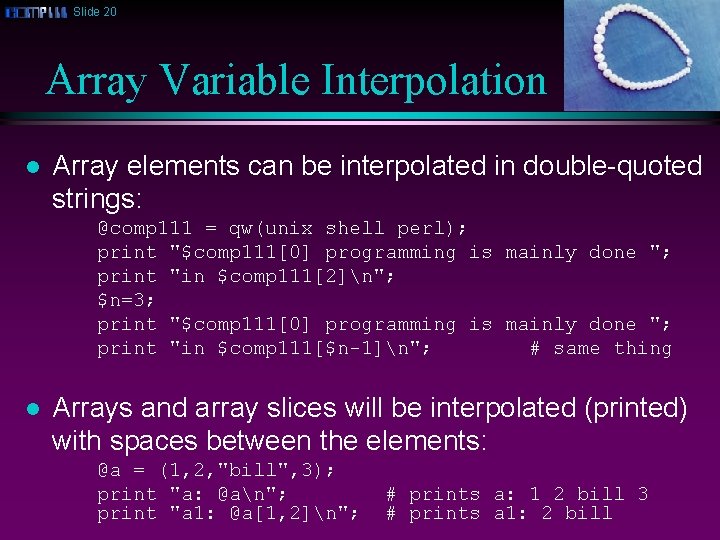
Slide 20 Array Variable Interpolation l Array elements can be interpolated in double-quoted strings: @comp 111 = qw(unix shell perl); print "$comp 111[0] programming is mainly done "; print "in $comp 111[2]n"; $n=3; print "$comp 111[0] programming is mainly done "; print "in $comp 111[$n-1]n"; # same thing l Arrays and array slices will be interpolated (printed) with spaces between the elements: @a = (1, 2, "bill", 3); print "a: @an"; print "a 1: @a[1, 2]n"; # prints a: 1 2 bill 3 # prints a 1: 2 bill
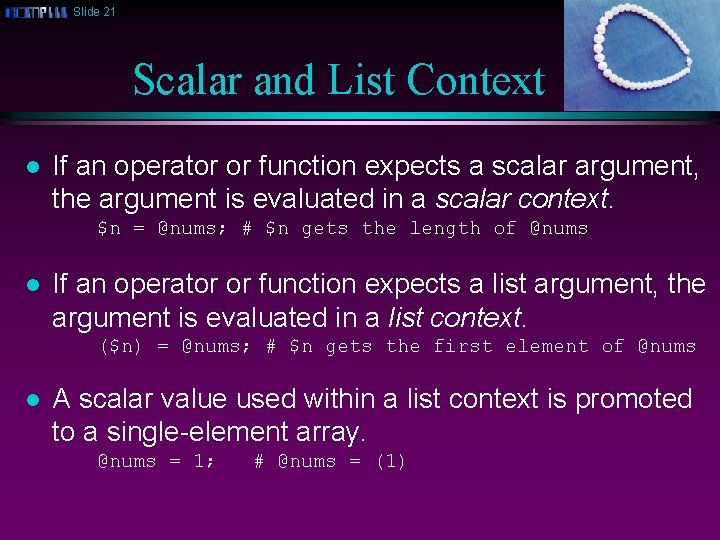
Slide 21 Scalar and List Context l If an operator or function expects a scalar argument, the argument is evaluated in a scalar context. $n = @nums; # $n gets the length of @nums l If an operator or function expects a list argument, the argument is evaluated in a list context. ($n) = @nums; # $n gets the first element of @nums l A scalar value used within a list context is promoted to a single-element array. @nums = 1; # @nums = (1)
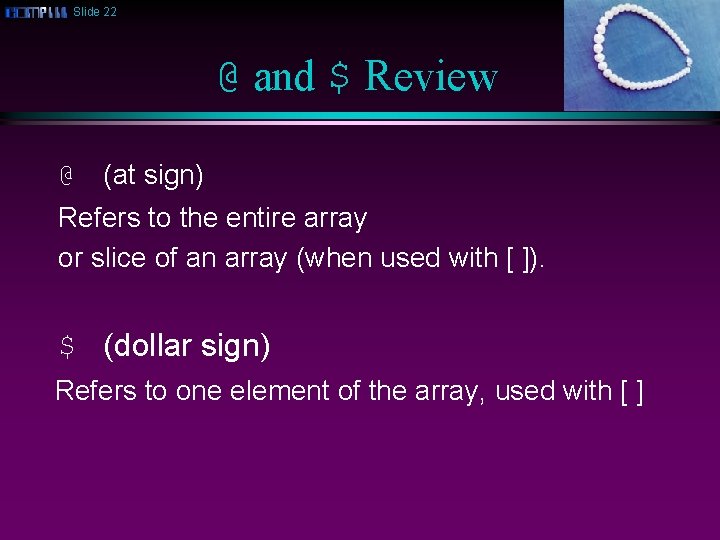
Slide 22 @ and $ Review @ (at sign) Refers to the entire array or slice of an array (when used with [ ]). $ (dollar sign) Refers to one element of the array, used with [ ]
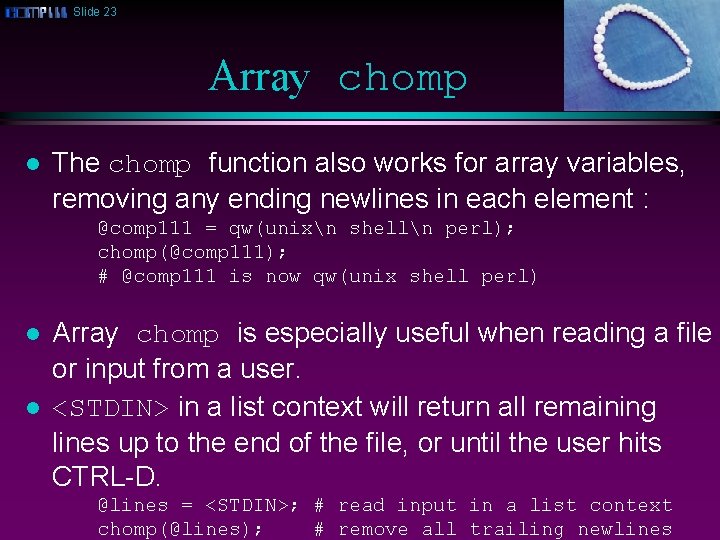
Slide 23 Array chomp l The chomp function also works for array variables, removing any ending newlines in each element : @comp 111 = qw(unixn shelln perl); chomp(@comp 111); # @comp 111 is now qw(unix shell perl) l l Array chomp is especially useful when reading a file or input from a user. <STDIN> in a list context will return all remaining lines up to the end of the file, or until the user hits CTRL-D. @lines = <STDIN>; # read input in a list context chomp(@lines); # remove all trailing newlines
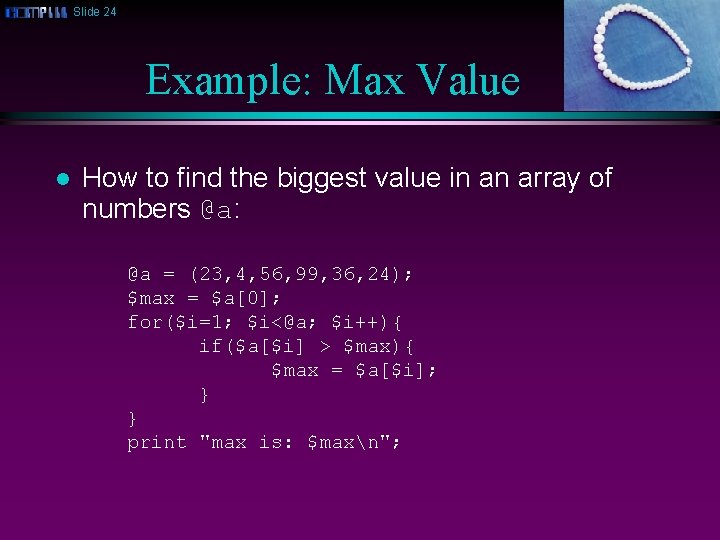
Slide 24 Example: Max Value l How to find the biggest value in an array of numbers @a: @a = (23, 4, 56, 99, 36, 24); $max = $a[0]; for($i=1; $i<@a; $i++){ if($a[$i] > $max){ $max = $a[$i]; } } print "max is: $maxn";