Scoping and Recursion CSC 171 FALL 2001 LECTURE
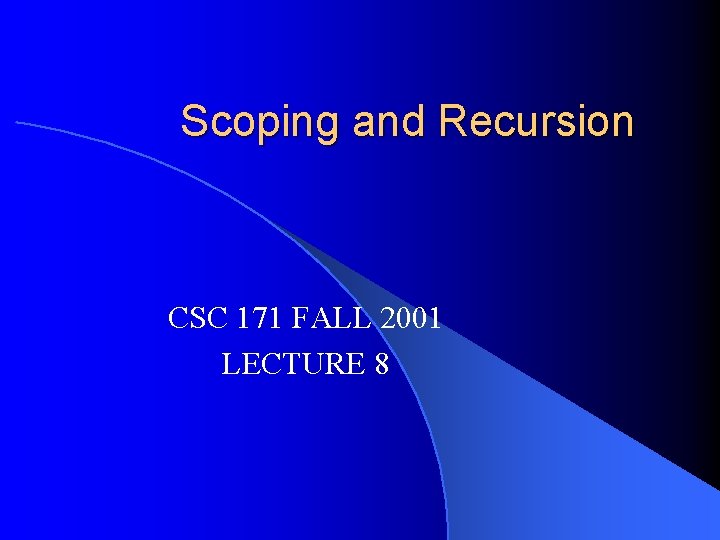
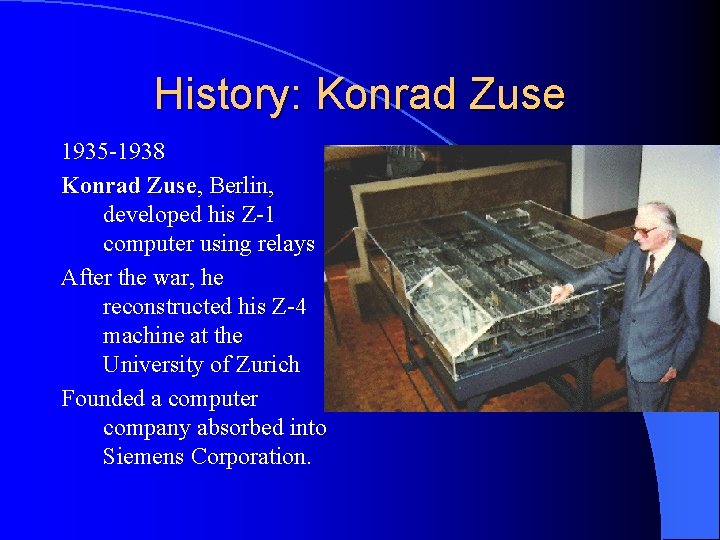
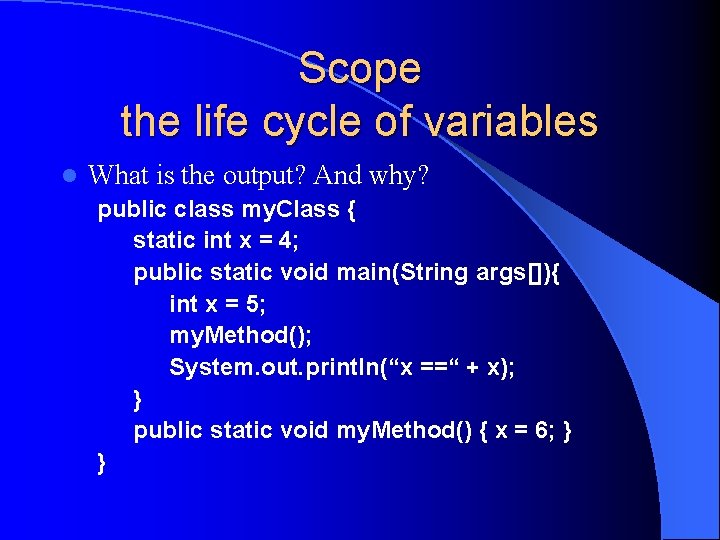
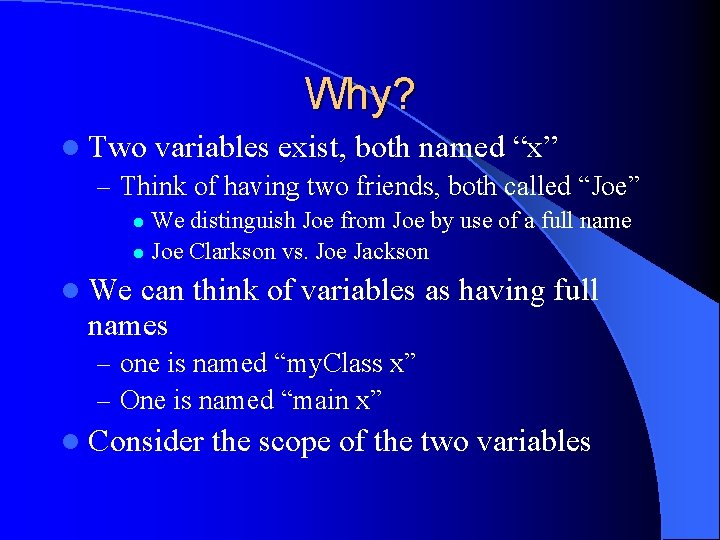
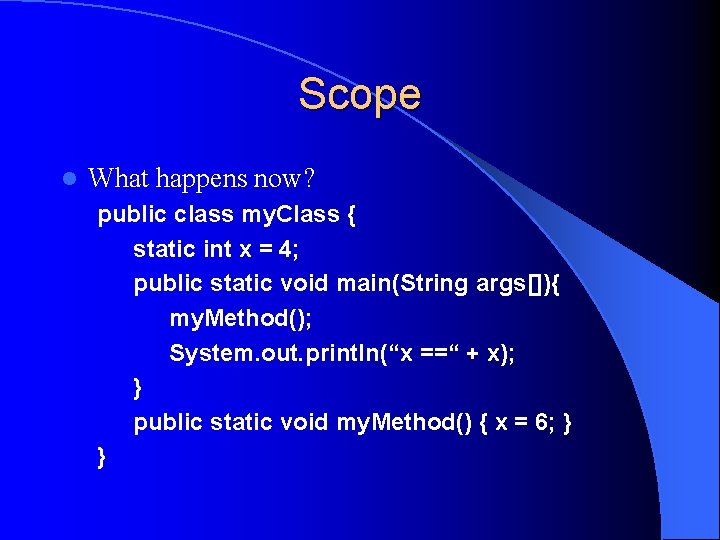
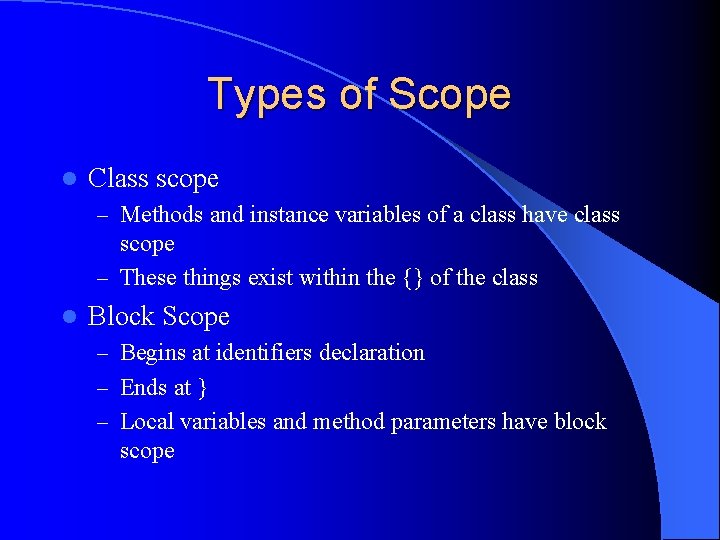
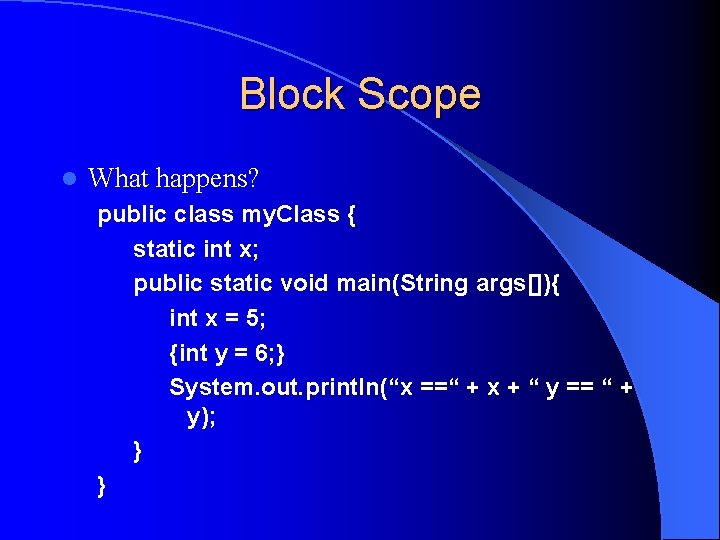
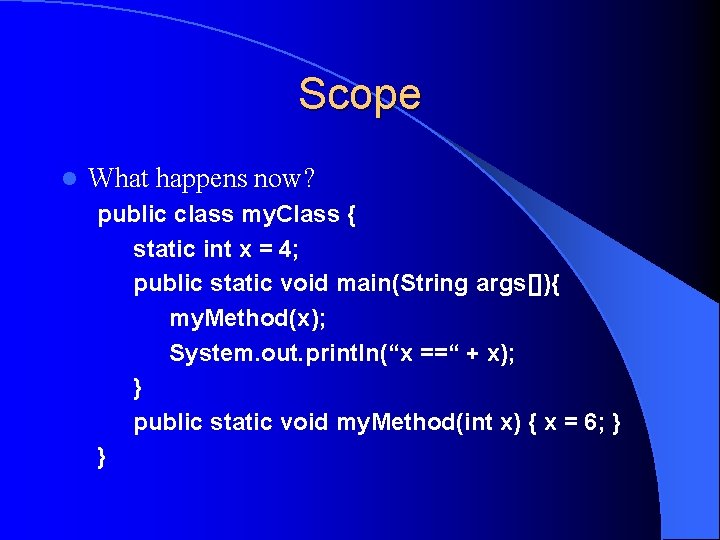
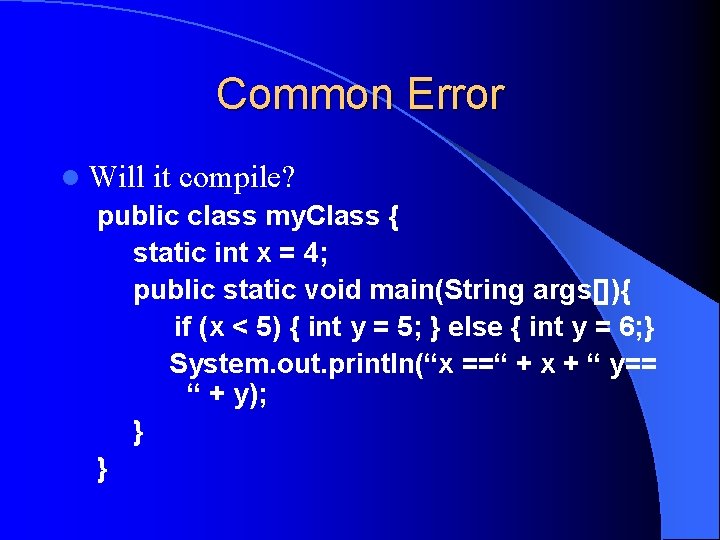
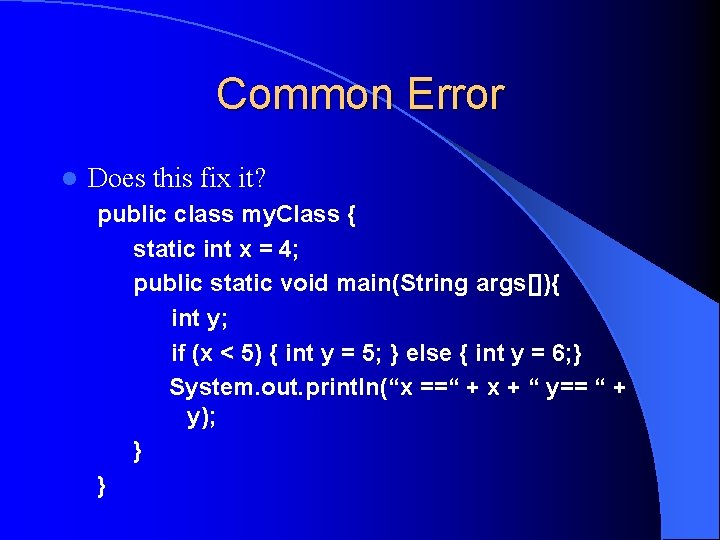
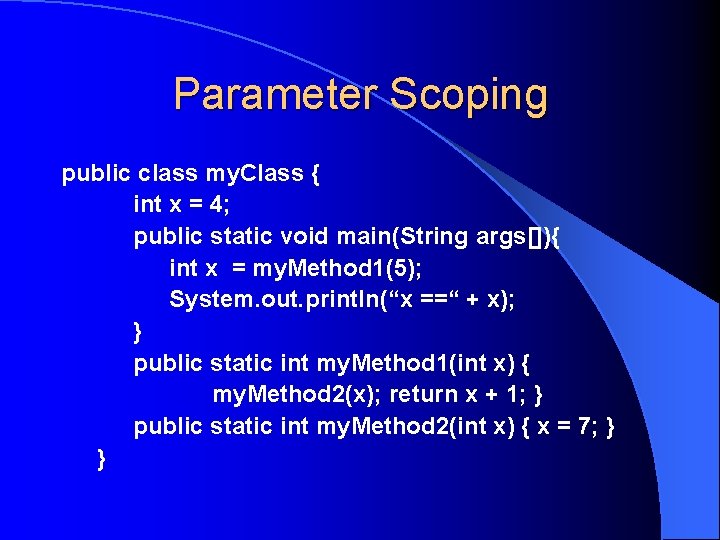
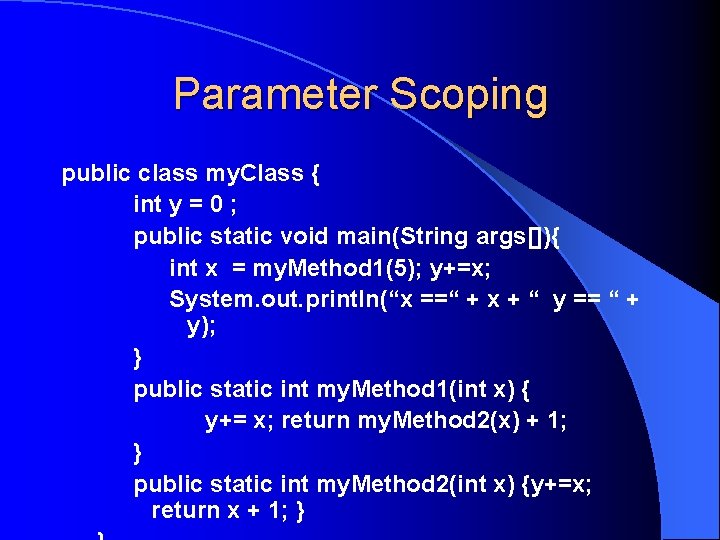
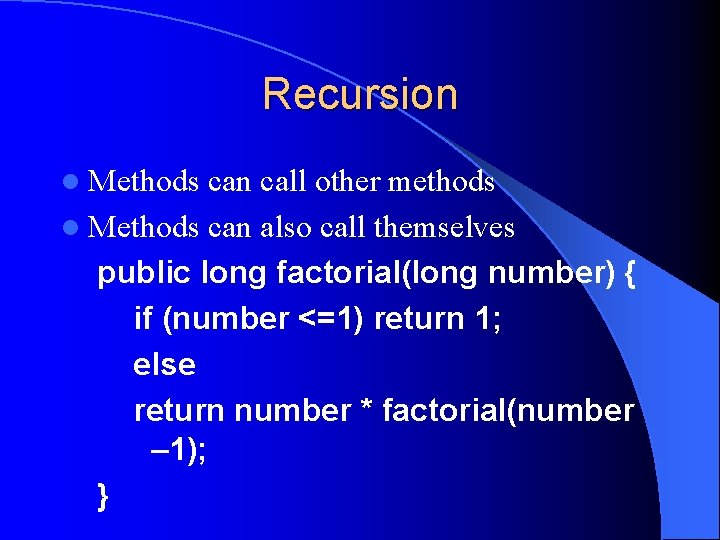
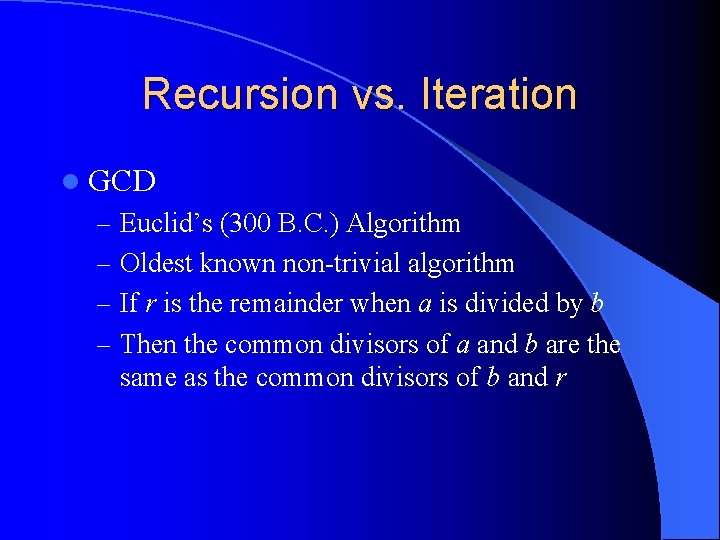
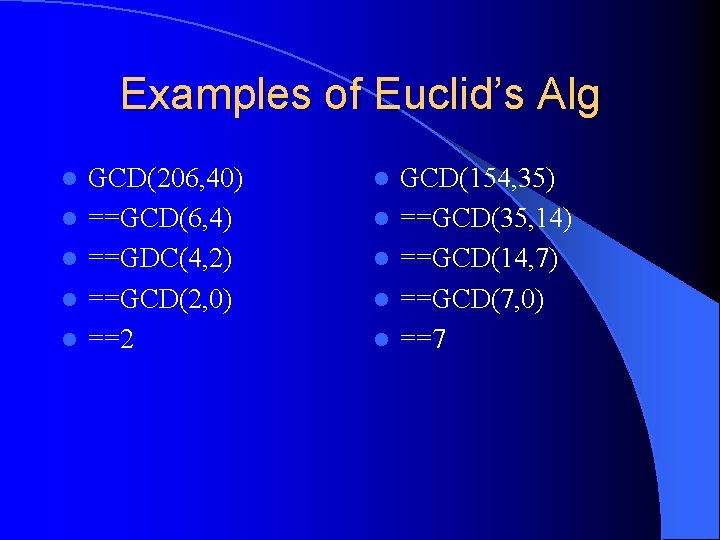
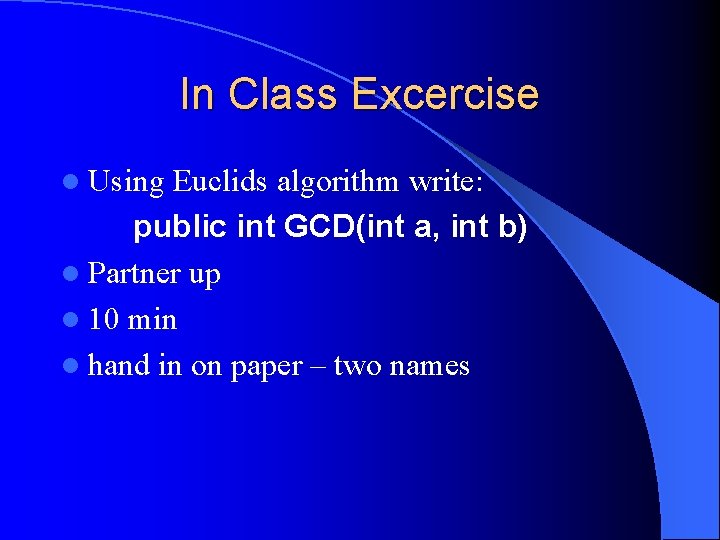
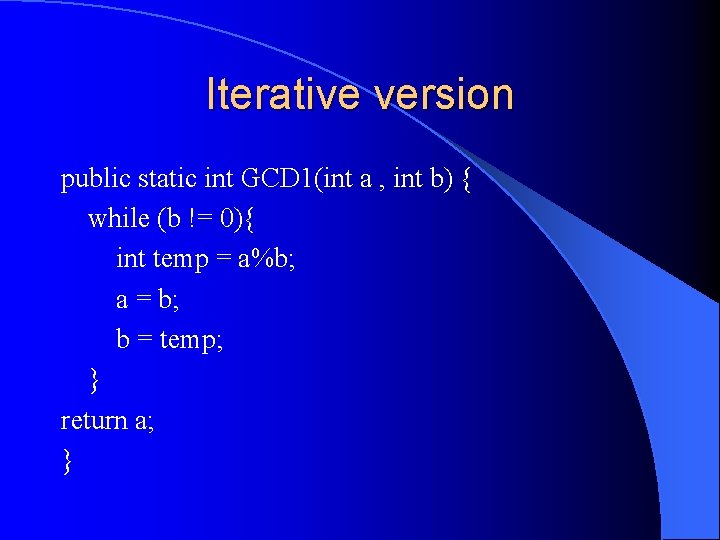
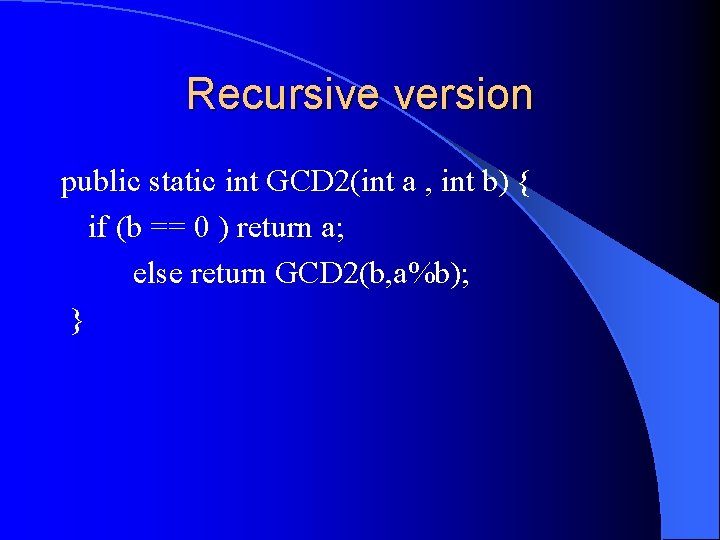
- Slides: 18
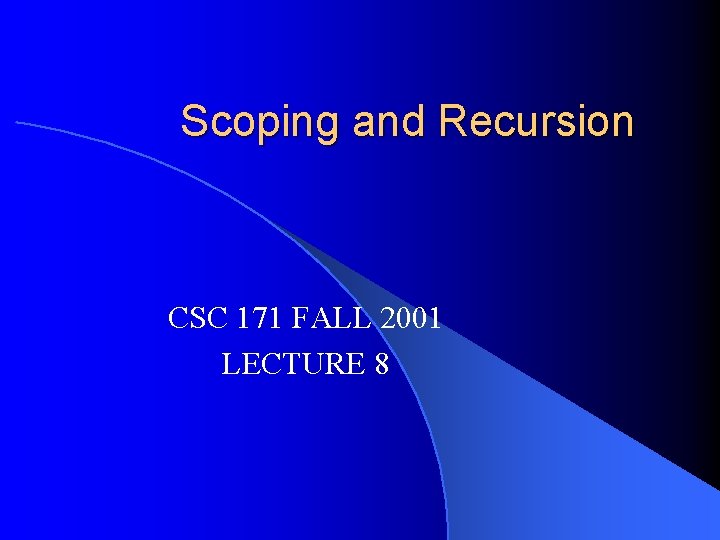
Scoping and Recursion CSC 171 FALL 2001 LECTURE 8
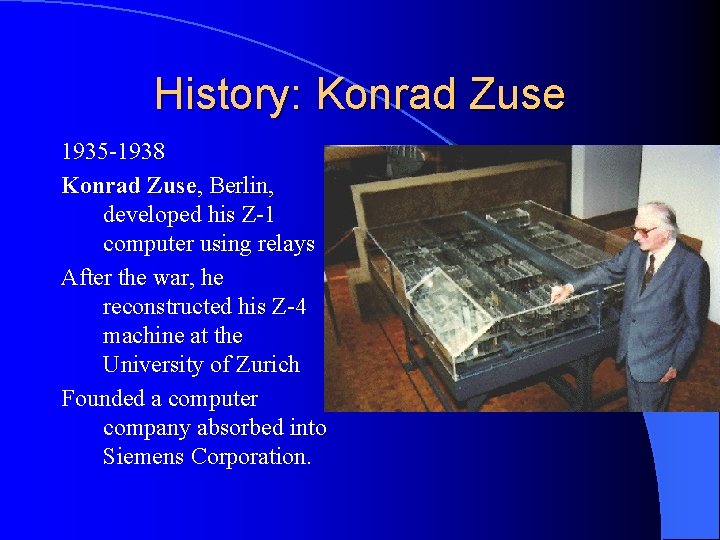
History: Konrad Zuse 1935 -1938 Konrad Zuse, Berlin, developed his Z-1 computer using relays After the war, he reconstructed his Z-4 machine at the University of Zurich Founded a computer company absorbed into Siemens Corporation.
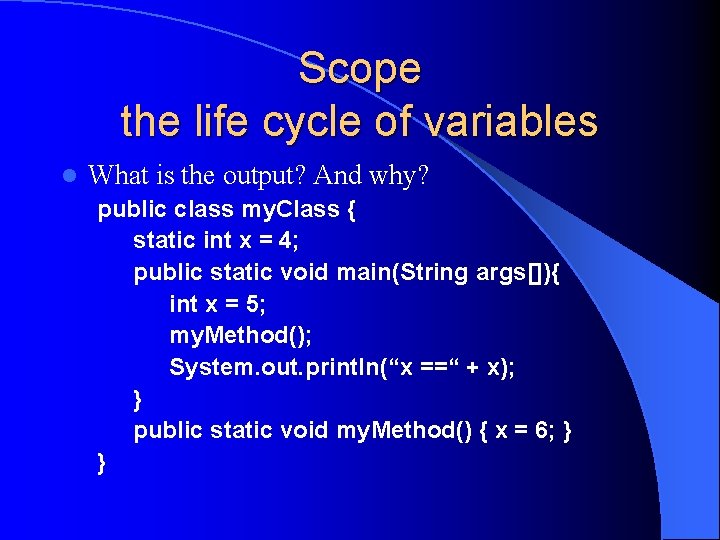
Scope the life cycle of variables l What is the output? And why? public class my. Class { static int x = 4; public static void main(String args[]){ int x = 5; my. Method(); System. out. println(“x ==“ + x); } public static void my. Method() { x = 6; } }
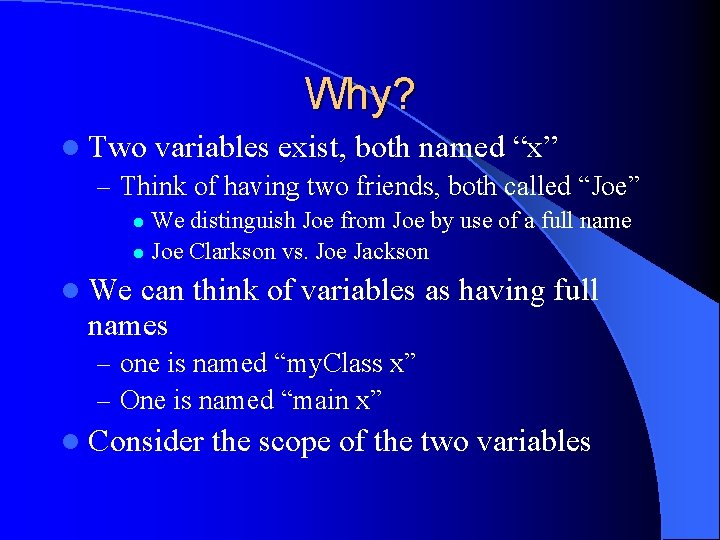
Why? l Two variables exist, both named “x” – Think of having two friends, both called “Joe” We distinguish Joe from Joe by use of a full name l Joe Clarkson vs. Joe Jackson l l We can think of variables as having full names – one is named “my. Class x” – One is named “main x” l Consider the scope of the two variables
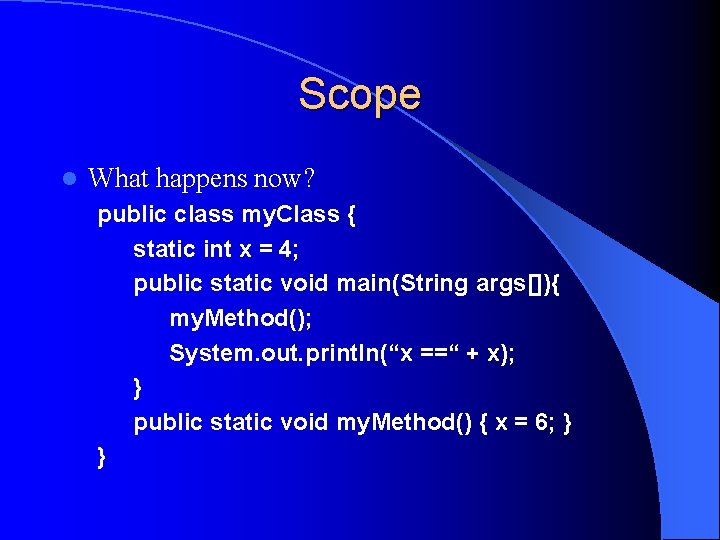
Scope l What happens now? public class my. Class { static int x = 4; public static void main(String args[]){ my. Method(); System. out. println(“x ==“ + x); } public static void my. Method() { x = 6; } }
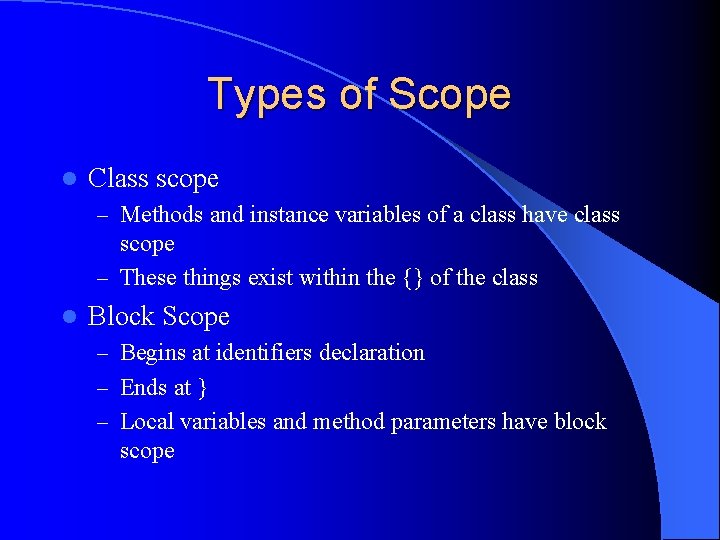
Types of Scope l Class scope – Methods and instance variables of a class have class scope – These things exist within the {} of the class l Block Scope – Begins at identifiers declaration – Ends at } – Local variables and method parameters have block scope
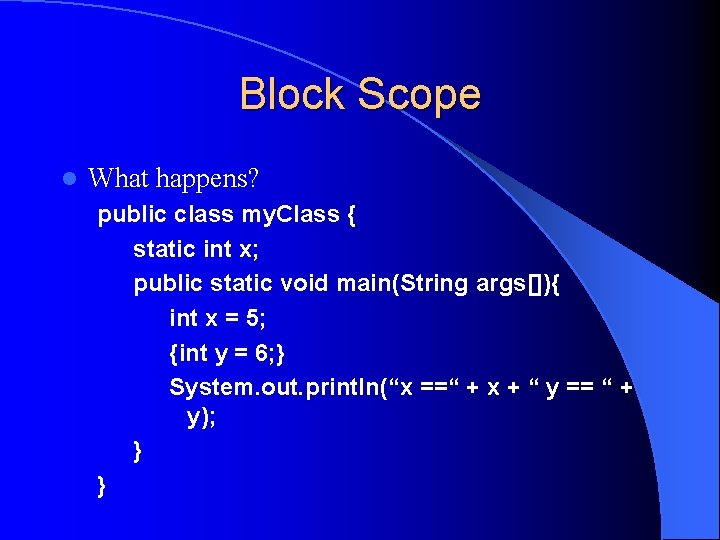
Block Scope l What happens? public class my. Class { static int x; public static void main(String args[]){ int x = 5; {int y = 6; } System. out. println(“x ==“ + x + “ y == “ + y); } }
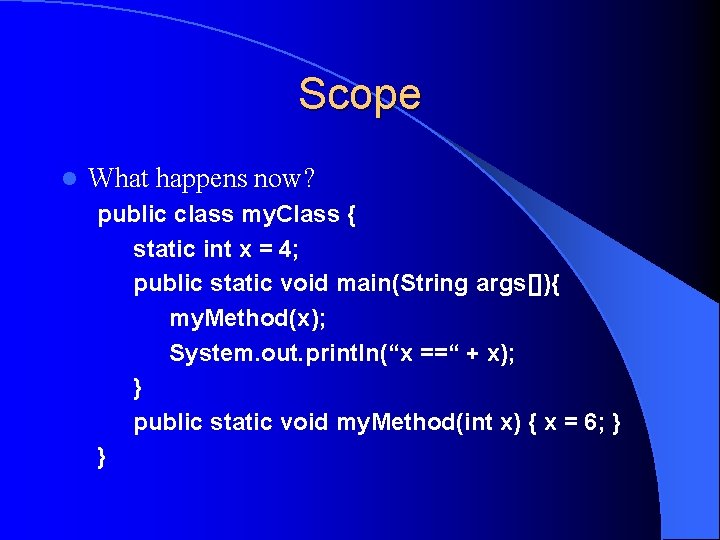
Scope l What happens now? public class my. Class { static int x = 4; public static void main(String args[]){ my. Method(x); System. out. println(“x ==“ + x); } public static void my. Method(int x) { x = 6; } }
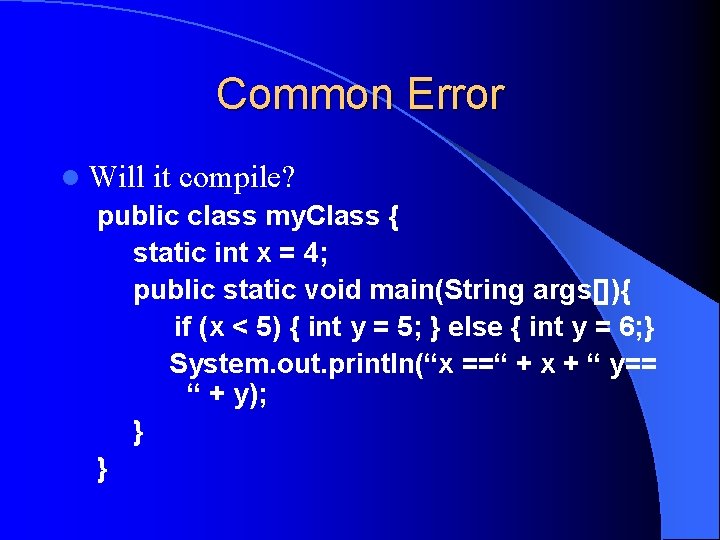
Common Error l Will it compile? public class my. Class { static int x = 4; public static void main(String args[]){ if (x < 5) { int y = 5; } else { int y = 6; } System. out. println(“x ==“ + x + “ y== “ + y); } }
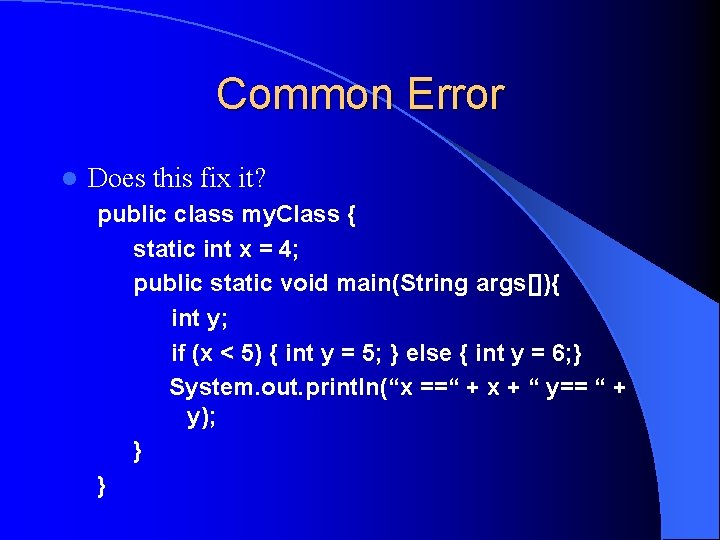
Common Error l Does this fix it? public class my. Class { static int x = 4; public static void main(String args[]){ int y; if (x < 5) { int y = 5; } else { int y = 6; } System. out. println(“x ==“ + x + “ y== “ + y); } }
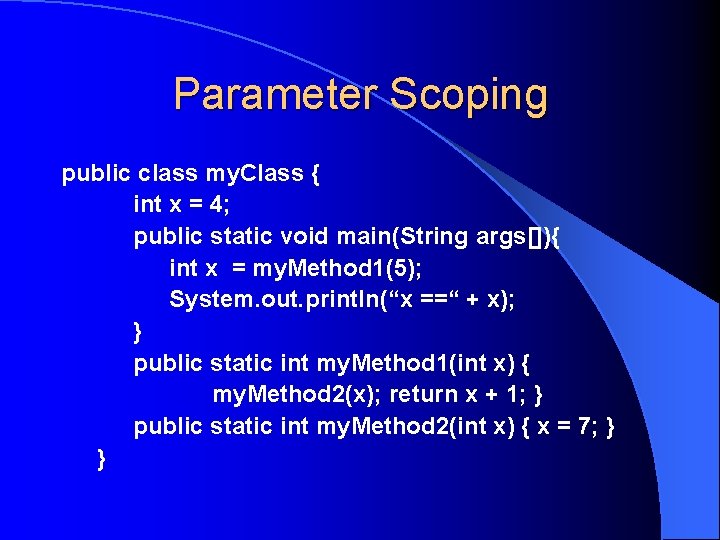
Parameter Scoping public class my. Class { int x = 4; public static void main(String args[]){ int x = my. Method 1(5); System. out. println(“x ==“ + x); } public static int my. Method 1(int x) { my. Method 2(x); return x + 1; } public static int my. Method 2(int x) { x = 7; } }
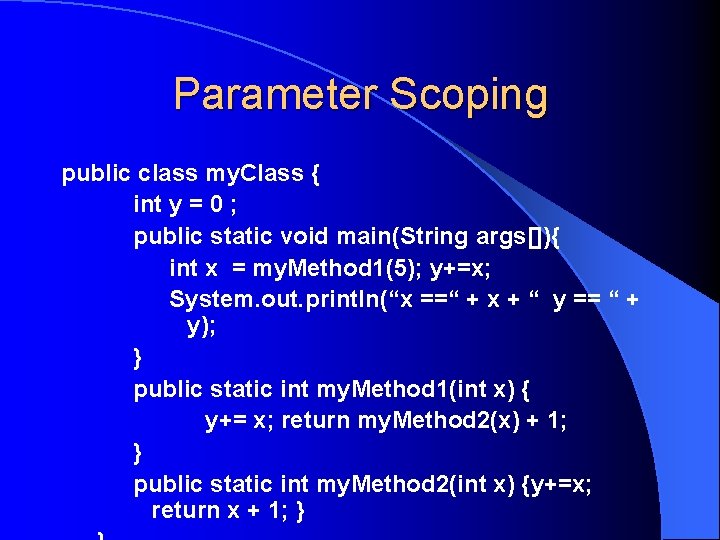
Parameter Scoping public class my. Class { int y = 0 ; public static void main(String args[]){ int x = my. Method 1(5); y+=x; System. out. println(“x ==“ + x + “ y == “ + y); } public static int my. Method 1(int x) { y+= x; return my. Method 2(x) + 1; } public static int my. Method 2(int x) {y+=x; return x + 1; }
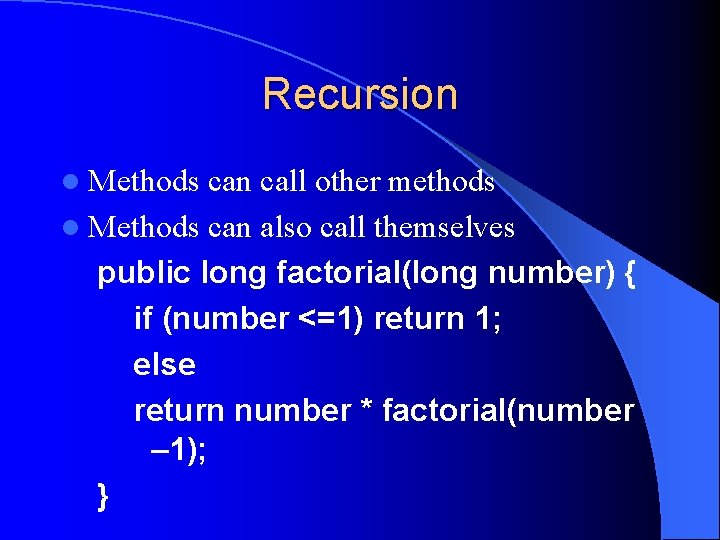
Recursion l Methods can call other methods l Methods can also call themselves public long factorial(long number) { if (number <=1) return 1; else return number * factorial(number – 1); }
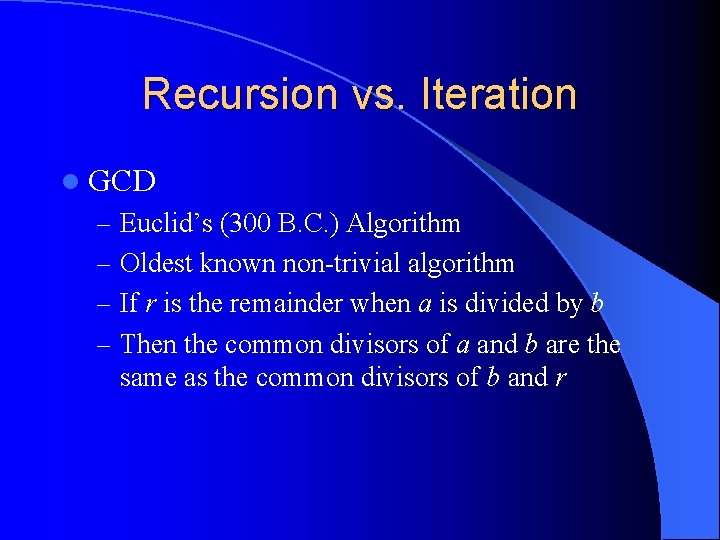
Recursion vs. Iteration l GCD – Euclid’s (300 B. C. ) Algorithm – Oldest known non-trivial algorithm – If r is the remainder when a is divided by b – Then the common divisors of a and b are the same as the common divisors of b and r
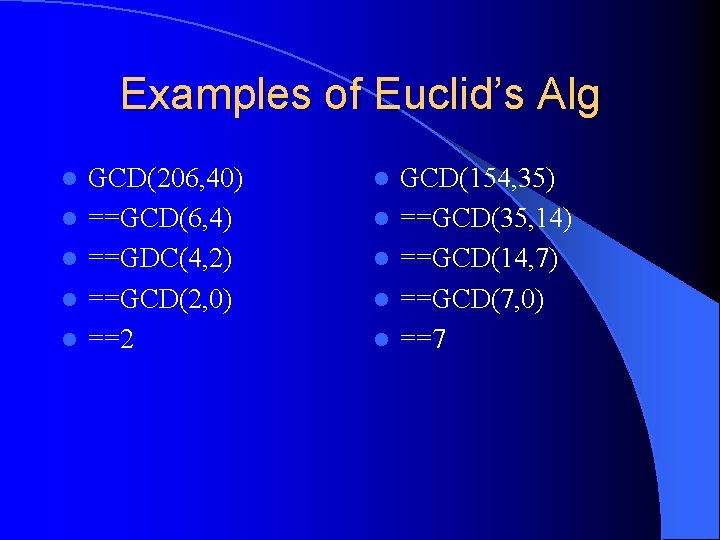
Examples of Euclid’s Alg l l l GCD(206, 40) ==GCD(6, 4) ==GDC(4, 2) ==GCD(2, 0) ==2 l l l GCD(154, 35) ==GCD(35, 14) ==GCD(14, 7) ==GCD(7, 0) ==7
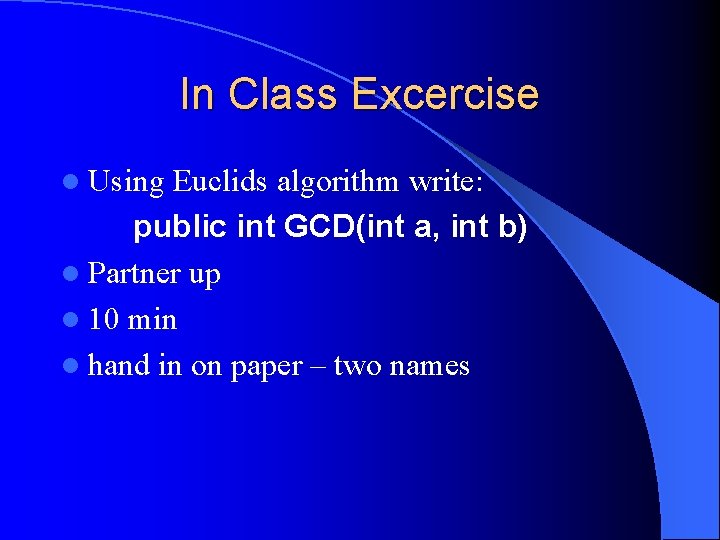
In Class Excercise l Using Euclids algorithm write: public int GCD(int a, int b) l Partner up l 10 min l hand in on paper – two names
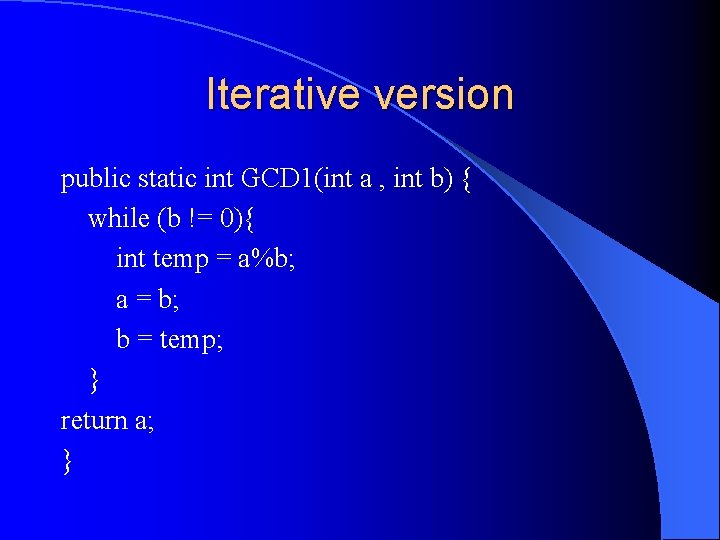
Iterative version public static int GCD 1(int a , int b) { while (b != 0){ int temp = a%b; a = b; b = temp; } return a; }
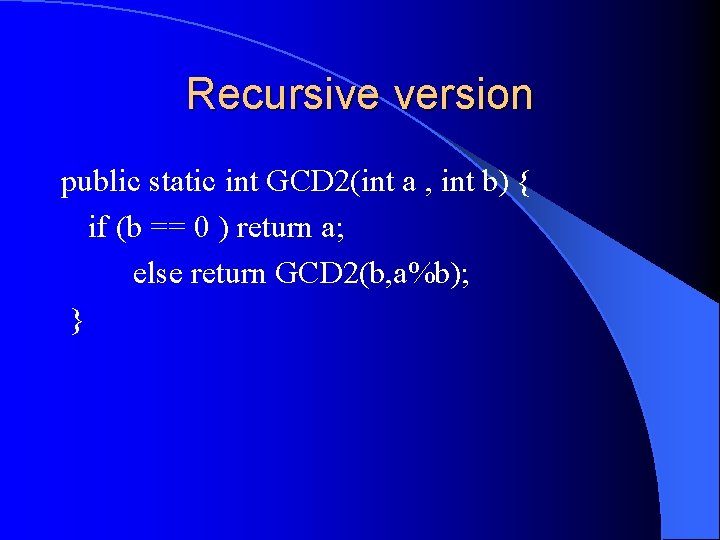
Recursive version public static int GCD 2(int a , int b) { if (b == 0 ) return a; else return GCD 2(b, a%b); }
To understand recursion you must understand recursion
How do you undertake community scoping
Problem scoping
Project scoping exercise
Project scoping
Dynamic scoping
01:640:244 lecture notes - lecture 15: plat, idah, farad
Pg 171
29052007
Present progressive irregular forms (p. 171)
Nonlinear pricing example
Ai 171
Ai 171
Tax allowance meaning
171 nomreli mekteb
171 nomreli mekteb
Decret 171/2015
Hazmat material table
Cs 171 uci