Recursive descent parsing The Stack n One easy
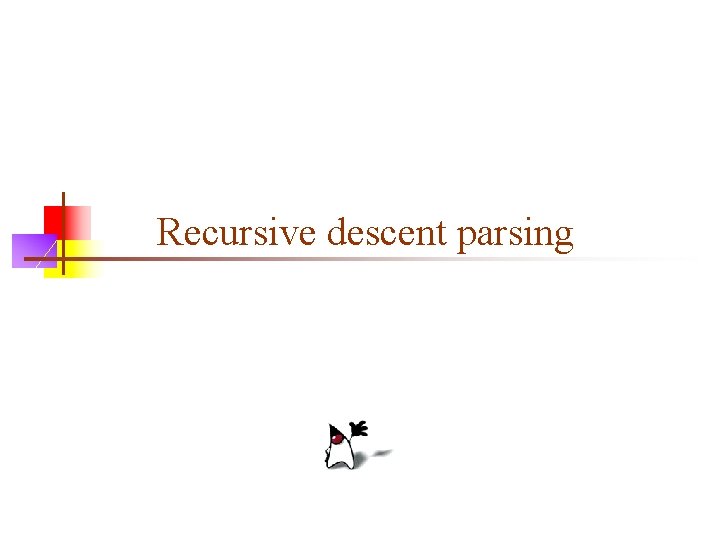
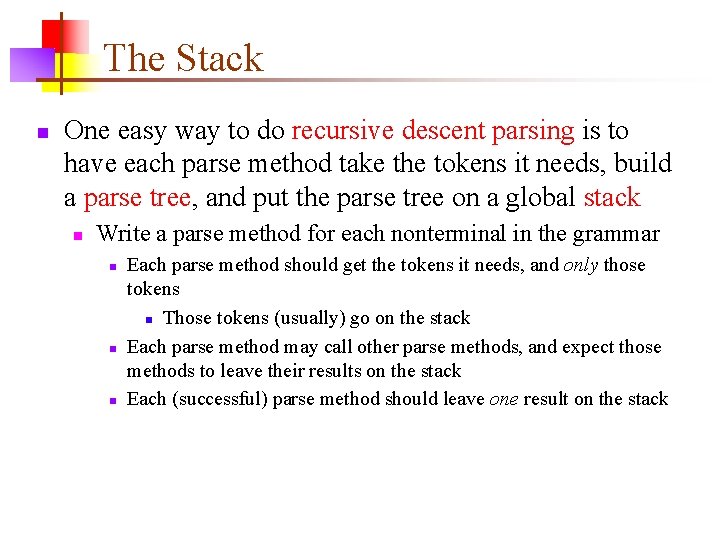
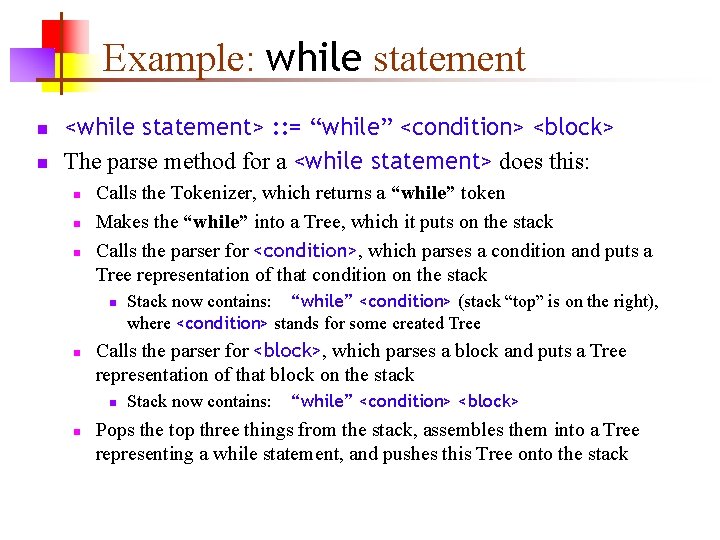
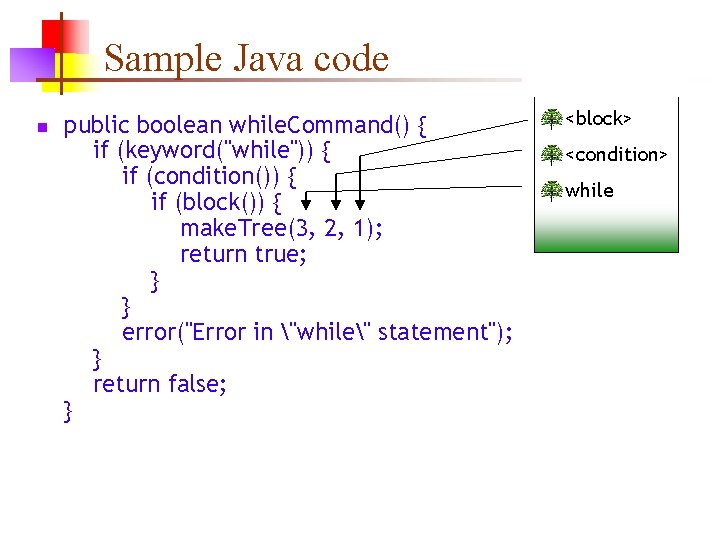
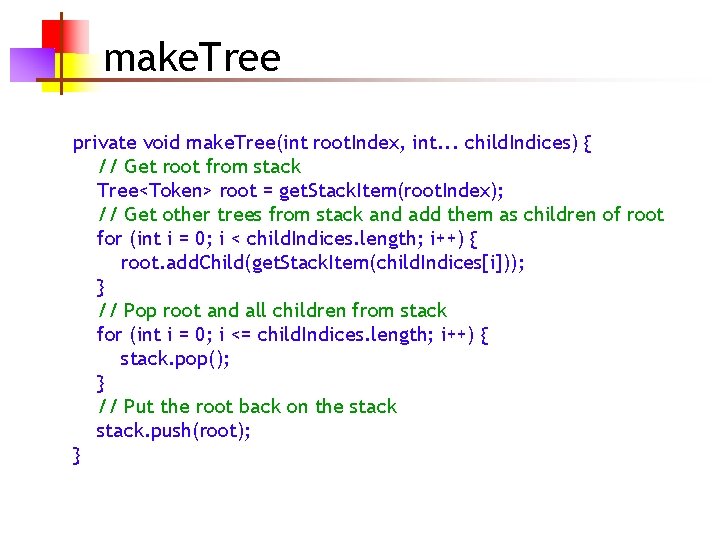
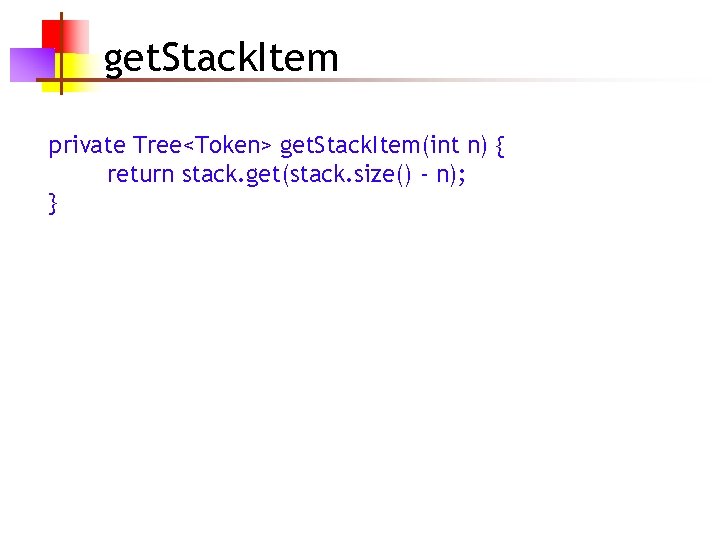
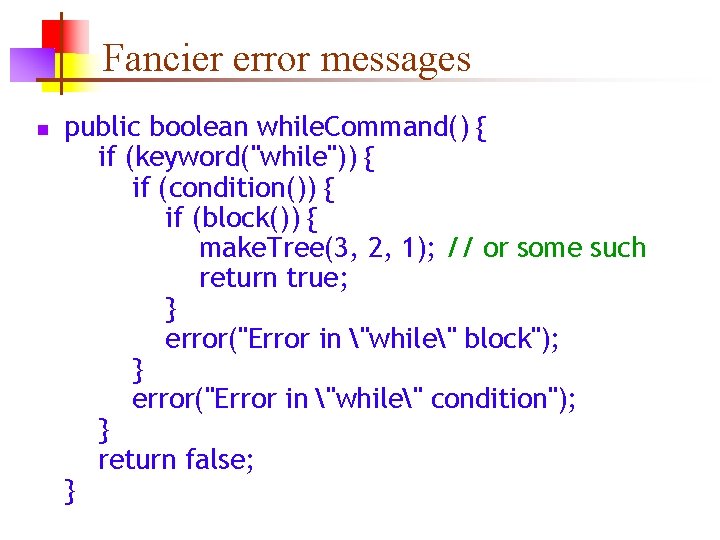
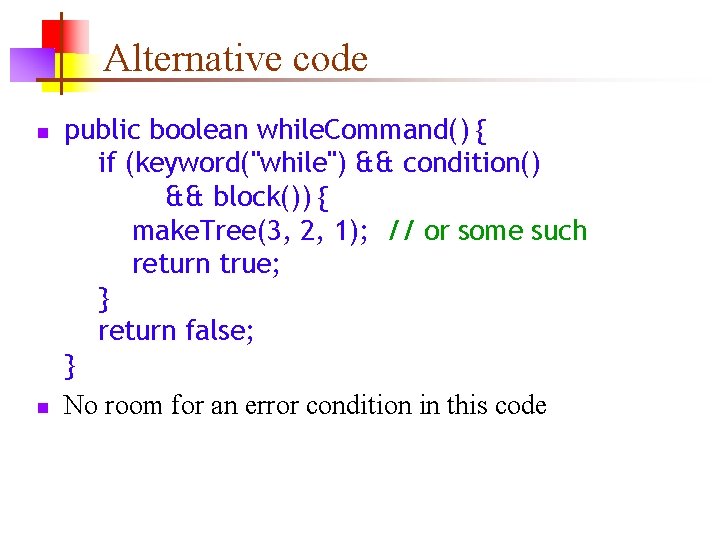
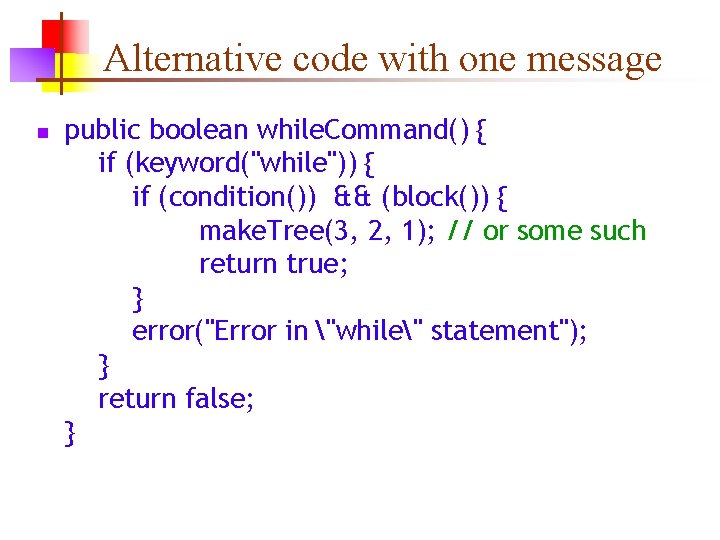
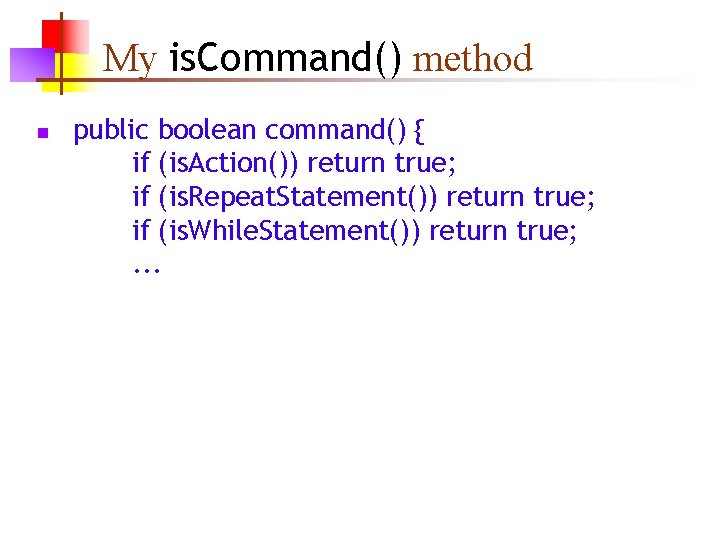
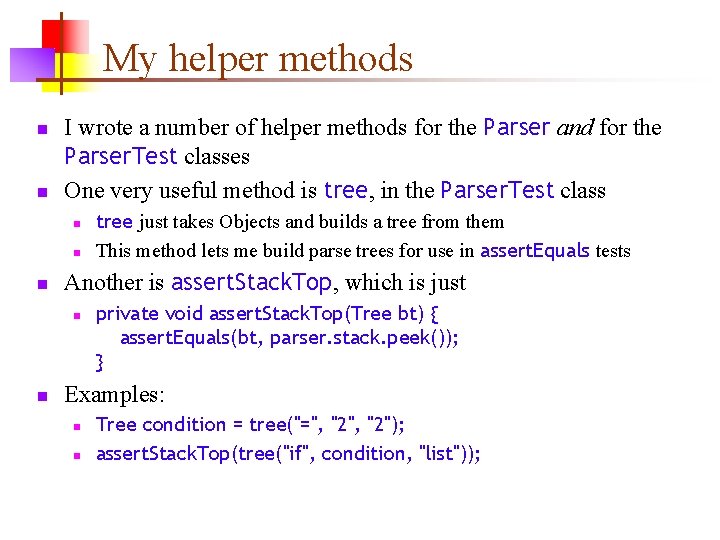
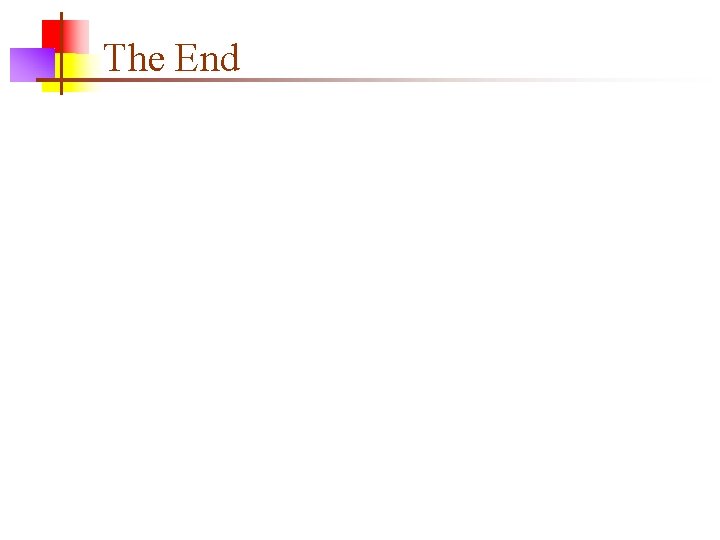
- Slides: 12
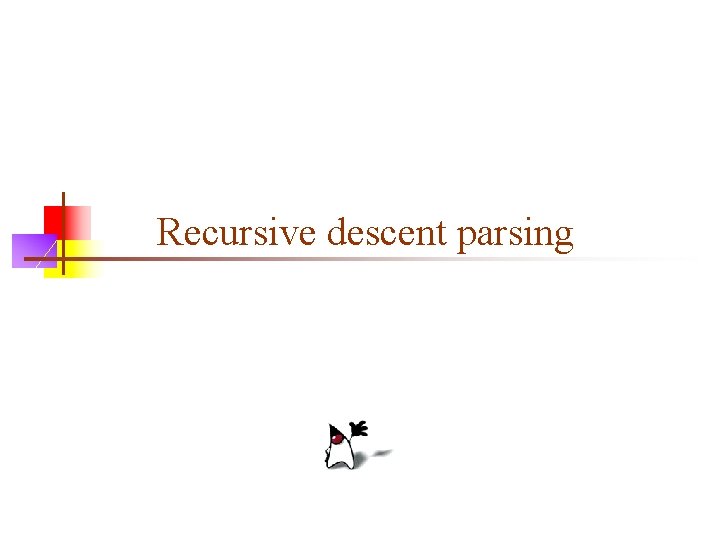
Recursive descent parsing
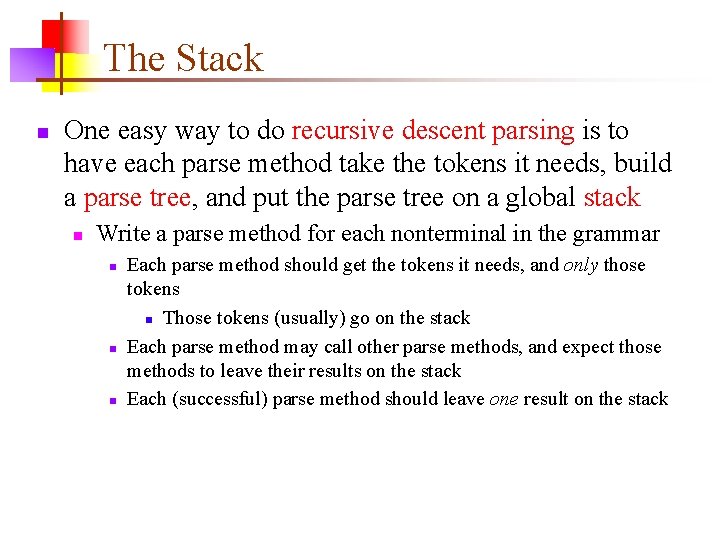
The Stack n One easy way to do recursive descent parsing is to have each parse method take the tokens it needs, build a parse tree, and put the parse tree on a global stack n Write a parse method for each nonterminal in the grammar n n n Each parse method should get the tokens it needs, and only those tokens n Those tokens (usually) go on the stack Each parse method may call other parse methods, and expect those methods to leave their results on the stack Each (successful) parse method should leave one result on the stack
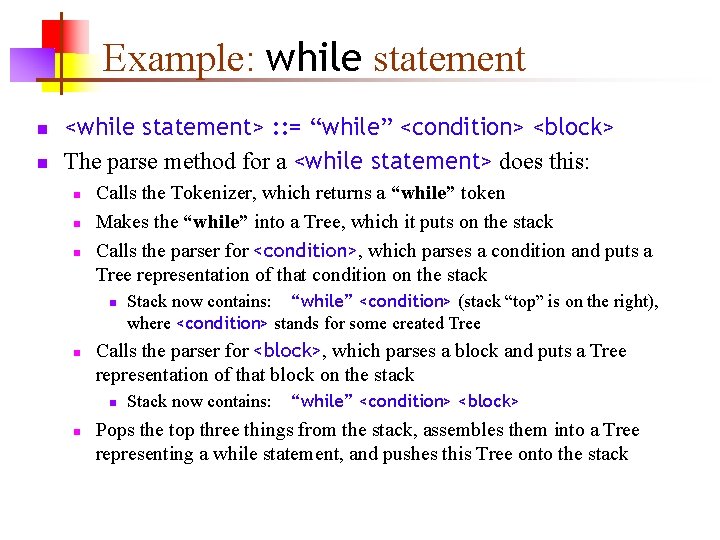
Example: while statement n n <while statement> : : = “while” <condition> <block> The parse method for a <while statement> does this: n n n Calls the Tokenizer, which returns a “while” token Makes the “while” into a Tree, which it puts on the stack Calls the parser for <condition>, which parses a condition and puts a Tree representation of that condition on the stack n n Calls the parser for <block>, which parses a block and puts a Tree representation of that block on the stack n n Stack now contains: “while” <condition> (stack “top” is on the right), where <condition> stands for some created Tree Stack now contains: “while” <condition> <block> Pops the top three things from the stack, assembles them into a Tree representing a while statement, and pushes this Tree onto the stack
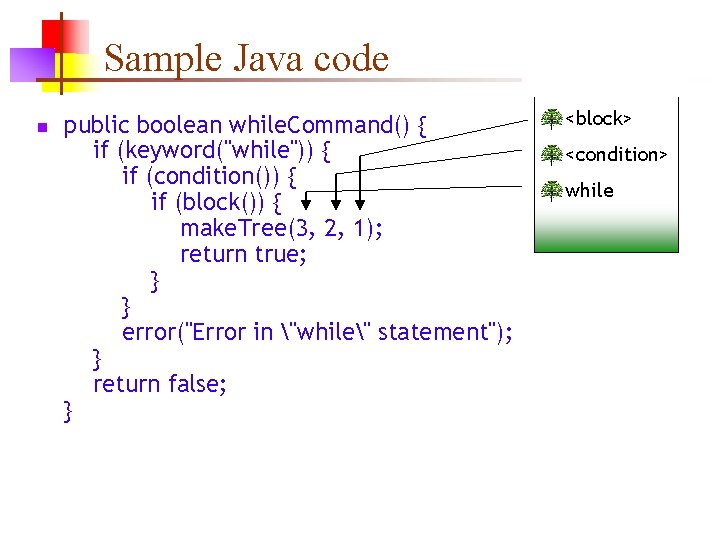
Sample Java code n public boolean while. Command() { if (keyword("while")) { if (condition()) { if (block()) { make. Tree(3, 2, 1); return true; } } error("Error in "while" statement"); } return false; } <block> <condition> while
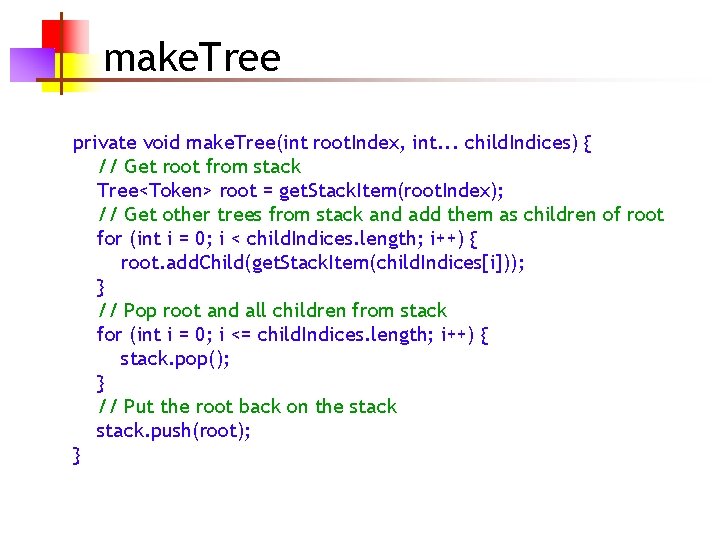
make. Tree private void make. Tree(int root. Index, int. . . child. Indices) { // Get root from stack Tree<Token> root = get. Stack. Item(root. Index); // Get other trees from stack and add them as children of root for (int i = 0; i < child. Indices. length; i++) { root. add. Child(get. Stack. Item(child. Indices[i])); } // Pop root and all children from stack for (int i = 0; i <= child. Indices. length; i++) { stack. pop(); } // Put the root back on the stack. push(root); }
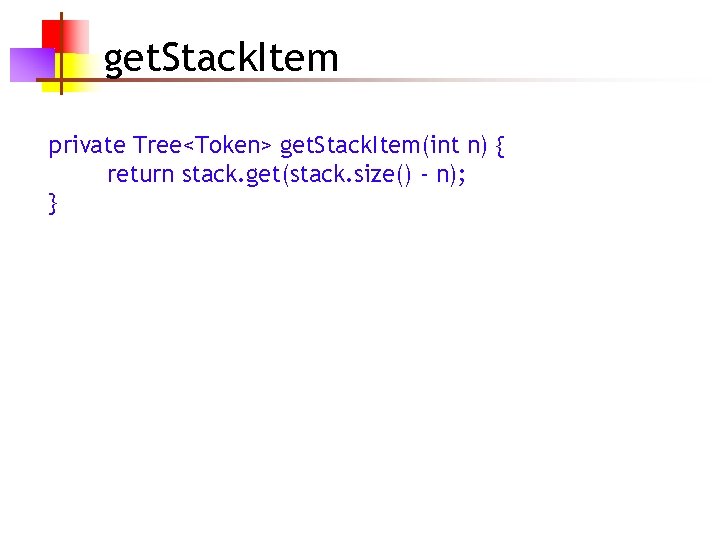
get. Stack. Item private Tree<Token> get. Stack. Item(int n) { return stack. get(stack. size() - n); }
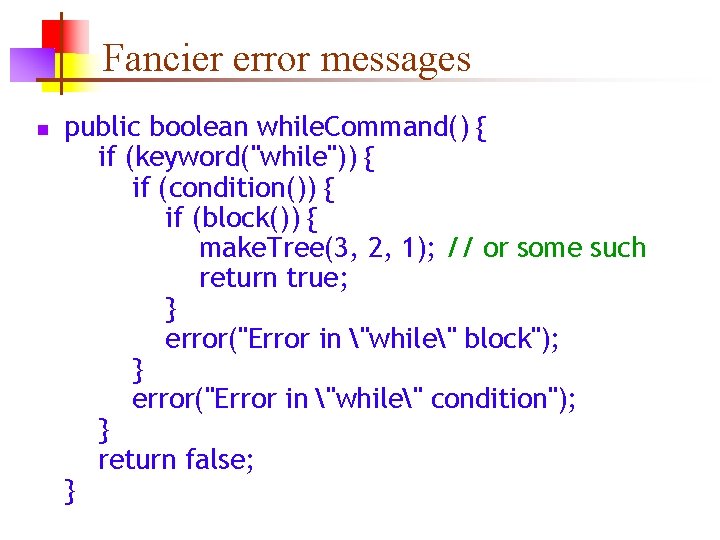
Fancier error messages n public boolean while. Command() { if (keyword("while")) { if (condition()) { if (block()) { make. Tree(3, 2, 1); // or some such return true; } error("Error in "while" block"); } error("Error in "while" condition"); } return false; }
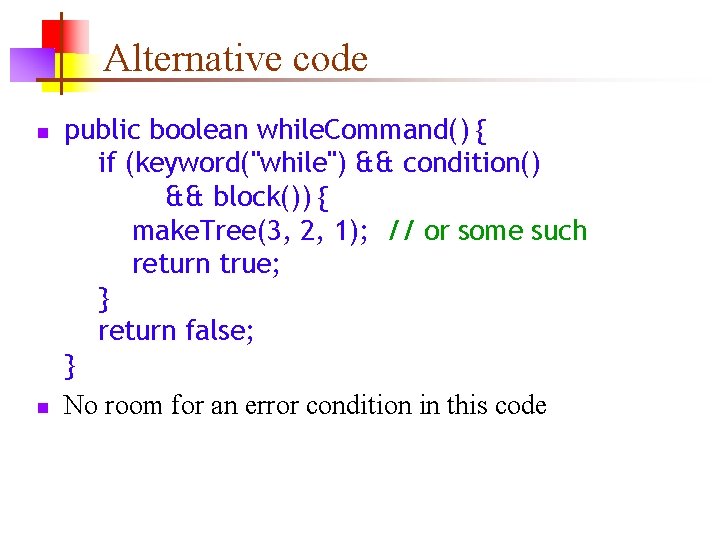
Alternative code n n public boolean while. Command() { if (keyword("while") && condition() && block()) { make. Tree(3, 2, 1); // or some such return true; } return false; } No room for an error condition in this code
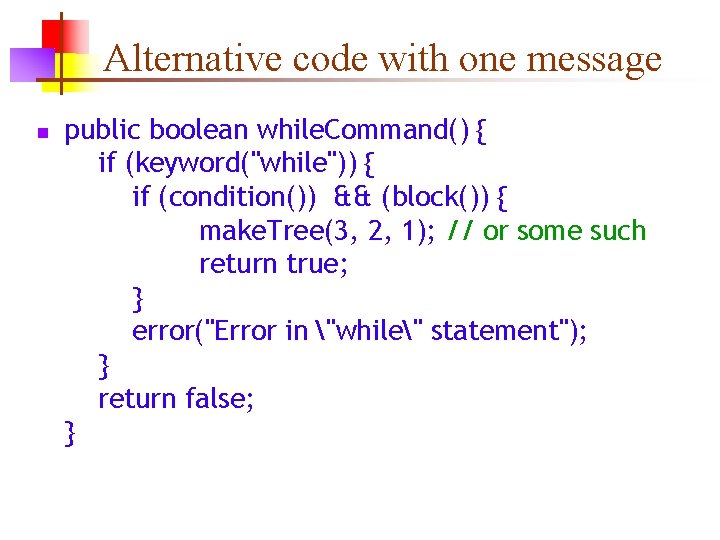
Alternative code with one message n public boolean while. Command() { if (keyword("while")) { if (condition()) && (block()) { make. Tree(3, 2, 1); // or some such return true; } error("Error in "while" statement"); } return false; }
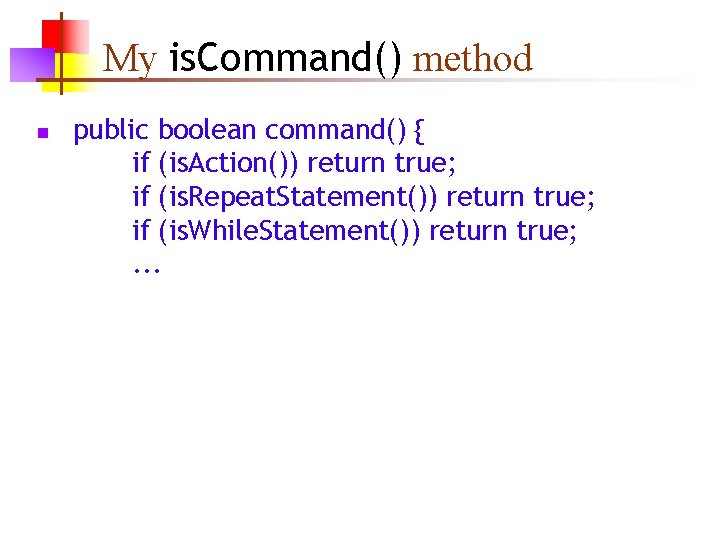
My is. Command() method n public boolean command() { if (is. Action()) return true; if (is. Repeat. Statement()) return true; if (is. While. Statement()) return true; . . .
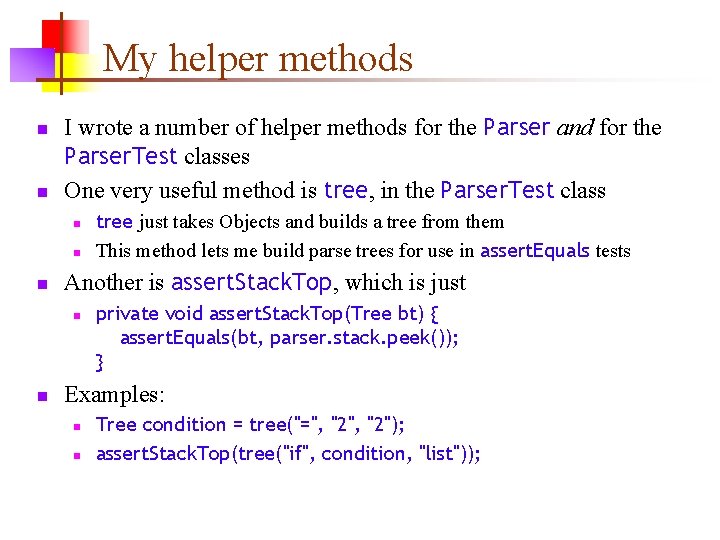
My helper methods n n I wrote a number of helper methods for the Parser and for the Parser. Test classes One very useful method is tree, in the Parser. Test class n n n Another is assert. Stack. Top, which is just n n tree just takes Objects and builds a tree from them This method lets me build parse trees for use in assert. Equals tests private void assert. Stack. Top(Tree bt) { assert. Equals(bt, parser. stack. peek()); } Examples: n n Tree condition = tree("=", "2"); assert. Stack. Top(tree("if", condition, "list"));
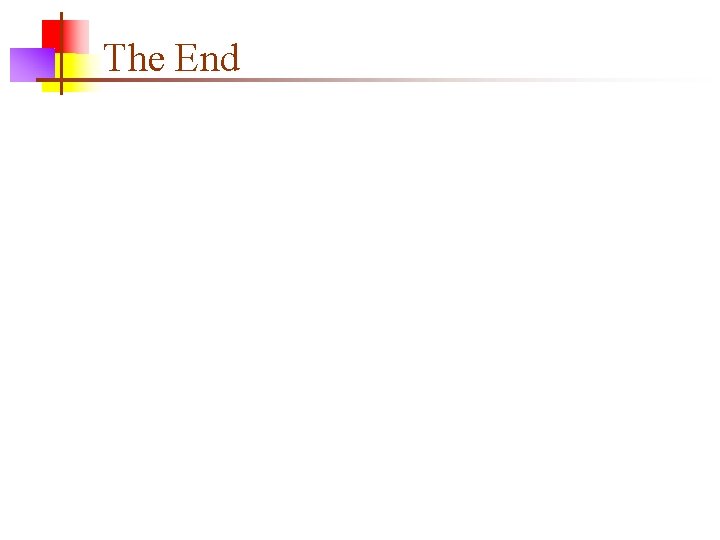
The End
Top-down parser
Recursive descent parsing
How to make a recursive descent parser
Non recursive predictive parsing
Eliminate left recursion calculator
Recursive descent parser
Limitations of recursive descent parser
Nltk recursive descent parser
Recursive descent parser
Recursive descent parser left recursion
Non recursive algorithm examples
Stack smashing vs stack overflow
Stack grows downwards