Recursive descent parsing The Stack n One easy
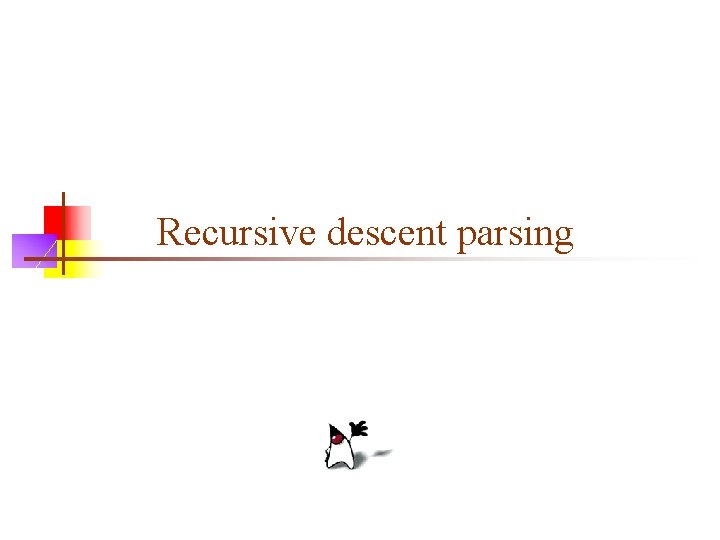
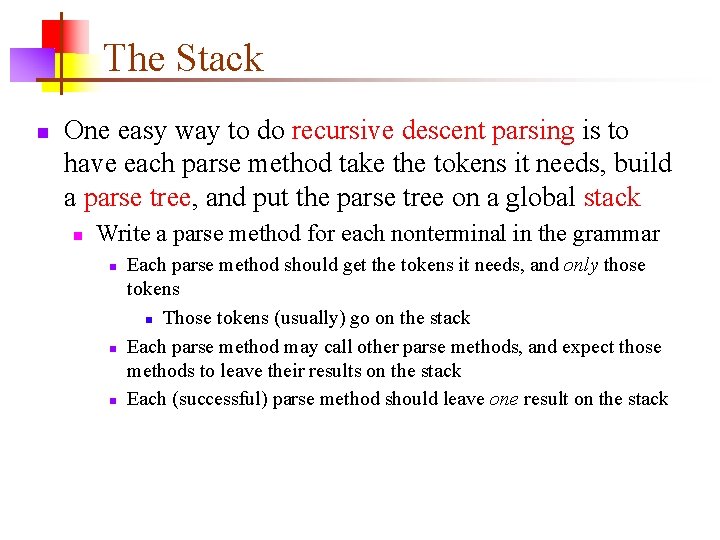
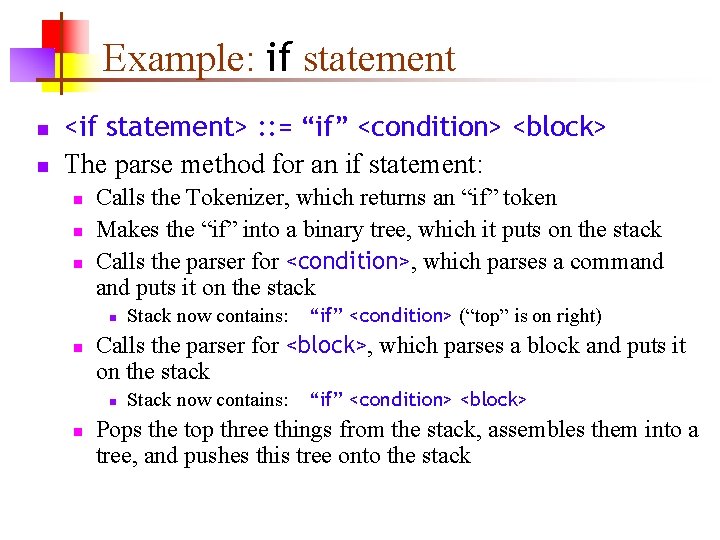
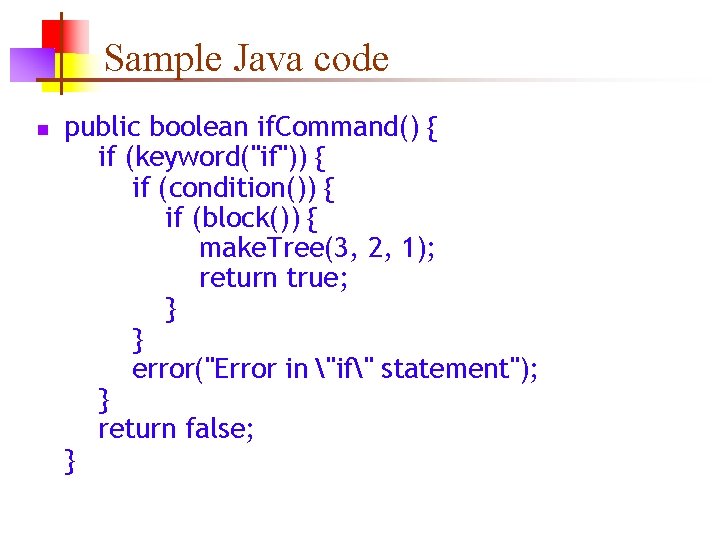
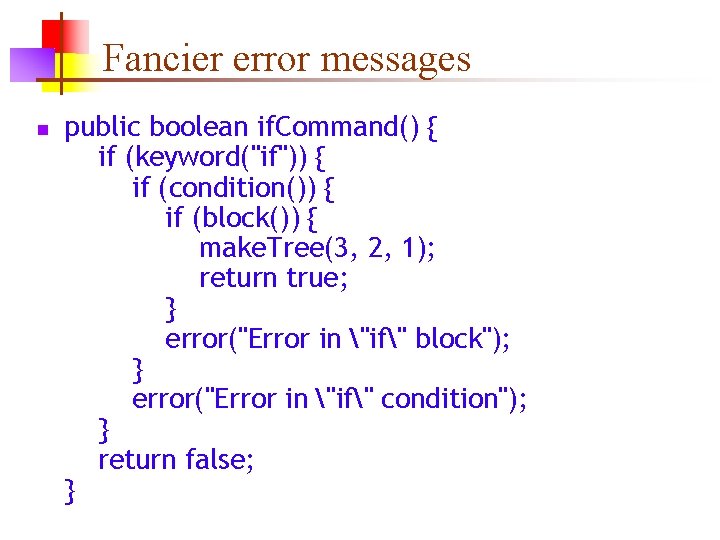
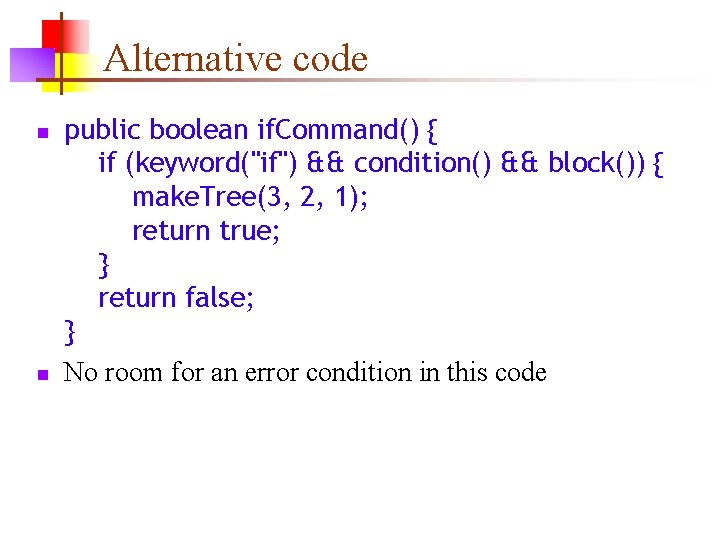
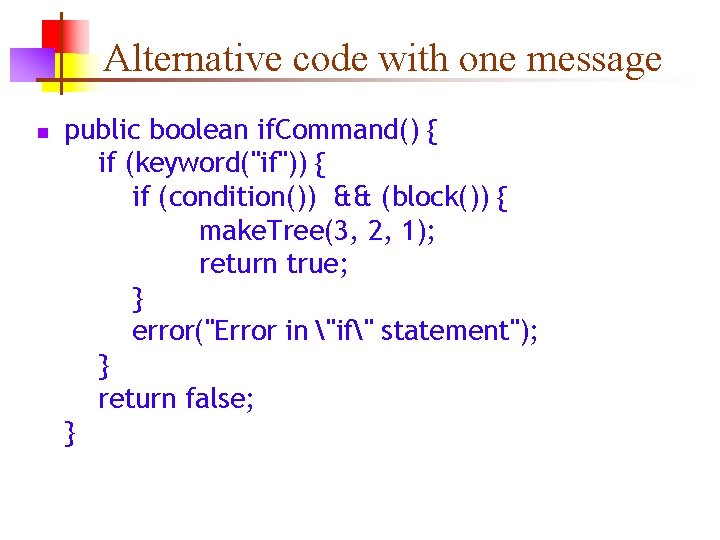
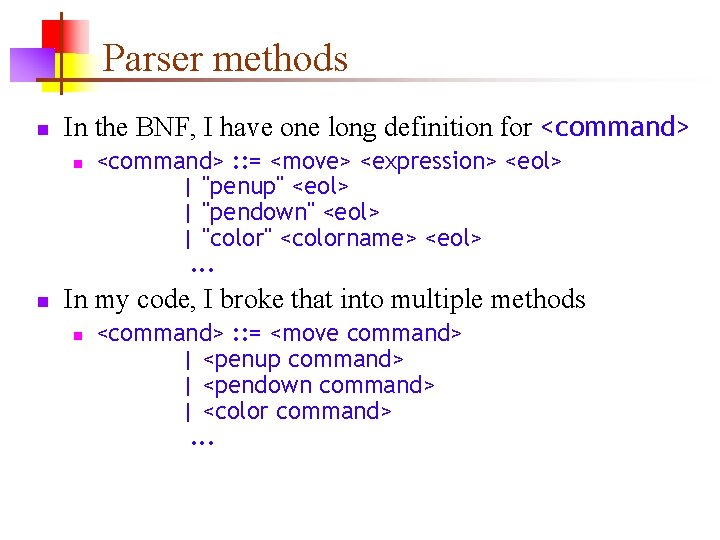
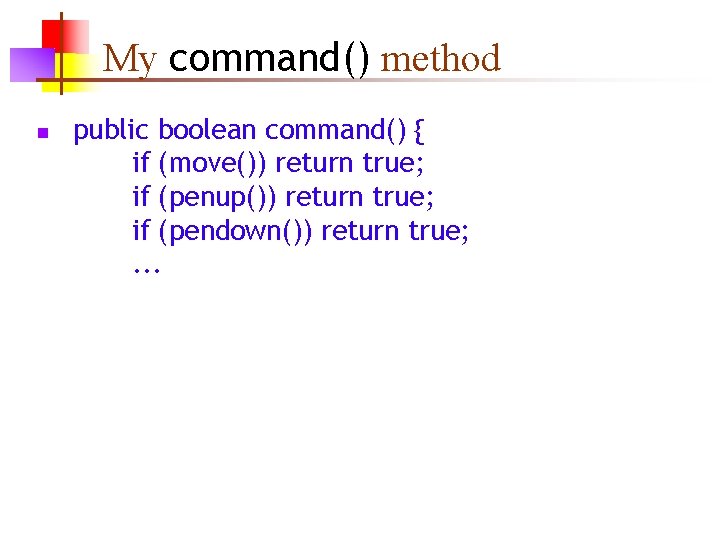
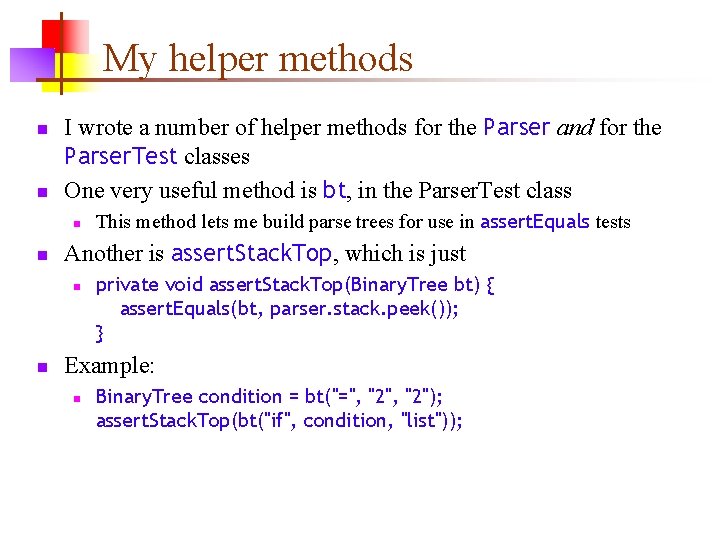
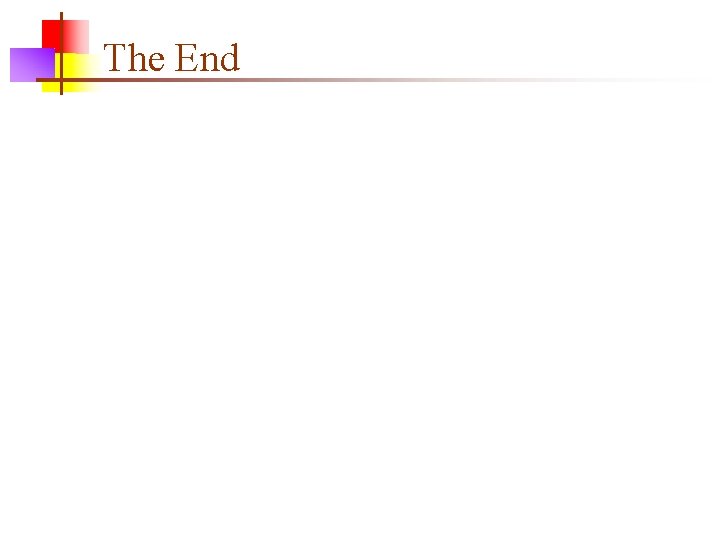
- Slides: 11
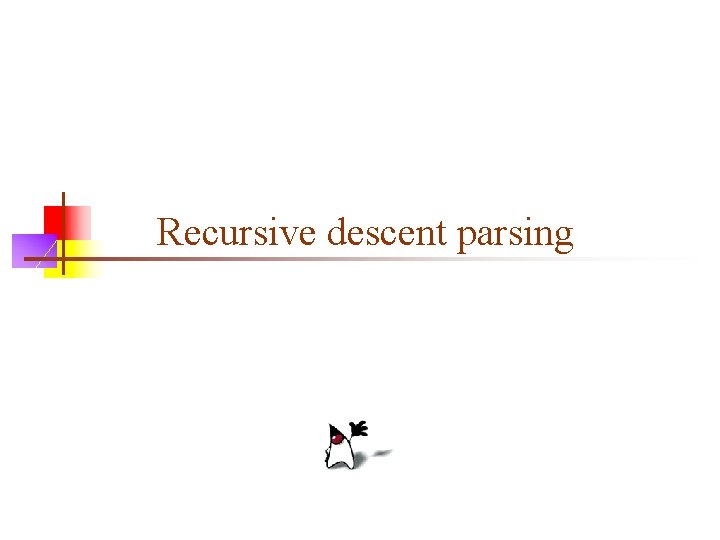
Recursive descent parsing
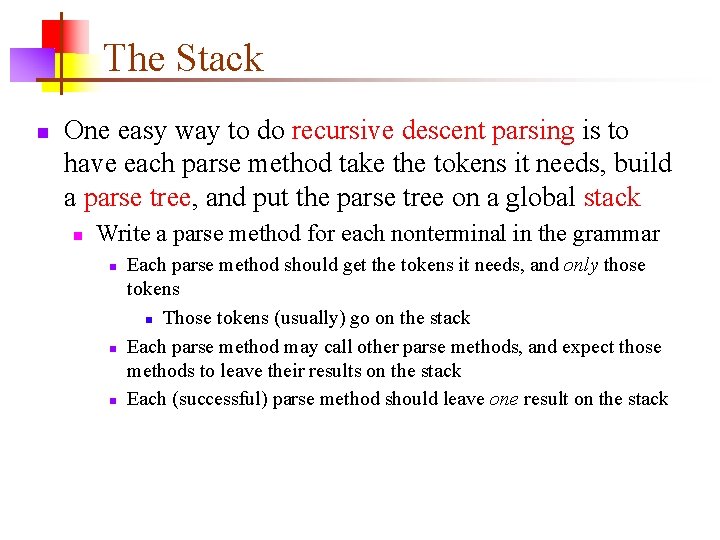
The Stack n One easy way to do recursive descent parsing is to have each parse method take the tokens it needs, build a parse tree, and put the parse tree on a global stack n Write a parse method for each nonterminal in the grammar n n n Each parse method should get the tokens it needs, and only those tokens n Those tokens (usually) go on the stack Each parse method may call other parse methods, and expect those methods to leave their results on the stack Each (successful) parse method should leave one result on the stack
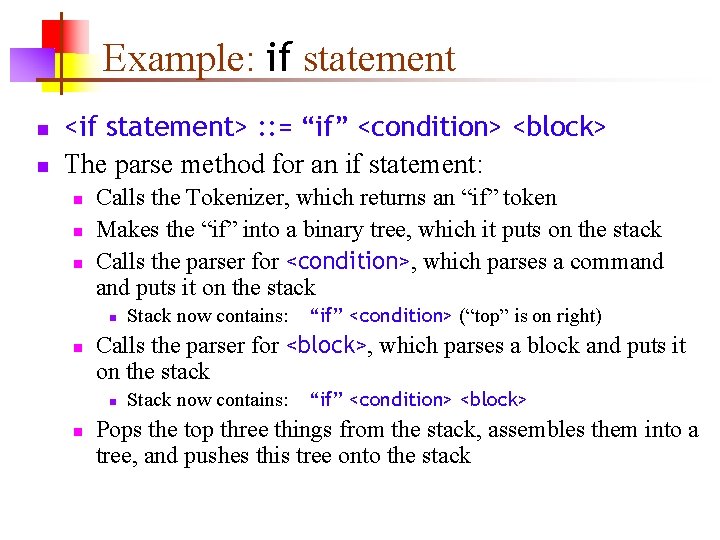
Example: if statement n n <if statement> : : = “if” <condition> <block> The parse method for an if statement: n n n Calls the Tokenizer, which returns an “if” token Makes the “if” into a binary tree, which it puts on the stack Calls the parser for <condition>, which parses a command puts it on the stack n n “if” <condition> (“top” is on right) Calls the parser for <block>, which parses a block and puts it on the stack n n Stack now contains: “if” <condition> <block> Pops the top three things from the stack, assembles them into a tree, and pushes this tree onto the stack
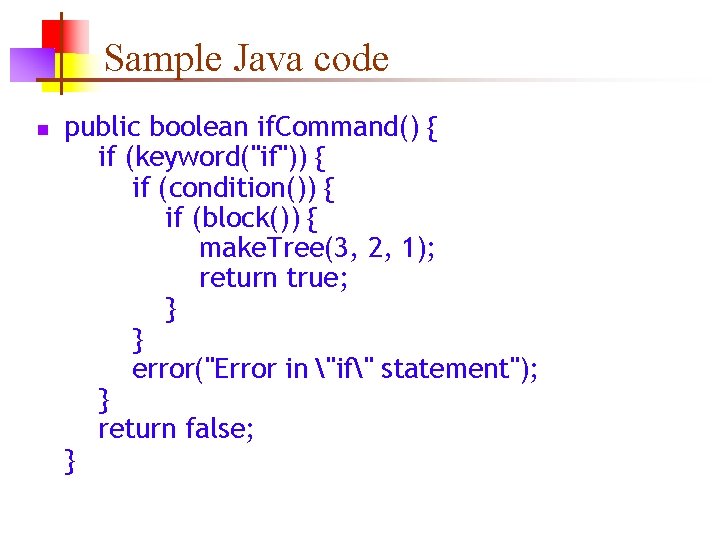
Sample Java code n public boolean if. Command() { if (keyword("if")) { if (condition()) { if (block()) { make. Tree(3, 2, 1); return true; } } error("Error in "if" statement"); } return false; }
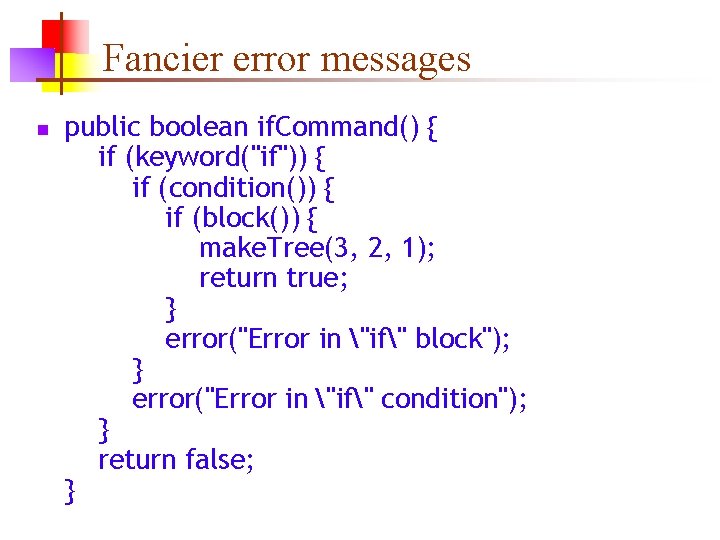
Fancier error messages n public boolean if. Command() { if (keyword("if")) { if (condition()) { if (block()) { make. Tree(3, 2, 1); return true; } error("Error in "if" block"); } error("Error in "if" condition"); } return false; }
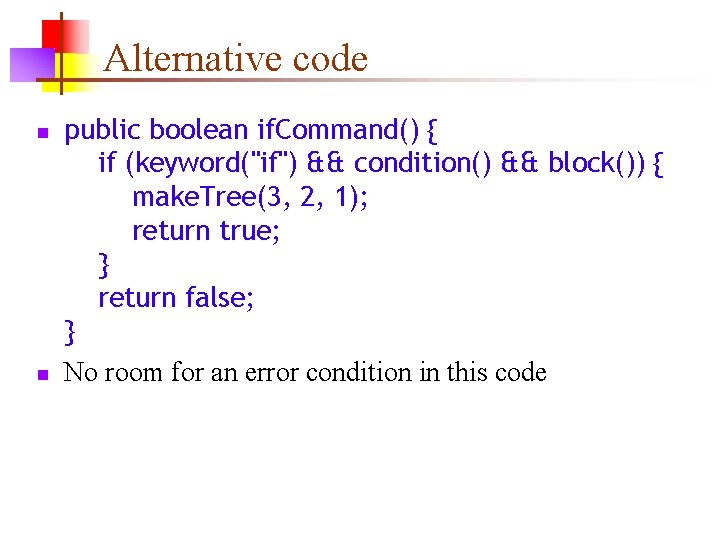
Alternative code n n public boolean if. Command() { if (keyword("if") && condition() && block()) { make. Tree(3, 2, 1); return true; } return false; } No room for an error condition in this code
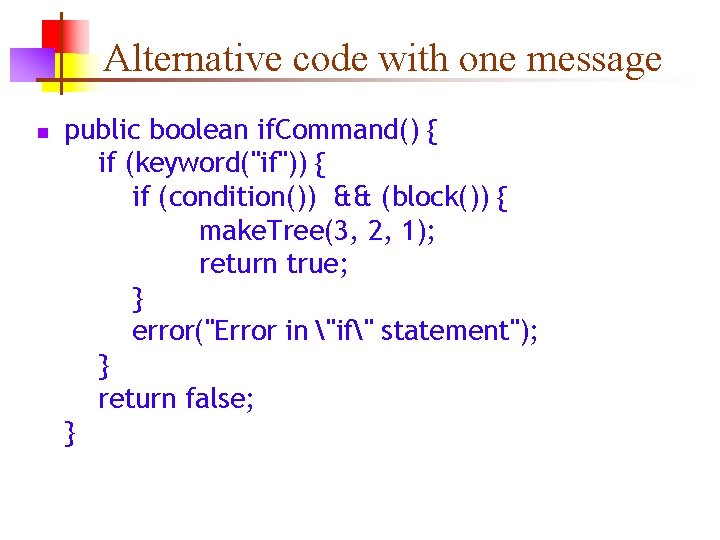
Alternative code with one message n public boolean if. Command() { if (keyword("if")) { if (condition()) && (block()) { make. Tree(3, 2, 1); return true; } error("Error in "if" statement"); } return false; }
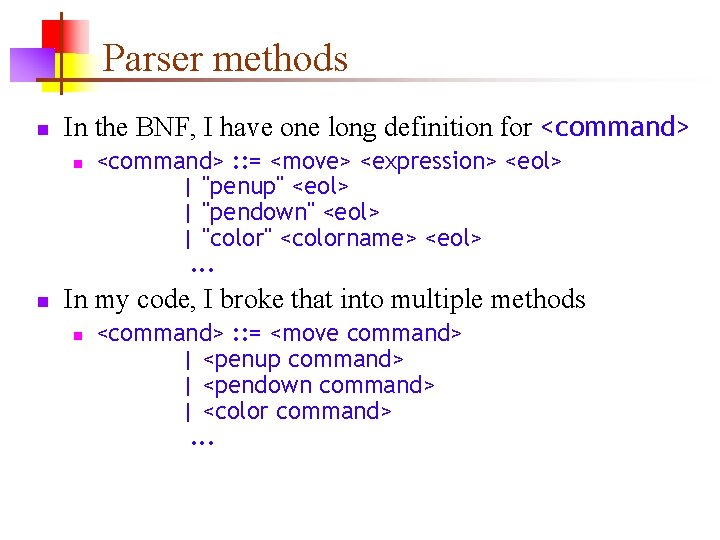
Parser methods n In the BNF, I have one long definition for <command> n n <command> : : = <move> <expression> <eol> | "penup" <eol> | "pendown" <eol> | "color" <colorname> <eol>. . . In my code, I broke that into multiple methods n <command> : : = <move command> | <penup command> | <pendown command> | <color command>. . .
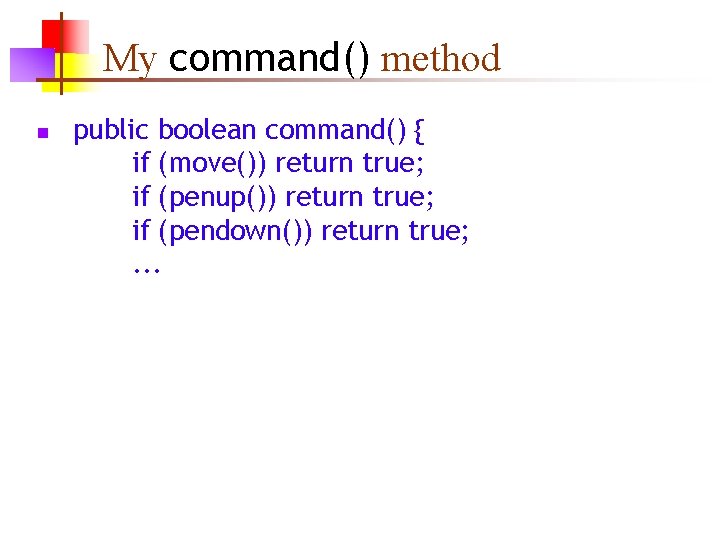
My command() method n public boolean command() { if (move()) return true; if (penup()) return true; if (pendown()) return true; . . .
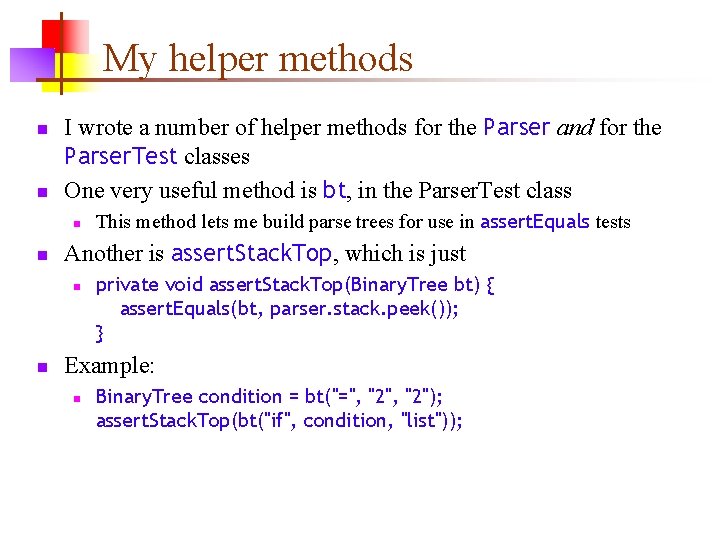
My helper methods n n I wrote a number of helper methods for the Parser and for the Parser. Test classes One very useful method is bt, in the Parser. Test class n n Another is assert. Stack. Top, which is just n n This method lets me build parse trees for use in assert. Equals tests private void assert. Stack. Top(Binary. Tree bt) { assert. Equals(bt, parser. stack. peek()); } Example: n Binary. Tree condition = bt("=", "2"); assert. Stack. Top(bt("if", condition, "list"));
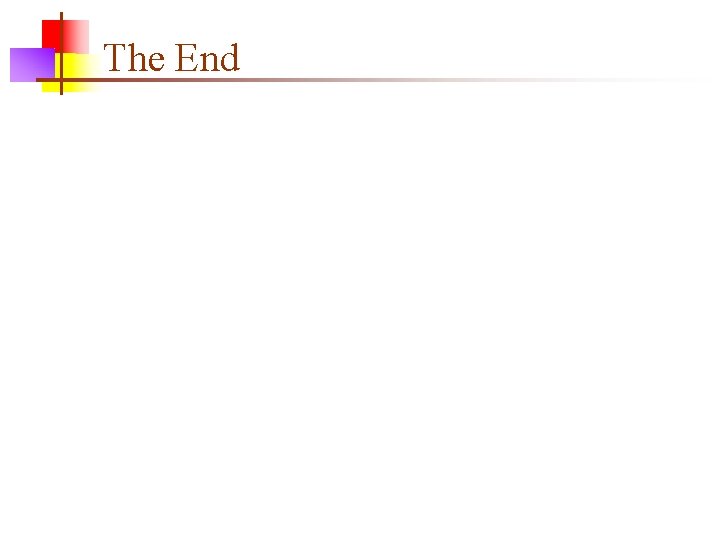
The End
Top down parsing
Recursive descent parsing
Predictive parsing
Non recursive predictive parsing
Recursive descent parser calculator java
Recursive descent parser
Limitations of recursive descent parser
Nltk recursive descent parser
Recursive descent parser
Left factoring algorithm
Summarize the general plan for non-recursive algorithms.
Stack smash attack