Python Spring 2020 Tufts University Instructor Joel Grodstein
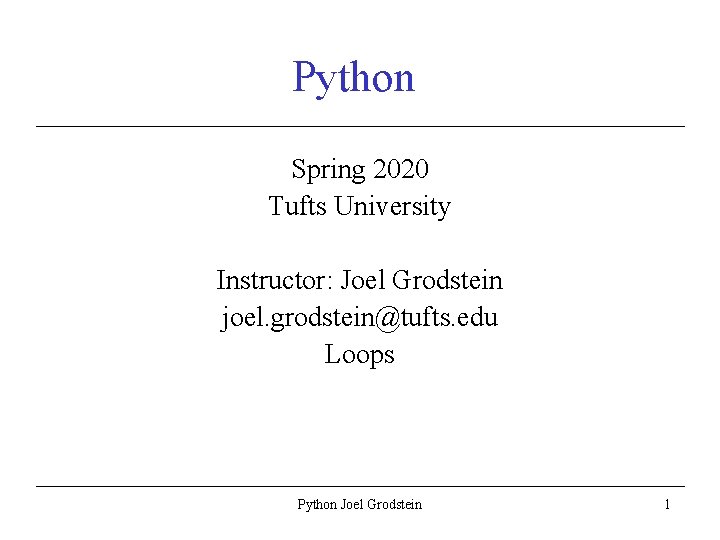
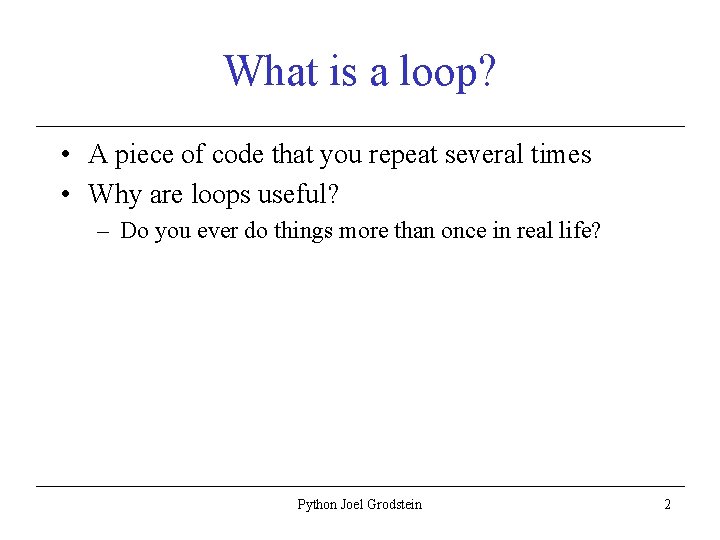
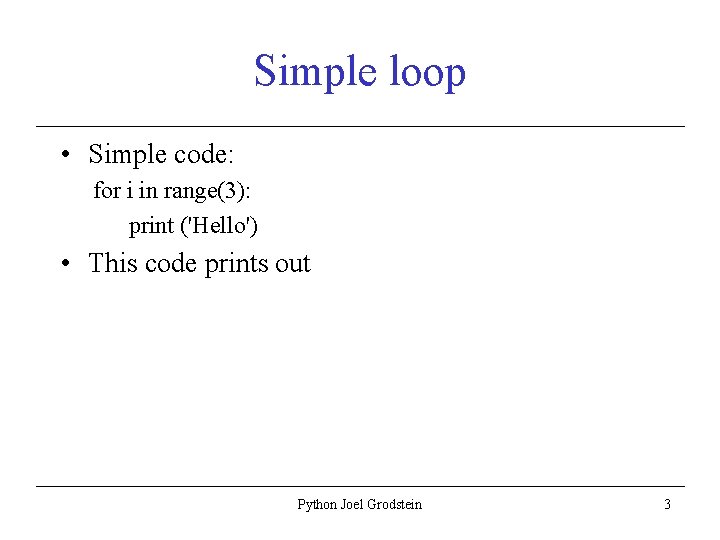
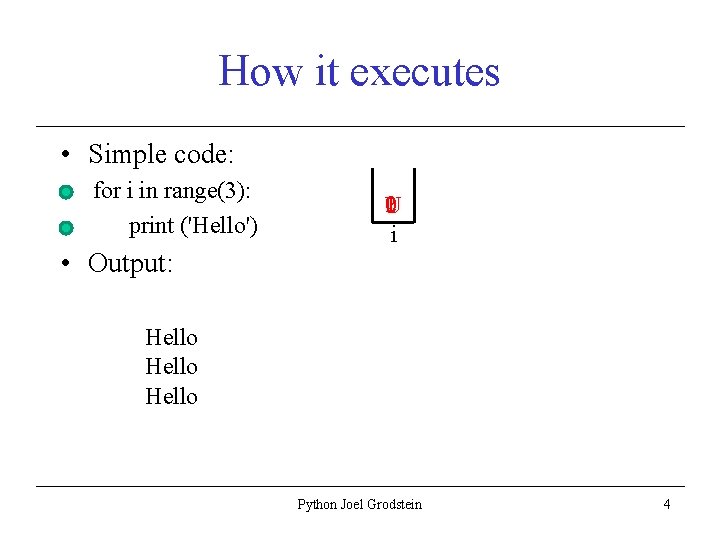
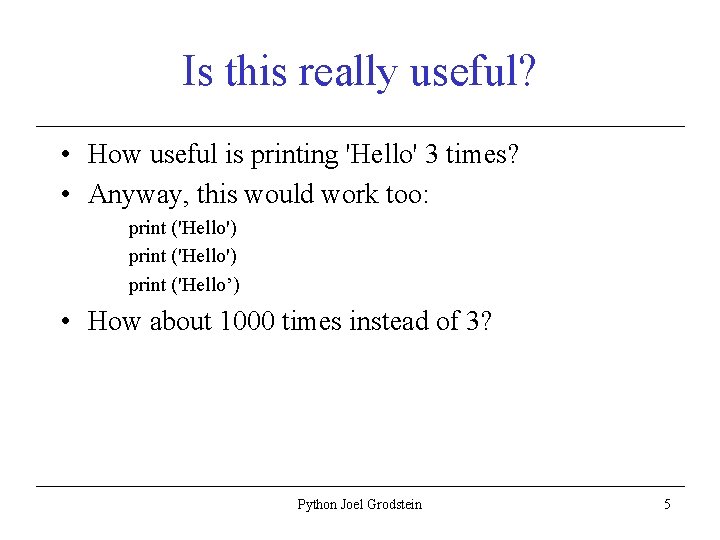
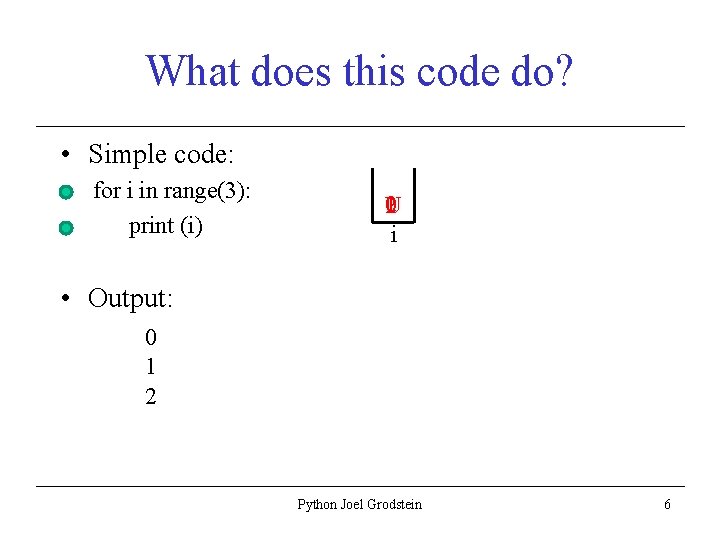
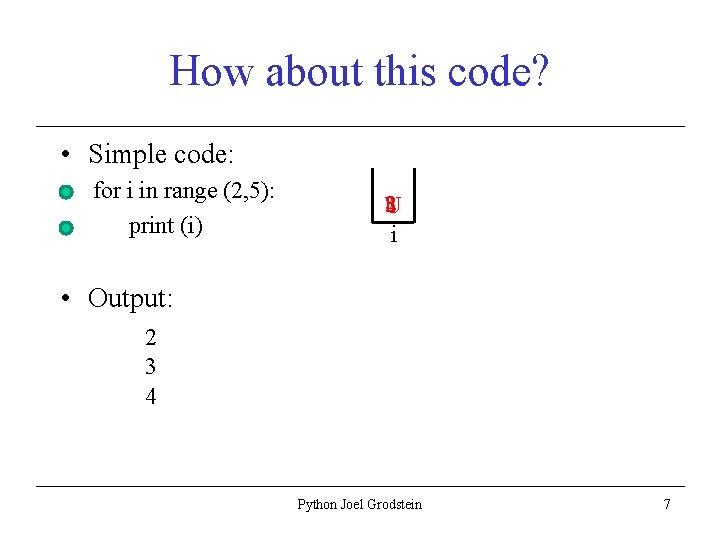
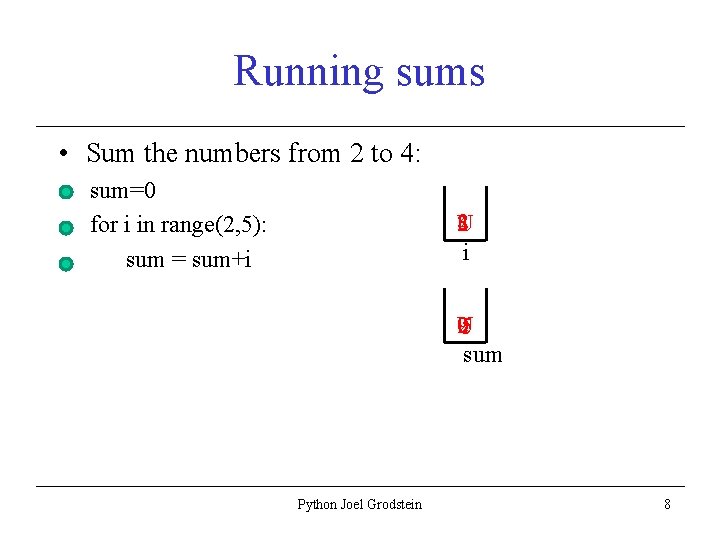
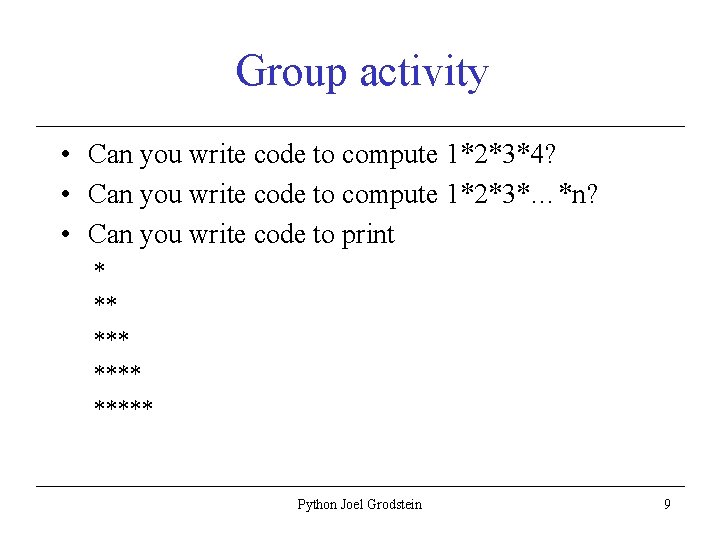
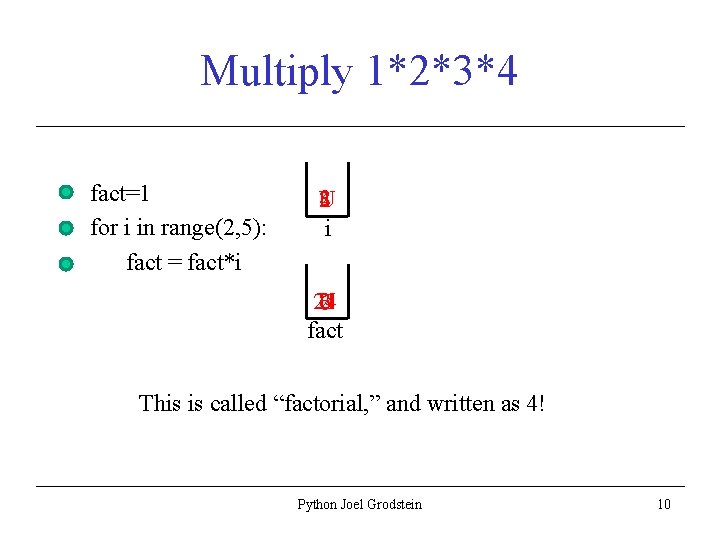
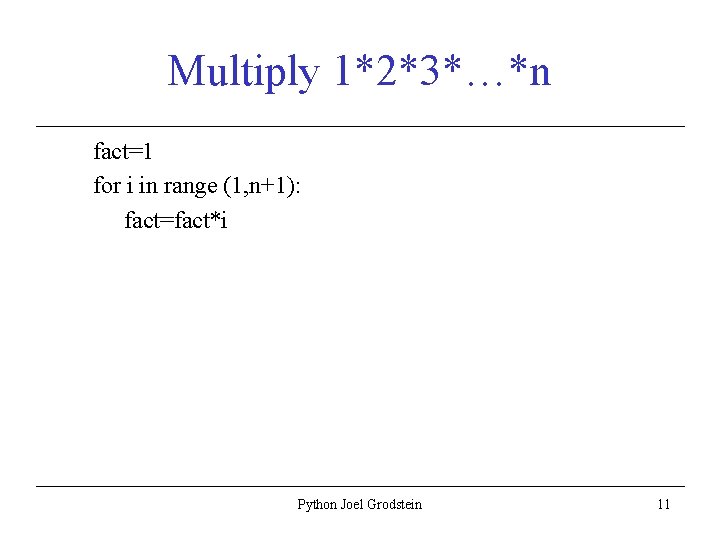
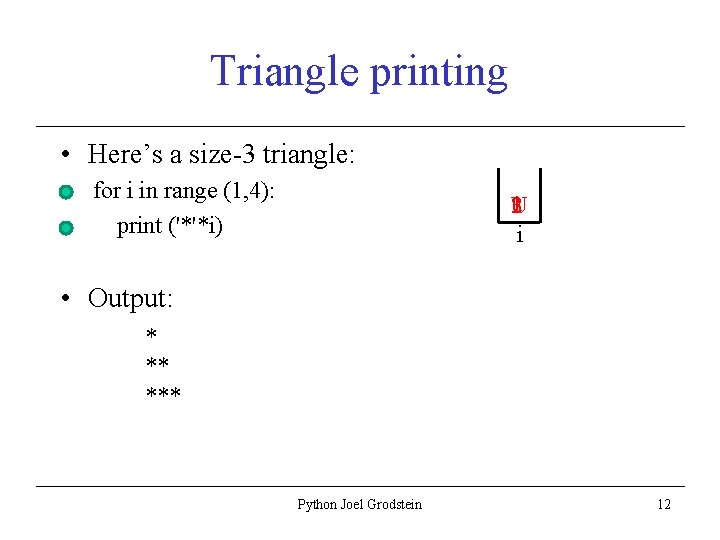
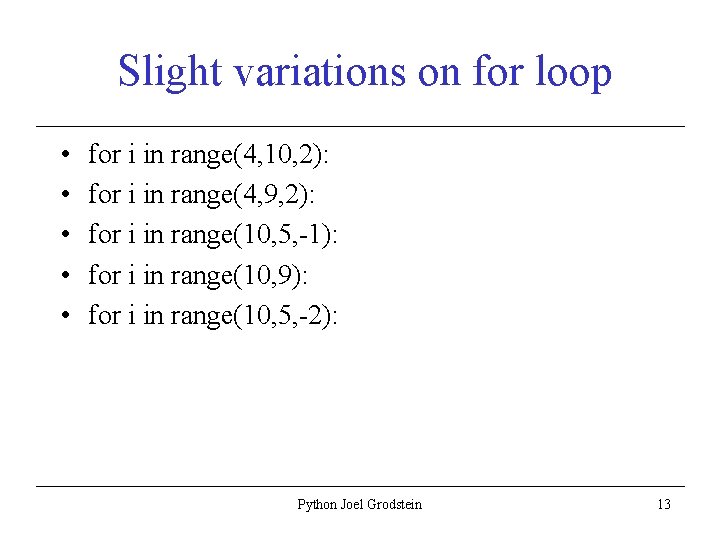
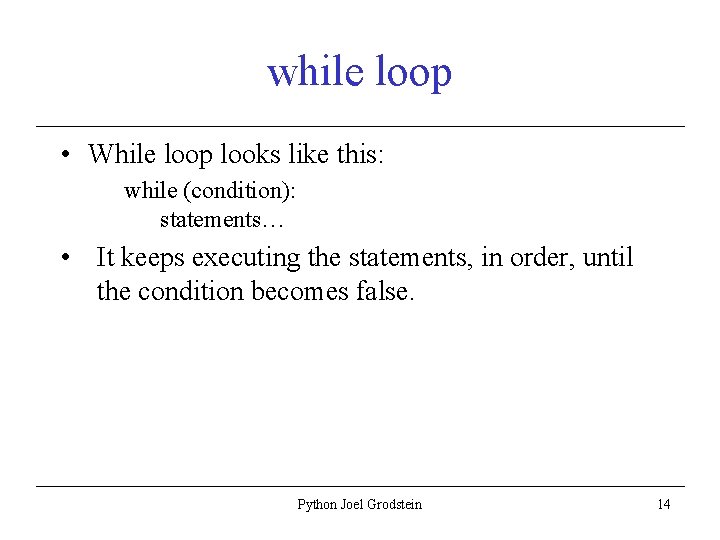
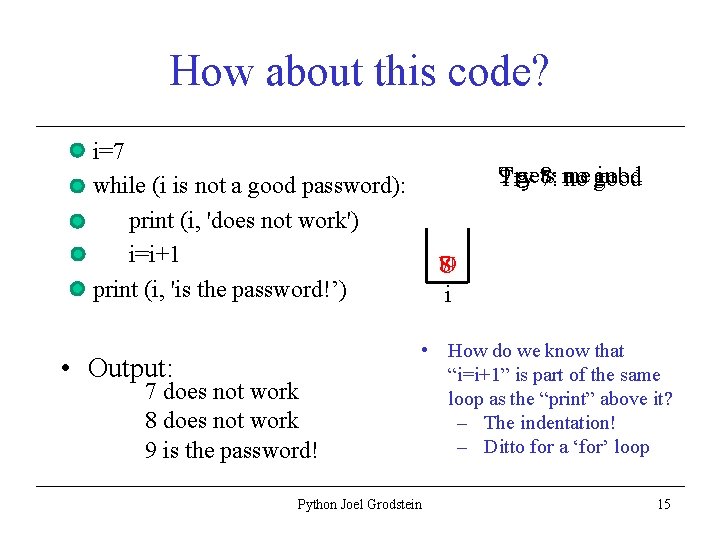
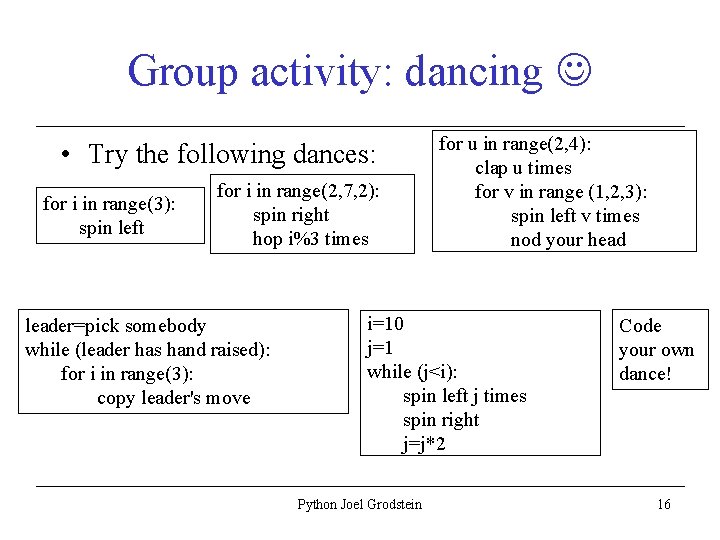
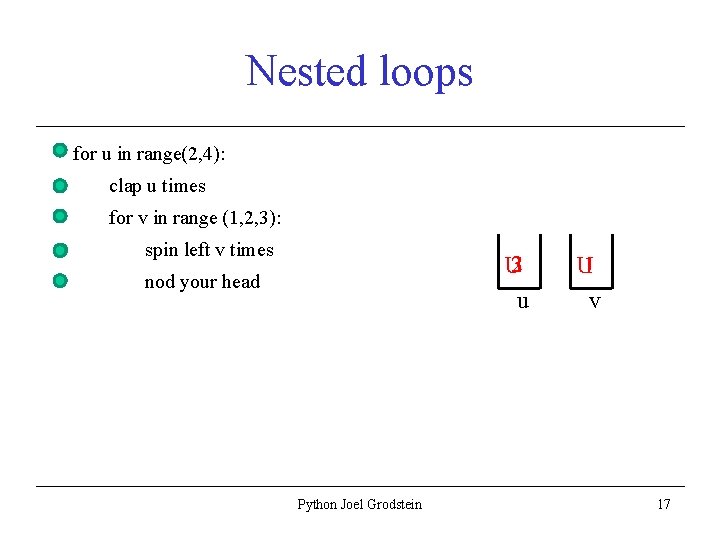
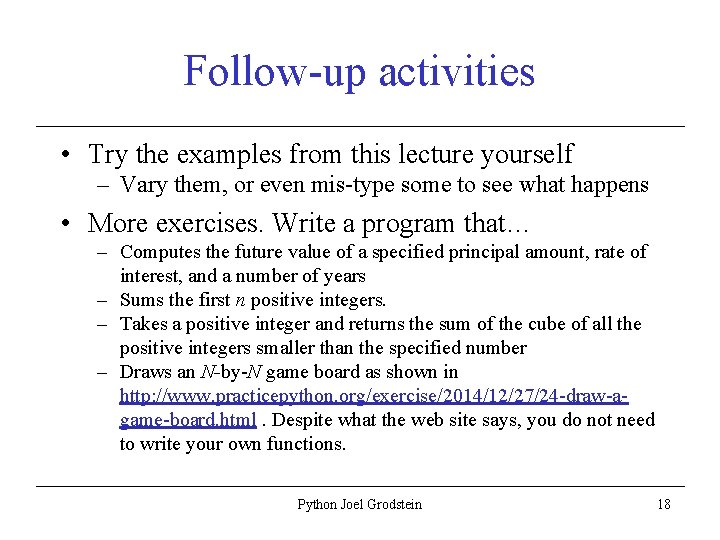
- Slides: 18
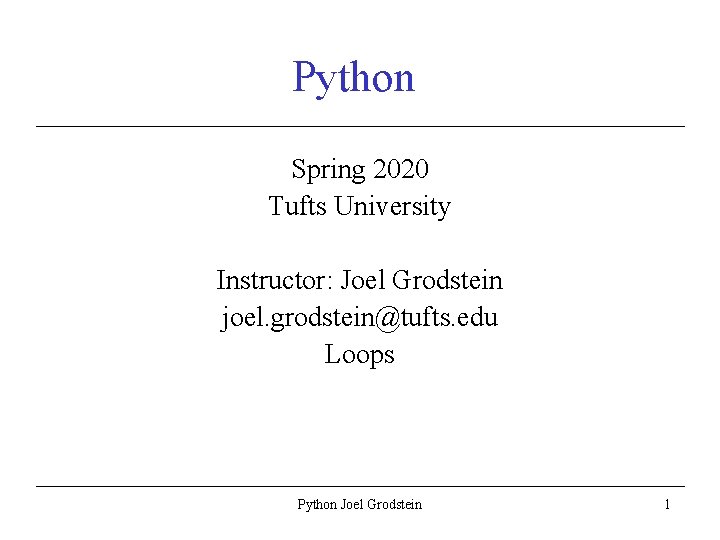
Python Spring 2020 Tufts University Instructor: Joel Grodstein joel. grodstein@tufts. edu Loops Python Joel Grodstein 1
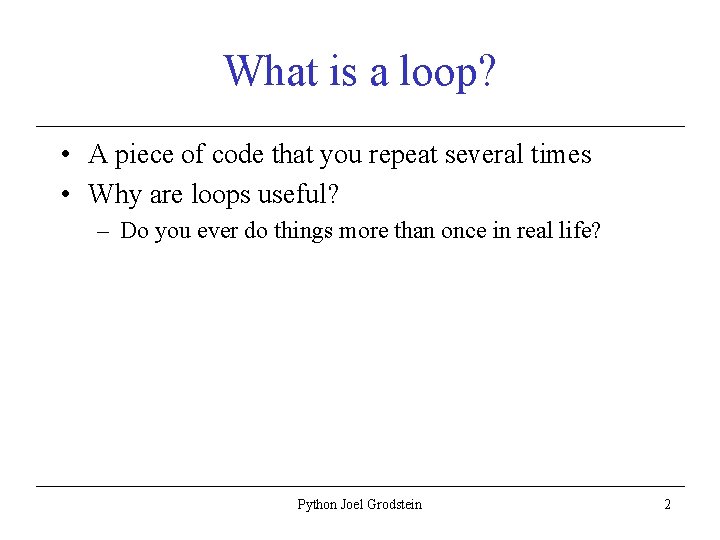
What is a loop? • A piece of code that you repeat several times • Why are loops useful? – Do you ever do things more than once in real life? Python Joel Grodstein 2
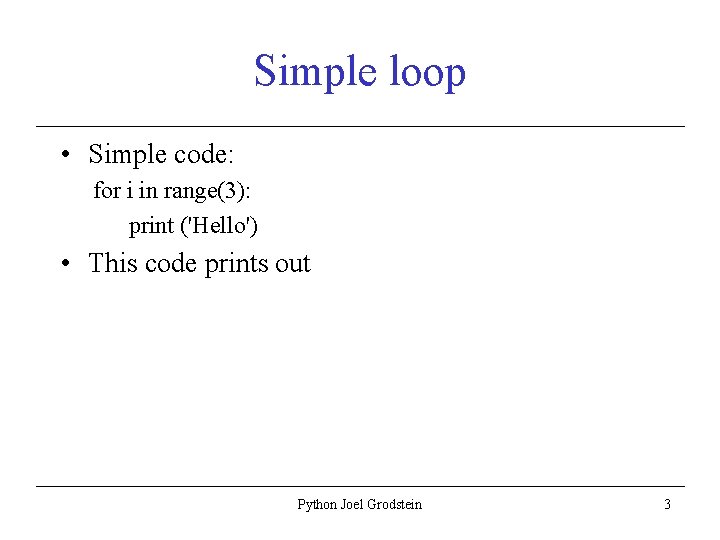
Simple loop • Simple code: for i in range(3): print ('Hello') • This code prints out Python Joel Grodstein 3
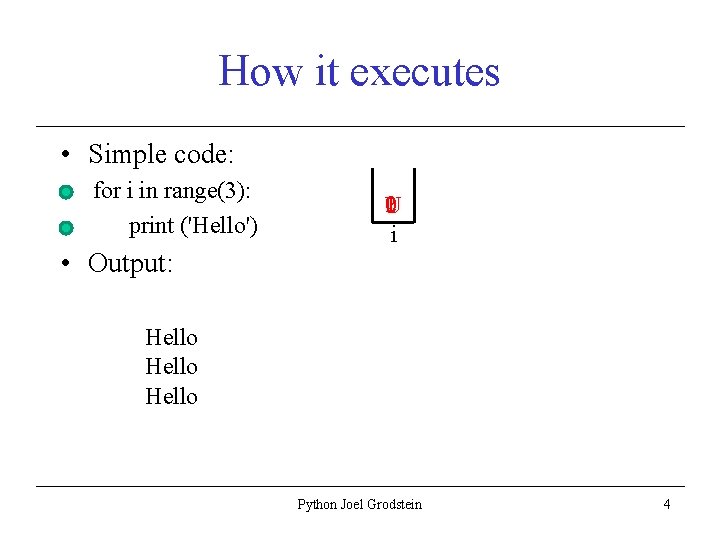
How it executes • Simple code: for i in range(3): print ('Hello') • Output: 201 U i Hello Python Joel Grodstein 4
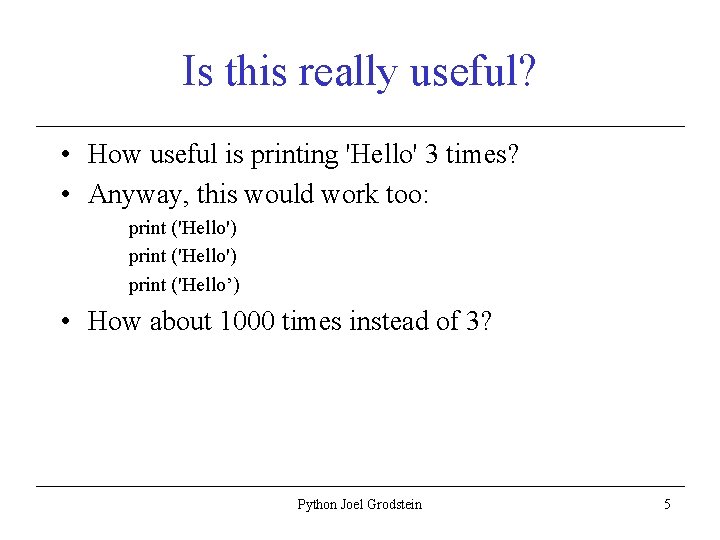
Is this really useful? • How useful is printing 'Hello' 3 times? • Anyway, this would work too: print ('Hello') print ('Hello’) • How about 1000 times instead of 3? Python Joel Grodstein 5
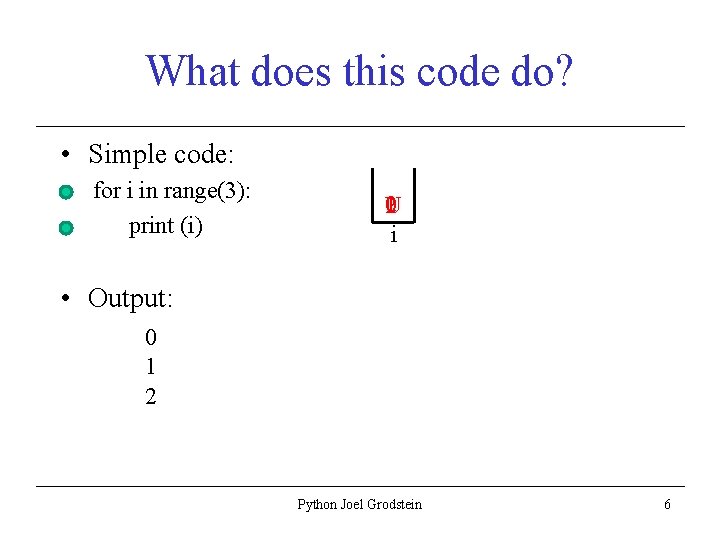
What does this code do? • Simple code: for i in range(3): print (i) 01 U 2 i • Output: 0 1 2 Python Joel Grodstein 6
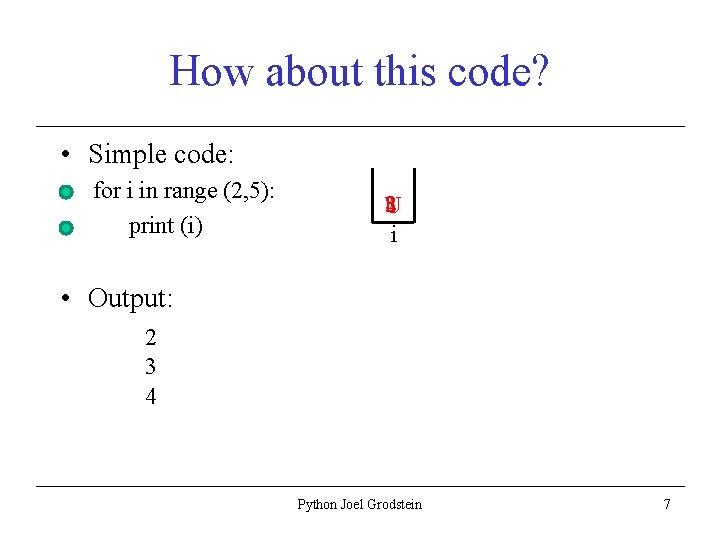
How about this code? • Simple code: for i in range (2, 5): print (i) 32 U 4 i • Output: 2 3 4 Python Joel Grodstein 7
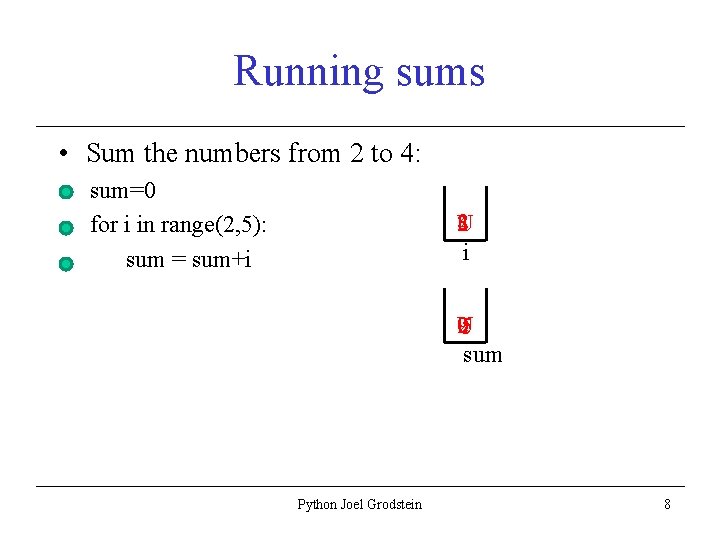
Running sums • Sum the numbers from 2 to 4: sum=0 for i in range(2, 5): sum = sum+i 23 U 4 i 0295 U sum Python Joel Grodstein 8
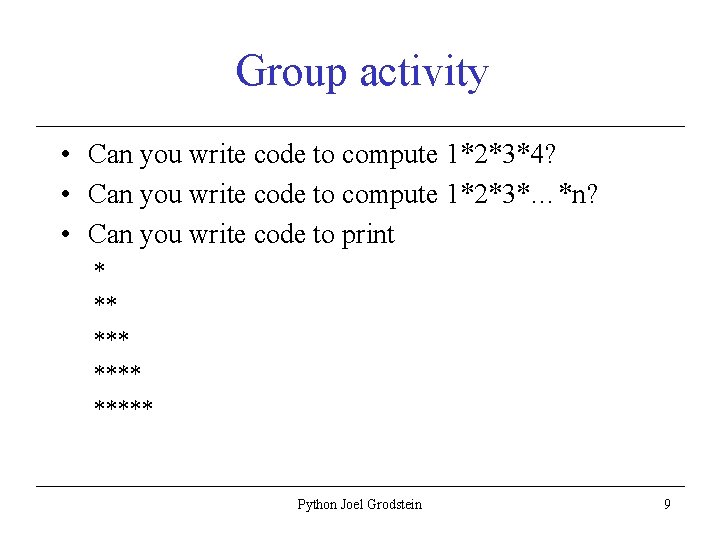
Group activity • Can you write code to compute 1*2*3*4? • Can you write code to compute 1*2*3*…*n? • Can you write code to print * ** ***** Python Joel Grodstein 9
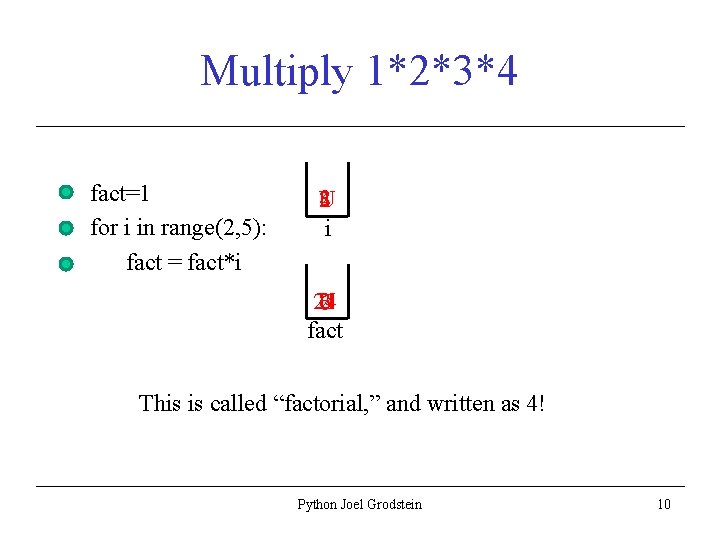
Multiply 1*2*3*4 fact=1 for i in range(2, 5): fact = fact*i 23 U 4 i 24 2 U 61 fact This is called “factorial, ” and written as 4! Python Joel Grodstein 10
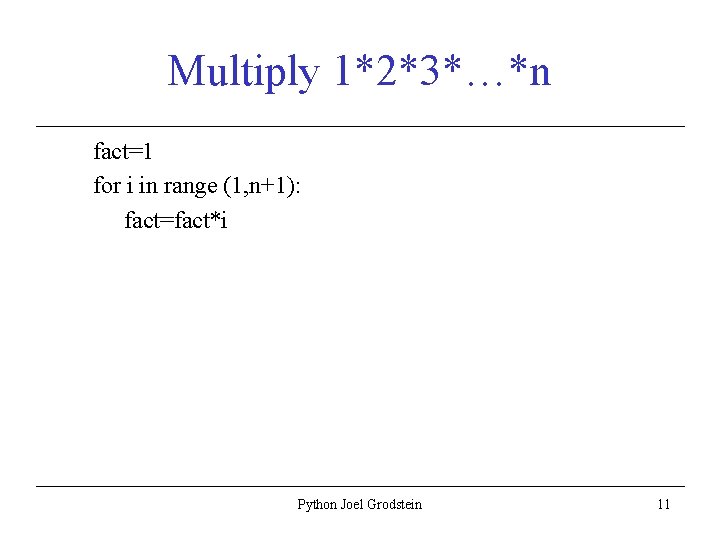
Multiply 1*2*3*…*n fact=1 for i in range (1, n+1): fact=fact*i Python Joel Grodstein 11
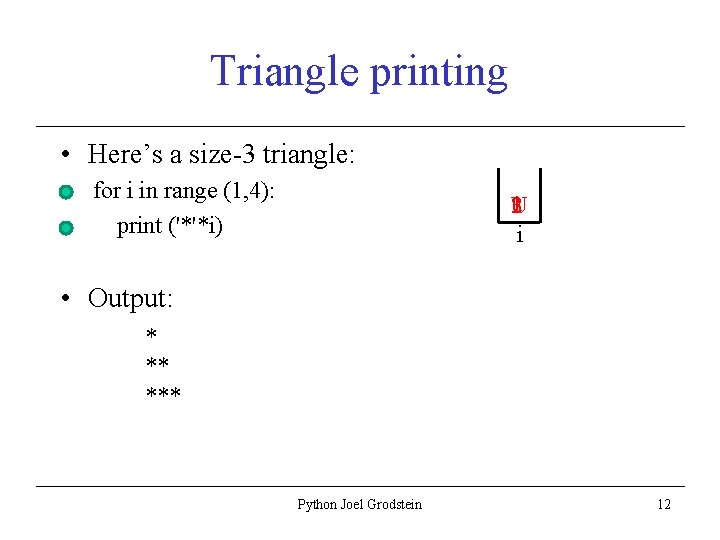
Triangle printing • Here’s a size-3 triangle: for i in range (1, 4): print ('*'*i) 12 U 3 i • Output: * ** *** Python Joel Grodstein 12
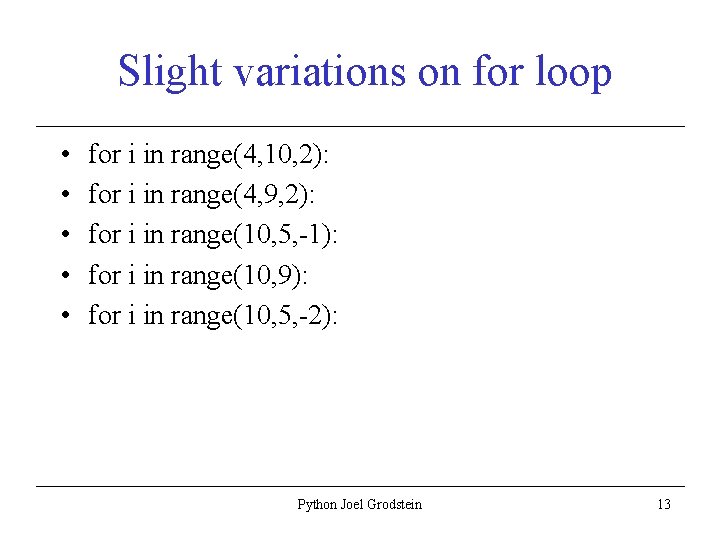
Slight variations on for loop • • • for i in range(4, 10, 2): for i in range(4, 9, 2): for i in range(10, 5, -1): for i in range(10, 9): for i in range(10, 5, -2): Python Joel Grodstein 13
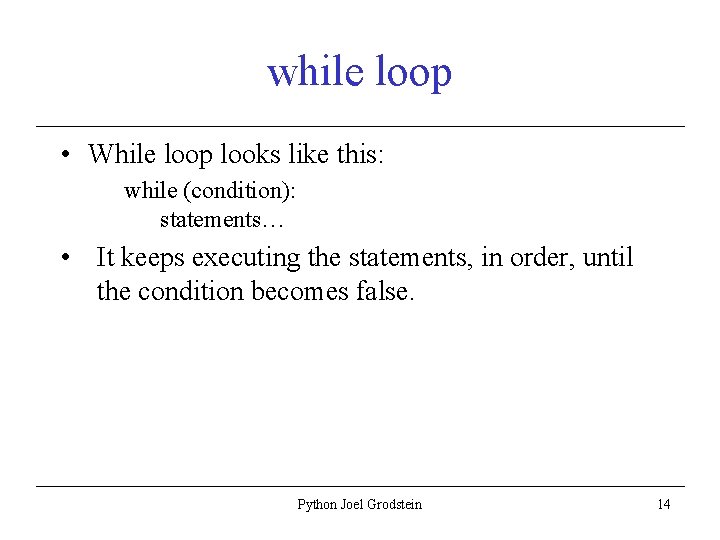
while loop • While loop looks like this: while (condition): statements… • It keeps executing the statements, in order, until the condition becomes false. Python Joel Grodstein 14
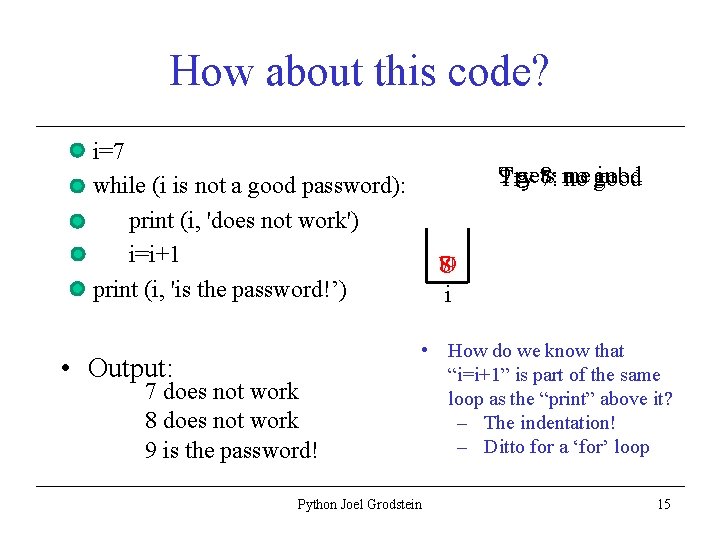
How about this code? i=7 while (i is not a good password): print (i, 'does not work') i=i+1 print (i, 'is the password!’) • Output: 7 does not work 8 does not work 9 is the password! 9 gets Try 8: no good in! 7: me 87 U 9 i • How do we know that “i=i+1” is part of the same loop as the “print” above it? – The indentation! – Ditto for a ‘for’ loop Python Joel Grodstein 15
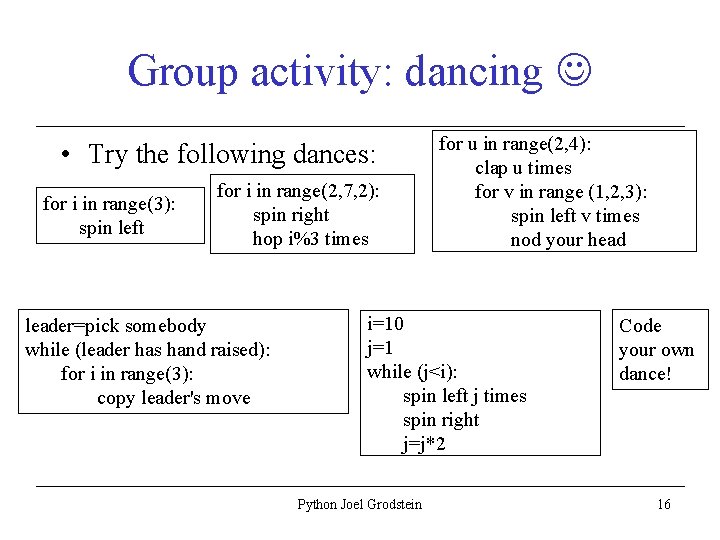
Group activity: dancing • Try the following dances: for i in range(3): spin left for i in range(2, 7, 2): spin right hop i%3 times leader=pick somebody while (leader has hand raised): for i in range(3): copy leader's move for u in range(2, 4): clap u times for v in range (1, 2, 3): spin left v times nod your head i=10 j=1 while (j<i): spin left j times spin right j=j*2 Python Joel Grodstein Code your own dance! 16
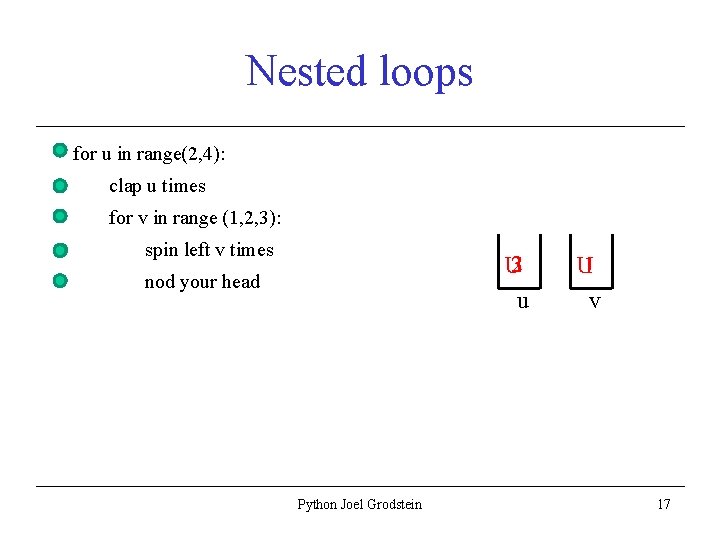
Nested loops for u in range(2, 4): clap u times for v in range (1, 2, 3): spin left v times U 32 u nod your head Python Joel Grodstein U 1 v 17
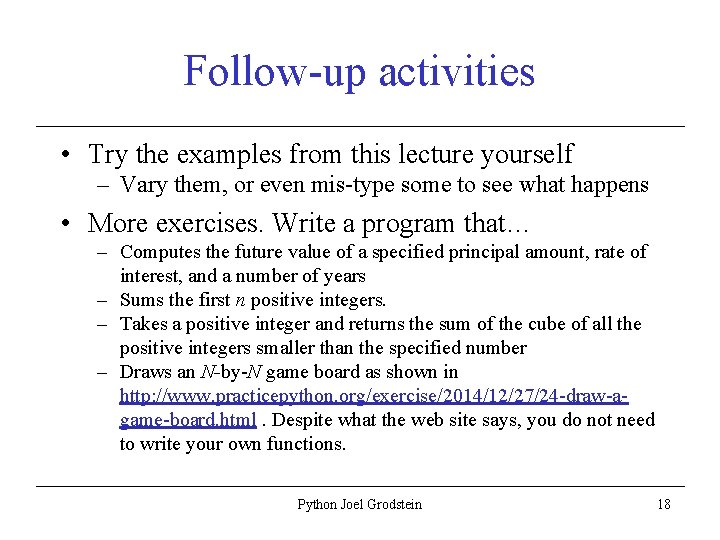
Follow-up activities • Try the examples from this lecture yourself – Vary them, or even mis-type some to see what happens • More exercises. Write a program that… – Computes the future value of a specified principal amount, rate of interest, and a number of years – Sums the first n positive integers. – Takes a positive integer and returns the sum of the cube of all the positive integers smaller than the specified number – Draws an N-by-N game board as shown in http: //www. practicepython. org/exercise/2014/12/27/24 -draw-agame-board. html. Despite what the web site says, you do not need to write your own functions. Python Joel Grodstein 18
Joel grodstein
Joel grodstein
Is tufts liberal arts
Cast of spring, summer, fall, winter... and spring
Summer winter autumn spring months
Spring io conference 2020
Math enrichment spring 2020
Cs162 spring 2020
Mem8000
Tufts anesthesiology residency
Tufts ilvs
Borders
Tufts science and technology center
Neuromodulator
Tufts tusk
Tufts navigator gym reimbursement
Enamel tufts
Enamel spindle
Tufts cluster