Programming Example Highest GPA Input The program reads
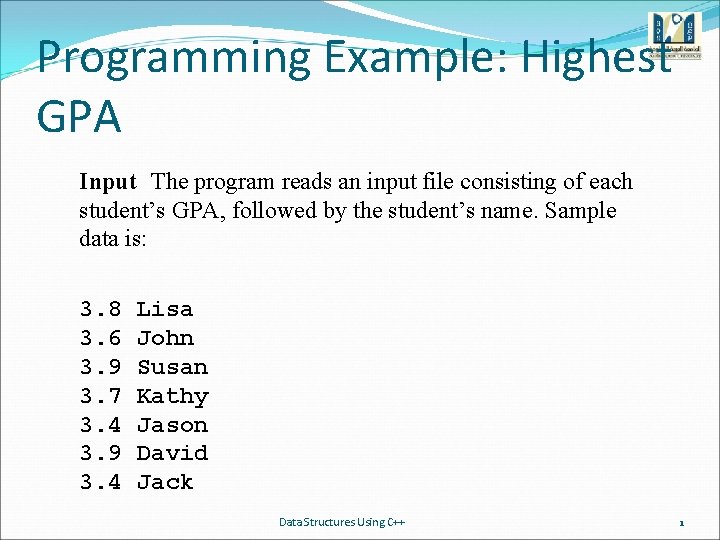
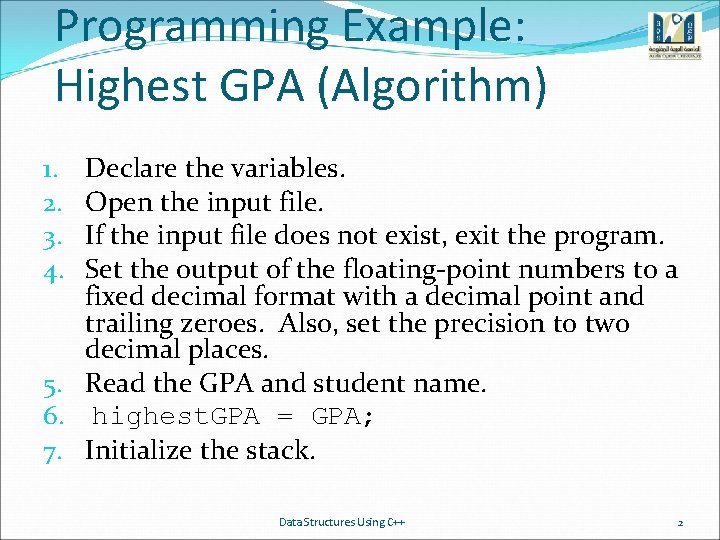
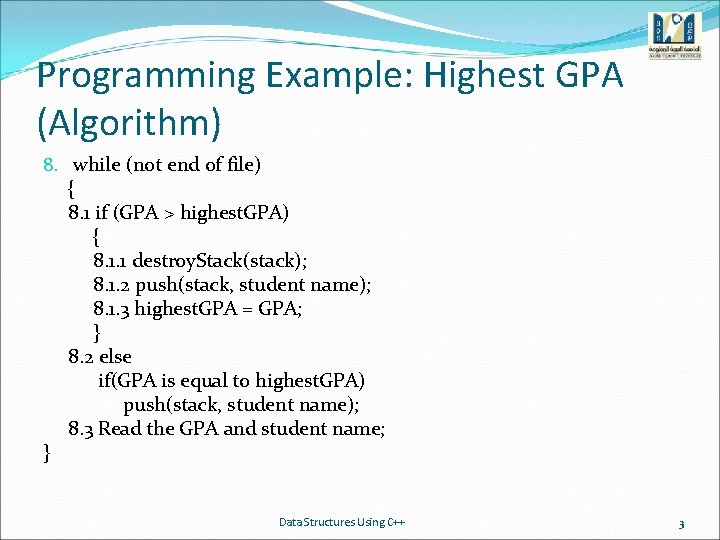
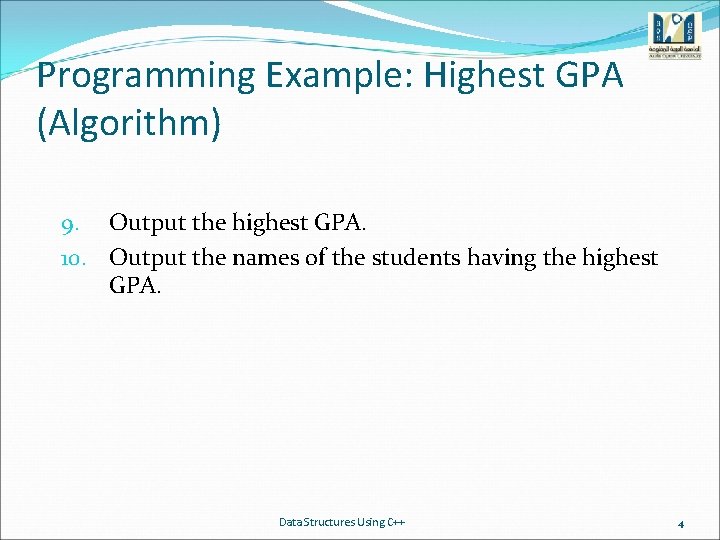
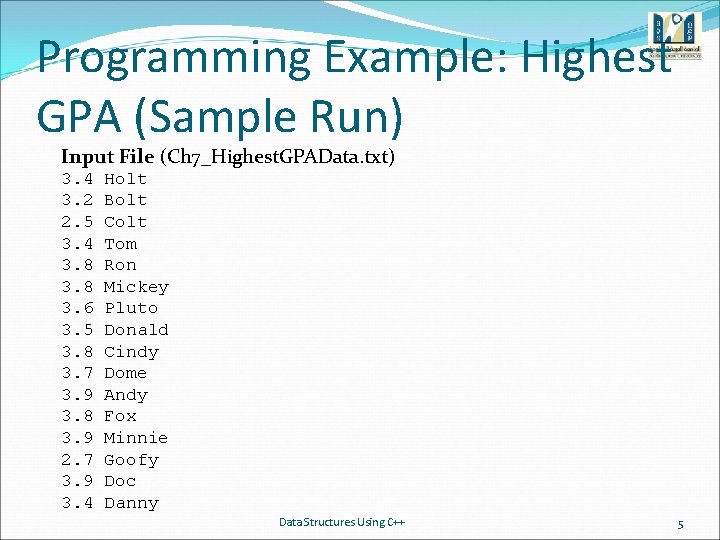
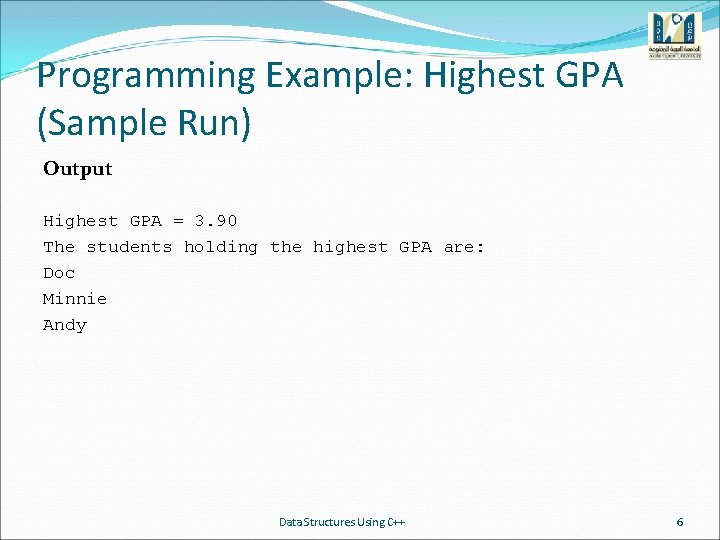
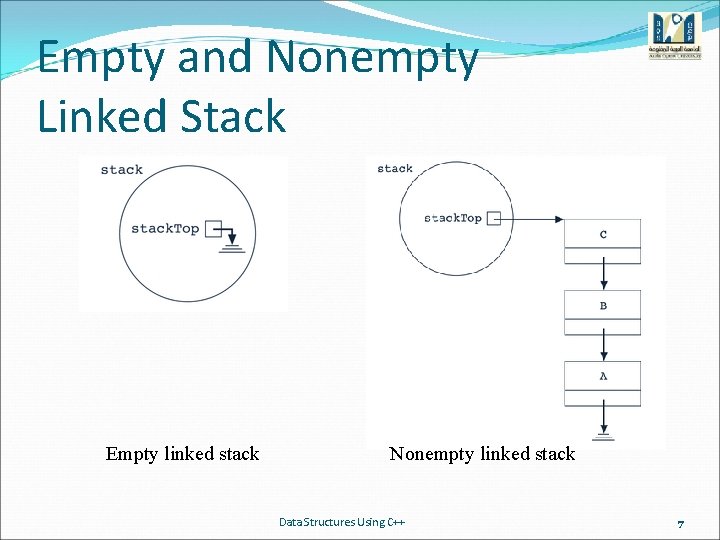
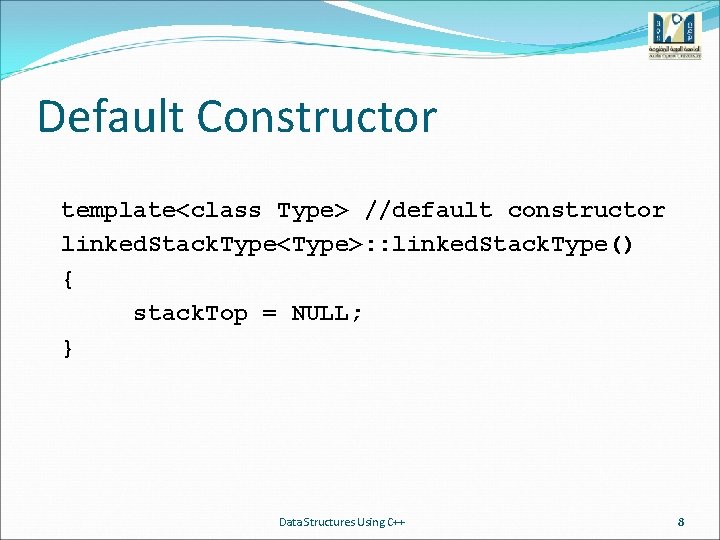
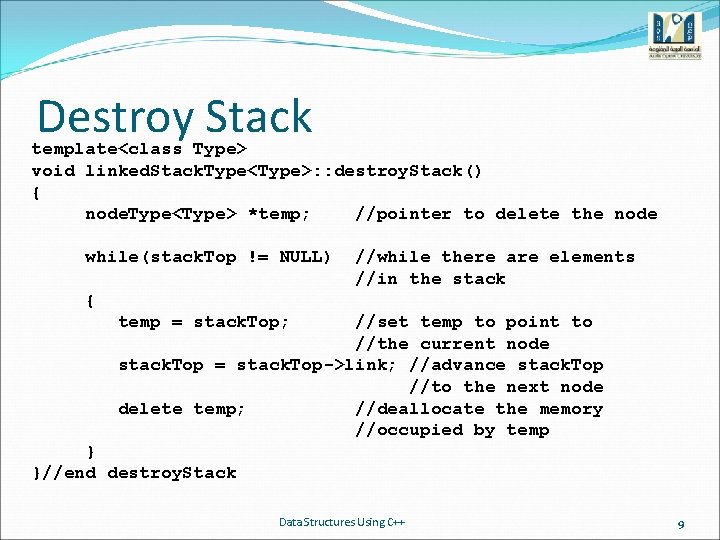
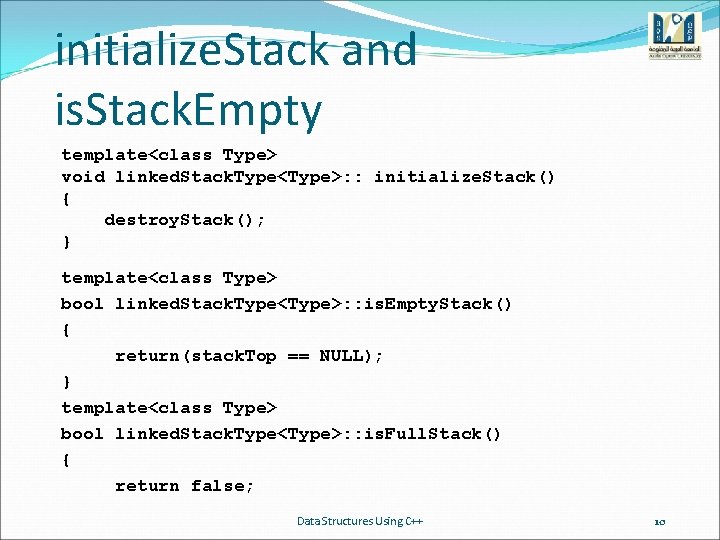
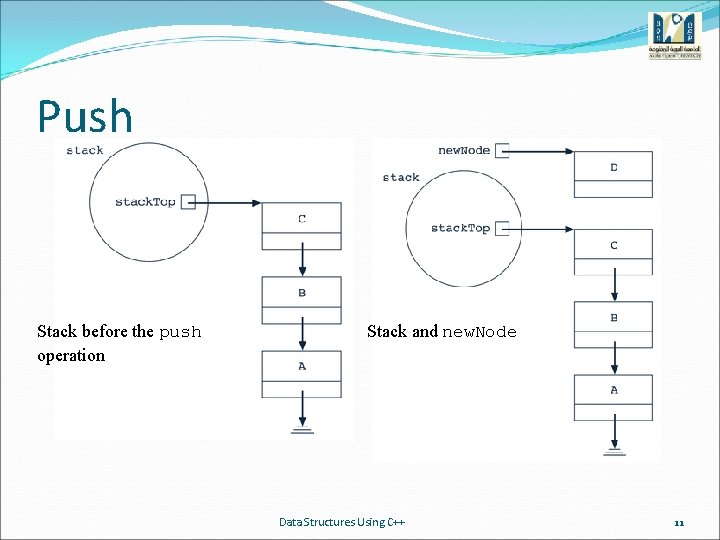
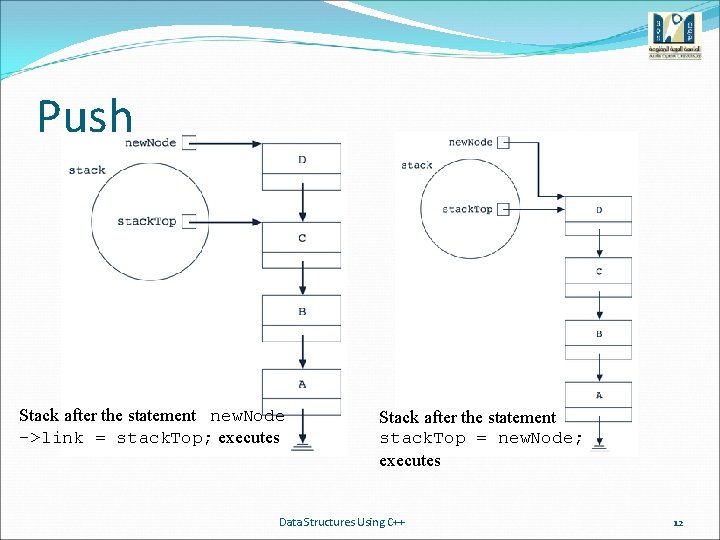
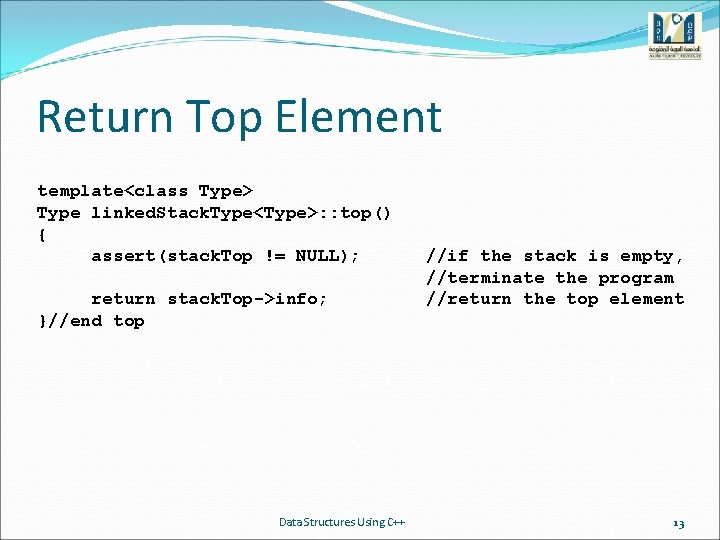
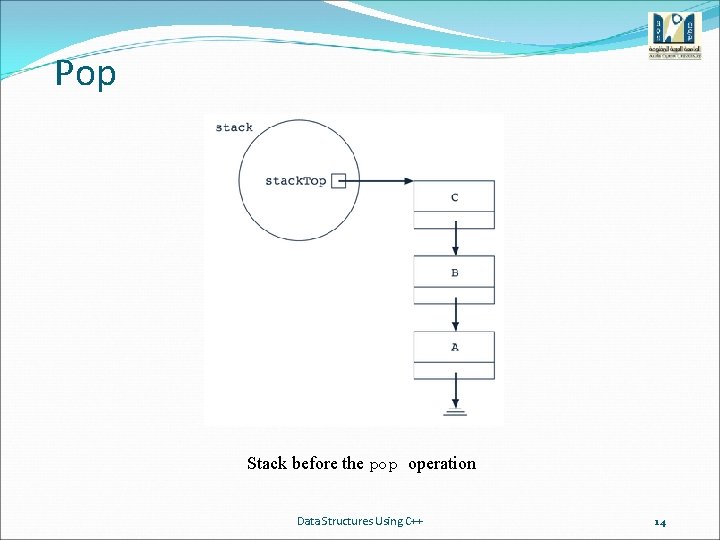
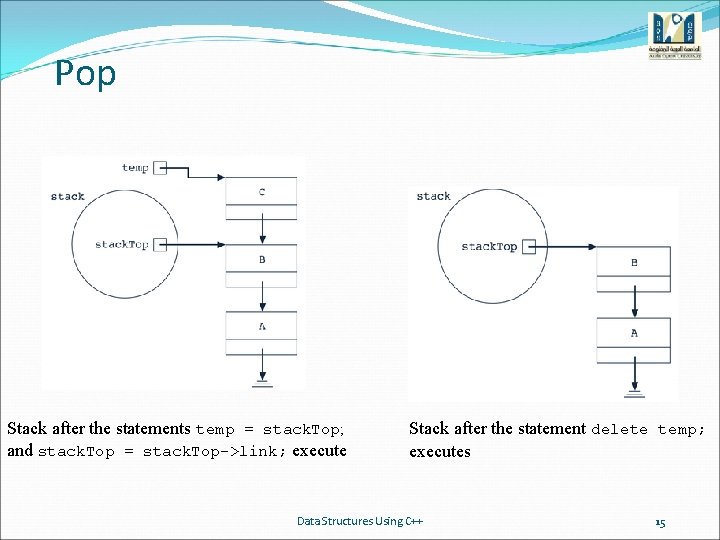
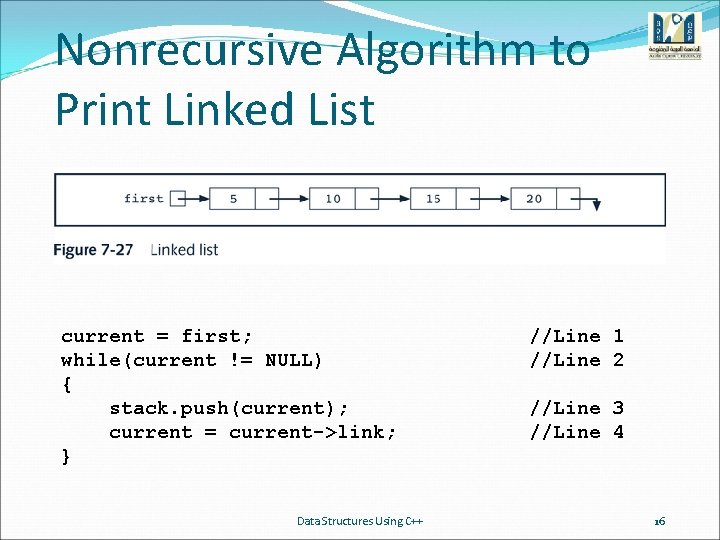
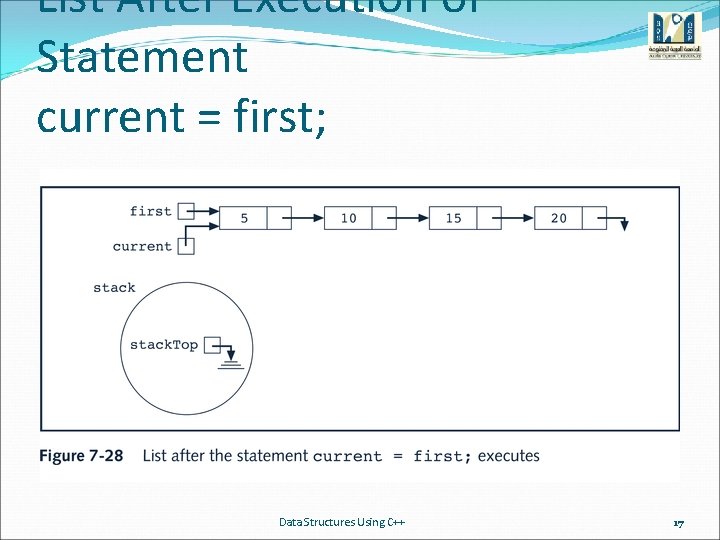
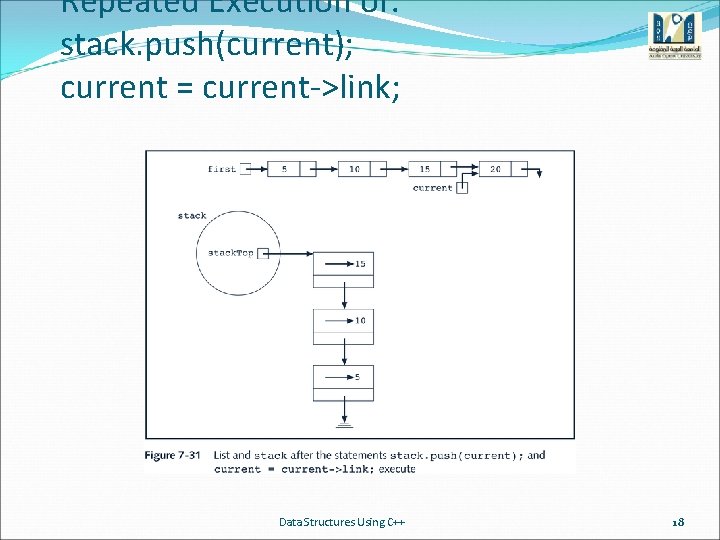
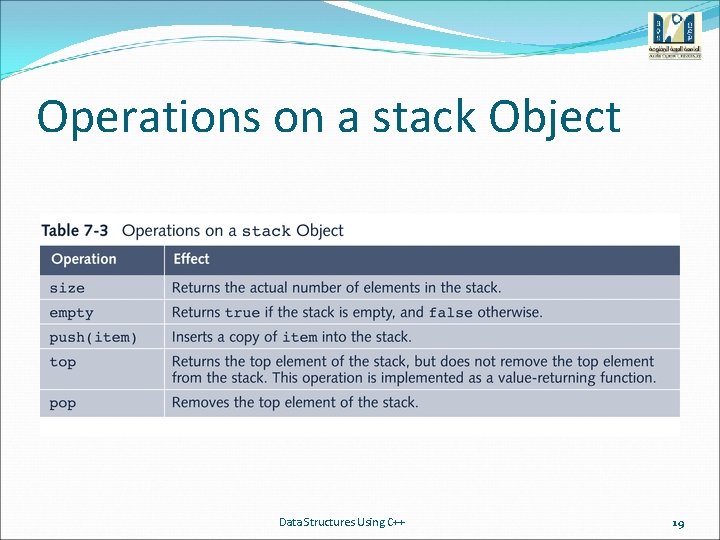
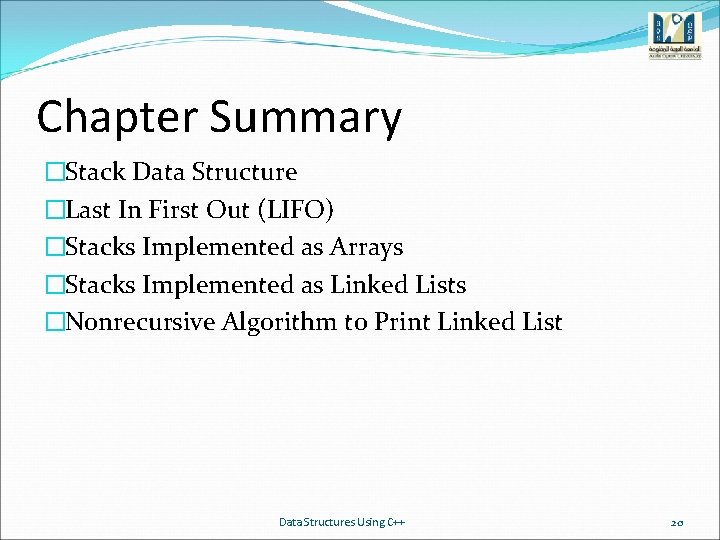
- Slides: 20
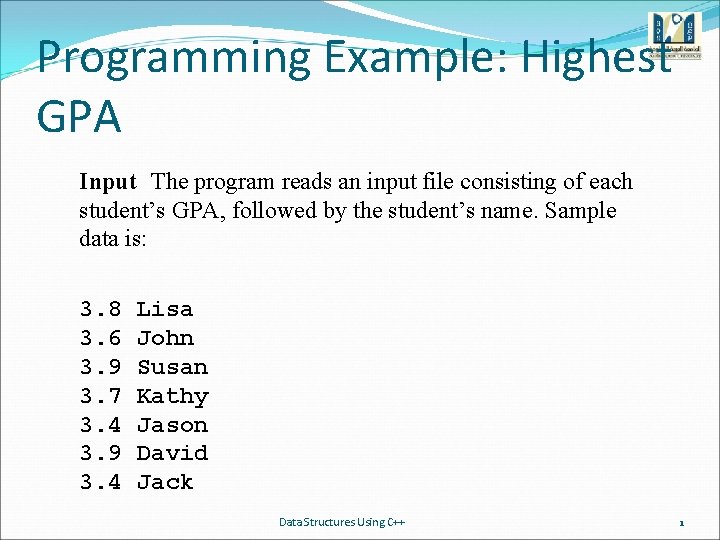
Programming Example: Highest GPA Input The program reads an input file consisting of each student’s GPA, followed by the student’s name. Sample data is: 3. 8 3. 6 3. 9 3. 7 3. 4 3. 9 3. 4 Lisa John Susan Kathy Jason David Jack Data Structures Using C++ 1
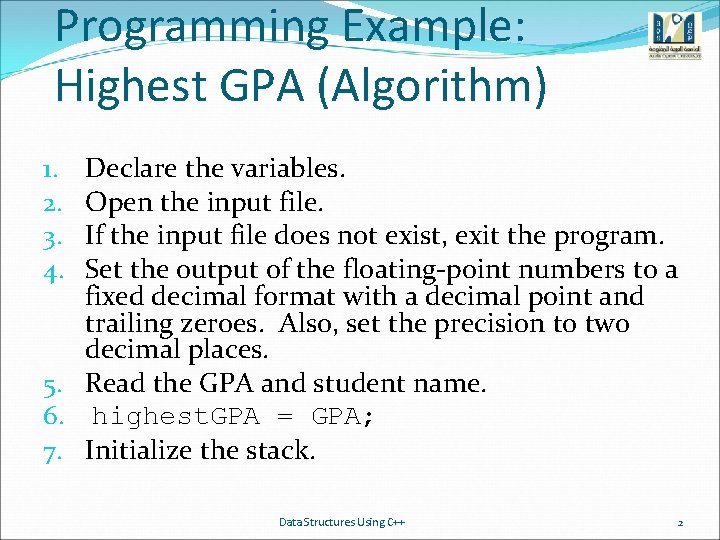
Programming Example: Highest GPA (Algorithm) Declare the variables. Open the input file. If the input file does not exist, exit the program. Set the output of the floating-point numbers to a fixed decimal format with a decimal point and trailing zeroes. Also, set the precision to two decimal places. 5. Read the GPA and student name. 6. highest. GPA = GPA; 7. Initialize the stack. 1. 2. 3. 4. Data Structures Using C++ 2
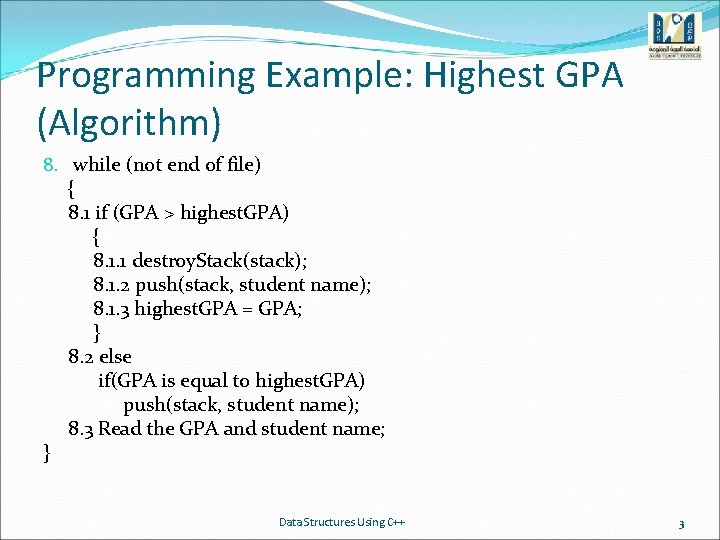
Programming Example: Highest GPA (Algorithm) 8. while (not end of file) { 8. 1 if (GPA > highest. GPA) { 8. 1. 1 destroy. Stack(stack); 8. 1. 2 push(stack, student name); 8. 1. 3 highest. GPA = GPA; } 8. 2 else if(GPA is equal to highest. GPA) push(stack, student name); 8. 3 Read the GPA and student name; } Data Structures Using C++ 3
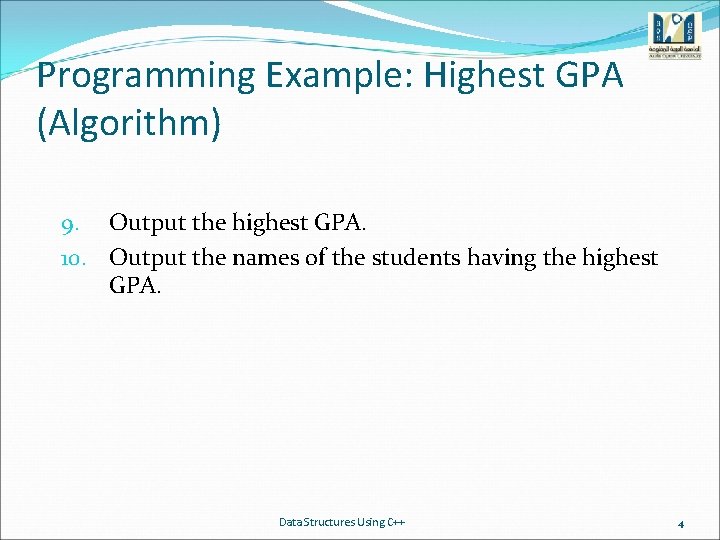
Programming Example: Highest GPA (Algorithm) 9. Output the highest GPA. 10. Output the names of the students having the highest GPA. Data Structures Using C++ 4
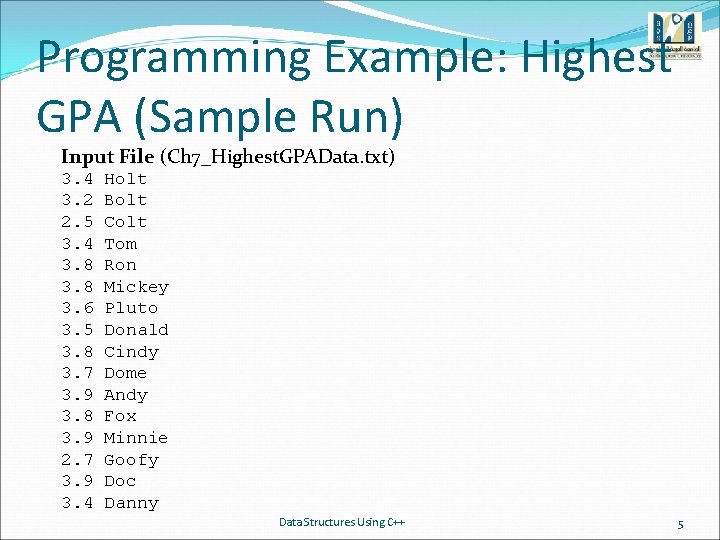
Programming Example: Highest GPA (Sample Run) Input File (Ch 7_Highest. GPAData. txt) 3. 4 3. 2 2. 5 3. 4 3. 8 3. 6 3. 5 3. 8 3. 7 3. 9 3. 8 3. 9 2. 7 3. 9 3. 4 Holt Bolt Colt Tom Ron Mickey Pluto Donald Cindy Dome Andy Fox Minnie Goofy Doc Danny Data Structures Using C++ 5
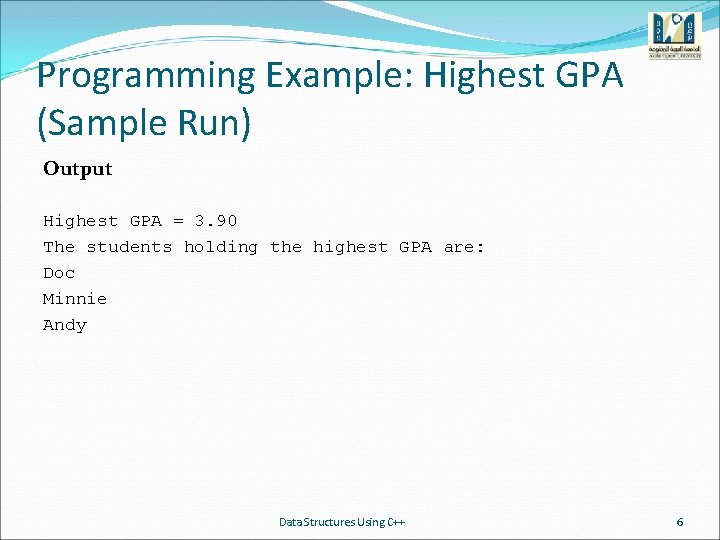
Programming Example: Highest GPA (Sample Run) Output Highest GPA = 3. 90 The students holding the highest GPA are: Doc Minnie Andy Data Structures Using C++ 6
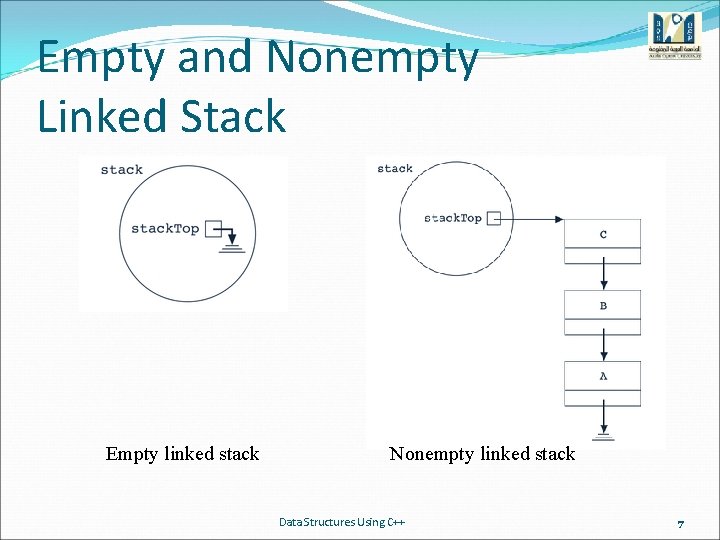
Empty and Nonempty Linked Stack Empty linked stack Nonempty linked stack Data Structures Using C++ 7
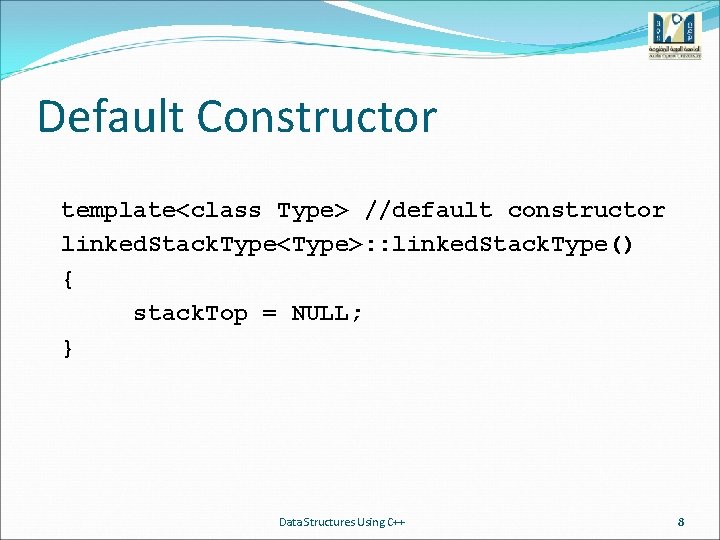
Default Constructor template<class Type> //default constructor linked. Stack. Type<Type>: : linked. Stack. Type() { stack. Top = NULL; } Data Structures Using C++ 8
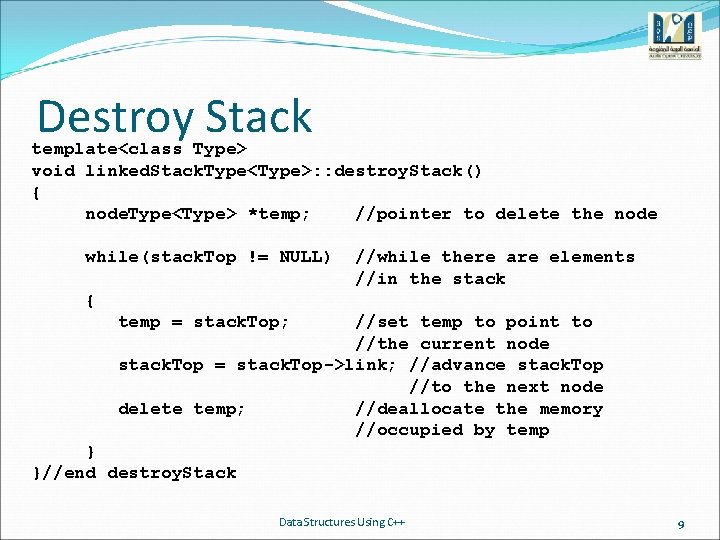
Destroy Stack template<class Type> void linked. Stack. Type<Type>: : destroy. Stack() { node. Type<Type> *temp; //pointer to delete the node while(stack. Top != NULL) //while there are elements //in the stack { temp = stack. Top; //set temp to point to //the current node stack. Top = stack. Top->link; //advance stack. Top //to the next node delete temp; //deallocate the memory //occupied by temp } }//end destroy. Stack Data Structures Using C++ 9
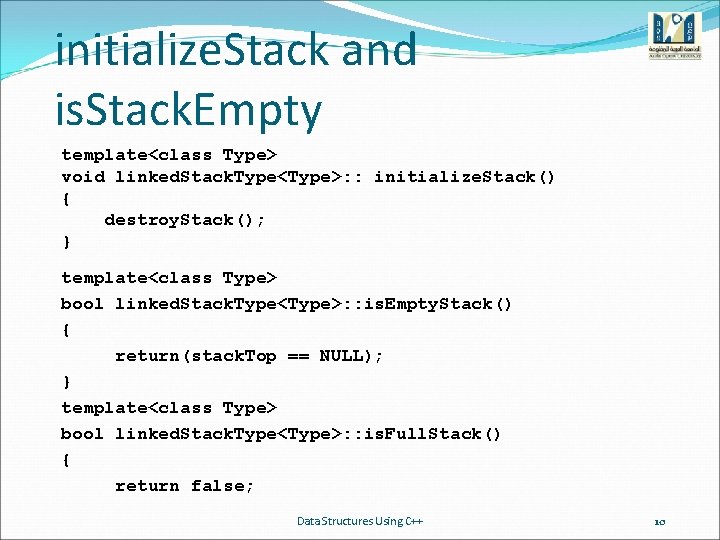
initialize. Stack and is. Stack. Empty template<class Type> void linked. Stack. Type<Type>: : initialize. Stack() { destroy. Stack(); } template<class Type> bool linked. Stack. Type<Type>: : is. Empty. Stack() { return(stack. Top == NULL); } template<class Type> bool linked. Stack. Type<Type>: : is. Full. Stack() { return false; Data Structures Using C++ 10
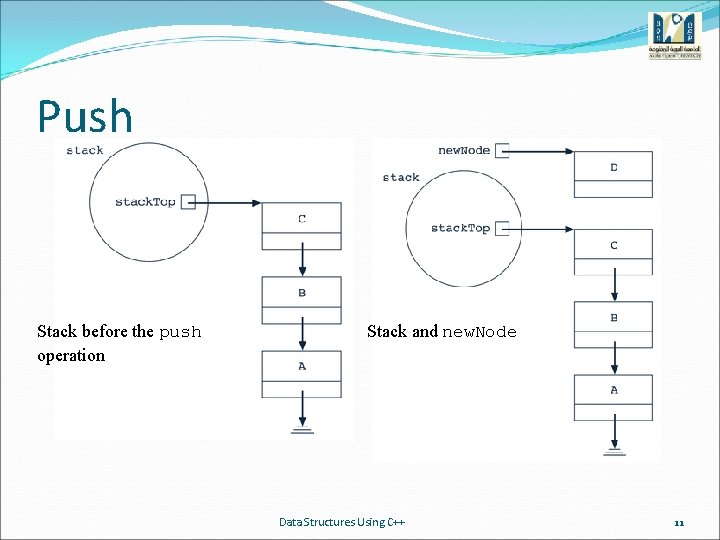
Push Stack before the push operation Stack and new. Node Data Structures Using C++ 11
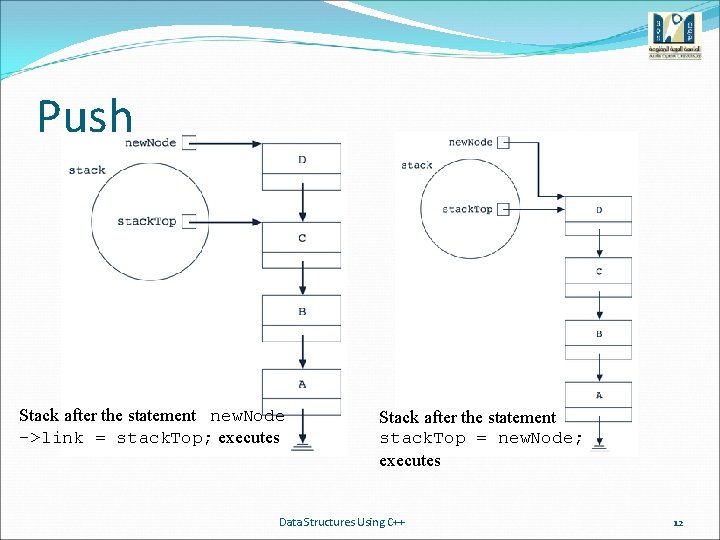
Push Stack after the statement new. Node ->link = stack. Top; executes Stack after the statement stack. Top = new. Node; executes Data Structures Using C++ 12
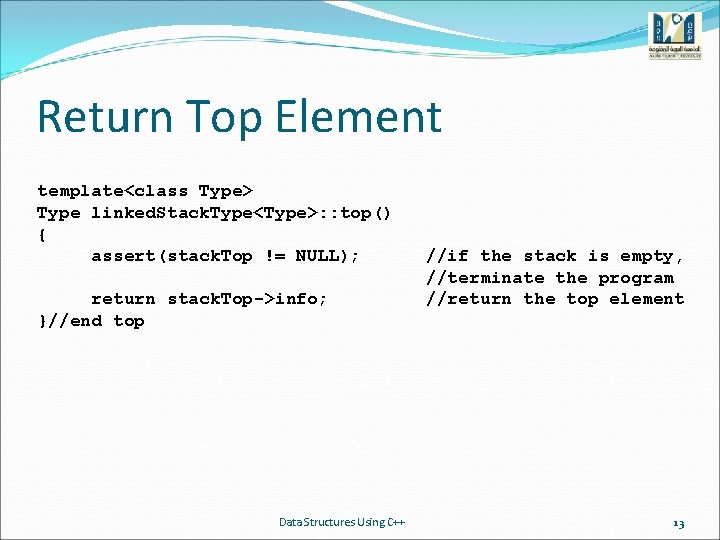
Return Top Element template<class Type> Type linked. Stack. Type<Type>: : top() { assert(stack. Top != NULL); return stack. Top->info; }//end top Data Structures Using C++ //if the stack is empty, //terminate the program //return the top element 13
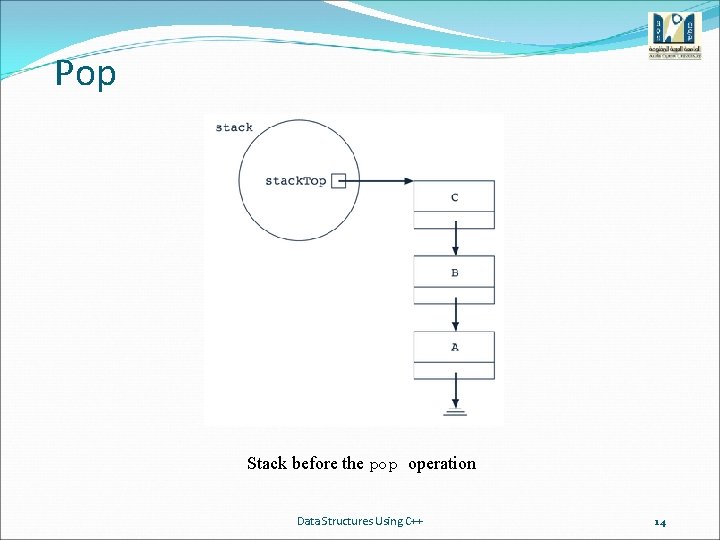
Pop Stack before the pop operation Data Structures Using C++ 14
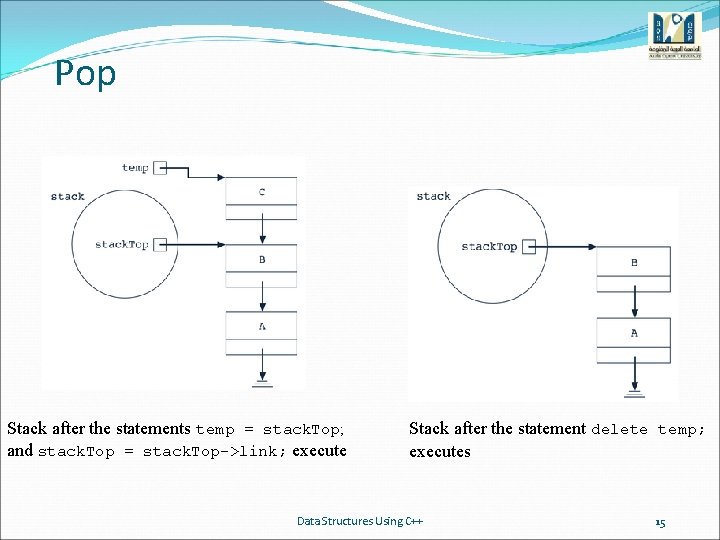
Pop Stack after the statements temp = stack. Top; and stack. Top = stack. Top->link; execute Stack after the statement delete temp; executes Data Structures Using C++ 15
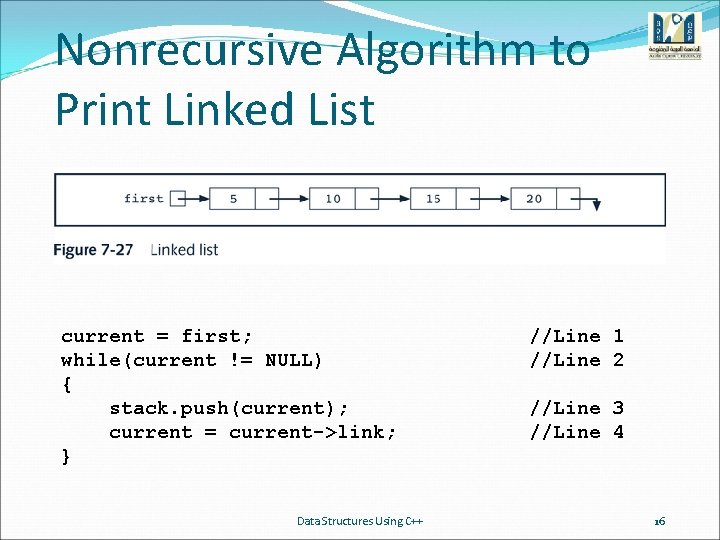
Nonrecursive Algorithm to Print Linked List current = first; while(current != NULL) { stack. push(current); current = current->link; } Data Structures Using C++ //Line 1 //Line 2 //Line 3 //Line 4 16
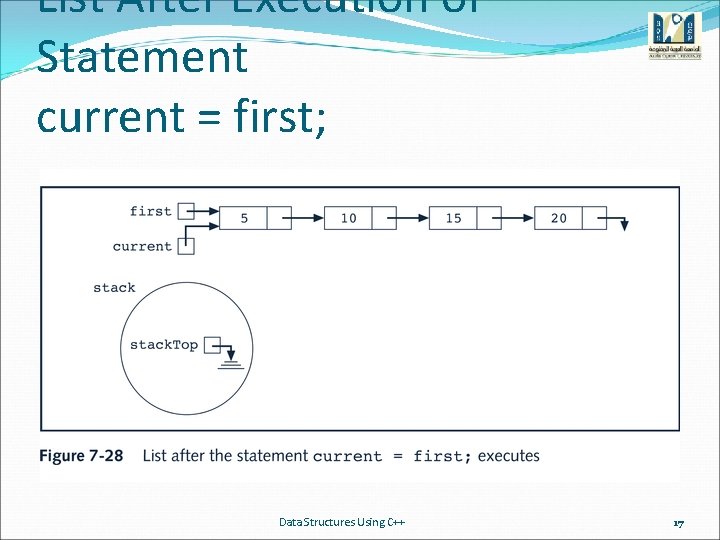
List After Execution of Statement current = first; Data Structures Using C++ 17
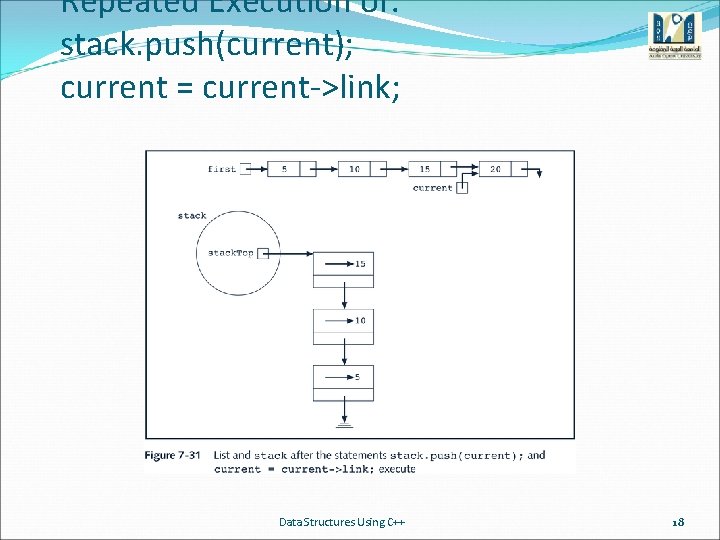
Repeated Execution of: stack. push(current); current = current->link; Data Structures Using C++ 18
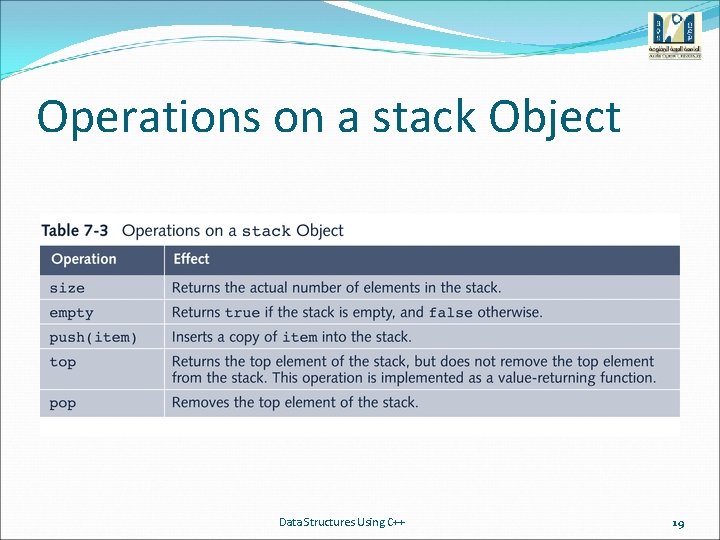
Operations on a stack Object Data Structures Using C++ 19
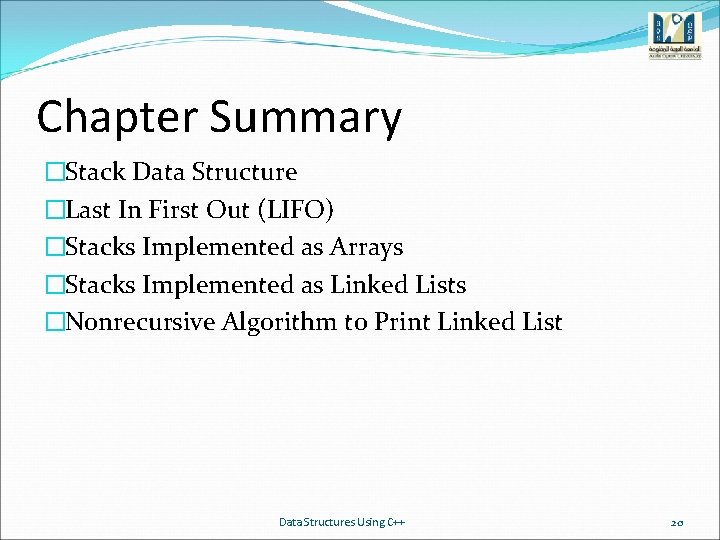
Chapter Summary �Stack Data Structure �Last In First Out (LIFO) �Stacks Implemented as Arrays �Stacks Implemented as Linked Lists �Nonrecursive Algorithm to Print Linked List Data Structures Using C++ 20
Core course gpa
Cumulative gpa vs core gpa
Hoghest gpa
Highest weighted gpa
Input device introduction
Scanners produce text and graphics on a physical medium
Input devices adalah
Finely tuned input and roughly tuned input
Present simple
Andy sometimes comics
Look junko is jumping into the water
Bowtie2
Label computer parts
Nebraska reads approved assessments
Bandage github
The raven stanza 9 summary
Saskatchewan reads
Uscs micrometer reads in
Tyshane went swimming with friends
Fgood reads
When everybody has short hair what does the rebel do answer