OVERVIEW OF CLIENTSIDE SCRIPTING Welcome to clientside scripting
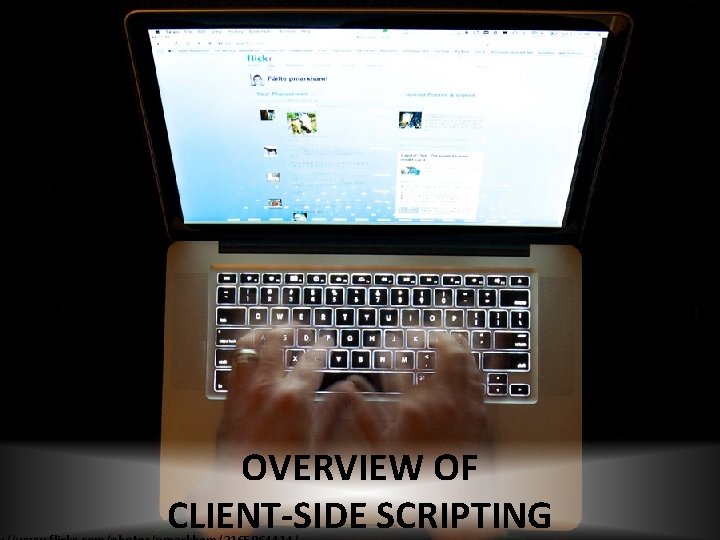
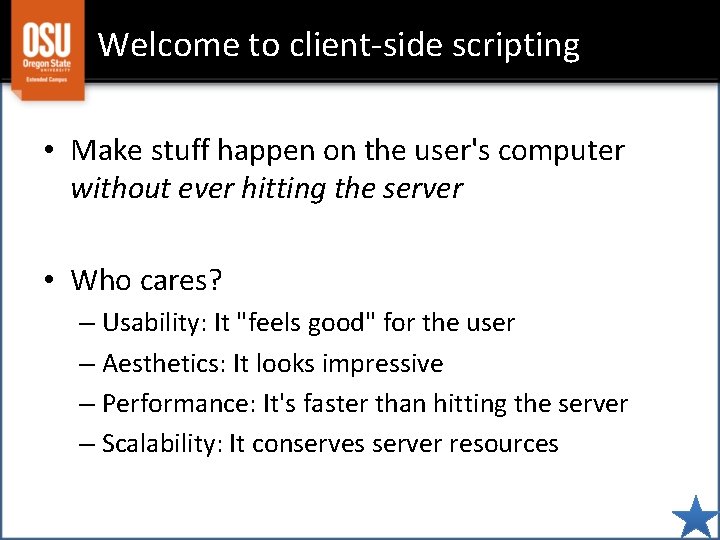
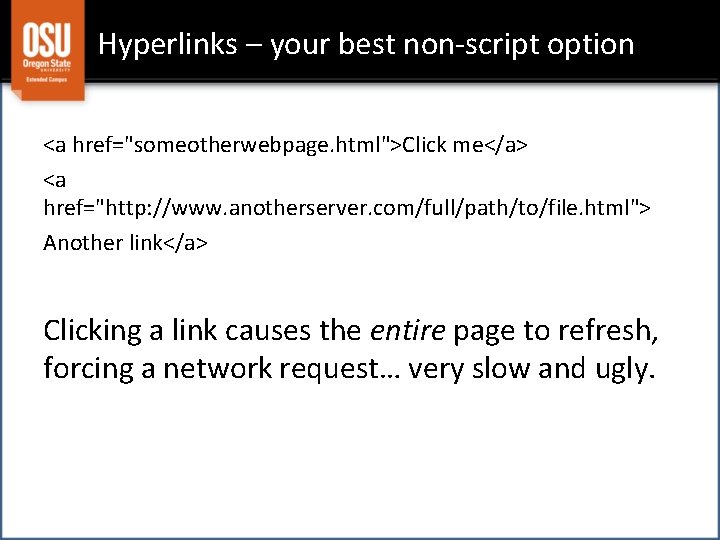
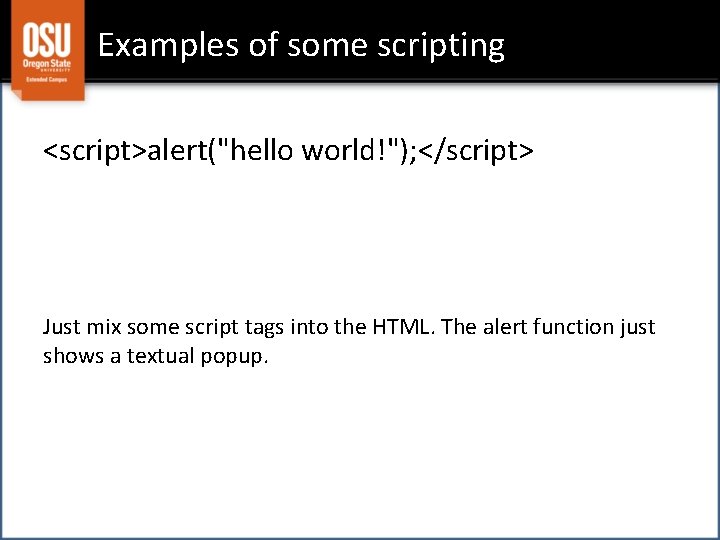
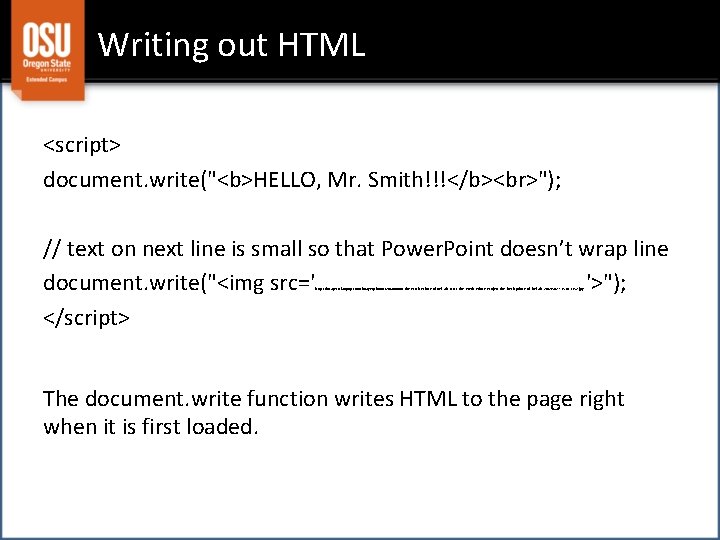
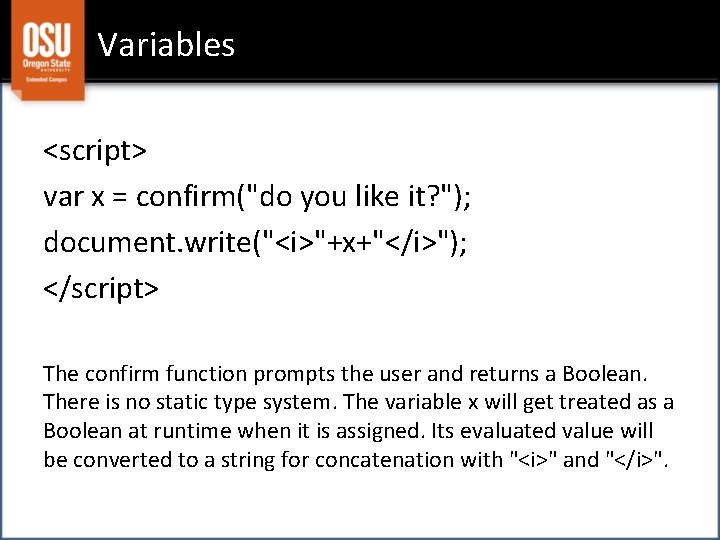
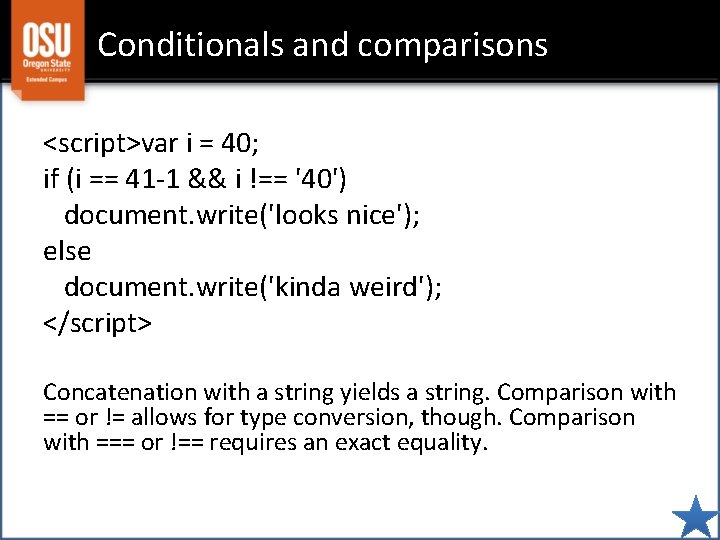
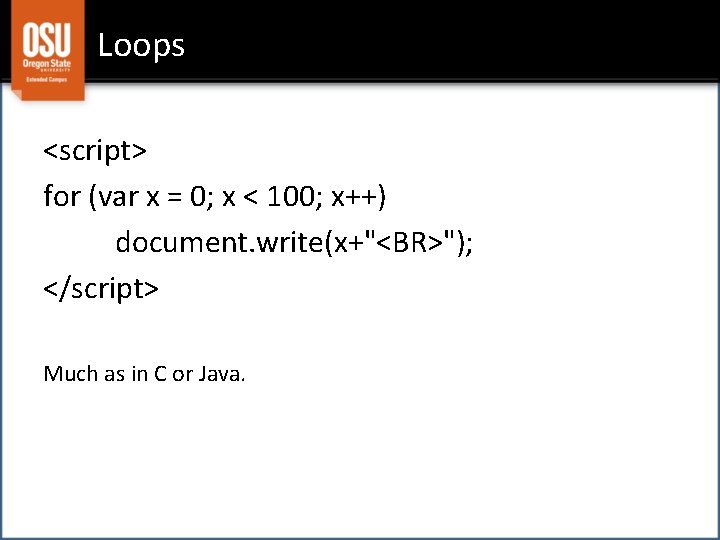
![Arrays <script> var myarray = ["A", "B", "C", "D"]; document. write(myarray[1]+"ob is the man!"); Arrays <script> var myarray = ["A", "B", "C", "D"]; document. write(myarray[1]+"ob is the man!");](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-9.jpg)
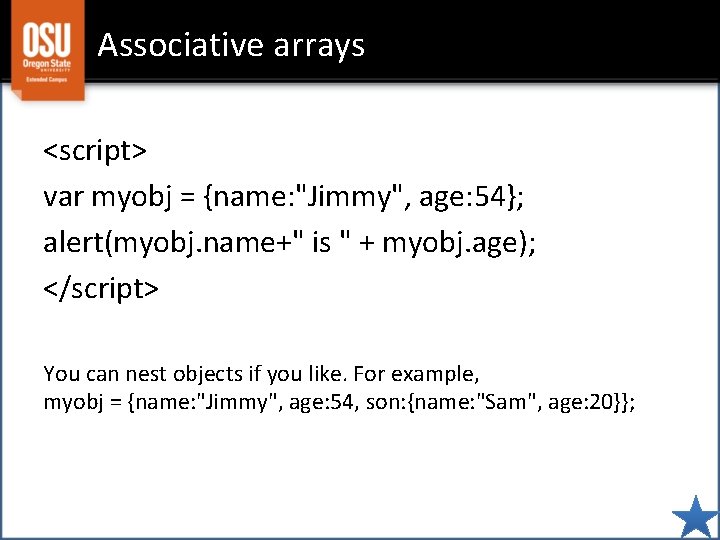
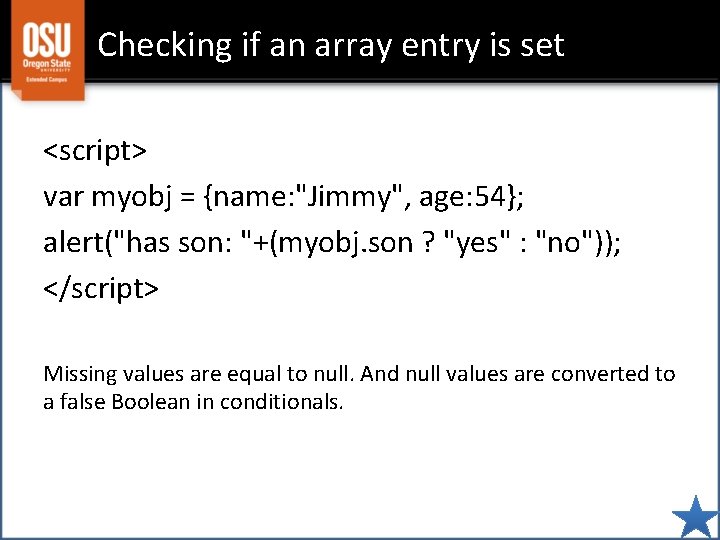
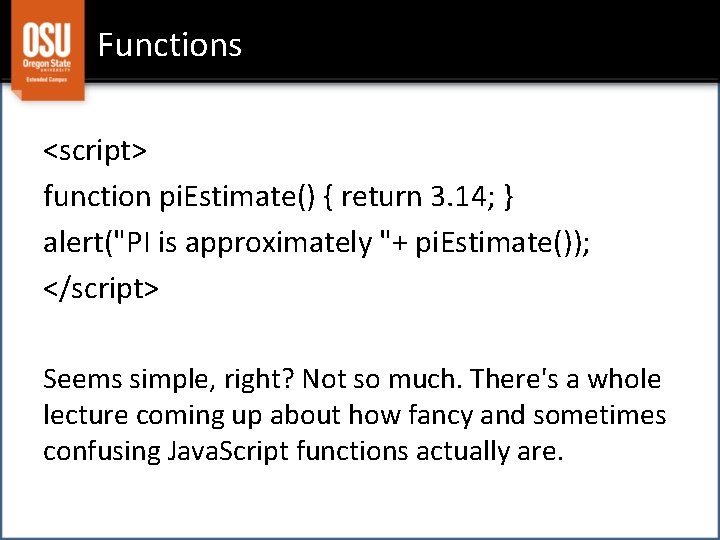
![Regular expressions <script> var isint = /^-? [0 -9]+$/; var strs = ["888", "bob", Regular expressions <script> var isint = /^-? [0 -9]+$/; var strs = ["888", "bob",](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-13.jpg)
![Regular expressions cheat sheet [0 -9] means any digit [a-z] means any lowercase letter Regular expressions cheat sheet [0 -9] means any digit [a-z] means any lowercase letter](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-14.jpg)
![Regular expressions examples • [0 -9]{5} means five digits • [A-Z][a-z]+ means an uppercase Regular expressions examples • [0 -9]{5} means five digits • [A-Z][a-z]+ means an uppercase](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-15.jpg)
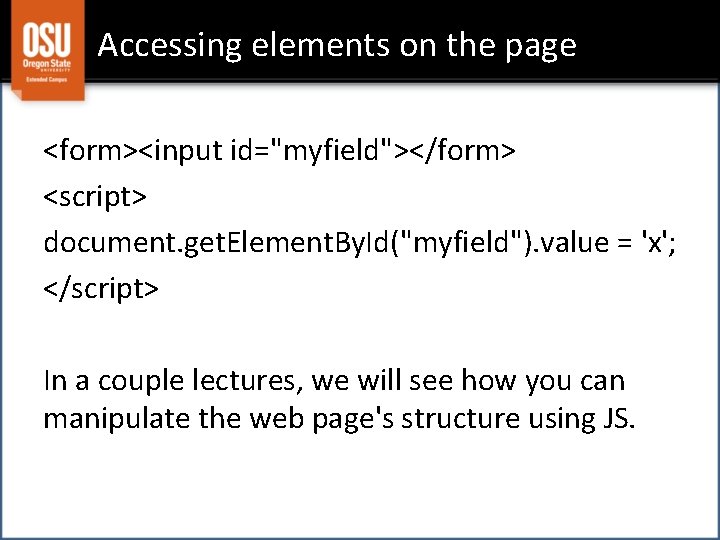
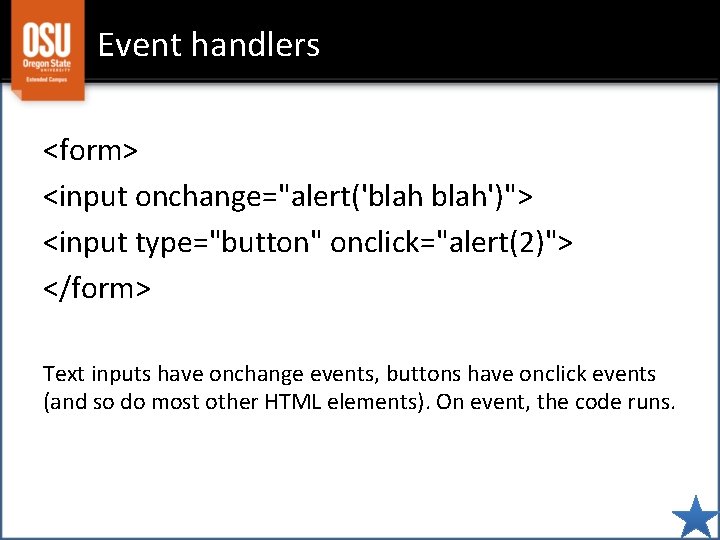
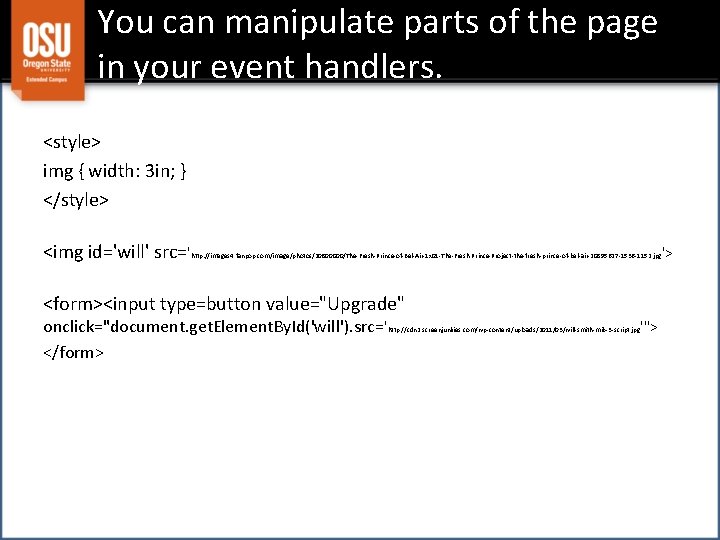
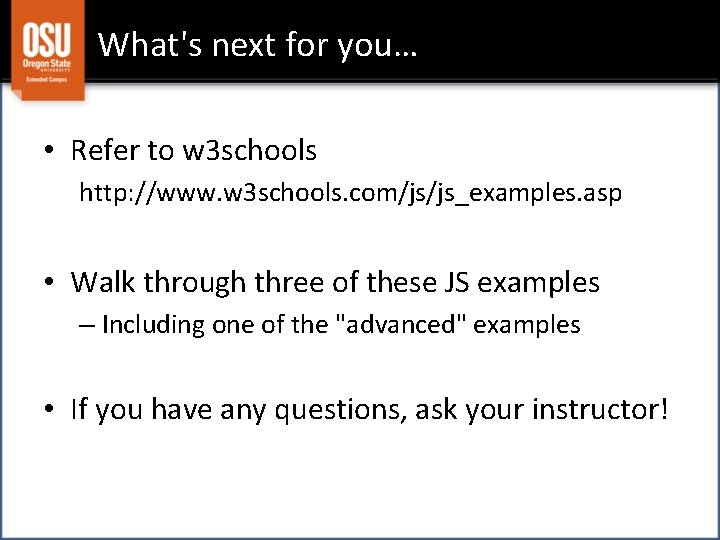
- Slides: 19
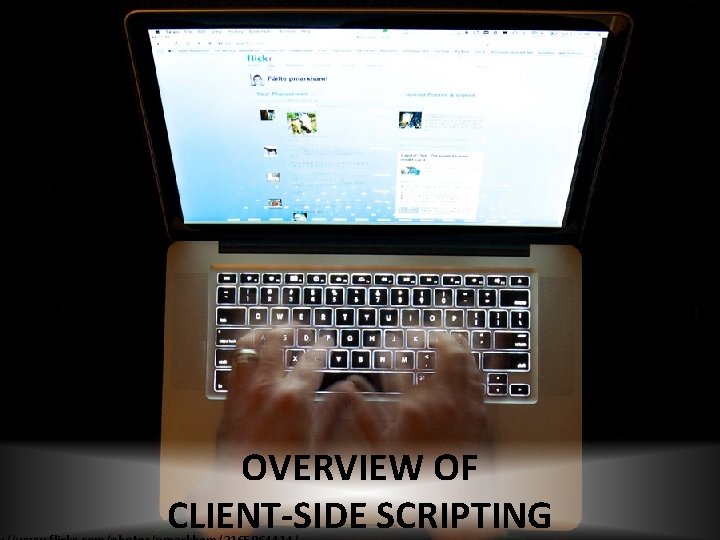
OVERVIEW OF CLIENT-SIDE SCRIPTING
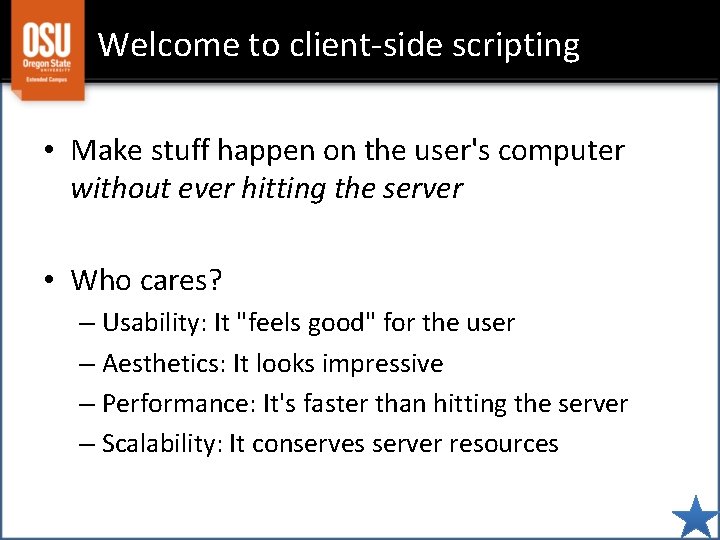
Welcome to client-side scripting • Make stuff happen on the user's computer without ever hitting the server • Who cares? – Usability: It "feels good" for the user – Aesthetics: It looks impressive – Performance: It's faster than hitting the server – Scalability: It conserves server resources
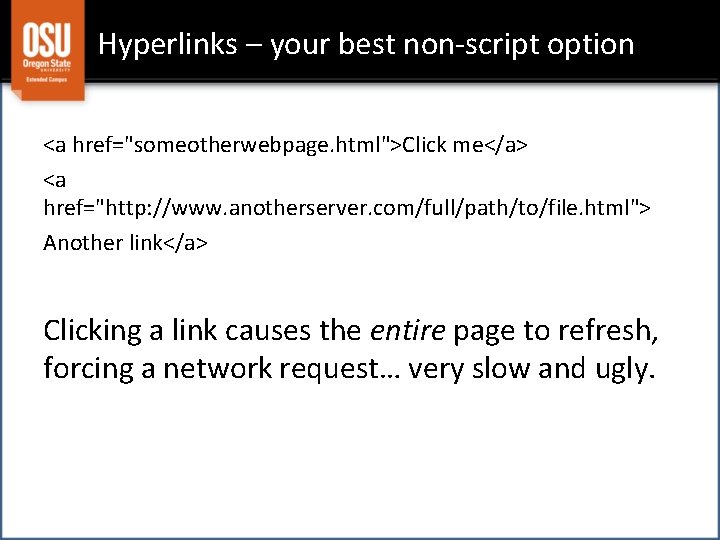
Hyperlinks – your best non-script option <a href="someotherwebpage. html">Click me</a> <a href="http: //www. anotherserver. com/full/path/to/file. html"> Another link</a> Clicking a link causes the entire page to refresh, forcing a network request… very slow and ugly.
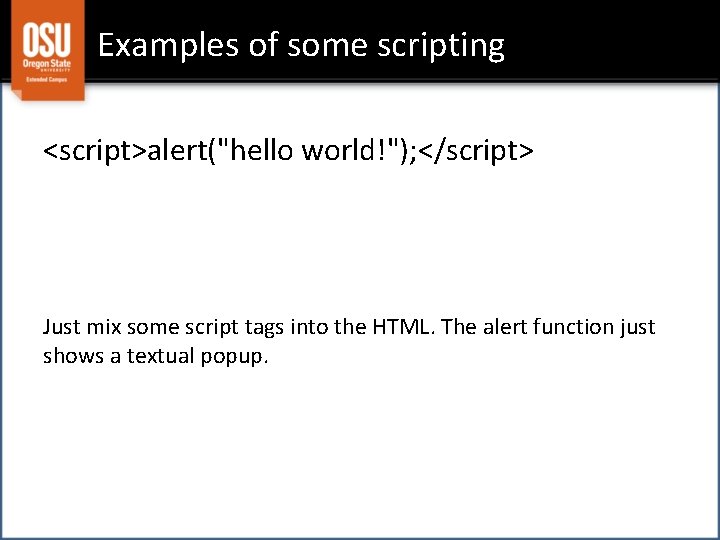
Examples of some scripting <script>alert("hello world!"); </script> Just mix some script tags into the HTML. The alert function just shows a textual popup.
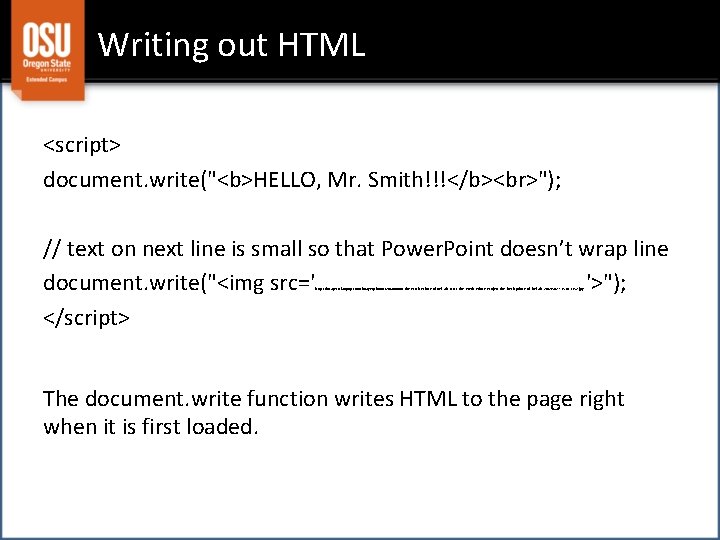
Writing out HTML <script> document. write("<b>HELLO, Mr. Smith!!!</b> "); // text on next line is small so that Power. Point doesn’t wrap line document. write("<img src=' '>"); </script> http: //images 4. fanpop. com/image/photos/20800000/The-Fresh-Prince-of-Bel-Air-1 x 01 -The-Fresh-Prince-Project-the-fresh-prince-of-bel-air-20895627 -1536 -1152. jpg The document. write function writes HTML to the page right when it is first loaded.
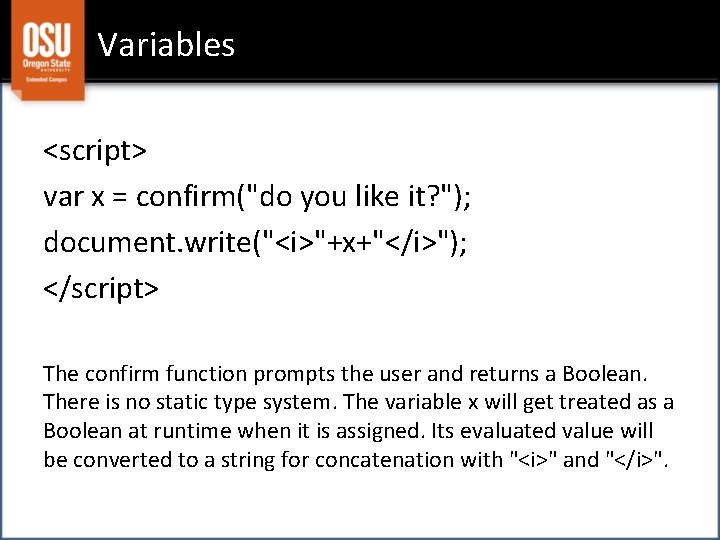
Variables <script> var x = confirm("do you like it? "); document. write("<i>"+x+"</i>"); </script> The confirm function prompts the user and returns a Boolean. There is no static type system. The variable x will get treated as a Boolean at runtime when it is assigned. Its evaluated value will be converted to a string for concatenation with "<i>" and "</i>".
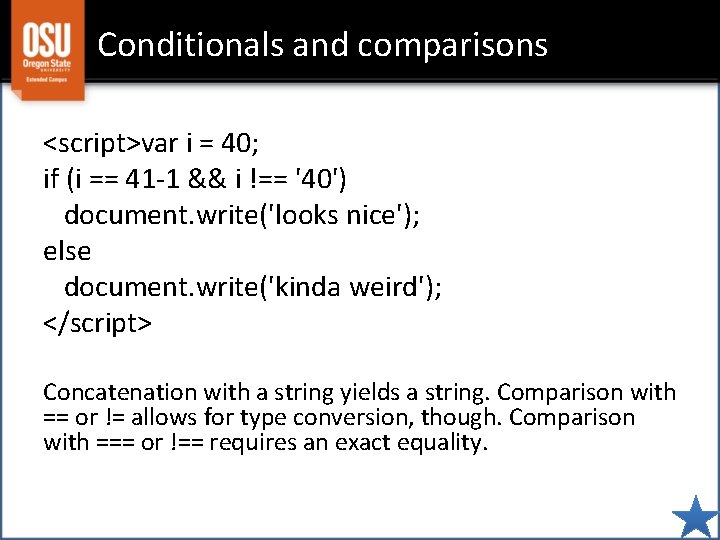
Conditionals and comparisons <script>var i = 40; if (i == 41 -1 && i !== '40') document. write('looks nice'); else document. write('kinda weird'); </script> Concatenation with a string yields a string. Comparison with == or != allows for type conversion, though. Comparison with === or !== requires an exact equality.
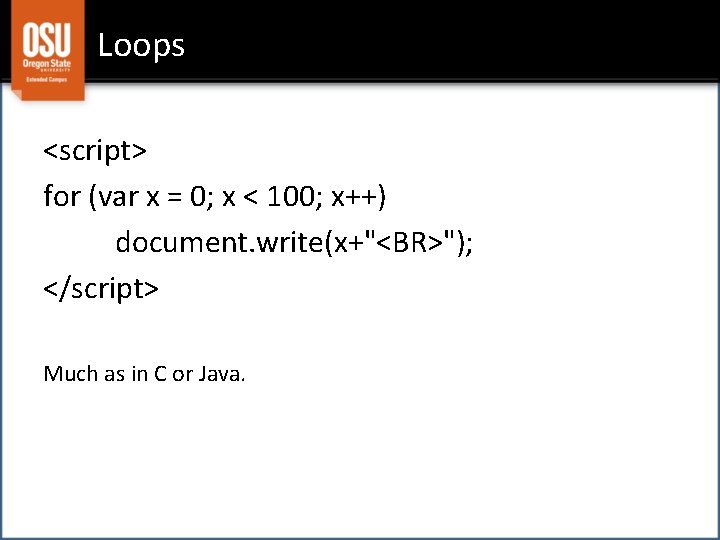
Loops <script> for (var x = 0; x < 100; x++) document. write(x+"<BR>"); </script> Much as in C or Java.
![Arrays script var myarray A B C D document writemyarray1ob is the man Arrays <script> var myarray = ["A", "B", "C", "D"]; document. write(myarray[1]+"ob is the man!");](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-9.jpg)
Arrays <script> var myarray = ["A", "B", "C", "D"]; document. write(myarray[1]+"ob is the man!"); </script> Each array object is indexed from 0 and has a. length property that indicates its number of elements.
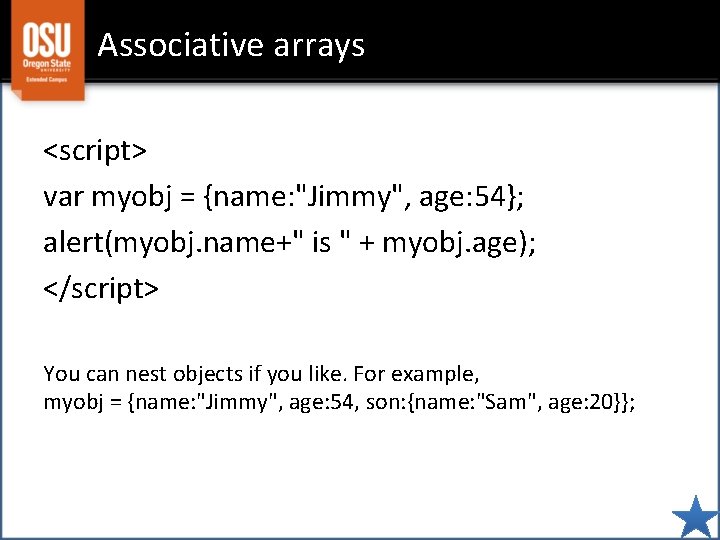
Associative arrays <script> var myobj = {name: "Jimmy", age: 54}; alert(myobj. name+" is " + myobj. age); </script> You can nest objects if you like. For example, myobj = {name: "Jimmy", age: 54, son: {name: "Sam", age: 20}};
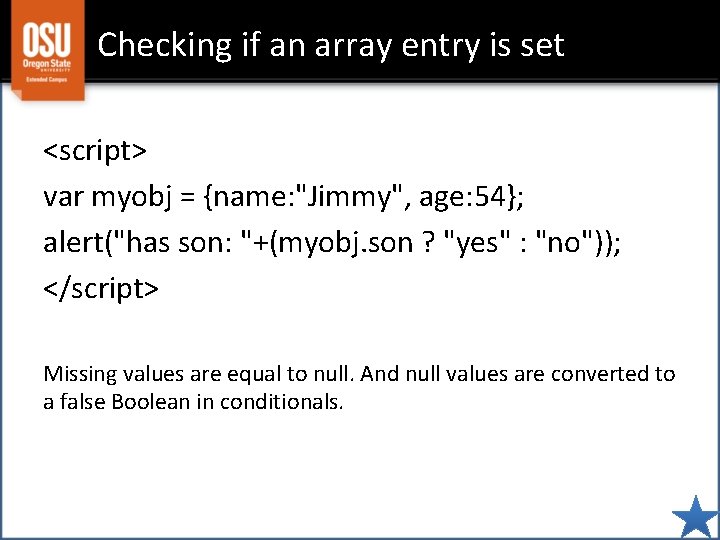
Checking if an array entry is set <script> var myobj = {name: "Jimmy", age: 54}; alert("has son: "+(myobj. son ? "yes" : "no")); </script> Missing values are equal to null. And null values are converted to a false Boolean in conditionals.
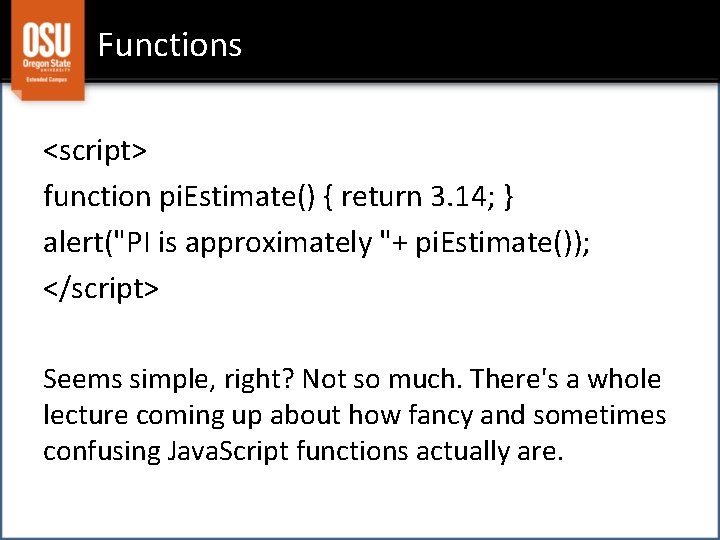
Functions <script> function pi. Estimate() { return 3. 14; } alert("PI is approximately "+ pi. Estimate()); </script> Seems simple, right? Not so much. There's a whole lecture coming up about how fancy and sometimes confusing Java. Script functions actually are.
![Regular expressions script var isint 0 9 var strs 888 bob Regular expressions <script> var isint = /^-? [0 -9]+$/; var strs = ["888", "bob",](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-13.jpg)
Regular expressions <script> var isint = /^-? [0 -9]+$/; var strs = ["888", "bob", "-282"]; for (var i = 0; i < strs. length; i++) document. write(strs[i]+": "+isint. test(strs[i])+"<BR>"); </script> The test function returns true if the string matches the pattern described by the regular expression, false otherwise.
![Regular expressions cheat sheet 0 9 means any digit az means any lowercase letter Regular expressions cheat sheet [0 -9] means any digit [a-z] means any lowercase letter](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-14.jpg)
Regular expressions cheat sheet [0 -9] means any digit [a-z] means any lowercase letter [A-Z] means any uppercase letter (x|y|z) means x or y or z * means 0 or more + means 1 or more ? means 0 or 1 {4 -5} means four or five ^ means starts with $ means ends with. means just about any character is how you escape the special characters above
![Regular expressions examples 0 95 means five digits AZaz means an uppercase Regular expressions examples • [0 -9]{5} means five digits • [A-Z][a-z]+ means an uppercase](https://slidetodoc.com/presentation_image/ca2587ac3a58e3e25ae6cfcd2a4c7819/image-15.jpg)
Regular expressions examples • [0 -9]{5} means five digits • [A-Z][a-z]+ means an uppercase followed by at least one lowercase (maybe more) • (Powerpoint|Open Office) means "Powerpoint" or "Open Office" • (Rubicon|Rider) v. [0 -9] matches "Rubicon v. 3. 2" and "Rider v. 1. 1" and "Rider v. 7. 2", etc
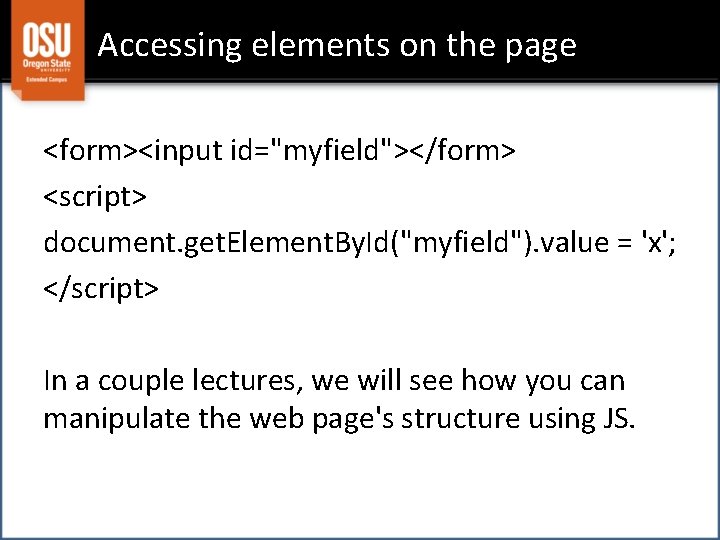
Accessing elements on the page <form><input id="myfield"></form> <script> document. get. Element. By. Id("myfield"). value = 'x'; </script> In a couple lectures, we will see how you can manipulate the web page's structure using JS.
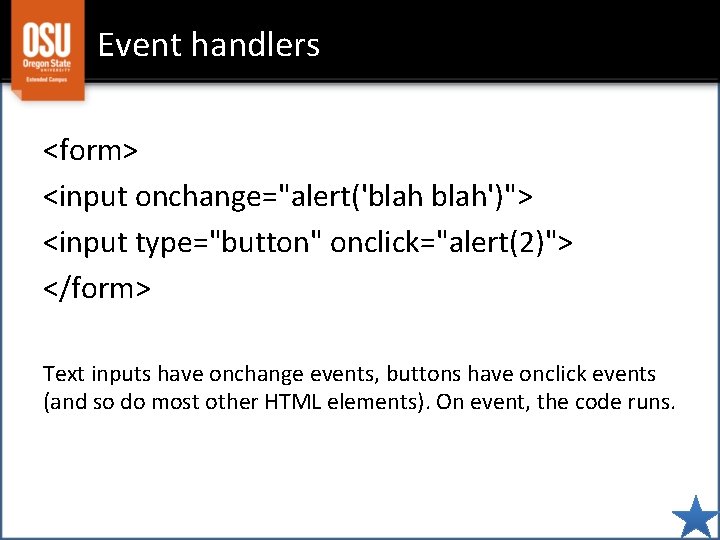
Event handlers <form> <input onchange="alert('blah')"> <input type="button" onclick="alert(2)"> </form> Text inputs have onchange events, buttons have onclick events (and so do most other HTML elements). On event, the code runs.
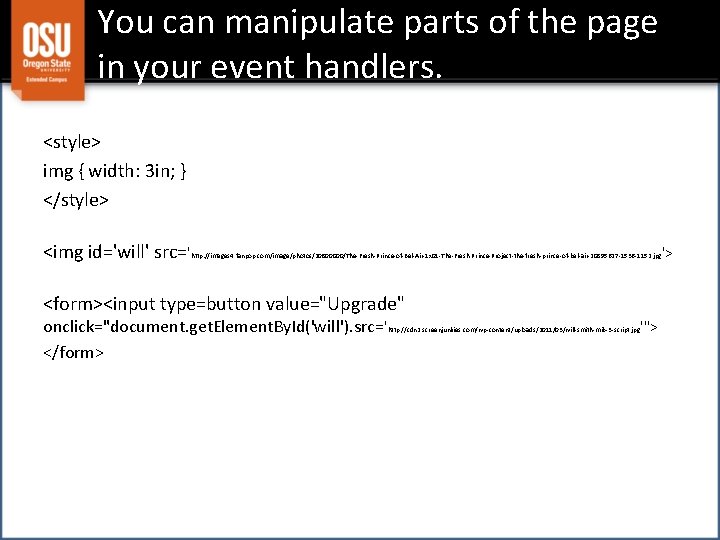
You can manipulate parts of the page in your event handlers. <style> img { width: 3 in; } </style> <img id='will' src=' '> http: //images 4. fanpop. com/image/photos/20800000/The-Fresh-Prince-of-Bel-Air-1 x 01 -The-Fresh-Prince-Project-the-fresh-prince-of-bel-air-20895627 -1536 -1152. jpg <form><input type=button value="Upgrade" onclick="document. get. Element. By. Id('will'). src=' </form> http: //cdn 2. screenjunkies. com/wp-content/uploads/2011/03/will-smith-mib-3 -script. jpg '">
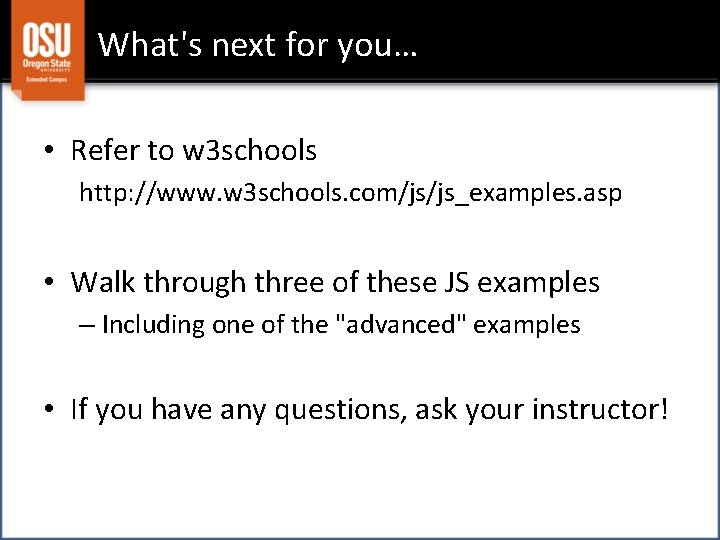
What's next for you… • Refer to w 3 schools http: //www. w 3 schools. com/js/js_examples. asp • Walk through three of these JS examples – Including one of the "advanced" examples • If you have any questions, ask your instructor!
Wise men three clever are we
Papercut uwl
Tabular editor
Pauline welby
Shifting script vorlage
Introduction to scripting languages
Scripting image
Paraview python scripting
Papercut job ticketing
Server side scripting
Gel script
Alleeg
Innovative features of scripting languages
Interpreted language vs compiled language
Perl shell scripting
Lumerical scripting language
Neoload scripting
Linden scripting language
Parallelism in a modest proposal
Marketo program tokens