More DOM Manipulating DOM trees n n DOM
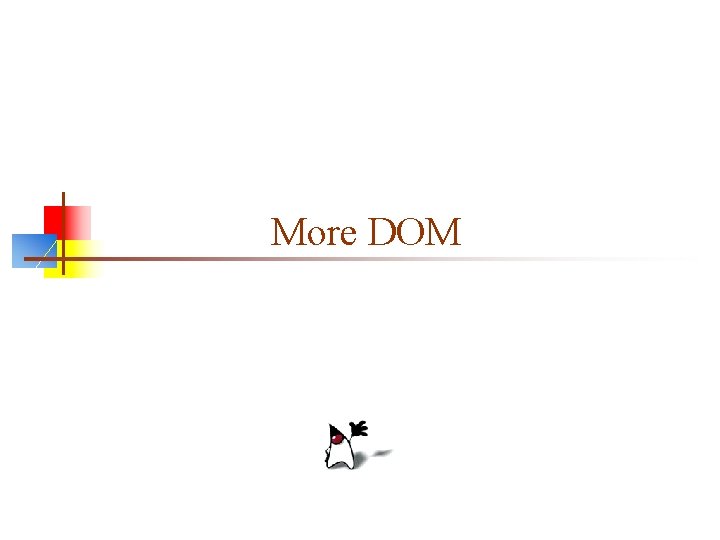
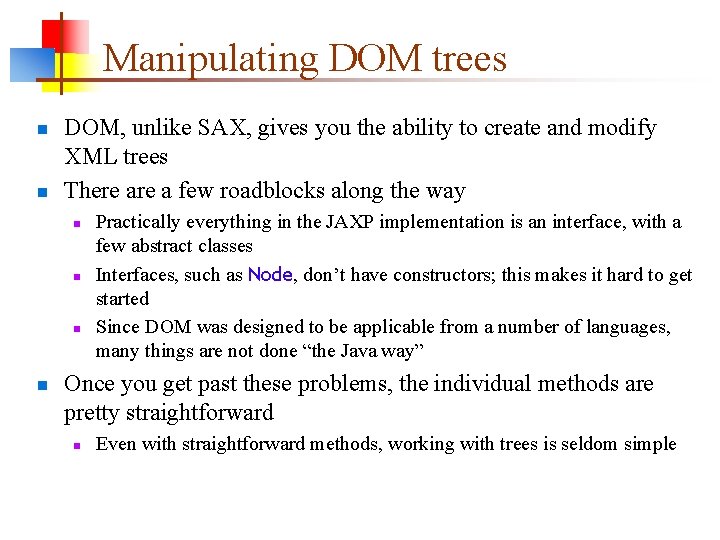
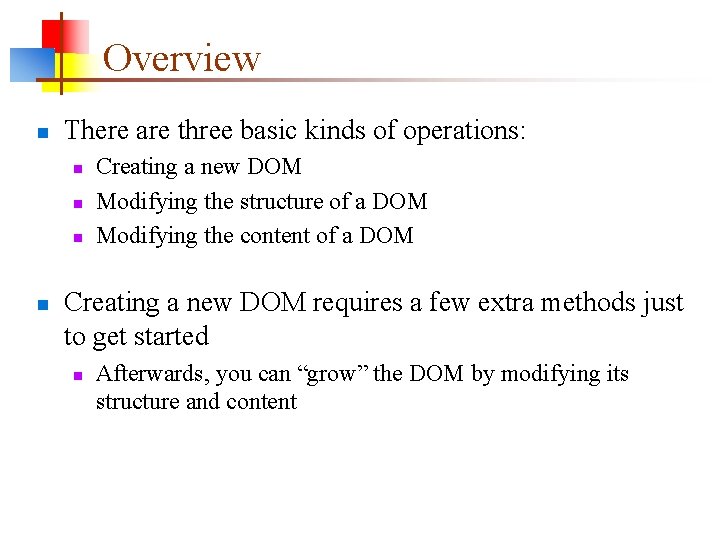
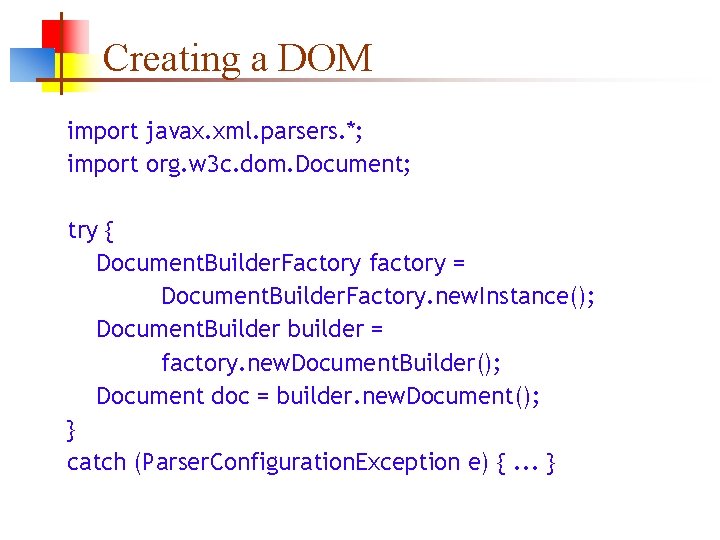
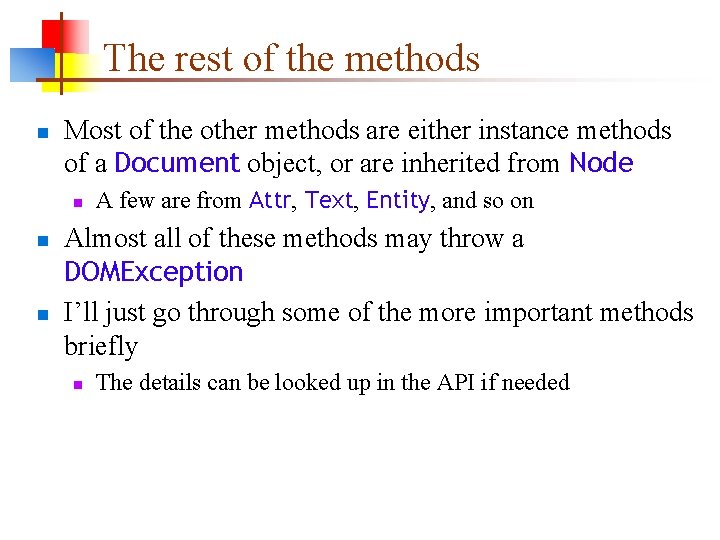
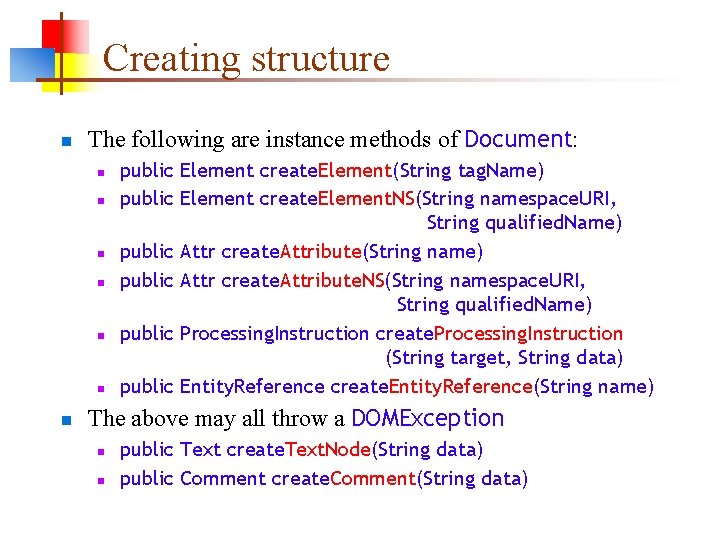
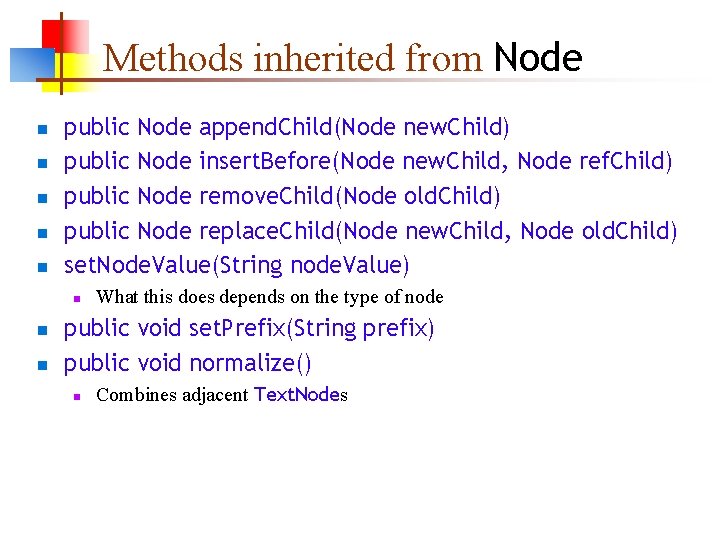
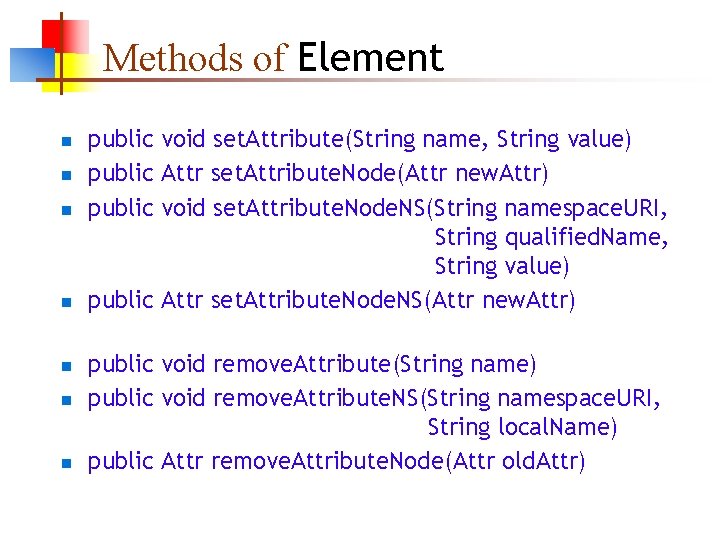
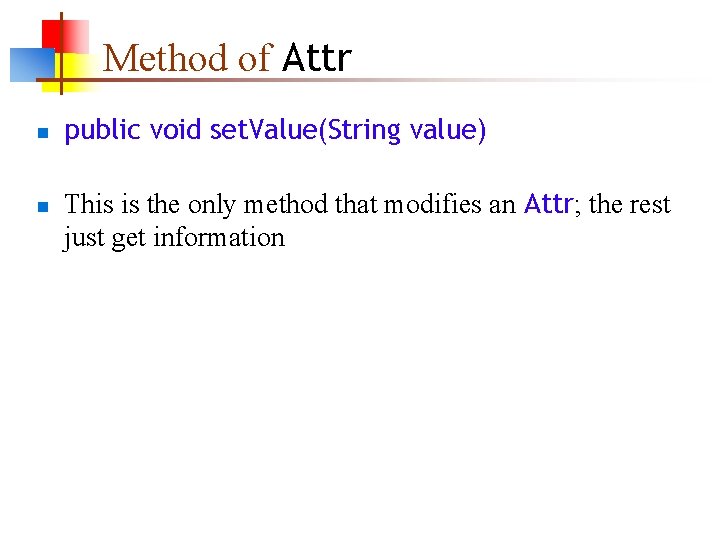
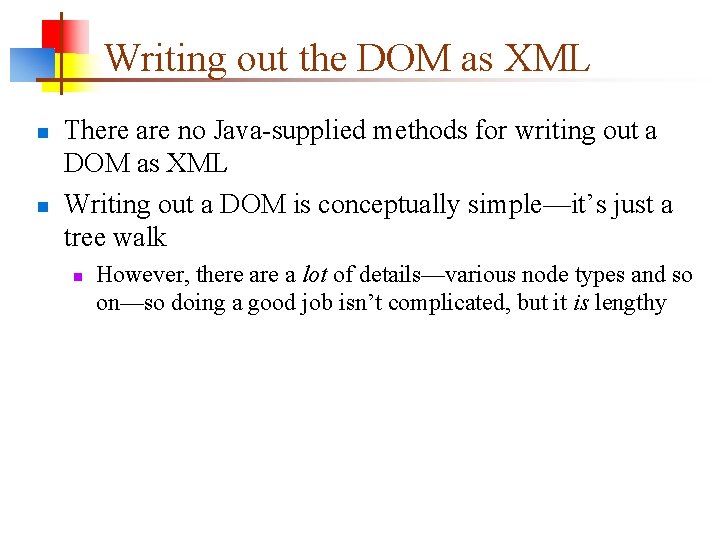
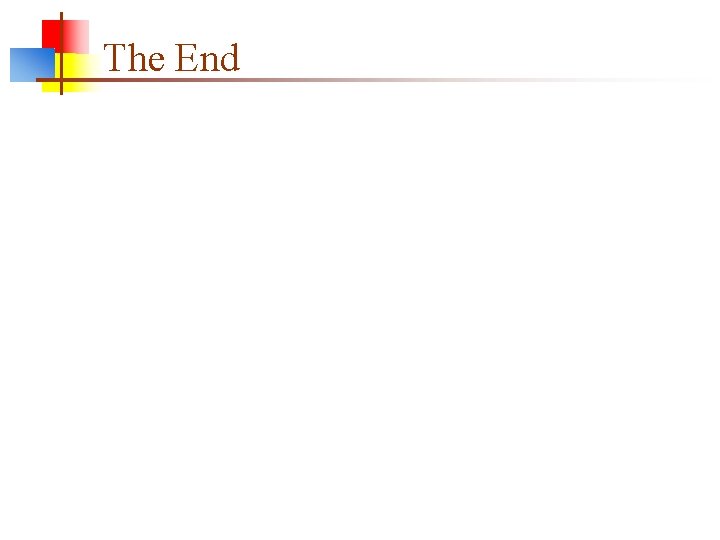
- Slides: 11
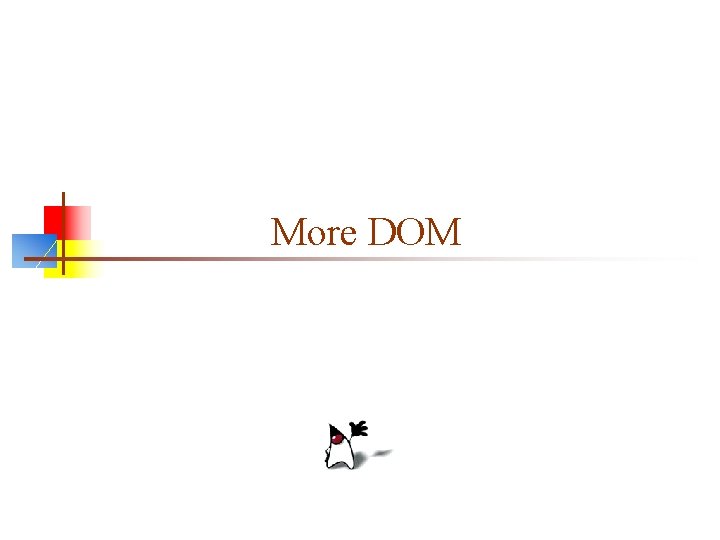
More DOM
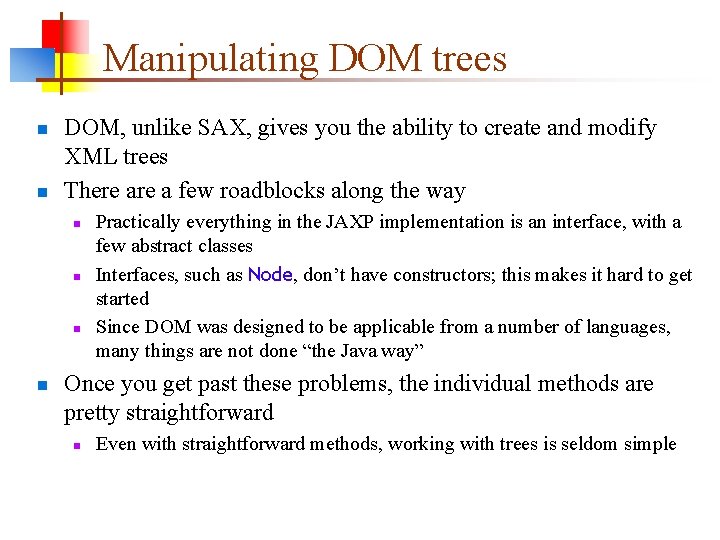
Manipulating DOM trees n n DOM, unlike SAX, gives you the ability to create and modify XML trees There a few roadblocks along the way n n Practically everything in the JAXP implementation is an interface, with a few abstract classes Interfaces, such as Node, don’t have constructors; this makes it hard to get started Since DOM was designed to be applicable from a number of languages, many things are not done “the Java way” Once you get past these problems, the individual methods are pretty straightforward n Even with straightforward methods, working with trees is seldom simple
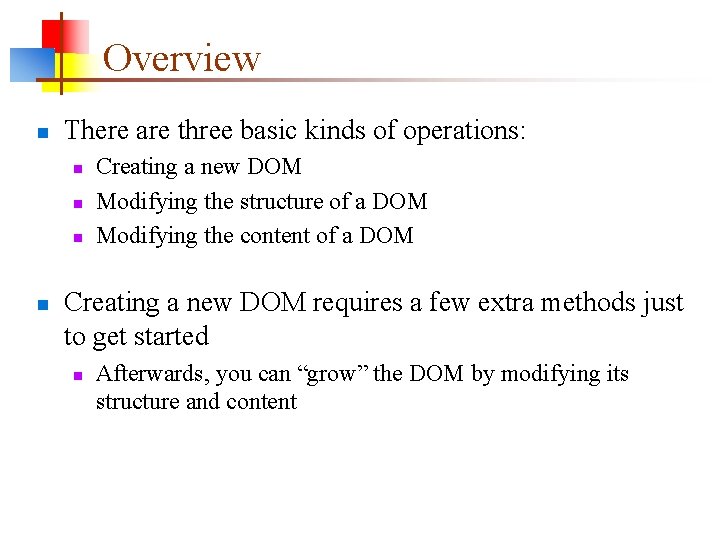
Overview n There are three basic kinds of operations: n n Creating a new DOM Modifying the structure of a DOM Modifying the content of a DOM Creating a new DOM requires a few extra methods just to get started n Afterwards, you can “grow” the DOM by modifying its structure and content
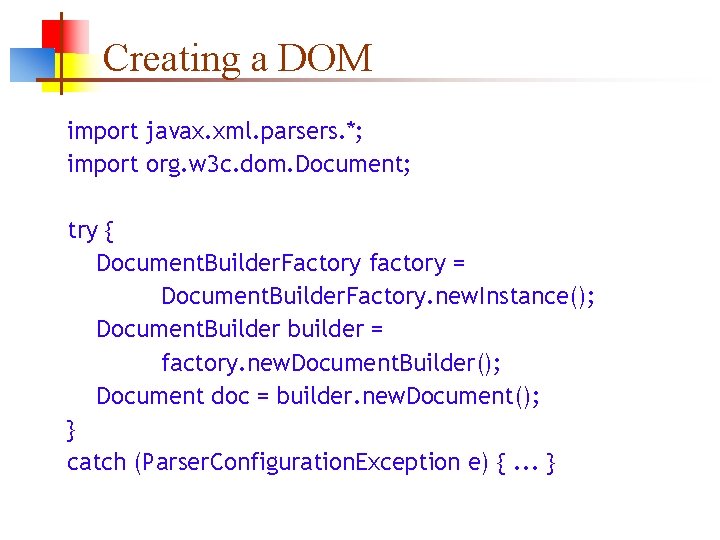
Creating a DOM import javax. xml. parsers. *; import org. w 3 c. dom. Document; try { Document. Builder. Factory factory = Document. Builder. Factory. new. Instance(); Document. Builder builder = factory. new. Document. Builder(); Document doc = builder. new. Document(); } catch (Parser. Configuration. Exception e) {. . . }
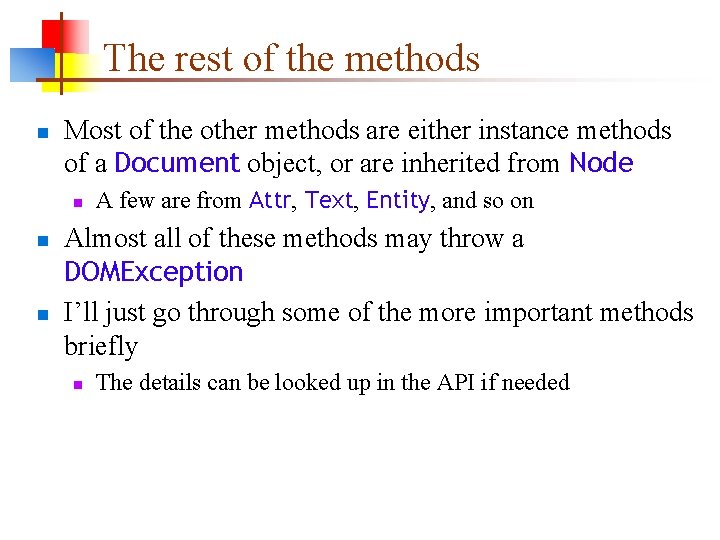
The rest of the methods n Most of the other methods are either instance methods of a Document object, or are inherited from Node n n n A few are from Attr, Text, Entity, and so on Almost all of these methods may throw a DOMException I’ll just go through some of the more important methods briefly n The details can be looked up in the API if needed
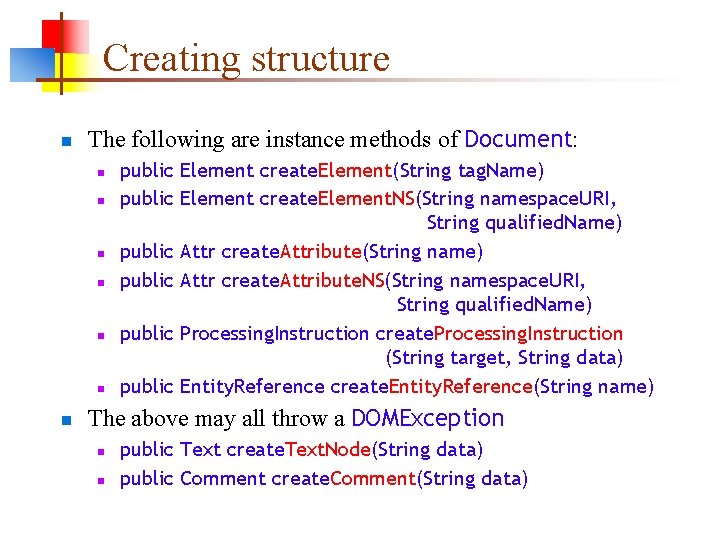
Creating structure n The following are instance methods of Document: n n n n public Element create. Element(String tag. Name) public Element create. Element. NS(String namespace. URI, String qualified. Name) public Attr create. Attribute(String name) public Attr create. Attribute. NS(String namespace. URI, String qualified. Name) public Processing. Instruction create. Processing. Instruction (String target, String data) public Entity. Reference create. Entity. Reference(String name) The above may all throw a DOMException n n public Text create. Text. Node(String data) public Comment create. Comment(String data)
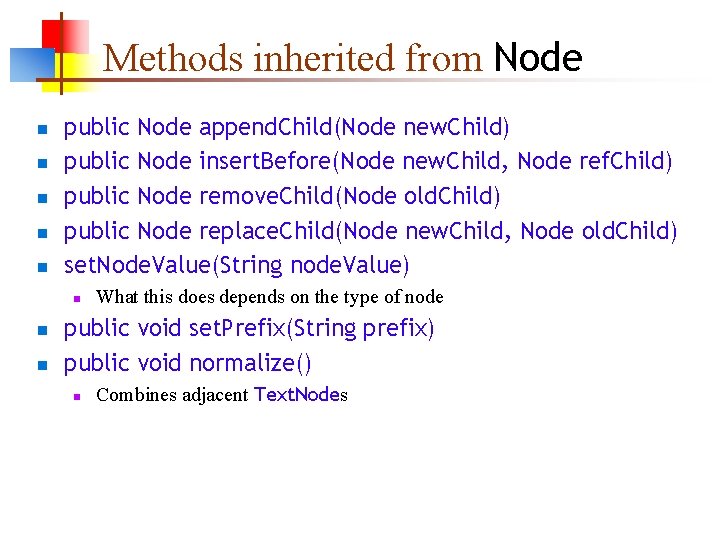
Methods inherited from Node n n n public Node append. Child(Node new. Child) public Node insert. Before(Node new. Child, Node ref. Child) public Node remove. Child(Node old. Child) public Node replace. Child(Node new. Child, Node old. Child) set. Node. Value(String node. Value) n n n What this does depends on the type of node public void set. Prefix(String prefix) public void normalize() n Combines adjacent Text. Nodes
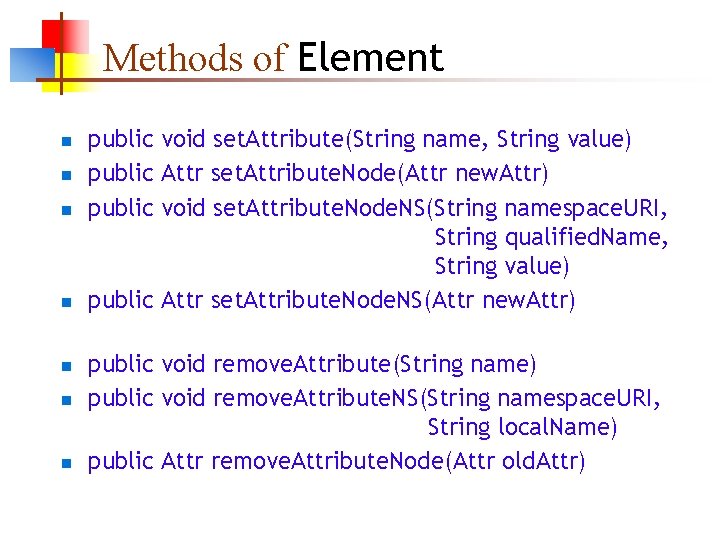
Methods of Element n n n n public void set. Attribute(String name, String value) public Attr set. Attribute. Node(Attr new. Attr) public void set. Attribute. Node. NS(String namespace. URI, String qualified. Name, String value) public Attr set. Attribute. Node. NS(Attr new. Attr) public void remove. Attribute(String name) public void remove. Attribute. NS(String namespace. URI, String local. Name) public Attr remove. Attribute. Node(Attr old. Attr)
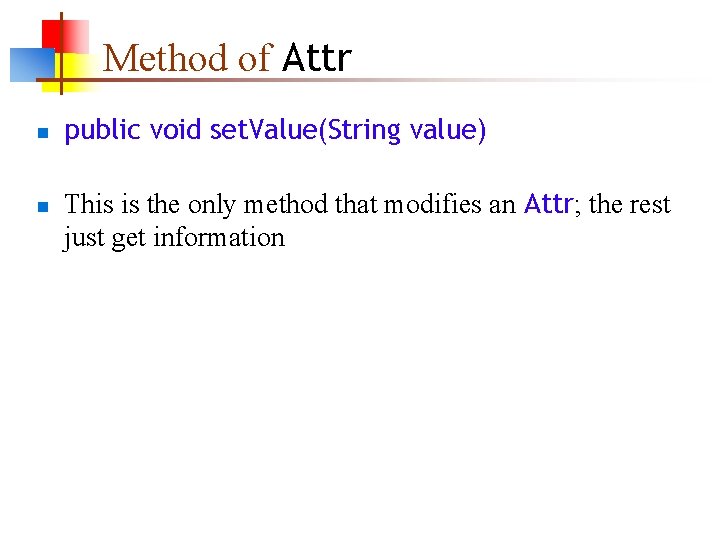
Method of Attr n n public void set. Value(String value) This is the only method that modifies an Attr; the rest just get information
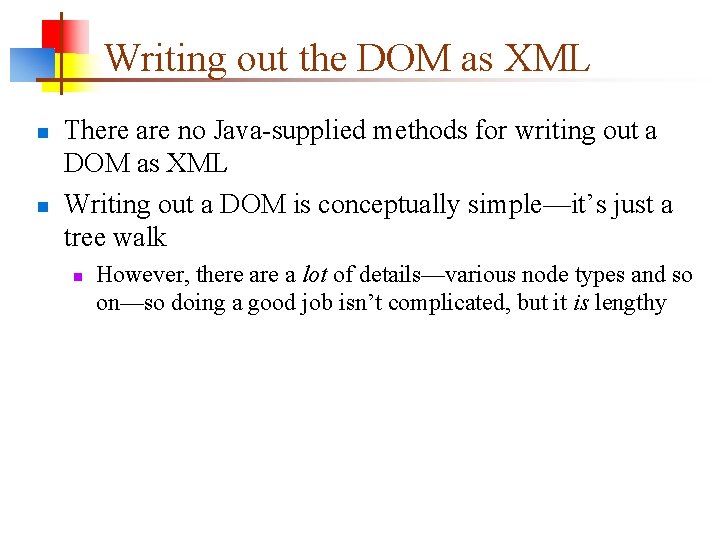
Writing out the DOM as XML n n There are no Java-supplied methods for writing out a DOM as XML Writing out a DOM is conceptually simple—it’s just a tree walk n However, there a lot of details—various node types and so on—so doing a good job isn’t complicated, but it is lengthy
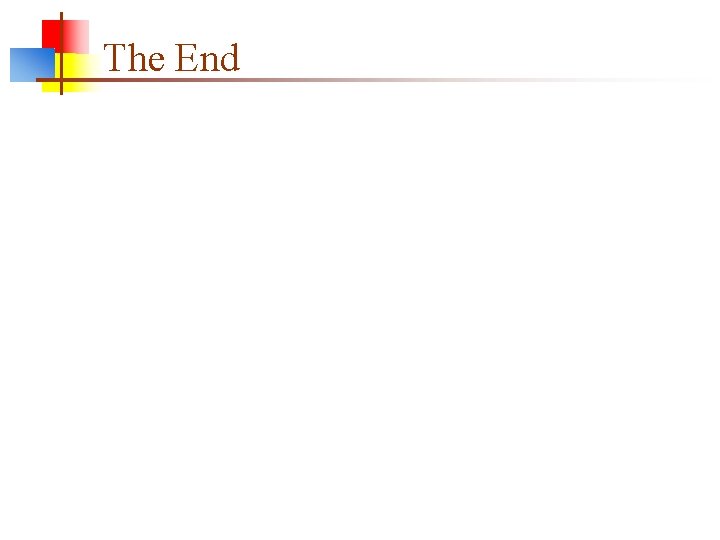
The End
More more more i want more more more more we praise you
More more more i want more more more more we praise you
Planting more trees is called
Process of manipulating images and sounds
What are accessibility features for staar
Medicated scalp lotion definition
Instructing conversing manipulating exploring
Manipulating large data sets
Multiply algebraic fractions
Section 13-2 manipulating dna
Manipulating dna answer key
Manipulating surds