Module 3 Part 5 Putting it all together
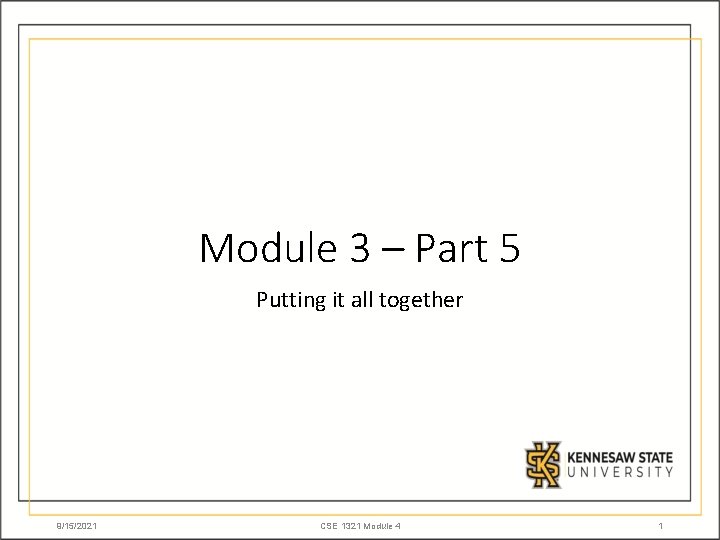
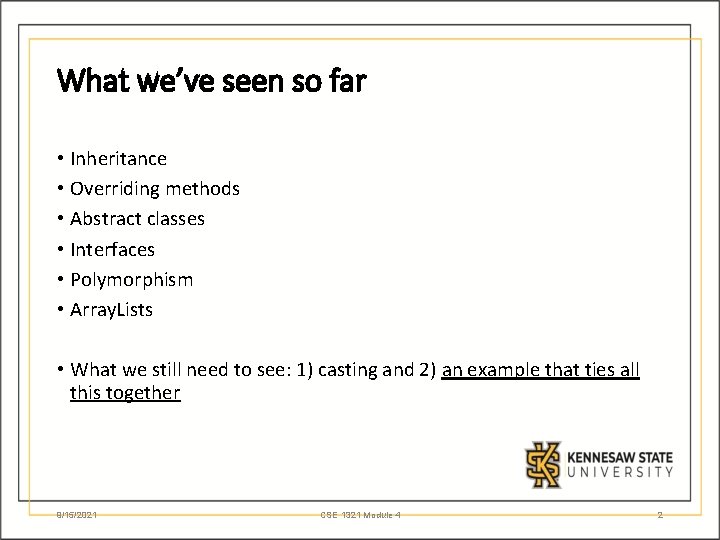
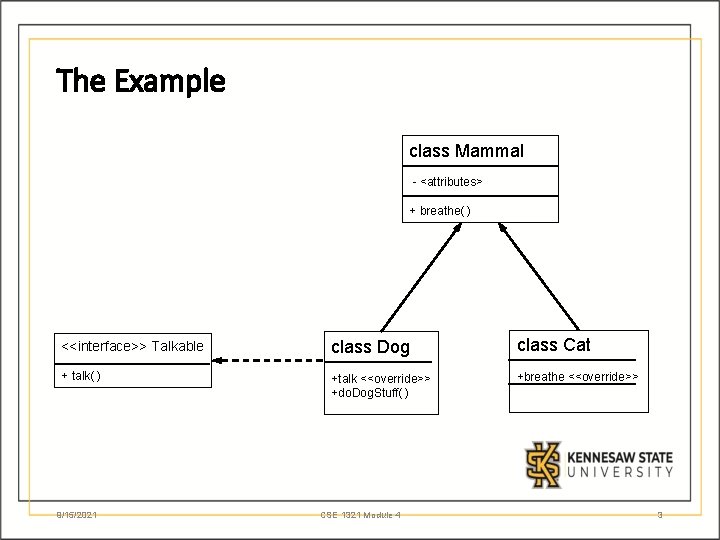
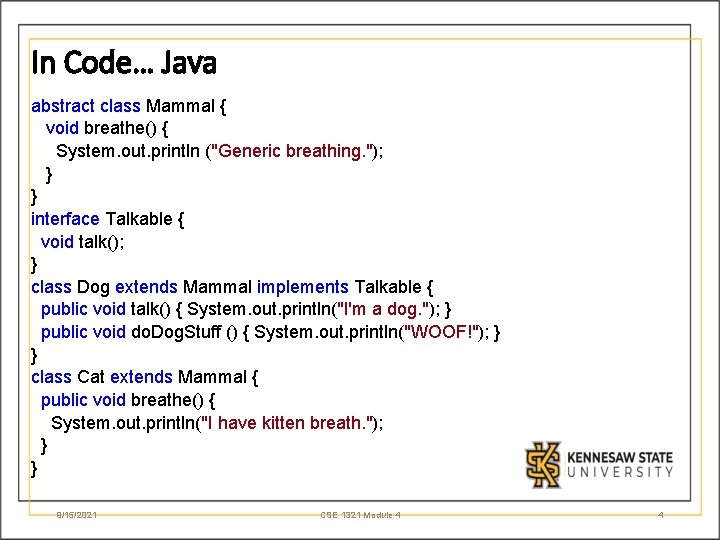
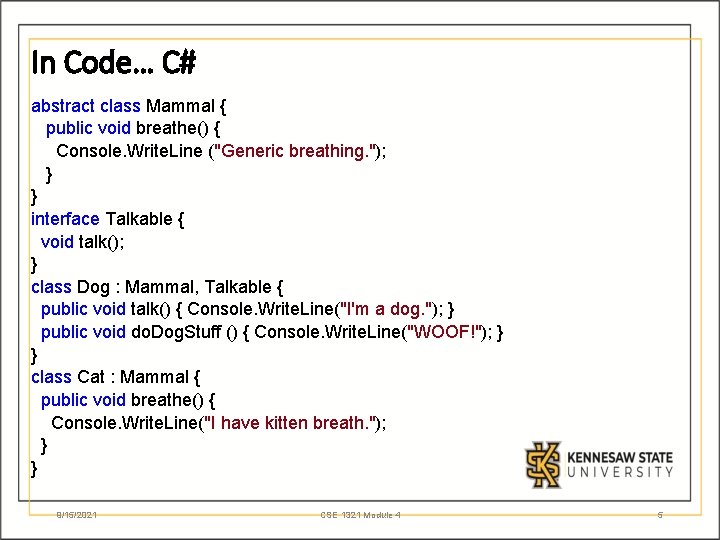
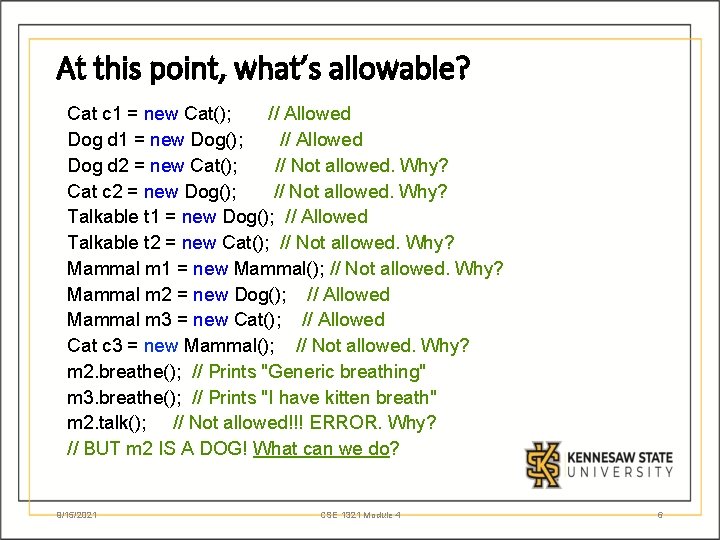
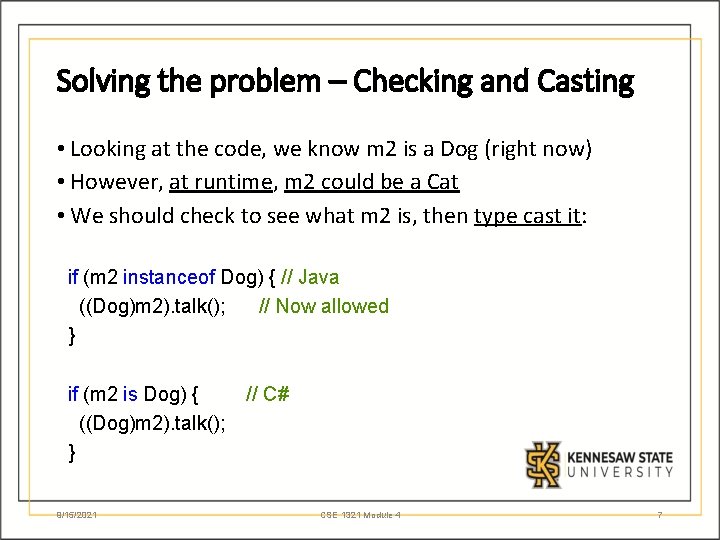
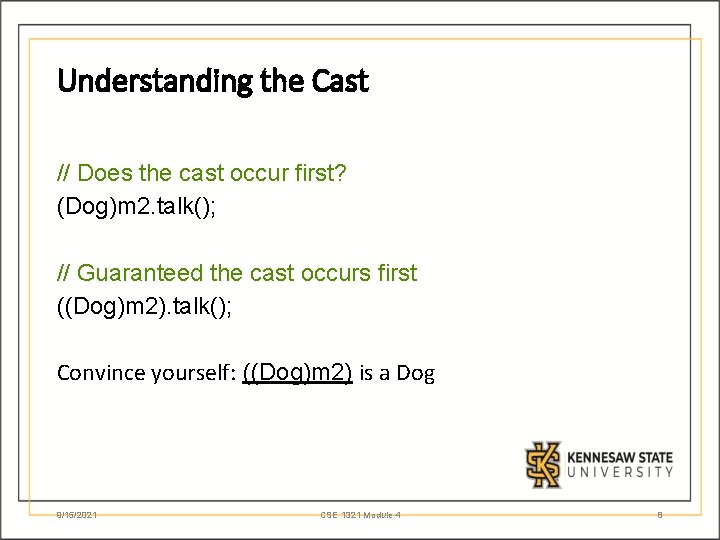
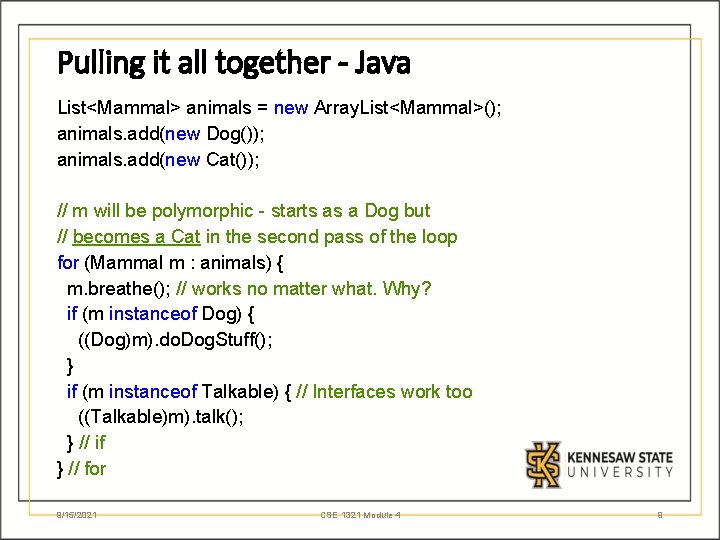
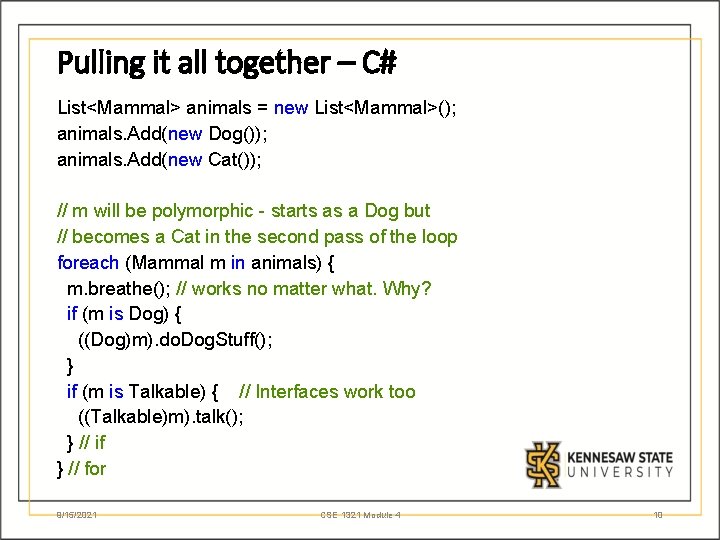
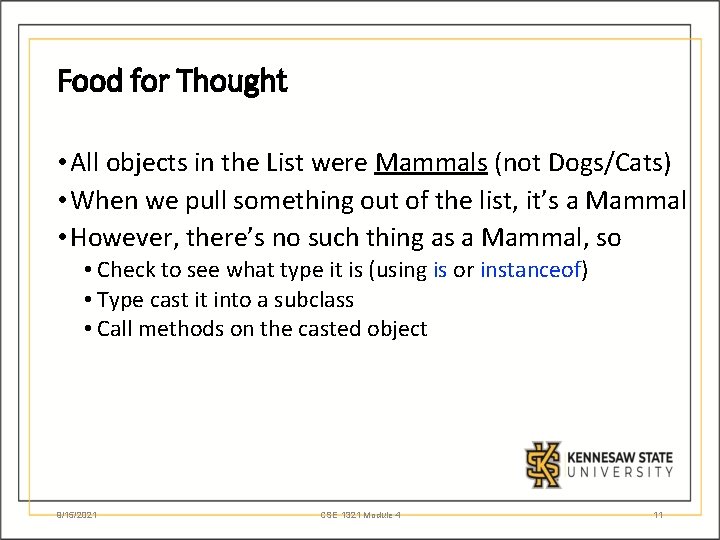
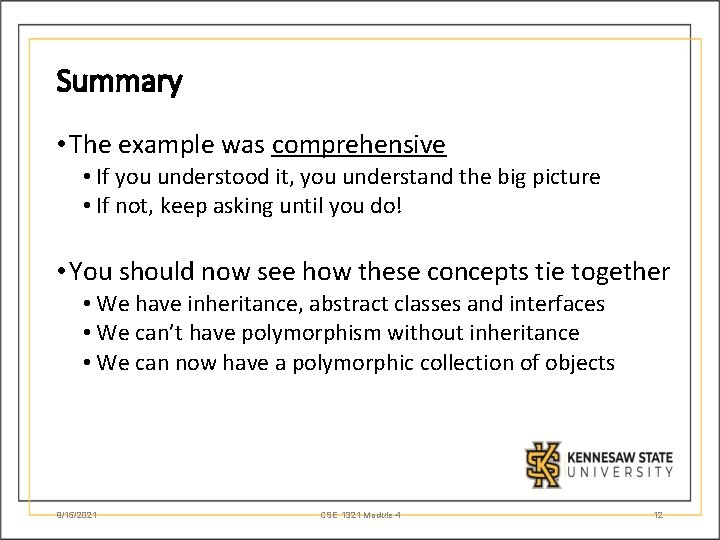
- Slides: 12
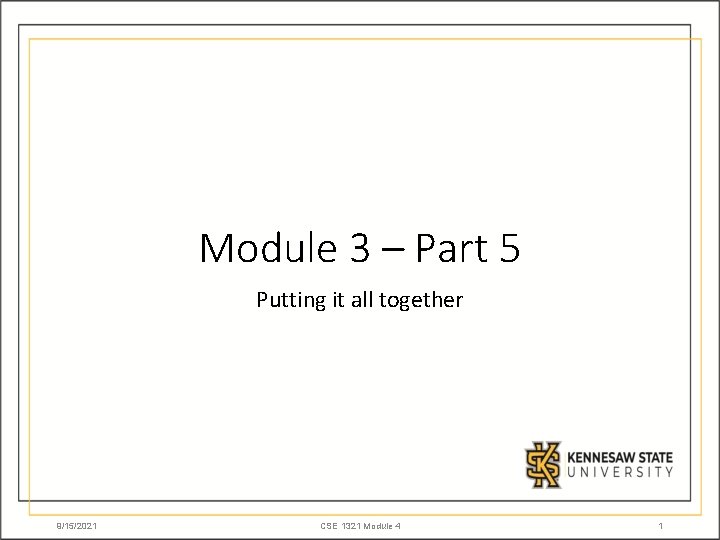
Module 3 – Part 5 Putting it all together 9/15/2021 CSE 1321 Module 4 1
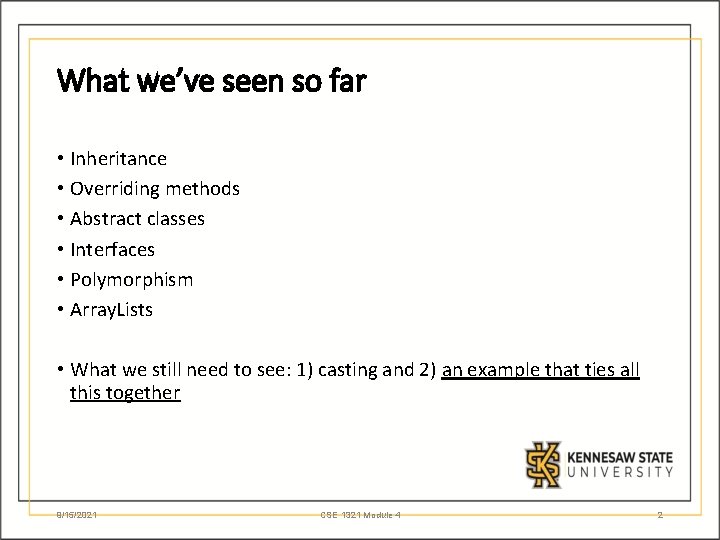
What we’ve seen so far • Inheritance • Overriding methods • Abstract classes • Interfaces • Polymorphism • Array. Lists • What we still need to see: 1) casting and 2) an example that ties all this together 9/15/2021 CSE 1321 Module 4 2
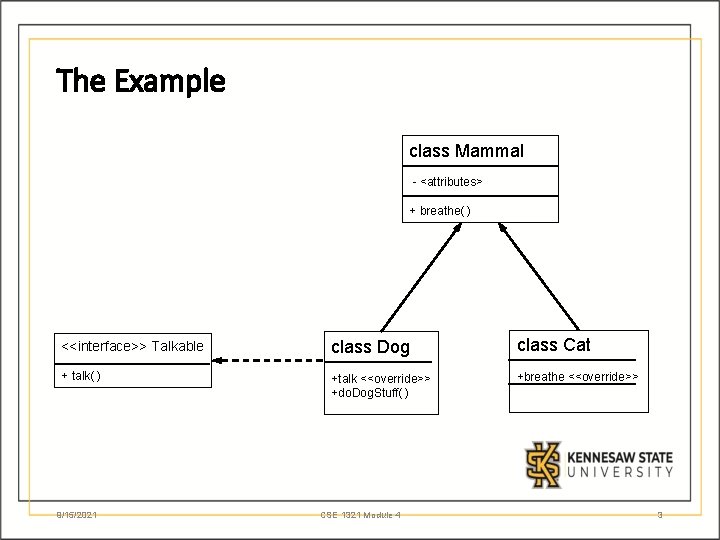
The Example class Mammal - <attributes> + breathe( ) <<interface>> Talkable class Dog class Cat + talk( ) +talk <<override>> +do. Dog. Stuff( ) +breathe <<override>> 9/15/2021 CSE 1321 Module 4 3
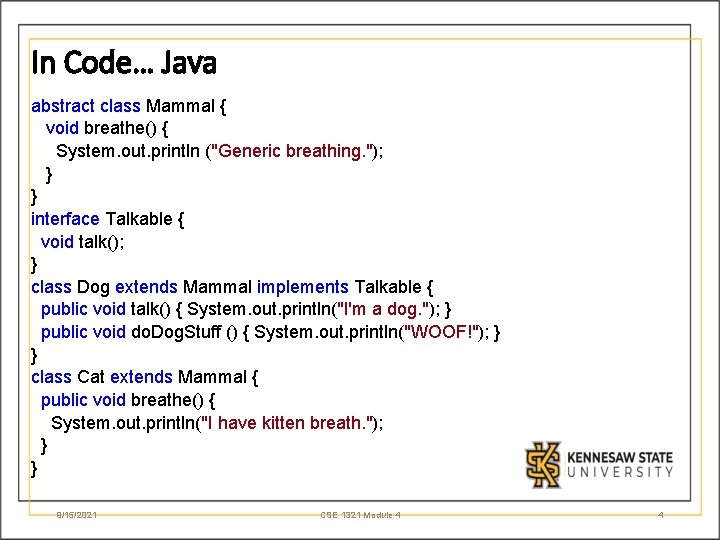
In Code… Java abstract class Mammal { void breathe() { System. out. println ("Generic breathing. "); } } interface Talkable { void talk(); } class Dog extends Mammal implements Talkable { public void talk() { System. out. println("I'm a dog. "); } public void do. Dog. Stuff () { System. out. println("WOOF!"); } } class Cat extends Mammal { public void breathe() { System. out. println("I have kitten breath. "); } } 9/15/2021 CSE 1321 Module 4 4
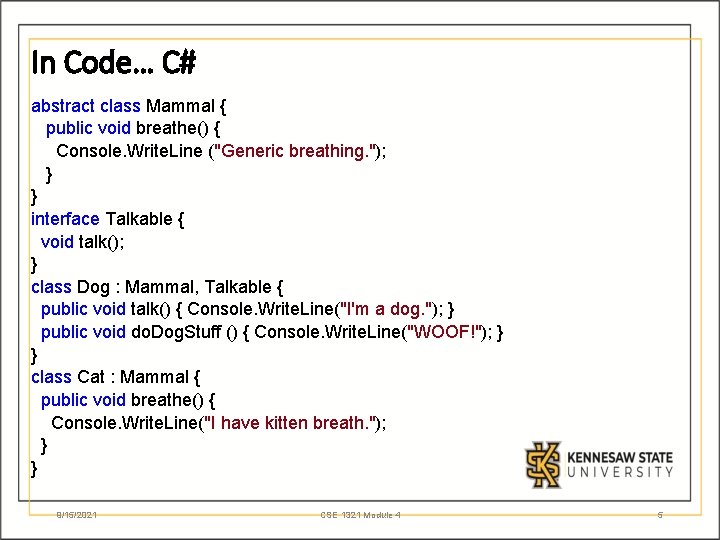
In Code… C# abstract class Mammal { public void breathe() { Console. Write. Line ("Generic breathing. "); } } interface Talkable { void talk(); } class Dog : Mammal, Talkable { public void talk() { Console. Write. Line("I'm a dog. "); } public void do. Dog. Stuff () { Console. Write. Line("WOOF!"); } } class Cat : Mammal { public void breathe() { Console. Write. Line("I have kitten breath. "); } } 9/15/2021 CSE 1321 Module 4 5
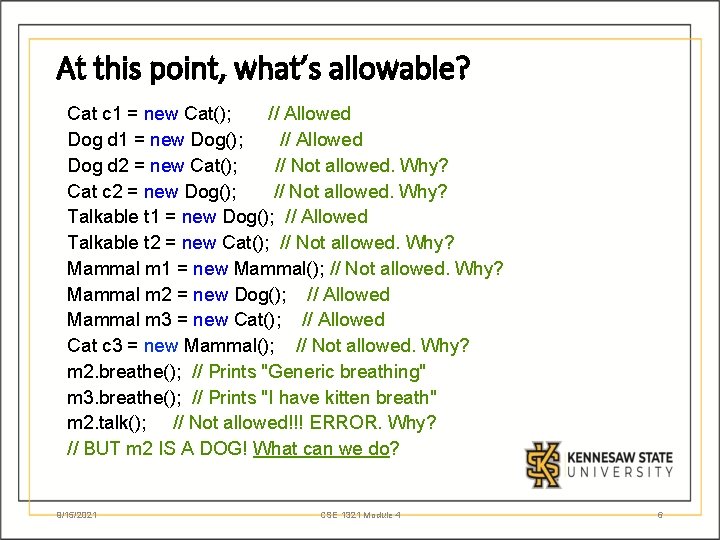
At this point, what’s allowable? Cat c 1 = new Cat(); // Allowed Dog d 1 = new Dog(); // Allowed Dog d 2 = new Cat(); // Not allowed. Why? Cat c 2 = new Dog(); // Not allowed. Why? Talkable t 1 = new Dog(); // Allowed Talkable t 2 = new Cat(); // Not allowed. Why? Mammal m 1 = new Mammal(); // Not allowed. Why? Mammal m 2 = new Dog(); // Allowed Mammal m 3 = new Cat(); // Allowed Cat c 3 = new Mammal(); // Not allowed. Why? m 2. breathe(); // Prints "Generic breathing" m 3. breathe(); // Prints "I have kitten breath" m 2. talk(); // Not allowed!!! ERROR. Why? // BUT m 2 IS A DOG! What can we do? 9/15/2021 CSE 1321 Module 4 6
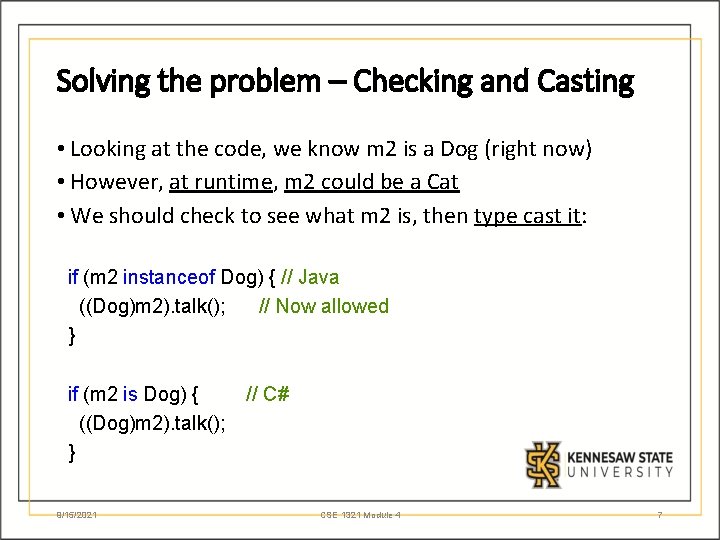
Solving the problem – Checking and Casting • Looking at the code, we know m 2 is a Dog (right now) • However, at runtime, m 2 could be a Cat • We should check to see what m 2 is, then type cast it: if (m 2 instanceof Dog) { // Java ((Dog)m 2). talk(); // Now allowed } if (m 2 is Dog) { ((Dog)m 2). talk(); } 9/15/2021 // C# CSE 1321 Module 4 7
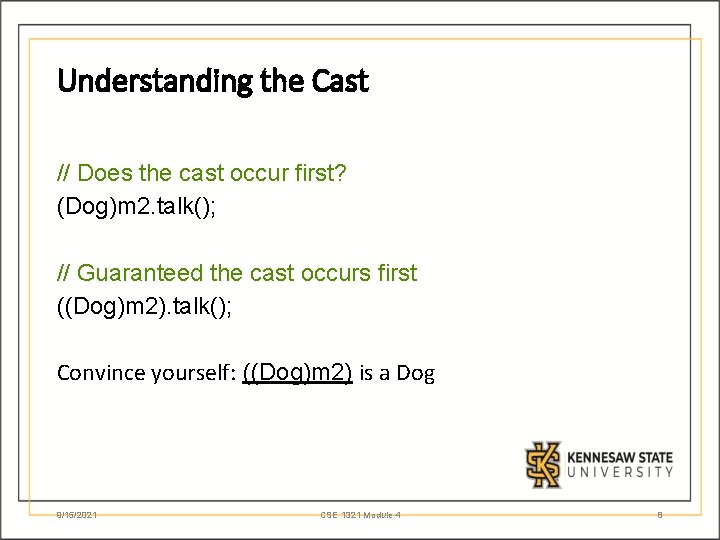
Understanding the Cast // Does the cast occur first? (Dog)m 2. talk(); // Guaranteed the cast occurs first ((Dog)m 2). talk(); Convince yourself: ((Dog)m 2) is a Dog 9/15/2021 CSE 1321 Module 4 8
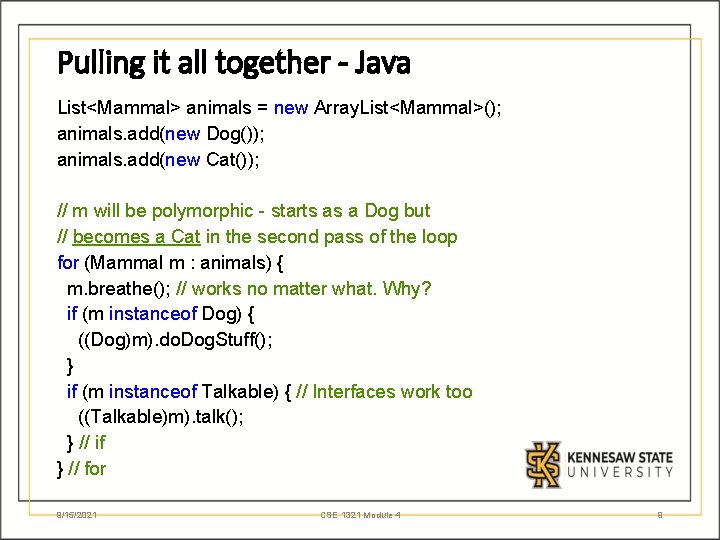
Pulling it all together - Java List<Mammal> animals = new Array. List<Mammal>(); animals. add(new Dog()); animals. add(new Cat()); // m will be polymorphic - starts as a Dog but // becomes a Cat in the second pass of the loop for (Mammal m : animals) { m. breathe(); // works no matter what. Why? if (m instanceof Dog) { ((Dog)m). do. Dog. Stuff(); } if (m instanceof Talkable) { // Interfaces work too ((Talkable)m). talk(); } // if } // for 9/15/2021 CSE 1321 Module 4 9
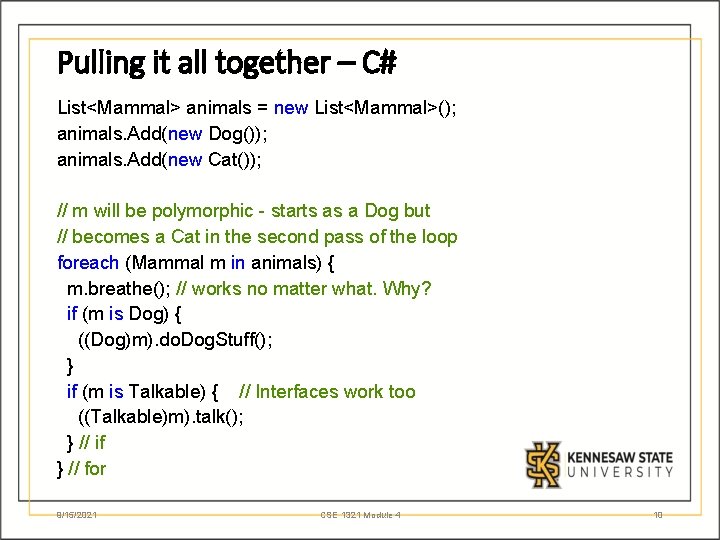
Pulling it all together – C# List<Mammal> animals = new List<Mammal>(); animals. Add(new Dog()); animals. Add(new Cat()); // m will be polymorphic - starts as a Dog but // becomes a Cat in the second pass of the loop foreach (Mammal m in animals) { m. breathe(); // works no matter what. Why? if (m is Dog) { ((Dog)m). do. Dog. Stuff(); } if (m is Talkable) { // Interfaces work too ((Talkable)m). talk(); } // if } // for 9/15/2021 CSE 1321 Module 4 10
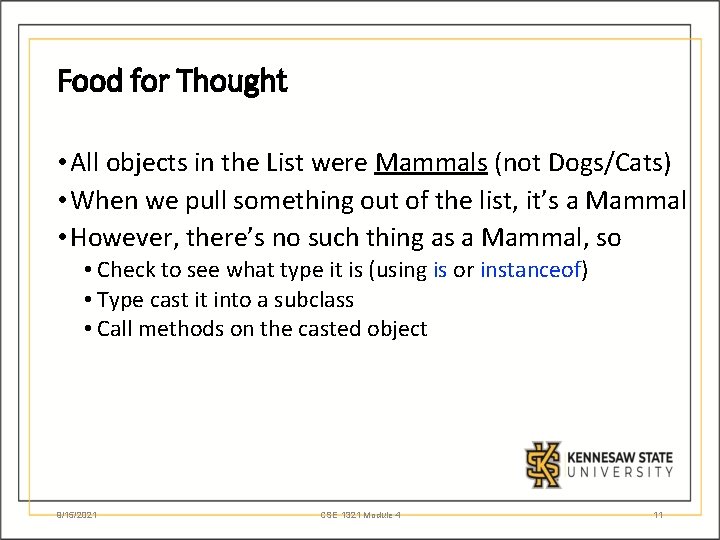
Food for Thought • All objects in the List were Mammals (not Dogs/Cats) • When we pull something out of the list, it’s a Mammal • However, there’s no such thing as a Mammal, so • Check to see what type it is (using is or instanceof) • Type cast it into a subclass • Call methods on the casted object 9/15/2021 CSE 1321 Module 4 11
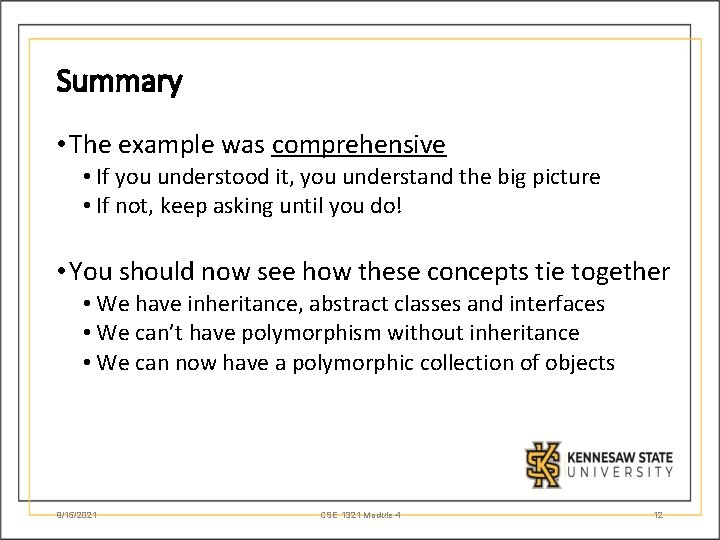
Summary • The example was comprehensive • If you understood it, you understand the big picture • If not, keep asking until you do! • You should now see how these concepts tie together • We have inheritance, abstract classes and interfaces • We can’t have polymorphism without inheritance • We can now have a polymorphic collection of objects 9/15/2021 CSE 1321 Module 4 12
Practice putting it all together part 1 fill in the blank
Putting it all together motion answer key
Together
Bridge introduction paragraph examples
6x²y³÷xy²= _______.
Putting letters together
Package mypackage class first class body
Strategic order of main points
Putting two words together
Putting the pieces together case study answer key
Putting things together is called
Putting it all to bed during project closeout includes
What fires together wires together